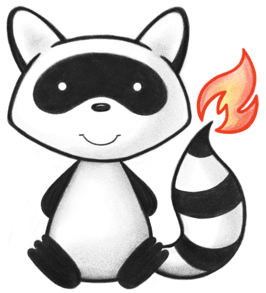
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.ConceptMapEquivalence; 038import org.hl7.fhir.dstu2.model.Enumerations.ConceptMapEquivalenceEnumFactory; 039import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus; 040import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory; 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 047import org.hl7.fhir.exceptions.FHIRException; 048import org.hl7.fhir.utilities.Utilities; 049 050/** 051 * A statement of relationships from one set of concepts to one or more other 052 * concepts - either code systems or data elements, or classes in class models. 053 */ 054@ResourceDef(name = "ConceptMap", profile = "http://hl7.org/fhir/Profile/ConceptMap") 055public class ConceptMap extends DomainResource { 056 057 @Block() 058 public static class ConceptMapContactComponent extends BackboneElement implements IBaseBackboneElement { 059 /** 060 * The name of an individual to contact regarding the concept map. 061 */ 062 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 063 @Description(shortDefinition = "Name of a individual to contact", formalDefinition = "The name of an individual to contact regarding the concept map.") 064 protected StringType name; 065 066 /** 067 * Contact details for individual (if a name was provided) or the publisher. 068 */ 069 @Child(name = "telecom", type = { 070 ContactPoint.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 071 @Description(shortDefinition = "Contact details for individual or publisher", formalDefinition = "Contact details for individual (if a name was provided) or the publisher.") 072 protected List<ContactPoint> telecom; 073 074 private static final long serialVersionUID = -1179697803L; 075 076 /* 077 * Constructor 078 */ 079 public ConceptMapContactComponent() { 080 super(); 081 } 082 083 /** 084 * @return {@link #name} (The name of an individual to contact regarding the 085 * concept map.). This is the underlying object with id, value and 086 * extensions. The accessor "getName" gives direct access to the value 087 */ 088 public StringType getNameElement() { 089 if (this.name == null) 090 if (Configuration.errorOnAutoCreate()) 091 throw new Error("Attempt to auto-create ConceptMapContactComponent.name"); 092 else if (Configuration.doAutoCreate()) 093 this.name = new StringType(); // bb 094 return this.name; 095 } 096 097 public boolean hasNameElement() { 098 return this.name != null && !this.name.isEmpty(); 099 } 100 101 public boolean hasName() { 102 return this.name != null && !this.name.isEmpty(); 103 } 104 105 /** 106 * @param value {@link #name} (The name of an individual to contact regarding 107 * the concept map.). This is the underlying object with id, value 108 * and extensions. The accessor "getName" gives direct access to 109 * the value 110 */ 111 public ConceptMapContactComponent setNameElement(StringType value) { 112 this.name = value; 113 return this; 114 } 115 116 /** 117 * @return The name of an individual to contact regarding the concept map. 118 */ 119 public String getName() { 120 return this.name == null ? null : this.name.getValue(); 121 } 122 123 /** 124 * @param value The name of an individual to contact regarding the concept map. 125 */ 126 public ConceptMapContactComponent setName(String value) { 127 if (Utilities.noString(value)) 128 this.name = null; 129 else { 130 if (this.name == null) 131 this.name = new StringType(); 132 this.name.setValue(value); 133 } 134 return this; 135 } 136 137 /** 138 * @return {@link #telecom} (Contact details for individual (if a name was 139 * provided) or the publisher.) 140 */ 141 public List<ContactPoint> getTelecom() { 142 if (this.telecom == null) 143 this.telecom = new ArrayList<ContactPoint>(); 144 return this.telecom; 145 } 146 147 public boolean hasTelecom() { 148 if (this.telecom == null) 149 return false; 150 for (ContactPoint item : this.telecom) 151 if (!item.isEmpty()) 152 return true; 153 return false; 154 } 155 156 /** 157 * @return {@link #telecom} (Contact details for individual (if a name was 158 * provided) or the publisher.) 159 */ 160 // syntactic sugar 161 public ContactPoint addTelecom() { // 3 162 ContactPoint t = new ContactPoint(); 163 if (this.telecom == null) 164 this.telecom = new ArrayList<ContactPoint>(); 165 this.telecom.add(t); 166 return t; 167 } 168 169 // syntactic sugar 170 public ConceptMapContactComponent addTelecom(ContactPoint t) { // 3 171 if (t == null) 172 return this; 173 if (this.telecom == null) 174 this.telecom = new ArrayList<ContactPoint>(); 175 this.telecom.add(t); 176 return this; 177 } 178 179 protected void listChildren(List<Property> childrenList) { 180 super.listChildren(childrenList); 181 childrenList.add(new Property("name", "string", "The name of an individual to contact regarding the concept map.", 182 0, java.lang.Integer.MAX_VALUE, name)); 183 childrenList.add(new Property("telecom", "ContactPoint", 184 "Contact details for individual (if a name was provided) or the publisher.", 0, java.lang.Integer.MAX_VALUE, 185 telecom)); 186 } 187 188 @Override 189 public void setProperty(String name, Base value) throws FHIRException { 190 if (name.equals("name")) 191 this.name = castToString(value); // StringType 192 else if (name.equals("telecom")) 193 this.getTelecom().add(castToContactPoint(value)); 194 else 195 super.setProperty(name, value); 196 } 197 198 @Override 199 public Base addChild(String name) throws FHIRException { 200 if (name.equals("name")) { 201 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.name"); 202 } else if (name.equals("telecom")) { 203 return addTelecom(); 204 } else 205 return super.addChild(name); 206 } 207 208 public ConceptMapContactComponent copy() { 209 ConceptMapContactComponent dst = new ConceptMapContactComponent(); 210 copyValues(dst); 211 dst.name = name == null ? null : name.copy(); 212 if (telecom != null) { 213 dst.telecom = new ArrayList<ContactPoint>(); 214 for (ContactPoint i : telecom) 215 dst.telecom.add(i.copy()); 216 } 217 ; 218 return dst; 219 } 220 221 @Override 222 public boolean equalsDeep(Base other) { 223 if (!super.equalsDeep(other)) 224 return false; 225 if (!(other instanceof ConceptMapContactComponent)) 226 return false; 227 ConceptMapContactComponent o = (ConceptMapContactComponent) other; 228 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 229 } 230 231 @Override 232 public boolean equalsShallow(Base other) { 233 if (!super.equalsShallow(other)) 234 return false; 235 if (!(other instanceof ConceptMapContactComponent)) 236 return false; 237 ConceptMapContactComponent o = (ConceptMapContactComponent) other; 238 return compareValues(name, o.name, true); 239 } 240 241 public boolean isEmpty() { 242 return super.isEmpty() && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()); 243 } 244 245 public String fhirType() { 246 return "ConceptMap.contact"; 247 248 } 249 250 } 251 252 @Block() 253 public static class SourceElementComponent extends BackboneElement implements IBaseBackboneElement { 254 /** 255 * An absolute URI that identifies the Code System (if the source is a value set 256 * that crosses more than one code system). 257 */ 258 @Child(name = "codeSystem", type = { 259 UriType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 260 @Description(shortDefinition = "Code System (if value set crosses code systems)", formalDefinition = "An absolute URI that identifies the Code System (if the source is a value set that crosses more than one code system).") 261 protected UriType codeSystem; 262 263 /** 264 * Identity (code or path) or the element/item being mapped. 265 */ 266 @Child(name = "code", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 267 @Description(shortDefinition = "Identifies element being mapped", formalDefinition = "Identity (code or path) or the element/item being mapped.") 268 protected CodeType code; 269 270 /** 271 * A concept from the target value set that this concept maps to. 272 */ 273 @Child(name = "target", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 274 @Description(shortDefinition = "Concept in target system for element", formalDefinition = "A concept from the target value set that this concept maps to.") 275 protected List<TargetElementComponent> target; 276 277 private static final long serialVersionUID = -458143877L; 278 279 /* 280 * Constructor 281 */ 282 public SourceElementComponent() { 283 super(); 284 } 285 286 /** 287 * @return {@link #codeSystem} (An absolute URI that identifies the Code System 288 * (if the source is a value set that crosses more than one code 289 * system).). This is the underlying object with id, value and 290 * extensions. The accessor "getCodeSystem" gives direct access to the 291 * value 292 */ 293 public UriType getCodeSystemElement() { 294 if (this.codeSystem == null) 295 if (Configuration.errorOnAutoCreate()) 296 throw new Error("Attempt to auto-create SourceElementComponent.codeSystem"); 297 else if (Configuration.doAutoCreate()) 298 this.codeSystem = new UriType(); // bb 299 return this.codeSystem; 300 } 301 302 public boolean hasCodeSystemElement() { 303 return this.codeSystem != null && !this.codeSystem.isEmpty(); 304 } 305 306 public boolean hasCodeSystem() { 307 return this.codeSystem != null && !this.codeSystem.isEmpty(); 308 } 309 310 /** 311 * @param value {@link #codeSystem} (An absolute URI that identifies the Code 312 * System (if the source is a value set that crosses more than one 313 * code system).). This is the underlying object with id, value and 314 * extensions. The accessor "getCodeSystem" gives direct access to 315 * the value 316 */ 317 public SourceElementComponent setCodeSystemElement(UriType value) { 318 this.codeSystem = value; 319 return this; 320 } 321 322 /** 323 * @return An absolute URI that identifies the Code System (if the source is a 324 * value set that crosses more than one code system). 325 */ 326 public String getCodeSystem() { 327 return this.codeSystem == null ? null : this.codeSystem.getValue(); 328 } 329 330 /** 331 * @param value An absolute URI that identifies the Code System (if the source 332 * is a value set that crosses more than one code system). 333 */ 334 public SourceElementComponent setCodeSystem(String value) { 335 if (Utilities.noString(value)) 336 this.codeSystem = null; 337 else { 338 if (this.codeSystem == null) 339 this.codeSystem = new UriType(); 340 this.codeSystem.setValue(value); 341 } 342 return this; 343 } 344 345 /** 346 * @return {@link #code} (Identity (code or path) or the element/item being 347 * mapped.). This is the underlying object with id, value and 348 * extensions. The accessor "getCode" gives direct access to the value 349 */ 350 public CodeType getCodeElement() { 351 if (this.code == null) 352 if (Configuration.errorOnAutoCreate()) 353 throw new Error("Attempt to auto-create SourceElementComponent.code"); 354 else if (Configuration.doAutoCreate()) 355 this.code = new CodeType(); // bb 356 return this.code; 357 } 358 359 public boolean hasCodeElement() { 360 return this.code != null && !this.code.isEmpty(); 361 } 362 363 public boolean hasCode() { 364 return this.code != null && !this.code.isEmpty(); 365 } 366 367 /** 368 * @param value {@link #code} (Identity (code or path) or the element/item being 369 * mapped.). This is the underlying object with id, value and 370 * extensions. The accessor "getCode" gives direct access to the 371 * value 372 */ 373 public SourceElementComponent setCodeElement(CodeType value) { 374 this.code = value; 375 return this; 376 } 377 378 /** 379 * @return Identity (code or path) or the element/item being mapped. 380 */ 381 public String getCode() { 382 return this.code == null ? null : this.code.getValue(); 383 } 384 385 /** 386 * @param value Identity (code or path) or the element/item being mapped. 387 */ 388 public SourceElementComponent setCode(String value) { 389 if (Utilities.noString(value)) 390 this.code = null; 391 else { 392 if (this.code == null) 393 this.code = new CodeType(); 394 this.code.setValue(value); 395 } 396 return this; 397 } 398 399 /** 400 * @return {@link #target} (A concept from the target value set that this 401 * concept maps to.) 402 */ 403 public List<TargetElementComponent> getTarget() { 404 if (this.target == null) 405 this.target = new ArrayList<TargetElementComponent>(); 406 return this.target; 407 } 408 409 public boolean hasTarget() { 410 if (this.target == null) 411 return false; 412 for (TargetElementComponent item : this.target) 413 if (!item.isEmpty()) 414 return true; 415 return false; 416 } 417 418 /** 419 * @return {@link #target} (A concept from the target value set that this 420 * concept maps to.) 421 */ 422 // syntactic sugar 423 public TargetElementComponent addTarget() { // 3 424 TargetElementComponent t = new TargetElementComponent(); 425 if (this.target == null) 426 this.target = new ArrayList<TargetElementComponent>(); 427 this.target.add(t); 428 return t; 429 } 430 431 // syntactic sugar 432 public SourceElementComponent addTarget(TargetElementComponent t) { // 3 433 if (t == null) 434 return this; 435 if (this.target == null) 436 this.target = new ArrayList<TargetElementComponent>(); 437 this.target.add(t); 438 return this; 439 } 440 441 protected void listChildren(List<Property> childrenList) { 442 super.listChildren(childrenList); 443 childrenList.add(new Property("codeSystem", "uri", 444 "An absolute URI that identifies the Code System (if the source is a value set that crosses more than one code system).", 445 0, java.lang.Integer.MAX_VALUE, codeSystem)); 446 childrenList.add(new Property("code", "code", "Identity (code or path) or the element/item being mapped.", 0, 447 java.lang.Integer.MAX_VALUE, code)); 448 childrenList.add(new Property("target", "", "A concept from the target value set that this concept maps to.", 0, 449 java.lang.Integer.MAX_VALUE, target)); 450 } 451 452 @Override 453 public void setProperty(String name, Base value) throws FHIRException { 454 if (name.equals("codeSystem")) 455 this.codeSystem = castToUri(value); // UriType 456 else if (name.equals("code")) 457 this.code = castToCode(value); // CodeType 458 else if (name.equals("target")) 459 this.getTarget().add((TargetElementComponent) value); 460 else 461 super.setProperty(name, value); 462 } 463 464 @Override 465 public Base addChild(String name) throws FHIRException { 466 if (name.equals("codeSystem")) { 467 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.codeSystem"); 468 } else if (name.equals("code")) { 469 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.code"); 470 } else if (name.equals("target")) { 471 return addTarget(); 472 } else 473 return super.addChild(name); 474 } 475 476 public SourceElementComponent copy() { 477 SourceElementComponent dst = new SourceElementComponent(); 478 copyValues(dst); 479 dst.codeSystem = codeSystem == null ? null : codeSystem.copy(); 480 dst.code = code == null ? null : code.copy(); 481 if (target != null) { 482 dst.target = new ArrayList<TargetElementComponent>(); 483 for (TargetElementComponent i : target) 484 dst.target.add(i.copy()); 485 } 486 ; 487 return dst; 488 } 489 490 @Override 491 public boolean equalsDeep(Base other) { 492 if (!super.equalsDeep(other)) 493 return false; 494 if (!(other instanceof SourceElementComponent)) 495 return false; 496 SourceElementComponent o = (SourceElementComponent) other; 497 return compareDeep(codeSystem, o.codeSystem, true) && compareDeep(code, o.code, true) 498 && compareDeep(target, o.target, true); 499 } 500 501 @Override 502 public boolean equalsShallow(Base other) { 503 if (!super.equalsShallow(other)) 504 return false; 505 if (!(other instanceof SourceElementComponent)) 506 return false; 507 SourceElementComponent o = (SourceElementComponent) other; 508 return compareValues(codeSystem, o.codeSystem, true) && compareValues(code, o.code, true); 509 } 510 511 public boolean isEmpty() { 512 return super.isEmpty() && (codeSystem == null || codeSystem.isEmpty()) && (code == null || code.isEmpty()) 513 && (target == null || target.isEmpty()); 514 } 515 516 public String fhirType() { 517 return "ConceptMap.element"; 518 519 } 520 521 } 522 523 @Block() 524 public static class TargetElementComponent extends BackboneElement implements IBaseBackboneElement { 525 /** 526 * An absolute URI that identifies the code system of the target code (if the 527 * target is a value set that cross code systems). 528 */ 529 @Child(name = "codeSystem", type = { 530 UriType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 531 @Description(shortDefinition = "System of the target (if necessary)", formalDefinition = "An absolute URI that identifies the code system of the target code (if the target is a value set that cross code systems).") 532 protected UriType codeSystem; 533 534 /** 535 * Identity (code or path) or the element/item that the map refers to. 536 */ 537 @Child(name = "code", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 538 @Description(shortDefinition = "Code that identifies the target element", formalDefinition = "Identity (code or path) or the element/item that the map refers to.") 539 protected CodeType code; 540 541 /** 542 * The equivalence between the source and target concepts (counting for the 543 * dependencies and products). The equivalence is read from target to source 544 * (e.g. the target is 'wider' than the source). 545 */ 546 @Child(name = "equivalence", type = { 547 CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = false) 548 @Description(shortDefinition = "equivalent | equal | wider | subsumes | narrower | specializes | inexact | unmatched | disjoint", formalDefinition = "The equivalence between the source and target concepts (counting for the dependencies and products). The equivalence is read from target to source (e.g. the target is 'wider' than the source).") 549 protected Enumeration<ConceptMapEquivalence> equivalence; 550 551 /** 552 * A description of status/issues in mapping that conveys additional information 553 * not represented in the structured data. 554 */ 555 @Child(name = "comments", type = { 556 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 557 @Description(shortDefinition = "Description of status/issues in mapping", formalDefinition = "A description of status/issues in mapping that conveys additional information not represented in the structured data.") 558 protected StringType comments; 559 560 /** 561 * A set of additional dependencies for this mapping to hold. This mapping is 562 * only applicable if the specified element can be resolved, and it has the 563 * specified value. 564 */ 565 @Child(name = "dependsOn", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 566 @Description(shortDefinition = "Other elements required for this mapping (from context)", formalDefinition = "A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified element can be resolved, and it has the specified value.") 567 protected List<OtherElementComponent> dependsOn; 568 569 /** 570 * A set of additional outcomes from this mapping to other elements. To properly 571 * execute this mapping, the specified element must be mapped to some data 572 * element or source that is in context. The mapping may still be useful without 573 * a place for the additional data elements, but the equivalence cannot be 574 * relied on. 575 */ 576 @Child(name = "product", type = { 577 OtherElementComponent.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 578 @Description(shortDefinition = "Other concepts that this mapping also produces", formalDefinition = "A set of additional outcomes from this mapping to other elements. To properly execute this mapping, the specified element must be mapped to some data element or source that is in context. The mapping may still be useful without a place for the additional data elements, but the equivalence cannot be relied on.") 579 protected List<OtherElementComponent> product; 580 581 private static final long serialVersionUID = -804990059L; 582 583 /* 584 * Constructor 585 */ 586 public TargetElementComponent() { 587 super(); 588 } 589 590 /* 591 * Constructor 592 */ 593 public TargetElementComponent(Enumeration<ConceptMapEquivalence> equivalence) { 594 super(); 595 this.equivalence = equivalence; 596 } 597 598 /** 599 * @return {@link #codeSystem} (An absolute URI that identifies the code system 600 * of the target code (if the target is a value set that cross code 601 * systems).). This is the underlying object with id, value and 602 * extensions. The accessor "getCodeSystem" gives direct access to the 603 * value 604 */ 605 public UriType getCodeSystemElement() { 606 if (this.codeSystem == null) 607 if (Configuration.errorOnAutoCreate()) 608 throw new Error("Attempt to auto-create TargetElementComponent.codeSystem"); 609 else if (Configuration.doAutoCreate()) 610 this.codeSystem = new UriType(); // bb 611 return this.codeSystem; 612 } 613 614 public boolean hasCodeSystemElement() { 615 return this.codeSystem != null && !this.codeSystem.isEmpty(); 616 } 617 618 public boolean hasCodeSystem() { 619 return this.codeSystem != null && !this.codeSystem.isEmpty(); 620 } 621 622 /** 623 * @param value {@link #codeSystem} (An absolute URI that identifies the code 624 * system of the target code (if the target is a value set that 625 * cross code systems).). This is the underlying object with id, 626 * value and extensions. The accessor "getCodeSystem" gives direct 627 * access to the value 628 */ 629 public TargetElementComponent setCodeSystemElement(UriType value) { 630 this.codeSystem = value; 631 return this; 632 } 633 634 /** 635 * @return An absolute URI that identifies the code system of the target code 636 * (if the target is a value set that cross code systems). 637 */ 638 public String getCodeSystem() { 639 return this.codeSystem == null ? null : this.codeSystem.getValue(); 640 } 641 642 /** 643 * @param value An absolute URI that identifies the code system of the target 644 * code (if the target is a value set that cross code systems). 645 */ 646 public TargetElementComponent setCodeSystem(String value) { 647 if (Utilities.noString(value)) 648 this.codeSystem = null; 649 else { 650 if (this.codeSystem == null) 651 this.codeSystem = new UriType(); 652 this.codeSystem.setValue(value); 653 } 654 return this; 655 } 656 657 /** 658 * @return {@link #code} (Identity (code or path) or the element/item that the 659 * map refers to.). This is the underlying object with id, value and 660 * extensions. The accessor "getCode" gives direct access to the value 661 */ 662 public CodeType getCodeElement() { 663 if (this.code == null) 664 if (Configuration.errorOnAutoCreate()) 665 throw new Error("Attempt to auto-create TargetElementComponent.code"); 666 else if (Configuration.doAutoCreate()) 667 this.code = new CodeType(); // bb 668 return this.code; 669 } 670 671 public boolean hasCodeElement() { 672 return this.code != null && !this.code.isEmpty(); 673 } 674 675 public boolean hasCode() { 676 return this.code != null && !this.code.isEmpty(); 677 } 678 679 /** 680 * @param value {@link #code} (Identity (code or path) or the element/item that 681 * the map refers to.). This is the underlying object with id, 682 * value and extensions. The accessor "getCode" gives direct access 683 * to the value 684 */ 685 public TargetElementComponent setCodeElement(CodeType value) { 686 this.code = value; 687 return this; 688 } 689 690 /** 691 * @return Identity (code or path) or the element/item that the map refers to. 692 */ 693 public String getCode() { 694 return this.code == null ? null : this.code.getValue(); 695 } 696 697 /** 698 * @param value Identity (code or path) or the element/item that the map refers 699 * to. 700 */ 701 public TargetElementComponent setCode(String value) { 702 if (Utilities.noString(value)) 703 this.code = null; 704 else { 705 if (this.code == null) 706 this.code = new CodeType(); 707 this.code.setValue(value); 708 } 709 return this; 710 } 711 712 /** 713 * @return {@link #equivalence} (The equivalence between the source and target 714 * concepts (counting for the dependencies and products). The 715 * equivalence is read from target to source (e.g. the target is 'wider' 716 * than the source).). This is the underlying object with id, value and 717 * extensions. The accessor "getEquivalence" gives direct access to the 718 * value 719 */ 720 public Enumeration<ConceptMapEquivalence> getEquivalenceElement() { 721 if (this.equivalence == null) 722 if (Configuration.errorOnAutoCreate()) 723 throw new Error("Attempt to auto-create TargetElementComponent.equivalence"); 724 else if (Configuration.doAutoCreate()) 725 this.equivalence = new Enumeration<ConceptMapEquivalence>(new ConceptMapEquivalenceEnumFactory()); // bb 726 return this.equivalence; 727 } 728 729 public boolean hasEquivalenceElement() { 730 return this.equivalence != null && !this.equivalence.isEmpty(); 731 } 732 733 public boolean hasEquivalence() { 734 return this.equivalence != null && !this.equivalence.isEmpty(); 735 } 736 737 /** 738 * @param value {@link #equivalence} (The equivalence between the source and 739 * target concepts (counting for the dependencies and products). 740 * The equivalence is read from target to source (e.g. the target 741 * is 'wider' than the source).). This is the underlying object 742 * with id, value and extensions. The accessor "getEquivalence" 743 * gives direct access to the value 744 */ 745 public TargetElementComponent setEquivalenceElement(Enumeration<ConceptMapEquivalence> value) { 746 this.equivalence = value; 747 return this; 748 } 749 750 /** 751 * @return The equivalence between the source and target concepts (counting for 752 * the dependencies and products). The equivalence is read from target 753 * to source (e.g. the target is 'wider' than the source). 754 */ 755 public ConceptMapEquivalence getEquivalence() { 756 return this.equivalence == null ? null : this.equivalence.getValue(); 757 } 758 759 /** 760 * @param value The equivalence between the source and target concepts (counting 761 * for the dependencies and products). The equivalence is read from 762 * target to source (e.g. the target is 'wider' than the source). 763 */ 764 public TargetElementComponent setEquivalence(ConceptMapEquivalence value) { 765 if (this.equivalence == null) 766 this.equivalence = new Enumeration<ConceptMapEquivalence>(new ConceptMapEquivalenceEnumFactory()); 767 this.equivalence.setValue(value); 768 return this; 769 } 770 771 /** 772 * @return {@link #comments} (A description of status/issues in mapping that 773 * conveys additional information not represented in the structured 774 * data.). This is the underlying object with id, value and extensions. 775 * The accessor "getComments" gives direct access to the value 776 */ 777 public StringType getCommentsElement() { 778 if (this.comments == null) 779 if (Configuration.errorOnAutoCreate()) 780 throw new Error("Attempt to auto-create TargetElementComponent.comments"); 781 else if (Configuration.doAutoCreate()) 782 this.comments = new StringType(); // bb 783 return this.comments; 784 } 785 786 public boolean hasCommentsElement() { 787 return this.comments != null && !this.comments.isEmpty(); 788 } 789 790 public boolean hasComments() { 791 return this.comments != null && !this.comments.isEmpty(); 792 } 793 794 /** 795 * @param value {@link #comments} (A description of status/issues in mapping 796 * that conveys additional information not represented in the 797 * structured data.). This is the underlying object with id, value 798 * and extensions. The accessor "getComments" gives direct access 799 * to the value 800 */ 801 public TargetElementComponent setCommentsElement(StringType value) { 802 this.comments = value; 803 return this; 804 } 805 806 /** 807 * @return A description of status/issues in mapping that conveys additional 808 * information not represented in the structured data. 809 */ 810 public String getComments() { 811 return this.comments == null ? null : this.comments.getValue(); 812 } 813 814 /** 815 * @param value A description of status/issues in mapping that conveys 816 * additional information not represented in the structured data. 817 */ 818 public TargetElementComponent setComments(String value) { 819 if (Utilities.noString(value)) 820 this.comments = null; 821 else { 822 if (this.comments == null) 823 this.comments = new StringType(); 824 this.comments.setValue(value); 825 } 826 return this; 827 } 828 829 /** 830 * @return {@link #dependsOn} (A set of additional dependencies for this mapping 831 * to hold. This mapping is only applicable if the specified element can 832 * be resolved, and it has the specified value.) 833 */ 834 public List<OtherElementComponent> getDependsOn() { 835 if (this.dependsOn == null) 836 this.dependsOn = new ArrayList<OtherElementComponent>(); 837 return this.dependsOn; 838 } 839 840 public boolean hasDependsOn() { 841 if (this.dependsOn == null) 842 return false; 843 for (OtherElementComponent item : this.dependsOn) 844 if (!item.isEmpty()) 845 return true; 846 return false; 847 } 848 849 /** 850 * @return {@link #dependsOn} (A set of additional dependencies for this mapping 851 * to hold. This mapping is only applicable if the specified element can 852 * be resolved, and it has the specified value.) 853 */ 854 // syntactic sugar 855 public OtherElementComponent addDependsOn() { // 3 856 OtherElementComponent t = new OtherElementComponent(); 857 if (this.dependsOn == null) 858 this.dependsOn = new ArrayList<OtherElementComponent>(); 859 this.dependsOn.add(t); 860 return t; 861 } 862 863 // syntactic sugar 864 public TargetElementComponent addDependsOn(OtherElementComponent t) { // 3 865 if (t == null) 866 return this; 867 if (this.dependsOn == null) 868 this.dependsOn = new ArrayList<OtherElementComponent>(); 869 this.dependsOn.add(t); 870 return this; 871 } 872 873 /** 874 * @return {@link #product} (A set of additional outcomes from this mapping to 875 * other elements. To properly execute this mapping, the specified 876 * element must be mapped to some data element or source that is in 877 * context. The mapping may still be useful without a place for the 878 * additional data elements, but the equivalence cannot be relied on.) 879 */ 880 public List<OtherElementComponent> getProduct() { 881 if (this.product == null) 882 this.product = new ArrayList<OtherElementComponent>(); 883 return this.product; 884 } 885 886 public boolean hasProduct() { 887 if (this.product == null) 888 return false; 889 for (OtherElementComponent item : this.product) 890 if (!item.isEmpty()) 891 return true; 892 return false; 893 } 894 895 /** 896 * @return {@link #product} (A set of additional outcomes from this mapping to 897 * other elements. To properly execute this mapping, the specified 898 * element must be mapped to some data element or source that is in 899 * context. The mapping may still be useful without a place for the 900 * additional data elements, but the equivalence cannot be relied on.) 901 */ 902 // syntactic sugar 903 public OtherElementComponent addProduct() { // 3 904 OtherElementComponent t = new OtherElementComponent(); 905 if (this.product == null) 906 this.product = new ArrayList<OtherElementComponent>(); 907 this.product.add(t); 908 return t; 909 } 910 911 // syntactic sugar 912 public TargetElementComponent addProduct(OtherElementComponent t) { // 3 913 if (t == null) 914 return this; 915 if (this.product == null) 916 this.product = new ArrayList<OtherElementComponent>(); 917 this.product.add(t); 918 return this; 919 } 920 921 protected void listChildren(List<Property> childrenList) { 922 super.listChildren(childrenList); 923 childrenList.add(new Property("codeSystem", "uri", 924 "An absolute URI that identifies the code system of the target code (if the target is a value set that cross code systems).", 925 0, java.lang.Integer.MAX_VALUE, codeSystem)); 926 childrenList.add(new Property("code", "code", 927 "Identity (code or path) or the element/item that the map refers to.", 0, java.lang.Integer.MAX_VALUE, code)); 928 childrenList.add(new Property("equivalence", "code", 929 "The equivalence between the source and target concepts (counting for the dependencies and products). The equivalence is read from target to source (e.g. the target is 'wider' than the source).", 930 0, java.lang.Integer.MAX_VALUE, equivalence)); 931 childrenList.add(new Property("comments", "string", 932 "A description of status/issues in mapping that conveys additional information not represented in the structured data.", 933 0, java.lang.Integer.MAX_VALUE, comments)); 934 childrenList.add(new Property("dependsOn", "", 935 "A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified element can be resolved, and it has the specified value.", 936 0, java.lang.Integer.MAX_VALUE, dependsOn)); 937 childrenList.add(new Property("product", "@ConceptMap.element.target.dependsOn", 938 "A set of additional outcomes from this mapping to other elements. To properly execute this mapping, the specified element must be mapped to some data element or source that is in context. The mapping may still be useful without a place for the additional data elements, but the equivalence cannot be relied on.", 939 0, java.lang.Integer.MAX_VALUE, product)); 940 } 941 942 @Override 943 public void setProperty(String name, Base value) throws FHIRException { 944 if (name.equals("codeSystem")) 945 this.codeSystem = castToUri(value); // UriType 946 else if (name.equals("code")) 947 this.code = castToCode(value); // CodeType 948 else if (name.equals("equivalence")) 949 this.equivalence = new ConceptMapEquivalenceEnumFactory().fromType(value); // Enumeration<ConceptMapEquivalence> 950 else if (name.equals("comments")) 951 this.comments = castToString(value); // StringType 952 else if (name.equals("dependsOn")) 953 this.getDependsOn().add((OtherElementComponent) value); 954 else if (name.equals("product")) 955 this.getProduct().add((OtherElementComponent) value); 956 else 957 super.setProperty(name, value); 958 } 959 960 @Override 961 public Base addChild(String name) throws FHIRException { 962 if (name.equals("codeSystem")) { 963 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.codeSystem"); 964 } else if (name.equals("code")) { 965 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.code"); 966 } else if (name.equals("equivalence")) { 967 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.equivalence"); 968 } else if (name.equals("comments")) { 969 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.comments"); 970 } else if (name.equals("dependsOn")) { 971 return addDependsOn(); 972 } else if (name.equals("product")) { 973 return addProduct(); 974 } else 975 return super.addChild(name); 976 } 977 978 public TargetElementComponent copy() { 979 TargetElementComponent dst = new TargetElementComponent(); 980 copyValues(dst); 981 dst.codeSystem = codeSystem == null ? null : codeSystem.copy(); 982 dst.code = code == null ? null : code.copy(); 983 dst.equivalence = equivalence == null ? null : equivalence.copy(); 984 dst.comments = comments == null ? null : comments.copy(); 985 if (dependsOn != null) { 986 dst.dependsOn = new ArrayList<OtherElementComponent>(); 987 for (OtherElementComponent i : dependsOn) 988 dst.dependsOn.add(i.copy()); 989 } 990 ; 991 if (product != null) { 992 dst.product = new ArrayList<OtherElementComponent>(); 993 for (OtherElementComponent i : product) 994 dst.product.add(i.copy()); 995 } 996 ; 997 return dst; 998 } 999 1000 @Override 1001 public boolean equalsDeep(Base other) { 1002 if (!super.equalsDeep(other)) 1003 return false; 1004 if (!(other instanceof TargetElementComponent)) 1005 return false; 1006 TargetElementComponent o = (TargetElementComponent) other; 1007 return compareDeep(codeSystem, o.codeSystem, true) && compareDeep(code, o.code, true) 1008 && compareDeep(equivalence, o.equivalence, true) && compareDeep(comments, o.comments, true) 1009 && compareDeep(dependsOn, o.dependsOn, true) && compareDeep(product, o.product, true); 1010 } 1011 1012 @Override 1013 public boolean equalsShallow(Base other) { 1014 if (!super.equalsShallow(other)) 1015 return false; 1016 if (!(other instanceof TargetElementComponent)) 1017 return false; 1018 TargetElementComponent o = (TargetElementComponent) other; 1019 return compareValues(codeSystem, o.codeSystem, true) && compareValues(code, o.code, true) 1020 && compareValues(equivalence, o.equivalence, true) && compareValues(comments, o.comments, true); 1021 } 1022 1023 public boolean isEmpty() { 1024 return super.isEmpty() && (codeSystem == null || codeSystem.isEmpty()) && (code == null || code.isEmpty()) 1025 && (equivalence == null || equivalence.isEmpty()) && (comments == null || comments.isEmpty()) 1026 && (dependsOn == null || dependsOn.isEmpty()) && (product == null || product.isEmpty()); 1027 } 1028 1029 public String fhirType() { 1030 return "ConceptMap.element.target"; 1031 1032 } 1033 1034 } 1035 1036 @Block() 1037 public static class OtherElementComponent extends BackboneElement implements IBaseBackboneElement { 1038 /** 1039 * A reference to a specific concept that holds a coded value. This can be an 1040 * element in a FHIR resource, or a specific reference to a data element in a 1041 * different specification (e.g. HL7 v2) or a general reference to a kind of 1042 * data field, or a reference to a value set with an appropriately narrow 1043 * definition. 1044 */ 1045 @Child(name = "element", type = { UriType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1046 @Description(shortDefinition = "Reference to element/field/ValueSet mapping depends on", formalDefinition = "A reference to a specific concept that holds a coded value. This can be an element in a FHIR resource, or a specific reference to a data element in a different specification (e.g. HL7 v2) or a general reference to a kind of data field, or a reference to a value set with an appropriately narrow definition.") 1047 protected UriType element; 1048 1049 /** 1050 * An absolute URI that identifies the code system of the dependency code (if 1051 * the source/dependency is a value set that crosses code systems). 1052 */ 1053 @Child(name = "codeSystem", type = { 1054 UriType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1055 @Description(shortDefinition = "Code System (if necessary)", formalDefinition = "An absolute URI that identifies the code system of the dependency code (if the source/dependency is a value set that crosses code systems).") 1056 protected UriType codeSystem; 1057 1058 /** 1059 * Identity (code or path) or the element/item/ValueSet that the map depends on 1060 * / refers to. 1061 */ 1062 @Child(name = "code", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 1063 @Description(shortDefinition = "Value of the referenced element", formalDefinition = "Identity (code or path) or the element/item/ValueSet that the map depends on / refers to.") 1064 protected StringType code; 1065 1066 private static final long serialVersionUID = 1488522448L; 1067 1068 /* 1069 * Constructor 1070 */ 1071 public OtherElementComponent() { 1072 super(); 1073 } 1074 1075 /* 1076 * Constructor 1077 */ 1078 public OtherElementComponent(UriType element, UriType codeSystem, StringType code) { 1079 super(); 1080 this.element = element; 1081 this.codeSystem = codeSystem; 1082 this.code = code; 1083 } 1084 1085 /** 1086 * @return {@link #element} (A reference to a specific concept that holds a 1087 * coded value. This can be an element in a FHIR resource, or a specific 1088 * reference to a data element in a different specification (e.g. HL7 1089 * v2) or a general reference to a kind of data field, or a reference to 1090 * a value set with an appropriately narrow definition.). This is the 1091 * underlying object with id, value and extensions. The accessor 1092 * "getElement" gives direct access to the value 1093 */ 1094 public UriType getElementElement() { 1095 if (this.element == null) 1096 if (Configuration.errorOnAutoCreate()) 1097 throw new Error("Attempt to auto-create OtherElementComponent.element"); 1098 else if (Configuration.doAutoCreate()) 1099 this.element = new UriType(); // bb 1100 return this.element; 1101 } 1102 1103 public boolean hasElementElement() { 1104 return this.element != null && !this.element.isEmpty(); 1105 } 1106 1107 public boolean hasElement() { 1108 return this.element != null && !this.element.isEmpty(); 1109 } 1110 1111 /** 1112 * @param value {@link #element} (A reference to a specific concept that holds a 1113 * coded value. This can be an element in a FHIR resource, or a 1114 * specific reference to a data element in a different 1115 * specification (e.g. HL7 v2) or a general reference to a kind of 1116 * data field, or a reference to a value set with an appropriately 1117 * narrow definition.). This is the underlying object with id, 1118 * value and extensions. The accessor "getElement" gives direct 1119 * access to the value 1120 */ 1121 public OtherElementComponent setElementElement(UriType value) { 1122 this.element = value; 1123 return this; 1124 } 1125 1126 /** 1127 * @return A reference to a specific concept that holds a coded value. This can 1128 * be an element in a FHIR resource, or a specific reference to a data 1129 * element in a different specification (e.g. HL7 v2) or a general 1130 * reference to a kind of data field, or a reference to a value set with 1131 * an appropriately narrow definition. 1132 */ 1133 public String getElement() { 1134 return this.element == null ? null : this.element.getValue(); 1135 } 1136 1137 /** 1138 * @param value A reference to a specific concept that holds a coded value. This 1139 * can be an element in a FHIR resource, or a specific reference to 1140 * a data element in a different specification (e.g. HL7 v2) or a 1141 * general reference to a kind of data field, or a reference to a 1142 * value set with an appropriately narrow definition. 1143 */ 1144 public OtherElementComponent setElement(String value) { 1145 if (this.element == null) 1146 this.element = new UriType(); 1147 this.element.setValue(value); 1148 return this; 1149 } 1150 1151 /** 1152 * @return {@link #codeSystem} (An absolute URI that identifies the code system 1153 * of the dependency code (if the source/dependency is a value set that 1154 * crosses code systems).). This is the underlying object with id, value 1155 * and extensions. The accessor "getCodeSystem" gives direct access to 1156 * the value 1157 */ 1158 public UriType getCodeSystemElement() { 1159 if (this.codeSystem == null) 1160 if (Configuration.errorOnAutoCreate()) 1161 throw new Error("Attempt to auto-create OtherElementComponent.codeSystem"); 1162 else if (Configuration.doAutoCreate()) 1163 this.codeSystem = new UriType(); // bb 1164 return this.codeSystem; 1165 } 1166 1167 public boolean hasCodeSystemElement() { 1168 return this.codeSystem != null && !this.codeSystem.isEmpty(); 1169 } 1170 1171 public boolean hasCodeSystem() { 1172 return this.codeSystem != null && !this.codeSystem.isEmpty(); 1173 } 1174 1175 /** 1176 * @param value {@link #codeSystem} (An absolute URI that identifies the code 1177 * system of the dependency code (if the source/dependency is a 1178 * value set that crosses code systems).). This is the underlying 1179 * object with id, value and extensions. The accessor 1180 * "getCodeSystem" gives direct access to the value 1181 */ 1182 public OtherElementComponent setCodeSystemElement(UriType value) { 1183 this.codeSystem = value; 1184 return this; 1185 } 1186 1187 /** 1188 * @return An absolute URI that identifies the code system of the dependency 1189 * code (if the source/dependency is a value set that crosses code 1190 * systems). 1191 */ 1192 public String getCodeSystem() { 1193 return this.codeSystem == null ? null : this.codeSystem.getValue(); 1194 } 1195 1196 /** 1197 * @param value An absolute URI that identifies the code system of the 1198 * dependency code (if the source/dependency is a value set that 1199 * crosses code systems). 1200 */ 1201 public OtherElementComponent setCodeSystem(String value) { 1202 if (this.codeSystem == null) 1203 this.codeSystem = new UriType(); 1204 this.codeSystem.setValue(value); 1205 return this; 1206 } 1207 1208 /** 1209 * @return {@link #code} (Identity (code or path) or the element/item/ValueSet 1210 * that the map depends on / refers to.). This is the underlying object 1211 * with id, value and extensions. The accessor "getCode" gives direct 1212 * access to the value 1213 */ 1214 public StringType getCodeElement() { 1215 if (this.code == null) 1216 if (Configuration.errorOnAutoCreate()) 1217 throw new Error("Attempt to auto-create OtherElementComponent.code"); 1218 else if (Configuration.doAutoCreate()) 1219 this.code = new StringType(); // bb 1220 return this.code; 1221 } 1222 1223 public boolean hasCodeElement() { 1224 return this.code != null && !this.code.isEmpty(); 1225 } 1226 1227 public boolean hasCode() { 1228 return this.code != null && !this.code.isEmpty(); 1229 } 1230 1231 /** 1232 * @param value {@link #code} (Identity (code or path) or the 1233 * element/item/ValueSet that the map depends on / refers to.). 1234 * This is the underlying object with id, value and extensions. The 1235 * accessor "getCode" gives direct access to the value 1236 */ 1237 public OtherElementComponent setCodeElement(StringType value) { 1238 this.code = value; 1239 return this; 1240 } 1241 1242 /** 1243 * @return Identity (code or path) or the element/item/ValueSet that the map 1244 * depends on / refers to. 1245 */ 1246 public String getCode() { 1247 return this.code == null ? null : this.code.getValue(); 1248 } 1249 1250 /** 1251 * @param value Identity (code or path) or the element/item/ValueSet that the 1252 * map depends on / refers to. 1253 */ 1254 public OtherElementComponent setCode(String value) { 1255 if (this.code == null) 1256 this.code = new StringType(); 1257 this.code.setValue(value); 1258 return this; 1259 } 1260 1261 protected void listChildren(List<Property> childrenList) { 1262 super.listChildren(childrenList); 1263 childrenList.add(new Property("element", "uri", 1264 "A reference to a specific concept that holds a coded value. This can be an element in a FHIR resource, or a specific reference to a data element in a different specification (e.g. HL7 v2) or a general reference to a kind of data field, or a reference to a value set with an appropriately narrow definition.", 1265 0, java.lang.Integer.MAX_VALUE, element)); 1266 childrenList.add(new Property("codeSystem", "uri", 1267 "An absolute URI that identifies the code system of the dependency code (if the source/dependency is a value set that crosses code systems).", 1268 0, java.lang.Integer.MAX_VALUE, codeSystem)); 1269 childrenList.add(new Property("code", "string", 1270 "Identity (code or path) or the element/item/ValueSet that the map depends on / refers to.", 0, 1271 java.lang.Integer.MAX_VALUE, code)); 1272 } 1273 1274 @Override 1275 public void setProperty(String name, Base value) throws FHIRException { 1276 if (name.equals("element")) 1277 this.element = castToUri(value); // UriType 1278 else if (name.equals("codeSystem")) 1279 this.codeSystem = castToUri(value); // UriType 1280 else if (name.equals("code")) 1281 this.code = castToString(value); // StringType 1282 else 1283 super.setProperty(name, value); 1284 } 1285 1286 @Override 1287 public Base addChild(String name) throws FHIRException { 1288 if (name.equals("element")) { 1289 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.element"); 1290 } else if (name.equals("codeSystem")) { 1291 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.codeSystem"); 1292 } else if (name.equals("code")) { 1293 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.code"); 1294 } else 1295 return super.addChild(name); 1296 } 1297 1298 public OtherElementComponent copy() { 1299 OtherElementComponent dst = new OtherElementComponent(); 1300 copyValues(dst); 1301 dst.element = element == null ? null : element.copy(); 1302 dst.codeSystem = codeSystem == null ? null : codeSystem.copy(); 1303 dst.code = code == null ? null : code.copy(); 1304 return dst; 1305 } 1306 1307 @Override 1308 public boolean equalsDeep(Base other) { 1309 if (!super.equalsDeep(other)) 1310 return false; 1311 if (!(other instanceof OtherElementComponent)) 1312 return false; 1313 OtherElementComponent o = (OtherElementComponent) other; 1314 return compareDeep(element, o.element, true) && compareDeep(codeSystem, o.codeSystem, true) 1315 && compareDeep(code, o.code, true); 1316 } 1317 1318 @Override 1319 public boolean equalsShallow(Base other) { 1320 if (!super.equalsShallow(other)) 1321 return false; 1322 if (!(other instanceof OtherElementComponent)) 1323 return false; 1324 OtherElementComponent o = (OtherElementComponent) other; 1325 return compareValues(element, o.element, true) && compareValues(codeSystem, o.codeSystem, true) 1326 && compareValues(code, o.code, true); 1327 } 1328 1329 public boolean isEmpty() { 1330 return super.isEmpty() && (element == null || element.isEmpty()) && (codeSystem == null || codeSystem.isEmpty()) 1331 && (code == null || code.isEmpty()); 1332 } 1333 1334 public String fhirType() { 1335 return "ConceptMap.element.target.dependsOn"; 1336 1337 } 1338 1339 } 1340 1341 /** 1342 * An absolute URL that is used to identify this concept map when it is 1343 * referenced in a specification, model, design or an instance. This SHALL be a 1344 * URL, SHOULD be globally unique, and SHOULD be an address at which this 1345 * concept map is (or will be) published. 1346 */ 1347 @Child(name = "url", type = { UriType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 1348 @Description(shortDefinition = "Globally unique logical id for concept map", formalDefinition = "An absolute URL that is used to identify this concept map when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this concept map is (or will be) published.") 1349 protected UriType url; 1350 1351 /** 1352 * Formal identifier that is used to identify this concept map when it is 1353 * represented in other formats, or referenced in a specification, model, design 1354 * or an instance. 1355 */ 1356 @Child(name = "identifier", type = { 1357 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1358 @Description(shortDefinition = "Additional identifier for the concept map", formalDefinition = "Formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance.") 1359 protected Identifier identifier; 1360 1361 /** 1362 * The identifier that is used to identify this version of the concept map when 1363 * it is referenced in a specification, model, design or instance. This is an 1364 * arbitrary value managed by the profile author manually and the value should 1365 * be a timestamp. 1366 */ 1367 @Child(name = "version", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1368 @Description(shortDefinition = "Logical id for this version of the concept map", formalDefinition = "The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the profile author manually and the value should be a timestamp.") 1369 protected StringType version; 1370 1371 /** 1372 * A free text natural language name describing the concept map. 1373 */ 1374 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1375 @Description(shortDefinition = "Informal name for this concept map", formalDefinition = "A free text natural language name describing the concept map.") 1376 protected StringType name; 1377 1378 /** 1379 * The status of the concept map. 1380 */ 1381 @Child(name = "status", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = true, summary = true) 1382 @Description(shortDefinition = "draft | active | retired", formalDefinition = "The status of the concept map.") 1383 protected Enumeration<ConformanceResourceStatus> status; 1384 1385 /** 1386 * This ConceptMap was authored for testing purposes (or 1387 * education/evaluation/marketing), and is not intended to be used for genuine 1388 * usage. 1389 */ 1390 @Child(name = "experimental", type = { 1391 BooleanType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1392 @Description(shortDefinition = "If for testing purposes, not real usage", formalDefinition = "This ConceptMap was authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.") 1393 protected BooleanType experimental; 1394 1395 /** 1396 * The name of the individual or organization that published the concept map. 1397 */ 1398 @Child(name = "publisher", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1399 @Description(shortDefinition = "Name of the publisher (organization or individual)", formalDefinition = "The name of the individual or organization that published the concept map.") 1400 protected StringType publisher; 1401 1402 /** 1403 * Contacts to assist a user in finding and communicating with the publisher. 1404 */ 1405 @Child(name = "contact", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1406 @Description(shortDefinition = "Contact details of the publisher", formalDefinition = "Contacts to assist a user in finding and communicating with the publisher.") 1407 protected List<ConceptMapContactComponent> contact; 1408 1409 /** 1410 * The date this version of the concept map was published. The date must change 1411 * when the business version changes, if it does, and it must change if the 1412 * status code changes. In addition, it should change when the substantive 1413 * content of the concept map changes. 1414 */ 1415 @Child(name = "date", type = { DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1416 @Description(shortDefinition = "Date for given status", formalDefinition = "The date this version of the concept map was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes.") 1417 protected DateTimeType date; 1418 1419 /** 1420 * A free text natural language description of the use of the concept map - 1421 * reason for definition, conditions of use, etc. 1422 */ 1423 @Child(name = "description", type = { 1424 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1425 @Description(shortDefinition = "Human language description of the concept map", formalDefinition = "A free text natural language description of the use of the concept map - reason for definition, conditions of use, etc.") 1426 protected StringType description; 1427 1428 /** 1429 * The content was developed with a focus and intent of supporting the contexts 1430 * that are listed. These terms may be used to assist with indexing and 1431 * searching of concept map instances. 1432 */ 1433 @Child(name = "useContext", type = { 1434 CodeableConcept.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1435 @Description(shortDefinition = "Content intends to support these contexts", formalDefinition = "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of concept map instances.") 1436 protected List<CodeableConcept> useContext; 1437 1438 /** 1439 * Explains why this concept map is needed and why it has been constrained as it 1440 * has. 1441 */ 1442 @Child(name = "requirements", type = { 1443 StringType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 1444 @Description(shortDefinition = "Why needed", formalDefinition = "Explains why this concept map is needed and why it has been constrained as it has.") 1445 protected StringType requirements; 1446 1447 /** 1448 * A copyright statement relating to the concept map and/or its contents. 1449 */ 1450 @Child(name = "copyright", type = { 1451 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1452 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the concept map and/or its contents.") 1453 protected StringType copyright; 1454 1455 /** 1456 * The source value set that specifies the concepts that are being mapped. 1457 */ 1458 @Child(name = "source", type = { UriType.class, ValueSet.class, 1459 StructureDefinition.class }, order = 13, min = 1, max = 1, modifier = false, summary = true) 1460 @Description(shortDefinition = "Identifies the source of the concepts which are being mapped", formalDefinition = "The source value set that specifies the concepts that are being mapped.") 1461 protected Type source; 1462 1463 /** 1464 * The target value set provides context to the mappings. Note that the mapping 1465 * is made between concepts, not between value sets, but the value set provides 1466 * important context about how the concept mapping choices are made. 1467 */ 1468 @Child(name = "target", type = { UriType.class, ValueSet.class, 1469 StructureDefinition.class }, order = 14, min = 1, max = 1, modifier = false, summary = true) 1470 @Description(shortDefinition = "Provides context to the mappings", formalDefinition = "The target value set provides context to the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.") 1471 protected Type target; 1472 1473 /** 1474 * Mappings for an individual concept in the source to one or more concepts in 1475 * the target. 1476 */ 1477 @Child(name = "element", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1478 @Description(shortDefinition = "Mappings for a concept from the source set", formalDefinition = "Mappings for an individual concept in the source to one or more concepts in the target.") 1479 protected List<SourceElementComponent> element; 1480 1481 private static final long serialVersionUID = 1687563642L; 1482 1483 /* 1484 * Constructor 1485 */ 1486 public ConceptMap() { 1487 super(); 1488 } 1489 1490 /* 1491 * Constructor 1492 */ 1493 public ConceptMap(Enumeration<ConformanceResourceStatus> status, Type source, Type target) { 1494 super(); 1495 this.status = status; 1496 this.source = source; 1497 this.target = target; 1498 } 1499 1500 /** 1501 * @return {@link #url} (An absolute URL that is used to identify this concept 1502 * map when it is referenced in a specification, model, design or an 1503 * instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD 1504 * be an address at which this concept map is (or will be) published.). 1505 * This is the underlying object with id, value and extensions. The 1506 * accessor "getUrl" gives direct access to the value 1507 */ 1508 public UriType getUrlElement() { 1509 if (this.url == null) 1510 if (Configuration.errorOnAutoCreate()) 1511 throw new Error("Attempt to auto-create ConceptMap.url"); 1512 else if (Configuration.doAutoCreate()) 1513 this.url = new UriType(); // bb 1514 return this.url; 1515 } 1516 1517 public boolean hasUrlElement() { 1518 return this.url != null && !this.url.isEmpty(); 1519 } 1520 1521 public boolean hasUrl() { 1522 return this.url != null && !this.url.isEmpty(); 1523 } 1524 1525 /** 1526 * @param value {@link #url} (An absolute URL that is used to identify this 1527 * concept map when it is referenced in a specification, model, 1528 * design or an instance. This SHALL be a URL, SHOULD be globally 1529 * unique, and SHOULD be an address at which this concept map is 1530 * (or will be) published.). This is the underlying object with id, 1531 * value and extensions. The accessor "getUrl" gives direct access 1532 * to the value 1533 */ 1534 public ConceptMap setUrlElement(UriType value) { 1535 this.url = value; 1536 return this; 1537 } 1538 1539 /** 1540 * @return An absolute URL that is used to identify this concept map when it is 1541 * referenced in a specification, model, design or an instance. This 1542 * SHALL be a URL, SHOULD be globally unique, and SHOULD be an address 1543 * at which this concept map is (or will be) published. 1544 */ 1545 public String getUrl() { 1546 return this.url == null ? null : this.url.getValue(); 1547 } 1548 1549 /** 1550 * @param value An absolute URL that is used to identify this concept map when 1551 * it is referenced in a specification, model, design or an 1552 * instance. This SHALL be a URL, SHOULD be globally unique, and 1553 * SHOULD be an address at which this concept map is (or will be) 1554 * published. 1555 */ 1556 public ConceptMap setUrl(String value) { 1557 if (Utilities.noString(value)) 1558 this.url = null; 1559 else { 1560 if (this.url == null) 1561 this.url = new UriType(); 1562 this.url.setValue(value); 1563 } 1564 return this; 1565 } 1566 1567 /** 1568 * @return {@link #identifier} (Formal identifier that is used to identify this 1569 * concept map when it is represented in other formats, or referenced in 1570 * a specification, model, design or an instance.) 1571 */ 1572 public Identifier getIdentifier() { 1573 if (this.identifier == null) 1574 if (Configuration.errorOnAutoCreate()) 1575 throw new Error("Attempt to auto-create ConceptMap.identifier"); 1576 else if (Configuration.doAutoCreate()) 1577 this.identifier = new Identifier(); // cc 1578 return this.identifier; 1579 } 1580 1581 public boolean hasIdentifier() { 1582 return this.identifier != null && !this.identifier.isEmpty(); 1583 } 1584 1585 /** 1586 * @param value {@link #identifier} (Formal identifier that is used to identify 1587 * this concept map when it is represented in other formats, or 1588 * referenced in a specification, model, design or an instance.) 1589 */ 1590 public ConceptMap setIdentifier(Identifier value) { 1591 this.identifier = value; 1592 return this; 1593 } 1594 1595 /** 1596 * @return {@link #version} (The identifier that is used to identify this 1597 * version of the concept map when it is referenced in a specification, 1598 * model, design or instance. This is an arbitrary value managed by the 1599 * profile author manually and the value should be a timestamp.). This 1600 * is the underlying object with id, value and extensions. The accessor 1601 * "getVersion" gives direct access to the value 1602 */ 1603 public StringType getVersionElement() { 1604 if (this.version == null) 1605 if (Configuration.errorOnAutoCreate()) 1606 throw new Error("Attempt to auto-create ConceptMap.version"); 1607 else if (Configuration.doAutoCreate()) 1608 this.version = new StringType(); // bb 1609 return this.version; 1610 } 1611 1612 public boolean hasVersionElement() { 1613 return this.version != null && !this.version.isEmpty(); 1614 } 1615 1616 public boolean hasVersion() { 1617 return this.version != null && !this.version.isEmpty(); 1618 } 1619 1620 /** 1621 * @param value {@link #version} (The identifier that is used to identify this 1622 * version of the concept map when it is referenced in a 1623 * specification, model, design or instance. This is an arbitrary 1624 * value managed by the profile author manually and the value 1625 * should be a timestamp.). This is the underlying object with id, 1626 * value and extensions. The accessor "getVersion" gives direct 1627 * access to the value 1628 */ 1629 public ConceptMap setVersionElement(StringType value) { 1630 this.version = value; 1631 return this; 1632 } 1633 1634 /** 1635 * @return The identifier that is used to identify this version of the concept 1636 * map when it is referenced in a specification, model, design or 1637 * instance. This is an arbitrary value managed by the profile author 1638 * manually and the value should be a timestamp. 1639 */ 1640 public String getVersion() { 1641 return this.version == null ? null : this.version.getValue(); 1642 } 1643 1644 /** 1645 * @param value The identifier that is used to identify this version of the 1646 * concept map when it is referenced in a specification, model, 1647 * design or instance. This is an arbitrary value managed by the 1648 * profile author manually and the value should be a timestamp. 1649 */ 1650 public ConceptMap setVersion(String value) { 1651 if (Utilities.noString(value)) 1652 this.version = null; 1653 else { 1654 if (this.version == null) 1655 this.version = new StringType(); 1656 this.version.setValue(value); 1657 } 1658 return this; 1659 } 1660 1661 /** 1662 * @return {@link #name} (A free text natural language name describing the 1663 * concept map.). This is the underlying object with id, value and 1664 * extensions. The accessor "getName" gives direct access to the value 1665 */ 1666 public StringType getNameElement() { 1667 if (this.name == null) 1668 if (Configuration.errorOnAutoCreate()) 1669 throw new Error("Attempt to auto-create ConceptMap.name"); 1670 else if (Configuration.doAutoCreate()) 1671 this.name = new StringType(); // bb 1672 return this.name; 1673 } 1674 1675 public boolean hasNameElement() { 1676 return this.name != null && !this.name.isEmpty(); 1677 } 1678 1679 public boolean hasName() { 1680 return this.name != null && !this.name.isEmpty(); 1681 } 1682 1683 /** 1684 * @param value {@link #name} (A free text natural language name describing the 1685 * concept map.). This is the underlying object with id, value and 1686 * extensions. The accessor "getName" gives direct access to the 1687 * value 1688 */ 1689 public ConceptMap setNameElement(StringType value) { 1690 this.name = value; 1691 return this; 1692 } 1693 1694 /** 1695 * @return A free text natural language name describing the concept map. 1696 */ 1697 public String getName() { 1698 return this.name == null ? null : this.name.getValue(); 1699 } 1700 1701 /** 1702 * @param value A free text natural language name describing the concept map. 1703 */ 1704 public ConceptMap setName(String value) { 1705 if (Utilities.noString(value)) 1706 this.name = null; 1707 else { 1708 if (this.name == null) 1709 this.name = new StringType(); 1710 this.name.setValue(value); 1711 } 1712 return this; 1713 } 1714 1715 /** 1716 * @return {@link #status} (The status of the concept map.). This is the 1717 * underlying object with id, value and extensions. The accessor 1718 * "getStatus" gives direct access to the value 1719 */ 1720 public Enumeration<ConformanceResourceStatus> getStatusElement() { 1721 if (this.status == null) 1722 if (Configuration.errorOnAutoCreate()) 1723 throw new Error("Attempt to auto-create ConceptMap.status"); 1724 else if (Configuration.doAutoCreate()) 1725 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); // bb 1726 return this.status; 1727 } 1728 1729 public boolean hasStatusElement() { 1730 return this.status != null && !this.status.isEmpty(); 1731 } 1732 1733 public boolean hasStatus() { 1734 return this.status != null && !this.status.isEmpty(); 1735 } 1736 1737 /** 1738 * @param value {@link #status} (The status of the concept map.). This is the 1739 * underlying object with id, value and extensions. The accessor 1740 * "getStatus" gives direct access to the value 1741 */ 1742 public ConceptMap setStatusElement(Enumeration<ConformanceResourceStatus> value) { 1743 this.status = value; 1744 return this; 1745 } 1746 1747 /** 1748 * @return The status of the concept map. 1749 */ 1750 public ConformanceResourceStatus getStatus() { 1751 return this.status == null ? null : this.status.getValue(); 1752 } 1753 1754 /** 1755 * @param value The status of the concept map. 1756 */ 1757 public ConceptMap setStatus(ConformanceResourceStatus value) { 1758 if (this.status == null) 1759 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); 1760 this.status.setValue(value); 1761 return this; 1762 } 1763 1764 /** 1765 * @return {@link #experimental} (This ConceptMap was authored for testing 1766 * purposes (or education/evaluation/marketing), and is not intended to 1767 * be used for genuine usage.). This is the underlying object with id, 1768 * value and extensions. The accessor "getExperimental" gives direct 1769 * access to the value 1770 */ 1771 public BooleanType getExperimentalElement() { 1772 if (this.experimental == null) 1773 if (Configuration.errorOnAutoCreate()) 1774 throw new Error("Attempt to auto-create ConceptMap.experimental"); 1775 else if (Configuration.doAutoCreate()) 1776 this.experimental = new BooleanType(); // bb 1777 return this.experimental; 1778 } 1779 1780 public boolean hasExperimentalElement() { 1781 return this.experimental != null && !this.experimental.isEmpty(); 1782 } 1783 1784 public boolean hasExperimental() { 1785 return this.experimental != null && !this.experimental.isEmpty(); 1786 } 1787 1788 /** 1789 * @param value {@link #experimental} (This ConceptMap was authored for testing 1790 * purposes (or education/evaluation/marketing), and is not 1791 * intended to be used for genuine usage.). This is the underlying 1792 * object with id, value and extensions. The accessor 1793 * "getExperimental" gives direct access to the value 1794 */ 1795 public ConceptMap setExperimentalElement(BooleanType value) { 1796 this.experimental = value; 1797 return this; 1798 } 1799 1800 /** 1801 * @return This ConceptMap was authored for testing purposes (or 1802 * education/evaluation/marketing), and is not intended to be used for 1803 * genuine usage. 1804 */ 1805 public boolean getExperimental() { 1806 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1807 } 1808 1809 /** 1810 * @param value This ConceptMap was authored for testing purposes (or 1811 * education/evaluation/marketing), and is not intended to be used 1812 * for genuine usage. 1813 */ 1814 public ConceptMap setExperimental(boolean value) { 1815 if (this.experimental == null) 1816 this.experimental = new BooleanType(); 1817 this.experimental.setValue(value); 1818 return this; 1819 } 1820 1821 /** 1822 * @return {@link #publisher} (The name of the individual or organization that 1823 * published the concept map.). This is the underlying object with id, 1824 * value and extensions. The accessor "getPublisher" gives direct access 1825 * to the value 1826 */ 1827 public StringType getPublisherElement() { 1828 if (this.publisher == null) 1829 if (Configuration.errorOnAutoCreate()) 1830 throw new Error("Attempt to auto-create ConceptMap.publisher"); 1831 else if (Configuration.doAutoCreate()) 1832 this.publisher = new StringType(); // bb 1833 return this.publisher; 1834 } 1835 1836 public boolean hasPublisherElement() { 1837 return this.publisher != null && !this.publisher.isEmpty(); 1838 } 1839 1840 public boolean hasPublisher() { 1841 return this.publisher != null && !this.publisher.isEmpty(); 1842 } 1843 1844 /** 1845 * @param value {@link #publisher} (The name of the individual or organization 1846 * that published the concept map.). This is the underlying object 1847 * with id, value and extensions. The accessor "getPublisher" gives 1848 * direct access to the value 1849 */ 1850 public ConceptMap setPublisherElement(StringType value) { 1851 this.publisher = value; 1852 return this; 1853 } 1854 1855 /** 1856 * @return The name of the individual or organization that published the concept 1857 * map. 1858 */ 1859 public String getPublisher() { 1860 return this.publisher == null ? null : this.publisher.getValue(); 1861 } 1862 1863 /** 1864 * @param value The name of the individual or organization that published the 1865 * concept map. 1866 */ 1867 public ConceptMap setPublisher(String value) { 1868 if (Utilities.noString(value)) 1869 this.publisher = null; 1870 else { 1871 if (this.publisher == null) 1872 this.publisher = new StringType(); 1873 this.publisher.setValue(value); 1874 } 1875 return this; 1876 } 1877 1878 /** 1879 * @return {@link #contact} (Contacts to assist a user in finding and 1880 * communicating with the publisher.) 1881 */ 1882 public List<ConceptMapContactComponent> getContact() { 1883 if (this.contact == null) 1884 this.contact = new ArrayList<ConceptMapContactComponent>(); 1885 return this.contact; 1886 } 1887 1888 public boolean hasContact() { 1889 if (this.contact == null) 1890 return false; 1891 for (ConceptMapContactComponent item : this.contact) 1892 if (!item.isEmpty()) 1893 return true; 1894 return false; 1895 } 1896 1897 /** 1898 * @return {@link #contact} (Contacts to assist a user in finding and 1899 * communicating with the publisher.) 1900 */ 1901 // syntactic sugar 1902 public ConceptMapContactComponent addContact() { // 3 1903 ConceptMapContactComponent t = new ConceptMapContactComponent(); 1904 if (this.contact == null) 1905 this.contact = new ArrayList<ConceptMapContactComponent>(); 1906 this.contact.add(t); 1907 return t; 1908 } 1909 1910 // syntactic sugar 1911 public ConceptMap addContact(ConceptMapContactComponent t) { // 3 1912 if (t == null) 1913 return this; 1914 if (this.contact == null) 1915 this.contact = new ArrayList<ConceptMapContactComponent>(); 1916 this.contact.add(t); 1917 return this; 1918 } 1919 1920 /** 1921 * @return {@link #date} (The date this version of the concept map was 1922 * published. The date must change when the business version changes, if 1923 * it does, and it must change if the status code changes. In addition, 1924 * it should change when the substantive content of the concept map 1925 * changes.). This is the underlying object with id, value and 1926 * extensions. The accessor "getDate" gives direct access to the value 1927 */ 1928 public DateTimeType getDateElement() { 1929 if (this.date == null) 1930 if (Configuration.errorOnAutoCreate()) 1931 throw new Error("Attempt to auto-create ConceptMap.date"); 1932 else if (Configuration.doAutoCreate()) 1933 this.date = new DateTimeType(); // bb 1934 return this.date; 1935 } 1936 1937 public boolean hasDateElement() { 1938 return this.date != null && !this.date.isEmpty(); 1939 } 1940 1941 public boolean hasDate() { 1942 return this.date != null && !this.date.isEmpty(); 1943 } 1944 1945 /** 1946 * @param value {@link #date} (The date this version of the concept map was 1947 * published. The date must change when the business version 1948 * changes, if it does, and it must change if the status code 1949 * changes. In addition, it should change when the substantive 1950 * content of the concept map changes.). This is the underlying 1951 * object with id, value and extensions. The accessor "getDate" 1952 * gives direct access to the value 1953 */ 1954 public ConceptMap setDateElement(DateTimeType value) { 1955 this.date = value; 1956 return this; 1957 } 1958 1959 /** 1960 * @return The date this version of the concept map was published. The date must 1961 * change when the business version changes, if it does, and it must 1962 * change if the status code changes. In addition, it should change when 1963 * the substantive content of the concept map changes. 1964 */ 1965 public Date getDate() { 1966 return this.date == null ? null : this.date.getValue(); 1967 } 1968 1969 /** 1970 * @param value The date this version of the concept map was published. The date 1971 * must change when the business version changes, if it does, and 1972 * it must change if the status code changes. In addition, it 1973 * should change when the substantive content of the concept map 1974 * changes. 1975 */ 1976 public ConceptMap setDate(Date value) { 1977 if (value == null) 1978 this.date = null; 1979 else { 1980 if (this.date == null) 1981 this.date = new DateTimeType(); 1982 this.date.setValue(value); 1983 } 1984 return this; 1985 } 1986 1987 /** 1988 * @return {@link #description} (A free text natural language description of the 1989 * use of the concept map - reason for definition, conditions of use, 1990 * etc.). This is the underlying object with id, value and extensions. 1991 * The accessor "getDescription" gives direct access to the value 1992 */ 1993 public StringType getDescriptionElement() { 1994 if (this.description == null) 1995 if (Configuration.errorOnAutoCreate()) 1996 throw new Error("Attempt to auto-create ConceptMap.description"); 1997 else if (Configuration.doAutoCreate()) 1998 this.description = new StringType(); // bb 1999 return this.description; 2000 } 2001 2002 public boolean hasDescriptionElement() { 2003 return this.description != null && !this.description.isEmpty(); 2004 } 2005 2006 public boolean hasDescription() { 2007 return this.description != null && !this.description.isEmpty(); 2008 } 2009 2010 /** 2011 * @param value {@link #description} (A free text natural language description 2012 * of the use of the concept map - reason for definition, 2013 * conditions of use, etc.). This is the underlying object with id, 2014 * value and extensions. The accessor "getDescription" gives direct 2015 * access to the value 2016 */ 2017 public ConceptMap setDescriptionElement(StringType value) { 2018 this.description = value; 2019 return this; 2020 } 2021 2022 /** 2023 * @return A free text natural language description of the use of the concept 2024 * map - reason for definition, conditions of use, etc. 2025 */ 2026 public String getDescription() { 2027 return this.description == null ? null : this.description.getValue(); 2028 } 2029 2030 /** 2031 * @param value A free text natural language description of the use of the 2032 * concept map - reason for definition, conditions of use, etc. 2033 */ 2034 public ConceptMap setDescription(String value) { 2035 if (Utilities.noString(value)) 2036 this.description = null; 2037 else { 2038 if (this.description == null) 2039 this.description = new StringType(); 2040 this.description.setValue(value); 2041 } 2042 return this; 2043 } 2044 2045 /** 2046 * @return {@link #useContext} (The content was developed with a focus and 2047 * intent of supporting the contexts that are listed. These terms may be 2048 * used to assist with indexing and searching of concept map instances.) 2049 */ 2050 public List<CodeableConcept> getUseContext() { 2051 if (this.useContext == null) 2052 this.useContext = new ArrayList<CodeableConcept>(); 2053 return this.useContext; 2054 } 2055 2056 public boolean hasUseContext() { 2057 if (this.useContext == null) 2058 return false; 2059 for (CodeableConcept item : this.useContext) 2060 if (!item.isEmpty()) 2061 return true; 2062 return false; 2063 } 2064 2065 /** 2066 * @return {@link #useContext} (The content was developed with a focus and 2067 * intent of supporting the contexts that are listed. These terms may be 2068 * used to assist with indexing and searching of concept map instances.) 2069 */ 2070 // syntactic sugar 2071 public CodeableConcept addUseContext() { // 3 2072 CodeableConcept t = new CodeableConcept(); 2073 if (this.useContext == null) 2074 this.useContext = new ArrayList<CodeableConcept>(); 2075 this.useContext.add(t); 2076 return t; 2077 } 2078 2079 // syntactic sugar 2080 public ConceptMap addUseContext(CodeableConcept t) { // 3 2081 if (t == null) 2082 return this; 2083 if (this.useContext == null) 2084 this.useContext = new ArrayList<CodeableConcept>(); 2085 this.useContext.add(t); 2086 return this; 2087 } 2088 2089 /** 2090 * @return {@link #requirements} (Explains why this concept map is needed and 2091 * why it has been constrained as it has.). This is the underlying 2092 * object with id, value and extensions. The accessor "getRequirements" 2093 * gives direct access to the value 2094 */ 2095 public StringType getRequirementsElement() { 2096 if (this.requirements == null) 2097 if (Configuration.errorOnAutoCreate()) 2098 throw new Error("Attempt to auto-create ConceptMap.requirements"); 2099 else if (Configuration.doAutoCreate()) 2100 this.requirements = new StringType(); // bb 2101 return this.requirements; 2102 } 2103 2104 public boolean hasRequirementsElement() { 2105 return this.requirements != null && !this.requirements.isEmpty(); 2106 } 2107 2108 public boolean hasRequirements() { 2109 return this.requirements != null && !this.requirements.isEmpty(); 2110 } 2111 2112 /** 2113 * @param value {@link #requirements} (Explains why this concept map is needed 2114 * and why it has been constrained as it has.). This is the 2115 * underlying object with id, value and extensions. The accessor 2116 * "getRequirements" gives direct access to the value 2117 */ 2118 public ConceptMap setRequirementsElement(StringType value) { 2119 this.requirements = value; 2120 return this; 2121 } 2122 2123 /** 2124 * @return Explains why this concept map is needed and why it has been 2125 * constrained as it has. 2126 */ 2127 public String getRequirements() { 2128 return this.requirements == null ? null : this.requirements.getValue(); 2129 } 2130 2131 /** 2132 * @param value Explains why this concept map is needed and why it has been 2133 * constrained as it has. 2134 */ 2135 public ConceptMap setRequirements(String value) { 2136 if (Utilities.noString(value)) 2137 this.requirements = null; 2138 else { 2139 if (this.requirements == null) 2140 this.requirements = new StringType(); 2141 this.requirements.setValue(value); 2142 } 2143 return this; 2144 } 2145 2146 /** 2147 * @return {@link #copyright} (A copyright statement relating to the concept map 2148 * and/or its contents.). This is the underlying object with id, value 2149 * and extensions. The accessor "getCopyright" gives direct access to 2150 * the value 2151 */ 2152 public StringType getCopyrightElement() { 2153 if (this.copyright == null) 2154 if (Configuration.errorOnAutoCreate()) 2155 throw new Error("Attempt to auto-create ConceptMap.copyright"); 2156 else if (Configuration.doAutoCreate()) 2157 this.copyright = new StringType(); // bb 2158 return this.copyright; 2159 } 2160 2161 public boolean hasCopyrightElement() { 2162 return this.copyright != null && !this.copyright.isEmpty(); 2163 } 2164 2165 public boolean hasCopyright() { 2166 return this.copyright != null && !this.copyright.isEmpty(); 2167 } 2168 2169 /** 2170 * @param value {@link #copyright} (A copyright statement relating to the 2171 * concept map and/or its contents.). This is the underlying object 2172 * with id, value and extensions. The accessor "getCopyright" gives 2173 * direct access to the value 2174 */ 2175 public ConceptMap setCopyrightElement(StringType value) { 2176 this.copyright = value; 2177 return this; 2178 } 2179 2180 /** 2181 * @return A copyright statement relating to the concept map and/or its 2182 * contents. 2183 */ 2184 public String getCopyright() { 2185 return this.copyright == null ? null : this.copyright.getValue(); 2186 } 2187 2188 /** 2189 * @param value A copyright statement relating to the concept map and/or its 2190 * contents. 2191 */ 2192 public ConceptMap setCopyright(String value) { 2193 if (Utilities.noString(value)) 2194 this.copyright = null; 2195 else { 2196 if (this.copyright == null) 2197 this.copyright = new StringType(); 2198 this.copyright.setValue(value); 2199 } 2200 return this; 2201 } 2202 2203 /** 2204 * @return {@link #source} (The source value set that specifies the concepts 2205 * that are being mapped.) 2206 */ 2207 public Type getSource() { 2208 return this.source; 2209 } 2210 2211 /** 2212 * @return {@link #source} (The source value set that specifies the concepts 2213 * that are being mapped.) 2214 */ 2215 public UriType getSourceUriType() throws FHIRException { 2216 if (!(this.source instanceof UriType)) 2217 throw new FHIRException( 2218 "Type mismatch: the type UriType was expected, but " + this.source.getClass().getName() + " was encountered"); 2219 return (UriType) this.source; 2220 } 2221 2222 public boolean hasSourceUriType() { 2223 return this.source instanceof UriType; 2224 } 2225 2226 /** 2227 * @return {@link #source} (The source value set that specifies the concepts 2228 * that are being mapped.) 2229 */ 2230 public Reference getSourceReference() throws FHIRException { 2231 if (!(this.source instanceof Reference)) 2232 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.source.getClass().getName() 2233 + " was encountered"); 2234 return (Reference) this.source; 2235 } 2236 2237 public boolean hasSourceReference() { 2238 return this.source instanceof Reference; 2239 } 2240 2241 public boolean hasSource() { 2242 return this.source != null && !this.source.isEmpty(); 2243 } 2244 2245 /** 2246 * @param value {@link #source} (The source value set that specifies the 2247 * concepts that are being mapped.) 2248 */ 2249 public ConceptMap setSource(Type value) { 2250 this.source = value; 2251 return this; 2252 } 2253 2254 /** 2255 * @return {@link #target} (The target value set provides context to the 2256 * mappings. Note that the mapping is made between concepts, not between 2257 * value sets, but the value set provides important context about how 2258 * the concept mapping choices are made.) 2259 */ 2260 public Type getTarget() { 2261 return this.target; 2262 } 2263 2264 /** 2265 * @return {@link #target} (The target value set provides context to the 2266 * mappings. Note that the mapping is made between concepts, not between 2267 * value sets, but the value set provides important context about how 2268 * the concept mapping choices are made.) 2269 */ 2270 public UriType getTargetUriType() throws FHIRException { 2271 if (!(this.target instanceof UriType)) 2272 throw new FHIRException( 2273 "Type mismatch: the type UriType was expected, but " + this.target.getClass().getName() + " was encountered"); 2274 return (UriType) this.target; 2275 } 2276 2277 public boolean hasTargetUriType() { 2278 return this.target instanceof UriType; 2279 } 2280 2281 /** 2282 * @return {@link #target} (The target value set provides context to the 2283 * mappings. Note that the mapping is made between concepts, not between 2284 * value sets, but the value set provides important context about how 2285 * the concept mapping choices are made.) 2286 */ 2287 public Reference getTargetReference() throws FHIRException { 2288 if (!(this.target instanceof Reference)) 2289 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.target.getClass().getName() 2290 + " was encountered"); 2291 return (Reference) this.target; 2292 } 2293 2294 public boolean hasTargetReference() { 2295 return this.target instanceof Reference; 2296 } 2297 2298 public boolean hasTarget() { 2299 return this.target != null && !this.target.isEmpty(); 2300 } 2301 2302 /** 2303 * @param value {@link #target} (The target value set provides context to the 2304 * mappings. Note that the mapping is made between concepts, not 2305 * between value sets, but the value set provides important context 2306 * about how the concept mapping choices are made.) 2307 */ 2308 public ConceptMap setTarget(Type value) { 2309 this.target = value; 2310 return this; 2311 } 2312 2313 /** 2314 * @return {@link #element} (Mappings for an individual concept in the source to 2315 * one or more concepts in the target.) 2316 */ 2317 public List<SourceElementComponent> getElement() { 2318 if (this.element == null) 2319 this.element = new ArrayList<SourceElementComponent>(); 2320 return this.element; 2321 } 2322 2323 public boolean hasElement() { 2324 if (this.element == null) 2325 return false; 2326 for (SourceElementComponent item : this.element) 2327 if (!item.isEmpty()) 2328 return true; 2329 return false; 2330 } 2331 2332 /** 2333 * @return {@link #element} (Mappings for an individual concept in the source to 2334 * one or more concepts in the target.) 2335 */ 2336 // syntactic sugar 2337 public SourceElementComponent addElement() { // 3 2338 SourceElementComponent t = new SourceElementComponent(); 2339 if (this.element == null) 2340 this.element = new ArrayList<SourceElementComponent>(); 2341 this.element.add(t); 2342 return t; 2343 } 2344 2345 // syntactic sugar 2346 public ConceptMap addElement(SourceElementComponent t) { // 3 2347 if (t == null) 2348 return this; 2349 if (this.element == null) 2350 this.element = new ArrayList<SourceElementComponent>(); 2351 this.element.add(t); 2352 return this; 2353 } 2354 2355 protected void listChildren(List<Property> childrenList) { 2356 super.listChildren(childrenList); 2357 childrenList.add(new Property("url", "uri", 2358 "An absolute URL that is used to identify this concept map when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this concept map is (or will be) published.", 2359 0, java.lang.Integer.MAX_VALUE, url)); 2360 childrenList.add(new Property("identifier", "Identifier", 2361 "Formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 2362 0, java.lang.Integer.MAX_VALUE, identifier)); 2363 childrenList.add(new Property("version", "string", 2364 "The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the profile author manually and the value should be a timestamp.", 2365 0, java.lang.Integer.MAX_VALUE, version)); 2366 childrenList.add(new Property("name", "string", "A free text natural language name describing the concept map.", 0, 2367 java.lang.Integer.MAX_VALUE, name)); 2368 childrenList 2369 .add(new Property("status", "code", "The status of the concept map.", 0, java.lang.Integer.MAX_VALUE, status)); 2370 childrenList.add(new Property("experimental", "boolean", 2371 "This ConceptMap was authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 2372 0, java.lang.Integer.MAX_VALUE, experimental)); 2373 childrenList.add(new Property("publisher", "string", 2374 "The name of the individual or organization that published the concept map.", 0, java.lang.Integer.MAX_VALUE, 2375 publisher)); 2376 childrenList 2377 .add(new Property("contact", "", "Contacts to assist a user in finding and communicating with the publisher.", 2378 0, java.lang.Integer.MAX_VALUE, contact)); 2379 childrenList.add(new Property("date", "dateTime", 2380 "The date this version of the concept map was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes.", 2381 0, java.lang.Integer.MAX_VALUE, date)); 2382 childrenList.add(new Property("description", "string", 2383 "A free text natural language description of the use of the concept map - reason for definition, conditions of use, etc.", 2384 0, java.lang.Integer.MAX_VALUE, description)); 2385 childrenList.add(new Property("useContext", "CodeableConcept", 2386 "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of concept map instances.", 2387 0, java.lang.Integer.MAX_VALUE, useContext)); 2388 childrenList.add(new Property("requirements", "string", 2389 "Explains why this concept map is needed and why it has been constrained as it has.", 0, 2390 java.lang.Integer.MAX_VALUE, requirements)); 2391 childrenList.add( 2392 new Property("copyright", "string", "A copyright statement relating to the concept map and/or its contents.", 0, 2393 java.lang.Integer.MAX_VALUE, copyright)); 2394 childrenList.add(new Property("source[x]", "uri|Reference(ValueSet|StructureDefinition)", 2395 "The source value set that specifies the concepts that are being mapped.", 0, java.lang.Integer.MAX_VALUE, 2396 source)); 2397 childrenList.add(new Property("target[x]", "uri|Reference(ValueSet|StructureDefinition)", 2398 "The target value set provides context to the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.", 2399 0, java.lang.Integer.MAX_VALUE, target)); 2400 childrenList.add(new Property("element", "", 2401 "Mappings for an individual concept in the source to one or more concepts in the target.", 0, 2402 java.lang.Integer.MAX_VALUE, element)); 2403 } 2404 2405 @Override 2406 public void setProperty(String name, Base value) throws FHIRException { 2407 if (name.equals("url")) 2408 this.url = castToUri(value); // UriType 2409 else if (name.equals("identifier")) 2410 this.identifier = castToIdentifier(value); // Identifier 2411 else if (name.equals("version")) 2412 this.version = castToString(value); // StringType 2413 else if (name.equals("name")) 2414 this.name = castToString(value); // StringType 2415 else if (name.equals("status")) 2416 this.status = new ConformanceResourceStatusEnumFactory().fromType(value); // Enumeration<ConformanceResourceStatus> 2417 else if (name.equals("experimental")) 2418 this.experimental = castToBoolean(value); // BooleanType 2419 else if (name.equals("publisher")) 2420 this.publisher = castToString(value); // StringType 2421 else if (name.equals("contact")) 2422 this.getContact().add((ConceptMapContactComponent) value); 2423 else if (name.equals("date")) 2424 this.date = castToDateTime(value); // DateTimeType 2425 else if (name.equals("description")) 2426 this.description = castToString(value); // StringType 2427 else if (name.equals("useContext")) 2428 this.getUseContext().add(castToCodeableConcept(value)); 2429 else if (name.equals("requirements")) 2430 this.requirements = castToString(value); // StringType 2431 else if (name.equals("copyright")) 2432 this.copyright = castToString(value); // StringType 2433 else if (name.equals("source[x]")) 2434 this.source = (Type) value; // Type 2435 else if (name.equals("target[x]")) 2436 this.target = (Type) value; // Type 2437 else if (name.equals("element")) 2438 this.getElement().add((SourceElementComponent) value); 2439 else 2440 super.setProperty(name, value); 2441 } 2442 2443 @Override 2444 public Base addChild(String name) throws FHIRException { 2445 if (name.equals("url")) { 2446 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.url"); 2447 } else if (name.equals("identifier")) { 2448 this.identifier = new Identifier(); 2449 return this.identifier; 2450 } else if (name.equals("version")) { 2451 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.version"); 2452 } else if (name.equals("name")) { 2453 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.name"); 2454 } else if (name.equals("status")) { 2455 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.status"); 2456 } else if (name.equals("experimental")) { 2457 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.experimental"); 2458 } else if (name.equals("publisher")) { 2459 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.publisher"); 2460 } else if (name.equals("contact")) { 2461 return addContact(); 2462 } else if (name.equals("date")) { 2463 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.date"); 2464 } else if (name.equals("description")) { 2465 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.description"); 2466 } else if (name.equals("useContext")) { 2467 return addUseContext(); 2468 } else if (name.equals("requirements")) { 2469 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.requirements"); 2470 } else if (name.equals("copyright")) { 2471 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.copyright"); 2472 } else if (name.equals("sourceUri")) { 2473 this.source = new UriType(); 2474 return this.source; 2475 } else if (name.equals("sourceReference")) { 2476 this.source = new Reference(); 2477 return this.source; 2478 } else if (name.equals("targetUri")) { 2479 this.target = new UriType(); 2480 return this.target; 2481 } else if (name.equals("targetReference")) { 2482 this.target = new Reference(); 2483 return this.target; 2484 } else if (name.equals("element")) { 2485 return addElement(); 2486 } else 2487 return super.addChild(name); 2488 } 2489 2490 public String fhirType() { 2491 return "ConceptMap"; 2492 2493 } 2494 2495 public ConceptMap copy() { 2496 ConceptMap dst = new ConceptMap(); 2497 copyValues(dst); 2498 dst.url = url == null ? null : url.copy(); 2499 dst.identifier = identifier == null ? null : identifier.copy(); 2500 dst.version = version == null ? null : version.copy(); 2501 dst.name = name == null ? null : name.copy(); 2502 dst.status = status == null ? null : status.copy(); 2503 dst.experimental = experimental == null ? null : experimental.copy(); 2504 dst.publisher = publisher == null ? null : publisher.copy(); 2505 if (contact != null) { 2506 dst.contact = new ArrayList<ConceptMapContactComponent>(); 2507 for (ConceptMapContactComponent i : contact) 2508 dst.contact.add(i.copy()); 2509 } 2510 ; 2511 dst.date = date == null ? null : date.copy(); 2512 dst.description = description == null ? null : description.copy(); 2513 if (useContext != null) { 2514 dst.useContext = new ArrayList<CodeableConcept>(); 2515 for (CodeableConcept i : useContext) 2516 dst.useContext.add(i.copy()); 2517 } 2518 ; 2519 dst.requirements = requirements == null ? null : requirements.copy(); 2520 dst.copyright = copyright == null ? null : copyright.copy(); 2521 dst.source = source == null ? null : source.copy(); 2522 dst.target = target == null ? null : target.copy(); 2523 if (element != null) { 2524 dst.element = new ArrayList<SourceElementComponent>(); 2525 for (SourceElementComponent i : element) 2526 dst.element.add(i.copy()); 2527 } 2528 ; 2529 return dst; 2530 } 2531 2532 protected ConceptMap typedCopy() { 2533 return copy(); 2534 } 2535 2536 @Override 2537 public boolean equalsDeep(Base other) { 2538 if (!super.equalsDeep(other)) 2539 return false; 2540 if (!(other instanceof ConceptMap)) 2541 return false; 2542 ConceptMap o = (ConceptMap) other; 2543 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) 2544 && compareDeep(version, o.version, true) && compareDeep(name, o.name, true) 2545 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 2546 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 2547 && compareDeep(date, o.date, true) && compareDeep(description, o.description, true) 2548 && compareDeep(useContext, o.useContext, true) && compareDeep(requirements, o.requirements, true) 2549 && compareDeep(copyright, o.copyright, true) && compareDeep(source, o.source, true) 2550 && compareDeep(target, o.target, true) && compareDeep(element, o.element, true); 2551 } 2552 2553 @Override 2554 public boolean equalsShallow(Base other) { 2555 if (!super.equalsShallow(other)) 2556 return false; 2557 if (!(other instanceof ConceptMap)) 2558 return false; 2559 ConceptMap o = (ConceptMap) other; 2560 return compareValues(url, o.url, true) && compareValues(version, o.version, true) 2561 && compareValues(name, o.name, true) && compareValues(status, o.status, true) 2562 && compareValues(experimental, o.experimental, true) && compareValues(publisher, o.publisher, true) 2563 && compareValues(date, o.date, true) && compareValues(description, o.description, true) 2564 && compareValues(requirements, o.requirements, true) && compareValues(copyright, o.copyright, true); 2565 } 2566 2567 public boolean isEmpty() { 2568 return super.isEmpty() && (url == null || url.isEmpty()) && (identifier == null || identifier.isEmpty()) 2569 && (version == null || version.isEmpty()) && (name == null || name.isEmpty()) 2570 && (status == null || status.isEmpty()) && (experimental == null || experimental.isEmpty()) 2571 && (publisher == null || publisher.isEmpty()) && (contact == null || contact.isEmpty()) 2572 && (date == null || date.isEmpty()) && (description == null || description.isEmpty()) 2573 && (useContext == null || useContext.isEmpty()) && (requirements == null || requirements.isEmpty()) 2574 && (copyright == null || copyright.isEmpty()) && (source == null || source.isEmpty()) 2575 && (target == null || target.isEmpty()) && (element == null || element.isEmpty()); 2576 } 2577 2578 @Override 2579 public ResourceType getResourceType() { 2580 return ResourceType.ConceptMap; 2581 } 2582 2583 @SearchParamDefinition(name = "date", path = "ConceptMap.date", description = "The concept map publication date", type = "date") 2584 public static final String SP_DATE = "date"; 2585 @SearchParamDefinition(name = "identifier", path = "ConceptMap.identifier", description = "Additional identifier for the concept map", type = "token") 2586 public static final String SP_IDENTIFIER = "identifier"; 2587 @SearchParamDefinition(name = "product", path = "ConceptMap.element.target.product.element", description = "Reference to element/field/ValueSet mapping depends on", type = "uri") 2588 public static final String SP_PRODUCT = "product"; 2589 @SearchParamDefinition(name = "dependson", path = "ConceptMap.element.target.dependsOn.element", description = "Reference to element/field/ValueSet mapping depends on", type = "uri") 2590 public static final String SP_DEPENDSON = "dependson"; 2591 @SearchParamDefinition(name = "description", path = "ConceptMap.description", description = "Text search in the description of the concept map", type = "string") 2592 public static final String SP_DESCRIPTION = "description"; 2593 @SearchParamDefinition(name = "targetsystem", path = "ConceptMap.element.target.codeSystem", description = "System of the target (if necessary)", type = "uri") 2594 public static final String SP_TARGETSYSTEM = "targetsystem"; 2595 @SearchParamDefinition(name = "source", path = "ConceptMap.sourceReference", description = "Identifies the source of the concepts which are being mapped", type = "reference") 2596 public static final String SP_SOURCE = "source"; 2597 @SearchParamDefinition(name = "version", path = "ConceptMap.version", description = "The version identifier of the concept map", type = "token") 2598 public static final String SP_VERSION = "version"; 2599 @SearchParamDefinition(name = "sourcesystem", path = "ConceptMap.element.codeSystem", description = "Code System (if value set crosses code systems)", type = "uri") 2600 public static final String SP_SOURCESYSTEM = "sourcesystem"; 2601 @SearchParamDefinition(name = "url", path = "ConceptMap.url", description = "The URL of the concept map", type = "uri") 2602 public static final String SP_URL = "url"; 2603 @SearchParamDefinition(name = "target", path = "ConceptMap.target[x]", description = "Provides context to the mappings", type = "reference") 2604 public static final String SP_TARGET = "target"; 2605 @SearchParamDefinition(name = "sourcecode", path = "ConceptMap.element.code", description = "Identifies element being mapped", type = "token") 2606 public static final String SP_SOURCECODE = "sourcecode"; 2607 @SearchParamDefinition(name = "sourceuri", path = "ConceptMap.sourceUri", description = "Identifies the source of the concepts which are being mapped", type = "reference") 2608 public static final String SP_SOURCEURI = "sourceuri"; 2609 @SearchParamDefinition(name = "name", path = "ConceptMap.name", description = "Name of the concept map", type = "string") 2610 public static final String SP_NAME = "name"; 2611 @SearchParamDefinition(name = "context", path = "ConceptMap.useContext", description = "A use context assigned to the concept map", type = "token") 2612 public static final String SP_CONTEXT = "context"; 2613 @SearchParamDefinition(name = "publisher", path = "ConceptMap.publisher", description = "Name of the publisher of the concept map", type = "string") 2614 public static final String SP_PUBLISHER = "publisher"; 2615 @SearchParamDefinition(name = "targetcode", path = "ConceptMap.element.target.code", description = "Code that identifies the target element", type = "token") 2616 public static final String SP_TARGETCODE = "targetcode"; 2617 @SearchParamDefinition(name = "status", path = "ConceptMap.status", description = "Status of the concept map", type = "token") 2618 public static final String SP_STATUS = "status"; 2619 2620}