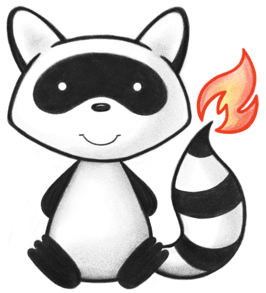
001package org.hl7.fhir.dstu2.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.utilities.Utilities; 047 048/** 049 * A formal agreement between parties regarding the conduct of business, 050 * exchange of information or other matters. 051 */ 052@ResourceDef(name = "Contract", profile = "http://hl7.org/fhir/Profile/Contract") 053public class Contract extends DomainResource { 054 055 @Block() 056 public static class ActorComponent extends BackboneElement implements IBaseBackboneElement { 057 /** 058 * Who or what actors are assigned roles in this Contract. 059 */ 060 @Child(name = "entity", type = { Contract.class, Device.class, Group.class, Location.class, Organization.class, 061 Patient.class, Practitioner.class, RelatedPerson.class, 062 Substance.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 063 @Description(shortDefinition = "Contract Actor Type", formalDefinition = "Who or what actors are assigned roles in this Contract.") 064 protected Reference entity; 065 066 /** 067 * The actual object that is the target of the reference (Who or what actors are 068 * assigned roles in this Contract.) 069 */ 070 protected Resource entityTarget; 071 072 /** 073 * Role type of actors assigned roles in this Contract. 074 */ 075 @Child(name = "role", type = { 076 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 077 @Description(shortDefinition = "Contract Actor Role", formalDefinition = "Role type of actors assigned roles in this Contract.") 078 protected List<CodeableConcept> role; 079 080 private static final long serialVersionUID = 1371245689L; 081 082 /* 083 * Constructor 084 */ 085 public ActorComponent() { 086 super(); 087 } 088 089 /* 090 * Constructor 091 */ 092 public ActorComponent(Reference entity) { 093 super(); 094 this.entity = entity; 095 } 096 097 /** 098 * @return {@link #entity} (Who or what actors are assigned roles in this 099 * Contract.) 100 */ 101 public Reference getEntity() { 102 if (this.entity == null) 103 if (Configuration.errorOnAutoCreate()) 104 throw new Error("Attempt to auto-create ActorComponent.entity"); 105 else if (Configuration.doAutoCreate()) 106 this.entity = new Reference(); // cc 107 return this.entity; 108 } 109 110 public boolean hasEntity() { 111 return this.entity != null && !this.entity.isEmpty(); 112 } 113 114 /** 115 * @param value {@link #entity} (Who or what actors are assigned roles in this 116 * Contract.) 117 */ 118 public ActorComponent setEntity(Reference value) { 119 this.entity = value; 120 return this; 121 } 122 123 /** 124 * @return {@link #entity} The actual object that is the target of the 125 * reference. The reference library doesn't populate this, but you can 126 * use it to hold the resource if you resolve it. (Who or what actors 127 * are assigned roles in this Contract.) 128 */ 129 public Resource getEntityTarget() { 130 return this.entityTarget; 131 } 132 133 /** 134 * @param value {@link #entity} The actual object that is the target of the 135 * reference. The reference library doesn't use these, but you can 136 * use it to hold the resource if you resolve it. (Who or what 137 * actors are assigned roles in this Contract.) 138 */ 139 public ActorComponent setEntityTarget(Resource value) { 140 this.entityTarget = value; 141 return this; 142 } 143 144 /** 145 * @return {@link #role} (Role type of actors assigned roles in this Contract.) 146 */ 147 public List<CodeableConcept> getRole() { 148 if (this.role == null) 149 this.role = new ArrayList<CodeableConcept>(); 150 return this.role; 151 } 152 153 public boolean hasRole() { 154 if (this.role == null) 155 return false; 156 for (CodeableConcept item : this.role) 157 if (!item.isEmpty()) 158 return true; 159 return false; 160 } 161 162 /** 163 * @return {@link #role} (Role type of actors assigned roles in this Contract.) 164 */ 165 // syntactic sugar 166 public CodeableConcept addRole() { // 3 167 CodeableConcept t = new CodeableConcept(); 168 if (this.role == null) 169 this.role = new ArrayList<CodeableConcept>(); 170 this.role.add(t); 171 return t; 172 } 173 174 // syntactic sugar 175 public ActorComponent addRole(CodeableConcept t) { // 3 176 if (t == null) 177 return this; 178 if (this.role == null) 179 this.role = new ArrayList<CodeableConcept>(); 180 this.role.add(t); 181 return this; 182 } 183 184 protected void listChildren(List<Property> childrenList) { 185 super.listChildren(childrenList); 186 childrenList.add(new Property("entity", 187 "Reference(Contract|Device|Group|Location|Organization|Patient|Practitioner|RelatedPerson|Substance)", 188 "Who or what actors are assigned roles in this Contract.", 0, java.lang.Integer.MAX_VALUE, entity)); 189 childrenList.add(new Property("role", "CodeableConcept", "Role type of actors assigned roles in this Contract.", 190 0, java.lang.Integer.MAX_VALUE, role)); 191 } 192 193 @Override 194 public void setProperty(String name, Base value) throws FHIRException { 195 if (name.equals("entity")) 196 this.entity = castToReference(value); // Reference 197 else if (name.equals("role")) 198 this.getRole().add(castToCodeableConcept(value)); 199 else 200 super.setProperty(name, value); 201 } 202 203 @Override 204 public Base addChild(String name) throws FHIRException { 205 if (name.equals("entity")) { 206 this.entity = new Reference(); 207 return this.entity; 208 } else if (name.equals("role")) { 209 return addRole(); 210 } else 211 return super.addChild(name); 212 } 213 214 public ActorComponent copy() { 215 ActorComponent dst = new ActorComponent(); 216 copyValues(dst); 217 dst.entity = entity == null ? null : entity.copy(); 218 if (role != null) { 219 dst.role = new ArrayList<CodeableConcept>(); 220 for (CodeableConcept i : role) 221 dst.role.add(i.copy()); 222 } 223 ; 224 return dst; 225 } 226 227 @Override 228 public boolean equalsDeep(Base other) { 229 if (!super.equalsDeep(other)) 230 return false; 231 if (!(other instanceof ActorComponent)) 232 return false; 233 ActorComponent o = (ActorComponent) other; 234 return compareDeep(entity, o.entity, true) && compareDeep(role, o.role, true); 235 } 236 237 @Override 238 public boolean equalsShallow(Base other) { 239 if (!super.equalsShallow(other)) 240 return false; 241 if (!(other instanceof ActorComponent)) 242 return false; 243 ActorComponent o = (ActorComponent) other; 244 return true; 245 } 246 247 public boolean isEmpty() { 248 return super.isEmpty() && (entity == null || entity.isEmpty()) && (role == null || role.isEmpty()); 249 } 250 251 public String fhirType() { 252 return "Contract.actor"; 253 254 } 255 256 } 257 258 @Block() 259 public static class ValuedItemComponent extends BackboneElement implements IBaseBackboneElement { 260 /** 261 * Specific type of Contract Valued Item that may be priced. 262 */ 263 @Child(name = "entity", type = { 264 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 265 @Description(shortDefinition = "Contract Valued Item Type", formalDefinition = "Specific type of Contract Valued Item that may be priced.") 266 protected Type entity; 267 268 /** 269 * Identifies a Contract Valued Item instance. 270 */ 271 @Child(name = "identifier", type = { 272 Identifier.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 273 @Description(shortDefinition = "Contract Valued Item Identifier", formalDefinition = "Identifies a Contract Valued Item instance.") 274 protected Identifier identifier; 275 276 /** 277 * Indicates the time during which this Contract ValuedItem information is 278 * effective. 279 */ 280 @Child(name = "effectiveTime", type = { 281 DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 282 @Description(shortDefinition = "Contract Valued Item Effective Tiem", formalDefinition = "Indicates the time during which this Contract ValuedItem information is effective.") 283 protected DateTimeType effectiveTime; 284 285 /** 286 * Specifies the units by which the Contract Valued Item is measured or counted, 287 * and quantifies the countable or measurable Contract Valued Item instances. 288 */ 289 @Child(name = "quantity", type = { 290 SimpleQuantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 291 @Description(shortDefinition = "Count of Contract Valued Items", formalDefinition = "Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.") 292 protected SimpleQuantity quantity; 293 294 /** 295 * A Contract Valued Item unit valuation measure. 296 */ 297 @Child(name = "unitPrice", type = { Money.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 298 @Description(shortDefinition = "Contract Valued Item fee, charge, or cost", formalDefinition = "A Contract Valued Item unit valuation measure.") 299 protected Money unitPrice; 300 301 /** 302 * A real number that represents a multiplier used in determining the overall 303 * value of the Contract Valued Item delivered. The concept of a Factor allows 304 * for a discount or surcharge multiplier to be applied to a monetary amount. 305 */ 306 @Child(name = "factor", type = { 307 DecimalType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 308 @Description(shortDefinition = "Contract Valued Item Price Scaling Factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 309 protected DecimalType factor; 310 311 /** 312 * An amount that expresses the weighting (based on difficulty, cost and/or 313 * resource intensiveness) associated with the Contract Valued Item delivered. 314 * The concept of Points allows for assignment of point values for a Contract 315 * Valued Item, such that a monetary amount can be assigned to each point. 316 */ 317 @Child(name = "points", type = { 318 DecimalType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 319 @Description(shortDefinition = "Contract Valued Item Difficulty Scaling Factor", formalDefinition = "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.") 320 protected DecimalType points; 321 322 /** 323 * Expresses the product of the Contract Valued Item unitQuantity and the 324 * unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per 325 * Point) * factor Number * points = net Amount. Quantity, factor and points are 326 * assumed to be 1 if not supplied. 327 */ 328 @Child(name = "net", type = { Money.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 329 @Description(shortDefinition = "Total Contract Valued Item Value", formalDefinition = "Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.") 330 protected Money net; 331 332 private static final long serialVersionUID = 1782449516L; 333 334 /* 335 * Constructor 336 */ 337 public ValuedItemComponent() { 338 super(); 339 } 340 341 /** 342 * @return {@link #entity} (Specific type of Contract Valued Item that may be 343 * priced.) 344 */ 345 public Type getEntity() { 346 return this.entity; 347 } 348 349 /** 350 * @return {@link #entity} (Specific type of Contract Valued Item that may be 351 * priced.) 352 */ 353 public CodeableConcept getEntityCodeableConcept() throws FHIRException { 354 if (!(this.entity instanceof CodeableConcept)) 355 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 356 + this.entity.getClass().getName() + " was encountered"); 357 return (CodeableConcept) this.entity; 358 } 359 360 public boolean hasEntityCodeableConcept() { 361 return this.entity instanceof CodeableConcept; 362 } 363 364 /** 365 * @return {@link #entity} (Specific type of Contract Valued Item that may be 366 * priced.) 367 */ 368 public Reference getEntityReference() throws FHIRException { 369 if (!(this.entity instanceof Reference)) 370 throw new FHIRException("Type mismatch: the type Reference was expected, but " 371 + this.entity.getClass().getName() + " was encountered"); 372 return (Reference) this.entity; 373 } 374 375 public boolean hasEntityReference() { 376 return this.entity instanceof Reference; 377 } 378 379 public boolean hasEntity() { 380 return this.entity != null && !this.entity.isEmpty(); 381 } 382 383 /** 384 * @param value {@link #entity} (Specific type of Contract Valued Item that may 385 * be priced.) 386 */ 387 public ValuedItemComponent setEntity(Type value) { 388 this.entity = value; 389 return this; 390 } 391 392 /** 393 * @return {@link #identifier} (Identifies a Contract Valued Item instance.) 394 */ 395 public Identifier getIdentifier() { 396 if (this.identifier == null) 397 if (Configuration.errorOnAutoCreate()) 398 throw new Error("Attempt to auto-create ValuedItemComponent.identifier"); 399 else if (Configuration.doAutoCreate()) 400 this.identifier = new Identifier(); // cc 401 return this.identifier; 402 } 403 404 public boolean hasIdentifier() { 405 return this.identifier != null && !this.identifier.isEmpty(); 406 } 407 408 /** 409 * @param value {@link #identifier} (Identifies a Contract Valued Item 410 * instance.) 411 */ 412 public ValuedItemComponent setIdentifier(Identifier value) { 413 this.identifier = value; 414 return this; 415 } 416 417 /** 418 * @return {@link #effectiveTime} (Indicates the time during which this Contract 419 * ValuedItem information is effective.). This is the underlying object 420 * with id, value and extensions. The accessor "getEffectiveTime" gives 421 * direct access to the value 422 */ 423 public DateTimeType getEffectiveTimeElement() { 424 if (this.effectiveTime == null) 425 if (Configuration.errorOnAutoCreate()) 426 throw new Error("Attempt to auto-create ValuedItemComponent.effectiveTime"); 427 else if (Configuration.doAutoCreate()) 428 this.effectiveTime = new DateTimeType(); // bb 429 return this.effectiveTime; 430 } 431 432 public boolean hasEffectiveTimeElement() { 433 return this.effectiveTime != null && !this.effectiveTime.isEmpty(); 434 } 435 436 public boolean hasEffectiveTime() { 437 return this.effectiveTime != null && !this.effectiveTime.isEmpty(); 438 } 439 440 /** 441 * @param value {@link #effectiveTime} (Indicates the time during which this 442 * Contract ValuedItem information is effective.). This is the 443 * underlying object with id, value and extensions. The accessor 444 * "getEffectiveTime" gives direct access to the value 445 */ 446 public ValuedItemComponent setEffectiveTimeElement(DateTimeType value) { 447 this.effectiveTime = value; 448 return this; 449 } 450 451 /** 452 * @return Indicates the time during which this Contract ValuedItem information 453 * is effective. 454 */ 455 public Date getEffectiveTime() { 456 return this.effectiveTime == null ? null : this.effectiveTime.getValue(); 457 } 458 459 /** 460 * @param value Indicates the time during which this Contract ValuedItem 461 * information is effective. 462 */ 463 public ValuedItemComponent setEffectiveTime(Date value) { 464 if (value == null) 465 this.effectiveTime = null; 466 else { 467 if (this.effectiveTime == null) 468 this.effectiveTime = new DateTimeType(); 469 this.effectiveTime.setValue(value); 470 } 471 return this; 472 } 473 474 /** 475 * @return {@link #quantity} (Specifies the units by which the Contract Valued 476 * Item is measured or counted, and quantifies the countable or 477 * measurable Contract Valued Item instances.) 478 */ 479 public SimpleQuantity getQuantity() { 480 if (this.quantity == null) 481 if (Configuration.errorOnAutoCreate()) 482 throw new Error("Attempt to auto-create ValuedItemComponent.quantity"); 483 else if (Configuration.doAutoCreate()) 484 this.quantity = new SimpleQuantity(); // cc 485 return this.quantity; 486 } 487 488 public boolean hasQuantity() { 489 return this.quantity != null && !this.quantity.isEmpty(); 490 } 491 492 /** 493 * @param value {@link #quantity} (Specifies the units by which the Contract 494 * Valued Item is measured or counted, and quantifies the countable 495 * or measurable Contract Valued Item instances.) 496 */ 497 public ValuedItemComponent setQuantity(SimpleQuantity value) { 498 this.quantity = value; 499 return this; 500 } 501 502 /** 503 * @return {@link #unitPrice} (A Contract Valued Item unit valuation measure.) 504 */ 505 public Money getUnitPrice() { 506 if (this.unitPrice == null) 507 if (Configuration.errorOnAutoCreate()) 508 throw new Error("Attempt to auto-create ValuedItemComponent.unitPrice"); 509 else if (Configuration.doAutoCreate()) 510 this.unitPrice = new Money(); // cc 511 return this.unitPrice; 512 } 513 514 public boolean hasUnitPrice() { 515 return this.unitPrice != null && !this.unitPrice.isEmpty(); 516 } 517 518 /** 519 * @param value {@link #unitPrice} (A Contract Valued Item unit valuation 520 * measure.) 521 */ 522 public ValuedItemComponent setUnitPrice(Money value) { 523 this.unitPrice = value; 524 return this; 525 } 526 527 /** 528 * @return {@link #factor} (A real number that represents a multiplier used in 529 * determining the overall value of the Contract Valued Item delivered. 530 * The concept of a Factor allows for a discount or surcharge multiplier 531 * to be applied to a monetary amount.). This is the underlying object 532 * with id, value and extensions. The accessor "getFactor" gives direct 533 * access to the value 534 */ 535 public DecimalType getFactorElement() { 536 if (this.factor == null) 537 if (Configuration.errorOnAutoCreate()) 538 throw new Error("Attempt to auto-create ValuedItemComponent.factor"); 539 else if (Configuration.doAutoCreate()) 540 this.factor = new DecimalType(); // bb 541 return this.factor; 542 } 543 544 public boolean hasFactorElement() { 545 return this.factor != null && !this.factor.isEmpty(); 546 } 547 548 public boolean hasFactor() { 549 return this.factor != null && !this.factor.isEmpty(); 550 } 551 552 /** 553 * @param value {@link #factor} (A real number that represents a multiplier used 554 * in determining the overall value of the Contract Valued Item 555 * delivered. The concept of a Factor allows for a discount or 556 * surcharge multiplier to be applied to a monetary amount.). This 557 * is the underlying object with id, value and extensions. The 558 * accessor "getFactor" gives direct access to the value 559 */ 560 public ValuedItemComponent setFactorElement(DecimalType value) { 561 this.factor = value; 562 return this; 563 } 564 565 /** 566 * @return A real number that represents a multiplier used in determining the 567 * overall value of the Contract Valued Item delivered. The concept of a 568 * Factor allows for a discount or surcharge multiplier to be applied to 569 * a monetary amount. 570 */ 571 public BigDecimal getFactor() { 572 return this.factor == null ? null : this.factor.getValue(); 573 } 574 575 /** 576 * @param value A real number that represents a multiplier used in determining 577 * the overall value of the Contract Valued Item delivered. The 578 * concept of a Factor allows for a discount or surcharge 579 * multiplier to be applied to a monetary amount. 580 */ 581 public ValuedItemComponent setFactor(BigDecimal value) { 582 if (value == null) 583 this.factor = null; 584 else { 585 if (this.factor == null) 586 this.factor = new DecimalType(); 587 this.factor.setValue(value); 588 } 589 return this; 590 } 591 592 /** 593 * @return {@link #points} (An amount that expresses the weighting (based on 594 * difficulty, cost and/or resource intensiveness) associated with the 595 * Contract Valued Item delivered. The concept of Points allows for 596 * assignment of point values for a Contract Valued Item, such that a 597 * monetary amount can be assigned to each point.). This is the 598 * underlying object with id, value and extensions. The accessor 599 * "getPoints" gives direct access to the value 600 */ 601 public DecimalType getPointsElement() { 602 if (this.points == null) 603 if (Configuration.errorOnAutoCreate()) 604 throw new Error("Attempt to auto-create ValuedItemComponent.points"); 605 else if (Configuration.doAutoCreate()) 606 this.points = new DecimalType(); // bb 607 return this.points; 608 } 609 610 public boolean hasPointsElement() { 611 return this.points != null && !this.points.isEmpty(); 612 } 613 614 public boolean hasPoints() { 615 return this.points != null && !this.points.isEmpty(); 616 } 617 618 /** 619 * @param value {@link #points} (An amount that expresses the weighting (based 620 * on difficulty, cost and/or resource intensiveness) associated 621 * with the Contract Valued Item delivered. The concept of Points 622 * allows for assignment of point values for a Contract Valued 623 * Item, such that a monetary amount can be assigned to each 624 * point.). This is the underlying object with id, value and 625 * extensions. The accessor "getPoints" gives direct access to the 626 * value 627 */ 628 public ValuedItemComponent setPointsElement(DecimalType value) { 629 this.points = value; 630 return this; 631 } 632 633 /** 634 * @return An amount that expresses the weighting (based on difficulty, cost 635 * and/or resource intensiveness) associated with the Contract Valued 636 * Item delivered. The concept of Points allows for assignment of point 637 * values for a Contract Valued Item, such that a monetary amount can be 638 * assigned to each point. 639 */ 640 public BigDecimal getPoints() { 641 return this.points == null ? null : this.points.getValue(); 642 } 643 644 /** 645 * @param value An amount that expresses the weighting (based on difficulty, 646 * cost and/or resource intensiveness) associated with the Contract 647 * Valued Item delivered. The concept of Points allows for 648 * assignment of point values for a Contract Valued Item, such that 649 * a monetary amount can be assigned to each point. 650 */ 651 public ValuedItemComponent setPoints(BigDecimal value) { 652 if (value == null) 653 this.points = null; 654 else { 655 if (this.points == null) 656 this.points = new DecimalType(); 657 this.points.setValue(value); 658 } 659 return this; 660 } 661 662 /** 663 * @return {@link #net} (Expresses the product of the Contract Valued Item 664 * unitQuantity and the unitPriceAmt. For example, the formula: unit 665 * Quantity * unit Price (Cost per Point) * factor Number * points = net 666 * Amount. Quantity, factor and points are assumed to be 1 if not 667 * supplied.) 668 */ 669 public Money getNet() { 670 if (this.net == null) 671 if (Configuration.errorOnAutoCreate()) 672 throw new Error("Attempt to auto-create ValuedItemComponent.net"); 673 else if (Configuration.doAutoCreate()) 674 this.net = new Money(); // cc 675 return this.net; 676 } 677 678 public boolean hasNet() { 679 return this.net != null && !this.net.isEmpty(); 680 } 681 682 /** 683 * @param value {@link #net} (Expresses the product of the Contract Valued Item 684 * unitQuantity and the unitPriceAmt. For example, the formula: 685 * unit Quantity * unit Price (Cost per Point) * factor Number * 686 * points = net Amount. Quantity, factor and points are assumed to 687 * be 1 if not supplied.) 688 */ 689 public ValuedItemComponent setNet(Money value) { 690 this.net = value; 691 return this; 692 } 693 694 protected void listChildren(List<Property> childrenList) { 695 super.listChildren(childrenList); 696 childrenList.add(new Property("entity[x]", "CodeableConcept|Reference(Any)", 697 "Specific type of Contract Valued Item that may be priced.", 0, java.lang.Integer.MAX_VALUE, entity)); 698 childrenList.add(new Property("identifier", "Identifier", "Identifies a Contract Valued Item instance.", 0, 699 java.lang.Integer.MAX_VALUE, identifier)); 700 childrenList.add(new Property("effectiveTime", "dateTime", 701 "Indicates the time during which this Contract ValuedItem information is effective.", 0, 702 java.lang.Integer.MAX_VALUE, effectiveTime)); 703 childrenList.add(new Property("quantity", "SimpleQuantity", 704 "Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.", 705 0, java.lang.Integer.MAX_VALUE, quantity)); 706 childrenList.add(new Property("unitPrice", "Money", "A Contract Valued Item unit valuation measure.", 0, 707 java.lang.Integer.MAX_VALUE, unitPrice)); 708 childrenList.add(new Property("factor", "decimal", 709 "A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 710 0, java.lang.Integer.MAX_VALUE, factor)); 711 childrenList.add(new Property("points", "decimal", 712 "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.", 713 0, java.lang.Integer.MAX_VALUE, points)); 714 childrenList.add(new Property("net", "Money", 715 "Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 716 0, java.lang.Integer.MAX_VALUE, net)); 717 } 718 719 @Override 720 public void setProperty(String name, Base value) throws FHIRException { 721 if (name.equals("entity[x]")) 722 this.entity = (Type) value; // Type 723 else if (name.equals("identifier")) 724 this.identifier = castToIdentifier(value); // Identifier 725 else if (name.equals("effectiveTime")) 726 this.effectiveTime = castToDateTime(value); // DateTimeType 727 else if (name.equals("quantity")) 728 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 729 else if (name.equals("unitPrice")) 730 this.unitPrice = castToMoney(value); // Money 731 else if (name.equals("factor")) 732 this.factor = castToDecimal(value); // DecimalType 733 else if (name.equals("points")) 734 this.points = castToDecimal(value); // DecimalType 735 else if (name.equals("net")) 736 this.net = castToMoney(value); // Money 737 else 738 super.setProperty(name, value); 739 } 740 741 @Override 742 public Base addChild(String name) throws FHIRException { 743 if (name.equals("entityCodeableConcept")) { 744 this.entity = new CodeableConcept(); 745 return this.entity; 746 } else if (name.equals("entityReference")) { 747 this.entity = new Reference(); 748 return this.entity; 749 } else if (name.equals("identifier")) { 750 this.identifier = new Identifier(); 751 return this.identifier; 752 } else if (name.equals("effectiveTime")) { 753 throw new FHIRException("Cannot call addChild on a singleton property Contract.effectiveTime"); 754 } else if (name.equals("quantity")) { 755 this.quantity = new SimpleQuantity(); 756 return this.quantity; 757 } else if (name.equals("unitPrice")) { 758 this.unitPrice = new Money(); 759 return this.unitPrice; 760 } else if (name.equals("factor")) { 761 throw new FHIRException("Cannot call addChild on a singleton property Contract.factor"); 762 } else if (name.equals("points")) { 763 throw new FHIRException("Cannot call addChild on a singleton property Contract.points"); 764 } else if (name.equals("net")) { 765 this.net = new Money(); 766 return this.net; 767 } else 768 return super.addChild(name); 769 } 770 771 public ValuedItemComponent copy() { 772 ValuedItemComponent dst = new ValuedItemComponent(); 773 copyValues(dst); 774 dst.entity = entity == null ? null : entity.copy(); 775 dst.identifier = identifier == null ? null : identifier.copy(); 776 dst.effectiveTime = effectiveTime == null ? null : effectiveTime.copy(); 777 dst.quantity = quantity == null ? null : quantity.copy(); 778 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 779 dst.factor = factor == null ? null : factor.copy(); 780 dst.points = points == null ? null : points.copy(); 781 dst.net = net == null ? null : net.copy(); 782 return dst; 783 } 784 785 @Override 786 public boolean equalsDeep(Base other) { 787 if (!super.equalsDeep(other)) 788 return false; 789 if (!(other instanceof ValuedItemComponent)) 790 return false; 791 ValuedItemComponent o = (ValuedItemComponent) other; 792 return compareDeep(entity, o.entity, true) && compareDeep(identifier, o.identifier, true) 793 && compareDeep(effectiveTime, o.effectiveTime, true) && compareDeep(quantity, o.quantity, true) 794 && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 795 && compareDeep(points, o.points, true) && compareDeep(net, o.net, true); 796 } 797 798 @Override 799 public boolean equalsShallow(Base other) { 800 if (!super.equalsShallow(other)) 801 return false; 802 if (!(other instanceof ValuedItemComponent)) 803 return false; 804 ValuedItemComponent o = (ValuedItemComponent) other; 805 return compareValues(effectiveTime, o.effectiveTime, true) && compareValues(factor, o.factor, true) 806 && compareValues(points, o.points, true); 807 } 808 809 public boolean isEmpty() { 810 return super.isEmpty() && (entity == null || entity.isEmpty()) && (identifier == null || identifier.isEmpty()) 811 && (effectiveTime == null || effectiveTime.isEmpty()) && (quantity == null || quantity.isEmpty()) 812 && (unitPrice == null || unitPrice.isEmpty()) && (factor == null || factor.isEmpty()) 813 && (points == null || points.isEmpty()) && (net == null || net.isEmpty()); 814 } 815 816 public String fhirType() { 817 return "Contract.valuedItem"; 818 819 } 820 821 } 822 823 @Block() 824 public static class SignatoryComponent extends BackboneElement implements IBaseBackboneElement { 825 /** 826 * Role of this Contract signer, e.g. notary, grantee. 827 */ 828 @Child(name = "type", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 829 @Description(shortDefinition = "Contract Signer Type", formalDefinition = "Role of this Contract signer, e.g. notary, grantee.") 830 protected Coding type; 831 832 /** 833 * Party which is a signator to this Contract. 834 */ 835 @Child(name = "party", type = { Organization.class, Patient.class, Practitioner.class, 836 RelatedPerson.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 837 @Description(shortDefinition = "Contract Signatory Party", formalDefinition = "Party which is a signator to this Contract.") 838 protected Reference party; 839 840 /** 841 * The actual object that is the target of the reference (Party which is a 842 * signator to this Contract.) 843 */ 844 protected Resource partyTarget; 845 846 /** 847 * Legally binding Contract DSIG signature contents in Base64. 848 */ 849 @Child(name = "signature", type = { 850 StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 851 @Description(shortDefinition = "Contract Documentation Signature", formalDefinition = "Legally binding Contract DSIG signature contents in Base64.") 852 protected StringType signature; 853 854 private static final long serialVersionUID = -1870392043L; 855 856 /* 857 * Constructor 858 */ 859 public SignatoryComponent() { 860 super(); 861 } 862 863 /* 864 * Constructor 865 */ 866 public SignatoryComponent(Coding type, Reference party, StringType signature) { 867 super(); 868 this.type = type; 869 this.party = party; 870 this.signature = signature; 871 } 872 873 /** 874 * @return {@link #type} (Role of this Contract signer, e.g. notary, grantee.) 875 */ 876 public Coding getType() { 877 if (this.type == null) 878 if (Configuration.errorOnAutoCreate()) 879 throw new Error("Attempt to auto-create SignatoryComponent.type"); 880 else if (Configuration.doAutoCreate()) 881 this.type = new Coding(); // cc 882 return this.type; 883 } 884 885 public boolean hasType() { 886 return this.type != null && !this.type.isEmpty(); 887 } 888 889 /** 890 * @param value {@link #type} (Role of this Contract signer, e.g. notary, 891 * grantee.) 892 */ 893 public SignatoryComponent setType(Coding value) { 894 this.type = value; 895 return this; 896 } 897 898 /** 899 * @return {@link #party} (Party which is a signator to this Contract.) 900 */ 901 public Reference getParty() { 902 if (this.party == null) 903 if (Configuration.errorOnAutoCreate()) 904 throw new Error("Attempt to auto-create SignatoryComponent.party"); 905 else if (Configuration.doAutoCreate()) 906 this.party = new Reference(); // cc 907 return this.party; 908 } 909 910 public boolean hasParty() { 911 return this.party != null && !this.party.isEmpty(); 912 } 913 914 /** 915 * @param value {@link #party} (Party which is a signator to this Contract.) 916 */ 917 public SignatoryComponent setParty(Reference value) { 918 this.party = value; 919 return this; 920 } 921 922 /** 923 * @return {@link #party} The actual object that is the target of the reference. 924 * The reference library doesn't populate this, but you can use it to 925 * hold the resource if you resolve it. (Party which is a signator to 926 * this Contract.) 927 */ 928 public Resource getPartyTarget() { 929 return this.partyTarget; 930 } 931 932 /** 933 * @param value {@link #party} The actual object that is the target of the 934 * reference. The reference library doesn't use these, but you can 935 * use it to hold the resource if you resolve it. (Party which is a 936 * signator to this Contract.) 937 */ 938 public SignatoryComponent setPartyTarget(Resource value) { 939 this.partyTarget = value; 940 return this; 941 } 942 943 /** 944 * @return {@link #signature} (Legally binding Contract DSIG signature contents 945 * in Base64.). This is the underlying object with id, value and 946 * extensions. The accessor "getSignature" gives direct access to the 947 * value 948 */ 949 public StringType getSignatureElement() { 950 if (this.signature == null) 951 if (Configuration.errorOnAutoCreate()) 952 throw new Error("Attempt to auto-create SignatoryComponent.signature"); 953 else if (Configuration.doAutoCreate()) 954 this.signature = new StringType(); // bb 955 return this.signature; 956 } 957 958 public boolean hasSignatureElement() { 959 return this.signature != null && !this.signature.isEmpty(); 960 } 961 962 public boolean hasSignature() { 963 return this.signature != null && !this.signature.isEmpty(); 964 } 965 966 /** 967 * @param value {@link #signature} (Legally binding Contract DSIG signature 968 * contents in Base64.). This is the underlying object with id, 969 * value and extensions. The accessor "getSignature" gives direct 970 * access to the value 971 */ 972 public SignatoryComponent setSignatureElement(StringType value) { 973 this.signature = value; 974 return this; 975 } 976 977 /** 978 * @return Legally binding Contract DSIG signature contents in Base64. 979 */ 980 public String getSignature() { 981 return this.signature == null ? null : this.signature.getValue(); 982 } 983 984 /** 985 * @param value Legally binding Contract DSIG signature contents in Base64. 986 */ 987 public SignatoryComponent setSignature(String value) { 988 if (this.signature == null) 989 this.signature = new StringType(); 990 this.signature.setValue(value); 991 return this; 992 } 993 994 protected void listChildren(List<Property> childrenList) { 995 super.listChildren(childrenList); 996 childrenList.add(new Property("type", "Coding", "Role of this Contract signer, e.g. notary, grantee.", 0, 997 java.lang.Integer.MAX_VALUE, type)); 998 childrenList.add(new Property("party", "Reference(Organization|Patient|Practitioner|RelatedPerson)", 999 "Party which is a signator to this Contract.", 0, java.lang.Integer.MAX_VALUE, party)); 1000 childrenList.add(new Property("signature", "string", 1001 "Legally binding Contract DSIG signature contents in Base64.", 0, java.lang.Integer.MAX_VALUE, signature)); 1002 } 1003 1004 @Override 1005 public void setProperty(String name, Base value) throws FHIRException { 1006 if (name.equals("type")) 1007 this.type = castToCoding(value); // Coding 1008 else if (name.equals("party")) 1009 this.party = castToReference(value); // Reference 1010 else if (name.equals("signature")) 1011 this.signature = castToString(value); // StringType 1012 else 1013 super.setProperty(name, value); 1014 } 1015 1016 @Override 1017 public Base addChild(String name) throws FHIRException { 1018 if (name.equals("type")) { 1019 this.type = new Coding(); 1020 return this.type; 1021 } else if (name.equals("party")) { 1022 this.party = new Reference(); 1023 return this.party; 1024 } else if (name.equals("signature")) { 1025 throw new FHIRException("Cannot call addChild on a singleton property Contract.signature"); 1026 } else 1027 return super.addChild(name); 1028 } 1029 1030 public SignatoryComponent copy() { 1031 SignatoryComponent dst = new SignatoryComponent(); 1032 copyValues(dst); 1033 dst.type = type == null ? null : type.copy(); 1034 dst.party = party == null ? null : party.copy(); 1035 dst.signature = signature == null ? null : signature.copy(); 1036 return dst; 1037 } 1038 1039 @Override 1040 public boolean equalsDeep(Base other) { 1041 if (!super.equalsDeep(other)) 1042 return false; 1043 if (!(other instanceof SignatoryComponent)) 1044 return false; 1045 SignatoryComponent o = (SignatoryComponent) other; 1046 return compareDeep(type, o.type, true) && compareDeep(party, o.party, true) 1047 && compareDeep(signature, o.signature, true); 1048 } 1049 1050 @Override 1051 public boolean equalsShallow(Base other) { 1052 if (!super.equalsShallow(other)) 1053 return false; 1054 if (!(other instanceof SignatoryComponent)) 1055 return false; 1056 SignatoryComponent o = (SignatoryComponent) other; 1057 return compareValues(signature, o.signature, true); 1058 } 1059 1060 public boolean isEmpty() { 1061 return super.isEmpty() && (type == null || type.isEmpty()) && (party == null || party.isEmpty()) 1062 && (signature == null || signature.isEmpty()); 1063 } 1064 1065 public String fhirType() { 1066 return "Contract.signer"; 1067 1068 } 1069 1070 } 1071 1072 @Block() 1073 public static class TermComponent extends BackboneElement implements IBaseBackboneElement { 1074 /** 1075 * Unique identifier for this particular Contract Provision. 1076 */ 1077 @Child(name = "identifier", type = { 1078 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1079 @Description(shortDefinition = "Contract Term identifier", formalDefinition = "Unique identifier for this particular Contract Provision.") 1080 protected Identifier identifier; 1081 1082 /** 1083 * When this Contract Provision was issued. 1084 */ 1085 @Child(name = "issued", type = { 1086 DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1087 @Description(shortDefinition = "Contract Term Issue Date Time", formalDefinition = "When this Contract Provision was issued.") 1088 protected DateTimeType issued; 1089 1090 /** 1091 * Relevant time or time-period when this Contract Provision is applicable. 1092 */ 1093 @Child(name = "applies", type = { Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1094 @Description(shortDefinition = "Contract Term Effective Time", formalDefinition = "Relevant time or time-period when this Contract Provision is applicable.") 1095 protected Period applies; 1096 1097 /** 1098 * Type of Contract Provision such as specific requirements, purposes for 1099 * actions, obligations, prohibitions, e.g. life time maximum benefit. 1100 */ 1101 @Child(name = "type", type = { 1102 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1103 @Description(shortDefinition = "Contract Term Type", formalDefinition = "Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.") 1104 protected CodeableConcept type; 1105 1106 /** 1107 * Subtype of this Contract Provision, e.g. life time maximum payment for a 1108 * contract term for specific valued item, e.g. disability payment. 1109 */ 1110 @Child(name = "subType", type = { 1111 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1112 @Description(shortDefinition = "Contract Term Subtype", formalDefinition = "Subtype of this Contract Provision, e.g. life time maximum payment for a contract term for specific valued item, e.g. disability payment.") 1113 protected CodeableConcept subType; 1114 1115 /** 1116 * Who or what this Contract Provision is about. 1117 */ 1118 @Child(name = "subject", type = {}, order = 6, min = 0, max = 1, modifier = false, summary = false) 1119 @Description(shortDefinition = "Subject of this Contract Term", formalDefinition = "Who or what this Contract Provision is about.") 1120 protected Reference subject; 1121 1122 /** 1123 * The actual object that is the target of the reference (Who or what this 1124 * Contract Provision is about.) 1125 */ 1126 protected Resource subjectTarget; 1127 1128 /** 1129 * Action stipulated by this Contract Provision. 1130 */ 1131 @Child(name = "action", type = { 1132 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1133 @Description(shortDefinition = "Contract Term Action", formalDefinition = "Action stipulated by this Contract Provision.") 1134 protected List<CodeableConcept> action; 1135 1136 /** 1137 * Reason or purpose for the action stipulated by this Contract Provision. 1138 */ 1139 @Child(name = "actionReason", type = { 1140 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1141 @Description(shortDefinition = "Contract Term Action Reason", formalDefinition = "Reason or purpose for the action stipulated by this Contract Provision.") 1142 protected List<CodeableConcept> actionReason; 1143 1144 /** 1145 * List of actors participating in this Contract Provision. 1146 */ 1147 @Child(name = "actor", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1148 @Description(shortDefinition = "Contract Term Actor List", formalDefinition = "List of actors participating in this Contract Provision.") 1149 protected List<TermActorComponent> actor; 1150 1151 /** 1152 * Human readable form of this Contract Provision. 1153 */ 1154 @Child(name = "text", type = { StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1155 @Description(shortDefinition = "Human readable Contract term text", formalDefinition = "Human readable form of this Contract Provision.") 1156 protected StringType text; 1157 1158 /** 1159 * Contract Provision Valued Item List. 1160 */ 1161 @Child(name = "valuedItem", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1162 @Description(shortDefinition = "Contract Term Valued Item", formalDefinition = "Contract Provision Valued Item List.") 1163 protected List<TermValuedItemComponent> valuedItem; 1164 1165 /** 1166 * Nested group of Contract Provisions. 1167 */ 1168 @Child(name = "group", type = { 1169 TermComponent.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1170 @Description(shortDefinition = "Nested Contract Term Group", formalDefinition = "Nested group of Contract Provisions.") 1171 protected List<TermComponent> group; 1172 1173 private static final long serialVersionUID = -1137577465L; 1174 1175 /* 1176 * Constructor 1177 */ 1178 public TermComponent() { 1179 super(); 1180 } 1181 1182 /** 1183 * @return {@link #identifier} (Unique identifier for this particular Contract 1184 * Provision.) 1185 */ 1186 public Identifier getIdentifier() { 1187 if (this.identifier == null) 1188 if (Configuration.errorOnAutoCreate()) 1189 throw new Error("Attempt to auto-create TermComponent.identifier"); 1190 else if (Configuration.doAutoCreate()) 1191 this.identifier = new Identifier(); // cc 1192 return this.identifier; 1193 } 1194 1195 public boolean hasIdentifier() { 1196 return this.identifier != null && !this.identifier.isEmpty(); 1197 } 1198 1199 /** 1200 * @param value {@link #identifier} (Unique identifier for this particular 1201 * Contract Provision.) 1202 */ 1203 public TermComponent setIdentifier(Identifier value) { 1204 this.identifier = value; 1205 return this; 1206 } 1207 1208 /** 1209 * @return {@link #issued} (When this Contract Provision was issued.). This is 1210 * the underlying object with id, value and extensions. The accessor 1211 * "getIssued" gives direct access to the value 1212 */ 1213 public DateTimeType getIssuedElement() { 1214 if (this.issued == null) 1215 if (Configuration.errorOnAutoCreate()) 1216 throw new Error("Attempt to auto-create TermComponent.issued"); 1217 else if (Configuration.doAutoCreate()) 1218 this.issued = new DateTimeType(); // bb 1219 return this.issued; 1220 } 1221 1222 public boolean hasIssuedElement() { 1223 return this.issued != null && !this.issued.isEmpty(); 1224 } 1225 1226 public boolean hasIssued() { 1227 return this.issued != null && !this.issued.isEmpty(); 1228 } 1229 1230 /** 1231 * @param value {@link #issued} (When this Contract Provision was issued.). This 1232 * is the underlying object with id, value and extensions. The 1233 * accessor "getIssued" gives direct access to the value 1234 */ 1235 public TermComponent setIssuedElement(DateTimeType value) { 1236 this.issued = value; 1237 return this; 1238 } 1239 1240 /** 1241 * @return When this Contract Provision was issued. 1242 */ 1243 public Date getIssued() { 1244 return this.issued == null ? null : this.issued.getValue(); 1245 } 1246 1247 /** 1248 * @param value When this Contract Provision was issued. 1249 */ 1250 public TermComponent setIssued(Date value) { 1251 if (value == null) 1252 this.issued = null; 1253 else { 1254 if (this.issued == null) 1255 this.issued = new DateTimeType(); 1256 this.issued.setValue(value); 1257 } 1258 return this; 1259 } 1260 1261 /** 1262 * @return {@link #applies} (Relevant time or time-period when this Contract 1263 * Provision is applicable.) 1264 */ 1265 public Period getApplies() { 1266 if (this.applies == null) 1267 if (Configuration.errorOnAutoCreate()) 1268 throw new Error("Attempt to auto-create TermComponent.applies"); 1269 else if (Configuration.doAutoCreate()) 1270 this.applies = new Period(); // cc 1271 return this.applies; 1272 } 1273 1274 public boolean hasApplies() { 1275 return this.applies != null && !this.applies.isEmpty(); 1276 } 1277 1278 /** 1279 * @param value {@link #applies} (Relevant time or time-period when this 1280 * Contract Provision is applicable.) 1281 */ 1282 public TermComponent setApplies(Period value) { 1283 this.applies = value; 1284 return this; 1285 } 1286 1287 /** 1288 * @return {@link #type} (Type of Contract Provision such as specific 1289 * requirements, purposes for actions, obligations, prohibitions, e.g. 1290 * life time maximum benefit.) 1291 */ 1292 public CodeableConcept getType() { 1293 if (this.type == null) 1294 if (Configuration.errorOnAutoCreate()) 1295 throw new Error("Attempt to auto-create TermComponent.type"); 1296 else if (Configuration.doAutoCreate()) 1297 this.type = new CodeableConcept(); // cc 1298 return this.type; 1299 } 1300 1301 public boolean hasType() { 1302 return this.type != null && !this.type.isEmpty(); 1303 } 1304 1305 /** 1306 * @param value {@link #type} (Type of Contract Provision such as specific 1307 * requirements, purposes for actions, obligations, prohibitions, 1308 * e.g. life time maximum benefit.) 1309 */ 1310 public TermComponent setType(CodeableConcept value) { 1311 this.type = value; 1312 return this; 1313 } 1314 1315 /** 1316 * @return {@link #subType} (Subtype of this Contract Provision, e.g. life time 1317 * maximum payment for a contract term for specific valued item, e.g. 1318 * disability payment.) 1319 */ 1320 public CodeableConcept getSubType() { 1321 if (this.subType == null) 1322 if (Configuration.errorOnAutoCreate()) 1323 throw new Error("Attempt to auto-create TermComponent.subType"); 1324 else if (Configuration.doAutoCreate()) 1325 this.subType = new CodeableConcept(); // cc 1326 return this.subType; 1327 } 1328 1329 public boolean hasSubType() { 1330 return this.subType != null && !this.subType.isEmpty(); 1331 } 1332 1333 /** 1334 * @param value {@link #subType} (Subtype of this Contract Provision, e.g. life 1335 * time maximum payment for a contract term for specific valued 1336 * item, e.g. disability payment.) 1337 */ 1338 public TermComponent setSubType(CodeableConcept value) { 1339 this.subType = value; 1340 return this; 1341 } 1342 1343 /** 1344 * @return {@link #subject} (Who or what this Contract Provision is about.) 1345 */ 1346 public Reference getSubject() { 1347 if (this.subject == null) 1348 if (Configuration.errorOnAutoCreate()) 1349 throw new Error("Attempt to auto-create TermComponent.subject"); 1350 else if (Configuration.doAutoCreate()) 1351 this.subject = new Reference(); // cc 1352 return this.subject; 1353 } 1354 1355 public boolean hasSubject() { 1356 return this.subject != null && !this.subject.isEmpty(); 1357 } 1358 1359 /** 1360 * @param value {@link #subject} (Who or what this Contract Provision is about.) 1361 */ 1362 public TermComponent setSubject(Reference value) { 1363 this.subject = value; 1364 return this; 1365 } 1366 1367 /** 1368 * @return {@link #subject} The actual object that is the target of the 1369 * reference. The reference library doesn't populate this, but you can 1370 * use it to hold the resource if you resolve it. (Who or what this 1371 * Contract Provision is about.) 1372 */ 1373 public Resource getSubjectTarget() { 1374 return this.subjectTarget; 1375 } 1376 1377 /** 1378 * @param value {@link #subject} The actual object that is the target of the 1379 * reference. The reference library doesn't use these, but you can 1380 * use it to hold the resource if you resolve it. (Who or what this 1381 * Contract Provision is about.) 1382 */ 1383 public TermComponent setSubjectTarget(Resource value) { 1384 this.subjectTarget = value; 1385 return this; 1386 } 1387 1388 /** 1389 * @return {@link #action} (Action stipulated by this Contract Provision.) 1390 */ 1391 public List<CodeableConcept> getAction() { 1392 if (this.action == null) 1393 this.action = new ArrayList<CodeableConcept>(); 1394 return this.action; 1395 } 1396 1397 public boolean hasAction() { 1398 if (this.action == null) 1399 return false; 1400 for (CodeableConcept item : this.action) 1401 if (!item.isEmpty()) 1402 return true; 1403 return false; 1404 } 1405 1406 /** 1407 * @return {@link #action} (Action stipulated by this Contract Provision.) 1408 */ 1409 // syntactic sugar 1410 public CodeableConcept addAction() { // 3 1411 CodeableConcept t = new CodeableConcept(); 1412 if (this.action == null) 1413 this.action = new ArrayList<CodeableConcept>(); 1414 this.action.add(t); 1415 return t; 1416 } 1417 1418 // syntactic sugar 1419 public TermComponent addAction(CodeableConcept t) { // 3 1420 if (t == null) 1421 return this; 1422 if (this.action == null) 1423 this.action = new ArrayList<CodeableConcept>(); 1424 this.action.add(t); 1425 return this; 1426 } 1427 1428 /** 1429 * @return {@link #actionReason} (Reason or purpose for the action stipulated by 1430 * this Contract Provision.) 1431 */ 1432 public List<CodeableConcept> getActionReason() { 1433 if (this.actionReason == null) 1434 this.actionReason = new ArrayList<CodeableConcept>(); 1435 return this.actionReason; 1436 } 1437 1438 public boolean hasActionReason() { 1439 if (this.actionReason == null) 1440 return false; 1441 for (CodeableConcept item : this.actionReason) 1442 if (!item.isEmpty()) 1443 return true; 1444 return false; 1445 } 1446 1447 /** 1448 * @return {@link #actionReason} (Reason or purpose for the action stipulated by 1449 * this Contract Provision.) 1450 */ 1451 // syntactic sugar 1452 public CodeableConcept addActionReason() { // 3 1453 CodeableConcept t = new CodeableConcept(); 1454 if (this.actionReason == null) 1455 this.actionReason = new ArrayList<CodeableConcept>(); 1456 this.actionReason.add(t); 1457 return t; 1458 } 1459 1460 // syntactic sugar 1461 public TermComponent addActionReason(CodeableConcept t) { // 3 1462 if (t == null) 1463 return this; 1464 if (this.actionReason == null) 1465 this.actionReason = new ArrayList<CodeableConcept>(); 1466 this.actionReason.add(t); 1467 return this; 1468 } 1469 1470 /** 1471 * @return {@link #actor} (List of actors participating in this Contract 1472 * Provision.) 1473 */ 1474 public List<TermActorComponent> getActor() { 1475 if (this.actor == null) 1476 this.actor = new ArrayList<TermActorComponent>(); 1477 return this.actor; 1478 } 1479 1480 public boolean hasActor() { 1481 if (this.actor == null) 1482 return false; 1483 for (TermActorComponent item : this.actor) 1484 if (!item.isEmpty()) 1485 return true; 1486 return false; 1487 } 1488 1489 /** 1490 * @return {@link #actor} (List of actors participating in this Contract 1491 * Provision.) 1492 */ 1493 // syntactic sugar 1494 public TermActorComponent addActor() { // 3 1495 TermActorComponent t = new TermActorComponent(); 1496 if (this.actor == null) 1497 this.actor = new ArrayList<TermActorComponent>(); 1498 this.actor.add(t); 1499 return t; 1500 } 1501 1502 // syntactic sugar 1503 public TermComponent addActor(TermActorComponent t) { // 3 1504 if (t == null) 1505 return this; 1506 if (this.actor == null) 1507 this.actor = new ArrayList<TermActorComponent>(); 1508 this.actor.add(t); 1509 return this; 1510 } 1511 1512 /** 1513 * @return {@link #text} (Human readable form of this Contract Provision.). This 1514 * is the underlying object with id, value and extensions. The accessor 1515 * "getText" gives direct access to the value 1516 */ 1517 public StringType getTextElement() { 1518 if (this.text == null) 1519 if (Configuration.errorOnAutoCreate()) 1520 throw new Error("Attempt to auto-create TermComponent.text"); 1521 else if (Configuration.doAutoCreate()) 1522 this.text = new StringType(); // bb 1523 return this.text; 1524 } 1525 1526 public boolean hasTextElement() { 1527 return this.text != null && !this.text.isEmpty(); 1528 } 1529 1530 public boolean hasText() { 1531 return this.text != null && !this.text.isEmpty(); 1532 } 1533 1534 /** 1535 * @param value {@link #text} (Human readable form of this Contract Provision.). 1536 * This is the underlying object with id, value and extensions. The 1537 * accessor "getText" gives direct access to the value 1538 */ 1539 public TermComponent setTextElement(StringType value) { 1540 this.text = value; 1541 return this; 1542 } 1543 1544 /** 1545 * @return Human readable form of this Contract Provision. 1546 */ 1547 public String getText() { 1548 return this.text == null ? null : this.text.getValue(); 1549 } 1550 1551 /** 1552 * @param value Human readable form of this Contract Provision. 1553 */ 1554 public TermComponent setText(String value) { 1555 if (Utilities.noString(value)) 1556 this.text = null; 1557 else { 1558 if (this.text == null) 1559 this.text = new StringType(); 1560 this.text.setValue(value); 1561 } 1562 return this; 1563 } 1564 1565 /** 1566 * @return {@link #valuedItem} (Contract Provision Valued Item List.) 1567 */ 1568 public List<TermValuedItemComponent> getValuedItem() { 1569 if (this.valuedItem == null) 1570 this.valuedItem = new ArrayList<TermValuedItemComponent>(); 1571 return this.valuedItem; 1572 } 1573 1574 public boolean hasValuedItem() { 1575 if (this.valuedItem == null) 1576 return false; 1577 for (TermValuedItemComponent item : this.valuedItem) 1578 if (!item.isEmpty()) 1579 return true; 1580 return false; 1581 } 1582 1583 /** 1584 * @return {@link #valuedItem} (Contract Provision Valued Item List.) 1585 */ 1586 // syntactic sugar 1587 public TermValuedItemComponent addValuedItem() { // 3 1588 TermValuedItemComponent t = new TermValuedItemComponent(); 1589 if (this.valuedItem == null) 1590 this.valuedItem = new ArrayList<TermValuedItemComponent>(); 1591 this.valuedItem.add(t); 1592 return t; 1593 } 1594 1595 // syntactic sugar 1596 public TermComponent addValuedItem(TermValuedItemComponent t) { // 3 1597 if (t == null) 1598 return this; 1599 if (this.valuedItem == null) 1600 this.valuedItem = new ArrayList<TermValuedItemComponent>(); 1601 this.valuedItem.add(t); 1602 return this; 1603 } 1604 1605 /** 1606 * @return {@link #group} (Nested group of Contract Provisions.) 1607 */ 1608 public List<TermComponent> getGroup() { 1609 if (this.group == null) 1610 this.group = new ArrayList<TermComponent>(); 1611 return this.group; 1612 } 1613 1614 public boolean hasGroup() { 1615 if (this.group == null) 1616 return false; 1617 for (TermComponent item : this.group) 1618 if (!item.isEmpty()) 1619 return true; 1620 return false; 1621 } 1622 1623 /** 1624 * @return {@link #group} (Nested group of Contract Provisions.) 1625 */ 1626 // syntactic sugar 1627 public TermComponent addGroup() { // 3 1628 TermComponent t = new TermComponent(); 1629 if (this.group == null) 1630 this.group = new ArrayList<TermComponent>(); 1631 this.group.add(t); 1632 return t; 1633 } 1634 1635 // syntactic sugar 1636 public TermComponent addGroup(TermComponent t) { // 3 1637 if (t == null) 1638 return this; 1639 if (this.group == null) 1640 this.group = new ArrayList<TermComponent>(); 1641 this.group.add(t); 1642 return this; 1643 } 1644 1645 protected void listChildren(List<Property> childrenList) { 1646 super.listChildren(childrenList); 1647 childrenList.add(new Property("identifier", "Identifier", 1648 "Unique identifier for this particular Contract Provision.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1649 childrenList.add(new Property("issued", "dateTime", "When this Contract Provision was issued.", 0, 1650 java.lang.Integer.MAX_VALUE, issued)); 1651 childrenList.add( 1652 new Property("applies", "Period", "Relevant time or time-period when this Contract Provision is applicable.", 1653 0, java.lang.Integer.MAX_VALUE, applies)); 1654 childrenList.add(new Property("type", "CodeableConcept", 1655 "Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.", 1656 0, java.lang.Integer.MAX_VALUE, type)); 1657 childrenList.add(new Property("subType", "CodeableConcept", 1658 "Subtype of this Contract Provision, e.g. life time maximum payment for a contract term for specific valued item, e.g. disability payment.", 1659 0, java.lang.Integer.MAX_VALUE, subType)); 1660 childrenList.add(new Property("subject", "Reference(Any)", "Who or what this Contract Provision is about.", 0, 1661 java.lang.Integer.MAX_VALUE, subject)); 1662 childrenList.add(new Property("action", "CodeableConcept", "Action stipulated by this Contract Provision.", 0, 1663 java.lang.Integer.MAX_VALUE, action)); 1664 childrenList.add(new Property("actionReason", "CodeableConcept", 1665 "Reason or purpose for the action stipulated by this Contract Provision.", 0, java.lang.Integer.MAX_VALUE, 1666 actionReason)); 1667 childrenList.add(new Property("actor", "", "List of actors participating in this Contract Provision.", 0, 1668 java.lang.Integer.MAX_VALUE, actor)); 1669 childrenList.add(new Property("text", "string", "Human readable form of this Contract Provision.", 0, 1670 java.lang.Integer.MAX_VALUE, text)); 1671 childrenList.add(new Property("valuedItem", "", "Contract Provision Valued Item List.", 0, 1672 java.lang.Integer.MAX_VALUE, valuedItem)); 1673 childrenList.add(new Property("group", "@Contract.term", "Nested group of Contract Provisions.", 0, 1674 java.lang.Integer.MAX_VALUE, group)); 1675 } 1676 1677 @Override 1678 public void setProperty(String name, Base value) throws FHIRException { 1679 if (name.equals("identifier")) 1680 this.identifier = castToIdentifier(value); // Identifier 1681 else if (name.equals("issued")) 1682 this.issued = castToDateTime(value); // DateTimeType 1683 else if (name.equals("applies")) 1684 this.applies = castToPeriod(value); // Period 1685 else if (name.equals("type")) 1686 this.type = castToCodeableConcept(value); // CodeableConcept 1687 else if (name.equals("subType")) 1688 this.subType = castToCodeableConcept(value); // CodeableConcept 1689 else if (name.equals("subject")) 1690 this.subject = castToReference(value); // Reference 1691 else if (name.equals("action")) 1692 this.getAction().add(castToCodeableConcept(value)); 1693 else if (name.equals("actionReason")) 1694 this.getActionReason().add(castToCodeableConcept(value)); 1695 else if (name.equals("actor")) 1696 this.getActor().add((TermActorComponent) value); 1697 else if (name.equals("text")) 1698 this.text = castToString(value); // StringType 1699 else if (name.equals("valuedItem")) 1700 this.getValuedItem().add((TermValuedItemComponent) value); 1701 else if (name.equals("group")) 1702 this.getGroup().add((TermComponent) value); 1703 else 1704 super.setProperty(name, value); 1705 } 1706 1707 @Override 1708 public Base addChild(String name) throws FHIRException { 1709 if (name.equals("identifier")) { 1710 this.identifier = new Identifier(); 1711 return this.identifier; 1712 } else if (name.equals("issued")) { 1713 throw new FHIRException("Cannot call addChild on a singleton property Contract.issued"); 1714 } else if (name.equals("applies")) { 1715 this.applies = new Period(); 1716 return this.applies; 1717 } else if (name.equals("type")) { 1718 this.type = new CodeableConcept(); 1719 return this.type; 1720 } else if (name.equals("subType")) { 1721 this.subType = new CodeableConcept(); 1722 return this.subType; 1723 } else if (name.equals("subject")) { 1724 this.subject = new Reference(); 1725 return this.subject; 1726 } else if (name.equals("action")) { 1727 return addAction(); 1728 } else if (name.equals("actionReason")) { 1729 return addActionReason(); 1730 } else if (name.equals("actor")) { 1731 return addActor(); 1732 } else if (name.equals("text")) { 1733 throw new FHIRException("Cannot call addChild on a singleton property Contract.text"); 1734 } else if (name.equals("valuedItem")) { 1735 return addValuedItem(); 1736 } else if (name.equals("group")) { 1737 return addGroup(); 1738 } else 1739 return super.addChild(name); 1740 } 1741 1742 public TermComponent copy() { 1743 TermComponent dst = new TermComponent(); 1744 copyValues(dst); 1745 dst.identifier = identifier == null ? null : identifier.copy(); 1746 dst.issued = issued == null ? null : issued.copy(); 1747 dst.applies = applies == null ? null : applies.copy(); 1748 dst.type = type == null ? null : type.copy(); 1749 dst.subType = subType == null ? null : subType.copy(); 1750 dst.subject = subject == null ? null : subject.copy(); 1751 if (action != null) { 1752 dst.action = new ArrayList<CodeableConcept>(); 1753 for (CodeableConcept i : action) 1754 dst.action.add(i.copy()); 1755 } 1756 ; 1757 if (actionReason != null) { 1758 dst.actionReason = new ArrayList<CodeableConcept>(); 1759 for (CodeableConcept i : actionReason) 1760 dst.actionReason.add(i.copy()); 1761 } 1762 ; 1763 if (actor != null) { 1764 dst.actor = new ArrayList<TermActorComponent>(); 1765 for (TermActorComponent i : actor) 1766 dst.actor.add(i.copy()); 1767 } 1768 ; 1769 dst.text = text == null ? null : text.copy(); 1770 if (valuedItem != null) { 1771 dst.valuedItem = new ArrayList<TermValuedItemComponent>(); 1772 for (TermValuedItemComponent i : valuedItem) 1773 dst.valuedItem.add(i.copy()); 1774 } 1775 ; 1776 if (group != null) { 1777 dst.group = new ArrayList<TermComponent>(); 1778 for (TermComponent i : group) 1779 dst.group.add(i.copy()); 1780 } 1781 ; 1782 return dst; 1783 } 1784 1785 @Override 1786 public boolean equalsDeep(Base other) { 1787 if (!super.equalsDeep(other)) 1788 return false; 1789 if (!(other instanceof TermComponent)) 1790 return false; 1791 TermComponent o = (TermComponent) other; 1792 return compareDeep(identifier, o.identifier, true) && compareDeep(issued, o.issued, true) 1793 && compareDeep(applies, o.applies, true) && compareDeep(type, o.type, true) 1794 && compareDeep(subType, o.subType, true) && compareDeep(subject, o.subject, true) 1795 && compareDeep(action, o.action, true) && compareDeep(actionReason, o.actionReason, true) 1796 && compareDeep(actor, o.actor, true) && compareDeep(text, o.text, true) 1797 && compareDeep(valuedItem, o.valuedItem, true) && compareDeep(group, o.group, true); 1798 } 1799 1800 @Override 1801 public boolean equalsShallow(Base other) { 1802 if (!super.equalsShallow(other)) 1803 return false; 1804 if (!(other instanceof TermComponent)) 1805 return false; 1806 TermComponent o = (TermComponent) other; 1807 return compareValues(issued, o.issued, true) && compareValues(text, o.text, true); 1808 } 1809 1810 public boolean isEmpty() { 1811 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (issued == null || issued.isEmpty()) 1812 && (applies == null || applies.isEmpty()) && (type == null || type.isEmpty()) 1813 && (subType == null || subType.isEmpty()) && (subject == null || subject.isEmpty()) 1814 && (action == null || action.isEmpty()) && (actionReason == null || actionReason.isEmpty()) 1815 && (actor == null || actor.isEmpty()) && (text == null || text.isEmpty()) 1816 && (valuedItem == null || valuedItem.isEmpty()) && (group == null || group.isEmpty()); 1817 } 1818 1819 public String fhirType() { 1820 return "Contract.term"; 1821 1822 } 1823 1824 } 1825 1826 @Block() 1827 public static class TermActorComponent extends BackboneElement implements IBaseBackboneElement { 1828 /** 1829 * The actor assigned a role in this Contract Provision. 1830 */ 1831 @Child(name = "entity", type = { Contract.class, Device.class, Group.class, Location.class, Organization.class, 1832 Patient.class, Practitioner.class, RelatedPerson.class, 1833 Substance.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1834 @Description(shortDefinition = "Contract Term Actor", formalDefinition = "The actor assigned a role in this Contract Provision.") 1835 protected Reference entity; 1836 1837 /** 1838 * The actual object that is the target of the reference (The actor assigned a 1839 * role in this Contract Provision.) 1840 */ 1841 protected Resource entityTarget; 1842 1843 /** 1844 * Role played by the actor assigned this role in this Contract Provision. 1845 */ 1846 @Child(name = "role", type = { 1847 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1848 @Description(shortDefinition = "Contract Term Actor Role", formalDefinition = "Role played by the actor assigned this role in this Contract Provision.") 1849 protected List<CodeableConcept> role; 1850 1851 private static final long serialVersionUID = 1371245689L; 1852 1853 /* 1854 * Constructor 1855 */ 1856 public TermActorComponent() { 1857 super(); 1858 } 1859 1860 /* 1861 * Constructor 1862 */ 1863 public TermActorComponent(Reference entity) { 1864 super(); 1865 this.entity = entity; 1866 } 1867 1868 /** 1869 * @return {@link #entity} (The actor assigned a role in this Contract 1870 * Provision.) 1871 */ 1872 public Reference getEntity() { 1873 if (this.entity == null) 1874 if (Configuration.errorOnAutoCreate()) 1875 throw new Error("Attempt to auto-create TermActorComponent.entity"); 1876 else if (Configuration.doAutoCreate()) 1877 this.entity = new Reference(); // cc 1878 return this.entity; 1879 } 1880 1881 public boolean hasEntity() { 1882 return this.entity != null && !this.entity.isEmpty(); 1883 } 1884 1885 /** 1886 * @param value {@link #entity} (The actor assigned a role in this Contract 1887 * Provision.) 1888 */ 1889 public TermActorComponent setEntity(Reference value) { 1890 this.entity = value; 1891 return this; 1892 } 1893 1894 /** 1895 * @return {@link #entity} The actual object that is the target of the 1896 * reference. The reference library doesn't populate this, but you can 1897 * use it to hold the resource if you resolve it. (The actor assigned a 1898 * role in this Contract Provision.) 1899 */ 1900 public Resource getEntityTarget() { 1901 return this.entityTarget; 1902 } 1903 1904 /** 1905 * @param value {@link #entity} The actual object that is the target of the 1906 * reference. The reference library doesn't use these, but you can 1907 * use it to hold the resource if you resolve it. (The actor 1908 * assigned a role in this Contract Provision.) 1909 */ 1910 public TermActorComponent setEntityTarget(Resource value) { 1911 this.entityTarget = value; 1912 return this; 1913 } 1914 1915 /** 1916 * @return {@link #role} (Role played by the actor assigned this role in this 1917 * Contract Provision.) 1918 */ 1919 public List<CodeableConcept> getRole() { 1920 if (this.role == null) 1921 this.role = new ArrayList<CodeableConcept>(); 1922 return this.role; 1923 } 1924 1925 public boolean hasRole() { 1926 if (this.role == null) 1927 return false; 1928 for (CodeableConcept item : this.role) 1929 if (!item.isEmpty()) 1930 return true; 1931 return false; 1932 } 1933 1934 /** 1935 * @return {@link #role} (Role played by the actor assigned this role in this 1936 * Contract Provision.) 1937 */ 1938 // syntactic sugar 1939 public CodeableConcept addRole() { // 3 1940 CodeableConcept t = new CodeableConcept(); 1941 if (this.role == null) 1942 this.role = new ArrayList<CodeableConcept>(); 1943 this.role.add(t); 1944 return t; 1945 } 1946 1947 // syntactic sugar 1948 public TermActorComponent addRole(CodeableConcept t) { // 3 1949 if (t == null) 1950 return this; 1951 if (this.role == null) 1952 this.role = new ArrayList<CodeableConcept>(); 1953 this.role.add(t); 1954 return this; 1955 } 1956 1957 protected void listChildren(List<Property> childrenList) { 1958 super.listChildren(childrenList); 1959 childrenList.add(new Property("entity", 1960 "Reference(Contract|Device|Group|Location|Organization|Patient|Practitioner|RelatedPerson|Substance)", 1961 "The actor assigned a role in this Contract Provision.", 0, java.lang.Integer.MAX_VALUE, entity)); 1962 childrenList.add(new Property("role", "CodeableConcept", 1963 "Role played by the actor assigned this role in this Contract Provision.", 0, java.lang.Integer.MAX_VALUE, 1964 role)); 1965 } 1966 1967 @Override 1968 public void setProperty(String name, Base value) throws FHIRException { 1969 if (name.equals("entity")) 1970 this.entity = castToReference(value); // Reference 1971 else if (name.equals("role")) 1972 this.getRole().add(castToCodeableConcept(value)); 1973 else 1974 super.setProperty(name, value); 1975 } 1976 1977 @Override 1978 public Base addChild(String name) throws FHIRException { 1979 if (name.equals("entity")) { 1980 this.entity = new Reference(); 1981 return this.entity; 1982 } else if (name.equals("role")) { 1983 return addRole(); 1984 } else 1985 return super.addChild(name); 1986 } 1987 1988 public TermActorComponent copy() { 1989 TermActorComponent dst = new TermActorComponent(); 1990 copyValues(dst); 1991 dst.entity = entity == null ? null : entity.copy(); 1992 if (role != null) { 1993 dst.role = new ArrayList<CodeableConcept>(); 1994 for (CodeableConcept i : role) 1995 dst.role.add(i.copy()); 1996 } 1997 ; 1998 return dst; 1999 } 2000 2001 @Override 2002 public boolean equalsDeep(Base other) { 2003 if (!super.equalsDeep(other)) 2004 return false; 2005 if (!(other instanceof TermActorComponent)) 2006 return false; 2007 TermActorComponent o = (TermActorComponent) other; 2008 return compareDeep(entity, o.entity, true) && compareDeep(role, o.role, true); 2009 } 2010 2011 @Override 2012 public boolean equalsShallow(Base other) { 2013 if (!super.equalsShallow(other)) 2014 return false; 2015 if (!(other instanceof TermActorComponent)) 2016 return false; 2017 TermActorComponent o = (TermActorComponent) other; 2018 return true; 2019 } 2020 2021 public boolean isEmpty() { 2022 return super.isEmpty() && (entity == null || entity.isEmpty()) && (role == null || role.isEmpty()); 2023 } 2024 2025 public String fhirType() { 2026 return "Contract.term.actor"; 2027 2028 } 2029 2030 } 2031 2032 @Block() 2033 public static class TermValuedItemComponent extends BackboneElement implements IBaseBackboneElement { 2034 /** 2035 * Specific type of Contract Provision Valued Item that may be priced. 2036 */ 2037 @Child(name = "entity", type = { 2038 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2039 @Description(shortDefinition = "Contract Term Valued Item Type", formalDefinition = "Specific type of Contract Provision Valued Item that may be priced.") 2040 protected Type entity; 2041 2042 /** 2043 * Identifies a Contract Provision Valued Item instance. 2044 */ 2045 @Child(name = "identifier", type = { 2046 Identifier.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2047 @Description(shortDefinition = "Contract Term Valued Item Identifier", formalDefinition = "Identifies a Contract Provision Valued Item instance.") 2048 protected Identifier identifier; 2049 2050 /** 2051 * Indicates the time during which this Contract Term ValuedItem information is 2052 * effective. 2053 */ 2054 @Child(name = "effectiveTime", type = { 2055 DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2056 @Description(shortDefinition = "Contract Term Valued Item Effective Tiem", formalDefinition = "Indicates the time during which this Contract Term ValuedItem information is effective.") 2057 protected DateTimeType effectiveTime; 2058 2059 /** 2060 * Specifies the units by which the Contract Provision Valued Item is measured 2061 * or counted, and quantifies the countable or measurable Contract Term Valued 2062 * Item instances. 2063 */ 2064 @Child(name = "quantity", type = { 2065 SimpleQuantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2066 @Description(shortDefinition = "Contract Term Valued Item Count", formalDefinition = "Specifies the units by which the Contract Provision Valued Item is measured or counted, and quantifies the countable or measurable Contract Term Valued Item instances.") 2067 protected SimpleQuantity quantity; 2068 2069 /** 2070 * A Contract Provision Valued Item unit valuation measure. 2071 */ 2072 @Child(name = "unitPrice", type = { Money.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2073 @Description(shortDefinition = "Contract Term Valued Item fee, charge, or cost", formalDefinition = "A Contract Provision Valued Item unit valuation measure.") 2074 protected Money unitPrice; 2075 2076 /** 2077 * A real number that represents a multiplier used in determining the overall 2078 * value of the Contract Provision Valued Item delivered. The concept of a 2079 * Factor allows for a discount or surcharge multiplier to be applied to a 2080 * monetary amount. 2081 */ 2082 @Child(name = "factor", type = { 2083 DecimalType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 2084 @Description(shortDefinition = "Contract Term Valued Item Price Scaling Factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of the Contract Provision Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 2085 protected DecimalType factor; 2086 2087 /** 2088 * An amount that expresses the weighting (based on difficulty, cost and/or 2089 * resource intensiveness) associated with the Contract Provision Valued Item 2090 * delivered. The concept of Points allows for assignment of point values for a 2091 * Contract ProvisionValued Item, such that a monetary amount can be assigned to 2092 * each point. 2093 */ 2094 @Child(name = "points", type = { 2095 DecimalType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 2096 @Description(shortDefinition = "Contract Term Valued Item Difficulty Scaling Factor", formalDefinition = "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Provision Valued Item delivered. The concept of Points allows for assignment of point values for a Contract ProvisionValued Item, such that a monetary amount can be assigned to each point.") 2097 protected DecimalType points; 2098 2099 /** 2100 * Expresses the product of the Contract Provision Valued Item unitQuantity and 2101 * the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost 2102 * per Point) * factor Number * points = net Amount. Quantity, factor and points 2103 * are assumed to be 1 if not supplied. 2104 */ 2105 @Child(name = "net", type = { Money.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 2106 @Description(shortDefinition = "Total Contract Term Valued Item Value", formalDefinition = "Expresses the product of the Contract Provision Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.") 2107 protected Money net; 2108 2109 private static final long serialVersionUID = 1782449516L; 2110 2111 /* 2112 * Constructor 2113 */ 2114 public TermValuedItemComponent() { 2115 super(); 2116 } 2117 2118 /** 2119 * @return {@link #entity} (Specific type of Contract Provision Valued Item that 2120 * may be priced.) 2121 */ 2122 public Type getEntity() { 2123 return this.entity; 2124 } 2125 2126 /** 2127 * @return {@link #entity} (Specific type of Contract Provision Valued Item that 2128 * may be priced.) 2129 */ 2130 public CodeableConcept getEntityCodeableConcept() throws FHIRException { 2131 if (!(this.entity instanceof CodeableConcept)) 2132 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2133 + this.entity.getClass().getName() + " was encountered"); 2134 return (CodeableConcept) this.entity; 2135 } 2136 2137 public boolean hasEntityCodeableConcept() { 2138 return this.entity instanceof CodeableConcept; 2139 } 2140 2141 /** 2142 * @return {@link #entity} (Specific type of Contract Provision Valued Item that 2143 * may be priced.) 2144 */ 2145 public Reference getEntityReference() throws FHIRException { 2146 if (!(this.entity instanceof Reference)) 2147 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2148 + this.entity.getClass().getName() + " was encountered"); 2149 return (Reference) this.entity; 2150 } 2151 2152 public boolean hasEntityReference() { 2153 return this.entity instanceof Reference; 2154 } 2155 2156 public boolean hasEntity() { 2157 return this.entity != null && !this.entity.isEmpty(); 2158 } 2159 2160 /** 2161 * @param value {@link #entity} (Specific type of Contract Provision Valued Item 2162 * that may be priced.) 2163 */ 2164 public TermValuedItemComponent setEntity(Type value) { 2165 this.entity = value; 2166 return this; 2167 } 2168 2169 /** 2170 * @return {@link #identifier} (Identifies a Contract Provision Valued Item 2171 * instance.) 2172 */ 2173 public Identifier getIdentifier() { 2174 if (this.identifier == null) 2175 if (Configuration.errorOnAutoCreate()) 2176 throw new Error("Attempt to auto-create TermValuedItemComponent.identifier"); 2177 else if (Configuration.doAutoCreate()) 2178 this.identifier = new Identifier(); // cc 2179 return this.identifier; 2180 } 2181 2182 public boolean hasIdentifier() { 2183 return this.identifier != null && !this.identifier.isEmpty(); 2184 } 2185 2186 /** 2187 * @param value {@link #identifier} (Identifies a Contract Provision Valued Item 2188 * instance.) 2189 */ 2190 public TermValuedItemComponent setIdentifier(Identifier value) { 2191 this.identifier = value; 2192 return this; 2193 } 2194 2195 /** 2196 * @return {@link #effectiveTime} (Indicates the time during which this Contract 2197 * Term ValuedItem information is effective.). This is the underlying 2198 * object with id, value and extensions. The accessor "getEffectiveTime" 2199 * gives direct access to the value 2200 */ 2201 public DateTimeType getEffectiveTimeElement() { 2202 if (this.effectiveTime == null) 2203 if (Configuration.errorOnAutoCreate()) 2204 throw new Error("Attempt to auto-create TermValuedItemComponent.effectiveTime"); 2205 else if (Configuration.doAutoCreate()) 2206 this.effectiveTime = new DateTimeType(); // bb 2207 return this.effectiveTime; 2208 } 2209 2210 public boolean hasEffectiveTimeElement() { 2211 return this.effectiveTime != null && !this.effectiveTime.isEmpty(); 2212 } 2213 2214 public boolean hasEffectiveTime() { 2215 return this.effectiveTime != null && !this.effectiveTime.isEmpty(); 2216 } 2217 2218 /** 2219 * @param value {@link #effectiveTime} (Indicates the time during which this 2220 * Contract Term ValuedItem information is effective.). This is the 2221 * underlying object with id, value and extensions. The accessor 2222 * "getEffectiveTime" gives direct access to the value 2223 */ 2224 public TermValuedItemComponent setEffectiveTimeElement(DateTimeType value) { 2225 this.effectiveTime = value; 2226 return this; 2227 } 2228 2229 /** 2230 * @return Indicates the time during which this Contract Term ValuedItem 2231 * information is effective. 2232 */ 2233 public Date getEffectiveTime() { 2234 return this.effectiveTime == null ? null : this.effectiveTime.getValue(); 2235 } 2236 2237 /** 2238 * @param value Indicates the time during which this Contract Term ValuedItem 2239 * information is effective. 2240 */ 2241 public TermValuedItemComponent setEffectiveTime(Date value) { 2242 if (value == null) 2243 this.effectiveTime = null; 2244 else { 2245 if (this.effectiveTime == null) 2246 this.effectiveTime = new DateTimeType(); 2247 this.effectiveTime.setValue(value); 2248 } 2249 return this; 2250 } 2251 2252 /** 2253 * @return {@link #quantity} (Specifies the units by which the Contract 2254 * Provision Valued Item is measured or counted, and quantifies the 2255 * countable or measurable Contract Term Valued Item instances.) 2256 */ 2257 public SimpleQuantity getQuantity() { 2258 if (this.quantity == null) 2259 if (Configuration.errorOnAutoCreate()) 2260 throw new Error("Attempt to auto-create TermValuedItemComponent.quantity"); 2261 else if (Configuration.doAutoCreate()) 2262 this.quantity = new SimpleQuantity(); // cc 2263 return this.quantity; 2264 } 2265 2266 public boolean hasQuantity() { 2267 return this.quantity != null && !this.quantity.isEmpty(); 2268 } 2269 2270 /** 2271 * @param value {@link #quantity} (Specifies the units by which the Contract 2272 * Provision Valued Item is measured or counted, and quantifies the 2273 * countable or measurable Contract Term Valued Item instances.) 2274 */ 2275 public TermValuedItemComponent setQuantity(SimpleQuantity value) { 2276 this.quantity = value; 2277 return this; 2278 } 2279 2280 /** 2281 * @return {@link #unitPrice} (A Contract Provision Valued Item unit valuation 2282 * measure.) 2283 */ 2284 public Money getUnitPrice() { 2285 if (this.unitPrice == null) 2286 if (Configuration.errorOnAutoCreate()) 2287 throw new Error("Attempt to auto-create TermValuedItemComponent.unitPrice"); 2288 else if (Configuration.doAutoCreate()) 2289 this.unitPrice = new Money(); // cc 2290 return this.unitPrice; 2291 } 2292 2293 public boolean hasUnitPrice() { 2294 return this.unitPrice != null && !this.unitPrice.isEmpty(); 2295 } 2296 2297 /** 2298 * @param value {@link #unitPrice} (A Contract Provision Valued Item unit 2299 * valuation measure.) 2300 */ 2301 public TermValuedItemComponent setUnitPrice(Money value) { 2302 this.unitPrice = value; 2303 return this; 2304 } 2305 2306 /** 2307 * @return {@link #factor} (A real number that represents a multiplier used in 2308 * determining the overall value of the Contract Provision Valued Item 2309 * delivered. The concept of a Factor allows for a discount or surcharge 2310 * multiplier to be applied to a monetary amount.). This is the 2311 * underlying object with id, value and extensions. The accessor 2312 * "getFactor" gives direct access to the value 2313 */ 2314 public DecimalType getFactorElement() { 2315 if (this.factor == null) 2316 if (Configuration.errorOnAutoCreate()) 2317 throw new Error("Attempt to auto-create TermValuedItemComponent.factor"); 2318 else if (Configuration.doAutoCreate()) 2319 this.factor = new DecimalType(); // bb 2320 return this.factor; 2321 } 2322 2323 public boolean hasFactorElement() { 2324 return this.factor != null && !this.factor.isEmpty(); 2325 } 2326 2327 public boolean hasFactor() { 2328 return this.factor != null && !this.factor.isEmpty(); 2329 } 2330 2331 /** 2332 * @param value {@link #factor} (A real number that represents a multiplier used 2333 * in determining the overall value of the Contract Provision 2334 * Valued Item delivered. The concept of a Factor allows for a 2335 * discount or surcharge multiplier to be applied to a monetary 2336 * amount.). This is the underlying object with id, value and 2337 * extensions. The accessor "getFactor" gives direct access to the 2338 * value 2339 */ 2340 public TermValuedItemComponent setFactorElement(DecimalType value) { 2341 this.factor = value; 2342 return this; 2343 } 2344 2345 /** 2346 * @return A real number that represents a multiplier used in determining the 2347 * overall value of the Contract Provision Valued Item delivered. The 2348 * concept of a Factor allows for a discount or surcharge multiplier to 2349 * be applied to a monetary amount. 2350 */ 2351 public BigDecimal getFactor() { 2352 return this.factor == null ? null : this.factor.getValue(); 2353 } 2354 2355 /** 2356 * @param value A real number that represents a multiplier used in determining 2357 * the overall value of the Contract Provision Valued Item 2358 * delivered. The concept of a Factor allows for a discount or 2359 * surcharge multiplier to be applied to a monetary amount. 2360 */ 2361 public TermValuedItemComponent setFactor(BigDecimal value) { 2362 if (value == null) 2363 this.factor = null; 2364 else { 2365 if (this.factor == null) 2366 this.factor = new DecimalType(); 2367 this.factor.setValue(value); 2368 } 2369 return this; 2370 } 2371 2372 /** 2373 * @return {@link #points} (An amount that expresses the weighting (based on 2374 * difficulty, cost and/or resource intensiveness) associated with the 2375 * Contract Provision Valued Item delivered. The concept of Points 2376 * allows for assignment of point values for a Contract ProvisionValued 2377 * Item, such that a monetary amount can be assigned to each point.). 2378 * This is the underlying object with id, value and extensions. The 2379 * accessor "getPoints" gives direct access to the value 2380 */ 2381 public DecimalType getPointsElement() { 2382 if (this.points == null) 2383 if (Configuration.errorOnAutoCreate()) 2384 throw new Error("Attempt to auto-create TermValuedItemComponent.points"); 2385 else if (Configuration.doAutoCreate()) 2386 this.points = new DecimalType(); // bb 2387 return this.points; 2388 } 2389 2390 public boolean hasPointsElement() { 2391 return this.points != null && !this.points.isEmpty(); 2392 } 2393 2394 public boolean hasPoints() { 2395 return this.points != null && !this.points.isEmpty(); 2396 } 2397 2398 /** 2399 * @param value {@link #points} (An amount that expresses the weighting (based 2400 * on difficulty, cost and/or resource intensiveness) associated 2401 * with the Contract Provision Valued Item delivered. The concept 2402 * of Points allows for assignment of point values for a Contract 2403 * ProvisionValued Item, such that a monetary amount can be 2404 * assigned to each point.). This is the underlying object with id, 2405 * value and extensions. The accessor "getPoints" gives direct 2406 * access to the value 2407 */ 2408 public TermValuedItemComponent setPointsElement(DecimalType value) { 2409 this.points = value; 2410 return this; 2411 } 2412 2413 /** 2414 * @return An amount that expresses the weighting (based on difficulty, cost 2415 * and/or resource intensiveness) associated with the Contract Provision 2416 * Valued Item delivered. The concept of Points allows for assignment of 2417 * point values for a Contract ProvisionValued Item, such that a 2418 * monetary amount can be assigned to each point. 2419 */ 2420 public BigDecimal getPoints() { 2421 return this.points == null ? null : this.points.getValue(); 2422 } 2423 2424 /** 2425 * @param value An amount that expresses the weighting (based on difficulty, 2426 * cost and/or resource intensiveness) associated with the Contract 2427 * Provision Valued Item delivered. The concept of Points allows 2428 * for assignment of point values for a Contract ProvisionValued 2429 * Item, such that a monetary amount can be assigned to each point. 2430 */ 2431 public TermValuedItemComponent setPoints(BigDecimal value) { 2432 if (value == null) 2433 this.points = null; 2434 else { 2435 if (this.points == null) 2436 this.points = new DecimalType(); 2437 this.points.setValue(value); 2438 } 2439 return this; 2440 } 2441 2442 /** 2443 * @return {@link #net} (Expresses the product of the Contract Provision Valued 2444 * Item unitQuantity and the unitPriceAmt. For example, the formula: 2445 * unit Quantity * unit Price (Cost per Point) * factor Number * points 2446 * = net Amount. Quantity, factor and points are assumed to be 1 if not 2447 * supplied.) 2448 */ 2449 public Money getNet() { 2450 if (this.net == null) 2451 if (Configuration.errorOnAutoCreate()) 2452 throw new Error("Attempt to auto-create TermValuedItemComponent.net"); 2453 else if (Configuration.doAutoCreate()) 2454 this.net = new Money(); // cc 2455 return this.net; 2456 } 2457 2458 public boolean hasNet() { 2459 return this.net != null && !this.net.isEmpty(); 2460 } 2461 2462 /** 2463 * @param value {@link #net} (Expresses the product of the Contract Provision 2464 * Valued Item unitQuantity and the unitPriceAmt. For example, the 2465 * formula: unit Quantity * unit Price (Cost per Point) * factor 2466 * Number * points = net Amount. Quantity, factor and points are 2467 * assumed to be 1 if not supplied.) 2468 */ 2469 public TermValuedItemComponent setNet(Money value) { 2470 this.net = value; 2471 return this; 2472 } 2473 2474 protected void listChildren(List<Property> childrenList) { 2475 super.listChildren(childrenList); 2476 childrenList.add(new Property("entity[x]", "CodeableConcept|Reference(Any)", 2477 "Specific type of Contract Provision Valued Item that may be priced.", 0, java.lang.Integer.MAX_VALUE, 2478 entity)); 2479 childrenList.add(new Property("identifier", "Identifier", "Identifies a Contract Provision Valued Item instance.", 2480 0, java.lang.Integer.MAX_VALUE, identifier)); 2481 childrenList.add(new Property("effectiveTime", "dateTime", 2482 "Indicates the time during which this Contract Term ValuedItem information is effective.", 0, 2483 java.lang.Integer.MAX_VALUE, effectiveTime)); 2484 childrenList.add(new Property("quantity", "SimpleQuantity", 2485 "Specifies the units by which the Contract Provision Valued Item is measured or counted, and quantifies the countable or measurable Contract Term Valued Item instances.", 2486 0, java.lang.Integer.MAX_VALUE, quantity)); 2487 childrenList.add(new Property("unitPrice", "Money", "A Contract Provision Valued Item unit valuation measure.", 0, 2488 java.lang.Integer.MAX_VALUE, unitPrice)); 2489 childrenList.add(new Property("factor", "decimal", 2490 "A real number that represents a multiplier used in determining the overall value of the Contract Provision Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 2491 0, java.lang.Integer.MAX_VALUE, factor)); 2492 childrenList.add(new Property("points", "decimal", 2493 "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Provision Valued Item delivered. The concept of Points allows for assignment of point values for a Contract ProvisionValued Item, such that a monetary amount can be assigned to each point.", 2494 0, java.lang.Integer.MAX_VALUE, points)); 2495 childrenList.add(new Property("net", "Money", 2496 "Expresses the product of the Contract Provision Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 2497 0, java.lang.Integer.MAX_VALUE, net)); 2498 } 2499 2500 @Override 2501 public void setProperty(String name, Base value) throws FHIRException { 2502 if (name.equals("entity[x]")) 2503 this.entity = (Type) value; // Type 2504 else if (name.equals("identifier")) 2505 this.identifier = castToIdentifier(value); // Identifier 2506 else if (name.equals("effectiveTime")) 2507 this.effectiveTime = castToDateTime(value); // DateTimeType 2508 else if (name.equals("quantity")) 2509 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 2510 else if (name.equals("unitPrice")) 2511 this.unitPrice = castToMoney(value); // Money 2512 else if (name.equals("factor")) 2513 this.factor = castToDecimal(value); // DecimalType 2514 else if (name.equals("points")) 2515 this.points = castToDecimal(value); // DecimalType 2516 else if (name.equals("net")) 2517 this.net = castToMoney(value); // Money 2518 else 2519 super.setProperty(name, value); 2520 } 2521 2522 @Override 2523 public Base addChild(String name) throws FHIRException { 2524 if (name.equals("entityCodeableConcept")) { 2525 this.entity = new CodeableConcept(); 2526 return this.entity; 2527 } else if (name.equals("entityReference")) { 2528 this.entity = new Reference(); 2529 return this.entity; 2530 } else if (name.equals("identifier")) { 2531 this.identifier = new Identifier(); 2532 return this.identifier; 2533 } else if (name.equals("effectiveTime")) { 2534 throw new FHIRException("Cannot call addChild on a singleton property Contract.effectiveTime"); 2535 } else if (name.equals("quantity")) { 2536 this.quantity = new SimpleQuantity(); 2537 return this.quantity; 2538 } else if (name.equals("unitPrice")) { 2539 this.unitPrice = new Money(); 2540 return this.unitPrice; 2541 } else if (name.equals("factor")) { 2542 throw new FHIRException("Cannot call addChild on a singleton property Contract.factor"); 2543 } else if (name.equals("points")) { 2544 throw new FHIRException("Cannot call addChild on a singleton property Contract.points"); 2545 } else if (name.equals("net")) { 2546 this.net = new Money(); 2547 return this.net; 2548 } else 2549 return super.addChild(name); 2550 } 2551 2552 public TermValuedItemComponent copy() { 2553 TermValuedItemComponent dst = new TermValuedItemComponent(); 2554 copyValues(dst); 2555 dst.entity = entity == null ? null : entity.copy(); 2556 dst.identifier = identifier == null ? null : identifier.copy(); 2557 dst.effectiveTime = effectiveTime == null ? null : effectiveTime.copy(); 2558 dst.quantity = quantity == null ? null : quantity.copy(); 2559 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 2560 dst.factor = factor == null ? null : factor.copy(); 2561 dst.points = points == null ? null : points.copy(); 2562 dst.net = net == null ? null : net.copy(); 2563 return dst; 2564 } 2565 2566 @Override 2567 public boolean equalsDeep(Base other) { 2568 if (!super.equalsDeep(other)) 2569 return false; 2570 if (!(other instanceof TermValuedItemComponent)) 2571 return false; 2572 TermValuedItemComponent o = (TermValuedItemComponent) other; 2573 return compareDeep(entity, o.entity, true) && compareDeep(identifier, o.identifier, true) 2574 && compareDeep(effectiveTime, o.effectiveTime, true) && compareDeep(quantity, o.quantity, true) 2575 && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 2576 && compareDeep(points, o.points, true) && compareDeep(net, o.net, true); 2577 } 2578 2579 @Override 2580 public boolean equalsShallow(Base other) { 2581 if (!super.equalsShallow(other)) 2582 return false; 2583 if (!(other instanceof TermValuedItemComponent)) 2584 return false; 2585 TermValuedItemComponent o = (TermValuedItemComponent) other; 2586 return compareValues(effectiveTime, o.effectiveTime, true) && compareValues(factor, o.factor, true) 2587 && compareValues(points, o.points, true); 2588 } 2589 2590 public boolean isEmpty() { 2591 return super.isEmpty() && (entity == null || entity.isEmpty()) && (identifier == null || identifier.isEmpty()) 2592 && (effectiveTime == null || effectiveTime.isEmpty()) && (quantity == null || quantity.isEmpty()) 2593 && (unitPrice == null || unitPrice.isEmpty()) && (factor == null || factor.isEmpty()) 2594 && (points == null || points.isEmpty()) && (net == null || net.isEmpty()); 2595 } 2596 2597 public String fhirType() { 2598 return "Contract.term.valuedItem"; 2599 2600 } 2601 2602 } 2603 2604 @Block() 2605 public static class FriendlyLanguageComponent extends BackboneElement implements IBaseBackboneElement { 2606 /** 2607 * Human readable rendering of this Contract in a format and representation 2608 * intended to enhance comprehension and ensure understandability. 2609 */ 2610 @Child(name = "content", type = { Attachment.class, Composition.class, DocumentReference.class, 2611 QuestionnaireResponse.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2612 @Description(shortDefinition = "Easily comprehended representation of this Contract", formalDefinition = "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.") 2613 protected Type content; 2614 2615 private static final long serialVersionUID = -1763459053L; 2616 2617 /* 2618 * Constructor 2619 */ 2620 public FriendlyLanguageComponent() { 2621 super(); 2622 } 2623 2624 /* 2625 * Constructor 2626 */ 2627 public FriendlyLanguageComponent(Type content) { 2628 super(); 2629 this.content = content; 2630 } 2631 2632 /** 2633 * @return {@link #content} (Human readable rendering of this Contract in a 2634 * format and representation intended to enhance comprehension and 2635 * ensure understandability.) 2636 */ 2637 public Type getContent() { 2638 return this.content; 2639 } 2640 2641 /** 2642 * @return {@link #content} (Human readable rendering of this Contract in a 2643 * format and representation intended to enhance comprehension and 2644 * ensure understandability.) 2645 */ 2646 public Attachment getContentAttachment() throws FHIRException { 2647 if (!(this.content instanceof Attachment)) 2648 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 2649 + this.content.getClass().getName() + " was encountered"); 2650 return (Attachment) this.content; 2651 } 2652 2653 public boolean hasContentAttachment() { 2654 return this.content instanceof Attachment; 2655 } 2656 2657 /** 2658 * @return {@link #content} (Human readable rendering of this Contract in a 2659 * format and representation intended to enhance comprehension and 2660 * ensure understandability.) 2661 */ 2662 public Reference getContentReference() throws FHIRException { 2663 if (!(this.content instanceof Reference)) 2664 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2665 + this.content.getClass().getName() + " was encountered"); 2666 return (Reference) this.content; 2667 } 2668 2669 public boolean hasContentReference() { 2670 return this.content instanceof Reference; 2671 } 2672 2673 public boolean hasContent() { 2674 return this.content != null && !this.content.isEmpty(); 2675 } 2676 2677 /** 2678 * @param value {@link #content} (Human readable rendering of this Contract in a 2679 * format and representation intended to enhance comprehension and 2680 * ensure understandability.) 2681 */ 2682 public FriendlyLanguageComponent setContent(Type value) { 2683 this.content = value; 2684 return this; 2685 } 2686 2687 protected void listChildren(List<Property> childrenList) { 2688 super.listChildren(childrenList); 2689 childrenList.add(new Property("content[x]", 2690 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 2691 "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 2692 0, java.lang.Integer.MAX_VALUE, content)); 2693 } 2694 2695 @Override 2696 public void setProperty(String name, Base value) throws FHIRException { 2697 if (name.equals("content[x]")) 2698 this.content = (Type) value; // Type 2699 else 2700 super.setProperty(name, value); 2701 } 2702 2703 @Override 2704 public Base addChild(String name) throws FHIRException { 2705 if (name.equals("contentAttachment")) { 2706 this.content = new Attachment(); 2707 return this.content; 2708 } else if (name.equals("contentReference")) { 2709 this.content = new Reference(); 2710 return this.content; 2711 } else 2712 return super.addChild(name); 2713 } 2714 2715 public FriendlyLanguageComponent copy() { 2716 FriendlyLanguageComponent dst = new FriendlyLanguageComponent(); 2717 copyValues(dst); 2718 dst.content = content == null ? null : content.copy(); 2719 return dst; 2720 } 2721 2722 @Override 2723 public boolean equalsDeep(Base other) { 2724 if (!super.equalsDeep(other)) 2725 return false; 2726 if (!(other instanceof FriendlyLanguageComponent)) 2727 return false; 2728 FriendlyLanguageComponent o = (FriendlyLanguageComponent) other; 2729 return compareDeep(content, o.content, true); 2730 } 2731 2732 @Override 2733 public boolean equalsShallow(Base other) { 2734 if (!super.equalsShallow(other)) 2735 return false; 2736 if (!(other instanceof FriendlyLanguageComponent)) 2737 return false; 2738 FriendlyLanguageComponent o = (FriendlyLanguageComponent) other; 2739 return true; 2740 } 2741 2742 public boolean isEmpty() { 2743 return super.isEmpty() && (content == null || content.isEmpty()); 2744 } 2745 2746 public String fhirType() { 2747 return "Contract.friendly"; 2748 2749 } 2750 2751 } 2752 2753 @Block() 2754 public static class LegalLanguageComponent extends BackboneElement implements IBaseBackboneElement { 2755 /** 2756 * Contract legal text in human renderable form. 2757 */ 2758 @Child(name = "content", type = { Attachment.class, Composition.class, DocumentReference.class, 2759 QuestionnaireResponse.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2760 @Description(shortDefinition = "Contract Legal Text", formalDefinition = "Contract legal text in human renderable form.") 2761 protected Type content; 2762 2763 private static final long serialVersionUID = -1763459053L; 2764 2765 /* 2766 * Constructor 2767 */ 2768 public LegalLanguageComponent() { 2769 super(); 2770 } 2771 2772 /* 2773 * Constructor 2774 */ 2775 public LegalLanguageComponent(Type content) { 2776 super(); 2777 this.content = content; 2778 } 2779 2780 /** 2781 * @return {@link #content} (Contract legal text in human renderable form.) 2782 */ 2783 public Type getContent() { 2784 return this.content; 2785 } 2786 2787 /** 2788 * @return {@link #content} (Contract legal text in human renderable form.) 2789 */ 2790 public Attachment getContentAttachment() throws FHIRException { 2791 if (!(this.content instanceof Attachment)) 2792 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 2793 + this.content.getClass().getName() + " was encountered"); 2794 return (Attachment) this.content; 2795 } 2796 2797 public boolean hasContentAttachment() { 2798 return this.content instanceof Attachment; 2799 } 2800 2801 /** 2802 * @return {@link #content} (Contract legal text in human renderable form.) 2803 */ 2804 public Reference getContentReference() throws FHIRException { 2805 if (!(this.content instanceof Reference)) 2806 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2807 + this.content.getClass().getName() + " was encountered"); 2808 return (Reference) this.content; 2809 } 2810 2811 public boolean hasContentReference() { 2812 return this.content instanceof Reference; 2813 } 2814 2815 public boolean hasContent() { 2816 return this.content != null && !this.content.isEmpty(); 2817 } 2818 2819 /** 2820 * @param value {@link #content} (Contract legal text in human renderable form.) 2821 */ 2822 public LegalLanguageComponent setContent(Type value) { 2823 this.content = value; 2824 return this; 2825 } 2826 2827 protected void listChildren(List<Property> childrenList) { 2828 super.listChildren(childrenList); 2829 childrenList 2830 .add(new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 2831 "Contract legal text in human renderable form.", 0, java.lang.Integer.MAX_VALUE, content)); 2832 } 2833 2834 @Override 2835 public void setProperty(String name, Base value) throws FHIRException { 2836 if (name.equals("content[x]")) 2837 this.content = (Type) value; // Type 2838 else 2839 super.setProperty(name, value); 2840 } 2841 2842 @Override 2843 public Base addChild(String name) throws FHIRException { 2844 if (name.equals("contentAttachment")) { 2845 this.content = new Attachment(); 2846 return this.content; 2847 } else if (name.equals("contentReference")) { 2848 this.content = new Reference(); 2849 return this.content; 2850 } else 2851 return super.addChild(name); 2852 } 2853 2854 public LegalLanguageComponent copy() { 2855 LegalLanguageComponent dst = new LegalLanguageComponent(); 2856 copyValues(dst); 2857 dst.content = content == null ? null : content.copy(); 2858 return dst; 2859 } 2860 2861 @Override 2862 public boolean equalsDeep(Base other) { 2863 if (!super.equalsDeep(other)) 2864 return false; 2865 if (!(other instanceof LegalLanguageComponent)) 2866 return false; 2867 LegalLanguageComponent o = (LegalLanguageComponent) other; 2868 return compareDeep(content, o.content, true); 2869 } 2870 2871 @Override 2872 public boolean equalsShallow(Base other) { 2873 if (!super.equalsShallow(other)) 2874 return false; 2875 if (!(other instanceof LegalLanguageComponent)) 2876 return false; 2877 LegalLanguageComponent o = (LegalLanguageComponent) other; 2878 return true; 2879 } 2880 2881 public boolean isEmpty() { 2882 return super.isEmpty() && (content == null || content.isEmpty()); 2883 } 2884 2885 public String fhirType() { 2886 return "Contract.legal"; 2887 2888 } 2889 2890 } 2891 2892 @Block() 2893 public static class ComputableLanguageComponent extends BackboneElement implements IBaseBackboneElement { 2894 /** 2895 * Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, 2896 * SecPal). 2897 */ 2898 @Child(name = "content", type = { Attachment.class, 2899 DocumentReference.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2900 @Description(shortDefinition = "Computable Contract Rules", formalDefinition = "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).") 2901 protected Type content; 2902 2903 private static final long serialVersionUID = -1763459053L; 2904 2905 /* 2906 * Constructor 2907 */ 2908 public ComputableLanguageComponent() { 2909 super(); 2910 } 2911 2912 /* 2913 * Constructor 2914 */ 2915 public ComputableLanguageComponent(Type content) { 2916 super(); 2917 this.content = content; 2918 } 2919 2920 /** 2921 * @return {@link #content} (Computable Contract conveyed using a policy rule 2922 * language (e.g. XACML, DKAL, SecPal).) 2923 */ 2924 public Type getContent() { 2925 return this.content; 2926 } 2927 2928 /** 2929 * @return {@link #content} (Computable Contract conveyed using a policy rule 2930 * language (e.g. XACML, DKAL, SecPal).) 2931 */ 2932 public Attachment getContentAttachment() throws FHIRException { 2933 if (!(this.content instanceof Attachment)) 2934 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 2935 + this.content.getClass().getName() + " was encountered"); 2936 return (Attachment) this.content; 2937 } 2938 2939 public boolean hasContentAttachment() { 2940 return this.content instanceof Attachment; 2941 } 2942 2943 /** 2944 * @return {@link #content} (Computable Contract conveyed using a policy rule 2945 * language (e.g. XACML, DKAL, SecPal).) 2946 */ 2947 public Reference getContentReference() throws FHIRException { 2948 if (!(this.content instanceof Reference)) 2949 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2950 + this.content.getClass().getName() + " was encountered"); 2951 return (Reference) this.content; 2952 } 2953 2954 public boolean hasContentReference() { 2955 return this.content instanceof Reference; 2956 } 2957 2958 public boolean hasContent() { 2959 return this.content != null && !this.content.isEmpty(); 2960 } 2961 2962 /** 2963 * @param value {@link #content} (Computable Contract conveyed using a policy 2964 * rule language (e.g. XACML, DKAL, SecPal).) 2965 */ 2966 public ComputableLanguageComponent setContent(Type value) { 2967 this.content = value; 2968 return this; 2969 } 2970 2971 protected void listChildren(List<Property> childrenList) { 2972 super.listChildren(childrenList); 2973 childrenList.add(new Property("content[x]", "Attachment|Reference(DocumentReference)", 2974 "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 2975 java.lang.Integer.MAX_VALUE, content)); 2976 } 2977 2978 @Override 2979 public void setProperty(String name, Base value) throws FHIRException { 2980 if (name.equals("content[x]")) 2981 this.content = (Type) value; // Type 2982 else 2983 super.setProperty(name, value); 2984 } 2985 2986 @Override 2987 public Base addChild(String name) throws FHIRException { 2988 if (name.equals("contentAttachment")) { 2989 this.content = new Attachment(); 2990 return this.content; 2991 } else if (name.equals("contentReference")) { 2992 this.content = new Reference(); 2993 return this.content; 2994 } else 2995 return super.addChild(name); 2996 } 2997 2998 public ComputableLanguageComponent copy() { 2999 ComputableLanguageComponent dst = new ComputableLanguageComponent(); 3000 copyValues(dst); 3001 dst.content = content == null ? null : content.copy(); 3002 return dst; 3003 } 3004 3005 @Override 3006 public boolean equalsDeep(Base other) { 3007 if (!super.equalsDeep(other)) 3008 return false; 3009 if (!(other instanceof ComputableLanguageComponent)) 3010 return false; 3011 ComputableLanguageComponent o = (ComputableLanguageComponent) other; 3012 return compareDeep(content, o.content, true); 3013 } 3014 3015 @Override 3016 public boolean equalsShallow(Base other) { 3017 if (!super.equalsShallow(other)) 3018 return false; 3019 if (!(other instanceof ComputableLanguageComponent)) 3020 return false; 3021 ComputableLanguageComponent o = (ComputableLanguageComponent) other; 3022 return true; 3023 } 3024 3025 public boolean isEmpty() { 3026 return super.isEmpty() && (content == null || content.isEmpty()); 3027 } 3028 3029 public String fhirType() { 3030 return "Contract.rule"; 3031 3032 } 3033 3034 } 3035 3036 /** 3037 * Unique identifier for this Contract. 3038 */ 3039 @Child(name = "identifier", type = { 3040 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 3041 @Description(shortDefinition = "Contract identifier", formalDefinition = "Unique identifier for this Contract.") 3042 protected Identifier identifier; 3043 3044 /** 3045 * When this Contract was issued. 3046 */ 3047 @Child(name = "issued", type = { DateTimeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 3048 @Description(shortDefinition = "When this Contract was issued", formalDefinition = "When this Contract was issued.") 3049 protected DateTimeType issued; 3050 3051 /** 3052 * Relevant time or time-period when this Contract is applicable. 3053 */ 3054 @Child(name = "applies", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 3055 @Description(shortDefinition = "Effective time", formalDefinition = "Relevant time or time-period when this Contract is applicable.") 3056 protected Period applies; 3057 3058 /** 3059 * Who and/or what this Contract is about: typically a Patient, Organization, or 3060 * valued items such as goods and services. 3061 */ 3062 @Child(name = "subject", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3063 @Description(shortDefinition = "Subject of this Contract", formalDefinition = "Who and/or what this Contract is about: typically a Patient, Organization, or valued items such as goods and services.") 3064 protected List<Reference> subject; 3065 /** 3066 * The actual objects that are the target of the reference (Who and/or what this 3067 * Contract is about: typically a Patient, Organization, or valued items such as 3068 * goods and services.) 3069 */ 3070 protected List<Resource> subjectTarget; 3071 3072 /** 3073 * A formally or informally recognized grouping of people, principals, 3074 * organizations, or jurisdictions formed for the purpose of achieving some form 3075 * of collective action such as the promulgation, administration and enforcement 3076 * of contracts and policies. 3077 */ 3078 @Child(name = "authority", type = { 3079 Organization.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3080 @Description(shortDefinition = "Authority under which this Contract has standing", formalDefinition = "A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies.") 3081 protected List<Reference> authority; 3082 /** 3083 * The actual objects that are the target of the reference (A formally or 3084 * informally recognized grouping of people, principals, organizations, or 3085 * jurisdictions formed for the purpose of achieving some form of collective 3086 * action such as the promulgation, administration and enforcement of contracts 3087 * and policies.) 3088 */ 3089 protected List<Organization> authorityTarget; 3090 3091 /** 3092 * Recognized governance framework or system operating with a circumscribed 3093 * scope in accordance with specified principles, policies, processes or 3094 * procedures for managing rights, actions, or behaviors of parties or 3095 * principals relative to resources. 3096 */ 3097 @Child(name = "domain", type = { 3098 Location.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3099 @Description(shortDefinition = "Domain in which this Contract applies", formalDefinition = "Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources.") 3100 protected List<Reference> domain; 3101 /** 3102 * The actual objects that are the target of the reference (Recognized 3103 * governance framework or system operating with a circumscribed scope in 3104 * accordance with specified principles, policies, processes or procedures for 3105 * managing rights, actions, or behaviors of parties or principals relative to 3106 * resources.) 3107 */ 3108 protected List<Location> domainTarget; 3109 3110 /** 3111 * Type of Contract such as an insurance policy, real estate contract, a will, 3112 * power of attorny, Privacy or Security policy , trust framework agreement, 3113 * etc. 3114 */ 3115 @Child(name = "type", type = { CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 3116 @Description(shortDefinition = "Contract Tyoe", formalDefinition = "Type of Contract such as an insurance policy, real estate contract, a will, power of attorny, Privacy or Security policy , trust framework agreement, etc.") 3117 protected CodeableConcept type; 3118 3119 /** 3120 * More specific type or specialization of an overarching or more general 3121 * contract such as auto insurance, home owner insurance, prenupial agreement, 3122 * Advanced-Directive, or privacy consent. 3123 */ 3124 @Child(name = "subType", type = { 3125 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3126 @Description(shortDefinition = "Contract Subtype", formalDefinition = "More specific type or specialization of an overarching or more general contract such as auto insurance, home owner insurance, prenupial agreement, Advanced-Directive, or privacy consent.") 3127 protected List<CodeableConcept> subType; 3128 3129 /** 3130 * Action stipulated by this Contract. 3131 */ 3132 @Child(name = "action", type = { 3133 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3134 @Description(shortDefinition = "Contract Action", formalDefinition = "Action stipulated by this Contract.") 3135 protected List<CodeableConcept> action; 3136 3137 /** 3138 * Reason for action stipulated by this Contract. 3139 */ 3140 @Child(name = "actionReason", type = { 3141 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3142 @Description(shortDefinition = "Contract Action Reason", formalDefinition = "Reason for action stipulated by this Contract.") 3143 protected List<CodeableConcept> actionReason; 3144 3145 /** 3146 * List of Contract actors. 3147 */ 3148 @Child(name = "actor", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3149 @Description(shortDefinition = "Contract Actor", formalDefinition = "List of Contract actors.") 3150 protected List<ActorComponent> actor; 3151 3152 /** 3153 * Contract Valued Item List. 3154 */ 3155 @Child(name = "valuedItem", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3156 @Description(shortDefinition = "Contract Valued Item", formalDefinition = "Contract Valued Item List.") 3157 protected List<ValuedItemComponent> valuedItem; 3158 3159 /** 3160 * Party signing this Contract. 3161 */ 3162 @Child(name = "signer", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3163 @Description(shortDefinition = "Contract Signer", formalDefinition = "Party signing this Contract.") 3164 protected List<SignatoryComponent> signer; 3165 3166 /** 3167 * One or more Contract Provisions, which may be related and conveyed as a 3168 * group, and may contain nested groups. 3169 */ 3170 @Child(name = "term", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3171 @Description(shortDefinition = "Contract Term List", formalDefinition = "One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups.") 3172 protected List<TermComponent> term; 3173 3174 /** 3175 * Legally binding Contract: This is the signed and legally recognized 3176 * representation of the Contract, which is considered the "source of truth" and 3177 * which would be the basis for legal action related to enforcement of this 3178 * Contract. 3179 */ 3180 @Child(name = "binding", type = { Attachment.class, Composition.class, DocumentReference.class, 3181 QuestionnaireResponse.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 3182 @Description(shortDefinition = "Binding Contract", formalDefinition = "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.") 3183 protected Type binding; 3184 3185 /** 3186 * The "patient friendly language" versionof the Contract in whole or in parts. 3187 * "Patient friendly language" means the representation of the Contract and 3188 * Contract Provisions in a manner that is readily accessible and understandable 3189 * by a layperson in accordance with best practices for communication styles 3190 * that ensure that those agreeing to or signing the Contract understand the 3191 * roles, actions, obligations, responsibilities, and implication of the 3192 * agreement. 3193 */ 3194 @Child(name = "friendly", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3195 @Description(shortDefinition = "Contract Friendly Language", formalDefinition = "The \"patient friendly language\" versionof the Contract in whole or in parts. \"Patient friendly language\" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement.") 3196 protected List<FriendlyLanguageComponent> friendly; 3197 3198 /** 3199 * List of Legal expressions or representations of this Contract. 3200 */ 3201 @Child(name = "legal", type = {}, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3202 @Description(shortDefinition = "Contract Legal Language", formalDefinition = "List of Legal expressions or representations of this Contract.") 3203 protected List<LegalLanguageComponent> legal; 3204 3205 /** 3206 * List of Computable Policy Rule Language Representations of this Contract. 3207 */ 3208 @Child(name = "rule", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3209 @Description(shortDefinition = "Computable Contract Language", formalDefinition = "List of Computable Policy Rule Language Representations of this Contract.") 3210 protected List<ComputableLanguageComponent> rule; 3211 3212 private static final long serialVersionUID = -1785608373L; 3213 3214 /* 3215 * Constructor 3216 */ 3217 public Contract() { 3218 super(); 3219 } 3220 3221 /** 3222 * @return {@link #identifier} (Unique identifier for this Contract.) 3223 */ 3224 public Identifier getIdentifier() { 3225 if (this.identifier == null) 3226 if (Configuration.errorOnAutoCreate()) 3227 throw new Error("Attempt to auto-create Contract.identifier"); 3228 else if (Configuration.doAutoCreate()) 3229 this.identifier = new Identifier(); // cc 3230 return this.identifier; 3231 } 3232 3233 public boolean hasIdentifier() { 3234 return this.identifier != null && !this.identifier.isEmpty(); 3235 } 3236 3237 /** 3238 * @param value {@link #identifier} (Unique identifier for this Contract.) 3239 */ 3240 public Contract setIdentifier(Identifier value) { 3241 this.identifier = value; 3242 return this; 3243 } 3244 3245 /** 3246 * @return {@link #issued} (When this Contract was issued.). This is the 3247 * underlying object with id, value and extensions. The accessor 3248 * "getIssued" gives direct access to the value 3249 */ 3250 public DateTimeType getIssuedElement() { 3251 if (this.issued == null) 3252 if (Configuration.errorOnAutoCreate()) 3253 throw new Error("Attempt to auto-create Contract.issued"); 3254 else if (Configuration.doAutoCreate()) 3255 this.issued = new DateTimeType(); // bb 3256 return this.issued; 3257 } 3258 3259 public boolean hasIssuedElement() { 3260 return this.issued != null && !this.issued.isEmpty(); 3261 } 3262 3263 public boolean hasIssued() { 3264 return this.issued != null && !this.issued.isEmpty(); 3265 } 3266 3267 /** 3268 * @param value {@link #issued} (When this Contract was issued.). This is the 3269 * underlying object with id, value and extensions. The accessor 3270 * "getIssued" gives direct access to the value 3271 */ 3272 public Contract setIssuedElement(DateTimeType value) { 3273 this.issued = value; 3274 return this; 3275 } 3276 3277 /** 3278 * @return When this Contract was issued. 3279 */ 3280 public Date getIssued() { 3281 return this.issued == null ? null : this.issued.getValue(); 3282 } 3283 3284 /** 3285 * @param value When this Contract was issued. 3286 */ 3287 public Contract setIssued(Date value) { 3288 if (value == null) 3289 this.issued = null; 3290 else { 3291 if (this.issued == null) 3292 this.issued = new DateTimeType(); 3293 this.issued.setValue(value); 3294 } 3295 return this; 3296 } 3297 3298 /** 3299 * @return {@link #applies} (Relevant time or time-period when this Contract is 3300 * applicable.) 3301 */ 3302 public Period getApplies() { 3303 if (this.applies == null) 3304 if (Configuration.errorOnAutoCreate()) 3305 throw new Error("Attempt to auto-create Contract.applies"); 3306 else if (Configuration.doAutoCreate()) 3307 this.applies = new Period(); // cc 3308 return this.applies; 3309 } 3310 3311 public boolean hasApplies() { 3312 return this.applies != null && !this.applies.isEmpty(); 3313 } 3314 3315 /** 3316 * @param value {@link #applies} (Relevant time or time-period when this 3317 * Contract is applicable.) 3318 */ 3319 public Contract setApplies(Period value) { 3320 this.applies = value; 3321 return this; 3322 } 3323 3324 /** 3325 * @return {@link #subject} (Who and/or what this Contract is about: typically a 3326 * Patient, Organization, or valued items such as goods and services.) 3327 */ 3328 public List<Reference> getSubject() { 3329 if (this.subject == null) 3330 this.subject = new ArrayList<Reference>(); 3331 return this.subject; 3332 } 3333 3334 public boolean hasSubject() { 3335 if (this.subject == null) 3336 return false; 3337 for (Reference item : this.subject) 3338 if (!item.isEmpty()) 3339 return true; 3340 return false; 3341 } 3342 3343 /** 3344 * @return {@link #subject} (Who and/or what this Contract is about: typically a 3345 * Patient, Organization, or valued items such as goods and services.) 3346 */ 3347 // syntactic sugar 3348 public Reference addSubject() { // 3 3349 Reference t = new Reference(); 3350 if (this.subject == null) 3351 this.subject = new ArrayList<Reference>(); 3352 this.subject.add(t); 3353 return t; 3354 } 3355 3356 // syntactic sugar 3357 public Contract addSubject(Reference t) { // 3 3358 if (t == null) 3359 return this; 3360 if (this.subject == null) 3361 this.subject = new ArrayList<Reference>(); 3362 this.subject.add(t); 3363 return this; 3364 } 3365 3366 /** 3367 * @return {@link #subject} (The actual objects that are the target of the 3368 * reference. The reference library doesn't populate this, but you can 3369 * use this to hold the resources if you resolvethemt. Who and/or what 3370 * this Contract is about: typically a Patient, Organization, or valued 3371 * items such as goods and services.) 3372 */ 3373 public List<Resource> getSubjectTarget() { 3374 if (this.subjectTarget == null) 3375 this.subjectTarget = new ArrayList<Resource>(); 3376 return this.subjectTarget; 3377 } 3378 3379 /** 3380 * @return {@link #authority} (A formally or informally recognized grouping of 3381 * people, principals, organizations, or jurisdictions formed for the 3382 * purpose of achieving some form of collective action such as the 3383 * promulgation, administration and enforcement of contracts and 3384 * policies.) 3385 */ 3386 public List<Reference> getAuthority() { 3387 if (this.authority == null) 3388 this.authority = new ArrayList<Reference>(); 3389 return this.authority; 3390 } 3391 3392 public boolean hasAuthority() { 3393 if (this.authority == null) 3394 return false; 3395 for (Reference item : this.authority) 3396 if (!item.isEmpty()) 3397 return true; 3398 return false; 3399 } 3400 3401 /** 3402 * @return {@link #authority} (A formally or informally recognized grouping of 3403 * people, principals, organizations, or jurisdictions formed for the 3404 * purpose of achieving some form of collective action such as the 3405 * promulgation, administration and enforcement of contracts and 3406 * policies.) 3407 */ 3408 // syntactic sugar 3409 public Reference addAuthority() { // 3 3410 Reference t = new Reference(); 3411 if (this.authority == null) 3412 this.authority = new ArrayList<Reference>(); 3413 this.authority.add(t); 3414 return t; 3415 } 3416 3417 // syntactic sugar 3418 public Contract addAuthority(Reference t) { // 3 3419 if (t == null) 3420 return this; 3421 if (this.authority == null) 3422 this.authority = new ArrayList<Reference>(); 3423 this.authority.add(t); 3424 return this; 3425 } 3426 3427 /** 3428 * @return {@link #authority} (The actual objects that are the target of the 3429 * reference. The reference library doesn't populate this, but you can 3430 * use this to hold the resources if you resolvethemt. A formally or 3431 * informally recognized grouping of people, principals, organizations, 3432 * or jurisdictions formed for the purpose of achieving some form of 3433 * collective action such as the promulgation, administration and 3434 * enforcement of contracts and policies.) 3435 */ 3436 public List<Organization> getAuthorityTarget() { 3437 if (this.authorityTarget == null) 3438 this.authorityTarget = new ArrayList<Organization>(); 3439 return this.authorityTarget; 3440 } 3441 3442 // syntactic sugar 3443 /** 3444 * @return {@link #authority} (Add an actual object that is the target of the 3445 * reference. The reference library doesn't use these, but you can use 3446 * this to hold the resources if you resolvethemt. A formally or 3447 * informally recognized grouping of people, principals, organizations, 3448 * or jurisdictions formed for the purpose of achieving some form of 3449 * collective action such as the promulgation, administration and 3450 * enforcement of contracts and policies.) 3451 */ 3452 public Organization addAuthorityTarget() { 3453 Organization r = new Organization(); 3454 if (this.authorityTarget == null) 3455 this.authorityTarget = new ArrayList<Organization>(); 3456 this.authorityTarget.add(r); 3457 return r; 3458 } 3459 3460 /** 3461 * @return {@link #domain} (Recognized governance framework or system operating 3462 * with a circumscribed scope in accordance with specified principles, 3463 * policies, processes or procedures for managing rights, actions, or 3464 * behaviors of parties or principals relative to resources.) 3465 */ 3466 public List<Reference> getDomain() { 3467 if (this.domain == null) 3468 this.domain = new ArrayList<Reference>(); 3469 return this.domain; 3470 } 3471 3472 public boolean hasDomain() { 3473 if (this.domain == null) 3474 return false; 3475 for (Reference item : this.domain) 3476 if (!item.isEmpty()) 3477 return true; 3478 return false; 3479 } 3480 3481 /** 3482 * @return {@link #domain} (Recognized governance framework or system operating 3483 * with a circumscribed scope in accordance with specified principles, 3484 * policies, processes or procedures for managing rights, actions, or 3485 * behaviors of parties or principals relative to resources.) 3486 */ 3487 // syntactic sugar 3488 public Reference addDomain() { // 3 3489 Reference t = new Reference(); 3490 if (this.domain == null) 3491 this.domain = new ArrayList<Reference>(); 3492 this.domain.add(t); 3493 return t; 3494 } 3495 3496 // syntactic sugar 3497 public Contract addDomain(Reference t) { // 3 3498 if (t == null) 3499 return this; 3500 if (this.domain == null) 3501 this.domain = new ArrayList<Reference>(); 3502 this.domain.add(t); 3503 return this; 3504 } 3505 3506 /** 3507 * @return {@link #domain} (The actual objects that are the target of the 3508 * reference. The reference library doesn't populate this, but you can 3509 * use this to hold the resources if you resolvethemt. Recognized 3510 * governance framework or system operating with a circumscribed scope 3511 * in accordance with specified principles, policies, processes or 3512 * procedures for managing rights, actions, or behaviors of parties or 3513 * principals relative to resources.) 3514 */ 3515 public List<Location> getDomainTarget() { 3516 if (this.domainTarget == null) 3517 this.domainTarget = new ArrayList<Location>(); 3518 return this.domainTarget; 3519 } 3520 3521 // syntactic sugar 3522 /** 3523 * @return {@link #domain} (Add an actual object that is the target of the 3524 * reference. The reference library doesn't use these, but you can use 3525 * this to hold the resources if you resolvethemt. Recognized governance 3526 * framework or system operating with a circumscribed scope in 3527 * accordance with specified principles, policies, processes or 3528 * procedures for managing rights, actions, or behaviors of parties or 3529 * principals relative to resources.) 3530 */ 3531 public Location addDomainTarget() { 3532 Location r = new Location(); 3533 if (this.domainTarget == null) 3534 this.domainTarget = new ArrayList<Location>(); 3535 this.domainTarget.add(r); 3536 return r; 3537 } 3538 3539 /** 3540 * @return {@link #type} (Type of Contract such as an insurance policy, real 3541 * estate contract, a will, power of attorny, Privacy or Security policy 3542 * , trust framework agreement, etc.) 3543 */ 3544 public CodeableConcept getType() { 3545 if (this.type == null) 3546 if (Configuration.errorOnAutoCreate()) 3547 throw new Error("Attempt to auto-create Contract.type"); 3548 else if (Configuration.doAutoCreate()) 3549 this.type = new CodeableConcept(); // cc 3550 return this.type; 3551 } 3552 3553 public boolean hasType() { 3554 return this.type != null && !this.type.isEmpty(); 3555 } 3556 3557 /** 3558 * @param value {@link #type} (Type of Contract such as an insurance policy, 3559 * real estate contract, a will, power of attorny, Privacy or 3560 * Security policy , trust framework agreement, etc.) 3561 */ 3562 public Contract setType(CodeableConcept value) { 3563 this.type = value; 3564 return this; 3565 } 3566 3567 /** 3568 * @return {@link #subType} (More specific type or specialization of an 3569 * overarching or more general contract such as auto insurance, home 3570 * owner insurance, prenupial agreement, Advanced-Directive, or privacy 3571 * consent.) 3572 */ 3573 public List<CodeableConcept> getSubType() { 3574 if (this.subType == null) 3575 this.subType = new ArrayList<CodeableConcept>(); 3576 return this.subType; 3577 } 3578 3579 public boolean hasSubType() { 3580 if (this.subType == null) 3581 return false; 3582 for (CodeableConcept item : this.subType) 3583 if (!item.isEmpty()) 3584 return true; 3585 return false; 3586 } 3587 3588 /** 3589 * @return {@link #subType} (More specific type or specialization of an 3590 * overarching or more general contract such as auto insurance, home 3591 * owner insurance, prenupial agreement, Advanced-Directive, or privacy 3592 * consent.) 3593 */ 3594 // syntactic sugar 3595 public CodeableConcept addSubType() { // 3 3596 CodeableConcept t = new CodeableConcept(); 3597 if (this.subType == null) 3598 this.subType = new ArrayList<CodeableConcept>(); 3599 this.subType.add(t); 3600 return t; 3601 } 3602 3603 // syntactic sugar 3604 public Contract addSubType(CodeableConcept t) { // 3 3605 if (t == null) 3606 return this; 3607 if (this.subType == null) 3608 this.subType = new ArrayList<CodeableConcept>(); 3609 this.subType.add(t); 3610 return this; 3611 } 3612 3613 /** 3614 * @return {@link #action} (Action stipulated by this Contract.) 3615 */ 3616 public List<CodeableConcept> getAction() { 3617 if (this.action == null) 3618 this.action = new ArrayList<CodeableConcept>(); 3619 return this.action; 3620 } 3621 3622 public boolean hasAction() { 3623 if (this.action == null) 3624 return false; 3625 for (CodeableConcept item : this.action) 3626 if (!item.isEmpty()) 3627 return true; 3628 return false; 3629 } 3630 3631 /** 3632 * @return {@link #action} (Action stipulated by this Contract.) 3633 */ 3634 // syntactic sugar 3635 public CodeableConcept addAction() { // 3 3636 CodeableConcept t = new CodeableConcept(); 3637 if (this.action == null) 3638 this.action = new ArrayList<CodeableConcept>(); 3639 this.action.add(t); 3640 return t; 3641 } 3642 3643 // syntactic sugar 3644 public Contract addAction(CodeableConcept t) { // 3 3645 if (t == null) 3646 return this; 3647 if (this.action == null) 3648 this.action = new ArrayList<CodeableConcept>(); 3649 this.action.add(t); 3650 return this; 3651 } 3652 3653 /** 3654 * @return {@link #actionReason} (Reason for action stipulated by this 3655 * Contract.) 3656 */ 3657 public List<CodeableConcept> getActionReason() { 3658 if (this.actionReason == null) 3659 this.actionReason = new ArrayList<CodeableConcept>(); 3660 return this.actionReason; 3661 } 3662 3663 public boolean hasActionReason() { 3664 if (this.actionReason == null) 3665 return false; 3666 for (CodeableConcept item : this.actionReason) 3667 if (!item.isEmpty()) 3668 return true; 3669 return false; 3670 } 3671 3672 /** 3673 * @return {@link #actionReason} (Reason for action stipulated by this 3674 * Contract.) 3675 */ 3676 // syntactic sugar 3677 public CodeableConcept addActionReason() { // 3 3678 CodeableConcept t = new CodeableConcept(); 3679 if (this.actionReason == null) 3680 this.actionReason = new ArrayList<CodeableConcept>(); 3681 this.actionReason.add(t); 3682 return t; 3683 } 3684 3685 // syntactic sugar 3686 public Contract addActionReason(CodeableConcept t) { // 3 3687 if (t == null) 3688 return this; 3689 if (this.actionReason == null) 3690 this.actionReason = new ArrayList<CodeableConcept>(); 3691 this.actionReason.add(t); 3692 return this; 3693 } 3694 3695 /** 3696 * @return {@link #actor} (List of Contract actors.) 3697 */ 3698 public List<ActorComponent> getActor() { 3699 if (this.actor == null) 3700 this.actor = new ArrayList<ActorComponent>(); 3701 return this.actor; 3702 } 3703 3704 public boolean hasActor() { 3705 if (this.actor == null) 3706 return false; 3707 for (ActorComponent item : this.actor) 3708 if (!item.isEmpty()) 3709 return true; 3710 return false; 3711 } 3712 3713 /** 3714 * @return {@link #actor} (List of Contract actors.) 3715 */ 3716 // syntactic sugar 3717 public ActorComponent addActor() { // 3 3718 ActorComponent t = new ActorComponent(); 3719 if (this.actor == null) 3720 this.actor = new ArrayList<ActorComponent>(); 3721 this.actor.add(t); 3722 return t; 3723 } 3724 3725 // syntactic sugar 3726 public Contract addActor(ActorComponent t) { // 3 3727 if (t == null) 3728 return this; 3729 if (this.actor == null) 3730 this.actor = new ArrayList<ActorComponent>(); 3731 this.actor.add(t); 3732 return this; 3733 } 3734 3735 /** 3736 * @return {@link #valuedItem} (Contract Valued Item List.) 3737 */ 3738 public List<ValuedItemComponent> getValuedItem() { 3739 if (this.valuedItem == null) 3740 this.valuedItem = new ArrayList<ValuedItemComponent>(); 3741 return this.valuedItem; 3742 } 3743 3744 public boolean hasValuedItem() { 3745 if (this.valuedItem == null) 3746 return false; 3747 for (ValuedItemComponent item : this.valuedItem) 3748 if (!item.isEmpty()) 3749 return true; 3750 return false; 3751 } 3752 3753 /** 3754 * @return {@link #valuedItem} (Contract Valued Item List.) 3755 */ 3756 // syntactic sugar 3757 public ValuedItemComponent addValuedItem() { // 3 3758 ValuedItemComponent t = new ValuedItemComponent(); 3759 if (this.valuedItem == null) 3760 this.valuedItem = new ArrayList<ValuedItemComponent>(); 3761 this.valuedItem.add(t); 3762 return t; 3763 } 3764 3765 // syntactic sugar 3766 public Contract addValuedItem(ValuedItemComponent t) { // 3 3767 if (t == null) 3768 return this; 3769 if (this.valuedItem == null) 3770 this.valuedItem = new ArrayList<ValuedItemComponent>(); 3771 this.valuedItem.add(t); 3772 return this; 3773 } 3774 3775 /** 3776 * @return {@link #signer} (Party signing this Contract.) 3777 */ 3778 public List<SignatoryComponent> getSigner() { 3779 if (this.signer == null) 3780 this.signer = new ArrayList<SignatoryComponent>(); 3781 return this.signer; 3782 } 3783 3784 public boolean hasSigner() { 3785 if (this.signer == null) 3786 return false; 3787 for (SignatoryComponent item : this.signer) 3788 if (!item.isEmpty()) 3789 return true; 3790 return false; 3791 } 3792 3793 /** 3794 * @return {@link #signer} (Party signing this Contract.) 3795 */ 3796 // syntactic sugar 3797 public SignatoryComponent addSigner() { // 3 3798 SignatoryComponent t = new SignatoryComponent(); 3799 if (this.signer == null) 3800 this.signer = new ArrayList<SignatoryComponent>(); 3801 this.signer.add(t); 3802 return t; 3803 } 3804 3805 // syntactic sugar 3806 public Contract addSigner(SignatoryComponent t) { // 3 3807 if (t == null) 3808 return this; 3809 if (this.signer == null) 3810 this.signer = new ArrayList<SignatoryComponent>(); 3811 this.signer.add(t); 3812 return this; 3813 } 3814 3815 /** 3816 * @return {@link #term} (One or more Contract Provisions, which may be related 3817 * and conveyed as a group, and may contain nested groups.) 3818 */ 3819 public List<TermComponent> getTerm() { 3820 if (this.term == null) 3821 this.term = new ArrayList<TermComponent>(); 3822 return this.term; 3823 } 3824 3825 public boolean hasTerm() { 3826 if (this.term == null) 3827 return false; 3828 for (TermComponent item : this.term) 3829 if (!item.isEmpty()) 3830 return true; 3831 return false; 3832 } 3833 3834 /** 3835 * @return {@link #term} (One or more Contract Provisions, which may be related 3836 * and conveyed as a group, and may contain nested groups.) 3837 */ 3838 // syntactic sugar 3839 public TermComponent addTerm() { // 3 3840 TermComponent t = new TermComponent(); 3841 if (this.term == null) 3842 this.term = new ArrayList<TermComponent>(); 3843 this.term.add(t); 3844 return t; 3845 } 3846 3847 // syntactic sugar 3848 public Contract addTerm(TermComponent t) { // 3 3849 if (t == null) 3850 return this; 3851 if (this.term == null) 3852 this.term = new ArrayList<TermComponent>(); 3853 this.term.add(t); 3854 return this; 3855 } 3856 3857 /** 3858 * @return {@link #binding} (Legally binding Contract: This is the signed and 3859 * legally recognized representation of the Contract, which is 3860 * considered the "source of truth" and which would be the basis for 3861 * legal action related to enforcement of this Contract.) 3862 */ 3863 public Type getBinding() { 3864 return this.binding; 3865 } 3866 3867 /** 3868 * @return {@link #binding} (Legally binding Contract: This is the signed and 3869 * legally recognized representation of the Contract, which is 3870 * considered the "source of truth" and which would be the basis for 3871 * legal action related to enforcement of this Contract.) 3872 */ 3873 public Attachment getBindingAttachment() throws FHIRException { 3874 if (!(this.binding instanceof Attachment)) 3875 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 3876 + this.binding.getClass().getName() + " was encountered"); 3877 return (Attachment) this.binding; 3878 } 3879 3880 public boolean hasBindingAttachment() { 3881 return this.binding instanceof Attachment; 3882 } 3883 3884 /** 3885 * @return {@link #binding} (Legally binding Contract: This is the signed and 3886 * legally recognized representation of the Contract, which is 3887 * considered the "source of truth" and which would be the basis for 3888 * legal action related to enforcement of this Contract.) 3889 */ 3890 public Reference getBindingReference() throws FHIRException { 3891 if (!(this.binding instanceof Reference)) 3892 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.binding.getClass().getName() 3893 + " was encountered"); 3894 return (Reference) this.binding; 3895 } 3896 3897 public boolean hasBindingReference() { 3898 return this.binding instanceof Reference; 3899 } 3900 3901 public boolean hasBinding() { 3902 return this.binding != null && !this.binding.isEmpty(); 3903 } 3904 3905 /** 3906 * @param value {@link #binding} (Legally binding Contract: This is the signed 3907 * and legally recognized representation of the Contract, which is 3908 * considered the "source of truth" and which would be the basis 3909 * for legal action related to enforcement of this Contract.) 3910 */ 3911 public Contract setBinding(Type value) { 3912 this.binding = value; 3913 return this; 3914 } 3915 3916 /** 3917 * @return {@link #friendly} (The "patient friendly language" versionof the 3918 * Contract in whole or in parts. "Patient friendly language" means the 3919 * representation of the Contract and Contract Provisions in a manner 3920 * that is readily accessible and understandable by a layperson in 3921 * accordance with best practices for communication styles that ensure 3922 * that those agreeing to or signing the Contract understand the roles, 3923 * actions, obligations, responsibilities, and implication of the 3924 * agreement.) 3925 */ 3926 public List<FriendlyLanguageComponent> getFriendly() { 3927 if (this.friendly == null) 3928 this.friendly = new ArrayList<FriendlyLanguageComponent>(); 3929 return this.friendly; 3930 } 3931 3932 public boolean hasFriendly() { 3933 if (this.friendly == null) 3934 return false; 3935 for (FriendlyLanguageComponent item : this.friendly) 3936 if (!item.isEmpty()) 3937 return true; 3938 return false; 3939 } 3940 3941 /** 3942 * @return {@link #friendly} (The "patient friendly language" versionof the 3943 * Contract in whole or in parts. "Patient friendly language" means the 3944 * representation of the Contract and Contract Provisions in a manner 3945 * that is readily accessible and understandable by a layperson in 3946 * accordance with best practices for communication styles that ensure 3947 * that those agreeing to or signing the Contract understand the roles, 3948 * actions, obligations, responsibilities, and implication of the 3949 * agreement.) 3950 */ 3951 // syntactic sugar 3952 public FriendlyLanguageComponent addFriendly() { // 3 3953 FriendlyLanguageComponent t = new FriendlyLanguageComponent(); 3954 if (this.friendly == null) 3955 this.friendly = new ArrayList<FriendlyLanguageComponent>(); 3956 this.friendly.add(t); 3957 return t; 3958 } 3959 3960 // syntactic sugar 3961 public Contract addFriendly(FriendlyLanguageComponent t) { // 3 3962 if (t == null) 3963 return this; 3964 if (this.friendly == null) 3965 this.friendly = new ArrayList<FriendlyLanguageComponent>(); 3966 this.friendly.add(t); 3967 return this; 3968 } 3969 3970 /** 3971 * @return {@link #legal} (List of Legal expressions or representations of this 3972 * Contract.) 3973 */ 3974 public List<LegalLanguageComponent> getLegal() { 3975 if (this.legal == null) 3976 this.legal = new ArrayList<LegalLanguageComponent>(); 3977 return this.legal; 3978 } 3979 3980 public boolean hasLegal() { 3981 if (this.legal == null) 3982 return false; 3983 for (LegalLanguageComponent item : this.legal) 3984 if (!item.isEmpty()) 3985 return true; 3986 return false; 3987 } 3988 3989 /** 3990 * @return {@link #legal} (List of Legal expressions or representations of this 3991 * Contract.) 3992 */ 3993 // syntactic sugar 3994 public LegalLanguageComponent addLegal() { // 3 3995 LegalLanguageComponent t = new LegalLanguageComponent(); 3996 if (this.legal == null) 3997 this.legal = new ArrayList<LegalLanguageComponent>(); 3998 this.legal.add(t); 3999 return t; 4000 } 4001 4002 // syntactic sugar 4003 public Contract addLegal(LegalLanguageComponent t) { // 3 4004 if (t == null) 4005 return this; 4006 if (this.legal == null) 4007 this.legal = new ArrayList<LegalLanguageComponent>(); 4008 this.legal.add(t); 4009 return this; 4010 } 4011 4012 /** 4013 * @return {@link #rule} (List of Computable Policy Rule Language 4014 * Representations of this Contract.) 4015 */ 4016 public List<ComputableLanguageComponent> getRule() { 4017 if (this.rule == null) 4018 this.rule = new ArrayList<ComputableLanguageComponent>(); 4019 return this.rule; 4020 } 4021 4022 public boolean hasRule() { 4023 if (this.rule == null) 4024 return false; 4025 for (ComputableLanguageComponent item : this.rule) 4026 if (!item.isEmpty()) 4027 return true; 4028 return false; 4029 } 4030 4031 /** 4032 * @return {@link #rule} (List of Computable Policy Rule Language 4033 * Representations of this Contract.) 4034 */ 4035 // syntactic sugar 4036 public ComputableLanguageComponent addRule() { // 3 4037 ComputableLanguageComponent t = new ComputableLanguageComponent(); 4038 if (this.rule == null) 4039 this.rule = new ArrayList<ComputableLanguageComponent>(); 4040 this.rule.add(t); 4041 return t; 4042 } 4043 4044 // syntactic sugar 4045 public Contract addRule(ComputableLanguageComponent t) { // 3 4046 if (t == null) 4047 return this; 4048 if (this.rule == null) 4049 this.rule = new ArrayList<ComputableLanguageComponent>(); 4050 this.rule.add(t); 4051 return this; 4052 } 4053 4054 protected void listChildren(List<Property> childrenList) { 4055 super.listChildren(childrenList); 4056 childrenList.add(new Property("identifier", "Identifier", "Unique identifier for this Contract.", 0, 4057 java.lang.Integer.MAX_VALUE, identifier)); 4058 childrenList.add( 4059 new Property("issued", "dateTime", "When this Contract was issued.", 0, java.lang.Integer.MAX_VALUE, issued)); 4060 childrenList.add(new Property("applies", "Period", "Relevant time or time-period when this Contract is applicable.", 4061 0, java.lang.Integer.MAX_VALUE, applies)); 4062 childrenList.add(new Property("subject", "Reference(Any)", 4063 "Who and/or what this Contract is about: typically a Patient, Organization, or valued items such as goods and services.", 4064 0, java.lang.Integer.MAX_VALUE, subject)); 4065 childrenList.add(new Property("authority", "Reference(Organization)", 4066 "A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies.", 4067 0, java.lang.Integer.MAX_VALUE, authority)); 4068 childrenList.add(new Property("domain", "Reference(Location)", 4069 "Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources.", 4070 0, java.lang.Integer.MAX_VALUE, domain)); 4071 childrenList.add(new Property("type", "CodeableConcept", 4072 "Type of Contract such as an insurance policy, real estate contract, a will, power of attorny, Privacy or Security policy , trust framework agreement, etc.", 4073 0, java.lang.Integer.MAX_VALUE, type)); 4074 childrenList.add(new Property("subType", "CodeableConcept", 4075 "More specific type or specialization of an overarching or more general contract such as auto insurance, home owner insurance, prenupial agreement, Advanced-Directive, or privacy consent.", 4076 0, java.lang.Integer.MAX_VALUE, subType)); 4077 childrenList.add(new Property("action", "CodeableConcept", "Action stipulated by this Contract.", 0, 4078 java.lang.Integer.MAX_VALUE, action)); 4079 childrenList.add(new Property("actionReason", "CodeableConcept", "Reason for action stipulated by this Contract.", 4080 0, java.lang.Integer.MAX_VALUE, actionReason)); 4081 childrenList.add(new Property("actor", "", "List of Contract actors.", 0, java.lang.Integer.MAX_VALUE, actor)); 4082 childrenList 4083 .add(new Property("valuedItem", "", "Contract Valued Item List.", 0, java.lang.Integer.MAX_VALUE, valuedItem)); 4084 childrenList 4085 .add(new Property("signer", "", "Party signing this Contract.", 0, java.lang.Integer.MAX_VALUE, signer)); 4086 childrenList.add(new Property("term", "", 4087 "One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups.", 4088 0, java.lang.Integer.MAX_VALUE, term)); 4089 childrenList.add(new Property("binding[x]", 4090 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 4091 "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 4092 0, java.lang.Integer.MAX_VALUE, binding)); 4093 childrenList.add(new Property("friendly", "", 4094 "The \"patient friendly language\" versionof the Contract in whole or in parts. \"Patient friendly language\" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement.", 4095 0, java.lang.Integer.MAX_VALUE, friendly)); 4096 childrenList.add(new Property("legal", "", "List of Legal expressions or representations of this Contract.", 0, 4097 java.lang.Integer.MAX_VALUE, legal)); 4098 childrenList 4099 .add(new Property("rule", "", "List of Computable Policy Rule Language Representations of this Contract.", 0, 4100 java.lang.Integer.MAX_VALUE, rule)); 4101 } 4102 4103 @Override 4104 public void setProperty(String name, Base value) throws FHIRException { 4105 if (name.equals("identifier")) 4106 this.identifier = castToIdentifier(value); // Identifier 4107 else if (name.equals("issued")) 4108 this.issued = castToDateTime(value); // DateTimeType 4109 else if (name.equals("applies")) 4110 this.applies = castToPeriod(value); // Period 4111 else if (name.equals("subject")) 4112 this.getSubject().add(castToReference(value)); 4113 else if (name.equals("authority")) 4114 this.getAuthority().add(castToReference(value)); 4115 else if (name.equals("domain")) 4116 this.getDomain().add(castToReference(value)); 4117 else if (name.equals("type")) 4118 this.type = castToCodeableConcept(value); // CodeableConcept 4119 else if (name.equals("subType")) 4120 this.getSubType().add(castToCodeableConcept(value)); 4121 else if (name.equals("action")) 4122 this.getAction().add(castToCodeableConcept(value)); 4123 else if (name.equals("actionReason")) 4124 this.getActionReason().add(castToCodeableConcept(value)); 4125 else if (name.equals("actor")) 4126 this.getActor().add((ActorComponent) value); 4127 else if (name.equals("valuedItem")) 4128 this.getValuedItem().add((ValuedItemComponent) value); 4129 else if (name.equals("signer")) 4130 this.getSigner().add((SignatoryComponent) value); 4131 else if (name.equals("term")) 4132 this.getTerm().add((TermComponent) value); 4133 else if (name.equals("binding[x]")) 4134 this.binding = (Type) value; // Type 4135 else if (name.equals("friendly")) 4136 this.getFriendly().add((FriendlyLanguageComponent) value); 4137 else if (name.equals("legal")) 4138 this.getLegal().add((LegalLanguageComponent) value); 4139 else if (name.equals("rule")) 4140 this.getRule().add((ComputableLanguageComponent) value); 4141 else 4142 super.setProperty(name, value); 4143 } 4144 4145 @Override 4146 public Base addChild(String name) throws FHIRException { 4147 if (name.equals("identifier")) { 4148 this.identifier = new Identifier(); 4149 return this.identifier; 4150 } else if (name.equals("issued")) { 4151 throw new FHIRException("Cannot call addChild on a singleton property Contract.issued"); 4152 } else if (name.equals("applies")) { 4153 this.applies = new Period(); 4154 return this.applies; 4155 } else if (name.equals("subject")) { 4156 return addSubject(); 4157 } else if (name.equals("authority")) { 4158 return addAuthority(); 4159 } else if (name.equals("domain")) { 4160 return addDomain(); 4161 } else if (name.equals("type")) { 4162 this.type = new CodeableConcept(); 4163 return this.type; 4164 } else if (name.equals("subType")) { 4165 return addSubType(); 4166 } else if (name.equals("action")) { 4167 return addAction(); 4168 } else if (name.equals("actionReason")) { 4169 return addActionReason(); 4170 } else if (name.equals("actor")) { 4171 return addActor(); 4172 } else if (name.equals("valuedItem")) { 4173 return addValuedItem(); 4174 } else if (name.equals("signer")) { 4175 return addSigner(); 4176 } else if (name.equals("term")) { 4177 return addTerm(); 4178 } else if (name.equals("bindingAttachment")) { 4179 this.binding = new Attachment(); 4180 return this.binding; 4181 } else if (name.equals("bindingReference")) { 4182 this.binding = new Reference(); 4183 return this.binding; 4184 } else if (name.equals("friendly")) { 4185 return addFriendly(); 4186 } else if (name.equals("legal")) { 4187 return addLegal(); 4188 } else if (name.equals("rule")) { 4189 return addRule(); 4190 } else 4191 return super.addChild(name); 4192 } 4193 4194 public String fhirType() { 4195 return "Contract"; 4196 4197 } 4198 4199 public Contract copy() { 4200 Contract dst = new Contract(); 4201 copyValues(dst); 4202 dst.identifier = identifier == null ? null : identifier.copy(); 4203 dst.issued = issued == null ? null : issued.copy(); 4204 dst.applies = applies == null ? null : applies.copy(); 4205 if (subject != null) { 4206 dst.subject = new ArrayList<Reference>(); 4207 for (Reference i : subject) 4208 dst.subject.add(i.copy()); 4209 } 4210 ; 4211 if (authority != null) { 4212 dst.authority = new ArrayList<Reference>(); 4213 for (Reference i : authority) 4214 dst.authority.add(i.copy()); 4215 } 4216 ; 4217 if (domain != null) { 4218 dst.domain = new ArrayList<Reference>(); 4219 for (Reference i : domain) 4220 dst.domain.add(i.copy()); 4221 } 4222 ; 4223 dst.type = type == null ? null : type.copy(); 4224 if (subType != null) { 4225 dst.subType = new ArrayList<CodeableConcept>(); 4226 for (CodeableConcept i : subType) 4227 dst.subType.add(i.copy()); 4228 } 4229 ; 4230 if (action != null) { 4231 dst.action = new ArrayList<CodeableConcept>(); 4232 for (CodeableConcept i : action) 4233 dst.action.add(i.copy()); 4234 } 4235 ; 4236 if (actionReason != null) { 4237 dst.actionReason = new ArrayList<CodeableConcept>(); 4238 for (CodeableConcept i : actionReason) 4239 dst.actionReason.add(i.copy()); 4240 } 4241 ; 4242 if (actor != null) { 4243 dst.actor = new ArrayList<ActorComponent>(); 4244 for (ActorComponent i : actor) 4245 dst.actor.add(i.copy()); 4246 } 4247 ; 4248 if (valuedItem != null) { 4249 dst.valuedItem = new ArrayList<ValuedItemComponent>(); 4250 for (ValuedItemComponent i : valuedItem) 4251 dst.valuedItem.add(i.copy()); 4252 } 4253 ; 4254 if (signer != null) { 4255 dst.signer = new ArrayList<SignatoryComponent>(); 4256 for (SignatoryComponent i : signer) 4257 dst.signer.add(i.copy()); 4258 } 4259 ; 4260 if (term != null) { 4261 dst.term = new ArrayList<TermComponent>(); 4262 for (TermComponent i : term) 4263 dst.term.add(i.copy()); 4264 } 4265 ; 4266 dst.binding = binding == null ? null : binding.copy(); 4267 if (friendly != null) { 4268 dst.friendly = new ArrayList<FriendlyLanguageComponent>(); 4269 for (FriendlyLanguageComponent i : friendly) 4270 dst.friendly.add(i.copy()); 4271 } 4272 ; 4273 if (legal != null) { 4274 dst.legal = new ArrayList<LegalLanguageComponent>(); 4275 for (LegalLanguageComponent i : legal) 4276 dst.legal.add(i.copy()); 4277 } 4278 ; 4279 if (rule != null) { 4280 dst.rule = new ArrayList<ComputableLanguageComponent>(); 4281 for (ComputableLanguageComponent i : rule) 4282 dst.rule.add(i.copy()); 4283 } 4284 ; 4285 return dst; 4286 } 4287 4288 protected Contract typedCopy() { 4289 return copy(); 4290 } 4291 4292 @Override 4293 public boolean equalsDeep(Base other) { 4294 if (!super.equalsDeep(other)) 4295 return false; 4296 if (!(other instanceof Contract)) 4297 return false; 4298 Contract o = (Contract) other; 4299 return compareDeep(identifier, o.identifier, true) && compareDeep(issued, o.issued, true) 4300 && compareDeep(applies, o.applies, true) && compareDeep(subject, o.subject, true) 4301 && compareDeep(authority, o.authority, true) && compareDeep(domain, o.domain, true) 4302 && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) 4303 && compareDeep(action, o.action, true) && compareDeep(actionReason, o.actionReason, true) 4304 && compareDeep(actor, o.actor, true) && compareDeep(valuedItem, o.valuedItem, true) 4305 && compareDeep(signer, o.signer, true) && compareDeep(term, o.term, true) 4306 && compareDeep(binding, o.binding, true) && compareDeep(friendly, o.friendly, true) 4307 && compareDeep(legal, o.legal, true) && compareDeep(rule, o.rule, true); 4308 } 4309 4310 @Override 4311 public boolean equalsShallow(Base other) { 4312 if (!super.equalsShallow(other)) 4313 return false; 4314 if (!(other instanceof Contract)) 4315 return false; 4316 Contract o = (Contract) other; 4317 return compareValues(issued, o.issued, true); 4318 } 4319 4320 public boolean isEmpty() { 4321 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (issued == null || issued.isEmpty()) 4322 && (applies == null || applies.isEmpty()) && (subject == null || subject.isEmpty()) 4323 && (authority == null || authority.isEmpty()) && (domain == null || domain.isEmpty()) 4324 && (type == null || type.isEmpty()) && (subType == null || subType.isEmpty()) 4325 && (action == null || action.isEmpty()) && (actionReason == null || actionReason.isEmpty()) 4326 && (actor == null || actor.isEmpty()) && (valuedItem == null || valuedItem.isEmpty()) 4327 && (signer == null || signer.isEmpty()) && (term == null || term.isEmpty()) 4328 && (binding == null || binding.isEmpty()) && (friendly == null || friendly.isEmpty()) 4329 && (legal == null || legal.isEmpty()) && (rule == null || rule.isEmpty()); 4330 } 4331 4332 @Override 4333 public ResourceType getResourceType() { 4334 return ResourceType.Contract; 4335 } 4336 4337 @SearchParamDefinition(name = "actor", path = "Contract.actor.entity", description = "Contract Actor Type", type = "reference") 4338 public static final String SP_ACTOR = "actor"; 4339 @SearchParamDefinition(name = "identifier", path = "Contract.identifier", description = "The identity of the contract", type = "token") 4340 public static final String SP_IDENTIFIER = "identifier"; 4341 @SearchParamDefinition(name = "subject", path = "Contract.subject", description = "The identity of the target of the contract", type = "reference") 4342 public static final String SP_SUBJECT = "subject"; 4343 @SearchParamDefinition(name = "patient", path = "Contract.subject", description = "The identity of the target of the contract (if a patient)", type = "reference") 4344 public static final String SP_PATIENT = "patient"; 4345 @SearchParamDefinition(name = "signer", path = "Contract.signer.party", description = "Contract Signatory Party", type = "reference") 4346 public static final String SP_SIGNER = "signer"; 4347 4348}