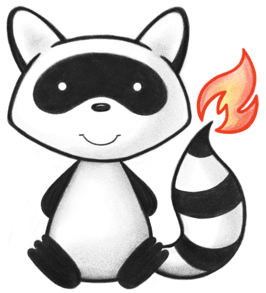
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus; 038import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory; 039import org.hl7.fhir.dstu2.model.Enumerations.SearchParamType; 040import org.hl7.fhir.dstu2.model.Enumerations.SearchParamTypeEnumFactory; 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 047import org.hl7.fhir.instance.model.api.IBaseConformance; 048import org.hl7.fhir.exceptions.FHIRException; 049import org.hl7.fhir.utilities.Utilities; 050 051/** 052 * A conformance statement is a set of capabilities of a FHIR Server that may be 053 * used as a statement of actual server functionality or a statement of required 054 * or desired server implementation. 055 */ 056@ResourceDef(name = "Conformance", profile = "http://hl7.org/fhir/Profile/Conformance") 057public class Conformance extends DomainResource implements IBaseConformance { 058 059 public enum ConformanceStatementKind { 060 /** 061 * The Conformance instance represents the present capabilities of a specific 062 * system instance. This is the kind returned by OPTIONS for a FHIR server 063 * end-point. 064 */ 065 INSTANCE, 066 /** 067 * The Conformance instance represents the capabilities of a system or piece of 068 * software, independent of a particular installation. 069 */ 070 CAPABILITY, 071 /** 072 * The Conformance instance represents a set of requirements for other systems 073 * to meet; e.g. as part of an implementation guide or 'request for proposal'. 074 */ 075 REQUIREMENTS, 076 /** 077 * added to help the parsers 078 */ 079 NULL; 080 081 public static ConformanceStatementKind fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("instance".equals(codeString)) 085 return INSTANCE; 086 if ("capability".equals(codeString)) 087 return CAPABILITY; 088 if ("requirements".equals(codeString)) 089 return REQUIREMENTS; 090 throw new FHIRException("Unknown ConformanceStatementKind code '" + codeString + "'"); 091 } 092 093 public String toCode() { 094 switch (this) { 095 case INSTANCE: 096 return "instance"; 097 case CAPABILITY: 098 return "capability"; 099 case REQUIREMENTS: 100 return "requirements"; 101 case NULL: 102 return null; 103 default: 104 return "?"; 105 } 106 } 107 108 public String getSystem() { 109 switch (this) { 110 case INSTANCE: 111 return "http://hl7.org/fhir/conformance-statement-kind"; 112 case CAPABILITY: 113 return "http://hl7.org/fhir/conformance-statement-kind"; 114 case REQUIREMENTS: 115 return "http://hl7.org/fhir/conformance-statement-kind"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getDefinition() { 124 switch (this) { 125 case INSTANCE: 126 return "The Conformance instance represents the present capabilities of a specific system instance. This is the kind returned by OPTIONS for a FHIR server end-point."; 127 case CAPABILITY: 128 return "The Conformance instance represents the capabilities of a system or piece of software, independent of a particular installation."; 129 case REQUIREMENTS: 130 return "The Conformance instance represents a set of requirements for other systems to meet; e.g. as part of an implementation guide or 'request for proposal'."; 131 case NULL: 132 return null; 133 default: 134 return "?"; 135 } 136 } 137 138 public String getDisplay() { 139 switch (this) { 140 case INSTANCE: 141 return "Instance"; 142 case CAPABILITY: 143 return "Capability"; 144 case REQUIREMENTS: 145 return "Requirements"; 146 case NULL: 147 return null; 148 default: 149 return "?"; 150 } 151 } 152 } 153 154 public static class ConformanceStatementKindEnumFactory implements EnumFactory<ConformanceStatementKind> { 155 public ConformanceStatementKind fromCode(String codeString) throws IllegalArgumentException { 156 if (codeString == null || "".equals(codeString)) 157 if (codeString == null || "".equals(codeString)) 158 return null; 159 if ("instance".equals(codeString)) 160 return ConformanceStatementKind.INSTANCE; 161 if ("capability".equals(codeString)) 162 return ConformanceStatementKind.CAPABILITY; 163 if ("requirements".equals(codeString)) 164 return ConformanceStatementKind.REQUIREMENTS; 165 throw new IllegalArgumentException("Unknown ConformanceStatementKind code '" + codeString + "'"); 166 } 167 168 public Enumeration<ConformanceStatementKind> fromType(Base code) throws FHIRException { 169 if (code == null || code.isEmpty()) 170 return null; 171 String codeString = ((PrimitiveType) code).asStringValue(); 172 if (codeString == null || "".equals(codeString)) 173 return null; 174 if ("instance".equals(codeString)) 175 return new Enumeration<ConformanceStatementKind>(this, ConformanceStatementKind.INSTANCE); 176 if ("capability".equals(codeString)) 177 return new Enumeration<ConformanceStatementKind>(this, ConformanceStatementKind.CAPABILITY); 178 if ("requirements".equals(codeString)) 179 return new Enumeration<ConformanceStatementKind>(this, ConformanceStatementKind.REQUIREMENTS); 180 throw new FHIRException("Unknown ConformanceStatementKind code '" + codeString + "'"); 181 } 182 183 public String toCode(ConformanceStatementKind code) 184 { 185 if (code == ConformanceStatementKind.NULL) 186 return null; 187 if (code == ConformanceStatementKind.INSTANCE) 188 return "instance"; 189 if (code == ConformanceStatementKind.CAPABILITY) 190 return "capability"; 191 if (code == ConformanceStatementKind.REQUIREMENTS) 192 return "requirements"; 193 return "?"; 194 } 195 } 196 197 public enum UnknownContentCode { 198 /** 199 * The application does not accept either unknown elements or extensions. 200 */ 201 NO, 202 /** 203 * The application accepts unknown extensions, but not unknown elements. 204 */ 205 EXTENSIONS, 206 /** 207 * The application accepts unknown elements, but not unknown extensions. 208 */ 209 ELEMENTS, 210 /** 211 * The application accepts unknown elements and extensions. 212 */ 213 BOTH, 214 /** 215 * added to help the parsers 216 */ 217 NULL; 218 219 public static UnknownContentCode fromCode(String codeString) throws FHIRException { 220 if (codeString == null || "".equals(codeString)) 221 return null; 222 if ("no".equals(codeString)) 223 return NO; 224 if ("extensions".equals(codeString)) 225 return EXTENSIONS; 226 if ("elements".equals(codeString)) 227 return ELEMENTS; 228 if ("both".equals(codeString)) 229 return BOTH; 230 throw new FHIRException("Unknown UnknownContentCode code '" + codeString + "'"); 231 } 232 233 public String toCode() { 234 switch (this) { 235 case NO: 236 return "no"; 237 case EXTENSIONS: 238 return "extensions"; 239 case ELEMENTS: 240 return "elements"; 241 case BOTH: 242 return "both"; 243 case NULL: 244 return null; 245 default: 246 return "?"; 247 } 248 } 249 250 public String getSystem() { 251 switch (this) { 252 case NO: 253 return "http://hl7.org/fhir/unknown-content-code"; 254 case EXTENSIONS: 255 return "http://hl7.org/fhir/unknown-content-code"; 256 case ELEMENTS: 257 return "http://hl7.org/fhir/unknown-content-code"; 258 case BOTH: 259 return "http://hl7.org/fhir/unknown-content-code"; 260 case NULL: 261 return null; 262 default: 263 return "?"; 264 } 265 } 266 267 public String getDefinition() { 268 switch (this) { 269 case NO: 270 return "The application does not accept either unknown elements or extensions."; 271 case EXTENSIONS: 272 return "The application accepts unknown extensions, but not unknown elements."; 273 case ELEMENTS: 274 return "The application accepts unknown elements, but not unknown extensions."; 275 case BOTH: 276 return "The application accepts unknown elements and extensions."; 277 case NULL: 278 return null; 279 default: 280 return "?"; 281 } 282 } 283 284 public String getDisplay() { 285 switch (this) { 286 case NO: 287 return "Neither Elements or Extensions"; 288 case EXTENSIONS: 289 return "Unknown Extensions"; 290 case ELEMENTS: 291 return "Unknown Elements"; 292 case BOTH: 293 return "Unknown Elements and Extensions"; 294 case NULL: 295 return null; 296 default: 297 return "?"; 298 } 299 } 300 } 301 302 public static class UnknownContentCodeEnumFactory implements EnumFactory<UnknownContentCode> { 303 public UnknownContentCode fromCode(String codeString) throws IllegalArgumentException { 304 if (codeString == null || "".equals(codeString)) 305 if (codeString == null || "".equals(codeString)) 306 return null; 307 if ("no".equals(codeString)) 308 return UnknownContentCode.NO; 309 if ("extensions".equals(codeString)) 310 return UnknownContentCode.EXTENSIONS; 311 if ("elements".equals(codeString)) 312 return UnknownContentCode.ELEMENTS; 313 if ("both".equals(codeString)) 314 return UnknownContentCode.BOTH; 315 throw new IllegalArgumentException("Unknown UnknownContentCode code '" + codeString + "'"); 316 } 317 318 public Enumeration<UnknownContentCode> fromType(Base code) throws FHIRException { 319 if (code == null || code.isEmpty()) 320 return null; 321 String codeString = ((PrimitiveType) code).asStringValue(); 322 if (codeString == null || "".equals(codeString)) 323 return null; 324 if ("no".equals(codeString)) 325 return new Enumeration<UnknownContentCode>(this, UnknownContentCode.NO); 326 if ("extensions".equals(codeString)) 327 return new Enumeration<UnknownContentCode>(this, UnknownContentCode.EXTENSIONS); 328 if ("elements".equals(codeString)) 329 return new Enumeration<UnknownContentCode>(this, UnknownContentCode.ELEMENTS); 330 if ("both".equals(codeString)) 331 return new Enumeration<UnknownContentCode>(this, UnknownContentCode.BOTH); 332 throw new FHIRException("Unknown UnknownContentCode code '" + codeString + "'"); 333 } 334 335 public String toCode(UnknownContentCode code) 336 { 337 if (code == UnknownContentCode.NULL) 338 return null; 339 if (code == UnknownContentCode.NO) 340 return "no"; 341 if (code == UnknownContentCode.EXTENSIONS) 342 return "extensions"; 343 if (code == UnknownContentCode.ELEMENTS) 344 return "elements"; 345 if (code == UnknownContentCode.BOTH) 346 return "both"; 347 return "?"; 348 } 349 } 350 351 public enum RestfulConformanceMode { 352 /** 353 * The application acts as a client for this resource. 354 */ 355 CLIENT, 356 /** 357 * The application acts as a server for this resource. 358 */ 359 SERVER, 360 /** 361 * added to help the parsers 362 */ 363 NULL; 364 365 public static RestfulConformanceMode fromCode(String codeString) throws FHIRException { 366 if (codeString == null || "".equals(codeString)) 367 return null; 368 if ("client".equals(codeString)) 369 return CLIENT; 370 if ("server".equals(codeString)) 371 return SERVER; 372 throw new FHIRException("Unknown RestfulConformanceMode code '" + codeString + "'"); 373 } 374 375 public String toCode() { 376 switch (this) { 377 case CLIENT: 378 return "client"; 379 case SERVER: 380 return "server"; 381 case NULL: 382 return null; 383 default: 384 return "?"; 385 } 386 } 387 388 public String getSystem() { 389 switch (this) { 390 case CLIENT: 391 return "http://hl7.org/fhir/restful-conformance-mode"; 392 case SERVER: 393 return "http://hl7.org/fhir/restful-conformance-mode"; 394 case NULL: 395 return null; 396 default: 397 return "?"; 398 } 399 } 400 401 public String getDefinition() { 402 switch (this) { 403 case CLIENT: 404 return "The application acts as a client for this resource."; 405 case SERVER: 406 return "The application acts as a server for this resource."; 407 case NULL: 408 return null; 409 default: 410 return "?"; 411 } 412 } 413 414 public String getDisplay() { 415 switch (this) { 416 case CLIENT: 417 return "Client"; 418 case SERVER: 419 return "Server"; 420 case NULL: 421 return null; 422 default: 423 return "?"; 424 } 425 } 426 } 427 428 public static class RestfulConformanceModeEnumFactory implements EnumFactory<RestfulConformanceMode> { 429 public RestfulConformanceMode fromCode(String codeString) throws IllegalArgumentException { 430 if (codeString == null || "".equals(codeString)) 431 if (codeString == null || "".equals(codeString)) 432 return null; 433 if ("client".equals(codeString)) 434 return RestfulConformanceMode.CLIENT; 435 if ("server".equals(codeString)) 436 return RestfulConformanceMode.SERVER; 437 throw new IllegalArgumentException("Unknown RestfulConformanceMode code '" + codeString + "'"); 438 } 439 440 public Enumeration<RestfulConformanceMode> fromType(Base code) throws FHIRException { 441 if (code == null || code.isEmpty()) 442 return null; 443 String codeString = ((PrimitiveType) code).asStringValue(); 444 if (codeString == null || "".equals(codeString)) 445 return null; 446 if ("client".equals(codeString)) 447 return new Enumeration<RestfulConformanceMode>(this, RestfulConformanceMode.CLIENT); 448 if ("server".equals(codeString)) 449 return new Enumeration<RestfulConformanceMode>(this, RestfulConformanceMode.SERVER); 450 throw new FHIRException("Unknown RestfulConformanceMode code '" + codeString + "'"); 451 } 452 453 public String toCode(RestfulConformanceMode code) 454 { 455 if (code == RestfulConformanceMode.NULL) 456 return null; 457 if (code == RestfulConformanceMode.CLIENT) 458 return "client"; 459 if (code == RestfulConformanceMode.SERVER) 460 return "server"; 461 return "?"; 462 } 463 } 464 465 public enum TypeRestfulInteraction { 466 /** 467 * null 468 */ 469 READ, 470 /** 471 * null 472 */ 473 VREAD, 474 /** 475 * null 476 */ 477 UPDATE, 478 /** 479 * null 480 */ 481 DELETE, 482 /** 483 * null 484 */ 485 HISTORYINSTANCE, 486 /** 487 * null 488 */ 489 VALIDATE, 490 /** 491 * null 492 */ 493 HISTORYTYPE, 494 /** 495 * null 496 */ 497 CREATE, 498 /** 499 * null 500 */ 501 SEARCHTYPE, 502 /** 503 * added to help the parsers 504 */ 505 NULL; 506 507 public static TypeRestfulInteraction fromCode(String codeString) throws FHIRException { 508 if (codeString == null || "".equals(codeString)) 509 return null; 510 if ("read".equals(codeString)) 511 return READ; 512 if ("vread".equals(codeString)) 513 return VREAD; 514 if ("update".equals(codeString)) 515 return UPDATE; 516 if ("delete".equals(codeString)) 517 return DELETE; 518 if ("history-instance".equals(codeString)) 519 return HISTORYINSTANCE; 520 if ("validate".equals(codeString)) 521 return VALIDATE; 522 if ("history-type".equals(codeString)) 523 return HISTORYTYPE; 524 if ("create".equals(codeString)) 525 return CREATE; 526 if ("search-type".equals(codeString)) 527 return SEARCHTYPE; 528 throw new FHIRException("Unknown TypeRestfulInteraction code '" + codeString + "'"); 529 } 530 531 public String toCode() { 532 switch (this) { 533 case READ: 534 return "read"; 535 case VREAD: 536 return "vread"; 537 case UPDATE: 538 return "update"; 539 case DELETE: 540 return "delete"; 541 case HISTORYINSTANCE: 542 return "history-instance"; 543 case VALIDATE: 544 return "validate"; 545 case HISTORYTYPE: 546 return "history-type"; 547 case CREATE: 548 return "create"; 549 case SEARCHTYPE: 550 return "search-type"; 551 case NULL: 552 return null; 553 default: 554 return "?"; 555 } 556 } 557 558 public String getSystem() { 559 switch (this) { 560 case READ: 561 return "http://hl7.org/fhir/restful-interaction"; 562 case VREAD: 563 return "http://hl7.org/fhir/restful-interaction"; 564 case UPDATE: 565 return "http://hl7.org/fhir/restful-interaction"; 566 case DELETE: 567 return "http://hl7.org/fhir/restful-interaction"; 568 case HISTORYINSTANCE: 569 return "http://hl7.org/fhir/restful-interaction"; 570 case VALIDATE: 571 return "http://hl7.org/fhir/restful-interaction"; 572 case HISTORYTYPE: 573 return "http://hl7.org/fhir/restful-interaction"; 574 case CREATE: 575 return "http://hl7.org/fhir/restful-interaction"; 576 case SEARCHTYPE: 577 return "http://hl7.org/fhir/restful-interaction"; 578 case NULL: 579 return null; 580 default: 581 return "?"; 582 } 583 } 584 585 public String getDefinition() { 586 switch (this) { 587 case READ: 588 return ""; 589 case VREAD: 590 return ""; 591 case UPDATE: 592 return ""; 593 case DELETE: 594 return ""; 595 case HISTORYINSTANCE: 596 return ""; 597 case VALIDATE: 598 return ""; 599 case HISTORYTYPE: 600 return ""; 601 case CREATE: 602 return ""; 603 case SEARCHTYPE: 604 return ""; 605 case NULL: 606 return null; 607 default: 608 return "?"; 609 } 610 } 611 612 public String getDisplay() { 613 switch (this) { 614 case READ: 615 return "read"; 616 case VREAD: 617 return "vread"; 618 case UPDATE: 619 return "update"; 620 case DELETE: 621 return "delete"; 622 case HISTORYINSTANCE: 623 return "history-instance"; 624 case VALIDATE: 625 return "validate"; 626 case HISTORYTYPE: 627 return "history-type"; 628 case CREATE: 629 return "create"; 630 case SEARCHTYPE: 631 return "search-type"; 632 case NULL: 633 return null; 634 default: 635 return "?"; 636 } 637 } 638 } 639 640 public static class TypeRestfulInteractionEnumFactory implements EnumFactory<TypeRestfulInteraction> { 641 public TypeRestfulInteraction fromCode(String codeString) throws IllegalArgumentException { 642 if (codeString == null || "".equals(codeString)) 643 if (codeString == null || "".equals(codeString)) 644 return null; 645 if ("read".equals(codeString)) 646 return TypeRestfulInteraction.READ; 647 if ("vread".equals(codeString)) 648 return TypeRestfulInteraction.VREAD; 649 if ("update".equals(codeString)) 650 return TypeRestfulInteraction.UPDATE; 651 if ("delete".equals(codeString)) 652 return TypeRestfulInteraction.DELETE; 653 if ("history-instance".equals(codeString)) 654 return TypeRestfulInteraction.HISTORYINSTANCE; 655 if ("validate".equals(codeString)) 656 return TypeRestfulInteraction.VALIDATE; 657 if ("history-type".equals(codeString)) 658 return TypeRestfulInteraction.HISTORYTYPE; 659 if ("create".equals(codeString)) 660 return TypeRestfulInteraction.CREATE; 661 if ("search-type".equals(codeString)) 662 return TypeRestfulInteraction.SEARCHTYPE; 663 throw new IllegalArgumentException("Unknown TypeRestfulInteraction code '" + codeString + "'"); 664 } 665 666 public Enumeration<TypeRestfulInteraction> fromType(Base code) throws FHIRException { 667 if (code == null || code.isEmpty()) 668 return null; 669 String codeString = ((PrimitiveType) code).asStringValue(); 670 if (codeString == null || "".equals(codeString)) 671 return null; 672 if ("read".equals(codeString)) 673 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.READ); 674 if ("vread".equals(codeString)) 675 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.VREAD); 676 if ("update".equals(codeString)) 677 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.UPDATE); 678 if ("delete".equals(codeString)) 679 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.DELETE); 680 if ("history-instance".equals(codeString)) 681 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.HISTORYINSTANCE); 682 if ("validate".equals(codeString)) 683 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.VALIDATE); 684 if ("history-type".equals(codeString)) 685 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.HISTORYTYPE); 686 if ("create".equals(codeString)) 687 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.CREATE); 688 if ("search-type".equals(codeString)) 689 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.SEARCHTYPE); 690 throw new FHIRException("Unknown TypeRestfulInteraction code '" + codeString + "'"); 691 } 692 693 public String toCode(TypeRestfulInteraction code) 694 { 695 if (code == TypeRestfulInteraction.NULL) 696 return null; 697 if (code == TypeRestfulInteraction.READ) 698 return "read"; 699 if (code == TypeRestfulInteraction.VREAD) 700 return "vread"; 701 if (code == TypeRestfulInteraction.UPDATE) 702 return "update"; 703 if (code == TypeRestfulInteraction.DELETE) 704 return "delete"; 705 if (code == TypeRestfulInteraction.HISTORYINSTANCE) 706 return "history-instance"; 707 if (code == TypeRestfulInteraction.VALIDATE) 708 return "validate"; 709 if (code == TypeRestfulInteraction.HISTORYTYPE) 710 return "history-type"; 711 if (code == TypeRestfulInteraction.CREATE) 712 return "create"; 713 if (code == TypeRestfulInteraction.SEARCHTYPE) 714 return "search-type"; 715 return "?"; 716 } 717 } 718 719 public enum ResourceVersionPolicy { 720 /** 721 * VersionId meta-property is not supported (server) or used (client). 722 */ 723 NOVERSION, 724 /** 725 * VersionId meta-property is supported (server) or used (client). 726 */ 727 VERSIONED, 728 /** 729 * VersionId is must be correct for updates (server) or will be specified 730 * (If-match header) for updates (client). 731 */ 732 VERSIONEDUPDATE, 733 /** 734 * added to help the parsers 735 */ 736 NULL; 737 738 public static ResourceVersionPolicy fromCode(String codeString) throws FHIRException { 739 if (codeString == null || "".equals(codeString)) 740 return null; 741 if ("no-version".equals(codeString)) 742 return NOVERSION; 743 if ("versioned".equals(codeString)) 744 return VERSIONED; 745 if ("versioned-update".equals(codeString)) 746 return VERSIONEDUPDATE; 747 throw new FHIRException("Unknown ResourceVersionPolicy code '" + codeString + "'"); 748 } 749 750 public String toCode() { 751 switch (this) { 752 case NOVERSION: 753 return "no-version"; 754 case VERSIONED: 755 return "versioned"; 756 case VERSIONEDUPDATE: 757 return "versioned-update"; 758 case NULL: 759 return null; 760 default: 761 return "?"; 762 } 763 } 764 765 public String getSystem() { 766 switch (this) { 767 case NOVERSION: 768 return "http://hl7.org/fhir/versioning-policy"; 769 case VERSIONED: 770 return "http://hl7.org/fhir/versioning-policy"; 771 case VERSIONEDUPDATE: 772 return "http://hl7.org/fhir/versioning-policy"; 773 case NULL: 774 return null; 775 default: 776 return "?"; 777 } 778 } 779 780 public String getDefinition() { 781 switch (this) { 782 case NOVERSION: 783 return "VersionId meta-property is not supported (server) or used (client)."; 784 case VERSIONED: 785 return "VersionId meta-property is supported (server) or used (client)."; 786 case VERSIONEDUPDATE: 787 return "VersionId is must be correct for updates (server) or will be specified (If-match header) for updates (client)."; 788 case NULL: 789 return null; 790 default: 791 return "?"; 792 } 793 } 794 795 public String getDisplay() { 796 switch (this) { 797 case NOVERSION: 798 return "No VersionId Support"; 799 case VERSIONED: 800 return "Versioned"; 801 case VERSIONEDUPDATE: 802 return "VersionId tracked fully"; 803 case NULL: 804 return null; 805 default: 806 return "?"; 807 } 808 } 809 } 810 811 public static class ResourceVersionPolicyEnumFactory implements EnumFactory<ResourceVersionPolicy> { 812 public ResourceVersionPolicy fromCode(String codeString) throws IllegalArgumentException { 813 if (codeString == null || "".equals(codeString)) 814 if (codeString == null || "".equals(codeString)) 815 return null; 816 if ("no-version".equals(codeString)) 817 return ResourceVersionPolicy.NOVERSION; 818 if ("versioned".equals(codeString)) 819 return ResourceVersionPolicy.VERSIONED; 820 if ("versioned-update".equals(codeString)) 821 return ResourceVersionPolicy.VERSIONEDUPDATE; 822 throw new IllegalArgumentException("Unknown ResourceVersionPolicy code '" + codeString + "'"); 823 } 824 825 public Enumeration<ResourceVersionPolicy> fromType(Base code) throws FHIRException { 826 if (code == null || code.isEmpty()) 827 return null; 828 String codeString = ((PrimitiveType) code).asStringValue(); 829 if (codeString == null || "".equals(codeString)) 830 return null; 831 if ("no-version".equals(codeString)) 832 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.NOVERSION); 833 if ("versioned".equals(codeString)) 834 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.VERSIONED); 835 if ("versioned-update".equals(codeString)) 836 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.VERSIONEDUPDATE); 837 throw new FHIRException("Unknown ResourceVersionPolicy code '" + codeString + "'"); 838 } 839 840 public String toCode(ResourceVersionPolicy code) 841 { 842 if (code == ResourceVersionPolicy.NULL) 843 return null; 844 if (code == ResourceVersionPolicy.NOVERSION) 845 return "no-version"; 846 if (code == ResourceVersionPolicy.VERSIONED) 847 return "versioned"; 848 if (code == ResourceVersionPolicy.VERSIONEDUPDATE) 849 return "versioned-update"; 850 return "?"; 851 } 852 } 853 854 public enum ConditionalDeleteStatus { 855 /** 856 * No support for conditional deletes. 857 */ 858 NOTSUPPORTED, 859 /** 860 * Conditional deletes are supported, but only single resources at a time. 861 */ 862 SINGLE, 863 /** 864 * Conditional deletes are supported, and multiple resources can be deleted in a 865 * single interaction. 866 */ 867 MULTIPLE, 868 /** 869 * added to help the parsers 870 */ 871 NULL; 872 873 public static ConditionalDeleteStatus fromCode(String codeString) throws FHIRException { 874 if (codeString == null || "".equals(codeString)) 875 return null; 876 if ("not-supported".equals(codeString)) 877 return NOTSUPPORTED; 878 if ("single".equals(codeString)) 879 return SINGLE; 880 if ("multiple".equals(codeString)) 881 return MULTIPLE; 882 throw new FHIRException("Unknown ConditionalDeleteStatus code '" + codeString + "'"); 883 } 884 885 public String toCode() { 886 switch (this) { 887 case NOTSUPPORTED: 888 return "not-supported"; 889 case SINGLE: 890 return "single"; 891 case MULTIPLE: 892 return "multiple"; 893 case NULL: 894 return null; 895 default: 896 return "?"; 897 } 898 } 899 900 public String getSystem() { 901 switch (this) { 902 case NOTSUPPORTED: 903 return "http://hl7.org/fhir/conditional-delete-status"; 904 case SINGLE: 905 return "http://hl7.org/fhir/conditional-delete-status"; 906 case MULTIPLE: 907 return "http://hl7.org/fhir/conditional-delete-status"; 908 case NULL: 909 return null; 910 default: 911 return "?"; 912 } 913 } 914 915 public String getDefinition() { 916 switch (this) { 917 case NOTSUPPORTED: 918 return "No support for conditional deletes."; 919 case SINGLE: 920 return "Conditional deletes are supported, but only single resources at a time."; 921 case MULTIPLE: 922 return "Conditional deletes are supported, and multiple resources can be deleted in a single interaction."; 923 case NULL: 924 return null; 925 default: 926 return "?"; 927 } 928 } 929 930 public String getDisplay() { 931 switch (this) { 932 case NOTSUPPORTED: 933 return "Not Supported"; 934 case SINGLE: 935 return "Single Deletes Supported"; 936 case MULTIPLE: 937 return "Multiple Deletes Supported"; 938 case NULL: 939 return null; 940 default: 941 return "?"; 942 } 943 } 944 } 945 946 public static class ConditionalDeleteStatusEnumFactory implements EnumFactory<ConditionalDeleteStatus> { 947 public ConditionalDeleteStatus fromCode(String codeString) throws IllegalArgumentException { 948 if (codeString == null || "".equals(codeString)) 949 if (codeString == null || "".equals(codeString)) 950 return null; 951 if ("not-supported".equals(codeString)) 952 return ConditionalDeleteStatus.NOTSUPPORTED; 953 if ("single".equals(codeString)) 954 return ConditionalDeleteStatus.SINGLE; 955 if ("multiple".equals(codeString)) 956 return ConditionalDeleteStatus.MULTIPLE; 957 throw new IllegalArgumentException("Unknown ConditionalDeleteStatus code '" + codeString + "'"); 958 } 959 960 public Enumeration<ConditionalDeleteStatus> fromType(Base code) throws FHIRException { 961 if (code == null || code.isEmpty()) 962 return null; 963 String codeString = ((PrimitiveType) code).asStringValue(); 964 if (codeString == null || "".equals(codeString)) 965 return null; 966 if ("not-supported".equals(codeString)) 967 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.NOTSUPPORTED); 968 if ("single".equals(codeString)) 969 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.SINGLE); 970 if ("multiple".equals(codeString)) 971 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.MULTIPLE); 972 throw new FHIRException("Unknown ConditionalDeleteStatus code '" + codeString + "'"); 973 } 974 975 public String toCode(ConditionalDeleteStatus code) 976 { 977 if (code == ConditionalDeleteStatus.NULL) 978 return null; 979 if (code == ConditionalDeleteStatus.NOTSUPPORTED) 980 return "not-supported"; 981 if (code == ConditionalDeleteStatus.SINGLE) 982 return "single"; 983 if (code == ConditionalDeleteStatus.MULTIPLE) 984 return "multiple"; 985 return "?"; 986 } 987 } 988 989 public enum SearchModifierCode { 990 /** 991 * The search parameter returns resources that have a value or not. 992 */ 993 MISSING, 994 /** 995 * The search parameter returns resources that have a value that exactly matches 996 * the supplied parameter (the whole string, including casing and accents). 997 */ 998 EXACT, 999 /** 1000 * The search parameter returns resources that include the supplied parameter 1001 * value anywhere within the field being searched. 1002 */ 1003 CONTAINS, 1004 /** 1005 * The search parameter returns resources that do not contain a match . 1006 */ 1007 NOT, 1008 /** 1009 * The search parameter is processed as a string that searches text associated 1010 * with the code/value - either CodeableConcept.text, Coding.display, or 1011 * Identifier.type.text. 1012 */ 1013 TEXT, 1014 /** 1015 * The search parameter is a URI (relative or absolute) that identifies a value 1016 * set, and the search parameter tests whether the coding is in the specified 1017 * value set. 1018 */ 1019 IN, 1020 /** 1021 * The search parameter is a URI (relative or absolute) that identifies a value 1022 * set, and the search parameter tests whether the coding is not in the 1023 * specified value set. 1024 */ 1025 NOTIN, 1026 /** 1027 * The search parameter tests whether the value in a resource is subsumed by the 1028 * specified value (is-a, or hierarchical relationships). 1029 */ 1030 BELOW, 1031 /** 1032 * The search parameter tests whether the value in a resource subsumes the 1033 * specified value (is-a, or hierarchical relationships). 1034 */ 1035 ABOVE, 1036 /** 1037 * The search parameter only applies to the Resource Type specified as a 1038 * modifier (e.g. the modifier is not actually :type, but :Patient etc.). 1039 */ 1040 TYPE, 1041 /** 1042 * added to help the parsers 1043 */ 1044 NULL; 1045 1046 public static SearchModifierCode fromCode(String codeString) throws FHIRException { 1047 if (codeString == null || "".equals(codeString)) 1048 return null; 1049 if ("missing".equals(codeString)) 1050 return MISSING; 1051 if ("exact".equals(codeString)) 1052 return EXACT; 1053 if ("contains".equals(codeString)) 1054 return CONTAINS; 1055 if ("not".equals(codeString)) 1056 return NOT; 1057 if ("text".equals(codeString)) 1058 return TEXT; 1059 if ("in".equals(codeString)) 1060 return IN; 1061 if ("not-in".equals(codeString)) 1062 return NOTIN; 1063 if ("below".equals(codeString)) 1064 return BELOW; 1065 if ("above".equals(codeString)) 1066 return ABOVE; 1067 if ("type".equals(codeString)) 1068 return TYPE; 1069 throw new FHIRException("Unknown SearchModifierCode code '" + codeString + "'"); 1070 } 1071 1072 public String toCode() { 1073 switch (this) { 1074 case MISSING: 1075 return "missing"; 1076 case EXACT: 1077 return "exact"; 1078 case CONTAINS: 1079 return "contains"; 1080 case NOT: 1081 return "not"; 1082 case TEXT: 1083 return "text"; 1084 case IN: 1085 return "in"; 1086 case NOTIN: 1087 return "not-in"; 1088 case BELOW: 1089 return "below"; 1090 case ABOVE: 1091 return "above"; 1092 case TYPE: 1093 return "type"; 1094 case NULL: 1095 return null; 1096 default: 1097 return "?"; 1098 } 1099 } 1100 1101 public String getSystem() { 1102 switch (this) { 1103 case MISSING: 1104 return "http://hl7.org/fhir/search-modifier-code"; 1105 case EXACT: 1106 return "http://hl7.org/fhir/search-modifier-code"; 1107 case CONTAINS: 1108 return "http://hl7.org/fhir/search-modifier-code"; 1109 case NOT: 1110 return "http://hl7.org/fhir/search-modifier-code"; 1111 case TEXT: 1112 return "http://hl7.org/fhir/search-modifier-code"; 1113 case IN: 1114 return "http://hl7.org/fhir/search-modifier-code"; 1115 case NOTIN: 1116 return "http://hl7.org/fhir/search-modifier-code"; 1117 case BELOW: 1118 return "http://hl7.org/fhir/search-modifier-code"; 1119 case ABOVE: 1120 return "http://hl7.org/fhir/search-modifier-code"; 1121 case TYPE: 1122 return "http://hl7.org/fhir/search-modifier-code"; 1123 case NULL: 1124 return null; 1125 default: 1126 return "?"; 1127 } 1128 } 1129 1130 public String getDefinition() { 1131 switch (this) { 1132 case MISSING: 1133 return "The search parameter returns resources that have a value or not."; 1134 case EXACT: 1135 return "The search parameter returns resources that have a value that exactly matches the supplied parameter (the whole string, including casing and accents)."; 1136 case CONTAINS: 1137 return "The search parameter returns resources that include the supplied parameter value anywhere within the field being searched."; 1138 case NOT: 1139 return "The search parameter returns resources that do not contain a match ."; 1140 case TEXT: 1141 return "The search parameter is processed as a string that searches text associated with the code/value - either CodeableConcept.text, Coding.display, or Identifier.type.text."; 1142 case IN: 1143 return "The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is in the specified value set."; 1144 case NOTIN: 1145 return "The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is not in the specified value set."; 1146 case BELOW: 1147 return "The search parameter tests whether the value in a resource is subsumed by the specified value (is-a, or hierarchical relationships)."; 1148 case ABOVE: 1149 return "The search parameter tests whether the value in a resource subsumes the specified value (is-a, or hierarchical relationships)."; 1150 case TYPE: 1151 return "The search parameter only applies to the Resource Type specified as a modifier (e.g. the modifier is not actually :type, but :Patient etc.)."; 1152 case NULL: 1153 return null; 1154 default: 1155 return "?"; 1156 } 1157 } 1158 1159 public String getDisplay() { 1160 switch (this) { 1161 case MISSING: 1162 return "Missing"; 1163 case EXACT: 1164 return "Exact"; 1165 case CONTAINS: 1166 return "Contains"; 1167 case NOT: 1168 return "Not"; 1169 case TEXT: 1170 return "Text"; 1171 case IN: 1172 return "In"; 1173 case NOTIN: 1174 return "Not In"; 1175 case BELOW: 1176 return "Below"; 1177 case ABOVE: 1178 return "Above"; 1179 case TYPE: 1180 return "Type"; 1181 case NULL: 1182 return null; 1183 default: 1184 return "?"; 1185 } 1186 } 1187 } 1188 1189 public static class SearchModifierCodeEnumFactory implements EnumFactory<SearchModifierCode> { 1190 public SearchModifierCode fromCode(String codeString) throws IllegalArgumentException { 1191 if (codeString == null || "".equals(codeString)) 1192 if (codeString == null || "".equals(codeString)) 1193 return null; 1194 if ("missing".equals(codeString)) 1195 return SearchModifierCode.MISSING; 1196 if ("exact".equals(codeString)) 1197 return SearchModifierCode.EXACT; 1198 if ("contains".equals(codeString)) 1199 return SearchModifierCode.CONTAINS; 1200 if ("not".equals(codeString)) 1201 return SearchModifierCode.NOT; 1202 if ("text".equals(codeString)) 1203 return SearchModifierCode.TEXT; 1204 if ("in".equals(codeString)) 1205 return SearchModifierCode.IN; 1206 if ("not-in".equals(codeString)) 1207 return SearchModifierCode.NOTIN; 1208 if ("below".equals(codeString)) 1209 return SearchModifierCode.BELOW; 1210 if ("above".equals(codeString)) 1211 return SearchModifierCode.ABOVE; 1212 if ("type".equals(codeString)) 1213 return SearchModifierCode.TYPE; 1214 throw new IllegalArgumentException("Unknown SearchModifierCode code '" + codeString + "'"); 1215 } 1216 1217 public Enumeration<SearchModifierCode> fromType(Base code) throws FHIRException { 1218 if (code == null || code.isEmpty()) 1219 return null; 1220 String codeString = ((PrimitiveType) code).asStringValue(); 1221 if (codeString == null || "".equals(codeString)) 1222 return null; 1223 if ("missing".equals(codeString)) 1224 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.MISSING); 1225 if ("exact".equals(codeString)) 1226 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.EXACT); 1227 if ("contains".equals(codeString)) 1228 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.CONTAINS); 1229 if ("not".equals(codeString)) 1230 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.NOT); 1231 if ("text".equals(codeString)) 1232 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.TEXT); 1233 if ("in".equals(codeString)) 1234 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.IN); 1235 if ("not-in".equals(codeString)) 1236 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.NOTIN); 1237 if ("below".equals(codeString)) 1238 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.BELOW); 1239 if ("above".equals(codeString)) 1240 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.ABOVE); 1241 if ("type".equals(codeString)) 1242 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.TYPE); 1243 throw new FHIRException("Unknown SearchModifierCode code '" + codeString + "'"); 1244 } 1245 1246 public String toCode(SearchModifierCode code) 1247 { 1248 if (code == SearchModifierCode.NULL) 1249 return null; 1250 if (code == SearchModifierCode.MISSING) 1251 return "missing"; 1252 if (code == SearchModifierCode.EXACT) 1253 return "exact"; 1254 if (code == SearchModifierCode.CONTAINS) 1255 return "contains"; 1256 if (code == SearchModifierCode.NOT) 1257 return "not"; 1258 if (code == SearchModifierCode.TEXT) 1259 return "text"; 1260 if (code == SearchModifierCode.IN) 1261 return "in"; 1262 if (code == SearchModifierCode.NOTIN) 1263 return "not-in"; 1264 if (code == SearchModifierCode.BELOW) 1265 return "below"; 1266 if (code == SearchModifierCode.ABOVE) 1267 return "above"; 1268 if (code == SearchModifierCode.TYPE) 1269 return "type"; 1270 return "?"; 1271 } 1272 } 1273 1274 public enum SystemRestfulInteraction { 1275 /** 1276 * null 1277 */ 1278 TRANSACTION, 1279 /** 1280 * null 1281 */ 1282 SEARCHSYSTEM, 1283 /** 1284 * null 1285 */ 1286 HISTORYSYSTEM, 1287 /** 1288 * added to help the parsers 1289 */ 1290 NULL; 1291 1292 public static SystemRestfulInteraction fromCode(String codeString) throws FHIRException { 1293 if (codeString == null || "".equals(codeString)) 1294 return null; 1295 if ("transaction".equals(codeString)) 1296 return TRANSACTION; 1297 if ("search-system".equals(codeString)) 1298 return SEARCHSYSTEM; 1299 if ("history-system".equals(codeString)) 1300 return HISTORYSYSTEM; 1301 throw new FHIRException("Unknown SystemRestfulInteraction code '" + codeString + "'"); 1302 } 1303 1304 public String toCode() { 1305 switch (this) { 1306 case TRANSACTION: 1307 return "transaction"; 1308 case SEARCHSYSTEM: 1309 return "search-system"; 1310 case HISTORYSYSTEM: 1311 return "history-system"; 1312 case NULL: 1313 return null; 1314 default: 1315 return "?"; 1316 } 1317 } 1318 1319 public String getSystem() { 1320 switch (this) { 1321 case TRANSACTION: 1322 return "http://hl7.org/fhir/restful-interaction"; 1323 case SEARCHSYSTEM: 1324 return "http://hl7.org/fhir/restful-interaction"; 1325 case HISTORYSYSTEM: 1326 return "http://hl7.org/fhir/restful-interaction"; 1327 case NULL: 1328 return null; 1329 default: 1330 return "?"; 1331 } 1332 } 1333 1334 public String getDefinition() { 1335 switch (this) { 1336 case TRANSACTION: 1337 return ""; 1338 case SEARCHSYSTEM: 1339 return ""; 1340 case HISTORYSYSTEM: 1341 return ""; 1342 case NULL: 1343 return null; 1344 default: 1345 return "?"; 1346 } 1347 } 1348 1349 public String getDisplay() { 1350 switch (this) { 1351 case TRANSACTION: 1352 return "transaction"; 1353 case SEARCHSYSTEM: 1354 return "search-system"; 1355 case HISTORYSYSTEM: 1356 return "history-system"; 1357 case NULL: 1358 return null; 1359 default: 1360 return "?"; 1361 } 1362 } 1363 } 1364 1365 public static class SystemRestfulInteractionEnumFactory implements EnumFactory<SystemRestfulInteraction> { 1366 public SystemRestfulInteraction fromCode(String codeString) throws IllegalArgumentException { 1367 if (codeString == null || "".equals(codeString)) 1368 if (codeString == null || "".equals(codeString)) 1369 return null; 1370 if ("transaction".equals(codeString)) 1371 return SystemRestfulInteraction.TRANSACTION; 1372 if ("search-system".equals(codeString)) 1373 return SystemRestfulInteraction.SEARCHSYSTEM; 1374 if ("history-system".equals(codeString)) 1375 return SystemRestfulInteraction.HISTORYSYSTEM; 1376 throw new IllegalArgumentException("Unknown SystemRestfulInteraction code '" + codeString + "'"); 1377 } 1378 1379 public Enumeration<SystemRestfulInteraction> fromType(Base code) throws FHIRException { 1380 if (code == null || code.isEmpty()) 1381 return null; 1382 String codeString = ((PrimitiveType) code).asStringValue(); 1383 if (codeString == null || "".equals(codeString)) 1384 return null; 1385 if ("transaction".equals(codeString)) 1386 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.TRANSACTION); 1387 if ("search-system".equals(codeString)) 1388 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.SEARCHSYSTEM); 1389 if ("history-system".equals(codeString)) 1390 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.HISTORYSYSTEM); 1391 throw new FHIRException("Unknown SystemRestfulInteraction code '" + codeString + "'"); 1392 } 1393 1394 public String toCode(SystemRestfulInteraction code) 1395 { 1396 if (code == SystemRestfulInteraction.NULL) 1397 return null; 1398 if (code == SystemRestfulInteraction.TRANSACTION) 1399 return "transaction"; 1400 if (code == SystemRestfulInteraction.SEARCHSYSTEM) 1401 return "search-system"; 1402 if (code == SystemRestfulInteraction.HISTORYSYSTEM) 1403 return "history-system"; 1404 return "?"; 1405 } 1406 } 1407 1408 public enum TransactionMode { 1409 /** 1410 * Neither batch or transaction is supported. 1411 */ 1412 NOTSUPPORTED, 1413 /** 1414 * Batches are supported. 1415 */ 1416 BATCH, 1417 /** 1418 * Transactions are supported. 1419 */ 1420 TRANSACTION, 1421 /** 1422 * Both batches and transactions are supported. 1423 */ 1424 BOTH, 1425 /** 1426 * added to help the parsers 1427 */ 1428 NULL; 1429 1430 public static TransactionMode fromCode(String codeString) throws FHIRException { 1431 if (codeString == null || "".equals(codeString)) 1432 return null; 1433 if ("not-supported".equals(codeString)) 1434 return NOTSUPPORTED; 1435 if ("batch".equals(codeString)) 1436 return BATCH; 1437 if ("transaction".equals(codeString)) 1438 return TRANSACTION; 1439 if ("both".equals(codeString)) 1440 return BOTH; 1441 throw new FHIRException("Unknown TransactionMode code '" + codeString + "'"); 1442 } 1443 1444 public String toCode() { 1445 switch (this) { 1446 case NOTSUPPORTED: 1447 return "not-supported"; 1448 case BATCH: 1449 return "batch"; 1450 case TRANSACTION: 1451 return "transaction"; 1452 case BOTH: 1453 return "both"; 1454 case NULL: 1455 return null; 1456 default: 1457 return "?"; 1458 } 1459 } 1460 1461 public String getSystem() { 1462 switch (this) { 1463 case NOTSUPPORTED: 1464 return "http://hl7.org/fhir/transaction-mode"; 1465 case BATCH: 1466 return "http://hl7.org/fhir/transaction-mode"; 1467 case TRANSACTION: 1468 return "http://hl7.org/fhir/transaction-mode"; 1469 case BOTH: 1470 return "http://hl7.org/fhir/transaction-mode"; 1471 case NULL: 1472 return null; 1473 default: 1474 return "?"; 1475 } 1476 } 1477 1478 public String getDefinition() { 1479 switch (this) { 1480 case NOTSUPPORTED: 1481 return "Neither batch or transaction is supported."; 1482 case BATCH: 1483 return "Batches are supported."; 1484 case TRANSACTION: 1485 return "Transactions are supported."; 1486 case BOTH: 1487 return "Both batches and transactions are supported."; 1488 case NULL: 1489 return null; 1490 default: 1491 return "?"; 1492 } 1493 } 1494 1495 public String getDisplay() { 1496 switch (this) { 1497 case NOTSUPPORTED: 1498 return "None"; 1499 case BATCH: 1500 return "Batches supported"; 1501 case TRANSACTION: 1502 return "Transactions Supported"; 1503 case BOTH: 1504 return "Batches & Transactions"; 1505 case NULL: 1506 return null; 1507 default: 1508 return "?"; 1509 } 1510 } 1511 } 1512 1513 public static class TransactionModeEnumFactory implements EnumFactory<TransactionMode> { 1514 public TransactionMode fromCode(String codeString) throws IllegalArgumentException { 1515 if (codeString == null || "".equals(codeString)) 1516 if (codeString == null || "".equals(codeString)) 1517 return null; 1518 if ("not-supported".equals(codeString)) 1519 return TransactionMode.NOTSUPPORTED; 1520 if ("batch".equals(codeString)) 1521 return TransactionMode.BATCH; 1522 if ("transaction".equals(codeString)) 1523 return TransactionMode.TRANSACTION; 1524 if ("both".equals(codeString)) 1525 return TransactionMode.BOTH; 1526 throw new IllegalArgumentException("Unknown TransactionMode code '" + codeString + "'"); 1527 } 1528 1529 public Enumeration<TransactionMode> fromType(Base code) throws FHIRException { 1530 if (code == null || code.isEmpty()) 1531 return null; 1532 String codeString = ((PrimitiveType) code).asStringValue(); 1533 if (codeString == null || "".equals(codeString)) 1534 return null; 1535 if ("not-supported".equals(codeString)) 1536 return new Enumeration<TransactionMode>(this, TransactionMode.NOTSUPPORTED); 1537 if ("batch".equals(codeString)) 1538 return new Enumeration<TransactionMode>(this, TransactionMode.BATCH); 1539 if ("transaction".equals(codeString)) 1540 return new Enumeration<TransactionMode>(this, TransactionMode.TRANSACTION); 1541 if ("both".equals(codeString)) 1542 return new Enumeration<TransactionMode>(this, TransactionMode.BOTH); 1543 throw new FHIRException("Unknown TransactionMode code '" + codeString + "'"); 1544 } 1545 1546 public String toCode(TransactionMode code) 1547 { 1548 if (code == TransactionMode.NULL) 1549 return null; 1550 if (code == TransactionMode.NOTSUPPORTED) 1551 return "not-supported"; 1552 if (code == TransactionMode.BATCH) 1553 return "batch"; 1554 if (code == TransactionMode.TRANSACTION) 1555 return "transaction"; 1556 if (code == TransactionMode.BOTH) 1557 return "both"; 1558 return "?"; 1559 } 1560 } 1561 1562 public enum MessageSignificanceCategory { 1563 /** 1564 * The message represents/requests a change that should not be processed more 1565 * than once; e.g. Making a booking for an appointment. 1566 */ 1567 CONSEQUENCE, 1568 /** 1569 * The message represents a response to query for current information. 1570 * Retrospective processing is wrong and/or wasteful. 1571 */ 1572 CURRENCY, 1573 /** 1574 * The content is not necessarily intended to be current, and it can be 1575 * reprocessed, though there may be version issues created by processing old 1576 * notifications. 1577 */ 1578 NOTIFICATION, 1579 /** 1580 * added to help the parsers 1581 */ 1582 NULL; 1583 1584 public static MessageSignificanceCategory fromCode(String codeString) throws FHIRException { 1585 if (codeString == null || "".equals(codeString)) 1586 return null; 1587 if ("Consequence".equals(codeString)) 1588 return CONSEQUENCE; 1589 if ("Currency".equals(codeString)) 1590 return CURRENCY; 1591 if ("Notification".equals(codeString)) 1592 return NOTIFICATION; 1593 throw new FHIRException("Unknown MessageSignificanceCategory code '" + codeString + "'"); 1594 } 1595 1596 public String toCode() { 1597 switch (this) { 1598 case CONSEQUENCE: 1599 return "Consequence"; 1600 case CURRENCY: 1601 return "Currency"; 1602 case NOTIFICATION: 1603 return "Notification"; 1604 case NULL: 1605 return null; 1606 default: 1607 return "?"; 1608 } 1609 } 1610 1611 public String getSystem() { 1612 switch (this) { 1613 case CONSEQUENCE: 1614 return "http://hl7.org/fhir/message-significance-category"; 1615 case CURRENCY: 1616 return "http://hl7.org/fhir/message-significance-category"; 1617 case NOTIFICATION: 1618 return "http://hl7.org/fhir/message-significance-category"; 1619 case NULL: 1620 return null; 1621 default: 1622 return "?"; 1623 } 1624 } 1625 1626 public String getDefinition() { 1627 switch (this) { 1628 case CONSEQUENCE: 1629 return "The message represents/requests a change that should not be processed more than once; e.g. Making a booking for an appointment."; 1630 case CURRENCY: 1631 return "The message represents a response to query for current information. Retrospective processing is wrong and/or wasteful."; 1632 case NOTIFICATION: 1633 return "The content is not necessarily intended to be current, and it can be reprocessed, though there may be version issues created by processing old notifications."; 1634 case NULL: 1635 return null; 1636 default: 1637 return "?"; 1638 } 1639 } 1640 1641 public String getDisplay() { 1642 switch (this) { 1643 case CONSEQUENCE: 1644 return "Consequence"; 1645 case CURRENCY: 1646 return "Currency"; 1647 case NOTIFICATION: 1648 return "Notification"; 1649 case NULL: 1650 return null; 1651 default: 1652 return "?"; 1653 } 1654 } 1655 } 1656 1657 public static class MessageSignificanceCategoryEnumFactory implements EnumFactory<MessageSignificanceCategory> { 1658 public MessageSignificanceCategory fromCode(String codeString) throws IllegalArgumentException { 1659 if (codeString == null || "".equals(codeString)) 1660 if (codeString == null || "".equals(codeString)) 1661 return null; 1662 if ("Consequence".equals(codeString)) 1663 return MessageSignificanceCategory.CONSEQUENCE; 1664 if ("Currency".equals(codeString)) 1665 return MessageSignificanceCategory.CURRENCY; 1666 if ("Notification".equals(codeString)) 1667 return MessageSignificanceCategory.NOTIFICATION; 1668 throw new IllegalArgumentException("Unknown MessageSignificanceCategory code '" + codeString + "'"); 1669 } 1670 1671 public Enumeration<MessageSignificanceCategory> fromType(Base code) throws FHIRException { 1672 if (code == null || code.isEmpty()) 1673 return null; 1674 String codeString = ((PrimitiveType) code).asStringValue(); 1675 if (codeString == null || "".equals(codeString)) 1676 return null; 1677 if ("Consequence".equals(codeString)) 1678 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.CONSEQUENCE); 1679 if ("Currency".equals(codeString)) 1680 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.CURRENCY); 1681 if ("Notification".equals(codeString)) 1682 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.NOTIFICATION); 1683 throw new FHIRException("Unknown MessageSignificanceCategory code '" + codeString + "'"); 1684 } 1685 1686 public String toCode(MessageSignificanceCategory code) 1687 { 1688 if (code == MessageSignificanceCategory.NULL) 1689 return null; 1690 if (code == MessageSignificanceCategory.CONSEQUENCE) 1691 return "Consequence"; 1692 if (code == MessageSignificanceCategory.CURRENCY) 1693 return "Currency"; 1694 if (code == MessageSignificanceCategory.NOTIFICATION) 1695 return "Notification"; 1696 return "?"; 1697 } 1698 } 1699 1700 public enum ConformanceEventMode { 1701 /** 1702 * The application sends requests and receives responses. 1703 */ 1704 SENDER, 1705 /** 1706 * The application receives requests and sends responses. 1707 */ 1708 RECEIVER, 1709 /** 1710 * added to help the parsers 1711 */ 1712 NULL; 1713 1714 public static ConformanceEventMode fromCode(String codeString) throws FHIRException { 1715 if (codeString == null || "".equals(codeString)) 1716 return null; 1717 if ("sender".equals(codeString)) 1718 return SENDER; 1719 if ("receiver".equals(codeString)) 1720 return RECEIVER; 1721 throw new FHIRException("Unknown ConformanceEventMode code '" + codeString + "'"); 1722 } 1723 1724 public String toCode() { 1725 switch (this) { 1726 case SENDER: 1727 return "sender"; 1728 case RECEIVER: 1729 return "receiver"; 1730 case NULL: 1731 return null; 1732 default: 1733 return "?"; 1734 } 1735 } 1736 1737 public String getSystem() { 1738 switch (this) { 1739 case SENDER: 1740 return "http://hl7.org/fhir/message-conformance-event-mode"; 1741 case RECEIVER: 1742 return "http://hl7.org/fhir/message-conformance-event-mode"; 1743 case NULL: 1744 return null; 1745 default: 1746 return "?"; 1747 } 1748 } 1749 1750 public String getDefinition() { 1751 switch (this) { 1752 case SENDER: 1753 return "The application sends requests and receives responses."; 1754 case RECEIVER: 1755 return "The application receives requests and sends responses."; 1756 case NULL: 1757 return null; 1758 default: 1759 return "?"; 1760 } 1761 } 1762 1763 public String getDisplay() { 1764 switch (this) { 1765 case SENDER: 1766 return "Sender"; 1767 case RECEIVER: 1768 return "Receiver"; 1769 case NULL: 1770 return null; 1771 default: 1772 return "?"; 1773 } 1774 } 1775 } 1776 1777 public static class ConformanceEventModeEnumFactory implements EnumFactory<ConformanceEventMode> { 1778 public ConformanceEventMode fromCode(String codeString) throws IllegalArgumentException { 1779 if (codeString == null || "".equals(codeString)) 1780 if (codeString == null || "".equals(codeString)) 1781 return null; 1782 if ("sender".equals(codeString)) 1783 return ConformanceEventMode.SENDER; 1784 if ("receiver".equals(codeString)) 1785 return ConformanceEventMode.RECEIVER; 1786 throw new IllegalArgumentException("Unknown ConformanceEventMode code '" + codeString + "'"); 1787 } 1788 1789 public Enumeration<ConformanceEventMode> fromType(Base code) throws FHIRException { 1790 if (code == null || code.isEmpty()) 1791 return null; 1792 String codeString = ((PrimitiveType) code).asStringValue(); 1793 if (codeString == null || "".equals(codeString)) 1794 return null; 1795 if ("sender".equals(codeString)) 1796 return new Enumeration<ConformanceEventMode>(this, ConformanceEventMode.SENDER); 1797 if ("receiver".equals(codeString)) 1798 return new Enumeration<ConformanceEventMode>(this, ConformanceEventMode.RECEIVER); 1799 throw new FHIRException("Unknown ConformanceEventMode code '" + codeString + "'"); 1800 } 1801 1802 public String toCode(ConformanceEventMode code) 1803 { 1804 if (code == ConformanceEventMode.NULL) 1805 return null; 1806 if (code == ConformanceEventMode.SENDER) 1807 return "sender"; 1808 if (code == ConformanceEventMode.RECEIVER) 1809 return "receiver"; 1810 return "?"; 1811 } 1812 } 1813 1814 public enum DocumentMode { 1815 /** 1816 * The application produces documents of the specified type. 1817 */ 1818 PRODUCER, 1819 /** 1820 * The application consumes documents of the specified type. 1821 */ 1822 CONSUMER, 1823 /** 1824 * added to help the parsers 1825 */ 1826 NULL; 1827 1828 public static DocumentMode fromCode(String codeString) throws FHIRException { 1829 if (codeString == null || "".equals(codeString)) 1830 return null; 1831 if ("producer".equals(codeString)) 1832 return PRODUCER; 1833 if ("consumer".equals(codeString)) 1834 return CONSUMER; 1835 throw new FHIRException("Unknown DocumentMode code '" + codeString + "'"); 1836 } 1837 1838 public String toCode() { 1839 switch (this) { 1840 case PRODUCER: 1841 return "producer"; 1842 case CONSUMER: 1843 return "consumer"; 1844 case NULL: 1845 return null; 1846 default: 1847 return "?"; 1848 } 1849 } 1850 1851 public String getSystem() { 1852 switch (this) { 1853 case PRODUCER: 1854 return "http://hl7.org/fhir/document-mode"; 1855 case CONSUMER: 1856 return "http://hl7.org/fhir/document-mode"; 1857 case NULL: 1858 return null; 1859 default: 1860 return "?"; 1861 } 1862 } 1863 1864 public String getDefinition() { 1865 switch (this) { 1866 case PRODUCER: 1867 return "The application produces documents of the specified type."; 1868 case CONSUMER: 1869 return "The application consumes documents of the specified type."; 1870 case NULL: 1871 return null; 1872 default: 1873 return "?"; 1874 } 1875 } 1876 1877 public String getDisplay() { 1878 switch (this) { 1879 case PRODUCER: 1880 return "Producer"; 1881 case CONSUMER: 1882 return "Consumer"; 1883 case NULL: 1884 return null; 1885 default: 1886 return "?"; 1887 } 1888 } 1889 } 1890 1891 public static class DocumentModeEnumFactory implements EnumFactory<DocumentMode> { 1892 public DocumentMode fromCode(String codeString) throws IllegalArgumentException { 1893 if (codeString == null || "".equals(codeString)) 1894 if (codeString == null || "".equals(codeString)) 1895 return null; 1896 if ("producer".equals(codeString)) 1897 return DocumentMode.PRODUCER; 1898 if ("consumer".equals(codeString)) 1899 return DocumentMode.CONSUMER; 1900 throw new IllegalArgumentException("Unknown DocumentMode code '" + codeString + "'"); 1901 } 1902 1903 public Enumeration<DocumentMode> fromType(Base code) throws FHIRException { 1904 if (code == null || code.isEmpty()) 1905 return null; 1906 String codeString = ((PrimitiveType) code).asStringValue(); 1907 if (codeString == null || "".equals(codeString)) 1908 return null; 1909 if ("producer".equals(codeString)) 1910 return new Enumeration<DocumentMode>(this, DocumentMode.PRODUCER); 1911 if ("consumer".equals(codeString)) 1912 return new Enumeration<DocumentMode>(this, DocumentMode.CONSUMER); 1913 throw new FHIRException("Unknown DocumentMode code '" + codeString + "'"); 1914 } 1915 1916 public String toCode(DocumentMode code) 1917 { 1918 if (code == DocumentMode.NULL) 1919 return null; 1920 if (code == DocumentMode.PRODUCER) 1921 return "producer"; 1922 if (code == DocumentMode.CONSUMER) 1923 return "consumer"; 1924 return "?"; 1925 } 1926 } 1927 1928 @Block() 1929 public static class ConformanceContactComponent extends BackboneElement implements IBaseBackboneElement { 1930 /** 1931 * The name of an individual to contact regarding the conformance. 1932 */ 1933 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1934 @Description(shortDefinition = "Name of a individual to contact", formalDefinition = "The name of an individual to contact regarding the conformance.") 1935 protected StringType name; 1936 1937 /** 1938 * Contact details for individual (if a name was provided) or the publisher. 1939 */ 1940 @Child(name = "telecom", type = { 1941 ContactPoint.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1942 @Description(shortDefinition = "Contact details for individual or publisher", formalDefinition = "Contact details for individual (if a name was provided) or the publisher.") 1943 protected List<ContactPoint> telecom; 1944 1945 private static final long serialVersionUID = -1179697803L; 1946 1947 /* 1948 * Constructor 1949 */ 1950 public ConformanceContactComponent() { 1951 super(); 1952 } 1953 1954 /** 1955 * @return {@link #name} (The name of an individual to contact regarding the 1956 * conformance.). This is the underlying object with id, value and 1957 * extensions. The accessor "getName" gives direct access to the value 1958 */ 1959 public StringType getNameElement() { 1960 if (this.name == null) 1961 if (Configuration.errorOnAutoCreate()) 1962 throw new Error("Attempt to auto-create ConformanceContactComponent.name"); 1963 else if (Configuration.doAutoCreate()) 1964 this.name = new StringType(); // bb 1965 return this.name; 1966 } 1967 1968 public boolean hasNameElement() { 1969 return this.name != null && !this.name.isEmpty(); 1970 } 1971 1972 public boolean hasName() { 1973 return this.name != null && !this.name.isEmpty(); 1974 } 1975 1976 /** 1977 * @param value {@link #name} (The name of an individual to contact regarding 1978 * the conformance.). This is the underlying object with id, value 1979 * and extensions. The accessor "getName" gives direct access to 1980 * the value 1981 */ 1982 public ConformanceContactComponent setNameElement(StringType value) { 1983 this.name = value; 1984 return this; 1985 } 1986 1987 /** 1988 * @return The name of an individual to contact regarding the conformance. 1989 */ 1990 public String getName() { 1991 return this.name == null ? null : this.name.getValue(); 1992 } 1993 1994 /** 1995 * @param value The name of an individual to contact regarding the conformance. 1996 */ 1997 public ConformanceContactComponent setName(String value) { 1998 if (Utilities.noString(value)) 1999 this.name = null; 2000 else { 2001 if (this.name == null) 2002 this.name = new StringType(); 2003 this.name.setValue(value); 2004 } 2005 return this; 2006 } 2007 2008 /** 2009 * @return {@link #telecom} (Contact details for individual (if a name was 2010 * provided) or the publisher.) 2011 */ 2012 public List<ContactPoint> getTelecom() { 2013 if (this.telecom == null) 2014 this.telecom = new ArrayList<ContactPoint>(); 2015 return this.telecom; 2016 } 2017 2018 public boolean hasTelecom() { 2019 if (this.telecom == null) 2020 return false; 2021 for (ContactPoint item : this.telecom) 2022 if (!item.isEmpty()) 2023 return true; 2024 return false; 2025 } 2026 2027 /** 2028 * @return {@link #telecom} (Contact details for individual (if a name was 2029 * provided) or the publisher.) 2030 */ 2031 // syntactic sugar 2032 public ContactPoint addTelecom() { // 3 2033 ContactPoint t = new ContactPoint(); 2034 if (this.telecom == null) 2035 this.telecom = new ArrayList<ContactPoint>(); 2036 this.telecom.add(t); 2037 return t; 2038 } 2039 2040 // syntactic sugar 2041 public ConformanceContactComponent addTelecom(ContactPoint t) { // 3 2042 if (t == null) 2043 return this; 2044 if (this.telecom == null) 2045 this.telecom = new ArrayList<ContactPoint>(); 2046 this.telecom.add(t); 2047 return this; 2048 } 2049 2050 protected void listChildren(List<Property> childrenList) { 2051 super.listChildren(childrenList); 2052 childrenList.add(new Property("name", "string", "The name of an individual to contact regarding the conformance.", 2053 0, java.lang.Integer.MAX_VALUE, name)); 2054 childrenList.add(new Property("telecom", "ContactPoint", 2055 "Contact details for individual (if a name was provided) or the publisher.", 0, java.lang.Integer.MAX_VALUE, 2056 telecom)); 2057 } 2058 2059 @Override 2060 public void setProperty(String name, Base value) throws FHIRException { 2061 if (name.equals("name")) 2062 this.name = castToString(value); // StringType 2063 else if (name.equals("telecom")) 2064 this.getTelecom().add(castToContactPoint(value)); 2065 else 2066 super.setProperty(name, value); 2067 } 2068 2069 @Override 2070 public Base addChild(String name) throws FHIRException { 2071 if (name.equals("name")) { 2072 throw new FHIRException("Cannot call addChild on a singleton property Conformance.name"); 2073 } else if (name.equals("telecom")) { 2074 return addTelecom(); 2075 } else 2076 return super.addChild(name); 2077 } 2078 2079 public ConformanceContactComponent copy() { 2080 ConformanceContactComponent dst = new ConformanceContactComponent(); 2081 copyValues(dst); 2082 dst.name = name == null ? null : name.copy(); 2083 if (telecom != null) { 2084 dst.telecom = new ArrayList<ContactPoint>(); 2085 for (ContactPoint i : telecom) 2086 dst.telecom.add(i.copy()); 2087 } 2088 ; 2089 return dst; 2090 } 2091 2092 @Override 2093 public boolean equalsDeep(Base other) { 2094 if (!super.equalsDeep(other)) 2095 return false; 2096 if (!(other instanceof ConformanceContactComponent)) 2097 return false; 2098 ConformanceContactComponent o = (ConformanceContactComponent) other; 2099 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 2100 } 2101 2102 @Override 2103 public boolean equalsShallow(Base other) { 2104 if (!super.equalsShallow(other)) 2105 return false; 2106 if (!(other instanceof ConformanceContactComponent)) 2107 return false; 2108 ConformanceContactComponent o = (ConformanceContactComponent) other; 2109 return compareValues(name, o.name, true); 2110 } 2111 2112 public boolean isEmpty() { 2113 return super.isEmpty() && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()); 2114 } 2115 2116 public String fhirType() { 2117 return "Conformance.contact"; 2118 2119 } 2120 2121 } 2122 2123 @Block() 2124 public static class ConformanceSoftwareComponent extends BackboneElement implements IBaseBackboneElement { 2125 /** 2126 * Name software is known by. 2127 */ 2128 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2129 @Description(shortDefinition = "A name the software is known by", formalDefinition = "Name software is known by.") 2130 protected StringType name; 2131 2132 /** 2133 * The version identifier for the software covered by this statement. 2134 */ 2135 @Child(name = "version", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2136 @Description(shortDefinition = "Version covered by this statement", formalDefinition = "The version identifier for the software covered by this statement.") 2137 protected StringType version; 2138 2139 /** 2140 * Date this version of the software released. 2141 */ 2142 @Child(name = "releaseDate", type = { 2143 DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2144 @Description(shortDefinition = "Date this version released", formalDefinition = "Date this version of the software released.") 2145 protected DateTimeType releaseDate; 2146 2147 private static final long serialVersionUID = 1819769027L; 2148 2149 /* 2150 * Constructor 2151 */ 2152 public ConformanceSoftwareComponent() { 2153 super(); 2154 } 2155 2156 /* 2157 * Constructor 2158 */ 2159 public ConformanceSoftwareComponent(StringType name) { 2160 super(); 2161 this.name = name; 2162 } 2163 2164 /** 2165 * @return {@link #name} (Name software is known by.). This is the underlying 2166 * object with id, value and extensions. The accessor "getName" gives 2167 * direct access to the value 2168 */ 2169 public StringType getNameElement() { 2170 if (this.name == null) 2171 if (Configuration.errorOnAutoCreate()) 2172 throw new Error("Attempt to auto-create ConformanceSoftwareComponent.name"); 2173 else if (Configuration.doAutoCreate()) 2174 this.name = new StringType(); // bb 2175 return this.name; 2176 } 2177 2178 public boolean hasNameElement() { 2179 return this.name != null && !this.name.isEmpty(); 2180 } 2181 2182 public boolean hasName() { 2183 return this.name != null && !this.name.isEmpty(); 2184 } 2185 2186 /** 2187 * @param value {@link #name} (Name software is known by.). This is the 2188 * underlying object with id, value and extensions. The accessor 2189 * "getName" gives direct access to the value 2190 */ 2191 public ConformanceSoftwareComponent setNameElement(StringType value) { 2192 this.name = value; 2193 return this; 2194 } 2195 2196 /** 2197 * @return Name software is known by. 2198 */ 2199 public String getName() { 2200 return this.name == null ? null : this.name.getValue(); 2201 } 2202 2203 /** 2204 * @param value Name software is known by. 2205 */ 2206 public ConformanceSoftwareComponent setName(String value) { 2207 if (this.name == null) 2208 this.name = new StringType(); 2209 this.name.setValue(value); 2210 return this; 2211 } 2212 2213 /** 2214 * @return {@link #version} (The version identifier for the software covered by 2215 * this statement.). This is the underlying object with id, value and 2216 * extensions. The accessor "getVersion" gives direct access to the 2217 * value 2218 */ 2219 public StringType getVersionElement() { 2220 if (this.version == null) 2221 if (Configuration.errorOnAutoCreate()) 2222 throw new Error("Attempt to auto-create ConformanceSoftwareComponent.version"); 2223 else if (Configuration.doAutoCreate()) 2224 this.version = new StringType(); // bb 2225 return this.version; 2226 } 2227 2228 public boolean hasVersionElement() { 2229 return this.version != null && !this.version.isEmpty(); 2230 } 2231 2232 public boolean hasVersion() { 2233 return this.version != null && !this.version.isEmpty(); 2234 } 2235 2236 /** 2237 * @param value {@link #version} (The version identifier for the software 2238 * covered by this statement.). This is the underlying object with 2239 * id, value and extensions. The accessor "getVersion" gives direct 2240 * access to the value 2241 */ 2242 public ConformanceSoftwareComponent setVersionElement(StringType value) { 2243 this.version = value; 2244 return this; 2245 } 2246 2247 /** 2248 * @return The version identifier for the software covered by this statement. 2249 */ 2250 public String getVersion() { 2251 return this.version == null ? null : this.version.getValue(); 2252 } 2253 2254 /** 2255 * @param value The version identifier for the software covered by this 2256 * statement. 2257 */ 2258 public ConformanceSoftwareComponent setVersion(String value) { 2259 if (Utilities.noString(value)) 2260 this.version = null; 2261 else { 2262 if (this.version == null) 2263 this.version = new StringType(); 2264 this.version.setValue(value); 2265 } 2266 return this; 2267 } 2268 2269 /** 2270 * @return {@link #releaseDate} (Date this version of the software released.). 2271 * This is the underlying object with id, value and extensions. The 2272 * accessor "getReleaseDate" gives direct access to the value 2273 */ 2274 public DateTimeType getReleaseDateElement() { 2275 if (this.releaseDate == null) 2276 if (Configuration.errorOnAutoCreate()) 2277 throw new Error("Attempt to auto-create ConformanceSoftwareComponent.releaseDate"); 2278 else if (Configuration.doAutoCreate()) 2279 this.releaseDate = new DateTimeType(); // bb 2280 return this.releaseDate; 2281 } 2282 2283 public boolean hasReleaseDateElement() { 2284 return this.releaseDate != null && !this.releaseDate.isEmpty(); 2285 } 2286 2287 public boolean hasReleaseDate() { 2288 return this.releaseDate != null && !this.releaseDate.isEmpty(); 2289 } 2290 2291 /** 2292 * @param value {@link #releaseDate} (Date this version of the software 2293 * released.). This is the underlying object with id, value and 2294 * extensions. The accessor "getReleaseDate" gives direct access to 2295 * the value 2296 */ 2297 public ConformanceSoftwareComponent setReleaseDateElement(DateTimeType value) { 2298 this.releaseDate = value; 2299 return this; 2300 } 2301 2302 /** 2303 * @return Date this version of the software released. 2304 */ 2305 public Date getReleaseDate() { 2306 return this.releaseDate == null ? null : this.releaseDate.getValue(); 2307 } 2308 2309 /** 2310 * @param value Date this version of the software released. 2311 */ 2312 public ConformanceSoftwareComponent setReleaseDate(Date value) { 2313 if (value == null) 2314 this.releaseDate = null; 2315 else { 2316 if (this.releaseDate == null) 2317 this.releaseDate = new DateTimeType(); 2318 this.releaseDate.setValue(value); 2319 } 2320 return this; 2321 } 2322 2323 protected void listChildren(List<Property> childrenList) { 2324 super.listChildren(childrenList); 2325 childrenList 2326 .add(new Property("name", "string", "Name software is known by.", 0, java.lang.Integer.MAX_VALUE, name)); 2327 childrenList 2328 .add(new Property("version", "string", "The version identifier for the software covered by this statement.", 2329 0, java.lang.Integer.MAX_VALUE, version)); 2330 childrenList.add(new Property("releaseDate", "dateTime", "Date this version of the software released.", 0, 2331 java.lang.Integer.MAX_VALUE, releaseDate)); 2332 } 2333 2334 @Override 2335 public void setProperty(String name, Base value) throws FHIRException { 2336 if (name.equals("name")) 2337 this.name = castToString(value); // StringType 2338 else if (name.equals("version")) 2339 this.version = castToString(value); // StringType 2340 else if (name.equals("releaseDate")) 2341 this.releaseDate = castToDateTime(value); // DateTimeType 2342 else 2343 super.setProperty(name, value); 2344 } 2345 2346 @Override 2347 public Base addChild(String name) throws FHIRException { 2348 if (name.equals("name")) { 2349 throw new FHIRException("Cannot call addChild on a singleton property Conformance.name"); 2350 } else if (name.equals("version")) { 2351 throw new FHIRException("Cannot call addChild on a singleton property Conformance.version"); 2352 } else if (name.equals("releaseDate")) { 2353 throw new FHIRException("Cannot call addChild on a singleton property Conformance.releaseDate"); 2354 } else 2355 return super.addChild(name); 2356 } 2357 2358 public ConformanceSoftwareComponent copy() { 2359 ConformanceSoftwareComponent dst = new ConformanceSoftwareComponent(); 2360 copyValues(dst); 2361 dst.name = name == null ? null : name.copy(); 2362 dst.version = version == null ? null : version.copy(); 2363 dst.releaseDate = releaseDate == null ? null : releaseDate.copy(); 2364 return dst; 2365 } 2366 2367 @Override 2368 public boolean equalsDeep(Base other) { 2369 if (!super.equalsDeep(other)) 2370 return false; 2371 if (!(other instanceof ConformanceSoftwareComponent)) 2372 return false; 2373 ConformanceSoftwareComponent o = (ConformanceSoftwareComponent) other; 2374 return compareDeep(name, o.name, true) && compareDeep(version, o.version, true) 2375 && compareDeep(releaseDate, o.releaseDate, true); 2376 } 2377 2378 @Override 2379 public boolean equalsShallow(Base other) { 2380 if (!super.equalsShallow(other)) 2381 return false; 2382 if (!(other instanceof ConformanceSoftwareComponent)) 2383 return false; 2384 ConformanceSoftwareComponent o = (ConformanceSoftwareComponent) other; 2385 return compareValues(name, o.name, true) && compareValues(version, o.version, true) 2386 && compareValues(releaseDate, o.releaseDate, true); 2387 } 2388 2389 public boolean isEmpty() { 2390 return super.isEmpty() && (name == null || name.isEmpty()) && (version == null || version.isEmpty()) 2391 && (releaseDate == null || releaseDate.isEmpty()); 2392 } 2393 2394 public String fhirType() { 2395 return "Conformance.software"; 2396 2397 } 2398 2399 } 2400 2401 @Block() 2402 public static class ConformanceImplementationComponent extends BackboneElement implements IBaseBackboneElement { 2403 /** 2404 * Information about the specific installation that this conformance statement 2405 * relates to. 2406 */ 2407 @Child(name = "description", type = { 2408 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2409 @Description(shortDefinition = "Describes this specific instance", formalDefinition = "Information about the specific installation that this conformance statement relates to.") 2410 protected StringType description; 2411 2412 /** 2413 * An absolute base URL for the implementation. This forms the base for REST 2414 * interfaces as well as the mailbox and document interfaces. 2415 */ 2416 @Child(name = "url", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2417 @Description(shortDefinition = "Base URL for the installation", formalDefinition = "An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.") 2418 protected UriType url; 2419 2420 private static final long serialVersionUID = -289238508L; 2421 2422 /* 2423 * Constructor 2424 */ 2425 public ConformanceImplementationComponent() { 2426 super(); 2427 } 2428 2429 /* 2430 * Constructor 2431 */ 2432 public ConformanceImplementationComponent(StringType description) { 2433 super(); 2434 this.description = description; 2435 } 2436 2437 /** 2438 * @return {@link #description} (Information about the specific installation 2439 * that this conformance statement relates to.). This is the underlying 2440 * object with id, value and extensions. The accessor "getDescription" 2441 * gives direct access to the value 2442 */ 2443 public StringType getDescriptionElement() { 2444 if (this.description == null) 2445 if (Configuration.errorOnAutoCreate()) 2446 throw new Error("Attempt to auto-create ConformanceImplementationComponent.description"); 2447 else if (Configuration.doAutoCreate()) 2448 this.description = new StringType(); // bb 2449 return this.description; 2450 } 2451 2452 public boolean hasDescriptionElement() { 2453 return this.description != null && !this.description.isEmpty(); 2454 } 2455 2456 public boolean hasDescription() { 2457 return this.description != null && !this.description.isEmpty(); 2458 } 2459 2460 /** 2461 * @param value {@link #description} (Information about the specific 2462 * installation that this conformance statement relates to.). This 2463 * is the underlying object with id, value and extensions. The 2464 * accessor "getDescription" gives direct access to the value 2465 */ 2466 public ConformanceImplementationComponent setDescriptionElement(StringType value) { 2467 this.description = value; 2468 return this; 2469 } 2470 2471 /** 2472 * @return Information about the specific installation that this conformance 2473 * statement relates to. 2474 */ 2475 public String getDescription() { 2476 return this.description == null ? null : this.description.getValue(); 2477 } 2478 2479 /** 2480 * @param value Information about the specific installation that this 2481 * conformance statement relates to. 2482 */ 2483 public ConformanceImplementationComponent setDescription(String value) { 2484 if (this.description == null) 2485 this.description = new StringType(); 2486 this.description.setValue(value); 2487 return this; 2488 } 2489 2490 /** 2491 * @return {@link #url} (An absolute base URL for the implementation. This forms 2492 * the base for REST interfaces as well as the mailbox and document 2493 * interfaces.). This is the underlying object with id, value and 2494 * extensions. The accessor "getUrl" gives direct access to the value 2495 */ 2496 public UriType getUrlElement() { 2497 if (this.url == null) 2498 if (Configuration.errorOnAutoCreate()) 2499 throw new Error("Attempt to auto-create ConformanceImplementationComponent.url"); 2500 else if (Configuration.doAutoCreate()) 2501 this.url = new UriType(); // bb 2502 return this.url; 2503 } 2504 2505 public boolean hasUrlElement() { 2506 return this.url != null && !this.url.isEmpty(); 2507 } 2508 2509 public boolean hasUrl() { 2510 return this.url != null && !this.url.isEmpty(); 2511 } 2512 2513 /** 2514 * @param value {@link #url} (An absolute base URL for the implementation. This 2515 * forms the base for REST interfaces as well as the mailbox and 2516 * document interfaces.). This is the underlying object with id, 2517 * value and extensions. The accessor "getUrl" gives direct access 2518 * to the value 2519 */ 2520 public ConformanceImplementationComponent setUrlElement(UriType value) { 2521 this.url = value; 2522 return this; 2523 } 2524 2525 /** 2526 * @return An absolute base URL for the implementation. This forms the base for 2527 * REST interfaces as well as the mailbox and document interfaces. 2528 */ 2529 public String getUrl() { 2530 return this.url == null ? null : this.url.getValue(); 2531 } 2532 2533 /** 2534 * @param value An absolute base URL for the implementation. This forms the base 2535 * for REST interfaces as well as the mailbox and document 2536 * interfaces. 2537 */ 2538 public ConformanceImplementationComponent setUrl(String value) { 2539 if (Utilities.noString(value)) 2540 this.url = null; 2541 else { 2542 if (this.url == null) 2543 this.url = new UriType(); 2544 this.url.setValue(value); 2545 } 2546 return this; 2547 } 2548 2549 protected void listChildren(List<Property> childrenList) { 2550 super.listChildren(childrenList); 2551 childrenList.add(new Property("description", "string", 2552 "Information about the specific installation that this conformance statement relates to.", 0, 2553 java.lang.Integer.MAX_VALUE, description)); 2554 childrenList.add(new Property("url", "uri", 2555 "An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.", 2556 0, java.lang.Integer.MAX_VALUE, url)); 2557 } 2558 2559 @Override 2560 public void setProperty(String name, Base value) throws FHIRException { 2561 if (name.equals("description")) 2562 this.description = castToString(value); // StringType 2563 else if (name.equals("url")) 2564 this.url = castToUri(value); // UriType 2565 else 2566 super.setProperty(name, value); 2567 } 2568 2569 @Override 2570 public Base addChild(String name) throws FHIRException { 2571 if (name.equals("description")) { 2572 throw new FHIRException("Cannot call addChild on a singleton property Conformance.description"); 2573 } else if (name.equals("url")) { 2574 throw new FHIRException("Cannot call addChild on a singleton property Conformance.url"); 2575 } else 2576 return super.addChild(name); 2577 } 2578 2579 public ConformanceImplementationComponent copy() { 2580 ConformanceImplementationComponent dst = new ConformanceImplementationComponent(); 2581 copyValues(dst); 2582 dst.description = description == null ? null : description.copy(); 2583 dst.url = url == null ? null : url.copy(); 2584 return dst; 2585 } 2586 2587 @Override 2588 public boolean equalsDeep(Base other) { 2589 if (!super.equalsDeep(other)) 2590 return false; 2591 if (!(other instanceof ConformanceImplementationComponent)) 2592 return false; 2593 ConformanceImplementationComponent o = (ConformanceImplementationComponent) other; 2594 return compareDeep(description, o.description, true) && compareDeep(url, o.url, true); 2595 } 2596 2597 @Override 2598 public boolean equalsShallow(Base other) { 2599 if (!super.equalsShallow(other)) 2600 return false; 2601 if (!(other instanceof ConformanceImplementationComponent)) 2602 return false; 2603 ConformanceImplementationComponent o = (ConformanceImplementationComponent) other; 2604 return compareValues(description, o.description, true) && compareValues(url, o.url, true); 2605 } 2606 2607 public boolean isEmpty() { 2608 return super.isEmpty() && (description == null || description.isEmpty()) && (url == null || url.isEmpty()); 2609 } 2610 2611 public String fhirType() { 2612 return "Conformance.implementation"; 2613 2614 } 2615 2616 } 2617 2618 @Block() 2619 public static class ConformanceRestComponent extends BackboneElement implements IBaseBackboneElement { 2620 /** 2621 * Identifies whether this portion of the statement is describing ability to 2622 * initiate or receive restful operations. 2623 */ 2624 @Child(name = "mode", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2625 @Description(shortDefinition = "client | server", formalDefinition = "Identifies whether this portion of the statement is describing ability to initiate or receive restful operations.") 2626 protected Enumeration<RestfulConformanceMode> mode; 2627 2628 /** 2629 * Information about the system's restful capabilities that apply across all 2630 * applications, such as security. 2631 */ 2632 @Child(name = "documentation", type = { 2633 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2634 @Description(shortDefinition = "General description of implementation", formalDefinition = "Information about the system's restful capabilities that apply across all applications, such as security.") 2635 protected StringType documentation; 2636 2637 /** 2638 * Information about security implementation from an interface perspective - 2639 * what a client needs to know. 2640 */ 2641 @Child(name = "security", type = {}, order = 3, min = 0, max = 1, modifier = false, summary = false) 2642 @Description(shortDefinition = "Information about security of implementation", formalDefinition = "Information about security implementation from an interface perspective - what a client needs to know.") 2643 protected ConformanceRestSecurityComponent security; 2644 2645 /** 2646 * A specification of the restful capabilities of the solution for a specific 2647 * resource type. 2648 */ 2649 @Child(name = "resource", type = {}, order = 4, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2650 @Description(shortDefinition = "Resource served on the REST interface", formalDefinition = "A specification of the restful capabilities of the solution for a specific resource type.") 2651 protected List<ConformanceRestResourceComponent> resource; 2652 2653 /** 2654 * A specification of restful operations supported by the system. 2655 */ 2656 @Child(name = "interaction", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2657 @Description(shortDefinition = "What operations are supported?", formalDefinition = "A specification of restful operations supported by the system.") 2658 protected List<SystemInteractionComponent> interaction; 2659 2660 /** 2661 * A code that indicates how transactions are supported. 2662 */ 2663 @Child(name = "transactionMode", type = { 2664 CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 2665 @Description(shortDefinition = "not-supported | batch | transaction | both", formalDefinition = "A code that indicates how transactions are supported.") 2666 protected Enumeration<TransactionMode> transactionMode; 2667 2668 /** 2669 * Search parameters that are supported for searching all resources for 2670 * implementations to support and/or make use of - either references to ones 2671 * defined in the specification, or additional ones defined for/by the 2672 * implementation. 2673 */ 2674 @Child(name = "searchParam", type = { 2675 ConformanceRestResourceSearchParamComponent.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2676 @Description(shortDefinition = "Search params for searching all resources", formalDefinition = "Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.") 2677 protected List<ConformanceRestResourceSearchParamComponent> searchParam; 2678 2679 /** 2680 * Definition of an operation or a named query and with its parameters and their 2681 * meaning and type. 2682 */ 2683 @Child(name = "operation", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2684 @Description(shortDefinition = "Definition of an operation or a custom query", formalDefinition = "Definition of an operation or a named query and with its parameters and their meaning and type.") 2685 protected List<ConformanceRestOperationComponent> operation; 2686 2687 /** 2688 * An absolute URI which is a reference to the definition of a compartment 2689 * hosted by the system. 2690 */ 2691 @Child(name = "compartment", type = { 2692 UriType.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2693 @Description(shortDefinition = "Compartments served/used by system", formalDefinition = "An absolute URI which is a reference to the definition of a compartment hosted by the system.") 2694 protected List<UriType> compartment; 2695 2696 private static final long serialVersionUID = 931983837L; 2697 2698 /* 2699 * Constructor 2700 */ 2701 public ConformanceRestComponent() { 2702 super(); 2703 } 2704 2705 /* 2706 * Constructor 2707 */ 2708 public ConformanceRestComponent(Enumeration<RestfulConformanceMode> mode) { 2709 super(); 2710 this.mode = mode; 2711 } 2712 2713 /** 2714 * @return {@link #mode} (Identifies whether this portion of the statement is 2715 * describing ability to initiate or receive restful operations.). This 2716 * is the underlying object with id, value and extensions. The accessor 2717 * "getMode" gives direct access to the value 2718 */ 2719 public Enumeration<RestfulConformanceMode> getModeElement() { 2720 if (this.mode == null) 2721 if (Configuration.errorOnAutoCreate()) 2722 throw new Error("Attempt to auto-create ConformanceRestComponent.mode"); 2723 else if (Configuration.doAutoCreate()) 2724 this.mode = new Enumeration<RestfulConformanceMode>(new RestfulConformanceModeEnumFactory()); // bb 2725 return this.mode; 2726 } 2727 2728 public boolean hasModeElement() { 2729 return this.mode != null && !this.mode.isEmpty(); 2730 } 2731 2732 public boolean hasMode() { 2733 return this.mode != null && !this.mode.isEmpty(); 2734 } 2735 2736 /** 2737 * @param value {@link #mode} (Identifies whether this portion of the statement 2738 * is describing ability to initiate or receive restful 2739 * operations.). This is the underlying object with id, value and 2740 * extensions. The accessor "getMode" gives direct access to the 2741 * value 2742 */ 2743 public ConformanceRestComponent setModeElement(Enumeration<RestfulConformanceMode> value) { 2744 this.mode = value; 2745 return this; 2746 } 2747 2748 /** 2749 * @return Identifies whether this portion of the statement is describing 2750 * ability to initiate or receive restful operations. 2751 */ 2752 public RestfulConformanceMode getMode() { 2753 return this.mode == null ? null : this.mode.getValue(); 2754 } 2755 2756 /** 2757 * @param value Identifies whether this portion of the statement is describing 2758 * ability to initiate or receive restful operations. 2759 */ 2760 public ConformanceRestComponent setMode(RestfulConformanceMode value) { 2761 if (this.mode == null) 2762 this.mode = new Enumeration<RestfulConformanceMode>(new RestfulConformanceModeEnumFactory()); 2763 this.mode.setValue(value); 2764 return this; 2765 } 2766 2767 /** 2768 * @return {@link #documentation} (Information about the system's restful 2769 * capabilities that apply across all applications, such as security.). 2770 * This is the underlying object with id, value and extensions. The 2771 * accessor "getDocumentation" gives direct access to the value 2772 */ 2773 public StringType getDocumentationElement() { 2774 if (this.documentation == null) 2775 if (Configuration.errorOnAutoCreate()) 2776 throw new Error("Attempt to auto-create ConformanceRestComponent.documentation"); 2777 else if (Configuration.doAutoCreate()) 2778 this.documentation = new StringType(); // bb 2779 return this.documentation; 2780 } 2781 2782 public boolean hasDocumentationElement() { 2783 return this.documentation != null && !this.documentation.isEmpty(); 2784 } 2785 2786 public boolean hasDocumentation() { 2787 return this.documentation != null && !this.documentation.isEmpty(); 2788 } 2789 2790 /** 2791 * @param value {@link #documentation} (Information about the system's restful 2792 * capabilities that apply across all applications, such as 2793 * security.). This is the underlying object with id, value and 2794 * extensions. The accessor "getDocumentation" gives direct access 2795 * to the value 2796 */ 2797 public ConformanceRestComponent setDocumentationElement(StringType value) { 2798 this.documentation = value; 2799 return this; 2800 } 2801 2802 /** 2803 * @return Information about the system's restful capabilities that apply across 2804 * all applications, such as security. 2805 */ 2806 public String getDocumentation() { 2807 return this.documentation == null ? null : this.documentation.getValue(); 2808 } 2809 2810 /** 2811 * @param value Information about the system's restful capabilities that apply 2812 * across all applications, such as security. 2813 */ 2814 public ConformanceRestComponent setDocumentation(String value) { 2815 if (Utilities.noString(value)) 2816 this.documentation = null; 2817 else { 2818 if (this.documentation == null) 2819 this.documentation = new StringType(); 2820 this.documentation.setValue(value); 2821 } 2822 return this; 2823 } 2824 2825 /** 2826 * @return {@link #security} (Information about security implementation from an 2827 * interface perspective - what a client needs to know.) 2828 */ 2829 public ConformanceRestSecurityComponent getSecurity() { 2830 if (this.security == null) 2831 if (Configuration.errorOnAutoCreate()) 2832 throw new Error("Attempt to auto-create ConformanceRestComponent.security"); 2833 else if (Configuration.doAutoCreate()) 2834 this.security = new ConformanceRestSecurityComponent(); // cc 2835 return this.security; 2836 } 2837 2838 public boolean hasSecurity() { 2839 return this.security != null && !this.security.isEmpty(); 2840 } 2841 2842 /** 2843 * @param value {@link #security} (Information about security implementation 2844 * from an interface perspective - what a client needs to know.) 2845 */ 2846 public ConformanceRestComponent setSecurity(ConformanceRestSecurityComponent value) { 2847 this.security = value; 2848 return this; 2849 } 2850 2851 /** 2852 * @return {@link #resource} (A specification of the restful capabilities of the 2853 * solution for a specific resource type.) 2854 */ 2855 public List<ConformanceRestResourceComponent> getResource() { 2856 if (this.resource == null) 2857 this.resource = new ArrayList<ConformanceRestResourceComponent>(); 2858 return this.resource; 2859 } 2860 2861 public boolean hasResource() { 2862 if (this.resource == null) 2863 return false; 2864 for (ConformanceRestResourceComponent item : this.resource) 2865 if (!item.isEmpty()) 2866 return true; 2867 return false; 2868 } 2869 2870 /** 2871 * @return {@link #resource} (A specification of the restful capabilities of the 2872 * solution for a specific resource type.) 2873 */ 2874 // syntactic sugar 2875 public ConformanceRestResourceComponent addResource() { // 3 2876 ConformanceRestResourceComponent t = new ConformanceRestResourceComponent(); 2877 if (this.resource == null) 2878 this.resource = new ArrayList<ConformanceRestResourceComponent>(); 2879 this.resource.add(t); 2880 return t; 2881 } 2882 2883 // syntactic sugar 2884 public ConformanceRestComponent addResource(ConformanceRestResourceComponent t) { // 3 2885 if (t == null) 2886 return this; 2887 if (this.resource == null) 2888 this.resource = new ArrayList<ConformanceRestResourceComponent>(); 2889 this.resource.add(t); 2890 return this; 2891 } 2892 2893 /** 2894 * @return {@link #interaction} (A specification of restful operations supported 2895 * by the system.) 2896 */ 2897 public List<SystemInteractionComponent> getInteraction() { 2898 if (this.interaction == null) 2899 this.interaction = new ArrayList<SystemInteractionComponent>(); 2900 return this.interaction; 2901 } 2902 2903 public boolean hasInteraction() { 2904 if (this.interaction == null) 2905 return false; 2906 for (SystemInteractionComponent item : this.interaction) 2907 if (!item.isEmpty()) 2908 return true; 2909 return false; 2910 } 2911 2912 /** 2913 * @return {@link #interaction} (A specification of restful operations supported 2914 * by the system.) 2915 */ 2916 // syntactic sugar 2917 public SystemInteractionComponent addInteraction() { // 3 2918 SystemInteractionComponent t = new SystemInteractionComponent(); 2919 if (this.interaction == null) 2920 this.interaction = new ArrayList<SystemInteractionComponent>(); 2921 this.interaction.add(t); 2922 return t; 2923 } 2924 2925 // syntactic sugar 2926 public ConformanceRestComponent addInteraction(SystemInteractionComponent t) { // 3 2927 if (t == null) 2928 return this; 2929 if (this.interaction == null) 2930 this.interaction = new ArrayList<SystemInteractionComponent>(); 2931 this.interaction.add(t); 2932 return this; 2933 } 2934 2935 /** 2936 * @return {@link #transactionMode} (A code that indicates how transactions are 2937 * supported.). This is the underlying object with id, value and 2938 * extensions. The accessor "getTransactionMode" gives direct access to 2939 * the value 2940 */ 2941 public Enumeration<TransactionMode> getTransactionModeElement() { 2942 if (this.transactionMode == null) 2943 if (Configuration.errorOnAutoCreate()) 2944 throw new Error("Attempt to auto-create ConformanceRestComponent.transactionMode"); 2945 else if (Configuration.doAutoCreate()) 2946 this.transactionMode = new Enumeration<TransactionMode>(new TransactionModeEnumFactory()); // bb 2947 return this.transactionMode; 2948 } 2949 2950 public boolean hasTransactionModeElement() { 2951 return this.transactionMode != null && !this.transactionMode.isEmpty(); 2952 } 2953 2954 public boolean hasTransactionMode() { 2955 return this.transactionMode != null && !this.transactionMode.isEmpty(); 2956 } 2957 2958 /** 2959 * @param value {@link #transactionMode} (A code that indicates how transactions 2960 * are supported.). This is the underlying object with id, value 2961 * and extensions. The accessor "getTransactionMode" gives direct 2962 * access to the value 2963 */ 2964 public ConformanceRestComponent setTransactionModeElement(Enumeration<TransactionMode> value) { 2965 this.transactionMode = value; 2966 return this; 2967 } 2968 2969 /** 2970 * @return A code that indicates how transactions are supported. 2971 */ 2972 public TransactionMode getTransactionMode() { 2973 return this.transactionMode == null ? null : this.transactionMode.getValue(); 2974 } 2975 2976 /** 2977 * @param value A code that indicates how transactions are supported. 2978 */ 2979 public ConformanceRestComponent setTransactionMode(TransactionMode value) { 2980 if (value == null) 2981 this.transactionMode = null; 2982 else { 2983 if (this.transactionMode == null) 2984 this.transactionMode = new Enumeration<TransactionMode>(new TransactionModeEnumFactory()); 2985 this.transactionMode.setValue(value); 2986 } 2987 return this; 2988 } 2989 2990 /** 2991 * @return {@link #searchParam} (Search parameters that are supported for 2992 * searching all resources for implementations to support and/or make 2993 * use of - either references to ones defined in the specification, or 2994 * additional ones defined for/by the implementation.) 2995 */ 2996 public List<ConformanceRestResourceSearchParamComponent> getSearchParam() { 2997 if (this.searchParam == null) 2998 this.searchParam = new ArrayList<ConformanceRestResourceSearchParamComponent>(); 2999 return this.searchParam; 3000 } 3001 3002 public boolean hasSearchParam() { 3003 if (this.searchParam == null) 3004 return false; 3005 for (ConformanceRestResourceSearchParamComponent item : this.searchParam) 3006 if (!item.isEmpty()) 3007 return true; 3008 return false; 3009 } 3010 3011 /** 3012 * @return {@link #searchParam} (Search parameters that are supported for 3013 * searching all resources for implementations to support and/or make 3014 * use of - either references to ones defined in the specification, or 3015 * additional ones defined for/by the implementation.) 3016 */ 3017 // syntactic sugar 3018 public ConformanceRestResourceSearchParamComponent addSearchParam() { // 3 3019 ConformanceRestResourceSearchParamComponent t = new ConformanceRestResourceSearchParamComponent(); 3020 if (this.searchParam == null) 3021 this.searchParam = new ArrayList<ConformanceRestResourceSearchParamComponent>(); 3022 this.searchParam.add(t); 3023 return t; 3024 } 3025 3026 // syntactic sugar 3027 public ConformanceRestComponent addSearchParam(ConformanceRestResourceSearchParamComponent t) { // 3 3028 if (t == null) 3029 return this; 3030 if (this.searchParam == null) 3031 this.searchParam = new ArrayList<ConformanceRestResourceSearchParamComponent>(); 3032 this.searchParam.add(t); 3033 return this; 3034 } 3035 3036 /** 3037 * @return {@link #operation} (Definition of an operation or a named query and 3038 * with its parameters and their meaning and type.) 3039 */ 3040 public List<ConformanceRestOperationComponent> getOperation() { 3041 if (this.operation == null) 3042 this.operation = new ArrayList<ConformanceRestOperationComponent>(); 3043 return this.operation; 3044 } 3045 3046 public boolean hasOperation() { 3047 if (this.operation == null) 3048 return false; 3049 for (ConformanceRestOperationComponent item : this.operation) 3050 if (!item.isEmpty()) 3051 return true; 3052 return false; 3053 } 3054 3055 /** 3056 * @return {@link #operation} (Definition of an operation or a named query and 3057 * with its parameters and their meaning and type.) 3058 */ 3059 // syntactic sugar 3060 public ConformanceRestOperationComponent addOperation() { // 3 3061 ConformanceRestOperationComponent t = new ConformanceRestOperationComponent(); 3062 if (this.operation == null) 3063 this.operation = new ArrayList<ConformanceRestOperationComponent>(); 3064 this.operation.add(t); 3065 return t; 3066 } 3067 3068 // syntactic sugar 3069 public ConformanceRestComponent addOperation(ConformanceRestOperationComponent t) { // 3 3070 if (t == null) 3071 return this; 3072 if (this.operation == null) 3073 this.operation = new ArrayList<ConformanceRestOperationComponent>(); 3074 this.operation.add(t); 3075 return this; 3076 } 3077 3078 /** 3079 * @return {@link #compartment} (An absolute URI which is a reference to the 3080 * definition of a compartment hosted by the system.) 3081 */ 3082 public List<UriType> getCompartment() { 3083 if (this.compartment == null) 3084 this.compartment = new ArrayList<UriType>(); 3085 return this.compartment; 3086 } 3087 3088 public boolean hasCompartment() { 3089 if (this.compartment == null) 3090 return false; 3091 for (UriType item : this.compartment) 3092 if (!item.isEmpty()) 3093 return true; 3094 return false; 3095 } 3096 3097 /** 3098 * @return {@link #compartment} (An absolute URI which is a reference to the 3099 * definition of a compartment hosted by the system.) 3100 */ 3101 // syntactic sugar 3102 public UriType addCompartmentElement() {// 2 3103 UriType t = new UriType(); 3104 if (this.compartment == null) 3105 this.compartment = new ArrayList<UriType>(); 3106 this.compartment.add(t); 3107 return t; 3108 } 3109 3110 /** 3111 * @param value {@link #compartment} (An absolute URI which is a reference to 3112 * the definition of a compartment hosted by the system.) 3113 */ 3114 public ConformanceRestComponent addCompartment(String value) { // 1 3115 UriType t = new UriType(); 3116 t.setValue(value); 3117 if (this.compartment == null) 3118 this.compartment = new ArrayList<UriType>(); 3119 this.compartment.add(t); 3120 return this; 3121 } 3122 3123 /** 3124 * @param value {@link #compartment} (An absolute URI which is a reference to 3125 * the definition of a compartment hosted by the system.) 3126 */ 3127 public boolean hasCompartment(String value) { 3128 if (this.compartment == null) 3129 return false; 3130 for (UriType v : this.compartment) 3131 if (v.equals(value)) // uri 3132 return true; 3133 return false; 3134 } 3135 3136 protected void listChildren(List<Property> childrenList) { 3137 super.listChildren(childrenList); 3138 childrenList.add(new Property("mode", "code", 3139 "Identifies whether this portion of the statement is describing ability to initiate or receive restful operations.", 3140 0, java.lang.Integer.MAX_VALUE, mode)); 3141 childrenList.add(new Property("documentation", "string", 3142 "Information about the system's restful capabilities that apply across all applications, such as security.", 3143 0, java.lang.Integer.MAX_VALUE, documentation)); 3144 childrenList.add(new Property("security", "", 3145 "Information about security implementation from an interface perspective - what a client needs to know.", 0, 3146 java.lang.Integer.MAX_VALUE, security)); 3147 childrenList.add(new Property("resource", "", 3148 "A specification of the restful capabilities of the solution for a specific resource type.", 0, 3149 java.lang.Integer.MAX_VALUE, resource)); 3150 childrenList.add(new Property("interaction", "", "A specification of restful operations supported by the system.", 3151 0, java.lang.Integer.MAX_VALUE, interaction)); 3152 childrenList.add(new Property("transactionMode", "code", "A code that indicates how transactions are supported.", 3153 0, java.lang.Integer.MAX_VALUE, transactionMode)); 3154 childrenList.add(new Property("searchParam", "@Conformance.rest.resource.searchParam", 3155 "Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 3156 0, java.lang.Integer.MAX_VALUE, searchParam)); 3157 childrenList.add(new Property("operation", "", 3158 "Definition of an operation or a named query and with its parameters and their meaning and type.", 0, 3159 java.lang.Integer.MAX_VALUE, operation)); 3160 childrenList.add(new Property("compartment", "uri", 3161 "An absolute URI which is a reference to the definition of a compartment hosted by the system.", 0, 3162 java.lang.Integer.MAX_VALUE, compartment)); 3163 } 3164 3165 @Override 3166 public void setProperty(String name, Base value) throws FHIRException { 3167 if (name.equals("mode")) 3168 this.mode = new RestfulConformanceModeEnumFactory().fromType(value); // Enumeration<RestfulConformanceMode> 3169 else if (name.equals("documentation")) 3170 this.documentation = castToString(value); // StringType 3171 else if (name.equals("security")) 3172 this.security = (ConformanceRestSecurityComponent) value; // ConformanceRestSecurityComponent 3173 else if (name.equals("resource")) 3174 this.getResource().add((ConformanceRestResourceComponent) value); 3175 else if (name.equals("interaction")) 3176 this.getInteraction().add((SystemInteractionComponent) value); 3177 else if (name.equals("transactionMode")) 3178 this.transactionMode = new TransactionModeEnumFactory().fromType(value); // Enumeration<TransactionMode> 3179 else if (name.equals("searchParam")) 3180 this.getSearchParam().add((ConformanceRestResourceSearchParamComponent) value); 3181 else if (name.equals("operation")) 3182 this.getOperation().add((ConformanceRestOperationComponent) value); 3183 else if (name.equals("compartment")) 3184 this.getCompartment().add(castToUri(value)); 3185 else 3186 super.setProperty(name, value); 3187 } 3188 3189 @Override 3190 public Base addChild(String name) throws FHIRException { 3191 if (name.equals("mode")) { 3192 throw new FHIRException("Cannot call addChild on a singleton property Conformance.mode"); 3193 } else if (name.equals("documentation")) { 3194 throw new FHIRException("Cannot call addChild on a singleton property Conformance.documentation"); 3195 } else if (name.equals("security")) { 3196 this.security = new ConformanceRestSecurityComponent(); 3197 return this.security; 3198 } else if (name.equals("resource")) { 3199 return addResource(); 3200 } else if (name.equals("interaction")) { 3201 return addInteraction(); 3202 } else if (name.equals("transactionMode")) { 3203 throw new FHIRException("Cannot call addChild on a singleton property Conformance.transactionMode"); 3204 } else if (name.equals("searchParam")) { 3205 return addSearchParam(); 3206 } else if (name.equals("operation")) { 3207 return addOperation(); 3208 } else if (name.equals("compartment")) { 3209 throw new FHIRException("Cannot call addChild on a singleton property Conformance.compartment"); 3210 } else 3211 return super.addChild(name); 3212 } 3213 3214 public ConformanceRestComponent copy() { 3215 ConformanceRestComponent dst = new ConformanceRestComponent(); 3216 copyValues(dst); 3217 dst.mode = mode == null ? null : mode.copy(); 3218 dst.documentation = documentation == null ? null : documentation.copy(); 3219 dst.security = security == null ? null : security.copy(); 3220 if (resource != null) { 3221 dst.resource = new ArrayList<ConformanceRestResourceComponent>(); 3222 for (ConformanceRestResourceComponent i : resource) 3223 dst.resource.add(i.copy()); 3224 } 3225 ; 3226 if (interaction != null) { 3227 dst.interaction = new ArrayList<SystemInteractionComponent>(); 3228 for (SystemInteractionComponent i : interaction) 3229 dst.interaction.add(i.copy()); 3230 } 3231 ; 3232 dst.transactionMode = transactionMode == null ? null : transactionMode.copy(); 3233 if (searchParam != null) { 3234 dst.searchParam = new ArrayList<ConformanceRestResourceSearchParamComponent>(); 3235 for (ConformanceRestResourceSearchParamComponent i : searchParam) 3236 dst.searchParam.add(i.copy()); 3237 } 3238 ; 3239 if (operation != null) { 3240 dst.operation = new ArrayList<ConformanceRestOperationComponent>(); 3241 for (ConformanceRestOperationComponent i : operation) 3242 dst.operation.add(i.copy()); 3243 } 3244 ; 3245 if (compartment != null) { 3246 dst.compartment = new ArrayList<UriType>(); 3247 for (UriType i : compartment) 3248 dst.compartment.add(i.copy()); 3249 } 3250 ; 3251 return dst; 3252 } 3253 3254 @Override 3255 public boolean equalsDeep(Base other) { 3256 if (!super.equalsDeep(other)) 3257 return false; 3258 if (!(other instanceof ConformanceRestComponent)) 3259 return false; 3260 ConformanceRestComponent o = (ConformanceRestComponent) other; 3261 return compareDeep(mode, o.mode, true) && compareDeep(documentation, o.documentation, true) 3262 && compareDeep(security, o.security, true) && compareDeep(resource, o.resource, true) 3263 && compareDeep(interaction, o.interaction, true) && compareDeep(transactionMode, o.transactionMode, true) 3264 && compareDeep(searchParam, o.searchParam, true) && compareDeep(operation, o.operation, true) 3265 && compareDeep(compartment, o.compartment, true); 3266 } 3267 3268 @Override 3269 public boolean equalsShallow(Base other) { 3270 if (!super.equalsShallow(other)) 3271 return false; 3272 if (!(other instanceof ConformanceRestComponent)) 3273 return false; 3274 ConformanceRestComponent o = (ConformanceRestComponent) other; 3275 return compareValues(mode, o.mode, true) && compareValues(documentation, o.documentation, true) 3276 && compareValues(transactionMode, o.transactionMode, true) && compareValues(compartment, o.compartment, true); 3277 } 3278 3279 public boolean isEmpty() { 3280 return super.isEmpty() && (mode == null || mode.isEmpty()) && (documentation == null || documentation.isEmpty()) 3281 && (security == null || security.isEmpty()) && (resource == null || resource.isEmpty()) 3282 && (interaction == null || interaction.isEmpty()) && (transactionMode == null || transactionMode.isEmpty()) 3283 && (searchParam == null || searchParam.isEmpty()) && (operation == null || operation.isEmpty()) 3284 && (compartment == null || compartment.isEmpty()); 3285 } 3286 3287 public String fhirType() { 3288 return "Conformance.rest"; 3289 3290 } 3291 3292 } 3293 3294 @Block() 3295 public static class ConformanceRestSecurityComponent extends BackboneElement implements IBaseBackboneElement { 3296 /** 3297 * Server adds CORS headers when responding to requests - this enables 3298 * javascript applications to use the server. 3299 */ 3300 @Child(name = "cors", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 3301 @Description(shortDefinition = "Adds CORS Headers (http://enable-cors.org/)", formalDefinition = "Server adds CORS headers when responding to requests - this enables javascript applications to use the server.") 3302 protected BooleanType cors; 3303 3304 /** 3305 * Types of security services are supported/required by the system. 3306 */ 3307 @Child(name = "service", type = { 3308 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3309 @Description(shortDefinition = "OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | Certificates", formalDefinition = "Types of security services are supported/required by the system.") 3310 protected List<CodeableConcept> service; 3311 3312 /** 3313 * General description of how security works. 3314 */ 3315 @Child(name = "description", type = { 3316 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3317 @Description(shortDefinition = "General description of how security works", formalDefinition = "General description of how security works.") 3318 protected StringType description; 3319 3320 /** 3321 * Certificates associated with security profiles. 3322 */ 3323 @Child(name = "certificate", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3324 @Description(shortDefinition = "Certificates associated with security profiles", formalDefinition = "Certificates associated with security profiles.") 3325 protected List<ConformanceRestSecurityCertificateComponent> certificate; 3326 3327 private static final long serialVersionUID = 391663952L; 3328 3329 /* 3330 * Constructor 3331 */ 3332 public ConformanceRestSecurityComponent() { 3333 super(); 3334 } 3335 3336 /** 3337 * @return {@link #cors} (Server adds CORS headers when responding to requests - 3338 * this enables javascript applications to use the server.). This is the 3339 * underlying object with id, value and extensions. The accessor 3340 * "getCors" gives direct access to the value 3341 */ 3342 public BooleanType getCorsElement() { 3343 if (this.cors == null) 3344 if (Configuration.errorOnAutoCreate()) 3345 throw new Error("Attempt to auto-create ConformanceRestSecurityComponent.cors"); 3346 else if (Configuration.doAutoCreate()) 3347 this.cors = new BooleanType(); // bb 3348 return this.cors; 3349 } 3350 3351 public boolean hasCorsElement() { 3352 return this.cors != null && !this.cors.isEmpty(); 3353 } 3354 3355 public boolean hasCors() { 3356 return this.cors != null && !this.cors.isEmpty(); 3357 } 3358 3359 /** 3360 * @param value {@link #cors} (Server adds CORS headers when responding to 3361 * requests - this enables javascript applications to use the 3362 * server.). This is the underlying object with id, value and 3363 * extensions. The accessor "getCors" gives direct access to the 3364 * value 3365 */ 3366 public ConformanceRestSecurityComponent setCorsElement(BooleanType value) { 3367 this.cors = value; 3368 return this; 3369 } 3370 3371 /** 3372 * @return Server adds CORS headers when responding to requests - this enables 3373 * javascript applications to use the server. 3374 */ 3375 public boolean getCors() { 3376 return this.cors == null || this.cors.isEmpty() ? false : this.cors.getValue(); 3377 } 3378 3379 /** 3380 * @param value Server adds CORS headers when responding to requests - this 3381 * enables javascript applications to use the server. 3382 */ 3383 public ConformanceRestSecurityComponent setCors(boolean value) { 3384 if (this.cors == null) 3385 this.cors = new BooleanType(); 3386 this.cors.setValue(value); 3387 return this; 3388 } 3389 3390 /** 3391 * @return {@link #service} (Types of security services are supported/required 3392 * by the system.) 3393 */ 3394 public List<CodeableConcept> getService() { 3395 if (this.service == null) 3396 this.service = new ArrayList<CodeableConcept>(); 3397 return this.service; 3398 } 3399 3400 public boolean hasService() { 3401 if (this.service == null) 3402 return false; 3403 for (CodeableConcept item : this.service) 3404 if (!item.isEmpty()) 3405 return true; 3406 return false; 3407 } 3408 3409 /** 3410 * @return {@link #service} (Types of security services are supported/required 3411 * by the system.) 3412 */ 3413 // syntactic sugar 3414 public CodeableConcept addService() { // 3 3415 CodeableConcept t = new CodeableConcept(); 3416 if (this.service == null) 3417 this.service = new ArrayList<CodeableConcept>(); 3418 this.service.add(t); 3419 return t; 3420 } 3421 3422 // syntactic sugar 3423 public ConformanceRestSecurityComponent addService(CodeableConcept t) { // 3 3424 if (t == null) 3425 return this; 3426 if (this.service == null) 3427 this.service = new ArrayList<CodeableConcept>(); 3428 this.service.add(t); 3429 return this; 3430 } 3431 3432 /** 3433 * @return {@link #description} (General description of how security works.). 3434 * This is the underlying object with id, value and extensions. The 3435 * accessor "getDescription" gives direct access to the value 3436 */ 3437 public StringType getDescriptionElement() { 3438 if (this.description == null) 3439 if (Configuration.errorOnAutoCreate()) 3440 throw new Error("Attempt to auto-create ConformanceRestSecurityComponent.description"); 3441 else if (Configuration.doAutoCreate()) 3442 this.description = new StringType(); // bb 3443 return this.description; 3444 } 3445 3446 public boolean hasDescriptionElement() { 3447 return this.description != null && !this.description.isEmpty(); 3448 } 3449 3450 public boolean hasDescription() { 3451 return this.description != null && !this.description.isEmpty(); 3452 } 3453 3454 /** 3455 * @param value {@link #description} (General description of how security 3456 * works.). This is the underlying object with id, value and 3457 * extensions. The accessor "getDescription" gives direct access to 3458 * the value 3459 */ 3460 public ConformanceRestSecurityComponent setDescriptionElement(StringType value) { 3461 this.description = value; 3462 return this; 3463 } 3464 3465 /** 3466 * @return General description of how security works. 3467 */ 3468 public String getDescription() { 3469 return this.description == null ? null : this.description.getValue(); 3470 } 3471 3472 /** 3473 * @param value General description of how security works. 3474 */ 3475 public ConformanceRestSecurityComponent setDescription(String value) { 3476 if (Utilities.noString(value)) 3477 this.description = null; 3478 else { 3479 if (this.description == null) 3480 this.description = new StringType(); 3481 this.description.setValue(value); 3482 } 3483 return this; 3484 } 3485 3486 /** 3487 * @return {@link #certificate} (Certificates associated with security 3488 * profiles.) 3489 */ 3490 public List<ConformanceRestSecurityCertificateComponent> getCertificate() { 3491 if (this.certificate == null) 3492 this.certificate = new ArrayList<ConformanceRestSecurityCertificateComponent>(); 3493 return this.certificate; 3494 } 3495 3496 public boolean hasCertificate() { 3497 if (this.certificate == null) 3498 return false; 3499 for (ConformanceRestSecurityCertificateComponent item : this.certificate) 3500 if (!item.isEmpty()) 3501 return true; 3502 return false; 3503 } 3504 3505 /** 3506 * @return {@link #certificate} (Certificates associated with security 3507 * profiles.) 3508 */ 3509 // syntactic sugar 3510 public ConformanceRestSecurityCertificateComponent addCertificate() { // 3 3511 ConformanceRestSecurityCertificateComponent t = new ConformanceRestSecurityCertificateComponent(); 3512 if (this.certificate == null) 3513 this.certificate = new ArrayList<ConformanceRestSecurityCertificateComponent>(); 3514 this.certificate.add(t); 3515 return t; 3516 } 3517 3518 // syntactic sugar 3519 public ConformanceRestSecurityComponent addCertificate(ConformanceRestSecurityCertificateComponent t) { // 3 3520 if (t == null) 3521 return this; 3522 if (this.certificate == null) 3523 this.certificate = new ArrayList<ConformanceRestSecurityCertificateComponent>(); 3524 this.certificate.add(t); 3525 return this; 3526 } 3527 3528 protected void listChildren(List<Property> childrenList) { 3529 super.listChildren(childrenList); 3530 childrenList.add(new Property("cors", "boolean", 3531 "Server adds CORS headers when responding to requests - this enables javascript applications to use the server.", 3532 0, java.lang.Integer.MAX_VALUE, cors)); 3533 childrenList.add(new Property("service", "CodeableConcept", 3534 "Types of security services are supported/required by the system.", 0, java.lang.Integer.MAX_VALUE, service)); 3535 childrenList.add(new Property("description", "string", "General description of how security works.", 0, 3536 java.lang.Integer.MAX_VALUE, description)); 3537 childrenList.add(new Property("certificate", "", "Certificates associated with security profiles.", 0, 3538 java.lang.Integer.MAX_VALUE, certificate)); 3539 } 3540 3541 @Override 3542 public void setProperty(String name, Base value) throws FHIRException { 3543 if (name.equals("cors")) 3544 this.cors = castToBoolean(value); // BooleanType 3545 else if (name.equals("service")) 3546 this.getService().add(castToCodeableConcept(value)); 3547 else if (name.equals("description")) 3548 this.description = castToString(value); // StringType 3549 else if (name.equals("certificate")) 3550 this.getCertificate().add((ConformanceRestSecurityCertificateComponent) value); 3551 else 3552 super.setProperty(name, value); 3553 } 3554 3555 @Override 3556 public Base addChild(String name) throws FHIRException { 3557 if (name.equals("cors")) { 3558 throw new FHIRException("Cannot call addChild on a singleton property Conformance.cors"); 3559 } else if (name.equals("service")) { 3560 return addService(); 3561 } else if (name.equals("description")) { 3562 throw new FHIRException("Cannot call addChild on a singleton property Conformance.description"); 3563 } else if (name.equals("certificate")) { 3564 return addCertificate(); 3565 } else 3566 return super.addChild(name); 3567 } 3568 3569 public ConformanceRestSecurityComponent copy() { 3570 ConformanceRestSecurityComponent dst = new ConformanceRestSecurityComponent(); 3571 copyValues(dst); 3572 dst.cors = cors == null ? null : cors.copy(); 3573 if (service != null) { 3574 dst.service = new ArrayList<CodeableConcept>(); 3575 for (CodeableConcept i : service) 3576 dst.service.add(i.copy()); 3577 } 3578 ; 3579 dst.description = description == null ? null : description.copy(); 3580 if (certificate != null) { 3581 dst.certificate = new ArrayList<ConformanceRestSecurityCertificateComponent>(); 3582 for (ConformanceRestSecurityCertificateComponent i : certificate) 3583 dst.certificate.add(i.copy()); 3584 } 3585 ; 3586 return dst; 3587 } 3588 3589 @Override 3590 public boolean equalsDeep(Base other) { 3591 if (!super.equalsDeep(other)) 3592 return false; 3593 if (!(other instanceof ConformanceRestSecurityComponent)) 3594 return false; 3595 ConformanceRestSecurityComponent o = (ConformanceRestSecurityComponent) other; 3596 return compareDeep(cors, o.cors, true) && compareDeep(service, o.service, true) 3597 && compareDeep(description, o.description, true) && compareDeep(certificate, o.certificate, true); 3598 } 3599 3600 @Override 3601 public boolean equalsShallow(Base other) { 3602 if (!super.equalsShallow(other)) 3603 return false; 3604 if (!(other instanceof ConformanceRestSecurityComponent)) 3605 return false; 3606 ConformanceRestSecurityComponent o = (ConformanceRestSecurityComponent) other; 3607 return compareValues(cors, o.cors, true) && compareValues(description, o.description, true); 3608 } 3609 3610 public boolean isEmpty() { 3611 return super.isEmpty() && (cors == null || cors.isEmpty()) && (service == null || service.isEmpty()) 3612 && (description == null || description.isEmpty()) && (certificate == null || certificate.isEmpty()); 3613 } 3614 3615 public String fhirType() { 3616 return "Conformance.rest.security"; 3617 3618 } 3619 3620 } 3621 3622 @Block() 3623 public static class ConformanceRestSecurityCertificateComponent extends BackboneElement 3624 implements IBaseBackboneElement { 3625 /** 3626 * Mime type for certificate. 3627 */ 3628 @Child(name = "type", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 3629 @Description(shortDefinition = "Mime type for certificate", formalDefinition = "Mime type for certificate.") 3630 protected CodeType type; 3631 3632 /** 3633 * Actual certificate. 3634 */ 3635 @Child(name = "blob", type = { 3636 Base64BinaryType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3637 @Description(shortDefinition = "Actual certificate", formalDefinition = "Actual certificate.") 3638 protected Base64BinaryType blob; 3639 3640 private static final long serialVersionUID = 2092655854L; 3641 3642 /* 3643 * Constructor 3644 */ 3645 public ConformanceRestSecurityCertificateComponent() { 3646 super(); 3647 } 3648 3649 /** 3650 * @return {@link #type} (Mime type for certificate.). This is the underlying 3651 * object with id, value and extensions. The accessor "getType" gives 3652 * direct access to the value 3653 */ 3654 public CodeType getTypeElement() { 3655 if (this.type == null) 3656 if (Configuration.errorOnAutoCreate()) 3657 throw new Error("Attempt to auto-create ConformanceRestSecurityCertificateComponent.type"); 3658 else if (Configuration.doAutoCreate()) 3659 this.type = new CodeType(); // bb 3660 return this.type; 3661 } 3662 3663 public boolean hasTypeElement() { 3664 return this.type != null && !this.type.isEmpty(); 3665 } 3666 3667 public boolean hasType() { 3668 return this.type != null && !this.type.isEmpty(); 3669 } 3670 3671 /** 3672 * @param value {@link #type} (Mime type for certificate.). This is the 3673 * underlying object with id, value and extensions. The accessor 3674 * "getType" gives direct access to the value 3675 */ 3676 public ConformanceRestSecurityCertificateComponent setTypeElement(CodeType value) { 3677 this.type = value; 3678 return this; 3679 } 3680 3681 /** 3682 * @return Mime type for certificate. 3683 */ 3684 public String getType() { 3685 return this.type == null ? null : this.type.getValue(); 3686 } 3687 3688 /** 3689 * @param value Mime type for certificate. 3690 */ 3691 public ConformanceRestSecurityCertificateComponent setType(String value) { 3692 if (Utilities.noString(value)) 3693 this.type = null; 3694 else { 3695 if (this.type == null) 3696 this.type = new CodeType(); 3697 this.type.setValue(value); 3698 } 3699 return this; 3700 } 3701 3702 /** 3703 * @return {@link #blob} (Actual certificate.). This is the underlying object 3704 * with id, value and extensions. The accessor "getBlob" gives direct 3705 * access to the value 3706 */ 3707 public Base64BinaryType getBlobElement() { 3708 if (this.blob == null) 3709 if (Configuration.errorOnAutoCreate()) 3710 throw new Error("Attempt to auto-create ConformanceRestSecurityCertificateComponent.blob"); 3711 else if (Configuration.doAutoCreate()) 3712 this.blob = new Base64BinaryType(); // bb 3713 return this.blob; 3714 } 3715 3716 public boolean hasBlobElement() { 3717 return this.blob != null && !this.blob.isEmpty(); 3718 } 3719 3720 public boolean hasBlob() { 3721 return this.blob != null && !this.blob.isEmpty(); 3722 } 3723 3724 /** 3725 * @param value {@link #blob} (Actual certificate.). This is the underlying 3726 * object with id, value and extensions. The accessor "getBlob" 3727 * gives direct access to the value 3728 */ 3729 public ConformanceRestSecurityCertificateComponent setBlobElement(Base64BinaryType value) { 3730 this.blob = value; 3731 return this; 3732 } 3733 3734 /** 3735 * @return Actual certificate. 3736 */ 3737 public byte[] getBlob() { 3738 return this.blob == null ? null : this.blob.getValue(); 3739 } 3740 3741 /** 3742 * @param value Actual certificate. 3743 */ 3744 public ConformanceRestSecurityCertificateComponent setBlob(byte[] value) { 3745 if (value == null) 3746 this.blob = null; 3747 else { 3748 if (this.blob == null) 3749 this.blob = new Base64BinaryType(); 3750 this.blob.setValue(value); 3751 } 3752 return this; 3753 } 3754 3755 protected void listChildren(List<Property> childrenList) { 3756 super.listChildren(childrenList); 3757 childrenList 3758 .add(new Property("type", "code", "Mime type for certificate.", 0, java.lang.Integer.MAX_VALUE, type)); 3759 childrenList 3760 .add(new Property("blob", "base64Binary", "Actual certificate.", 0, java.lang.Integer.MAX_VALUE, blob)); 3761 } 3762 3763 @Override 3764 public void setProperty(String name, Base value) throws FHIRException { 3765 if (name.equals("type")) 3766 this.type = castToCode(value); // CodeType 3767 else if (name.equals("blob")) 3768 this.blob = castToBase64Binary(value); // Base64BinaryType 3769 else 3770 super.setProperty(name, value); 3771 } 3772 3773 @Override 3774 public Base addChild(String name) throws FHIRException { 3775 if (name.equals("type")) { 3776 throw new FHIRException("Cannot call addChild on a singleton property Conformance.type"); 3777 } else if (name.equals("blob")) { 3778 throw new FHIRException("Cannot call addChild on a singleton property Conformance.blob"); 3779 } else 3780 return super.addChild(name); 3781 } 3782 3783 public ConformanceRestSecurityCertificateComponent copy() { 3784 ConformanceRestSecurityCertificateComponent dst = new ConformanceRestSecurityCertificateComponent(); 3785 copyValues(dst); 3786 dst.type = type == null ? null : type.copy(); 3787 dst.blob = blob == null ? null : blob.copy(); 3788 return dst; 3789 } 3790 3791 @Override 3792 public boolean equalsDeep(Base other) { 3793 if (!super.equalsDeep(other)) 3794 return false; 3795 if (!(other instanceof ConformanceRestSecurityCertificateComponent)) 3796 return false; 3797 ConformanceRestSecurityCertificateComponent o = (ConformanceRestSecurityCertificateComponent) other; 3798 return compareDeep(type, o.type, true) && compareDeep(blob, o.blob, true); 3799 } 3800 3801 @Override 3802 public boolean equalsShallow(Base other) { 3803 if (!super.equalsShallow(other)) 3804 return false; 3805 if (!(other instanceof ConformanceRestSecurityCertificateComponent)) 3806 return false; 3807 ConformanceRestSecurityCertificateComponent o = (ConformanceRestSecurityCertificateComponent) other; 3808 return compareValues(type, o.type, true) && compareValues(blob, o.blob, true); 3809 } 3810 3811 public boolean isEmpty() { 3812 return super.isEmpty() && (type == null || type.isEmpty()) && (blob == null || blob.isEmpty()); 3813 } 3814 3815 public String fhirType() { 3816 return "Conformance.rest.security.certificate"; 3817 3818 } 3819 3820 } 3821 3822 @Block() 3823 public static class ConformanceRestResourceComponent extends BackboneElement implements IBaseBackboneElement { 3824 /** 3825 * A type of resource exposed via the restful interface. 3826 */ 3827 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 3828 @Description(shortDefinition = "A resource type that is supported", formalDefinition = "A type of resource exposed via the restful interface.") 3829 protected CodeType type; 3830 3831 /** 3832 * A specification of the profile that describes the solution's overall support 3833 * for the resource, including any constraints on cardinality, bindings, lengths 3834 * or other limitations. See further discussion in [Using 3835 * Profiles]{profiling.html#profile-uses}. 3836 */ 3837 @Child(name = "profile", type = { 3838 StructureDefinition.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3839 @Description(shortDefinition = "Base System profile for all uses of resource", formalDefinition = "A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles]{profiling.html#profile-uses}.") 3840 protected Reference profile; 3841 3842 /** 3843 * The actual object that is the target of the reference (A specification of the 3844 * profile that describes the solution's overall support for the resource, 3845 * including any constraints on cardinality, bindings, lengths or other 3846 * limitations. See further discussion in [Using 3847 * Profiles]{profiling.html#profile-uses}.) 3848 */ 3849 protected StructureDefinition profileTarget; 3850 3851 /** 3852 * Identifies a restful operation supported by the solution. 3853 */ 3854 @Child(name = "interaction", type = {}, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3855 @Description(shortDefinition = "What operations are supported?", formalDefinition = "Identifies a restful operation supported by the solution.") 3856 protected List<ResourceInteractionComponent> interaction; 3857 3858 /** 3859 * This field is set to no-version to specify that the system does not support 3860 * (server) or use (client) versioning for this resource type. If this has some 3861 * other value, the server must at least correctly track and populate the 3862 * versionId meta-property on resources. If the value is 'versioned-update', 3863 * then the server supports all the versioning features, including using e-tags 3864 * for version integrity in the API. 3865 */ 3866 @Child(name = "versioning", type = { 3867 CodeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 3868 @Description(shortDefinition = "no-version | versioned | versioned-update", formalDefinition = "This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.") 3869 protected Enumeration<ResourceVersionPolicy> versioning; 3870 3871 /** 3872 * A flag for whether the server is able to return past versions as part of the 3873 * vRead operation. 3874 */ 3875 @Child(name = "readHistory", type = { 3876 BooleanType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 3877 @Description(shortDefinition = "Whether vRead can return past versions", formalDefinition = "A flag for whether the server is able to return past versions as part of the vRead operation.") 3878 protected BooleanType readHistory; 3879 3880 /** 3881 * A flag to indicate that the server allows or needs to allow the client to 3882 * create new identities on the server (e.g. that is, the client PUTs to a 3883 * location where there is no existing resource). Allowing this operation means 3884 * that the server allows the client to create new identities on the server. 3885 */ 3886 @Child(name = "updateCreate", type = { 3887 BooleanType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 3888 @Description(shortDefinition = "If update can commit to a new identity", formalDefinition = "A flag to indicate that the server allows or needs to allow the client to create new identities on the server (e.g. that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.") 3889 protected BooleanType updateCreate; 3890 3891 /** 3892 * A flag that indicates that the server supports conditional create. 3893 */ 3894 @Child(name = "conditionalCreate", type = { 3895 BooleanType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 3896 @Description(shortDefinition = "If allows/uses conditional create", formalDefinition = "A flag that indicates that the server supports conditional create.") 3897 protected BooleanType conditionalCreate; 3898 3899 /** 3900 * A flag that indicates that the server supports conditional update. 3901 */ 3902 @Child(name = "conditionalUpdate", type = { 3903 BooleanType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 3904 @Description(shortDefinition = "If allows/uses conditional update", formalDefinition = "A flag that indicates that the server supports conditional update.") 3905 protected BooleanType conditionalUpdate; 3906 3907 /** 3908 * A code that indicates how the server supports conditional delete. 3909 */ 3910 @Child(name = "conditionalDelete", type = { 3911 CodeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 3912 @Description(shortDefinition = "not-supported | single | multiple - how conditional delete is supported", formalDefinition = "A code that indicates how the server supports conditional delete.") 3913 protected Enumeration<ConditionalDeleteStatus> conditionalDelete; 3914 3915 /** 3916 * A list of _include values supported by the server. 3917 */ 3918 @Child(name = "searchInclude", type = { 3919 StringType.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3920 @Description(shortDefinition = "_include values supported by the server", formalDefinition = "A list of _include values supported by the server.") 3921 protected List<StringType> searchInclude; 3922 3923 /** 3924 * A list of _revinclude (reverse include) values supported by the server. 3925 */ 3926 @Child(name = "searchRevInclude", type = { 3927 StringType.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3928 @Description(shortDefinition = "_revinclude values supported by the server", formalDefinition = "A list of _revinclude (reverse include) values supported by the server.") 3929 protected List<StringType> searchRevInclude; 3930 3931 /** 3932 * Search parameters for implementations to support and/or make use of - either 3933 * references to ones defined in the specification, or additional ones defined 3934 * for/by the implementation. 3935 */ 3936 @Child(name = "searchParam", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3937 @Description(shortDefinition = "Search params supported by implementation", formalDefinition = "Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.") 3938 protected List<ConformanceRestResourceSearchParamComponent> searchParam; 3939 3940 private static final long serialVersionUID = 1781959905L; 3941 3942 /* 3943 * Constructor 3944 */ 3945 public ConformanceRestResourceComponent() { 3946 super(); 3947 } 3948 3949 /* 3950 * Constructor 3951 */ 3952 public ConformanceRestResourceComponent(CodeType type) { 3953 super(); 3954 this.type = type; 3955 } 3956 3957 /** 3958 * @return {@link #type} (A type of resource exposed via the restful 3959 * interface.). This is the underlying object with id, value and 3960 * extensions. The accessor "getType" gives direct access to the value 3961 */ 3962 public CodeType getTypeElement() { 3963 if (this.type == null) 3964 if (Configuration.errorOnAutoCreate()) 3965 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.type"); 3966 else if (Configuration.doAutoCreate()) 3967 this.type = new CodeType(); // bb 3968 return this.type; 3969 } 3970 3971 public boolean hasTypeElement() { 3972 return this.type != null && !this.type.isEmpty(); 3973 } 3974 3975 public boolean hasType() { 3976 return this.type != null && !this.type.isEmpty(); 3977 } 3978 3979 /** 3980 * @param value {@link #type} (A type of resource exposed via the restful 3981 * interface.). This is the underlying object with id, value and 3982 * extensions. The accessor "getType" gives direct access to the 3983 * value 3984 */ 3985 public ConformanceRestResourceComponent setTypeElement(CodeType value) { 3986 this.type = value; 3987 return this; 3988 } 3989 3990 /** 3991 * @return A type of resource exposed via the restful interface. 3992 */ 3993 public String getType() { 3994 return this.type == null ? null : this.type.getValue(); 3995 } 3996 3997 /** 3998 * @param value A type of resource exposed via the restful interface. 3999 */ 4000 public ConformanceRestResourceComponent setType(String value) { 4001 if (this.type == null) 4002 this.type = new CodeType(); 4003 this.type.setValue(value); 4004 return this; 4005 } 4006 4007 /** 4008 * @return {@link #profile} (A specification of the profile that describes the 4009 * solution's overall support for the resource, including any 4010 * constraints on cardinality, bindings, lengths or other limitations. 4011 * See further discussion in [Using 4012 * Profiles]{profiling.html#profile-uses}.) 4013 */ 4014 public Reference getProfile() { 4015 if (this.profile == null) 4016 if (Configuration.errorOnAutoCreate()) 4017 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.profile"); 4018 else if (Configuration.doAutoCreate()) 4019 this.profile = new Reference(); // cc 4020 return this.profile; 4021 } 4022 4023 public boolean hasProfile() { 4024 return this.profile != null && !this.profile.isEmpty(); 4025 } 4026 4027 /** 4028 * @param value {@link #profile} (A specification of the profile that describes 4029 * the solution's overall support for the resource, including any 4030 * constraints on cardinality, bindings, lengths or other 4031 * limitations. See further discussion in [Using 4032 * Profiles]{profiling.html#profile-uses}.) 4033 */ 4034 public ConformanceRestResourceComponent setProfile(Reference value) { 4035 this.profile = value; 4036 return this; 4037 } 4038 4039 /** 4040 * @return {@link #profile} The actual object that is the target of the 4041 * reference. The reference library doesn't populate this, but you can 4042 * use it to hold the resource if you resolve it. (A specification of 4043 * the profile that describes the solution's overall support for the 4044 * resource, including any constraints on cardinality, bindings, lengths 4045 * or other limitations. See further discussion in [Using 4046 * Profiles]{profiling.html#profile-uses}.) 4047 */ 4048 public StructureDefinition getProfileTarget() { 4049 if (this.profileTarget == null) 4050 if (Configuration.errorOnAutoCreate()) 4051 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.profile"); 4052 else if (Configuration.doAutoCreate()) 4053 this.profileTarget = new StructureDefinition(); // aa 4054 return this.profileTarget; 4055 } 4056 4057 /** 4058 * @param value {@link #profile} The actual object that is the target of the 4059 * reference. The reference library doesn't use these, but you can 4060 * use it to hold the resource if you resolve it. (A specification 4061 * of the profile that describes the solution's overall support for 4062 * the resource, including any constraints on cardinality, 4063 * bindings, lengths or other limitations. See further discussion 4064 * in [Using Profiles]{profiling.html#profile-uses}.) 4065 */ 4066 public ConformanceRestResourceComponent setProfileTarget(StructureDefinition value) { 4067 this.profileTarget = value; 4068 return this; 4069 } 4070 4071 /** 4072 * @return {@link #interaction} (Identifies a restful operation supported by the 4073 * solution.) 4074 */ 4075 public List<ResourceInteractionComponent> getInteraction() { 4076 if (this.interaction == null) 4077 this.interaction = new ArrayList<ResourceInteractionComponent>(); 4078 return this.interaction; 4079 } 4080 4081 public boolean hasInteraction() { 4082 if (this.interaction == null) 4083 return false; 4084 for (ResourceInteractionComponent item : this.interaction) 4085 if (!item.isEmpty()) 4086 return true; 4087 return false; 4088 } 4089 4090 /** 4091 * @return {@link #interaction} (Identifies a restful operation supported by the 4092 * solution.) 4093 */ 4094 // syntactic sugar 4095 public ResourceInteractionComponent addInteraction() { // 3 4096 ResourceInteractionComponent t = new ResourceInteractionComponent(); 4097 if (this.interaction == null) 4098 this.interaction = new ArrayList<ResourceInteractionComponent>(); 4099 this.interaction.add(t); 4100 return t; 4101 } 4102 4103 // syntactic sugar 4104 public ConformanceRestResourceComponent addInteraction(ResourceInteractionComponent t) { // 3 4105 if (t == null) 4106 return this; 4107 if (this.interaction == null) 4108 this.interaction = new ArrayList<ResourceInteractionComponent>(); 4109 this.interaction.add(t); 4110 return this; 4111 } 4112 4113 /** 4114 * @return {@link #versioning} (This field is set to no-version to specify that 4115 * the system does not support (server) or use (client) versioning for 4116 * this resource type. If this has some other value, the server must at 4117 * least correctly track and populate the versionId meta-property on 4118 * resources. If the value is 'versioned-update', then the server 4119 * supports all the versioning features, including using e-tags for 4120 * version integrity in the API.). This is the underlying object with 4121 * id, value and extensions. The accessor "getVersioning" gives direct 4122 * access to the value 4123 */ 4124 public Enumeration<ResourceVersionPolicy> getVersioningElement() { 4125 if (this.versioning == null) 4126 if (Configuration.errorOnAutoCreate()) 4127 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.versioning"); 4128 else if (Configuration.doAutoCreate()) 4129 this.versioning = new Enumeration<ResourceVersionPolicy>(new ResourceVersionPolicyEnumFactory()); // bb 4130 return this.versioning; 4131 } 4132 4133 public boolean hasVersioningElement() { 4134 return this.versioning != null && !this.versioning.isEmpty(); 4135 } 4136 4137 public boolean hasVersioning() { 4138 return this.versioning != null && !this.versioning.isEmpty(); 4139 } 4140 4141 /** 4142 * @param value {@link #versioning} (This field is set to no-version to specify 4143 * that the system does not support (server) or use (client) 4144 * versioning for this resource type. If this has some other value, 4145 * the server must at least correctly track and populate the 4146 * versionId meta-property on resources. If the value is 4147 * 'versioned-update', then the server supports all the versioning 4148 * features, including using e-tags for version integrity in the 4149 * API.). This is the underlying object with id, value and 4150 * extensions. The accessor "getVersioning" gives direct access to 4151 * the value 4152 */ 4153 public ConformanceRestResourceComponent setVersioningElement(Enumeration<ResourceVersionPolicy> value) { 4154 this.versioning = value; 4155 return this; 4156 } 4157 4158 /** 4159 * @return This field is set to no-version to specify that the system does not 4160 * support (server) or use (client) versioning for this resource type. 4161 * If this has some other value, the server must at least correctly 4162 * track and populate the versionId meta-property on resources. If the 4163 * value is 'versioned-update', then the server supports all the 4164 * versioning features, including using e-tags for version integrity in 4165 * the API. 4166 */ 4167 public ResourceVersionPolicy getVersioning() { 4168 return this.versioning == null ? null : this.versioning.getValue(); 4169 } 4170 4171 /** 4172 * @param value This field is set to no-version to specify that the system does 4173 * not support (server) or use (client) versioning for this 4174 * resource type. If this has some other value, the server must at 4175 * least correctly track and populate the versionId meta-property 4176 * on resources. If the value is 'versioned-update', then the 4177 * server supports all the versioning features, including using 4178 * e-tags for version integrity in the API. 4179 */ 4180 public ConformanceRestResourceComponent setVersioning(ResourceVersionPolicy value) { 4181 if (value == null) 4182 this.versioning = null; 4183 else { 4184 if (this.versioning == null) 4185 this.versioning = new Enumeration<ResourceVersionPolicy>(new ResourceVersionPolicyEnumFactory()); 4186 this.versioning.setValue(value); 4187 } 4188 return this; 4189 } 4190 4191 /** 4192 * @return {@link #readHistory} (A flag for whether the server is able to return 4193 * past versions as part of the vRead operation.). This is the 4194 * underlying object with id, value and extensions. The accessor 4195 * "getReadHistory" gives direct access to the value 4196 */ 4197 public BooleanType getReadHistoryElement() { 4198 if (this.readHistory == null) 4199 if (Configuration.errorOnAutoCreate()) 4200 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.readHistory"); 4201 else if (Configuration.doAutoCreate()) 4202 this.readHistory = new BooleanType(); // bb 4203 return this.readHistory; 4204 } 4205 4206 public boolean hasReadHistoryElement() { 4207 return this.readHistory != null && !this.readHistory.isEmpty(); 4208 } 4209 4210 public boolean hasReadHistory() { 4211 return this.readHistory != null && !this.readHistory.isEmpty(); 4212 } 4213 4214 /** 4215 * @param value {@link #readHistory} (A flag for whether the server is able to 4216 * return past versions as part of the vRead operation.). This is 4217 * the underlying object with id, value and extensions. The 4218 * accessor "getReadHistory" gives direct access to the value 4219 */ 4220 public ConformanceRestResourceComponent setReadHistoryElement(BooleanType value) { 4221 this.readHistory = value; 4222 return this; 4223 } 4224 4225 /** 4226 * @return A flag for whether the server is able to return past versions as part 4227 * of the vRead operation. 4228 */ 4229 public boolean getReadHistory() { 4230 return this.readHistory == null || this.readHistory.isEmpty() ? false : this.readHistory.getValue(); 4231 } 4232 4233 /** 4234 * @param value A flag for whether the server is able to return past versions as 4235 * part of the vRead operation. 4236 */ 4237 public ConformanceRestResourceComponent setReadHistory(boolean value) { 4238 if (this.readHistory == null) 4239 this.readHistory = new BooleanType(); 4240 this.readHistory.setValue(value); 4241 return this; 4242 } 4243 4244 /** 4245 * @return {@link #updateCreate} (A flag to indicate that the server allows or 4246 * needs to allow the client to create new identities on the server 4247 * (e.g. that is, the client PUTs to a location where there is no 4248 * existing resource). Allowing this operation means that the server 4249 * allows the client to create new identities on the server.). This is 4250 * the underlying object with id, value and extensions. The accessor 4251 * "getUpdateCreate" gives direct access to the value 4252 */ 4253 public BooleanType getUpdateCreateElement() { 4254 if (this.updateCreate == null) 4255 if (Configuration.errorOnAutoCreate()) 4256 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.updateCreate"); 4257 else if (Configuration.doAutoCreate()) 4258 this.updateCreate = new BooleanType(); // bb 4259 return this.updateCreate; 4260 } 4261 4262 public boolean hasUpdateCreateElement() { 4263 return this.updateCreate != null && !this.updateCreate.isEmpty(); 4264 } 4265 4266 public boolean hasUpdateCreate() { 4267 return this.updateCreate != null && !this.updateCreate.isEmpty(); 4268 } 4269 4270 /** 4271 * @param value {@link #updateCreate} (A flag to indicate that the server allows 4272 * or needs to allow the client to create new identities on the 4273 * server (e.g. that is, the client PUTs to a location where there 4274 * is no existing resource). Allowing this operation means that the 4275 * server allows the client to create new identities on the 4276 * server.). This is the underlying object with id, value and 4277 * extensions. The accessor "getUpdateCreate" gives direct access 4278 * to the value 4279 */ 4280 public ConformanceRestResourceComponent setUpdateCreateElement(BooleanType value) { 4281 this.updateCreate = value; 4282 return this; 4283 } 4284 4285 /** 4286 * @return A flag to indicate that the server allows or needs to allow the 4287 * client to create new identities on the server (e.g. that is, the 4288 * client PUTs to a location where there is no existing resource). 4289 * Allowing this operation means that the server allows the client to 4290 * create new identities on the server. 4291 */ 4292 public boolean getUpdateCreate() { 4293 return this.updateCreate == null || this.updateCreate.isEmpty() ? false : this.updateCreate.getValue(); 4294 } 4295 4296 /** 4297 * @param value A flag to indicate that the server allows or needs to allow the 4298 * client to create new identities on the server (e.g. that is, the 4299 * client PUTs to a location where there is no existing resource). 4300 * Allowing this operation means that the server allows the client 4301 * to create new identities on the server. 4302 */ 4303 public ConformanceRestResourceComponent setUpdateCreate(boolean value) { 4304 if (this.updateCreate == null) 4305 this.updateCreate = new BooleanType(); 4306 this.updateCreate.setValue(value); 4307 return this; 4308 } 4309 4310 /** 4311 * @return {@link #conditionalCreate} (A flag that indicates that the server 4312 * supports conditional create.). This is the underlying object with id, 4313 * value and extensions. The accessor "getConditionalCreate" gives 4314 * direct access to the value 4315 */ 4316 public BooleanType getConditionalCreateElement() { 4317 if (this.conditionalCreate == null) 4318 if (Configuration.errorOnAutoCreate()) 4319 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.conditionalCreate"); 4320 else if (Configuration.doAutoCreate()) 4321 this.conditionalCreate = new BooleanType(); // bb 4322 return this.conditionalCreate; 4323 } 4324 4325 public boolean hasConditionalCreateElement() { 4326 return this.conditionalCreate != null && !this.conditionalCreate.isEmpty(); 4327 } 4328 4329 public boolean hasConditionalCreate() { 4330 return this.conditionalCreate != null && !this.conditionalCreate.isEmpty(); 4331 } 4332 4333 /** 4334 * @param value {@link #conditionalCreate} (A flag that indicates that the 4335 * server supports conditional create.). This is the underlying 4336 * object with id, value and extensions. The accessor 4337 * "getConditionalCreate" gives direct access to the value 4338 */ 4339 public ConformanceRestResourceComponent setConditionalCreateElement(BooleanType value) { 4340 this.conditionalCreate = value; 4341 return this; 4342 } 4343 4344 /** 4345 * @return A flag that indicates that the server supports conditional create. 4346 */ 4347 public boolean getConditionalCreate() { 4348 return this.conditionalCreate == null || this.conditionalCreate.isEmpty() ? false 4349 : this.conditionalCreate.getValue(); 4350 } 4351 4352 /** 4353 * @param value A flag that indicates that the server supports conditional 4354 * create. 4355 */ 4356 public ConformanceRestResourceComponent setConditionalCreate(boolean value) { 4357 if (this.conditionalCreate == null) 4358 this.conditionalCreate = new BooleanType(); 4359 this.conditionalCreate.setValue(value); 4360 return this; 4361 } 4362 4363 /** 4364 * @return {@link #conditionalUpdate} (A flag that indicates that the server 4365 * supports conditional update.). This is the underlying object with id, 4366 * value and extensions. The accessor "getConditionalUpdate" gives 4367 * direct access to the value 4368 */ 4369 public BooleanType getConditionalUpdateElement() { 4370 if (this.conditionalUpdate == null) 4371 if (Configuration.errorOnAutoCreate()) 4372 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.conditionalUpdate"); 4373 else if (Configuration.doAutoCreate()) 4374 this.conditionalUpdate = new BooleanType(); // bb 4375 return this.conditionalUpdate; 4376 } 4377 4378 public boolean hasConditionalUpdateElement() { 4379 return this.conditionalUpdate != null && !this.conditionalUpdate.isEmpty(); 4380 } 4381 4382 public boolean hasConditionalUpdate() { 4383 return this.conditionalUpdate != null && !this.conditionalUpdate.isEmpty(); 4384 } 4385 4386 /** 4387 * @param value {@link #conditionalUpdate} (A flag that indicates that the 4388 * server supports conditional update.). This is the underlying 4389 * object with id, value and extensions. The accessor 4390 * "getConditionalUpdate" gives direct access to the value 4391 */ 4392 public ConformanceRestResourceComponent setConditionalUpdateElement(BooleanType value) { 4393 this.conditionalUpdate = value; 4394 return this; 4395 } 4396 4397 /** 4398 * @return A flag that indicates that the server supports conditional update. 4399 */ 4400 public boolean getConditionalUpdate() { 4401 return this.conditionalUpdate == null || this.conditionalUpdate.isEmpty() ? false 4402 : this.conditionalUpdate.getValue(); 4403 } 4404 4405 /** 4406 * @param value A flag that indicates that the server supports conditional 4407 * update. 4408 */ 4409 public ConformanceRestResourceComponent setConditionalUpdate(boolean value) { 4410 if (this.conditionalUpdate == null) 4411 this.conditionalUpdate = new BooleanType(); 4412 this.conditionalUpdate.setValue(value); 4413 return this; 4414 } 4415 4416 /** 4417 * @return {@link #conditionalDelete} (A code that indicates how the server 4418 * supports conditional delete.). This is the underlying object with id, 4419 * value and extensions. The accessor "getConditionalDelete" gives 4420 * direct access to the value 4421 */ 4422 public Enumeration<ConditionalDeleteStatus> getConditionalDeleteElement() { 4423 if (this.conditionalDelete == null) 4424 if (Configuration.errorOnAutoCreate()) 4425 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.conditionalDelete"); 4426 else if (Configuration.doAutoCreate()) 4427 this.conditionalDelete = new Enumeration<ConditionalDeleteStatus>(new ConditionalDeleteStatusEnumFactory()); // bb 4428 return this.conditionalDelete; 4429 } 4430 4431 public boolean hasConditionalDeleteElement() { 4432 return this.conditionalDelete != null && !this.conditionalDelete.isEmpty(); 4433 } 4434 4435 public boolean hasConditionalDelete() { 4436 return this.conditionalDelete != null && !this.conditionalDelete.isEmpty(); 4437 } 4438 4439 /** 4440 * @param value {@link #conditionalDelete} (A code that indicates how the server 4441 * supports conditional delete.). This is the underlying object 4442 * with id, value and extensions. The accessor 4443 * "getConditionalDelete" gives direct access to the value 4444 */ 4445 public ConformanceRestResourceComponent setConditionalDeleteElement(Enumeration<ConditionalDeleteStatus> value) { 4446 this.conditionalDelete = value; 4447 return this; 4448 } 4449 4450 /** 4451 * @return A code that indicates how the server supports conditional delete. 4452 */ 4453 public ConditionalDeleteStatus getConditionalDelete() { 4454 return this.conditionalDelete == null ? null : this.conditionalDelete.getValue(); 4455 } 4456 4457 /** 4458 * @param value A code that indicates how the server supports conditional 4459 * delete. 4460 */ 4461 public ConformanceRestResourceComponent setConditionalDelete(ConditionalDeleteStatus value) { 4462 if (value == null) 4463 this.conditionalDelete = null; 4464 else { 4465 if (this.conditionalDelete == null) 4466 this.conditionalDelete = new Enumeration<ConditionalDeleteStatus>(new ConditionalDeleteStatusEnumFactory()); 4467 this.conditionalDelete.setValue(value); 4468 } 4469 return this; 4470 } 4471 4472 /** 4473 * @return {@link #searchInclude} (A list of _include values supported by the 4474 * server.) 4475 */ 4476 public List<StringType> getSearchInclude() { 4477 if (this.searchInclude == null) 4478 this.searchInclude = new ArrayList<StringType>(); 4479 return this.searchInclude; 4480 } 4481 4482 public boolean hasSearchInclude() { 4483 if (this.searchInclude == null) 4484 return false; 4485 for (StringType item : this.searchInclude) 4486 if (!item.isEmpty()) 4487 return true; 4488 return false; 4489 } 4490 4491 /** 4492 * @return {@link #searchInclude} (A list of _include values supported by the 4493 * server.) 4494 */ 4495 // syntactic sugar 4496 public StringType addSearchIncludeElement() {// 2 4497 StringType t = new StringType(); 4498 if (this.searchInclude == null) 4499 this.searchInclude = new ArrayList<StringType>(); 4500 this.searchInclude.add(t); 4501 return t; 4502 } 4503 4504 /** 4505 * @param value {@link #searchInclude} (A list of _include values supported by 4506 * the server.) 4507 */ 4508 public ConformanceRestResourceComponent addSearchInclude(String value) { // 1 4509 StringType t = new StringType(); 4510 t.setValue(value); 4511 if (this.searchInclude == null) 4512 this.searchInclude = new ArrayList<StringType>(); 4513 this.searchInclude.add(t); 4514 return this; 4515 } 4516 4517 /** 4518 * @param value {@link #searchInclude} (A list of _include values supported by 4519 * the server.) 4520 */ 4521 public boolean hasSearchInclude(String value) { 4522 if (this.searchInclude == null) 4523 return false; 4524 for (StringType v : this.searchInclude) 4525 if (v.equals(value)) // string 4526 return true; 4527 return false; 4528 } 4529 4530 /** 4531 * @return {@link #searchRevInclude} (A list of _revinclude (reverse include) 4532 * values supported by the server.) 4533 */ 4534 public List<StringType> getSearchRevInclude() { 4535 if (this.searchRevInclude == null) 4536 this.searchRevInclude = new ArrayList<StringType>(); 4537 return this.searchRevInclude; 4538 } 4539 4540 public boolean hasSearchRevInclude() { 4541 if (this.searchRevInclude == null) 4542 return false; 4543 for (StringType item : this.searchRevInclude) 4544 if (!item.isEmpty()) 4545 return true; 4546 return false; 4547 } 4548 4549 /** 4550 * @return {@link #searchRevInclude} (A list of _revinclude (reverse include) 4551 * values supported by the server.) 4552 */ 4553 // syntactic sugar 4554 public StringType addSearchRevIncludeElement() {// 2 4555 StringType t = new StringType(); 4556 if (this.searchRevInclude == null) 4557 this.searchRevInclude = new ArrayList<StringType>(); 4558 this.searchRevInclude.add(t); 4559 return t; 4560 } 4561 4562 /** 4563 * @param value {@link #searchRevInclude} (A list of _revinclude (reverse 4564 * include) values supported by the server.) 4565 */ 4566 public ConformanceRestResourceComponent addSearchRevInclude(String value) { // 1 4567 StringType t = new StringType(); 4568 t.setValue(value); 4569 if (this.searchRevInclude == null) 4570 this.searchRevInclude = new ArrayList<StringType>(); 4571 this.searchRevInclude.add(t); 4572 return this; 4573 } 4574 4575 /** 4576 * @param value {@link #searchRevInclude} (A list of _revinclude (reverse 4577 * include) values supported by the server.) 4578 */ 4579 public boolean hasSearchRevInclude(String value) { 4580 if (this.searchRevInclude == null) 4581 return false; 4582 for (StringType v : this.searchRevInclude) 4583 if (v.equals(value)) // string 4584 return true; 4585 return false; 4586 } 4587 4588 /** 4589 * @return {@link #searchParam} (Search parameters for implementations to 4590 * support and/or make use of - either references to ones defined in the 4591 * specification, or additional ones defined for/by the implementation.) 4592 */ 4593 public List<ConformanceRestResourceSearchParamComponent> getSearchParam() { 4594 if (this.searchParam == null) 4595 this.searchParam = new ArrayList<ConformanceRestResourceSearchParamComponent>(); 4596 return this.searchParam; 4597 } 4598 4599 public boolean hasSearchParam() { 4600 if (this.searchParam == null) 4601 return false; 4602 for (ConformanceRestResourceSearchParamComponent item : this.searchParam) 4603 if (!item.isEmpty()) 4604 return true; 4605 return false; 4606 } 4607 4608 /** 4609 * @return {@link #searchParam} (Search parameters for implementations to 4610 * support and/or make use of - either references to ones defined in the 4611 * specification, or additional ones defined for/by the implementation.) 4612 */ 4613 // syntactic sugar 4614 public ConformanceRestResourceSearchParamComponent addSearchParam() { // 3 4615 ConformanceRestResourceSearchParamComponent t = new ConformanceRestResourceSearchParamComponent(); 4616 if (this.searchParam == null) 4617 this.searchParam = new ArrayList<ConformanceRestResourceSearchParamComponent>(); 4618 this.searchParam.add(t); 4619 return t; 4620 } 4621 4622 // syntactic sugar 4623 public ConformanceRestResourceComponent addSearchParam(ConformanceRestResourceSearchParamComponent t) { // 3 4624 if (t == null) 4625 return this; 4626 if (this.searchParam == null) 4627 this.searchParam = new ArrayList<ConformanceRestResourceSearchParamComponent>(); 4628 this.searchParam.add(t); 4629 return this; 4630 } 4631 4632 protected void listChildren(List<Property> childrenList) { 4633 super.listChildren(childrenList); 4634 childrenList.add(new Property("type", "code", "A type of resource exposed via the restful interface.", 0, 4635 java.lang.Integer.MAX_VALUE, type)); 4636 childrenList.add(new Property("profile", "Reference(StructureDefinition)", 4637 "A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles]{profiling.html#profile-uses}.", 4638 0, java.lang.Integer.MAX_VALUE, profile)); 4639 childrenList.add(new Property("interaction", "", "Identifies a restful operation supported by the solution.", 0, 4640 java.lang.Integer.MAX_VALUE, interaction)); 4641 childrenList.add(new Property("versioning", "code", 4642 "This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.", 4643 0, java.lang.Integer.MAX_VALUE, versioning)); 4644 childrenList.add(new Property("readHistory", "boolean", 4645 "A flag for whether the server is able to return past versions as part of the vRead operation.", 0, 4646 java.lang.Integer.MAX_VALUE, readHistory)); 4647 childrenList.add(new Property("updateCreate", "boolean", 4648 "A flag to indicate that the server allows or needs to allow the client to create new identities on the server (e.g. that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.", 4649 0, java.lang.Integer.MAX_VALUE, updateCreate)); 4650 childrenList.add(new Property("conditionalCreate", "boolean", 4651 "A flag that indicates that the server supports conditional create.", 0, java.lang.Integer.MAX_VALUE, 4652 conditionalCreate)); 4653 childrenList.add(new Property("conditionalUpdate", "boolean", 4654 "A flag that indicates that the server supports conditional update.", 0, java.lang.Integer.MAX_VALUE, 4655 conditionalUpdate)); 4656 childrenList.add( 4657 new Property("conditionalDelete", "code", "A code that indicates how the server supports conditional delete.", 4658 0, java.lang.Integer.MAX_VALUE, conditionalDelete)); 4659 childrenList.add(new Property("searchInclude", "string", "A list of _include values supported by the server.", 0, 4660 java.lang.Integer.MAX_VALUE, searchInclude)); 4661 childrenList.add(new Property("searchRevInclude", "string", 4662 "A list of _revinclude (reverse include) values supported by the server.", 0, java.lang.Integer.MAX_VALUE, 4663 searchRevInclude)); 4664 childrenList.add(new Property("searchParam", "", 4665 "Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 4666 0, java.lang.Integer.MAX_VALUE, searchParam)); 4667 } 4668 4669 @Override 4670 public void setProperty(String name, Base value) throws FHIRException { 4671 if (name.equals("type")) 4672 this.type = castToCode(value); // CodeType 4673 else if (name.equals("profile")) 4674 this.profile = castToReference(value); // Reference 4675 else if (name.equals("interaction")) 4676 this.getInteraction().add((ResourceInteractionComponent) value); 4677 else if (name.equals("versioning")) 4678 this.versioning = new ResourceVersionPolicyEnumFactory().fromType(value); // Enumeration<ResourceVersionPolicy> 4679 else if (name.equals("readHistory")) 4680 this.readHistory = castToBoolean(value); // BooleanType 4681 else if (name.equals("updateCreate")) 4682 this.updateCreate = castToBoolean(value); // BooleanType 4683 else if (name.equals("conditionalCreate")) 4684 this.conditionalCreate = castToBoolean(value); // BooleanType 4685 else if (name.equals("conditionalUpdate")) 4686 this.conditionalUpdate = castToBoolean(value); // BooleanType 4687 else if (name.equals("conditionalDelete")) 4688 this.conditionalDelete = new ConditionalDeleteStatusEnumFactory().fromType(value); // Enumeration<ConditionalDeleteStatus> 4689 else if (name.equals("searchInclude")) 4690 this.getSearchInclude().add(castToString(value)); 4691 else if (name.equals("searchRevInclude")) 4692 this.getSearchRevInclude().add(castToString(value)); 4693 else if (name.equals("searchParam")) 4694 this.getSearchParam().add((ConformanceRestResourceSearchParamComponent) value); 4695 else 4696 super.setProperty(name, value); 4697 } 4698 4699 @Override 4700 public Base addChild(String name) throws FHIRException { 4701 if (name.equals("type")) { 4702 throw new FHIRException("Cannot call addChild on a singleton property Conformance.type"); 4703 } else if (name.equals("profile")) { 4704 this.profile = new Reference(); 4705 return this.profile; 4706 } else if (name.equals("interaction")) { 4707 return addInteraction(); 4708 } else if (name.equals("versioning")) { 4709 throw new FHIRException("Cannot call addChild on a singleton property Conformance.versioning"); 4710 } else if (name.equals("readHistory")) { 4711 throw new FHIRException("Cannot call addChild on a singleton property Conformance.readHistory"); 4712 } else if (name.equals("updateCreate")) { 4713 throw new FHIRException("Cannot call addChild on a singleton property Conformance.updateCreate"); 4714 } else if (name.equals("conditionalCreate")) { 4715 throw new FHIRException("Cannot call addChild on a singleton property Conformance.conditionalCreate"); 4716 } else if (name.equals("conditionalUpdate")) { 4717 throw new FHIRException("Cannot call addChild on a singleton property Conformance.conditionalUpdate"); 4718 } else if (name.equals("conditionalDelete")) { 4719 throw new FHIRException("Cannot call addChild on a singleton property Conformance.conditionalDelete"); 4720 } else if (name.equals("searchInclude")) { 4721 throw new FHIRException("Cannot call addChild on a singleton property Conformance.searchInclude"); 4722 } else if (name.equals("searchRevInclude")) { 4723 throw new FHIRException("Cannot call addChild on a singleton property Conformance.searchRevInclude"); 4724 } else if (name.equals("searchParam")) { 4725 return addSearchParam(); 4726 } else 4727 return super.addChild(name); 4728 } 4729 4730 public ConformanceRestResourceComponent copy() { 4731 ConformanceRestResourceComponent dst = new ConformanceRestResourceComponent(); 4732 copyValues(dst); 4733 dst.type = type == null ? null : type.copy(); 4734 dst.profile = profile == null ? null : profile.copy(); 4735 if (interaction != null) { 4736 dst.interaction = new ArrayList<ResourceInteractionComponent>(); 4737 for (ResourceInteractionComponent i : interaction) 4738 dst.interaction.add(i.copy()); 4739 } 4740 ; 4741 dst.versioning = versioning == null ? null : versioning.copy(); 4742 dst.readHistory = readHistory == null ? null : readHistory.copy(); 4743 dst.updateCreate = updateCreate == null ? null : updateCreate.copy(); 4744 dst.conditionalCreate = conditionalCreate == null ? null : conditionalCreate.copy(); 4745 dst.conditionalUpdate = conditionalUpdate == null ? null : conditionalUpdate.copy(); 4746 dst.conditionalDelete = conditionalDelete == null ? null : conditionalDelete.copy(); 4747 if (searchInclude != null) { 4748 dst.searchInclude = new ArrayList<StringType>(); 4749 for (StringType i : searchInclude) 4750 dst.searchInclude.add(i.copy()); 4751 } 4752 ; 4753 if (searchRevInclude != null) { 4754 dst.searchRevInclude = new ArrayList<StringType>(); 4755 for (StringType i : searchRevInclude) 4756 dst.searchRevInclude.add(i.copy()); 4757 } 4758 ; 4759 if (searchParam != null) { 4760 dst.searchParam = new ArrayList<ConformanceRestResourceSearchParamComponent>(); 4761 for (ConformanceRestResourceSearchParamComponent i : searchParam) 4762 dst.searchParam.add(i.copy()); 4763 } 4764 ; 4765 return dst; 4766 } 4767 4768 @Override 4769 public boolean equalsDeep(Base other) { 4770 if (!super.equalsDeep(other)) 4771 return false; 4772 if (!(other instanceof ConformanceRestResourceComponent)) 4773 return false; 4774 ConformanceRestResourceComponent o = (ConformanceRestResourceComponent) other; 4775 return compareDeep(type, o.type, true) && compareDeep(profile, o.profile, true) 4776 && compareDeep(interaction, o.interaction, true) && compareDeep(versioning, o.versioning, true) 4777 && compareDeep(readHistory, o.readHistory, true) && compareDeep(updateCreate, o.updateCreate, true) 4778 && compareDeep(conditionalCreate, o.conditionalCreate, true) 4779 && compareDeep(conditionalUpdate, o.conditionalUpdate, true) 4780 && compareDeep(conditionalDelete, o.conditionalDelete, true) 4781 && compareDeep(searchInclude, o.searchInclude, true) 4782 && compareDeep(searchRevInclude, o.searchRevInclude, true) && compareDeep(searchParam, o.searchParam, true); 4783 } 4784 4785 @Override 4786 public boolean equalsShallow(Base other) { 4787 if (!super.equalsShallow(other)) 4788 return false; 4789 if (!(other instanceof ConformanceRestResourceComponent)) 4790 return false; 4791 ConformanceRestResourceComponent o = (ConformanceRestResourceComponent) other; 4792 return compareValues(type, o.type, true) && compareValues(versioning, o.versioning, true) 4793 && compareValues(readHistory, o.readHistory, true) && compareValues(updateCreate, o.updateCreate, true) 4794 && compareValues(conditionalCreate, o.conditionalCreate, true) 4795 && compareValues(conditionalUpdate, o.conditionalUpdate, true) 4796 && compareValues(conditionalDelete, o.conditionalDelete, true) 4797 && compareValues(searchInclude, o.searchInclude, true) 4798 && compareValues(searchRevInclude, o.searchRevInclude, true); 4799 } 4800 4801 public boolean isEmpty() { 4802 return super.isEmpty() && (type == null || type.isEmpty()) && (profile == null || profile.isEmpty()) 4803 && (interaction == null || interaction.isEmpty()) && (versioning == null || versioning.isEmpty()) 4804 && (readHistory == null || readHistory.isEmpty()) && (updateCreate == null || updateCreate.isEmpty()) 4805 && (conditionalCreate == null || conditionalCreate.isEmpty()) 4806 && (conditionalUpdate == null || conditionalUpdate.isEmpty()) 4807 && (conditionalDelete == null || conditionalDelete.isEmpty()) 4808 && (searchInclude == null || searchInclude.isEmpty()) 4809 && (searchRevInclude == null || searchRevInclude.isEmpty()) && (searchParam == null || searchParam.isEmpty()); 4810 } 4811 4812 public String fhirType() { 4813 return "Conformance.rest.resource"; 4814 4815 } 4816 4817 } 4818 4819 @Block() 4820 public static class ResourceInteractionComponent extends BackboneElement implements IBaseBackboneElement { 4821 /** 4822 * Coded identifier of the operation, supported by the system resource. 4823 */ 4824 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4825 @Description(shortDefinition = "read | vread | update | delete | history-instance | validate | history-type | create | search-type", formalDefinition = "Coded identifier of the operation, supported by the system resource.") 4826 protected Enumeration<TypeRestfulInteraction> code; 4827 4828 /** 4829 * Guidance specific to the implementation of this operation, such as 'delete is 4830 * a logical delete' or 'updates are only allowed with version id' or 'creates 4831 * permitted from pre-authorized certificates only'. 4832 */ 4833 @Child(name = "documentation", type = { 4834 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 4835 @Description(shortDefinition = "Anything special about operation behavior", formalDefinition = "Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.") 4836 protected StringType documentation; 4837 4838 private static final long serialVersionUID = -437507806L; 4839 4840 /* 4841 * Constructor 4842 */ 4843 public ResourceInteractionComponent() { 4844 super(); 4845 } 4846 4847 /* 4848 * Constructor 4849 */ 4850 public ResourceInteractionComponent(Enumeration<TypeRestfulInteraction> code) { 4851 super(); 4852 this.code = code; 4853 } 4854 4855 /** 4856 * @return {@link #code} (Coded identifier of the operation, supported by the 4857 * system resource.). This is the underlying object with id, value and 4858 * extensions. The accessor "getCode" gives direct access to the value 4859 */ 4860 public Enumeration<TypeRestfulInteraction> getCodeElement() { 4861 if (this.code == null) 4862 if (Configuration.errorOnAutoCreate()) 4863 throw new Error("Attempt to auto-create ResourceInteractionComponent.code"); 4864 else if (Configuration.doAutoCreate()) 4865 this.code = new Enumeration<TypeRestfulInteraction>(new TypeRestfulInteractionEnumFactory()); // bb 4866 return this.code; 4867 } 4868 4869 public boolean hasCodeElement() { 4870 return this.code != null && !this.code.isEmpty(); 4871 } 4872 4873 public boolean hasCode() { 4874 return this.code != null && !this.code.isEmpty(); 4875 } 4876 4877 /** 4878 * @param value {@link #code} (Coded identifier of the operation, supported by 4879 * the system resource.). This is the underlying object with id, 4880 * value and extensions. The accessor "getCode" gives direct access 4881 * to the value 4882 */ 4883 public ResourceInteractionComponent setCodeElement(Enumeration<TypeRestfulInteraction> value) { 4884 this.code = value; 4885 return this; 4886 } 4887 4888 /** 4889 * @return Coded identifier of the operation, supported by the system resource. 4890 */ 4891 public TypeRestfulInteraction getCode() { 4892 return this.code == null ? null : this.code.getValue(); 4893 } 4894 4895 /** 4896 * @param value Coded identifier of the operation, supported by the system 4897 * resource. 4898 */ 4899 public ResourceInteractionComponent setCode(TypeRestfulInteraction value) { 4900 if (this.code == null) 4901 this.code = new Enumeration<TypeRestfulInteraction>(new TypeRestfulInteractionEnumFactory()); 4902 this.code.setValue(value); 4903 return this; 4904 } 4905 4906 /** 4907 * @return {@link #documentation} (Guidance specific to the implementation of 4908 * this operation, such as 'delete is a logical delete' or 'updates are 4909 * only allowed with version id' or 'creates permitted from 4910 * pre-authorized certificates only'.). This is the underlying object 4911 * with id, value and extensions. The accessor "getDocumentation" gives 4912 * direct access to the value 4913 */ 4914 public StringType getDocumentationElement() { 4915 if (this.documentation == null) 4916 if (Configuration.errorOnAutoCreate()) 4917 throw new Error("Attempt to auto-create ResourceInteractionComponent.documentation"); 4918 else if (Configuration.doAutoCreate()) 4919 this.documentation = new StringType(); // bb 4920 return this.documentation; 4921 } 4922 4923 public boolean hasDocumentationElement() { 4924 return this.documentation != null && !this.documentation.isEmpty(); 4925 } 4926 4927 public boolean hasDocumentation() { 4928 return this.documentation != null && !this.documentation.isEmpty(); 4929 } 4930 4931 /** 4932 * @param value {@link #documentation} (Guidance specific to the implementation 4933 * of this operation, such as 'delete is a logical delete' or 4934 * 'updates are only allowed with version id' or 'creates permitted 4935 * from pre-authorized certificates only'.). This is the underlying 4936 * object with id, value and extensions. The accessor 4937 * "getDocumentation" gives direct access to the value 4938 */ 4939 public ResourceInteractionComponent setDocumentationElement(StringType value) { 4940 this.documentation = value; 4941 return this; 4942 } 4943 4944 /** 4945 * @return Guidance specific to the implementation of this operation, such as 4946 * 'delete is a logical delete' or 'updates are only allowed with 4947 * version id' or 'creates permitted from pre-authorized certificates 4948 * only'. 4949 */ 4950 public String getDocumentation() { 4951 return this.documentation == null ? null : this.documentation.getValue(); 4952 } 4953 4954 /** 4955 * @param value Guidance specific to the implementation of this operation, such 4956 * as 'delete is a logical delete' or 'updates are only allowed 4957 * with version id' or 'creates permitted from pre-authorized 4958 * certificates only'. 4959 */ 4960 public ResourceInteractionComponent setDocumentation(String value) { 4961 if (Utilities.noString(value)) 4962 this.documentation = null; 4963 else { 4964 if (this.documentation == null) 4965 this.documentation = new StringType(); 4966 this.documentation.setValue(value); 4967 } 4968 return this; 4969 } 4970 4971 protected void listChildren(List<Property> childrenList) { 4972 super.listChildren(childrenList); 4973 childrenList 4974 .add(new Property("code", "code", "Coded identifier of the operation, supported by the system resource.", 0, 4975 java.lang.Integer.MAX_VALUE, code)); 4976 childrenList.add(new Property("documentation", "string", 4977 "Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.", 4978 0, java.lang.Integer.MAX_VALUE, documentation)); 4979 } 4980 4981 @Override 4982 public void setProperty(String name, Base value) throws FHIRException { 4983 if (name.equals("code")) 4984 this.code = new TypeRestfulInteractionEnumFactory().fromType(value); // Enumeration<TypeRestfulInteraction> 4985 else if (name.equals("documentation")) 4986 this.documentation = castToString(value); // StringType 4987 else 4988 super.setProperty(name, value); 4989 } 4990 4991 @Override 4992 public Base addChild(String name) throws FHIRException { 4993 if (name.equals("code")) { 4994 throw new FHIRException("Cannot call addChild on a singleton property Conformance.code"); 4995 } else if (name.equals("documentation")) { 4996 throw new FHIRException("Cannot call addChild on a singleton property Conformance.documentation"); 4997 } else 4998 return super.addChild(name); 4999 } 5000 5001 public ResourceInteractionComponent copy() { 5002 ResourceInteractionComponent dst = new ResourceInteractionComponent(); 5003 copyValues(dst); 5004 dst.code = code == null ? null : code.copy(); 5005 dst.documentation = documentation == null ? null : documentation.copy(); 5006 return dst; 5007 } 5008 5009 @Override 5010 public boolean equalsDeep(Base other) { 5011 if (!super.equalsDeep(other)) 5012 return false; 5013 if (!(other instanceof ResourceInteractionComponent)) 5014 return false; 5015 ResourceInteractionComponent o = (ResourceInteractionComponent) other; 5016 return compareDeep(code, o.code, true) && compareDeep(documentation, o.documentation, true); 5017 } 5018 5019 @Override 5020 public boolean equalsShallow(Base other) { 5021 if (!super.equalsShallow(other)) 5022 return false; 5023 if (!(other instanceof ResourceInteractionComponent)) 5024 return false; 5025 ResourceInteractionComponent o = (ResourceInteractionComponent) other; 5026 return compareValues(code, o.code, true) && compareValues(documentation, o.documentation, true); 5027 } 5028 5029 public boolean isEmpty() { 5030 return super.isEmpty() && (code == null || code.isEmpty()) && (documentation == null || documentation.isEmpty()); 5031 } 5032 5033 public String fhirType() { 5034 return "Conformance.rest.resource.interaction"; 5035 5036 } 5037 5038 } 5039 5040 @Block() 5041 public static class ConformanceRestResourceSearchParamComponent extends BackboneElement 5042 implements IBaseBackboneElement { 5043 /** 5044 * The name of the search parameter used in the interface. 5045 */ 5046 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 5047 @Description(shortDefinition = "Name of search parameter", formalDefinition = "The name of the search parameter used in the interface.") 5048 protected StringType name; 5049 5050 /** 5051 * An absolute URI that is a formal reference to where this parameter was first 5052 * defined, so that a client can be confident of the meaning of the search 5053 * parameter (a reference to [[[SearchParameter.url]]]). 5054 */ 5055 @Child(name = "definition", type = { 5056 UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5057 @Description(shortDefinition = "Source of definition for parameter", formalDefinition = "An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]).") 5058 protected UriType definition; 5059 5060 /** 5061 * The type of value a search parameter refers to, and how the content is 5062 * interpreted. 5063 */ 5064 @Child(name = "type", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 5065 @Description(shortDefinition = "number | date | string | token | reference | composite | quantity | uri", formalDefinition = "The type of value a search parameter refers to, and how the content is interpreted.") 5066 protected Enumeration<SearchParamType> type; 5067 5068 /** 5069 * This allows documentation of any distinct behaviors about how the search 5070 * parameter is used. For example, text matching algorithms. 5071 */ 5072 @Child(name = "documentation", type = { 5073 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 5074 @Description(shortDefinition = "Server-specific usage", formalDefinition = "This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.") 5075 protected StringType documentation; 5076 5077 /** 5078 * Types of resource (if a resource is referenced). 5079 */ 5080 @Child(name = "target", type = { 5081 CodeType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5082 @Description(shortDefinition = "Types of resource (if a resource reference)", formalDefinition = "Types of resource (if a resource is referenced).") 5083 protected List<CodeType> target; 5084 5085 /** 5086 * A modifier supported for the search parameter. 5087 */ 5088 @Child(name = "modifier", type = { 5089 CodeType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5090 @Description(shortDefinition = "missing | exact | contains | not | text | in | not-in | below | above | type", formalDefinition = "A modifier supported for the search parameter.") 5091 protected List<Enumeration<SearchModifierCode>> modifier; 5092 5093 /** 5094 * Contains the names of any search parameters which may be chained to the 5095 * containing search parameter. Chained parameters may be added to search 5096 * parameters of type reference, and specify that resources will only be 5097 * returned if they contain a reference to a resource which matches the chained 5098 * parameter value. Values for this field should be drawn from 5099 * Conformance.rest.resource.searchParam.name on the target resource type. 5100 */ 5101 @Child(name = "chain", type = { 5102 StringType.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5103 @Description(shortDefinition = "Chained names supported", formalDefinition = "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from Conformance.rest.resource.searchParam.name on the target resource type.") 5104 protected List<StringType> chain; 5105 5106 private static final long serialVersionUID = -1020405086L; 5107 5108 /* 5109 * Constructor 5110 */ 5111 public ConformanceRestResourceSearchParamComponent() { 5112 super(); 5113 } 5114 5115 /* 5116 * Constructor 5117 */ 5118 public ConformanceRestResourceSearchParamComponent(StringType name, Enumeration<SearchParamType> type) { 5119 super(); 5120 this.name = name; 5121 this.type = type; 5122 } 5123 5124 /** 5125 * @return {@link #name} (The name of the search parameter used in the 5126 * interface.). This is the underlying object with id, value and 5127 * extensions. The accessor "getName" gives direct access to the value 5128 */ 5129 public StringType getNameElement() { 5130 if (this.name == null) 5131 if (Configuration.errorOnAutoCreate()) 5132 throw new Error("Attempt to auto-create ConformanceRestResourceSearchParamComponent.name"); 5133 else if (Configuration.doAutoCreate()) 5134 this.name = new StringType(); // bb 5135 return this.name; 5136 } 5137 5138 public boolean hasNameElement() { 5139 return this.name != null && !this.name.isEmpty(); 5140 } 5141 5142 public boolean hasName() { 5143 return this.name != null && !this.name.isEmpty(); 5144 } 5145 5146 /** 5147 * @param value {@link #name} (The name of the search parameter used in the 5148 * interface.). This is the underlying object with id, value and 5149 * extensions. The accessor "getName" gives direct access to the 5150 * value 5151 */ 5152 public ConformanceRestResourceSearchParamComponent setNameElement(StringType value) { 5153 this.name = value; 5154 return this; 5155 } 5156 5157 /** 5158 * @return The name of the search parameter used in the interface. 5159 */ 5160 public String getName() { 5161 return this.name == null ? null : this.name.getValue(); 5162 } 5163 5164 /** 5165 * @param value The name of the search parameter used in the interface. 5166 */ 5167 public ConformanceRestResourceSearchParamComponent setName(String value) { 5168 if (this.name == null) 5169 this.name = new StringType(); 5170 this.name.setValue(value); 5171 return this; 5172 } 5173 5174 /** 5175 * @return {@link #definition} (An absolute URI that is a formal reference to 5176 * where this parameter was first defined, so that a client can be 5177 * confident of the meaning of the search parameter (a reference to 5178 * [[[SearchParameter.url]]]).). This is the underlying object with id, 5179 * value and extensions. The accessor "getDefinition" gives direct 5180 * access to the value 5181 */ 5182 public UriType getDefinitionElement() { 5183 if (this.definition == null) 5184 if (Configuration.errorOnAutoCreate()) 5185 throw new Error("Attempt to auto-create ConformanceRestResourceSearchParamComponent.definition"); 5186 else if (Configuration.doAutoCreate()) 5187 this.definition = new UriType(); // bb 5188 return this.definition; 5189 } 5190 5191 public boolean hasDefinitionElement() { 5192 return this.definition != null && !this.definition.isEmpty(); 5193 } 5194 5195 public boolean hasDefinition() { 5196 return this.definition != null && !this.definition.isEmpty(); 5197 } 5198 5199 /** 5200 * @param value {@link #definition} (An absolute URI that is a formal reference 5201 * to where this parameter was first defined, so that a client can 5202 * be confident of the meaning of the search parameter (a reference 5203 * to [[[SearchParameter.url]]]).). This is the underlying object 5204 * with id, value and extensions. The accessor "getDefinition" 5205 * gives direct access to the value 5206 */ 5207 public ConformanceRestResourceSearchParamComponent setDefinitionElement(UriType value) { 5208 this.definition = value; 5209 return this; 5210 } 5211 5212 /** 5213 * @return An absolute URI that is a formal reference to where this parameter 5214 * was first defined, so that a client can be confident of the meaning 5215 * of the search parameter (a reference to [[[SearchParameter.url]]]). 5216 */ 5217 public String getDefinition() { 5218 return this.definition == null ? null : this.definition.getValue(); 5219 } 5220 5221 /** 5222 * @param value An absolute URI that is a formal reference to where this 5223 * parameter was first defined, so that a client can be confident 5224 * of the meaning of the search parameter (a reference to 5225 * [[[SearchParameter.url]]]). 5226 */ 5227 public ConformanceRestResourceSearchParamComponent setDefinition(String value) { 5228 if (Utilities.noString(value)) 5229 this.definition = null; 5230 else { 5231 if (this.definition == null) 5232 this.definition = new UriType(); 5233 this.definition.setValue(value); 5234 } 5235 return this; 5236 } 5237 5238 /** 5239 * @return {@link #type} (The type of value a search parameter refers to, and 5240 * how the content is interpreted.). This is the underlying object with 5241 * id, value and extensions. The accessor "getType" gives direct access 5242 * to the value 5243 */ 5244 public Enumeration<SearchParamType> getTypeElement() { 5245 if (this.type == null) 5246 if (Configuration.errorOnAutoCreate()) 5247 throw new Error("Attempt to auto-create ConformanceRestResourceSearchParamComponent.type"); 5248 else if (Configuration.doAutoCreate()) 5249 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 5250 return this.type; 5251 } 5252 5253 public boolean hasTypeElement() { 5254 return this.type != null && !this.type.isEmpty(); 5255 } 5256 5257 public boolean hasType() { 5258 return this.type != null && !this.type.isEmpty(); 5259 } 5260 5261 /** 5262 * @param value {@link #type} (The type of value a search parameter refers to, 5263 * and how the content is interpreted.). This is the underlying 5264 * object with id, value and extensions. The accessor "getType" 5265 * gives direct access to the value 5266 */ 5267 public ConformanceRestResourceSearchParamComponent setTypeElement(Enumeration<SearchParamType> value) { 5268 this.type = value; 5269 return this; 5270 } 5271 5272 /** 5273 * @return The type of value a search parameter refers to, and how the content 5274 * is interpreted. 5275 */ 5276 public SearchParamType getType() { 5277 return this.type == null ? null : this.type.getValue(); 5278 } 5279 5280 /** 5281 * @param value The type of value a search parameter refers to, and how the 5282 * content is interpreted. 5283 */ 5284 public ConformanceRestResourceSearchParamComponent setType(SearchParamType value) { 5285 if (this.type == null) 5286 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 5287 this.type.setValue(value); 5288 return this; 5289 } 5290 5291 /** 5292 * @return {@link #documentation} (This allows documentation of any distinct 5293 * behaviors about how the search parameter is used. For example, text 5294 * matching algorithms.). This is the underlying object with id, value 5295 * and extensions. The accessor "getDocumentation" gives direct access 5296 * to the value 5297 */ 5298 public StringType getDocumentationElement() { 5299 if (this.documentation == null) 5300 if (Configuration.errorOnAutoCreate()) 5301 throw new Error("Attempt to auto-create ConformanceRestResourceSearchParamComponent.documentation"); 5302 else if (Configuration.doAutoCreate()) 5303 this.documentation = new StringType(); // bb 5304 return this.documentation; 5305 } 5306 5307 public boolean hasDocumentationElement() { 5308 return this.documentation != null && !this.documentation.isEmpty(); 5309 } 5310 5311 public boolean hasDocumentation() { 5312 return this.documentation != null && !this.documentation.isEmpty(); 5313 } 5314 5315 /** 5316 * @param value {@link #documentation} (This allows documentation of any 5317 * distinct behaviors about how the search parameter is used. For 5318 * example, text matching algorithms.). This is the underlying 5319 * object with id, value and extensions. The accessor 5320 * "getDocumentation" gives direct access to the value 5321 */ 5322 public ConformanceRestResourceSearchParamComponent setDocumentationElement(StringType value) { 5323 this.documentation = value; 5324 return this; 5325 } 5326 5327 /** 5328 * @return This allows documentation of any distinct behaviors about how the 5329 * search parameter is used. For example, text matching algorithms. 5330 */ 5331 public String getDocumentation() { 5332 return this.documentation == null ? null : this.documentation.getValue(); 5333 } 5334 5335 /** 5336 * @param value This allows documentation of any distinct behaviors about how 5337 * the search parameter is used. For example, text matching 5338 * algorithms. 5339 */ 5340 public ConformanceRestResourceSearchParamComponent setDocumentation(String value) { 5341 if (Utilities.noString(value)) 5342 this.documentation = null; 5343 else { 5344 if (this.documentation == null) 5345 this.documentation = new StringType(); 5346 this.documentation.setValue(value); 5347 } 5348 return this; 5349 } 5350 5351 /** 5352 * @return {@link #target} (Types of resource (if a resource is referenced).) 5353 */ 5354 public List<CodeType> getTarget() { 5355 if (this.target == null) 5356 this.target = new ArrayList<CodeType>(); 5357 return this.target; 5358 } 5359 5360 public boolean hasTarget() { 5361 if (this.target == null) 5362 return false; 5363 for (CodeType item : this.target) 5364 if (!item.isEmpty()) 5365 return true; 5366 return false; 5367 } 5368 5369 /** 5370 * @return {@link #target} (Types of resource (if a resource is referenced).) 5371 */ 5372 // syntactic sugar 5373 public CodeType addTargetElement() {// 2 5374 CodeType t = new CodeType(); 5375 if (this.target == null) 5376 this.target = new ArrayList<CodeType>(); 5377 this.target.add(t); 5378 return t; 5379 } 5380 5381 /** 5382 * @param value {@link #target} (Types of resource (if a resource is 5383 * referenced).) 5384 */ 5385 public ConformanceRestResourceSearchParamComponent addTarget(String value) { // 1 5386 CodeType t = new CodeType(); 5387 t.setValue(value); 5388 if (this.target == null) 5389 this.target = new ArrayList<CodeType>(); 5390 this.target.add(t); 5391 return this; 5392 } 5393 5394 /** 5395 * @param value {@link #target} (Types of resource (if a resource is 5396 * referenced).) 5397 */ 5398 public boolean hasTarget(String value) { 5399 if (this.target == null) 5400 return false; 5401 for (CodeType v : this.target) 5402 if (v.equals(value)) // code 5403 return true; 5404 return false; 5405 } 5406 5407 /** 5408 * @return {@link #modifier} (A modifier supported for the search parameter.) 5409 */ 5410 public List<Enumeration<SearchModifierCode>> getModifier() { 5411 if (this.modifier == null) 5412 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 5413 return this.modifier; 5414 } 5415 5416 public boolean hasModifier() { 5417 if (this.modifier == null) 5418 return false; 5419 for (Enumeration<SearchModifierCode> item : this.modifier) 5420 if (!item.isEmpty()) 5421 return true; 5422 return false; 5423 } 5424 5425 /** 5426 * @return {@link #modifier} (A modifier supported for the search parameter.) 5427 */ 5428 // syntactic sugar 5429 public Enumeration<SearchModifierCode> addModifierElement() {// 2 5430 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 5431 if (this.modifier == null) 5432 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 5433 this.modifier.add(t); 5434 return t; 5435 } 5436 5437 /** 5438 * @param value {@link #modifier} (A modifier supported for the search 5439 * parameter.) 5440 */ 5441 public ConformanceRestResourceSearchParamComponent addModifier(SearchModifierCode value) { // 1 5442 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 5443 t.setValue(value); 5444 if (this.modifier == null) 5445 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 5446 this.modifier.add(t); 5447 return this; 5448 } 5449 5450 /** 5451 * @param value {@link #modifier} (A modifier supported for the search 5452 * parameter.) 5453 */ 5454 public boolean hasModifier(SearchModifierCode value) { 5455 if (this.modifier == null) 5456 return false; 5457 for (Enumeration<SearchModifierCode> v : this.modifier) 5458 if (v.equals(value)) // code 5459 return true; 5460 return false; 5461 } 5462 5463 /** 5464 * @return {@link #chain} (Contains the names of any search parameters which may 5465 * be chained to the containing search parameter. Chained parameters may 5466 * be added to search parameters of type reference, and specify that 5467 * resources will only be returned if they contain a reference to a 5468 * resource which matches the chained parameter value. Values for this 5469 * field should be drawn from Conformance.rest.resource.searchParam.name 5470 * on the target resource type.) 5471 */ 5472 public List<StringType> getChain() { 5473 if (this.chain == null) 5474 this.chain = new ArrayList<StringType>(); 5475 return this.chain; 5476 } 5477 5478 public boolean hasChain() { 5479 if (this.chain == null) 5480 return false; 5481 for (StringType item : this.chain) 5482 if (!item.isEmpty()) 5483 return true; 5484 return false; 5485 } 5486 5487 /** 5488 * @return {@link #chain} (Contains the names of any search parameters which may 5489 * be chained to the containing search parameter. Chained parameters may 5490 * be added to search parameters of type reference, and specify that 5491 * resources will only be returned if they contain a reference to a 5492 * resource which matches the chained parameter value. Values for this 5493 * field should be drawn from Conformance.rest.resource.searchParam.name 5494 * on the target resource type.) 5495 */ 5496 // syntactic sugar 5497 public StringType addChainElement() {// 2 5498 StringType t = new StringType(); 5499 if (this.chain == null) 5500 this.chain = new ArrayList<StringType>(); 5501 this.chain.add(t); 5502 return t; 5503 } 5504 5505 /** 5506 * @param value {@link #chain} (Contains the names of any search parameters 5507 * which may be chained to the containing search parameter. Chained 5508 * parameters may be added to search parameters of type reference, 5509 * and specify that resources will only be returned if they contain 5510 * a reference to a resource which matches the chained parameter 5511 * value. Values for this field should be drawn from 5512 * Conformance.rest.resource.searchParam.name on the target 5513 * resource type.) 5514 */ 5515 public ConformanceRestResourceSearchParamComponent addChain(String value) { // 1 5516 StringType t = new StringType(); 5517 t.setValue(value); 5518 if (this.chain == null) 5519 this.chain = new ArrayList<StringType>(); 5520 this.chain.add(t); 5521 return this; 5522 } 5523 5524 /** 5525 * @param value {@link #chain} (Contains the names of any search parameters 5526 * which may be chained to the containing search parameter. Chained 5527 * parameters may be added to search parameters of type reference, 5528 * and specify that resources will only be returned if they contain 5529 * a reference to a resource which matches the chained parameter 5530 * value. Values for this field should be drawn from 5531 * Conformance.rest.resource.searchParam.name on the target 5532 * resource type.) 5533 */ 5534 public boolean hasChain(String value) { 5535 if (this.chain == null) 5536 return false; 5537 for (StringType v : this.chain) 5538 if (v.equals(value)) // string 5539 return true; 5540 return false; 5541 } 5542 5543 protected void listChildren(List<Property> childrenList) { 5544 super.listChildren(childrenList); 5545 childrenList.add(new Property("name", "string", "The name of the search parameter used in the interface.", 0, 5546 java.lang.Integer.MAX_VALUE, name)); 5547 childrenList.add(new Property("definition", "uri", 5548 "An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]).", 5549 0, java.lang.Integer.MAX_VALUE, definition)); 5550 childrenList.add(new Property("type", "code", 5551 "The type of value a search parameter refers to, and how the content is interpreted.", 0, 5552 java.lang.Integer.MAX_VALUE, type)); 5553 childrenList.add(new Property("documentation", "string", 5554 "This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.", 5555 0, java.lang.Integer.MAX_VALUE, documentation)); 5556 childrenList.add(new Property("target", "code", "Types of resource (if a resource is referenced).", 0, 5557 java.lang.Integer.MAX_VALUE, target)); 5558 childrenList.add(new Property("modifier", "code", "A modifier supported for the search parameter.", 0, 5559 java.lang.Integer.MAX_VALUE, modifier)); 5560 childrenList.add(new Property("chain", "string", 5561 "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from Conformance.rest.resource.searchParam.name on the target resource type.", 5562 0, java.lang.Integer.MAX_VALUE, chain)); 5563 } 5564 5565 @Override 5566 public void setProperty(String name, Base value) throws FHIRException { 5567 if (name.equals("name")) 5568 this.name = castToString(value); // StringType 5569 else if (name.equals("definition")) 5570 this.definition = castToUri(value); // UriType 5571 else if (name.equals("type")) 5572 this.type = new SearchParamTypeEnumFactory().fromType(value); // Enumeration<SearchParamType> 5573 else if (name.equals("documentation")) 5574 this.documentation = castToString(value); // StringType 5575 else if (name.equals("target")) 5576 this.getTarget().add(castToCode(value)); 5577 else if (name.equals("modifier")) 5578 this.getModifier().add(new SearchModifierCodeEnumFactory().fromType(value)); 5579 else if (name.equals("chain")) 5580 this.getChain().add(castToString(value)); 5581 else 5582 super.setProperty(name, value); 5583 } 5584 5585 @Override 5586 public Base addChild(String name) throws FHIRException { 5587 if (name.equals("name")) { 5588 throw new FHIRException("Cannot call addChild on a singleton property Conformance.name"); 5589 } else if (name.equals("definition")) { 5590 throw new FHIRException("Cannot call addChild on a singleton property Conformance.definition"); 5591 } else if (name.equals("type")) { 5592 throw new FHIRException("Cannot call addChild on a singleton property Conformance.type"); 5593 } else if (name.equals("documentation")) { 5594 throw new FHIRException("Cannot call addChild on a singleton property Conformance.documentation"); 5595 } else if (name.equals("target")) { 5596 throw new FHIRException("Cannot call addChild on a singleton property Conformance.target"); 5597 } else if (name.equals("modifier")) { 5598 throw new FHIRException("Cannot call addChild on a singleton property Conformance.modifier"); 5599 } else if (name.equals("chain")) { 5600 throw new FHIRException("Cannot call addChild on a singleton property Conformance.chain"); 5601 } else 5602 return super.addChild(name); 5603 } 5604 5605 public ConformanceRestResourceSearchParamComponent copy() { 5606 ConformanceRestResourceSearchParamComponent dst = new ConformanceRestResourceSearchParamComponent(); 5607 copyValues(dst); 5608 dst.name = name == null ? null : name.copy(); 5609 dst.definition = definition == null ? null : definition.copy(); 5610 dst.type = type == null ? null : type.copy(); 5611 dst.documentation = documentation == null ? null : documentation.copy(); 5612 if (target != null) { 5613 dst.target = new ArrayList<CodeType>(); 5614 for (CodeType i : target) 5615 dst.target.add(i.copy()); 5616 } 5617 ; 5618 if (modifier != null) { 5619 dst.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 5620 for (Enumeration<SearchModifierCode> i : modifier) 5621 dst.modifier.add(i.copy()); 5622 } 5623 ; 5624 if (chain != null) { 5625 dst.chain = new ArrayList<StringType>(); 5626 for (StringType i : chain) 5627 dst.chain.add(i.copy()); 5628 } 5629 ; 5630 return dst; 5631 } 5632 5633 @Override 5634 public boolean equalsDeep(Base other) { 5635 if (!super.equalsDeep(other)) 5636 return false; 5637 if (!(other instanceof ConformanceRestResourceSearchParamComponent)) 5638 return false; 5639 ConformanceRestResourceSearchParamComponent o = (ConformanceRestResourceSearchParamComponent) other; 5640 return compareDeep(name, o.name, true) && compareDeep(definition, o.definition, true) 5641 && compareDeep(type, o.type, true) && compareDeep(documentation, o.documentation, true) 5642 && compareDeep(target, o.target, true) && compareDeep(modifier, o.modifier, true) 5643 && compareDeep(chain, o.chain, true); 5644 } 5645 5646 @Override 5647 public boolean equalsShallow(Base other) { 5648 if (!super.equalsShallow(other)) 5649 return false; 5650 if (!(other instanceof ConformanceRestResourceSearchParamComponent)) 5651 return false; 5652 ConformanceRestResourceSearchParamComponent o = (ConformanceRestResourceSearchParamComponent) other; 5653 return compareValues(name, o.name, true) && compareValues(definition, o.definition, true) 5654 && compareValues(type, o.type, true) && compareValues(documentation, o.documentation, true) 5655 && compareValues(target, o.target, true) && compareValues(modifier, o.modifier, true) 5656 && compareValues(chain, o.chain, true); 5657 } 5658 5659 public boolean isEmpty() { 5660 return super.isEmpty() && (name == null || name.isEmpty()) && (definition == null || definition.isEmpty()) 5661 && (type == null || type.isEmpty()) && (documentation == null || documentation.isEmpty()) 5662 && (target == null || target.isEmpty()) && (modifier == null || modifier.isEmpty()) 5663 && (chain == null || chain.isEmpty()); 5664 } 5665 5666 public String fhirType() { 5667 return "Conformance.rest.resource.searchParam"; 5668 5669 } 5670 5671 } 5672 5673 @Block() 5674 public static class SystemInteractionComponent extends BackboneElement implements IBaseBackboneElement { 5675 /** 5676 * A coded identifier of the operation, supported by the system. 5677 */ 5678 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 5679 @Description(shortDefinition = "transaction | search-system | history-system", formalDefinition = "A coded identifier of the operation, supported by the system.") 5680 protected Enumeration<SystemRestfulInteraction> code; 5681 5682 /** 5683 * Guidance specific to the implementation of this operation, such as 5684 * limitations on the kind of transactions allowed, or information about system 5685 * wide search is implemented. 5686 */ 5687 @Child(name = "documentation", type = { 5688 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5689 @Description(shortDefinition = "Anything special about operation behavior", formalDefinition = "Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.") 5690 protected StringType documentation; 5691 5692 private static final long serialVersionUID = 510675287L; 5693 5694 /* 5695 * Constructor 5696 */ 5697 public SystemInteractionComponent() { 5698 super(); 5699 } 5700 5701 /* 5702 * Constructor 5703 */ 5704 public SystemInteractionComponent(Enumeration<SystemRestfulInteraction> code) { 5705 super(); 5706 this.code = code; 5707 } 5708 5709 /** 5710 * @return {@link #code} (A coded identifier of the operation, supported by the 5711 * system.). This is the underlying object with id, value and 5712 * extensions. The accessor "getCode" gives direct access to the value 5713 */ 5714 public Enumeration<SystemRestfulInteraction> getCodeElement() { 5715 if (this.code == null) 5716 if (Configuration.errorOnAutoCreate()) 5717 throw new Error("Attempt to auto-create SystemInteractionComponent.code"); 5718 else if (Configuration.doAutoCreate()) 5719 this.code = new Enumeration<SystemRestfulInteraction>(new SystemRestfulInteractionEnumFactory()); // bb 5720 return this.code; 5721 } 5722 5723 public boolean hasCodeElement() { 5724 return this.code != null && !this.code.isEmpty(); 5725 } 5726 5727 public boolean hasCode() { 5728 return this.code != null && !this.code.isEmpty(); 5729 } 5730 5731 /** 5732 * @param value {@link #code} (A coded identifier of the operation, supported by 5733 * the system.). This is the underlying object with id, value and 5734 * extensions. The accessor "getCode" gives direct access to the 5735 * value 5736 */ 5737 public SystemInteractionComponent setCodeElement(Enumeration<SystemRestfulInteraction> value) { 5738 this.code = value; 5739 return this; 5740 } 5741 5742 /** 5743 * @return A coded identifier of the operation, supported by the system. 5744 */ 5745 public SystemRestfulInteraction getCode() { 5746 return this.code == null ? null : this.code.getValue(); 5747 } 5748 5749 /** 5750 * @param value A coded identifier of the operation, supported by the system. 5751 */ 5752 public SystemInteractionComponent setCode(SystemRestfulInteraction value) { 5753 if (this.code == null) 5754 this.code = new Enumeration<SystemRestfulInteraction>(new SystemRestfulInteractionEnumFactory()); 5755 this.code.setValue(value); 5756 return this; 5757 } 5758 5759 /** 5760 * @return {@link #documentation} (Guidance specific to the implementation of 5761 * this operation, such as limitations on the kind of transactions 5762 * allowed, or information about system wide search is implemented.). 5763 * This is the underlying object with id, value and extensions. The 5764 * accessor "getDocumentation" gives direct access to the value 5765 */ 5766 public StringType getDocumentationElement() { 5767 if (this.documentation == null) 5768 if (Configuration.errorOnAutoCreate()) 5769 throw new Error("Attempt to auto-create SystemInteractionComponent.documentation"); 5770 else if (Configuration.doAutoCreate()) 5771 this.documentation = new StringType(); // bb 5772 return this.documentation; 5773 } 5774 5775 public boolean hasDocumentationElement() { 5776 return this.documentation != null && !this.documentation.isEmpty(); 5777 } 5778 5779 public boolean hasDocumentation() { 5780 return this.documentation != null && !this.documentation.isEmpty(); 5781 } 5782 5783 /** 5784 * @param value {@link #documentation} (Guidance specific to the implementation 5785 * of this operation, such as limitations on the kind of 5786 * transactions allowed, or information about system wide search is 5787 * implemented.). This is the underlying object with id, value and 5788 * extensions. The accessor "getDocumentation" gives direct access 5789 * to the value 5790 */ 5791 public SystemInteractionComponent setDocumentationElement(StringType value) { 5792 this.documentation = value; 5793 return this; 5794 } 5795 5796 /** 5797 * @return Guidance specific to the implementation of this operation, such as 5798 * limitations on the kind of transactions allowed, or information about 5799 * system wide search is implemented. 5800 */ 5801 public String getDocumentation() { 5802 return this.documentation == null ? null : this.documentation.getValue(); 5803 } 5804 5805 /** 5806 * @param value Guidance specific to the implementation of this operation, such 5807 * as limitations on the kind of transactions allowed, or 5808 * information about system wide search is implemented. 5809 */ 5810 public SystemInteractionComponent setDocumentation(String value) { 5811 if (Utilities.noString(value)) 5812 this.documentation = null; 5813 else { 5814 if (this.documentation == null) 5815 this.documentation = new StringType(); 5816 this.documentation.setValue(value); 5817 } 5818 return this; 5819 } 5820 5821 protected void listChildren(List<Property> childrenList) { 5822 super.listChildren(childrenList); 5823 childrenList.add(new Property("code", "code", "A coded identifier of the operation, supported by the system.", 0, 5824 java.lang.Integer.MAX_VALUE, code)); 5825 childrenList.add(new Property("documentation", "string", 5826 "Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.", 5827 0, java.lang.Integer.MAX_VALUE, documentation)); 5828 } 5829 5830 @Override 5831 public void setProperty(String name, Base value) throws FHIRException { 5832 if (name.equals("code")) 5833 this.code = new SystemRestfulInteractionEnumFactory().fromType(value); // Enumeration<SystemRestfulInteraction> 5834 else if (name.equals("documentation")) 5835 this.documentation = castToString(value); // StringType 5836 else 5837 super.setProperty(name, value); 5838 } 5839 5840 @Override 5841 public Base addChild(String name) throws FHIRException { 5842 if (name.equals("code")) { 5843 throw new FHIRException("Cannot call addChild on a singleton property Conformance.code"); 5844 } else if (name.equals("documentation")) { 5845 throw new FHIRException("Cannot call addChild on a singleton property Conformance.documentation"); 5846 } else 5847 return super.addChild(name); 5848 } 5849 5850 public SystemInteractionComponent copy() { 5851 SystemInteractionComponent dst = new SystemInteractionComponent(); 5852 copyValues(dst); 5853 dst.code = code == null ? null : code.copy(); 5854 dst.documentation = documentation == null ? null : documentation.copy(); 5855 return dst; 5856 } 5857 5858 @Override 5859 public boolean equalsDeep(Base other) { 5860 if (!super.equalsDeep(other)) 5861 return false; 5862 if (!(other instanceof SystemInteractionComponent)) 5863 return false; 5864 SystemInteractionComponent o = (SystemInteractionComponent) other; 5865 return compareDeep(code, o.code, true) && compareDeep(documentation, o.documentation, true); 5866 } 5867 5868 @Override 5869 public boolean equalsShallow(Base other) { 5870 if (!super.equalsShallow(other)) 5871 return false; 5872 if (!(other instanceof SystemInteractionComponent)) 5873 return false; 5874 SystemInteractionComponent o = (SystemInteractionComponent) other; 5875 return compareValues(code, o.code, true) && compareValues(documentation, o.documentation, true); 5876 } 5877 5878 public boolean isEmpty() { 5879 return super.isEmpty() && (code == null || code.isEmpty()) && (documentation == null || documentation.isEmpty()); 5880 } 5881 5882 public String fhirType() { 5883 return "Conformance.rest.interaction"; 5884 5885 } 5886 5887 } 5888 5889 @Block() 5890 public static class ConformanceRestOperationComponent extends BackboneElement implements IBaseBackboneElement { 5891 /** 5892 * The name of a query, which is used in the _query parameter when the query is 5893 * called. 5894 */ 5895 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 5896 @Description(shortDefinition = "Name by which the operation/query is invoked", formalDefinition = "The name of a query, which is used in the _query parameter when the query is called.") 5897 protected StringType name; 5898 5899 /** 5900 * Where the formal definition can be found. 5901 */ 5902 @Child(name = "definition", type = { 5903 OperationDefinition.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 5904 @Description(shortDefinition = "The defined operation/query", formalDefinition = "Where the formal definition can be found.") 5905 protected Reference definition; 5906 5907 /** 5908 * The actual object that is the target of the reference (Where the formal 5909 * definition can be found.) 5910 */ 5911 protected OperationDefinition definitionTarget; 5912 5913 private static final long serialVersionUID = 122107272L; 5914 5915 /* 5916 * Constructor 5917 */ 5918 public ConformanceRestOperationComponent() { 5919 super(); 5920 } 5921 5922 /* 5923 * Constructor 5924 */ 5925 public ConformanceRestOperationComponent(StringType name, Reference definition) { 5926 super(); 5927 this.name = name; 5928 this.definition = definition; 5929 } 5930 5931 /** 5932 * @return {@link #name} (The name of a query, which is used in the _query 5933 * parameter when the query is called.). This is the underlying object 5934 * with id, value and extensions. The accessor "getName" gives direct 5935 * access to the value 5936 */ 5937 public StringType getNameElement() { 5938 if (this.name == null) 5939 if (Configuration.errorOnAutoCreate()) 5940 throw new Error("Attempt to auto-create ConformanceRestOperationComponent.name"); 5941 else if (Configuration.doAutoCreate()) 5942 this.name = new StringType(); // bb 5943 return this.name; 5944 } 5945 5946 public boolean hasNameElement() { 5947 return this.name != null && !this.name.isEmpty(); 5948 } 5949 5950 public boolean hasName() { 5951 return this.name != null && !this.name.isEmpty(); 5952 } 5953 5954 /** 5955 * @param value {@link #name} (The name of a query, which is used in the _query 5956 * parameter when the query is called.). This is the underlying 5957 * object with id, value and extensions. The accessor "getName" 5958 * gives direct access to the value 5959 */ 5960 public ConformanceRestOperationComponent setNameElement(StringType value) { 5961 this.name = value; 5962 return this; 5963 } 5964 5965 /** 5966 * @return The name of a query, which is used in the _query parameter when the 5967 * query is called. 5968 */ 5969 public String getName() { 5970 return this.name == null ? null : this.name.getValue(); 5971 } 5972 5973 /** 5974 * @param value The name of a query, which is used in the _query parameter when 5975 * the query is called. 5976 */ 5977 public ConformanceRestOperationComponent setName(String value) { 5978 if (this.name == null) 5979 this.name = new StringType(); 5980 this.name.setValue(value); 5981 return this; 5982 } 5983 5984 /** 5985 * @return {@link #definition} (Where the formal definition can be found.) 5986 */ 5987 public Reference getDefinition() { 5988 if (this.definition == null) 5989 if (Configuration.errorOnAutoCreate()) 5990 throw new Error("Attempt to auto-create ConformanceRestOperationComponent.definition"); 5991 else if (Configuration.doAutoCreate()) 5992 this.definition = new Reference(); // cc 5993 return this.definition; 5994 } 5995 5996 public boolean hasDefinition() { 5997 return this.definition != null && !this.definition.isEmpty(); 5998 } 5999 6000 /** 6001 * @param value {@link #definition} (Where the formal definition can be found.) 6002 */ 6003 public ConformanceRestOperationComponent setDefinition(Reference value) { 6004 this.definition = value; 6005 return this; 6006 } 6007 6008 /** 6009 * @return {@link #definition} The actual object that is the target of the 6010 * reference. The reference library doesn't populate this, but you can 6011 * use it to hold the resource if you resolve it. (Where the formal 6012 * definition can be found.) 6013 */ 6014 public OperationDefinition getDefinitionTarget() { 6015 if (this.definitionTarget == null) 6016 if (Configuration.errorOnAutoCreate()) 6017 throw new Error("Attempt to auto-create ConformanceRestOperationComponent.definition"); 6018 else if (Configuration.doAutoCreate()) 6019 this.definitionTarget = new OperationDefinition(); // aa 6020 return this.definitionTarget; 6021 } 6022 6023 /** 6024 * @param value {@link #definition} The actual object that is the target of the 6025 * reference. The reference library doesn't use these, but you can 6026 * use it to hold the resource if you resolve it. (Where the formal 6027 * definition can be found.) 6028 */ 6029 public ConformanceRestOperationComponent setDefinitionTarget(OperationDefinition value) { 6030 this.definitionTarget = value; 6031 return this; 6032 } 6033 6034 protected void listChildren(List<Property> childrenList) { 6035 super.listChildren(childrenList); 6036 childrenList.add(new Property("name", "string", 6037 "The name of a query, which is used in the _query parameter when the query is called.", 0, 6038 java.lang.Integer.MAX_VALUE, name)); 6039 childrenList.add(new Property("definition", "Reference(OperationDefinition)", 6040 "Where the formal definition can be found.", 0, java.lang.Integer.MAX_VALUE, definition)); 6041 } 6042 6043 @Override 6044 public void setProperty(String name, Base value) throws FHIRException { 6045 if (name.equals("name")) 6046 this.name = castToString(value); // StringType 6047 else if (name.equals("definition")) 6048 this.definition = castToReference(value); // Reference 6049 else 6050 super.setProperty(name, value); 6051 } 6052 6053 @Override 6054 public Base addChild(String name) throws FHIRException { 6055 if (name.equals("name")) { 6056 throw new FHIRException("Cannot call addChild on a singleton property Conformance.name"); 6057 } else if (name.equals("definition")) { 6058 this.definition = new Reference(); 6059 return this.definition; 6060 } else 6061 return super.addChild(name); 6062 } 6063 6064 public ConformanceRestOperationComponent copy() { 6065 ConformanceRestOperationComponent dst = new ConformanceRestOperationComponent(); 6066 copyValues(dst); 6067 dst.name = name == null ? null : name.copy(); 6068 dst.definition = definition == null ? null : definition.copy(); 6069 return dst; 6070 } 6071 6072 @Override 6073 public boolean equalsDeep(Base other) { 6074 if (!super.equalsDeep(other)) 6075 return false; 6076 if (!(other instanceof ConformanceRestOperationComponent)) 6077 return false; 6078 ConformanceRestOperationComponent o = (ConformanceRestOperationComponent) other; 6079 return compareDeep(name, o.name, true) && compareDeep(definition, o.definition, true); 6080 } 6081 6082 @Override 6083 public boolean equalsShallow(Base other) { 6084 if (!super.equalsShallow(other)) 6085 return false; 6086 if (!(other instanceof ConformanceRestOperationComponent)) 6087 return false; 6088 ConformanceRestOperationComponent o = (ConformanceRestOperationComponent) other; 6089 return compareValues(name, o.name, true); 6090 } 6091 6092 public boolean isEmpty() { 6093 return super.isEmpty() && (name == null || name.isEmpty()) && (definition == null || definition.isEmpty()); 6094 } 6095 6096 public String fhirType() { 6097 return "Conformance.rest.operation"; 6098 6099 } 6100 6101 } 6102 6103 @Block() 6104 public static class ConformanceMessagingComponent extends BackboneElement implements IBaseBackboneElement { 6105 /** 6106 * An endpoint (network accessible address) to which messages and/or replies are 6107 * to be sent. 6108 */ 6109 @Child(name = "endpoint", type = {}, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6110 @Description(shortDefinition = "A messaging service end-point", formalDefinition = "An endpoint (network accessible address) to which messages and/or replies are to be sent.") 6111 protected List<ConformanceMessagingEndpointComponent> endpoint; 6112 6113 /** 6114 * Length if the receiver's reliable messaging cache in minutes (if a receiver) 6115 * or how long the cache length on the receiver should be (if a sender). 6116 */ 6117 @Child(name = "reliableCache", type = { 6118 UnsignedIntType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6119 @Description(shortDefinition = "Reliable Message Cache Length (min)", formalDefinition = "Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).") 6120 protected UnsignedIntType reliableCache; 6121 6122 /** 6123 * Documentation about the system's messaging capabilities for this endpoint not 6124 * otherwise documented by the conformance statement. For example, process for 6125 * becoming an authorized messaging exchange partner. 6126 */ 6127 @Child(name = "documentation", type = { 6128 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6129 @Description(shortDefinition = "Messaging interface behavior details", formalDefinition = "Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the conformance statement. For example, process for becoming an authorized messaging exchange partner.") 6130 protected StringType documentation; 6131 6132 /** 6133 * A description of the solution's support for an event at this end-point. 6134 */ 6135 @Child(name = "event", type = {}, order = 4, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6136 @Description(shortDefinition = "Declare support for this event", formalDefinition = "A description of the solution's support for an event at this end-point.") 6137 protected List<ConformanceMessagingEventComponent> event; 6138 6139 private static final long serialVersionUID = -712362545L; 6140 6141 /* 6142 * Constructor 6143 */ 6144 public ConformanceMessagingComponent() { 6145 super(); 6146 } 6147 6148 /** 6149 * @return {@link #endpoint} (An endpoint (network accessible address) to which 6150 * messages and/or replies are to be sent.) 6151 */ 6152 public List<ConformanceMessagingEndpointComponent> getEndpoint() { 6153 if (this.endpoint == null) 6154 this.endpoint = new ArrayList<ConformanceMessagingEndpointComponent>(); 6155 return this.endpoint; 6156 } 6157 6158 public boolean hasEndpoint() { 6159 if (this.endpoint == null) 6160 return false; 6161 for (ConformanceMessagingEndpointComponent item : this.endpoint) 6162 if (!item.isEmpty()) 6163 return true; 6164 return false; 6165 } 6166 6167 /** 6168 * @return {@link #endpoint} (An endpoint (network accessible address) to which 6169 * messages and/or replies are to be sent.) 6170 */ 6171 // syntactic sugar 6172 public ConformanceMessagingEndpointComponent addEndpoint() { // 3 6173 ConformanceMessagingEndpointComponent t = new ConformanceMessagingEndpointComponent(); 6174 if (this.endpoint == null) 6175 this.endpoint = new ArrayList<ConformanceMessagingEndpointComponent>(); 6176 this.endpoint.add(t); 6177 return t; 6178 } 6179 6180 // syntactic sugar 6181 public ConformanceMessagingComponent addEndpoint(ConformanceMessagingEndpointComponent t) { // 3 6182 if (t == null) 6183 return this; 6184 if (this.endpoint == null) 6185 this.endpoint = new ArrayList<ConformanceMessagingEndpointComponent>(); 6186 this.endpoint.add(t); 6187 return this; 6188 } 6189 6190 /** 6191 * @return {@link #reliableCache} (Length if the receiver's reliable messaging 6192 * cache in minutes (if a receiver) or how long the cache length on the 6193 * receiver should be (if a sender).). This is the underlying object 6194 * with id, value and extensions. The accessor "getReliableCache" gives 6195 * direct access to the value 6196 */ 6197 public UnsignedIntType getReliableCacheElement() { 6198 if (this.reliableCache == null) 6199 if (Configuration.errorOnAutoCreate()) 6200 throw new Error("Attempt to auto-create ConformanceMessagingComponent.reliableCache"); 6201 else if (Configuration.doAutoCreate()) 6202 this.reliableCache = new UnsignedIntType(); // bb 6203 return this.reliableCache; 6204 } 6205 6206 public boolean hasReliableCacheElement() { 6207 return this.reliableCache != null && !this.reliableCache.isEmpty(); 6208 } 6209 6210 public boolean hasReliableCache() { 6211 return this.reliableCache != null && !this.reliableCache.isEmpty(); 6212 } 6213 6214 /** 6215 * @param value {@link #reliableCache} (Length if the receiver's reliable 6216 * messaging cache in minutes (if a receiver) or how long the cache 6217 * length on the receiver should be (if a sender).). This is the 6218 * underlying object with id, value and extensions. The accessor 6219 * "getReliableCache" gives direct access to the value 6220 */ 6221 public ConformanceMessagingComponent setReliableCacheElement(UnsignedIntType value) { 6222 this.reliableCache = value; 6223 return this; 6224 } 6225 6226 /** 6227 * @return Length if the receiver's reliable messaging cache in minutes (if a 6228 * receiver) or how long the cache length on the receiver should be (if 6229 * a sender). 6230 */ 6231 public int getReliableCache() { 6232 return this.reliableCache == null || this.reliableCache.isEmpty() ? 0 : this.reliableCache.getValue(); 6233 } 6234 6235 /** 6236 * @param value Length if the receiver's reliable messaging cache in minutes (if 6237 * a receiver) or how long the cache length on the receiver should 6238 * be (if a sender). 6239 */ 6240 public ConformanceMessagingComponent setReliableCache(int value) { 6241 if (this.reliableCache == null) 6242 this.reliableCache = new UnsignedIntType(); 6243 this.reliableCache.setValue(value); 6244 return this; 6245 } 6246 6247 /** 6248 * @return {@link #documentation} (Documentation about the system's messaging 6249 * capabilities for this endpoint not otherwise documented by the 6250 * conformance statement. For example, process for becoming an 6251 * authorized messaging exchange partner.). This is the underlying 6252 * object with id, value and extensions. The accessor "getDocumentation" 6253 * gives direct access to the value 6254 */ 6255 public StringType getDocumentationElement() { 6256 if (this.documentation == null) 6257 if (Configuration.errorOnAutoCreate()) 6258 throw new Error("Attempt to auto-create ConformanceMessagingComponent.documentation"); 6259 else if (Configuration.doAutoCreate()) 6260 this.documentation = new StringType(); // bb 6261 return this.documentation; 6262 } 6263 6264 public boolean hasDocumentationElement() { 6265 return this.documentation != null && !this.documentation.isEmpty(); 6266 } 6267 6268 public boolean hasDocumentation() { 6269 return this.documentation != null && !this.documentation.isEmpty(); 6270 } 6271 6272 /** 6273 * @param value {@link #documentation} (Documentation about the system's 6274 * messaging capabilities for this endpoint not otherwise 6275 * documented by the conformance statement. For example, process 6276 * for becoming an authorized messaging exchange partner.). This is 6277 * the underlying object with id, value and extensions. The 6278 * accessor "getDocumentation" gives direct access to the value 6279 */ 6280 public ConformanceMessagingComponent setDocumentationElement(StringType value) { 6281 this.documentation = value; 6282 return this; 6283 } 6284 6285 /** 6286 * @return Documentation about the system's messaging capabilities for this 6287 * endpoint not otherwise documented by the conformance statement. For 6288 * example, process for becoming an authorized messaging exchange 6289 * partner. 6290 */ 6291 public String getDocumentation() { 6292 return this.documentation == null ? null : this.documentation.getValue(); 6293 } 6294 6295 /** 6296 * @param value Documentation about the system's messaging capabilities for this 6297 * endpoint not otherwise documented by the conformance statement. 6298 * For example, process for becoming an authorized messaging 6299 * exchange partner. 6300 */ 6301 public ConformanceMessagingComponent setDocumentation(String value) { 6302 if (Utilities.noString(value)) 6303 this.documentation = null; 6304 else { 6305 if (this.documentation == null) 6306 this.documentation = new StringType(); 6307 this.documentation.setValue(value); 6308 } 6309 return this; 6310 } 6311 6312 /** 6313 * @return {@link #event} (A description of the solution's support for an event 6314 * at this end-point.) 6315 */ 6316 public List<ConformanceMessagingEventComponent> getEvent() { 6317 if (this.event == null) 6318 this.event = new ArrayList<ConformanceMessagingEventComponent>(); 6319 return this.event; 6320 } 6321 6322 public boolean hasEvent() { 6323 if (this.event == null) 6324 return false; 6325 for (ConformanceMessagingEventComponent item : this.event) 6326 if (!item.isEmpty()) 6327 return true; 6328 return false; 6329 } 6330 6331 /** 6332 * @return {@link #event} (A description of the solution's support for an event 6333 * at this end-point.) 6334 */ 6335 // syntactic sugar 6336 public ConformanceMessagingEventComponent addEvent() { // 3 6337 ConformanceMessagingEventComponent t = new ConformanceMessagingEventComponent(); 6338 if (this.event == null) 6339 this.event = new ArrayList<ConformanceMessagingEventComponent>(); 6340 this.event.add(t); 6341 return t; 6342 } 6343 6344 // syntactic sugar 6345 public ConformanceMessagingComponent addEvent(ConformanceMessagingEventComponent t) { // 3 6346 if (t == null) 6347 return this; 6348 if (this.event == null) 6349 this.event = new ArrayList<ConformanceMessagingEventComponent>(); 6350 this.event.add(t); 6351 return this; 6352 } 6353 6354 protected void listChildren(List<Property> childrenList) { 6355 super.listChildren(childrenList); 6356 childrenList.add(new Property("endpoint", "", 6357 "An endpoint (network accessible address) to which messages and/or replies are to be sent.", 0, 6358 java.lang.Integer.MAX_VALUE, endpoint)); 6359 childrenList.add(new Property("reliableCache", "unsignedInt", 6360 "Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).", 6361 0, java.lang.Integer.MAX_VALUE, reliableCache)); 6362 childrenList.add(new Property("documentation", "string", 6363 "Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the conformance statement. For example, process for becoming an authorized messaging exchange partner.", 6364 0, java.lang.Integer.MAX_VALUE, documentation)); 6365 childrenList 6366 .add(new Property("event", "", "A description of the solution's support for an event at this end-point.", 0, 6367 java.lang.Integer.MAX_VALUE, event)); 6368 } 6369 6370 @Override 6371 public void setProperty(String name, Base value) throws FHIRException { 6372 if (name.equals("endpoint")) 6373 this.getEndpoint().add((ConformanceMessagingEndpointComponent) value); 6374 else if (name.equals("reliableCache")) 6375 this.reliableCache = castToUnsignedInt(value); // UnsignedIntType 6376 else if (name.equals("documentation")) 6377 this.documentation = castToString(value); // StringType 6378 else if (name.equals("event")) 6379 this.getEvent().add((ConformanceMessagingEventComponent) value); 6380 else 6381 super.setProperty(name, value); 6382 } 6383 6384 @Override 6385 public Base addChild(String name) throws FHIRException { 6386 if (name.equals("endpoint")) { 6387 return addEndpoint(); 6388 } else if (name.equals("reliableCache")) { 6389 throw new FHIRException("Cannot call addChild on a singleton property Conformance.reliableCache"); 6390 } else if (name.equals("documentation")) { 6391 throw new FHIRException("Cannot call addChild on a singleton property Conformance.documentation"); 6392 } else if (name.equals("event")) { 6393 return addEvent(); 6394 } else 6395 return super.addChild(name); 6396 } 6397 6398 public ConformanceMessagingComponent copy() { 6399 ConformanceMessagingComponent dst = new ConformanceMessagingComponent(); 6400 copyValues(dst); 6401 if (endpoint != null) { 6402 dst.endpoint = new ArrayList<ConformanceMessagingEndpointComponent>(); 6403 for (ConformanceMessagingEndpointComponent i : endpoint) 6404 dst.endpoint.add(i.copy()); 6405 } 6406 ; 6407 dst.reliableCache = reliableCache == null ? null : reliableCache.copy(); 6408 dst.documentation = documentation == null ? null : documentation.copy(); 6409 if (event != null) { 6410 dst.event = new ArrayList<ConformanceMessagingEventComponent>(); 6411 for (ConformanceMessagingEventComponent i : event) 6412 dst.event.add(i.copy()); 6413 } 6414 ; 6415 return dst; 6416 } 6417 6418 @Override 6419 public boolean equalsDeep(Base other) { 6420 if (!super.equalsDeep(other)) 6421 return false; 6422 if (!(other instanceof ConformanceMessagingComponent)) 6423 return false; 6424 ConformanceMessagingComponent o = (ConformanceMessagingComponent) other; 6425 return compareDeep(endpoint, o.endpoint, true) && compareDeep(reliableCache, o.reliableCache, true) 6426 && compareDeep(documentation, o.documentation, true) && compareDeep(event, o.event, true); 6427 } 6428 6429 @Override 6430 public boolean equalsShallow(Base other) { 6431 if (!super.equalsShallow(other)) 6432 return false; 6433 if (!(other instanceof ConformanceMessagingComponent)) 6434 return false; 6435 ConformanceMessagingComponent o = (ConformanceMessagingComponent) other; 6436 return compareValues(reliableCache, o.reliableCache, true) && compareValues(documentation, o.documentation, true); 6437 } 6438 6439 public boolean isEmpty() { 6440 return super.isEmpty() && (endpoint == null || endpoint.isEmpty()) 6441 && (reliableCache == null || reliableCache.isEmpty()) && (documentation == null || documentation.isEmpty()) 6442 && (event == null || event.isEmpty()); 6443 } 6444 6445 public String fhirType() { 6446 return "Conformance.messaging"; 6447 6448 } 6449 6450 } 6451 6452 @Block() 6453 public static class ConformanceMessagingEndpointComponent extends BackboneElement implements IBaseBackboneElement { 6454 /** 6455 * A list of the messaging transport protocol(s) identifiers, supported by this 6456 * endpoint. 6457 */ 6458 @Child(name = "protocol", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6459 @Description(shortDefinition = "http | ftp | mllp +", formalDefinition = "A list of the messaging transport protocol(s) identifiers, supported by this endpoint.") 6460 protected Coding protocol; 6461 6462 /** 6463 * The network address of the end-point. For solutions that do not use network 6464 * addresses for routing, it can be just an identifier. 6465 */ 6466 @Child(name = "address", type = { UriType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 6467 @Description(shortDefinition = "Address of end-point", formalDefinition = "The network address of the end-point. For solutions that do not use network addresses for routing, it can be just an identifier.") 6468 protected UriType address; 6469 6470 private static final long serialVersionUID = 1294656428L; 6471 6472 /* 6473 * Constructor 6474 */ 6475 public ConformanceMessagingEndpointComponent() { 6476 super(); 6477 } 6478 6479 /* 6480 * Constructor 6481 */ 6482 public ConformanceMessagingEndpointComponent(Coding protocol, UriType address) { 6483 super(); 6484 this.protocol = protocol; 6485 this.address = address; 6486 } 6487 6488 /** 6489 * @return {@link #protocol} (A list of the messaging transport protocol(s) 6490 * identifiers, supported by this endpoint.) 6491 */ 6492 public Coding getProtocol() { 6493 if (this.protocol == null) 6494 if (Configuration.errorOnAutoCreate()) 6495 throw new Error("Attempt to auto-create ConformanceMessagingEndpointComponent.protocol"); 6496 else if (Configuration.doAutoCreate()) 6497 this.protocol = new Coding(); // cc 6498 return this.protocol; 6499 } 6500 6501 public boolean hasProtocol() { 6502 return this.protocol != null && !this.protocol.isEmpty(); 6503 } 6504 6505 /** 6506 * @param value {@link #protocol} (A list of the messaging transport protocol(s) 6507 * identifiers, supported by this endpoint.) 6508 */ 6509 public ConformanceMessagingEndpointComponent setProtocol(Coding value) { 6510 this.protocol = value; 6511 return this; 6512 } 6513 6514 /** 6515 * @return {@link #address} (The network address of the end-point. For solutions 6516 * that do not use network addresses for routing, it can be just an 6517 * identifier.). This is the underlying object with id, value and 6518 * extensions. The accessor "getAddress" gives direct access to the 6519 * value 6520 */ 6521 public UriType getAddressElement() { 6522 if (this.address == null) 6523 if (Configuration.errorOnAutoCreate()) 6524 throw new Error("Attempt to auto-create ConformanceMessagingEndpointComponent.address"); 6525 else if (Configuration.doAutoCreate()) 6526 this.address = new UriType(); // bb 6527 return this.address; 6528 } 6529 6530 public boolean hasAddressElement() { 6531 return this.address != null && !this.address.isEmpty(); 6532 } 6533 6534 public boolean hasAddress() { 6535 return this.address != null && !this.address.isEmpty(); 6536 } 6537 6538 /** 6539 * @param value {@link #address} (The network address of the end-point. For 6540 * solutions that do not use network addresses for routing, it can 6541 * be just an identifier.). This is the underlying object with id, 6542 * value and extensions. The accessor "getAddress" gives direct 6543 * access to the value 6544 */ 6545 public ConformanceMessagingEndpointComponent setAddressElement(UriType value) { 6546 this.address = value; 6547 return this; 6548 } 6549 6550 /** 6551 * @return The network address of the end-point. For solutions that do not use 6552 * network addresses for routing, it can be just an identifier. 6553 */ 6554 public String getAddress() { 6555 return this.address == null ? null : this.address.getValue(); 6556 } 6557 6558 /** 6559 * @param value The network address of the end-point. For solutions that do not 6560 * use network addresses for routing, it can be just an identifier. 6561 */ 6562 public ConformanceMessagingEndpointComponent setAddress(String value) { 6563 if (this.address == null) 6564 this.address = new UriType(); 6565 this.address.setValue(value); 6566 return this; 6567 } 6568 6569 protected void listChildren(List<Property> childrenList) { 6570 super.listChildren(childrenList); 6571 childrenList.add(new Property("protocol", "Coding", 6572 "A list of the messaging transport protocol(s) identifiers, supported by this endpoint.", 0, 6573 java.lang.Integer.MAX_VALUE, protocol)); 6574 childrenList.add(new Property("address", "uri", 6575 "The network address of the end-point. For solutions that do not use network addresses for routing, it can be just an identifier.", 6576 0, java.lang.Integer.MAX_VALUE, address)); 6577 } 6578 6579 @Override 6580 public void setProperty(String name, Base value) throws FHIRException { 6581 if (name.equals("protocol")) 6582 this.protocol = castToCoding(value); // Coding 6583 else if (name.equals("address")) 6584 this.address = castToUri(value); // UriType 6585 else 6586 super.setProperty(name, value); 6587 } 6588 6589 @Override 6590 public Base addChild(String name) throws FHIRException { 6591 if (name.equals("protocol")) { 6592 this.protocol = new Coding(); 6593 return this.protocol; 6594 } else if (name.equals("address")) { 6595 throw new FHIRException("Cannot call addChild on a singleton property Conformance.address"); 6596 } else 6597 return super.addChild(name); 6598 } 6599 6600 public ConformanceMessagingEndpointComponent copy() { 6601 ConformanceMessagingEndpointComponent dst = new ConformanceMessagingEndpointComponent(); 6602 copyValues(dst); 6603 dst.protocol = protocol == null ? null : protocol.copy(); 6604 dst.address = address == null ? null : address.copy(); 6605 return dst; 6606 } 6607 6608 @Override 6609 public boolean equalsDeep(Base other) { 6610 if (!super.equalsDeep(other)) 6611 return false; 6612 if (!(other instanceof ConformanceMessagingEndpointComponent)) 6613 return false; 6614 ConformanceMessagingEndpointComponent o = (ConformanceMessagingEndpointComponent) other; 6615 return compareDeep(protocol, o.protocol, true) && compareDeep(address, o.address, true); 6616 } 6617 6618 @Override 6619 public boolean equalsShallow(Base other) { 6620 if (!super.equalsShallow(other)) 6621 return false; 6622 if (!(other instanceof ConformanceMessagingEndpointComponent)) 6623 return false; 6624 ConformanceMessagingEndpointComponent o = (ConformanceMessagingEndpointComponent) other; 6625 return compareValues(address, o.address, true); 6626 } 6627 6628 public boolean isEmpty() { 6629 return super.isEmpty() && (protocol == null || protocol.isEmpty()) && (address == null || address.isEmpty()); 6630 } 6631 6632 public String fhirType() { 6633 return "Conformance.messaging.endpoint"; 6634 6635 } 6636 6637 } 6638 6639 @Block() 6640 public static class ConformanceMessagingEventComponent extends BackboneElement implements IBaseBackboneElement { 6641 /** 6642 * A coded identifier of a supported messaging event. 6643 */ 6644 @Child(name = "code", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6645 @Description(shortDefinition = "Event type", formalDefinition = "A coded identifier of a supported messaging event.") 6646 protected Coding code; 6647 6648 /** 6649 * The impact of the content of the message. 6650 */ 6651 @Child(name = "category", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6652 @Description(shortDefinition = "Consequence | Currency | Notification", formalDefinition = "The impact of the content of the message.") 6653 protected Enumeration<MessageSignificanceCategory> category; 6654 6655 /** 6656 * The mode of this event declaration - whether application is sender or 6657 * receiver. 6658 */ 6659 @Child(name = "mode", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 6660 @Description(shortDefinition = "sender | receiver", formalDefinition = "The mode of this event declaration - whether application is sender or receiver.") 6661 protected Enumeration<ConformanceEventMode> mode; 6662 6663 /** 6664 * A resource associated with the event. This is the resource that defines the 6665 * event. 6666 */ 6667 @Child(name = "focus", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 6668 @Description(shortDefinition = "Resource that's focus of message", formalDefinition = "A resource associated with the event. This is the resource that defines the event.") 6669 protected CodeType focus; 6670 6671 /** 6672 * Information about the request for this event. 6673 */ 6674 @Child(name = "request", type = { 6675 StructureDefinition.class }, order = 5, min = 1, max = 1, modifier = false, summary = false) 6676 @Description(shortDefinition = "Profile that describes the request", formalDefinition = "Information about the request for this event.") 6677 protected Reference request; 6678 6679 /** 6680 * The actual object that is the target of the reference (Information about the 6681 * request for this event.) 6682 */ 6683 protected StructureDefinition requestTarget; 6684 6685 /** 6686 * Information about the response for this event. 6687 */ 6688 @Child(name = "response", type = { 6689 StructureDefinition.class }, order = 6, min = 1, max = 1, modifier = false, summary = false) 6690 @Description(shortDefinition = "Profile that describes the response", formalDefinition = "Information about the response for this event.") 6691 protected Reference response; 6692 6693 /** 6694 * The actual object that is the target of the reference (Information about the 6695 * response for this event.) 6696 */ 6697 protected StructureDefinition responseTarget; 6698 6699 /** 6700 * Guidance on how this event is handled, such as internal system trigger 6701 * points, business rules, etc. 6702 */ 6703 @Child(name = "documentation", type = { 6704 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 6705 @Description(shortDefinition = "Endpoint-specific event documentation", formalDefinition = "Guidance on how this event is handled, such as internal system trigger points, business rules, etc.") 6706 protected StringType documentation; 6707 6708 private static final long serialVersionUID = -47031390L; 6709 6710 /* 6711 * Constructor 6712 */ 6713 public ConformanceMessagingEventComponent() { 6714 super(); 6715 } 6716 6717 /* 6718 * Constructor 6719 */ 6720 public ConformanceMessagingEventComponent(Coding code, Enumeration<ConformanceEventMode> mode, CodeType focus, 6721 Reference request, Reference response) { 6722 super(); 6723 this.code = code; 6724 this.mode = mode; 6725 this.focus = focus; 6726 this.request = request; 6727 this.response = response; 6728 } 6729 6730 /** 6731 * @return {@link #code} (A coded identifier of a supported messaging event.) 6732 */ 6733 public Coding getCode() { 6734 if (this.code == null) 6735 if (Configuration.errorOnAutoCreate()) 6736 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.code"); 6737 else if (Configuration.doAutoCreate()) 6738 this.code = new Coding(); // cc 6739 return this.code; 6740 } 6741 6742 public boolean hasCode() { 6743 return this.code != null && !this.code.isEmpty(); 6744 } 6745 6746 /** 6747 * @param value {@link #code} (A coded identifier of a supported messaging 6748 * event.) 6749 */ 6750 public ConformanceMessagingEventComponent setCode(Coding value) { 6751 this.code = value; 6752 return this; 6753 } 6754 6755 /** 6756 * @return {@link #category} (The impact of the content of the message.). This 6757 * is the underlying object with id, value and extensions. The accessor 6758 * "getCategory" gives direct access to the value 6759 */ 6760 public Enumeration<MessageSignificanceCategory> getCategoryElement() { 6761 if (this.category == null) 6762 if (Configuration.errorOnAutoCreate()) 6763 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.category"); 6764 else if (Configuration.doAutoCreate()) 6765 this.category = new Enumeration<MessageSignificanceCategory>(new MessageSignificanceCategoryEnumFactory()); // bb 6766 return this.category; 6767 } 6768 6769 public boolean hasCategoryElement() { 6770 return this.category != null && !this.category.isEmpty(); 6771 } 6772 6773 public boolean hasCategory() { 6774 return this.category != null && !this.category.isEmpty(); 6775 } 6776 6777 /** 6778 * @param value {@link #category} (The impact of the content of the message.). 6779 * This is the underlying object with id, value and extensions. The 6780 * accessor "getCategory" gives direct access to the value 6781 */ 6782 public ConformanceMessagingEventComponent setCategoryElement(Enumeration<MessageSignificanceCategory> value) { 6783 this.category = value; 6784 return this; 6785 } 6786 6787 /** 6788 * @return The impact of the content of the message. 6789 */ 6790 public MessageSignificanceCategory getCategory() { 6791 return this.category == null ? null : this.category.getValue(); 6792 } 6793 6794 /** 6795 * @param value The impact of the content of the message. 6796 */ 6797 public ConformanceMessagingEventComponent setCategory(MessageSignificanceCategory value) { 6798 if (value == null) 6799 this.category = null; 6800 else { 6801 if (this.category == null) 6802 this.category = new Enumeration<MessageSignificanceCategory>(new MessageSignificanceCategoryEnumFactory()); 6803 this.category.setValue(value); 6804 } 6805 return this; 6806 } 6807 6808 /** 6809 * @return {@link #mode} (The mode of this event declaration - whether 6810 * application is sender or receiver.). This is the underlying object 6811 * with id, value and extensions. The accessor "getMode" gives direct 6812 * access to the value 6813 */ 6814 public Enumeration<ConformanceEventMode> getModeElement() { 6815 if (this.mode == null) 6816 if (Configuration.errorOnAutoCreate()) 6817 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.mode"); 6818 else if (Configuration.doAutoCreate()) 6819 this.mode = new Enumeration<ConformanceEventMode>(new ConformanceEventModeEnumFactory()); // bb 6820 return this.mode; 6821 } 6822 6823 public boolean hasModeElement() { 6824 return this.mode != null && !this.mode.isEmpty(); 6825 } 6826 6827 public boolean hasMode() { 6828 return this.mode != null && !this.mode.isEmpty(); 6829 } 6830 6831 /** 6832 * @param value {@link #mode} (The mode of this event declaration - whether 6833 * application is sender or receiver.). This is the underlying 6834 * object with id, value and extensions. The accessor "getMode" 6835 * gives direct access to the value 6836 */ 6837 public ConformanceMessagingEventComponent setModeElement(Enumeration<ConformanceEventMode> value) { 6838 this.mode = value; 6839 return this; 6840 } 6841 6842 /** 6843 * @return The mode of this event declaration - whether application is sender or 6844 * receiver. 6845 */ 6846 public ConformanceEventMode getMode() { 6847 return this.mode == null ? null : this.mode.getValue(); 6848 } 6849 6850 /** 6851 * @param value The mode of this event declaration - whether application is 6852 * sender or receiver. 6853 */ 6854 public ConformanceMessagingEventComponent setMode(ConformanceEventMode value) { 6855 if (this.mode == null) 6856 this.mode = new Enumeration<ConformanceEventMode>(new ConformanceEventModeEnumFactory()); 6857 this.mode.setValue(value); 6858 return this; 6859 } 6860 6861 /** 6862 * @return {@link #focus} (A resource associated with the event. This is the 6863 * resource that defines the event.). This is the underlying object with 6864 * id, value and extensions. The accessor "getFocus" gives direct access 6865 * to the value 6866 */ 6867 public CodeType getFocusElement() { 6868 if (this.focus == null) 6869 if (Configuration.errorOnAutoCreate()) 6870 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.focus"); 6871 else if (Configuration.doAutoCreate()) 6872 this.focus = new CodeType(); // bb 6873 return this.focus; 6874 } 6875 6876 public boolean hasFocusElement() { 6877 return this.focus != null && !this.focus.isEmpty(); 6878 } 6879 6880 public boolean hasFocus() { 6881 return this.focus != null && !this.focus.isEmpty(); 6882 } 6883 6884 /** 6885 * @param value {@link #focus} (A resource associated with the event. This is 6886 * the resource that defines the event.). This is the underlying 6887 * object with id, value and extensions. The accessor "getFocus" 6888 * gives direct access to the value 6889 */ 6890 public ConformanceMessagingEventComponent setFocusElement(CodeType value) { 6891 this.focus = value; 6892 return this; 6893 } 6894 6895 /** 6896 * @return A resource associated with the event. This is the resource that 6897 * defines the event. 6898 */ 6899 public String getFocus() { 6900 return this.focus == null ? null : this.focus.getValue(); 6901 } 6902 6903 /** 6904 * @param value A resource associated with the event. This is the resource that 6905 * defines the event. 6906 */ 6907 public ConformanceMessagingEventComponent setFocus(String value) { 6908 if (this.focus == null) 6909 this.focus = new CodeType(); 6910 this.focus.setValue(value); 6911 return this; 6912 } 6913 6914 /** 6915 * @return {@link #request} (Information about the request for this event.) 6916 */ 6917 public Reference getRequest() { 6918 if (this.request == null) 6919 if (Configuration.errorOnAutoCreate()) 6920 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.request"); 6921 else if (Configuration.doAutoCreate()) 6922 this.request = new Reference(); // cc 6923 return this.request; 6924 } 6925 6926 public boolean hasRequest() { 6927 return this.request != null && !this.request.isEmpty(); 6928 } 6929 6930 /** 6931 * @param value {@link #request} (Information about the request for this event.) 6932 */ 6933 public ConformanceMessagingEventComponent setRequest(Reference value) { 6934 this.request = value; 6935 return this; 6936 } 6937 6938 /** 6939 * @return {@link #request} The actual object that is the target of the 6940 * reference. The reference library doesn't populate this, but you can 6941 * use it to hold the resource if you resolve it. (Information about the 6942 * request for this event.) 6943 */ 6944 public StructureDefinition getRequestTarget() { 6945 if (this.requestTarget == null) 6946 if (Configuration.errorOnAutoCreate()) 6947 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.request"); 6948 else if (Configuration.doAutoCreate()) 6949 this.requestTarget = new StructureDefinition(); // aa 6950 return this.requestTarget; 6951 } 6952 6953 /** 6954 * @param value {@link #request} The actual object that is the target of the 6955 * reference. The reference library doesn't use these, but you can 6956 * use it to hold the resource if you resolve it. (Information 6957 * about the request for this event.) 6958 */ 6959 public ConformanceMessagingEventComponent setRequestTarget(StructureDefinition value) { 6960 this.requestTarget = value; 6961 return this; 6962 } 6963 6964 /** 6965 * @return {@link #response} (Information about the response for this event.) 6966 */ 6967 public Reference getResponse() { 6968 if (this.response == null) 6969 if (Configuration.errorOnAutoCreate()) 6970 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.response"); 6971 else if (Configuration.doAutoCreate()) 6972 this.response = new Reference(); // cc 6973 return this.response; 6974 } 6975 6976 public boolean hasResponse() { 6977 return this.response != null && !this.response.isEmpty(); 6978 } 6979 6980 /** 6981 * @param value {@link #response} (Information about the response for this 6982 * event.) 6983 */ 6984 public ConformanceMessagingEventComponent setResponse(Reference value) { 6985 this.response = value; 6986 return this; 6987 } 6988 6989 /** 6990 * @return {@link #response} The actual object that is the target of the 6991 * reference. The reference library doesn't populate this, but you can 6992 * use it to hold the resource if you resolve it. (Information about the 6993 * response for this event.) 6994 */ 6995 public StructureDefinition getResponseTarget() { 6996 if (this.responseTarget == null) 6997 if (Configuration.errorOnAutoCreate()) 6998 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.response"); 6999 else if (Configuration.doAutoCreate()) 7000 this.responseTarget = new StructureDefinition(); // aa 7001 return this.responseTarget; 7002 } 7003 7004 /** 7005 * @param value {@link #response} The actual object that is the target of the 7006 * reference. The reference library doesn't use these, but you can 7007 * use it to hold the resource if you resolve it. (Information 7008 * about the response for this event.) 7009 */ 7010 public ConformanceMessagingEventComponent setResponseTarget(StructureDefinition value) { 7011 this.responseTarget = value; 7012 return this; 7013 } 7014 7015 /** 7016 * @return {@link #documentation} (Guidance on how this event is handled, such 7017 * as internal system trigger points, business rules, etc.). This is the 7018 * underlying object with id, value and extensions. The accessor 7019 * "getDocumentation" gives direct access to the value 7020 */ 7021 public StringType getDocumentationElement() { 7022 if (this.documentation == null) 7023 if (Configuration.errorOnAutoCreate()) 7024 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.documentation"); 7025 else if (Configuration.doAutoCreate()) 7026 this.documentation = new StringType(); // bb 7027 return this.documentation; 7028 } 7029 7030 public boolean hasDocumentationElement() { 7031 return this.documentation != null && !this.documentation.isEmpty(); 7032 } 7033 7034 public boolean hasDocumentation() { 7035 return this.documentation != null && !this.documentation.isEmpty(); 7036 } 7037 7038 /** 7039 * @param value {@link #documentation} (Guidance on how this event is handled, 7040 * such as internal system trigger points, business rules, etc.). 7041 * This is the underlying object with id, value and extensions. The 7042 * accessor "getDocumentation" gives direct access to the value 7043 */ 7044 public ConformanceMessagingEventComponent setDocumentationElement(StringType value) { 7045 this.documentation = value; 7046 return this; 7047 } 7048 7049 /** 7050 * @return Guidance on how this event is handled, such as internal system 7051 * trigger points, business rules, etc. 7052 */ 7053 public String getDocumentation() { 7054 return this.documentation == null ? null : this.documentation.getValue(); 7055 } 7056 7057 /** 7058 * @param value Guidance on how this event is handled, such as internal system 7059 * trigger points, business rules, etc. 7060 */ 7061 public ConformanceMessagingEventComponent setDocumentation(String value) { 7062 if (Utilities.noString(value)) 7063 this.documentation = null; 7064 else { 7065 if (this.documentation == null) 7066 this.documentation = new StringType(); 7067 this.documentation.setValue(value); 7068 } 7069 return this; 7070 } 7071 7072 protected void listChildren(List<Property> childrenList) { 7073 super.listChildren(childrenList); 7074 childrenList.add(new Property("code", "Coding", "A coded identifier of a supported messaging event.", 0, 7075 java.lang.Integer.MAX_VALUE, code)); 7076 childrenList.add(new Property("category", "code", "The impact of the content of the message.", 0, 7077 java.lang.Integer.MAX_VALUE, category)); 7078 childrenList.add(new Property("mode", "code", 7079 "The mode of this event declaration - whether application is sender or receiver.", 0, 7080 java.lang.Integer.MAX_VALUE, mode)); 7081 childrenList.add(new Property("focus", "code", 7082 "A resource associated with the event. This is the resource that defines the event.", 0, 7083 java.lang.Integer.MAX_VALUE, focus)); 7084 childrenList.add(new Property("request", "Reference(StructureDefinition)", 7085 "Information about the request for this event.", 0, java.lang.Integer.MAX_VALUE, request)); 7086 childrenList.add(new Property("response", "Reference(StructureDefinition)", 7087 "Information about the response for this event.", 0, java.lang.Integer.MAX_VALUE, response)); 7088 childrenList.add(new Property("documentation", "string", 7089 "Guidance on how this event is handled, such as internal system trigger points, business rules, etc.", 0, 7090 java.lang.Integer.MAX_VALUE, documentation)); 7091 } 7092 7093 @Override 7094 public void setProperty(String name, Base value) throws FHIRException { 7095 if (name.equals("code")) 7096 this.code = castToCoding(value); // Coding 7097 else if (name.equals("category")) 7098 this.category = new MessageSignificanceCategoryEnumFactory().fromType(value); // Enumeration<MessageSignificanceCategory> 7099 else if (name.equals("mode")) 7100 this.mode = new ConformanceEventModeEnumFactory().fromType(value); // Enumeration<ConformanceEventMode> 7101 else if (name.equals("focus")) 7102 this.focus = castToCode(value); // CodeType 7103 else if (name.equals("request")) 7104 this.request = castToReference(value); // Reference 7105 else if (name.equals("response")) 7106 this.response = castToReference(value); // Reference 7107 else if (name.equals("documentation")) 7108 this.documentation = castToString(value); // StringType 7109 else 7110 super.setProperty(name, value); 7111 } 7112 7113 @Override 7114 public Base addChild(String name) throws FHIRException { 7115 if (name.equals("code")) { 7116 this.code = new Coding(); 7117 return this.code; 7118 } else if (name.equals("category")) { 7119 throw new FHIRException("Cannot call addChild on a singleton property Conformance.category"); 7120 } else if (name.equals("mode")) { 7121 throw new FHIRException("Cannot call addChild on a singleton property Conformance.mode"); 7122 } else if (name.equals("focus")) { 7123 throw new FHIRException("Cannot call addChild on a singleton property Conformance.focus"); 7124 } else if (name.equals("request")) { 7125 this.request = new Reference(); 7126 return this.request; 7127 } else if (name.equals("response")) { 7128 this.response = new Reference(); 7129 return this.response; 7130 } else if (name.equals("documentation")) { 7131 throw new FHIRException("Cannot call addChild on a singleton property Conformance.documentation"); 7132 } else 7133 return super.addChild(name); 7134 } 7135 7136 public ConformanceMessagingEventComponent copy() { 7137 ConformanceMessagingEventComponent dst = new ConformanceMessagingEventComponent(); 7138 copyValues(dst); 7139 dst.code = code == null ? null : code.copy(); 7140 dst.category = category == null ? null : category.copy(); 7141 dst.mode = mode == null ? null : mode.copy(); 7142 dst.focus = focus == null ? null : focus.copy(); 7143 dst.request = request == null ? null : request.copy(); 7144 dst.response = response == null ? null : response.copy(); 7145 dst.documentation = documentation == null ? null : documentation.copy(); 7146 return dst; 7147 } 7148 7149 @Override 7150 public boolean equalsDeep(Base other) { 7151 if (!super.equalsDeep(other)) 7152 return false; 7153 if (!(other instanceof ConformanceMessagingEventComponent)) 7154 return false; 7155 ConformanceMessagingEventComponent o = (ConformanceMessagingEventComponent) other; 7156 return compareDeep(code, o.code, true) && compareDeep(category, o.category, true) 7157 && compareDeep(mode, o.mode, true) && compareDeep(focus, o.focus, true) 7158 && compareDeep(request, o.request, true) && compareDeep(response, o.response, true) 7159 && compareDeep(documentation, o.documentation, true); 7160 } 7161 7162 @Override 7163 public boolean equalsShallow(Base other) { 7164 if (!super.equalsShallow(other)) 7165 return false; 7166 if (!(other instanceof ConformanceMessagingEventComponent)) 7167 return false; 7168 ConformanceMessagingEventComponent o = (ConformanceMessagingEventComponent) other; 7169 return compareValues(category, o.category, true) && compareValues(mode, o.mode, true) 7170 && compareValues(focus, o.focus, true) && compareValues(documentation, o.documentation, true); 7171 } 7172 7173 public boolean isEmpty() { 7174 return super.isEmpty() && (code == null || code.isEmpty()) && (category == null || category.isEmpty()) 7175 && (mode == null || mode.isEmpty()) && (focus == null || focus.isEmpty()) 7176 && (request == null || request.isEmpty()) && (response == null || response.isEmpty()) 7177 && (documentation == null || documentation.isEmpty()); 7178 } 7179 7180 public String fhirType() { 7181 return "Conformance.messaging.event"; 7182 7183 } 7184 7185 } 7186 7187 @Block() 7188 public static class ConformanceDocumentComponent extends BackboneElement implements IBaseBackboneElement { 7189 /** 7190 * Mode of this document declaration - whether application is producer or 7191 * consumer. 7192 */ 7193 @Child(name = "mode", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 7194 @Description(shortDefinition = "producer | consumer", formalDefinition = "Mode of this document declaration - whether application is producer or consumer.") 7195 protected Enumeration<DocumentMode> mode; 7196 7197 /** 7198 * A description of how the application supports or uses the specified document 7199 * profile. For example, when are documents created, what action is taken with 7200 * consumed documents, etc. 7201 */ 7202 @Child(name = "documentation", type = { 7203 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 7204 @Description(shortDefinition = "Description of document support", formalDefinition = "A description of how the application supports or uses the specified document profile. For example, when are documents created, what action is taken with consumed documents, etc.") 7205 protected StringType documentation; 7206 7207 /** 7208 * A constraint on a resource used in the document. 7209 */ 7210 @Child(name = "profile", type = { 7211 StructureDefinition.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 7212 @Description(shortDefinition = "Constraint on a resource used in the document", formalDefinition = "A constraint on a resource used in the document.") 7213 protected Reference profile; 7214 7215 /** 7216 * The actual object that is the target of the reference (A constraint on a 7217 * resource used in the document.) 7218 */ 7219 protected StructureDefinition profileTarget; 7220 7221 private static final long serialVersionUID = -1059555053L; 7222 7223 /* 7224 * Constructor 7225 */ 7226 public ConformanceDocumentComponent() { 7227 super(); 7228 } 7229 7230 /* 7231 * Constructor 7232 */ 7233 public ConformanceDocumentComponent(Enumeration<DocumentMode> mode, Reference profile) { 7234 super(); 7235 this.mode = mode; 7236 this.profile = profile; 7237 } 7238 7239 /** 7240 * @return {@link #mode} (Mode of this document declaration - whether 7241 * application is producer or consumer.). This is the underlying object 7242 * with id, value and extensions. The accessor "getMode" gives direct 7243 * access to the value 7244 */ 7245 public Enumeration<DocumentMode> getModeElement() { 7246 if (this.mode == null) 7247 if (Configuration.errorOnAutoCreate()) 7248 throw new Error("Attempt to auto-create ConformanceDocumentComponent.mode"); 7249 else if (Configuration.doAutoCreate()) 7250 this.mode = new Enumeration<DocumentMode>(new DocumentModeEnumFactory()); // bb 7251 return this.mode; 7252 } 7253 7254 public boolean hasModeElement() { 7255 return this.mode != null && !this.mode.isEmpty(); 7256 } 7257 7258 public boolean hasMode() { 7259 return this.mode != null && !this.mode.isEmpty(); 7260 } 7261 7262 /** 7263 * @param value {@link #mode} (Mode of this document declaration - whether 7264 * application is producer or consumer.). This is the underlying 7265 * object with id, value and extensions. The accessor "getMode" 7266 * gives direct access to the value 7267 */ 7268 public ConformanceDocumentComponent setModeElement(Enumeration<DocumentMode> value) { 7269 this.mode = value; 7270 return this; 7271 } 7272 7273 /** 7274 * @return Mode of this document declaration - whether application is producer 7275 * or consumer. 7276 */ 7277 public DocumentMode getMode() { 7278 return this.mode == null ? null : this.mode.getValue(); 7279 } 7280 7281 /** 7282 * @param value Mode of this document declaration - whether application is 7283 * producer or consumer. 7284 */ 7285 public ConformanceDocumentComponent setMode(DocumentMode value) { 7286 if (this.mode == null) 7287 this.mode = new Enumeration<DocumentMode>(new DocumentModeEnumFactory()); 7288 this.mode.setValue(value); 7289 return this; 7290 } 7291 7292 /** 7293 * @return {@link #documentation} (A description of how the application supports 7294 * or uses the specified document profile. For example, when are 7295 * documents created, what action is taken with consumed documents, 7296 * etc.). This is the underlying object with id, value and extensions. 7297 * The accessor "getDocumentation" gives direct access to the value 7298 */ 7299 public StringType getDocumentationElement() { 7300 if (this.documentation == null) 7301 if (Configuration.errorOnAutoCreate()) 7302 throw new Error("Attempt to auto-create ConformanceDocumentComponent.documentation"); 7303 else if (Configuration.doAutoCreate()) 7304 this.documentation = new StringType(); // bb 7305 return this.documentation; 7306 } 7307 7308 public boolean hasDocumentationElement() { 7309 return this.documentation != null && !this.documentation.isEmpty(); 7310 } 7311 7312 public boolean hasDocumentation() { 7313 return this.documentation != null && !this.documentation.isEmpty(); 7314 } 7315 7316 /** 7317 * @param value {@link #documentation} (A description of how the application 7318 * supports or uses the specified document profile. For example, 7319 * when are documents created, what action is taken with consumed 7320 * documents, etc.). This is the underlying object with id, value 7321 * and extensions. The accessor "getDocumentation" gives direct 7322 * access to the value 7323 */ 7324 public ConformanceDocumentComponent setDocumentationElement(StringType value) { 7325 this.documentation = value; 7326 return this; 7327 } 7328 7329 /** 7330 * @return A description of how the application supports or uses the specified 7331 * document profile. For example, when are documents created, what 7332 * action is taken with consumed documents, etc. 7333 */ 7334 public String getDocumentation() { 7335 return this.documentation == null ? null : this.documentation.getValue(); 7336 } 7337 7338 /** 7339 * @param value A description of how the application supports or uses the 7340 * specified document profile. For example, when are documents 7341 * created, what action is taken with consumed documents, etc. 7342 */ 7343 public ConformanceDocumentComponent setDocumentation(String value) { 7344 if (Utilities.noString(value)) 7345 this.documentation = null; 7346 else { 7347 if (this.documentation == null) 7348 this.documentation = new StringType(); 7349 this.documentation.setValue(value); 7350 } 7351 return this; 7352 } 7353 7354 /** 7355 * @return {@link #profile} (A constraint on a resource used in the document.) 7356 */ 7357 public Reference getProfile() { 7358 if (this.profile == null) 7359 if (Configuration.errorOnAutoCreate()) 7360 throw new Error("Attempt to auto-create ConformanceDocumentComponent.profile"); 7361 else if (Configuration.doAutoCreate()) 7362 this.profile = new Reference(); // cc 7363 return this.profile; 7364 } 7365 7366 public boolean hasProfile() { 7367 return this.profile != null && !this.profile.isEmpty(); 7368 } 7369 7370 /** 7371 * @param value {@link #profile} (A constraint on a resource used in the 7372 * document.) 7373 */ 7374 public ConformanceDocumentComponent setProfile(Reference value) { 7375 this.profile = value; 7376 return this; 7377 } 7378 7379 /** 7380 * @return {@link #profile} The actual object that is the target of the 7381 * reference. The reference library doesn't populate this, but you can 7382 * use it to hold the resource if you resolve it. (A constraint on a 7383 * resource used in the document.) 7384 */ 7385 public StructureDefinition getProfileTarget() { 7386 if (this.profileTarget == null) 7387 if (Configuration.errorOnAutoCreate()) 7388 throw new Error("Attempt to auto-create ConformanceDocumentComponent.profile"); 7389 else if (Configuration.doAutoCreate()) 7390 this.profileTarget = new StructureDefinition(); // aa 7391 return this.profileTarget; 7392 } 7393 7394 /** 7395 * @param value {@link #profile} The actual object that is the target of the 7396 * reference. The reference library doesn't use these, but you can 7397 * use it to hold the resource if you resolve it. (A constraint on 7398 * a resource used in the document.) 7399 */ 7400 public ConformanceDocumentComponent setProfileTarget(StructureDefinition value) { 7401 this.profileTarget = value; 7402 return this; 7403 } 7404 7405 protected void listChildren(List<Property> childrenList) { 7406 super.listChildren(childrenList); 7407 childrenList.add(new Property("mode", "code", 7408 "Mode of this document declaration - whether application is producer or consumer.", 0, 7409 java.lang.Integer.MAX_VALUE, mode)); 7410 childrenList.add(new Property("documentation", "string", 7411 "A description of how the application supports or uses the specified document profile. For example, when are documents created, what action is taken with consumed documents, etc.", 7412 0, java.lang.Integer.MAX_VALUE, documentation)); 7413 childrenList.add(new Property("profile", "Reference(StructureDefinition)", 7414 "A constraint on a resource used in the document.", 0, java.lang.Integer.MAX_VALUE, profile)); 7415 } 7416 7417 @Override 7418 public void setProperty(String name, Base value) throws FHIRException { 7419 if (name.equals("mode")) 7420 this.mode = new DocumentModeEnumFactory().fromType(value); // Enumeration<DocumentMode> 7421 else if (name.equals("documentation")) 7422 this.documentation = castToString(value); // StringType 7423 else if (name.equals("profile")) 7424 this.profile = castToReference(value); // Reference 7425 else 7426 super.setProperty(name, value); 7427 } 7428 7429 @Override 7430 public Base addChild(String name) throws FHIRException { 7431 if (name.equals("mode")) { 7432 throw new FHIRException("Cannot call addChild on a singleton property Conformance.mode"); 7433 } else if (name.equals("documentation")) { 7434 throw new FHIRException("Cannot call addChild on a singleton property Conformance.documentation"); 7435 } else if (name.equals("profile")) { 7436 this.profile = new Reference(); 7437 return this.profile; 7438 } else 7439 return super.addChild(name); 7440 } 7441 7442 public ConformanceDocumentComponent copy() { 7443 ConformanceDocumentComponent dst = new ConformanceDocumentComponent(); 7444 copyValues(dst); 7445 dst.mode = mode == null ? null : mode.copy(); 7446 dst.documentation = documentation == null ? null : documentation.copy(); 7447 dst.profile = profile == null ? null : profile.copy(); 7448 return dst; 7449 } 7450 7451 @Override 7452 public boolean equalsDeep(Base other) { 7453 if (!super.equalsDeep(other)) 7454 return false; 7455 if (!(other instanceof ConformanceDocumentComponent)) 7456 return false; 7457 ConformanceDocumentComponent o = (ConformanceDocumentComponent) other; 7458 return compareDeep(mode, o.mode, true) && compareDeep(documentation, o.documentation, true) 7459 && compareDeep(profile, o.profile, true); 7460 } 7461 7462 @Override 7463 public boolean equalsShallow(Base other) { 7464 if (!super.equalsShallow(other)) 7465 return false; 7466 if (!(other instanceof ConformanceDocumentComponent)) 7467 return false; 7468 ConformanceDocumentComponent o = (ConformanceDocumentComponent) other; 7469 return compareValues(mode, o.mode, true) && compareValues(documentation, o.documentation, true); 7470 } 7471 7472 public boolean isEmpty() { 7473 return super.isEmpty() && (mode == null || mode.isEmpty()) && (documentation == null || documentation.isEmpty()) 7474 && (profile == null || profile.isEmpty()); 7475 } 7476 7477 public String fhirType() { 7478 return "Conformance.document"; 7479 7480 } 7481 7482 } 7483 7484 /** 7485 * An absolute URL that is used to identify this conformance statement when it 7486 * is referenced in a specification, model, design or an instance. This SHALL be 7487 * a URL, SHOULD be globally unique, and SHOULD be an address at which this 7488 * conformance statement is (or will be) published. 7489 */ 7490 @Child(name = "url", type = { UriType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 7491 @Description(shortDefinition = "Logical uri to reference this statement", formalDefinition = "An absolute URL that is used to identify this conformance statement when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this conformance statement is (or will be) published.") 7492 protected UriType url; 7493 7494 /** 7495 * The identifier that is used to identify this version of the conformance 7496 * statement when it is referenced in a specification, model, design or 7497 * instance. This is an arbitrary value managed by the profile author manually 7498 * and the value should be a timestamp. 7499 */ 7500 @Child(name = "version", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 7501 @Description(shortDefinition = "Logical id for this version of the statement", formalDefinition = "The identifier that is used to identify this version of the conformance statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the profile author manually and the value should be a timestamp.") 7502 protected StringType version; 7503 7504 /** 7505 * A free text natural language name identifying the conformance statement. 7506 */ 7507 @Child(name = "name", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 7508 @Description(shortDefinition = "Informal name for this conformance statement", formalDefinition = "A free text natural language name identifying the conformance statement.") 7509 protected StringType name; 7510 7511 /** 7512 * The status of this conformance statement. 7513 */ 7514 @Child(name = "status", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = true, summary = true) 7515 @Description(shortDefinition = "draft | active | retired", formalDefinition = "The status of this conformance statement.") 7516 protected Enumeration<ConformanceResourceStatus> status; 7517 7518 /** 7519 * A flag to indicate that this conformance statement is authored for testing 7520 * purposes (or education/evaluation/marketing), and is not intended to be used 7521 * for genuine usage. 7522 */ 7523 @Child(name = "experimental", type = { 7524 BooleanType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 7525 @Description(shortDefinition = "If for testing purposes, not real usage", formalDefinition = "A flag to indicate that this conformance statement is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.") 7526 protected BooleanType experimental; 7527 7528 /** 7529 * The name of the individual or organization that published the conformance. 7530 */ 7531 @Child(name = "publisher", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 7532 @Description(shortDefinition = "Name of the publisher (Organization or individual)", formalDefinition = "The name of the individual or organization that published the conformance.") 7533 protected StringType publisher; 7534 7535 /** 7536 * Contacts to assist a user in finding and communicating with the publisher. 7537 */ 7538 @Child(name = "contact", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 7539 @Description(shortDefinition = "Contact details of the publisher", formalDefinition = "Contacts to assist a user in finding and communicating with the publisher.") 7540 protected List<ConformanceContactComponent> contact; 7541 7542 /** 7543 * The date (and optionally time) when the conformance statement was published. 7544 * The date must change when the business version changes, if it does, and it 7545 * must change if the status code changes. In addition, it should change when 7546 * the substantive content of the conformance statement changes. 7547 */ 7548 @Child(name = "date", type = { DateTimeType.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 7549 @Description(shortDefinition = "Publication Date(/time)", formalDefinition = "The date (and optionally time) when the conformance statement was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the conformance statement changes.") 7550 protected DateTimeType date; 7551 7552 /** 7553 * A free text natural language description of the conformance statement and its 7554 * use. Typically, this is used when the conformance statement describes a 7555 * desired rather than an actual solution, for example as a formal expression of 7556 * requirements as part of an RFP. 7557 */ 7558 @Child(name = "description", type = { 7559 StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 7560 @Description(shortDefinition = "Human description of the conformance statement", formalDefinition = "A free text natural language description of the conformance statement and its use. Typically, this is used when the conformance statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.") 7561 protected StringType description; 7562 7563 /** 7564 * Explains why this conformance statement is needed and why it's been 7565 * constrained as it has. 7566 */ 7567 @Child(name = "requirements", type = { 7568 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 7569 @Description(shortDefinition = "Why is this needed?", formalDefinition = "Explains why this conformance statement is needed and why it's been constrained as it has.") 7570 protected StringType requirements; 7571 7572 /** 7573 * A copyright statement relating to the conformance statement and/or its 7574 * contents. Copyright statements are generally legal restrictions on the use 7575 * and publishing of the details of the system described by the conformance 7576 * statement. 7577 */ 7578 @Child(name = "copyright", type = { 7579 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 7580 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the conformance statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the details of the system described by the conformance statement.") 7581 protected StringType copyright; 7582 7583 /** 7584 * The way that this statement is intended to be used, to describe an actual 7585 * running instance of software, a particular product (kind not instance of 7586 * software) or a class of implementation (e.g. a desired purchase). 7587 */ 7588 @Child(name = "kind", type = { CodeType.class }, order = 11, min = 1, max = 1, modifier = false, summary = true) 7589 @Description(shortDefinition = "instance | capability | requirements", formalDefinition = "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind not instance of software) or a class of implementation (e.g. a desired purchase).") 7590 protected Enumeration<ConformanceStatementKind> kind; 7591 7592 /** 7593 * Software that is covered by this conformance statement. It is used when the 7594 * conformance statement describes the capabilities of a particular software 7595 * version, independent of an installation. 7596 */ 7597 @Child(name = "software", type = {}, order = 12, min = 0, max = 1, modifier = false, summary = true) 7598 @Description(shortDefinition = "Software that is covered by this conformance statement", formalDefinition = "Software that is covered by this conformance statement. It is used when the conformance statement describes the capabilities of a particular software version, independent of an installation.") 7599 protected ConformanceSoftwareComponent software; 7600 7601 /** 7602 * Identifies a specific implementation instance that is described by the 7603 * conformance statement - i.e. a particular installation, rather than the 7604 * capabilities of a software program. 7605 */ 7606 @Child(name = "implementation", type = {}, order = 13, min = 0, max = 1, modifier = false, summary = true) 7607 @Description(shortDefinition = "If this describes a specific instance", formalDefinition = "Identifies a specific implementation instance that is described by the conformance statement - i.e. a particular installation, rather than the capabilities of a software program.") 7608 protected ConformanceImplementationComponent implementation; 7609 7610 /** 7611 * The version of the FHIR specification on which this conformance statement is 7612 * based. 7613 */ 7614 @Child(name = "fhirVersion", type = { IdType.class }, order = 14, min = 1, max = 1, modifier = false, summary = true) 7615 @Description(shortDefinition = "FHIR Version the system uses", formalDefinition = "The version of the FHIR specification on which this conformance statement is based.") 7616 protected IdType fhirVersion; 7617 7618 /** 7619 * A code that indicates whether the application accepts unknown elements or 7620 * extensions when reading resources. 7621 */ 7622 @Child(name = "acceptUnknown", type = { 7623 CodeType.class }, order = 15, min = 1, max = 1, modifier = false, summary = true) 7624 @Description(shortDefinition = "no | extensions | elements | both", formalDefinition = "A code that indicates whether the application accepts unknown elements or extensions when reading resources.") 7625 protected Enumeration<UnknownContentCode> acceptUnknown; 7626 7627 /** 7628 * A list of the formats supported by this implementation using their content 7629 * types. 7630 */ 7631 @Child(name = "format", type = { 7632 CodeType.class }, order = 16, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 7633 @Description(shortDefinition = "formats supported (xml | json | mime type)", formalDefinition = "A list of the formats supported by this implementation using their content types.") 7634 protected List<CodeType> format; 7635 7636 /** 7637 * A list of profiles that represent different use cases supported by the 7638 * system. For a server, "supported by the system" means the system 7639 * hosts/produces a set of resources that are conformant to a particular 7640 * profile, and allows clients that use its services to search using this 7641 * profile and to find appropriate data. For a client, it means the system will 7642 * search by this profile and process data according to the guidance implicit in 7643 * the profile. See further discussion in [Using 7644 * Profiles]{profiling.html#profile-uses}. 7645 */ 7646 @Child(name = "profile", type = { 7647 StructureDefinition.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7648 @Description(shortDefinition = "Profiles for use cases supported", formalDefinition = "A list of profiles that represent different use cases supported by the system. For a server, \"supported by the system\" means the system hosts/produces a set of resources that are conformant to a particular profile, and allows clients that use its services to search using this profile and to find appropriate data. For a client, it means the system will search by this profile and process data according to the guidance implicit in the profile. See further discussion in [Using Profiles]{profiling.html#profile-uses}.") 7649 protected List<Reference> profile; 7650 /** 7651 * The actual objects that are the target of the reference (A list of profiles 7652 * that represent different use cases supported by the system. For a server, 7653 * "supported by the system" means the system hosts/produces a set of resources 7654 * that are conformant to a particular profile, and allows clients that use its 7655 * services to search using this profile and to find appropriate data. For a 7656 * client, it means the system will search by this profile and process data 7657 * according to the guidance implicit in the profile. See further discussion in 7658 * [Using Profiles]{profiling.html#profile-uses}.) 7659 */ 7660 protected List<StructureDefinition> profileTarget; 7661 7662 /** 7663 * A definition of the restful capabilities of the solution, if any. 7664 */ 7665 @Child(name = "rest", type = {}, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 7666 @Description(shortDefinition = "If the endpoint is a RESTful one", formalDefinition = "A definition of the restful capabilities of the solution, if any.") 7667 protected List<ConformanceRestComponent> rest; 7668 7669 /** 7670 * A description of the messaging capabilities of the solution. 7671 */ 7672 @Child(name = "messaging", type = {}, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7673 @Description(shortDefinition = "If messaging is supported", formalDefinition = "A description of the messaging capabilities of the solution.") 7674 protected List<ConformanceMessagingComponent> messaging; 7675 7676 /** 7677 * A document definition. 7678 */ 7679 @Child(name = "document", type = {}, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7680 @Description(shortDefinition = "Document definition", formalDefinition = "A document definition.") 7681 protected List<ConformanceDocumentComponent> document; 7682 7683 private static final long serialVersionUID = 1863739648L; 7684 7685 /* 7686 * Constructor 7687 */ 7688 public Conformance() { 7689 super(); 7690 } 7691 7692 /* 7693 * Constructor 7694 */ 7695 public Conformance(DateTimeType date, Enumeration<ConformanceStatementKind> kind, IdType fhirVersion, 7696 Enumeration<UnknownContentCode> acceptUnknown) { 7697 super(); 7698 this.date = date; 7699 this.kind = kind; 7700 this.fhirVersion = fhirVersion; 7701 this.acceptUnknown = acceptUnknown; 7702 } 7703 7704 /** 7705 * @return {@link #url} (An absolute URL that is used to identify this 7706 * conformance statement when it is referenced in a specification, 7707 * model, design or an instance. This SHALL be a URL, SHOULD be globally 7708 * unique, and SHOULD be an address at which this conformance statement 7709 * is (or will be) published.). This is the underlying object with id, 7710 * value and extensions. The accessor "getUrl" gives direct access to 7711 * the value 7712 */ 7713 public UriType getUrlElement() { 7714 if (this.url == null) 7715 if (Configuration.errorOnAutoCreate()) 7716 throw new Error("Attempt to auto-create Conformance.url"); 7717 else if (Configuration.doAutoCreate()) 7718 this.url = new UriType(); // bb 7719 return this.url; 7720 } 7721 7722 public boolean hasUrlElement() { 7723 return this.url != null && !this.url.isEmpty(); 7724 } 7725 7726 public boolean hasUrl() { 7727 return this.url != null && !this.url.isEmpty(); 7728 } 7729 7730 /** 7731 * @param value {@link #url} (An absolute URL that is used to identify this 7732 * conformance statement when it is referenced in a specification, 7733 * model, design or an instance. This SHALL be a URL, SHOULD be 7734 * globally unique, and SHOULD be an address at which this 7735 * conformance statement is (or will be) published.). This is the 7736 * underlying object with id, value and extensions. The accessor 7737 * "getUrl" gives direct access to the value 7738 */ 7739 public Conformance setUrlElement(UriType value) { 7740 this.url = value; 7741 return this; 7742 } 7743 7744 /** 7745 * @return An absolute URL that is used to identify this conformance statement 7746 * when it is referenced in a specification, model, design or an 7747 * instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD 7748 * be an address at which this conformance statement is (or will be) 7749 * published. 7750 */ 7751 public String getUrl() { 7752 return this.url == null ? null : this.url.getValue(); 7753 } 7754 7755 /** 7756 * @param value An absolute URL that is used to identify this conformance 7757 * statement when it is referenced in a specification, model, 7758 * design or an instance. This SHALL be a URL, SHOULD be globally 7759 * unique, and SHOULD be an address at which this conformance 7760 * statement is (or will be) published. 7761 */ 7762 public Conformance setUrl(String value) { 7763 if (Utilities.noString(value)) 7764 this.url = null; 7765 else { 7766 if (this.url == null) 7767 this.url = new UriType(); 7768 this.url.setValue(value); 7769 } 7770 return this; 7771 } 7772 7773 /** 7774 * @return {@link #version} (The identifier that is used to identify this 7775 * version of the conformance statement when it is referenced in a 7776 * specification, model, design or instance. This is an arbitrary value 7777 * managed by the profile author manually and the value should be a 7778 * timestamp.). This is the underlying object with id, value and 7779 * extensions. The accessor "getVersion" gives direct access to the 7780 * value 7781 */ 7782 public StringType getVersionElement() { 7783 if (this.version == null) 7784 if (Configuration.errorOnAutoCreate()) 7785 throw new Error("Attempt to auto-create Conformance.version"); 7786 else if (Configuration.doAutoCreate()) 7787 this.version = new StringType(); // bb 7788 return this.version; 7789 } 7790 7791 public boolean hasVersionElement() { 7792 return this.version != null && !this.version.isEmpty(); 7793 } 7794 7795 public boolean hasVersion() { 7796 return this.version != null && !this.version.isEmpty(); 7797 } 7798 7799 /** 7800 * @param value {@link #version} (The identifier that is used to identify this 7801 * version of the conformance statement when it is referenced in a 7802 * specification, model, design or instance. This is an arbitrary 7803 * value managed by the profile author manually and the value 7804 * should be a timestamp.). This is the underlying object with id, 7805 * value and extensions. The accessor "getVersion" gives direct 7806 * access to the value 7807 */ 7808 public Conformance setVersionElement(StringType value) { 7809 this.version = value; 7810 return this; 7811 } 7812 7813 /** 7814 * @return The identifier that is used to identify this version of the 7815 * conformance statement when it is referenced in a specification, 7816 * model, design or instance. This is an arbitrary value managed by the 7817 * profile author manually and the value should be a timestamp. 7818 */ 7819 public String getVersion() { 7820 return this.version == null ? null : this.version.getValue(); 7821 } 7822 7823 /** 7824 * @param value The identifier that is used to identify this version of the 7825 * conformance statement when it is referenced in a specification, 7826 * model, design or instance. This is an arbitrary value managed by 7827 * the profile author manually and the value should be a timestamp. 7828 */ 7829 public Conformance setVersion(String value) { 7830 if (Utilities.noString(value)) 7831 this.version = null; 7832 else { 7833 if (this.version == null) 7834 this.version = new StringType(); 7835 this.version.setValue(value); 7836 } 7837 return this; 7838 } 7839 7840 /** 7841 * @return {@link #name} (A free text natural language name identifying the 7842 * conformance statement.). This is the underlying object with id, value 7843 * and extensions. The accessor "getName" gives direct access to the 7844 * value 7845 */ 7846 public StringType getNameElement() { 7847 if (this.name == null) 7848 if (Configuration.errorOnAutoCreate()) 7849 throw new Error("Attempt to auto-create Conformance.name"); 7850 else if (Configuration.doAutoCreate()) 7851 this.name = new StringType(); // bb 7852 return this.name; 7853 } 7854 7855 public boolean hasNameElement() { 7856 return this.name != null && !this.name.isEmpty(); 7857 } 7858 7859 public boolean hasName() { 7860 return this.name != null && !this.name.isEmpty(); 7861 } 7862 7863 /** 7864 * @param value {@link #name} (A free text natural language name identifying the 7865 * conformance statement.). This is the underlying object with id, 7866 * value and extensions. The accessor "getName" gives direct access 7867 * to the value 7868 */ 7869 public Conformance setNameElement(StringType value) { 7870 this.name = value; 7871 return this; 7872 } 7873 7874 /** 7875 * @return A free text natural language name identifying the conformance 7876 * statement. 7877 */ 7878 public String getName() { 7879 return this.name == null ? null : this.name.getValue(); 7880 } 7881 7882 /** 7883 * @param value A free text natural language name identifying the conformance 7884 * statement. 7885 */ 7886 public Conformance setName(String value) { 7887 if (Utilities.noString(value)) 7888 this.name = null; 7889 else { 7890 if (this.name == null) 7891 this.name = new StringType(); 7892 this.name.setValue(value); 7893 } 7894 return this; 7895 } 7896 7897 /** 7898 * @return {@link #status} (The status of this conformance statement.). This is 7899 * the underlying object with id, value and extensions. The accessor 7900 * "getStatus" gives direct access to the value 7901 */ 7902 public Enumeration<ConformanceResourceStatus> getStatusElement() { 7903 if (this.status == null) 7904 if (Configuration.errorOnAutoCreate()) 7905 throw new Error("Attempt to auto-create Conformance.status"); 7906 else if (Configuration.doAutoCreate()) 7907 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); // bb 7908 return this.status; 7909 } 7910 7911 public boolean hasStatusElement() { 7912 return this.status != null && !this.status.isEmpty(); 7913 } 7914 7915 public boolean hasStatus() { 7916 return this.status != null && !this.status.isEmpty(); 7917 } 7918 7919 /** 7920 * @param value {@link #status} (The status of this conformance statement.). 7921 * This is the underlying object with id, value and extensions. The 7922 * accessor "getStatus" gives direct access to the value 7923 */ 7924 public Conformance setStatusElement(Enumeration<ConformanceResourceStatus> value) { 7925 this.status = value; 7926 return this; 7927 } 7928 7929 /** 7930 * @return The status of this conformance statement. 7931 */ 7932 public ConformanceResourceStatus getStatus() { 7933 return this.status == null ? null : this.status.getValue(); 7934 } 7935 7936 /** 7937 * @param value The status of this conformance statement. 7938 */ 7939 public Conformance setStatus(ConformanceResourceStatus value) { 7940 if (value == null) 7941 this.status = null; 7942 else { 7943 if (this.status == null) 7944 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); 7945 this.status.setValue(value); 7946 } 7947 return this; 7948 } 7949 7950 /** 7951 * @return {@link #experimental} (A flag to indicate that this conformance 7952 * statement is authored for testing purposes (or 7953 * education/evaluation/marketing), and is not intended to be used for 7954 * genuine usage.). This is the underlying object with id, value and 7955 * extensions. The accessor "getExperimental" gives direct access to the 7956 * value 7957 */ 7958 public BooleanType getExperimentalElement() { 7959 if (this.experimental == null) 7960 if (Configuration.errorOnAutoCreate()) 7961 throw new Error("Attempt to auto-create Conformance.experimental"); 7962 else if (Configuration.doAutoCreate()) 7963 this.experimental = new BooleanType(); // bb 7964 return this.experimental; 7965 } 7966 7967 public boolean hasExperimentalElement() { 7968 return this.experimental != null && !this.experimental.isEmpty(); 7969 } 7970 7971 public boolean hasExperimental() { 7972 return this.experimental != null && !this.experimental.isEmpty(); 7973 } 7974 7975 /** 7976 * @param value {@link #experimental} (A flag to indicate that this conformance 7977 * statement is authored for testing purposes (or 7978 * education/evaluation/marketing), and is not intended to be used 7979 * for genuine usage.). This is the underlying object with id, 7980 * value and extensions. The accessor "getExperimental" gives 7981 * direct access to the value 7982 */ 7983 public Conformance setExperimentalElement(BooleanType value) { 7984 this.experimental = value; 7985 return this; 7986 } 7987 7988 /** 7989 * @return A flag to indicate that this conformance statement is authored for 7990 * testing purposes (or education/evaluation/marketing), and is not 7991 * intended to be used for genuine usage. 7992 */ 7993 public boolean getExperimental() { 7994 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 7995 } 7996 7997 /** 7998 * @param value A flag to indicate that this conformance statement is authored 7999 * for testing purposes (or education/evaluation/marketing), and is 8000 * not intended to be used for genuine usage. 8001 */ 8002 public Conformance setExperimental(boolean value) { 8003 if (this.experimental == null) 8004 this.experimental = new BooleanType(); 8005 this.experimental.setValue(value); 8006 return this; 8007 } 8008 8009 /** 8010 * @return {@link #publisher} (The name of the individual or organization that 8011 * published the conformance.). This is the underlying object with id, 8012 * value and extensions. The accessor "getPublisher" gives direct access 8013 * to the value 8014 */ 8015 public StringType getPublisherElement() { 8016 if (this.publisher == null) 8017 if (Configuration.errorOnAutoCreate()) 8018 throw new Error("Attempt to auto-create Conformance.publisher"); 8019 else if (Configuration.doAutoCreate()) 8020 this.publisher = new StringType(); // bb 8021 return this.publisher; 8022 } 8023 8024 public boolean hasPublisherElement() { 8025 return this.publisher != null && !this.publisher.isEmpty(); 8026 } 8027 8028 public boolean hasPublisher() { 8029 return this.publisher != null && !this.publisher.isEmpty(); 8030 } 8031 8032 /** 8033 * @param value {@link #publisher} (The name of the individual or organization 8034 * that published the conformance.). This is the underlying object 8035 * with id, value and extensions. The accessor "getPublisher" gives 8036 * direct access to the value 8037 */ 8038 public Conformance setPublisherElement(StringType value) { 8039 this.publisher = value; 8040 return this; 8041 } 8042 8043 /** 8044 * @return The name of the individual or organization that published the 8045 * conformance. 8046 */ 8047 public String getPublisher() { 8048 return this.publisher == null ? null : this.publisher.getValue(); 8049 } 8050 8051 /** 8052 * @param value The name of the individual or organization that published the 8053 * conformance. 8054 */ 8055 public Conformance setPublisher(String value) { 8056 if (Utilities.noString(value)) 8057 this.publisher = null; 8058 else { 8059 if (this.publisher == null) 8060 this.publisher = new StringType(); 8061 this.publisher.setValue(value); 8062 } 8063 return this; 8064 } 8065 8066 /** 8067 * @return {@link #contact} (Contacts to assist a user in finding and 8068 * communicating with the publisher.) 8069 */ 8070 public List<ConformanceContactComponent> getContact() { 8071 if (this.contact == null) 8072 this.contact = new ArrayList<ConformanceContactComponent>(); 8073 return this.contact; 8074 } 8075 8076 public boolean hasContact() { 8077 if (this.contact == null) 8078 return false; 8079 for (ConformanceContactComponent item : this.contact) 8080 if (!item.isEmpty()) 8081 return true; 8082 return false; 8083 } 8084 8085 /** 8086 * @return {@link #contact} (Contacts to assist a user in finding and 8087 * communicating with the publisher.) 8088 */ 8089 // syntactic sugar 8090 public ConformanceContactComponent addContact() { // 3 8091 ConformanceContactComponent t = new ConformanceContactComponent(); 8092 if (this.contact == null) 8093 this.contact = new ArrayList<ConformanceContactComponent>(); 8094 this.contact.add(t); 8095 return t; 8096 } 8097 8098 // syntactic sugar 8099 public Conformance addContact(ConformanceContactComponent t) { // 3 8100 if (t == null) 8101 return this; 8102 if (this.contact == null) 8103 this.contact = new ArrayList<ConformanceContactComponent>(); 8104 this.contact.add(t); 8105 return this; 8106 } 8107 8108 /** 8109 * @return {@link #date} (The date (and optionally time) when the conformance 8110 * statement was published. The date must change when the business 8111 * version changes, if it does, and it must change if the status code 8112 * changes. In addition, it should change when the substantive content 8113 * of the conformance statement changes.). This is the underlying object 8114 * with id, value and extensions. The accessor "getDate" gives direct 8115 * access to the value 8116 */ 8117 public DateTimeType getDateElement() { 8118 if (this.date == null) 8119 if (Configuration.errorOnAutoCreate()) 8120 throw new Error("Attempt to auto-create Conformance.date"); 8121 else if (Configuration.doAutoCreate()) 8122 this.date = new DateTimeType(); // bb 8123 return this.date; 8124 } 8125 8126 public boolean hasDateElement() { 8127 return this.date != null && !this.date.isEmpty(); 8128 } 8129 8130 public boolean hasDate() { 8131 return this.date != null && !this.date.isEmpty(); 8132 } 8133 8134 /** 8135 * @param value {@link #date} (The date (and optionally time) when the 8136 * conformance statement was published. The date must change when 8137 * the business version changes, if it does, and it must change if 8138 * the status code changes. In addition, it should change when the 8139 * substantive content of the conformance statement changes.). This 8140 * is the underlying object with id, value and extensions. The 8141 * accessor "getDate" gives direct access to the value 8142 */ 8143 public Conformance setDateElement(DateTimeType value) { 8144 this.date = value; 8145 return this; 8146 } 8147 8148 /** 8149 * @return The date (and optionally time) when the conformance statement was 8150 * published. The date must change when the business version changes, if 8151 * it does, and it must change if the status code changes. In addition, 8152 * it should change when the substantive content of the conformance 8153 * statement changes. 8154 */ 8155 public Date getDate() { 8156 return this.date == null ? null : this.date.getValue(); 8157 } 8158 8159 /** 8160 * @param value The date (and optionally time) when the conformance statement 8161 * was published. The date must change when the business version 8162 * changes, if it does, and it must change if the status code 8163 * changes. In addition, it should change when the substantive 8164 * content of the conformance statement changes. 8165 */ 8166 public Conformance setDate(Date value) { 8167 if (this.date == null) 8168 this.date = new DateTimeType(); 8169 this.date.setValue(value); 8170 return this; 8171 } 8172 8173 /** 8174 * @return {@link #description} (A free text natural language description of the 8175 * conformance statement and its use. Typically, this is used when the 8176 * conformance statement describes a desired rather than an actual 8177 * solution, for example as a formal expression of requirements as part 8178 * of an RFP.). This is the underlying object with id, value and 8179 * extensions. The accessor "getDescription" gives direct access to the 8180 * value 8181 */ 8182 public StringType getDescriptionElement() { 8183 if (this.description == null) 8184 if (Configuration.errorOnAutoCreate()) 8185 throw new Error("Attempt to auto-create Conformance.description"); 8186 else if (Configuration.doAutoCreate()) 8187 this.description = new StringType(); // bb 8188 return this.description; 8189 } 8190 8191 public boolean hasDescriptionElement() { 8192 return this.description != null && !this.description.isEmpty(); 8193 } 8194 8195 public boolean hasDescription() { 8196 return this.description != null && !this.description.isEmpty(); 8197 } 8198 8199 /** 8200 * @param value {@link #description} (A free text natural language description 8201 * of the conformance statement and its use. Typically, this is 8202 * used when the conformance statement describes a desired rather 8203 * than an actual solution, for example as a formal expression of 8204 * requirements as part of an RFP.). This is the underlying object 8205 * with id, value and extensions. The accessor "getDescription" 8206 * gives direct access to the value 8207 */ 8208 public Conformance setDescriptionElement(StringType value) { 8209 this.description = value; 8210 return this; 8211 } 8212 8213 /** 8214 * @return A free text natural language description of the conformance statement 8215 * and its use. Typically, this is used when the conformance statement 8216 * describes a desired rather than an actual solution, for example as a 8217 * formal expression of requirements as part of an RFP. 8218 */ 8219 public String getDescription() { 8220 return this.description == null ? null : this.description.getValue(); 8221 } 8222 8223 /** 8224 * @param value A free text natural language description of the conformance 8225 * statement and its use. Typically, this is used when the 8226 * conformance statement describes a desired rather than an actual 8227 * solution, for example as a formal expression of requirements as 8228 * part of an RFP. 8229 */ 8230 public Conformance setDescription(String value) { 8231 if (Utilities.noString(value)) 8232 this.description = null; 8233 else { 8234 if (this.description == null) 8235 this.description = new StringType(); 8236 this.description.setValue(value); 8237 } 8238 return this; 8239 } 8240 8241 /** 8242 * @return {@link #requirements} (Explains why this conformance statement is 8243 * needed and why it's been constrained as it has.). This is the 8244 * underlying object with id, value and extensions. The accessor 8245 * "getRequirements" gives direct access to the value 8246 */ 8247 public StringType getRequirementsElement() { 8248 if (this.requirements == null) 8249 if (Configuration.errorOnAutoCreate()) 8250 throw new Error("Attempt to auto-create Conformance.requirements"); 8251 else if (Configuration.doAutoCreate()) 8252 this.requirements = new StringType(); // bb 8253 return this.requirements; 8254 } 8255 8256 public boolean hasRequirementsElement() { 8257 return this.requirements != null && !this.requirements.isEmpty(); 8258 } 8259 8260 public boolean hasRequirements() { 8261 return this.requirements != null && !this.requirements.isEmpty(); 8262 } 8263 8264 /** 8265 * @param value {@link #requirements} (Explains why this conformance statement 8266 * is needed and why it's been constrained as it has.). This is the 8267 * underlying object with id, value and extensions. The accessor 8268 * "getRequirements" gives direct access to the value 8269 */ 8270 public Conformance setRequirementsElement(StringType value) { 8271 this.requirements = value; 8272 return this; 8273 } 8274 8275 /** 8276 * @return Explains why this conformance statement is needed and why it's been 8277 * constrained as it has. 8278 */ 8279 public String getRequirements() { 8280 return this.requirements == null ? null : this.requirements.getValue(); 8281 } 8282 8283 /** 8284 * @param value Explains why this conformance statement is needed and why it's 8285 * been constrained as it has. 8286 */ 8287 public Conformance setRequirements(String value) { 8288 if (Utilities.noString(value)) 8289 this.requirements = null; 8290 else { 8291 if (this.requirements == null) 8292 this.requirements = new StringType(); 8293 this.requirements.setValue(value); 8294 } 8295 return this; 8296 } 8297 8298 /** 8299 * @return {@link #copyright} (A copyright statement relating to the conformance 8300 * statement and/or its contents. Copyright statements are generally 8301 * legal restrictions on the use and publishing of the details of the 8302 * system described by the conformance statement.). This is the 8303 * underlying object with id, value and extensions. The accessor 8304 * "getCopyright" gives direct access to the value 8305 */ 8306 public StringType getCopyrightElement() { 8307 if (this.copyright == null) 8308 if (Configuration.errorOnAutoCreate()) 8309 throw new Error("Attempt to auto-create Conformance.copyright"); 8310 else if (Configuration.doAutoCreate()) 8311 this.copyright = new StringType(); // bb 8312 return this.copyright; 8313 } 8314 8315 public boolean hasCopyrightElement() { 8316 return this.copyright != null && !this.copyright.isEmpty(); 8317 } 8318 8319 public boolean hasCopyright() { 8320 return this.copyright != null && !this.copyright.isEmpty(); 8321 } 8322 8323 /** 8324 * @param value {@link #copyright} (A copyright statement relating to the 8325 * conformance statement and/or its contents. Copyright statements 8326 * are generally legal restrictions on the use and publishing of 8327 * the details of the system described by the conformance 8328 * statement.). This is the underlying object with id, value and 8329 * extensions. The accessor "getCopyright" gives direct access to 8330 * the value 8331 */ 8332 public Conformance setCopyrightElement(StringType value) { 8333 this.copyright = value; 8334 return this; 8335 } 8336 8337 /** 8338 * @return A copyright statement relating to the conformance statement and/or 8339 * its contents. Copyright statements are generally legal restrictions 8340 * on the use and publishing of the details of the system described by 8341 * the conformance statement. 8342 */ 8343 public String getCopyright() { 8344 return this.copyright == null ? null : this.copyright.getValue(); 8345 } 8346 8347 /** 8348 * @param value A copyright statement relating to the conformance statement 8349 * and/or its contents. Copyright statements are generally legal 8350 * restrictions on the use and publishing of the details of the 8351 * system described by the conformance statement. 8352 */ 8353 public Conformance setCopyright(String value) { 8354 if (Utilities.noString(value)) 8355 this.copyright = null; 8356 else { 8357 if (this.copyright == null) 8358 this.copyright = new StringType(); 8359 this.copyright.setValue(value); 8360 } 8361 return this; 8362 } 8363 8364 /** 8365 * @return {@link #kind} (The way that this statement is intended to be used, to 8366 * describe an actual running instance of software, a particular product 8367 * (kind not instance of software) or a class of implementation (e.g. a 8368 * desired purchase).). This is the underlying object with id, value and 8369 * extensions. The accessor "getKind" gives direct access to the value 8370 */ 8371 public Enumeration<ConformanceStatementKind> getKindElement() { 8372 if (this.kind == null) 8373 if (Configuration.errorOnAutoCreate()) 8374 throw new Error("Attempt to auto-create Conformance.kind"); 8375 else if (Configuration.doAutoCreate()) 8376 this.kind = new Enumeration<ConformanceStatementKind>(new ConformanceStatementKindEnumFactory()); // bb 8377 return this.kind; 8378 } 8379 8380 public boolean hasKindElement() { 8381 return this.kind != null && !this.kind.isEmpty(); 8382 } 8383 8384 public boolean hasKind() { 8385 return this.kind != null && !this.kind.isEmpty(); 8386 } 8387 8388 /** 8389 * @param value {@link #kind} (The way that this statement is intended to be 8390 * used, to describe an actual running instance of software, a 8391 * particular product (kind not instance of software) or a class of 8392 * implementation (e.g. a desired purchase).). This is the 8393 * underlying object with id, value and extensions. The accessor 8394 * "getKind" gives direct access to the value 8395 */ 8396 public Conformance setKindElement(Enumeration<ConformanceStatementKind> value) { 8397 this.kind = value; 8398 return this; 8399 } 8400 8401 /** 8402 * @return The way that this statement is intended to be used, to describe an 8403 * actual running instance of software, a particular product (kind not 8404 * instance of software) or a class of implementation (e.g. a desired 8405 * purchase). 8406 */ 8407 public ConformanceStatementKind getKind() { 8408 return this.kind == null ? null : this.kind.getValue(); 8409 } 8410 8411 /** 8412 * @param value The way that this statement is intended to be used, to describe 8413 * an actual running instance of software, a particular product 8414 * (kind not instance of software) or a class of implementation 8415 * (e.g. a desired purchase). 8416 */ 8417 public Conformance setKind(ConformanceStatementKind value) { 8418 if (this.kind == null) 8419 this.kind = new Enumeration<ConformanceStatementKind>(new ConformanceStatementKindEnumFactory()); 8420 this.kind.setValue(value); 8421 return this; 8422 } 8423 8424 /** 8425 * @return {@link #software} (Software that is covered by this conformance 8426 * statement. It is used when the conformance statement describes the 8427 * capabilities of a particular software version, independent of an 8428 * installation.) 8429 */ 8430 public ConformanceSoftwareComponent getSoftware() { 8431 if (this.software == null) 8432 if (Configuration.errorOnAutoCreate()) 8433 throw new Error("Attempt to auto-create Conformance.software"); 8434 else if (Configuration.doAutoCreate()) 8435 this.software = new ConformanceSoftwareComponent(); // cc 8436 return this.software; 8437 } 8438 8439 public boolean hasSoftware() { 8440 return this.software != null && !this.software.isEmpty(); 8441 } 8442 8443 /** 8444 * @param value {@link #software} (Software that is covered by this conformance 8445 * statement. It is used when the conformance statement describes 8446 * the capabilities of a particular software version, independent 8447 * of an installation.) 8448 */ 8449 public Conformance setSoftware(ConformanceSoftwareComponent value) { 8450 this.software = value; 8451 return this; 8452 } 8453 8454 /** 8455 * @return {@link #implementation} (Identifies a specific implementation 8456 * instance that is described by the conformance statement - i.e. a 8457 * particular installation, rather than the capabilities of a software 8458 * program.) 8459 */ 8460 public ConformanceImplementationComponent getImplementation() { 8461 if (this.implementation == null) 8462 if (Configuration.errorOnAutoCreate()) 8463 throw new Error("Attempt to auto-create Conformance.implementation"); 8464 else if (Configuration.doAutoCreate()) 8465 this.implementation = new ConformanceImplementationComponent(); // cc 8466 return this.implementation; 8467 } 8468 8469 public boolean hasImplementation() { 8470 return this.implementation != null && !this.implementation.isEmpty(); 8471 } 8472 8473 /** 8474 * @param value {@link #implementation} (Identifies a specific implementation 8475 * instance that is described by the conformance statement - i.e. a 8476 * particular installation, rather than the capabilities of a 8477 * software program.) 8478 */ 8479 public Conformance setImplementation(ConformanceImplementationComponent value) { 8480 this.implementation = value; 8481 return this; 8482 } 8483 8484 /** 8485 * @return {@link #fhirVersion} (The version of the FHIR specification on which 8486 * this conformance statement is based.). This is the underlying object 8487 * with id, value and extensions. The accessor "getFhirVersion" gives 8488 * direct access to the value 8489 */ 8490 public IdType getFhirVersionElement() { 8491 if (this.fhirVersion == null) 8492 if (Configuration.errorOnAutoCreate()) 8493 throw new Error("Attempt to auto-create Conformance.fhirVersion"); 8494 else if (Configuration.doAutoCreate()) 8495 this.fhirVersion = new IdType(); // bb 8496 return this.fhirVersion; 8497 } 8498 8499 public boolean hasFhirVersionElement() { 8500 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 8501 } 8502 8503 public boolean hasFhirVersion() { 8504 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 8505 } 8506 8507 /** 8508 * @param value {@link #fhirVersion} (The version of the FHIR specification on 8509 * which this conformance statement is based.). This is the 8510 * underlying object with id, value and extensions. The accessor 8511 * "getFhirVersion" gives direct access to the value 8512 */ 8513 public Conformance setFhirVersionElement(IdType value) { 8514 this.fhirVersion = value; 8515 return this; 8516 } 8517 8518 /** 8519 * @return The version of the FHIR specification on which this conformance 8520 * statement is based. 8521 */ 8522 public String getFhirVersion() { 8523 return this.fhirVersion == null ? null : this.fhirVersion.getValue(); 8524 } 8525 8526 /** 8527 * @param value The version of the FHIR specification on which this conformance 8528 * statement is based. 8529 */ 8530 public Conformance setFhirVersion(String value) { 8531 if (this.fhirVersion == null) 8532 this.fhirVersion = new IdType(); 8533 this.fhirVersion.setValue(value); 8534 return this; 8535 } 8536 8537 /** 8538 * @return {@link #acceptUnknown} (A code that indicates whether the application 8539 * accepts unknown elements or extensions when reading resources.). This 8540 * is the underlying object with id, value and extensions. The accessor 8541 * "getAcceptUnknown" gives direct access to the value 8542 */ 8543 public Enumeration<UnknownContentCode> getAcceptUnknownElement() { 8544 if (this.acceptUnknown == null) 8545 if (Configuration.errorOnAutoCreate()) 8546 throw new Error("Attempt to auto-create Conformance.acceptUnknown"); 8547 else if (Configuration.doAutoCreate()) 8548 this.acceptUnknown = new Enumeration<UnknownContentCode>(new UnknownContentCodeEnumFactory()); // bb 8549 return this.acceptUnknown; 8550 } 8551 8552 public boolean hasAcceptUnknownElement() { 8553 return this.acceptUnknown != null && !this.acceptUnknown.isEmpty(); 8554 } 8555 8556 public boolean hasAcceptUnknown() { 8557 return this.acceptUnknown != null && !this.acceptUnknown.isEmpty(); 8558 } 8559 8560 /** 8561 * @param value {@link #acceptUnknown} (A code that indicates whether the 8562 * application accepts unknown elements or extensions when reading 8563 * resources.). This is the underlying object with id, value and 8564 * extensions. The accessor "getAcceptUnknown" gives direct access 8565 * to the value 8566 */ 8567 public Conformance setAcceptUnknownElement(Enumeration<UnknownContentCode> value) { 8568 this.acceptUnknown = value; 8569 return this; 8570 } 8571 8572 /** 8573 * @return A code that indicates whether the application accepts unknown 8574 * elements or extensions when reading resources. 8575 */ 8576 public UnknownContentCode getAcceptUnknown() { 8577 return this.acceptUnknown == null ? null : this.acceptUnknown.getValue(); 8578 } 8579 8580 /** 8581 * @param value A code that indicates whether the application accepts unknown 8582 * elements or extensions when reading resources. 8583 */ 8584 public Conformance setAcceptUnknown(UnknownContentCode value) { 8585 if (this.acceptUnknown == null) 8586 this.acceptUnknown = new Enumeration<UnknownContentCode>(new UnknownContentCodeEnumFactory()); 8587 this.acceptUnknown.setValue(value); 8588 return this; 8589 } 8590 8591 /** 8592 * @return {@link #format} (A list of the formats supported by this 8593 * implementation using their content types.) 8594 */ 8595 public List<CodeType> getFormat() { 8596 if (this.format == null) 8597 this.format = new ArrayList<CodeType>(); 8598 return this.format; 8599 } 8600 8601 public boolean hasFormat() { 8602 if (this.format == null) 8603 return false; 8604 for (CodeType item : this.format) 8605 if (!item.isEmpty()) 8606 return true; 8607 return false; 8608 } 8609 8610 /** 8611 * @return {@link #format} (A list of the formats supported by this 8612 * implementation using their content types.) 8613 */ 8614 // syntactic sugar 8615 public CodeType addFormatElement() {// 2 8616 CodeType t = new CodeType(); 8617 if (this.format == null) 8618 this.format = new ArrayList<CodeType>(); 8619 this.format.add(t); 8620 return t; 8621 } 8622 8623 /** 8624 * @param value {@link #format} (A list of the formats supported by this 8625 * implementation using their content types.) 8626 */ 8627 public Conformance addFormat(String value) { // 1 8628 CodeType t = new CodeType(); 8629 t.setValue(value); 8630 if (this.format == null) 8631 this.format = new ArrayList<CodeType>(); 8632 this.format.add(t); 8633 return this; 8634 } 8635 8636 /** 8637 * @param value {@link #format} (A list of the formats supported by this 8638 * implementation using their content types.) 8639 */ 8640 public boolean hasFormat(String value) { 8641 if (this.format == null) 8642 return false; 8643 for (CodeType v : this.format) 8644 if (v.equals(value)) // code 8645 return true; 8646 return false; 8647 } 8648 8649 /** 8650 * @return {@link #profile} (A list of profiles that represent different use 8651 * cases supported by the system. For a server, "supported by the 8652 * system" means the system hosts/produces a set of resources that are 8653 * conformant to a particular profile, and allows clients that use its 8654 * services to search using this profile and to find appropriate data. 8655 * For a client, it means the system will search by this profile and 8656 * process data according to the guidance implicit in the profile. See 8657 * further discussion in [Using Profiles]{profiling.html#profile-uses}.) 8658 */ 8659 public List<Reference> getProfile() { 8660 if (this.profile == null) 8661 this.profile = new ArrayList<Reference>(); 8662 return this.profile; 8663 } 8664 8665 public boolean hasProfile() { 8666 if (this.profile == null) 8667 return false; 8668 for (Reference item : this.profile) 8669 if (!item.isEmpty()) 8670 return true; 8671 return false; 8672 } 8673 8674 /** 8675 * @return {@link #profile} (A list of profiles that represent different use 8676 * cases supported by the system. For a server, "supported by the 8677 * system" means the system hosts/produces a set of resources that are 8678 * conformant to a particular profile, and allows clients that use its 8679 * services to search using this profile and to find appropriate data. 8680 * For a client, it means the system will search by this profile and 8681 * process data according to the guidance implicit in the profile. See 8682 * further discussion in [Using Profiles]{profiling.html#profile-uses}.) 8683 */ 8684 // syntactic sugar 8685 public Reference addProfile() { // 3 8686 Reference t = new Reference(); 8687 if (this.profile == null) 8688 this.profile = new ArrayList<Reference>(); 8689 this.profile.add(t); 8690 return t; 8691 } 8692 8693 // syntactic sugar 8694 public Conformance addProfile(Reference t) { // 3 8695 if (t == null) 8696 return this; 8697 if (this.profile == null) 8698 this.profile = new ArrayList<Reference>(); 8699 this.profile.add(t); 8700 return this; 8701 } 8702 8703 /** 8704 * @return {@link #profile} (The actual objects that are the target of the 8705 * reference. The reference library doesn't populate this, but you can 8706 * use this to hold the resources if you resolvethemt. A list of 8707 * profiles that represent different use cases supported by the system. 8708 * For a server, "supported by the system" means the system 8709 * hosts/produces a set of resources that are conformant to a particular 8710 * profile, and allows clients that use its services to search using 8711 * this profile and to find appropriate data. For a client, it means the 8712 * system will search by this profile and process data according to the 8713 * guidance implicit in the profile. See further discussion in [Using 8714 * Profiles]{profiling.html#profile-uses}.) 8715 */ 8716 public List<StructureDefinition> getProfileTarget() { 8717 if (this.profileTarget == null) 8718 this.profileTarget = new ArrayList<StructureDefinition>(); 8719 return this.profileTarget; 8720 } 8721 8722 // syntactic sugar 8723 /** 8724 * @return {@link #profile} (Add an actual object that is the target of the 8725 * reference. The reference library doesn't use these, but you can use 8726 * this to hold the resources if you resolvethemt. A list of profiles 8727 * that represent different use cases supported by the system. For a 8728 * server, "supported by the system" means the system hosts/produces a 8729 * set of resources that are conformant to a particular profile, and 8730 * allows clients that use its services to search using this profile and 8731 * to find appropriate data. For a client, it means the system will 8732 * search by this profile and process data according to the guidance 8733 * implicit in the profile. See further discussion in [Using 8734 * Profiles]{profiling.html#profile-uses}.) 8735 */ 8736 public StructureDefinition addProfileTarget() { 8737 StructureDefinition r = new StructureDefinition(); 8738 if (this.profileTarget == null) 8739 this.profileTarget = new ArrayList<StructureDefinition>(); 8740 this.profileTarget.add(r); 8741 return r; 8742 } 8743 8744 /** 8745 * @return {@link #rest} (A definition of the restful capabilities of the 8746 * solution, if any.) 8747 */ 8748 public List<ConformanceRestComponent> getRest() { 8749 if (this.rest == null) 8750 this.rest = new ArrayList<ConformanceRestComponent>(); 8751 return this.rest; 8752 } 8753 8754 public boolean hasRest() { 8755 if (this.rest == null) 8756 return false; 8757 for (ConformanceRestComponent item : this.rest) 8758 if (!item.isEmpty()) 8759 return true; 8760 return false; 8761 } 8762 8763 /** 8764 * @return {@link #rest} (A definition of the restful capabilities of the 8765 * solution, if any.) 8766 */ 8767 // syntactic sugar 8768 public ConformanceRestComponent addRest() { // 3 8769 ConformanceRestComponent t = new ConformanceRestComponent(); 8770 if (this.rest == null) 8771 this.rest = new ArrayList<ConformanceRestComponent>(); 8772 this.rest.add(t); 8773 return t; 8774 } 8775 8776 // syntactic sugar 8777 public Conformance addRest(ConformanceRestComponent t) { // 3 8778 if (t == null) 8779 return this; 8780 if (this.rest == null) 8781 this.rest = new ArrayList<ConformanceRestComponent>(); 8782 this.rest.add(t); 8783 return this; 8784 } 8785 8786 /** 8787 * @return {@link #messaging} (A description of the messaging capabilities of 8788 * the solution.) 8789 */ 8790 public List<ConformanceMessagingComponent> getMessaging() { 8791 if (this.messaging == null) 8792 this.messaging = new ArrayList<ConformanceMessagingComponent>(); 8793 return this.messaging; 8794 } 8795 8796 public boolean hasMessaging() { 8797 if (this.messaging == null) 8798 return false; 8799 for (ConformanceMessagingComponent item : this.messaging) 8800 if (!item.isEmpty()) 8801 return true; 8802 return false; 8803 } 8804 8805 /** 8806 * @return {@link #messaging} (A description of the messaging capabilities of 8807 * the solution.) 8808 */ 8809 // syntactic sugar 8810 public ConformanceMessagingComponent addMessaging() { // 3 8811 ConformanceMessagingComponent t = new ConformanceMessagingComponent(); 8812 if (this.messaging == null) 8813 this.messaging = new ArrayList<ConformanceMessagingComponent>(); 8814 this.messaging.add(t); 8815 return t; 8816 } 8817 8818 // syntactic sugar 8819 public Conformance addMessaging(ConformanceMessagingComponent t) { // 3 8820 if (t == null) 8821 return this; 8822 if (this.messaging == null) 8823 this.messaging = new ArrayList<ConformanceMessagingComponent>(); 8824 this.messaging.add(t); 8825 return this; 8826 } 8827 8828 /** 8829 * @return {@link #document} (A document definition.) 8830 */ 8831 public List<ConformanceDocumentComponent> getDocument() { 8832 if (this.document == null) 8833 this.document = new ArrayList<ConformanceDocumentComponent>(); 8834 return this.document; 8835 } 8836 8837 public boolean hasDocument() { 8838 if (this.document == null) 8839 return false; 8840 for (ConformanceDocumentComponent item : this.document) 8841 if (!item.isEmpty()) 8842 return true; 8843 return false; 8844 } 8845 8846 /** 8847 * @return {@link #document} (A document definition.) 8848 */ 8849 // syntactic sugar 8850 public ConformanceDocumentComponent addDocument() { // 3 8851 ConformanceDocumentComponent t = new ConformanceDocumentComponent(); 8852 if (this.document == null) 8853 this.document = new ArrayList<ConformanceDocumentComponent>(); 8854 this.document.add(t); 8855 return t; 8856 } 8857 8858 // syntactic sugar 8859 public Conformance addDocument(ConformanceDocumentComponent t) { // 3 8860 if (t == null) 8861 return this; 8862 if (this.document == null) 8863 this.document = new ArrayList<ConformanceDocumentComponent>(); 8864 this.document.add(t); 8865 return this; 8866 } 8867 8868 protected void listChildren(List<Property> childrenList) { 8869 super.listChildren(childrenList); 8870 childrenList.add(new Property("url", "uri", 8871 "An absolute URL that is used to identify this conformance statement when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this conformance statement is (or will be) published.", 8872 0, java.lang.Integer.MAX_VALUE, url)); 8873 childrenList.add(new Property("version", "string", 8874 "The identifier that is used to identify this version of the conformance statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the profile author manually and the value should be a timestamp.", 8875 0, java.lang.Integer.MAX_VALUE, version)); 8876 childrenList 8877 .add(new Property("name", "string", "A free text natural language name identifying the conformance statement.", 8878 0, java.lang.Integer.MAX_VALUE, name)); 8879 childrenList.add(new Property("status", "code", "The status of this conformance statement.", 0, 8880 java.lang.Integer.MAX_VALUE, status)); 8881 childrenList.add(new Property("experimental", "boolean", 8882 "A flag to indicate that this conformance statement is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 8883 0, java.lang.Integer.MAX_VALUE, experimental)); 8884 childrenList.add(new Property("publisher", "string", 8885 "The name of the individual or organization that published the conformance.", 0, java.lang.Integer.MAX_VALUE, 8886 publisher)); 8887 childrenList 8888 .add(new Property("contact", "", "Contacts to assist a user in finding and communicating with the publisher.", 8889 0, java.lang.Integer.MAX_VALUE, contact)); 8890 childrenList.add(new Property("date", "dateTime", 8891 "The date (and optionally time) when the conformance statement was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the conformance statement changes.", 8892 0, java.lang.Integer.MAX_VALUE, date)); 8893 childrenList.add(new Property("description", "string", 8894 "A free text natural language description of the conformance statement and its use. Typically, this is used when the conformance statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.", 8895 0, java.lang.Integer.MAX_VALUE, description)); 8896 childrenList.add(new Property("requirements", "string", 8897 "Explains why this conformance statement is needed and why it's been constrained as it has.", 0, 8898 java.lang.Integer.MAX_VALUE, requirements)); 8899 childrenList.add(new Property("copyright", "string", 8900 "A copyright statement relating to the conformance statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the details of the system described by the conformance statement.", 8901 0, java.lang.Integer.MAX_VALUE, copyright)); 8902 childrenList.add(new Property("kind", "code", 8903 "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind not instance of software) or a class of implementation (e.g. a desired purchase).", 8904 0, java.lang.Integer.MAX_VALUE, kind)); 8905 childrenList.add(new Property("software", "", 8906 "Software that is covered by this conformance statement. It is used when the conformance statement describes the capabilities of a particular software version, independent of an installation.", 8907 0, java.lang.Integer.MAX_VALUE, software)); 8908 childrenList.add(new Property("implementation", "", 8909 "Identifies a specific implementation instance that is described by the conformance statement - i.e. a particular installation, rather than the capabilities of a software program.", 8910 0, java.lang.Integer.MAX_VALUE, implementation)); 8911 childrenList.add(new Property("fhirVersion", "id", 8912 "The version of the FHIR specification on which this conformance statement is based.", 0, 8913 java.lang.Integer.MAX_VALUE, fhirVersion)); 8914 childrenList.add(new Property("acceptUnknown", "code", 8915 "A code that indicates whether the application accepts unknown elements or extensions when reading resources.", 8916 0, java.lang.Integer.MAX_VALUE, acceptUnknown)); 8917 childrenList.add(new Property("format", "code", 8918 "A list of the formats supported by this implementation using their content types.", 0, 8919 java.lang.Integer.MAX_VALUE, format)); 8920 childrenList.add(new Property("profile", "Reference(StructureDefinition)", 8921 "A list of profiles that represent different use cases supported by the system. For a server, \"supported by the system\" means the system hosts/produces a set of resources that are conformant to a particular profile, and allows clients that use its services to search using this profile and to find appropriate data. For a client, it means the system will search by this profile and process data according to the guidance implicit in the profile. See further discussion in [Using Profiles]{profiling.html#profile-uses}.", 8922 0, java.lang.Integer.MAX_VALUE, profile)); 8923 childrenList.add(new Property("rest", "", "A definition of the restful capabilities of the solution, if any.", 0, 8924 java.lang.Integer.MAX_VALUE, rest)); 8925 childrenList.add(new Property("messaging", "", "A description of the messaging capabilities of the solution.", 0, 8926 java.lang.Integer.MAX_VALUE, messaging)); 8927 childrenList.add(new Property("document", "", "A document definition.", 0, java.lang.Integer.MAX_VALUE, document)); 8928 } 8929 8930 @Override 8931 public void setProperty(String name, Base value) throws FHIRException { 8932 if (name.equals("url")) 8933 this.url = castToUri(value); // UriType 8934 else if (name.equals("version")) 8935 this.version = castToString(value); // StringType 8936 else if (name.equals("name")) 8937 this.name = castToString(value); // StringType 8938 else if (name.equals("status")) 8939 this.status = new ConformanceResourceStatusEnumFactory().fromType(value); // Enumeration<ConformanceResourceStatus> 8940 else if (name.equals("experimental")) 8941 this.experimental = castToBoolean(value); // BooleanType 8942 else if (name.equals("publisher")) 8943 this.publisher = castToString(value); // StringType 8944 else if (name.equals("contact")) 8945 this.getContact().add((ConformanceContactComponent) value); 8946 else if (name.equals("date")) 8947 this.date = castToDateTime(value); // DateTimeType 8948 else if (name.equals("description")) 8949 this.description = castToString(value); // StringType 8950 else if (name.equals("requirements")) 8951 this.requirements = castToString(value); // StringType 8952 else if (name.equals("copyright")) 8953 this.copyright = castToString(value); // StringType 8954 else if (name.equals("kind")) 8955 this.kind = new ConformanceStatementKindEnumFactory().fromType(value); // Enumeration<ConformanceStatementKind> 8956 else if (name.equals("software")) 8957 this.software = (ConformanceSoftwareComponent) value; // ConformanceSoftwareComponent 8958 else if (name.equals("implementation")) 8959 this.implementation = (ConformanceImplementationComponent) value; // ConformanceImplementationComponent 8960 else if (name.equals("fhirVersion")) 8961 this.fhirVersion = castToId(value); // IdType 8962 else if (name.equals("acceptUnknown")) 8963 this.acceptUnknown = new UnknownContentCodeEnumFactory().fromType(value); // Enumeration<UnknownContentCode> 8964 else if (name.equals("format")) 8965 this.getFormat().add(castToCode(value)); 8966 else if (name.equals("profile")) 8967 this.getProfile().add(castToReference(value)); 8968 else if (name.equals("rest")) 8969 this.getRest().add((ConformanceRestComponent) value); 8970 else if (name.equals("messaging")) 8971 this.getMessaging().add((ConformanceMessagingComponent) value); 8972 else if (name.equals("document")) 8973 this.getDocument().add((ConformanceDocumentComponent) value); 8974 else 8975 super.setProperty(name, value); 8976 } 8977 8978 @Override 8979 public Base addChild(String name) throws FHIRException { 8980 if (name.equals("url")) { 8981 throw new FHIRException("Cannot call addChild on a singleton property Conformance.url"); 8982 } else if (name.equals("version")) { 8983 throw new FHIRException("Cannot call addChild on a singleton property Conformance.version"); 8984 } else if (name.equals("name")) { 8985 throw new FHIRException("Cannot call addChild on a singleton property Conformance.name"); 8986 } else if (name.equals("status")) { 8987 throw new FHIRException("Cannot call addChild on a singleton property Conformance.status"); 8988 } else if (name.equals("experimental")) { 8989 throw new FHIRException("Cannot call addChild on a singleton property Conformance.experimental"); 8990 } else if (name.equals("publisher")) { 8991 throw new FHIRException("Cannot call addChild on a singleton property Conformance.publisher"); 8992 } else if (name.equals("contact")) { 8993 return addContact(); 8994 } else if (name.equals("date")) { 8995 throw new FHIRException("Cannot call addChild on a singleton property Conformance.date"); 8996 } else if (name.equals("description")) { 8997 throw new FHIRException("Cannot call addChild on a singleton property Conformance.description"); 8998 } else if (name.equals("requirements")) { 8999 throw new FHIRException("Cannot call addChild on a singleton property Conformance.requirements"); 9000 } else if (name.equals("copyright")) { 9001 throw new FHIRException("Cannot call addChild on a singleton property Conformance.copyright"); 9002 } else if (name.equals("kind")) { 9003 throw new FHIRException("Cannot call addChild on a singleton property Conformance.kind"); 9004 } else if (name.equals("software")) { 9005 this.software = new ConformanceSoftwareComponent(); 9006 return this.software; 9007 } else if (name.equals("implementation")) { 9008 this.implementation = new ConformanceImplementationComponent(); 9009 return this.implementation; 9010 } else if (name.equals("fhirVersion")) { 9011 throw new FHIRException("Cannot call addChild on a singleton property Conformance.fhirVersion"); 9012 } else if (name.equals("acceptUnknown")) { 9013 throw new FHIRException("Cannot call addChild on a singleton property Conformance.acceptUnknown"); 9014 } else if (name.equals("format")) { 9015 throw new FHIRException("Cannot call addChild on a singleton property Conformance.format"); 9016 } else if (name.equals("profile")) { 9017 return addProfile(); 9018 } else if (name.equals("rest")) { 9019 return addRest(); 9020 } else if (name.equals("messaging")) { 9021 return addMessaging(); 9022 } else if (name.equals("document")) { 9023 return addDocument(); 9024 } else 9025 return super.addChild(name); 9026 } 9027 9028 public String fhirType() { 9029 return "Conformance"; 9030 9031 } 9032 9033 public Conformance copy() { 9034 Conformance dst = new Conformance(); 9035 copyValues(dst); 9036 dst.url = url == null ? null : url.copy(); 9037 dst.version = version == null ? null : version.copy(); 9038 dst.name = name == null ? null : name.copy(); 9039 dst.status = status == null ? null : status.copy(); 9040 dst.experimental = experimental == null ? null : experimental.copy(); 9041 dst.publisher = publisher == null ? null : publisher.copy(); 9042 if (contact != null) { 9043 dst.contact = new ArrayList<ConformanceContactComponent>(); 9044 for (ConformanceContactComponent i : contact) 9045 dst.contact.add(i.copy()); 9046 } 9047 ; 9048 dst.date = date == null ? null : date.copy(); 9049 dst.description = description == null ? null : description.copy(); 9050 dst.requirements = requirements == null ? null : requirements.copy(); 9051 dst.copyright = copyright == null ? null : copyright.copy(); 9052 dst.kind = kind == null ? null : kind.copy(); 9053 dst.software = software == null ? null : software.copy(); 9054 dst.implementation = implementation == null ? null : implementation.copy(); 9055 dst.fhirVersion = fhirVersion == null ? null : fhirVersion.copy(); 9056 dst.acceptUnknown = acceptUnknown == null ? null : acceptUnknown.copy(); 9057 if (format != null) { 9058 dst.format = new ArrayList<CodeType>(); 9059 for (CodeType i : format) 9060 dst.format.add(i.copy()); 9061 } 9062 ; 9063 if (profile != null) { 9064 dst.profile = new ArrayList<Reference>(); 9065 for (Reference i : profile) 9066 dst.profile.add(i.copy()); 9067 } 9068 ; 9069 if (rest != null) { 9070 dst.rest = new ArrayList<ConformanceRestComponent>(); 9071 for (ConformanceRestComponent i : rest) 9072 dst.rest.add(i.copy()); 9073 } 9074 ; 9075 if (messaging != null) { 9076 dst.messaging = new ArrayList<ConformanceMessagingComponent>(); 9077 for (ConformanceMessagingComponent i : messaging) 9078 dst.messaging.add(i.copy()); 9079 } 9080 ; 9081 if (document != null) { 9082 dst.document = new ArrayList<ConformanceDocumentComponent>(); 9083 for (ConformanceDocumentComponent i : document) 9084 dst.document.add(i.copy()); 9085 } 9086 ; 9087 return dst; 9088 } 9089 9090 protected Conformance typedCopy() { 9091 return copy(); 9092 } 9093 9094 @Override 9095 public boolean equalsDeep(Base other) { 9096 if (!super.equalsDeep(other)) 9097 return false; 9098 if (!(other instanceof Conformance)) 9099 return false; 9100 Conformance o = (Conformance) other; 9101 return compareDeep(url, o.url, true) && compareDeep(version, o.version, true) && compareDeep(name, o.name, true) 9102 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 9103 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 9104 && compareDeep(date, o.date, true) && compareDeep(description, o.description, true) 9105 && compareDeep(requirements, o.requirements, true) && compareDeep(copyright, o.copyright, true) 9106 && compareDeep(kind, o.kind, true) && compareDeep(software, o.software, true) 9107 && compareDeep(implementation, o.implementation, true) && compareDeep(fhirVersion, o.fhirVersion, true) 9108 && compareDeep(acceptUnknown, o.acceptUnknown, true) && compareDeep(format, o.format, true) 9109 && compareDeep(profile, o.profile, true) && compareDeep(rest, o.rest, true) 9110 && compareDeep(messaging, o.messaging, true) && compareDeep(document, o.document, true); 9111 } 9112 9113 @Override 9114 public boolean equalsShallow(Base other) { 9115 if (!super.equalsShallow(other)) 9116 return false; 9117 if (!(other instanceof Conformance)) 9118 return false; 9119 Conformance o = (Conformance) other; 9120 return compareValues(url, o.url, true) && compareValues(version, o.version, true) 9121 && compareValues(name, o.name, true) && compareValues(status, o.status, true) 9122 && compareValues(experimental, o.experimental, true) && compareValues(publisher, o.publisher, true) 9123 && compareValues(date, o.date, true) && compareValues(description, o.description, true) 9124 && compareValues(requirements, o.requirements, true) && compareValues(copyright, o.copyright, true) 9125 && compareValues(kind, o.kind, true) && compareValues(fhirVersion, o.fhirVersion, true) 9126 && compareValues(acceptUnknown, o.acceptUnknown, true) && compareValues(format, o.format, true); 9127 } 9128 9129 public boolean isEmpty() { 9130 return super.isEmpty() && (url == null || url.isEmpty()) && (version == null || version.isEmpty()) 9131 && (name == null || name.isEmpty()) && (status == null || status.isEmpty()) 9132 && (experimental == null || experimental.isEmpty()) && (publisher == null || publisher.isEmpty()) 9133 && (contact == null || contact.isEmpty()) && (date == null || date.isEmpty()) 9134 && (description == null || description.isEmpty()) && (requirements == null || requirements.isEmpty()) 9135 && (copyright == null || copyright.isEmpty()) && (kind == null || kind.isEmpty()) 9136 && (software == null || software.isEmpty()) && (implementation == null || implementation.isEmpty()) 9137 && (fhirVersion == null || fhirVersion.isEmpty()) && (acceptUnknown == null || acceptUnknown.isEmpty()) 9138 && (format == null || format.isEmpty()) && (profile == null || profile.isEmpty()) 9139 && (rest == null || rest.isEmpty()) && (messaging == null || messaging.isEmpty()) 9140 && (document == null || document.isEmpty()); 9141 } 9142 9143 @Override 9144 public ResourceType getResourceType() { 9145 return ResourceType.Conformance; 9146 } 9147 9148 @SearchParamDefinition(name = "date", path = "Conformance.date", description = "The conformance statement publication date", type = "date") 9149 public static final String SP_DATE = "date"; 9150 @SearchParamDefinition(name = "software", path = "Conformance.software.name", description = "Part of a the name of a software application", type = "string") 9151 public static final String SP_SOFTWARE = "software"; 9152 @SearchParamDefinition(name = "resource", path = "Conformance.rest.resource.type", description = "Name of a resource mentioned in a conformance statement", type = "token") 9153 public static final String SP_RESOURCE = "resource"; 9154 @SearchParamDefinition(name = "profile", path = "Conformance.rest.resource.profile", description = "A profile id invoked in a conformance statement", type = "reference") 9155 public static final String SP_PROFILE = "profile"; 9156 @SearchParamDefinition(name = "format", path = "Conformance.format", description = "formats supported (xml | json | mime type)", type = "token") 9157 public static final String SP_FORMAT = "format"; 9158 @SearchParamDefinition(name = "description", path = "Conformance.description", description = "Text search in the description of the conformance statement", type = "string") 9159 public static final String SP_DESCRIPTION = "description"; 9160 @SearchParamDefinition(name = "fhirversion", path = "Conformance.version", description = "The version of FHIR", type = "token") 9161 public static final String SP_FHIRVERSION = "fhirversion"; 9162 @SearchParamDefinition(name = "version", path = "Conformance.version", description = "The version identifier of the conformance statement", type = "token") 9163 public static final String SP_VERSION = "version"; 9164 @SearchParamDefinition(name = "url", path = "Conformance.url", description = "The uri that identifies the conformance statement", type = "uri") 9165 public static final String SP_URL = "url"; 9166 @SearchParamDefinition(name = "supported-profile", path = "Conformance.profile", description = "Profiles for use cases supported", type = "reference") 9167 public static final String SP_SUPPORTEDPROFILE = "supported-profile"; 9168 @SearchParamDefinition(name = "mode", path = "Conformance.rest.mode", description = "Mode - restful (server/client) or messaging (sender/receiver)", type = "token") 9169 public static final String SP_MODE = "mode"; 9170 @SearchParamDefinition(name = "security", path = "Conformance.rest.security.service", description = "OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | Certificates", type = "token") 9171 public static final String SP_SECURITY = "security"; 9172 @SearchParamDefinition(name = "name", path = "Conformance.name", description = "Name of the conformance statement", type = "string") 9173 public static final String SP_NAME = "name"; 9174 @SearchParamDefinition(name = "publisher", path = "Conformance.publisher", description = "Name of the publisher of the conformance statement", type = "string") 9175 public static final String SP_PUBLISHER = "publisher"; 9176 @SearchParamDefinition(name = "event", path = "Conformance.messaging.event.code", description = "Event code in a conformance statement", type = "token") 9177 public static final String SP_EVENT = "event"; 9178 @SearchParamDefinition(name = "status", path = "Conformance.status", description = "The current status of the conformance statement", type = "token") 9179 public static final String SP_STATUS = "status"; 9180 9181}