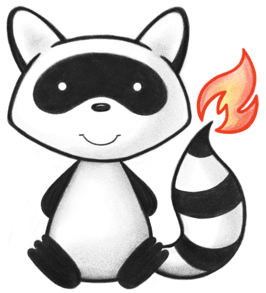
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus; 038import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory; 039import org.hl7.fhir.dstu2.model.Enumerations.SearchParamType; 040import org.hl7.fhir.dstu2.model.Enumerations.SearchParamTypeEnumFactory; 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 047import org.hl7.fhir.instance.model.api.IBaseConformance; 048import org.hl7.fhir.exceptions.FHIRException; 049import org.hl7.fhir.utilities.Utilities; 050 051/** 052 * A conformance statement is a set of capabilities of a FHIR Server that may be 053 * used as a statement of actual server functionality or a statement of required 054 * or desired server implementation. 055 */ 056@ResourceDef(name = "Conformance", profile = "http://hl7.org/fhir/Profile/Conformance") 057public class Conformance extends DomainResource implements IBaseConformance { 058 059 public enum ConformanceStatementKind { 060 /** 061 * The Conformance instance represents the present capabilities of a specific 062 * system instance. This is the kind returned by OPTIONS for a FHIR server 063 * end-point. 064 */ 065 INSTANCE, 066 /** 067 * The Conformance instance represents the capabilities of a system or piece of 068 * software, independent of a particular installation. 069 */ 070 CAPABILITY, 071 /** 072 * The Conformance instance represents a set of requirements for other systems 073 * to meet; e.g. as part of an implementation guide or 'request for proposal'. 074 */ 075 REQUIREMENTS, 076 /** 077 * added to help the parsers 078 */ 079 NULL; 080 081 public static ConformanceStatementKind fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("instance".equals(codeString)) 085 return INSTANCE; 086 if ("capability".equals(codeString)) 087 return CAPABILITY; 088 if ("requirements".equals(codeString)) 089 return REQUIREMENTS; 090 throw new FHIRException("Unknown ConformanceStatementKind code '" + codeString + "'"); 091 } 092 093 public String toCode() { 094 switch (this) { 095 case INSTANCE: 096 return "instance"; 097 case CAPABILITY: 098 return "capability"; 099 case REQUIREMENTS: 100 return "requirements"; 101 case NULL: 102 return null; 103 default: 104 return "?"; 105 } 106 } 107 108 public String getSystem() { 109 switch (this) { 110 case INSTANCE: 111 return "http://hl7.org/fhir/conformance-statement-kind"; 112 case CAPABILITY: 113 return "http://hl7.org/fhir/conformance-statement-kind"; 114 case REQUIREMENTS: 115 return "http://hl7.org/fhir/conformance-statement-kind"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getDefinition() { 124 switch (this) { 125 case INSTANCE: 126 return "The Conformance instance represents the present capabilities of a specific system instance. This is the kind returned by OPTIONS for a FHIR server end-point."; 127 case CAPABILITY: 128 return "The Conformance instance represents the capabilities of a system or piece of software, independent of a particular installation."; 129 case REQUIREMENTS: 130 return "The Conformance instance represents a set of requirements for other systems to meet; e.g. as part of an implementation guide or 'request for proposal'."; 131 case NULL: 132 return null; 133 default: 134 return "?"; 135 } 136 } 137 138 public String getDisplay() { 139 switch (this) { 140 case INSTANCE: 141 return "Instance"; 142 case CAPABILITY: 143 return "Capability"; 144 case REQUIREMENTS: 145 return "Requirements"; 146 case NULL: 147 return null; 148 default: 149 return "?"; 150 } 151 } 152 } 153 154 public static class ConformanceStatementKindEnumFactory implements EnumFactory<ConformanceStatementKind> { 155 public ConformanceStatementKind fromCode(String codeString) throws IllegalArgumentException { 156 if (codeString == null || "".equals(codeString)) 157 if (codeString == null || "".equals(codeString)) 158 return null; 159 if ("instance".equals(codeString)) 160 return ConformanceStatementKind.INSTANCE; 161 if ("capability".equals(codeString)) 162 return ConformanceStatementKind.CAPABILITY; 163 if ("requirements".equals(codeString)) 164 return ConformanceStatementKind.REQUIREMENTS; 165 throw new IllegalArgumentException("Unknown ConformanceStatementKind code '" + codeString + "'"); 166 } 167 168 public Enumeration<ConformanceStatementKind> fromType(Base code) throws FHIRException { 169 if (code == null || code.isEmpty()) 170 return null; 171 String codeString = ((PrimitiveType) code).asStringValue(); 172 if (codeString == null || "".equals(codeString)) 173 return null; 174 if ("instance".equals(codeString)) 175 return new Enumeration<ConformanceStatementKind>(this, ConformanceStatementKind.INSTANCE); 176 if ("capability".equals(codeString)) 177 return new Enumeration<ConformanceStatementKind>(this, ConformanceStatementKind.CAPABILITY); 178 if ("requirements".equals(codeString)) 179 return new Enumeration<ConformanceStatementKind>(this, ConformanceStatementKind.REQUIREMENTS); 180 throw new FHIRException("Unknown ConformanceStatementKind code '" + codeString + "'"); 181 } 182 183 public String toCode(ConformanceStatementKind code) { 184 if (code == ConformanceStatementKind.INSTANCE) 185 return "instance"; 186 if (code == ConformanceStatementKind.CAPABILITY) 187 return "capability"; 188 if (code == ConformanceStatementKind.REQUIREMENTS) 189 return "requirements"; 190 return "?"; 191 } 192 } 193 194 public enum UnknownContentCode { 195 /** 196 * The application does not accept either unknown elements or extensions. 197 */ 198 NO, 199 /** 200 * The application accepts unknown extensions, but not unknown elements. 201 */ 202 EXTENSIONS, 203 /** 204 * The application accepts unknown elements, but not unknown extensions. 205 */ 206 ELEMENTS, 207 /** 208 * The application accepts unknown elements and extensions. 209 */ 210 BOTH, 211 /** 212 * added to help the parsers 213 */ 214 NULL; 215 216 public static UnknownContentCode fromCode(String codeString) throws FHIRException { 217 if (codeString == null || "".equals(codeString)) 218 return null; 219 if ("no".equals(codeString)) 220 return NO; 221 if ("extensions".equals(codeString)) 222 return EXTENSIONS; 223 if ("elements".equals(codeString)) 224 return ELEMENTS; 225 if ("both".equals(codeString)) 226 return BOTH; 227 throw new FHIRException("Unknown UnknownContentCode code '" + codeString + "'"); 228 } 229 230 public String toCode() { 231 switch (this) { 232 case NO: 233 return "no"; 234 case EXTENSIONS: 235 return "extensions"; 236 case ELEMENTS: 237 return "elements"; 238 case BOTH: 239 return "both"; 240 case NULL: 241 return null; 242 default: 243 return "?"; 244 } 245 } 246 247 public String getSystem() { 248 switch (this) { 249 case NO: 250 return "http://hl7.org/fhir/unknown-content-code"; 251 case EXTENSIONS: 252 return "http://hl7.org/fhir/unknown-content-code"; 253 case ELEMENTS: 254 return "http://hl7.org/fhir/unknown-content-code"; 255 case BOTH: 256 return "http://hl7.org/fhir/unknown-content-code"; 257 case NULL: 258 return null; 259 default: 260 return "?"; 261 } 262 } 263 264 public String getDefinition() { 265 switch (this) { 266 case NO: 267 return "The application does not accept either unknown elements or extensions."; 268 case EXTENSIONS: 269 return "The application accepts unknown extensions, but not unknown elements."; 270 case ELEMENTS: 271 return "The application accepts unknown elements, but not unknown extensions."; 272 case BOTH: 273 return "The application accepts unknown elements and extensions."; 274 case NULL: 275 return null; 276 default: 277 return "?"; 278 } 279 } 280 281 public String getDisplay() { 282 switch (this) { 283 case NO: 284 return "Neither Elements or Extensions"; 285 case EXTENSIONS: 286 return "Unknown Extensions"; 287 case ELEMENTS: 288 return "Unknown Elements"; 289 case BOTH: 290 return "Unknown Elements and Extensions"; 291 case NULL: 292 return null; 293 default: 294 return "?"; 295 } 296 } 297 } 298 299 public static class UnknownContentCodeEnumFactory implements EnumFactory<UnknownContentCode> { 300 public UnknownContentCode fromCode(String codeString) throws IllegalArgumentException { 301 if (codeString == null || "".equals(codeString)) 302 if (codeString == null || "".equals(codeString)) 303 return null; 304 if ("no".equals(codeString)) 305 return UnknownContentCode.NO; 306 if ("extensions".equals(codeString)) 307 return UnknownContentCode.EXTENSIONS; 308 if ("elements".equals(codeString)) 309 return UnknownContentCode.ELEMENTS; 310 if ("both".equals(codeString)) 311 return UnknownContentCode.BOTH; 312 throw new IllegalArgumentException("Unknown UnknownContentCode code '" + codeString + "'"); 313 } 314 315 public Enumeration<UnknownContentCode> fromType(Base code) throws FHIRException { 316 if (code == null || code.isEmpty()) 317 return null; 318 String codeString = ((PrimitiveType) code).asStringValue(); 319 if (codeString == null || "".equals(codeString)) 320 return null; 321 if ("no".equals(codeString)) 322 return new Enumeration<UnknownContentCode>(this, UnknownContentCode.NO); 323 if ("extensions".equals(codeString)) 324 return new Enumeration<UnknownContentCode>(this, UnknownContentCode.EXTENSIONS); 325 if ("elements".equals(codeString)) 326 return new Enumeration<UnknownContentCode>(this, UnknownContentCode.ELEMENTS); 327 if ("both".equals(codeString)) 328 return new Enumeration<UnknownContentCode>(this, UnknownContentCode.BOTH); 329 throw new FHIRException("Unknown UnknownContentCode code '" + codeString + "'"); 330 } 331 332 public String toCode(UnknownContentCode code) { 333 if (code == UnknownContentCode.NO) 334 return "no"; 335 if (code == UnknownContentCode.EXTENSIONS) 336 return "extensions"; 337 if (code == UnknownContentCode.ELEMENTS) 338 return "elements"; 339 if (code == UnknownContentCode.BOTH) 340 return "both"; 341 return "?"; 342 } 343 } 344 345 public enum RestfulConformanceMode { 346 /** 347 * The application acts as a client for this resource. 348 */ 349 CLIENT, 350 /** 351 * The application acts as a server for this resource. 352 */ 353 SERVER, 354 /** 355 * added to help the parsers 356 */ 357 NULL; 358 359 public static RestfulConformanceMode fromCode(String codeString) throws FHIRException { 360 if (codeString == null || "".equals(codeString)) 361 return null; 362 if ("client".equals(codeString)) 363 return CLIENT; 364 if ("server".equals(codeString)) 365 return SERVER; 366 throw new FHIRException("Unknown RestfulConformanceMode code '" + codeString + "'"); 367 } 368 369 public String toCode() { 370 switch (this) { 371 case CLIENT: 372 return "client"; 373 case SERVER: 374 return "server"; 375 case NULL: 376 return null; 377 default: 378 return "?"; 379 } 380 } 381 382 public String getSystem() { 383 switch (this) { 384 case CLIENT: 385 return "http://hl7.org/fhir/restful-conformance-mode"; 386 case SERVER: 387 return "http://hl7.org/fhir/restful-conformance-mode"; 388 case NULL: 389 return null; 390 default: 391 return "?"; 392 } 393 } 394 395 public String getDefinition() { 396 switch (this) { 397 case CLIENT: 398 return "The application acts as a client for this resource."; 399 case SERVER: 400 return "The application acts as a server for this resource."; 401 case NULL: 402 return null; 403 default: 404 return "?"; 405 } 406 } 407 408 public String getDisplay() { 409 switch (this) { 410 case CLIENT: 411 return "Client"; 412 case SERVER: 413 return "Server"; 414 case NULL: 415 return null; 416 default: 417 return "?"; 418 } 419 } 420 } 421 422 public static class RestfulConformanceModeEnumFactory implements EnumFactory<RestfulConformanceMode> { 423 public RestfulConformanceMode fromCode(String codeString) throws IllegalArgumentException { 424 if (codeString == null || "".equals(codeString)) 425 if (codeString == null || "".equals(codeString)) 426 return null; 427 if ("client".equals(codeString)) 428 return RestfulConformanceMode.CLIENT; 429 if ("server".equals(codeString)) 430 return RestfulConformanceMode.SERVER; 431 throw new IllegalArgumentException("Unknown RestfulConformanceMode code '" + codeString + "'"); 432 } 433 434 public Enumeration<RestfulConformanceMode> fromType(Base code) throws FHIRException { 435 if (code == null || code.isEmpty()) 436 return null; 437 String codeString = ((PrimitiveType) code).asStringValue(); 438 if (codeString == null || "".equals(codeString)) 439 return null; 440 if ("client".equals(codeString)) 441 return new Enumeration<RestfulConformanceMode>(this, RestfulConformanceMode.CLIENT); 442 if ("server".equals(codeString)) 443 return new Enumeration<RestfulConformanceMode>(this, RestfulConformanceMode.SERVER); 444 throw new FHIRException("Unknown RestfulConformanceMode code '" + codeString + "'"); 445 } 446 447 public String toCode(RestfulConformanceMode code) { 448 if (code == RestfulConformanceMode.CLIENT) 449 return "client"; 450 if (code == RestfulConformanceMode.SERVER) 451 return "server"; 452 return "?"; 453 } 454 } 455 456 public enum TypeRestfulInteraction { 457 /** 458 * null 459 */ 460 READ, 461 /** 462 * null 463 */ 464 VREAD, 465 /** 466 * null 467 */ 468 UPDATE, 469 /** 470 * null 471 */ 472 DELETE, 473 /** 474 * null 475 */ 476 HISTORYINSTANCE, 477 /** 478 * null 479 */ 480 VALIDATE, 481 /** 482 * null 483 */ 484 HISTORYTYPE, 485 /** 486 * null 487 */ 488 CREATE, 489 /** 490 * null 491 */ 492 SEARCHTYPE, 493 /** 494 * added to help the parsers 495 */ 496 NULL; 497 498 public static TypeRestfulInteraction fromCode(String codeString) throws FHIRException { 499 if (codeString == null || "".equals(codeString)) 500 return null; 501 if ("read".equals(codeString)) 502 return READ; 503 if ("vread".equals(codeString)) 504 return VREAD; 505 if ("update".equals(codeString)) 506 return UPDATE; 507 if ("delete".equals(codeString)) 508 return DELETE; 509 if ("history-instance".equals(codeString)) 510 return HISTORYINSTANCE; 511 if ("validate".equals(codeString)) 512 return VALIDATE; 513 if ("history-type".equals(codeString)) 514 return HISTORYTYPE; 515 if ("create".equals(codeString)) 516 return CREATE; 517 if ("search-type".equals(codeString)) 518 return SEARCHTYPE; 519 throw new FHIRException("Unknown TypeRestfulInteraction code '" + codeString + "'"); 520 } 521 522 public String toCode() { 523 switch (this) { 524 case READ: 525 return "read"; 526 case VREAD: 527 return "vread"; 528 case UPDATE: 529 return "update"; 530 case DELETE: 531 return "delete"; 532 case HISTORYINSTANCE: 533 return "history-instance"; 534 case VALIDATE: 535 return "validate"; 536 case HISTORYTYPE: 537 return "history-type"; 538 case CREATE: 539 return "create"; 540 case SEARCHTYPE: 541 return "search-type"; 542 case NULL: 543 return null; 544 default: 545 return "?"; 546 } 547 } 548 549 public String getSystem() { 550 switch (this) { 551 case READ: 552 return "http://hl7.org/fhir/restful-interaction"; 553 case VREAD: 554 return "http://hl7.org/fhir/restful-interaction"; 555 case UPDATE: 556 return "http://hl7.org/fhir/restful-interaction"; 557 case DELETE: 558 return "http://hl7.org/fhir/restful-interaction"; 559 case HISTORYINSTANCE: 560 return "http://hl7.org/fhir/restful-interaction"; 561 case VALIDATE: 562 return "http://hl7.org/fhir/restful-interaction"; 563 case HISTORYTYPE: 564 return "http://hl7.org/fhir/restful-interaction"; 565 case CREATE: 566 return "http://hl7.org/fhir/restful-interaction"; 567 case SEARCHTYPE: 568 return "http://hl7.org/fhir/restful-interaction"; 569 case NULL: 570 return null; 571 default: 572 return "?"; 573 } 574 } 575 576 public String getDefinition() { 577 switch (this) { 578 case READ: 579 return ""; 580 case VREAD: 581 return ""; 582 case UPDATE: 583 return ""; 584 case DELETE: 585 return ""; 586 case HISTORYINSTANCE: 587 return ""; 588 case VALIDATE: 589 return ""; 590 case HISTORYTYPE: 591 return ""; 592 case CREATE: 593 return ""; 594 case SEARCHTYPE: 595 return ""; 596 case NULL: 597 return null; 598 default: 599 return "?"; 600 } 601 } 602 603 public String getDisplay() { 604 switch (this) { 605 case READ: 606 return "read"; 607 case VREAD: 608 return "vread"; 609 case UPDATE: 610 return "update"; 611 case DELETE: 612 return "delete"; 613 case HISTORYINSTANCE: 614 return "history-instance"; 615 case VALIDATE: 616 return "validate"; 617 case HISTORYTYPE: 618 return "history-type"; 619 case CREATE: 620 return "create"; 621 case SEARCHTYPE: 622 return "search-type"; 623 case NULL: 624 return null; 625 default: 626 return "?"; 627 } 628 } 629 } 630 631 public static class TypeRestfulInteractionEnumFactory implements EnumFactory<TypeRestfulInteraction> { 632 public TypeRestfulInteraction fromCode(String codeString) throws IllegalArgumentException { 633 if (codeString == null || "".equals(codeString)) 634 if (codeString == null || "".equals(codeString)) 635 return null; 636 if ("read".equals(codeString)) 637 return TypeRestfulInteraction.READ; 638 if ("vread".equals(codeString)) 639 return TypeRestfulInteraction.VREAD; 640 if ("update".equals(codeString)) 641 return TypeRestfulInteraction.UPDATE; 642 if ("delete".equals(codeString)) 643 return TypeRestfulInteraction.DELETE; 644 if ("history-instance".equals(codeString)) 645 return TypeRestfulInteraction.HISTORYINSTANCE; 646 if ("validate".equals(codeString)) 647 return TypeRestfulInteraction.VALIDATE; 648 if ("history-type".equals(codeString)) 649 return TypeRestfulInteraction.HISTORYTYPE; 650 if ("create".equals(codeString)) 651 return TypeRestfulInteraction.CREATE; 652 if ("search-type".equals(codeString)) 653 return TypeRestfulInteraction.SEARCHTYPE; 654 throw new IllegalArgumentException("Unknown TypeRestfulInteraction code '" + codeString + "'"); 655 } 656 657 public Enumeration<TypeRestfulInteraction> fromType(Base code) throws FHIRException { 658 if (code == null || code.isEmpty()) 659 return null; 660 String codeString = ((PrimitiveType) code).asStringValue(); 661 if (codeString == null || "".equals(codeString)) 662 return null; 663 if ("read".equals(codeString)) 664 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.READ); 665 if ("vread".equals(codeString)) 666 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.VREAD); 667 if ("update".equals(codeString)) 668 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.UPDATE); 669 if ("delete".equals(codeString)) 670 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.DELETE); 671 if ("history-instance".equals(codeString)) 672 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.HISTORYINSTANCE); 673 if ("validate".equals(codeString)) 674 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.VALIDATE); 675 if ("history-type".equals(codeString)) 676 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.HISTORYTYPE); 677 if ("create".equals(codeString)) 678 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.CREATE); 679 if ("search-type".equals(codeString)) 680 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.SEARCHTYPE); 681 throw new FHIRException("Unknown TypeRestfulInteraction code '" + codeString + "'"); 682 } 683 684 public String toCode(TypeRestfulInteraction code) { 685 if (code == TypeRestfulInteraction.READ) 686 return "read"; 687 if (code == TypeRestfulInteraction.VREAD) 688 return "vread"; 689 if (code == TypeRestfulInteraction.UPDATE) 690 return "update"; 691 if (code == TypeRestfulInteraction.DELETE) 692 return "delete"; 693 if (code == TypeRestfulInteraction.HISTORYINSTANCE) 694 return "history-instance"; 695 if (code == TypeRestfulInteraction.VALIDATE) 696 return "validate"; 697 if (code == TypeRestfulInteraction.HISTORYTYPE) 698 return "history-type"; 699 if (code == TypeRestfulInteraction.CREATE) 700 return "create"; 701 if (code == TypeRestfulInteraction.SEARCHTYPE) 702 return "search-type"; 703 return "?"; 704 } 705 } 706 707 public enum ResourceVersionPolicy { 708 /** 709 * VersionId meta-property is not supported (server) or used (client). 710 */ 711 NOVERSION, 712 /** 713 * VersionId meta-property is supported (server) or used (client). 714 */ 715 VERSIONED, 716 /** 717 * VersionId is must be correct for updates (server) or will be specified 718 * (If-match header) for updates (client). 719 */ 720 VERSIONEDUPDATE, 721 /** 722 * added to help the parsers 723 */ 724 NULL; 725 726 public static ResourceVersionPolicy fromCode(String codeString) throws FHIRException { 727 if (codeString == null || "".equals(codeString)) 728 return null; 729 if ("no-version".equals(codeString)) 730 return NOVERSION; 731 if ("versioned".equals(codeString)) 732 return VERSIONED; 733 if ("versioned-update".equals(codeString)) 734 return VERSIONEDUPDATE; 735 throw new FHIRException("Unknown ResourceVersionPolicy code '" + codeString + "'"); 736 } 737 738 public String toCode() { 739 switch (this) { 740 case NOVERSION: 741 return "no-version"; 742 case VERSIONED: 743 return "versioned"; 744 case VERSIONEDUPDATE: 745 return "versioned-update"; 746 case NULL: 747 return null; 748 default: 749 return "?"; 750 } 751 } 752 753 public String getSystem() { 754 switch (this) { 755 case NOVERSION: 756 return "http://hl7.org/fhir/versioning-policy"; 757 case VERSIONED: 758 return "http://hl7.org/fhir/versioning-policy"; 759 case VERSIONEDUPDATE: 760 return "http://hl7.org/fhir/versioning-policy"; 761 case NULL: 762 return null; 763 default: 764 return "?"; 765 } 766 } 767 768 public String getDefinition() { 769 switch (this) { 770 case NOVERSION: 771 return "VersionId meta-property is not supported (server) or used (client)."; 772 case VERSIONED: 773 return "VersionId meta-property is supported (server) or used (client)."; 774 case VERSIONEDUPDATE: 775 return "VersionId is must be correct for updates (server) or will be specified (If-match header) for updates (client)."; 776 case NULL: 777 return null; 778 default: 779 return "?"; 780 } 781 } 782 783 public String getDisplay() { 784 switch (this) { 785 case NOVERSION: 786 return "No VersionId Support"; 787 case VERSIONED: 788 return "Versioned"; 789 case VERSIONEDUPDATE: 790 return "VersionId tracked fully"; 791 case NULL: 792 return null; 793 default: 794 return "?"; 795 } 796 } 797 } 798 799 public static class ResourceVersionPolicyEnumFactory implements EnumFactory<ResourceVersionPolicy> { 800 public ResourceVersionPolicy fromCode(String codeString) throws IllegalArgumentException { 801 if (codeString == null || "".equals(codeString)) 802 if (codeString == null || "".equals(codeString)) 803 return null; 804 if ("no-version".equals(codeString)) 805 return ResourceVersionPolicy.NOVERSION; 806 if ("versioned".equals(codeString)) 807 return ResourceVersionPolicy.VERSIONED; 808 if ("versioned-update".equals(codeString)) 809 return ResourceVersionPolicy.VERSIONEDUPDATE; 810 throw new IllegalArgumentException("Unknown ResourceVersionPolicy code '" + codeString + "'"); 811 } 812 813 public Enumeration<ResourceVersionPolicy> fromType(Base code) throws FHIRException { 814 if (code == null || code.isEmpty()) 815 return null; 816 String codeString = ((PrimitiveType) code).asStringValue(); 817 if (codeString == null || "".equals(codeString)) 818 return null; 819 if ("no-version".equals(codeString)) 820 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.NOVERSION); 821 if ("versioned".equals(codeString)) 822 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.VERSIONED); 823 if ("versioned-update".equals(codeString)) 824 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.VERSIONEDUPDATE); 825 throw new FHIRException("Unknown ResourceVersionPolicy code '" + codeString + "'"); 826 } 827 828 public String toCode(ResourceVersionPolicy code) { 829 if (code == ResourceVersionPolicy.NOVERSION) 830 return "no-version"; 831 if (code == ResourceVersionPolicy.VERSIONED) 832 return "versioned"; 833 if (code == ResourceVersionPolicy.VERSIONEDUPDATE) 834 return "versioned-update"; 835 return "?"; 836 } 837 } 838 839 public enum ConditionalDeleteStatus { 840 /** 841 * No support for conditional deletes. 842 */ 843 NOTSUPPORTED, 844 /** 845 * Conditional deletes are supported, but only single resources at a time. 846 */ 847 SINGLE, 848 /** 849 * Conditional deletes are supported, and multiple resources can be deleted in a 850 * single interaction. 851 */ 852 MULTIPLE, 853 /** 854 * added to help the parsers 855 */ 856 NULL; 857 858 public static ConditionalDeleteStatus fromCode(String codeString) throws FHIRException { 859 if (codeString == null || "".equals(codeString)) 860 return null; 861 if ("not-supported".equals(codeString)) 862 return NOTSUPPORTED; 863 if ("single".equals(codeString)) 864 return SINGLE; 865 if ("multiple".equals(codeString)) 866 return MULTIPLE; 867 throw new FHIRException("Unknown ConditionalDeleteStatus code '" + codeString + "'"); 868 } 869 870 public String toCode() { 871 switch (this) { 872 case NOTSUPPORTED: 873 return "not-supported"; 874 case SINGLE: 875 return "single"; 876 case MULTIPLE: 877 return "multiple"; 878 case NULL: 879 return null; 880 default: 881 return "?"; 882 } 883 } 884 885 public String getSystem() { 886 switch (this) { 887 case NOTSUPPORTED: 888 return "http://hl7.org/fhir/conditional-delete-status"; 889 case SINGLE: 890 return "http://hl7.org/fhir/conditional-delete-status"; 891 case MULTIPLE: 892 return "http://hl7.org/fhir/conditional-delete-status"; 893 case NULL: 894 return null; 895 default: 896 return "?"; 897 } 898 } 899 900 public String getDefinition() { 901 switch (this) { 902 case NOTSUPPORTED: 903 return "No support for conditional deletes."; 904 case SINGLE: 905 return "Conditional deletes are supported, but only single resources at a time."; 906 case MULTIPLE: 907 return "Conditional deletes are supported, and multiple resources can be deleted in a single interaction."; 908 case NULL: 909 return null; 910 default: 911 return "?"; 912 } 913 } 914 915 public String getDisplay() { 916 switch (this) { 917 case NOTSUPPORTED: 918 return "Not Supported"; 919 case SINGLE: 920 return "Single Deletes Supported"; 921 case MULTIPLE: 922 return "Multiple Deletes Supported"; 923 case NULL: 924 return null; 925 default: 926 return "?"; 927 } 928 } 929 } 930 931 public static class ConditionalDeleteStatusEnumFactory implements EnumFactory<ConditionalDeleteStatus> { 932 public ConditionalDeleteStatus fromCode(String codeString) throws IllegalArgumentException { 933 if (codeString == null || "".equals(codeString)) 934 if (codeString == null || "".equals(codeString)) 935 return null; 936 if ("not-supported".equals(codeString)) 937 return ConditionalDeleteStatus.NOTSUPPORTED; 938 if ("single".equals(codeString)) 939 return ConditionalDeleteStatus.SINGLE; 940 if ("multiple".equals(codeString)) 941 return ConditionalDeleteStatus.MULTIPLE; 942 throw new IllegalArgumentException("Unknown ConditionalDeleteStatus code '" + codeString + "'"); 943 } 944 945 public Enumeration<ConditionalDeleteStatus> fromType(Base code) throws FHIRException { 946 if (code == null || code.isEmpty()) 947 return null; 948 String codeString = ((PrimitiveType) code).asStringValue(); 949 if (codeString == null || "".equals(codeString)) 950 return null; 951 if ("not-supported".equals(codeString)) 952 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.NOTSUPPORTED); 953 if ("single".equals(codeString)) 954 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.SINGLE); 955 if ("multiple".equals(codeString)) 956 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.MULTIPLE); 957 throw new FHIRException("Unknown ConditionalDeleteStatus code '" + codeString + "'"); 958 } 959 960 public String toCode(ConditionalDeleteStatus code) { 961 if (code == ConditionalDeleteStatus.NOTSUPPORTED) 962 return "not-supported"; 963 if (code == ConditionalDeleteStatus.SINGLE) 964 return "single"; 965 if (code == ConditionalDeleteStatus.MULTIPLE) 966 return "multiple"; 967 return "?"; 968 } 969 } 970 971 public enum SearchModifierCode { 972 /** 973 * The search parameter returns resources that have a value or not. 974 */ 975 MISSING, 976 /** 977 * The search parameter returns resources that have a value that exactly matches 978 * the supplied parameter (the whole string, including casing and accents). 979 */ 980 EXACT, 981 /** 982 * The search parameter returns resources that include the supplied parameter 983 * value anywhere within the field being searched. 984 */ 985 CONTAINS, 986 /** 987 * The search parameter returns resources that do not contain a match . 988 */ 989 NOT, 990 /** 991 * The search parameter is processed as a string that searches text associated 992 * with the code/value - either CodeableConcept.text, Coding.display, or 993 * Identifier.type.text. 994 */ 995 TEXT, 996 /** 997 * The search parameter is a URI (relative or absolute) that identifies a value 998 * set, and the search parameter tests whether the coding is in the specified 999 * value set. 1000 */ 1001 IN, 1002 /** 1003 * The search parameter is a URI (relative or absolute) that identifies a value 1004 * set, and the search parameter tests whether the coding is not in the 1005 * specified value set. 1006 */ 1007 NOTIN, 1008 /** 1009 * The search parameter tests whether the value in a resource is subsumed by the 1010 * specified value (is-a, or hierarchical relationships). 1011 */ 1012 BELOW, 1013 /** 1014 * The search parameter tests whether the value in a resource subsumes the 1015 * specified value (is-a, or hierarchical relationships). 1016 */ 1017 ABOVE, 1018 /** 1019 * The search parameter only applies to the Resource Type specified as a 1020 * modifier (e.g. the modifier is not actually :type, but :Patient etc.). 1021 */ 1022 TYPE, 1023 /** 1024 * added to help the parsers 1025 */ 1026 NULL; 1027 1028 public static SearchModifierCode fromCode(String codeString) throws FHIRException { 1029 if (codeString == null || "".equals(codeString)) 1030 return null; 1031 if ("missing".equals(codeString)) 1032 return MISSING; 1033 if ("exact".equals(codeString)) 1034 return EXACT; 1035 if ("contains".equals(codeString)) 1036 return CONTAINS; 1037 if ("not".equals(codeString)) 1038 return NOT; 1039 if ("text".equals(codeString)) 1040 return TEXT; 1041 if ("in".equals(codeString)) 1042 return IN; 1043 if ("not-in".equals(codeString)) 1044 return NOTIN; 1045 if ("below".equals(codeString)) 1046 return BELOW; 1047 if ("above".equals(codeString)) 1048 return ABOVE; 1049 if ("type".equals(codeString)) 1050 return TYPE; 1051 throw new FHIRException("Unknown SearchModifierCode code '" + codeString + "'"); 1052 } 1053 1054 public String toCode() { 1055 switch (this) { 1056 case MISSING: 1057 return "missing"; 1058 case EXACT: 1059 return "exact"; 1060 case CONTAINS: 1061 return "contains"; 1062 case NOT: 1063 return "not"; 1064 case TEXT: 1065 return "text"; 1066 case IN: 1067 return "in"; 1068 case NOTIN: 1069 return "not-in"; 1070 case BELOW: 1071 return "below"; 1072 case ABOVE: 1073 return "above"; 1074 case TYPE: 1075 return "type"; 1076 case NULL: 1077 return null; 1078 default: 1079 return "?"; 1080 } 1081 } 1082 1083 public String getSystem() { 1084 switch (this) { 1085 case MISSING: 1086 return "http://hl7.org/fhir/search-modifier-code"; 1087 case EXACT: 1088 return "http://hl7.org/fhir/search-modifier-code"; 1089 case CONTAINS: 1090 return "http://hl7.org/fhir/search-modifier-code"; 1091 case NOT: 1092 return "http://hl7.org/fhir/search-modifier-code"; 1093 case TEXT: 1094 return "http://hl7.org/fhir/search-modifier-code"; 1095 case IN: 1096 return "http://hl7.org/fhir/search-modifier-code"; 1097 case NOTIN: 1098 return "http://hl7.org/fhir/search-modifier-code"; 1099 case BELOW: 1100 return "http://hl7.org/fhir/search-modifier-code"; 1101 case ABOVE: 1102 return "http://hl7.org/fhir/search-modifier-code"; 1103 case TYPE: 1104 return "http://hl7.org/fhir/search-modifier-code"; 1105 case NULL: 1106 return null; 1107 default: 1108 return "?"; 1109 } 1110 } 1111 1112 public String getDefinition() { 1113 switch (this) { 1114 case MISSING: 1115 return "The search parameter returns resources that have a value or not."; 1116 case EXACT: 1117 return "The search parameter returns resources that have a value that exactly matches the supplied parameter (the whole string, including casing and accents)."; 1118 case CONTAINS: 1119 return "The search parameter returns resources that include the supplied parameter value anywhere within the field being searched."; 1120 case NOT: 1121 return "The search parameter returns resources that do not contain a match ."; 1122 case TEXT: 1123 return "The search parameter is processed as a string that searches text associated with the code/value - either CodeableConcept.text, Coding.display, or Identifier.type.text."; 1124 case IN: 1125 return "The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is in the specified value set."; 1126 case NOTIN: 1127 return "The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is not in the specified value set."; 1128 case BELOW: 1129 return "The search parameter tests whether the value in a resource is subsumed by the specified value (is-a, or hierarchical relationships)."; 1130 case ABOVE: 1131 return "The search parameter tests whether the value in a resource subsumes the specified value (is-a, or hierarchical relationships)."; 1132 case TYPE: 1133 return "The search parameter only applies to the Resource Type specified as a modifier (e.g. the modifier is not actually :type, but :Patient etc.)."; 1134 case NULL: 1135 return null; 1136 default: 1137 return "?"; 1138 } 1139 } 1140 1141 public String getDisplay() { 1142 switch (this) { 1143 case MISSING: 1144 return "Missing"; 1145 case EXACT: 1146 return "Exact"; 1147 case CONTAINS: 1148 return "Contains"; 1149 case NOT: 1150 return "Not"; 1151 case TEXT: 1152 return "Text"; 1153 case IN: 1154 return "In"; 1155 case NOTIN: 1156 return "Not In"; 1157 case BELOW: 1158 return "Below"; 1159 case ABOVE: 1160 return "Above"; 1161 case TYPE: 1162 return "Type"; 1163 case NULL: 1164 return null; 1165 default: 1166 return "?"; 1167 } 1168 } 1169 } 1170 1171 public static class SearchModifierCodeEnumFactory implements EnumFactory<SearchModifierCode> { 1172 public SearchModifierCode fromCode(String codeString) throws IllegalArgumentException { 1173 if (codeString == null || "".equals(codeString)) 1174 if (codeString == null || "".equals(codeString)) 1175 return null; 1176 if ("missing".equals(codeString)) 1177 return SearchModifierCode.MISSING; 1178 if ("exact".equals(codeString)) 1179 return SearchModifierCode.EXACT; 1180 if ("contains".equals(codeString)) 1181 return SearchModifierCode.CONTAINS; 1182 if ("not".equals(codeString)) 1183 return SearchModifierCode.NOT; 1184 if ("text".equals(codeString)) 1185 return SearchModifierCode.TEXT; 1186 if ("in".equals(codeString)) 1187 return SearchModifierCode.IN; 1188 if ("not-in".equals(codeString)) 1189 return SearchModifierCode.NOTIN; 1190 if ("below".equals(codeString)) 1191 return SearchModifierCode.BELOW; 1192 if ("above".equals(codeString)) 1193 return SearchModifierCode.ABOVE; 1194 if ("type".equals(codeString)) 1195 return SearchModifierCode.TYPE; 1196 throw new IllegalArgumentException("Unknown SearchModifierCode code '" + codeString + "'"); 1197 } 1198 1199 public Enumeration<SearchModifierCode> fromType(Base code) throws FHIRException { 1200 if (code == null || code.isEmpty()) 1201 return null; 1202 String codeString = ((PrimitiveType) code).asStringValue(); 1203 if (codeString == null || "".equals(codeString)) 1204 return null; 1205 if ("missing".equals(codeString)) 1206 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.MISSING); 1207 if ("exact".equals(codeString)) 1208 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.EXACT); 1209 if ("contains".equals(codeString)) 1210 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.CONTAINS); 1211 if ("not".equals(codeString)) 1212 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.NOT); 1213 if ("text".equals(codeString)) 1214 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.TEXT); 1215 if ("in".equals(codeString)) 1216 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.IN); 1217 if ("not-in".equals(codeString)) 1218 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.NOTIN); 1219 if ("below".equals(codeString)) 1220 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.BELOW); 1221 if ("above".equals(codeString)) 1222 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.ABOVE); 1223 if ("type".equals(codeString)) 1224 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.TYPE); 1225 throw new FHIRException("Unknown SearchModifierCode code '" + codeString + "'"); 1226 } 1227 1228 public String toCode(SearchModifierCode code) { 1229 if (code == SearchModifierCode.MISSING) 1230 return "missing"; 1231 if (code == SearchModifierCode.EXACT) 1232 return "exact"; 1233 if (code == SearchModifierCode.CONTAINS) 1234 return "contains"; 1235 if (code == SearchModifierCode.NOT) 1236 return "not"; 1237 if (code == SearchModifierCode.TEXT) 1238 return "text"; 1239 if (code == SearchModifierCode.IN) 1240 return "in"; 1241 if (code == SearchModifierCode.NOTIN) 1242 return "not-in"; 1243 if (code == SearchModifierCode.BELOW) 1244 return "below"; 1245 if (code == SearchModifierCode.ABOVE) 1246 return "above"; 1247 if (code == SearchModifierCode.TYPE) 1248 return "type"; 1249 return "?"; 1250 } 1251 } 1252 1253 public enum SystemRestfulInteraction { 1254 /** 1255 * null 1256 */ 1257 TRANSACTION, 1258 /** 1259 * null 1260 */ 1261 SEARCHSYSTEM, 1262 /** 1263 * null 1264 */ 1265 HISTORYSYSTEM, 1266 /** 1267 * added to help the parsers 1268 */ 1269 NULL; 1270 1271 public static SystemRestfulInteraction fromCode(String codeString) throws FHIRException { 1272 if (codeString == null || "".equals(codeString)) 1273 return null; 1274 if ("transaction".equals(codeString)) 1275 return TRANSACTION; 1276 if ("search-system".equals(codeString)) 1277 return SEARCHSYSTEM; 1278 if ("history-system".equals(codeString)) 1279 return HISTORYSYSTEM; 1280 throw new FHIRException("Unknown SystemRestfulInteraction code '" + codeString + "'"); 1281 } 1282 1283 public String toCode() { 1284 switch (this) { 1285 case TRANSACTION: 1286 return "transaction"; 1287 case SEARCHSYSTEM: 1288 return "search-system"; 1289 case HISTORYSYSTEM: 1290 return "history-system"; 1291 case NULL: 1292 return null; 1293 default: 1294 return "?"; 1295 } 1296 } 1297 1298 public String getSystem() { 1299 switch (this) { 1300 case TRANSACTION: 1301 return "http://hl7.org/fhir/restful-interaction"; 1302 case SEARCHSYSTEM: 1303 return "http://hl7.org/fhir/restful-interaction"; 1304 case HISTORYSYSTEM: 1305 return "http://hl7.org/fhir/restful-interaction"; 1306 case NULL: 1307 return null; 1308 default: 1309 return "?"; 1310 } 1311 } 1312 1313 public String getDefinition() { 1314 switch (this) { 1315 case TRANSACTION: 1316 return ""; 1317 case SEARCHSYSTEM: 1318 return ""; 1319 case HISTORYSYSTEM: 1320 return ""; 1321 case NULL: 1322 return null; 1323 default: 1324 return "?"; 1325 } 1326 } 1327 1328 public String getDisplay() { 1329 switch (this) { 1330 case TRANSACTION: 1331 return "transaction"; 1332 case SEARCHSYSTEM: 1333 return "search-system"; 1334 case HISTORYSYSTEM: 1335 return "history-system"; 1336 case NULL: 1337 return null; 1338 default: 1339 return "?"; 1340 } 1341 } 1342 } 1343 1344 public static class SystemRestfulInteractionEnumFactory implements EnumFactory<SystemRestfulInteraction> { 1345 public SystemRestfulInteraction fromCode(String codeString) throws IllegalArgumentException { 1346 if (codeString == null || "".equals(codeString)) 1347 if (codeString == null || "".equals(codeString)) 1348 return null; 1349 if ("transaction".equals(codeString)) 1350 return SystemRestfulInteraction.TRANSACTION; 1351 if ("search-system".equals(codeString)) 1352 return SystemRestfulInteraction.SEARCHSYSTEM; 1353 if ("history-system".equals(codeString)) 1354 return SystemRestfulInteraction.HISTORYSYSTEM; 1355 throw new IllegalArgumentException("Unknown SystemRestfulInteraction code '" + codeString + "'"); 1356 } 1357 1358 public Enumeration<SystemRestfulInteraction> fromType(Base code) throws FHIRException { 1359 if (code == null || code.isEmpty()) 1360 return null; 1361 String codeString = ((PrimitiveType) code).asStringValue(); 1362 if (codeString == null || "".equals(codeString)) 1363 return null; 1364 if ("transaction".equals(codeString)) 1365 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.TRANSACTION); 1366 if ("search-system".equals(codeString)) 1367 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.SEARCHSYSTEM); 1368 if ("history-system".equals(codeString)) 1369 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.HISTORYSYSTEM); 1370 throw new FHIRException("Unknown SystemRestfulInteraction code '" + codeString + "'"); 1371 } 1372 1373 public String toCode(SystemRestfulInteraction code) { 1374 if (code == SystemRestfulInteraction.TRANSACTION) 1375 return "transaction"; 1376 if (code == SystemRestfulInteraction.SEARCHSYSTEM) 1377 return "search-system"; 1378 if (code == SystemRestfulInteraction.HISTORYSYSTEM) 1379 return "history-system"; 1380 return "?"; 1381 } 1382 } 1383 1384 public enum TransactionMode { 1385 /** 1386 * Neither batch or transaction is supported. 1387 */ 1388 NOTSUPPORTED, 1389 /** 1390 * Batches are supported. 1391 */ 1392 BATCH, 1393 /** 1394 * Transactions are supported. 1395 */ 1396 TRANSACTION, 1397 /** 1398 * Both batches and transactions are supported. 1399 */ 1400 BOTH, 1401 /** 1402 * added to help the parsers 1403 */ 1404 NULL; 1405 1406 public static TransactionMode fromCode(String codeString) throws FHIRException { 1407 if (codeString == null || "".equals(codeString)) 1408 return null; 1409 if ("not-supported".equals(codeString)) 1410 return NOTSUPPORTED; 1411 if ("batch".equals(codeString)) 1412 return BATCH; 1413 if ("transaction".equals(codeString)) 1414 return TRANSACTION; 1415 if ("both".equals(codeString)) 1416 return BOTH; 1417 throw new FHIRException("Unknown TransactionMode code '" + codeString + "'"); 1418 } 1419 1420 public String toCode() { 1421 switch (this) { 1422 case NOTSUPPORTED: 1423 return "not-supported"; 1424 case BATCH: 1425 return "batch"; 1426 case TRANSACTION: 1427 return "transaction"; 1428 case BOTH: 1429 return "both"; 1430 case NULL: 1431 return null; 1432 default: 1433 return "?"; 1434 } 1435 } 1436 1437 public String getSystem() { 1438 switch (this) { 1439 case NOTSUPPORTED: 1440 return "http://hl7.org/fhir/transaction-mode"; 1441 case BATCH: 1442 return "http://hl7.org/fhir/transaction-mode"; 1443 case TRANSACTION: 1444 return "http://hl7.org/fhir/transaction-mode"; 1445 case BOTH: 1446 return "http://hl7.org/fhir/transaction-mode"; 1447 case NULL: 1448 return null; 1449 default: 1450 return "?"; 1451 } 1452 } 1453 1454 public String getDefinition() { 1455 switch (this) { 1456 case NOTSUPPORTED: 1457 return "Neither batch or transaction is supported."; 1458 case BATCH: 1459 return "Batches are supported."; 1460 case TRANSACTION: 1461 return "Transactions are supported."; 1462 case BOTH: 1463 return "Both batches and transactions are supported."; 1464 case NULL: 1465 return null; 1466 default: 1467 return "?"; 1468 } 1469 } 1470 1471 public String getDisplay() { 1472 switch (this) { 1473 case NOTSUPPORTED: 1474 return "None"; 1475 case BATCH: 1476 return "Batches supported"; 1477 case TRANSACTION: 1478 return "Transactions Supported"; 1479 case BOTH: 1480 return "Batches & Transactions"; 1481 case NULL: 1482 return null; 1483 default: 1484 return "?"; 1485 } 1486 } 1487 } 1488 1489 public static class TransactionModeEnumFactory implements EnumFactory<TransactionMode> { 1490 public TransactionMode fromCode(String codeString) throws IllegalArgumentException { 1491 if (codeString == null || "".equals(codeString)) 1492 if (codeString == null || "".equals(codeString)) 1493 return null; 1494 if ("not-supported".equals(codeString)) 1495 return TransactionMode.NOTSUPPORTED; 1496 if ("batch".equals(codeString)) 1497 return TransactionMode.BATCH; 1498 if ("transaction".equals(codeString)) 1499 return TransactionMode.TRANSACTION; 1500 if ("both".equals(codeString)) 1501 return TransactionMode.BOTH; 1502 throw new IllegalArgumentException("Unknown TransactionMode code '" + codeString + "'"); 1503 } 1504 1505 public Enumeration<TransactionMode> fromType(Base code) throws FHIRException { 1506 if (code == null || code.isEmpty()) 1507 return null; 1508 String codeString = ((PrimitiveType) code).asStringValue(); 1509 if (codeString == null || "".equals(codeString)) 1510 return null; 1511 if ("not-supported".equals(codeString)) 1512 return new Enumeration<TransactionMode>(this, TransactionMode.NOTSUPPORTED); 1513 if ("batch".equals(codeString)) 1514 return new Enumeration<TransactionMode>(this, TransactionMode.BATCH); 1515 if ("transaction".equals(codeString)) 1516 return new Enumeration<TransactionMode>(this, TransactionMode.TRANSACTION); 1517 if ("both".equals(codeString)) 1518 return new Enumeration<TransactionMode>(this, TransactionMode.BOTH); 1519 throw new FHIRException("Unknown TransactionMode code '" + codeString + "'"); 1520 } 1521 1522 public String toCode(TransactionMode code) { 1523 if (code == TransactionMode.NOTSUPPORTED) 1524 return "not-supported"; 1525 if (code == TransactionMode.BATCH) 1526 return "batch"; 1527 if (code == TransactionMode.TRANSACTION) 1528 return "transaction"; 1529 if (code == TransactionMode.BOTH) 1530 return "both"; 1531 return "?"; 1532 } 1533 } 1534 1535 public enum MessageSignificanceCategory { 1536 /** 1537 * The message represents/requests a change that should not be processed more 1538 * than once; e.g. Making a booking for an appointment. 1539 */ 1540 CONSEQUENCE, 1541 /** 1542 * The message represents a response to query for current information. 1543 * Retrospective processing is wrong and/or wasteful. 1544 */ 1545 CURRENCY, 1546 /** 1547 * The content is not necessarily intended to be current, and it can be 1548 * reprocessed, though there may be version issues created by processing old 1549 * notifications. 1550 */ 1551 NOTIFICATION, 1552 /** 1553 * added to help the parsers 1554 */ 1555 NULL; 1556 1557 public static MessageSignificanceCategory fromCode(String codeString) throws FHIRException { 1558 if (codeString == null || "".equals(codeString)) 1559 return null; 1560 if ("Consequence".equals(codeString)) 1561 return CONSEQUENCE; 1562 if ("Currency".equals(codeString)) 1563 return CURRENCY; 1564 if ("Notification".equals(codeString)) 1565 return NOTIFICATION; 1566 throw new FHIRException("Unknown MessageSignificanceCategory code '" + codeString + "'"); 1567 } 1568 1569 public String toCode() { 1570 switch (this) { 1571 case CONSEQUENCE: 1572 return "Consequence"; 1573 case CURRENCY: 1574 return "Currency"; 1575 case NOTIFICATION: 1576 return "Notification"; 1577 case NULL: 1578 return null; 1579 default: 1580 return "?"; 1581 } 1582 } 1583 1584 public String getSystem() { 1585 switch (this) { 1586 case CONSEQUENCE: 1587 return "http://hl7.org/fhir/message-significance-category"; 1588 case CURRENCY: 1589 return "http://hl7.org/fhir/message-significance-category"; 1590 case NOTIFICATION: 1591 return "http://hl7.org/fhir/message-significance-category"; 1592 case NULL: 1593 return null; 1594 default: 1595 return "?"; 1596 } 1597 } 1598 1599 public String getDefinition() { 1600 switch (this) { 1601 case CONSEQUENCE: 1602 return "The message represents/requests a change that should not be processed more than once; e.g. Making a booking for an appointment."; 1603 case CURRENCY: 1604 return "The message represents a response to query for current information. Retrospective processing is wrong and/or wasteful."; 1605 case NOTIFICATION: 1606 return "The content is not necessarily intended to be current, and it can be reprocessed, though there may be version issues created by processing old notifications."; 1607 case NULL: 1608 return null; 1609 default: 1610 return "?"; 1611 } 1612 } 1613 1614 public String getDisplay() { 1615 switch (this) { 1616 case CONSEQUENCE: 1617 return "Consequence"; 1618 case CURRENCY: 1619 return "Currency"; 1620 case NOTIFICATION: 1621 return "Notification"; 1622 case NULL: 1623 return null; 1624 default: 1625 return "?"; 1626 } 1627 } 1628 } 1629 1630 public static class MessageSignificanceCategoryEnumFactory implements EnumFactory<MessageSignificanceCategory> { 1631 public MessageSignificanceCategory fromCode(String codeString) throws IllegalArgumentException { 1632 if (codeString == null || "".equals(codeString)) 1633 if (codeString == null || "".equals(codeString)) 1634 return null; 1635 if ("Consequence".equals(codeString)) 1636 return MessageSignificanceCategory.CONSEQUENCE; 1637 if ("Currency".equals(codeString)) 1638 return MessageSignificanceCategory.CURRENCY; 1639 if ("Notification".equals(codeString)) 1640 return MessageSignificanceCategory.NOTIFICATION; 1641 throw new IllegalArgumentException("Unknown MessageSignificanceCategory code '" + codeString + "'"); 1642 } 1643 1644 public Enumeration<MessageSignificanceCategory> fromType(Base code) throws FHIRException { 1645 if (code == null || code.isEmpty()) 1646 return null; 1647 String codeString = ((PrimitiveType) code).asStringValue(); 1648 if (codeString == null || "".equals(codeString)) 1649 return null; 1650 if ("Consequence".equals(codeString)) 1651 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.CONSEQUENCE); 1652 if ("Currency".equals(codeString)) 1653 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.CURRENCY); 1654 if ("Notification".equals(codeString)) 1655 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.NOTIFICATION); 1656 throw new FHIRException("Unknown MessageSignificanceCategory code '" + codeString + "'"); 1657 } 1658 1659 public String toCode(MessageSignificanceCategory code) { 1660 if (code == MessageSignificanceCategory.CONSEQUENCE) 1661 return "Consequence"; 1662 if (code == MessageSignificanceCategory.CURRENCY) 1663 return "Currency"; 1664 if (code == MessageSignificanceCategory.NOTIFICATION) 1665 return "Notification"; 1666 return "?"; 1667 } 1668 } 1669 1670 public enum ConformanceEventMode { 1671 /** 1672 * The application sends requests and receives responses. 1673 */ 1674 SENDER, 1675 /** 1676 * The application receives requests and sends responses. 1677 */ 1678 RECEIVER, 1679 /** 1680 * added to help the parsers 1681 */ 1682 NULL; 1683 1684 public static ConformanceEventMode fromCode(String codeString) throws FHIRException { 1685 if (codeString == null || "".equals(codeString)) 1686 return null; 1687 if ("sender".equals(codeString)) 1688 return SENDER; 1689 if ("receiver".equals(codeString)) 1690 return RECEIVER; 1691 throw new FHIRException("Unknown ConformanceEventMode code '" + codeString + "'"); 1692 } 1693 1694 public String toCode() { 1695 switch (this) { 1696 case SENDER: 1697 return "sender"; 1698 case RECEIVER: 1699 return "receiver"; 1700 case NULL: 1701 return null; 1702 default: 1703 return "?"; 1704 } 1705 } 1706 1707 public String getSystem() { 1708 switch (this) { 1709 case SENDER: 1710 return "http://hl7.org/fhir/message-conformance-event-mode"; 1711 case RECEIVER: 1712 return "http://hl7.org/fhir/message-conformance-event-mode"; 1713 case NULL: 1714 return null; 1715 default: 1716 return "?"; 1717 } 1718 } 1719 1720 public String getDefinition() { 1721 switch (this) { 1722 case SENDER: 1723 return "The application sends requests and receives responses."; 1724 case RECEIVER: 1725 return "The application receives requests and sends responses."; 1726 case NULL: 1727 return null; 1728 default: 1729 return "?"; 1730 } 1731 } 1732 1733 public String getDisplay() { 1734 switch (this) { 1735 case SENDER: 1736 return "Sender"; 1737 case RECEIVER: 1738 return "Receiver"; 1739 case NULL: 1740 return null; 1741 default: 1742 return "?"; 1743 } 1744 } 1745 } 1746 1747 public static class ConformanceEventModeEnumFactory implements EnumFactory<ConformanceEventMode> { 1748 public ConformanceEventMode fromCode(String codeString) throws IllegalArgumentException { 1749 if (codeString == null || "".equals(codeString)) 1750 if (codeString == null || "".equals(codeString)) 1751 return null; 1752 if ("sender".equals(codeString)) 1753 return ConformanceEventMode.SENDER; 1754 if ("receiver".equals(codeString)) 1755 return ConformanceEventMode.RECEIVER; 1756 throw new IllegalArgumentException("Unknown ConformanceEventMode code '" + codeString + "'"); 1757 } 1758 1759 public Enumeration<ConformanceEventMode> fromType(Base code) throws FHIRException { 1760 if (code == null || code.isEmpty()) 1761 return null; 1762 String codeString = ((PrimitiveType) code).asStringValue(); 1763 if (codeString == null || "".equals(codeString)) 1764 return null; 1765 if ("sender".equals(codeString)) 1766 return new Enumeration<ConformanceEventMode>(this, ConformanceEventMode.SENDER); 1767 if ("receiver".equals(codeString)) 1768 return new Enumeration<ConformanceEventMode>(this, ConformanceEventMode.RECEIVER); 1769 throw new FHIRException("Unknown ConformanceEventMode code '" + codeString + "'"); 1770 } 1771 1772 public String toCode(ConformanceEventMode code) { 1773 if (code == ConformanceEventMode.SENDER) 1774 return "sender"; 1775 if (code == ConformanceEventMode.RECEIVER) 1776 return "receiver"; 1777 return "?"; 1778 } 1779 } 1780 1781 public enum DocumentMode { 1782 /** 1783 * The application produces documents of the specified type. 1784 */ 1785 PRODUCER, 1786 /** 1787 * The application consumes documents of the specified type. 1788 */ 1789 CONSUMER, 1790 /** 1791 * added to help the parsers 1792 */ 1793 NULL; 1794 1795 public static DocumentMode fromCode(String codeString) throws FHIRException { 1796 if (codeString == null || "".equals(codeString)) 1797 return null; 1798 if ("producer".equals(codeString)) 1799 return PRODUCER; 1800 if ("consumer".equals(codeString)) 1801 return CONSUMER; 1802 throw new FHIRException("Unknown DocumentMode code '" + codeString + "'"); 1803 } 1804 1805 public String toCode() { 1806 switch (this) { 1807 case PRODUCER: 1808 return "producer"; 1809 case CONSUMER: 1810 return "consumer"; 1811 case NULL: 1812 return null; 1813 default: 1814 return "?"; 1815 } 1816 } 1817 1818 public String getSystem() { 1819 switch (this) { 1820 case PRODUCER: 1821 return "http://hl7.org/fhir/document-mode"; 1822 case CONSUMER: 1823 return "http://hl7.org/fhir/document-mode"; 1824 case NULL: 1825 return null; 1826 default: 1827 return "?"; 1828 } 1829 } 1830 1831 public String getDefinition() { 1832 switch (this) { 1833 case PRODUCER: 1834 return "The application produces documents of the specified type."; 1835 case CONSUMER: 1836 return "The application consumes documents of the specified type."; 1837 case NULL: 1838 return null; 1839 default: 1840 return "?"; 1841 } 1842 } 1843 1844 public String getDisplay() { 1845 switch (this) { 1846 case PRODUCER: 1847 return "Producer"; 1848 case CONSUMER: 1849 return "Consumer"; 1850 case NULL: 1851 return null; 1852 default: 1853 return "?"; 1854 } 1855 } 1856 } 1857 1858 public static class DocumentModeEnumFactory implements EnumFactory<DocumentMode> { 1859 public DocumentMode fromCode(String codeString) throws IllegalArgumentException { 1860 if (codeString == null || "".equals(codeString)) 1861 if (codeString == null || "".equals(codeString)) 1862 return null; 1863 if ("producer".equals(codeString)) 1864 return DocumentMode.PRODUCER; 1865 if ("consumer".equals(codeString)) 1866 return DocumentMode.CONSUMER; 1867 throw new IllegalArgumentException("Unknown DocumentMode code '" + codeString + "'"); 1868 } 1869 1870 public Enumeration<DocumentMode> fromType(Base code) throws FHIRException { 1871 if (code == null || code.isEmpty()) 1872 return null; 1873 String codeString = ((PrimitiveType) code).asStringValue(); 1874 if (codeString == null || "".equals(codeString)) 1875 return null; 1876 if ("producer".equals(codeString)) 1877 return new Enumeration<DocumentMode>(this, DocumentMode.PRODUCER); 1878 if ("consumer".equals(codeString)) 1879 return new Enumeration<DocumentMode>(this, DocumentMode.CONSUMER); 1880 throw new FHIRException("Unknown DocumentMode code '" + codeString + "'"); 1881 } 1882 1883 public String toCode(DocumentMode code) { 1884 if (code == DocumentMode.PRODUCER) 1885 return "producer"; 1886 if (code == DocumentMode.CONSUMER) 1887 return "consumer"; 1888 return "?"; 1889 } 1890 } 1891 1892 @Block() 1893 public static class ConformanceContactComponent extends BackboneElement implements IBaseBackboneElement { 1894 /** 1895 * The name of an individual to contact regarding the conformance. 1896 */ 1897 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1898 @Description(shortDefinition = "Name of a individual to contact", formalDefinition = "The name of an individual to contact regarding the conformance.") 1899 protected StringType name; 1900 1901 /** 1902 * Contact details for individual (if a name was provided) or the publisher. 1903 */ 1904 @Child(name = "telecom", type = { 1905 ContactPoint.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1906 @Description(shortDefinition = "Contact details for individual or publisher", formalDefinition = "Contact details for individual (if a name was provided) or the publisher.") 1907 protected List<ContactPoint> telecom; 1908 1909 private static final long serialVersionUID = -1179697803L; 1910 1911 /* 1912 * Constructor 1913 */ 1914 public ConformanceContactComponent() { 1915 super(); 1916 } 1917 1918 /** 1919 * @return {@link #name} (The name of an individual to contact regarding the 1920 * conformance.). This is the underlying object with id, value and 1921 * extensions. The accessor "getName" gives direct access to the value 1922 */ 1923 public StringType getNameElement() { 1924 if (this.name == null) 1925 if (Configuration.errorOnAutoCreate()) 1926 throw new Error("Attempt to auto-create ConformanceContactComponent.name"); 1927 else if (Configuration.doAutoCreate()) 1928 this.name = new StringType(); // bb 1929 return this.name; 1930 } 1931 1932 public boolean hasNameElement() { 1933 return this.name != null && !this.name.isEmpty(); 1934 } 1935 1936 public boolean hasName() { 1937 return this.name != null && !this.name.isEmpty(); 1938 } 1939 1940 /** 1941 * @param value {@link #name} (The name of an individual to contact regarding 1942 * the conformance.). This is the underlying object with id, value 1943 * and extensions. The accessor "getName" gives direct access to 1944 * the value 1945 */ 1946 public ConformanceContactComponent setNameElement(StringType value) { 1947 this.name = value; 1948 return this; 1949 } 1950 1951 /** 1952 * @return The name of an individual to contact regarding the conformance. 1953 */ 1954 public String getName() { 1955 return this.name == null ? null : this.name.getValue(); 1956 } 1957 1958 /** 1959 * @param value The name of an individual to contact regarding the conformance. 1960 */ 1961 public ConformanceContactComponent setName(String value) { 1962 if (Utilities.noString(value)) 1963 this.name = null; 1964 else { 1965 if (this.name == null) 1966 this.name = new StringType(); 1967 this.name.setValue(value); 1968 } 1969 return this; 1970 } 1971 1972 /** 1973 * @return {@link #telecom} (Contact details for individual (if a name was 1974 * provided) or the publisher.) 1975 */ 1976 public List<ContactPoint> getTelecom() { 1977 if (this.telecom == null) 1978 this.telecom = new ArrayList<ContactPoint>(); 1979 return this.telecom; 1980 } 1981 1982 public boolean hasTelecom() { 1983 if (this.telecom == null) 1984 return false; 1985 for (ContactPoint item : this.telecom) 1986 if (!item.isEmpty()) 1987 return true; 1988 return false; 1989 } 1990 1991 /** 1992 * @return {@link #telecom} (Contact details for individual (if a name was 1993 * provided) or the publisher.) 1994 */ 1995 // syntactic sugar 1996 public ContactPoint addTelecom() { // 3 1997 ContactPoint t = new ContactPoint(); 1998 if (this.telecom == null) 1999 this.telecom = new ArrayList<ContactPoint>(); 2000 this.telecom.add(t); 2001 return t; 2002 } 2003 2004 // syntactic sugar 2005 public ConformanceContactComponent addTelecom(ContactPoint t) { // 3 2006 if (t == null) 2007 return this; 2008 if (this.telecom == null) 2009 this.telecom = new ArrayList<ContactPoint>(); 2010 this.telecom.add(t); 2011 return this; 2012 } 2013 2014 protected void listChildren(List<Property> childrenList) { 2015 super.listChildren(childrenList); 2016 childrenList.add(new Property("name", "string", "The name of an individual to contact regarding the conformance.", 2017 0, java.lang.Integer.MAX_VALUE, name)); 2018 childrenList.add(new Property("telecom", "ContactPoint", 2019 "Contact details for individual (if a name was provided) or the publisher.", 0, java.lang.Integer.MAX_VALUE, 2020 telecom)); 2021 } 2022 2023 @Override 2024 public void setProperty(String name, Base value) throws FHIRException { 2025 if (name.equals("name")) 2026 this.name = castToString(value); // StringType 2027 else if (name.equals("telecom")) 2028 this.getTelecom().add(castToContactPoint(value)); 2029 else 2030 super.setProperty(name, value); 2031 } 2032 2033 @Override 2034 public Base addChild(String name) throws FHIRException { 2035 if (name.equals("name")) { 2036 throw new FHIRException("Cannot call addChild on a singleton property Conformance.name"); 2037 } else if (name.equals("telecom")) { 2038 return addTelecom(); 2039 } else 2040 return super.addChild(name); 2041 } 2042 2043 public ConformanceContactComponent copy() { 2044 ConformanceContactComponent dst = new ConformanceContactComponent(); 2045 copyValues(dst); 2046 dst.name = name == null ? null : name.copy(); 2047 if (telecom != null) { 2048 dst.telecom = new ArrayList<ContactPoint>(); 2049 for (ContactPoint i : telecom) 2050 dst.telecom.add(i.copy()); 2051 } 2052 ; 2053 return dst; 2054 } 2055 2056 @Override 2057 public boolean equalsDeep(Base other) { 2058 if (!super.equalsDeep(other)) 2059 return false; 2060 if (!(other instanceof ConformanceContactComponent)) 2061 return false; 2062 ConformanceContactComponent o = (ConformanceContactComponent) other; 2063 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 2064 } 2065 2066 @Override 2067 public boolean equalsShallow(Base other) { 2068 if (!super.equalsShallow(other)) 2069 return false; 2070 if (!(other instanceof ConformanceContactComponent)) 2071 return false; 2072 ConformanceContactComponent o = (ConformanceContactComponent) other; 2073 return compareValues(name, o.name, true); 2074 } 2075 2076 public boolean isEmpty() { 2077 return super.isEmpty() && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()); 2078 } 2079 2080 public String fhirType() { 2081 return "Conformance.contact"; 2082 2083 } 2084 2085 } 2086 2087 @Block() 2088 public static class ConformanceSoftwareComponent extends BackboneElement implements IBaseBackboneElement { 2089 /** 2090 * Name software is known by. 2091 */ 2092 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2093 @Description(shortDefinition = "A name the software is known by", formalDefinition = "Name software is known by.") 2094 protected StringType name; 2095 2096 /** 2097 * The version identifier for the software covered by this statement. 2098 */ 2099 @Child(name = "version", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2100 @Description(shortDefinition = "Version covered by this statement", formalDefinition = "The version identifier for the software covered by this statement.") 2101 protected StringType version; 2102 2103 /** 2104 * Date this version of the software released. 2105 */ 2106 @Child(name = "releaseDate", type = { 2107 DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2108 @Description(shortDefinition = "Date this version released", formalDefinition = "Date this version of the software released.") 2109 protected DateTimeType releaseDate; 2110 2111 private static final long serialVersionUID = 1819769027L; 2112 2113 /* 2114 * Constructor 2115 */ 2116 public ConformanceSoftwareComponent() { 2117 super(); 2118 } 2119 2120 /* 2121 * Constructor 2122 */ 2123 public ConformanceSoftwareComponent(StringType name) { 2124 super(); 2125 this.name = name; 2126 } 2127 2128 /** 2129 * @return {@link #name} (Name software is known by.). This is the underlying 2130 * object with id, value and extensions. The accessor "getName" gives 2131 * direct access to the value 2132 */ 2133 public StringType getNameElement() { 2134 if (this.name == null) 2135 if (Configuration.errorOnAutoCreate()) 2136 throw new Error("Attempt to auto-create ConformanceSoftwareComponent.name"); 2137 else if (Configuration.doAutoCreate()) 2138 this.name = new StringType(); // bb 2139 return this.name; 2140 } 2141 2142 public boolean hasNameElement() { 2143 return this.name != null && !this.name.isEmpty(); 2144 } 2145 2146 public boolean hasName() { 2147 return this.name != null && !this.name.isEmpty(); 2148 } 2149 2150 /** 2151 * @param value {@link #name} (Name software is known by.). This is the 2152 * underlying object with id, value and extensions. The accessor 2153 * "getName" gives direct access to the value 2154 */ 2155 public ConformanceSoftwareComponent setNameElement(StringType value) { 2156 this.name = value; 2157 return this; 2158 } 2159 2160 /** 2161 * @return Name software is known by. 2162 */ 2163 public String getName() { 2164 return this.name == null ? null : this.name.getValue(); 2165 } 2166 2167 /** 2168 * @param value Name software is known by. 2169 */ 2170 public ConformanceSoftwareComponent setName(String value) { 2171 if (this.name == null) 2172 this.name = new StringType(); 2173 this.name.setValue(value); 2174 return this; 2175 } 2176 2177 /** 2178 * @return {@link #version} (The version identifier for the software covered by 2179 * this statement.). This is the underlying object with id, value and 2180 * extensions. The accessor "getVersion" gives direct access to the 2181 * value 2182 */ 2183 public StringType getVersionElement() { 2184 if (this.version == null) 2185 if (Configuration.errorOnAutoCreate()) 2186 throw new Error("Attempt to auto-create ConformanceSoftwareComponent.version"); 2187 else if (Configuration.doAutoCreate()) 2188 this.version = new StringType(); // bb 2189 return this.version; 2190 } 2191 2192 public boolean hasVersionElement() { 2193 return this.version != null && !this.version.isEmpty(); 2194 } 2195 2196 public boolean hasVersion() { 2197 return this.version != null && !this.version.isEmpty(); 2198 } 2199 2200 /** 2201 * @param value {@link #version} (The version identifier for the software 2202 * covered by this statement.). This is the underlying object with 2203 * id, value and extensions. The accessor "getVersion" gives direct 2204 * access to the value 2205 */ 2206 public ConformanceSoftwareComponent setVersionElement(StringType value) { 2207 this.version = value; 2208 return this; 2209 } 2210 2211 /** 2212 * @return The version identifier for the software covered by this statement. 2213 */ 2214 public String getVersion() { 2215 return this.version == null ? null : this.version.getValue(); 2216 } 2217 2218 /** 2219 * @param value The version identifier for the software covered by this 2220 * statement. 2221 */ 2222 public ConformanceSoftwareComponent setVersion(String value) { 2223 if (Utilities.noString(value)) 2224 this.version = null; 2225 else { 2226 if (this.version == null) 2227 this.version = new StringType(); 2228 this.version.setValue(value); 2229 } 2230 return this; 2231 } 2232 2233 /** 2234 * @return {@link #releaseDate} (Date this version of the software released.). 2235 * This is the underlying object with id, value and extensions. The 2236 * accessor "getReleaseDate" gives direct access to the value 2237 */ 2238 public DateTimeType getReleaseDateElement() { 2239 if (this.releaseDate == null) 2240 if (Configuration.errorOnAutoCreate()) 2241 throw new Error("Attempt to auto-create ConformanceSoftwareComponent.releaseDate"); 2242 else if (Configuration.doAutoCreate()) 2243 this.releaseDate = new DateTimeType(); // bb 2244 return this.releaseDate; 2245 } 2246 2247 public boolean hasReleaseDateElement() { 2248 return this.releaseDate != null && !this.releaseDate.isEmpty(); 2249 } 2250 2251 public boolean hasReleaseDate() { 2252 return this.releaseDate != null && !this.releaseDate.isEmpty(); 2253 } 2254 2255 /** 2256 * @param value {@link #releaseDate} (Date this version of the software 2257 * released.). This is the underlying object with id, value and 2258 * extensions. The accessor "getReleaseDate" gives direct access to 2259 * the value 2260 */ 2261 public ConformanceSoftwareComponent setReleaseDateElement(DateTimeType value) { 2262 this.releaseDate = value; 2263 return this; 2264 } 2265 2266 /** 2267 * @return Date this version of the software released. 2268 */ 2269 public Date getReleaseDate() { 2270 return this.releaseDate == null ? null : this.releaseDate.getValue(); 2271 } 2272 2273 /** 2274 * @param value Date this version of the software released. 2275 */ 2276 public ConformanceSoftwareComponent setReleaseDate(Date value) { 2277 if (value == null) 2278 this.releaseDate = null; 2279 else { 2280 if (this.releaseDate == null) 2281 this.releaseDate = new DateTimeType(); 2282 this.releaseDate.setValue(value); 2283 } 2284 return this; 2285 } 2286 2287 protected void listChildren(List<Property> childrenList) { 2288 super.listChildren(childrenList); 2289 childrenList 2290 .add(new Property("name", "string", "Name software is known by.", 0, java.lang.Integer.MAX_VALUE, name)); 2291 childrenList 2292 .add(new Property("version", "string", "The version identifier for the software covered by this statement.", 2293 0, java.lang.Integer.MAX_VALUE, version)); 2294 childrenList.add(new Property("releaseDate", "dateTime", "Date this version of the software released.", 0, 2295 java.lang.Integer.MAX_VALUE, releaseDate)); 2296 } 2297 2298 @Override 2299 public void setProperty(String name, Base value) throws FHIRException { 2300 if (name.equals("name")) 2301 this.name = castToString(value); // StringType 2302 else if (name.equals("version")) 2303 this.version = castToString(value); // StringType 2304 else if (name.equals("releaseDate")) 2305 this.releaseDate = castToDateTime(value); // DateTimeType 2306 else 2307 super.setProperty(name, value); 2308 } 2309 2310 @Override 2311 public Base addChild(String name) throws FHIRException { 2312 if (name.equals("name")) { 2313 throw new FHIRException("Cannot call addChild on a singleton property Conformance.name"); 2314 } else if (name.equals("version")) { 2315 throw new FHIRException("Cannot call addChild on a singleton property Conformance.version"); 2316 } else if (name.equals("releaseDate")) { 2317 throw new FHIRException("Cannot call addChild on a singleton property Conformance.releaseDate"); 2318 } else 2319 return super.addChild(name); 2320 } 2321 2322 public ConformanceSoftwareComponent copy() { 2323 ConformanceSoftwareComponent dst = new ConformanceSoftwareComponent(); 2324 copyValues(dst); 2325 dst.name = name == null ? null : name.copy(); 2326 dst.version = version == null ? null : version.copy(); 2327 dst.releaseDate = releaseDate == null ? null : releaseDate.copy(); 2328 return dst; 2329 } 2330 2331 @Override 2332 public boolean equalsDeep(Base other) { 2333 if (!super.equalsDeep(other)) 2334 return false; 2335 if (!(other instanceof ConformanceSoftwareComponent)) 2336 return false; 2337 ConformanceSoftwareComponent o = (ConformanceSoftwareComponent) other; 2338 return compareDeep(name, o.name, true) && compareDeep(version, o.version, true) 2339 && compareDeep(releaseDate, o.releaseDate, true); 2340 } 2341 2342 @Override 2343 public boolean equalsShallow(Base other) { 2344 if (!super.equalsShallow(other)) 2345 return false; 2346 if (!(other instanceof ConformanceSoftwareComponent)) 2347 return false; 2348 ConformanceSoftwareComponent o = (ConformanceSoftwareComponent) other; 2349 return compareValues(name, o.name, true) && compareValues(version, o.version, true) 2350 && compareValues(releaseDate, o.releaseDate, true); 2351 } 2352 2353 public boolean isEmpty() { 2354 return super.isEmpty() && (name == null || name.isEmpty()) && (version == null || version.isEmpty()) 2355 && (releaseDate == null || releaseDate.isEmpty()); 2356 } 2357 2358 public String fhirType() { 2359 return "Conformance.software"; 2360 2361 } 2362 2363 } 2364 2365 @Block() 2366 public static class ConformanceImplementationComponent extends BackboneElement implements IBaseBackboneElement { 2367 /** 2368 * Information about the specific installation that this conformance statement 2369 * relates to. 2370 */ 2371 @Child(name = "description", type = { 2372 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2373 @Description(shortDefinition = "Describes this specific instance", formalDefinition = "Information about the specific installation that this conformance statement relates to.") 2374 protected StringType description; 2375 2376 /** 2377 * An absolute base URL for the implementation. This forms the base for REST 2378 * interfaces as well as the mailbox and document interfaces. 2379 */ 2380 @Child(name = "url", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2381 @Description(shortDefinition = "Base URL for the installation", formalDefinition = "An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.") 2382 protected UriType url; 2383 2384 private static final long serialVersionUID = -289238508L; 2385 2386 /* 2387 * Constructor 2388 */ 2389 public ConformanceImplementationComponent() { 2390 super(); 2391 } 2392 2393 /* 2394 * Constructor 2395 */ 2396 public ConformanceImplementationComponent(StringType description) { 2397 super(); 2398 this.description = description; 2399 } 2400 2401 /** 2402 * @return {@link #description} (Information about the specific installation 2403 * that this conformance statement relates to.). This is the underlying 2404 * object with id, value and extensions. The accessor "getDescription" 2405 * gives direct access to the value 2406 */ 2407 public StringType getDescriptionElement() { 2408 if (this.description == null) 2409 if (Configuration.errorOnAutoCreate()) 2410 throw new Error("Attempt to auto-create ConformanceImplementationComponent.description"); 2411 else if (Configuration.doAutoCreate()) 2412 this.description = new StringType(); // bb 2413 return this.description; 2414 } 2415 2416 public boolean hasDescriptionElement() { 2417 return this.description != null && !this.description.isEmpty(); 2418 } 2419 2420 public boolean hasDescription() { 2421 return this.description != null && !this.description.isEmpty(); 2422 } 2423 2424 /** 2425 * @param value {@link #description} (Information about the specific 2426 * installation that this conformance statement relates to.). This 2427 * is the underlying object with id, value and extensions. The 2428 * accessor "getDescription" gives direct access to the value 2429 */ 2430 public ConformanceImplementationComponent setDescriptionElement(StringType value) { 2431 this.description = value; 2432 return this; 2433 } 2434 2435 /** 2436 * @return Information about the specific installation that this conformance 2437 * statement relates to. 2438 */ 2439 public String getDescription() { 2440 return this.description == null ? null : this.description.getValue(); 2441 } 2442 2443 /** 2444 * @param value Information about the specific installation that this 2445 * conformance statement relates to. 2446 */ 2447 public ConformanceImplementationComponent setDescription(String value) { 2448 if (this.description == null) 2449 this.description = new StringType(); 2450 this.description.setValue(value); 2451 return this; 2452 } 2453 2454 /** 2455 * @return {@link #url} (An absolute base URL for the implementation. This forms 2456 * the base for REST interfaces as well as the mailbox and document 2457 * interfaces.). This is the underlying object with id, value and 2458 * extensions. The accessor "getUrl" gives direct access to the value 2459 */ 2460 public UriType getUrlElement() { 2461 if (this.url == null) 2462 if (Configuration.errorOnAutoCreate()) 2463 throw new Error("Attempt to auto-create ConformanceImplementationComponent.url"); 2464 else if (Configuration.doAutoCreate()) 2465 this.url = new UriType(); // bb 2466 return this.url; 2467 } 2468 2469 public boolean hasUrlElement() { 2470 return this.url != null && !this.url.isEmpty(); 2471 } 2472 2473 public boolean hasUrl() { 2474 return this.url != null && !this.url.isEmpty(); 2475 } 2476 2477 /** 2478 * @param value {@link #url} (An absolute base URL for the implementation. This 2479 * forms the base for REST interfaces as well as the mailbox and 2480 * document interfaces.). This is the underlying object with id, 2481 * value and extensions. The accessor "getUrl" gives direct access 2482 * to the value 2483 */ 2484 public ConformanceImplementationComponent setUrlElement(UriType value) { 2485 this.url = value; 2486 return this; 2487 } 2488 2489 /** 2490 * @return An absolute base URL for the implementation. This forms the base for 2491 * REST interfaces as well as the mailbox and document interfaces. 2492 */ 2493 public String getUrl() { 2494 return this.url == null ? null : this.url.getValue(); 2495 } 2496 2497 /** 2498 * @param value An absolute base URL for the implementation. This forms the base 2499 * for REST interfaces as well as the mailbox and document 2500 * interfaces. 2501 */ 2502 public ConformanceImplementationComponent setUrl(String value) { 2503 if (Utilities.noString(value)) 2504 this.url = null; 2505 else { 2506 if (this.url == null) 2507 this.url = new UriType(); 2508 this.url.setValue(value); 2509 } 2510 return this; 2511 } 2512 2513 protected void listChildren(List<Property> childrenList) { 2514 super.listChildren(childrenList); 2515 childrenList.add(new Property("description", "string", 2516 "Information about the specific installation that this conformance statement relates to.", 0, 2517 java.lang.Integer.MAX_VALUE, description)); 2518 childrenList.add(new Property("url", "uri", 2519 "An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.", 2520 0, java.lang.Integer.MAX_VALUE, url)); 2521 } 2522 2523 @Override 2524 public void setProperty(String name, Base value) throws FHIRException { 2525 if (name.equals("description")) 2526 this.description = castToString(value); // StringType 2527 else if (name.equals("url")) 2528 this.url = castToUri(value); // UriType 2529 else 2530 super.setProperty(name, value); 2531 } 2532 2533 @Override 2534 public Base addChild(String name) throws FHIRException { 2535 if (name.equals("description")) { 2536 throw new FHIRException("Cannot call addChild on a singleton property Conformance.description"); 2537 } else if (name.equals("url")) { 2538 throw new FHIRException("Cannot call addChild on a singleton property Conformance.url"); 2539 } else 2540 return super.addChild(name); 2541 } 2542 2543 public ConformanceImplementationComponent copy() { 2544 ConformanceImplementationComponent dst = new ConformanceImplementationComponent(); 2545 copyValues(dst); 2546 dst.description = description == null ? null : description.copy(); 2547 dst.url = url == null ? null : url.copy(); 2548 return dst; 2549 } 2550 2551 @Override 2552 public boolean equalsDeep(Base other) { 2553 if (!super.equalsDeep(other)) 2554 return false; 2555 if (!(other instanceof ConformanceImplementationComponent)) 2556 return false; 2557 ConformanceImplementationComponent o = (ConformanceImplementationComponent) other; 2558 return compareDeep(description, o.description, true) && compareDeep(url, o.url, true); 2559 } 2560 2561 @Override 2562 public boolean equalsShallow(Base other) { 2563 if (!super.equalsShallow(other)) 2564 return false; 2565 if (!(other instanceof ConformanceImplementationComponent)) 2566 return false; 2567 ConformanceImplementationComponent o = (ConformanceImplementationComponent) other; 2568 return compareValues(description, o.description, true) && compareValues(url, o.url, true); 2569 } 2570 2571 public boolean isEmpty() { 2572 return super.isEmpty() && (description == null || description.isEmpty()) && (url == null || url.isEmpty()); 2573 } 2574 2575 public String fhirType() { 2576 return "Conformance.implementation"; 2577 2578 } 2579 2580 } 2581 2582 @Block() 2583 public static class ConformanceRestComponent extends BackboneElement implements IBaseBackboneElement { 2584 /** 2585 * Identifies whether this portion of the statement is describing ability to 2586 * initiate or receive restful operations. 2587 */ 2588 @Child(name = "mode", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2589 @Description(shortDefinition = "client | server", formalDefinition = "Identifies whether this portion of the statement is describing ability to initiate or receive restful operations.") 2590 protected Enumeration<RestfulConformanceMode> mode; 2591 2592 /** 2593 * Information about the system's restful capabilities that apply across all 2594 * applications, such as security. 2595 */ 2596 @Child(name = "documentation", type = { 2597 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2598 @Description(shortDefinition = "General description of implementation", formalDefinition = "Information about the system's restful capabilities that apply across all applications, such as security.") 2599 protected StringType documentation; 2600 2601 /** 2602 * Information about security implementation from an interface perspective - 2603 * what a client needs to know. 2604 */ 2605 @Child(name = "security", type = {}, order = 3, min = 0, max = 1, modifier = false, summary = false) 2606 @Description(shortDefinition = "Information about security of implementation", formalDefinition = "Information about security implementation from an interface perspective - what a client needs to know.") 2607 protected ConformanceRestSecurityComponent security; 2608 2609 /** 2610 * A specification of the restful capabilities of the solution for a specific 2611 * resource type. 2612 */ 2613 @Child(name = "resource", type = {}, order = 4, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2614 @Description(shortDefinition = "Resource served on the REST interface", formalDefinition = "A specification of the restful capabilities of the solution for a specific resource type.") 2615 protected List<ConformanceRestResourceComponent> resource; 2616 2617 /** 2618 * A specification of restful operations supported by the system. 2619 */ 2620 @Child(name = "interaction", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2621 @Description(shortDefinition = "What operations are supported?", formalDefinition = "A specification of restful operations supported by the system.") 2622 protected List<SystemInteractionComponent> interaction; 2623 2624 /** 2625 * A code that indicates how transactions are supported. 2626 */ 2627 @Child(name = "transactionMode", type = { 2628 CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 2629 @Description(shortDefinition = "not-supported | batch | transaction | both", formalDefinition = "A code that indicates how transactions are supported.") 2630 protected Enumeration<TransactionMode> transactionMode; 2631 2632 /** 2633 * Search parameters that are supported for searching all resources for 2634 * implementations to support and/or make use of - either references to ones 2635 * defined in the specification, or additional ones defined for/by the 2636 * implementation. 2637 */ 2638 @Child(name = "searchParam", type = { 2639 ConformanceRestResourceSearchParamComponent.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2640 @Description(shortDefinition = "Search params for searching all resources", formalDefinition = "Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.") 2641 protected List<ConformanceRestResourceSearchParamComponent> searchParam; 2642 2643 /** 2644 * Definition of an operation or a named query and with its parameters and their 2645 * meaning and type. 2646 */ 2647 @Child(name = "operation", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2648 @Description(shortDefinition = "Definition of an operation or a custom query", formalDefinition = "Definition of an operation or a named query and with its parameters and their meaning and type.") 2649 protected List<ConformanceRestOperationComponent> operation; 2650 2651 /** 2652 * An absolute URI which is a reference to the definition of a compartment 2653 * hosted by the system. 2654 */ 2655 @Child(name = "compartment", type = { 2656 UriType.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2657 @Description(shortDefinition = "Compartments served/used by system", formalDefinition = "An absolute URI which is a reference to the definition of a compartment hosted by the system.") 2658 protected List<UriType> compartment; 2659 2660 private static final long serialVersionUID = 931983837L; 2661 2662 /* 2663 * Constructor 2664 */ 2665 public ConformanceRestComponent() { 2666 super(); 2667 } 2668 2669 /* 2670 * Constructor 2671 */ 2672 public ConformanceRestComponent(Enumeration<RestfulConformanceMode> mode) { 2673 super(); 2674 this.mode = mode; 2675 } 2676 2677 /** 2678 * @return {@link #mode} (Identifies whether this portion of the statement is 2679 * describing ability to initiate or receive restful operations.). This 2680 * is the underlying object with id, value and extensions. The accessor 2681 * "getMode" gives direct access to the value 2682 */ 2683 public Enumeration<RestfulConformanceMode> getModeElement() { 2684 if (this.mode == null) 2685 if (Configuration.errorOnAutoCreate()) 2686 throw new Error("Attempt to auto-create ConformanceRestComponent.mode"); 2687 else if (Configuration.doAutoCreate()) 2688 this.mode = new Enumeration<RestfulConformanceMode>(new RestfulConformanceModeEnumFactory()); // bb 2689 return this.mode; 2690 } 2691 2692 public boolean hasModeElement() { 2693 return this.mode != null && !this.mode.isEmpty(); 2694 } 2695 2696 public boolean hasMode() { 2697 return this.mode != null && !this.mode.isEmpty(); 2698 } 2699 2700 /** 2701 * @param value {@link #mode} (Identifies whether this portion of the statement 2702 * is describing ability to initiate or receive restful 2703 * operations.). This is the underlying object with id, value and 2704 * extensions. The accessor "getMode" gives direct access to the 2705 * value 2706 */ 2707 public ConformanceRestComponent setModeElement(Enumeration<RestfulConformanceMode> value) { 2708 this.mode = value; 2709 return this; 2710 } 2711 2712 /** 2713 * @return Identifies whether this portion of the statement is describing 2714 * ability to initiate or receive restful operations. 2715 */ 2716 public RestfulConformanceMode getMode() { 2717 return this.mode == null ? null : this.mode.getValue(); 2718 } 2719 2720 /** 2721 * @param value Identifies whether this portion of the statement is describing 2722 * ability to initiate or receive restful operations. 2723 */ 2724 public ConformanceRestComponent setMode(RestfulConformanceMode value) { 2725 if (this.mode == null) 2726 this.mode = new Enumeration<RestfulConformanceMode>(new RestfulConformanceModeEnumFactory()); 2727 this.mode.setValue(value); 2728 return this; 2729 } 2730 2731 /** 2732 * @return {@link #documentation} (Information about the system's restful 2733 * capabilities that apply across all applications, such as security.). 2734 * This is the underlying object with id, value and extensions. The 2735 * accessor "getDocumentation" gives direct access to the value 2736 */ 2737 public StringType getDocumentationElement() { 2738 if (this.documentation == null) 2739 if (Configuration.errorOnAutoCreate()) 2740 throw new Error("Attempt to auto-create ConformanceRestComponent.documentation"); 2741 else if (Configuration.doAutoCreate()) 2742 this.documentation = new StringType(); // bb 2743 return this.documentation; 2744 } 2745 2746 public boolean hasDocumentationElement() { 2747 return this.documentation != null && !this.documentation.isEmpty(); 2748 } 2749 2750 public boolean hasDocumentation() { 2751 return this.documentation != null && !this.documentation.isEmpty(); 2752 } 2753 2754 /** 2755 * @param value {@link #documentation} (Information about the system's restful 2756 * capabilities that apply across all applications, such as 2757 * security.). This is the underlying object with id, value and 2758 * extensions. The accessor "getDocumentation" gives direct access 2759 * to the value 2760 */ 2761 public ConformanceRestComponent setDocumentationElement(StringType value) { 2762 this.documentation = value; 2763 return this; 2764 } 2765 2766 /** 2767 * @return Information about the system's restful capabilities that apply across 2768 * all applications, such as security. 2769 */ 2770 public String getDocumentation() { 2771 return this.documentation == null ? null : this.documentation.getValue(); 2772 } 2773 2774 /** 2775 * @param value Information about the system's restful capabilities that apply 2776 * across all applications, such as security. 2777 */ 2778 public ConformanceRestComponent setDocumentation(String value) { 2779 if (Utilities.noString(value)) 2780 this.documentation = null; 2781 else { 2782 if (this.documentation == null) 2783 this.documentation = new StringType(); 2784 this.documentation.setValue(value); 2785 } 2786 return this; 2787 } 2788 2789 /** 2790 * @return {@link #security} (Information about security implementation from an 2791 * interface perspective - what a client needs to know.) 2792 */ 2793 public ConformanceRestSecurityComponent getSecurity() { 2794 if (this.security == null) 2795 if (Configuration.errorOnAutoCreate()) 2796 throw new Error("Attempt to auto-create ConformanceRestComponent.security"); 2797 else if (Configuration.doAutoCreate()) 2798 this.security = new ConformanceRestSecurityComponent(); // cc 2799 return this.security; 2800 } 2801 2802 public boolean hasSecurity() { 2803 return this.security != null && !this.security.isEmpty(); 2804 } 2805 2806 /** 2807 * @param value {@link #security} (Information about security implementation 2808 * from an interface perspective - what a client needs to know.) 2809 */ 2810 public ConformanceRestComponent setSecurity(ConformanceRestSecurityComponent value) { 2811 this.security = value; 2812 return this; 2813 } 2814 2815 /** 2816 * @return {@link #resource} (A specification of the restful capabilities of the 2817 * solution for a specific resource type.) 2818 */ 2819 public List<ConformanceRestResourceComponent> getResource() { 2820 if (this.resource == null) 2821 this.resource = new ArrayList<ConformanceRestResourceComponent>(); 2822 return this.resource; 2823 } 2824 2825 public boolean hasResource() { 2826 if (this.resource == null) 2827 return false; 2828 for (ConformanceRestResourceComponent item : this.resource) 2829 if (!item.isEmpty()) 2830 return true; 2831 return false; 2832 } 2833 2834 /** 2835 * @return {@link #resource} (A specification of the restful capabilities of the 2836 * solution for a specific resource type.) 2837 */ 2838 // syntactic sugar 2839 public ConformanceRestResourceComponent addResource() { // 3 2840 ConformanceRestResourceComponent t = new ConformanceRestResourceComponent(); 2841 if (this.resource == null) 2842 this.resource = new ArrayList<ConformanceRestResourceComponent>(); 2843 this.resource.add(t); 2844 return t; 2845 } 2846 2847 // syntactic sugar 2848 public ConformanceRestComponent addResource(ConformanceRestResourceComponent t) { // 3 2849 if (t == null) 2850 return this; 2851 if (this.resource == null) 2852 this.resource = new ArrayList<ConformanceRestResourceComponent>(); 2853 this.resource.add(t); 2854 return this; 2855 } 2856 2857 /** 2858 * @return {@link #interaction} (A specification of restful operations supported 2859 * by the system.) 2860 */ 2861 public List<SystemInteractionComponent> getInteraction() { 2862 if (this.interaction == null) 2863 this.interaction = new ArrayList<SystemInteractionComponent>(); 2864 return this.interaction; 2865 } 2866 2867 public boolean hasInteraction() { 2868 if (this.interaction == null) 2869 return false; 2870 for (SystemInteractionComponent item : this.interaction) 2871 if (!item.isEmpty()) 2872 return true; 2873 return false; 2874 } 2875 2876 /** 2877 * @return {@link #interaction} (A specification of restful operations supported 2878 * by the system.) 2879 */ 2880 // syntactic sugar 2881 public SystemInteractionComponent addInteraction() { // 3 2882 SystemInteractionComponent t = new SystemInteractionComponent(); 2883 if (this.interaction == null) 2884 this.interaction = new ArrayList<SystemInteractionComponent>(); 2885 this.interaction.add(t); 2886 return t; 2887 } 2888 2889 // syntactic sugar 2890 public ConformanceRestComponent addInteraction(SystemInteractionComponent t) { // 3 2891 if (t == null) 2892 return this; 2893 if (this.interaction == null) 2894 this.interaction = new ArrayList<SystemInteractionComponent>(); 2895 this.interaction.add(t); 2896 return this; 2897 } 2898 2899 /** 2900 * @return {@link #transactionMode} (A code that indicates how transactions are 2901 * supported.). This is the underlying object with id, value and 2902 * extensions. The accessor "getTransactionMode" gives direct access to 2903 * the value 2904 */ 2905 public Enumeration<TransactionMode> getTransactionModeElement() { 2906 if (this.transactionMode == null) 2907 if (Configuration.errorOnAutoCreate()) 2908 throw new Error("Attempt to auto-create ConformanceRestComponent.transactionMode"); 2909 else if (Configuration.doAutoCreate()) 2910 this.transactionMode = new Enumeration<TransactionMode>(new TransactionModeEnumFactory()); // bb 2911 return this.transactionMode; 2912 } 2913 2914 public boolean hasTransactionModeElement() { 2915 return this.transactionMode != null && !this.transactionMode.isEmpty(); 2916 } 2917 2918 public boolean hasTransactionMode() { 2919 return this.transactionMode != null && !this.transactionMode.isEmpty(); 2920 } 2921 2922 /** 2923 * @param value {@link #transactionMode} (A code that indicates how transactions 2924 * are supported.). This is the underlying object with id, value 2925 * and extensions. The accessor "getTransactionMode" gives direct 2926 * access to the value 2927 */ 2928 public ConformanceRestComponent setTransactionModeElement(Enumeration<TransactionMode> value) { 2929 this.transactionMode = value; 2930 return this; 2931 } 2932 2933 /** 2934 * @return A code that indicates how transactions are supported. 2935 */ 2936 public TransactionMode getTransactionMode() { 2937 return this.transactionMode == null ? null : this.transactionMode.getValue(); 2938 } 2939 2940 /** 2941 * @param value A code that indicates how transactions are supported. 2942 */ 2943 public ConformanceRestComponent setTransactionMode(TransactionMode value) { 2944 if (value == null) 2945 this.transactionMode = null; 2946 else { 2947 if (this.transactionMode == null) 2948 this.transactionMode = new Enumeration<TransactionMode>(new TransactionModeEnumFactory()); 2949 this.transactionMode.setValue(value); 2950 } 2951 return this; 2952 } 2953 2954 /** 2955 * @return {@link #searchParam} (Search parameters that are supported for 2956 * searching all resources for implementations to support and/or make 2957 * use of - either references to ones defined in the specification, or 2958 * additional ones defined for/by the implementation.) 2959 */ 2960 public List<ConformanceRestResourceSearchParamComponent> getSearchParam() { 2961 if (this.searchParam == null) 2962 this.searchParam = new ArrayList<ConformanceRestResourceSearchParamComponent>(); 2963 return this.searchParam; 2964 } 2965 2966 public boolean hasSearchParam() { 2967 if (this.searchParam == null) 2968 return false; 2969 for (ConformanceRestResourceSearchParamComponent item : this.searchParam) 2970 if (!item.isEmpty()) 2971 return true; 2972 return false; 2973 } 2974 2975 /** 2976 * @return {@link #searchParam} (Search parameters that are supported for 2977 * searching all resources for implementations to support and/or make 2978 * use of - either references to ones defined in the specification, or 2979 * additional ones defined for/by the implementation.) 2980 */ 2981 // syntactic sugar 2982 public ConformanceRestResourceSearchParamComponent addSearchParam() { // 3 2983 ConformanceRestResourceSearchParamComponent t = new ConformanceRestResourceSearchParamComponent(); 2984 if (this.searchParam == null) 2985 this.searchParam = new ArrayList<ConformanceRestResourceSearchParamComponent>(); 2986 this.searchParam.add(t); 2987 return t; 2988 } 2989 2990 // syntactic sugar 2991 public ConformanceRestComponent addSearchParam(ConformanceRestResourceSearchParamComponent t) { // 3 2992 if (t == null) 2993 return this; 2994 if (this.searchParam == null) 2995 this.searchParam = new ArrayList<ConformanceRestResourceSearchParamComponent>(); 2996 this.searchParam.add(t); 2997 return this; 2998 } 2999 3000 /** 3001 * @return {@link #operation} (Definition of an operation or a named query and 3002 * with its parameters and their meaning and type.) 3003 */ 3004 public List<ConformanceRestOperationComponent> getOperation() { 3005 if (this.operation == null) 3006 this.operation = new ArrayList<ConformanceRestOperationComponent>(); 3007 return this.operation; 3008 } 3009 3010 public boolean hasOperation() { 3011 if (this.operation == null) 3012 return false; 3013 for (ConformanceRestOperationComponent item : this.operation) 3014 if (!item.isEmpty()) 3015 return true; 3016 return false; 3017 } 3018 3019 /** 3020 * @return {@link #operation} (Definition of an operation or a named query and 3021 * with its parameters and their meaning and type.) 3022 */ 3023 // syntactic sugar 3024 public ConformanceRestOperationComponent addOperation() { // 3 3025 ConformanceRestOperationComponent t = new ConformanceRestOperationComponent(); 3026 if (this.operation == null) 3027 this.operation = new ArrayList<ConformanceRestOperationComponent>(); 3028 this.operation.add(t); 3029 return t; 3030 } 3031 3032 // syntactic sugar 3033 public ConformanceRestComponent addOperation(ConformanceRestOperationComponent t) { // 3 3034 if (t == null) 3035 return this; 3036 if (this.operation == null) 3037 this.operation = new ArrayList<ConformanceRestOperationComponent>(); 3038 this.operation.add(t); 3039 return this; 3040 } 3041 3042 /** 3043 * @return {@link #compartment} (An absolute URI which is a reference to the 3044 * definition of a compartment hosted by the system.) 3045 */ 3046 public List<UriType> getCompartment() { 3047 if (this.compartment == null) 3048 this.compartment = new ArrayList<UriType>(); 3049 return this.compartment; 3050 } 3051 3052 public boolean hasCompartment() { 3053 if (this.compartment == null) 3054 return false; 3055 for (UriType item : this.compartment) 3056 if (!item.isEmpty()) 3057 return true; 3058 return false; 3059 } 3060 3061 /** 3062 * @return {@link #compartment} (An absolute URI which is a reference to the 3063 * definition of a compartment hosted by the system.) 3064 */ 3065 // syntactic sugar 3066 public UriType addCompartmentElement() {// 2 3067 UriType t = new UriType(); 3068 if (this.compartment == null) 3069 this.compartment = new ArrayList<UriType>(); 3070 this.compartment.add(t); 3071 return t; 3072 } 3073 3074 /** 3075 * @param value {@link #compartment} (An absolute URI which is a reference to 3076 * the definition of a compartment hosted by the system.) 3077 */ 3078 public ConformanceRestComponent addCompartment(String value) { // 1 3079 UriType t = new UriType(); 3080 t.setValue(value); 3081 if (this.compartment == null) 3082 this.compartment = new ArrayList<UriType>(); 3083 this.compartment.add(t); 3084 return this; 3085 } 3086 3087 /** 3088 * @param value {@link #compartment} (An absolute URI which is a reference to 3089 * the definition of a compartment hosted by the system.) 3090 */ 3091 public boolean hasCompartment(String value) { 3092 if (this.compartment == null) 3093 return false; 3094 for (UriType v : this.compartment) 3095 if (v.equals(value)) // uri 3096 return true; 3097 return false; 3098 } 3099 3100 protected void listChildren(List<Property> childrenList) { 3101 super.listChildren(childrenList); 3102 childrenList.add(new Property("mode", "code", 3103 "Identifies whether this portion of the statement is describing ability to initiate or receive restful operations.", 3104 0, java.lang.Integer.MAX_VALUE, mode)); 3105 childrenList.add(new Property("documentation", "string", 3106 "Information about the system's restful capabilities that apply across all applications, such as security.", 3107 0, java.lang.Integer.MAX_VALUE, documentation)); 3108 childrenList.add(new Property("security", "", 3109 "Information about security implementation from an interface perspective - what a client needs to know.", 0, 3110 java.lang.Integer.MAX_VALUE, security)); 3111 childrenList.add(new Property("resource", "", 3112 "A specification of the restful capabilities of the solution for a specific resource type.", 0, 3113 java.lang.Integer.MAX_VALUE, resource)); 3114 childrenList.add(new Property("interaction", "", "A specification of restful operations supported by the system.", 3115 0, java.lang.Integer.MAX_VALUE, interaction)); 3116 childrenList.add(new Property("transactionMode", "code", "A code that indicates how transactions are supported.", 3117 0, java.lang.Integer.MAX_VALUE, transactionMode)); 3118 childrenList.add(new Property("searchParam", "@Conformance.rest.resource.searchParam", 3119 "Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 3120 0, java.lang.Integer.MAX_VALUE, searchParam)); 3121 childrenList.add(new Property("operation", "", 3122 "Definition of an operation or a named query and with its parameters and their meaning and type.", 0, 3123 java.lang.Integer.MAX_VALUE, operation)); 3124 childrenList.add(new Property("compartment", "uri", 3125 "An absolute URI which is a reference to the definition of a compartment hosted by the system.", 0, 3126 java.lang.Integer.MAX_VALUE, compartment)); 3127 } 3128 3129 @Override 3130 public void setProperty(String name, Base value) throws FHIRException { 3131 if (name.equals("mode")) 3132 this.mode = new RestfulConformanceModeEnumFactory().fromType(value); // Enumeration<RestfulConformanceMode> 3133 else if (name.equals("documentation")) 3134 this.documentation = castToString(value); // StringType 3135 else if (name.equals("security")) 3136 this.security = (ConformanceRestSecurityComponent) value; // ConformanceRestSecurityComponent 3137 else if (name.equals("resource")) 3138 this.getResource().add((ConformanceRestResourceComponent) value); 3139 else if (name.equals("interaction")) 3140 this.getInteraction().add((SystemInteractionComponent) value); 3141 else if (name.equals("transactionMode")) 3142 this.transactionMode = new TransactionModeEnumFactory().fromType(value); // Enumeration<TransactionMode> 3143 else if (name.equals("searchParam")) 3144 this.getSearchParam().add((ConformanceRestResourceSearchParamComponent) value); 3145 else if (name.equals("operation")) 3146 this.getOperation().add((ConformanceRestOperationComponent) value); 3147 else if (name.equals("compartment")) 3148 this.getCompartment().add(castToUri(value)); 3149 else 3150 super.setProperty(name, value); 3151 } 3152 3153 @Override 3154 public Base addChild(String name) throws FHIRException { 3155 if (name.equals("mode")) { 3156 throw new FHIRException("Cannot call addChild on a singleton property Conformance.mode"); 3157 } else if (name.equals("documentation")) { 3158 throw new FHIRException("Cannot call addChild on a singleton property Conformance.documentation"); 3159 } else if (name.equals("security")) { 3160 this.security = new ConformanceRestSecurityComponent(); 3161 return this.security; 3162 } else if (name.equals("resource")) { 3163 return addResource(); 3164 } else if (name.equals("interaction")) { 3165 return addInteraction(); 3166 } else if (name.equals("transactionMode")) { 3167 throw new FHIRException("Cannot call addChild on a singleton property Conformance.transactionMode"); 3168 } else if (name.equals("searchParam")) { 3169 return addSearchParam(); 3170 } else if (name.equals("operation")) { 3171 return addOperation(); 3172 } else if (name.equals("compartment")) { 3173 throw new FHIRException("Cannot call addChild on a singleton property Conformance.compartment"); 3174 } else 3175 return super.addChild(name); 3176 } 3177 3178 public ConformanceRestComponent copy() { 3179 ConformanceRestComponent dst = new ConformanceRestComponent(); 3180 copyValues(dst); 3181 dst.mode = mode == null ? null : mode.copy(); 3182 dst.documentation = documentation == null ? null : documentation.copy(); 3183 dst.security = security == null ? null : security.copy(); 3184 if (resource != null) { 3185 dst.resource = new ArrayList<ConformanceRestResourceComponent>(); 3186 for (ConformanceRestResourceComponent i : resource) 3187 dst.resource.add(i.copy()); 3188 } 3189 ; 3190 if (interaction != null) { 3191 dst.interaction = new ArrayList<SystemInteractionComponent>(); 3192 for (SystemInteractionComponent i : interaction) 3193 dst.interaction.add(i.copy()); 3194 } 3195 ; 3196 dst.transactionMode = transactionMode == null ? null : transactionMode.copy(); 3197 if (searchParam != null) { 3198 dst.searchParam = new ArrayList<ConformanceRestResourceSearchParamComponent>(); 3199 for (ConformanceRestResourceSearchParamComponent i : searchParam) 3200 dst.searchParam.add(i.copy()); 3201 } 3202 ; 3203 if (operation != null) { 3204 dst.operation = new ArrayList<ConformanceRestOperationComponent>(); 3205 for (ConformanceRestOperationComponent i : operation) 3206 dst.operation.add(i.copy()); 3207 } 3208 ; 3209 if (compartment != null) { 3210 dst.compartment = new ArrayList<UriType>(); 3211 for (UriType i : compartment) 3212 dst.compartment.add(i.copy()); 3213 } 3214 ; 3215 return dst; 3216 } 3217 3218 @Override 3219 public boolean equalsDeep(Base other) { 3220 if (!super.equalsDeep(other)) 3221 return false; 3222 if (!(other instanceof ConformanceRestComponent)) 3223 return false; 3224 ConformanceRestComponent o = (ConformanceRestComponent) other; 3225 return compareDeep(mode, o.mode, true) && compareDeep(documentation, o.documentation, true) 3226 && compareDeep(security, o.security, true) && compareDeep(resource, o.resource, true) 3227 && compareDeep(interaction, o.interaction, true) && compareDeep(transactionMode, o.transactionMode, true) 3228 && compareDeep(searchParam, o.searchParam, true) && compareDeep(operation, o.operation, true) 3229 && compareDeep(compartment, o.compartment, true); 3230 } 3231 3232 @Override 3233 public boolean equalsShallow(Base other) { 3234 if (!super.equalsShallow(other)) 3235 return false; 3236 if (!(other instanceof ConformanceRestComponent)) 3237 return false; 3238 ConformanceRestComponent o = (ConformanceRestComponent) other; 3239 return compareValues(mode, o.mode, true) && compareValues(documentation, o.documentation, true) 3240 && compareValues(transactionMode, o.transactionMode, true) && compareValues(compartment, o.compartment, true); 3241 } 3242 3243 public boolean isEmpty() { 3244 return super.isEmpty() && (mode == null || mode.isEmpty()) && (documentation == null || documentation.isEmpty()) 3245 && (security == null || security.isEmpty()) && (resource == null || resource.isEmpty()) 3246 && (interaction == null || interaction.isEmpty()) && (transactionMode == null || transactionMode.isEmpty()) 3247 && (searchParam == null || searchParam.isEmpty()) && (operation == null || operation.isEmpty()) 3248 && (compartment == null || compartment.isEmpty()); 3249 } 3250 3251 public String fhirType() { 3252 return "Conformance.rest"; 3253 3254 } 3255 3256 } 3257 3258 @Block() 3259 public static class ConformanceRestSecurityComponent extends BackboneElement implements IBaseBackboneElement { 3260 /** 3261 * Server adds CORS headers when responding to requests - this enables 3262 * javascript applications to use the server. 3263 */ 3264 @Child(name = "cors", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 3265 @Description(shortDefinition = "Adds CORS Headers (http://enable-cors.org/)", formalDefinition = "Server adds CORS headers when responding to requests - this enables javascript applications to use the server.") 3266 protected BooleanType cors; 3267 3268 /** 3269 * Types of security services are supported/required by the system. 3270 */ 3271 @Child(name = "service", type = { 3272 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3273 @Description(shortDefinition = "OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | Certificates", formalDefinition = "Types of security services are supported/required by the system.") 3274 protected List<CodeableConcept> service; 3275 3276 /** 3277 * General description of how security works. 3278 */ 3279 @Child(name = "description", type = { 3280 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3281 @Description(shortDefinition = "General description of how security works", formalDefinition = "General description of how security works.") 3282 protected StringType description; 3283 3284 /** 3285 * Certificates associated with security profiles. 3286 */ 3287 @Child(name = "certificate", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3288 @Description(shortDefinition = "Certificates associated with security profiles", formalDefinition = "Certificates associated with security profiles.") 3289 protected List<ConformanceRestSecurityCertificateComponent> certificate; 3290 3291 private static final long serialVersionUID = 391663952L; 3292 3293 /* 3294 * Constructor 3295 */ 3296 public ConformanceRestSecurityComponent() { 3297 super(); 3298 } 3299 3300 /** 3301 * @return {@link #cors} (Server adds CORS headers when responding to requests - 3302 * this enables javascript applications to use the server.). This is the 3303 * underlying object with id, value and extensions. The accessor 3304 * "getCors" gives direct access to the value 3305 */ 3306 public BooleanType getCorsElement() { 3307 if (this.cors == null) 3308 if (Configuration.errorOnAutoCreate()) 3309 throw new Error("Attempt to auto-create ConformanceRestSecurityComponent.cors"); 3310 else if (Configuration.doAutoCreate()) 3311 this.cors = new BooleanType(); // bb 3312 return this.cors; 3313 } 3314 3315 public boolean hasCorsElement() { 3316 return this.cors != null && !this.cors.isEmpty(); 3317 } 3318 3319 public boolean hasCors() { 3320 return this.cors != null && !this.cors.isEmpty(); 3321 } 3322 3323 /** 3324 * @param value {@link #cors} (Server adds CORS headers when responding to 3325 * requests - this enables javascript applications to use the 3326 * server.). This is the underlying object with id, value and 3327 * extensions. The accessor "getCors" gives direct access to the 3328 * value 3329 */ 3330 public ConformanceRestSecurityComponent setCorsElement(BooleanType value) { 3331 this.cors = value; 3332 return this; 3333 } 3334 3335 /** 3336 * @return Server adds CORS headers when responding to requests - this enables 3337 * javascript applications to use the server. 3338 */ 3339 public boolean getCors() { 3340 return this.cors == null || this.cors.isEmpty() ? false : this.cors.getValue(); 3341 } 3342 3343 /** 3344 * @param value Server adds CORS headers when responding to requests - this 3345 * enables javascript applications to use the server. 3346 */ 3347 public ConformanceRestSecurityComponent setCors(boolean value) { 3348 if (this.cors == null) 3349 this.cors = new BooleanType(); 3350 this.cors.setValue(value); 3351 return this; 3352 } 3353 3354 /** 3355 * @return {@link #service} (Types of security services are supported/required 3356 * by the system.) 3357 */ 3358 public List<CodeableConcept> getService() { 3359 if (this.service == null) 3360 this.service = new ArrayList<CodeableConcept>(); 3361 return this.service; 3362 } 3363 3364 public boolean hasService() { 3365 if (this.service == null) 3366 return false; 3367 for (CodeableConcept item : this.service) 3368 if (!item.isEmpty()) 3369 return true; 3370 return false; 3371 } 3372 3373 /** 3374 * @return {@link #service} (Types of security services are supported/required 3375 * by the system.) 3376 */ 3377 // syntactic sugar 3378 public CodeableConcept addService() { // 3 3379 CodeableConcept t = new CodeableConcept(); 3380 if (this.service == null) 3381 this.service = new ArrayList<CodeableConcept>(); 3382 this.service.add(t); 3383 return t; 3384 } 3385 3386 // syntactic sugar 3387 public ConformanceRestSecurityComponent addService(CodeableConcept t) { // 3 3388 if (t == null) 3389 return this; 3390 if (this.service == null) 3391 this.service = new ArrayList<CodeableConcept>(); 3392 this.service.add(t); 3393 return this; 3394 } 3395 3396 /** 3397 * @return {@link #description} (General description of how security works.). 3398 * This is the underlying object with id, value and extensions. The 3399 * accessor "getDescription" gives direct access to the value 3400 */ 3401 public StringType getDescriptionElement() { 3402 if (this.description == null) 3403 if (Configuration.errorOnAutoCreate()) 3404 throw new Error("Attempt to auto-create ConformanceRestSecurityComponent.description"); 3405 else if (Configuration.doAutoCreate()) 3406 this.description = new StringType(); // bb 3407 return this.description; 3408 } 3409 3410 public boolean hasDescriptionElement() { 3411 return this.description != null && !this.description.isEmpty(); 3412 } 3413 3414 public boolean hasDescription() { 3415 return this.description != null && !this.description.isEmpty(); 3416 } 3417 3418 /** 3419 * @param value {@link #description} (General description of how security 3420 * works.). This is the underlying object with id, value and 3421 * extensions. The accessor "getDescription" gives direct access to 3422 * the value 3423 */ 3424 public ConformanceRestSecurityComponent setDescriptionElement(StringType value) { 3425 this.description = value; 3426 return this; 3427 } 3428 3429 /** 3430 * @return General description of how security works. 3431 */ 3432 public String getDescription() { 3433 return this.description == null ? null : this.description.getValue(); 3434 } 3435 3436 /** 3437 * @param value General description of how security works. 3438 */ 3439 public ConformanceRestSecurityComponent setDescription(String value) { 3440 if (Utilities.noString(value)) 3441 this.description = null; 3442 else { 3443 if (this.description == null) 3444 this.description = new StringType(); 3445 this.description.setValue(value); 3446 } 3447 return this; 3448 } 3449 3450 /** 3451 * @return {@link #certificate} (Certificates associated with security 3452 * profiles.) 3453 */ 3454 public List<ConformanceRestSecurityCertificateComponent> getCertificate() { 3455 if (this.certificate == null) 3456 this.certificate = new ArrayList<ConformanceRestSecurityCertificateComponent>(); 3457 return this.certificate; 3458 } 3459 3460 public boolean hasCertificate() { 3461 if (this.certificate == null) 3462 return false; 3463 for (ConformanceRestSecurityCertificateComponent item : this.certificate) 3464 if (!item.isEmpty()) 3465 return true; 3466 return false; 3467 } 3468 3469 /** 3470 * @return {@link #certificate} (Certificates associated with security 3471 * profiles.) 3472 */ 3473 // syntactic sugar 3474 public ConformanceRestSecurityCertificateComponent addCertificate() { // 3 3475 ConformanceRestSecurityCertificateComponent t = new ConformanceRestSecurityCertificateComponent(); 3476 if (this.certificate == null) 3477 this.certificate = new ArrayList<ConformanceRestSecurityCertificateComponent>(); 3478 this.certificate.add(t); 3479 return t; 3480 } 3481 3482 // syntactic sugar 3483 public ConformanceRestSecurityComponent addCertificate(ConformanceRestSecurityCertificateComponent t) { // 3 3484 if (t == null) 3485 return this; 3486 if (this.certificate == null) 3487 this.certificate = new ArrayList<ConformanceRestSecurityCertificateComponent>(); 3488 this.certificate.add(t); 3489 return this; 3490 } 3491 3492 protected void listChildren(List<Property> childrenList) { 3493 super.listChildren(childrenList); 3494 childrenList.add(new Property("cors", "boolean", 3495 "Server adds CORS headers when responding to requests - this enables javascript applications to use the server.", 3496 0, java.lang.Integer.MAX_VALUE, cors)); 3497 childrenList.add(new Property("service", "CodeableConcept", 3498 "Types of security services are supported/required by the system.", 0, java.lang.Integer.MAX_VALUE, service)); 3499 childrenList.add(new Property("description", "string", "General description of how security works.", 0, 3500 java.lang.Integer.MAX_VALUE, description)); 3501 childrenList.add(new Property("certificate", "", "Certificates associated with security profiles.", 0, 3502 java.lang.Integer.MAX_VALUE, certificate)); 3503 } 3504 3505 @Override 3506 public void setProperty(String name, Base value) throws FHIRException { 3507 if (name.equals("cors")) 3508 this.cors = castToBoolean(value); // BooleanType 3509 else if (name.equals("service")) 3510 this.getService().add(castToCodeableConcept(value)); 3511 else if (name.equals("description")) 3512 this.description = castToString(value); // StringType 3513 else if (name.equals("certificate")) 3514 this.getCertificate().add((ConformanceRestSecurityCertificateComponent) value); 3515 else 3516 super.setProperty(name, value); 3517 } 3518 3519 @Override 3520 public Base addChild(String name) throws FHIRException { 3521 if (name.equals("cors")) { 3522 throw new FHIRException("Cannot call addChild on a singleton property Conformance.cors"); 3523 } else if (name.equals("service")) { 3524 return addService(); 3525 } else if (name.equals("description")) { 3526 throw new FHIRException("Cannot call addChild on a singleton property Conformance.description"); 3527 } else if (name.equals("certificate")) { 3528 return addCertificate(); 3529 } else 3530 return super.addChild(name); 3531 } 3532 3533 public ConformanceRestSecurityComponent copy() { 3534 ConformanceRestSecurityComponent dst = new ConformanceRestSecurityComponent(); 3535 copyValues(dst); 3536 dst.cors = cors == null ? null : cors.copy(); 3537 if (service != null) { 3538 dst.service = new ArrayList<CodeableConcept>(); 3539 for (CodeableConcept i : service) 3540 dst.service.add(i.copy()); 3541 } 3542 ; 3543 dst.description = description == null ? null : description.copy(); 3544 if (certificate != null) { 3545 dst.certificate = new ArrayList<ConformanceRestSecurityCertificateComponent>(); 3546 for (ConformanceRestSecurityCertificateComponent i : certificate) 3547 dst.certificate.add(i.copy()); 3548 } 3549 ; 3550 return dst; 3551 } 3552 3553 @Override 3554 public boolean equalsDeep(Base other) { 3555 if (!super.equalsDeep(other)) 3556 return false; 3557 if (!(other instanceof ConformanceRestSecurityComponent)) 3558 return false; 3559 ConformanceRestSecurityComponent o = (ConformanceRestSecurityComponent) other; 3560 return compareDeep(cors, o.cors, true) && compareDeep(service, o.service, true) 3561 && compareDeep(description, o.description, true) && compareDeep(certificate, o.certificate, true); 3562 } 3563 3564 @Override 3565 public boolean equalsShallow(Base other) { 3566 if (!super.equalsShallow(other)) 3567 return false; 3568 if (!(other instanceof ConformanceRestSecurityComponent)) 3569 return false; 3570 ConformanceRestSecurityComponent o = (ConformanceRestSecurityComponent) other; 3571 return compareValues(cors, o.cors, true) && compareValues(description, o.description, true); 3572 } 3573 3574 public boolean isEmpty() { 3575 return super.isEmpty() && (cors == null || cors.isEmpty()) && (service == null || service.isEmpty()) 3576 && (description == null || description.isEmpty()) && (certificate == null || certificate.isEmpty()); 3577 } 3578 3579 public String fhirType() { 3580 return "Conformance.rest.security"; 3581 3582 } 3583 3584 } 3585 3586 @Block() 3587 public static class ConformanceRestSecurityCertificateComponent extends BackboneElement 3588 implements IBaseBackboneElement { 3589 /** 3590 * Mime type for certificate. 3591 */ 3592 @Child(name = "type", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 3593 @Description(shortDefinition = "Mime type for certificate", formalDefinition = "Mime type for certificate.") 3594 protected CodeType type; 3595 3596 /** 3597 * Actual certificate. 3598 */ 3599 @Child(name = "blob", type = { 3600 Base64BinaryType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3601 @Description(shortDefinition = "Actual certificate", formalDefinition = "Actual certificate.") 3602 protected Base64BinaryType blob; 3603 3604 private static final long serialVersionUID = 2092655854L; 3605 3606 /* 3607 * Constructor 3608 */ 3609 public ConformanceRestSecurityCertificateComponent() { 3610 super(); 3611 } 3612 3613 /** 3614 * @return {@link #type} (Mime type for certificate.). This is the underlying 3615 * object with id, value and extensions. The accessor "getType" gives 3616 * direct access to the value 3617 */ 3618 public CodeType getTypeElement() { 3619 if (this.type == null) 3620 if (Configuration.errorOnAutoCreate()) 3621 throw new Error("Attempt to auto-create ConformanceRestSecurityCertificateComponent.type"); 3622 else if (Configuration.doAutoCreate()) 3623 this.type = new CodeType(); // bb 3624 return this.type; 3625 } 3626 3627 public boolean hasTypeElement() { 3628 return this.type != null && !this.type.isEmpty(); 3629 } 3630 3631 public boolean hasType() { 3632 return this.type != null && !this.type.isEmpty(); 3633 } 3634 3635 /** 3636 * @param value {@link #type} (Mime type for certificate.). This is the 3637 * underlying object with id, value and extensions. The accessor 3638 * "getType" gives direct access to the value 3639 */ 3640 public ConformanceRestSecurityCertificateComponent setTypeElement(CodeType value) { 3641 this.type = value; 3642 return this; 3643 } 3644 3645 /** 3646 * @return Mime type for certificate. 3647 */ 3648 public String getType() { 3649 return this.type == null ? null : this.type.getValue(); 3650 } 3651 3652 /** 3653 * @param value Mime type for certificate. 3654 */ 3655 public ConformanceRestSecurityCertificateComponent setType(String value) { 3656 if (Utilities.noString(value)) 3657 this.type = null; 3658 else { 3659 if (this.type == null) 3660 this.type = new CodeType(); 3661 this.type.setValue(value); 3662 } 3663 return this; 3664 } 3665 3666 /** 3667 * @return {@link #blob} (Actual certificate.). This is the underlying object 3668 * with id, value and extensions. The accessor "getBlob" gives direct 3669 * access to the value 3670 */ 3671 public Base64BinaryType getBlobElement() { 3672 if (this.blob == null) 3673 if (Configuration.errorOnAutoCreate()) 3674 throw new Error("Attempt to auto-create ConformanceRestSecurityCertificateComponent.blob"); 3675 else if (Configuration.doAutoCreate()) 3676 this.blob = new Base64BinaryType(); // bb 3677 return this.blob; 3678 } 3679 3680 public boolean hasBlobElement() { 3681 return this.blob != null && !this.blob.isEmpty(); 3682 } 3683 3684 public boolean hasBlob() { 3685 return this.blob != null && !this.blob.isEmpty(); 3686 } 3687 3688 /** 3689 * @param value {@link #blob} (Actual certificate.). This is the underlying 3690 * object with id, value and extensions. The accessor "getBlob" 3691 * gives direct access to the value 3692 */ 3693 public ConformanceRestSecurityCertificateComponent setBlobElement(Base64BinaryType value) { 3694 this.blob = value; 3695 return this; 3696 } 3697 3698 /** 3699 * @return Actual certificate. 3700 */ 3701 public byte[] getBlob() { 3702 return this.blob == null ? null : this.blob.getValue(); 3703 } 3704 3705 /** 3706 * @param value Actual certificate. 3707 */ 3708 public ConformanceRestSecurityCertificateComponent setBlob(byte[] value) { 3709 if (value == null) 3710 this.blob = null; 3711 else { 3712 if (this.blob == null) 3713 this.blob = new Base64BinaryType(); 3714 this.blob.setValue(value); 3715 } 3716 return this; 3717 } 3718 3719 protected void listChildren(List<Property> childrenList) { 3720 super.listChildren(childrenList); 3721 childrenList 3722 .add(new Property("type", "code", "Mime type for certificate.", 0, java.lang.Integer.MAX_VALUE, type)); 3723 childrenList 3724 .add(new Property("blob", "base64Binary", "Actual certificate.", 0, java.lang.Integer.MAX_VALUE, blob)); 3725 } 3726 3727 @Override 3728 public void setProperty(String name, Base value) throws FHIRException { 3729 if (name.equals("type")) 3730 this.type = castToCode(value); // CodeType 3731 else if (name.equals("blob")) 3732 this.blob = castToBase64Binary(value); // Base64BinaryType 3733 else 3734 super.setProperty(name, value); 3735 } 3736 3737 @Override 3738 public Base addChild(String name) throws FHIRException { 3739 if (name.equals("type")) { 3740 throw new FHIRException("Cannot call addChild on a singleton property Conformance.type"); 3741 } else if (name.equals("blob")) { 3742 throw new FHIRException("Cannot call addChild on a singleton property Conformance.blob"); 3743 } else 3744 return super.addChild(name); 3745 } 3746 3747 public ConformanceRestSecurityCertificateComponent copy() { 3748 ConformanceRestSecurityCertificateComponent dst = new ConformanceRestSecurityCertificateComponent(); 3749 copyValues(dst); 3750 dst.type = type == null ? null : type.copy(); 3751 dst.blob = blob == null ? null : blob.copy(); 3752 return dst; 3753 } 3754 3755 @Override 3756 public boolean equalsDeep(Base other) { 3757 if (!super.equalsDeep(other)) 3758 return false; 3759 if (!(other instanceof ConformanceRestSecurityCertificateComponent)) 3760 return false; 3761 ConformanceRestSecurityCertificateComponent o = (ConformanceRestSecurityCertificateComponent) other; 3762 return compareDeep(type, o.type, true) && compareDeep(blob, o.blob, true); 3763 } 3764 3765 @Override 3766 public boolean equalsShallow(Base other) { 3767 if (!super.equalsShallow(other)) 3768 return false; 3769 if (!(other instanceof ConformanceRestSecurityCertificateComponent)) 3770 return false; 3771 ConformanceRestSecurityCertificateComponent o = (ConformanceRestSecurityCertificateComponent) other; 3772 return compareValues(type, o.type, true) && compareValues(blob, o.blob, true); 3773 } 3774 3775 public boolean isEmpty() { 3776 return super.isEmpty() && (type == null || type.isEmpty()) && (blob == null || blob.isEmpty()); 3777 } 3778 3779 public String fhirType() { 3780 return "Conformance.rest.security.certificate"; 3781 3782 } 3783 3784 } 3785 3786 @Block() 3787 public static class ConformanceRestResourceComponent extends BackboneElement implements IBaseBackboneElement { 3788 /** 3789 * A type of resource exposed via the restful interface. 3790 */ 3791 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 3792 @Description(shortDefinition = "A resource type that is supported", formalDefinition = "A type of resource exposed via the restful interface.") 3793 protected CodeType type; 3794 3795 /** 3796 * A specification of the profile that describes the solution's overall support 3797 * for the resource, including any constraints on cardinality, bindings, lengths 3798 * or other limitations. See further discussion in [Using 3799 * Profiles]{profiling.html#profile-uses}. 3800 */ 3801 @Child(name = "profile", type = { 3802 StructureDefinition.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3803 @Description(shortDefinition = "Base System profile for all uses of resource", formalDefinition = "A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles]{profiling.html#profile-uses}.") 3804 protected Reference profile; 3805 3806 /** 3807 * The actual object that is the target of the reference (A specification of the 3808 * profile that describes the solution's overall support for the resource, 3809 * including any constraints on cardinality, bindings, lengths or other 3810 * limitations. See further discussion in [Using 3811 * Profiles]{profiling.html#profile-uses}.) 3812 */ 3813 protected StructureDefinition profileTarget; 3814 3815 /** 3816 * Identifies a restful operation supported by the solution. 3817 */ 3818 @Child(name = "interaction", type = {}, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3819 @Description(shortDefinition = "What operations are supported?", formalDefinition = "Identifies a restful operation supported by the solution.") 3820 protected List<ResourceInteractionComponent> interaction; 3821 3822 /** 3823 * This field is set to no-version to specify that the system does not support 3824 * (server) or use (client) versioning for this resource type. If this has some 3825 * other value, the server must at least correctly track and populate the 3826 * versionId meta-property on resources. If the value is 'versioned-update', 3827 * then the server supports all the versioning features, including using e-tags 3828 * for version integrity in the API. 3829 */ 3830 @Child(name = "versioning", type = { 3831 CodeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 3832 @Description(shortDefinition = "no-version | versioned | versioned-update", formalDefinition = "This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.") 3833 protected Enumeration<ResourceVersionPolicy> versioning; 3834 3835 /** 3836 * A flag for whether the server is able to return past versions as part of the 3837 * vRead operation. 3838 */ 3839 @Child(name = "readHistory", type = { 3840 BooleanType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 3841 @Description(shortDefinition = "Whether vRead can return past versions", formalDefinition = "A flag for whether the server is able to return past versions as part of the vRead operation.") 3842 protected BooleanType readHistory; 3843 3844 /** 3845 * A flag to indicate that the server allows or needs to allow the client to 3846 * create new identities on the server (e.g. that is, the client PUTs to a 3847 * location where there is no existing resource). Allowing this operation means 3848 * that the server allows the client to create new identities on the server. 3849 */ 3850 @Child(name = "updateCreate", type = { 3851 BooleanType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 3852 @Description(shortDefinition = "If update can commit to a new identity", formalDefinition = "A flag to indicate that the server allows or needs to allow the client to create new identities on the server (e.g. that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.") 3853 protected BooleanType updateCreate; 3854 3855 /** 3856 * A flag that indicates that the server supports conditional create. 3857 */ 3858 @Child(name = "conditionalCreate", type = { 3859 BooleanType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 3860 @Description(shortDefinition = "If allows/uses conditional create", formalDefinition = "A flag that indicates that the server supports conditional create.") 3861 protected BooleanType conditionalCreate; 3862 3863 /** 3864 * A flag that indicates that the server supports conditional update. 3865 */ 3866 @Child(name = "conditionalUpdate", type = { 3867 BooleanType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 3868 @Description(shortDefinition = "If allows/uses conditional update", formalDefinition = "A flag that indicates that the server supports conditional update.") 3869 protected BooleanType conditionalUpdate; 3870 3871 /** 3872 * A code that indicates how the server supports conditional delete. 3873 */ 3874 @Child(name = "conditionalDelete", type = { 3875 CodeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 3876 @Description(shortDefinition = "not-supported | single | multiple - how conditional delete is supported", formalDefinition = "A code that indicates how the server supports conditional delete.") 3877 protected Enumeration<ConditionalDeleteStatus> conditionalDelete; 3878 3879 /** 3880 * A list of _include values supported by the server. 3881 */ 3882 @Child(name = "searchInclude", type = { 3883 StringType.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3884 @Description(shortDefinition = "_include values supported by the server", formalDefinition = "A list of _include values supported by the server.") 3885 protected List<StringType> searchInclude; 3886 3887 /** 3888 * A list of _revinclude (reverse include) values supported by the server. 3889 */ 3890 @Child(name = "searchRevInclude", type = { 3891 StringType.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3892 @Description(shortDefinition = "_revinclude values supported by the server", formalDefinition = "A list of _revinclude (reverse include) values supported by the server.") 3893 protected List<StringType> searchRevInclude; 3894 3895 /** 3896 * Search parameters for implementations to support and/or make use of - either 3897 * references to ones defined in the specification, or additional ones defined 3898 * for/by the implementation. 3899 */ 3900 @Child(name = "searchParam", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3901 @Description(shortDefinition = "Search params supported by implementation", formalDefinition = "Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.") 3902 protected List<ConformanceRestResourceSearchParamComponent> searchParam; 3903 3904 private static final long serialVersionUID = 1781959905L; 3905 3906 /* 3907 * Constructor 3908 */ 3909 public ConformanceRestResourceComponent() { 3910 super(); 3911 } 3912 3913 /* 3914 * Constructor 3915 */ 3916 public ConformanceRestResourceComponent(CodeType type) { 3917 super(); 3918 this.type = type; 3919 } 3920 3921 /** 3922 * @return {@link #type} (A type of resource exposed via the restful 3923 * interface.). This is the underlying object with id, value and 3924 * extensions. The accessor "getType" gives direct access to the value 3925 */ 3926 public CodeType getTypeElement() { 3927 if (this.type == null) 3928 if (Configuration.errorOnAutoCreate()) 3929 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.type"); 3930 else if (Configuration.doAutoCreate()) 3931 this.type = new CodeType(); // bb 3932 return this.type; 3933 } 3934 3935 public boolean hasTypeElement() { 3936 return this.type != null && !this.type.isEmpty(); 3937 } 3938 3939 public boolean hasType() { 3940 return this.type != null && !this.type.isEmpty(); 3941 } 3942 3943 /** 3944 * @param value {@link #type} (A type of resource exposed via the restful 3945 * interface.). This is the underlying object with id, value and 3946 * extensions. The accessor "getType" gives direct access to the 3947 * value 3948 */ 3949 public ConformanceRestResourceComponent setTypeElement(CodeType value) { 3950 this.type = value; 3951 return this; 3952 } 3953 3954 /** 3955 * @return A type of resource exposed via the restful interface. 3956 */ 3957 public String getType() { 3958 return this.type == null ? null : this.type.getValue(); 3959 } 3960 3961 /** 3962 * @param value A type of resource exposed via the restful interface. 3963 */ 3964 public ConformanceRestResourceComponent setType(String value) { 3965 if (this.type == null) 3966 this.type = new CodeType(); 3967 this.type.setValue(value); 3968 return this; 3969 } 3970 3971 /** 3972 * @return {@link #profile} (A specification of the profile that describes the 3973 * solution's overall support for the resource, including any 3974 * constraints on cardinality, bindings, lengths or other limitations. 3975 * See further discussion in [Using 3976 * Profiles]{profiling.html#profile-uses}.) 3977 */ 3978 public Reference getProfile() { 3979 if (this.profile == null) 3980 if (Configuration.errorOnAutoCreate()) 3981 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.profile"); 3982 else if (Configuration.doAutoCreate()) 3983 this.profile = new Reference(); // cc 3984 return this.profile; 3985 } 3986 3987 public boolean hasProfile() { 3988 return this.profile != null && !this.profile.isEmpty(); 3989 } 3990 3991 /** 3992 * @param value {@link #profile} (A specification of the profile that describes 3993 * the solution's overall support for the resource, including any 3994 * constraints on cardinality, bindings, lengths or other 3995 * limitations. See further discussion in [Using 3996 * Profiles]{profiling.html#profile-uses}.) 3997 */ 3998 public ConformanceRestResourceComponent setProfile(Reference value) { 3999 this.profile = value; 4000 return this; 4001 } 4002 4003 /** 4004 * @return {@link #profile} The actual object that is the target of the 4005 * reference. The reference library doesn't populate this, but you can 4006 * use it to hold the resource if you resolve it. (A specification of 4007 * the profile that describes the solution's overall support for the 4008 * resource, including any constraints on cardinality, bindings, lengths 4009 * or other limitations. See further discussion in [Using 4010 * Profiles]{profiling.html#profile-uses}.) 4011 */ 4012 public StructureDefinition getProfileTarget() { 4013 if (this.profileTarget == null) 4014 if (Configuration.errorOnAutoCreate()) 4015 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.profile"); 4016 else if (Configuration.doAutoCreate()) 4017 this.profileTarget = new StructureDefinition(); // aa 4018 return this.profileTarget; 4019 } 4020 4021 /** 4022 * @param value {@link #profile} The actual object that is the target of the 4023 * reference. The reference library doesn't use these, but you can 4024 * use it to hold the resource if you resolve it. (A specification 4025 * of the profile that describes the solution's overall support for 4026 * the resource, including any constraints on cardinality, 4027 * bindings, lengths or other limitations. See further discussion 4028 * in [Using Profiles]{profiling.html#profile-uses}.) 4029 */ 4030 public ConformanceRestResourceComponent setProfileTarget(StructureDefinition value) { 4031 this.profileTarget = value; 4032 return this; 4033 } 4034 4035 /** 4036 * @return {@link #interaction} (Identifies a restful operation supported by the 4037 * solution.) 4038 */ 4039 public List<ResourceInteractionComponent> getInteraction() { 4040 if (this.interaction == null) 4041 this.interaction = new ArrayList<ResourceInteractionComponent>(); 4042 return this.interaction; 4043 } 4044 4045 public boolean hasInteraction() { 4046 if (this.interaction == null) 4047 return false; 4048 for (ResourceInteractionComponent item : this.interaction) 4049 if (!item.isEmpty()) 4050 return true; 4051 return false; 4052 } 4053 4054 /** 4055 * @return {@link #interaction} (Identifies a restful operation supported by the 4056 * solution.) 4057 */ 4058 // syntactic sugar 4059 public ResourceInteractionComponent addInteraction() { // 3 4060 ResourceInteractionComponent t = new ResourceInteractionComponent(); 4061 if (this.interaction == null) 4062 this.interaction = new ArrayList<ResourceInteractionComponent>(); 4063 this.interaction.add(t); 4064 return t; 4065 } 4066 4067 // syntactic sugar 4068 public ConformanceRestResourceComponent addInteraction(ResourceInteractionComponent t) { // 3 4069 if (t == null) 4070 return this; 4071 if (this.interaction == null) 4072 this.interaction = new ArrayList<ResourceInteractionComponent>(); 4073 this.interaction.add(t); 4074 return this; 4075 } 4076 4077 /** 4078 * @return {@link #versioning} (This field is set to no-version to specify that 4079 * the system does not support (server) or use (client) versioning for 4080 * this resource type. If this has some other value, the server must at 4081 * least correctly track and populate the versionId meta-property on 4082 * resources. If the value is 'versioned-update', then the server 4083 * supports all the versioning features, including using e-tags for 4084 * version integrity in the API.). This is the underlying object with 4085 * id, value and extensions. The accessor "getVersioning" gives direct 4086 * access to the value 4087 */ 4088 public Enumeration<ResourceVersionPolicy> getVersioningElement() { 4089 if (this.versioning == null) 4090 if (Configuration.errorOnAutoCreate()) 4091 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.versioning"); 4092 else if (Configuration.doAutoCreate()) 4093 this.versioning = new Enumeration<ResourceVersionPolicy>(new ResourceVersionPolicyEnumFactory()); // bb 4094 return this.versioning; 4095 } 4096 4097 public boolean hasVersioningElement() { 4098 return this.versioning != null && !this.versioning.isEmpty(); 4099 } 4100 4101 public boolean hasVersioning() { 4102 return this.versioning != null && !this.versioning.isEmpty(); 4103 } 4104 4105 /** 4106 * @param value {@link #versioning} (This field is set to no-version to specify 4107 * that the system does not support (server) or use (client) 4108 * versioning for this resource type. If this has some other value, 4109 * the server must at least correctly track and populate the 4110 * versionId meta-property on resources. If the value is 4111 * 'versioned-update', then the server supports all the versioning 4112 * features, including using e-tags for version integrity in the 4113 * API.). This is the underlying object with id, value and 4114 * extensions. The accessor "getVersioning" gives direct access to 4115 * the value 4116 */ 4117 public ConformanceRestResourceComponent setVersioningElement(Enumeration<ResourceVersionPolicy> value) { 4118 this.versioning = value; 4119 return this; 4120 } 4121 4122 /** 4123 * @return This field is set to no-version to specify that the system does not 4124 * support (server) or use (client) versioning for this resource type. 4125 * If this has some other value, the server must at least correctly 4126 * track and populate the versionId meta-property on resources. If the 4127 * value is 'versioned-update', then the server supports all the 4128 * versioning features, including using e-tags for version integrity in 4129 * the API. 4130 */ 4131 public ResourceVersionPolicy getVersioning() { 4132 return this.versioning == null ? null : this.versioning.getValue(); 4133 } 4134 4135 /** 4136 * @param value This field is set to no-version to specify that the system does 4137 * not support (server) or use (client) versioning for this 4138 * resource type. If this has some other value, the server must at 4139 * least correctly track and populate the versionId meta-property 4140 * on resources. If the value is 'versioned-update', then the 4141 * server supports all the versioning features, including using 4142 * e-tags for version integrity in the API. 4143 */ 4144 public ConformanceRestResourceComponent setVersioning(ResourceVersionPolicy value) { 4145 if (value == null) 4146 this.versioning = null; 4147 else { 4148 if (this.versioning == null) 4149 this.versioning = new Enumeration<ResourceVersionPolicy>(new ResourceVersionPolicyEnumFactory()); 4150 this.versioning.setValue(value); 4151 } 4152 return this; 4153 } 4154 4155 /** 4156 * @return {@link #readHistory} (A flag for whether the server is able to return 4157 * past versions as part of the vRead operation.). This is the 4158 * underlying object with id, value and extensions. The accessor 4159 * "getReadHistory" gives direct access to the value 4160 */ 4161 public BooleanType getReadHistoryElement() { 4162 if (this.readHistory == null) 4163 if (Configuration.errorOnAutoCreate()) 4164 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.readHistory"); 4165 else if (Configuration.doAutoCreate()) 4166 this.readHistory = new BooleanType(); // bb 4167 return this.readHistory; 4168 } 4169 4170 public boolean hasReadHistoryElement() { 4171 return this.readHistory != null && !this.readHistory.isEmpty(); 4172 } 4173 4174 public boolean hasReadHistory() { 4175 return this.readHistory != null && !this.readHistory.isEmpty(); 4176 } 4177 4178 /** 4179 * @param value {@link #readHistory} (A flag for whether the server is able to 4180 * return past versions as part of the vRead operation.). This is 4181 * the underlying object with id, value and extensions. The 4182 * accessor "getReadHistory" gives direct access to the value 4183 */ 4184 public ConformanceRestResourceComponent setReadHistoryElement(BooleanType value) { 4185 this.readHistory = value; 4186 return this; 4187 } 4188 4189 /** 4190 * @return A flag for whether the server is able to return past versions as part 4191 * of the vRead operation. 4192 */ 4193 public boolean getReadHistory() { 4194 return this.readHistory == null || this.readHistory.isEmpty() ? false : this.readHistory.getValue(); 4195 } 4196 4197 /** 4198 * @param value A flag for whether the server is able to return past versions as 4199 * part of the vRead operation. 4200 */ 4201 public ConformanceRestResourceComponent setReadHistory(boolean value) { 4202 if (this.readHistory == null) 4203 this.readHistory = new BooleanType(); 4204 this.readHistory.setValue(value); 4205 return this; 4206 } 4207 4208 /** 4209 * @return {@link #updateCreate} (A flag to indicate that the server allows or 4210 * needs to allow the client to create new identities on the server 4211 * (e.g. that is, the client PUTs to a location where there is no 4212 * existing resource). Allowing this operation means that the server 4213 * allows the client to create new identities on the server.). This is 4214 * the underlying object with id, value and extensions. The accessor 4215 * "getUpdateCreate" gives direct access to the value 4216 */ 4217 public BooleanType getUpdateCreateElement() { 4218 if (this.updateCreate == null) 4219 if (Configuration.errorOnAutoCreate()) 4220 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.updateCreate"); 4221 else if (Configuration.doAutoCreate()) 4222 this.updateCreate = new BooleanType(); // bb 4223 return this.updateCreate; 4224 } 4225 4226 public boolean hasUpdateCreateElement() { 4227 return this.updateCreate != null && !this.updateCreate.isEmpty(); 4228 } 4229 4230 public boolean hasUpdateCreate() { 4231 return this.updateCreate != null && !this.updateCreate.isEmpty(); 4232 } 4233 4234 /** 4235 * @param value {@link #updateCreate} (A flag to indicate that the server allows 4236 * or needs to allow the client to create new identities on the 4237 * server (e.g. that is, the client PUTs to a location where there 4238 * is no existing resource). Allowing this operation means that the 4239 * server allows the client to create new identities on the 4240 * server.). This is the underlying object with id, value and 4241 * extensions. The accessor "getUpdateCreate" gives direct access 4242 * to the value 4243 */ 4244 public ConformanceRestResourceComponent setUpdateCreateElement(BooleanType value) { 4245 this.updateCreate = value; 4246 return this; 4247 } 4248 4249 /** 4250 * @return A flag to indicate that the server allows or needs to allow the 4251 * client to create new identities on the server (e.g. that is, the 4252 * client PUTs to a location where there is no existing resource). 4253 * Allowing this operation means that the server allows the client to 4254 * create new identities on the server. 4255 */ 4256 public boolean getUpdateCreate() { 4257 return this.updateCreate == null || this.updateCreate.isEmpty() ? false : this.updateCreate.getValue(); 4258 } 4259 4260 /** 4261 * @param value A flag to indicate that the server allows or needs to allow the 4262 * client to create new identities on the server (e.g. that is, the 4263 * client PUTs to a location where there is no existing resource). 4264 * Allowing this operation means that the server allows the client 4265 * to create new identities on the server. 4266 */ 4267 public ConformanceRestResourceComponent setUpdateCreate(boolean value) { 4268 if (this.updateCreate == null) 4269 this.updateCreate = new BooleanType(); 4270 this.updateCreate.setValue(value); 4271 return this; 4272 } 4273 4274 /** 4275 * @return {@link #conditionalCreate} (A flag that indicates that the server 4276 * supports conditional create.). This is the underlying object with id, 4277 * value and extensions. The accessor "getConditionalCreate" gives 4278 * direct access to the value 4279 */ 4280 public BooleanType getConditionalCreateElement() { 4281 if (this.conditionalCreate == null) 4282 if (Configuration.errorOnAutoCreate()) 4283 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.conditionalCreate"); 4284 else if (Configuration.doAutoCreate()) 4285 this.conditionalCreate = new BooleanType(); // bb 4286 return this.conditionalCreate; 4287 } 4288 4289 public boolean hasConditionalCreateElement() { 4290 return this.conditionalCreate != null && !this.conditionalCreate.isEmpty(); 4291 } 4292 4293 public boolean hasConditionalCreate() { 4294 return this.conditionalCreate != null && !this.conditionalCreate.isEmpty(); 4295 } 4296 4297 /** 4298 * @param value {@link #conditionalCreate} (A flag that indicates that the 4299 * server supports conditional create.). This is the underlying 4300 * object with id, value and extensions. The accessor 4301 * "getConditionalCreate" gives direct access to the value 4302 */ 4303 public ConformanceRestResourceComponent setConditionalCreateElement(BooleanType value) { 4304 this.conditionalCreate = value; 4305 return this; 4306 } 4307 4308 /** 4309 * @return A flag that indicates that the server supports conditional create. 4310 */ 4311 public boolean getConditionalCreate() { 4312 return this.conditionalCreate == null || this.conditionalCreate.isEmpty() ? false 4313 : this.conditionalCreate.getValue(); 4314 } 4315 4316 /** 4317 * @param value A flag that indicates that the server supports conditional 4318 * create. 4319 */ 4320 public ConformanceRestResourceComponent setConditionalCreate(boolean value) { 4321 if (this.conditionalCreate == null) 4322 this.conditionalCreate = new BooleanType(); 4323 this.conditionalCreate.setValue(value); 4324 return this; 4325 } 4326 4327 /** 4328 * @return {@link #conditionalUpdate} (A flag that indicates that the server 4329 * supports conditional update.). This is the underlying object with id, 4330 * value and extensions. The accessor "getConditionalUpdate" gives 4331 * direct access to the value 4332 */ 4333 public BooleanType getConditionalUpdateElement() { 4334 if (this.conditionalUpdate == null) 4335 if (Configuration.errorOnAutoCreate()) 4336 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.conditionalUpdate"); 4337 else if (Configuration.doAutoCreate()) 4338 this.conditionalUpdate = new BooleanType(); // bb 4339 return this.conditionalUpdate; 4340 } 4341 4342 public boolean hasConditionalUpdateElement() { 4343 return this.conditionalUpdate != null && !this.conditionalUpdate.isEmpty(); 4344 } 4345 4346 public boolean hasConditionalUpdate() { 4347 return this.conditionalUpdate != null && !this.conditionalUpdate.isEmpty(); 4348 } 4349 4350 /** 4351 * @param value {@link #conditionalUpdate} (A flag that indicates that the 4352 * server supports conditional update.). This is the underlying 4353 * object with id, value and extensions. The accessor 4354 * "getConditionalUpdate" gives direct access to the value 4355 */ 4356 public ConformanceRestResourceComponent setConditionalUpdateElement(BooleanType value) { 4357 this.conditionalUpdate = value; 4358 return this; 4359 } 4360 4361 /** 4362 * @return A flag that indicates that the server supports conditional update. 4363 */ 4364 public boolean getConditionalUpdate() { 4365 return this.conditionalUpdate == null || this.conditionalUpdate.isEmpty() ? false 4366 : this.conditionalUpdate.getValue(); 4367 } 4368 4369 /** 4370 * @param value A flag that indicates that the server supports conditional 4371 * update. 4372 */ 4373 public ConformanceRestResourceComponent setConditionalUpdate(boolean value) { 4374 if (this.conditionalUpdate == null) 4375 this.conditionalUpdate = new BooleanType(); 4376 this.conditionalUpdate.setValue(value); 4377 return this; 4378 } 4379 4380 /** 4381 * @return {@link #conditionalDelete} (A code that indicates how the server 4382 * supports conditional delete.). This is the underlying object with id, 4383 * value and extensions. The accessor "getConditionalDelete" gives 4384 * direct access to the value 4385 */ 4386 public Enumeration<ConditionalDeleteStatus> getConditionalDeleteElement() { 4387 if (this.conditionalDelete == null) 4388 if (Configuration.errorOnAutoCreate()) 4389 throw new Error("Attempt to auto-create ConformanceRestResourceComponent.conditionalDelete"); 4390 else if (Configuration.doAutoCreate()) 4391 this.conditionalDelete = new Enumeration<ConditionalDeleteStatus>(new ConditionalDeleteStatusEnumFactory()); // bb 4392 return this.conditionalDelete; 4393 } 4394 4395 public boolean hasConditionalDeleteElement() { 4396 return this.conditionalDelete != null && !this.conditionalDelete.isEmpty(); 4397 } 4398 4399 public boolean hasConditionalDelete() { 4400 return this.conditionalDelete != null && !this.conditionalDelete.isEmpty(); 4401 } 4402 4403 /** 4404 * @param value {@link #conditionalDelete} (A code that indicates how the server 4405 * supports conditional delete.). This is the underlying object 4406 * with id, value and extensions. The accessor 4407 * "getConditionalDelete" gives direct access to the value 4408 */ 4409 public ConformanceRestResourceComponent setConditionalDeleteElement(Enumeration<ConditionalDeleteStatus> value) { 4410 this.conditionalDelete = value; 4411 return this; 4412 } 4413 4414 /** 4415 * @return A code that indicates how the server supports conditional delete. 4416 */ 4417 public ConditionalDeleteStatus getConditionalDelete() { 4418 return this.conditionalDelete == null ? null : this.conditionalDelete.getValue(); 4419 } 4420 4421 /** 4422 * @param value A code that indicates how the server supports conditional 4423 * delete. 4424 */ 4425 public ConformanceRestResourceComponent setConditionalDelete(ConditionalDeleteStatus value) { 4426 if (value == null) 4427 this.conditionalDelete = null; 4428 else { 4429 if (this.conditionalDelete == null) 4430 this.conditionalDelete = new Enumeration<ConditionalDeleteStatus>(new ConditionalDeleteStatusEnumFactory()); 4431 this.conditionalDelete.setValue(value); 4432 } 4433 return this; 4434 } 4435 4436 /** 4437 * @return {@link #searchInclude} (A list of _include values supported by the 4438 * server.) 4439 */ 4440 public List<StringType> getSearchInclude() { 4441 if (this.searchInclude == null) 4442 this.searchInclude = new ArrayList<StringType>(); 4443 return this.searchInclude; 4444 } 4445 4446 public boolean hasSearchInclude() { 4447 if (this.searchInclude == null) 4448 return false; 4449 for (StringType item : this.searchInclude) 4450 if (!item.isEmpty()) 4451 return true; 4452 return false; 4453 } 4454 4455 /** 4456 * @return {@link #searchInclude} (A list of _include values supported by the 4457 * server.) 4458 */ 4459 // syntactic sugar 4460 public StringType addSearchIncludeElement() {// 2 4461 StringType t = new StringType(); 4462 if (this.searchInclude == null) 4463 this.searchInclude = new ArrayList<StringType>(); 4464 this.searchInclude.add(t); 4465 return t; 4466 } 4467 4468 /** 4469 * @param value {@link #searchInclude} (A list of _include values supported by 4470 * the server.) 4471 */ 4472 public ConformanceRestResourceComponent addSearchInclude(String value) { // 1 4473 StringType t = new StringType(); 4474 t.setValue(value); 4475 if (this.searchInclude == null) 4476 this.searchInclude = new ArrayList<StringType>(); 4477 this.searchInclude.add(t); 4478 return this; 4479 } 4480 4481 /** 4482 * @param value {@link #searchInclude} (A list of _include values supported by 4483 * the server.) 4484 */ 4485 public boolean hasSearchInclude(String value) { 4486 if (this.searchInclude == null) 4487 return false; 4488 for (StringType v : this.searchInclude) 4489 if (v.equals(value)) // string 4490 return true; 4491 return false; 4492 } 4493 4494 /** 4495 * @return {@link #searchRevInclude} (A list of _revinclude (reverse include) 4496 * values supported by the server.) 4497 */ 4498 public List<StringType> getSearchRevInclude() { 4499 if (this.searchRevInclude == null) 4500 this.searchRevInclude = new ArrayList<StringType>(); 4501 return this.searchRevInclude; 4502 } 4503 4504 public boolean hasSearchRevInclude() { 4505 if (this.searchRevInclude == null) 4506 return false; 4507 for (StringType item : this.searchRevInclude) 4508 if (!item.isEmpty()) 4509 return true; 4510 return false; 4511 } 4512 4513 /** 4514 * @return {@link #searchRevInclude} (A list of _revinclude (reverse include) 4515 * values supported by the server.) 4516 */ 4517 // syntactic sugar 4518 public StringType addSearchRevIncludeElement() {// 2 4519 StringType t = new StringType(); 4520 if (this.searchRevInclude == null) 4521 this.searchRevInclude = new ArrayList<StringType>(); 4522 this.searchRevInclude.add(t); 4523 return t; 4524 } 4525 4526 /** 4527 * @param value {@link #searchRevInclude} (A list of _revinclude (reverse 4528 * include) values supported by the server.) 4529 */ 4530 public ConformanceRestResourceComponent addSearchRevInclude(String value) { // 1 4531 StringType t = new StringType(); 4532 t.setValue(value); 4533 if (this.searchRevInclude == null) 4534 this.searchRevInclude = new ArrayList<StringType>(); 4535 this.searchRevInclude.add(t); 4536 return this; 4537 } 4538 4539 /** 4540 * @param value {@link #searchRevInclude} (A list of _revinclude (reverse 4541 * include) values supported by the server.) 4542 */ 4543 public boolean hasSearchRevInclude(String value) { 4544 if (this.searchRevInclude == null) 4545 return false; 4546 for (StringType v : this.searchRevInclude) 4547 if (v.equals(value)) // string 4548 return true; 4549 return false; 4550 } 4551 4552 /** 4553 * @return {@link #searchParam} (Search parameters for implementations to 4554 * support and/or make use of - either references to ones defined in the 4555 * specification, or additional ones defined for/by the implementation.) 4556 */ 4557 public List<ConformanceRestResourceSearchParamComponent> getSearchParam() { 4558 if (this.searchParam == null) 4559 this.searchParam = new ArrayList<ConformanceRestResourceSearchParamComponent>(); 4560 return this.searchParam; 4561 } 4562 4563 public boolean hasSearchParam() { 4564 if (this.searchParam == null) 4565 return false; 4566 for (ConformanceRestResourceSearchParamComponent item : this.searchParam) 4567 if (!item.isEmpty()) 4568 return true; 4569 return false; 4570 } 4571 4572 /** 4573 * @return {@link #searchParam} (Search parameters for implementations to 4574 * support and/or make use of - either references to ones defined in the 4575 * specification, or additional ones defined for/by the implementation.) 4576 */ 4577 // syntactic sugar 4578 public ConformanceRestResourceSearchParamComponent addSearchParam() { // 3 4579 ConformanceRestResourceSearchParamComponent t = new ConformanceRestResourceSearchParamComponent(); 4580 if (this.searchParam == null) 4581 this.searchParam = new ArrayList<ConformanceRestResourceSearchParamComponent>(); 4582 this.searchParam.add(t); 4583 return t; 4584 } 4585 4586 // syntactic sugar 4587 public ConformanceRestResourceComponent addSearchParam(ConformanceRestResourceSearchParamComponent t) { // 3 4588 if (t == null) 4589 return this; 4590 if (this.searchParam == null) 4591 this.searchParam = new ArrayList<ConformanceRestResourceSearchParamComponent>(); 4592 this.searchParam.add(t); 4593 return this; 4594 } 4595 4596 protected void listChildren(List<Property> childrenList) { 4597 super.listChildren(childrenList); 4598 childrenList.add(new Property("type", "code", "A type of resource exposed via the restful interface.", 0, 4599 java.lang.Integer.MAX_VALUE, type)); 4600 childrenList.add(new Property("profile", "Reference(StructureDefinition)", 4601 "A specification of the profile that describes the solution's overall support for the resource, including any constraints on cardinality, bindings, lengths or other limitations. See further discussion in [Using Profiles]{profiling.html#profile-uses}.", 4602 0, java.lang.Integer.MAX_VALUE, profile)); 4603 childrenList.add(new Property("interaction", "", "Identifies a restful operation supported by the solution.", 0, 4604 java.lang.Integer.MAX_VALUE, interaction)); 4605 childrenList.add(new Property("versioning", "code", 4606 "This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.", 4607 0, java.lang.Integer.MAX_VALUE, versioning)); 4608 childrenList.add(new Property("readHistory", "boolean", 4609 "A flag for whether the server is able to return past versions as part of the vRead operation.", 0, 4610 java.lang.Integer.MAX_VALUE, readHistory)); 4611 childrenList.add(new Property("updateCreate", "boolean", 4612 "A flag to indicate that the server allows or needs to allow the client to create new identities on the server (e.g. that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.", 4613 0, java.lang.Integer.MAX_VALUE, updateCreate)); 4614 childrenList.add(new Property("conditionalCreate", "boolean", 4615 "A flag that indicates that the server supports conditional create.", 0, java.lang.Integer.MAX_VALUE, 4616 conditionalCreate)); 4617 childrenList.add(new Property("conditionalUpdate", "boolean", 4618 "A flag that indicates that the server supports conditional update.", 0, java.lang.Integer.MAX_VALUE, 4619 conditionalUpdate)); 4620 childrenList.add( 4621 new Property("conditionalDelete", "code", "A code that indicates how the server supports conditional delete.", 4622 0, java.lang.Integer.MAX_VALUE, conditionalDelete)); 4623 childrenList.add(new Property("searchInclude", "string", "A list of _include values supported by the server.", 0, 4624 java.lang.Integer.MAX_VALUE, searchInclude)); 4625 childrenList.add(new Property("searchRevInclude", "string", 4626 "A list of _revinclude (reverse include) values supported by the server.", 0, java.lang.Integer.MAX_VALUE, 4627 searchRevInclude)); 4628 childrenList.add(new Property("searchParam", "", 4629 "Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 4630 0, java.lang.Integer.MAX_VALUE, searchParam)); 4631 } 4632 4633 @Override 4634 public void setProperty(String name, Base value) throws FHIRException { 4635 if (name.equals("type")) 4636 this.type = castToCode(value); // CodeType 4637 else if (name.equals("profile")) 4638 this.profile = castToReference(value); // Reference 4639 else if (name.equals("interaction")) 4640 this.getInteraction().add((ResourceInteractionComponent) value); 4641 else if (name.equals("versioning")) 4642 this.versioning = new ResourceVersionPolicyEnumFactory().fromType(value); // Enumeration<ResourceVersionPolicy> 4643 else if (name.equals("readHistory")) 4644 this.readHistory = castToBoolean(value); // BooleanType 4645 else if (name.equals("updateCreate")) 4646 this.updateCreate = castToBoolean(value); // BooleanType 4647 else if (name.equals("conditionalCreate")) 4648 this.conditionalCreate = castToBoolean(value); // BooleanType 4649 else if (name.equals("conditionalUpdate")) 4650 this.conditionalUpdate = castToBoolean(value); // BooleanType 4651 else if (name.equals("conditionalDelete")) 4652 this.conditionalDelete = new ConditionalDeleteStatusEnumFactory().fromType(value); // Enumeration<ConditionalDeleteStatus> 4653 else if (name.equals("searchInclude")) 4654 this.getSearchInclude().add(castToString(value)); 4655 else if (name.equals("searchRevInclude")) 4656 this.getSearchRevInclude().add(castToString(value)); 4657 else if (name.equals("searchParam")) 4658 this.getSearchParam().add((ConformanceRestResourceSearchParamComponent) value); 4659 else 4660 super.setProperty(name, value); 4661 } 4662 4663 @Override 4664 public Base addChild(String name) throws FHIRException { 4665 if (name.equals("type")) { 4666 throw new FHIRException("Cannot call addChild on a singleton property Conformance.type"); 4667 } else if (name.equals("profile")) { 4668 this.profile = new Reference(); 4669 return this.profile; 4670 } else if (name.equals("interaction")) { 4671 return addInteraction(); 4672 } else if (name.equals("versioning")) { 4673 throw new FHIRException("Cannot call addChild on a singleton property Conformance.versioning"); 4674 } else if (name.equals("readHistory")) { 4675 throw new FHIRException("Cannot call addChild on a singleton property Conformance.readHistory"); 4676 } else if (name.equals("updateCreate")) { 4677 throw new FHIRException("Cannot call addChild on a singleton property Conformance.updateCreate"); 4678 } else if (name.equals("conditionalCreate")) { 4679 throw new FHIRException("Cannot call addChild on a singleton property Conformance.conditionalCreate"); 4680 } else if (name.equals("conditionalUpdate")) { 4681 throw new FHIRException("Cannot call addChild on a singleton property Conformance.conditionalUpdate"); 4682 } else if (name.equals("conditionalDelete")) { 4683 throw new FHIRException("Cannot call addChild on a singleton property Conformance.conditionalDelete"); 4684 } else if (name.equals("searchInclude")) { 4685 throw new FHIRException("Cannot call addChild on a singleton property Conformance.searchInclude"); 4686 } else if (name.equals("searchRevInclude")) { 4687 throw new FHIRException("Cannot call addChild on a singleton property Conformance.searchRevInclude"); 4688 } else if (name.equals("searchParam")) { 4689 return addSearchParam(); 4690 } else 4691 return super.addChild(name); 4692 } 4693 4694 public ConformanceRestResourceComponent copy() { 4695 ConformanceRestResourceComponent dst = new ConformanceRestResourceComponent(); 4696 copyValues(dst); 4697 dst.type = type == null ? null : type.copy(); 4698 dst.profile = profile == null ? null : profile.copy(); 4699 if (interaction != null) { 4700 dst.interaction = new ArrayList<ResourceInteractionComponent>(); 4701 for (ResourceInteractionComponent i : interaction) 4702 dst.interaction.add(i.copy()); 4703 } 4704 ; 4705 dst.versioning = versioning == null ? null : versioning.copy(); 4706 dst.readHistory = readHistory == null ? null : readHistory.copy(); 4707 dst.updateCreate = updateCreate == null ? null : updateCreate.copy(); 4708 dst.conditionalCreate = conditionalCreate == null ? null : conditionalCreate.copy(); 4709 dst.conditionalUpdate = conditionalUpdate == null ? null : conditionalUpdate.copy(); 4710 dst.conditionalDelete = conditionalDelete == null ? null : conditionalDelete.copy(); 4711 if (searchInclude != null) { 4712 dst.searchInclude = new ArrayList<StringType>(); 4713 for (StringType i : searchInclude) 4714 dst.searchInclude.add(i.copy()); 4715 } 4716 ; 4717 if (searchRevInclude != null) { 4718 dst.searchRevInclude = new ArrayList<StringType>(); 4719 for (StringType i : searchRevInclude) 4720 dst.searchRevInclude.add(i.copy()); 4721 } 4722 ; 4723 if (searchParam != null) { 4724 dst.searchParam = new ArrayList<ConformanceRestResourceSearchParamComponent>(); 4725 for (ConformanceRestResourceSearchParamComponent i : searchParam) 4726 dst.searchParam.add(i.copy()); 4727 } 4728 ; 4729 return dst; 4730 } 4731 4732 @Override 4733 public boolean equalsDeep(Base other) { 4734 if (!super.equalsDeep(other)) 4735 return false; 4736 if (!(other instanceof ConformanceRestResourceComponent)) 4737 return false; 4738 ConformanceRestResourceComponent o = (ConformanceRestResourceComponent) other; 4739 return compareDeep(type, o.type, true) && compareDeep(profile, o.profile, true) 4740 && compareDeep(interaction, o.interaction, true) && compareDeep(versioning, o.versioning, true) 4741 && compareDeep(readHistory, o.readHistory, true) && compareDeep(updateCreate, o.updateCreate, true) 4742 && compareDeep(conditionalCreate, o.conditionalCreate, true) 4743 && compareDeep(conditionalUpdate, o.conditionalUpdate, true) 4744 && compareDeep(conditionalDelete, o.conditionalDelete, true) 4745 && compareDeep(searchInclude, o.searchInclude, true) 4746 && compareDeep(searchRevInclude, o.searchRevInclude, true) && compareDeep(searchParam, o.searchParam, true); 4747 } 4748 4749 @Override 4750 public boolean equalsShallow(Base other) { 4751 if (!super.equalsShallow(other)) 4752 return false; 4753 if (!(other instanceof ConformanceRestResourceComponent)) 4754 return false; 4755 ConformanceRestResourceComponent o = (ConformanceRestResourceComponent) other; 4756 return compareValues(type, o.type, true) && compareValues(versioning, o.versioning, true) 4757 && compareValues(readHistory, o.readHistory, true) && compareValues(updateCreate, o.updateCreate, true) 4758 && compareValues(conditionalCreate, o.conditionalCreate, true) 4759 && compareValues(conditionalUpdate, o.conditionalUpdate, true) 4760 && compareValues(conditionalDelete, o.conditionalDelete, true) 4761 && compareValues(searchInclude, o.searchInclude, true) 4762 && compareValues(searchRevInclude, o.searchRevInclude, true); 4763 } 4764 4765 public boolean isEmpty() { 4766 return super.isEmpty() && (type == null || type.isEmpty()) && (profile == null || profile.isEmpty()) 4767 && (interaction == null || interaction.isEmpty()) && (versioning == null || versioning.isEmpty()) 4768 && (readHistory == null || readHistory.isEmpty()) && (updateCreate == null || updateCreate.isEmpty()) 4769 && (conditionalCreate == null || conditionalCreate.isEmpty()) 4770 && (conditionalUpdate == null || conditionalUpdate.isEmpty()) 4771 && (conditionalDelete == null || conditionalDelete.isEmpty()) 4772 && (searchInclude == null || searchInclude.isEmpty()) 4773 && (searchRevInclude == null || searchRevInclude.isEmpty()) && (searchParam == null || searchParam.isEmpty()); 4774 } 4775 4776 public String fhirType() { 4777 return "Conformance.rest.resource"; 4778 4779 } 4780 4781 } 4782 4783 @Block() 4784 public static class ResourceInteractionComponent extends BackboneElement implements IBaseBackboneElement { 4785 /** 4786 * Coded identifier of the operation, supported by the system resource. 4787 */ 4788 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4789 @Description(shortDefinition = "read | vread | update | delete | history-instance | validate | history-type | create | search-type", formalDefinition = "Coded identifier of the operation, supported by the system resource.") 4790 protected Enumeration<TypeRestfulInteraction> code; 4791 4792 /** 4793 * Guidance specific to the implementation of this operation, such as 'delete is 4794 * a logical delete' or 'updates are only allowed with version id' or 'creates 4795 * permitted from pre-authorized certificates only'. 4796 */ 4797 @Child(name = "documentation", type = { 4798 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 4799 @Description(shortDefinition = "Anything special about operation behavior", formalDefinition = "Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.") 4800 protected StringType documentation; 4801 4802 private static final long serialVersionUID = -437507806L; 4803 4804 /* 4805 * Constructor 4806 */ 4807 public ResourceInteractionComponent() { 4808 super(); 4809 } 4810 4811 /* 4812 * Constructor 4813 */ 4814 public ResourceInteractionComponent(Enumeration<TypeRestfulInteraction> code) { 4815 super(); 4816 this.code = code; 4817 } 4818 4819 /** 4820 * @return {@link #code} (Coded identifier of the operation, supported by the 4821 * system resource.). This is the underlying object with id, value and 4822 * extensions. The accessor "getCode" gives direct access to the value 4823 */ 4824 public Enumeration<TypeRestfulInteraction> getCodeElement() { 4825 if (this.code == null) 4826 if (Configuration.errorOnAutoCreate()) 4827 throw new Error("Attempt to auto-create ResourceInteractionComponent.code"); 4828 else if (Configuration.doAutoCreate()) 4829 this.code = new Enumeration<TypeRestfulInteraction>(new TypeRestfulInteractionEnumFactory()); // bb 4830 return this.code; 4831 } 4832 4833 public boolean hasCodeElement() { 4834 return this.code != null && !this.code.isEmpty(); 4835 } 4836 4837 public boolean hasCode() { 4838 return this.code != null && !this.code.isEmpty(); 4839 } 4840 4841 /** 4842 * @param value {@link #code} (Coded identifier of the operation, supported by 4843 * the system resource.). This is the underlying object with id, 4844 * value and extensions. The accessor "getCode" gives direct access 4845 * to the value 4846 */ 4847 public ResourceInteractionComponent setCodeElement(Enumeration<TypeRestfulInteraction> value) { 4848 this.code = value; 4849 return this; 4850 } 4851 4852 /** 4853 * @return Coded identifier of the operation, supported by the system resource. 4854 */ 4855 public TypeRestfulInteraction getCode() { 4856 return this.code == null ? null : this.code.getValue(); 4857 } 4858 4859 /** 4860 * @param value Coded identifier of the operation, supported by the system 4861 * resource. 4862 */ 4863 public ResourceInteractionComponent setCode(TypeRestfulInteraction value) { 4864 if (this.code == null) 4865 this.code = new Enumeration<TypeRestfulInteraction>(new TypeRestfulInteractionEnumFactory()); 4866 this.code.setValue(value); 4867 return this; 4868 } 4869 4870 /** 4871 * @return {@link #documentation} (Guidance specific to the implementation of 4872 * this operation, such as 'delete is a logical delete' or 'updates are 4873 * only allowed with version id' or 'creates permitted from 4874 * pre-authorized certificates only'.). This is the underlying object 4875 * with id, value and extensions. The accessor "getDocumentation" gives 4876 * direct access to the value 4877 */ 4878 public StringType getDocumentationElement() { 4879 if (this.documentation == null) 4880 if (Configuration.errorOnAutoCreate()) 4881 throw new Error("Attempt to auto-create ResourceInteractionComponent.documentation"); 4882 else if (Configuration.doAutoCreate()) 4883 this.documentation = new StringType(); // bb 4884 return this.documentation; 4885 } 4886 4887 public boolean hasDocumentationElement() { 4888 return this.documentation != null && !this.documentation.isEmpty(); 4889 } 4890 4891 public boolean hasDocumentation() { 4892 return this.documentation != null && !this.documentation.isEmpty(); 4893 } 4894 4895 /** 4896 * @param value {@link #documentation} (Guidance specific to the implementation 4897 * of this operation, such as 'delete is a logical delete' or 4898 * 'updates are only allowed with version id' or 'creates permitted 4899 * from pre-authorized certificates only'.). This is the underlying 4900 * object with id, value and extensions. The accessor 4901 * "getDocumentation" gives direct access to the value 4902 */ 4903 public ResourceInteractionComponent setDocumentationElement(StringType value) { 4904 this.documentation = value; 4905 return this; 4906 } 4907 4908 /** 4909 * @return Guidance specific to the implementation of this operation, such as 4910 * 'delete is a logical delete' or 'updates are only allowed with 4911 * version id' or 'creates permitted from pre-authorized certificates 4912 * only'. 4913 */ 4914 public String getDocumentation() { 4915 return this.documentation == null ? null : this.documentation.getValue(); 4916 } 4917 4918 /** 4919 * @param value Guidance specific to the implementation of this operation, such 4920 * as 'delete is a logical delete' or 'updates are only allowed 4921 * with version id' or 'creates permitted from pre-authorized 4922 * certificates only'. 4923 */ 4924 public ResourceInteractionComponent setDocumentation(String value) { 4925 if (Utilities.noString(value)) 4926 this.documentation = null; 4927 else { 4928 if (this.documentation == null) 4929 this.documentation = new StringType(); 4930 this.documentation.setValue(value); 4931 } 4932 return this; 4933 } 4934 4935 protected void listChildren(List<Property> childrenList) { 4936 super.listChildren(childrenList); 4937 childrenList 4938 .add(new Property("code", "code", "Coded identifier of the operation, supported by the system resource.", 0, 4939 java.lang.Integer.MAX_VALUE, code)); 4940 childrenList.add(new Property("documentation", "string", 4941 "Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.", 4942 0, java.lang.Integer.MAX_VALUE, documentation)); 4943 } 4944 4945 @Override 4946 public void setProperty(String name, Base value) throws FHIRException { 4947 if (name.equals("code")) 4948 this.code = new TypeRestfulInteractionEnumFactory().fromType(value); // Enumeration<TypeRestfulInteraction> 4949 else if (name.equals("documentation")) 4950 this.documentation = castToString(value); // StringType 4951 else 4952 super.setProperty(name, value); 4953 } 4954 4955 @Override 4956 public Base addChild(String name) throws FHIRException { 4957 if (name.equals("code")) { 4958 throw new FHIRException("Cannot call addChild on a singleton property Conformance.code"); 4959 } else if (name.equals("documentation")) { 4960 throw new FHIRException("Cannot call addChild on a singleton property Conformance.documentation"); 4961 } else 4962 return super.addChild(name); 4963 } 4964 4965 public ResourceInteractionComponent copy() { 4966 ResourceInteractionComponent dst = new ResourceInteractionComponent(); 4967 copyValues(dst); 4968 dst.code = code == null ? null : code.copy(); 4969 dst.documentation = documentation == null ? null : documentation.copy(); 4970 return dst; 4971 } 4972 4973 @Override 4974 public boolean equalsDeep(Base other) { 4975 if (!super.equalsDeep(other)) 4976 return false; 4977 if (!(other instanceof ResourceInteractionComponent)) 4978 return false; 4979 ResourceInteractionComponent o = (ResourceInteractionComponent) other; 4980 return compareDeep(code, o.code, true) && compareDeep(documentation, o.documentation, true); 4981 } 4982 4983 @Override 4984 public boolean equalsShallow(Base other) { 4985 if (!super.equalsShallow(other)) 4986 return false; 4987 if (!(other instanceof ResourceInteractionComponent)) 4988 return false; 4989 ResourceInteractionComponent o = (ResourceInteractionComponent) other; 4990 return compareValues(code, o.code, true) && compareValues(documentation, o.documentation, true); 4991 } 4992 4993 public boolean isEmpty() { 4994 return super.isEmpty() && (code == null || code.isEmpty()) && (documentation == null || documentation.isEmpty()); 4995 } 4996 4997 public String fhirType() { 4998 return "Conformance.rest.resource.interaction"; 4999 5000 } 5001 5002 } 5003 5004 @Block() 5005 public static class ConformanceRestResourceSearchParamComponent extends BackboneElement 5006 implements IBaseBackboneElement { 5007 /** 5008 * The name of the search parameter used in the interface. 5009 */ 5010 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 5011 @Description(shortDefinition = "Name of search parameter", formalDefinition = "The name of the search parameter used in the interface.") 5012 protected StringType name; 5013 5014 /** 5015 * An absolute URI that is a formal reference to where this parameter was first 5016 * defined, so that a client can be confident of the meaning of the search 5017 * parameter (a reference to [[[SearchParameter.url]]]). 5018 */ 5019 @Child(name = "definition", type = { 5020 UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5021 @Description(shortDefinition = "Source of definition for parameter", formalDefinition = "An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]).") 5022 protected UriType definition; 5023 5024 /** 5025 * The type of value a search parameter refers to, and how the content is 5026 * interpreted. 5027 */ 5028 @Child(name = "type", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 5029 @Description(shortDefinition = "number | date | string | token | reference | composite | quantity | uri", formalDefinition = "The type of value a search parameter refers to, and how the content is interpreted.") 5030 protected Enumeration<SearchParamType> type; 5031 5032 /** 5033 * This allows documentation of any distinct behaviors about how the search 5034 * parameter is used. For example, text matching algorithms. 5035 */ 5036 @Child(name = "documentation", type = { 5037 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 5038 @Description(shortDefinition = "Server-specific usage", formalDefinition = "This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.") 5039 protected StringType documentation; 5040 5041 /** 5042 * Types of resource (if a resource is referenced). 5043 */ 5044 @Child(name = "target", type = { 5045 CodeType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5046 @Description(shortDefinition = "Types of resource (if a resource reference)", formalDefinition = "Types of resource (if a resource is referenced).") 5047 protected List<CodeType> target; 5048 5049 /** 5050 * A modifier supported for the search parameter. 5051 */ 5052 @Child(name = "modifier", type = { 5053 CodeType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5054 @Description(shortDefinition = "missing | exact | contains | not | text | in | not-in | below | above | type", formalDefinition = "A modifier supported for the search parameter.") 5055 protected List<Enumeration<SearchModifierCode>> modifier; 5056 5057 /** 5058 * Contains the names of any search parameters which may be chained to the 5059 * containing search parameter. Chained parameters may be added to search 5060 * parameters of type reference, and specify that resources will only be 5061 * returned if they contain a reference to a resource which matches the chained 5062 * parameter value. Values for this field should be drawn from 5063 * Conformance.rest.resource.searchParam.name on the target resource type. 5064 */ 5065 @Child(name = "chain", type = { 5066 StringType.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5067 @Description(shortDefinition = "Chained names supported", formalDefinition = "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from Conformance.rest.resource.searchParam.name on the target resource type.") 5068 protected List<StringType> chain; 5069 5070 private static final long serialVersionUID = -1020405086L; 5071 5072 /* 5073 * Constructor 5074 */ 5075 public ConformanceRestResourceSearchParamComponent() { 5076 super(); 5077 } 5078 5079 /* 5080 * Constructor 5081 */ 5082 public ConformanceRestResourceSearchParamComponent(StringType name, Enumeration<SearchParamType> type) { 5083 super(); 5084 this.name = name; 5085 this.type = type; 5086 } 5087 5088 /** 5089 * @return {@link #name} (The name of the search parameter used in the 5090 * interface.). This is the underlying object with id, value and 5091 * extensions. The accessor "getName" gives direct access to the value 5092 */ 5093 public StringType getNameElement() { 5094 if (this.name == null) 5095 if (Configuration.errorOnAutoCreate()) 5096 throw new Error("Attempt to auto-create ConformanceRestResourceSearchParamComponent.name"); 5097 else if (Configuration.doAutoCreate()) 5098 this.name = new StringType(); // bb 5099 return this.name; 5100 } 5101 5102 public boolean hasNameElement() { 5103 return this.name != null && !this.name.isEmpty(); 5104 } 5105 5106 public boolean hasName() { 5107 return this.name != null && !this.name.isEmpty(); 5108 } 5109 5110 /** 5111 * @param value {@link #name} (The name of the search parameter used in the 5112 * interface.). This is the underlying object with id, value and 5113 * extensions. The accessor "getName" gives direct access to the 5114 * value 5115 */ 5116 public ConformanceRestResourceSearchParamComponent setNameElement(StringType value) { 5117 this.name = value; 5118 return this; 5119 } 5120 5121 /** 5122 * @return The name of the search parameter used in the interface. 5123 */ 5124 public String getName() { 5125 return this.name == null ? null : this.name.getValue(); 5126 } 5127 5128 /** 5129 * @param value The name of the search parameter used in the interface. 5130 */ 5131 public ConformanceRestResourceSearchParamComponent setName(String value) { 5132 if (this.name == null) 5133 this.name = new StringType(); 5134 this.name.setValue(value); 5135 return this; 5136 } 5137 5138 /** 5139 * @return {@link #definition} (An absolute URI that is a formal reference to 5140 * where this parameter was first defined, so that a client can be 5141 * confident of the meaning of the search parameter (a reference to 5142 * [[[SearchParameter.url]]]).). This is the underlying object with id, 5143 * value and extensions. The accessor "getDefinition" gives direct 5144 * access to the value 5145 */ 5146 public UriType getDefinitionElement() { 5147 if (this.definition == null) 5148 if (Configuration.errorOnAutoCreate()) 5149 throw new Error("Attempt to auto-create ConformanceRestResourceSearchParamComponent.definition"); 5150 else if (Configuration.doAutoCreate()) 5151 this.definition = new UriType(); // bb 5152 return this.definition; 5153 } 5154 5155 public boolean hasDefinitionElement() { 5156 return this.definition != null && !this.definition.isEmpty(); 5157 } 5158 5159 public boolean hasDefinition() { 5160 return this.definition != null && !this.definition.isEmpty(); 5161 } 5162 5163 /** 5164 * @param value {@link #definition} (An absolute URI that is a formal reference 5165 * to where this parameter was first defined, so that a client can 5166 * be confident of the meaning of the search parameter (a reference 5167 * to [[[SearchParameter.url]]]).). This is the underlying object 5168 * with id, value and extensions. The accessor "getDefinition" 5169 * gives direct access to the value 5170 */ 5171 public ConformanceRestResourceSearchParamComponent setDefinitionElement(UriType value) { 5172 this.definition = value; 5173 return this; 5174 } 5175 5176 /** 5177 * @return An absolute URI that is a formal reference to where this parameter 5178 * was first defined, so that a client can be confident of the meaning 5179 * of the search parameter (a reference to [[[SearchParameter.url]]]). 5180 */ 5181 public String getDefinition() { 5182 return this.definition == null ? null : this.definition.getValue(); 5183 } 5184 5185 /** 5186 * @param value An absolute URI that is a formal reference to where this 5187 * parameter was first defined, so that a client can be confident 5188 * of the meaning of the search parameter (a reference to 5189 * [[[SearchParameter.url]]]). 5190 */ 5191 public ConformanceRestResourceSearchParamComponent setDefinition(String value) { 5192 if (Utilities.noString(value)) 5193 this.definition = null; 5194 else { 5195 if (this.definition == null) 5196 this.definition = new UriType(); 5197 this.definition.setValue(value); 5198 } 5199 return this; 5200 } 5201 5202 /** 5203 * @return {@link #type} (The type of value a search parameter refers to, and 5204 * how the content is interpreted.). This is the underlying object with 5205 * id, value and extensions. The accessor "getType" gives direct access 5206 * to the value 5207 */ 5208 public Enumeration<SearchParamType> getTypeElement() { 5209 if (this.type == null) 5210 if (Configuration.errorOnAutoCreate()) 5211 throw new Error("Attempt to auto-create ConformanceRestResourceSearchParamComponent.type"); 5212 else if (Configuration.doAutoCreate()) 5213 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 5214 return this.type; 5215 } 5216 5217 public boolean hasTypeElement() { 5218 return this.type != null && !this.type.isEmpty(); 5219 } 5220 5221 public boolean hasType() { 5222 return this.type != null && !this.type.isEmpty(); 5223 } 5224 5225 /** 5226 * @param value {@link #type} (The type of value a search parameter refers to, 5227 * and how the content is interpreted.). This is the underlying 5228 * object with id, value and extensions. The accessor "getType" 5229 * gives direct access to the value 5230 */ 5231 public ConformanceRestResourceSearchParamComponent setTypeElement(Enumeration<SearchParamType> value) { 5232 this.type = value; 5233 return this; 5234 } 5235 5236 /** 5237 * @return The type of value a search parameter refers to, and how the content 5238 * is interpreted. 5239 */ 5240 public SearchParamType getType() { 5241 return this.type == null ? null : this.type.getValue(); 5242 } 5243 5244 /** 5245 * @param value The type of value a search parameter refers to, and how the 5246 * content is interpreted. 5247 */ 5248 public ConformanceRestResourceSearchParamComponent setType(SearchParamType value) { 5249 if (this.type == null) 5250 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 5251 this.type.setValue(value); 5252 return this; 5253 } 5254 5255 /** 5256 * @return {@link #documentation} (This allows documentation of any distinct 5257 * behaviors about how the search parameter is used. For example, text 5258 * matching algorithms.). This is the underlying object with id, value 5259 * and extensions. The accessor "getDocumentation" gives direct access 5260 * to the value 5261 */ 5262 public StringType getDocumentationElement() { 5263 if (this.documentation == null) 5264 if (Configuration.errorOnAutoCreate()) 5265 throw new Error("Attempt to auto-create ConformanceRestResourceSearchParamComponent.documentation"); 5266 else if (Configuration.doAutoCreate()) 5267 this.documentation = new StringType(); // bb 5268 return this.documentation; 5269 } 5270 5271 public boolean hasDocumentationElement() { 5272 return this.documentation != null && !this.documentation.isEmpty(); 5273 } 5274 5275 public boolean hasDocumentation() { 5276 return this.documentation != null && !this.documentation.isEmpty(); 5277 } 5278 5279 /** 5280 * @param value {@link #documentation} (This allows documentation of any 5281 * distinct behaviors about how the search parameter is used. For 5282 * example, text matching algorithms.). This is the underlying 5283 * object with id, value and extensions. The accessor 5284 * "getDocumentation" gives direct access to the value 5285 */ 5286 public ConformanceRestResourceSearchParamComponent setDocumentationElement(StringType value) { 5287 this.documentation = value; 5288 return this; 5289 } 5290 5291 /** 5292 * @return This allows documentation of any distinct behaviors about how the 5293 * search parameter is used. For example, text matching algorithms. 5294 */ 5295 public String getDocumentation() { 5296 return this.documentation == null ? null : this.documentation.getValue(); 5297 } 5298 5299 /** 5300 * @param value This allows documentation of any distinct behaviors about how 5301 * the search parameter is used. For example, text matching 5302 * algorithms. 5303 */ 5304 public ConformanceRestResourceSearchParamComponent setDocumentation(String value) { 5305 if (Utilities.noString(value)) 5306 this.documentation = null; 5307 else { 5308 if (this.documentation == null) 5309 this.documentation = new StringType(); 5310 this.documentation.setValue(value); 5311 } 5312 return this; 5313 } 5314 5315 /** 5316 * @return {@link #target} (Types of resource (if a resource is referenced).) 5317 */ 5318 public List<CodeType> getTarget() { 5319 if (this.target == null) 5320 this.target = new ArrayList<CodeType>(); 5321 return this.target; 5322 } 5323 5324 public boolean hasTarget() { 5325 if (this.target == null) 5326 return false; 5327 for (CodeType item : this.target) 5328 if (!item.isEmpty()) 5329 return true; 5330 return false; 5331 } 5332 5333 /** 5334 * @return {@link #target} (Types of resource (if a resource is referenced).) 5335 */ 5336 // syntactic sugar 5337 public CodeType addTargetElement() {// 2 5338 CodeType t = new CodeType(); 5339 if (this.target == null) 5340 this.target = new ArrayList<CodeType>(); 5341 this.target.add(t); 5342 return t; 5343 } 5344 5345 /** 5346 * @param value {@link #target} (Types of resource (if a resource is 5347 * referenced).) 5348 */ 5349 public ConformanceRestResourceSearchParamComponent addTarget(String value) { // 1 5350 CodeType t = new CodeType(); 5351 t.setValue(value); 5352 if (this.target == null) 5353 this.target = new ArrayList<CodeType>(); 5354 this.target.add(t); 5355 return this; 5356 } 5357 5358 /** 5359 * @param value {@link #target} (Types of resource (if a resource is 5360 * referenced).) 5361 */ 5362 public boolean hasTarget(String value) { 5363 if (this.target == null) 5364 return false; 5365 for (CodeType v : this.target) 5366 if (v.equals(value)) // code 5367 return true; 5368 return false; 5369 } 5370 5371 /** 5372 * @return {@link #modifier} (A modifier supported for the search parameter.) 5373 */ 5374 public List<Enumeration<SearchModifierCode>> getModifier() { 5375 if (this.modifier == null) 5376 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 5377 return this.modifier; 5378 } 5379 5380 public boolean hasModifier() { 5381 if (this.modifier == null) 5382 return false; 5383 for (Enumeration<SearchModifierCode> item : this.modifier) 5384 if (!item.isEmpty()) 5385 return true; 5386 return false; 5387 } 5388 5389 /** 5390 * @return {@link #modifier} (A modifier supported for the search parameter.) 5391 */ 5392 // syntactic sugar 5393 public Enumeration<SearchModifierCode> addModifierElement() {// 2 5394 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 5395 if (this.modifier == null) 5396 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 5397 this.modifier.add(t); 5398 return t; 5399 } 5400 5401 /** 5402 * @param value {@link #modifier} (A modifier supported for the search 5403 * parameter.) 5404 */ 5405 public ConformanceRestResourceSearchParamComponent addModifier(SearchModifierCode value) { // 1 5406 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 5407 t.setValue(value); 5408 if (this.modifier == null) 5409 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 5410 this.modifier.add(t); 5411 return this; 5412 } 5413 5414 /** 5415 * @param value {@link #modifier} (A modifier supported for the search 5416 * parameter.) 5417 */ 5418 public boolean hasModifier(SearchModifierCode value) { 5419 if (this.modifier == null) 5420 return false; 5421 for (Enumeration<SearchModifierCode> v : this.modifier) 5422 if (v.equals(value)) // code 5423 return true; 5424 return false; 5425 } 5426 5427 /** 5428 * @return {@link #chain} (Contains the names of any search parameters which may 5429 * be chained to the containing search parameter. Chained parameters may 5430 * be added to search parameters of type reference, and specify that 5431 * resources will only be returned if they contain a reference to a 5432 * resource which matches the chained parameter value. Values for this 5433 * field should be drawn from Conformance.rest.resource.searchParam.name 5434 * on the target resource type.) 5435 */ 5436 public List<StringType> getChain() { 5437 if (this.chain == null) 5438 this.chain = new ArrayList<StringType>(); 5439 return this.chain; 5440 } 5441 5442 public boolean hasChain() { 5443 if (this.chain == null) 5444 return false; 5445 for (StringType item : this.chain) 5446 if (!item.isEmpty()) 5447 return true; 5448 return false; 5449 } 5450 5451 /** 5452 * @return {@link #chain} (Contains the names of any search parameters which may 5453 * be chained to the containing search parameter. Chained parameters may 5454 * be added to search parameters of type reference, and specify that 5455 * resources will only be returned if they contain a reference to a 5456 * resource which matches the chained parameter value. Values for this 5457 * field should be drawn from Conformance.rest.resource.searchParam.name 5458 * on the target resource type.) 5459 */ 5460 // syntactic sugar 5461 public StringType addChainElement() {// 2 5462 StringType t = new StringType(); 5463 if (this.chain == null) 5464 this.chain = new ArrayList<StringType>(); 5465 this.chain.add(t); 5466 return t; 5467 } 5468 5469 /** 5470 * @param value {@link #chain} (Contains the names of any search parameters 5471 * which may be chained to the containing search parameter. Chained 5472 * parameters may be added to search parameters of type reference, 5473 * and specify that resources will only be returned if they contain 5474 * a reference to a resource which matches the chained parameter 5475 * value. Values for this field should be drawn from 5476 * Conformance.rest.resource.searchParam.name on the target 5477 * resource type.) 5478 */ 5479 public ConformanceRestResourceSearchParamComponent addChain(String value) { // 1 5480 StringType t = new StringType(); 5481 t.setValue(value); 5482 if (this.chain == null) 5483 this.chain = new ArrayList<StringType>(); 5484 this.chain.add(t); 5485 return this; 5486 } 5487 5488 /** 5489 * @param value {@link #chain} (Contains the names of any search parameters 5490 * which may be chained to the containing search parameter. Chained 5491 * parameters may be added to search parameters of type reference, 5492 * and specify that resources will only be returned if they contain 5493 * a reference to a resource which matches the chained parameter 5494 * value. Values for this field should be drawn from 5495 * Conformance.rest.resource.searchParam.name on the target 5496 * resource type.) 5497 */ 5498 public boolean hasChain(String value) { 5499 if (this.chain == null) 5500 return false; 5501 for (StringType v : this.chain) 5502 if (v.equals(value)) // string 5503 return true; 5504 return false; 5505 } 5506 5507 protected void listChildren(List<Property> childrenList) { 5508 super.listChildren(childrenList); 5509 childrenList.add(new Property("name", "string", "The name of the search parameter used in the interface.", 0, 5510 java.lang.Integer.MAX_VALUE, name)); 5511 childrenList.add(new Property("definition", "uri", 5512 "An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [[[SearchParameter.url]]]).", 5513 0, java.lang.Integer.MAX_VALUE, definition)); 5514 childrenList.add(new Property("type", "code", 5515 "The type of value a search parameter refers to, and how the content is interpreted.", 0, 5516 java.lang.Integer.MAX_VALUE, type)); 5517 childrenList.add(new Property("documentation", "string", 5518 "This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.", 5519 0, java.lang.Integer.MAX_VALUE, documentation)); 5520 childrenList.add(new Property("target", "code", "Types of resource (if a resource is referenced).", 0, 5521 java.lang.Integer.MAX_VALUE, target)); 5522 childrenList.add(new Property("modifier", "code", "A modifier supported for the search parameter.", 0, 5523 java.lang.Integer.MAX_VALUE, modifier)); 5524 childrenList.add(new Property("chain", "string", 5525 "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference, and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from Conformance.rest.resource.searchParam.name on the target resource type.", 5526 0, java.lang.Integer.MAX_VALUE, chain)); 5527 } 5528 5529 @Override 5530 public void setProperty(String name, Base value) throws FHIRException { 5531 if (name.equals("name")) 5532 this.name = castToString(value); // StringType 5533 else if (name.equals("definition")) 5534 this.definition = castToUri(value); // UriType 5535 else if (name.equals("type")) 5536 this.type = new SearchParamTypeEnumFactory().fromType(value); // Enumeration<SearchParamType> 5537 else if (name.equals("documentation")) 5538 this.documentation = castToString(value); // StringType 5539 else if (name.equals("target")) 5540 this.getTarget().add(castToCode(value)); 5541 else if (name.equals("modifier")) 5542 this.getModifier().add(new SearchModifierCodeEnumFactory().fromType(value)); 5543 else if (name.equals("chain")) 5544 this.getChain().add(castToString(value)); 5545 else 5546 super.setProperty(name, value); 5547 } 5548 5549 @Override 5550 public Base addChild(String name) throws FHIRException { 5551 if (name.equals("name")) { 5552 throw new FHIRException("Cannot call addChild on a singleton property Conformance.name"); 5553 } else if (name.equals("definition")) { 5554 throw new FHIRException("Cannot call addChild on a singleton property Conformance.definition"); 5555 } else if (name.equals("type")) { 5556 throw new FHIRException("Cannot call addChild on a singleton property Conformance.type"); 5557 } else if (name.equals("documentation")) { 5558 throw new FHIRException("Cannot call addChild on a singleton property Conformance.documentation"); 5559 } else if (name.equals("target")) { 5560 throw new FHIRException("Cannot call addChild on a singleton property Conformance.target"); 5561 } else if (name.equals("modifier")) { 5562 throw new FHIRException("Cannot call addChild on a singleton property Conformance.modifier"); 5563 } else if (name.equals("chain")) { 5564 throw new FHIRException("Cannot call addChild on a singleton property Conformance.chain"); 5565 } else 5566 return super.addChild(name); 5567 } 5568 5569 public ConformanceRestResourceSearchParamComponent copy() { 5570 ConformanceRestResourceSearchParamComponent dst = new ConformanceRestResourceSearchParamComponent(); 5571 copyValues(dst); 5572 dst.name = name == null ? null : name.copy(); 5573 dst.definition = definition == null ? null : definition.copy(); 5574 dst.type = type == null ? null : type.copy(); 5575 dst.documentation = documentation == null ? null : documentation.copy(); 5576 if (target != null) { 5577 dst.target = new ArrayList<CodeType>(); 5578 for (CodeType i : target) 5579 dst.target.add(i.copy()); 5580 } 5581 ; 5582 if (modifier != null) { 5583 dst.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 5584 for (Enumeration<SearchModifierCode> i : modifier) 5585 dst.modifier.add(i.copy()); 5586 } 5587 ; 5588 if (chain != null) { 5589 dst.chain = new ArrayList<StringType>(); 5590 for (StringType i : chain) 5591 dst.chain.add(i.copy()); 5592 } 5593 ; 5594 return dst; 5595 } 5596 5597 @Override 5598 public boolean equalsDeep(Base other) { 5599 if (!super.equalsDeep(other)) 5600 return false; 5601 if (!(other instanceof ConformanceRestResourceSearchParamComponent)) 5602 return false; 5603 ConformanceRestResourceSearchParamComponent o = (ConformanceRestResourceSearchParamComponent) other; 5604 return compareDeep(name, o.name, true) && compareDeep(definition, o.definition, true) 5605 && compareDeep(type, o.type, true) && compareDeep(documentation, o.documentation, true) 5606 && compareDeep(target, o.target, true) && compareDeep(modifier, o.modifier, true) 5607 && compareDeep(chain, o.chain, true); 5608 } 5609 5610 @Override 5611 public boolean equalsShallow(Base other) { 5612 if (!super.equalsShallow(other)) 5613 return false; 5614 if (!(other instanceof ConformanceRestResourceSearchParamComponent)) 5615 return false; 5616 ConformanceRestResourceSearchParamComponent o = (ConformanceRestResourceSearchParamComponent) other; 5617 return compareValues(name, o.name, true) && compareValues(definition, o.definition, true) 5618 && compareValues(type, o.type, true) && compareValues(documentation, o.documentation, true) 5619 && compareValues(target, o.target, true) && compareValues(modifier, o.modifier, true) 5620 && compareValues(chain, o.chain, true); 5621 } 5622 5623 public boolean isEmpty() { 5624 return super.isEmpty() && (name == null || name.isEmpty()) && (definition == null || definition.isEmpty()) 5625 && (type == null || type.isEmpty()) && (documentation == null || documentation.isEmpty()) 5626 && (target == null || target.isEmpty()) && (modifier == null || modifier.isEmpty()) 5627 && (chain == null || chain.isEmpty()); 5628 } 5629 5630 public String fhirType() { 5631 return "Conformance.rest.resource.searchParam"; 5632 5633 } 5634 5635 } 5636 5637 @Block() 5638 public static class SystemInteractionComponent extends BackboneElement implements IBaseBackboneElement { 5639 /** 5640 * A coded identifier of the operation, supported by the system. 5641 */ 5642 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 5643 @Description(shortDefinition = "transaction | search-system | history-system", formalDefinition = "A coded identifier of the operation, supported by the system.") 5644 protected Enumeration<SystemRestfulInteraction> code; 5645 5646 /** 5647 * Guidance specific to the implementation of this operation, such as 5648 * limitations on the kind of transactions allowed, or information about system 5649 * wide search is implemented. 5650 */ 5651 @Child(name = "documentation", type = { 5652 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5653 @Description(shortDefinition = "Anything special about operation behavior", formalDefinition = "Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.") 5654 protected StringType documentation; 5655 5656 private static final long serialVersionUID = 510675287L; 5657 5658 /* 5659 * Constructor 5660 */ 5661 public SystemInteractionComponent() { 5662 super(); 5663 } 5664 5665 /* 5666 * Constructor 5667 */ 5668 public SystemInteractionComponent(Enumeration<SystemRestfulInteraction> code) { 5669 super(); 5670 this.code = code; 5671 } 5672 5673 /** 5674 * @return {@link #code} (A coded identifier of the operation, supported by the 5675 * system.). This is the underlying object with id, value and 5676 * extensions. The accessor "getCode" gives direct access to the value 5677 */ 5678 public Enumeration<SystemRestfulInteraction> getCodeElement() { 5679 if (this.code == null) 5680 if (Configuration.errorOnAutoCreate()) 5681 throw new Error("Attempt to auto-create SystemInteractionComponent.code"); 5682 else if (Configuration.doAutoCreate()) 5683 this.code = new Enumeration<SystemRestfulInteraction>(new SystemRestfulInteractionEnumFactory()); // bb 5684 return this.code; 5685 } 5686 5687 public boolean hasCodeElement() { 5688 return this.code != null && !this.code.isEmpty(); 5689 } 5690 5691 public boolean hasCode() { 5692 return this.code != null && !this.code.isEmpty(); 5693 } 5694 5695 /** 5696 * @param value {@link #code} (A coded identifier of the operation, supported by 5697 * the system.). This is the underlying object with id, value and 5698 * extensions. The accessor "getCode" gives direct access to the 5699 * value 5700 */ 5701 public SystemInteractionComponent setCodeElement(Enumeration<SystemRestfulInteraction> value) { 5702 this.code = value; 5703 return this; 5704 } 5705 5706 /** 5707 * @return A coded identifier of the operation, supported by the system. 5708 */ 5709 public SystemRestfulInteraction getCode() { 5710 return this.code == null ? null : this.code.getValue(); 5711 } 5712 5713 /** 5714 * @param value A coded identifier of the operation, supported by the system. 5715 */ 5716 public SystemInteractionComponent setCode(SystemRestfulInteraction value) { 5717 if (this.code == null) 5718 this.code = new Enumeration<SystemRestfulInteraction>(new SystemRestfulInteractionEnumFactory()); 5719 this.code.setValue(value); 5720 return this; 5721 } 5722 5723 /** 5724 * @return {@link #documentation} (Guidance specific to the implementation of 5725 * this operation, such as limitations on the kind of transactions 5726 * allowed, or information about system wide search is implemented.). 5727 * This is the underlying object with id, value and extensions. The 5728 * accessor "getDocumentation" gives direct access to the value 5729 */ 5730 public StringType getDocumentationElement() { 5731 if (this.documentation == null) 5732 if (Configuration.errorOnAutoCreate()) 5733 throw new Error("Attempt to auto-create SystemInteractionComponent.documentation"); 5734 else if (Configuration.doAutoCreate()) 5735 this.documentation = new StringType(); // bb 5736 return this.documentation; 5737 } 5738 5739 public boolean hasDocumentationElement() { 5740 return this.documentation != null && !this.documentation.isEmpty(); 5741 } 5742 5743 public boolean hasDocumentation() { 5744 return this.documentation != null && !this.documentation.isEmpty(); 5745 } 5746 5747 /** 5748 * @param value {@link #documentation} (Guidance specific to the implementation 5749 * of this operation, such as limitations on the kind of 5750 * transactions allowed, or information about system wide search is 5751 * implemented.). This is the underlying object with id, value and 5752 * extensions. The accessor "getDocumentation" gives direct access 5753 * to the value 5754 */ 5755 public SystemInteractionComponent setDocumentationElement(StringType value) { 5756 this.documentation = value; 5757 return this; 5758 } 5759 5760 /** 5761 * @return Guidance specific to the implementation of this operation, such as 5762 * limitations on the kind of transactions allowed, or information about 5763 * system wide search is implemented. 5764 */ 5765 public String getDocumentation() { 5766 return this.documentation == null ? null : this.documentation.getValue(); 5767 } 5768 5769 /** 5770 * @param value Guidance specific to the implementation of this operation, such 5771 * as limitations on the kind of transactions allowed, or 5772 * information about system wide search is implemented. 5773 */ 5774 public SystemInteractionComponent setDocumentation(String value) { 5775 if (Utilities.noString(value)) 5776 this.documentation = null; 5777 else { 5778 if (this.documentation == null) 5779 this.documentation = new StringType(); 5780 this.documentation.setValue(value); 5781 } 5782 return this; 5783 } 5784 5785 protected void listChildren(List<Property> childrenList) { 5786 super.listChildren(childrenList); 5787 childrenList.add(new Property("code", "code", "A coded identifier of the operation, supported by the system.", 0, 5788 java.lang.Integer.MAX_VALUE, code)); 5789 childrenList.add(new Property("documentation", "string", 5790 "Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.", 5791 0, java.lang.Integer.MAX_VALUE, documentation)); 5792 } 5793 5794 @Override 5795 public void setProperty(String name, Base value) throws FHIRException { 5796 if (name.equals("code")) 5797 this.code = new SystemRestfulInteractionEnumFactory().fromType(value); // Enumeration<SystemRestfulInteraction> 5798 else if (name.equals("documentation")) 5799 this.documentation = castToString(value); // StringType 5800 else 5801 super.setProperty(name, value); 5802 } 5803 5804 @Override 5805 public Base addChild(String name) throws FHIRException { 5806 if (name.equals("code")) { 5807 throw new FHIRException("Cannot call addChild on a singleton property Conformance.code"); 5808 } else if (name.equals("documentation")) { 5809 throw new FHIRException("Cannot call addChild on a singleton property Conformance.documentation"); 5810 } else 5811 return super.addChild(name); 5812 } 5813 5814 public SystemInteractionComponent copy() { 5815 SystemInteractionComponent dst = new SystemInteractionComponent(); 5816 copyValues(dst); 5817 dst.code = code == null ? null : code.copy(); 5818 dst.documentation = documentation == null ? null : documentation.copy(); 5819 return dst; 5820 } 5821 5822 @Override 5823 public boolean equalsDeep(Base other) { 5824 if (!super.equalsDeep(other)) 5825 return false; 5826 if (!(other instanceof SystemInteractionComponent)) 5827 return false; 5828 SystemInteractionComponent o = (SystemInteractionComponent) other; 5829 return compareDeep(code, o.code, true) && compareDeep(documentation, o.documentation, true); 5830 } 5831 5832 @Override 5833 public boolean equalsShallow(Base other) { 5834 if (!super.equalsShallow(other)) 5835 return false; 5836 if (!(other instanceof SystemInteractionComponent)) 5837 return false; 5838 SystemInteractionComponent o = (SystemInteractionComponent) other; 5839 return compareValues(code, o.code, true) && compareValues(documentation, o.documentation, true); 5840 } 5841 5842 public boolean isEmpty() { 5843 return super.isEmpty() && (code == null || code.isEmpty()) && (documentation == null || documentation.isEmpty()); 5844 } 5845 5846 public String fhirType() { 5847 return "Conformance.rest.interaction"; 5848 5849 } 5850 5851 } 5852 5853 @Block() 5854 public static class ConformanceRestOperationComponent extends BackboneElement implements IBaseBackboneElement { 5855 /** 5856 * The name of a query, which is used in the _query parameter when the query is 5857 * called. 5858 */ 5859 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 5860 @Description(shortDefinition = "Name by which the operation/query is invoked", formalDefinition = "The name of a query, which is used in the _query parameter when the query is called.") 5861 protected StringType name; 5862 5863 /** 5864 * Where the formal definition can be found. 5865 */ 5866 @Child(name = "definition", type = { 5867 OperationDefinition.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 5868 @Description(shortDefinition = "The defined operation/query", formalDefinition = "Where the formal definition can be found.") 5869 protected Reference definition; 5870 5871 /** 5872 * The actual object that is the target of the reference (Where the formal 5873 * definition can be found.) 5874 */ 5875 protected OperationDefinition definitionTarget; 5876 5877 private static final long serialVersionUID = 122107272L; 5878 5879 /* 5880 * Constructor 5881 */ 5882 public ConformanceRestOperationComponent() { 5883 super(); 5884 } 5885 5886 /* 5887 * Constructor 5888 */ 5889 public ConformanceRestOperationComponent(StringType name, Reference definition) { 5890 super(); 5891 this.name = name; 5892 this.definition = definition; 5893 } 5894 5895 /** 5896 * @return {@link #name} (The name of a query, which is used in the _query 5897 * parameter when the query is called.). This is the underlying object 5898 * with id, value and extensions. The accessor "getName" gives direct 5899 * access to the value 5900 */ 5901 public StringType getNameElement() { 5902 if (this.name == null) 5903 if (Configuration.errorOnAutoCreate()) 5904 throw new Error("Attempt to auto-create ConformanceRestOperationComponent.name"); 5905 else if (Configuration.doAutoCreate()) 5906 this.name = new StringType(); // bb 5907 return this.name; 5908 } 5909 5910 public boolean hasNameElement() { 5911 return this.name != null && !this.name.isEmpty(); 5912 } 5913 5914 public boolean hasName() { 5915 return this.name != null && !this.name.isEmpty(); 5916 } 5917 5918 /** 5919 * @param value {@link #name} (The name of a query, which is used in the _query 5920 * parameter when the query is called.). This is the underlying 5921 * object with id, value and extensions. The accessor "getName" 5922 * gives direct access to the value 5923 */ 5924 public ConformanceRestOperationComponent setNameElement(StringType value) { 5925 this.name = value; 5926 return this; 5927 } 5928 5929 /** 5930 * @return The name of a query, which is used in the _query parameter when the 5931 * query is called. 5932 */ 5933 public String getName() { 5934 return this.name == null ? null : this.name.getValue(); 5935 } 5936 5937 /** 5938 * @param value The name of a query, which is used in the _query parameter when 5939 * the query is called. 5940 */ 5941 public ConformanceRestOperationComponent setName(String value) { 5942 if (this.name == null) 5943 this.name = new StringType(); 5944 this.name.setValue(value); 5945 return this; 5946 } 5947 5948 /** 5949 * @return {@link #definition} (Where the formal definition can be found.) 5950 */ 5951 public Reference getDefinition() { 5952 if (this.definition == null) 5953 if (Configuration.errorOnAutoCreate()) 5954 throw new Error("Attempt to auto-create ConformanceRestOperationComponent.definition"); 5955 else if (Configuration.doAutoCreate()) 5956 this.definition = new Reference(); // cc 5957 return this.definition; 5958 } 5959 5960 public boolean hasDefinition() { 5961 return this.definition != null && !this.definition.isEmpty(); 5962 } 5963 5964 /** 5965 * @param value {@link #definition} (Where the formal definition can be found.) 5966 */ 5967 public ConformanceRestOperationComponent setDefinition(Reference value) { 5968 this.definition = value; 5969 return this; 5970 } 5971 5972 /** 5973 * @return {@link #definition} The actual object that is the target of the 5974 * reference. The reference library doesn't populate this, but you can 5975 * use it to hold the resource if you resolve it. (Where the formal 5976 * definition can be found.) 5977 */ 5978 public OperationDefinition getDefinitionTarget() { 5979 if (this.definitionTarget == null) 5980 if (Configuration.errorOnAutoCreate()) 5981 throw new Error("Attempt to auto-create ConformanceRestOperationComponent.definition"); 5982 else if (Configuration.doAutoCreate()) 5983 this.definitionTarget = new OperationDefinition(); // aa 5984 return this.definitionTarget; 5985 } 5986 5987 /** 5988 * @param value {@link #definition} The actual object that is the target of the 5989 * reference. The reference library doesn't use these, but you can 5990 * use it to hold the resource if you resolve it. (Where the formal 5991 * definition can be found.) 5992 */ 5993 public ConformanceRestOperationComponent setDefinitionTarget(OperationDefinition value) { 5994 this.definitionTarget = value; 5995 return this; 5996 } 5997 5998 protected void listChildren(List<Property> childrenList) { 5999 super.listChildren(childrenList); 6000 childrenList.add(new Property("name", "string", 6001 "The name of a query, which is used in the _query parameter when the query is called.", 0, 6002 java.lang.Integer.MAX_VALUE, name)); 6003 childrenList.add(new Property("definition", "Reference(OperationDefinition)", 6004 "Where the formal definition can be found.", 0, java.lang.Integer.MAX_VALUE, definition)); 6005 } 6006 6007 @Override 6008 public void setProperty(String name, Base value) throws FHIRException { 6009 if (name.equals("name")) 6010 this.name = castToString(value); // StringType 6011 else if (name.equals("definition")) 6012 this.definition = castToReference(value); // Reference 6013 else 6014 super.setProperty(name, value); 6015 } 6016 6017 @Override 6018 public Base addChild(String name) throws FHIRException { 6019 if (name.equals("name")) { 6020 throw new FHIRException("Cannot call addChild on a singleton property Conformance.name"); 6021 } else if (name.equals("definition")) { 6022 this.definition = new Reference(); 6023 return this.definition; 6024 } else 6025 return super.addChild(name); 6026 } 6027 6028 public ConformanceRestOperationComponent copy() { 6029 ConformanceRestOperationComponent dst = new ConformanceRestOperationComponent(); 6030 copyValues(dst); 6031 dst.name = name == null ? null : name.copy(); 6032 dst.definition = definition == null ? null : definition.copy(); 6033 return dst; 6034 } 6035 6036 @Override 6037 public boolean equalsDeep(Base other) { 6038 if (!super.equalsDeep(other)) 6039 return false; 6040 if (!(other instanceof ConformanceRestOperationComponent)) 6041 return false; 6042 ConformanceRestOperationComponent o = (ConformanceRestOperationComponent) other; 6043 return compareDeep(name, o.name, true) && compareDeep(definition, o.definition, true); 6044 } 6045 6046 @Override 6047 public boolean equalsShallow(Base other) { 6048 if (!super.equalsShallow(other)) 6049 return false; 6050 if (!(other instanceof ConformanceRestOperationComponent)) 6051 return false; 6052 ConformanceRestOperationComponent o = (ConformanceRestOperationComponent) other; 6053 return compareValues(name, o.name, true); 6054 } 6055 6056 public boolean isEmpty() { 6057 return super.isEmpty() && (name == null || name.isEmpty()) && (definition == null || definition.isEmpty()); 6058 } 6059 6060 public String fhirType() { 6061 return "Conformance.rest.operation"; 6062 6063 } 6064 6065 } 6066 6067 @Block() 6068 public static class ConformanceMessagingComponent extends BackboneElement implements IBaseBackboneElement { 6069 /** 6070 * An endpoint (network accessible address) to which messages and/or replies are 6071 * to be sent. 6072 */ 6073 @Child(name = "endpoint", type = {}, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6074 @Description(shortDefinition = "A messaging service end-point", formalDefinition = "An endpoint (network accessible address) to which messages and/or replies are to be sent.") 6075 protected List<ConformanceMessagingEndpointComponent> endpoint; 6076 6077 /** 6078 * Length if the receiver's reliable messaging cache in minutes (if a receiver) 6079 * or how long the cache length on the receiver should be (if a sender). 6080 */ 6081 @Child(name = "reliableCache", type = { 6082 UnsignedIntType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6083 @Description(shortDefinition = "Reliable Message Cache Length (min)", formalDefinition = "Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).") 6084 protected UnsignedIntType reliableCache; 6085 6086 /** 6087 * Documentation about the system's messaging capabilities for this endpoint not 6088 * otherwise documented by the conformance statement. For example, process for 6089 * becoming an authorized messaging exchange partner. 6090 */ 6091 @Child(name = "documentation", type = { 6092 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6093 @Description(shortDefinition = "Messaging interface behavior details", formalDefinition = "Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the conformance statement. For example, process for becoming an authorized messaging exchange partner.") 6094 protected StringType documentation; 6095 6096 /** 6097 * A description of the solution's support for an event at this end-point. 6098 */ 6099 @Child(name = "event", type = {}, order = 4, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6100 @Description(shortDefinition = "Declare support for this event", formalDefinition = "A description of the solution's support for an event at this end-point.") 6101 protected List<ConformanceMessagingEventComponent> event; 6102 6103 private static final long serialVersionUID = -712362545L; 6104 6105 /* 6106 * Constructor 6107 */ 6108 public ConformanceMessagingComponent() { 6109 super(); 6110 } 6111 6112 /** 6113 * @return {@link #endpoint} (An endpoint (network accessible address) to which 6114 * messages and/or replies are to be sent.) 6115 */ 6116 public List<ConformanceMessagingEndpointComponent> getEndpoint() { 6117 if (this.endpoint == null) 6118 this.endpoint = new ArrayList<ConformanceMessagingEndpointComponent>(); 6119 return this.endpoint; 6120 } 6121 6122 public boolean hasEndpoint() { 6123 if (this.endpoint == null) 6124 return false; 6125 for (ConformanceMessagingEndpointComponent item : this.endpoint) 6126 if (!item.isEmpty()) 6127 return true; 6128 return false; 6129 } 6130 6131 /** 6132 * @return {@link #endpoint} (An endpoint (network accessible address) to which 6133 * messages and/or replies are to be sent.) 6134 */ 6135 // syntactic sugar 6136 public ConformanceMessagingEndpointComponent addEndpoint() { // 3 6137 ConformanceMessagingEndpointComponent t = new ConformanceMessagingEndpointComponent(); 6138 if (this.endpoint == null) 6139 this.endpoint = new ArrayList<ConformanceMessagingEndpointComponent>(); 6140 this.endpoint.add(t); 6141 return t; 6142 } 6143 6144 // syntactic sugar 6145 public ConformanceMessagingComponent addEndpoint(ConformanceMessagingEndpointComponent t) { // 3 6146 if (t == null) 6147 return this; 6148 if (this.endpoint == null) 6149 this.endpoint = new ArrayList<ConformanceMessagingEndpointComponent>(); 6150 this.endpoint.add(t); 6151 return this; 6152 } 6153 6154 /** 6155 * @return {@link #reliableCache} (Length if the receiver's reliable messaging 6156 * cache in minutes (if a receiver) or how long the cache length on the 6157 * receiver should be (if a sender).). This is the underlying object 6158 * with id, value and extensions. The accessor "getReliableCache" gives 6159 * direct access to the value 6160 */ 6161 public UnsignedIntType getReliableCacheElement() { 6162 if (this.reliableCache == null) 6163 if (Configuration.errorOnAutoCreate()) 6164 throw new Error("Attempt to auto-create ConformanceMessagingComponent.reliableCache"); 6165 else if (Configuration.doAutoCreate()) 6166 this.reliableCache = new UnsignedIntType(); // bb 6167 return this.reliableCache; 6168 } 6169 6170 public boolean hasReliableCacheElement() { 6171 return this.reliableCache != null && !this.reliableCache.isEmpty(); 6172 } 6173 6174 public boolean hasReliableCache() { 6175 return this.reliableCache != null && !this.reliableCache.isEmpty(); 6176 } 6177 6178 /** 6179 * @param value {@link #reliableCache} (Length if the receiver's reliable 6180 * messaging cache in minutes (if a receiver) or how long the cache 6181 * length on the receiver should be (if a sender).). This is the 6182 * underlying object with id, value and extensions. The accessor 6183 * "getReliableCache" gives direct access to the value 6184 */ 6185 public ConformanceMessagingComponent setReliableCacheElement(UnsignedIntType value) { 6186 this.reliableCache = value; 6187 return this; 6188 } 6189 6190 /** 6191 * @return Length if the receiver's reliable messaging cache in minutes (if a 6192 * receiver) or how long the cache length on the receiver should be (if 6193 * a sender). 6194 */ 6195 public int getReliableCache() { 6196 return this.reliableCache == null || this.reliableCache.isEmpty() ? 0 : this.reliableCache.getValue(); 6197 } 6198 6199 /** 6200 * @param value Length if the receiver's reliable messaging cache in minutes (if 6201 * a receiver) or how long the cache length on the receiver should 6202 * be (if a sender). 6203 */ 6204 public ConformanceMessagingComponent setReliableCache(int value) { 6205 if (this.reliableCache == null) 6206 this.reliableCache = new UnsignedIntType(); 6207 this.reliableCache.setValue(value); 6208 return this; 6209 } 6210 6211 /** 6212 * @return {@link #documentation} (Documentation about the system's messaging 6213 * capabilities for this endpoint not otherwise documented by the 6214 * conformance statement. For example, process for becoming an 6215 * authorized messaging exchange partner.). This is the underlying 6216 * object with id, value and extensions. The accessor "getDocumentation" 6217 * gives direct access to the value 6218 */ 6219 public StringType getDocumentationElement() { 6220 if (this.documentation == null) 6221 if (Configuration.errorOnAutoCreate()) 6222 throw new Error("Attempt to auto-create ConformanceMessagingComponent.documentation"); 6223 else if (Configuration.doAutoCreate()) 6224 this.documentation = new StringType(); // bb 6225 return this.documentation; 6226 } 6227 6228 public boolean hasDocumentationElement() { 6229 return this.documentation != null && !this.documentation.isEmpty(); 6230 } 6231 6232 public boolean hasDocumentation() { 6233 return this.documentation != null && !this.documentation.isEmpty(); 6234 } 6235 6236 /** 6237 * @param value {@link #documentation} (Documentation about the system's 6238 * messaging capabilities for this endpoint not otherwise 6239 * documented by the conformance statement. For example, process 6240 * for becoming an authorized messaging exchange partner.). This is 6241 * the underlying object with id, value and extensions. The 6242 * accessor "getDocumentation" gives direct access to the value 6243 */ 6244 public ConformanceMessagingComponent setDocumentationElement(StringType value) { 6245 this.documentation = value; 6246 return this; 6247 } 6248 6249 /** 6250 * @return Documentation about the system's messaging capabilities for this 6251 * endpoint not otherwise documented by the conformance statement. For 6252 * example, process for becoming an authorized messaging exchange 6253 * partner. 6254 */ 6255 public String getDocumentation() { 6256 return this.documentation == null ? null : this.documentation.getValue(); 6257 } 6258 6259 /** 6260 * @param value Documentation about the system's messaging capabilities for this 6261 * endpoint not otherwise documented by the conformance statement. 6262 * For example, process for becoming an authorized messaging 6263 * exchange partner. 6264 */ 6265 public ConformanceMessagingComponent setDocumentation(String value) { 6266 if (Utilities.noString(value)) 6267 this.documentation = null; 6268 else { 6269 if (this.documentation == null) 6270 this.documentation = new StringType(); 6271 this.documentation.setValue(value); 6272 } 6273 return this; 6274 } 6275 6276 /** 6277 * @return {@link #event} (A description of the solution's support for an event 6278 * at this end-point.) 6279 */ 6280 public List<ConformanceMessagingEventComponent> getEvent() { 6281 if (this.event == null) 6282 this.event = new ArrayList<ConformanceMessagingEventComponent>(); 6283 return this.event; 6284 } 6285 6286 public boolean hasEvent() { 6287 if (this.event == null) 6288 return false; 6289 for (ConformanceMessagingEventComponent item : this.event) 6290 if (!item.isEmpty()) 6291 return true; 6292 return false; 6293 } 6294 6295 /** 6296 * @return {@link #event} (A description of the solution's support for an event 6297 * at this end-point.) 6298 */ 6299 // syntactic sugar 6300 public ConformanceMessagingEventComponent addEvent() { // 3 6301 ConformanceMessagingEventComponent t = new ConformanceMessagingEventComponent(); 6302 if (this.event == null) 6303 this.event = new ArrayList<ConformanceMessagingEventComponent>(); 6304 this.event.add(t); 6305 return t; 6306 } 6307 6308 // syntactic sugar 6309 public ConformanceMessagingComponent addEvent(ConformanceMessagingEventComponent t) { // 3 6310 if (t == null) 6311 return this; 6312 if (this.event == null) 6313 this.event = new ArrayList<ConformanceMessagingEventComponent>(); 6314 this.event.add(t); 6315 return this; 6316 } 6317 6318 protected void listChildren(List<Property> childrenList) { 6319 super.listChildren(childrenList); 6320 childrenList.add(new Property("endpoint", "", 6321 "An endpoint (network accessible address) to which messages and/or replies are to be sent.", 0, 6322 java.lang.Integer.MAX_VALUE, endpoint)); 6323 childrenList.add(new Property("reliableCache", "unsignedInt", 6324 "Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).", 6325 0, java.lang.Integer.MAX_VALUE, reliableCache)); 6326 childrenList.add(new Property("documentation", "string", 6327 "Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the conformance statement. For example, process for becoming an authorized messaging exchange partner.", 6328 0, java.lang.Integer.MAX_VALUE, documentation)); 6329 childrenList 6330 .add(new Property("event", "", "A description of the solution's support for an event at this end-point.", 0, 6331 java.lang.Integer.MAX_VALUE, event)); 6332 } 6333 6334 @Override 6335 public void setProperty(String name, Base value) throws FHIRException { 6336 if (name.equals("endpoint")) 6337 this.getEndpoint().add((ConformanceMessagingEndpointComponent) value); 6338 else if (name.equals("reliableCache")) 6339 this.reliableCache = castToUnsignedInt(value); // UnsignedIntType 6340 else if (name.equals("documentation")) 6341 this.documentation = castToString(value); // StringType 6342 else if (name.equals("event")) 6343 this.getEvent().add((ConformanceMessagingEventComponent) value); 6344 else 6345 super.setProperty(name, value); 6346 } 6347 6348 @Override 6349 public Base addChild(String name) throws FHIRException { 6350 if (name.equals("endpoint")) { 6351 return addEndpoint(); 6352 } else if (name.equals("reliableCache")) { 6353 throw new FHIRException("Cannot call addChild on a singleton property Conformance.reliableCache"); 6354 } else if (name.equals("documentation")) { 6355 throw new FHIRException("Cannot call addChild on a singleton property Conformance.documentation"); 6356 } else if (name.equals("event")) { 6357 return addEvent(); 6358 } else 6359 return super.addChild(name); 6360 } 6361 6362 public ConformanceMessagingComponent copy() { 6363 ConformanceMessagingComponent dst = new ConformanceMessagingComponent(); 6364 copyValues(dst); 6365 if (endpoint != null) { 6366 dst.endpoint = new ArrayList<ConformanceMessagingEndpointComponent>(); 6367 for (ConformanceMessagingEndpointComponent i : endpoint) 6368 dst.endpoint.add(i.copy()); 6369 } 6370 ; 6371 dst.reliableCache = reliableCache == null ? null : reliableCache.copy(); 6372 dst.documentation = documentation == null ? null : documentation.copy(); 6373 if (event != null) { 6374 dst.event = new ArrayList<ConformanceMessagingEventComponent>(); 6375 for (ConformanceMessagingEventComponent i : event) 6376 dst.event.add(i.copy()); 6377 } 6378 ; 6379 return dst; 6380 } 6381 6382 @Override 6383 public boolean equalsDeep(Base other) { 6384 if (!super.equalsDeep(other)) 6385 return false; 6386 if (!(other instanceof ConformanceMessagingComponent)) 6387 return false; 6388 ConformanceMessagingComponent o = (ConformanceMessagingComponent) other; 6389 return compareDeep(endpoint, o.endpoint, true) && compareDeep(reliableCache, o.reliableCache, true) 6390 && compareDeep(documentation, o.documentation, true) && compareDeep(event, o.event, true); 6391 } 6392 6393 @Override 6394 public boolean equalsShallow(Base other) { 6395 if (!super.equalsShallow(other)) 6396 return false; 6397 if (!(other instanceof ConformanceMessagingComponent)) 6398 return false; 6399 ConformanceMessagingComponent o = (ConformanceMessagingComponent) other; 6400 return compareValues(reliableCache, o.reliableCache, true) && compareValues(documentation, o.documentation, true); 6401 } 6402 6403 public boolean isEmpty() { 6404 return super.isEmpty() && (endpoint == null || endpoint.isEmpty()) 6405 && (reliableCache == null || reliableCache.isEmpty()) && (documentation == null || documentation.isEmpty()) 6406 && (event == null || event.isEmpty()); 6407 } 6408 6409 public String fhirType() { 6410 return "Conformance.messaging"; 6411 6412 } 6413 6414 } 6415 6416 @Block() 6417 public static class ConformanceMessagingEndpointComponent extends BackboneElement implements IBaseBackboneElement { 6418 /** 6419 * A list of the messaging transport protocol(s) identifiers, supported by this 6420 * endpoint. 6421 */ 6422 @Child(name = "protocol", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6423 @Description(shortDefinition = "http | ftp | mllp +", formalDefinition = "A list of the messaging transport protocol(s) identifiers, supported by this endpoint.") 6424 protected Coding protocol; 6425 6426 /** 6427 * The network address of the end-point. For solutions that do not use network 6428 * addresses for routing, it can be just an identifier. 6429 */ 6430 @Child(name = "address", type = { UriType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 6431 @Description(shortDefinition = "Address of end-point", formalDefinition = "The network address of the end-point. For solutions that do not use network addresses for routing, it can be just an identifier.") 6432 protected UriType address; 6433 6434 private static final long serialVersionUID = 1294656428L; 6435 6436 /* 6437 * Constructor 6438 */ 6439 public ConformanceMessagingEndpointComponent() { 6440 super(); 6441 } 6442 6443 /* 6444 * Constructor 6445 */ 6446 public ConformanceMessagingEndpointComponent(Coding protocol, UriType address) { 6447 super(); 6448 this.protocol = protocol; 6449 this.address = address; 6450 } 6451 6452 /** 6453 * @return {@link #protocol} (A list of the messaging transport protocol(s) 6454 * identifiers, supported by this endpoint.) 6455 */ 6456 public Coding getProtocol() { 6457 if (this.protocol == null) 6458 if (Configuration.errorOnAutoCreate()) 6459 throw new Error("Attempt to auto-create ConformanceMessagingEndpointComponent.protocol"); 6460 else if (Configuration.doAutoCreate()) 6461 this.protocol = new Coding(); // cc 6462 return this.protocol; 6463 } 6464 6465 public boolean hasProtocol() { 6466 return this.protocol != null && !this.protocol.isEmpty(); 6467 } 6468 6469 /** 6470 * @param value {@link #protocol} (A list of the messaging transport protocol(s) 6471 * identifiers, supported by this endpoint.) 6472 */ 6473 public ConformanceMessagingEndpointComponent setProtocol(Coding value) { 6474 this.protocol = value; 6475 return this; 6476 } 6477 6478 /** 6479 * @return {@link #address} (The network address of the end-point. For solutions 6480 * that do not use network addresses for routing, it can be just an 6481 * identifier.). This is the underlying object with id, value and 6482 * extensions. The accessor "getAddress" gives direct access to the 6483 * value 6484 */ 6485 public UriType getAddressElement() { 6486 if (this.address == null) 6487 if (Configuration.errorOnAutoCreate()) 6488 throw new Error("Attempt to auto-create ConformanceMessagingEndpointComponent.address"); 6489 else if (Configuration.doAutoCreate()) 6490 this.address = new UriType(); // bb 6491 return this.address; 6492 } 6493 6494 public boolean hasAddressElement() { 6495 return this.address != null && !this.address.isEmpty(); 6496 } 6497 6498 public boolean hasAddress() { 6499 return this.address != null && !this.address.isEmpty(); 6500 } 6501 6502 /** 6503 * @param value {@link #address} (The network address of the end-point. For 6504 * solutions that do not use network addresses for routing, it can 6505 * be just an identifier.). This is the underlying object with id, 6506 * value and extensions. The accessor "getAddress" gives direct 6507 * access to the value 6508 */ 6509 public ConformanceMessagingEndpointComponent setAddressElement(UriType value) { 6510 this.address = value; 6511 return this; 6512 } 6513 6514 /** 6515 * @return The network address of the end-point. For solutions that do not use 6516 * network addresses for routing, it can be just an identifier. 6517 */ 6518 public String getAddress() { 6519 return this.address == null ? null : this.address.getValue(); 6520 } 6521 6522 /** 6523 * @param value The network address of the end-point. For solutions that do not 6524 * use network addresses for routing, it can be just an identifier. 6525 */ 6526 public ConformanceMessagingEndpointComponent setAddress(String value) { 6527 if (this.address == null) 6528 this.address = new UriType(); 6529 this.address.setValue(value); 6530 return this; 6531 } 6532 6533 protected void listChildren(List<Property> childrenList) { 6534 super.listChildren(childrenList); 6535 childrenList.add(new Property("protocol", "Coding", 6536 "A list of the messaging transport protocol(s) identifiers, supported by this endpoint.", 0, 6537 java.lang.Integer.MAX_VALUE, protocol)); 6538 childrenList.add(new Property("address", "uri", 6539 "The network address of the end-point. For solutions that do not use network addresses for routing, it can be just an identifier.", 6540 0, java.lang.Integer.MAX_VALUE, address)); 6541 } 6542 6543 @Override 6544 public void setProperty(String name, Base value) throws FHIRException { 6545 if (name.equals("protocol")) 6546 this.protocol = castToCoding(value); // Coding 6547 else if (name.equals("address")) 6548 this.address = castToUri(value); // UriType 6549 else 6550 super.setProperty(name, value); 6551 } 6552 6553 @Override 6554 public Base addChild(String name) throws FHIRException { 6555 if (name.equals("protocol")) { 6556 this.protocol = new Coding(); 6557 return this.protocol; 6558 } else if (name.equals("address")) { 6559 throw new FHIRException("Cannot call addChild on a singleton property Conformance.address"); 6560 } else 6561 return super.addChild(name); 6562 } 6563 6564 public ConformanceMessagingEndpointComponent copy() { 6565 ConformanceMessagingEndpointComponent dst = new ConformanceMessagingEndpointComponent(); 6566 copyValues(dst); 6567 dst.protocol = protocol == null ? null : protocol.copy(); 6568 dst.address = address == null ? null : address.copy(); 6569 return dst; 6570 } 6571 6572 @Override 6573 public boolean equalsDeep(Base other) { 6574 if (!super.equalsDeep(other)) 6575 return false; 6576 if (!(other instanceof ConformanceMessagingEndpointComponent)) 6577 return false; 6578 ConformanceMessagingEndpointComponent o = (ConformanceMessagingEndpointComponent) other; 6579 return compareDeep(protocol, o.protocol, true) && compareDeep(address, o.address, true); 6580 } 6581 6582 @Override 6583 public boolean equalsShallow(Base other) { 6584 if (!super.equalsShallow(other)) 6585 return false; 6586 if (!(other instanceof ConformanceMessagingEndpointComponent)) 6587 return false; 6588 ConformanceMessagingEndpointComponent o = (ConformanceMessagingEndpointComponent) other; 6589 return compareValues(address, o.address, true); 6590 } 6591 6592 public boolean isEmpty() { 6593 return super.isEmpty() && (protocol == null || protocol.isEmpty()) && (address == null || address.isEmpty()); 6594 } 6595 6596 public String fhirType() { 6597 return "Conformance.messaging.endpoint"; 6598 6599 } 6600 6601 } 6602 6603 @Block() 6604 public static class ConformanceMessagingEventComponent extends BackboneElement implements IBaseBackboneElement { 6605 /** 6606 * A coded identifier of a supported messaging event. 6607 */ 6608 @Child(name = "code", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6609 @Description(shortDefinition = "Event type", formalDefinition = "A coded identifier of a supported messaging event.") 6610 protected Coding code; 6611 6612 /** 6613 * The impact of the content of the message. 6614 */ 6615 @Child(name = "category", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6616 @Description(shortDefinition = "Consequence | Currency | Notification", formalDefinition = "The impact of the content of the message.") 6617 protected Enumeration<MessageSignificanceCategory> category; 6618 6619 /** 6620 * The mode of this event declaration - whether application is sender or 6621 * receiver. 6622 */ 6623 @Child(name = "mode", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 6624 @Description(shortDefinition = "sender | receiver", formalDefinition = "The mode of this event declaration - whether application is sender or receiver.") 6625 protected Enumeration<ConformanceEventMode> mode; 6626 6627 /** 6628 * A resource associated with the event. This is the resource that defines the 6629 * event. 6630 */ 6631 @Child(name = "focus", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 6632 @Description(shortDefinition = "Resource that's focus of message", formalDefinition = "A resource associated with the event. This is the resource that defines the event.") 6633 protected CodeType focus; 6634 6635 /** 6636 * Information about the request for this event. 6637 */ 6638 @Child(name = "request", type = { 6639 StructureDefinition.class }, order = 5, min = 1, max = 1, modifier = false, summary = false) 6640 @Description(shortDefinition = "Profile that describes the request", formalDefinition = "Information about the request for this event.") 6641 protected Reference request; 6642 6643 /** 6644 * The actual object that is the target of the reference (Information about the 6645 * request for this event.) 6646 */ 6647 protected StructureDefinition requestTarget; 6648 6649 /** 6650 * Information about the response for this event. 6651 */ 6652 @Child(name = "response", type = { 6653 StructureDefinition.class }, order = 6, min = 1, max = 1, modifier = false, summary = false) 6654 @Description(shortDefinition = "Profile that describes the response", formalDefinition = "Information about the response for this event.") 6655 protected Reference response; 6656 6657 /** 6658 * The actual object that is the target of the reference (Information about the 6659 * response for this event.) 6660 */ 6661 protected StructureDefinition responseTarget; 6662 6663 /** 6664 * Guidance on how this event is handled, such as internal system trigger 6665 * points, business rules, etc. 6666 */ 6667 @Child(name = "documentation", type = { 6668 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 6669 @Description(shortDefinition = "Endpoint-specific event documentation", formalDefinition = "Guidance on how this event is handled, such as internal system trigger points, business rules, etc.") 6670 protected StringType documentation; 6671 6672 private static final long serialVersionUID = -47031390L; 6673 6674 /* 6675 * Constructor 6676 */ 6677 public ConformanceMessagingEventComponent() { 6678 super(); 6679 } 6680 6681 /* 6682 * Constructor 6683 */ 6684 public ConformanceMessagingEventComponent(Coding code, Enumeration<ConformanceEventMode> mode, CodeType focus, 6685 Reference request, Reference response) { 6686 super(); 6687 this.code = code; 6688 this.mode = mode; 6689 this.focus = focus; 6690 this.request = request; 6691 this.response = response; 6692 } 6693 6694 /** 6695 * @return {@link #code} (A coded identifier of a supported messaging event.) 6696 */ 6697 public Coding getCode() { 6698 if (this.code == null) 6699 if (Configuration.errorOnAutoCreate()) 6700 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.code"); 6701 else if (Configuration.doAutoCreate()) 6702 this.code = new Coding(); // cc 6703 return this.code; 6704 } 6705 6706 public boolean hasCode() { 6707 return this.code != null && !this.code.isEmpty(); 6708 } 6709 6710 /** 6711 * @param value {@link #code} (A coded identifier of a supported messaging 6712 * event.) 6713 */ 6714 public ConformanceMessagingEventComponent setCode(Coding value) { 6715 this.code = value; 6716 return this; 6717 } 6718 6719 /** 6720 * @return {@link #category} (The impact of the content of the message.). This 6721 * is the underlying object with id, value and extensions. The accessor 6722 * "getCategory" gives direct access to the value 6723 */ 6724 public Enumeration<MessageSignificanceCategory> getCategoryElement() { 6725 if (this.category == null) 6726 if (Configuration.errorOnAutoCreate()) 6727 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.category"); 6728 else if (Configuration.doAutoCreate()) 6729 this.category = new Enumeration<MessageSignificanceCategory>(new MessageSignificanceCategoryEnumFactory()); // bb 6730 return this.category; 6731 } 6732 6733 public boolean hasCategoryElement() { 6734 return this.category != null && !this.category.isEmpty(); 6735 } 6736 6737 public boolean hasCategory() { 6738 return this.category != null && !this.category.isEmpty(); 6739 } 6740 6741 /** 6742 * @param value {@link #category} (The impact of the content of the message.). 6743 * This is the underlying object with id, value and extensions. The 6744 * accessor "getCategory" gives direct access to the value 6745 */ 6746 public ConformanceMessagingEventComponent setCategoryElement(Enumeration<MessageSignificanceCategory> value) { 6747 this.category = value; 6748 return this; 6749 } 6750 6751 /** 6752 * @return The impact of the content of the message. 6753 */ 6754 public MessageSignificanceCategory getCategory() { 6755 return this.category == null ? null : this.category.getValue(); 6756 } 6757 6758 /** 6759 * @param value The impact of the content of the message. 6760 */ 6761 public ConformanceMessagingEventComponent setCategory(MessageSignificanceCategory value) { 6762 if (value == null) 6763 this.category = null; 6764 else { 6765 if (this.category == null) 6766 this.category = new Enumeration<MessageSignificanceCategory>(new MessageSignificanceCategoryEnumFactory()); 6767 this.category.setValue(value); 6768 } 6769 return this; 6770 } 6771 6772 /** 6773 * @return {@link #mode} (The mode of this event declaration - whether 6774 * application is sender or receiver.). This is the underlying object 6775 * with id, value and extensions. The accessor "getMode" gives direct 6776 * access to the value 6777 */ 6778 public Enumeration<ConformanceEventMode> getModeElement() { 6779 if (this.mode == null) 6780 if (Configuration.errorOnAutoCreate()) 6781 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.mode"); 6782 else if (Configuration.doAutoCreate()) 6783 this.mode = new Enumeration<ConformanceEventMode>(new ConformanceEventModeEnumFactory()); // bb 6784 return this.mode; 6785 } 6786 6787 public boolean hasModeElement() { 6788 return this.mode != null && !this.mode.isEmpty(); 6789 } 6790 6791 public boolean hasMode() { 6792 return this.mode != null && !this.mode.isEmpty(); 6793 } 6794 6795 /** 6796 * @param value {@link #mode} (The mode of this event declaration - whether 6797 * application is sender or receiver.). This is the underlying 6798 * object with id, value and extensions. The accessor "getMode" 6799 * gives direct access to the value 6800 */ 6801 public ConformanceMessagingEventComponent setModeElement(Enumeration<ConformanceEventMode> value) { 6802 this.mode = value; 6803 return this; 6804 } 6805 6806 /** 6807 * @return The mode of this event declaration - whether application is sender or 6808 * receiver. 6809 */ 6810 public ConformanceEventMode getMode() { 6811 return this.mode == null ? null : this.mode.getValue(); 6812 } 6813 6814 /** 6815 * @param value The mode of this event declaration - whether application is 6816 * sender or receiver. 6817 */ 6818 public ConformanceMessagingEventComponent setMode(ConformanceEventMode value) { 6819 if (this.mode == null) 6820 this.mode = new Enumeration<ConformanceEventMode>(new ConformanceEventModeEnumFactory()); 6821 this.mode.setValue(value); 6822 return this; 6823 } 6824 6825 /** 6826 * @return {@link #focus} (A resource associated with the event. This is the 6827 * resource that defines the event.). This is the underlying object with 6828 * id, value and extensions. The accessor "getFocus" gives direct access 6829 * to the value 6830 */ 6831 public CodeType getFocusElement() { 6832 if (this.focus == null) 6833 if (Configuration.errorOnAutoCreate()) 6834 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.focus"); 6835 else if (Configuration.doAutoCreate()) 6836 this.focus = new CodeType(); // bb 6837 return this.focus; 6838 } 6839 6840 public boolean hasFocusElement() { 6841 return this.focus != null && !this.focus.isEmpty(); 6842 } 6843 6844 public boolean hasFocus() { 6845 return this.focus != null && !this.focus.isEmpty(); 6846 } 6847 6848 /** 6849 * @param value {@link #focus} (A resource associated with the event. This is 6850 * the resource that defines the event.). This is the underlying 6851 * object with id, value and extensions. The accessor "getFocus" 6852 * gives direct access to the value 6853 */ 6854 public ConformanceMessagingEventComponent setFocusElement(CodeType value) { 6855 this.focus = value; 6856 return this; 6857 } 6858 6859 /** 6860 * @return A resource associated with the event. This is the resource that 6861 * defines the event. 6862 */ 6863 public String getFocus() { 6864 return this.focus == null ? null : this.focus.getValue(); 6865 } 6866 6867 /** 6868 * @param value A resource associated with the event. This is the resource that 6869 * defines the event. 6870 */ 6871 public ConformanceMessagingEventComponent setFocus(String value) { 6872 if (this.focus == null) 6873 this.focus = new CodeType(); 6874 this.focus.setValue(value); 6875 return this; 6876 } 6877 6878 /** 6879 * @return {@link #request} (Information about the request for this event.) 6880 */ 6881 public Reference getRequest() { 6882 if (this.request == null) 6883 if (Configuration.errorOnAutoCreate()) 6884 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.request"); 6885 else if (Configuration.doAutoCreate()) 6886 this.request = new Reference(); // cc 6887 return this.request; 6888 } 6889 6890 public boolean hasRequest() { 6891 return this.request != null && !this.request.isEmpty(); 6892 } 6893 6894 /** 6895 * @param value {@link #request} (Information about the request for this event.) 6896 */ 6897 public ConformanceMessagingEventComponent setRequest(Reference value) { 6898 this.request = value; 6899 return this; 6900 } 6901 6902 /** 6903 * @return {@link #request} The actual object that is the target of the 6904 * reference. The reference library doesn't populate this, but you can 6905 * use it to hold the resource if you resolve it. (Information about the 6906 * request for this event.) 6907 */ 6908 public StructureDefinition getRequestTarget() { 6909 if (this.requestTarget == null) 6910 if (Configuration.errorOnAutoCreate()) 6911 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.request"); 6912 else if (Configuration.doAutoCreate()) 6913 this.requestTarget = new StructureDefinition(); // aa 6914 return this.requestTarget; 6915 } 6916 6917 /** 6918 * @param value {@link #request} The actual object that is the target of the 6919 * reference. The reference library doesn't use these, but you can 6920 * use it to hold the resource if you resolve it. (Information 6921 * about the request for this event.) 6922 */ 6923 public ConformanceMessagingEventComponent setRequestTarget(StructureDefinition value) { 6924 this.requestTarget = value; 6925 return this; 6926 } 6927 6928 /** 6929 * @return {@link #response} (Information about the response for this event.) 6930 */ 6931 public Reference getResponse() { 6932 if (this.response == null) 6933 if (Configuration.errorOnAutoCreate()) 6934 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.response"); 6935 else if (Configuration.doAutoCreate()) 6936 this.response = new Reference(); // cc 6937 return this.response; 6938 } 6939 6940 public boolean hasResponse() { 6941 return this.response != null && !this.response.isEmpty(); 6942 } 6943 6944 /** 6945 * @param value {@link #response} (Information about the response for this 6946 * event.) 6947 */ 6948 public ConformanceMessagingEventComponent setResponse(Reference value) { 6949 this.response = value; 6950 return this; 6951 } 6952 6953 /** 6954 * @return {@link #response} The actual object that is the target of the 6955 * reference. The reference library doesn't populate this, but you can 6956 * use it to hold the resource if you resolve it. (Information about the 6957 * response for this event.) 6958 */ 6959 public StructureDefinition getResponseTarget() { 6960 if (this.responseTarget == null) 6961 if (Configuration.errorOnAutoCreate()) 6962 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.response"); 6963 else if (Configuration.doAutoCreate()) 6964 this.responseTarget = new StructureDefinition(); // aa 6965 return this.responseTarget; 6966 } 6967 6968 /** 6969 * @param value {@link #response} The actual object that is the target of the 6970 * reference. The reference library doesn't use these, but you can 6971 * use it to hold the resource if you resolve it. (Information 6972 * about the response for this event.) 6973 */ 6974 public ConformanceMessagingEventComponent setResponseTarget(StructureDefinition value) { 6975 this.responseTarget = value; 6976 return this; 6977 } 6978 6979 /** 6980 * @return {@link #documentation} (Guidance on how this event is handled, such 6981 * as internal system trigger points, business rules, etc.). This is the 6982 * underlying object with id, value and extensions. The accessor 6983 * "getDocumentation" gives direct access to the value 6984 */ 6985 public StringType getDocumentationElement() { 6986 if (this.documentation == null) 6987 if (Configuration.errorOnAutoCreate()) 6988 throw new Error("Attempt to auto-create ConformanceMessagingEventComponent.documentation"); 6989 else if (Configuration.doAutoCreate()) 6990 this.documentation = new StringType(); // bb 6991 return this.documentation; 6992 } 6993 6994 public boolean hasDocumentationElement() { 6995 return this.documentation != null && !this.documentation.isEmpty(); 6996 } 6997 6998 public boolean hasDocumentation() { 6999 return this.documentation != null && !this.documentation.isEmpty(); 7000 } 7001 7002 /** 7003 * @param value {@link #documentation} (Guidance on how this event is handled, 7004 * such as internal system trigger points, business rules, etc.). 7005 * This is the underlying object with id, value and extensions. The 7006 * accessor "getDocumentation" gives direct access to the value 7007 */ 7008 public ConformanceMessagingEventComponent setDocumentationElement(StringType value) { 7009 this.documentation = value; 7010 return this; 7011 } 7012 7013 /** 7014 * @return Guidance on how this event is handled, such as internal system 7015 * trigger points, business rules, etc. 7016 */ 7017 public String getDocumentation() { 7018 return this.documentation == null ? null : this.documentation.getValue(); 7019 } 7020 7021 /** 7022 * @param value Guidance on how this event is handled, such as internal system 7023 * trigger points, business rules, etc. 7024 */ 7025 public ConformanceMessagingEventComponent setDocumentation(String value) { 7026 if (Utilities.noString(value)) 7027 this.documentation = null; 7028 else { 7029 if (this.documentation == null) 7030 this.documentation = new StringType(); 7031 this.documentation.setValue(value); 7032 } 7033 return this; 7034 } 7035 7036 protected void listChildren(List<Property> childrenList) { 7037 super.listChildren(childrenList); 7038 childrenList.add(new Property("code", "Coding", "A coded identifier of a supported messaging event.", 0, 7039 java.lang.Integer.MAX_VALUE, code)); 7040 childrenList.add(new Property("category", "code", "The impact of the content of the message.", 0, 7041 java.lang.Integer.MAX_VALUE, category)); 7042 childrenList.add(new Property("mode", "code", 7043 "The mode of this event declaration - whether application is sender or receiver.", 0, 7044 java.lang.Integer.MAX_VALUE, mode)); 7045 childrenList.add(new Property("focus", "code", 7046 "A resource associated with the event. This is the resource that defines the event.", 0, 7047 java.lang.Integer.MAX_VALUE, focus)); 7048 childrenList.add(new Property("request", "Reference(StructureDefinition)", 7049 "Information about the request for this event.", 0, java.lang.Integer.MAX_VALUE, request)); 7050 childrenList.add(new Property("response", "Reference(StructureDefinition)", 7051 "Information about the response for this event.", 0, java.lang.Integer.MAX_VALUE, response)); 7052 childrenList.add(new Property("documentation", "string", 7053 "Guidance on how this event is handled, such as internal system trigger points, business rules, etc.", 0, 7054 java.lang.Integer.MAX_VALUE, documentation)); 7055 } 7056 7057 @Override 7058 public void setProperty(String name, Base value) throws FHIRException { 7059 if (name.equals("code")) 7060 this.code = castToCoding(value); // Coding 7061 else if (name.equals("category")) 7062 this.category = new MessageSignificanceCategoryEnumFactory().fromType(value); // Enumeration<MessageSignificanceCategory> 7063 else if (name.equals("mode")) 7064 this.mode = new ConformanceEventModeEnumFactory().fromType(value); // Enumeration<ConformanceEventMode> 7065 else if (name.equals("focus")) 7066 this.focus = castToCode(value); // CodeType 7067 else if (name.equals("request")) 7068 this.request = castToReference(value); // Reference 7069 else if (name.equals("response")) 7070 this.response = castToReference(value); // Reference 7071 else if (name.equals("documentation")) 7072 this.documentation = castToString(value); // StringType 7073 else 7074 super.setProperty(name, value); 7075 } 7076 7077 @Override 7078 public Base addChild(String name) throws FHIRException { 7079 if (name.equals("code")) { 7080 this.code = new Coding(); 7081 return this.code; 7082 } else if (name.equals("category")) { 7083 throw new FHIRException("Cannot call addChild on a singleton property Conformance.category"); 7084 } else if (name.equals("mode")) { 7085 throw new FHIRException("Cannot call addChild on a singleton property Conformance.mode"); 7086 } else if (name.equals("focus")) { 7087 throw new FHIRException("Cannot call addChild on a singleton property Conformance.focus"); 7088 } else if (name.equals("request")) { 7089 this.request = new Reference(); 7090 return this.request; 7091 } else if (name.equals("response")) { 7092 this.response = new Reference(); 7093 return this.response; 7094 } else if (name.equals("documentation")) { 7095 throw new FHIRException("Cannot call addChild on a singleton property Conformance.documentation"); 7096 } else 7097 return super.addChild(name); 7098 } 7099 7100 public ConformanceMessagingEventComponent copy() { 7101 ConformanceMessagingEventComponent dst = new ConformanceMessagingEventComponent(); 7102 copyValues(dst); 7103 dst.code = code == null ? null : code.copy(); 7104 dst.category = category == null ? null : category.copy(); 7105 dst.mode = mode == null ? null : mode.copy(); 7106 dst.focus = focus == null ? null : focus.copy(); 7107 dst.request = request == null ? null : request.copy(); 7108 dst.response = response == null ? null : response.copy(); 7109 dst.documentation = documentation == null ? null : documentation.copy(); 7110 return dst; 7111 } 7112 7113 @Override 7114 public boolean equalsDeep(Base other) { 7115 if (!super.equalsDeep(other)) 7116 return false; 7117 if (!(other instanceof ConformanceMessagingEventComponent)) 7118 return false; 7119 ConformanceMessagingEventComponent o = (ConformanceMessagingEventComponent) other; 7120 return compareDeep(code, o.code, true) && compareDeep(category, o.category, true) 7121 && compareDeep(mode, o.mode, true) && compareDeep(focus, o.focus, true) 7122 && compareDeep(request, o.request, true) && compareDeep(response, o.response, true) 7123 && compareDeep(documentation, o.documentation, true); 7124 } 7125 7126 @Override 7127 public boolean equalsShallow(Base other) { 7128 if (!super.equalsShallow(other)) 7129 return false; 7130 if (!(other instanceof ConformanceMessagingEventComponent)) 7131 return false; 7132 ConformanceMessagingEventComponent o = (ConformanceMessagingEventComponent) other; 7133 return compareValues(category, o.category, true) && compareValues(mode, o.mode, true) 7134 && compareValues(focus, o.focus, true) && compareValues(documentation, o.documentation, true); 7135 } 7136 7137 public boolean isEmpty() { 7138 return super.isEmpty() && (code == null || code.isEmpty()) && (category == null || category.isEmpty()) 7139 && (mode == null || mode.isEmpty()) && (focus == null || focus.isEmpty()) 7140 && (request == null || request.isEmpty()) && (response == null || response.isEmpty()) 7141 && (documentation == null || documentation.isEmpty()); 7142 } 7143 7144 public String fhirType() { 7145 return "Conformance.messaging.event"; 7146 7147 } 7148 7149 } 7150 7151 @Block() 7152 public static class ConformanceDocumentComponent extends BackboneElement implements IBaseBackboneElement { 7153 /** 7154 * Mode of this document declaration - whether application is producer or 7155 * consumer. 7156 */ 7157 @Child(name = "mode", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 7158 @Description(shortDefinition = "producer | consumer", formalDefinition = "Mode of this document declaration - whether application is producer or consumer.") 7159 protected Enumeration<DocumentMode> mode; 7160 7161 /** 7162 * A description of how the application supports or uses the specified document 7163 * profile. For example, when are documents created, what action is taken with 7164 * consumed documents, etc. 7165 */ 7166 @Child(name = "documentation", type = { 7167 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 7168 @Description(shortDefinition = "Description of document support", formalDefinition = "A description of how the application supports or uses the specified document profile. For example, when are documents created, what action is taken with consumed documents, etc.") 7169 protected StringType documentation; 7170 7171 /** 7172 * A constraint on a resource used in the document. 7173 */ 7174 @Child(name = "profile", type = { 7175 StructureDefinition.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 7176 @Description(shortDefinition = "Constraint on a resource used in the document", formalDefinition = "A constraint on a resource used in the document.") 7177 protected Reference profile; 7178 7179 /** 7180 * The actual object that is the target of the reference (A constraint on a 7181 * resource used in the document.) 7182 */ 7183 protected StructureDefinition profileTarget; 7184 7185 private static final long serialVersionUID = -1059555053L; 7186 7187 /* 7188 * Constructor 7189 */ 7190 public ConformanceDocumentComponent() { 7191 super(); 7192 } 7193 7194 /* 7195 * Constructor 7196 */ 7197 public ConformanceDocumentComponent(Enumeration<DocumentMode> mode, Reference profile) { 7198 super(); 7199 this.mode = mode; 7200 this.profile = profile; 7201 } 7202 7203 /** 7204 * @return {@link #mode} (Mode of this document declaration - whether 7205 * application is producer or consumer.). This is the underlying object 7206 * with id, value and extensions. The accessor "getMode" gives direct 7207 * access to the value 7208 */ 7209 public Enumeration<DocumentMode> getModeElement() { 7210 if (this.mode == null) 7211 if (Configuration.errorOnAutoCreate()) 7212 throw new Error("Attempt to auto-create ConformanceDocumentComponent.mode"); 7213 else if (Configuration.doAutoCreate()) 7214 this.mode = new Enumeration<DocumentMode>(new DocumentModeEnumFactory()); // bb 7215 return this.mode; 7216 } 7217 7218 public boolean hasModeElement() { 7219 return this.mode != null && !this.mode.isEmpty(); 7220 } 7221 7222 public boolean hasMode() { 7223 return this.mode != null && !this.mode.isEmpty(); 7224 } 7225 7226 /** 7227 * @param value {@link #mode} (Mode of this document declaration - whether 7228 * application is producer or consumer.). This is the underlying 7229 * object with id, value and extensions. The accessor "getMode" 7230 * gives direct access to the value 7231 */ 7232 public ConformanceDocumentComponent setModeElement(Enumeration<DocumentMode> value) { 7233 this.mode = value; 7234 return this; 7235 } 7236 7237 /** 7238 * @return Mode of this document declaration - whether application is producer 7239 * or consumer. 7240 */ 7241 public DocumentMode getMode() { 7242 return this.mode == null ? null : this.mode.getValue(); 7243 } 7244 7245 /** 7246 * @param value Mode of this document declaration - whether application is 7247 * producer or consumer. 7248 */ 7249 public ConformanceDocumentComponent setMode(DocumentMode value) { 7250 if (this.mode == null) 7251 this.mode = new Enumeration<DocumentMode>(new DocumentModeEnumFactory()); 7252 this.mode.setValue(value); 7253 return this; 7254 } 7255 7256 /** 7257 * @return {@link #documentation} (A description of how the application supports 7258 * or uses the specified document profile. For example, when are 7259 * documents created, what action is taken with consumed documents, 7260 * etc.). This is the underlying object with id, value and extensions. 7261 * The accessor "getDocumentation" gives direct access to the value 7262 */ 7263 public StringType getDocumentationElement() { 7264 if (this.documentation == null) 7265 if (Configuration.errorOnAutoCreate()) 7266 throw new Error("Attempt to auto-create ConformanceDocumentComponent.documentation"); 7267 else if (Configuration.doAutoCreate()) 7268 this.documentation = new StringType(); // bb 7269 return this.documentation; 7270 } 7271 7272 public boolean hasDocumentationElement() { 7273 return this.documentation != null && !this.documentation.isEmpty(); 7274 } 7275 7276 public boolean hasDocumentation() { 7277 return this.documentation != null && !this.documentation.isEmpty(); 7278 } 7279 7280 /** 7281 * @param value {@link #documentation} (A description of how the application 7282 * supports or uses the specified document profile. For example, 7283 * when are documents created, what action is taken with consumed 7284 * documents, etc.). This is the underlying object with id, value 7285 * and extensions. The accessor "getDocumentation" gives direct 7286 * access to the value 7287 */ 7288 public ConformanceDocumentComponent setDocumentationElement(StringType value) { 7289 this.documentation = value; 7290 return this; 7291 } 7292 7293 /** 7294 * @return A description of how the application supports or uses the specified 7295 * document profile. For example, when are documents created, what 7296 * action is taken with consumed documents, etc. 7297 */ 7298 public String getDocumentation() { 7299 return this.documentation == null ? null : this.documentation.getValue(); 7300 } 7301 7302 /** 7303 * @param value A description of how the application supports or uses the 7304 * specified document profile. For example, when are documents 7305 * created, what action is taken with consumed documents, etc. 7306 */ 7307 public ConformanceDocumentComponent setDocumentation(String value) { 7308 if (Utilities.noString(value)) 7309 this.documentation = null; 7310 else { 7311 if (this.documentation == null) 7312 this.documentation = new StringType(); 7313 this.documentation.setValue(value); 7314 } 7315 return this; 7316 } 7317 7318 /** 7319 * @return {@link #profile} (A constraint on a resource used in the document.) 7320 */ 7321 public Reference getProfile() { 7322 if (this.profile == null) 7323 if (Configuration.errorOnAutoCreate()) 7324 throw new Error("Attempt to auto-create ConformanceDocumentComponent.profile"); 7325 else if (Configuration.doAutoCreate()) 7326 this.profile = new Reference(); // cc 7327 return this.profile; 7328 } 7329 7330 public boolean hasProfile() { 7331 return this.profile != null && !this.profile.isEmpty(); 7332 } 7333 7334 /** 7335 * @param value {@link #profile} (A constraint on a resource used in the 7336 * document.) 7337 */ 7338 public ConformanceDocumentComponent setProfile(Reference value) { 7339 this.profile = value; 7340 return this; 7341 } 7342 7343 /** 7344 * @return {@link #profile} The actual object that is the target of the 7345 * reference. The reference library doesn't populate this, but you can 7346 * use it to hold the resource if you resolve it. (A constraint on a 7347 * resource used in the document.) 7348 */ 7349 public StructureDefinition getProfileTarget() { 7350 if (this.profileTarget == null) 7351 if (Configuration.errorOnAutoCreate()) 7352 throw new Error("Attempt to auto-create ConformanceDocumentComponent.profile"); 7353 else if (Configuration.doAutoCreate()) 7354 this.profileTarget = new StructureDefinition(); // aa 7355 return this.profileTarget; 7356 } 7357 7358 /** 7359 * @param value {@link #profile} The actual object that is the target of the 7360 * reference. The reference library doesn't use these, but you can 7361 * use it to hold the resource if you resolve it. (A constraint on 7362 * a resource used in the document.) 7363 */ 7364 public ConformanceDocumentComponent setProfileTarget(StructureDefinition value) { 7365 this.profileTarget = value; 7366 return this; 7367 } 7368 7369 protected void listChildren(List<Property> childrenList) { 7370 super.listChildren(childrenList); 7371 childrenList.add(new Property("mode", "code", 7372 "Mode of this document declaration - whether application is producer or consumer.", 0, 7373 java.lang.Integer.MAX_VALUE, mode)); 7374 childrenList.add(new Property("documentation", "string", 7375 "A description of how the application supports or uses the specified document profile. For example, when are documents created, what action is taken with consumed documents, etc.", 7376 0, java.lang.Integer.MAX_VALUE, documentation)); 7377 childrenList.add(new Property("profile", "Reference(StructureDefinition)", 7378 "A constraint on a resource used in the document.", 0, java.lang.Integer.MAX_VALUE, profile)); 7379 } 7380 7381 @Override 7382 public void setProperty(String name, Base value) throws FHIRException { 7383 if (name.equals("mode")) 7384 this.mode = new DocumentModeEnumFactory().fromType(value); // Enumeration<DocumentMode> 7385 else if (name.equals("documentation")) 7386 this.documentation = castToString(value); // StringType 7387 else if (name.equals("profile")) 7388 this.profile = castToReference(value); // Reference 7389 else 7390 super.setProperty(name, value); 7391 } 7392 7393 @Override 7394 public Base addChild(String name) throws FHIRException { 7395 if (name.equals("mode")) { 7396 throw new FHIRException("Cannot call addChild on a singleton property Conformance.mode"); 7397 } else if (name.equals("documentation")) { 7398 throw new FHIRException("Cannot call addChild on a singleton property Conformance.documentation"); 7399 } else if (name.equals("profile")) { 7400 this.profile = new Reference(); 7401 return this.profile; 7402 } else 7403 return super.addChild(name); 7404 } 7405 7406 public ConformanceDocumentComponent copy() { 7407 ConformanceDocumentComponent dst = new ConformanceDocumentComponent(); 7408 copyValues(dst); 7409 dst.mode = mode == null ? null : mode.copy(); 7410 dst.documentation = documentation == null ? null : documentation.copy(); 7411 dst.profile = profile == null ? null : profile.copy(); 7412 return dst; 7413 } 7414 7415 @Override 7416 public boolean equalsDeep(Base other) { 7417 if (!super.equalsDeep(other)) 7418 return false; 7419 if (!(other instanceof ConformanceDocumentComponent)) 7420 return false; 7421 ConformanceDocumentComponent o = (ConformanceDocumentComponent) other; 7422 return compareDeep(mode, o.mode, true) && compareDeep(documentation, o.documentation, true) 7423 && compareDeep(profile, o.profile, true); 7424 } 7425 7426 @Override 7427 public boolean equalsShallow(Base other) { 7428 if (!super.equalsShallow(other)) 7429 return false; 7430 if (!(other instanceof ConformanceDocumentComponent)) 7431 return false; 7432 ConformanceDocumentComponent o = (ConformanceDocumentComponent) other; 7433 return compareValues(mode, o.mode, true) && compareValues(documentation, o.documentation, true); 7434 } 7435 7436 public boolean isEmpty() { 7437 return super.isEmpty() && (mode == null || mode.isEmpty()) && (documentation == null || documentation.isEmpty()) 7438 && (profile == null || profile.isEmpty()); 7439 } 7440 7441 public String fhirType() { 7442 return "Conformance.document"; 7443 7444 } 7445 7446 } 7447 7448 /** 7449 * An absolute URL that is used to identify this conformance statement when it 7450 * is referenced in a specification, model, design or an instance. This SHALL be 7451 * a URL, SHOULD be globally unique, and SHOULD be an address at which this 7452 * conformance statement is (or will be) published. 7453 */ 7454 @Child(name = "url", type = { UriType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 7455 @Description(shortDefinition = "Logical uri to reference this statement", formalDefinition = "An absolute URL that is used to identify this conformance statement when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this conformance statement is (or will be) published.") 7456 protected UriType url; 7457 7458 /** 7459 * The identifier that is used to identify this version of the conformance 7460 * statement when it is referenced in a specification, model, design or 7461 * instance. This is an arbitrary value managed by the profile author manually 7462 * and the value should be a timestamp. 7463 */ 7464 @Child(name = "version", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 7465 @Description(shortDefinition = "Logical id for this version of the statement", formalDefinition = "The identifier that is used to identify this version of the conformance statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the profile author manually and the value should be a timestamp.") 7466 protected StringType version; 7467 7468 /** 7469 * A free text natural language name identifying the conformance statement. 7470 */ 7471 @Child(name = "name", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 7472 @Description(shortDefinition = "Informal name for this conformance statement", formalDefinition = "A free text natural language name identifying the conformance statement.") 7473 protected StringType name; 7474 7475 /** 7476 * The status of this conformance statement. 7477 */ 7478 @Child(name = "status", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = true, summary = true) 7479 @Description(shortDefinition = "draft | active | retired", formalDefinition = "The status of this conformance statement.") 7480 protected Enumeration<ConformanceResourceStatus> status; 7481 7482 /** 7483 * A flag to indicate that this conformance statement is authored for testing 7484 * purposes (or education/evaluation/marketing), and is not intended to be used 7485 * for genuine usage. 7486 */ 7487 @Child(name = "experimental", type = { 7488 BooleanType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 7489 @Description(shortDefinition = "If for testing purposes, not real usage", formalDefinition = "A flag to indicate that this conformance statement is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.") 7490 protected BooleanType experimental; 7491 7492 /** 7493 * The name of the individual or organization that published the conformance. 7494 */ 7495 @Child(name = "publisher", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 7496 @Description(shortDefinition = "Name of the publisher (Organization or individual)", formalDefinition = "The name of the individual or organization that published the conformance.") 7497 protected StringType publisher; 7498 7499 /** 7500 * Contacts to assist a user in finding and communicating with the publisher. 7501 */ 7502 @Child(name = "contact", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 7503 @Description(shortDefinition = "Contact details of the publisher", formalDefinition = "Contacts to assist a user in finding and communicating with the publisher.") 7504 protected List<ConformanceContactComponent> contact; 7505 7506 /** 7507 * The date (and optionally time) when the conformance statement was published. 7508 * The date must change when the business version changes, if it does, and it 7509 * must change if the status code changes. In addition, it should change when 7510 * the substantive content of the conformance statement changes. 7511 */ 7512 @Child(name = "date", type = { DateTimeType.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 7513 @Description(shortDefinition = "Publication Date(/time)", formalDefinition = "The date (and optionally time) when the conformance statement was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the conformance statement changes.") 7514 protected DateTimeType date; 7515 7516 /** 7517 * A free text natural language description of the conformance statement and its 7518 * use. Typically, this is used when the conformance statement describes a 7519 * desired rather than an actual solution, for example as a formal expression of 7520 * requirements as part of an RFP. 7521 */ 7522 @Child(name = "description", type = { 7523 StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 7524 @Description(shortDefinition = "Human description of the conformance statement", formalDefinition = "A free text natural language description of the conformance statement and its use. Typically, this is used when the conformance statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.") 7525 protected StringType description; 7526 7527 /** 7528 * Explains why this conformance statement is needed and why it's been 7529 * constrained as it has. 7530 */ 7531 @Child(name = "requirements", type = { 7532 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 7533 @Description(shortDefinition = "Why is this needed?", formalDefinition = "Explains why this conformance statement is needed and why it's been constrained as it has.") 7534 protected StringType requirements; 7535 7536 /** 7537 * A copyright statement relating to the conformance statement and/or its 7538 * contents. Copyright statements are generally legal restrictions on the use 7539 * and publishing of the details of the system described by the conformance 7540 * statement. 7541 */ 7542 @Child(name = "copyright", type = { 7543 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 7544 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the conformance statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the details of the system described by the conformance statement.") 7545 protected StringType copyright; 7546 7547 /** 7548 * The way that this statement is intended to be used, to describe an actual 7549 * running instance of software, a particular product (kind not instance of 7550 * software) or a class of implementation (e.g. a desired purchase). 7551 */ 7552 @Child(name = "kind", type = { CodeType.class }, order = 11, min = 1, max = 1, modifier = false, summary = true) 7553 @Description(shortDefinition = "instance | capability | requirements", formalDefinition = "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind not instance of software) or a class of implementation (e.g. a desired purchase).") 7554 protected Enumeration<ConformanceStatementKind> kind; 7555 7556 /** 7557 * Software that is covered by this conformance statement. It is used when the 7558 * conformance statement describes the capabilities of a particular software 7559 * version, independent of an installation. 7560 */ 7561 @Child(name = "software", type = {}, order = 12, min = 0, max = 1, modifier = false, summary = true) 7562 @Description(shortDefinition = "Software that is covered by this conformance statement", formalDefinition = "Software that is covered by this conformance statement. It is used when the conformance statement describes the capabilities of a particular software version, independent of an installation.") 7563 protected ConformanceSoftwareComponent software; 7564 7565 /** 7566 * Identifies a specific implementation instance that is described by the 7567 * conformance statement - i.e. a particular installation, rather than the 7568 * capabilities of a software program. 7569 */ 7570 @Child(name = "implementation", type = {}, order = 13, min = 0, max = 1, modifier = false, summary = true) 7571 @Description(shortDefinition = "If this describes a specific instance", formalDefinition = "Identifies a specific implementation instance that is described by the conformance statement - i.e. a particular installation, rather than the capabilities of a software program.") 7572 protected ConformanceImplementationComponent implementation; 7573 7574 /** 7575 * The version of the FHIR specification on which this conformance statement is 7576 * based. 7577 */ 7578 @Child(name = "fhirVersion", type = { IdType.class }, order = 14, min = 1, max = 1, modifier = false, summary = true) 7579 @Description(shortDefinition = "FHIR Version the system uses", formalDefinition = "The version of the FHIR specification on which this conformance statement is based.") 7580 protected IdType fhirVersion; 7581 7582 /** 7583 * A code that indicates whether the application accepts unknown elements or 7584 * extensions when reading resources. 7585 */ 7586 @Child(name = "acceptUnknown", type = { 7587 CodeType.class }, order = 15, min = 1, max = 1, modifier = false, summary = true) 7588 @Description(shortDefinition = "no | extensions | elements | both", formalDefinition = "A code that indicates whether the application accepts unknown elements or extensions when reading resources.") 7589 protected Enumeration<UnknownContentCode> acceptUnknown; 7590 7591 /** 7592 * A list of the formats supported by this implementation using their content 7593 * types. 7594 */ 7595 @Child(name = "format", type = { 7596 CodeType.class }, order = 16, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 7597 @Description(shortDefinition = "formats supported (xml | json | mime type)", formalDefinition = "A list of the formats supported by this implementation using their content types.") 7598 protected List<CodeType> format; 7599 7600 /** 7601 * A list of profiles that represent different use cases supported by the 7602 * system. For a server, "supported by the system" means the system 7603 * hosts/produces a set of resources that are conformant to a particular 7604 * profile, and allows clients that use its services to search using this 7605 * profile and to find appropriate data. For a client, it means the system will 7606 * search by this profile and process data according to the guidance implicit in 7607 * the profile. See further discussion in [Using 7608 * Profiles]{profiling.html#profile-uses}. 7609 */ 7610 @Child(name = "profile", type = { 7611 StructureDefinition.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7612 @Description(shortDefinition = "Profiles for use cases supported", formalDefinition = "A list of profiles that represent different use cases supported by the system. For a server, \"supported by the system\" means the system hosts/produces a set of resources that are conformant to a particular profile, and allows clients that use its services to search using this profile and to find appropriate data. For a client, it means the system will search by this profile and process data according to the guidance implicit in the profile. See further discussion in [Using Profiles]{profiling.html#profile-uses}.") 7613 protected List<Reference> profile; 7614 /** 7615 * The actual objects that are the target of the reference (A list of profiles 7616 * that represent different use cases supported by the system. For a server, 7617 * "supported by the system" means the system hosts/produces a set of resources 7618 * that are conformant to a particular profile, and allows clients that use its 7619 * services to search using this profile and to find appropriate data. For a 7620 * client, it means the system will search by this profile and process data 7621 * according to the guidance implicit in the profile. See further discussion in 7622 * [Using Profiles]{profiling.html#profile-uses}.) 7623 */ 7624 protected List<StructureDefinition> profileTarget; 7625 7626 /** 7627 * A definition of the restful capabilities of the solution, if any. 7628 */ 7629 @Child(name = "rest", type = {}, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 7630 @Description(shortDefinition = "If the endpoint is a RESTful one", formalDefinition = "A definition of the restful capabilities of the solution, if any.") 7631 protected List<ConformanceRestComponent> rest; 7632 7633 /** 7634 * A description of the messaging capabilities of the solution. 7635 */ 7636 @Child(name = "messaging", type = {}, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7637 @Description(shortDefinition = "If messaging is supported", formalDefinition = "A description of the messaging capabilities of the solution.") 7638 protected List<ConformanceMessagingComponent> messaging; 7639 7640 /** 7641 * A document definition. 7642 */ 7643 @Child(name = "document", type = {}, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7644 @Description(shortDefinition = "Document definition", formalDefinition = "A document definition.") 7645 protected List<ConformanceDocumentComponent> document; 7646 7647 private static final long serialVersionUID = 1863739648L; 7648 7649 /* 7650 * Constructor 7651 */ 7652 public Conformance() { 7653 super(); 7654 } 7655 7656 /* 7657 * Constructor 7658 */ 7659 public Conformance(DateTimeType date, Enumeration<ConformanceStatementKind> kind, IdType fhirVersion, 7660 Enumeration<UnknownContentCode> acceptUnknown) { 7661 super(); 7662 this.date = date; 7663 this.kind = kind; 7664 this.fhirVersion = fhirVersion; 7665 this.acceptUnknown = acceptUnknown; 7666 } 7667 7668 /** 7669 * @return {@link #url} (An absolute URL that is used to identify this 7670 * conformance statement when it is referenced in a specification, 7671 * model, design or an instance. This SHALL be a URL, SHOULD be globally 7672 * unique, and SHOULD be an address at which this conformance statement 7673 * is (or will be) published.). This is the underlying object with id, 7674 * value and extensions. The accessor "getUrl" gives direct access to 7675 * the value 7676 */ 7677 public UriType getUrlElement() { 7678 if (this.url == null) 7679 if (Configuration.errorOnAutoCreate()) 7680 throw new Error("Attempt to auto-create Conformance.url"); 7681 else if (Configuration.doAutoCreate()) 7682 this.url = new UriType(); // bb 7683 return this.url; 7684 } 7685 7686 public boolean hasUrlElement() { 7687 return this.url != null && !this.url.isEmpty(); 7688 } 7689 7690 public boolean hasUrl() { 7691 return this.url != null && !this.url.isEmpty(); 7692 } 7693 7694 /** 7695 * @param value {@link #url} (An absolute URL that is used to identify this 7696 * conformance statement when it is referenced in a specification, 7697 * model, design or an instance. This SHALL be a URL, SHOULD be 7698 * globally unique, and SHOULD be an address at which this 7699 * conformance statement is (or will be) published.). This is the 7700 * underlying object with id, value and extensions. The accessor 7701 * "getUrl" gives direct access to the value 7702 */ 7703 public Conformance setUrlElement(UriType value) { 7704 this.url = value; 7705 return this; 7706 } 7707 7708 /** 7709 * @return An absolute URL that is used to identify this conformance statement 7710 * when it is referenced in a specification, model, design or an 7711 * instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD 7712 * be an address at which this conformance statement is (or will be) 7713 * published. 7714 */ 7715 public String getUrl() { 7716 return this.url == null ? null : this.url.getValue(); 7717 } 7718 7719 /** 7720 * @param value An absolute URL that is used to identify this conformance 7721 * statement when it is referenced in a specification, model, 7722 * design or an instance. This SHALL be a URL, SHOULD be globally 7723 * unique, and SHOULD be an address at which this conformance 7724 * statement is (or will be) published. 7725 */ 7726 public Conformance setUrl(String value) { 7727 if (Utilities.noString(value)) 7728 this.url = null; 7729 else { 7730 if (this.url == null) 7731 this.url = new UriType(); 7732 this.url.setValue(value); 7733 } 7734 return this; 7735 } 7736 7737 /** 7738 * @return {@link #version} (The identifier that is used to identify this 7739 * version of the conformance statement when it is referenced in a 7740 * specification, model, design or instance. This is an arbitrary value 7741 * managed by the profile author manually and the value should be a 7742 * timestamp.). This is the underlying object with id, value and 7743 * extensions. The accessor "getVersion" gives direct access to the 7744 * value 7745 */ 7746 public StringType getVersionElement() { 7747 if (this.version == null) 7748 if (Configuration.errorOnAutoCreate()) 7749 throw new Error("Attempt to auto-create Conformance.version"); 7750 else if (Configuration.doAutoCreate()) 7751 this.version = new StringType(); // bb 7752 return this.version; 7753 } 7754 7755 public boolean hasVersionElement() { 7756 return this.version != null && !this.version.isEmpty(); 7757 } 7758 7759 public boolean hasVersion() { 7760 return this.version != null && !this.version.isEmpty(); 7761 } 7762 7763 /** 7764 * @param value {@link #version} (The identifier that is used to identify this 7765 * version of the conformance statement when it is referenced in a 7766 * specification, model, design or instance. This is an arbitrary 7767 * value managed by the profile author manually and the value 7768 * should be a timestamp.). This is the underlying object with id, 7769 * value and extensions. The accessor "getVersion" gives direct 7770 * access to the value 7771 */ 7772 public Conformance setVersionElement(StringType value) { 7773 this.version = value; 7774 return this; 7775 } 7776 7777 /** 7778 * @return The identifier that is used to identify this version of the 7779 * conformance statement when it is referenced in a specification, 7780 * model, design or instance. This is an arbitrary value managed by the 7781 * profile author manually and the value should be a timestamp. 7782 */ 7783 public String getVersion() { 7784 return this.version == null ? null : this.version.getValue(); 7785 } 7786 7787 /** 7788 * @param value The identifier that is used to identify this version of the 7789 * conformance statement when it is referenced in a specification, 7790 * model, design or instance. This is an arbitrary value managed by 7791 * the profile author manually and the value should be a timestamp. 7792 */ 7793 public Conformance setVersion(String value) { 7794 if (Utilities.noString(value)) 7795 this.version = null; 7796 else { 7797 if (this.version == null) 7798 this.version = new StringType(); 7799 this.version.setValue(value); 7800 } 7801 return this; 7802 } 7803 7804 /** 7805 * @return {@link #name} (A free text natural language name identifying the 7806 * conformance statement.). This is the underlying object with id, value 7807 * and extensions. The accessor "getName" gives direct access to the 7808 * value 7809 */ 7810 public StringType getNameElement() { 7811 if (this.name == null) 7812 if (Configuration.errorOnAutoCreate()) 7813 throw new Error("Attempt to auto-create Conformance.name"); 7814 else if (Configuration.doAutoCreate()) 7815 this.name = new StringType(); // bb 7816 return this.name; 7817 } 7818 7819 public boolean hasNameElement() { 7820 return this.name != null && !this.name.isEmpty(); 7821 } 7822 7823 public boolean hasName() { 7824 return this.name != null && !this.name.isEmpty(); 7825 } 7826 7827 /** 7828 * @param value {@link #name} (A free text natural language name identifying the 7829 * conformance statement.). This is the underlying object with id, 7830 * value and extensions. The accessor "getName" gives direct access 7831 * to the value 7832 */ 7833 public Conformance setNameElement(StringType value) { 7834 this.name = value; 7835 return this; 7836 } 7837 7838 /** 7839 * @return A free text natural language name identifying the conformance 7840 * statement. 7841 */ 7842 public String getName() { 7843 return this.name == null ? null : this.name.getValue(); 7844 } 7845 7846 /** 7847 * @param value A free text natural language name identifying the conformance 7848 * statement. 7849 */ 7850 public Conformance setName(String value) { 7851 if (Utilities.noString(value)) 7852 this.name = null; 7853 else { 7854 if (this.name == null) 7855 this.name = new StringType(); 7856 this.name.setValue(value); 7857 } 7858 return this; 7859 } 7860 7861 /** 7862 * @return {@link #status} (The status of this conformance statement.). This is 7863 * the underlying object with id, value and extensions. The accessor 7864 * "getStatus" gives direct access to the value 7865 */ 7866 public Enumeration<ConformanceResourceStatus> getStatusElement() { 7867 if (this.status == null) 7868 if (Configuration.errorOnAutoCreate()) 7869 throw new Error("Attempt to auto-create Conformance.status"); 7870 else if (Configuration.doAutoCreate()) 7871 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); // bb 7872 return this.status; 7873 } 7874 7875 public boolean hasStatusElement() { 7876 return this.status != null && !this.status.isEmpty(); 7877 } 7878 7879 public boolean hasStatus() { 7880 return this.status != null && !this.status.isEmpty(); 7881 } 7882 7883 /** 7884 * @param value {@link #status} (The status of this conformance statement.). 7885 * This is the underlying object with id, value and extensions. The 7886 * accessor "getStatus" gives direct access to the value 7887 */ 7888 public Conformance setStatusElement(Enumeration<ConformanceResourceStatus> value) { 7889 this.status = value; 7890 return this; 7891 } 7892 7893 /** 7894 * @return The status of this conformance statement. 7895 */ 7896 public ConformanceResourceStatus getStatus() { 7897 return this.status == null ? null : this.status.getValue(); 7898 } 7899 7900 /** 7901 * @param value The status of this conformance statement. 7902 */ 7903 public Conformance setStatus(ConformanceResourceStatus value) { 7904 if (value == null) 7905 this.status = null; 7906 else { 7907 if (this.status == null) 7908 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); 7909 this.status.setValue(value); 7910 } 7911 return this; 7912 } 7913 7914 /** 7915 * @return {@link #experimental} (A flag to indicate that this conformance 7916 * statement is authored for testing purposes (or 7917 * education/evaluation/marketing), and is not intended to be used for 7918 * genuine usage.). This is the underlying object with id, value and 7919 * extensions. The accessor "getExperimental" gives direct access to the 7920 * value 7921 */ 7922 public BooleanType getExperimentalElement() { 7923 if (this.experimental == null) 7924 if (Configuration.errorOnAutoCreate()) 7925 throw new Error("Attempt to auto-create Conformance.experimental"); 7926 else if (Configuration.doAutoCreate()) 7927 this.experimental = new BooleanType(); // bb 7928 return this.experimental; 7929 } 7930 7931 public boolean hasExperimentalElement() { 7932 return this.experimental != null && !this.experimental.isEmpty(); 7933 } 7934 7935 public boolean hasExperimental() { 7936 return this.experimental != null && !this.experimental.isEmpty(); 7937 } 7938 7939 /** 7940 * @param value {@link #experimental} (A flag to indicate that this conformance 7941 * statement is authored for testing purposes (or 7942 * education/evaluation/marketing), and is not intended to be used 7943 * for genuine usage.). This is the underlying object with id, 7944 * value and extensions. The accessor "getExperimental" gives 7945 * direct access to the value 7946 */ 7947 public Conformance setExperimentalElement(BooleanType value) { 7948 this.experimental = value; 7949 return this; 7950 } 7951 7952 /** 7953 * @return A flag to indicate that this conformance statement is authored for 7954 * testing purposes (or education/evaluation/marketing), and is not 7955 * intended to be used for genuine usage. 7956 */ 7957 public boolean getExperimental() { 7958 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 7959 } 7960 7961 /** 7962 * @param value A flag to indicate that this conformance statement is authored 7963 * for testing purposes (or education/evaluation/marketing), and is 7964 * not intended to be used for genuine usage. 7965 */ 7966 public Conformance setExperimental(boolean value) { 7967 if (this.experimental == null) 7968 this.experimental = new BooleanType(); 7969 this.experimental.setValue(value); 7970 return this; 7971 } 7972 7973 /** 7974 * @return {@link #publisher} (The name of the individual or organization that 7975 * published the conformance.). This is the underlying object with id, 7976 * value and extensions. The accessor "getPublisher" gives direct access 7977 * to the value 7978 */ 7979 public StringType getPublisherElement() { 7980 if (this.publisher == null) 7981 if (Configuration.errorOnAutoCreate()) 7982 throw new Error("Attempt to auto-create Conformance.publisher"); 7983 else if (Configuration.doAutoCreate()) 7984 this.publisher = new StringType(); // bb 7985 return this.publisher; 7986 } 7987 7988 public boolean hasPublisherElement() { 7989 return this.publisher != null && !this.publisher.isEmpty(); 7990 } 7991 7992 public boolean hasPublisher() { 7993 return this.publisher != null && !this.publisher.isEmpty(); 7994 } 7995 7996 /** 7997 * @param value {@link #publisher} (The name of the individual or organization 7998 * that published the conformance.). This is the underlying object 7999 * with id, value and extensions. The accessor "getPublisher" gives 8000 * direct access to the value 8001 */ 8002 public Conformance setPublisherElement(StringType value) { 8003 this.publisher = value; 8004 return this; 8005 } 8006 8007 /** 8008 * @return The name of the individual or organization that published the 8009 * conformance. 8010 */ 8011 public String getPublisher() { 8012 return this.publisher == null ? null : this.publisher.getValue(); 8013 } 8014 8015 /** 8016 * @param value The name of the individual or organization that published the 8017 * conformance. 8018 */ 8019 public Conformance setPublisher(String value) { 8020 if (Utilities.noString(value)) 8021 this.publisher = null; 8022 else { 8023 if (this.publisher == null) 8024 this.publisher = new StringType(); 8025 this.publisher.setValue(value); 8026 } 8027 return this; 8028 } 8029 8030 /** 8031 * @return {@link #contact} (Contacts to assist a user in finding and 8032 * communicating with the publisher.) 8033 */ 8034 public List<ConformanceContactComponent> getContact() { 8035 if (this.contact == null) 8036 this.contact = new ArrayList<ConformanceContactComponent>(); 8037 return this.contact; 8038 } 8039 8040 public boolean hasContact() { 8041 if (this.contact == null) 8042 return false; 8043 for (ConformanceContactComponent item : this.contact) 8044 if (!item.isEmpty()) 8045 return true; 8046 return false; 8047 } 8048 8049 /** 8050 * @return {@link #contact} (Contacts to assist a user in finding and 8051 * communicating with the publisher.) 8052 */ 8053 // syntactic sugar 8054 public ConformanceContactComponent addContact() { // 3 8055 ConformanceContactComponent t = new ConformanceContactComponent(); 8056 if (this.contact == null) 8057 this.contact = new ArrayList<ConformanceContactComponent>(); 8058 this.contact.add(t); 8059 return t; 8060 } 8061 8062 // syntactic sugar 8063 public Conformance addContact(ConformanceContactComponent t) { // 3 8064 if (t == null) 8065 return this; 8066 if (this.contact == null) 8067 this.contact = new ArrayList<ConformanceContactComponent>(); 8068 this.contact.add(t); 8069 return this; 8070 } 8071 8072 /** 8073 * @return {@link #date} (The date (and optionally time) when the conformance 8074 * statement was published. The date must change when the business 8075 * version changes, if it does, and it must change if the status code 8076 * changes. In addition, it should change when the substantive content 8077 * of the conformance statement changes.). This is the underlying object 8078 * with id, value and extensions. The accessor "getDate" gives direct 8079 * access to the value 8080 */ 8081 public DateTimeType getDateElement() { 8082 if (this.date == null) 8083 if (Configuration.errorOnAutoCreate()) 8084 throw new Error("Attempt to auto-create Conformance.date"); 8085 else if (Configuration.doAutoCreate()) 8086 this.date = new DateTimeType(); // bb 8087 return this.date; 8088 } 8089 8090 public boolean hasDateElement() { 8091 return this.date != null && !this.date.isEmpty(); 8092 } 8093 8094 public boolean hasDate() { 8095 return this.date != null && !this.date.isEmpty(); 8096 } 8097 8098 /** 8099 * @param value {@link #date} (The date (and optionally time) when the 8100 * conformance statement was published. The date must change when 8101 * the business version changes, if it does, and it must change if 8102 * the status code changes. In addition, it should change when the 8103 * substantive content of the conformance statement changes.). This 8104 * is the underlying object with id, value and extensions. The 8105 * accessor "getDate" gives direct access to the value 8106 */ 8107 public Conformance setDateElement(DateTimeType value) { 8108 this.date = value; 8109 return this; 8110 } 8111 8112 /** 8113 * @return The date (and optionally time) when the conformance statement was 8114 * published. The date must change when the business version changes, if 8115 * it does, and it must change if the status code changes. In addition, 8116 * it should change when the substantive content of the conformance 8117 * statement changes. 8118 */ 8119 public Date getDate() { 8120 return this.date == null ? null : this.date.getValue(); 8121 } 8122 8123 /** 8124 * @param value The date (and optionally time) when the conformance statement 8125 * was published. The date must change when the business version 8126 * changes, if it does, and it must change if the status code 8127 * changes. In addition, it should change when the substantive 8128 * content of the conformance statement changes. 8129 */ 8130 public Conformance setDate(Date value) { 8131 if (this.date == null) 8132 this.date = new DateTimeType(); 8133 this.date.setValue(value); 8134 return this; 8135 } 8136 8137 /** 8138 * @return {@link #description} (A free text natural language description of the 8139 * conformance statement and its use. Typically, this is used when the 8140 * conformance statement describes a desired rather than an actual 8141 * solution, for example as a formal expression of requirements as part 8142 * of an RFP.). This is the underlying object with id, value and 8143 * extensions. The accessor "getDescription" gives direct access to the 8144 * value 8145 */ 8146 public StringType getDescriptionElement() { 8147 if (this.description == null) 8148 if (Configuration.errorOnAutoCreate()) 8149 throw new Error("Attempt to auto-create Conformance.description"); 8150 else if (Configuration.doAutoCreate()) 8151 this.description = new StringType(); // bb 8152 return this.description; 8153 } 8154 8155 public boolean hasDescriptionElement() { 8156 return this.description != null && !this.description.isEmpty(); 8157 } 8158 8159 public boolean hasDescription() { 8160 return this.description != null && !this.description.isEmpty(); 8161 } 8162 8163 /** 8164 * @param value {@link #description} (A free text natural language description 8165 * of the conformance statement and its use. Typically, this is 8166 * used when the conformance statement describes a desired rather 8167 * than an actual solution, for example as a formal expression of 8168 * requirements as part of an RFP.). This is the underlying object 8169 * with id, value and extensions. The accessor "getDescription" 8170 * gives direct access to the value 8171 */ 8172 public Conformance setDescriptionElement(StringType value) { 8173 this.description = value; 8174 return this; 8175 } 8176 8177 /** 8178 * @return A free text natural language description of the conformance statement 8179 * and its use. Typically, this is used when the conformance statement 8180 * describes a desired rather than an actual solution, for example as a 8181 * formal expression of requirements as part of an RFP. 8182 */ 8183 public String getDescription() { 8184 return this.description == null ? null : this.description.getValue(); 8185 } 8186 8187 /** 8188 * @param value A free text natural language description of the conformance 8189 * statement and its use. Typically, this is used when the 8190 * conformance statement describes a desired rather than an actual 8191 * solution, for example as a formal expression of requirements as 8192 * part of an RFP. 8193 */ 8194 public Conformance setDescription(String value) { 8195 if (Utilities.noString(value)) 8196 this.description = null; 8197 else { 8198 if (this.description == null) 8199 this.description = new StringType(); 8200 this.description.setValue(value); 8201 } 8202 return this; 8203 } 8204 8205 /** 8206 * @return {@link #requirements} (Explains why this conformance statement is 8207 * needed and why it's been constrained as it has.). This is the 8208 * underlying object with id, value and extensions. The accessor 8209 * "getRequirements" gives direct access to the value 8210 */ 8211 public StringType getRequirementsElement() { 8212 if (this.requirements == null) 8213 if (Configuration.errorOnAutoCreate()) 8214 throw new Error("Attempt to auto-create Conformance.requirements"); 8215 else if (Configuration.doAutoCreate()) 8216 this.requirements = new StringType(); // bb 8217 return this.requirements; 8218 } 8219 8220 public boolean hasRequirementsElement() { 8221 return this.requirements != null && !this.requirements.isEmpty(); 8222 } 8223 8224 public boolean hasRequirements() { 8225 return this.requirements != null && !this.requirements.isEmpty(); 8226 } 8227 8228 /** 8229 * @param value {@link #requirements} (Explains why this conformance statement 8230 * is needed and why it's been constrained as it has.). This is the 8231 * underlying object with id, value and extensions. The accessor 8232 * "getRequirements" gives direct access to the value 8233 */ 8234 public Conformance setRequirementsElement(StringType value) { 8235 this.requirements = value; 8236 return this; 8237 } 8238 8239 /** 8240 * @return Explains why this conformance statement is needed and why it's been 8241 * constrained as it has. 8242 */ 8243 public String getRequirements() { 8244 return this.requirements == null ? null : this.requirements.getValue(); 8245 } 8246 8247 /** 8248 * @param value Explains why this conformance statement is needed and why it's 8249 * been constrained as it has. 8250 */ 8251 public Conformance setRequirements(String value) { 8252 if (Utilities.noString(value)) 8253 this.requirements = null; 8254 else { 8255 if (this.requirements == null) 8256 this.requirements = new StringType(); 8257 this.requirements.setValue(value); 8258 } 8259 return this; 8260 } 8261 8262 /** 8263 * @return {@link #copyright} (A copyright statement relating to the conformance 8264 * statement and/or its contents. Copyright statements are generally 8265 * legal restrictions on the use and publishing of the details of the 8266 * system described by the conformance statement.). This is the 8267 * underlying object with id, value and extensions. The accessor 8268 * "getCopyright" gives direct access to the value 8269 */ 8270 public StringType getCopyrightElement() { 8271 if (this.copyright == null) 8272 if (Configuration.errorOnAutoCreate()) 8273 throw new Error("Attempt to auto-create Conformance.copyright"); 8274 else if (Configuration.doAutoCreate()) 8275 this.copyright = new StringType(); // bb 8276 return this.copyright; 8277 } 8278 8279 public boolean hasCopyrightElement() { 8280 return this.copyright != null && !this.copyright.isEmpty(); 8281 } 8282 8283 public boolean hasCopyright() { 8284 return this.copyright != null && !this.copyright.isEmpty(); 8285 } 8286 8287 /** 8288 * @param value {@link #copyright} (A copyright statement relating to the 8289 * conformance statement and/or its contents. Copyright statements 8290 * are generally legal restrictions on the use and publishing of 8291 * the details of the system described by the conformance 8292 * statement.). This is the underlying object with id, value and 8293 * extensions. The accessor "getCopyright" gives direct access to 8294 * the value 8295 */ 8296 public Conformance setCopyrightElement(StringType value) { 8297 this.copyright = value; 8298 return this; 8299 } 8300 8301 /** 8302 * @return A copyright statement relating to the conformance statement and/or 8303 * its contents. Copyright statements are generally legal restrictions 8304 * on the use and publishing of the details of the system described by 8305 * the conformance statement. 8306 */ 8307 public String getCopyright() { 8308 return this.copyright == null ? null : this.copyright.getValue(); 8309 } 8310 8311 /** 8312 * @param value A copyright statement relating to the conformance statement 8313 * and/or its contents. Copyright statements are generally legal 8314 * restrictions on the use and publishing of the details of the 8315 * system described by the conformance statement. 8316 */ 8317 public Conformance setCopyright(String value) { 8318 if (Utilities.noString(value)) 8319 this.copyright = null; 8320 else { 8321 if (this.copyright == null) 8322 this.copyright = new StringType(); 8323 this.copyright.setValue(value); 8324 } 8325 return this; 8326 } 8327 8328 /** 8329 * @return {@link #kind} (The way that this statement is intended to be used, to 8330 * describe an actual running instance of software, a particular product 8331 * (kind not instance of software) or a class of implementation (e.g. a 8332 * desired purchase).). This is the underlying object with id, value and 8333 * extensions. The accessor "getKind" gives direct access to the value 8334 */ 8335 public Enumeration<ConformanceStatementKind> getKindElement() { 8336 if (this.kind == null) 8337 if (Configuration.errorOnAutoCreate()) 8338 throw new Error("Attempt to auto-create Conformance.kind"); 8339 else if (Configuration.doAutoCreate()) 8340 this.kind = new Enumeration<ConformanceStatementKind>(new ConformanceStatementKindEnumFactory()); // bb 8341 return this.kind; 8342 } 8343 8344 public boolean hasKindElement() { 8345 return this.kind != null && !this.kind.isEmpty(); 8346 } 8347 8348 public boolean hasKind() { 8349 return this.kind != null && !this.kind.isEmpty(); 8350 } 8351 8352 /** 8353 * @param value {@link #kind} (The way that this statement is intended to be 8354 * used, to describe an actual running instance of software, a 8355 * particular product (kind not instance of software) or a class of 8356 * implementation (e.g. a desired purchase).). This is the 8357 * underlying object with id, value and extensions. The accessor 8358 * "getKind" gives direct access to the value 8359 */ 8360 public Conformance setKindElement(Enumeration<ConformanceStatementKind> value) { 8361 this.kind = value; 8362 return this; 8363 } 8364 8365 /** 8366 * @return The way that this statement is intended to be used, to describe an 8367 * actual running instance of software, a particular product (kind not 8368 * instance of software) or a class of implementation (e.g. a desired 8369 * purchase). 8370 */ 8371 public ConformanceStatementKind getKind() { 8372 return this.kind == null ? null : this.kind.getValue(); 8373 } 8374 8375 /** 8376 * @param value The way that this statement is intended to be used, to describe 8377 * an actual running instance of software, a particular product 8378 * (kind not instance of software) or a class of implementation 8379 * (e.g. a desired purchase). 8380 */ 8381 public Conformance setKind(ConformanceStatementKind value) { 8382 if (this.kind == null) 8383 this.kind = new Enumeration<ConformanceStatementKind>(new ConformanceStatementKindEnumFactory()); 8384 this.kind.setValue(value); 8385 return this; 8386 } 8387 8388 /** 8389 * @return {@link #software} (Software that is covered by this conformance 8390 * statement. It is used when the conformance statement describes the 8391 * capabilities of a particular software version, independent of an 8392 * installation.) 8393 */ 8394 public ConformanceSoftwareComponent getSoftware() { 8395 if (this.software == null) 8396 if (Configuration.errorOnAutoCreate()) 8397 throw new Error("Attempt to auto-create Conformance.software"); 8398 else if (Configuration.doAutoCreate()) 8399 this.software = new ConformanceSoftwareComponent(); // cc 8400 return this.software; 8401 } 8402 8403 public boolean hasSoftware() { 8404 return this.software != null && !this.software.isEmpty(); 8405 } 8406 8407 /** 8408 * @param value {@link #software} (Software that is covered by this conformance 8409 * statement. It is used when the conformance statement describes 8410 * the capabilities of a particular software version, independent 8411 * of an installation.) 8412 */ 8413 public Conformance setSoftware(ConformanceSoftwareComponent value) { 8414 this.software = value; 8415 return this; 8416 } 8417 8418 /** 8419 * @return {@link #implementation} (Identifies a specific implementation 8420 * instance that is described by the conformance statement - i.e. a 8421 * particular installation, rather than the capabilities of a software 8422 * program.) 8423 */ 8424 public ConformanceImplementationComponent getImplementation() { 8425 if (this.implementation == null) 8426 if (Configuration.errorOnAutoCreate()) 8427 throw new Error("Attempt to auto-create Conformance.implementation"); 8428 else if (Configuration.doAutoCreate()) 8429 this.implementation = new ConformanceImplementationComponent(); // cc 8430 return this.implementation; 8431 } 8432 8433 public boolean hasImplementation() { 8434 return this.implementation != null && !this.implementation.isEmpty(); 8435 } 8436 8437 /** 8438 * @param value {@link #implementation} (Identifies a specific implementation 8439 * instance that is described by the conformance statement - i.e. a 8440 * particular installation, rather than the capabilities of a 8441 * software program.) 8442 */ 8443 public Conformance setImplementation(ConformanceImplementationComponent value) { 8444 this.implementation = value; 8445 return this; 8446 } 8447 8448 /** 8449 * @return {@link #fhirVersion} (The version of the FHIR specification on which 8450 * this conformance statement is based.). This is the underlying object 8451 * with id, value and extensions. The accessor "getFhirVersion" gives 8452 * direct access to the value 8453 */ 8454 public IdType getFhirVersionElement() { 8455 if (this.fhirVersion == null) 8456 if (Configuration.errorOnAutoCreate()) 8457 throw new Error("Attempt to auto-create Conformance.fhirVersion"); 8458 else if (Configuration.doAutoCreate()) 8459 this.fhirVersion = new IdType(); // bb 8460 return this.fhirVersion; 8461 } 8462 8463 public boolean hasFhirVersionElement() { 8464 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 8465 } 8466 8467 public boolean hasFhirVersion() { 8468 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 8469 } 8470 8471 /** 8472 * @param value {@link #fhirVersion} (The version of the FHIR specification on 8473 * which this conformance statement is based.). This is the 8474 * underlying object with id, value and extensions. The accessor 8475 * "getFhirVersion" gives direct access to the value 8476 */ 8477 public Conformance setFhirVersionElement(IdType value) { 8478 this.fhirVersion = value; 8479 return this; 8480 } 8481 8482 /** 8483 * @return The version of the FHIR specification on which this conformance 8484 * statement is based. 8485 */ 8486 public String getFhirVersion() { 8487 return this.fhirVersion == null ? null : this.fhirVersion.getValue(); 8488 } 8489 8490 /** 8491 * @param value The version of the FHIR specification on which this conformance 8492 * statement is based. 8493 */ 8494 public Conformance setFhirVersion(String value) { 8495 if (this.fhirVersion == null) 8496 this.fhirVersion = new IdType(); 8497 this.fhirVersion.setValue(value); 8498 return this; 8499 } 8500 8501 /** 8502 * @return {@link #acceptUnknown} (A code that indicates whether the application 8503 * accepts unknown elements or extensions when reading resources.). This 8504 * is the underlying object with id, value and extensions. The accessor 8505 * "getAcceptUnknown" gives direct access to the value 8506 */ 8507 public Enumeration<UnknownContentCode> getAcceptUnknownElement() { 8508 if (this.acceptUnknown == null) 8509 if (Configuration.errorOnAutoCreate()) 8510 throw new Error("Attempt to auto-create Conformance.acceptUnknown"); 8511 else if (Configuration.doAutoCreate()) 8512 this.acceptUnknown = new Enumeration<UnknownContentCode>(new UnknownContentCodeEnumFactory()); // bb 8513 return this.acceptUnknown; 8514 } 8515 8516 public boolean hasAcceptUnknownElement() { 8517 return this.acceptUnknown != null && !this.acceptUnknown.isEmpty(); 8518 } 8519 8520 public boolean hasAcceptUnknown() { 8521 return this.acceptUnknown != null && !this.acceptUnknown.isEmpty(); 8522 } 8523 8524 /** 8525 * @param value {@link #acceptUnknown} (A code that indicates whether the 8526 * application accepts unknown elements or extensions when reading 8527 * resources.). This is the underlying object with id, value and 8528 * extensions. The accessor "getAcceptUnknown" gives direct access 8529 * to the value 8530 */ 8531 public Conformance setAcceptUnknownElement(Enumeration<UnknownContentCode> value) { 8532 this.acceptUnknown = value; 8533 return this; 8534 } 8535 8536 /** 8537 * @return A code that indicates whether the application accepts unknown 8538 * elements or extensions when reading resources. 8539 */ 8540 public UnknownContentCode getAcceptUnknown() { 8541 return this.acceptUnknown == null ? null : this.acceptUnknown.getValue(); 8542 } 8543 8544 /** 8545 * @param value A code that indicates whether the application accepts unknown 8546 * elements or extensions when reading resources. 8547 */ 8548 public Conformance setAcceptUnknown(UnknownContentCode value) { 8549 if (this.acceptUnknown == null) 8550 this.acceptUnknown = new Enumeration<UnknownContentCode>(new UnknownContentCodeEnumFactory()); 8551 this.acceptUnknown.setValue(value); 8552 return this; 8553 } 8554 8555 /** 8556 * @return {@link #format} (A list of the formats supported by this 8557 * implementation using their content types.) 8558 */ 8559 public List<CodeType> getFormat() { 8560 if (this.format == null) 8561 this.format = new ArrayList<CodeType>(); 8562 return this.format; 8563 } 8564 8565 public boolean hasFormat() { 8566 if (this.format == null) 8567 return false; 8568 for (CodeType item : this.format) 8569 if (!item.isEmpty()) 8570 return true; 8571 return false; 8572 } 8573 8574 /** 8575 * @return {@link #format} (A list of the formats supported by this 8576 * implementation using their content types.) 8577 */ 8578 // syntactic sugar 8579 public CodeType addFormatElement() {// 2 8580 CodeType t = new CodeType(); 8581 if (this.format == null) 8582 this.format = new ArrayList<CodeType>(); 8583 this.format.add(t); 8584 return t; 8585 } 8586 8587 /** 8588 * @param value {@link #format} (A list of the formats supported by this 8589 * implementation using their content types.) 8590 */ 8591 public Conformance addFormat(String value) { // 1 8592 CodeType t = new CodeType(); 8593 t.setValue(value); 8594 if (this.format == null) 8595 this.format = new ArrayList<CodeType>(); 8596 this.format.add(t); 8597 return this; 8598 } 8599 8600 /** 8601 * @param value {@link #format} (A list of the formats supported by this 8602 * implementation using their content types.) 8603 */ 8604 public boolean hasFormat(String value) { 8605 if (this.format == null) 8606 return false; 8607 for (CodeType v : this.format) 8608 if (v.equals(value)) // code 8609 return true; 8610 return false; 8611 } 8612 8613 /** 8614 * @return {@link #profile} (A list of profiles that represent different use 8615 * cases supported by the system. For a server, "supported by the 8616 * system" means the system hosts/produces a set of resources that are 8617 * conformant to a particular profile, and allows clients that use its 8618 * services to search using this profile and to find appropriate data. 8619 * For a client, it means the system will search by this profile and 8620 * process data according to the guidance implicit in the profile. See 8621 * further discussion in [Using Profiles]{profiling.html#profile-uses}.) 8622 */ 8623 public List<Reference> getProfile() { 8624 if (this.profile == null) 8625 this.profile = new ArrayList<Reference>(); 8626 return this.profile; 8627 } 8628 8629 public boolean hasProfile() { 8630 if (this.profile == null) 8631 return false; 8632 for (Reference item : this.profile) 8633 if (!item.isEmpty()) 8634 return true; 8635 return false; 8636 } 8637 8638 /** 8639 * @return {@link #profile} (A list of profiles that represent different use 8640 * cases supported by the system. For a server, "supported by the 8641 * system" means the system hosts/produces a set of resources that are 8642 * conformant to a particular profile, and allows clients that use its 8643 * services to search using this profile and to find appropriate data. 8644 * For a client, it means the system will search by this profile and 8645 * process data according to the guidance implicit in the profile. See 8646 * further discussion in [Using Profiles]{profiling.html#profile-uses}.) 8647 */ 8648 // syntactic sugar 8649 public Reference addProfile() { // 3 8650 Reference t = new Reference(); 8651 if (this.profile == null) 8652 this.profile = new ArrayList<Reference>(); 8653 this.profile.add(t); 8654 return t; 8655 } 8656 8657 // syntactic sugar 8658 public Conformance addProfile(Reference t) { // 3 8659 if (t == null) 8660 return this; 8661 if (this.profile == null) 8662 this.profile = new ArrayList<Reference>(); 8663 this.profile.add(t); 8664 return this; 8665 } 8666 8667 /** 8668 * @return {@link #profile} (The actual objects that are the target of the 8669 * reference. The reference library doesn't populate this, but you can 8670 * use this to hold the resources if you resolvethemt. A list of 8671 * profiles that represent different use cases supported by the system. 8672 * For a server, "supported by the system" means the system 8673 * hosts/produces a set of resources that are conformant to a particular 8674 * profile, and allows clients that use its services to search using 8675 * this profile and to find appropriate data. For a client, it means the 8676 * system will search by this profile and process data according to the 8677 * guidance implicit in the profile. See further discussion in [Using 8678 * Profiles]{profiling.html#profile-uses}.) 8679 */ 8680 public List<StructureDefinition> getProfileTarget() { 8681 if (this.profileTarget == null) 8682 this.profileTarget = new ArrayList<StructureDefinition>(); 8683 return this.profileTarget; 8684 } 8685 8686 // syntactic sugar 8687 /** 8688 * @return {@link #profile} (Add an actual object that is the target of the 8689 * reference. The reference library doesn't use these, but you can use 8690 * this to hold the resources if you resolvethemt. A list of profiles 8691 * that represent different use cases supported by the system. For a 8692 * server, "supported by the system" means the system hosts/produces a 8693 * set of resources that are conformant to a particular profile, and 8694 * allows clients that use its services to search using this profile and 8695 * to find appropriate data. For a client, it means the system will 8696 * search by this profile and process data according to the guidance 8697 * implicit in the profile. See further discussion in [Using 8698 * Profiles]{profiling.html#profile-uses}.) 8699 */ 8700 public StructureDefinition addProfileTarget() { 8701 StructureDefinition r = new StructureDefinition(); 8702 if (this.profileTarget == null) 8703 this.profileTarget = new ArrayList<StructureDefinition>(); 8704 this.profileTarget.add(r); 8705 return r; 8706 } 8707 8708 /** 8709 * @return {@link #rest} (A definition of the restful capabilities of the 8710 * solution, if any.) 8711 */ 8712 public List<ConformanceRestComponent> getRest() { 8713 if (this.rest == null) 8714 this.rest = new ArrayList<ConformanceRestComponent>(); 8715 return this.rest; 8716 } 8717 8718 public boolean hasRest() { 8719 if (this.rest == null) 8720 return false; 8721 for (ConformanceRestComponent item : this.rest) 8722 if (!item.isEmpty()) 8723 return true; 8724 return false; 8725 } 8726 8727 /** 8728 * @return {@link #rest} (A definition of the restful capabilities of the 8729 * solution, if any.) 8730 */ 8731 // syntactic sugar 8732 public ConformanceRestComponent addRest() { // 3 8733 ConformanceRestComponent t = new ConformanceRestComponent(); 8734 if (this.rest == null) 8735 this.rest = new ArrayList<ConformanceRestComponent>(); 8736 this.rest.add(t); 8737 return t; 8738 } 8739 8740 // syntactic sugar 8741 public Conformance addRest(ConformanceRestComponent t) { // 3 8742 if (t == null) 8743 return this; 8744 if (this.rest == null) 8745 this.rest = new ArrayList<ConformanceRestComponent>(); 8746 this.rest.add(t); 8747 return this; 8748 } 8749 8750 /** 8751 * @return {@link #messaging} (A description of the messaging capabilities of 8752 * the solution.) 8753 */ 8754 public List<ConformanceMessagingComponent> getMessaging() { 8755 if (this.messaging == null) 8756 this.messaging = new ArrayList<ConformanceMessagingComponent>(); 8757 return this.messaging; 8758 } 8759 8760 public boolean hasMessaging() { 8761 if (this.messaging == null) 8762 return false; 8763 for (ConformanceMessagingComponent item : this.messaging) 8764 if (!item.isEmpty()) 8765 return true; 8766 return false; 8767 } 8768 8769 /** 8770 * @return {@link #messaging} (A description of the messaging capabilities of 8771 * the solution.) 8772 */ 8773 // syntactic sugar 8774 public ConformanceMessagingComponent addMessaging() { // 3 8775 ConformanceMessagingComponent t = new ConformanceMessagingComponent(); 8776 if (this.messaging == null) 8777 this.messaging = new ArrayList<ConformanceMessagingComponent>(); 8778 this.messaging.add(t); 8779 return t; 8780 } 8781 8782 // syntactic sugar 8783 public Conformance addMessaging(ConformanceMessagingComponent t) { // 3 8784 if (t == null) 8785 return this; 8786 if (this.messaging == null) 8787 this.messaging = new ArrayList<ConformanceMessagingComponent>(); 8788 this.messaging.add(t); 8789 return this; 8790 } 8791 8792 /** 8793 * @return {@link #document} (A document definition.) 8794 */ 8795 public List<ConformanceDocumentComponent> getDocument() { 8796 if (this.document == null) 8797 this.document = new ArrayList<ConformanceDocumentComponent>(); 8798 return this.document; 8799 } 8800 8801 public boolean hasDocument() { 8802 if (this.document == null) 8803 return false; 8804 for (ConformanceDocumentComponent item : this.document) 8805 if (!item.isEmpty()) 8806 return true; 8807 return false; 8808 } 8809 8810 /** 8811 * @return {@link #document} (A document definition.) 8812 */ 8813 // syntactic sugar 8814 public ConformanceDocumentComponent addDocument() { // 3 8815 ConformanceDocumentComponent t = new ConformanceDocumentComponent(); 8816 if (this.document == null) 8817 this.document = new ArrayList<ConformanceDocumentComponent>(); 8818 this.document.add(t); 8819 return t; 8820 } 8821 8822 // syntactic sugar 8823 public Conformance addDocument(ConformanceDocumentComponent t) { // 3 8824 if (t == null) 8825 return this; 8826 if (this.document == null) 8827 this.document = new ArrayList<ConformanceDocumentComponent>(); 8828 this.document.add(t); 8829 return this; 8830 } 8831 8832 protected void listChildren(List<Property> childrenList) { 8833 super.listChildren(childrenList); 8834 childrenList.add(new Property("url", "uri", 8835 "An absolute URL that is used to identify this conformance statement when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this conformance statement is (or will be) published.", 8836 0, java.lang.Integer.MAX_VALUE, url)); 8837 childrenList.add(new Property("version", "string", 8838 "The identifier that is used to identify this version of the conformance statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the profile author manually and the value should be a timestamp.", 8839 0, java.lang.Integer.MAX_VALUE, version)); 8840 childrenList 8841 .add(new Property("name", "string", "A free text natural language name identifying the conformance statement.", 8842 0, java.lang.Integer.MAX_VALUE, name)); 8843 childrenList.add(new Property("status", "code", "The status of this conformance statement.", 0, 8844 java.lang.Integer.MAX_VALUE, status)); 8845 childrenList.add(new Property("experimental", "boolean", 8846 "A flag to indicate that this conformance statement is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 8847 0, java.lang.Integer.MAX_VALUE, experimental)); 8848 childrenList.add(new Property("publisher", "string", 8849 "The name of the individual or organization that published the conformance.", 0, java.lang.Integer.MAX_VALUE, 8850 publisher)); 8851 childrenList 8852 .add(new Property("contact", "", "Contacts to assist a user in finding and communicating with the publisher.", 8853 0, java.lang.Integer.MAX_VALUE, contact)); 8854 childrenList.add(new Property("date", "dateTime", 8855 "The date (and optionally time) when the conformance statement was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the conformance statement changes.", 8856 0, java.lang.Integer.MAX_VALUE, date)); 8857 childrenList.add(new Property("description", "string", 8858 "A free text natural language description of the conformance statement and its use. Typically, this is used when the conformance statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.", 8859 0, java.lang.Integer.MAX_VALUE, description)); 8860 childrenList.add(new Property("requirements", "string", 8861 "Explains why this conformance statement is needed and why it's been constrained as it has.", 0, 8862 java.lang.Integer.MAX_VALUE, requirements)); 8863 childrenList.add(new Property("copyright", "string", 8864 "A copyright statement relating to the conformance statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the details of the system described by the conformance statement.", 8865 0, java.lang.Integer.MAX_VALUE, copyright)); 8866 childrenList.add(new Property("kind", "code", 8867 "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind not instance of software) or a class of implementation (e.g. a desired purchase).", 8868 0, java.lang.Integer.MAX_VALUE, kind)); 8869 childrenList.add(new Property("software", "", 8870 "Software that is covered by this conformance statement. It is used when the conformance statement describes the capabilities of a particular software version, independent of an installation.", 8871 0, java.lang.Integer.MAX_VALUE, software)); 8872 childrenList.add(new Property("implementation", "", 8873 "Identifies a specific implementation instance that is described by the conformance statement - i.e. a particular installation, rather than the capabilities of a software program.", 8874 0, java.lang.Integer.MAX_VALUE, implementation)); 8875 childrenList.add(new Property("fhirVersion", "id", 8876 "The version of the FHIR specification on which this conformance statement is based.", 0, 8877 java.lang.Integer.MAX_VALUE, fhirVersion)); 8878 childrenList.add(new Property("acceptUnknown", "code", 8879 "A code that indicates whether the application accepts unknown elements or extensions when reading resources.", 8880 0, java.lang.Integer.MAX_VALUE, acceptUnknown)); 8881 childrenList.add(new Property("format", "code", 8882 "A list of the formats supported by this implementation using their content types.", 0, 8883 java.lang.Integer.MAX_VALUE, format)); 8884 childrenList.add(new Property("profile", "Reference(StructureDefinition)", 8885 "A list of profiles that represent different use cases supported by the system. For a server, \"supported by the system\" means the system hosts/produces a set of resources that are conformant to a particular profile, and allows clients that use its services to search using this profile and to find appropriate data. For a client, it means the system will search by this profile and process data according to the guidance implicit in the profile. See further discussion in [Using Profiles]{profiling.html#profile-uses}.", 8886 0, java.lang.Integer.MAX_VALUE, profile)); 8887 childrenList.add(new Property("rest", "", "A definition of the restful capabilities of the solution, if any.", 0, 8888 java.lang.Integer.MAX_VALUE, rest)); 8889 childrenList.add(new Property("messaging", "", "A description of the messaging capabilities of the solution.", 0, 8890 java.lang.Integer.MAX_VALUE, messaging)); 8891 childrenList.add(new Property("document", "", "A document definition.", 0, java.lang.Integer.MAX_VALUE, document)); 8892 } 8893 8894 @Override 8895 public void setProperty(String name, Base value) throws FHIRException { 8896 if (name.equals("url")) 8897 this.url = castToUri(value); // UriType 8898 else if (name.equals("version")) 8899 this.version = castToString(value); // StringType 8900 else if (name.equals("name")) 8901 this.name = castToString(value); // StringType 8902 else if (name.equals("status")) 8903 this.status = new ConformanceResourceStatusEnumFactory().fromType(value); // Enumeration<ConformanceResourceStatus> 8904 else if (name.equals("experimental")) 8905 this.experimental = castToBoolean(value); // BooleanType 8906 else if (name.equals("publisher")) 8907 this.publisher = castToString(value); // StringType 8908 else if (name.equals("contact")) 8909 this.getContact().add((ConformanceContactComponent) value); 8910 else if (name.equals("date")) 8911 this.date = castToDateTime(value); // DateTimeType 8912 else if (name.equals("description")) 8913 this.description = castToString(value); // StringType 8914 else if (name.equals("requirements")) 8915 this.requirements = castToString(value); // StringType 8916 else if (name.equals("copyright")) 8917 this.copyright = castToString(value); // StringType 8918 else if (name.equals("kind")) 8919 this.kind = new ConformanceStatementKindEnumFactory().fromType(value); // Enumeration<ConformanceStatementKind> 8920 else if (name.equals("software")) 8921 this.software = (ConformanceSoftwareComponent) value; // ConformanceSoftwareComponent 8922 else if (name.equals("implementation")) 8923 this.implementation = (ConformanceImplementationComponent) value; // ConformanceImplementationComponent 8924 else if (name.equals("fhirVersion")) 8925 this.fhirVersion = castToId(value); // IdType 8926 else if (name.equals("acceptUnknown")) 8927 this.acceptUnknown = new UnknownContentCodeEnumFactory().fromType(value); // Enumeration<UnknownContentCode> 8928 else if (name.equals("format")) 8929 this.getFormat().add(castToCode(value)); 8930 else if (name.equals("profile")) 8931 this.getProfile().add(castToReference(value)); 8932 else if (name.equals("rest")) 8933 this.getRest().add((ConformanceRestComponent) value); 8934 else if (name.equals("messaging")) 8935 this.getMessaging().add((ConformanceMessagingComponent) value); 8936 else if (name.equals("document")) 8937 this.getDocument().add((ConformanceDocumentComponent) value); 8938 else 8939 super.setProperty(name, value); 8940 } 8941 8942 @Override 8943 public Base addChild(String name) throws FHIRException { 8944 if (name.equals("url")) { 8945 throw new FHIRException("Cannot call addChild on a singleton property Conformance.url"); 8946 } else if (name.equals("version")) { 8947 throw new FHIRException("Cannot call addChild on a singleton property Conformance.version"); 8948 } else if (name.equals("name")) { 8949 throw new FHIRException("Cannot call addChild on a singleton property Conformance.name"); 8950 } else if (name.equals("status")) { 8951 throw new FHIRException("Cannot call addChild on a singleton property Conformance.status"); 8952 } else if (name.equals("experimental")) { 8953 throw new FHIRException("Cannot call addChild on a singleton property Conformance.experimental"); 8954 } else if (name.equals("publisher")) { 8955 throw new FHIRException("Cannot call addChild on a singleton property Conformance.publisher"); 8956 } else if (name.equals("contact")) { 8957 return addContact(); 8958 } else if (name.equals("date")) { 8959 throw new FHIRException("Cannot call addChild on a singleton property Conformance.date"); 8960 } else if (name.equals("description")) { 8961 throw new FHIRException("Cannot call addChild on a singleton property Conformance.description"); 8962 } else if (name.equals("requirements")) { 8963 throw new FHIRException("Cannot call addChild on a singleton property Conformance.requirements"); 8964 } else if (name.equals("copyright")) { 8965 throw new FHIRException("Cannot call addChild on a singleton property Conformance.copyright"); 8966 } else if (name.equals("kind")) { 8967 throw new FHIRException("Cannot call addChild on a singleton property Conformance.kind"); 8968 } else if (name.equals("software")) { 8969 this.software = new ConformanceSoftwareComponent(); 8970 return this.software; 8971 } else if (name.equals("implementation")) { 8972 this.implementation = new ConformanceImplementationComponent(); 8973 return this.implementation; 8974 } else if (name.equals("fhirVersion")) { 8975 throw new FHIRException("Cannot call addChild on a singleton property Conformance.fhirVersion"); 8976 } else if (name.equals("acceptUnknown")) { 8977 throw new FHIRException("Cannot call addChild on a singleton property Conformance.acceptUnknown"); 8978 } else if (name.equals("format")) { 8979 throw new FHIRException("Cannot call addChild on a singleton property Conformance.format"); 8980 } else if (name.equals("profile")) { 8981 return addProfile(); 8982 } else if (name.equals("rest")) { 8983 return addRest(); 8984 } else if (name.equals("messaging")) { 8985 return addMessaging(); 8986 } else if (name.equals("document")) { 8987 return addDocument(); 8988 } else 8989 return super.addChild(name); 8990 } 8991 8992 public String fhirType() { 8993 return "Conformance"; 8994 8995 } 8996 8997 public Conformance copy() { 8998 Conformance dst = new Conformance(); 8999 copyValues(dst); 9000 dst.url = url == null ? null : url.copy(); 9001 dst.version = version == null ? null : version.copy(); 9002 dst.name = name == null ? null : name.copy(); 9003 dst.status = status == null ? null : status.copy(); 9004 dst.experimental = experimental == null ? null : experimental.copy(); 9005 dst.publisher = publisher == null ? null : publisher.copy(); 9006 if (contact != null) { 9007 dst.contact = new ArrayList<ConformanceContactComponent>(); 9008 for (ConformanceContactComponent i : contact) 9009 dst.contact.add(i.copy()); 9010 } 9011 ; 9012 dst.date = date == null ? null : date.copy(); 9013 dst.description = description == null ? null : description.copy(); 9014 dst.requirements = requirements == null ? null : requirements.copy(); 9015 dst.copyright = copyright == null ? null : copyright.copy(); 9016 dst.kind = kind == null ? null : kind.copy(); 9017 dst.software = software == null ? null : software.copy(); 9018 dst.implementation = implementation == null ? null : implementation.copy(); 9019 dst.fhirVersion = fhirVersion == null ? null : fhirVersion.copy(); 9020 dst.acceptUnknown = acceptUnknown == null ? null : acceptUnknown.copy(); 9021 if (format != null) { 9022 dst.format = new ArrayList<CodeType>(); 9023 for (CodeType i : format) 9024 dst.format.add(i.copy()); 9025 } 9026 ; 9027 if (profile != null) { 9028 dst.profile = new ArrayList<Reference>(); 9029 for (Reference i : profile) 9030 dst.profile.add(i.copy()); 9031 } 9032 ; 9033 if (rest != null) { 9034 dst.rest = new ArrayList<ConformanceRestComponent>(); 9035 for (ConformanceRestComponent i : rest) 9036 dst.rest.add(i.copy()); 9037 } 9038 ; 9039 if (messaging != null) { 9040 dst.messaging = new ArrayList<ConformanceMessagingComponent>(); 9041 for (ConformanceMessagingComponent i : messaging) 9042 dst.messaging.add(i.copy()); 9043 } 9044 ; 9045 if (document != null) { 9046 dst.document = new ArrayList<ConformanceDocumentComponent>(); 9047 for (ConformanceDocumentComponent i : document) 9048 dst.document.add(i.copy()); 9049 } 9050 ; 9051 return dst; 9052 } 9053 9054 protected Conformance typedCopy() { 9055 return copy(); 9056 } 9057 9058 @Override 9059 public boolean equalsDeep(Base other) { 9060 if (!super.equalsDeep(other)) 9061 return false; 9062 if (!(other instanceof Conformance)) 9063 return false; 9064 Conformance o = (Conformance) other; 9065 return compareDeep(url, o.url, true) && compareDeep(version, o.version, true) && compareDeep(name, o.name, true) 9066 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 9067 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 9068 && compareDeep(date, o.date, true) && compareDeep(description, o.description, true) 9069 && compareDeep(requirements, o.requirements, true) && compareDeep(copyright, o.copyright, true) 9070 && compareDeep(kind, o.kind, true) && compareDeep(software, o.software, true) 9071 && compareDeep(implementation, o.implementation, true) && compareDeep(fhirVersion, o.fhirVersion, true) 9072 && compareDeep(acceptUnknown, o.acceptUnknown, true) && compareDeep(format, o.format, true) 9073 && compareDeep(profile, o.profile, true) && compareDeep(rest, o.rest, true) 9074 && compareDeep(messaging, o.messaging, true) && compareDeep(document, o.document, true); 9075 } 9076 9077 @Override 9078 public boolean equalsShallow(Base other) { 9079 if (!super.equalsShallow(other)) 9080 return false; 9081 if (!(other instanceof Conformance)) 9082 return false; 9083 Conformance o = (Conformance) other; 9084 return compareValues(url, o.url, true) && compareValues(version, o.version, true) 9085 && compareValues(name, o.name, true) && compareValues(status, o.status, true) 9086 && compareValues(experimental, o.experimental, true) && compareValues(publisher, o.publisher, true) 9087 && compareValues(date, o.date, true) && compareValues(description, o.description, true) 9088 && compareValues(requirements, o.requirements, true) && compareValues(copyright, o.copyright, true) 9089 && compareValues(kind, o.kind, true) && compareValues(fhirVersion, o.fhirVersion, true) 9090 && compareValues(acceptUnknown, o.acceptUnknown, true) && compareValues(format, o.format, true); 9091 } 9092 9093 public boolean isEmpty() { 9094 return super.isEmpty() && (url == null || url.isEmpty()) && (version == null || version.isEmpty()) 9095 && (name == null || name.isEmpty()) && (status == null || status.isEmpty()) 9096 && (experimental == null || experimental.isEmpty()) && (publisher == null || publisher.isEmpty()) 9097 && (contact == null || contact.isEmpty()) && (date == null || date.isEmpty()) 9098 && (description == null || description.isEmpty()) && (requirements == null || requirements.isEmpty()) 9099 && (copyright == null || copyright.isEmpty()) && (kind == null || kind.isEmpty()) 9100 && (software == null || software.isEmpty()) && (implementation == null || implementation.isEmpty()) 9101 && (fhirVersion == null || fhirVersion.isEmpty()) && (acceptUnknown == null || acceptUnknown.isEmpty()) 9102 && (format == null || format.isEmpty()) && (profile == null || profile.isEmpty()) 9103 && (rest == null || rest.isEmpty()) && (messaging == null || messaging.isEmpty()) 9104 && (document == null || document.isEmpty()); 9105 } 9106 9107 @Override 9108 public ResourceType getResourceType() { 9109 return ResourceType.Conformance; 9110 } 9111 9112 @SearchParamDefinition(name = "date", path = "Conformance.date", description = "The conformance statement publication date", type = "date") 9113 public static final String SP_DATE = "date"; 9114 @SearchParamDefinition(name = "software", path = "Conformance.software.name", description = "Part of a the name of a software application", type = "string") 9115 public static final String SP_SOFTWARE = "software"; 9116 @SearchParamDefinition(name = "resource", path = "Conformance.rest.resource.type", description = "Name of a resource mentioned in a conformance statement", type = "token") 9117 public static final String SP_RESOURCE = "resource"; 9118 @SearchParamDefinition(name = "profile", path = "Conformance.rest.resource.profile", description = "A profile id invoked in a conformance statement", type = "reference") 9119 public static final String SP_PROFILE = "profile"; 9120 @SearchParamDefinition(name = "format", path = "Conformance.format", description = "formats supported (xml | json | mime type)", type = "token") 9121 public static final String SP_FORMAT = "format"; 9122 @SearchParamDefinition(name = "description", path = "Conformance.description", description = "Text search in the description of the conformance statement", type = "string") 9123 public static final String SP_DESCRIPTION = "description"; 9124 @SearchParamDefinition(name = "fhirversion", path = "Conformance.version", description = "The version of FHIR", type = "token") 9125 public static final String SP_FHIRVERSION = "fhirversion"; 9126 @SearchParamDefinition(name = "version", path = "Conformance.version", description = "The version identifier of the conformance statement", type = "token") 9127 public static final String SP_VERSION = "version"; 9128 @SearchParamDefinition(name = "url", path = "Conformance.url", description = "The uri that identifies the conformance statement", type = "uri") 9129 public static final String SP_URL = "url"; 9130 @SearchParamDefinition(name = "supported-profile", path = "Conformance.profile", description = "Profiles for use cases supported", type = "reference") 9131 public static final String SP_SUPPORTEDPROFILE = "supported-profile"; 9132 @SearchParamDefinition(name = "mode", path = "Conformance.rest.mode", description = "Mode - restful (server/client) or messaging (sender/receiver)", type = "token") 9133 public static final String SP_MODE = "mode"; 9134 @SearchParamDefinition(name = "security", path = "Conformance.rest.security.service", description = "OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | Certificates", type = "token") 9135 public static final String SP_SECURITY = "security"; 9136 @SearchParamDefinition(name = "name", path = "Conformance.name", description = "Name of the conformance statement", type = "string") 9137 public static final String SP_NAME = "name"; 9138 @SearchParamDefinition(name = "publisher", path = "Conformance.publisher", description = "Name of the publisher of the conformance statement", type = "string") 9139 public static final String SP_PUBLISHER = "publisher"; 9140 @SearchParamDefinition(name = "event", path = "Conformance.messaging.event.code", description = "Event code in a conformance statement", type = "token") 9141 public static final String SP_EVENT = "event"; 9142 @SearchParamDefinition(name = "status", path = "Conformance.status", description = "The current status of the conformance statement", type = "token") 9143 public static final String SP_STATUS = "status"; 9144 9145}