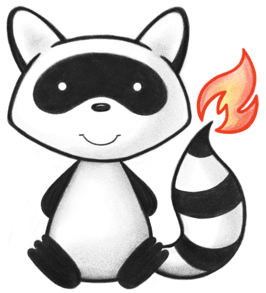
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044 045/** 046 * A request to convey information; e.g. the CDS system proposes that an alert 047 * be sent to a responsible provider, the CDS system proposes that the public 048 * health agency be notified about a reportable condition. 049 */ 050@ResourceDef(name = "CommunicationRequest", profile = "http://hl7.org/fhir/Profile/CommunicationRequest") 051public class CommunicationRequest extends DomainResource { 052 053 public enum CommunicationRequestStatus { 054 /** 055 * The request has been proposed. 056 */ 057 PROPOSED, 058 /** 059 * The request has been planned. 060 */ 061 PLANNED, 062 /** 063 * The request has been placed. 064 */ 065 REQUESTED, 066 /** 067 * The receiving system has received the request but not yet decided whether it 068 * will be performed. 069 */ 070 RECEIVED, 071 /** 072 * The receiving system has accepted the order, but work has not yet commenced. 073 */ 074 ACCEPTED, 075 /** 076 * The work to fulfill the order is happening. 077 */ 078 INPROGRESS, 079 /** 080 * The work has been complete, the report(s) released, and no further work is 081 * planned. 082 */ 083 COMPLETED, 084 /** 085 * The request has been held by originating system/user request. 086 */ 087 SUSPENDED, 088 /** 089 * The receiving system has declined to fulfill the request 090 */ 091 REJECTED, 092 /** 093 * The communication was attempted, but due to some procedural error, it could 094 * not be completed. 095 */ 096 FAILED, 097 /** 098 * added to help the parsers 099 */ 100 NULL; 101 102 public static CommunicationRequestStatus fromCode(String codeString) throws FHIRException { 103 if (codeString == null || "".equals(codeString)) 104 return null; 105 if ("proposed".equals(codeString)) 106 return PROPOSED; 107 if ("planned".equals(codeString)) 108 return PLANNED; 109 if ("requested".equals(codeString)) 110 return REQUESTED; 111 if ("received".equals(codeString)) 112 return RECEIVED; 113 if ("accepted".equals(codeString)) 114 return ACCEPTED; 115 if ("in-progress".equals(codeString)) 116 return INPROGRESS; 117 if ("completed".equals(codeString)) 118 return COMPLETED; 119 if ("suspended".equals(codeString)) 120 return SUSPENDED; 121 if ("rejected".equals(codeString)) 122 return REJECTED; 123 if ("failed".equals(codeString)) 124 return FAILED; 125 throw new FHIRException("Unknown CommunicationRequestStatus code '" + codeString + "'"); 126 } 127 128 public String toCode() { 129 switch (this) { 130 case PROPOSED: 131 return "proposed"; 132 case PLANNED: 133 return "planned"; 134 case REQUESTED: 135 return "requested"; 136 case RECEIVED: 137 return "received"; 138 case ACCEPTED: 139 return "accepted"; 140 case INPROGRESS: 141 return "in-progress"; 142 case COMPLETED: 143 return "completed"; 144 case SUSPENDED: 145 return "suspended"; 146 case REJECTED: 147 return "rejected"; 148 case FAILED: 149 return "failed"; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 157 public String getSystem() { 158 switch (this) { 159 case PROPOSED: 160 return "http://hl7.org/fhir/communication-request-status"; 161 case PLANNED: 162 return "http://hl7.org/fhir/communication-request-status"; 163 case REQUESTED: 164 return "http://hl7.org/fhir/communication-request-status"; 165 case RECEIVED: 166 return "http://hl7.org/fhir/communication-request-status"; 167 case ACCEPTED: 168 return "http://hl7.org/fhir/communication-request-status"; 169 case INPROGRESS: 170 return "http://hl7.org/fhir/communication-request-status"; 171 case COMPLETED: 172 return "http://hl7.org/fhir/communication-request-status"; 173 case SUSPENDED: 174 return "http://hl7.org/fhir/communication-request-status"; 175 case REJECTED: 176 return "http://hl7.org/fhir/communication-request-status"; 177 case FAILED: 178 return "http://hl7.org/fhir/communication-request-status"; 179 case NULL: 180 return null; 181 default: 182 return "?"; 183 } 184 } 185 186 public String getDefinition() { 187 switch (this) { 188 case PROPOSED: 189 return "The request has been proposed."; 190 case PLANNED: 191 return "The request has been planned."; 192 case REQUESTED: 193 return "The request has been placed."; 194 case RECEIVED: 195 return "The receiving system has received the request but not yet decided whether it will be performed."; 196 case ACCEPTED: 197 return "The receiving system has accepted the order, but work has not yet commenced."; 198 case INPROGRESS: 199 return "The work to fulfill the order is happening."; 200 case COMPLETED: 201 return "The work has been complete, the report(s) released, and no further work is planned."; 202 case SUSPENDED: 203 return "The request has been held by originating system/user request."; 204 case REJECTED: 205 return "The receiving system has declined to fulfill the request"; 206 case FAILED: 207 return "The communication was attempted, but due to some procedural error, it could not be completed."; 208 case NULL: 209 return null; 210 default: 211 return "?"; 212 } 213 } 214 215 public String getDisplay() { 216 switch (this) { 217 case PROPOSED: 218 return "Proposed"; 219 case PLANNED: 220 return "Planned"; 221 case REQUESTED: 222 return "Requested"; 223 case RECEIVED: 224 return "Received"; 225 case ACCEPTED: 226 return "Accepted"; 227 case INPROGRESS: 228 return "In Progress"; 229 case COMPLETED: 230 return "Completed"; 231 case SUSPENDED: 232 return "Suspended"; 233 case REJECTED: 234 return "Rejected"; 235 case FAILED: 236 return "Failed"; 237 case NULL: 238 return null; 239 default: 240 return "?"; 241 } 242 } 243 } 244 245 public static class CommunicationRequestStatusEnumFactory implements EnumFactory<CommunicationRequestStatus> { 246 public CommunicationRequestStatus fromCode(String codeString) throws IllegalArgumentException { 247 if (codeString == null || "".equals(codeString)) 248 if (codeString == null || "".equals(codeString)) 249 return null; 250 if ("proposed".equals(codeString)) 251 return CommunicationRequestStatus.PROPOSED; 252 if ("planned".equals(codeString)) 253 return CommunicationRequestStatus.PLANNED; 254 if ("requested".equals(codeString)) 255 return CommunicationRequestStatus.REQUESTED; 256 if ("received".equals(codeString)) 257 return CommunicationRequestStatus.RECEIVED; 258 if ("accepted".equals(codeString)) 259 return CommunicationRequestStatus.ACCEPTED; 260 if ("in-progress".equals(codeString)) 261 return CommunicationRequestStatus.INPROGRESS; 262 if ("completed".equals(codeString)) 263 return CommunicationRequestStatus.COMPLETED; 264 if ("suspended".equals(codeString)) 265 return CommunicationRequestStatus.SUSPENDED; 266 if ("rejected".equals(codeString)) 267 return CommunicationRequestStatus.REJECTED; 268 if ("failed".equals(codeString)) 269 return CommunicationRequestStatus.FAILED; 270 throw new IllegalArgumentException("Unknown CommunicationRequestStatus code '" + codeString + "'"); 271 } 272 273 public Enumeration<CommunicationRequestStatus> fromType(Base code) throws FHIRException { 274 if (code == null || code.isEmpty()) 275 return null; 276 String codeString = ((PrimitiveType) code).asStringValue(); 277 if (codeString == null || "".equals(codeString)) 278 return null; 279 if ("proposed".equals(codeString)) 280 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.PROPOSED); 281 if ("planned".equals(codeString)) 282 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.PLANNED); 283 if ("requested".equals(codeString)) 284 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.REQUESTED); 285 if ("received".equals(codeString)) 286 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.RECEIVED); 287 if ("accepted".equals(codeString)) 288 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.ACCEPTED); 289 if ("in-progress".equals(codeString)) 290 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.INPROGRESS); 291 if ("completed".equals(codeString)) 292 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.COMPLETED); 293 if ("suspended".equals(codeString)) 294 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.SUSPENDED); 295 if ("rejected".equals(codeString)) 296 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.REJECTED); 297 if ("failed".equals(codeString)) 298 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.FAILED); 299 throw new FHIRException("Unknown CommunicationRequestStatus code '" + codeString + "'"); 300 } 301 302 public String toCode(CommunicationRequestStatus code) 303 { 304 if (code == CommunicationRequestStatus.NULL) 305 return null; 306 if (code == CommunicationRequestStatus.PROPOSED) 307 return "proposed"; 308 if (code == CommunicationRequestStatus.PLANNED) 309 return "planned"; 310 if (code == CommunicationRequestStatus.REQUESTED) 311 return "requested"; 312 if (code == CommunicationRequestStatus.RECEIVED) 313 return "received"; 314 if (code == CommunicationRequestStatus.ACCEPTED) 315 return "accepted"; 316 if (code == CommunicationRequestStatus.INPROGRESS) 317 return "in-progress"; 318 if (code == CommunicationRequestStatus.COMPLETED) 319 return "completed"; 320 if (code == CommunicationRequestStatus.SUSPENDED) 321 return "suspended"; 322 if (code == CommunicationRequestStatus.REJECTED) 323 return "rejected"; 324 if (code == CommunicationRequestStatus.FAILED) 325 return "failed"; 326 return "?"; 327 } 328 } 329 330 @Block() 331 public static class CommunicationRequestPayloadComponent extends BackboneElement implements IBaseBackboneElement { 332 /** 333 * The communicated content (or for multi-part communications, one portion of 334 * the communication). 335 */ 336 @Child(name = "content", type = { StringType.class, 337 Attachment.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 338 @Description(shortDefinition = "Message part content", formalDefinition = "The communicated content (or for multi-part communications, one portion of the communication).") 339 protected Type content; 340 341 private static final long serialVersionUID = -1763459053L; 342 343 /* 344 * Constructor 345 */ 346 public CommunicationRequestPayloadComponent() { 347 super(); 348 } 349 350 /* 351 * Constructor 352 */ 353 public CommunicationRequestPayloadComponent(Type content) { 354 super(); 355 this.content = content; 356 } 357 358 /** 359 * @return {@link #content} (The communicated content (or for multi-part 360 * communications, one portion of the communication).) 361 */ 362 public Type getContent() { 363 return this.content; 364 } 365 366 /** 367 * @return {@link #content} (The communicated content (or for multi-part 368 * communications, one portion of the communication).) 369 */ 370 public StringType getContentStringType() throws FHIRException { 371 if (!(this.content instanceof StringType)) 372 throw new FHIRException("Type mismatch: the type StringType was expected, but " 373 + this.content.getClass().getName() + " was encountered"); 374 return (StringType) this.content; 375 } 376 377 public boolean hasContentStringType() { 378 return this.content instanceof StringType; 379 } 380 381 /** 382 * @return {@link #content} (The communicated content (or for multi-part 383 * communications, one portion of the communication).) 384 */ 385 public Attachment getContentAttachment() throws FHIRException { 386 if (!(this.content instanceof Attachment)) 387 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 388 + this.content.getClass().getName() + " was encountered"); 389 return (Attachment) this.content; 390 } 391 392 public boolean hasContentAttachment() { 393 return this.content instanceof Attachment; 394 } 395 396 /** 397 * @return {@link #content} (The communicated content (or for multi-part 398 * communications, one portion of the communication).) 399 */ 400 public Reference getContentReference() throws FHIRException { 401 if (!(this.content instanceof Reference)) 402 throw new FHIRException("Type mismatch: the type Reference was expected, but " 403 + this.content.getClass().getName() + " was encountered"); 404 return (Reference) this.content; 405 } 406 407 public boolean hasContentReference() { 408 return this.content instanceof Reference; 409 } 410 411 public boolean hasContent() { 412 return this.content != null && !this.content.isEmpty(); 413 } 414 415 /** 416 * @param value {@link #content} (The communicated content (or for multi-part 417 * communications, one portion of the communication).) 418 */ 419 public CommunicationRequestPayloadComponent setContent(Type value) { 420 this.content = value; 421 return this; 422 } 423 424 protected void listChildren(List<Property> childrenList) { 425 super.listChildren(childrenList); 426 childrenList.add(new Property("content[x]", "string|Attachment|Reference(Any)", 427 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 428 java.lang.Integer.MAX_VALUE, content)); 429 } 430 431 @Override 432 public void setProperty(String name, Base value) throws FHIRException { 433 if (name.equals("content[x]")) 434 this.content = (Type) value; // Type 435 else 436 super.setProperty(name, value); 437 } 438 439 @Override 440 public Base addChild(String name) throws FHIRException { 441 if (name.equals("contentString")) { 442 this.content = new StringType(); 443 return this.content; 444 } else if (name.equals("contentAttachment")) { 445 this.content = new Attachment(); 446 return this.content; 447 } else if (name.equals("contentReference")) { 448 this.content = new Reference(); 449 return this.content; 450 } else 451 return super.addChild(name); 452 } 453 454 public CommunicationRequestPayloadComponent copy() { 455 CommunicationRequestPayloadComponent dst = new CommunicationRequestPayloadComponent(); 456 copyValues(dst); 457 dst.content = content == null ? null : content.copy(); 458 return dst; 459 } 460 461 @Override 462 public boolean equalsDeep(Base other) { 463 if (!super.equalsDeep(other)) 464 return false; 465 if (!(other instanceof CommunicationRequestPayloadComponent)) 466 return false; 467 CommunicationRequestPayloadComponent o = (CommunicationRequestPayloadComponent) other; 468 return compareDeep(content, o.content, true); 469 } 470 471 @Override 472 public boolean equalsShallow(Base other) { 473 if (!super.equalsShallow(other)) 474 return false; 475 if (!(other instanceof CommunicationRequestPayloadComponent)) 476 return false; 477 CommunicationRequestPayloadComponent o = (CommunicationRequestPayloadComponent) other; 478 return true; 479 } 480 481 public boolean isEmpty() { 482 return super.isEmpty() && (content == null || content.isEmpty()); 483 } 484 485 public String fhirType() { 486 return "CommunicationRequest.payload"; 487 488 } 489 490 } 491 492 /** 493 * A unique ID of this request for reference purposes. It must be provided if 494 * user wants it returned as part of any output, otherwise it will be 495 * autogenerated, if needed, by CDS system. Does not need to be the actual ID of 496 * the source system. 497 */ 498 @Child(name = "identifier", type = { 499 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 500 @Description(shortDefinition = "Unique identifier", formalDefinition = "A unique ID of this request for reference purposes. It must be provided if user wants it returned as part of any output, otherwise it will be autogenerated, if needed, by CDS system. Does not need to be the actual ID of the source system.") 501 protected List<Identifier> identifier; 502 503 /** 504 * The type of message to be sent such as alert, notification, reminder, 505 * instruction, etc. 506 */ 507 @Child(name = "category", type = { 508 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 509 @Description(shortDefinition = "Message category", formalDefinition = "The type of message to be sent such as alert, notification, reminder, instruction, etc.") 510 protected CodeableConcept category; 511 512 /** 513 * The entity (e.g. person, organization, clinical information system, or 514 * device) which is to be the source of the communication. 515 */ 516 @Child(name = "sender", type = { Device.class, Organization.class, Patient.class, Practitioner.class, 517 RelatedPerson.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 518 @Description(shortDefinition = "Message sender", formalDefinition = "The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.") 519 protected Reference sender; 520 521 /** 522 * The actual object that is the target of the reference (The entity (e.g. 523 * person, organization, clinical information system, or device) which is to be 524 * the source of the communication.) 525 */ 526 protected Resource senderTarget; 527 528 /** 529 * The entity (e.g. person, organization, clinical information system, or 530 * device) which is the intended target of the communication. 531 */ 532 @Child(name = "recipient", type = { Device.class, Organization.class, Patient.class, Practitioner.class, 533 RelatedPerson.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 534 @Description(shortDefinition = "Message recipient", formalDefinition = "The entity (e.g. person, organization, clinical information system, or device) which is the intended target of the communication.") 535 protected List<Reference> recipient; 536 /** 537 * The actual objects that are the target of the reference (The entity (e.g. 538 * person, organization, clinical information system, or device) which is the 539 * intended target of the communication.) 540 */ 541 protected List<Resource> recipientTarget; 542 543 /** 544 * Text, attachment(s), or resource(s) to be communicated to the recipient. 545 */ 546 @Child(name = "payload", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 547 @Description(shortDefinition = "Message payload", formalDefinition = "Text, attachment(s), or resource(s) to be communicated to the recipient.") 548 protected List<CommunicationRequestPayloadComponent> payload; 549 550 /** 551 * A channel that was used for this communication (e.g. email, fax). 552 */ 553 @Child(name = "medium", type = { 554 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 555 @Description(shortDefinition = "A channel of communication", formalDefinition = "A channel that was used for this communication (e.g. email, fax).") 556 protected List<CodeableConcept> medium; 557 558 /** 559 * The responsible person who authorizes this order, e.g. physician. This may be 560 * different than the author of the order statement, e.g. clerk, who may have 561 * entered the statement into the order entry application. 562 */ 563 @Child(name = "requester", type = { Practitioner.class, Patient.class, 564 RelatedPerson.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 565 @Description(shortDefinition = "An individual who requested a communication", formalDefinition = "The responsible person who authorizes this order, e.g. physician. This may be different than the author of the order statement, e.g. clerk, who may have entered the statement into the order entry application.") 566 protected Reference requester; 567 568 /** 569 * The actual object that is the target of the reference (The responsible person 570 * who authorizes this order, e.g. physician. This may be different than the 571 * author of the order statement, e.g. clerk, who may have entered the statement 572 * into the order entry application.) 573 */ 574 protected Resource requesterTarget; 575 576 /** 577 * The status of the proposal or order. 578 */ 579 @Child(name = "status", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = true, summary = true) 580 @Description(shortDefinition = "proposed | planned | requested | received | accepted | in-progress | completed | suspended | rejected | failed", formalDefinition = "The status of the proposal or order.") 581 protected Enumeration<CommunicationRequestStatus> status; 582 583 /** 584 * The encounter within which the communication request was created. 585 */ 586 @Child(name = "encounter", type = { Encounter.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 587 @Description(shortDefinition = "Encounter leading to message", formalDefinition = "The encounter within which the communication request was created.") 588 protected Reference encounter; 589 590 /** 591 * The actual object that is the target of the reference (The encounter within 592 * which the communication request was created.) 593 */ 594 protected Encounter encounterTarget; 595 596 /** 597 * The time when this communication is to occur. 598 */ 599 @Child(name = "scheduled", type = { DateTimeType.class, 600 Period.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 601 @Description(shortDefinition = "When scheduled", formalDefinition = "The time when this communication is to occur.") 602 protected Type scheduled; 603 604 /** 605 * The reason or justification for the communication request. 606 */ 607 @Child(name = "reason", type = { 608 CodeableConcept.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 609 @Description(shortDefinition = "Indication for message", formalDefinition = "The reason or justification for the communication request.") 610 protected List<CodeableConcept> reason; 611 612 /** 613 * The time when the request was made. 614 */ 615 @Child(name = "requestedOn", type = { 616 DateTimeType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 617 @Description(shortDefinition = "When ordered or proposed", formalDefinition = "The time when the request was made.") 618 protected DateTimeType requestedOn; 619 620 /** 621 * The patient who is the focus of this communication request. 622 */ 623 @Child(name = "subject", type = { Patient.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 624 @Description(shortDefinition = "Focus of message", formalDefinition = "The patient who is the focus of this communication request.") 625 protected Reference subject; 626 627 /** 628 * The actual object that is the target of the reference (The patient who is the 629 * focus of this communication request.) 630 */ 631 protected Patient subjectTarget; 632 633 /** 634 * Characterizes how quickly the proposed act must be initiated. Includes 635 * concepts such as stat, urgent, routine. 636 */ 637 @Child(name = "priority", type = { 638 CodeableConcept.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 639 @Description(shortDefinition = "Message urgency", formalDefinition = "Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.") 640 protected CodeableConcept priority; 641 642 private static final long serialVersionUID = 146906020L; 643 644 /* 645 * Constructor 646 */ 647 public CommunicationRequest() { 648 super(); 649 } 650 651 /** 652 * @return {@link #identifier} (A unique ID of this request for reference 653 * purposes. It must be provided if user wants it returned as part of 654 * any output, otherwise it will be autogenerated, if needed, by CDS 655 * system. Does not need to be the actual ID of the source system.) 656 */ 657 public List<Identifier> getIdentifier() { 658 if (this.identifier == null) 659 this.identifier = new ArrayList<Identifier>(); 660 return this.identifier; 661 } 662 663 public boolean hasIdentifier() { 664 if (this.identifier == null) 665 return false; 666 for (Identifier item : this.identifier) 667 if (!item.isEmpty()) 668 return true; 669 return false; 670 } 671 672 /** 673 * @return {@link #identifier} (A unique ID of this request for reference 674 * purposes. It must be provided if user wants it returned as part of 675 * any output, otherwise it will be autogenerated, if needed, by CDS 676 * system. Does not need to be the actual ID of the source system.) 677 */ 678 // syntactic sugar 679 public Identifier addIdentifier() { // 3 680 Identifier t = new Identifier(); 681 if (this.identifier == null) 682 this.identifier = new ArrayList<Identifier>(); 683 this.identifier.add(t); 684 return t; 685 } 686 687 // syntactic sugar 688 public CommunicationRequest addIdentifier(Identifier t) { // 3 689 if (t == null) 690 return this; 691 if (this.identifier == null) 692 this.identifier = new ArrayList<Identifier>(); 693 this.identifier.add(t); 694 return this; 695 } 696 697 /** 698 * @return {@link #category} (The type of message to be sent such as alert, 699 * notification, reminder, instruction, etc.) 700 */ 701 public CodeableConcept getCategory() { 702 if (this.category == null) 703 if (Configuration.errorOnAutoCreate()) 704 throw new Error("Attempt to auto-create CommunicationRequest.category"); 705 else if (Configuration.doAutoCreate()) 706 this.category = new CodeableConcept(); // cc 707 return this.category; 708 } 709 710 public boolean hasCategory() { 711 return this.category != null && !this.category.isEmpty(); 712 } 713 714 /** 715 * @param value {@link #category} (The type of message to be sent such as alert, 716 * notification, reminder, instruction, etc.) 717 */ 718 public CommunicationRequest setCategory(CodeableConcept value) { 719 this.category = value; 720 return this; 721 } 722 723 /** 724 * @return {@link #sender} (The entity (e.g. person, organization, clinical 725 * information system, or device) which is to be the source of the 726 * communication.) 727 */ 728 public Reference getSender() { 729 if (this.sender == null) 730 if (Configuration.errorOnAutoCreate()) 731 throw new Error("Attempt to auto-create CommunicationRequest.sender"); 732 else if (Configuration.doAutoCreate()) 733 this.sender = new Reference(); // cc 734 return this.sender; 735 } 736 737 public boolean hasSender() { 738 return this.sender != null && !this.sender.isEmpty(); 739 } 740 741 /** 742 * @param value {@link #sender} (The entity (e.g. person, organization, clinical 743 * information system, or device) which is to be the source of the 744 * communication.) 745 */ 746 public CommunicationRequest setSender(Reference value) { 747 this.sender = value; 748 return this; 749 } 750 751 /** 752 * @return {@link #sender} The actual object that is the target of the 753 * reference. The reference library doesn't populate this, but you can 754 * use it to hold the resource if you resolve it. (The entity (e.g. 755 * person, organization, clinical information system, or device) which 756 * is to be the source of the communication.) 757 */ 758 public Resource getSenderTarget() { 759 return this.senderTarget; 760 } 761 762 /** 763 * @param value {@link #sender} The actual object that is the target of the 764 * reference. The reference library doesn't use these, but you can 765 * use it to hold the resource if you resolve it. (The entity (e.g. 766 * person, organization, clinical information system, or device) 767 * which is to be the source of the communication.) 768 */ 769 public CommunicationRequest setSenderTarget(Resource value) { 770 this.senderTarget = value; 771 return this; 772 } 773 774 /** 775 * @return {@link #recipient} (The entity (e.g. person, organization, clinical 776 * information system, or device) which is the intended target of the 777 * communication.) 778 */ 779 public List<Reference> getRecipient() { 780 if (this.recipient == null) 781 this.recipient = new ArrayList<Reference>(); 782 return this.recipient; 783 } 784 785 public boolean hasRecipient() { 786 if (this.recipient == null) 787 return false; 788 for (Reference item : this.recipient) 789 if (!item.isEmpty()) 790 return true; 791 return false; 792 } 793 794 /** 795 * @return {@link #recipient} (The entity (e.g. person, organization, clinical 796 * information system, or device) which is the intended target of the 797 * communication.) 798 */ 799 // syntactic sugar 800 public Reference addRecipient() { // 3 801 Reference t = new Reference(); 802 if (this.recipient == null) 803 this.recipient = new ArrayList<Reference>(); 804 this.recipient.add(t); 805 return t; 806 } 807 808 // syntactic sugar 809 public CommunicationRequest addRecipient(Reference t) { // 3 810 if (t == null) 811 return this; 812 if (this.recipient == null) 813 this.recipient = new ArrayList<Reference>(); 814 this.recipient.add(t); 815 return this; 816 } 817 818 /** 819 * @return {@link #recipient} (The actual objects that are the target of the 820 * reference. The reference library doesn't populate this, but you can 821 * use this to hold the resources if you resolvethemt. The entity (e.g. 822 * person, organization, clinical information system, or device) which 823 * is the intended target of the communication.) 824 */ 825 public List<Resource> getRecipientTarget() { 826 if (this.recipientTarget == null) 827 this.recipientTarget = new ArrayList<Resource>(); 828 return this.recipientTarget; 829 } 830 831 /** 832 * @return {@link #payload} (Text, attachment(s), or resource(s) to be 833 * communicated to the recipient.) 834 */ 835 public List<CommunicationRequestPayloadComponent> getPayload() { 836 if (this.payload == null) 837 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 838 return this.payload; 839 } 840 841 public boolean hasPayload() { 842 if (this.payload == null) 843 return false; 844 for (CommunicationRequestPayloadComponent item : this.payload) 845 if (!item.isEmpty()) 846 return true; 847 return false; 848 } 849 850 /** 851 * @return {@link #payload} (Text, attachment(s), or resource(s) to be 852 * communicated to the recipient.) 853 */ 854 // syntactic sugar 855 public CommunicationRequestPayloadComponent addPayload() { // 3 856 CommunicationRequestPayloadComponent t = new CommunicationRequestPayloadComponent(); 857 if (this.payload == null) 858 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 859 this.payload.add(t); 860 return t; 861 } 862 863 // syntactic sugar 864 public CommunicationRequest addPayload(CommunicationRequestPayloadComponent t) { // 3 865 if (t == null) 866 return this; 867 if (this.payload == null) 868 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 869 this.payload.add(t); 870 return this; 871 } 872 873 /** 874 * @return {@link #medium} (A channel that was used for this communication (e.g. 875 * email, fax).) 876 */ 877 public List<CodeableConcept> getMedium() { 878 if (this.medium == null) 879 this.medium = new ArrayList<CodeableConcept>(); 880 return this.medium; 881 } 882 883 public boolean hasMedium() { 884 if (this.medium == null) 885 return false; 886 for (CodeableConcept item : this.medium) 887 if (!item.isEmpty()) 888 return true; 889 return false; 890 } 891 892 /** 893 * @return {@link #medium} (A channel that was used for this communication (e.g. 894 * email, fax).) 895 */ 896 // syntactic sugar 897 public CodeableConcept addMedium() { // 3 898 CodeableConcept t = new CodeableConcept(); 899 if (this.medium == null) 900 this.medium = new ArrayList<CodeableConcept>(); 901 this.medium.add(t); 902 return t; 903 } 904 905 // syntactic sugar 906 public CommunicationRequest addMedium(CodeableConcept t) { // 3 907 if (t == null) 908 return this; 909 if (this.medium == null) 910 this.medium = new ArrayList<CodeableConcept>(); 911 this.medium.add(t); 912 return this; 913 } 914 915 /** 916 * @return {@link #requester} (The responsible person who authorizes this order, 917 * e.g. physician. This may be different than the author of the order 918 * statement, e.g. clerk, who may have entered the statement into the 919 * order entry application.) 920 */ 921 public Reference getRequester() { 922 if (this.requester == null) 923 if (Configuration.errorOnAutoCreate()) 924 throw new Error("Attempt to auto-create CommunicationRequest.requester"); 925 else if (Configuration.doAutoCreate()) 926 this.requester = new Reference(); // cc 927 return this.requester; 928 } 929 930 public boolean hasRequester() { 931 return this.requester != null && !this.requester.isEmpty(); 932 } 933 934 /** 935 * @param value {@link #requester} (The responsible person who authorizes this 936 * order, e.g. physician. This may be different than the author of 937 * the order statement, e.g. clerk, who may have entered the 938 * statement into the order entry application.) 939 */ 940 public CommunicationRequest setRequester(Reference value) { 941 this.requester = value; 942 return this; 943 } 944 945 /** 946 * @return {@link #requester} The actual object that is the target of the 947 * reference. The reference library doesn't populate this, but you can 948 * use it to hold the resource if you resolve it. (The responsible 949 * person who authorizes this order, e.g. physician. This may be 950 * different than the author of the order statement, e.g. clerk, who may 951 * have entered the statement into the order entry application.) 952 */ 953 public Resource getRequesterTarget() { 954 return this.requesterTarget; 955 } 956 957 /** 958 * @param value {@link #requester} The actual object that is the target of the 959 * reference. The reference library doesn't use these, but you can 960 * use it to hold the resource if you resolve it. (The responsible 961 * person who authorizes this order, e.g. physician. This may be 962 * different than the author of the order statement, e.g. clerk, 963 * who may have entered the statement into the order entry 964 * application.) 965 */ 966 public CommunicationRequest setRequesterTarget(Resource value) { 967 this.requesterTarget = value; 968 return this; 969 } 970 971 /** 972 * @return {@link #status} (The status of the proposal or order.). This is the 973 * underlying object with id, value and extensions. The accessor 974 * "getStatus" gives direct access to the value 975 */ 976 public Enumeration<CommunicationRequestStatus> getStatusElement() { 977 if (this.status == null) 978 if (Configuration.errorOnAutoCreate()) 979 throw new Error("Attempt to auto-create CommunicationRequest.status"); 980 else if (Configuration.doAutoCreate()) 981 this.status = new Enumeration<CommunicationRequestStatus>(new CommunicationRequestStatusEnumFactory()); // bb 982 return this.status; 983 } 984 985 public boolean hasStatusElement() { 986 return this.status != null && !this.status.isEmpty(); 987 } 988 989 public boolean hasStatus() { 990 return this.status != null && !this.status.isEmpty(); 991 } 992 993 /** 994 * @param value {@link #status} (The status of the proposal or order.). This is 995 * the underlying object with id, value and extensions. The 996 * accessor "getStatus" gives direct access to the value 997 */ 998 public CommunicationRequest setStatusElement(Enumeration<CommunicationRequestStatus> value) { 999 this.status = value; 1000 return this; 1001 } 1002 1003 /** 1004 * @return The status of the proposal or order. 1005 */ 1006 public CommunicationRequestStatus getStatus() { 1007 return this.status == null ? null : this.status.getValue(); 1008 } 1009 1010 /** 1011 * @param value The status of the proposal or order. 1012 */ 1013 public CommunicationRequest setStatus(CommunicationRequestStatus value) { 1014 if (value == null) 1015 this.status = null; 1016 else { 1017 if (this.status == null) 1018 this.status = new Enumeration<CommunicationRequestStatus>(new CommunicationRequestStatusEnumFactory()); 1019 this.status.setValue(value); 1020 } 1021 return this; 1022 } 1023 1024 /** 1025 * @return {@link #encounter} (The encounter within which the communication 1026 * request was created.) 1027 */ 1028 public Reference getEncounter() { 1029 if (this.encounter == null) 1030 if (Configuration.errorOnAutoCreate()) 1031 throw new Error("Attempt to auto-create CommunicationRequest.encounter"); 1032 else if (Configuration.doAutoCreate()) 1033 this.encounter = new Reference(); // cc 1034 return this.encounter; 1035 } 1036 1037 public boolean hasEncounter() { 1038 return this.encounter != null && !this.encounter.isEmpty(); 1039 } 1040 1041 /** 1042 * @param value {@link #encounter} (The encounter within which the communication 1043 * request was created.) 1044 */ 1045 public CommunicationRequest setEncounter(Reference value) { 1046 this.encounter = value; 1047 return this; 1048 } 1049 1050 /** 1051 * @return {@link #encounter} The actual object that is the target of the 1052 * reference. The reference library doesn't populate this, but you can 1053 * use it to hold the resource if you resolve it. (The encounter within 1054 * which the communication request was created.) 1055 */ 1056 public Encounter getEncounterTarget() { 1057 if (this.encounterTarget == null) 1058 if (Configuration.errorOnAutoCreate()) 1059 throw new Error("Attempt to auto-create CommunicationRequest.encounter"); 1060 else if (Configuration.doAutoCreate()) 1061 this.encounterTarget = new Encounter(); // aa 1062 return this.encounterTarget; 1063 } 1064 1065 /** 1066 * @param value {@link #encounter} The actual object that is the target of the 1067 * reference. The reference library doesn't use these, but you can 1068 * use it to hold the resource if you resolve it. (The encounter 1069 * within which the communication request was created.) 1070 */ 1071 public CommunicationRequest setEncounterTarget(Encounter value) { 1072 this.encounterTarget = value; 1073 return this; 1074 } 1075 1076 /** 1077 * @return {@link #scheduled} (The time when this communication is to occur.) 1078 */ 1079 public Type getScheduled() { 1080 return this.scheduled; 1081 } 1082 1083 /** 1084 * @return {@link #scheduled} (The time when this communication is to occur.) 1085 */ 1086 public DateTimeType getScheduledDateTimeType() throws FHIRException { 1087 if (!(this.scheduled instanceof DateTimeType)) 1088 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1089 + this.scheduled.getClass().getName() + " was encountered"); 1090 return (DateTimeType) this.scheduled; 1091 } 1092 1093 public boolean hasScheduledDateTimeType() { 1094 return this.scheduled instanceof DateTimeType; 1095 } 1096 1097 /** 1098 * @return {@link #scheduled} (The time when this communication is to occur.) 1099 */ 1100 public Period getScheduledPeriod() throws FHIRException { 1101 if (!(this.scheduled instanceof Period)) 1102 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.scheduled.getClass().getName() 1103 + " was encountered"); 1104 return (Period) this.scheduled; 1105 } 1106 1107 public boolean hasScheduledPeriod() { 1108 return this.scheduled instanceof Period; 1109 } 1110 1111 public boolean hasScheduled() { 1112 return this.scheduled != null && !this.scheduled.isEmpty(); 1113 } 1114 1115 /** 1116 * @param value {@link #scheduled} (The time when this communication is to 1117 * occur.) 1118 */ 1119 public CommunicationRequest setScheduled(Type value) { 1120 this.scheduled = value; 1121 return this; 1122 } 1123 1124 /** 1125 * @return {@link #reason} (The reason or justification for the communication 1126 * request.) 1127 */ 1128 public List<CodeableConcept> getReason() { 1129 if (this.reason == null) 1130 this.reason = new ArrayList<CodeableConcept>(); 1131 return this.reason; 1132 } 1133 1134 public boolean hasReason() { 1135 if (this.reason == null) 1136 return false; 1137 for (CodeableConcept item : this.reason) 1138 if (!item.isEmpty()) 1139 return true; 1140 return false; 1141 } 1142 1143 /** 1144 * @return {@link #reason} (The reason or justification for the communication 1145 * request.) 1146 */ 1147 // syntactic sugar 1148 public CodeableConcept addReason() { // 3 1149 CodeableConcept t = new CodeableConcept(); 1150 if (this.reason == null) 1151 this.reason = new ArrayList<CodeableConcept>(); 1152 this.reason.add(t); 1153 return t; 1154 } 1155 1156 // syntactic sugar 1157 public CommunicationRequest addReason(CodeableConcept t) { // 3 1158 if (t == null) 1159 return this; 1160 if (this.reason == null) 1161 this.reason = new ArrayList<CodeableConcept>(); 1162 this.reason.add(t); 1163 return this; 1164 } 1165 1166 /** 1167 * @return {@link #requestedOn} (The time when the request was made.). This is 1168 * the underlying object with id, value and extensions. The accessor 1169 * "getRequestedOn" gives direct access to the value 1170 */ 1171 public DateTimeType getRequestedOnElement() { 1172 if (this.requestedOn == null) 1173 if (Configuration.errorOnAutoCreate()) 1174 throw new Error("Attempt to auto-create CommunicationRequest.requestedOn"); 1175 else if (Configuration.doAutoCreate()) 1176 this.requestedOn = new DateTimeType(); // bb 1177 return this.requestedOn; 1178 } 1179 1180 public boolean hasRequestedOnElement() { 1181 return this.requestedOn != null && !this.requestedOn.isEmpty(); 1182 } 1183 1184 public boolean hasRequestedOn() { 1185 return this.requestedOn != null && !this.requestedOn.isEmpty(); 1186 } 1187 1188 /** 1189 * @param value {@link #requestedOn} (The time when the request was made.). This 1190 * is the underlying object with id, value and extensions. The 1191 * accessor "getRequestedOn" gives direct access to the value 1192 */ 1193 public CommunicationRequest setRequestedOnElement(DateTimeType value) { 1194 this.requestedOn = value; 1195 return this; 1196 } 1197 1198 /** 1199 * @return The time when the request was made. 1200 */ 1201 public Date getRequestedOn() { 1202 return this.requestedOn == null ? null : this.requestedOn.getValue(); 1203 } 1204 1205 /** 1206 * @param value The time when the request was made. 1207 */ 1208 public CommunicationRequest setRequestedOn(Date value) { 1209 if (value == null) 1210 this.requestedOn = null; 1211 else { 1212 if (this.requestedOn == null) 1213 this.requestedOn = new DateTimeType(); 1214 this.requestedOn.setValue(value); 1215 } 1216 return this; 1217 } 1218 1219 /** 1220 * @return {@link #subject} (The patient who is the focus of this communication 1221 * request.) 1222 */ 1223 public Reference getSubject() { 1224 if (this.subject == null) 1225 if (Configuration.errorOnAutoCreate()) 1226 throw new Error("Attempt to auto-create CommunicationRequest.subject"); 1227 else if (Configuration.doAutoCreate()) 1228 this.subject = new Reference(); // cc 1229 return this.subject; 1230 } 1231 1232 public boolean hasSubject() { 1233 return this.subject != null && !this.subject.isEmpty(); 1234 } 1235 1236 /** 1237 * @param value {@link #subject} (The patient who is the focus of this 1238 * communication request.) 1239 */ 1240 public CommunicationRequest setSubject(Reference value) { 1241 this.subject = value; 1242 return this; 1243 } 1244 1245 /** 1246 * @return {@link #subject} The actual object that is the target of the 1247 * reference. The reference library doesn't populate this, but you can 1248 * use it to hold the resource if you resolve it. (The patient who is 1249 * the focus of this communication request.) 1250 */ 1251 public Patient getSubjectTarget() { 1252 if (this.subjectTarget == null) 1253 if (Configuration.errorOnAutoCreate()) 1254 throw new Error("Attempt to auto-create CommunicationRequest.subject"); 1255 else if (Configuration.doAutoCreate()) 1256 this.subjectTarget = new Patient(); // aa 1257 return this.subjectTarget; 1258 } 1259 1260 /** 1261 * @param value {@link #subject} The actual object that is the target of the 1262 * reference. The reference library doesn't use these, but you can 1263 * use it to hold the resource if you resolve it. (The patient who 1264 * is the focus of this communication request.) 1265 */ 1266 public CommunicationRequest setSubjectTarget(Patient value) { 1267 this.subjectTarget = value; 1268 return this; 1269 } 1270 1271 /** 1272 * @return {@link #priority} (Characterizes how quickly the proposed act must be 1273 * initiated. Includes concepts such as stat, urgent, routine.) 1274 */ 1275 public CodeableConcept getPriority() { 1276 if (this.priority == null) 1277 if (Configuration.errorOnAutoCreate()) 1278 throw new Error("Attempt to auto-create CommunicationRequest.priority"); 1279 else if (Configuration.doAutoCreate()) 1280 this.priority = new CodeableConcept(); // cc 1281 return this.priority; 1282 } 1283 1284 public boolean hasPriority() { 1285 return this.priority != null && !this.priority.isEmpty(); 1286 } 1287 1288 /** 1289 * @param value {@link #priority} (Characterizes how quickly the proposed act 1290 * must be initiated. Includes concepts such as stat, urgent, 1291 * routine.) 1292 */ 1293 public CommunicationRequest setPriority(CodeableConcept value) { 1294 this.priority = value; 1295 return this; 1296 } 1297 1298 protected void listChildren(List<Property> childrenList) { 1299 super.listChildren(childrenList); 1300 childrenList.add(new Property("identifier", "Identifier", 1301 "A unique ID of this request for reference purposes. It must be provided if user wants it returned as part of any output, otherwise it will be autogenerated, if needed, by CDS system. Does not need to be the actual ID of the source system.", 1302 0, java.lang.Integer.MAX_VALUE, identifier)); 1303 childrenList.add(new Property("category", "CodeableConcept", 1304 "The type of message to be sent such as alert, notification, reminder, instruction, etc.", 0, 1305 java.lang.Integer.MAX_VALUE, category)); 1306 childrenList.add(new Property("sender", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson)", 1307 "The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.", 1308 0, java.lang.Integer.MAX_VALUE, sender)); 1309 childrenList.add(new Property("recipient", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson)", 1310 "The entity (e.g. person, organization, clinical information system, or device) which is the intended target of the communication.", 1311 0, java.lang.Integer.MAX_VALUE, recipient)); 1312 childrenList 1313 .add(new Property("payload", "", "Text, attachment(s), or resource(s) to be communicated to the recipient.", 0, 1314 java.lang.Integer.MAX_VALUE, payload)); 1315 childrenList.add(new Property("medium", "CodeableConcept", 1316 "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium)); 1317 childrenList.add(new Property("requester", "Reference(Practitioner|Patient|RelatedPerson)", 1318 "The responsible person who authorizes this order, e.g. physician. This may be different than the author of the order statement, e.g. clerk, who may have entered the statement into the order entry application.", 1319 0, java.lang.Integer.MAX_VALUE, requester)); 1320 childrenList.add( 1321 new Property("status", "code", "The status of the proposal or order.", 0, java.lang.Integer.MAX_VALUE, status)); 1322 childrenList.add(new Property("encounter", "Reference(Encounter)", 1323 "The encounter within which the communication request was created.", 0, java.lang.Integer.MAX_VALUE, 1324 encounter)); 1325 childrenList.add(new Property("scheduled[x]", "dateTime|Period", "The time when this communication is to occur.", 0, 1326 java.lang.Integer.MAX_VALUE, scheduled)); 1327 childrenList.add(new Property("reason", "CodeableConcept", 1328 "The reason or justification for the communication request.", 0, java.lang.Integer.MAX_VALUE, reason)); 1329 childrenList.add(new Property("requestedOn", "dateTime", "The time when the request was made.", 0, 1330 java.lang.Integer.MAX_VALUE, requestedOn)); 1331 childrenList.add(new Property("subject", "Reference(Patient)", 1332 "The patient who is the focus of this communication request.", 0, java.lang.Integer.MAX_VALUE, subject)); 1333 childrenList.add(new Property("priority", "CodeableConcept", 1334 "Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.", 1335 0, java.lang.Integer.MAX_VALUE, priority)); 1336 } 1337 1338 @Override 1339 public void setProperty(String name, Base value) throws FHIRException { 1340 if (name.equals("identifier")) 1341 this.getIdentifier().add(castToIdentifier(value)); 1342 else if (name.equals("category")) 1343 this.category = castToCodeableConcept(value); // CodeableConcept 1344 else if (name.equals("sender")) 1345 this.sender = castToReference(value); // Reference 1346 else if (name.equals("recipient")) 1347 this.getRecipient().add(castToReference(value)); 1348 else if (name.equals("payload")) 1349 this.getPayload().add((CommunicationRequestPayloadComponent) value); 1350 else if (name.equals("medium")) 1351 this.getMedium().add(castToCodeableConcept(value)); 1352 else if (name.equals("requester")) 1353 this.requester = castToReference(value); // Reference 1354 else if (name.equals("status")) 1355 this.status = new CommunicationRequestStatusEnumFactory().fromType(value); // Enumeration<CommunicationRequestStatus> 1356 else if (name.equals("encounter")) 1357 this.encounter = castToReference(value); // Reference 1358 else if (name.equals("scheduled[x]")) 1359 this.scheduled = (Type) value; // Type 1360 else if (name.equals("reason")) 1361 this.getReason().add(castToCodeableConcept(value)); 1362 else if (name.equals("requestedOn")) 1363 this.requestedOn = castToDateTime(value); // DateTimeType 1364 else if (name.equals("subject")) 1365 this.subject = castToReference(value); // Reference 1366 else if (name.equals("priority")) 1367 this.priority = castToCodeableConcept(value); // CodeableConcept 1368 else 1369 super.setProperty(name, value); 1370 } 1371 1372 @Override 1373 public Base addChild(String name) throws FHIRException { 1374 if (name.equals("identifier")) { 1375 return addIdentifier(); 1376 } else if (name.equals("category")) { 1377 this.category = new CodeableConcept(); 1378 return this.category; 1379 } else if (name.equals("sender")) { 1380 this.sender = new Reference(); 1381 return this.sender; 1382 } else if (name.equals("recipient")) { 1383 return addRecipient(); 1384 } else if (name.equals("payload")) { 1385 return addPayload(); 1386 } else if (name.equals("medium")) { 1387 return addMedium(); 1388 } else if (name.equals("requester")) { 1389 this.requester = new Reference(); 1390 return this.requester; 1391 } else if (name.equals("status")) { 1392 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.status"); 1393 } else if (name.equals("encounter")) { 1394 this.encounter = new Reference(); 1395 return this.encounter; 1396 } else if (name.equals("scheduledDateTime")) { 1397 this.scheduled = new DateTimeType(); 1398 return this.scheduled; 1399 } else if (name.equals("scheduledPeriod")) { 1400 this.scheduled = new Period(); 1401 return this.scheduled; 1402 } else if (name.equals("reason")) { 1403 return addReason(); 1404 } else if (name.equals("requestedOn")) { 1405 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.requestedOn"); 1406 } else if (name.equals("subject")) { 1407 this.subject = new Reference(); 1408 return this.subject; 1409 } else if (name.equals("priority")) { 1410 this.priority = new CodeableConcept(); 1411 return this.priority; 1412 } else 1413 return super.addChild(name); 1414 } 1415 1416 public String fhirType() { 1417 return "CommunicationRequest"; 1418 1419 } 1420 1421 public CommunicationRequest copy() { 1422 CommunicationRequest dst = new CommunicationRequest(); 1423 copyValues(dst); 1424 if (identifier != null) { 1425 dst.identifier = new ArrayList<Identifier>(); 1426 for (Identifier i : identifier) 1427 dst.identifier.add(i.copy()); 1428 } 1429 ; 1430 dst.category = category == null ? null : category.copy(); 1431 dst.sender = sender == null ? null : sender.copy(); 1432 if (recipient != null) { 1433 dst.recipient = new ArrayList<Reference>(); 1434 for (Reference i : recipient) 1435 dst.recipient.add(i.copy()); 1436 } 1437 ; 1438 if (payload != null) { 1439 dst.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1440 for (CommunicationRequestPayloadComponent i : payload) 1441 dst.payload.add(i.copy()); 1442 } 1443 ; 1444 if (medium != null) { 1445 dst.medium = new ArrayList<CodeableConcept>(); 1446 for (CodeableConcept i : medium) 1447 dst.medium.add(i.copy()); 1448 } 1449 ; 1450 dst.requester = requester == null ? null : requester.copy(); 1451 dst.status = status == null ? null : status.copy(); 1452 dst.encounter = encounter == null ? null : encounter.copy(); 1453 dst.scheduled = scheduled == null ? null : scheduled.copy(); 1454 if (reason != null) { 1455 dst.reason = new ArrayList<CodeableConcept>(); 1456 for (CodeableConcept i : reason) 1457 dst.reason.add(i.copy()); 1458 } 1459 ; 1460 dst.requestedOn = requestedOn == null ? null : requestedOn.copy(); 1461 dst.subject = subject == null ? null : subject.copy(); 1462 dst.priority = priority == null ? null : priority.copy(); 1463 return dst; 1464 } 1465 1466 protected CommunicationRequest typedCopy() { 1467 return copy(); 1468 } 1469 1470 @Override 1471 public boolean equalsDeep(Base other) { 1472 if (!super.equalsDeep(other)) 1473 return false; 1474 if (!(other instanceof CommunicationRequest)) 1475 return false; 1476 CommunicationRequest o = (CommunicationRequest) other; 1477 return compareDeep(identifier, o.identifier, true) && compareDeep(category, o.category, true) 1478 && compareDeep(sender, o.sender, true) && compareDeep(recipient, o.recipient, true) 1479 && compareDeep(payload, o.payload, true) && compareDeep(medium, o.medium, true) 1480 && compareDeep(requester, o.requester, true) && compareDeep(status, o.status, true) 1481 && compareDeep(encounter, o.encounter, true) && compareDeep(scheduled, o.scheduled, true) 1482 && compareDeep(reason, o.reason, true) && compareDeep(requestedOn, o.requestedOn, true) 1483 && compareDeep(subject, o.subject, true) && compareDeep(priority, o.priority, true); 1484 } 1485 1486 @Override 1487 public boolean equalsShallow(Base other) { 1488 if (!super.equalsShallow(other)) 1489 return false; 1490 if (!(other instanceof CommunicationRequest)) 1491 return false; 1492 CommunicationRequest o = (CommunicationRequest) other; 1493 return compareValues(status, o.status, true) && compareValues(requestedOn, o.requestedOn, true); 1494 } 1495 1496 public boolean isEmpty() { 1497 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (category == null || category.isEmpty()) 1498 && (sender == null || sender.isEmpty()) && (recipient == null || recipient.isEmpty()) 1499 && (payload == null || payload.isEmpty()) && (medium == null || medium.isEmpty()) 1500 && (requester == null || requester.isEmpty()) && (status == null || status.isEmpty()) 1501 && (encounter == null || encounter.isEmpty()) && (scheduled == null || scheduled.isEmpty()) 1502 && (reason == null || reason.isEmpty()) && (requestedOn == null || requestedOn.isEmpty()) 1503 && (subject == null || subject.isEmpty()) && (priority == null || priority.isEmpty()); 1504 } 1505 1506 @Override 1507 public ResourceType getResourceType() { 1508 return ResourceType.CommunicationRequest; 1509 } 1510 1511 @SearchParamDefinition(name = "requester", path = "CommunicationRequest.requester", description = "An individual who requested a communication", type = "reference") 1512 public static final String SP_REQUESTER = "requester"; 1513 @SearchParamDefinition(name = "identifier", path = "CommunicationRequest.identifier", description = "Unique identifier", type = "token") 1514 public static final String SP_IDENTIFIER = "identifier"; 1515 @SearchParamDefinition(name = "subject", path = "CommunicationRequest.subject", description = "Focus of message", type = "reference") 1516 public static final String SP_SUBJECT = "subject"; 1517 @SearchParamDefinition(name = "medium", path = "CommunicationRequest.medium", description = "A channel of communication", type = "token") 1518 public static final String SP_MEDIUM = "medium"; 1519 @SearchParamDefinition(name = "encounter", path = "CommunicationRequest.encounter", description = "Encounter leading to message", type = "reference") 1520 public static final String SP_ENCOUNTER = "encounter"; 1521 @SearchParamDefinition(name = "priority", path = "CommunicationRequest.priority", description = "Message urgency", type = "token") 1522 public static final String SP_PRIORITY = "priority"; 1523 @SearchParamDefinition(name = "requested", path = "CommunicationRequest.requestedOn", description = "When ordered or proposed", type = "date") 1524 public static final String SP_REQUESTED = "requested"; 1525 @SearchParamDefinition(name = "sender", path = "CommunicationRequest.sender", description = "Message sender", type = "reference") 1526 public static final String SP_SENDER = "sender"; 1527 @SearchParamDefinition(name = "patient", path = "CommunicationRequest.subject", description = "Focus of message", type = "reference") 1528 public static final String SP_PATIENT = "patient"; 1529 @SearchParamDefinition(name = "recipient", path = "CommunicationRequest.recipient", description = "Message recipient", type = "reference") 1530 public static final String SP_RECIPIENT = "recipient"; 1531 @SearchParamDefinition(name = "time", path = "CommunicationRequest.scheduledDateTime", description = "When scheduled", type = "date") 1532 public static final String SP_TIME = "time"; 1533 @SearchParamDefinition(name = "category", path = "CommunicationRequest.category", description = "Message category", type = "token") 1534 public static final String SP_CATEGORY = "category"; 1535 @SearchParamDefinition(name = "status", path = "CommunicationRequest.status", description = "proposed | planned | requested | received | accepted | in-progress | completed | suspended | rejected | failed", type = "token") 1536 public static final String SP_STATUS = "status"; 1537 1538}