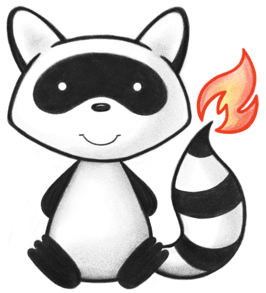
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * A set of healthcare-related information that is assembled together into a 048 * single logical document that provides a single coherent statement of meaning, 049 * establishes its own context and that has clinical attestation with regard to 050 * who is making the statement. While a Composition defines the structure, it 051 * does not actually contain the content: rather the full content of a document 052 * is contained in a Bundle, of which the Composition is the first resource 053 * contained. 054 */ 055@ResourceDef(name = "Composition", profile = "http://hl7.org/fhir/Profile/Composition") 056public class Composition extends DomainResource { 057 058 public enum CompositionStatus { 059 /** 060 * This is a preliminary composition or document (also known as initial or 061 * interim). The content may be incomplete or unverified. 062 */ 063 PRELIMINARY, 064 /** 065 * This version of the composition is complete and verified by an appropriate 066 * person and no further work is planned. Any subsequent updates would be on a 067 * new version of the composition. 068 */ 069 FINAL, 070 /** 071 * The composition content or the referenced resources have been modified 072 * (edited or added to) subsequent to being released as "final" and the 073 * composition is complete and verified by an authorized person. 074 */ 075 AMENDED, 076 /** 077 * The composition or document was originally created/issued in error, and this 078 * is an amendment that marks that the entire series should not be considered as 079 * valid. 080 */ 081 ENTEREDINERROR, 082 /** 083 * added to help the parsers 084 */ 085 NULL; 086 087 public static CompositionStatus fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("preliminary".equals(codeString)) 091 return PRELIMINARY; 092 if ("final".equals(codeString)) 093 return FINAL; 094 if ("amended".equals(codeString)) 095 return AMENDED; 096 if ("entered-in-error".equals(codeString)) 097 return ENTEREDINERROR; 098 throw new FHIRException("Unknown CompositionStatus code '" + codeString + "'"); 099 } 100 101 public String toCode() { 102 switch (this) { 103 case PRELIMINARY: 104 return "preliminary"; 105 case FINAL: 106 return "final"; 107 case AMENDED: 108 return "amended"; 109 case ENTEREDINERROR: 110 return "entered-in-error"; 111 case NULL: 112 return null; 113 default: 114 return "?"; 115 } 116 } 117 118 public String getSystem() { 119 switch (this) { 120 case PRELIMINARY: 121 return "http://hl7.org/fhir/composition-status"; 122 case FINAL: 123 return "http://hl7.org/fhir/composition-status"; 124 case AMENDED: 125 return "http://hl7.org/fhir/composition-status"; 126 case ENTEREDINERROR: 127 return "http://hl7.org/fhir/composition-status"; 128 case NULL: 129 return null; 130 default: 131 return "?"; 132 } 133 } 134 135 public String getDefinition() { 136 switch (this) { 137 case PRELIMINARY: 138 return "This is a preliminary composition or document (also known as initial or interim). The content may be incomplete or unverified."; 139 case FINAL: 140 return "This version of the composition is complete and verified by an appropriate person and no further work is planned. Any subsequent updates would be on a new version of the composition."; 141 case AMENDED: 142 return "The composition content or the referenced resources have been modified (edited or added to) subsequent to being released as \"final\" and the composition is complete and verified by an authorized person."; 143 case ENTEREDINERROR: 144 return "The composition or document was originally created/issued in error, and this is an amendment that marks that the entire series should not be considered as valid."; 145 case NULL: 146 return null; 147 default: 148 return "?"; 149 } 150 } 151 152 public String getDisplay() { 153 switch (this) { 154 case PRELIMINARY: 155 return "Preliminary"; 156 case FINAL: 157 return "Final"; 158 case AMENDED: 159 return "Amended"; 160 case ENTEREDINERROR: 161 return "Entered in Error"; 162 case NULL: 163 return null; 164 default: 165 return "?"; 166 } 167 } 168 } 169 170 public static class CompositionStatusEnumFactory implements EnumFactory<CompositionStatus> { 171 public CompositionStatus fromCode(String codeString) throws IllegalArgumentException { 172 if (codeString == null || "".equals(codeString)) 173 if (codeString == null || "".equals(codeString)) 174 return null; 175 if ("preliminary".equals(codeString)) 176 return CompositionStatus.PRELIMINARY; 177 if ("final".equals(codeString)) 178 return CompositionStatus.FINAL; 179 if ("amended".equals(codeString)) 180 return CompositionStatus.AMENDED; 181 if ("entered-in-error".equals(codeString)) 182 return CompositionStatus.ENTEREDINERROR; 183 throw new IllegalArgumentException("Unknown CompositionStatus code '" + codeString + "'"); 184 } 185 186 public Enumeration<CompositionStatus> fromType(Base code) throws FHIRException { 187 if (code == null || code.isEmpty()) 188 return null; 189 String codeString = ((PrimitiveType) code).asStringValue(); 190 if (codeString == null || "".equals(codeString)) 191 return null; 192 if ("preliminary".equals(codeString)) 193 return new Enumeration<CompositionStatus>(this, CompositionStatus.PRELIMINARY); 194 if ("final".equals(codeString)) 195 return new Enumeration<CompositionStatus>(this, CompositionStatus.FINAL); 196 if ("amended".equals(codeString)) 197 return new Enumeration<CompositionStatus>(this, CompositionStatus.AMENDED); 198 if ("entered-in-error".equals(codeString)) 199 return new Enumeration<CompositionStatus>(this, CompositionStatus.ENTEREDINERROR); 200 throw new FHIRException("Unknown CompositionStatus code '" + codeString + "'"); 201 } 202 203 public String toCode(CompositionStatus code) 204 { 205 if (code == CompositionStatus.NULL) 206 return null; 207 if (code == CompositionStatus.PRELIMINARY) 208 return "preliminary"; 209 if (code == CompositionStatus.FINAL) 210 return "final"; 211 if (code == CompositionStatus.AMENDED) 212 return "amended"; 213 if (code == CompositionStatus.ENTEREDINERROR) 214 return "entered-in-error"; 215 return "?"; 216 } 217 } 218 219 public enum CompositionAttestationMode { 220 /** 221 * The person authenticated the content in their personal capacity. 222 */ 223 PERSONAL, 224 /** 225 * The person authenticated the content in their professional capacity. 226 */ 227 PROFESSIONAL, 228 /** 229 * The person authenticated the content and accepted legal responsibility for 230 * its content. 231 */ 232 LEGAL, 233 /** 234 * The organization authenticated the content as consistent with their policies 235 * and procedures. 236 */ 237 OFFICIAL, 238 /** 239 * added to help the parsers 240 */ 241 NULL; 242 243 public static CompositionAttestationMode fromCode(String codeString) throws FHIRException { 244 if (codeString == null || "".equals(codeString)) 245 return null; 246 if ("personal".equals(codeString)) 247 return PERSONAL; 248 if ("professional".equals(codeString)) 249 return PROFESSIONAL; 250 if ("legal".equals(codeString)) 251 return LEGAL; 252 if ("official".equals(codeString)) 253 return OFFICIAL; 254 throw new FHIRException("Unknown CompositionAttestationMode code '" + codeString + "'"); 255 } 256 257 public String toCode() { 258 switch (this) { 259 case PERSONAL: 260 return "personal"; 261 case PROFESSIONAL: 262 return "professional"; 263 case LEGAL: 264 return "legal"; 265 case OFFICIAL: 266 return "official"; 267 case NULL: 268 return null; 269 default: 270 return "?"; 271 } 272 } 273 274 public String getSystem() { 275 switch (this) { 276 case PERSONAL: 277 return "http://hl7.org/fhir/composition-attestation-mode"; 278 case PROFESSIONAL: 279 return "http://hl7.org/fhir/composition-attestation-mode"; 280 case LEGAL: 281 return "http://hl7.org/fhir/composition-attestation-mode"; 282 case OFFICIAL: 283 return "http://hl7.org/fhir/composition-attestation-mode"; 284 case NULL: 285 return null; 286 default: 287 return "?"; 288 } 289 } 290 291 public String getDefinition() { 292 switch (this) { 293 case PERSONAL: 294 return "The person authenticated the content in their personal capacity."; 295 case PROFESSIONAL: 296 return "The person authenticated the content in their professional capacity."; 297 case LEGAL: 298 return "The person authenticated the content and accepted legal responsibility for its content."; 299 case OFFICIAL: 300 return "The organization authenticated the content as consistent with their policies and procedures."; 301 case NULL: 302 return null; 303 default: 304 return "?"; 305 } 306 } 307 308 public String getDisplay() { 309 switch (this) { 310 case PERSONAL: 311 return "Personal"; 312 case PROFESSIONAL: 313 return "Professional"; 314 case LEGAL: 315 return "Legal"; 316 case OFFICIAL: 317 return "Official"; 318 case NULL: 319 return null; 320 default: 321 return "?"; 322 } 323 } 324 } 325 326 public static class CompositionAttestationModeEnumFactory implements EnumFactory<CompositionAttestationMode> { 327 public CompositionAttestationMode fromCode(String codeString) throws IllegalArgumentException { 328 if (codeString == null || "".equals(codeString)) 329 if (codeString == null || "".equals(codeString)) 330 return null; 331 if ("personal".equals(codeString)) 332 return CompositionAttestationMode.PERSONAL; 333 if ("professional".equals(codeString)) 334 return CompositionAttestationMode.PROFESSIONAL; 335 if ("legal".equals(codeString)) 336 return CompositionAttestationMode.LEGAL; 337 if ("official".equals(codeString)) 338 return CompositionAttestationMode.OFFICIAL; 339 throw new IllegalArgumentException("Unknown CompositionAttestationMode code '" + codeString + "'"); 340 } 341 342 public Enumeration<CompositionAttestationMode> fromType(Base code) throws FHIRException { 343 if (code == null || code.isEmpty()) 344 return null; 345 String codeString = ((PrimitiveType) code).asStringValue(); 346 if (codeString == null || "".equals(codeString)) 347 return null; 348 if ("personal".equals(codeString)) 349 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.PERSONAL); 350 if ("professional".equals(codeString)) 351 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.PROFESSIONAL); 352 if ("legal".equals(codeString)) 353 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.LEGAL); 354 if ("official".equals(codeString)) 355 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.OFFICIAL); 356 throw new FHIRException("Unknown CompositionAttestationMode code '" + codeString + "'"); 357 } 358 359 public String toCode(CompositionAttestationMode code) 360 { 361 if (code == CompositionAttestationMode.NULL) 362 return null; 363 if (code == CompositionAttestationMode.PERSONAL) 364 return "personal"; 365 if (code == CompositionAttestationMode.PROFESSIONAL) 366 return "professional"; 367 if (code == CompositionAttestationMode.LEGAL) 368 return "legal"; 369 if (code == CompositionAttestationMode.OFFICIAL) 370 return "official"; 371 return "?"; 372 } 373 } 374 375 @Block() 376 public static class CompositionAttesterComponent extends BackboneElement implements IBaseBackboneElement { 377 /** 378 * The type of attestation the authenticator offers. 379 */ 380 @Child(name = "mode", type = { 381 CodeType.class }, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 382 @Description(shortDefinition = "personal | professional | legal | official", formalDefinition = "The type of attestation the authenticator offers.") 383 protected List<Enumeration<CompositionAttestationMode>> mode; 384 385 /** 386 * When composition was attested by the party. 387 */ 388 @Child(name = "time", type = { DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 389 @Description(shortDefinition = "When composition attested", formalDefinition = "When composition was attested by the party.") 390 protected DateTimeType time; 391 392 /** 393 * Who attested the composition in the specified way. 394 */ 395 @Child(name = "party", type = { Patient.class, Practitioner.class, 396 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 397 @Description(shortDefinition = "Who attested the composition", formalDefinition = "Who attested the composition in the specified way.") 398 protected Reference party; 399 400 /** 401 * The actual object that is the target of the reference (Who attested the 402 * composition in the specified way.) 403 */ 404 protected Resource partyTarget; 405 406 private static final long serialVersionUID = -436604745L; 407 408 /* 409 * Constructor 410 */ 411 public CompositionAttesterComponent() { 412 super(); 413 } 414 415 /** 416 * @return {@link #mode} (The type of attestation the authenticator offers.) 417 */ 418 public List<Enumeration<CompositionAttestationMode>> getMode() { 419 if (this.mode == null) 420 this.mode = new ArrayList<Enumeration<CompositionAttestationMode>>(); 421 return this.mode; 422 } 423 424 public boolean hasMode() { 425 if (this.mode == null) 426 return false; 427 for (Enumeration<CompositionAttestationMode> item : this.mode) 428 if (!item.isEmpty()) 429 return true; 430 return false; 431 } 432 433 /** 434 * @return Returns a reference to <code>this</code> for easy method chaining 435 */ 436 public CompositionAttesterComponent setMode(List<Enumeration<CompositionAttestationMode>> mode) { 437 this.mode = mode; 438 return this; 439 } 440 441 /** 442 * @return {@link #mode} (The type of attestation the authenticator offers.) 443 */ 444 // syntactic sugar 445 public Enumeration<CompositionAttestationMode> addModeElement() {// 2 446 Enumeration<CompositionAttestationMode> t = new Enumeration<CompositionAttestationMode>( 447 new CompositionAttestationModeEnumFactory()); 448 if (this.mode == null) 449 this.mode = new ArrayList<Enumeration<CompositionAttestationMode>>(); 450 this.mode.add(t); 451 return t; 452 } 453 454 /** 455 * @param value {@link #mode} (The type of attestation the authenticator 456 * offers.) 457 */ 458 public CompositionAttesterComponent addMode(CompositionAttestationMode value) { // 1 459 Enumeration<CompositionAttestationMode> t = new Enumeration<CompositionAttestationMode>( 460 new CompositionAttestationModeEnumFactory()); 461 t.setValue(value); 462 if (this.mode == null) 463 this.mode = new ArrayList<Enumeration<CompositionAttestationMode>>(); 464 this.mode.add(t); 465 return this; 466 } 467 468 /** 469 * @param value {@link #mode} (The type of attestation the authenticator 470 * offers.) 471 */ 472 public boolean hasMode(CompositionAttestationMode value) { 473 if (this.mode == null) 474 return false; 475 for (Enumeration<CompositionAttestationMode> v : this.mode) 476 if (v.equals(value)) // code 477 return true; 478 return false; 479 } 480 481 /** 482 * @return {@link #time} (When composition was attested by the party.). This is 483 * the underlying object with id, value and extensions. The accessor 484 * "getTime" gives direct access to the value 485 */ 486 public DateTimeType getTimeElement() { 487 if (this.time == null) 488 if (Configuration.errorOnAutoCreate()) 489 throw new Error("Attempt to auto-create CompositionAttesterComponent.time"); 490 else if (Configuration.doAutoCreate()) 491 this.time = new DateTimeType(); // bb 492 return this.time; 493 } 494 495 public boolean hasTimeElement() { 496 return this.time != null && !this.time.isEmpty(); 497 } 498 499 public boolean hasTime() { 500 return this.time != null && !this.time.isEmpty(); 501 } 502 503 /** 504 * @param value {@link #time} (When composition was attested by the party.). 505 * This is the underlying object with id, value and extensions. The 506 * accessor "getTime" gives direct access to the value 507 */ 508 public CompositionAttesterComponent setTimeElement(DateTimeType value) { 509 this.time = value; 510 return this; 511 } 512 513 /** 514 * @return When composition was attested by the party. 515 */ 516 public Date getTime() { 517 return this.time == null ? null : this.time.getValue(); 518 } 519 520 /** 521 * @param value When composition was attested by the party. 522 */ 523 public CompositionAttesterComponent setTime(Date value) { 524 if (value == null) 525 this.time = null; 526 else { 527 if (this.time == null) 528 this.time = new DateTimeType(); 529 this.time.setValue(value); 530 } 531 return this; 532 } 533 534 /** 535 * @return {@link #party} (Who attested the composition in the specified way.) 536 */ 537 public Reference getParty() { 538 if (this.party == null) 539 if (Configuration.errorOnAutoCreate()) 540 throw new Error("Attempt to auto-create CompositionAttesterComponent.party"); 541 else if (Configuration.doAutoCreate()) 542 this.party = new Reference(); // cc 543 return this.party; 544 } 545 546 public boolean hasParty() { 547 return this.party != null && !this.party.isEmpty(); 548 } 549 550 /** 551 * @param value {@link #party} (Who attested the composition in the specified 552 * way.) 553 */ 554 public CompositionAttesterComponent setParty(Reference value) { 555 this.party = value; 556 return this; 557 } 558 559 /** 560 * @return {@link #party} The actual object that is the target of the reference. 561 * The reference library doesn't populate this, but you can use it to 562 * hold the resource if you resolve it. (Who attested the composition in 563 * the specified way.) 564 */ 565 public Resource getPartyTarget() { 566 return this.partyTarget; 567 } 568 569 /** 570 * @param value {@link #party} The actual object that is the target of the 571 * reference. The reference library doesn't use these, but you can 572 * use it to hold the resource if you resolve it. (Who attested the 573 * composition in the specified way.) 574 */ 575 public CompositionAttesterComponent setPartyTarget(Resource value) { 576 this.partyTarget = value; 577 return this; 578 } 579 580 protected void listChildren(List<Property> childrenList) { 581 super.listChildren(childrenList); 582 childrenList.add(new Property("mode", "code", "The type of attestation the authenticator offers.", 0, 583 java.lang.Integer.MAX_VALUE, mode)); 584 childrenList.add(new Property("time", "dateTime", "When composition was attested by the party.", 0, 585 java.lang.Integer.MAX_VALUE, time)); 586 childrenList.add(new Property("party", "Reference(Patient|Practitioner|Organization)", 587 "Who attested the composition in the specified way.", 0, java.lang.Integer.MAX_VALUE, party)); 588 } 589 590 @Override 591 public void setProperty(String name, Base value) throws FHIRException { 592 if (name.equals("mode")) 593 this.getMode().add(new CompositionAttestationModeEnumFactory().fromType(value)); 594 else if (name.equals("time")) 595 this.time = castToDateTime(value); // DateTimeType 596 else if (name.equals("party")) 597 this.party = castToReference(value); // Reference 598 else 599 super.setProperty(name, value); 600 } 601 602 @Override 603 public Base addChild(String name) throws FHIRException { 604 if (name.equals("mode")) { 605 throw new FHIRException("Cannot call addChild on a singleton property Composition.mode"); 606 } else if (name.equals("time")) { 607 throw new FHIRException("Cannot call addChild on a singleton property Composition.time"); 608 } else if (name.equals("party")) { 609 this.party = new Reference(); 610 return this.party; 611 } else 612 return super.addChild(name); 613 } 614 615 public CompositionAttesterComponent copy() { 616 CompositionAttesterComponent dst = new CompositionAttesterComponent(); 617 copyValues(dst); 618 if (mode != null) { 619 dst.mode = new ArrayList<Enumeration<CompositionAttestationMode>>(); 620 for (Enumeration<CompositionAttestationMode> i : mode) 621 dst.mode.add(i.copy()); 622 } 623 ; 624 dst.time = time == null ? null : time.copy(); 625 dst.party = party == null ? null : party.copy(); 626 return dst; 627 } 628 629 @Override 630 public boolean equalsDeep(Base other) { 631 if (!super.equalsDeep(other)) 632 return false; 633 if (!(other instanceof CompositionAttesterComponent)) 634 return false; 635 CompositionAttesterComponent o = (CompositionAttesterComponent) other; 636 return compareDeep(mode, o.mode, true) && compareDeep(time, o.time, true) && compareDeep(party, o.party, true); 637 } 638 639 @Override 640 public boolean equalsShallow(Base other) { 641 if (!super.equalsShallow(other)) 642 return false; 643 if (!(other instanceof CompositionAttesterComponent)) 644 return false; 645 CompositionAttesterComponent o = (CompositionAttesterComponent) other; 646 return compareValues(mode, o.mode, true) && compareValues(time, o.time, true); 647 } 648 649 public boolean isEmpty() { 650 return super.isEmpty() && (mode == null || mode.isEmpty()) && (time == null || time.isEmpty()) 651 && (party == null || party.isEmpty()); 652 } 653 654 public String fhirType() { 655 return "Composition.attester"; 656 657 } 658 659 } 660 661 @Block() 662 public static class CompositionEventComponent extends BackboneElement implements IBaseBackboneElement { 663 /** 664 * This list of codes represents the main clinical acts, such as a colonoscopy 665 * or an appendectomy, being documented. In some cases, the event is inherent in 666 * the typeCode, such as a "History and Physical Report" in which the procedure 667 * being documented is necessarily a "History and Physical" act. 668 */ 669 @Child(name = "code", type = { 670 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 671 @Description(shortDefinition = "Code(s) that apply to the event being documented", formalDefinition = "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.") 672 protected List<CodeableConcept> code; 673 674 /** 675 * The period of time covered by the documentation. There is no assertion that 676 * the documentation is a complete representation for this period, only that it 677 * documents events during this time. 678 */ 679 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 680 @Description(shortDefinition = "The period covered by the documentation", formalDefinition = "The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time.") 681 protected Period period; 682 683 /** 684 * The description and/or reference of the event(s) being documented. For 685 * example, this could be used to document such a colonoscopy or an 686 * appendectomy. 687 */ 688 @Child(name = "detail", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 689 @Description(shortDefinition = "The event(s) being documented", formalDefinition = "The description and/or reference of the event(s) being documented. For example, this could be used to document such a colonoscopy or an appendectomy.") 690 protected List<Reference> detail; 691 /** 692 * The actual objects that are the target of the reference (The description 693 * and/or reference of the event(s) being documented. For example, this could be 694 * used to document such a colonoscopy or an appendectomy.) 695 */ 696 protected List<Resource> detailTarget; 697 698 private static final long serialVersionUID = -1581379774L; 699 700 /* 701 * Constructor 702 */ 703 public CompositionEventComponent() { 704 super(); 705 } 706 707 /** 708 * @return {@link #code} (This list of codes represents the main clinical acts, 709 * such as a colonoscopy or an appendectomy, being documented. In some 710 * cases, the event is inherent in the typeCode, such as a "History and 711 * Physical Report" in which the procedure being documented is 712 * necessarily a "History and Physical" act.) 713 */ 714 public List<CodeableConcept> getCode() { 715 if (this.code == null) 716 this.code = new ArrayList<CodeableConcept>(); 717 return this.code; 718 } 719 720 public boolean hasCode() { 721 if (this.code == null) 722 return false; 723 for (CodeableConcept item : this.code) 724 if (!item.isEmpty()) 725 return true; 726 return false; 727 } 728 729 /** 730 * @return {@link #code} (This list of codes represents the main clinical acts, 731 * such as a colonoscopy or an appendectomy, being documented. In some 732 * cases, the event is inherent in the typeCode, such as a "History and 733 * Physical Report" in which the procedure being documented is 734 * necessarily a "History and Physical" act.) 735 */ 736 // syntactic sugar 737 public CodeableConcept addCode() { // 3 738 CodeableConcept t = new CodeableConcept(); 739 if (this.code == null) 740 this.code = new ArrayList<CodeableConcept>(); 741 this.code.add(t); 742 return t; 743 } 744 745 // syntactic sugar 746 public CompositionEventComponent addCode(CodeableConcept t) { // 3 747 if (t == null) 748 return this; 749 if (this.code == null) 750 this.code = new ArrayList<CodeableConcept>(); 751 this.code.add(t); 752 return this; 753 } 754 755 /** 756 * @return {@link #period} (The period of time covered by the documentation. 757 * There is no assertion that the documentation is a complete 758 * representation for this period, only that it documents events during 759 * this time.) 760 */ 761 public Period getPeriod() { 762 if (this.period == null) 763 if (Configuration.errorOnAutoCreate()) 764 throw new Error("Attempt to auto-create CompositionEventComponent.period"); 765 else if (Configuration.doAutoCreate()) 766 this.period = new Period(); // cc 767 return this.period; 768 } 769 770 public boolean hasPeriod() { 771 return this.period != null && !this.period.isEmpty(); 772 } 773 774 /** 775 * @param value {@link #period} (The period of time covered by the 776 * documentation. There is no assertion that the documentation is a 777 * complete representation for this period, only that it documents 778 * events during this time.) 779 */ 780 public CompositionEventComponent setPeriod(Period value) { 781 this.period = value; 782 return this; 783 } 784 785 /** 786 * @return {@link #detail} (The description and/or reference of the event(s) 787 * being documented. For example, this could be used to document such a 788 * colonoscopy or an appendectomy.) 789 */ 790 public List<Reference> getDetail() { 791 if (this.detail == null) 792 this.detail = new ArrayList<Reference>(); 793 return this.detail; 794 } 795 796 public boolean hasDetail() { 797 if (this.detail == null) 798 return false; 799 for (Reference item : this.detail) 800 if (!item.isEmpty()) 801 return true; 802 return false; 803 } 804 805 /** 806 * @return {@link #detail} (The description and/or reference of the event(s) 807 * being documented. For example, this could be used to document such a 808 * colonoscopy or an appendectomy.) 809 */ 810 // syntactic sugar 811 public Reference addDetail() { // 3 812 Reference t = new Reference(); 813 if (this.detail == null) 814 this.detail = new ArrayList<Reference>(); 815 this.detail.add(t); 816 return t; 817 } 818 819 // syntactic sugar 820 public CompositionEventComponent addDetail(Reference t) { // 3 821 if (t == null) 822 return this; 823 if (this.detail == null) 824 this.detail = new ArrayList<Reference>(); 825 this.detail.add(t); 826 return this; 827 } 828 829 /** 830 * @return {@link #detail} (The actual objects that are the target of the 831 * reference. The reference library doesn't populate this, but you can 832 * use this to hold the resources if you resolvethemt. The description 833 * and/or reference of the event(s) being documented. For example, this 834 * could be used to document such a colonoscopy or an appendectomy.) 835 */ 836 public List<Resource> getDetailTarget() { 837 if (this.detailTarget == null) 838 this.detailTarget = new ArrayList<Resource>(); 839 return this.detailTarget; 840 } 841 842 protected void listChildren(List<Property> childrenList) { 843 super.listChildren(childrenList); 844 childrenList.add(new Property("code", "CodeableConcept", 845 "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 846 0, java.lang.Integer.MAX_VALUE, code)); 847 childrenList.add(new Property("period", "Period", 848 "The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time.", 849 0, java.lang.Integer.MAX_VALUE, period)); 850 childrenList.add(new Property("detail", "Reference(Any)", 851 "The description and/or reference of the event(s) being documented. For example, this could be used to document such a colonoscopy or an appendectomy.", 852 0, java.lang.Integer.MAX_VALUE, detail)); 853 } 854 855 @Override 856 public void setProperty(String name, Base value) throws FHIRException { 857 if (name.equals("code")) 858 this.getCode().add(castToCodeableConcept(value)); 859 else if (name.equals("period")) 860 this.period = castToPeriod(value); // Period 861 else if (name.equals("detail")) 862 this.getDetail().add(castToReference(value)); 863 else 864 super.setProperty(name, value); 865 } 866 867 @Override 868 public Base addChild(String name) throws FHIRException { 869 if (name.equals("code")) { 870 return addCode(); 871 } else if (name.equals("period")) { 872 this.period = new Period(); 873 return this.period; 874 } else if (name.equals("detail")) { 875 return addDetail(); 876 } else 877 return super.addChild(name); 878 } 879 880 public CompositionEventComponent copy() { 881 CompositionEventComponent dst = new CompositionEventComponent(); 882 copyValues(dst); 883 if (code != null) { 884 dst.code = new ArrayList<CodeableConcept>(); 885 for (CodeableConcept i : code) 886 dst.code.add(i.copy()); 887 } 888 ; 889 dst.period = period == null ? null : period.copy(); 890 if (detail != null) { 891 dst.detail = new ArrayList<Reference>(); 892 for (Reference i : detail) 893 dst.detail.add(i.copy()); 894 } 895 ; 896 return dst; 897 } 898 899 @Override 900 public boolean equalsDeep(Base other) { 901 if (!super.equalsDeep(other)) 902 return false; 903 if (!(other instanceof CompositionEventComponent)) 904 return false; 905 CompositionEventComponent o = (CompositionEventComponent) other; 906 return compareDeep(code, o.code, true) && compareDeep(period, o.period, true) 907 && compareDeep(detail, o.detail, true); 908 } 909 910 @Override 911 public boolean equalsShallow(Base other) { 912 if (!super.equalsShallow(other)) 913 return false; 914 if (!(other instanceof CompositionEventComponent)) 915 return false; 916 CompositionEventComponent o = (CompositionEventComponent) other; 917 return true; 918 } 919 920 public boolean isEmpty() { 921 return super.isEmpty() && (code == null || code.isEmpty()) && (period == null || period.isEmpty()) 922 && (detail == null || detail.isEmpty()); 923 } 924 925 public String fhirType() { 926 return "Composition.event"; 927 928 } 929 930 } 931 932 @Block() 933 public static class SectionComponent extends BackboneElement implements IBaseBackboneElement { 934 /** 935 * The label for this particular section. This will be part of the rendered 936 * content for the document, and is often used to build a table of contents. 937 */ 938 @Child(name = "title", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 939 @Description(shortDefinition = "Label for section (e.g. for ToC)", formalDefinition = "The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.") 940 protected StringType title; 941 942 /** 943 * A code identifying the kind of content contained within the section. This 944 * must be consistent with the section title. 945 */ 946 @Child(name = "code", type = { 947 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 948 @Description(shortDefinition = "Classification of section (recommended)", formalDefinition = "A code identifying the kind of content contained within the section. This must be consistent with the section title.") 949 protected CodeableConcept code; 950 951 /** 952 * A human-readable narrative that contains the attested content of the section, 953 * used to represent the content of the resource to a human. The narrative need 954 * not encode all the structured data, but is required to contain sufficient 955 * detail to make it "clinically safe" for a human to just read the narrative. 956 */ 957 @Child(name = "text", type = { Narrative.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 958 @Description(shortDefinition = "Text summary of the section, for human interpretation", formalDefinition = "A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative.") 959 protected Narrative text; 960 961 /** 962 * How the entry list was prepared - whether it is a working list that is 963 * suitable for being maintained on an ongoing basis, or if it represents a 964 * snapshot of a list of items from another source, or whether it is a prepared 965 * list where items may be marked as added, modified or deleted. 966 */ 967 @Child(name = "mode", type = { CodeType.class }, order = 4, min = 0, max = 1, modifier = true, summary = true) 968 @Description(shortDefinition = "working | snapshot | changes", formalDefinition = "How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.") 969 protected CodeType mode; 970 971 /** 972 * Specifies the order applied to the items in the section entries. 973 */ 974 @Child(name = "orderedBy", type = { 975 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 976 @Description(shortDefinition = "Order of section entries", formalDefinition = "Specifies the order applied to the items in the section entries.") 977 protected CodeableConcept orderedBy; 978 979 /** 980 * A reference to the actual resource from which the narrative in the section is 981 * derived. 982 */ 983 @Child(name = "entry", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 984 @Description(shortDefinition = "A reference to data that supports this section", formalDefinition = "A reference to the actual resource from which the narrative in the section is derived.") 985 protected List<Reference> entry; 986 /** 987 * The actual objects that are the target of the reference (A reference to the 988 * actual resource from which the narrative in the section is derived.) 989 */ 990 protected List<Resource> entryTarget; 991 992 /** 993 * If the section is empty, why the list is empty. An empty section typically 994 * has some text explaining the empty reason. 995 */ 996 @Child(name = "emptyReason", type = { 997 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 998 @Description(shortDefinition = "Why the section is empty", formalDefinition = "If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.") 999 protected CodeableConcept emptyReason; 1000 1001 /** 1002 * A nested sub-section within this section. 1003 */ 1004 @Child(name = "section", type = { 1005 SectionComponent.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1006 @Description(shortDefinition = "Nested Section", formalDefinition = "A nested sub-section within this section.") 1007 protected List<SectionComponent> section; 1008 1009 private static final long serialVersionUID = -726390626L; 1010 1011 /* 1012 * Constructor 1013 */ 1014 public SectionComponent() { 1015 super(); 1016 } 1017 1018 /** 1019 * @return {@link #title} (The label for this particular section. This will be 1020 * part of the rendered content for the document, and is often used to 1021 * build a table of contents.). This is the underlying object with id, 1022 * value and extensions. The accessor "getTitle" gives direct access to 1023 * the value 1024 */ 1025 public StringType getTitleElement() { 1026 if (this.title == null) 1027 if (Configuration.errorOnAutoCreate()) 1028 throw new Error("Attempt to auto-create SectionComponent.title"); 1029 else if (Configuration.doAutoCreate()) 1030 this.title = new StringType(); // bb 1031 return this.title; 1032 } 1033 1034 public boolean hasTitleElement() { 1035 return this.title != null && !this.title.isEmpty(); 1036 } 1037 1038 public boolean hasTitle() { 1039 return this.title != null && !this.title.isEmpty(); 1040 } 1041 1042 /** 1043 * @param value {@link #title} (The label for this particular section. This will 1044 * be part of the rendered content for the document, and is often 1045 * used to build a table of contents.). This is the underlying 1046 * object with id, value and extensions. The accessor "getTitle" 1047 * gives direct access to the value 1048 */ 1049 public SectionComponent setTitleElement(StringType value) { 1050 this.title = value; 1051 return this; 1052 } 1053 1054 /** 1055 * @return The label for this particular section. This will be part of the 1056 * rendered content for the document, and is often used to build a table 1057 * of contents. 1058 */ 1059 public String getTitle() { 1060 return this.title == null ? null : this.title.getValue(); 1061 } 1062 1063 /** 1064 * @param value The label for this particular section. This will be part of the 1065 * rendered content for the document, and is often used to build a 1066 * table of contents. 1067 */ 1068 public SectionComponent setTitle(String value) { 1069 if (Utilities.noString(value)) 1070 this.title = null; 1071 else { 1072 if (this.title == null) 1073 this.title = new StringType(); 1074 this.title.setValue(value); 1075 } 1076 return this; 1077 } 1078 1079 /** 1080 * @return {@link #code} (A code identifying the kind of content contained 1081 * within the section. This must be consistent with the section title.) 1082 */ 1083 public CodeableConcept getCode() { 1084 if (this.code == null) 1085 if (Configuration.errorOnAutoCreate()) 1086 throw new Error("Attempt to auto-create SectionComponent.code"); 1087 else if (Configuration.doAutoCreate()) 1088 this.code = new CodeableConcept(); // cc 1089 return this.code; 1090 } 1091 1092 public boolean hasCode() { 1093 return this.code != null && !this.code.isEmpty(); 1094 } 1095 1096 /** 1097 * @param value {@link #code} (A code identifying the kind of content contained 1098 * within the section. This must be consistent with the section 1099 * title.) 1100 */ 1101 public SectionComponent setCode(CodeableConcept value) { 1102 this.code = value; 1103 return this; 1104 } 1105 1106 /** 1107 * @return {@link #text} (A human-readable narrative that contains the attested 1108 * content of the section, used to represent the content of the resource 1109 * to a human. The narrative need not encode all the structured data, 1110 * but is required to contain sufficient detail to make it "clinically 1111 * safe" for a human to just read the narrative.) 1112 */ 1113 public Narrative getText() { 1114 if (this.text == null) 1115 if (Configuration.errorOnAutoCreate()) 1116 throw new Error("Attempt to auto-create SectionComponent.text"); 1117 else if (Configuration.doAutoCreate()) 1118 this.text = new Narrative(); // cc 1119 return this.text; 1120 } 1121 1122 public boolean hasText() { 1123 return this.text != null && !this.text.isEmpty(); 1124 } 1125 1126 /** 1127 * @param value {@link #text} (A human-readable narrative that contains the 1128 * attested content of the section, used to represent the content 1129 * of the resource to a human. The narrative need not encode all 1130 * the structured data, but is required to contain sufficient 1131 * detail to make it "clinically safe" for a human to just read the 1132 * narrative.) 1133 */ 1134 public SectionComponent setText(Narrative value) { 1135 this.text = value; 1136 return this; 1137 } 1138 1139 /** 1140 * @return {@link #mode} (How the entry list was prepared - whether it is a 1141 * working list that is suitable for being maintained on an ongoing 1142 * basis, or if it represents a snapshot of a list of items from another 1143 * source, or whether it is a prepared list where items may be marked as 1144 * added, modified or deleted.). This is the underlying object with id, 1145 * value and extensions. The accessor "getMode" gives direct access to 1146 * the value 1147 */ 1148 public CodeType getModeElement() { 1149 if (this.mode == null) 1150 if (Configuration.errorOnAutoCreate()) 1151 throw new Error("Attempt to auto-create SectionComponent.mode"); 1152 else if (Configuration.doAutoCreate()) 1153 this.mode = new CodeType(); // bb 1154 return this.mode; 1155 } 1156 1157 public boolean hasModeElement() { 1158 return this.mode != null && !this.mode.isEmpty(); 1159 } 1160 1161 public boolean hasMode() { 1162 return this.mode != null && !this.mode.isEmpty(); 1163 } 1164 1165 /** 1166 * @param value {@link #mode} (How the entry list was prepared - whether it is a 1167 * working list that is suitable for being maintained on an ongoing 1168 * basis, or if it represents a snapshot of a list of items from 1169 * another source, or whether it is a prepared list where items may 1170 * be marked as added, modified or deleted.). This is the 1171 * underlying object with id, value and extensions. The accessor 1172 * "getMode" gives direct access to the value 1173 */ 1174 public SectionComponent setModeElement(CodeType value) { 1175 this.mode = value; 1176 return this; 1177 } 1178 1179 /** 1180 * @return How the entry list was prepared - whether it is a working list that 1181 * is suitable for being maintained on an ongoing basis, or if it 1182 * represents a snapshot of a list of items from another source, or 1183 * whether it is a prepared list where items may be marked as added, 1184 * modified or deleted. 1185 */ 1186 public String getMode() { 1187 return this.mode == null ? null : this.mode.getValue(); 1188 } 1189 1190 /** 1191 * @param value How the entry list was prepared - whether it is a working list 1192 * that is suitable for being maintained on an ongoing basis, or if 1193 * it represents a snapshot of a list of items from another source, 1194 * or whether it is a prepared list where items may be marked as 1195 * added, modified or deleted. 1196 */ 1197 public SectionComponent setMode(String value) { 1198 if (Utilities.noString(value)) 1199 this.mode = null; 1200 else { 1201 if (this.mode == null) 1202 this.mode = new CodeType(); 1203 this.mode.setValue(value); 1204 } 1205 return this; 1206 } 1207 1208 /** 1209 * @return {@link #orderedBy} (Specifies the order applied to the items in the 1210 * section entries.) 1211 */ 1212 public CodeableConcept getOrderedBy() { 1213 if (this.orderedBy == null) 1214 if (Configuration.errorOnAutoCreate()) 1215 throw new Error("Attempt to auto-create SectionComponent.orderedBy"); 1216 else if (Configuration.doAutoCreate()) 1217 this.orderedBy = new CodeableConcept(); // cc 1218 return this.orderedBy; 1219 } 1220 1221 public boolean hasOrderedBy() { 1222 return this.orderedBy != null && !this.orderedBy.isEmpty(); 1223 } 1224 1225 /** 1226 * @param value {@link #orderedBy} (Specifies the order applied to the items in 1227 * the section entries.) 1228 */ 1229 public SectionComponent setOrderedBy(CodeableConcept value) { 1230 this.orderedBy = value; 1231 return this; 1232 } 1233 1234 /** 1235 * @return {@link #entry} (A reference to the actual resource from which the 1236 * narrative in the section is derived.) 1237 */ 1238 public List<Reference> getEntry() { 1239 if (this.entry == null) 1240 this.entry = new ArrayList<Reference>(); 1241 return this.entry; 1242 } 1243 1244 public boolean hasEntry() { 1245 if (this.entry == null) 1246 return false; 1247 for (Reference item : this.entry) 1248 if (!item.isEmpty()) 1249 return true; 1250 return false; 1251 } 1252 1253 /** 1254 * @return {@link #entry} (A reference to the actual resource from which the 1255 * narrative in the section is derived.) 1256 */ 1257 // syntactic sugar 1258 public Reference addEntry() { // 3 1259 Reference t = new Reference(); 1260 if (this.entry == null) 1261 this.entry = new ArrayList<Reference>(); 1262 this.entry.add(t); 1263 return t; 1264 } 1265 1266 // syntactic sugar 1267 public SectionComponent addEntry(Reference t) { // 3 1268 if (t == null) 1269 return this; 1270 if (this.entry == null) 1271 this.entry = new ArrayList<Reference>(); 1272 this.entry.add(t); 1273 return this; 1274 } 1275 1276 /** 1277 * @return {@link #entry} (The actual objects that are the target of the 1278 * reference. The reference library doesn't populate this, but you can 1279 * use this to hold the resources if you resolvethemt. A reference to 1280 * the actual resource from which the narrative in the section is 1281 * derived.) 1282 */ 1283 public List<Resource> getEntryTarget() { 1284 if (this.entryTarget == null) 1285 this.entryTarget = new ArrayList<Resource>(); 1286 return this.entryTarget; 1287 } 1288 1289 /** 1290 * @return {@link #emptyReason} (If the section is empty, why the list is empty. 1291 * An empty section typically has some text explaining the empty 1292 * reason.) 1293 */ 1294 public CodeableConcept getEmptyReason() { 1295 if (this.emptyReason == null) 1296 if (Configuration.errorOnAutoCreate()) 1297 throw new Error("Attempt to auto-create SectionComponent.emptyReason"); 1298 else if (Configuration.doAutoCreate()) 1299 this.emptyReason = new CodeableConcept(); // cc 1300 return this.emptyReason; 1301 } 1302 1303 public boolean hasEmptyReason() { 1304 return this.emptyReason != null && !this.emptyReason.isEmpty(); 1305 } 1306 1307 /** 1308 * @param value {@link #emptyReason} (If the section is empty, why the list is 1309 * empty. An empty section typically has some text explaining the 1310 * empty reason.) 1311 */ 1312 public SectionComponent setEmptyReason(CodeableConcept value) { 1313 this.emptyReason = value; 1314 return this; 1315 } 1316 1317 /** 1318 * @return {@link #section} (A nested sub-section within this section.) 1319 */ 1320 public List<SectionComponent> getSection() { 1321 if (this.section == null) 1322 this.section = new ArrayList<SectionComponent>(); 1323 return this.section; 1324 } 1325 1326 public boolean hasSection() { 1327 if (this.section == null) 1328 return false; 1329 for (SectionComponent item : this.section) 1330 if (!item.isEmpty()) 1331 return true; 1332 return false; 1333 } 1334 1335 /** 1336 * @return {@link #section} (A nested sub-section within this section.) 1337 */ 1338 // syntactic sugar 1339 public SectionComponent addSection() { // 3 1340 SectionComponent t = new SectionComponent(); 1341 if (this.section == null) 1342 this.section = new ArrayList<SectionComponent>(); 1343 this.section.add(t); 1344 return t; 1345 } 1346 1347 // syntactic sugar 1348 public SectionComponent addSection(SectionComponent t) { // 3 1349 if (t == null) 1350 return this; 1351 if (this.section == null) 1352 this.section = new ArrayList<SectionComponent>(); 1353 this.section.add(t); 1354 return this; 1355 } 1356 1357 protected void listChildren(List<Property> childrenList) { 1358 super.listChildren(childrenList); 1359 childrenList.add(new Property("title", "string", 1360 "The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.", 1361 0, java.lang.Integer.MAX_VALUE, title)); 1362 childrenList.add(new Property("code", "CodeableConcept", 1363 "A code identifying the kind of content contained within the section. This must be consistent with the section title.", 1364 0, java.lang.Integer.MAX_VALUE, code)); 1365 childrenList.add(new Property("text", "Narrative", 1366 "A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative.", 1367 0, java.lang.Integer.MAX_VALUE, text)); 1368 childrenList.add(new Property("mode", "code", 1369 "How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 1370 0, java.lang.Integer.MAX_VALUE, mode)); 1371 childrenList.add(new Property("orderedBy", "CodeableConcept", 1372 "Specifies the order applied to the items in the section entries.", 0, java.lang.Integer.MAX_VALUE, 1373 orderedBy)); 1374 childrenList.add(new Property("entry", "Reference(Any)", 1375 "A reference to the actual resource from which the narrative in the section is derived.", 0, 1376 java.lang.Integer.MAX_VALUE, entry)); 1377 childrenList.add(new Property("emptyReason", "CodeableConcept", 1378 "If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.", 1379 0, java.lang.Integer.MAX_VALUE, emptyReason)); 1380 childrenList.add(new Property("section", "@Composition.section", "A nested sub-section within this section.", 0, 1381 java.lang.Integer.MAX_VALUE, section)); 1382 } 1383 1384 @Override 1385 public void setProperty(String name, Base value) throws FHIRException { 1386 if (name.equals("title")) 1387 this.title = castToString(value); // StringType 1388 else if (name.equals("code")) 1389 this.code = castToCodeableConcept(value); // CodeableConcept 1390 else if (name.equals("text")) 1391 this.text = castToNarrative(value); // Narrative 1392 else if (name.equals("mode")) 1393 this.mode = castToCode(value); // CodeType 1394 else if (name.equals("orderedBy")) 1395 this.orderedBy = castToCodeableConcept(value); // CodeableConcept 1396 else if (name.equals("entry")) 1397 this.getEntry().add(castToReference(value)); 1398 else if (name.equals("emptyReason")) 1399 this.emptyReason = castToCodeableConcept(value); // CodeableConcept 1400 else if (name.equals("section")) 1401 this.getSection().add((SectionComponent) value); 1402 else 1403 super.setProperty(name, value); 1404 } 1405 1406 @Override 1407 public Base addChild(String name) throws FHIRException { 1408 if (name.equals("title")) { 1409 throw new FHIRException("Cannot call addChild on a singleton property Composition.title"); 1410 } else if (name.equals("code")) { 1411 this.code = new CodeableConcept(); 1412 return this.code; 1413 } else if (name.equals("text")) { 1414 this.text = new Narrative(); 1415 return this.text; 1416 } else if (name.equals("mode")) { 1417 throw new FHIRException("Cannot call addChild on a singleton property Composition.mode"); 1418 } else if (name.equals("orderedBy")) { 1419 this.orderedBy = new CodeableConcept(); 1420 return this.orderedBy; 1421 } else if (name.equals("entry")) { 1422 return addEntry(); 1423 } else if (name.equals("emptyReason")) { 1424 this.emptyReason = new CodeableConcept(); 1425 return this.emptyReason; 1426 } else if (name.equals("section")) { 1427 return addSection(); 1428 } else 1429 return super.addChild(name); 1430 } 1431 1432 public SectionComponent copy() { 1433 SectionComponent dst = new SectionComponent(); 1434 copyValues(dst); 1435 dst.title = title == null ? null : title.copy(); 1436 dst.code = code == null ? null : code.copy(); 1437 dst.text = text == null ? null : text.copy(); 1438 dst.mode = mode == null ? null : mode.copy(); 1439 dst.orderedBy = orderedBy == null ? null : orderedBy.copy(); 1440 if (entry != null) { 1441 dst.entry = new ArrayList<Reference>(); 1442 for (Reference i : entry) 1443 dst.entry.add(i.copy()); 1444 } 1445 ; 1446 dst.emptyReason = emptyReason == null ? null : emptyReason.copy(); 1447 if (section != null) { 1448 dst.section = new ArrayList<SectionComponent>(); 1449 for (SectionComponent i : section) 1450 dst.section.add(i.copy()); 1451 } 1452 ; 1453 return dst; 1454 } 1455 1456 @Override 1457 public boolean equalsDeep(Base other) { 1458 if (!super.equalsDeep(other)) 1459 return false; 1460 if (!(other instanceof SectionComponent)) 1461 return false; 1462 SectionComponent o = (SectionComponent) other; 1463 return compareDeep(title, o.title, true) && compareDeep(code, o.code, true) && compareDeep(text, o.text, true) 1464 && compareDeep(mode, o.mode, true) && compareDeep(orderedBy, o.orderedBy, true) 1465 && compareDeep(entry, o.entry, true) && compareDeep(emptyReason, o.emptyReason, true) 1466 && compareDeep(section, o.section, true); 1467 } 1468 1469 @Override 1470 public boolean equalsShallow(Base other) { 1471 if (!super.equalsShallow(other)) 1472 return false; 1473 if (!(other instanceof SectionComponent)) 1474 return false; 1475 SectionComponent o = (SectionComponent) other; 1476 return compareValues(title, o.title, true) && compareValues(mode, o.mode, true); 1477 } 1478 1479 public boolean isEmpty() { 1480 return super.isEmpty() && (title == null || title.isEmpty()) && (code == null || code.isEmpty()) 1481 && (text == null || text.isEmpty()) && (mode == null || mode.isEmpty()) 1482 && (orderedBy == null || orderedBy.isEmpty()) && (entry == null || entry.isEmpty()) 1483 && (emptyReason == null || emptyReason.isEmpty()) && (section == null || section.isEmpty()); 1484 } 1485 1486 public String fhirType() { 1487 return "Composition.section"; 1488 1489 } 1490 1491 } 1492 1493 /** 1494 * Logical identifier for the composition, assigned when created. This 1495 * identifier stays constant as the composition is changed over time. 1496 */ 1497 @Child(name = "identifier", type = { 1498 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 1499 @Description(shortDefinition = "Logical identifier of composition (version-independent)", formalDefinition = "Logical identifier for the composition, assigned when created. This identifier stays constant as the composition is changed over time.") 1500 protected Identifier identifier; 1501 1502 /** 1503 * The composition editing time, when the composition was last logically changed 1504 * by the author. 1505 */ 1506 @Child(name = "date", type = { DateTimeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1507 @Description(shortDefinition = "Composition editing time", formalDefinition = "The composition editing time, when the composition was last logically changed by the author.") 1508 protected DateTimeType date; 1509 1510 /** 1511 * Specifies the particular kind of composition (e.g. History and Physical, 1512 * Discharge Summary, Progress Note). This usually equates to the purpose of 1513 * making the composition. 1514 */ 1515 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1516 @Description(shortDefinition = "Kind of composition (LOINC if possible)", formalDefinition = "Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition.") 1517 protected CodeableConcept type; 1518 1519 /** 1520 * A categorization for the type of the composition - helps for indexing and 1521 * searching. This may be implied by or derived from the code specified in the 1522 * Composition Type. 1523 */ 1524 @Child(name = "class", type = { 1525 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1526 @Description(shortDefinition = "Categorization of Composition", formalDefinition = "A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type.") 1527 protected CodeableConcept class_; 1528 1529 /** 1530 * Official human-readable label for the composition. 1531 */ 1532 @Child(name = "title", type = { StringType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 1533 @Description(shortDefinition = "Human Readable name/title", formalDefinition = "Official human-readable label for the composition.") 1534 protected StringType title; 1535 1536 /** 1537 * The workflow/clinical status of this composition. The status is a marker for 1538 * the clinical standing of the document. 1539 */ 1540 @Child(name = "status", type = { CodeType.class }, order = 5, min = 1, max = 1, modifier = true, summary = true) 1541 @Description(shortDefinition = "preliminary | final | amended | entered-in-error", formalDefinition = "The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document.") 1542 protected Enumeration<CompositionStatus> status; 1543 1544 /** 1545 * The code specifying the level of confidentiality of the Composition. 1546 */ 1547 @Child(name = "confidentiality", type = { 1548 CodeType.class }, order = 6, min = 0, max = 1, modifier = true, summary = true) 1549 @Description(shortDefinition = "As defined by affinity domain", formalDefinition = "The code specifying the level of confidentiality of the Composition.") 1550 protected CodeType confidentiality; 1551 1552 /** 1553 * Who or what the composition is about. The composition can be about a person, 1554 * (patient or healthcare practitioner), a device (e.g. a machine) or even a 1555 * group of subjects (such as a document about a herd of livestock, or a set of 1556 * patients that share a common exposure). 1557 */ 1558 @Child(name = "subject", type = {}, order = 7, min = 1, max = 1, modifier = false, summary = true) 1559 @Description(shortDefinition = "Who and/or what the composition is about", formalDefinition = "Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).") 1560 protected Reference subject; 1561 1562 /** 1563 * The actual object that is the target of the reference (Who or what the 1564 * composition is about. The composition can be about a person, (patient or 1565 * healthcare practitioner), a device (e.g. a machine) or even a group of 1566 * subjects (such as a document about a herd of livestock, or a set of patients 1567 * that share a common exposure).) 1568 */ 1569 protected Resource subjectTarget; 1570 1571 /** 1572 * Identifies who is responsible for the information in the composition, not 1573 * necessarily who typed it in. 1574 */ 1575 @Child(name = "author", type = { Practitioner.class, Device.class, Patient.class, 1576 RelatedPerson.class }, order = 8, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1577 @Description(shortDefinition = "Who and/or what authored the composition", formalDefinition = "Identifies who is responsible for the information in the composition, not necessarily who typed it in.") 1578 protected List<Reference> author; 1579 /** 1580 * The actual objects that are the target of the reference (Identifies who is 1581 * responsible for the information in the composition, not necessarily who typed 1582 * it in.) 1583 */ 1584 protected List<Resource> authorTarget; 1585 1586 /** 1587 * A participant who has attested to the accuracy of the composition/document. 1588 */ 1589 @Child(name = "attester", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1590 @Description(shortDefinition = "Attests to accuracy of composition", formalDefinition = "A participant who has attested to the accuracy of the composition/document.") 1591 protected List<CompositionAttesterComponent> attester; 1592 1593 /** 1594 * Identifies the organization or group who is responsible for ongoing 1595 * maintenance of and access to the composition/document information. 1596 */ 1597 @Child(name = "custodian", type = { 1598 Organization.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1599 @Description(shortDefinition = "Organization which maintains the composition", formalDefinition = "Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.") 1600 protected Reference custodian; 1601 1602 /** 1603 * The actual object that is the target of the reference (Identifies the 1604 * organization or group who is responsible for ongoing maintenance of and 1605 * access to the composition/document information.) 1606 */ 1607 protected Organization custodianTarget; 1608 1609 /** 1610 * The clinical service, such as a colonoscopy or an appendectomy, being 1611 * documented. 1612 */ 1613 @Child(name = "event", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1614 @Description(shortDefinition = "The clinical service(s) being documented", formalDefinition = "The clinical service, such as a colonoscopy or an appendectomy, being documented.") 1615 protected List<CompositionEventComponent> event; 1616 1617 /** 1618 * Describes the clinical encounter or type of care this documentation is 1619 * associated with. 1620 */ 1621 @Child(name = "encounter", type = { Encounter.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1622 @Description(shortDefinition = "Context of the Composition", formalDefinition = "Describes the clinical encounter or type of care this documentation is associated with.") 1623 protected Reference encounter; 1624 1625 /** 1626 * The actual object that is the target of the reference (Describes the clinical 1627 * encounter or type of care this documentation is associated with.) 1628 */ 1629 protected Encounter encounterTarget; 1630 1631 /** 1632 * The root of the sections that make up the composition. 1633 */ 1634 @Child(name = "section", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1635 @Description(shortDefinition = "Composition is broken into sections", formalDefinition = "The root of the sections that make up the composition.") 1636 protected List<SectionComponent> section; 1637 1638 private static final long serialVersionUID = 2127852326L; 1639 1640 /* 1641 * Constructor 1642 */ 1643 public Composition() { 1644 super(); 1645 } 1646 1647 /* 1648 * Constructor 1649 */ 1650 public Composition(DateTimeType date, CodeableConcept type, StringType title, Enumeration<CompositionStatus> status, 1651 Reference subject) { 1652 super(); 1653 this.date = date; 1654 this.type = type; 1655 this.title = title; 1656 this.status = status; 1657 this.subject = subject; 1658 } 1659 1660 /** 1661 * @return {@link #identifier} (Logical identifier for the composition, assigned 1662 * when created. This identifier stays constant as the composition is 1663 * changed over time.) 1664 */ 1665 public Identifier getIdentifier() { 1666 if (this.identifier == null) 1667 if (Configuration.errorOnAutoCreate()) 1668 throw new Error("Attempt to auto-create Composition.identifier"); 1669 else if (Configuration.doAutoCreate()) 1670 this.identifier = new Identifier(); // cc 1671 return this.identifier; 1672 } 1673 1674 public boolean hasIdentifier() { 1675 return this.identifier != null && !this.identifier.isEmpty(); 1676 } 1677 1678 /** 1679 * @param value {@link #identifier} (Logical identifier for the composition, 1680 * assigned when created. This identifier stays constant as the 1681 * composition is changed over time.) 1682 */ 1683 public Composition setIdentifier(Identifier value) { 1684 this.identifier = value; 1685 return this; 1686 } 1687 1688 /** 1689 * @return {@link #date} (The composition editing time, when the composition was 1690 * last logically changed by the author.). This is the underlying object 1691 * with id, value and extensions. The accessor "getDate" gives direct 1692 * access to the value 1693 */ 1694 public DateTimeType getDateElement() { 1695 if (this.date == null) 1696 if (Configuration.errorOnAutoCreate()) 1697 throw new Error("Attempt to auto-create Composition.date"); 1698 else if (Configuration.doAutoCreate()) 1699 this.date = new DateTimeType(); // bb 1700 return this.date; 1701 } 1702 1703 public boolean hasDateElement() { 1704 return this.date != null && !this.date.isEmpty(); 1705 } 1706 1707 public boolean hasDate() { 1708 return this.date != null && !this.date.isEmpty(); 1709 } 1710 1711 /** 1712 * @param value {@link #date} (The composition editing time, when the 1713 * composition was last logically changed by the author.). This is 1714 * the underlying object with id, value and extensions. The 1715 * accessor "getDate" gives direct access to the value 1716 */ 1717 public Composition setDateElement(DateTimeType value) { 1718 this.date = value; 1719 return this; 1720 } 1721 1722 /** 1723 * @return The composition editing time, when the composition was last logically 1724 * changed by the author. 1725 */ 1726 public Date getDate() { 1727 return this.date == null ? null : this.date.getValue(); 1728 } 1729 1730 /** 1731 * @param value The composition editing time, when the composition was last 1732 * logically changed by the author. 1733 */ 1734 public Composition setDate(Date value) { 1735 if (this.date == null) 1736 this.date = new DateTimeType(); 1737 this.date.setValue(value); 1738 return this; 1739 } 1740 1741 /** 1742 * @return {@link #type} (Specifies the particular kind of composition (e.g. 1743 * History and Physical, Discharge Summary, Progress Note). This usually 1744 * equates to the purpose of making the composition.) 1745 */ 1746 public CodeableConcept getType() { 1747 if (this.type == null) 1748 if (Configuration.errorOnAutoCreate()) 1749 throw new Error("Attempt to auto-create Composition.type"); 1750 else if (Configuration.doAutoCreate()) 1751 this.type = new CodeableConcept(); // cc 1752 return this.type; 1753 } 1754 1755 public boolean hasType() { 1756 return this.type != null && !this.type.isEmpty(); 1757 } 1758 1759 /** 1760 * @param value {@link #type} (Specifies the particular kind of composition 1761 * (e.g. History and Physical, Discharge Summary, Progress Note). 1762 * This usually equates to the purpose of making the composition.) 1763 */ 1764 public Composition setType(CodeableConcept value) { 1765 this.type = value; 1766 return this; 1767 } 1768 1769 /** 1770 * @return {@link #class_} (A categorization for the type of the composition - 1771 * helps for indexing and searching. This may be implied by or derived 1772 * from the code specified in the Composition Type.) 1773 */ 1774 public CodeableConcept getClass_() { 1775 if (this.class_ == null) 1776 if (Configuration.errorOnAutoCreate()) 1777 throw new Error("Attempt to auto-create Composition.class_"); 1778 else if (Configuration.doAutoCreate()) 1779 this.class_ = new CodeableConcept(); // cc 1780 return this.class_; 1781 } 1782 1783 public boolean hasClass_() { 1784 return this.class_ != null && !this.class_.isEmpty(); 1785 } 1786 1787 /** 1788 * @param value {@link #class_} (A categorization for the type of the 1789 * composition - helps for indexing and searching. This may be 1790 * implied by or derived from the code specified in the Composition 1791 * Type.) 1792 */ 1793 public Composition setClass_(CodeableConcept value) { 1794 this.class_ = value; 1795 return this; 1796 } 1797 1798 /** 1799 * @return {@link #title} (Official human-readable label for the composition.). 1800 * This is the underlying object with id, value and extensions. The 1801 * accessor "getTitle" gives direct access to the value 1802 */ 1803 public StringType getTitleElement() { 1804 if (this.title == null) 1805 if (Configuration.errorOnAutoCreate()) 1806 throw new Error("Attempt to auto-create Composition.title"); 1807 else if (Configuration.doAutoCreate()) 1808 this.title = new StringType(); // bb 1809 return this.title; 1810 } 1811 1812 public boolean hasTitleElement() { 1813 return this.title != null && !this.title.isEmpty(); 1814 } 1815 1816 public boolean hasTitle() { 1817 return this.title != null && !this.title.isEmpty(); 1818 } 1819 1820 /** 1821 * @param value {@link #title} (Official human-readable label for the 1822 * composition.). This is the underlying object with id, value and 1823 * extensions. The accessor "getTitle" gives direct access to the 1824 * value 1825 */ 1826 public Composition setTitleElement(StringType value) { 1827 this.title = value; 1828 return this; 1829 } 1830 1831 /** 1832 * @return Official human-readable label for the composition. 1833 */ 1834 public String getTitle() { 1835 return this.title == null ? null : this.title.getValue(); 1836 } 1837 1838 /** 1839 * @param value Official human-readable label for the composition. 1840 */ 1841 public Composition setTitle(String value) { 1842 if (this.title == null) 1843 this.title = new StringType(); 1844 this.title.setValue(value); 1845 return this; 1846 } 1847 1848 /** 1849 * @return {@link #status} (The workflow/clinical status of this composition. 1850 * The status is a marker for the clinical standing of the document.). 1851 * This is the underlying object with id, value and extensions. The 1852 * accessor "getStatus" gives direct access to the value 1853 */ 1854 public Enumeration<CompositionStatus> getStatusElement() { 1855 if (this.status == null) 1856 if (Configuration.errorOnAutoCreate()) 1857 throw new Error("Attempt to auto-create Composition.status"); 1858 else if (Configuration.doAutoCreate()) 1859 this.status = new Enumeration<CompositionStatus>(new CompositionStatusEnumFactory()); // bb 1860 return this.status; 1861 } 1862 1863 public boolean hasStatusElement() { 1864 return this.status != null && !this.status.isEmpty(); 1865 } 1866 1867 public boolean hasStatus() { 1868 return this.status != null && !this.status.isEmpty(); 1869 } 1870 1871 /** 1872 * @param value {@link #status} (The workflow/clinical status of this 1873 * composition. The status is a marker for the clinical standing of 1874 * the document.). This is the underlying object with id, value and 1875 * extensions. The accessor "getStatus" gives direct access to the 1876 * value 1877 */ 1878 public Composition setStatusElement(Enumeration<CompositionStatus> value) { 1879 this.status = value; 1880 return this; 1881 } 1882 1883 /** 1884 * @return The workflow/clinical status of this composition. The status is a 1885 * marker for the clinical standing of the document. 1886 */ 1887 public CompositionStatus getStatus() { 1888 return this.status == null ? null : this.status.getValue(); 1889 } 1890 1891 /** 1892 * @param value The workflow/clinical status of this composition. The status is 1893 * a marker for the clinical standing of the document. 1894 */ 1895 public Composition setStatus(CompositionStatus value) { 1896 if (this.status == null) 1897 this.status = new Enumeration<CompositionStatus>(new CompositionStatusEnumFactory()); 1898 this.status.setValue(value); 1899 return this; 1900 } 1901 1902 /** 1903 * @return {@link #confidentiality} (The code specifying the level of 1904 * confidentiality of the Composition.). This is the underlying object 1905 * with id, value and extensions. The accessor "getConfidentiality" 1906 * gives direct access to the value 1907 */ 1908 public CodeType getConfidentialityElement() { 1909 if (this.confidentiality == null) 1910 if (Configuration.errorOnAutoCreate()) 1911 throw new Error("Attempt to auto-create Composition.confidentiality"); 1912 else if (Configuration.doAutoCreate()) 1913 this.confidentiality = new CodeType(); // bb 1914 return this.confidentiality; 1915 } 1916 1917 public boolean hasConfidentialityElement() { 1918 return this.confidentiality != null && !this.confidentiality.isEmpty(); 1919 } 1920 1921 public boolean hasConfidentiality() { 1922 return this.confidentiality != null && !this.confidentiality.isEmpty(); 1923 } 1924 1925 /** 1926 * @param value {@link #confidentiality} (The code specifying the level of 1927 * confidentiality of the Composition.). This is the underlying 1928 * object with id, value and extensions. The accessor 1929 * "getConfidentiality" gives direct access to the value 1930 */ 1931 public Composition setConfidentialityElement(CodeType value) { 1932 this.confidentiality = value; 1933 return this; 1934 } 1935 1936 /** 1937 * @return The code specifying the level of confidentiality of the Composition. 1938 */ 1939 public String getConfidentiality() { 1940 return this.confidentiality == null ? null : this.confidentiality.getValue(); 1941 } 1942 1943 /** 1944 * @param value The code specifying the level of confidentiality of the 1945 * Composition. 1946 */ 1947 public Composition setConfidentiality(String value) { 1948 if (Utilities.noString(value)) 1949 this.confidentiality = null; 1950 else { 1951 if (this.confidentiality == null) 1952 this.confidentiality = new CodeType(); 1953 this.confidentiality.setValue(value); 1954 } 1955 return this; 1956 } 1957 1958 /** 1959 * @return {@link #subject} (Who or what the composition is about. The 1960 * composition can be about a person, (patient or healthcare 1961 * practitioner), a device (e.g. a machine) or even a group of subjects 1962 * (such as a document about a herd of livestock, or a set of patients 1963 * that share a common exposure).) 1964 */ 1965 public Reference getSubject() { 1966 if (this.subject == null) 1967 if (Configuration.errorOnAutoCreate()) 1968 throw new Error("Attempt to auto-create Composition.subject"); 1969 else if (Configuration.doAutoCreate()) 1970 this.subject = new Reference(); // cc 1971 return this.subject; 1972 } 1973 1974 public boolean hasSubject() { 1975 return this.subject != null && !this.subject.isEmpty(); 1976 } 1977 1978 /** 1979 * @param value {@link #subject} (Who or what the composition is about. The 1980 * composition can be about a person, (patient or healthcare 1981 * practitioner), a device (e.g. a machine) or even a group of 1982 * subjects (such as a document about a herd of livestock, or a set 1983 * of patients that share a common exposure).) 1984 */ 1985 public Composition setSubject(Reference value) { 1986 this.subject = value; 1987 return this; 1988 } 1989 1990 /** 1991 * @return {@link #subject} The actual object that is the target of the 1992 * reference. The reference library doesn't populate this, but you can 1993 * use it to hold the resource if you resolve it. (Who or what the 1994 * composition is about. The composition can be about a person, (patient 1995 * or healthcare practitioner), a device (e.g. a machine) or even a 1996 * group of subjects (such as a document about a herd of livestock, or a 1997 * set of patients that share a common exposure).) 1998 */ 1999 public Resource getSubjectTarget() { 2000 return this.subjectTarget; 2001 } 2002 2003 /** 2004 * @param value {@link #subject} The actual object that is the target of the 2005 * reference. The reference library doesn't use these, but you can 2006 * use it to hold the resource if you resolve it. (Who or what the 2007 * composition is about. The composition can be about a person, 2008 * (patient or healthcare practitioner), a device (e.g. a machine) 2009 * or even a group of subjects (such as a document about a herd of 2010 * livestock, or a set of patients that share a common exposure).) 2011 */ 2012 public Composition setSubjectTarget(Resource value) { 2013 this.subjectTarget = value; 2014 return this; 2015 } 2016 2017 /** 2018 * @return {@link #author} (Identifies who is responsible for the information in 2019 * the composition, not necessarily who typed it in.) 2020 */ 2021 public List<Reference> getAuthor() { 2022 if (this.author == null) 2023 this.author = new ArrayList<Reference>(); 2024 return this.author; 2025 } 2026 2027 public boolean hasAuthor() { 2028 if (this.author == null) 2029 return false; 2030 for (Reference item : this.author) 2031 if (!item.isEmpty()) 2032 return true; 2033 return false; 2034 } 2035 2036 /** 2037 * @return {@link #author} (Identifies who is responsible for the information in 2038 * the composition, not necessarily who typed it in.) 2039 */ 2040 // syntactic sugar 2041 public Reference addAuthor() { // 3 2042 Reference t = new Reference(); 2043 if (this.author == null) 2044 this.author = new ArrayList<Reference>(); 2045 this.author.add(t); 2046 return t; 2047 } 2048 2049 // syntactic sugar 2050 public Composition addAuthor(Reference t) { // 3 2051 if (t == null) 2052 return this; 2053 if (this.author == null) 2054 this.author = new ArrayList<Reference>(); 2055 this.author.add(t); 2056 return this; 2057 } 2058 2059 /** 2060 * @return {@link #author} (The actual objects that are the target of the 2061 * reference. The reference library doesn't populate this, but you can 2062 * use this to hold the resources if you resolvethemt. Identifies who is 2063 * responsible for the information in the composition, not necessarily 2064 * who typed it in.) 2065 */ 2066 public List<Resource> getAuthorTarget() { 2067 if (this.authorTarget == null) 2068 this.authorTarget = new ArrayList<Resource>(); 2069 return this.authorTarget; 2070 } 2071 2072 /** 2073 * @return {@link #attester} (A participant who has attested to the accuracy of 2074 * the composition/document.) 2075 */ 2076 public List<CompositionAttesterComponent> getAttester() { 2077 if (this.attester == null) 2078 this.attester = new ArrayList<CompositionAttesterComponent>(); 2079 return this.attester; 2080 } 2081 2082 public boolean hasAttester() { 2083 if (this.attester == null) 2084 return false; 2085 for (CompositionAttesterComponent item : this.attester) 2086 if (!item.isEmpty()) 2087 return true; 2088 return false; 2089 } 2090 2091 /** 2092 * @return {@link #attester} (A participant who has attested to the accuracy of 2093 * the composition/document.) 2094 */ 2095 // syntactic sugar 2096 public CompositionAttesterComponent addAttester() { // 3 2097 CompositionAttesterComponent t = new CompositionAttesterComponent(); 2098 if (this.attester == null) 2099 this.attester = new ArrayList<CompositionAttesterComponent>(); 2100 this.attester.add(t); 2101 return t; 2102 } 2103 2104 // syntactic sugar 2105 public Composition addAttester(CompositionAttesterComponent t) { // 3 2106 if (t == null) 2107 return this; 2108 if (this.attester == null) 2109 this.attester = new ArrayList<CompositionAttesterComponent>(); 2110 this.attester.add(t); 2111 return this; 2112 } 2113 2114 /** 2115 * @return {@link #custodian} (Identifies the organization or group who is 2116 * responsible for ongoing maintenance of and access to the 2117 * composition/document information.) 2118 */ 2119 public Reference getCustodian() { 2120 if (this.custodian == null) 2121 if (Configuration.errorOnAutoCreate()) 2122 throw new Error("Attempt to auto-create Composition.custodian"); 2123 else if (Configuration.doAutoCreate()) 2124 this.custodian = new Reference(); // cc 2125 return this.custodian; 2126 } 2127 2128 public boolean hasCustodian() { 2129 return this.custodian != null && !this.custodian.isEmpty(); 2130 } 2131 2132 /** 2133 * @param value {@link #custodian} (Identifies the organization or group who is 2134 * responsible for ongoing maintenance of and access to the 2135 * composition/document information.) 2136 */ 2137 public Composition setCustodian(Reference value) { 2138 this.custodian = value; 2139 return this; 2140 } 2141 2142 /** 2143 * @return {@link #custodian} The actual object that is the target of the 2144 * reference. The reference library doesn't populate this, but you can 2145 * use it to hold the resource if you resolve it. (Identifies the 2146 * organization or group who is responsible for ongoing maintenance of 2147 * and access to the composition/document information.) 2148 */ 2149 public Organization getCustodianTarget() { 2150 if (this.custodianTarget == null) 2151 if (Configuration.errorOnAutoCreate()) 2152 throw new Error("Attempt to auto-create Composition.custodian"); 2153 else if (Configuration.doAutoCreate()) 2154 this.custodianTarget = new Organization(); // aa 2155 return this.custodianTarget; 2156 } 2157 2158 /** 2159 * @param value {@link #custodian} The actual object that is the target of the 2160 * reference. The reference library doesn't use these, but you can 2161 * use it to hold the resource if you resolve it. (Identifies the 2162 * organization or group who is responsible for ongoing maintenance 2163 * of and access to the composition/document information.) 2164 */ 2165 public Composition setCustodianTarget(Organization value) { 2166 this.custodianTarget = value; 2167 return this; 2168 } 2169 2170 /** 2171 * @return {@link #event} (The clinical service, such as a colonoscopy or an 2172 * appendectomy, being documented.) 2173 */ 2174 public List<CompositionEventComponent> getEvent() { 2175 if (this.event == null) 2176 this.event = new ArrayList<CompositionEventComponent>(); 2177 return this.event; 2178 } 2179 2180 public boolean hasEvent() { 2181 if (this.event == null) 2182 return false; 2183 for (CompositionEventComponent item : this.event) 2184 if (!item.isEmpty()) 2185 return true; 2186 return false; 2187 } 2188 2189 /** 2190 * @return {@link #event} (The clinical service, such as a colonoscopy or an 2191 * appendectomy, being documented.) 2192 */ 2193 // syntactic sugar 2194 public CompositionEventComponent addEvent() { // 3 2195 CompositionEventComponent t = new CompositionEventComponent(); 2196 if (this.event == null) 2197 this.event = new ArrayList<CompositionEventComponent>(); 2198 this.event.add(t); 2199 return t; 2200 } 2201 2202 // syntactic sugar 2203 public Composition addEvent(CompositionEventComponent t) { // 3 2204 if (t == null) 2205 return this; 2206 if (this.event == null) 2207 this.event = new ArrayList<CompositionEventComponent>(); 2208 this.event.add(t); 2209 return this; 2210 } 2211 2212 /** 2213 * @return {@link #encounter} (Describes the clinical encounter or type of care 2214 * this documentation is associated with.) 2215 */ 2216 public Reference getEncounter() { 2217 if (this.encounter == null) 2218 if (Configuration.errorOnAutoCreate()) 2219 throw new Error("Attempt to auto-create Composition.encounter"); 2220 else if (Configuration.doAutoCreate()) 2221 this.encounter = new Reference(); // cc 2222 return this.encounter; 2223 } 2224 2225 public boolean hasEncounter() { 2226 return this.encounter != null && !this.encounter.isEmpty(); 2227 } 2228 2229 /** 2230 * @param value {@link #encounter} (Describes the clinical encounter or type of 2231 * care this documentation is associated with.) 2232 */ 2233 public Composition setEncounter(Reference value) { 2234 this.encounter = value; 2235 return this; 2236 } 2237 2238 /** 2239 * @return {@link #encounter} The actual object that is the target of the 2240 * reference. The reference library doesn't populate this, but you can 2241 * use it to hold the resource if you resolve it. (Describes the 2242 * clinical encounter or type of care this documentation is associated 2243 * with.) 2244 */ 2245 public Encounter getEncounterTarget() { 2246 if (this.encounterTarget == null) 2247 if (Configuration.errorOnAutoCreate()) 2248 throw new Error("Attempt to auto-create Composition.encounter"); 2249 else if (Configuration.doAutoCreate()) 2250 this.encounterTarget = new Encounter(); // aa 2251 return this.encounterTarget; 2252 } 2253 2254 /** 2255 * @param value {@link #encounter} The actual object that is the target of the 2256 * reference. The reference library doesn't use these, but you can 2257 * use it to hold the resource if you resolve it. (Describes the 2258 * clinical encounter or type of care this documentation is 2259 * associated with.) 2260 */ 2261 public Composition setEncounterTarget(Encounter value) { 2262 this.encounterTarget = value; 2263 return this; 2264 } 2265 2266 /** 2267 * @return {@link #section} (The root of the sections that make up the 2268 * composition.) 2269 */ 2270 public List<SectionComponent> getSection() { 2271 if (this.section == null) 2272 this.section = new ArrayList<SectionComponent>(); 2273 return this.section; 2274 } 2275 2276 public boolean hasSection() { 2277 if (this.section == null) 2278 return false; 2279 for (SectionComponent item : this.section) 2280 if (!item.isEmpty()) 2281 return true; 2282 return false; 2283 } 2284 2285 /** 2286 * @return {@link #section} (The root of the sections that make up the 2287 * composition.) 2288 */ 2289 // syntactic sugar 2290 public SectionComponent addSection() { // 3 2291 SectionComponent t = new SectionComponent(); 2292 if (this.section == null) 2293 this.section = new ArrayList<SectionComponent>(); 2294 this.section.add(t); 2295 return t; 2296 } 2297 2298 // syntactic sugar 2299 public Composition addSection(SectionComponent t) { // 3 2300 if (t == null) 2301 return this; 2302 if (this.section == null) 2303 this.section = new ArrayList<SectionComponent>(); 2304 this.section.add(t); 2305 return this; 2306 } 2307 2308 protected void listChildren(List<Property> childrenList) { 2309 super.listChildren(childrenList); 2310 childrenList.add(new Property("identifier", "Identifier", 2311 "Logical identifier for the composition, assigned when created. This identifier stays constant as the composition is changed over time.", 2312 0, java.lang.Integer.MAX_VALUE, identifier)); 2313 childrenList.add(new Property("date", "dateTime", 2314 "The composition editing time, when the composition was last logically changed by the author.", 0, 2315 java.lang.Integer.MAX_VALUE, date)); 2316 childrenList.add(new Property("type", "CodeableConcept", 2317 "Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition.", 2318 0, java.lang.Integer.MAX_VALUE, type)); 2319 childrenList.add(new Property("class", "CodeableConcept", 2320 "A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type.", 2321 0, java.lang.Integer.MAX_VALUE, class_)); 2322 childrenList.add(new Property("title", "string", "Official human-readable label for the composition.", 0, 2323 java.lang.Integer.MAX_VALUE, title)); 2324 childrenList.add(new Property("status", "code", 2325 "The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document.", 2326 0, java.lang.Integer.MAX_VALUE, status)); 2327 childrenList.add( 2328 new Property("confidentiality", "code", "The code specifying the level of confidentiality of the Composition.", 2329 0, java.lang.Integer.MAX_VALUE, confidentiality)); 2330 childrenList.add(new Property("subject", "Reference(Any)", 2331 "Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).", 2332 0, java.lang.Integer.MAX_VALUE, subject)); 2333 childrenList.add(new Property("author", "Reference(Practitioner|Device|Patient|RelatedPerson)", 2334 "Identifies who is responsible for the information in the composition, not necessarily who typed it in.", 0, 2335 java.lang.Integer.MAX_VALUE, author)); 2336 childrenList 2337 .add(new Property("attester", "", "A participant who has attested to the accuracy of the composition/document.", 2338 0, java.lang.Integer.MAX_VALUE, attester)); 2339 childrenList.add(new Property("custodian", "Reference(Organization)", 2340 "Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.", 2341 0, java.lang.Integer.MAX_VALUE, custodian)); 2342 childrenList.add( 2343 new Property("event", "", "The clinical service, such as a colonoscopy or an appendectomy, being documented.", 2344 0, java.lang.Integer.MAX_VALUE, event)); 2345 childrenList.add(new Property("encounter", "Reference(Encounter)", 2346 "Describes the clinical encounter or type of care this documentation is associated with.", 0, 2347 java.lang.Integer.MAX_VALUE, encounter)); 2348 childrenList.add(new Property("section", "", "The root of the sections that make up the composition.", 0, 2349 java.lang.Integer.MAX_VALUE, section)); 2350 } 2351 2352 @Override 2353 public void setProperty(String name, Base value) throws FHIRException { 2354 if (name.equals("identifier")) 2355 this.identifier = castToIdentifier(value); // Identifier 2356 else if (name.equals("date")) 2357 this.date = castToDateTime(value); // DateTimeType 2358 else if (name.equals("type")) 2359 this.type = castToCodeableConcept(value); // CodeableConcept 2360 else if (name.equals("class")) 2361 this.class_ = castToCodeableConcept(value); // CodeableConcept 2362 else if (name.equals("title")) 2363 this.title = castToString(value); // StringType 2364 else if (name.equals("status")) 2365 this.status = new CompositionStatusEnumFactory().fromType(value); // Enumeration<CompositionStatus> 2366 else if (name.equals("confidentiality")) 2367 this.confidentiality = castToCode(value); // CodeType 2368 else if (name.equals("subject")) 2369 this.subject = castToReference(value); // Reference 2370 else if (name.equals("author")) 2371 this.getAuthor().add(castToReference(value)); 2372 else if (name.equals("attester")) 2373 this.getAttester().add((CompositionAttesterComponent) value); 2374 else if (name.equals("custodian")) 2375 this.custodian = castToReference(value); // Reference 2376 else if (name.equals("event")) 2377 this.getEvent().add((CompositionEventComponent) value); 2378 else if (name.equals("encounter")) 2379 this.encounter = castToReference(value); // Reference 2380 else if (name.equals("section")) 2381 this.getSection().add((SectionComponent) value); 2382 else 2383 super.setProperty(name, value); 2384 } 2385 2386 @Override 2387 public Base addChild(String name) throws FHIRException { 2388 if (name.equals("identifier")) { 2389 this.identifier = new Identifier(); 2390 return this.identifier; 2391 } else if (name.equals("date")) { 2392 throw new FHIRException("Cannot call addChild on a singleton property Composition.date"); 2393 } else if (name.equals("type")) { 2394 this.type = new CodeableConcept(); 2395 return this.type; 2396 } else if (name.equals("class")) { 2397 this.class_ = new CodeableConcept(); 2398 return this.class_; 2399 } else if (name.equals("title")) { 2400 throw new FHIRException("Cannot call addChild on a singleton property Composition.title"); 2401 } else if (name.equals("status")) { 2402 throw new FHIRException("Cannot call addChild on a singleton property Composition.status"); 2403 } else if (name.equals("confidentiality")) { 2404 throw new FHIRException("Cannot call addChild on a singleton property Composition.confidentiality"); 2405 } else if (name.equals("subject")) { 2406 this.subject = new Reference(); 2407 return this.subject; 2408 } else if (name.equals("author")) { 2409 return addAuthor(); 2410 } else if (name.equals("attester")) { 2411 return addAttester(); 2412 } else if (name.equals("custodian")) { 2413 this.custodian = new Reference(); 2414 return this.custodian; 2415 } else if (name.equals("event")) { 2416 return addEvent(); 2417 } else if (name.equals("encounter")) { 2418 this.encounter = new Reference(); 2419 return this.encounter; 2420 } else if (name.equals("section")) { 2421 return addSection(); 2422 } else 2423 return super.addChild(name); 2424 } 2425 2426 public String fhirType() { 2427 return "Composition"; 2428 2429 } 2430 2431 public Composition copy() { 2432 Composition dst = new Composition(); 2433 copyValues(dst); 2434 dst.identifier = identifier == null ? null : identifier.copy(); 2435 dst.date = date == null ? null : date.copy(); 2436 dst.type = type == null ? null : type.copy(); 2437 dst.class_ = class_ == null ? null : class_.copy(); 2438 dst.title = title == null ? null : title.copy(); 2439 dst.status = status == null ? null : status.copy(); 2440 dst.confidentiality = confidentiality == null ? null : confidentiality.copy(); 2441 dst.subject = subject == null ? null : subject.copy(); 2442 if (author != null) { 2443 dst.author = new ArrayList<Reference>(); 2444 for (Reference i : author) 2445 dst.author.add(i.copy()); 2446 } 2447 ; 2448 if (attester != null) { 2449 dst.attester = new ArrayList<CompositionAttesterComponent>(); 2450 for (CompositionAttesterComponent i : attester) 2451 dst.attester.add(i.copy()); 2452 } 2453 ; 2454 dst.custodian = custodian == null ? null : custodian.copy(); 2455 if (event != null) { 2456 dst.event = new ArrayList<CompositionEventComponent>(); 2457 for (CompositionEventComponent i : event) 2458 dst.event.add(i.copy()); 2459 } 2460 ; 2461 dst.encounter = encounter == null ? null : encounter.copy(); 2462 if (section != null) { 2463 dst.section = new ArrayList<SectionComponent>(); 2464 for (SectionComponent i : section) 2465 dst.section.add(i.copy()); 2466 } 2467 ; 2468 return dst; 2469 } 2470 2471 protected Composition typedCopy() { 2472 return copy(); 2473 } 2474 2475 @Override 2476 public boolean equalsDeep(Base other) { 2477 if (!super.equalsDeep(other)) 2478 return false; 2479 if (!(other instanceof Composition)) 2480 return false; 2481 Composition o = (Composition) other; 2482 return compareDeep(identifier, o.identifier, true) && compareDeep(date, o.date, true) 2483 && compareDeep(type, o.type, true) && compareDeep(class_, o.class_, true) && compareDeep(title, o.title, true) 2484 && compareDeep(status, o.status, true) && compareDeep(confidentiality, o.confidentiality, true) 2485 && compareDeep(subject, o.subject, true) && compareDeep(author, o.author, true) 2486 && compareDeep(attester, o.attester, true) && compareDeep(custodian, o.custodian, true) 2487 && compareDeep(event, o.event, true) && compareDeep(encounter, o.encounter, true) 2488 && compareDeep(section, o.section, true); 2489 } 2490 2491 @Override 2492 public boolean equalsShallow(Base other) { 2493 if (!super.equalsShallow(other)) 2494 return false; 2495 if (!(other instanceof Composition)) 2496 return false; 2497 Composition o = (Composition) other; 2498 return compareValues(date, o.date, true) && compareValues(title, o.title, true) 2499 && compareValues(status, o.status, true) && compareValues(confidentiality, o.confidentiality, true); 2500 } 2501 2502 public boolean isEmpty() { 2503 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (date == null || date.isEmpty()) 2504 && (type == null || type.isEmpty()) && (class_ == null || class_.isEmpty()) 2505 && (title == null || title.isEmpty()) && (status == null || status.isEmpty()) 2506 && (confidentiality == null || confidentiality.isEmpty()) && (subject == null || subject.isEmpty()) 2507 && (author == null || author.isEmpty()) && (attester == null || attester.isEmpty()) 2508 && (custodian == null || custodian.isEmpty()) && (event == null || event.isEmpty()) 2509 && (encounter == null || encounter.isEmpty()) && (section == null || section.isEmpty()); 2510 } 2511 2512 @Override 2513 public ResourceType getResourceType() { 2514 return ResourceType.Composition; 2515 } 2516 2517 @SearchParamDefinition(name = "date", path = "Composition.date", description = "Composition editing time", type = "date") 2518 public static final String SP_DATE = "date"; 2519 @SearchParamDefinition(name = "identifier", path = "Composition.identifier", description = "Logical identifier of composition (version-independent)", type = "token") 2520 public static final String SP_IDENTIFIER = "identifier"; 2521 @SearchParamDefinition(name = "period", path = "Composition.event.period", description = "The period covered by the documentation", type = "date") 2522 public static final String SP_PERIOD = "period"; 2523 @SearchParamDefinition(name = "subject", path = "Composition.subject", description = "Who and/or what the composition is about", type = "reference") 2524 public static final String SP_SUBJECT = "subject"; 2525 @SearchParamDefinition(name = "author", path = "Composition.author", description = "Who and/or what authored the composition", type = "reference") 2526 public static final String SP_AUTHOR = "author"; 2527 @SearchParamDefinition(name = "confidentiality", path = "Composition.confidentiality", description = "As defined by affinity domain", type = "token") 2528 public static final String SP_CONFIDENTIALITY = "confidentiality"; 2529 @SearchParamDefinition(name = "section", path = "Composition.section.code", description = "Classification of section (recommended)", type = "token") 2530 public static final String SP_SECTION = "section"; 2531 @SearchParamDefinition(name = "encounter", path = "Composition.encounter", description = "Context of the Composition", type = "reference") 2532 public static final String SP_ENCOUNTER = "encounter"; 2533 @SearchParamDefinition(name = "type", path = "Composition.type", description = "Kind of composition (LOINC if possible)", type = "token") 2534 public static final String SP_TYPE = "type"; 2535 @SearchParamDefinition(name = "title", path = "Composition.title", description = "Human Readable name/title", type = "string") 2536 public static final String SP_TITLE = "title"; 2537 @SearchParamDefinition(name = "attester", path = "Composition.attester.party", description = "Who attested the composition", type = "reference") 2538 public static final String SP_ATTESTER = "attester"; 2539 @SearchParamDefinition(name = "entry", path = "Composition.section.entry", description = "A reference to data that supports this section", type = "reference") 2540 public static final String SP_ENTRY = "entry"; 2541 @SearchParamDefinition(name = "patient", path = "Composition.subject", description = "Who and/or what the composition is about", type = "reference") 2542 public static final String SP_PATIENT = "patient"; 2543 @SearchParamDefinition(name = "context", path = "Composition.event.code", description = "Code(s) that apply to the event being documented", type = "token") 2544 public static final String SP_CONTEXT = "context"; 2545 @SearchParamDefinition(name = "class", path = "Composition.class", description = "Categorization of Composition", type = "token") 2546 public static final String SP_CLASS = "class"; 2547 @SearchParamDefinition(name = "status", path = "Composition.status", description = "preliminary | final | amended | entered-in-error", type = "token") 2548 public static final String SP_STATUS = "status"; 2549 2550}