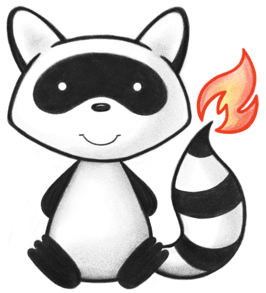
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Child; 037import ca.uhn.fhir.model.api.annotation.Description; 038import ca.uhn.fhir.model.api.annotation.ResourceDef; 039import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.utilities.Utilities; 042 043/** 044 * Financial instrument which may be used to pay for or reimburse health care 045 * products and services. 046 */ 047@ResourceDef(name = "Coverage", profile = "http://hl7.org/fhir/Profile/Coverage") 048public class Coverage extends DomainResource { 049 050 /** 051 * The program or plan underwriter or payor. 052 */ 053 @Child(name = "issuer", type = { Organization.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 054 @Description(shortDefinition = "An identifier for the plan issuer", formalDefinition = "The program or plan underwriter or payor.") 055 protected Reference issuer; 056 057 /** 058 * The actual object that is the target of the reference (The program or plan 059 * underwriter or payor.) 060 */ 061 protected Organization issuerTarget; 062 063 /** 064 * Business Identification Number (BIN number) used to identify the routing of 065 * eclaims if the insurer themselves don't have a BIN number for all of their 066 * business. 067 */ 068 @Child(name = "bin", type = { Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 069 @Description(shortDefinition = "BIN Number", formalDefinition = "Business Identification Number (BIN number) used to identify the routing of eclaims if the insurer themselves don't have a BIN number for all of their business.") 070 protected Identifier bin; 071 072 /** 073 * Time period during which the coverage is in force. A missing start date 074 * indicates the start date isn't known, a missing end date means the coverage 075 * is continuing to be in force. 076 */ 077 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 078 @Description(shortDefinition = "Coverage start and end dates", formalDefinition = "Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.") 079 protected Period period; 080 081 /** 082 * The type of coverage: social program, medical plan, accident coverage 083 * (workers compensation, auto), group health. 084 */ 085 @Child(name = "type", type = { Coding.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 086 @Description(shortDefinition = "Type of coverage", formalDefinition = "The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health.") 087 protected Coding type; 088 089 /** 090 * The id issued to the subscriber. 091 */ 092 @Child(name = "subscriberId", type = { 093 Identifier.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 094 @Description(shortDefinition = "Subscriber ID", formalDefinition = "The id issued to the subscriber.") 095 protected Identifier subscriberId; 096 097 /** 098 * The main (and possibly only) identifier for the coverage - often referred to 099 * as a Member Id, Subscriber Id, Certificate number or Personal Health Number 100 * or Case ID. 101 */ 102 @Child(name = "identifier", type = { 103 Identifier.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 104 @Description(shortDefinition = "The primary coverage ID", formalDefinition = "The main (and possibly only) identifier for the coverage - often referred to as a Member Id, Subscriber Id, Certificate number or Personal Health Number or Case ID.") 105 protected List<Identifier> identifier; 106 107 /** 108 * Identifies a style or collective of coverage issues by the underwriter, for 109 * example may be used to identify a class of coverage or employer group. May 110 * also be referred to as a Policy or Group ID. 111 */ 112 @Child(name = "group", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 113 @Description(shortDefinition = "An identifier for the group", formalDefinition = "Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage or employer group. May also be referred to as a Policy or Group ID.") 114 protected StringType group; 115 116 /** 117 * Identifies a style or collective of coverage issues by the underwriter, for 118 * example may be used to identify a class of coverage or employer group. May 119 * also be referred to as a Policy or Group ID. 120 */ 121 @Child(name = "plan", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 122 @Description(shortDefinition = "An identifier for the plan", formalDefinition = "Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage or employer group. May also be referred to as a Policy or Group ID.") 123 protected StringType plan; 124 125 /** 126 * Identifies a sub-style or sub-collective of coverage issues by the 127 * underwriter, for example may be used to identify a specific employer group 128 * within a class of employers. May be referred to as a Section or Division ID. 129 */ 130 @Child(name = "subPlan", type = { StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 131 @Description(shortDefinition = "An identifier for the subsection of the plan", formalDefinition = "Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a specific employer group within a class of employers. May be referred to as a Section or Division ID.") 132 protected StringType subPlan; 133 134 /** 135 * A unique identifier for a dependent under the coverage. 136 */ 137 @Child(name = "dependent", type = { 138 PositiveIntType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 139 @Description(shortDefinition = "The dependent number", formalDefinition = "A unique identifier for a dependent under the coverage.") 140 protected PositiveIntType dependent; 141 142 /** 143 * An optional counter for a particular instance of the identified coverage 144 * which increments upon each renewal. 145 */ 146 @Child(name = "sequence", type = { 147 PositiveIntType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 148 @Description(shortDefinition = "The plan instance or sequence counter", formalDefinition = "An optional counter for a particular instance of the identified coverage which increments upon each renewal.") 149 protected PositiveIntType sequence; 150 151 /** 152 * The party who 'owns' the insurance contractual relationship to the policy or 153 * to whom the benefit of the policy is due. 154 */ 155 @Child(name = "subscriber", type = { Patient.class }, order = 11, min = 0, max = 1, modifier = true, summary = false) 156 @Description(shortDefinition = "Plan holder information", formalDefinition = "The party who 'owns' the insurance contractual relationship to the policy or to whom the benefit of the policy is due.") 157 protected Reference subscriber; 158 159 /** 160 * The actual object that is the target of the reference (The party who 'owns' 161 * the insurance contractual relationship to the policy or to whom the benefit 162 * of the policy is due.) 163 */ 164 protected Patient subscriberTarget; 165 166 /** 167 * The identifier for a community of providers. 168 */ 169 @Child(name = "network", type = { Identifier.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 170 @Description(shortDefinition = "Insurer network", formalDefinition = "The identifier for a community of providers.") 171 protected Identifier network; 172 173 /** 174 * The policy(s) which constitute this insurance coverage. 175 */ 176 @Child(name = "contract", type = { 177 Contract.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 178 @Description(shortDefinition = "Contract details", formalDefinition = "The policy(s) which constitute this insurance coverage.") 179 protected List<Reference> contract; 180 /** 181 * The actual objects that are the target of the reference (The policy(s) which 182 * constitute this insurance coverage.) 183 */ 184 protected List<Contract> contractTarget; 185 186 private static final long serialVersionUID = -1312031251L; 187 188 /* 189 * Constructor 190 */ 191 public Coverage() { 192 super(); 193 } 194 195 /** 196 * @return {@link #issuer} (The program or plan underwriter or payor.) 197 */ 198 public Reference getIssuer() { 199 if (this.issuer == null) 200 if (Configuration.errorOnAutoCreate()) 201 throw new Error("Attempt to auto-create Coverage.issuer"); 202 else if (Configuration.doAutoCreate()) 203 this.issuer = new Reference(); // cc 204 return this.issuer; 205 } 206 207 public boolean hasIssuer() { 208 return this.issuer != null && !this.issuer.isEmpty(); 209 } 210 211 /** 212 * @param value {@link #issuer} (The program or plan underwriter or payor.) 213 */ 214 public Coverage setIssuer(Reference value) { 215 this.issuer = value; 216 return this; 217 } 218 219 /** 220 * @return {@link #issuer} The actual object that is the target of the 221 * reference. The reference library doesn't populate this, but you can 222 * use it to hold the resource if you resolve it. (The program or plan 223 * underwriter or payor.) 224 */ 225 public Organization getIssuerTarget() { 226 if (this.issuerTarget == null) 227 if (Configuration.errorOnAutoCreate()) 228 throw new Error("Attempt to auto-create Coverage.issuer"); 229 else if (Configuration.doAutoCreate()) 230 this.issuerTarget = new Organization(); // aa 231 return this.issuerTarget; 232 } 233 234 /** 235 * @param value {@link #issuer} The actual object that is the target of the 236 * reference. The reference library doesn't use these, but you can 237 * use it to hold the resource if you resolve it. (The program or 238 * plan underwriter or payor.) 239 */ 240 public Coverage setIssuerTarget(Organization value) { 241 this.issuerTarget = value; 242 return this; 243 } 244 245 /** 246 * @return {@link #bin} (Business Identification Number (BIN number) used to 247 * identify the routing of eclaims if the insurer themselves don't have 248 * a BIN number for all of their business.) 249 */ 250 public Identifier getBin() { 251 if (this.bin == null) 252 if (Configuration.errorOnAutoCreate()) 253 throw new Error("Attempt to auto-create Coverage.bin"); 254 else if (Configuration.doAutoCreate()) 255 this.bin = new Identifier(); // cc 256 return this.bin; 257 } 258 259 public boolean hasBin() { 260 return this.bin != null && !this.bin.isEmpty(); 261 } 262 263 /** 264 * @param value {@link #bin} (Business Identification Number (BIN number) used 265 * to identify the routing of eclaims if the insurer themselves 266 * don't have a BIN number for all of their business.) 267 */ 268 public Coverage setBin(Identifier value) { 269 this.bin = value; 270 return this; 271 } 272 273 /** 274 * @return {@link #period} (Time period during which the coverage is in force. A 275 * missing start date indicates the start date isn't known, a missing 276 * end date means the coverage is continuing to be in force.) 277 */ 278 public Period getPeriod() { 279 if (this.period == null) 280 if (Configuration.errorOnAutoCreate()) 281 throw new Error("Attempt to auto-create Coverage.period"); 282 else if (Configuration.doAutoCreate()) 283 this.period = new Period(); // cc 284 return this.period; 285 } 286 287 public boolean hasPeriod() { 288 return this.period != null && !this.period.isEmpty(); 289 } 290 291 /** 292 * @param value {@link #period} (Time period during which the coverage is in 293 * force. A missing start date indicates the start date isn't 294 * known, a missing end date means the coverage is continuing to be 295 * in force.) 296 */ 297 public Coverage setPeriod(Period value) { 298 this.period = value; 299 return this; 300 } 301 302 /** 303 * @return {@link #type} (The type of coverage: social program, medical plan, 304 * accident coverage (workers compensation, auto), group health.) 305 */ 306 public Coding getType() { 307 if (this.type == null) 308 if (Configuration.errorOnAutoCreate()) 309 throw new Error("Attempt to auto-create Coverage.type"); 310 else if (Configuration.doAutoCreate()) 311 this.type = new Coding(); // cc 312 return this.type; 313 } 314 315 public boolean hasType() { 316 return this.type != null && !this.type.isEmpty(); 317 } 318 319 /** 320 * @param value {@link #type} (The type of coverage: social program, medical 321 * plan, accident coverage (workers compensation, auto), group 322 * health.) 323 */ 324 public Coverage setType(Coding value) { 325 this.type = value; 326 return this; 327 } 328 329 /** 330 * @return {@link #subscriberId} (The id issued to the subscriber.) 331 */ 332 public Identifier getSubscriberId() { 333 if (this.subscriberId == null) 334 if (Configuration.errorOnAutoCreate()) 335 throw new Error("Attempt to auto-create Coverage.subscriberId"); 336 else if (Configuration.doAutoCreate()) 337 this.subscriberId = new Identifier(); // cc 338 return this.subscriberId; 339 } 340 341 public boolean hasSubscriberId() { 342 return this.subscriberId != null && !this.subscriberId.isEmpty(); 343 } 344 345 /** 346 * @param value {@link #subscriberId} (The id issued to the subscriber.) 347 */ 348 public Coverage setSubscriberId(Identifier value) { 349 this.subscriberId = value; 350 return this; 351 } 352 353 /** 354 * @return {@link #identifier} (The main (and possibly only) identifier for the 355 * coverage - often referred to as a Member Id, Subscriber Id, 356 * Certificate number or Personal Health Number or Case ID.) 357 */ 358 public List<Identifier> getIdentifier() { 359 if (this.identifier == null) 360 this.identifier = new ArrayList<Identifier>(); 361 return this.identifier; 362 } 363 364 public boolean hasIdentifier() { 365 if (this.identifier == null) 366 return false; 367 for (Identifier item : this.identifier) 368 if (!item.isEmpty()) 369 return true; 370 return false; 371 } 372 373 /** 374 * @return {@link #identifier} (The main (and possibly only) identifier for the 375 * coverage - often referred to as a Member Id, Subscriber Id, 376 * Certificate number or Personal Health Number or Case ID.) 377 */ 378 // syntactic sugar 379 public Identifier addIdentifier() { // 3 380 Identifier t = new Identifier(); 381 if (this.identifier == null) 382 this.identifier = new ArrayList<Identifier>(); 383 this.identifier.add(t); 384 return t; 385 } 386 387 // syntactic sugar 388 public Coverage addIdentifier(Identifier t) { // 3 389 if (t == null) 390 return this; 391 if (this.identifier == null) 392 this.identifier = new ArrayList<Identifier>(); 393 this.identifier.add(t); 394 return this; 395 } 396 397 /** 398 * @return {@link #group} (Identifies a style or collective of coverage issues 399 * by the underwriter, for example may be used to identify a class of 400 * coverage or employer group. May also be referred to as a Policy or 401 * Group ID.). This is the underlying object with id, value and 402 * extensions. The accessor "getGroup" gives direct access to the value 403 */ 404 public StringType getGroupElement() { 405 if (this.group == null) 406 if (Configuration.errorOnAutoCreate()) 407 throw new Error("Attempt to auto-create Coverage.group"); 408 else if (Configuration.doAutoCreate()) 409 this.group = new StringType(); // bb 410 return this.group; 411 } 412 413 public boolean hasGroupElement() { 414 return this.group != null && !this.group.isEmpty(); 415 } 416 417 public boolean hasGroup() { 418 return this.group != null && !this.group.isEmpty(); 419 } 420 421 /** 422 * @param value {@link #group} (Identifies a style or collective of coverage 423 * issues by the underwriter, for example may be used to identify a 424 * class of coverage or employer group. May also be referred to as 425 * a Policy or Group ID.). This is the underlying object with id, 426 * value and extensions. The accessor "getGroup" gives direct 427 * access to the value 428 */ 429 public Coverage setGroupElement(StringType value) { 430 this.group = value; 431 return this; 432 } 433 434 /** 435 * @return Identifies a style or collective of coverage issues by the 436 * underwriter, for example may be used to identify a class of coverage 437 * or employer group. May also be referred to as a Policy or Group ID. 438 */ 439 public String getGroup() { 440 return this.group == null ? null : this.group.getValue(); 441 } 442 443 /** 444 * @param value Identifies a style or collective of coverage issues by the 445 * underwriter, for example may be used to identify a class of 446 * coverage or employer group. May also be referred to as a Policy 447 * or Group ID. 448 */ 449 public Coverage setGroup(String value) { 450 if (Utilities.noString(value)) 451 this.group = null; 452 else { 453 if (this.group == null) 454 this.group = new StringType(); 455 this.group.setValue(value); 456 } 457 return this; 458 } 459 460 /** 461 * @return {@link #plan} (Identifies a style or collective of coverage issues by 462 * the underwriter, for example may be used to identify a class of 463 * coverage or employer group. May also be referred to as a Policy or 464 * Group ID.). This is the underlying object with id, value and 465 * extensions. The accessor "getPlan" gives direct access to the value 466 */ 467 public StringType getPlanElement() { 468 if (this.plan == null) 469 if (Configuration.errorOnAutoCreate()) 470 throw new Error("Attempt to auto-create Coverage.plan"); 471 else if (Configuration.doAutoCreate()) 472 this.plan = new StringType(); // bb 473 return this.plan; 474 } 475 476 public boolean hasPlanElement() { 477 return this.plan != null && !this.plan.isEmpty(); 478 } 479 480 public boolean hasPlan() { 481 return this.plan != null && !this.plan.isEmpty(); 482 } 483 484 /** 485 * @param value {@link #plan} (Identifies a style or collective of coverage 486 * issues by the underwriter, for example may be used to identify a 487 * class of coverage or employer group. May also be referred to as 488 * a Policy or Group ID.). This is the underlying object with id, 489 * value and extensions. The accessor "getPlan" gives direct access 490 * to the value 491 */ 492 public Coverage setPlanElement(StringType value) { 493 this.plan = value; 494 return this; 495 } 496 497 /** 498 * @return Identifies a style or collective of coverage issues by the 499 * underwriter, for example may be used to identify a class of coverage 500 * or employer group. May also be referred to as a Policy or Group ID. 501 */ 502 public String getPlan() { 503 return this.plan == null ? null : this.plan.getValue(); 504 } 505 506 /** 507 * @param value Identifies a style or collective of coverage issues by the 508 * underwriter, for example may be used to identify a class of 509 * coverage or employer group. May also be referred to as a Policy 510 * or Group ID. 511 */ 512 public Coverage setPlan(String value) { 513 if (Utilities.noString(value)) 514 this.plan = null; 515 else { 516 if (this.plan == null) 517 this.plan = new StringType(); 518 this.plan.setValue(value); 519 } 520 return this; 521 } 522 523 /** 524 * @return {@link #subPlan} (Identifies a sub-style or sub-collective of 525 * coverage issues by the underwriter, for example may be used to 526 * identify a specific employer group within a class of employers. May 527 * be referred to as a Section or Division ID.). This is the underlying 528 * object with id, value and extensions. The accessor "getSubPlan" gives 529 * direct access to the value 530 */ 531 public StringType getSubPlanElement() { 532 if (this.subPlan == null) 533 if (Configuration.errorOnAutoCreate()) 534 throw new Error("Attempt to auto-create Coverage.subPlan"); 535 else if (Configuration.doAutoCreate()) 536 this.subPlan = new StringType(); // bb 537 return this.subPlan; 538 } 539 540 public boolean hasSubPlanElement() { 541 return this.subPlan != null && !this.subPlan.isEmpty(); 542 } 543 544 public boolean hasSubPlan() { 545 return this.subPlan != null && !this.subPlan.isEmpty(); 546 } 547 548 /** 549 * @param value {@link #subPlan} (Identifies a sub-style or sub-collective of 550 * coverage issues by the underwriter, for example may be used to 551 * identify a specific employer group within a class of employers. 552 * May be referred to as a Section or Division ID.). This is the 553 * underlying object with id, value and extensions. The accessor 554 * "getSubPlan" gives direct access to the value 555 */ 556 public Coverage setSubPlanElement(StringType value) { 557 this.subPlan = value; 558 return this; 559 } 560 561 /** 562 * @return Identifies a sub-style or sub-collective of coverage issues by the 563 * underwriter, for example may be used to identify a specific employer 564 * group within a class of employers. May be referred to as a Section or 565 * Division ID. 566 */ 567 public String getSubPlan() { 568 return this.subPlan == null ? null : this.subPlan.getValue(); 569 } 570 571 /** 572 * @param value Identifies a sub-style or sub-collective of coverage issues by 573 * the underwriter, for example may be used to identify a specific 574 * employer group within a class of employers. May be referred to 575 * as a Section or Division ID. 576 */ 577 public Coverage setSubPlan(String value) { 578 if (Utilities.noString(value)) 579 this.subPlan = null; 580 else { 581 if (this.subPlan == null) 582 this.subPlan = new StringType(); 583 this.subPlan.setValue(value); 584 } 585 return this; 586 } 587 588 /** 589 * @return {@link #dependent} (A unique identifier for a dependent under the 590 * coverage.). This is the underlying object with id, value and 591 * extensions. The accessor "getDependent" gives direct access to the 592 * value 593 */ 594 public PositiveIntType getDependentElement() { 595 if (this.dependent == null) 596 if (Configuration.errorOnAutoCreate()) 597 throw new Error("Attempt to auto-create Coverage.dependent"); 598 else if (Configuration.doAutoCreate()) 599 this.dependent = new PositiveIntType(); // bb 600 return this.dependent; 601 } 602 603 public boolean hasDependentElement() { 604 return this.dependent != null && !this.dependent.isEmpty(); 605 } 606 607 public boolean hasDependent() { 608 return this.dependent != null && !this.dependent.isEmpty(); 609 } 610 611 /** 612 * @param value {@link #dependent} (A unique identifier for a dependent under 613 * the coverage.). This is the underlying object with id, value and 614 * extensions. The accessor "getDependent" gives direct access to 615 * the value 616 */ 617 public Coverage setDependentElement(PositiveIntType value) { 618 this.dependent = value; 619 return this; 620 } 621 622 /** 623 * @return A unique identifier for a dependent under the coverage. 624 */ 625 public int getDependent() { 626 return this.dependent == null || this.dependent.isEmpty() ? 0 : this.dependent.getValue(); 627 } 628 629 /** 630 * @param value A unique identifier for a dependent under the coverage. 631 */ 632 public Coverage setDependent(int value) { 633 if (this.dependent == null) 634 this.dependent = new PositiveIntType(); 635 this.dependent.setValue(value); 636 return this; 637 } 638 639 /** 640 * @return {@link #sequence} (An optional counter for a particular instance of 641 * the identified coverage which increments upon each renewal.). This is 642 * the underlying object with id, value and extensions. The accessor 643 * "getSequence" gives direct access to the value 644 */ 645 public PositiveIntType getSequenceElement() { 646 if (this.sequence == null) 647 if (Configuration.errorOnAutoCreate()) 648 throw new Error("Attempt to auto-create Coverage.sequence"); 649 else if (Configuration.doAutoCreate()) 650 this.sequence = new PositiveIntType(); // bb 651 return this.sequence; 652 } 653 654 public boolean hasSequenceElement() { 655 return this.sequence != null && !this.sequence.isEmpty(); 656 } 657 658 public boolean hasSequence() { 659 return this.sequence != null && !this.sequence.isEmpty(); 660 } 661 662 /** 663 * @param value {@link #sequence} (An optional counter for a particular instance 664 * of the identified coverage which increments upon each renewal.). 665 * This is the underlying object with id, value and extensions. The 666 * accessor "getSequence" gives direct access to the value 667 */ 668 public Coverage setSequenceElement(PositiveIntType value) { 669 this.sequence = value; 670 return this; 671 } 672 673 /** 674 * @return An optional counter for a particular instance of the identified 675 * coverage which increments upon each renewal. 676 */ 677 public int getSequence() { 678 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 679 } 680 681 /** 682 * @param value An optional counter for a particular instance of the identified 683 * coverage which increments upon each renewal. 684 */ 685 public Coverage setSequence(int value) { 686 if (this.sequence == null) 687 this.sequence = new PositiveIntType(); 688 this.sequence.setValue(value); 689 return this; 690 } 691 692 /** 693 * @return {@link #subscriber} (The party who 'owns' the insurance contractual 694 * relationship to the policy or to whom the benefit of the policy is 695 * due.) 696 */ 697 public Reference getSubscriber() { 698 if (this.subscriber == null) 699 if (Configuration.errorOnAutoCreate()) 700 throw new Error("Attempt to auto-create Coverage.subscriber"); 701 else if (Configuration.doAutoCreate()) 702 this.subscriber = new Reference(); // cc 703 return this.subscriber; 704 } 705 706 public boolean hasSubscriber() { 707 return this.subscriber != null && !this.subscriber.isEmpty(); 708 } 709 710 /** 711 * @param value {@link #subscriber} (The party who 'owns' the insurance 712 * contractual relationship to the policy or to whom the benefit of 713 * the policy is due.) 714 */ 715 public Coverage setSubscriber(Reference value) { 716 this.subscriber = value; 717 return this; 718 } 719 720 /** 721 * @return {@link #subscriber} The actual object that is the target of the 722 * reference. The reference library doesn't populate this, but you can 723 * use it to hold the resource if you resolve it. (The party who 'owns' 724 * the insurance contractual relationship to the policy or to whom the 725 * benefit of the policy is due.) 726 */ 727 public Patient getSubscriberTarget() { 728 if (this.subscriberTarget == null) 729 if (Configuration.errorOnAutoCreate()) 730 throw new Error("Attempt to auto-create Coverage.subscriber"); 731 else if (Configuration.doAutoCreate()) 732 this.subscriberTarget = new Patient(); // aa 733 return this.subscriberTarget; 734 } 735 736 /** 737 * @param value {@link #subscriber} The actual object that is the target of the 738 * reference. The reference library doesn't use these, but you can 739 * use it to hold the resource if you resolve it. (The party who 740 * 'owns' the insurance contractual relationship to the policy or 741 * to whom the benefit of the policy is due.) 742 */ 743 public Coverage setSubscriberTarget(Patient value) { 744 this.subscriberTarget = value; 745 return this; 746 } 747 748 /** 749 * @return {@link #network} (The identifier for a community of providers.) 750 */ 751 public Identifier getNetwork() { 752 if (this.network == null) 753 if (Configuration.errorOnAutoCreate()) 754 throw new Error("Attempt to auto-create Coverage.network"); 755 else if (Configuration.doAutoCreate()) 756 this.network = new Identifier(); // cc 757 return this.network; 758 } 759 760 public boolean hasNetwork() { 761 return this.network != null && !this.network.isEmpty(); 762 } 763 764 /** 765 * @param value {@link #network} (The identifier for a community of providers.) 766 */ 767 public Coverage setNetwork(Identifier value) { 768 this.network = value; 769 return this; 770 } 771 772 /** 773 * @return {@link #contract} (The policy(s) which constitute this insurance 774 * coverage.) 775 */ 776 public List<Reference> getContract() { 777 if (this.contract == null) 778 this.contract = new ArrayList<Reference>(); 779 return this.contract; 780 } 781 782 public boolean hasContract() { 783 if (this.contract == null) 784 return false; 785 for (Reference item : this.contract) 786 if (!item.isEmpty()) 787 return true; 788 return false; 789 } 790 791 /** 792 * @return {@link #contract} (The policy(s) which constitute this insurance 793 * coverage.) 794 */ 795 // syntactic sugar 796 public Reference addContract() { // 3 797 Reference t = new Reference(); 798 if (this.contract == null) 799 this.contract = new ArrayList<Reference>(); 800 this.contract.add(t); 801 return t; 802 } 803 804 // syntactic sugar 805 public Coverage addContract(Reference t) { // 3 806 if (t == null) 807 return this; 808 if (this.contract == null) 809 this.contract = new ArrayList<Reference>(); 810 this.contract.add(t); 811 return this; 812 } 813 814 /** 815 * @return {@link #contract} (The actual objects that are the target of the 816 * reference. The reference library doesn't populate this, but you can 817 * use this to hold the resources if you resolvethemt. The policy(s) 818 * which constitute this insurance coverage.) 819 */ 820 public List<Contract> getContractTarget() { 821 if (this.contractTarget == null) 822 this.contractTarget = new ArrayList<Contract>(); 823 return this.contractTarget; 824 } 825 826 // syntactic sugar 827 /** 828 * @return {@link #contract} (Add an actual object that is the target of the 829 * reference. The reference library doesn't use these, but you can use 830 * this to hold the resources if you resolvethemt. The policy(s) which 831 * constitute this insurance coverage.) 832 */ 833 public Contract addContractTarget() { 834 Contract r = new Contract(); 835 if (this.contractTarget == null) 836 this.contractTarget = new ArrayList<Contract>(); 837 this.contractTarget.add(r); 838 return r; 839 } 840 841 protected void listChildren(List<Property> childrenList) { 842 super.listChildren(childrenList); 843 childrenList.add(new Property("issuer", "Reference(Organization)", "The program or plan underwriter or payor.", 0, 844 java.lang.Integer.MAX_VALUE, issuer)); 845 childrenList.add(new Property("bin", "Identifier", 846 "Business Identification Number (BIN number) used to identify the routing of eclaims if the insurer themselves don't have a BIN number for all of their business.", 847 0, java.lang.Integer.MAX_VALUE, bin)); 848 childrenList.add(new Property("period", "Period", 849 "Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.", 850 0, java.lang.Integer.MAX_VALUE, period)); 851 childrenList.add(new Property("type", "Coding", 852 "The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health.", 853 0, java.lang.Integer.MAX_VALUE, type)); 854 childrenList.add(new Property("subscriberId", "Identifier", "The id issued to the subscriber.", 0, 855 java.lang.Integer.MAX_VALUE, subscriberId)); 856 childrenList.add(new Property("identifier", "Identifier", 857 "The main (and possibly only) identifier for the coverage - often referred to as a Member Id, Subscriber Id, Certificate number or Personal Health Number or Case ID.", 858 0, java.lang.Integer.MAX_VALUE, identifier)); 859 childrenList.add(new Property("group", "string", 860 "Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage or employer group. May also be referred to as a Policy or Group ID.", 861 0, java.lang.Integer.MAX_VALUE, group)); 862 childrenList.add(new Property("plan", "string", 863 "Identifies a style or collective of coverage issues by the underwriter, for example may be used to identify a class of coverage or employer group. May also be referred to as a Policy or Group ID.", 864 0, java.lang.Integer.MAX_VALUE, plan)); 865 childrenList.add(new Property("subPlan", "string", 866 "Identifies a sub-style or sub-collective of coverage issues by the underwriter, for example may be used to identify a specific employer group within a class of employers. May be referred to as a Section or Division ID.", 867 0, java.lang.Integer.MAX_VALUE, subPlan)); 868 childrenList.add(new Property("dependent", "positiveInt", "A unique identifier for a dependent under the coverage.", 869 0, java.lang.Integer.MAX_VALUE, dependent)); 870 childrenList.add(new Property("sequence", "positiveInt", 871 "An optional counter for a particular instance of the identified coverage which increments upon each renewal.", 872 0, java.lang.Integer.MAX_VALUE, sequence)); 873 childrenList.add(new Property("subscriber", "Reference(Patient)", 874 "The party who 'owns' the insurance contractual relationship to the policy or to whom the benefit of the policy is due.", 875 0, java.lang.Integer.MAX_VALUE, subscriber)); 876 childrenList.add(new Property("network", "Identifier", "The identifier for a community of providers.", 0, 877 java.lang.Integer.MAX_VALUE, network)); 878 childrenList.add(new Property("contract", "Reference(Contract)", 879 "The policy(s) which constitute this insurance coverage.", 0, java.lang.Integer.MAX_VALUE, contract)); 880 } 881 882 @Override 883 public void setProperty(String name, Base value) throws FHIRException { 884 if (name.equals("issuer")) 885 this.issuer = castToReference(value); // Reference 886 else if (name.equals("bin")) 887 this.bin = castToIdentifier(value); // Identifier 888 else if (name.equals("period")) 889 this.period = castToPeriod(value); // Period 890 else if (name.equals("type")) 891 this.type = castToCoding(value); // Coding 892 else if (name.equals("subscriberId")) 893 this.subscriberId = castToIdentifier(value); // Identifier 894 else if (name.equals("identifier")) 895 this.getIdentifier().add(castToIdentifier(value)); 896 else if (name.equals("group")) 897 this.group = castToString(value); // StringType 898 else if (name.equals("plan")) 899 this.plan = castToString(value); // StringType 900 else if (name.equals("subPlan")) 901 this.subPlan = castToString(value); // StringType 902 else if (name.equals("dependent")) 903 this.dependent = castToPositiveInt(value); // PositiveIntType 904 else if (name.equals("sequence")) 905 this.sequence = castToPositiveInt(value); // PositiveIntType 906 else if (name.equals("subscriber")) 907 this.subscriber = castToReference(value); // Reference 908 else if (name.equals("network")) 909 this.network = castToIdentifier(value); // Identifier 910 else if (name.equals("contract")) 911 this.getContract().add(castToReference(value)); 912 else 913 super.setProperty(name, value); 914 } 915 916 @Override 917 public Base addChild(String name) throws FHIRException { 918 if (name.equals("issuer")) { 919 this.issuer = new Reference(); 920 return this.issuer; 921 } else if (name.equals("bin")) { 922 this.bin = new Identifier(); 923 return this.bin; 924 } else if (name.equals("period")) { 925 this.period = new Period(); 926 return this.period; 927 } else if (name.equals("type")) { 928 this.type = new Coding(); 929 return this.type; 930 } else if (name.equals("subscriberId")) { 931 this.subscriberId = new Identifier(); 932 return this.subscriberId; 933 } else if (name.equals("identifier")) { 934 return addIdentifier(); 935 } else if (name.equals("group")) { 936 throw new FHIRException("Cannot call addChild on a singleton property Coverage.group"); 937 } else if (name.equals("plan")) { 938 throw new FHIRException("Cannot call addChild on a singleton property Coverage.plan"); 939 } else if (name.equals("subPlan")) { 940 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subPlan"); 941 } else if (name.equals("dependent")) { 942 throw new FHIRException("Cannot call addChild on a singleton property Coverage.dependent"); 943 } else if (name.equals("sequence")) { 944 throw new FHIRException("Cannot call addChild on a singleton property Coverage.sequence"); 945 } else if (name.equals("subscriber")) { 946 this.subscriber = new Reference(); 947 return this.subscriber; 948 } else if (name.equals("network")) { 949 this.network = new Identifier(); 950 return this.network; 951 } else if (name.equals("contract")) { 952 return addContract(); 953 } else 954 return super.addChild(name); 955 } 956 957 public String fhirType() { 958 return "Coverage"; 959 960 } 961 962 public Coverage copy() { 963 Coverage dst = new Coverage(); 964 copyValues(dst); 965 dst.issuer = issuer == null ? null : issuer.copy(); 966 dst.bin = bin == null ? null : bin.copy(); 967 dst.period = period == null ? null : period.copy(); 968 dst.type = type == null ? null : type.copy(); 969 dst.subscriberId = subscriberId == null ? null : subscriberId.copy(); 970 if (identifier != null) { 971 dst.identifier = new ArrayList<Identifier>(); 972 for (Identifier i : identifier) 973 dst.identifier.add(i.copy()); 974 } 975 ; 976 dst.group = group == null ? null : group.copy(); 977 dst.plan = plan == null ? null : plan.copy(); 978 dst.subPlan = subPlan == null ? null : subPlan.copy(); 979 dst.dependent = dependent == null ? null : dependent.copy(); 980 dst.sequence = sequence == null ? null : sequence.copy(); 981 dst.subscriber = subscriber == null ? null : subscriber.copy(); 982 dst.network = network == null ? null : network.copy(); 983 if (contract != null) { 984 dst.contract = new ArrayList<Reference>(); 985 for (Reference i : contract) 986 dst.contract.add(i.copy()); 987 } 988 ; 989 return dst; 990 } 991 992 protected Coverage typedCopy() { 993 return copy(); 994 } 995 996 @Override 997 public boolean equalsDeep(Base other) { 998 if (!super.equalsDeep(other)) 999 return false; 1000 if (!(other instanceof Coverage)) 1001 return false; 1002 Coverage o = (Coverage) other; 1003 return compareDeep(issuer, o.issuer, true) && compareDeep(bin, o.bin, true) && compareDeep(period, o.period, true) 1004 && compareDeep(type, o.type, true) && compareDeep(subscriberId, o.subscriberId, true) 1005 && compareDeep(identifier, o.identifier, true) && compareDeep(group, o.group, true) 1006 && compareDeep(plan, o.plan, true) && compareDeep(subPlan, o.subPlan, true) 1007 && compareDeep(dependent, o.dependent, true) && compareDeep(sequence, o.sequence, true) 1008 && compareDeep(subscriber, o.subscriber, true) && compareDeep(network, o.network, true) 1009 && compareDeep(contract, o.contract, true); 1010 } 1011 1012 @Override 1013 public boolean equalsShallow(Base other) { 1014 if (!super.equalsShallow(other)) 1015 return false; 1016 if (!(other instanceof Coverage)) 1017 return false; 1018 Coverage o = (Coverage) other; 1019 return compareValues(group, o.group, true) && compareValues(plan, o.plan, true) 1020 && compareValues(subPlan, o.subPlan, true) && compareValues(dependent, o.dependent, true) 1021 && compareValues(sequence, o.sequence, true); 1022 } 1023 1024 public boolean isEmpty() { 1025 return super.isEmpty() && (issuer == null || issuer.isEmpty()) && (bin == null || bin.isEmpty()) 1026 && (period == null || period.isEmpty()) && (type == null || type.isEmpty()) 1027 && (subscriberId == null || subscriberId.isEmpty()) && (identifier == null || identifier.isEmpty()) 1028 && (group == null || group.isEmpty()) && (plan == null || plan.isEmpty()) 1029 && (subPlan == null || subPlan.isEmpty()) && (dependent == null || dependent.isEmpty()) 1030 && (sequence == null || sequence.isEmpty()) && (subscriber == null || subscriber.isEmpty()) 1031 && (network == null || network.isEmpty()) && (contract == null || contract.isEmpty()); 1032 } 1033 1034 @Override 1035 public ResourceType getResourceType() { 1036 return ResourceType.Coverage; 1037 } 1038 1039 @SearchParamDefinition(name = "identifier", path = "Coverage.identifier", description = "The primary identifier of the insured", type = "token") 1040 public static final String SP_IDENTIFIER = "identifier"; 1041 @SearchParamDefinition(name = "sequence", path = "Coverage.sequence", description = "Sequence number", type = "token") 1042 public static final String SP_SEQUENCE = "sequence"; 1043 @SearchParamDefinition(name = "subplan", path = "Coverage.subPlan", description = "Sub-plan identifier", type = "token") 1044 public static final String SP_SUBPLAN = "subplan"; 1045 @SearchParamDefinition(name = "type", path = "Coverage.type", description = "The kind of coverage", type = "token") 1046 public static final String SP_TYPE = "type"; 1047 @SearchParamDefinition(name = "plan", path = "Coverage.plan", description = "A plan or policy identifier", type = "token") 1048 public static final String SP_PLAN = "plan"; 1049 @SearchParamDefinition(name = "dependent", path = "Coverage.dependent", description = "Dependent number", type = "token") 1050 public static final String SP_DEPENDENT = "dependent"; 1051 @SearchParamDefinition(name = "issuer", path = "Coverage.issuer", description = "The identity of the insurer", type = "reference") 1052 public static final String SP_ISSUER = "issuer"; 1053 @SearchParamDefinition(name = "group", path = "Coverage.group", description = "Group identifier", type = "token") 1054 public static final String SP_GROUP = "group"; 1055 1056}