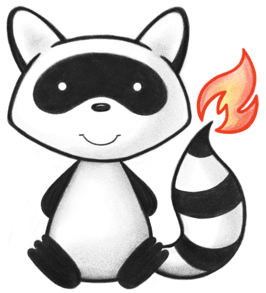
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * Use to record detailed information about conditions, problems or diagnoses 048 * recognized by a clinician. There are many uses including: recording a 049 * diagnosis during an encounter; populating a problem list or a summary 050 * statement, such as a discharge summary. 051 */ 052@ResourceDef(name = "Condition", profile = "http://hl7.org/fhir/Profile/Condition") 053public class Condition extends DomainResource { 054 055 public enum ConditionVerificationStatus { 056 /** 057 * This is a tentative diagnosis - still a candidate that is under 058 * consideration. 059 */ 060 PROVISIONAL, 061 /** 062 * One of a set of potential (and typically mutually exclusive) diagnosis 063 * asserted to further guide the diagnostic process and preliminary treatment. 064 */ 065 DIFFERENTIAL, 066 /** 067 * There is sufficient diagnostic and/or clinical evidence to treat this as a 068 * confirmed condition. 069 */ 070 CONFIRMED, 071 /** 072 * This condition has been ruled out by diagnostic and clinical evidence. 073 */ 074 REFUTED, 075 /** 076 * The statement was entered in error and is not valid. 077 */ 078 ENTEREDINERROR, 079 /** 080 * The condition status is unknown. Note that "unknown" is a value of last 081 * resort and every attempt should be made to provide a meaningful value other 082 * than "unknown". 083 */ 084 UNKNOWN, 085 /** 086 * added to help the parsers 087 */ 088 NULL; 089 090 public static ConditionVerificationStatus fromCode(String codeString) throws FHIRException { 091 if (codeString == null || "".equals(codeString)) 092 return null; 093 if ("provisional".equals(codeString)) 094 return PROVISIONAL; 095 if ("differential".equals(codeString)) 096 return DIFFERENTIAL; 097 if ("confirmed".equals(codeString)) 098 return CONFIRMED; 099 if ("refuted".equals(codeString)) 100 return REFUTED; 101 if ("entered-in-error".equals(codeString)) 102 return ENTEREDINERROR; 103 if ("unknown".equals(codeString)) 104 return UNKNOWN; 105 throw new FHIRException("Unknown ConditionVerificationStatus code '" + codeString + "'"); 106 } 107 108 public String toCode() { 109 switch (this) { 110 case PROVISIONAL: 111 return "provisional"; 112 case DIFFERENTIAL: 113 return "differential"; 114 case CONFIRMED: 115 return "confirmed"; 116 case REFUTED: 117 return "refuted"; 118 case ENTEREDINERROR: 119 return "entered-in-error"; 120 case UNKNOWN: 121 return "unknown"; 122 case NULL: 123 return null; 124 default: 125 return "?"; 126 } 127 } 128 129 public String getSystem() { 130 switch (this) { 131 case PROVISIONAL: 132 return "http://hl7.org/fhir/condition-ver-status"; 133 case DIFFERENTIAL: 134 return "http://hl7.org/fhir/condition-ver-status"; 135 case CONFIRMED: 136 return "http://hl7.org/fhir/condition-ver-status"; 137 case REFUTED: 138 return "http://hl7.org/fhir/condition-ver-status"; 139 case ENTEREDINERROR: 140 return "http://hl7.org/fhir/condition-ver-status"; 141 case UNKNOWN: 142 return "http://hl7.org/fhir/condition-ver-status"; 143 case NULL: 144 return null; 145 default: 146 return "?"; 147 } 148 } 149 150 public String getDefinition() { 151 switch (this) { 152 case PROVISIONAL: 153 return "This is a tentative diagnosis - still a candidate that is under consideration."; 154 case DIFFERENTIAL: 155 return "One of a set of potential (and typically mutually exclusive) diagnosis asserted to further guide the diagnostic process and preliminary treatment."; 156 case CONFIRMED: 157 return "There is sufficient diagnostic and/or clinical evidence to treat this as a confirmed condition."; 158 case REFUTED: 159 return "This condition has been ruled out by diagnostic and clinical evidence."; 160 case ENTEREDINERROR: 161 return "The statement was entered in error and is not valid."; 162 case UNKNOWN: 163 return "The condition status is unknown. Note that \"unknown\" is a value of last resort and every attempt should be made to provide a meaningful value other than \"unknown\"."; 164 case NULL: 165 return null; 166 default: 167 return "?"; 168 } 169 } 170 171 public String getDisplay() { 172 switch (this) { 173 case PROVISIONAL: 174 return "Provisional"; 175 case DIFFERENTIAL: 176 return "Differential"; 177 case CONFIRMED: 178 return "Confirmed"; 179 case REFUTED: 180 return "Refuted"; 181 case ENTEREDINERROR: 182 return "Entered In Error"; 183 case UNKNOWN: 184 return "Unknown"; 185 case NULL: 186 return null; 187 default: 188 return "?"; 189 } 190 } 191 } 192 193 public static class ConditionVerificationStatusEnumFactory implements EnumFactory<ConditionVerificationStatus> { 194 public ConditionVerificationStatus fromCode(String codeString) throws IllegalArgumentException { 195 if (codeString == null || "".equals(codeString)) 196 if (codeString == null || "".equals(codeString)) 197 return null; 198 if ("provisional".equals(codeString)) 199 return ConditionVerificationStatus.PROVISIONAL; 200 if ("differential".equals(codeString)) 201 return ConditionVerificationStatus.DIFFERENTIAL; 202 if ("confirmed".equals(codeString)) 203 return ConditionVerificationStatus.CONFIRMED; 204 if ("refuted".equals(codeString)) 205 return ConditionVerificationStatus.REFUTED; 206 if ("entered-in-error".equals(codeString)) 207 return ConditionVerificationStatus.ENTEREDINERROR; 208 if ("unknown".equals(codeString)) 209 return ConditionVerificationStatus.UNKNOWN; 210 throw new IllegalArgumentException("Unknown ConditionVerificationStatus code '" + codeString + "'"); 211 } 212 213 public Enumeration<ConditionVerificationStatus> fromType(Base code) throws FHIRException { 214 if (code == null || code.isEmpty()) 215 return null; 216 String codeString = ((PrimitiveType) code).asStringValue(); 217 if (codeString == null || "".equals(codeString)) 218 return null; 219 if ("provisional".equals(codeString)) 220 return new Enumeration<ConditionVerificationStatus>(this, ConditionVerificationStatus.PROVISIONAL); 221 if ("differential".equals(codeString)) 222 return new Enumeration<ConditionVerificationStatus>(this, ConditionVerificationStatus.DIFFERENTIAL); 223 if ("confirmed".equals(codeString)) 224 return new Enumeration<ConditionVerificationStatus>(this, ConditionVerificationStatus.CONFIRMED); 225 if ("refuted".equals(codeString)) 226 return new Enumeration<ConditionVerificationStatus>(this, ConditionVerificationStatus.REFUTED); 227 if ("entered-in-error".equals(codeString)) 228 return new Enumeration<ConditionVerificationStatus>(this, ConditionVerificationStatus.ENTEREDINERROR); 229 if ("unknown".equals(codeString)) 230 return new Enumeration<ConditionVerificationStatus>(this, ConditionVerificationStatus.UNKNOWN); 231 throw new FHIRException("Unknown ConditionVerificationStatus code '" + codeString + "'"); 232 } 233 234 public String toCode(ConditionVerificationStatus code) 235 { 236 if (code == ConditionVerificationStatus.NULL) 237 return null; 238 if (code == ConditionVerificationStatus.PROVISIONAL) 239 return "provisional"; 240 if (code == ConditionVerificationStatus.DIFFERENTIAL) 241 return "differential"; 242 if (code == ConditionVerificationStatus.CONFIRMED) 243 return "confirmed"; 244 if (code == ConditionVerificationStatus.REFUTED) 245 return "refuted"; 246 if (code == ConditionVerificationStatus.ENTEREDINERROR) 247 return "entered-in-error"; 248 if (code == ConditionVerificationStatus.UNKNOWN) 249 return "unknown"; 250 return "?"; 251 } 252 } 253 254 @Block() 255 public static class ConditionStageComponent extends BackboneElement implements IBaseBackboneElement { 256 /** 257 * A simple summary of the stage such as "Stage 3". The determination of the 258 * stage is disease-specific. 259 */ 260 @Child(name = "summary", type = { 261 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 262 @Description(shortDefinition = "Simple summary (disease specific)", formalDefinition = "A simple summary of the stage such as \"Stage 3\". The determination of the stage is disease-specific.") 263 protected CodeableConcept summary; 264 265 /** 266 * Reference to a formal record of the evidence on which the staging assessment 267 * is based. 268 */ 269 @Child(name = "assessment", type = { ClinicalImpression.class, DiagnosticReport.class, 270 Observation.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 271 @Description(shortDefinition = "Formal record of assessment", formalDefinition = "Reference to a formal record of the evidence on which the staging assessment is based.") 272 protected List<Reference> assessment; 273 /** 274 * The actual objects that are the target of the reference (Reference to a 275 * formal record of the evidence on which the staging assessment is based.) 276 */ 277 protected List<Resource> assessmentTarget; 278 279 private static final long serialVersionUID = -1961530405L; 280 281 /* 282 * Constructor 283 */ 284 public ConditionStageComponent() { 285 super(); 286 } 287 288 /** 289 * @return {@link #summary} (A simple summary of the stage such as "Stage 3". 290 * The determination of the stage is disease-specific.) 291 */ 292 public CodeableConcept getSummary() { 293 if (this.summary == null) 294 if (Configuration.errorOnAutoCreate()) 295 throw new Error("Attempt to auto-create ConditionStageComponent.summary"); 296 else if (Configuration.doAutoCreate()) 297 this.summary = new CodeableConcept(); // cc 298 return this.summary; 299 } 300 301 public boolean hasSummary() { 302 return this.summary != null && !this.summary.isEmpty(); 303 } 304 305 /** 306 * @param value {@link #summary} (A simple summary of the stage such as "Stage 307 * 3". The determination of the stage is disease-specific.) 308 */ 309 public ConditionStageComponent setSummary(CodeableConcept value) { 310 this.summary = value; 311 return this; 312 } 313 314 /** 315 * @return {@link #assessment} (Reference to a formal record of the evidence on 316 * which the staging assessment is based.) 317 */ 318 public List<Reference> getAssessment() { 319 if (this.assessment == null) 320 this.assessment = new ArrayList<Reference>(); 321 return this.assessment; 322 } 323 324 public boolean hasAssessment() { 325 if (this.assessment == null) 326 return false; 327 for (Reference item : this.assessment) 328 if (!item.isEmpty()) 329 return true; 330 return false; 331 } 332 333 /** 334 * @return {@link #assessment} (Reference to a formal record of the evidence on 335 * which the staging assessment is based.) 336 */ 337 // syntactic sugar 338 public Reference addAssessment() { // 3 339 Reference t = new Reference(); 340 if (this.assessment == null) 341 this.assessment = new ArrayList<Reference>(); 342 this.assessment.add(t); 343 return t; 344 } 345 346 // syntactic sugar 347 public ConditionStageComponent addAssessment(Reference t) { // 3 348 if (t == null) 349 return this; 350 if (this.assessment == null) 351 this.assessment = new ArrayList<Reference>(); 352 this.assessment.add(t); 353 return this; 354 } 355 356 /** 357 * @return {@link #assessment} (The actual objects that are the target of the 358 * reference. The reference library doesn't populate this, but you can 359 * use this to hold the resources if you resolvethemt. Reference to a 360 * formal record of the evidence on which the staging assessment is 361 * based.) 362 */ 363 public List<Resource> getAssessmentTarget() { 364 if (this.assessmentTarget == null) 365 this.assessmentTarget = new ArrayList<Resource>(); 366 return this.assessmentTarget; 367 } 368 369 protected void listChildren(List<Property> childrenList) { 370 super.listChildren(childrenList); 371 childrenList.add(new Property("summary", "CodeableConcept", 372 "A simple summary of the stage such as \"Stage 3\". The determination of the stage is disease-specific.", 0, 373 java.lang.Integer.MAX_VALUE, summary)); 374 childrenList.add(new Property("assessment", "Reference(ClinicalImpression|DiagnosticReport|Observation)", 375 "Reference to a formal record of the evidence on which the staging assessment is based.", 0, 376 java.lang.Integer.MAX_VALUE, assessment)); 377 } 378 379 @Override 380 public void setProperty(String name, Base value) throws FHIRException { 381 if (name.equals("summary")) 382 this.summary = castToCodeableConcept(value); // CodeableConcept 383 else if (name.equals("assessment")) 384 this.getAssessment().add(castToReference(value)); 385 else 386 super.setProperty(name, value); 387 } 388 389 @Override 390 public Base addChild(String name) throws FHIRException { 391 if (name.equals("summary")) { 392 this.summary = new CodeableConcept(); 393 return this.summary; 394 } else if (name.equals("assessment")) { 395 return addAssessment(); 396 } else 397 return super.addChild(name); 398 } 399 400 public ConditionStageComponent copy() { 401 ConditionStageComponent dst = new ConditionStageComponent(); 402 copyValues(dst); 403 dst.summary = summary == null ? null : summary.copy(); 404 if (assessment != null) { 405 dst.assessment = new ArrayList<Reference>(); 406 for (Reference i : assessment) 407 dst.assessment.add(i.copy()); 408 } 409 ; 410 return dst; 411 } 412 413 @Override 414 public boolean equalsDeep(Base other) { 415 if (!super.equalsDeep(other)) 416 return false; 417 if (!(other instanceof ConditionStageComponent)) 418 return false; 419 ConditionStageComponent o = (ConditionStageComponent) other; 420 return compareDeep(summary, o.summary, true) && compareDeep(assessment, o.assessment, true); 421 } 422 423 @Override 424 public boolean equalsShallow(Base other) { 425 if (!super.equalsShallow(other)) 426 return false; 427 if (!(other instanceof ConditionStageComponent)) 428 return false; 429 ConditionStageComponent o = (ConditionStageComponent) other; 430 return true; 431 } 432 433 public boolean isEmpty() { 434 return super.isEmpty() && (summary == null || summary.isEmpty()) && (assessment == null || assessment.isEmpty()); 435 } 436 437 public String fhirType() { 438 return "Condition.stage"; 439 440 } 441 442 } 443 444 @Block() 445 public static class ConditionEvidenceComponent extends BackboneElement implements IBaseBackboneElement { 446 /** 447 * A manifestation or symptom that led to the recording of this condition. 448 */ 449 @Child(name = "code", type = { 450 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 451 @Description(shortDefinition = "Manifestation/symptom", formalDefinition = "A manifestation or symptom that led to the recording of this condition.") 452 protected CodeableConcept code; 453 454 /** 455 * Links to other relevant information, including pathology reports. 456 */ 457 @Child(name = "detail", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 458 @Description(shortDefinition = "Supporting information found elsewhere", formalDefinition = "Links to other relevant information, including pathology reports.") 459 protected List<Reference> detail; 460 /** 461 * The actual objects that are the target of the reference (Links to other 462 * relevant information, including pathology reports.) 463 */ 464 protected List<Resource> detailTarget; 465 466 private static final long serialVersionUID = 945689926L; 467 468 /* 469 * Constructor 470 */ 471 public ConditionEvidenceComponent() { 472 super(); 473 } 474 475 /** 476 * @return {@link #code} (A manifestation or symptom that led to the recording 477 * of this condition.) 478 */ 479 public CodeableConcept getCode() { 480 if (this.code == null) 481 if (Configuration.errorOnAutoCreate()) 482 throw new Error("Attempt to auto-create ConditionEvidenceComponent.code"); 483 else if (Configuration.doAutoCreate()) 484 this.code = new CodeableConcept(); // cc 485 return this.code; 486 } 487 488 public boolean hasCode() { 489 return this.code != null && !this.code.isEmpty(); 490 } 491 492 /** 493 * @param value {@link #code} (A manifestation or symptom that led to the 494 * recording of this condition.) 495 */ 496 public ConditionEvidenceComponent setCode(CodeableConcept value) { 497 this.code = value; 498 return this; 499 } 500 501 /** 502 * @return {@link #detail} (Links to other relevant information, including 503 * pathology reports.) 504 */ 505 public List<Reference> getDetail() { 506 if (this.detail == null) 507 this.detail = new ArrayList<Reference>(); 508 return this.detail; 509 } 510 511 public boolean hasDetail() { 512 if (this.detail == null) 513 return false; 514 for (Reference item : this.detail) 515 if (!item.isEmpty()) 516 return true; 517 return false; 518 } 519 520 /** 521 * @return {@link #detail} (Links to other relevant information, including 522 * pathology reports.) 523 */ 524 // syntactic sugar 525 public Reference addDetail() { // 3 526 Reference t = new Reference(); 527 if (this.detail == null) 528 this.detail = new ArrayList<Reference>(); 529 this.detail.add(t); 530 return t; 531 } 532 533 // syntactic sugar 534 public ConditionEvidenceComponent addDetail(Reference t) { // 3 535 if (t == null) 536 return this; 537 if (this.detail == null) 538 this.detail = new ArrayList<Reference>(); 539 this.detail.add(t); 540 return this; 541 } 542 543 /** 544 * @return {@link #detail} (The actual objects that are the target of the 545 * reference. The reference library doesn't populate this, but you can 546 * use this to hold the resources if you resolvethemt. Links to other 547 * relevant information, including pathology reports.) 548 */ 549 public List<Resource> getDetailTarget() { 550 if (this.detailTarget == null) 551 this.detailTarget = new ArrayList<Resource>(); 552 return this.detailTarget; 553 } 554 555 protected void listChildren(List<Property> childrenList) { 556 super.listChildren(childrenList); 557 childrenList.add(new Property("code", "CodeableConcept", 558 "A manifestation or symptom that led to the recording of this condition.", 0, java.lang.Integer.MAX_VALUE, 559 code)); 560 childrenList.add(new Property("detail", "Reference(Any)", 561 "Links to other relevant information, including pathology reports.", 0, java.lang.Integer.MAX_VALUE, detail)); 562 } 563 564 @Override 565 public void setProperty(String name, Base value) throws FHIRException { 566 if (name.equals("code")) 567 this.code = castToCodeableConcept(value); // CodeableConcept 568 else if (name.equals("detail")) 569 this.getDetail().add(castToReference(value)); 570 else 571 super.setProperty(name, value); 572 } 573 574 @Override 575 public Base addChild(String name) throws FHIRException { 576 if (name.equals("code")) { 577 this.code = new CodeableConcept(); 578 return this.code; 579 } else if (name.equals("detail")) { 580 return addDetail(); 581 } else 582 return super.addChild(name); 583 } 584 585 public ConditionEvidenceComponent copy() { 586 ConditionEvidenceComponent dst = new ConditionEvidenceComponent(); 587 copyValues(dst); 588 dst.code = code == null ? null : code.copy(); 589 if (detail != null) { 590 dst.detail = new ArrayList<Reference>(); 591 for (Reference i : detail) 592 dst.detail.add(i.copy()); 593 } 594 ; 595 return dst; 596 } 597 598 @Override 599 public boolean equalsDeep(Base other) { 600 if (!super.equalsDeep(other)) 601 return false; 602 if (!(other instanceof ConditionEvidenceComponent)) 603 return false; 604 ConditionEvidenceComponent o = (ConditionEvidenceComponent) other; 605 return compareDeep(code, o.code, true) && compareDeep(detail, o.detail, true); 606 } 607 608 @Override 609 public boolean equalsShallow(Base other) { 610 if (!super.equalsShallow(other)) 611 return false; 612 if (!(other instanceof ConditionEvidenceComponent)) 613 return false; 614 ConditionEvidenceComponent o = (ConditionEvidenceComponent) other; 615 return true; 616 } 617 618 public boolean isEmpty() { 619 return super.isEmpty() && (code == null || code.isEmpty()) && (detail == null || detail.isEmpty()); 620 } 621 622 public String fhirType() { 623 return "Condition.evidence"; 624 625 } 626 627 } 628 629 /** 630 * This records identifiers associated with this condition that are defined by 631 * business processes and/or used to refer to it when a direct URL reference to 632 * the resource itself is not appropriate (e.g. in CDA documents, or in written 633 * / printed documentation). 634 */ 635 @Child(name = "identifier", type = { 636 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 637 @Description(shortDefinition = "External Ids for this condition", formalDefinition = "This records identifiers associated with this condition that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).") 638 protected List<Identifier> identifier; 639 640 /** 641 * Indicates the patient who the condition record is associated with. 642 */ 643 @Child(name = "patient", type = { Patient.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 644 @Description(shortDefinition = "Who has the condition?", formalDefinition = "Indicates the patient who the condition record is associated with.") 645 protected Reference patient; 646 647 /** 648 * The actual object that is the target of the reference (Indicates the patient 649 * who the condition record is associated with.) 650 */ 651 protected Patient patientTarget; 652 653 /** 654 * Encounter during which the condition was first asserted. 655 */ 656 @Child(name = "encounter", type = { Encounter.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 657 @Description(shortDefinition = "Encounter when condition first asserted", formalDefinition = "Encounter during which the condition was first asserted.") 658 protected Reference encounter; 659 660 /** 661 * The actual object that is the target of the reference (Encounter during which 662 * the condition was first asserted.) 663 */ 664 protected Encounter encounterTarget; 665 666 /** 667 * Individual who is making the condition statement. 668 */ 669 @Child(name = "asserter", type = { Practitioner.class, 670 Patient.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 671 @Description(shortDefinition = "Person who asserts this condition", formalDefinition = "Individual who is making the condition statement.") 672 protected Reference asserter; 673 674 /** 675 * The actual object that is the target of the reference (Individual who is 676 * making the condition statement.) 677 */ 678 protected Resource asserterTarget; 679 680 /** 681 * A date, when the Condition statement was documented. 682 */ 683 @Child(name = "dateRecorded", type = { 684 DateType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 685 @Description(shortDefinition = "When first entered", formalDefinition = "A date, when the Condition statement was documented.") 686 protected DateType dateRecorded; 687 688 /** 689 * Identification of the condition, problem or diagnosis. 690 */ 691 @Child(name = "code", type = { CodeableConcept.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 692 @Description(shortDefinition = "Identification of the condition, problem or diagnosis", formalDefinition = "Identification of the condition, problem or diagnosis.") 693 protected CodeableConcept code; 694 695 /** 696 * A category assigned to the condition. 697 */ 698 @Child(name = "category", type = { 699 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 700 @Description(shortDefinition = "complaint | symptom | finding | diagnosis", formalDefinition = "A category assigned to the condition.") 701 protected CodeableConcept category; 702 703 /** 704 * The clinical status of the condition. 705 */ 706 @Child(name = "clinicalStatus", type = { 707 CodeType.class }, order = 7, min = 0, max = 1, modifier = true, summary = true) 708 @Description(shortDefinition = "active | relapse | remission | resolved", formalDefinition = "The clinical status of the condition.") 709 protected CodeType clinicalStatus; 710 711 /** 712 * The verification status to support the clinical status of the condition. 713 */ 714 @Child(name = "verificationStatus", type = { 715 CodeType.class }, order = 8, min = 1, max = 1, modifier = true, summary = true) 716 @Description(shortDefinition = "provisional | differential | confirmed | refuted | entered-in-error | unknown", formalDefinition = "The verification status to support the clinical status of the condition.") 717 protected Enumeration<ConditionVerificationStatus> verificationStatus; 718 719 /** 720 * A subjective assessment of the severity of the condition as evaluated by the 721 * clinician. 722 */ 723 @Child(name = "severity", type = { 724 CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 725 @Description(shortDefinition = "Subjective severity of condition", formalDefinition = "A subjective assessment of the severity of the condition as evaluated by the clinician.") 726 protected CodeableConcept severity; 727 728 /** 729 * Estimated or actual date or date-time the condition began, in the opinion of 730 * the clinician. 731 */ 732 @Child(name = "onset", type = { DateTimeType.class, Age.class, Period.class, Range.class, 733 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 734 @Description(shortDefinition = "Estimated or actual date, date-time, or age", formalDefinition = "Estimated or actual date or date-time the condition began, in the opinion of the clinician.") 735 protected Type onset; 736 737 /** 738 * The date or estimated date that the condition resolved or went into 739 * remission. This is called "abatement" because of the many overloaded 740 * connotations associated with "remission" or "resolution" - Conditions are 741 * never really resolved, but they can abate. 742 */ 743 @Child(name = "abatement", type = { DateTimeType.class, Age.class, BooleanType.class, Period.class, Range.class, 744 StringType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 745 @Description(shortDefinition = "If/when in resolution/remission", formalDefinition = "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.") 746 protected Type abatement; 747 748 /** 749 * Clinical stage or grade of a condition. May include formal severity 750 * assessments. 751 */ 752 @Child(name = "stage", type = {}, order = 12, min = 0, max = 1, modifier = false, summary = true) 753 @Description(shortDefinition = "Stage/grade, usually assessed formally", formalDefinition = "Clinical stage or grade of a condition. May include formal severity assessments.") 754 protected ConditionStageComponent stage; 755 756 /** 757 * Supporting Evidence / manifestations that are the basis on which this 758 * condition is suspected or confirmed. 759 */ 760 @Child(name = "evidence", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 761 @Description(shortDefinition = "Supporting evidence", formalDefinition = "Supporting Evidence / manifestations that are the basis on which this condition is suspected or confirmed.") 762 protected List<ConditionEvidenceComponent> evidence; 763 764 /** 765 * The anatomical location where this condition manifests itself. 766 */ 767 @Child(name = "bodySite", type = { 768 CodeableConcept.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 769 @Description(shortDefinition = "Anatomical location, if relevant", formalDefinition = "The anatomical location where this condition manifests itself.") 770 protected List<CodeableConcept> bodySite; 771 772 /** 773 * Additional information about the Condition. This is a general notes/comments 774 * entry for description of the Condition, its diagnosis and prognosis. 775 */ 776 @Child(name = "notes", type = { StringType.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 777 @Description(shortDefinition = "Additional information about the Condition", formalDefinition = "Additional information about the Condition. This is a general notes/comments entry for description of the Condition, its diagnosis and prognosis.") 778 protected StringType notes; 779 780 private static final long serialVersionUID = -341227215L; 781 782 /* 783 * Constructor 784 */ 785 public Condition() { 786 super(); 787 } 788 789 /* 790 * Constructor 791 */ 792 public Condition(Reference patient, CodeableConcept code, 793 Enumeration<ConditionVerificationStatus> verificationStatus) { 794 super(); 795 this.patient = patient; 796 this.code = code; 797 this.verificationStatus = verificationStatus; 798 } 799 800 /** 801 * @return {@link #identifier} (This records identifiers associated with this 802 * condition that are defined by business processes and/or used to refer 803 * to it when a direct URL reference to the resource itself is not 804 * appropriate (e.g. in CDA documents, or in written / printed 805 * documentation).) 806 */ 807 public List<Identifier> getIdentifier() { 808 if (this.identifier == null) 809 this.identifier = new ArrayList<Identifier>(); 810 return this.identifier; 811 } 812 813 public boolean hasIdentifier() { 814 if (this.identifier == null) 815 return false; 816 for (Identifier item : this.identifier) 817 if (!item.isEmpty()) 818 return true; 819 return false; 820 } 821 822 /** 823 * @return {@link #identifier} (This records identifiers associated with this 824 * condition that are defined by business processes and/or used to refer 825 * to it when a direct URL reference to the resource itself is not 826 * appropriate (e.g. in CDA documents, or in written / printed 827 * documentation).) 828 */ 829 // syntactic sugar 830 public Identifier addIdentifier() { // 3 831 Identifier t = new Identifier(); 832 if (this.identifier == null) 833 this.identifier = new ArrayList<Identifier>(); 834 this.identifier.add(t); 835 return t; 836 } 837 838 // syntactic sugar 839 public Condition addIdentifier(Identifier t) { // 3 840 if (t == null) 841 return this; 842 if (this.identifier == null) 843 this.identifier = new ArrayList<Identifier>(); 844 this.identifier.add(t); 845 return this; 846 } 847 848 /** 849 * @return {@link #patient} (Indicates the patient who the condition record is 850 * associated with.) 851 */ 852 public Reference getPatient() { 853 if (this.patient == null) 854 if (Configuration.errorOnAutoCreate()) 855 throw new Error("Attempt to auto-create Condition.patient"); 856 else if (Configuration.doAutoCreate()) 857 this.patient = new Reference(); // cc 858 return this.patient; 859 } 860 861 public boolean hasPatient() { 862 return this.patient != null && !this.patient.isEmpty(); 863 } 864 865 /** 866 * @param value {@link #patient} (Indicates the patient who the condition record 867 * is associated with.) 868 */ 869 public Condition setPatient(Reference value) { 870 this.patient = value; 871 return this; 872 } 873 874 /** 875 * @return {@link #patient} The actual object that is the target of the 876 * reference. The reference library doesn't populate this, but you can 877 * use it to hold the resource if you resolve it. (Indicates the patient 878 * who the condition record is associated with.) 879 */ 880 public Patient getPatientTarget() { 881 if (this.patientTarget == null) 882 if (Configuration.errorOnAutoCreate()) 883 throw new Error("Attempt to auto-create Condition.patient"); 884 else if (Configuration.doAutoCreate()) 885 this.patientTarget = new Patient(); // aa 886 return this.patientTarget; 887 } 888 889 /** 890 * @param value {@link #patient} The actual object that is the target of the 891 * reference. The reference library doesn't use these, but you can 892 * use it to hold the resource if you resolve it. (Indicates the 893 * patient who the condition record is associated with.) 894 */ 895 public Condition setPatientTarget(Patient value) { 896 this.patientTarget = value; 897 return this; 898 } 899 900 /** 901 * @return {@link #encounter} (Encounter during which the condition was first 902 * asserted.) 903 */ 904 public Reference getEncounter() { 905 if (this.encounter == null) 906 if (Configuration.errorOnAutoCreate()) 907 throw new Error("Attempt to auto-create Condition.encounter"); 908 else if (Configuration.doAutoCreate()) 909 this.encounter = new Reference(); // cc 910 return this.encounter; 911 } 912 913 public boolean hasEncounter() { 914 return this.encounter != null && !this.encounter.isEmpty(); 915 } 916 917 /** 918 * @param value {@link #encounter} (Encounter during which the condition was 919 * first asserted.) 920 */ 921 public Condition setEncounter(Reference value) { 922 this.encounter = value; 923 return this; 924 } 925 926 /** 927 * @return {@link #encounter} The actual object that is the target of the 928 * reference. The reference library doesn't populate this, but you can 929 * use it to hold the resource if you resolve it. (Encounter during 930 * which the condition was first asserted.) 931 */ 932 public Encounter getEncounterTarget() { 933 if (this.encounterTarget == null) 934 if (Configuration.errorOnAutoCreate()) 935 throw new Error("Attempt to auto-create Condition.encounter"); 936 else if (Configuration.doAutoCreate()) 937 this.encounterTarget = new Encounter(); // aa 938 return this.encounterTarget; 939 } 940 941 /** 942 * @param value {@link #encounter} The actual object that is the target of the 943 * reference. The reference library doesn't use these, but you can 944 * use it to hold the resource if you resolve it. (Encounter during 945 * which the condition was first asserted.) 946 */ 947 public Condition setEncounterTarget(Encounter value) { 948 this.encounterTarget = value; 949 return this; 950 } 951 952 /** 953 * @return {@link #asserter} (Individual who is making the condition statement.) 954 */ 955 public Reference getAsserter() { 956 if (this.asserter == null) 957 if (Configuration.errorOnAutoCreate()) 958 throw new Error("Attempt to auto-create Condition.asserter"); 959 else if (Configuration.doAutoCreate()) 960 this.asserter = new Reference(); // cc 961 return this.asserter; 962 } 963 964 public boolean hasAsserter() { 965 return this.asserter != null && !this.asserter.isEmpty(); 966 } 967 968 /** 969 * @param value {@link #asserter} (Individual who is making the condition 970 * statement.) 971 */ 972 public Condition setAsserter(Reference value) { 973 this.asserter = value; 974 return this; 975 } 976 977 /** 978 * @return {@link #asserter} The actual object that is the target of the 979 * reference. The reference library doesn't populate this, but you can 980 * use it to hold the resource if you resolve it. (Individual who is 981 * making the condition statement.) 982 */ 983 public Resource getAsserterTarget() { 984 return this.asserterTarget; 985 } 986 987 /** 988 * @param value {@link #asserter} The actual object that is the target of the 989 * reference. The reference library doesn't use these, but you can 990 * use it to hold the resource if you resolve it. (Individual who 991 * is making the condition statement.) 992 */ 993 public Condition setAsserterTarget(Resource value) { 994 this.asserterTarget = value; 995 return this; 996 } 997 998 /** 999 * @return {@link #dateRecorded} (A date, when the Condition statement was 1000 * documented.). This is the underlying object with id, value and 1001 * extensions. The accessor "getDateRecorded" gives direct access to the 1002 * value 1003 */ 1004 public DateType getDateRecordedElement() { 1005 if (this.dateRecorded == null) 1006 if (Configuration.errorOnAutoCreate()) 1007 throw new Error("Attempt to auto-create Condition.dateRecorded"); 1008 else if (Configuration.doAutoCreate()) 1009 this.dateRecorded = new DateType(); // bb 1010 return this.dateRecorded; 1011 } 1012 1013 public boolean hasDateRecordedElement() { 1014 return this.dateRecorded != null && !this.dateRecorded.isEmpty(); 1015 } 1016 1017 public boolean hasDateRecorded() { 1018 return this.dateRecorded != null && !this.dateRecorded.isEmpty(); 1019 } 1020 1021 /** 1022 * @param value {@link #dateRecorded} (A date, when the Condition statement was 1023 * documented.). This is the underlying object with id, value and 1024 * extensions. The accessor "getDateRecorded" gives direct access 1025 * to the value 1026 */ 1027 public Condition setDateRecordedElement(DateType value) { 1028 this.dateRecorded = value; 1029 return this; 1030 } 1031 1032 /** 1033 * @return A date, when the Condition statement was documented. 1034 */ 1035 public Date getDateRecorded() { 1036 return this.dateRecorded == null ? null : this.dateRecorded.getValue(); 1037 } 1038 1039 /** 1040 * @param value A date, when the Condition statement was documented. 1041 */ 1042 public Condition setDateRecorded(Date value) { 1043 if (value == null) 1044 this.dateRecorded = null; 1045 else { 1046 if (this.dateRecorded == null) 1047 this.dateRecorded = new DateType(); 1048 this.dateRecorded.setValue(value); 1049 } 1050 return this; 1051 } 1052 1053 /** 1054 * @return {@link #code} (Identification of the condition, problem or 1055 * diagnosis.) 1056 */ 1057 public CodeableConcept getCode() { 1058 if (this.code == null) 1059 if (Configuration.errorOnAutoCreate()) 1060 throw new Error("Attempt to auto-create Condition.code"); 1061 else if (Configuration.doAutoCreate()) 1062 this.code = new CodeableConcept(); // cc 1063 return this.code; 1064 } 1065 1066 public boolean hasCode() { 1067 return this.code != null && !this.code.isEmpty(); 1068 } 1069 1070 /** 1071 * @param value {@link #code} (Identification of the condition, problem or 1072 * diagnosis.) 1073 */ 1074 public Condition setCode(CodeableConcept value) { 1075 this.code = value; 1076 return this; 1077 } 1078 1079 /** 1080 * @return {@link #category} (A category assigned to the condition.) 1081 */ 1082 public CodeableConcept getCategory() { 1083 if (this.category == null) 1084 if (Configuration.errorOnAutoCreate()) 1085 throw new Error("Attempt to auto-create Condition.category"); 1086 else if (Configuration.doAutoCreate()) 1087 this.category = new CodeableConcept(); // cc 1088 return this.category; 1089 } 1090 1091 public boolean hasCategory() { 1092 return this.category != null && !this.category.isEmpty(); 1093 } 1094 1095 /** 1096 * @param value {@link #category} (A category assigned to the condition.) 1097 */ 1098 public Condition setCategory(CodeableConcept value) { 1099 this.category = value; 1100 return this; 1101 } 1102 1103 /** 1104 * @return {@link #clinicalStatus} (The clinical status of the condition.). This 1105 * is the underlying object with id, value and extensions. The accessor 1106 * "getClinicalStatus" gives direct access to the value 1107 */ 1108 public CodeType getClinicalStatusElement() { 1109 if (this.clinicalStatus == null) 1110 if (Configuration.errorOnAutoCreate()) 1111 throw new Error("Attempt to auto-create Condition.clinicalStatus"); 1112 else if (Configuration.doAutoCreate()) 1113 this.clinicalStatus = new CodeType(); // bb 1114 return this.clinicalStatus; 1115 } 1116 1117 public boolean hasClinicalStatusElement() { 1118 return this.clinicalStatus != null && !this.clinicalStatus.isEmpty(); 1119 } 1120 1121 public boolean hasClinicalStatus() { 1122 return this.clinicalStatus != null && !this.clinicalStatus.isEmpty(); 1123 } 1124 1125 /** 1126 * @param value {@link #clinicalStatus} (The clinical status of the condition.). 1127 * This is the underlying object with id, value and extensions. The 1128 * accessor "getClinicalStatus" gives direct access to the value 1129 */ 1130 public Condition setClinicalStatusElement(CodeType value) { 1131 this.clinicalStatus = value; 1132 return this; 1133 } 1134 1135 /** 1136 * @return The clinical status of the condition. 1137 */ 1138 public String getClinicalStatus() { 1139 return this.clinicalStatus == null ? null : this.clinicalStatus.getValue(); 1140 } 1141 1142 /** 1143 * @param value The clinical status of the condition. 1144 */ 1145 public Condition setClinicalStatus(String value) { 1146 if (Utilities.noString(value)) 1147 this.clinicalStatus = null; 1148 else { 1149 if (this.clinicalStatus == null) 1150 this.clinicalStatus = new CodeType(); 1151 this.clinicalStatus.setValue(value); 1152 } 1153 return this; 1154 } 1155 1156 /** 1157 * @return {@link #verificationStatus} (The verification status to support the 1158 * clinical status of the condition.). This is the underlying object 1159 * with id, value and extensions. The accessor "getVerificationStatus" 1160 * gives direct access to the value 1161 */ 1162 public Enumeration<ConditionVerificationStatus> getVerificationStatusElement() { 1163 if (this.verificationStatus == null) 1164 if (Configuration.errorOnAutoCreate()) 1165 throw new Error("Attempt to auto-create Condition.verificationStatus"); 1166 else if (Configuration.doAutoCreate()) 1167 this.verificationStatus = new Enumeration<ConditionVerificationStatus>( 1168 new ConditionVerificationStatusEnumFactory()); // bb 1169 return this.verificationStatus; 1170 } 1171 1172 public boolean hasVerificationStatusElement() { 1173 return this.verificationStatus != null && !this.verificationStatus.isEmpty(); 1174 } 1175 1176 public boolean hasVerificationStatus() { 1177 return this.verificationStatus != null && !this.verificationStatus.isEmpty(); 1178 } 1179 1180 /** 1181 * @param value {@link #verificationStatus} (The verification status to support 1182 * the clinical status of the condition.). This is the underlying 1183 * object with id, value and extensions. The accessor 1184 * "getVerificationStatus" gives direct access to the value 1185 */ 1186 public Condition setVerificationStatusElement(Enumeration<ConditionVerificationStatus> value) { 1187 this.verificationStatus = value; 1188 return this; 1189 } 1190 1191 /** 1192 * @return The verification status to support the clinical status of the 1193 * condition. 1194 */ 1195 public ConditionVerificationStatus getVerificationStatus() { 1196 return this.verificationStatus == null ? null : this.verificationStatus.getValue(); 1197 } 1198 1199 /** 1200 * @param value The verification status to support the clinical status of the 1201 * condition. 1202 */ 1203 public Condition setVerificationStatus(ConditionVerificationStatus value) { 1204 if (this.verificationStatus == null) 1205 this.verificationStatus = new Enumeration<ConditionVerificationStatus>( 1206 new ConditionVerificationStatusEnumFactory()); 1207 this.verificationStatus.setValue(value); 1208 return this; 1209 } 1210 1211 /** 1212 * @return {@link #severity} (A subjective assessment of the severity of the 1213 * condition as evaluated by the clinician.) 1214 */ 1215 public CodeableConcept getSeverity() { 1216 if (this.severity == null) 1217 if (Configuration.errorOnAutoCreate()) 1218 throw new Error("Attempt to auto-create Condition.severity"); 1219 else if (Configuration.doAutoCreate()) 1220 this.severity = new CodeableConcept(); // cc 1221 return this.severity; 1222 } 1223 1224 public boolean hasSeverity() { 1225 return this.severity != null && !this.severity.isEmpty(); 1226 } 1227 1228 /** 1229 * @param value {@link #severity} (A subjective assessment of the severity of 1230 * the condition as evaluated by the clinician.) 1231 */ 1232 public Condition setSeverity(CodeableConcept value) { 1233 this.severity = value; 1234 return this; 1235 } 1236 1237 /** 1238 * @return {@link #onset} (Estimated or actual date or date-time the condition 1239 * began, in the opinion of the clinician.) 1240 */ 1241 public Type getOnset() { 1242 return this.onset; 1243 } 1244 1245 /** 1246 * @return {@link #onset} (Estimated or actual date or date-time the condition 1247 * began, in the opinion of the clinician.) 1248 */ 1249 public DateTimeType getOnsetDateTimeType() throws FHIRException { 1250 if (!(this.onset instanceof DateTimeType)) 1251 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1252 + this.onset.getClass().getName() + " was encountered"); 1253 return (DateTimeType) this.onset; 1254 } 1255 1256 public boolean hasOnsetDateTimeType() { 1257 return this.onset instanceof DateTimeType; 1258 } 1259 1260 /** 1261 * @return {@link #onset} (Estimated or actual date or date-time the condition 1262 * began, in the opinion of the clinician.) 1263 */ 1264 public Age getOnsetAge() throws FHIRException { 1265 if (!(this.onset instanceof Age)) 1266 throw new FHIRException( 1267 "Type mismatch: the type Age was expected, but " + this.onset.getClass().getName() + " was encountered"); 1268 return (Age) this.onset; 1269 } 1270 1271 public boolean hasOnsetAge() { 1272 return this.onset instanceof Age; 1273 } 1274 1275 /** 1276 * @return {@link #onset} (Estimated or actual date or date-time the condition 1277 * began, in the opinion of the clinician.) 1278 */ 1279 public Period getOnsetPeriod() throws FHIRException { 1280 if (!(this.onset instanceof Period)) 1281 throw new FHIRException( 1282 "Type mismatch: the type Period was expected, but " + this.onset.getClass().getName() + " was encountered"); 1283 return (Period) this.onset; 1284 } 1285 1286 public boolean hasOnsetPeriod() { 1287 return this.onset instanceof Period; 1288 } 1289 1290 /** 1291 * @return {@link #onset} (Estimated or actual date or date-time the condition 1292 * began, in the opinion of the clinician.) 1293 */ 1294 public Range getOnsetRange() throws FHIRException { 1295 if (!(this.onset instanceof Range)) 1296 throw new FHIRException( 1297 "Type mismatch: the type Range was expected, but " + this.onset.getClass().getName() + " was encountered"); 1298 return (Range) this.onset; 1299 } 1300 1301 public boolean hasOnsetRange() { 1302 return this.onset instanceof Range; 1303 } 1304 1305 /** 1306 * @return {@link #onset} (Estimated or actual date or date-time the condition 1307 * began, in the opinion of the clinician.) 1308 */ 1309 public StringType getOnsetStringType() throws FHIRException { 1310 if (!(this.onset instanceof StringType)) 1311 throw new FHIRException("Type mismatch: the type StringType was expected, but " + this.onset.getClass().getName() 1312 + " was encountered"); 1313 return (StringType) this.onset; 1314 } 1315 1316 public boolean hasOnsetStringType() { 1317 return this.onset instanceof StringType; 1318 } 1319 1320 public boolean hasOnset() { 1321 return this.onset != null && !this.onset.isEmpty(); 1322 } 1323 1324 /** 1325 * @param value {@link #onset} (Estimated or actual date or date-time the 1326 * condition began, in the opinion of the clinician.) 1327 */ 1328 public Condition setOnset(Type value) { 1329 this.onset = value; 1330 return this; 1331 } 1332 1333 /** 1334 * @return {@link #abatement} (The date or estimated date that the condition 1335 * resolved or went into remission. This is called "abatement" because 1336 * of the many overloaded connotations associated with "remission" or 1337 * "resolution" - Conditions are never really resolved, but they can 1338 * abate.) 1339 */ 1340 public Type getAbatement() { 1341 return this.abatement; 1342 } 1343 1344 /** 1345 * @return {@link #abatement} (The date or estimated date that the condition 1346 * resolved or went into remission. This is called "abatement" because 1347 * of the many overloaded connotations associated with "remission" or 1348 * "resolution" - Conditions are never really resolved, but they can 1349 * abate.) 1350 */ 1351 public DateTimeType getAbatementDateTimeType() throws FHIRException { 1352 if (!(this.abatement instanceof DateTimeType)) 1353 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1354 + this.abatement.getClass().getName() + " was encountered"); 1355 return (DateTimeType) this.abatement; 1356 } 1357 1358 public boolean hasAbatementDateTimeType() { 1359 return this.abatement instanceof DateTimeType; 1360 } 1361 1362 /** 1363 * @return {@link #abatement} (The date or estimated date that the condition 1364 * resolved or went into remission. This is called "abatement" because 1365 * of the many overloaded connotations associated with "remission" or 1366 * "resolution" - Conditions are never really resolved, but they can 1367 * abate.) 1368 */ 1369 public Age getAbatementAge() throws FHIRException { 1370 if (!(this.abatement instanceof Age)) 1371 throw new FHIRException( 1372 "Type mismatch: the type Age was expected, but " + this.abatement.getClass().getName() + " was encountered"); 1373 return (Age) this.abatement; 1374 } 1375 1376 public boolean hasAbatementAge() { 1377 return this.abatement instanceof Age; 1378 } 1379 1380 /** 1381 * @return {@link #abatement} (The date or estimated date that the condition 1382 * resolved or went into remission. This is called "abatement" because 1383 * of the many overloaded connotations associated with "remission" or 1384 * "resolution" - Conditions are never really resolved, but they can 1385 * abate.) 1386 */ 1387 public BooleanType getAbatementBooleanType() throws FHIRException { 1388 if (!(this.abatement instanceof BooleanType)) 1389 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1390 + this.abatement.getClass().getName() + " was encountered"); 1391 return (BooleanType) this.abatement; 1392 } 1393 1394 public boolean hasAbatementBooleanType() { 1395 return this.abatement instanceof BooleanType; 1396 } 1397 1398 /** 1399 * @return {@link #abatement} (The date or estimated date that the condition 1400 * resolved or went into remission. This is called "abatement" because 1401 * of the many overloaded connotations associated with "remission" or 1402 * "resolution" - Conditions are never really resolved, but they can 1403 * abate.) 1404 */ 1405 public Period getAbatementPeriod() throws FHIRException { 1406 if (!(this.abatement instanceof Period)) 1407 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.abatement.getClass().getName() 1408 + " was encountered"); 1409 return (Period) this.abatement; 1410 } 1411 1412 public boolean hasAbatementPeriod() { 1413 return this.abatement instanceof Period; 1414 } 1415 1416 /** 1417 * @return {@link #abatement} (The date or estimated date that the condition 1418 * resolved or went into remission. This is called "abatement" because 1419 * of the many overloaded connotations associated with "remission" or 1420 * "resolution" - Conditions are never really resolved, but they can 1421 * abate.) 1422 */ 1423 public Range getAbatementRange() throws FHIRException { 1424 if (!(this.abatement instanceof Range)) 1425 throw new FHIRException("Type mismatch: the type Range was expected, but " + this.abatement.getClass().getName() 1426 + " was encountered"); 1427 return (Range) this.abatement; 1428 } 1429 1430 public boolean hasAbatementRange() { 1431 return this.abatement instanceof Range; 1432 } 1433 1434 /** 1435 * @return {@link #abatement} (The date or estimated date that the condition 1436 * resolved or went into remission. This is called "abatement" because 1437 * of the many overloaded connotations associated with "remission" or 1438 * "resolution" - Conditions are never really resolved, but they can 1439 * abate.) 1440 */ 1441 public StringType getAbatementStringType() throws FHIRException { 1442 if (!(this.abatement instanceof StringType)) 1443 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1444 + this.abatement.getClass().getName() + " was encountered"); 1445 return (StringType) this.abatement; 1446 } 1447 1448 public boolean hasAbatementStringType() { 1449 return this.abatement instanceof StringType; 1450 } 1451 1452 public boolean hasAbatement() { 1453 return this.abatement != null && !this.abatement.isEmpty(); 1454 } 1455 1456 /** 1457 * @param value {@link #abatement} (The date or estimated date that the 1458 * condition resolved or went into remission. This is called 1459 * "abatement" because of the many overloaded connotations 1460 * associated with "remission" or "resolution" - Conditions are 1461 * never really resolved, but they can abate.) 1462 */ 1463 public Condition setAbatement(Type value) { 1464 this.abatement = value; 1465 return this; 1466 } 1467 1468 /** 1469 * @return {@link #stage} (Clinical stage or grade of a condition. May include 1470 * formal severity assessments.) 1471 */ 1472 public ConditionStageComponent getStage() { 1473 if (this.stage == null) 1474 if (Configuration.errorOnAutoCreate()) 1475 throw new Error("Attempt to auto-create Condition.stage"); 1476 else if (Configuration.doAutoCreate()) 1477 this.stage = new ConditionStageComponent(); // cc 1478 return this.stage; 1479 } 1480 1481 public boolean hasStage() { 1482 return this.stage != null && !this.stage.isEmpty(); 1483 } 1484 1485 /** 1486 * @param value {@link #stage} (Clinical stage or grade of a condition. May 1487 * include formal severity assessments.) 1488 */ 1489 public Condition setStage(ConditionStageComponent value) { 1490 this.stage = value; 1491 return this; 1492 } 1493 1494 /** 1495 * @return {@link #evidence} (Supporting Evidence / manifestations that are the 1496 * basis on which this condition is suspected or confirmed.) 1497 */ 1498 public List<ConditionEvidenceComponent> getEvidence() { 1499 if (this.evidence == null) 1500 this.evidence = new ArrayList<ConditionEvidenceComponent>(); 1501 return this.evidence; 1502 } 1503 1504 public boolean hasEvidence() { 1505 if (this.evidence == null) 1506 return false; 1507 for (ConditionEvidenceComponent item : this.evidence) 1508 if (!item.isEmpty()) 1509 return true; 1510 return false; 1511 } 1512 1513 /** 1514 * @return {@link #evidence} (Supporting Evidence / manifestations that are the 1515 * basis on which this condition is suspected or confirmed.) 1516 */ 1517 // syntactic sugar 1518 public ConditionEvidenceComponent addEvidence() { // 3 1519 ConditionEvidenceComponent t = new ConditionEvidenceComponent(); 1520 if (this.evidence == null) 1521 this.evidence = new ArrayList<ConditionEvidenceComponent>(); 1522 this.evidence.add(t); 1523 return t; 1524 } 1525 1526 // syntactic sugar 1527 public Condition addEvidence(ConditionEvidenceComponent t) { // 3 1528 if (t == null) 1529 return this; 1530 if (this.evidence == null) 1531 this.evidence = new ArrayList<ConditionEvidenceComponent>(); 1532 this.evidence.add(t); 1533 return this; 1534 } 1535 1536 /** 1537 * @return {@link #bodySite} (The anatomical location where this condition 1538 * manifests itself.) 1539 */ 1540 public List<CodeableConcept> getBodySite() { 1541 if (this.bodySite == null) 1542 this.bodySite = new ArrayList<CodeableConcept>(); 1543 return this.bodySite; 1544 } 1545 1546 public boolean hasBodySite() { 1547 if (this.bodySite == null) 1548 return false; 1549 for (CodeableConcept item : this.bodySite) 1550 if (!item.isEmpty()) 1551 return true; 1552 return false; 1553 } 1554 1555 /** 1556 * @return {@link #bodySite} (The anatomical location where this condition 1557 * manifests itself.) 1558 */ 1559 // syntactic sugar 1560 public CodeableConcept addBodySite() { // 3 1561 CodeableConcept t = new CodeableConcept(); 1562 if (this.bodySite == null) 1563 this.bodySite = new ArrayList<CodeableConcept>(); 1564 this.bodySite.add(t); 1565 return t; 1566 } 1567 1568 // syntactic sugar 1569 public Condition addBodySite(CodeableConcept t) { // 3 1570 if (t == null) 1571 return this; 1572 if (this.bodySite == null) 1573 this.bodySite = new ArrayList<CodeableConcept>(); 1574 this.bodySite.add(t); 1575 return this; 1576 } 1577 1578 /** 1579 * @return {@link #notes} (Additional information about the Condition. This is a 1580 * general notes/comments entry for description of the Condition, its 1581 * diagnosis and prognosis.). This is the underlying object with id, 1582 * value and extensions. The accessor "getNotes" gives direct access to 1583 * the value 1584 */ 1585 public StringType getNotesElement() { 1586 if (this.notes == null) 1587 if (Configuration.errorOnAutoCreate()) 1588 throw new Error("Attempt to auto-create Condition.notes"); 1589 else if (Configuration.doAutoCreate()) 1590 this.notes = new StringType(); // bb 1591 return this.notes; 1592 } 1593 1594 public boolean hasNotesElement() { 1595 return this.notes != null && !this.notes.isEmpty(); 1596 } 1597 1598 public boolean hasNotes() { 1599 return this.notes != null && !this.notes.isEmpty(); 1600 } 1601 1602 /** 1603 * @param value {@link #notes} (Additional information about the Condition. This 1604 * is a general notes/comments entry for description of the 1605 * Condition, its diagnosis and prognosis.). This is the underlying 1606 * object with id, value and extensions. The accessor "getNotes" 1607 * gives direct access to the value 1608 */ 1609 public Condition setNotesElement(StringType value) { 1610 this.notes = value; 1611 return this; 1612 } 1613 1614 /** 1615 * @return Additional information about the Condition. This is a general 1616 * notes/comments entry for description of the Condition, its diagnosis 1617 * and prognosis. 1618 */ 1619 public String getNotes() { 1620 return this.notes == null ? null : this.notes.getValue(); 1621 } 1622 1623 /** 1624 * @param value Additional information about the Condition. This is a general 1625 * notes/comments entry for description of the Condition, its 1626 * diagnosis and prognosis. 1627 */ 1628 public Condition setNotes(String value) { 1629 if (Utilities.noString(value)) 1630 this.notes = null; 1631 else { 1632 if (this.notes == null) 1633 this.notes = new StringType(); 1634 this.notes.setValue(value); 1635 } 1636 return this; 1637 } 1638 1639 protected void listChildren(List<Property> childrenList) { 1640 super.listChildren(childrenList); 1641 childrenList.add(new Property("identifier", "Identifier", 1642 "This records identifiers associated with this condition that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 1643 0, java.lang.Integer.MAX_VALUE, identifier)); 1644 childrenList.add(new Property("patient", "Reference(Patient)", 1645 "Indicates the patient who the condition record is associated with.", 0, java.lang.Integer.MAX_VALUE, patient)); 1646 childrenList.add(new Property("encounter", "Reference(Encounter)", 1647 "Encounter during which the condition was first asserted.", 0, java.lang.Integer.MAX_VALUE, encounter)); 1648 childrenList.add(new Property("asserter", "Reference(Practitioner|Patient)", 1649 "Individual who is making the condition statement.", 0, java.lang.Integer.MAX_VALUE, asserter)); 1650 childrenList.add(new Property("dateRecorded", "date", "A date, when the Condition statement was documented.", 0, 1651 java.lang.Integer.MAX_VALUE, dateRecorded)); 1652 childrenList.add(new Property("code", "CodeableConcept", "Identification of the condition, problem or diagnosis.", 1653 0, java.lang.Integer.MAX_VALUE, code)); 1654 childrenList.add(new Property("category", "CodeableConcept", "A category assigned to the condition.", 0, 1655 java.lang.Integer.MAX_VALUE, category)); 1656 childrenList.add(new Property("clinicalStatus", "code", "The clinical status of the condition.", 0, 1657 java.lang.Integer.MAX_VALUE, clinicalStatus)); 1658 childrenList.add(new Property("verificationStatus", "code", 1659 "The verification status to support the clinical status of the condition.", 0, java.lang.Integer.MAX_VALUE, 1660 verificationStatus)); 1661 childrenList.add(new Property("severity", "CodeableConcept", 1662 "A subjective assessment of the severity of the condition as evaluated by the clinician.", 0, 1663 java.lang.Integer.MAX_VALUE, severity)); 1664 childrenList.add(new Property("onset[x]", "dateTime|Age|Period|Range|string", 1665 "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1666 java.lang.Integer.MAX_VALUE, onset)); 1667 childrenList.add(new Property("abatement[x]", "dateTime|Age|boolean|Period|Range|string", 1668 "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 1669 0, java.lang.Integer.MAX_VALUE, abatement)); 1670 childrenList.add( 1671 new Property("stage", "", "Clinical stage or grade of a condition. May include formal severity assessments.", 0, 1672 java.lang.Integer.MAX_VALUE, stage)); 1673 childrenList.add(new Property("evidence", "", 1674 "Supporting Evidence / manifestations that are the basis on which this condition is suspected or confirmed.", 0, 1675 java.lang.Integer.MAX_VALUE, evidence)); 1676 childrenList.add(new Property("bodySite", "CodeableConcept", 1677 "The anatomical location where this condition manifests itself.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 1678 childrenList.add(new Property("notes", "string", 1679 "Additional information about the Condition. This is a general notes/comments entry for description of the Condition, its diagnosis and prognosis.", 1680 0, java.lang.Integer.MAX_VALUE, notes)); 1681 } 1682 1683 @Override 1684 public void setProperty(String name, Base value) throws FHIRException { 1685 if (name.equals("identifier")) 1686 this.getIdentifier().add(castToIdentifier(value)); 1687 else if (name.equals("patient")) 1688 this.patient = castToReference(value); // Reference 1689 else if (name.equals("encounter")) 1690 this.encounter = castToReference(value); // Reference 1691 else if (name.equals("asserter")) 1692 this.asserter = castToReference(value); // Reference 1693 else if (name.equals("dateRecorded")) 1694 this.dateRecorded = castToDate(value); // DateType 1695 else if (name.equals("code")) 1696 this.code = castToCodeableConcept(value); // CodeableConcept 1697 else if (name.equals("category")) 1698 this.category = castToCodeableConcept(value); // CodeableConcept 1699 else if (name.equals("clinicalStatus")) 1700 this.clinicalStatus = castToCode(value); // CodeType 1701 else if (name.equals("verificationStatus")) 1702 this.verificationStatus = new ConditionVerificationStatusEnumFactory().fromType(value); // Enumeration<ConditionVerificationStatus> 1703 else if (name.equals("severity")) 1704 this.severity = castToCodeableConcept(value); // CodeableConcept 1705 else if (name.equals("onset[x]")) 1706 this.onset = (Type) value; // Type 1707 else if (name.equals("abatement[x]")) 1708 this.abatement = (Type) value; // Type 1709 else if (name.equals("stage")) 1710 this.stage = (ConditionStageComponent) value; // ConditionStageComponent 1711 else if (name.equals("evidence")) 1712 this.getEvidence().add((ConditionEvidenceComponent) value); 1713 else if (name.equals("bodySite")) 1714 this.getBodySite().add(castToCodeableConcept(value)); 1715 else if (name.equals("notes")) 1716 this.notes = castToString(value); // StringType 1717 else 1718 super.setProperty(name, value); 1719 } 1720 1721 @Override 1722 public Base addChild(String name) throws FHIRException { 1723 if (name.equals("identifier")) { 1724 return addIdentifier(); 1725 } else if (name.equals("patient")) { 1726 this.patient = new Reference(); 1727 return this.patient; 1728 } else if (name.equals("encounter")) { 1729 this.encounter = new Reference(); 1730 return this.encounter; 1731 } else if (name.equals("asserter")) { 1732 this.asserter = new Reference(); 1733 return this.asserter; 1734 } else if (name.equals("dateRecorded")) { 1735 throw new FHIRException("Cannot call addChild on a singleton property Condition.dateRecorded"); 1736 } else if (name.equals("code")) { 1737 this.code = new CodeableConcept(); 1738 return this.code; 1739 } else if (name.equals("category")) { 1740 this.category = new CodeableConcept(); 1741 return this.category; 1742 } else if (name.equals("clinicalStatus")) { 1743 throw new FHIRException("Cannot call addChild on a singleton property Condition.clinicalStatus"); 1744 } else if (name.equals("verificationStatus")) { 1745 throw new FHIRException("Cannot call addChild on a singleton property Condition.verificationStatus"); 1746 } else if (name.equals("severity")) { 1747 this.severity = new CodeableConcept(); 1748 return this.severity; 1749 } else if (name.equals("onsetDateTime")) { 1750 this.onset = new DateTimeType(); 1751 return this.onset; 1752 } else if (name.equals("onsetAge")) { 1753 this.onset = new Age(); 1754 return this.onset; 1755 } else if (name.equals("onsetPeriod")) { 1756 this.onset = new Period(); 1757 return this.onset; 1758 } else if (name.equals("onsetRange")) { 1759 this.onset = new Range(); 1760 return this.onset; 1761 } else if (name.equals("onsetString")) { 1762 this.onset = new StringType(); 1763 return this.onset; 1764 } else if (name.equals("abatementDateTime")) { 1765 this.abatement = new DateTimeType(); 1766 return this.abatement; 1767 } else if (name.equals("abatementAge")) { 1768 this.abatement = new Age(); 1769 return this.abatement; 1770 } else if (name.equals("abatementBoolean")) { 1771 this.abatement = new BooleanType(); 1772 return this.abatement; 1773 } else if (name.equals("abatementPeriod")) { 1774 this.abatement = new Period(); 1775 return this.abatement; 1776 } else if (name.equals("abatementRange")) { 1777 this.abatement = new Range(); 1778 return this.abatement; 1779 } else if (name.equals("abatementString")) { 1780 this.abatement = new StringType(); 1781 return this.abatement; 1782 } else if (name.equals("stage")) { 1783 this.stage = new ConditionStageComponent(); 1784 return this.stage; 1785 } else if (name.equals("evidence")) { 1786 return addEvidence(); 1787 } else if (name.equals("bodySite")) { 1788 return addBodySite(); 1789 } else if (name.equals("notes")) { 1790 throw new FHIRException("Cannot call addChild on a singleton property Condition.notes"); 1791 } else 1792 return super.addChild(name); 1793 } 1794 1795 public String fhirType() { 1796 return "Condition"; 1797 1798 } 1799 1800 public Condition copy() { 1801 Condition dst = new Condition(); 1802 copyValues(dst); 1803 if (identifier != null) { 1804 dst.identifier = new ArrayList<Identifier>(); 1805 for (Identifier i : identifier) 1806 dst.identifier.add(i.copy()); 1807 } 1808 ; 1809 dst.patient = patient == null ? null : patient.copy(); 1810 dst.encounter = encounter == null ? null : encounter.copy(); 1811 dst.asserter = asserter == null ? null : asserter.copy(); 1812 dst.dateRecorded = dateRecorded == null ? null : dateRecorded.copy(); 1813 dst.code = code == null ? null : code.copy(); 1814 dst.category = category == null ? null : category.copy(); 1815 dst.clinicalStatus = clinicalStatus == null ? null : clinicalStatus.copy(); 1816 dst.verificationStatus = verificationStatus == null ? null : verificationStatus.copy(); 1817 dst.severity = severity == null ? null : severity.copy(); 1818 dst.onset = onset == null ? null : onset.copy(); 1819 dst.abatement = abatement == null ? null : abatement.copy(); 1820 dst.stage = stage == null ? null : stage.copy(); 1821 if (evidence != null) { 1822 dst.evidence = new ArrayList<ConditionEvidenceComponent>(); 1823 for (ConditionEvidenceComponent i : evidence) 1824 dst.evidence.add(i.copy()); 1825 } 1826 ; 1827 if (bodySite != null) { 1828 dst.bodySite = new ArrayList<CodeableConcept>(); 1829 for (CodeableConcept i : bodySite) 1830 dst.bodySite.add(i.copy()); 1831 } 1832 ; 1833 dst.notes = notes == null ? null : notes.copy(); 1834 return dst; 1835 } 1836 1837 protected Condition typedCopy() { 1838 return copy(); 1839 } 1840 1841 @Override 1842 public boolean equalsDeep(Base other) { 1843 if (!super.equalsDeep(other)) 1844 return false; 1845 if (!(other instanceof Condition)) 1846 return false; 1847 Condition o = (Condition) other; 1848 return compareDeep(identifier, o.identifier, true) && compareDeep(patient, o.patient, true) 1849 && compareDeep(encounter, o.encounter, true) && compareDeep(asserter, o.asserter, true) 1850 && compareDeep(dateRecorded, o.dateRecorded, true) && compareDeep(code, o.code, true) 1851 && compareDeep(category, o.category, true) && compareDeep(clinicalStatus, o.clinicalStatus, true) 1852 && compareDeep(verificationStatus, o.verificationStatus, true) && compareDeep(severity, o.severity, true) 1853 && compareDeep(onset, o.onset, true) && compareDeep(abatement, o.abatement, true) 1854 && compareDeep(stage, o.stage, true) && compareDeep(evidence, o.evidence, true) 1855 && compareDeep(bodySite, o.bodySite, true) && compareDeep(notes, o.notes, true); 1856 } 1857 1858 @Override 1859 public boolean equalsShallow(Base other) { 1860 if (!super.equalsShallow(other)) 1861 return false; 1862 if (!(other instanceof Condition)) 1863 return false; 1864 Condition o = (Condition) other; 1865 return compareValues(dateRecorded, o.dateRecorded, true) && compareValues(clinicalStatus, o.clinicalStatus, true) 1866 && compareValues(verificationStatus, o.verificationStatus, true) && compareValues(notes, o.notes, true); 1867 } 1868 1869 public boolean isEmpty() { 1870 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (patient == null || patient.isEmpty()) 1871 && (encounter == null || encounter.isEmpty()) && (asserter == null || asserter.isEmpty()) 1872 && (dateRecorded == null || dateRecorded.isEmpty()) && (code == null || code.isEmpty()) 1873 && (category == null || category.isEmpty()) && (clinicalStatus == null || clinicalStatus.isEmpty()) 1874 && (verificationStatus == null || verificationStatus.isEmpty()) && (severity == null || severity.isEmpty()) 1875 && (onset == null || onset.isEmpty()) && (abatement == null || abatement.isEmpty()) 1876 && (stage == null || stage.isEmpty()) && (evidence == null || evidence.isEmpty()) 1877 && (bodySite == null || bodySite.isEmpty()) && (notes == null || notes.isEmpty()); 1878 } 1879 1880 @Override 1881 public ResourceType getResourceType() { 1882 return ResourceType.Condition; 1883 } 1884 1885 @SearchParamDefinition(name = "severity", path = "Condition.severity", description = "The severity of the condition", type = "token") 1886 public static final String SP_SEVERITY = "severity"; 1887 @SearchParamDefinition(name = "identifier", path = "Condition.identifier", description = "A unique identifier of the condition record", type = "token") 1888 public static final String SP_IDENTIFIER = "identifier"; 1889 @SearchParamDefinition(name = "clinicalstatus", path = "Condition.clinicalStatus", description = "The clinical status of the condition", type = "token") 1890 public static final String SP_CLINICALSTATUS = "clinicalstatus"; 1891 @SearchParamDefinition(name = "onset-info", path = "Condition.onset[x]", description = "Other onsets (boolean, age, range, string)", type = "string") 1892 public static final String SP_ONSETINFO = "onset-info"; 1893 @SearchParamDefinition(name = "code", path = "Condition.code", description = "Code for the condition", type = "token") 1894 public static final String SP_CODE = "code"; 1895 @SearchParamDefinition(name = "evidence", path = "Condition.evidence.code", description = "Manifestation/symptom", type = "token") 1896 public static final String SP_EVIDENCE = "evidence"; 1897 @SearchParamDefinition(name = "encounter", path = "Condition.encounter", description = "Encounter when condition first asserted", type = "reference") 1898 public static final String SP_ENCOUNTER = "encounter"; 1899 @SearchParamDefinition(name = "onset", path = "Condition.onset[x]", description = "Date related onsets (dateTime and Period)", type = "date") 1900 public static final String SP_ONSET = "onset"; 1901 @SearchParamDefinition(name = "asserter", path = "Condition.asserter", description = "Person who asserts this condition", type = "reference") 1902 public static final String SP_ASSERTER = "asserter"; 1903 @SearchParamDefinition(name = "date-recorded", path = "Condition.dateRecorded", description = "A date, when the Condition statement was documented", type = "date") 1904 public static final String SP_DATERECORDED = "date-recorded"; 1905 @SearchParamDefinition(name = "stage", path = "Condition.stage.summary", description = "Simple summary (disease specific)", type = "token") 1906 public static final String SP_STAGE = "stage"; 1907 @SearchParamDefinition(name = "patient", path = "Condition.patient", description = "Who has the condition?", type = "reference") 1908 public static final String SP_PATIENT = "patient"; 1909 @SearchParamDefinition(name = "category", path = "Condition.category", description = "The category of the condition", type = "token") 1910 public static final String SP_CATEGORY = "category"; 1911 @SearchParamDefinition(name = "body-site", path = "Condition.bodySite", description = "Anatomical location, if relevant", type = "token") 1912 public static final String SP_BODYSITE = "body-site"; 1913 1914}