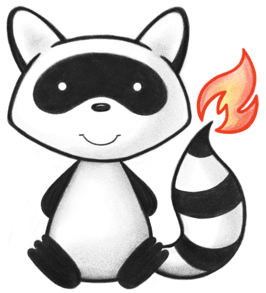
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.List; 034 035import ca.uhn.fhir.model.api.annotation.Child; 036import ca.uhn.fhir.model.api.annotation.DatatypeDef; 037import ca.uhn.fhir.model.api.annotation.Description; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.utilities.Utilities; 041 042/** 043 * Details for all kinds of technology mediated contact points for a person or 044 * organization, including telephone, email, etc. 045 */ 046@DatatypeDef(name = "ContactPoint") 047public class ContactPoint extends Type implements ICompositeType { 048 049 public enum ContactPointSystem { 050 /** 051 * The value is a telephone number used for voice calls. Use of full 052 * international numbers starting with + is recommended to enable automatic 053 * dialing support but not required. 054 */ 055 PHONE, 056 /** 057 * The value is a fax machine. Use of full international numbers starting with + 058 * is recommended to enable automatic dialing support but not required. 059 */ 060 FAX, 061 /** 062 * The value is an email address. 063 */ 064 EMAIL, 065 /** 066 * The value is a pager number. These may be local pager numbers that are only 067 * usable on a particular pager system. 068 */ 069 PAGER, 070 /** 071 * A contact that is not a phone, fax, or email address. The format of the value 072 * SHOULD be a URL. This is intended for various personal contacts including 073 * blogs, Twitter, Facebook, etc. Do not use for email addresses. If this is not 074 * a URL, then it will require human interpretation. 075 */ 076 OTHER, 077 /** 078 * added to help the parsers 079 */ 080 NULL; 081 082 public static ContactPointSystem fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("phone".equals(codeString)) 086 return PHONE; 087 if ("fax".equals(codeString)) 088 return FAX; 089 if ("email".equals(codeString)) 090 return EMAIL; 091 if ("pager".equals(codeString)) 092 return PAGER; 093 if ("other".equals(codeString)) 094 return OTHER; 095 throw new FHIRException("Unknown ContactPointSystem code '" + codeString + "'"); 096 } 097 098 public String toCode() { 099 switch (this) { 100 case PHONE: 101 return "phone"; 102 case FAX: 103 return "fax"; 104 case EMAIL: 105 return "email"; 106 case PAGER: 107 return "pager"; 108 case OTHER: 109 return "other"; 110 case NULL: 111 return null; 112 default: 113 return "?"; 114 } 115 } 116 117 public String getSystem() { 118 switch (this) { 119 case PHONE: 120 return "http://hl7.org/fhir/contact-point-system"; 121 case FAX: 122 return "http://hl7.org/fhir/contact-point-system"; 123 case EMAIL: 124 return "http://hl7.org/fhir/contact-point-system"; 125 case PAGER: 126 return "http://hl7.org/fhir/contact-point-system"; 127 case OTHER: 128 return "http://hl7.org/fhir/contact-point-system"; 129 case NULL: 130 return null; 131 default: 132 return "?"; 133 } 134 } 135 136 public String getDefinition() { 137 switch (this) { 138 case PHONE: 139 return "The value is a telephone number used for voice calls. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required."; 140 case FAX: 141 return "The value is a fax machine. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required."; 142 case EMAIL: 143 return "The value is an email address."; 144 case PAGER: 145 return "The value is a pager number. These may be local pager numbers that are only usable on a particular pager system."; 146 case OTHER: 147 return "A contact that is not a phone, fax, or email address. The format of the value SHOULD be a URL. This is intended for various personal contacts including blogs, Twitter, Facebook, etc. Do not use for email addresses. If this is not a URL, then it will require human interpretation."; 148 case NULL: 149 return null; 150 default: 151 return "?"; 152 } 153 } 154 155 public String getDisplay() { 156 switch (this) { 157 case PHONE: 158 return "Phone"; 159 case FAX: 160 return "Fax"; 161 case EMAIL: 162 return "Email"; 163 case PAGER: 164 return "Pager"; 165 case OTHER: 166 return "URL"; 167 case NULL: 168 return null; 169 default: 170 return "?"; 171 } 172 } 173 } 174 175 public static class ContactPointSystemEnumFactory implements EnumFactory<ContactPointSystem> { 176 public ContactPointSystem fromCode(String codeString) throws IllegalArgumentException { 177 if (codeString == null || "".equals(codeString)) 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("phone".equals(codeString)) 181 return ContactPointSystem.PHONE; 182 if ("fax".equals(codeString)) 183 return ContactPointSystem.FAX; 184 if ("email".equals(codeString)) 185 return ContactPointSystem.EMAIL; 186 if ("pager".equals(codeString)) 187 return ContactPointSystem.PAGER; 188 if ("other".equals(codeString)) 189 return ContactPointSystem.OTHER; 190 throw new IllegalArgumentException("Unknown ContactPointSystem code '" + codeString + "'"); 191 } 192 193 public Enumeration<ContactPointSystem> fromType(Base code) throws FHIRException { 194 if (code == null || code.isEmpty()) 195 return null; 196 String codeString = ((PrimitiveType) code).asStringValue(); 197 if (codeString == null || "".equals(codeString)) 198 return null; 199 if ("phone".equals(codeString)) 200 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.PHONE); 201 if ("fax".equals(codeString)) 202 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.FAX); 203 if ("email".equals(codeString)) 204 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.EMAIL); 205 if ("pager".equals(codeString)) 206 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.PAGER); 207 if ("other".equals(codeString)) 208 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.OTHER); 209 throw new FHIRException("Unknown ContactPointSystem code '" + codeString + "'"); 210 } 211 212 public String toCode(ContactPointSystem code) 213 { 214 if (code == ContactPointSystem.NULL) 215 return null; 216 if (code == ContactPointSystem.PHONE) 217 return "phone"; 218 if (code == ContactPointSystem.FAX) 219 return "fax"; 220 if (code == ContactPointSystem.EMAIL) 221 return "email"; 222 if (code == ContactPointSystem.PAGER) 223 return "pager"; 224 if (code == ContactPointSystem.OTHER) 225 return "other"; 226 return "?"; 227 } 228 } 229 230 public enum ContactPointUse { 231 /** 232 * A communication contact point at a home; attempted contacts for business 233 * purposes might intrude privacy and chances are one will contact family or 234 * other household members instead of the person one wishes to call. Typically 235 * used with urgent cases, or if no other contacts are available. 236 */ 237 HOME, 238 /** 239 * An office contact point. First choice for business related contacts during 240 * business hours. 241 */ 242 WORK, 243 /** 244 * A temporary contact point. The period can provide more detailed information. 245 */ 246 TEMP, 247 /** 248 * This contact point is no longer in use (or was never correct, but retained 249 * for records). 250 */ 251 OLD, 252 /** 253 * A telecommunication device that moves and stays with its owner. May have 254 * characteristics of all other use codes, suitable for urgent matters, not the 255 * first choice for routine business. 256 */ 257 MOBILE, 258 /** 259 * added to help the parsers 260 */ 261 NULL; 262 263 public static ContactPointUse fromCode(String codeString) throws FHIRException { 264 if (codeString == null || "".equals(codeString)) 265 return null; 266 if ("home".equals(codeString)) 267 return HOME; 268 if ("work".equals(codeString)) 269 return WORK; 270 if ("temp".equals(codeString)) 271 return TEMP; 272 if ("old".equals(codeString)) 273 return OLD; 274 if ("mobile".equals(codeString)) 275 return MOBILE; 276 throw new FHIRException("Unknown ContactPointUse code '" + codeString + "'"); 277 } 278 279 public String toCode() { 280 switch (this) { 281 case HOME: 282 return "home"; 283 case WORK: 284 return "work"; 285 case TEMP: 286 return "temp"; 287 case OLD: 288 return "old"; 289 case MOBILE: 290 return "mobile"; 291 case NULL: 292 return null; 293 default: 294 return "?"; 295 } 296 } 297 298 public String getSystem() { 299 switch (this) { 300 case HOME: 301 return "http://hl7.org/fhir/contact-point-use"; 302 case WORK: 303 return "http://hl7.org/fhir/contact-point-use"; 304 case TEMP: 305 return "http://hl7.org/fhir/contact-point-use"; 306 case OLD: 307 return "http://hl7.org/fhir/contact-point-use"; 308 case MOBILE: 309 return "http://hl7.org/fhir/contact-point-use"; 310 case NULL: 311 return null; 312 default: 313 return "?"; 314 } 315 } 316 317 public String getDefinition() { 318 switch (this) { 319 case HOME: 320 return "A communication contact point at a home; attempted contacts for business purposes might intrude privacy and chances are one will contact family or other household members instead of the person one wishes to call. Typically used with urgent cases, or if no other contacts are available."; 321 case WORK: 322 return "An office contact point. First choice for business related contacts during business hours."; 323 case TEMP: 324 return "A temporary contact point. The period can provide more detailed information."; 325 case OLD: 326 return "This contact point is no longer in use (or was never correct, but retained for records)."; 327 case MOBILE: 328 return "A telecommunication device that moves and stays with its owner. May have characteristics of all other use codes, suitable for urgent matters, not the first choice for routine business."; 329 case NULL: 330 return null; 331 default: 332 return "?"; 333 } 334 } 335 336 public String getDisplay() { 337 switch (this) { 338 case HOME: 339 return "Home"; 340 case WORK: 341 return "Work"; 342 case TEMP: 343 return "Temp"; 344 case OLD: 345 return "Old"; 346 case MOBILE: 347 return "Mobile"; 348 case NULL: 349 return null; 350 default: 351 return "?"; 352 } 353 } 354 } 355 356 public static class ContactPointUseEnumFactory implements EnumFactory<ContactPointUse> { 357 public ContactPointUse fromCode(String codeString) throws IllegalArgumentException { 358 if (codeString == null || "".equals(codeString)) 359 if (codeString == null || "".equals(codeString)) 360 return null; 361 if ("home".equals(codeString)) 362 return ContactPointUse.HOME; 363 if ("work".equals(codeString)) 364 return ContactPointUse.WORK; 365 if ("temp".equals(codeString)) 366 return ContactPointUse.TEMP; 367 if ("old".equals(codeString)) 368 return ContactPointUse.OLD; 369 if ("mobile".equals(codeString)) 370 return ContactPointUse.MOBILE; 371 throw new IllegalArgumentException("Unknown ContactPointUse code '" + codeString + "'"); 372 } 373 374 public Enumeration<ContactPointUse> fromType(Base code) throws FHIRException { 375 if (code == null || code.isEmpty()) 376 return null; 377 String codeString = ((PrimitiveType) code).asStringValue(); 378 if (codeString == null || "".equals(codeString)) 379 return null; 380 if ("home".equals(codeString)) 381 return new Enumeration<ContactPointUse>(this, ContactPointUse.HOME); 382 if ("work".equals(codeString)) 383 return new Enumeration<ContactPointUse>(this, ContactPointUse.WORK); 384 if ("temp".equals(codeString)) 385 return new Enumeration<ContactPointUse>(this, ContactPointUse.TEMP); 386 if ("old".equals(codeString)) 387 return new Enumeration<ContactPointUse>(this, ContactPointUse.OLD); 388 if ("mobile".equals(codeString)) 389 return new Enumeration<ContactPointUse>(this, ContactPointUse.MOBILE); 390 throw new FHIRException("Unknown ContactPointUse code '" + codeString + "'"); 391 } 392 393 public String toCode(ContactPointUse code) 394 { 395 if (code == ContactPointUse.NULL) 396 return null; 397 if (code == ContactPointUse.HOME) 398 return "home"; 399 if (code == ContactPointUse.WORK) 400 return "work"; 401 if (code == ContactPointUse.TEMP) 402 return "temp"; 403 if (code == ContactPointUse.OLD) 404 return "old"; 405 if (code == ContactPointUse.MOBILE) 406 return "mobile"; 407 return "?"; 408 } 409 } 410 411 /** 412 * Telecommunications form for contact point - what communications system is 413 * required to make use of the contact. 414 */ 415 @Child(name = "system", type = { CodeType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 416 @Description(shortDefinition = "phone | fax | email | pager | other", formalDefinition = "Telecommunications form for contact point - what communications system is required to make use of the contact.") 417 protected Enumeration<ContactPointSystem> system; 418 419 /** 420 * The actual contact point details, in a form that is meaningful to the 421 * designated communication system (i.e. phone number or email address). 422 */ 423 @Child(name = "value", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 424 @Description(shortDefinition = "The actual contact point details", formalDefinition = "The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address).") 425 protected StringType value; 426 427 /** 428 * Identifies the purpose for the contact point. 429 */ 430 @Child(name = "use", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = true, summary = true) 431 @Description(shortDefinition = "home | work | temp | old | mobile - purpose of this contact point", formalDefinition = "Identifies the purpose for the contact point.") 432 protected Enumeration<ContactPointUse> use; 433 434 /** 435 * Specifies a preferred order in which to use a set of contacts. Contacts are 436 * ranked with lower values coming before higher values. 437 */ 438 @Child(name = "rank", type = { PositiveIntType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 439 @Description(shortDefinition = "Specify preferred order of use (1 = highest)", formalDefinition = "Specifies a preferred order in which to use a set of contacts. Contacts are ranked with lower values coming before higher values.") 440 protected PositiveIntType rank; 441 442 /** 443 * Time period when the contact point was/is in use. 444 */ 445 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 446 @Description(shortDefinition = "Time period when the contact point was/is in use", formalDefinition = "Time period when the contact point was/is in use.") 447 protected Period period; 448 449 private static final long serialVersionUID = 1509610874L; 450 451 /* 452 * Constructor 453 */ 454 public ContactPoint() { 455 super(); 456 } 457 458 /** 459 * @return {@link #system} (Telecommunications form for contact point - what 460 * communications system is required to make use of the contact.). This 461 * is the underlying object with id, value and extensions. The accessor 462 * "getSystem" gives direct access to the value 463 */ 464 public Enumeration<ContactPointSystem> getSystemElement() { 465 if (this.system == null) 466 if (Configuration.errorOnAutoCreate()) 467 throw new Error("Attempt to auto-create ContactPoint.system"); 468 else if (Configuration.doAutoCreate()) 469 this.system = new Enumeration<ContactPointSystem>(new ContactPointSystemEnumFactory()); // bb 470 return this.system; 471 } 472 473 public boolean hasSystemElement() { 474 return this.system != null && !this.system.isEmpty(); 475 } 476 477 public boolean hasSystem() { 478 return this.system != null && !this.system.isEmpty(); 479 } 480 481 /** 482 * @param value {@link #system} (Telecommunications form for contact point - 483 * what communications system is required to make use of the 484 * contact.). This is the underlying object with id, value and 485 * extensions. The accessor "getSystem" gives direct access to the 486 * value 487 */ 488 public ContactPoint setSystemElement(Enumeration<ContactPointSystem> value) { 489 this.system = value; 490 return this; 491 } 492 493 /** 494 * @return Telecommunications form for contact point - what communications 495 * system is required to make use of the contact. 496 */ 497 public ContactPointSystem getSystem() { 498 return this.system == null ? null : this.system.getValue(); 499 } 500 501 /** 502 * @param value Telecommunications form for contact point - what communications 503 * system is required to make use of the contact. 504 */ 505 public ContactPoint setSystem(ContactPointSystem value) { 506 if (value == null) 507 this.system = null; 508 else { 509 if (this.system == null) 510 this.system = new Enumeration<ContactPointSystem>(new ContactPointSystemEnumFactory()); 511 this.system.setValue(value); 512 } 513 return this; 514 } 515 516 /** 517 * @return {@link #value} (The actual contact point details, in a form that is 518 * meaningful to the designated communication system (i.e. phone number 519 * or email address).). This is the underlying object with id, value and 520 * extensions. The accessor "getValue" gives direct access to the value 521 */ 522 public StringType getValueElement() { 523 if (this.value == null) 524 if (Configuration.errorOnAutoCreate()) 525 throw new Error("Attempt to auto-create ContactPoint.value"); 526 else if (Configuration.doAutoCreate()) 527 this.value = new StringType(); // bb 528 return this.value; 529 } 530 531 public boolean hasValueElement() { 532 return this.value != null && !this.value.isEmpty(); 533 } 534 535 public boolean hasValue() { 536 return this.value != null && !this.value.isEmpty(); 537 } 538 539 /** 540 * @param value {@link #value} (The actual contact point details, in a form that 541 * is meaningful to the designated communication system (i.e. phone 542 * number or email address).). This is the underlying object with 543 * id, value and extensions. The accessor "getValue" gives direct 544 * access to the value 545 */ 546 public ContactPoint setValueElement(StringType value) { 547 this.value = value; 548 return this; 549 } 550 551 /** 552 * @return The actual contact point details, in a form that is meaningful to the 553 * designated communication system (i.e. phone number or email address). 554 */ 555 public String getValue() { 556 return this.value == null ? null : this.value.getValue(); 557 } 558 559 /** 560 * @param value The actual contact point details, in a form that is meaningful 561 * to the designated communication system (i.e. phone number or 562 * email address). 563 */ 564 public ContactPoint setValue(String value) { 565 if (Utilities.noString(value)) 566 this.value = null; 567 else { 568 if (this.value == null) 569 this.value = new StringType(); 570 this.value.setValue(value); 571 } 572 return this; 573 } 574 575 /** 576 * @return {@link #use} (Identifies the purpose for the contact point.). This is 577 * the underlying object with id, value and extensions. The accessor 578 * "getUse" gives direct access to the value 579 */ 580 public Enumeration<ContactPointUse> getUseElement() { 581 if (this.use == null) 582 if (Configuration.errorOnAutoCreate()) 583 throw new Error("Attempt to auto-create ContactPoint.use"); 584 else if (Configuration.doAutoCreate()) 585 this.use = new Enumeration<ContactPointUse>(new ContactPointUseEnumFactory()); // bb 586 return this.use; 587 } 588 589 public boolean hasUseElement() { 590 return this.use != null && !this.use.isEmpty(); 591 } 592 593 public boolean hasUse() { 594 return this.use != null && !this.use.isEmpty(); 595 } 596 597 /** 598 * @param value {@link #use} (Identifies the purpose for the contact point.). 599 * This is the underlying object with id, value and extensions. The 600 * accessor "getUse" gives direct access to the value 601 */ 602 public ContactPoint setUseElement(Enumeration<ContactPointUse> value) { 603 this.use = value; 604 return this; 605 } 606 607 /** 608 * @return Identifies the purpose for the contact point. 609 */ 610 public ContactPointUse getUse() { 611 return this.use == null ? null : this.use.getValue(); 612 } 613 614 /** 615 * @param value Identifies the purpose for the contact point. 616 */ 617 public ContactPoint setUse(ContactPointUse value) { 618 if (value == null) 619 this.use = null; 620 else { 621 if (this.use == null) 622 this.use = new Enumeration<ContactPointUse>(new ContactPointUseEnumFactory()); 623 this.use.setValue(value); 624 } 625 return this; 626 } 627 628 /** 629 * @return {@link #rank} (Specifies a preferred order in which to use a set of 630 * contacts. Contacts are ranked with lower values coming before higher 631 * values.). This is the underlying object with id, value and 632 * extensions. The accessor "getRank" gives direct access to the value 633 */ 634 public PositiveIntType getRankElement() { 635 if (this.rank == null) 636 if (Configuration.errorOnAutoCreate()) 637 throw new Error("Attempt to auto-create ContactPoint.rank"); 638 else if (Configuration.doAutoCreate()) 639 this.rank = new PositiveIntType(); // bb 640 return this.rank; 641 } 642 643 public boolean hasRankElement() { 644 return this.rank != null && !this.rank.isEmpty(); 645 } 646 647 public boolean hasRank() { 648 return this.rank != null && !this.rank.isEmpty(); 649 } 650 651 /** 652 * @param value {@link #rank} (Specifies a preferred order in which to use a set 653 * of contacts. Contacts are ranked with lower values coming before 654 * higher values.). This is the underlying object with id, value 655 * and extensions. The accessor "getRank" gives direct access to 656 * the value 657 */ 658 public ContactPoint setRankElement(PositiveIntType value) { 659 this.rank = value; 660 return this; 661 } 662 663 /** 664 * @return Specifies a preferred order in which to use a set of contacts. 665 * Contacts are ranked with lower values coming before higher values. 666 */ 667 public int getRank() { 668 return this.rank == null || this.rank.isEmpty() ? 0 : this.rank.getValue(); 669 } 670 671 /** 672 * @param value Specifies a preferred order in which to use a set of contacts. 673 * Contacts are ranked with lower values coming before higher 674 * values. 675 */ 676 public ContactPoint setRank(int value) { 677 if (this.rank == null) 678 this.rank = new PositiveIntType(); 679 this.rank.setValue(value); 680 return this; 681 } 682 683 /** 684 * @return {@link #period} (Time period when the contact point was/is in use.) 685 */ 686 public Period getPeriod() { 687 if (this.period == null) 688 if (Configuration.errorOnAutoCreate()) 689 throw new Error("Attempt to auto-create ContactPoint.period"); 690 else if (Configuration.doAutoCreate()) 691 this.period = new Period(); // cc 692 return this.period; 693 } 694 695 public boolean hasPeriod() { 696 return this.period != null && !this.period.isEmpty(); 697 } 698 699 /** 700 * @param value {@link #period} (Time period when the contact point was/is in 701 * use.) 702 */ 703 public ContactPoint setPeriod(Period value) { 704 this.period = value; 705 return this; 706 } 707 708 protected void listChildren(List<Property> childrenList) { 709 super.listChildren(childrenList); 710 childrenList.add(new Property("system", "code", 711 "Telecommunications form for contact point - what communications system is required to make use of the contact.", 712 0, java.lang.Integer.MAX_VALUE, system)); 713 childrenList.add(new Property("value", "string", 714 "The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address).", 715 0, java.lang.Integer.MAX_VALUE, value)); 716 childrenList.add(new Property("use", "code", "Identifies the purpose for the contact point.", 0, 717 java.lang.Integer.MAX_VALUE, use)); 718 childrenList.add(new Property("rank", "positiveInt", 719 "Specifies a preferred order in which to use a set of contacts. Contacts are ranked with lower values coming before higher values.", 720 0, java.lang.Integer.MAX_VALUE, rank)); 721 childrenList.add(new Property("period", "Period", "Time period when the contact point was/is in use.", 0, 722 java.lang.Integer.MAX_VALUE, period)); 723 } 724 725 @Override 726 public void setProperty(String name, Base value) throws FHIRException { 727 if (name.equals("system")) 728 this.system = new ContactPointSystemEnumFactory().fromType(value); // Enumeration<ContactPointSystem> 729 else if (name.equals("value")) 730 this.value = castToString(value); // StringType 731 else if (name.equals("use")) 732 this.use = new ContactPointUseEnumFactory().fromType(value); // Enumeration<ContactPointUse> 733 else if (name.equals("rank")) 734 this.rank = castToPositiveInt(value); // PositiveIntType 735 else if (name.equals("period")) 736 this.period = castToPeriod(value); // Period 737 else 738 super.setProperty(name, value); 739 } 740 741 @Override 742 public Base addChild(String name) throws FHIRException { 743 if (name.equals("system")) { 744 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.system"); 745 } else if (name.equals("value")) { 746 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.value"); 747 } else if (name.equals("use")) { 748 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.use"); 749 } else if (name.equals("rank")) { 750 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.rank"); 751 } else if (name.equals("period")) { 752 this.period = new Period(); 753 return this.period; 754 } else 755 return super.addChild(name); 756 } 757 758 public String fhirType() { 759 return "ContactPoint"; 760 761 } 762 763 public ContactPoint copy() { 764 ContactPoint dst = new ContactPoint(); 765 copyValues(dst); 766 dst.system = system == null ? null : system.copy(); 767 dst.value = value == null ? null : value.copy(); 768 dst.use = use == null ? null : use.copy(); 769 dst.rank = rank == null ? null : rank.copy(); 770 dst.period = period == null ? null : period.copy(); 771 return dst; 772 } 773 774 protected ContactPoint typedCopy() { 775 return copy(); 776 } 777 778 @Override 779 public boolean equalsDeep(Base other) { 780 if (!super.equalsDeep(other)) 781 return false; 782 if (!(other instanceof ContactPoint)) 783 return false; 784 ContactPoint o = (ContactPoint) other; 785 return compareDeep(system, o.system, true) && compareDeep(value, o.value, true) && compareDeep(use, o.use, true) 786 && compareDeep(rank, o.rank, true) && compareDeep(period, o.period, true); 787 } 788 789 @Override 790 public boolean equalsShallow(Base other) { 791 if (!super.equalsShallow(other)) 792 return false; 793 if (!(other instanceof ContactPoint)) 794 return false; 795 ContactPoint o = (ContactPoint) other; 796 return compareValues(system, o.system, true) && compareValues(value, o.value, true) 797 && compareValues(use, o.use, true) && compareValues(rank, o.rank, true); 798 } 799 800 public boolean isEmpty() { 801 return super.isEmpty() && (system == null || system.isEmpty()) && (value == null || value.isEmpty()) 802 && (use == null || use.isEmpty()) && (rank == null || rank.isEmpty()) && (period == null || period.isEmpty()); 803 } 804 805}