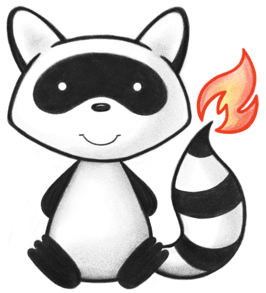
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus; 038import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.utilities.Utilities; 047 048/** 049 * The formal description of a single piece of information that can be gathered 050 * and reported. 051 */ 052@ResourceDef(name = "DataElement", profile = "http://hl7.org/fhir/Profile/DataElement") 053public class DataElement extends DomainResource { 054 055 public enum DataElementStringency { 056 /** 057 * The data element is sufficiently well-constrained that multiple pieces of 058 * data captured according to the constraints of the data element will be 059 * comparable (though in some cases, a degree of automated 060 * conversion/normalization may be required). 061 */ 062 COMPARABLE, 063 /** 064 * The data element is fully specified down to a single value set, single unit 065 * of measure, single data type, etc. Multiple pieces of data associated with 066 * this data element are fully comparable. 067 */ 068 FULLYSPECIFIED, 069 /** 070 * The data element allows multiple units of measure having equivalent meaning; 071 * e.g. "cc" (cubic centimeter) and "mL" (milliliter). 072 */ 073 EQUIVALENT, 074 /** 075 * The data element allows multiple units of measure that are convertable 076 * between each other (e.g. inches and centimeters) and/or allows data to be 077 * captured in multiple value sets for which a known mapping exists allowing 078 * conversion of meaning. 079 */ 080 CONVERTABLE, 081 /** 082 * A convertable data element where unit conversions are different only by a 083 * power of 10; e.g. g, mg, kg. 084 */ 085 SCALEABLE, 086 /** 087 * The data element is unconstrained in units, choice of data types and/or 088 * choice of vocabulary such that automated comparison of data captured using 089 * the data element is not possible. 090 */ 091 FLEXIBLE, 092 /** 093 * added to help the parsers 094 */ 095 NULL; 096 097 public static DataElementStringency fromCode(String codeString) throws FHIRException { 098 if (codeString == null || "".equals(codeString)) 099 return null; 100 if ("comparable".equals(codeString)) 101 return COMPARABLE; 102 if ("fully-specified".equals(codeString)) 103 return FULLYSPECIFIED; 104 if ("equivalent".equals(codeString)) 105 return EQUIVALENT; 106 if ("convertable".equals(codeString)) 107 return CONVERTABLE; 108 if ("scaleable".equals(codeString)) 109 return SCALEABLE; 110 if ("flexible".equals(codeString)) 111 return FLEXIBLE; 112 throw new FHIRException("Unknown DataElementStringency code '" + codeString + "'"); 113 } 114 115 public String toCode() { 116 switch (this) { 117 case COMPARABLE: 118 return "comparable"; 119 case FULLYSPECIFIED: 120 return "fully-specified"; 121 case EQUIVALENT: 122 return "equivalent"; 123 case CONVERTABLE: 124 return "convertable"; 125 case SCALEABLE: 126 return "scaleable"; 127 case FLEXIBLE: 128 return "flexible"; 129 case NULL: 130 return null; 131 default: 132 return "?"; 133 } 134 } 135 136 public String getSystem() { 137 switch (this) { 138 case COMPARABLE: 139 return "http://hl7.org/fhir/dataelement-stringency"; 140 case FULLYSPECIFIED: 141 return "http://hl7.org/fhir/dataelement-stringency"; 142 case EQUIVALENT: 143 return "http://hl7.org/fhir/dataelement-stringency"; 144 case CONVERTABLE: 145 return "http://hl7.org/fhir/dataelement-stringency"; 146 case SCALEABLE: 147 return "http://hl7.org/fhir/dataelement-stringency"; 148 case FLEXIBLE: 149 return "http://hl7.org/fhir/dataelement-stringency"; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 157 public String getDefinition() { 158 switch (this) { 159 case COMPARABLE: 160 return "The data element is sufficiently well-constrained that multiple pieces of data captured according to the constraints of the data element will be comparable (though in some cases, a degree of automated conversion/normalization may be required)."; 161 case FULLYSPECIFIED: 162 return "The data element is fully specified down to a single value set, single unit of measure, single data type, etc. Multiple pieces of data associated with this data element are fully comparable."; 163 case EQUIVALENT: 164 return "The data element allows multiple units of measure having equivalent meaning; e.g. \"cc\" (cubic centimeter) and \"mL\" (milliliter)."; 165 case CONVERTABLE: 166 return "The data element allows multiple units of measure that are convertable between each other (e.g. inches and centimeters) and/or allows data to be captured in multiple value sets for which a known mapping exists allowing conversion of meaning."; 167 case SCALEABLE: 168 return "A convertable data element where unit conversions are different only by a power of 10; e.g. g, mg, kg."; 169 case FLEXIBLE: 170 return "The data element is unconstrained in units, choice of data types and/or choice of vocabulary such that automated comparison of data captured using the data element is not possible."; 171 case NULL: 172 return null; 173 default: 174 return "?"; 175 } 176 } 177 178 public String getDisplay() { 179 switch (this) { 180 case COMPARABLE: 181 return "Comparable"; 182 case FULLYSPECIFIED: 183 return "Fully Specified"; 184 case EQUIVALENT: 185 return "Equivalent"; 186 case CONVERTABLE: 187 return "Convertable"; 188 case SCALEABLE: 189 return "Scaleable"; 190 case FLEXIBLE: 191 return "Flexible"; 192 case NULL: 193 return null; 194 default: 195 return "?"; 196 } 197 } 198 } 199 200 public static class DataElementStringencyEnumFactory implements EnumFactory<DataElementStringency> { 201 public DataElementStringency fromCode(String codeString) throws IllegalArgumentException { 202 if (codeString == null || "".equals(codeString)) 203 if (codeString == null || "".equals(codeString)) 204 return null; 205 if ("comparable".equals(codeString)) 206 return DataElementStringency.COMPARABLE; 207 if ("fully-specified".equals(codeString)) 208 return DataElementStringency.FULLYSPECIFIED; 209 if ("equivalent".equals(codeString)) 210 return DataElementStringency.EQUIVALENT; 211 if ("convertable".equals(codeString)) 212 return DataElementStringency.CONVERTABLE; 213 if ("scaleable".equals(codeString)) 214 return DataElementStringency.SCALEABLE; 215 if ("flexible".equals(codeString)) 216 return DataElementStringency.FLEXIBLE; 217 throw new IllegalArgumentException("Unknown DataElementStringency code '" + codeString + "'"); 218 } 219 220 public Enumeration<DataElementStringency> fromType(Base code) throws FHIRException { 221 if (code == null || code.isEmpty()) 222 return null; 223 String codeString = ((PrimitiveType) code).asStringValue(); 224 if (codeString == null || "".equals(codeString)) 225 return null; 226 if ("comparable".equals(codeString)) 227 return new Enumeration<DataElementStringency>(this, DataElementStringency.COMPARABLE); 228 if ("fully-specified".equals(codeString)) 229 return new Enumeration<DataElementStringency>(this, DataElementStringency.FULLYSPECIFIED); 230 if ("equivalent".equals(codeString)) 231 return new Enumeration<DataElementStringency>(this, DataElementStringency.EQUIVALENT); 232 if ("convertable".equals(codeString)) 233 return new Enumeration<DataElementStringency>(this, DataElementStringency.CONVERTABLE); 234 if ("scaleable".equals(codeString)) 235 return new Enumeration<DataElementStringency>(this, DataElementStringency.SCALEABLE); 236 if ("flexible".equals(codeString)) 237 return new Enumeration<DataElementStringency>(this, DataElementStringency.FLEXIBLE); 238 throw new FHIRException("Unknown DataElementStringency code '" + codeString + "'"); 239 } 240 241 public String toCode(DataElementStringency code) { 242 if (code == DataElementStringency.COMPARABLE) 243 return "comparable"; 244 if (code == DataElementStringency.FULLYSPECIFIED) 245 return "fully-specified"; 246 if (code == DataElementStringency.EQUIVALENT) 247 return "equivalent"; 248 if (code == DataElementStringency.CONVERTABLE) 249 return "convertable"; 250 if (code == DataElementStringency.SCALEABLE) 251 return "scaleable"; 252 if (code == DataElementStringency.FLEXIBLE) 253 return "flexible"; 254 return "?"; 255 } 256 } 257 258 @Block() 259 public static class DataElementContactComponent extends BackboneElement implements IBaseBackboneElement { 260 /** 261 * The name of an individual to contact regarding the data element. 262 */ 263 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 264 @Description(shortDefinition = "Name of a individual to contact", formalDefinition = "The name of an individual to contact regarding the data element.") 265 protected StringType name; 266 267 /** 268 * Contact details for individual (if a name was provided) or the publisher. 269 */ 270 @Child(name = "telecom", type = { 271 ContactPoint.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 272 @Description(shortDefinition = "Contact details for individual or publisher", formalDefinition = "Contact details for individual (if a name was provided) or the publisher.") 273 protected List<ContactPoint> telecom; 274 275 private static final long serialVersionUID = -1179697803L; 276 277 /* 278 * Constructor 279 */ 280 public DataElementContactComponent() { 281 super(); 282 } 283 284 /** 285 * @return {@link #name} (The name of an individual to contact regarding the 286 * data element.). This is the underlying object with id, value and 287 * extensions. The accessor "getName" gives direct access to the value 288 */ 289 public StringType getNameElement() { 290 if (this.name == null) 291 if (Configuration.errorOnAutoCreate()) 292 throw new Error("Attempt to auto-create DataElementContactComponent.name"); 293 else if (Configuration.doAutoCreate()) 294 this.name = new StringType(); // bb 295 return this.name; 296 } 297 298 public boolean hasNameElement() { 299 return this.name != null && !this.name.isEmpty(); 300 } 301 302 public boolean hasName() { 303 return this.name != null && !this.name.isEmpty(); 304 } 305 306 /** 307 * @param value {@link #name} (The name of an individual to contact regarding 308 * the data element.). This is the underlying object with id, value 309 * and extensions. The accessor "getName" gives direct access to 310 * the value 311 */ 312 public DataElementContactComponent setNameElement(StringType value) { 313 this.name = value; 314 return this; 315 } 316 317 /** 318 * @return The name of an individual to contact regarding the data element. 319 */ 320 public String getName() { 321 return this.name == null ? null : this.name.getValue(); 322 } 323 324 /** 325 * @param value The name of an individual to contact regarding the data element. 326 */ 327 public DataElementContactComponent setName(String value) { 328 if (Utilities.noString(value)) 329 this.name = null; 330 else { 331 if (this.name == null) 332 this.name = new StringType(); 333 this.name.setValue(value); 334 } 335 return this; 336 } 337 338 /** 339 * @return {@link #telecom} (Contact details for individual (if a name was 340 * provided) or the publisher.) 341 */ 342 public List<ContactPoint> getTelecom() { 343 if (this.telecom == null) 344 this.telecom = new ArrayList<ContactPoint>(); 345 return this.telecom; 346 } 347 348 public boolean hasTelecom() { 349 if (this.telecom == null) 350 return false; 351 for (ContactPoint item : this.telecom) 352 if (!item.isEmpty()) 353 return true; 354 return false; 355 } 356 357 /** 358 * @return {@link #telecom} (Contact details for individual (if a name was 359 * provided) or the publisher.) 360 */ 361 // syntactic sugar 362 public ContactPoint addTelecom() { // 3 363 ContactPoint t = new ContactPoint(); 364 if (this.telecom == null) 365 this.telecom = new ArrayList<ContactPoint>(); 366 this.telecom.add(t); 367 return t; 368 } 369 370 // syntactic sugar 371 public DataElementContactComponent addTelecom(ContactPoint t) { // 3 372 if (t == null) 373 return this; 374 if (this.telecom == null) 375 this.telecom = new ArrayList<ContactPoint>(); 376 this.telecom.add(t); 377 return this; 378 } 379 380 protected void listChildren(List<Property> childrenList) { 381 super.listChildren(childrenList); 382 childrenList.add(new Property("name", "string", 383 "The name of an individual to contact regarding the data element.", 0, java.lang.Integer.MAX_VALUE, name)); 384 childrenList.add(new Property("telecom", "ContactPoint", 385 "Contact details for individual (if a name was provided) or the publisher.", 0, java.lang.Integer.MAX_VALUE, 386 telecom)); 387 } 388 389 @Override 390 public void setProperty(String name, Base value) throws FHIRException { 391 if (name.equals("name")) 392 this.name = castToString(value); // StringType 393 else if (name.equals("telecom")) 394 this.getTelecom().add(castToContactPoint(value)); 395 else 396 super.setProperty(name, value); 397 } 398 399 @Override 400 public Base addChild(String name) throws FHIRException { 401 if (name.equals("name")) { 402 throw new FHIRException("Cannot call addChild on a singleton property DataElement.name"); 403 } else if (name.equals("telecom")) { 404 return addTelecom(); 405 } else 406 return super.addChild(name); 407 } 408 409 public DataElementContactComponent copy() { 410 DataElementContactComponent dst = new DataElementContactComponent(); 411 copyValues(dst); 412 dst.name = name == null ? null : name.copy(); 413 if (telecom != null) { 414 dst.telecom = new ArrayList<ContactPoint>(); 415 for (ContactPoint i : telecom) 416 dst.telecom.add(i.copy()); 417 } 418 ; 419 return dst; 420 } 421 422 @Override 423 public boolean equalsDeep(Base other) { 424 if (!super.equalsDeep(other)) 425 return false; 426 if (!(other instanceof DataElementContactComponent)) 427 return false; 428 DataElementContactComponent o = (DataElementContactComponent) other; 429 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 430 } 431 432 @Override 433 public boolean equalsShallow(Base other) { 434 if (!super.equalsShallow(other)) 435 return false; 436 if (!(other instanceof DataElementContactComponent)) 437 return false; 438 DataElementContactComponent o = (DataElementContactComponent) other; 439 return compareValues(name, o.name, true); 440 } 441 442 public boolean isEmpty() { 443 return super.isEmpty() && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()); 444 } 445 446 public String fhirType() { 447 return "DataElement.contact"; 448 449 } 450 451 } 452 453 @Block() 454 public static class DataElementMappingComponent extends BackboneElement implements IBaseBackboneElement { 455 /** 456 * An internal id that is used to identify this mapping set when specific 457 * mappings are made on a per-element basis. 458 */ 459 @Child(name = "identity", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 460 @Description(shortDefinition = "Internal id when this mapping is used", formalDefinition = "An internal id that is used to identify this mapping set when specific mappings are made on a per-element basis.") 461 protected IdType identity; 462 463 /** 464 * An absolute URI that identifies the specification that this mapping is 465 * expressed to. 466 */ 467 @Child(name = "uri", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 468 @Description(shortDefinition = "Identifies what this mapping refers to", formalDefinition = "An absolute URI that identifies the specification that this mapping is expressed to.") 469 protected UriType uri; 470 471 /** 472 * A name for the specification that is being mapped to. 473 */ 474 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 475 @Description(shortDefinition = "Names what this mapping refers to", formalDefinition = "A name for the specification that is being mapped to.") 476 protected StringType name; 477 478 /** 479 * Comments about this mapping, including version notes, issues, scope 480 * limitations, and other important notes for usage. 481 */ 482 @Child(name = "comments", type = { 483 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 484 @Description(shortDefinition = "Versions, Issues, Scope limitations etc.", formalDefinition = "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.") 485 protected StringType comments; 486 487 private static final long serialVersionUID = 299630820L; 488 489 /* 490 * Constructor 491 */ 492 public DataElementMappingComponent() { 493 super(); 494 } 495 496 /* 497 * Constructor 498 */ 499 public DataElementMappingComponent(IdType identity) { 500 super(); 501 this.identity = identity; 502 } 503 504 /** 505 * @return {@link #identity} (An internal id that is used to identify this 506 * mapping set when specific mappings are made on a per-element basis.). 507 * This is the underlying object with id, value and extensions. The 508 * accessor "getIdentity" gives direct access to the value 509 */ 510 public IdType getIdentityElement() { 511 if (this.identity == null) 512 if (Configuration.errorOnAutoCreate()) 513 throw new Error("Attempt to auto-create DataElementMappingComponent.identity"); 514 else if (Configuration.doAutoCreate()) 515 this.identity = new IdType(); // bb 516 return this.identity; 517 } 518 519 public boolean hasIdentityElement() { 520 return this.identity != null && !this.identity.isEmpty(); 521 } 522 523 public boolean hasIdentity() { 524 return this.identity != null && !this.identity.isEmpty(); 525 } 526 527 /** 528 * @param value {@link #identity} (An internal id that is used to identify this 529 * mapping set when specific mappings are made on a per-element 530 * basis.). This is the underlying object with id, value and 531 * extensions. The accessor "getIdentity" gives direct access to 532 * the value 533 */ 534 public DataElementMappingComponent setIdentityElement(IdType value) { 535 this.identity = value; 536 return this; 537 } 538 539 /** 540 * @return An internal id that is used to identify this mapping set when 541 * specific mappings are made on a per-element basis. 542 */ 543 public String getIdentity() { 544 return this.identity == null ? null : this.identity.getValue(); 545 } 546 547 /** 548 * @param value An internal id that is used to identify this mapping set when 549 * specific mappings are made on a per-element basis. 550 */ 551 public DataElementMappingComponent setIdentity(String value) { 552 if (this.identity == null) 553 this.identity = new IdType(); 554 this.identity.setValue(value); 555 return this; 556 } 557 558 /** 559 * @return {@link #uri} (An absolute URI that identifies the specification that 560 * this mapping is expressed to.). This is the underlying object with 561 * id, value and extensions. The accessor "getUri" gives direct access 562 * to the value 563 */ 564 public UriType getUriElement() { 565 if (this.uri == null) 566 if (Configuration.errorOnAutoCreate()) 567 throw new Error("Attempt to auto-create DataElementMappingComponent.uri"); 568 else if (Configuration.doAutoCreate()) 569 this.uri = new UriType(); // bb 570 return this.uri; 571 } 572 573 public boolean hasUriElement() { 574 return this.uri != null && !this.uri.isEmpty(); 575 } 576 577 public boolean hasUri() { 578 return this.uri != null && !this.uri.isEmpty(); 579 } 580 581 /** 582 * @param value {@link #uri} (An absolute URI that identifies the specification 583 * that this mapping is expressed to.). This is the underlying 584 * object with id, value and extensions. The accessor "getUri" 585 * gives direct access to the value 586 */ 587 public DataElementMappingComponent setUriElement(UriType value) { 588 this.uri = value; 589 return this; 590 } 591 592 /** 593 * @return An absolute URI that identifies the specification that this mapping 594 * is expressed to. 595 */ 596 public String getUri() { 597 return this.uri == null ? null : this.uri.getValue(); 598 } 599 600 /** 601 * @param value An absolute URI that identifies the specification that this 602 * mapping is expressed to. 603 */ 604 public DataElementMappingComponent setUri(String value) { 605 if (Utilities.noString(value)) 606 this.uri = null; 607 else { 608 if (this.uri == null) 609 this.uri = new UriType(); 610 this.uri.setValue(value); 611 } 612 return this; 613 } 614 615 /** 616 * @return {@link #name} (A name for the specification that is being mapped 617 * to.). This is the underlying object with id, value and extensions. 618 * The accessor "getName" gives direct access to the value 619 */ 620 public StringType getNameElement() { 621 if (this.name == null) 622 if (Configuration.errorOnAutoCreate()) 623 throw new Error("Attempt to auto-create DataElementMappingComponent.name"); 624 else if (Configuration.doAutoCreate()) 625 this.name = new StringType(); // bb 626 return this.name; 627 } 628 629 public boolean hasNameElement() { 630 return this.name != null && !this.name.isEmpty(); 631 } 632 633 public boolean hasName() { 634 return this.name != null && !this.name.isEmpty(); 635 } 636 637 /** 638 * @param value {@link #name} (A name for the specification that is being mapped 639 * to.). This is the underlying object with id, value and 640 * extensions. The accessor "getName" gives direct access to the 641 * value 642 */ 643 public DataElementMappingComponent setNameElement(StringType value) { 644 this.name = value; 645 return this; 646 } 647 648 /** 649 * @return A name for the specification that is being mapped to. 650 */ 651 public String getName() { 652 return this.name == null ? null : this.name.getValue(); 653 } 654 655 /** 656 * @param value A name for the specification that is being mapped to. 657 */ 658 public DataElementMappingComponent setName(String value) { 659 if (Utilities.noString(value)) 660 this.name = null; 661 else { 662 if (this.name == null) 663 this.name = new StringType(); 664 this.name.setValue(value); 665 } 666 return this; 667 } 668 669 /** 670 * @return {@link #comments} (Comments about this mapping, including version 671 * notes, issues, scope limitations, and other important notes for 672 * usage.). This is the underlying object with id, value and extensions. 673 * The accessor "getComments" gives direct access to the value 674 */ 675 public StringType getCommentsElement() { 676 if (this.comments == null) 677 if (Configuration.errorOnAutoCreate()) 678 throw new Error("Attempt to auto-create DataElementMappingComponent.comments"); 679 else if (Configuration.doAutoCreate()) 680 this.comments = new StringType(); // bb 681 return this.comments; 682 } 683 684 public boolean hasCommentsElement() { 685 return this.comments != null && !this.comments.isEmpty(); 686 } 687 688 public boolean hasComments() { 689 return this.comments != null && !this.comments.isEmpty(); 690 } 691 692 /** 693 * @param value {@link #comments} (Comments about this mapping, including 694 * version notes, issues, scope limitations, and other important 695 * notes for usage.). This is the underlying object with id, value 696 * and extensions. The accessor "getComments" gives direct access 697 * to the value 698 */ 699 public DataElementMappingComponent setCommentsElement(StringType value) { 700 this.comments = value; 701 return this; 702 } 703 704 /** 705 * @return Comments about this mapping, including version notes, issues, scope 706 * limitations, and other important notes for usage. 707 */ 708 public String getComments() { 709 return this.comments == null ? null : this.comments.getValue(); 710 } 711 712 /** 713 * @param value Comments about this mapping, including version notes, issues, 714 * scope limitations, and other important notes for usage. 715 */ 716 public DataElementMappingComponent setComments(String value) { 717 if (Utilities.noString(value)) 718 this.comments = null; 719 else { 720 if (this.comments == null) 721 this.comments = new StringType(); 722 this.comments.setValue(value); 723 } 724 return this; 725 } 726 727 protected void listChildren(List<Property> childrenList) { 728 super.listChildren(childrenList); 729 childrenList.add(new Property("identity", "id", 730 "An internal id that is used to identify this mapping set when specific mappings are made on a per-element basis.", 731 0, java.lang.Integer.MAX_VALUE, identity)); 732 childrenList.add(new Property("uri", "uri", 733 "An absolute URI that identifies the specification that this mapping is expressed to.", 0, 734 java.lang.Integer.MAX_VALUE, uri)); 735 childrenList.add(new Property("name", "string", "A name for the specification that is being mapped to.", 0, 736 java.lang.Integer.MAX_VALUE, name)); 737 childrenList.add(new Property("comments", "string", 738 "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.", 739 0, java.lang.Integer.MAX_VALUE, comments)); 740 } 741 742 @Override 743 public void setProperty(String name, Base value) throws FHIRException { 744 if (name.equals("identity")) 745 this.identity = castToId(value); // IdType 746 else if (name.equals("uri")) 747 this.uri = castToUri(value); // UriType 748 else if (name.equals("name")) 749 this.name = castToString(value); // StringType 750 else if (name.equals("comments")) 751 this.comments = castToString(value); // StringType 752 else 753 super.setProperty(name, value); 754 } 755 756 @Override 757 public Base addChild(String name) throws FHIRException { 758 if (name.equals("identity")) { 759 throw new FHIRException("Cannot call addChild on a singleton property DataElement.identity"); 760 } else if (name.equals("uri")) { 761 throw new FHIRException("Cannot call addChild on a singleton property DataElement.uri"); 762 } else if (name.equals("name")) { 763 throw new FHIRException("Cannot call addChild on a singleton property DataElement.name"); 764 } else if (name.equals("comments")) { 765 throw new FHIRException("Cannot call addChild on a singleton property DataElement.comments"); 766 } else 767 return super.addChild(name); 768 } 769 770 public DataElementMappingComponent copy() { 771 DataElementMappingComponent dst = new DataElementMappingComponent(); 772 copyValues(dst); 773 dst.identity = identity == null ? null : identity.copy(); 774 dst.uri = uri == null ? null : uri.copy(); 775 dst.name = name == null ? null : name.copy(); 776 dst.comments = comments == null ? null : comments.copy(); 777 return dst; 778 } 779 780 @Override 781 public boolean equalsDeep(Base other) { 782 if (!super.equalsDeep(other)) 783 return false; 784 if (!(other instanceof DataElementMappingComponent)) 785 return false; 786 DataElementMappingComponent o = (DataElementMappingComponent) other; 787 return compareDeep(identity, o.identity, true) && compareDeep(uri, o.uri, true) && compareDeep(name, o.name, true) 788 && compareDeep(comments, o.comments, true); 789 } 790 791 @Override 792 public boolean equalsShallow(Base other) { 793 if (!super.equalsShallow(other)) 794 return false; 795 if (!(other instanceof DataElementMappingComponent)) 796 return false; 797 DataElementMappingComponent o = (DataElementMappingComponent) other; 798 return compareValues(identity, o.identity, true) && compareValues(uri, o.uri, true) 799 && compareValues(name, o.name, true) && compareValues(comments, o.comments, true); 800 } 801 802 public boolean isEmpty() { 803 return super.isEmpty() && (identity == null || identity.isEmpty()) && (uri == null || uri.isEmpty()) 804 && (name == null || name.isEmpty()) && (comments == null || comments.isEmpty()); 805 } 806 807 public String fhirType() { 808 return "DataElement.mapping"; 809 810 } 811 812 } 813 814 /** 815 * An absolute URL that is used to identify this data element when it is 816 * referenced in a specification, model, design or an instance. This SHALL be a 817 * URL, SHOULD be globally unique, and SHOULD be an address at which this data 818 * element is (or will be) published. 819 */ 820 @Child(name = "url", type = { UriType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 821 @Description(shortDefinition = "Globally unique logical id for data element", formalDefinition = "An absolute URL that is used to identify this data element when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this data element is (or will be) published.") 822 protected UriType url; 823 824 /** 825 * Formal identifier that is used to identify this data element when it is 826 * represented in other formats, or referenced in a specification, model, design 827 * or an instance. 828 */ 829 @Child(name = "identifier", type = { 830 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 831 @Description(shortDefinition = "Logical id to reference this data element", formalDefinition = "Formal identifier that is used to identify this data element when it is represented in other formats, or referenced in a specification, model, design or an instance.") 832 protected List<Identifier> identifier; 833 834 /** 835 * The identifier that is used to identify this version of the data element when 836 * it is referenced in a StructureDefinition, Questionnaire or instance. This is 837 * an arbitrary value managed by the definition author manually. 838 */ 839 @Child(name = "version", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 840 @Description(shortDefinition = "Logical id for this version of the data element", formalDefinition = "The identifier that is used to identify this version of the data element when it is referenced in a StructureDefinition, Questionnaire or instance. This is an arbitrary value managed by the definition author manually.") 841 protected StringType version; 842 843 /** 844 * The term used by humans to refer to the data element. Should ideally be 845 * unique within the context in which the data element is expected to be used. 846 */ 847 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 848 @Description(shortDefinition = "Descriptive label for this element definition", formalDefinition = "The term used by humans to refer to the data element. Should ideally be unique within the context in which the data element is expected to be used.") 849 protected StringType name; 850 851 /** 852 * The status of the data element. 853 */ 854 @Child(name = "status", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = true, summary = true) 855 @Description(shortDefinition = "draft | active | retired", formalDefinition = "The status of the data element.") 856 protected Enumeration<ConformanceResourceStatus> status; 857 858 /** 859 * A flag to indicate that this search data element definition is authored for 860 * testing purposes (or education/evaluation/marketing), and is not intended to 861 * be used for genuine usage. 862 */ 863 @Child(name = "experimental", type = { 864 BooleanType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 865 @Description(shortDefinition = "If for testing purposes, not real usage", formalDefinition = "A flag to indicate that this search data element definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.") 866 protected BooleanType experimental; 867 868 /** 869 * The name of the individual or organization that published the data element. 870 */ 871 @Child(name = "publisher", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 872 @Description(shortDefinition = "Name of the publisher (Organization or individual)", formalDefinition = "The name of the individual or organization that published the data element.") 873 protected StringType publisher; 874 875 /** 876 * Contacts to assist a user in finding and communicating with the publisher. 877 */ 878 @Child(name = "contact", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 879 @Description(shortDefinition = "Contact details of the publisher", formalDefinition = "Contacts to assist a user in finding and communicating with the publisher.") 880 protected List<DataElementContactComponent> contact; 881 882 /** 883 * The date this version of the data element was published. The date must change 884 * when the business version changes, if it does, and it must change if the 885 * status code changes. In addition, it should change when the substantive 886 * content of the data element changes. 887 */ 888 @Child(name = "date", type = { DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 889 @Description(shortDefinition = "Date for this version of the data element", formalDefinition = "The date this version of the data element was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the data element changes.") 890 protected DateTimeType date; 891 892 /** 893 * The content was developed with a focus and intent of supporting the contexts 894 * that are listed. These terms may be used to assist with indexing and 895 * searching of data element definitions. 896 */ 897 @Child(name = "useContext", type = { 898 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 899 @Description(shortDefinition = "Content intends to support these contexts", formalDefinition = "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of data element definitions.") 900 protected List<CodeableConcept> useContext; 901 902 /** 903 * A copyright statement relating to the definition of the data element. 904 * Copyright statements are generally legal restrictions on the use and 905 * publishing of the details of the definition of the data element. 906 */ 907 @Child(name = "copyright", type = { 908 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 909 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the definition of the data element. Copyright statements are generally legal restrictions on the use and publishing of the details of the definition of the data element.") 910 protected StringType copyright; 911 912 /** 913 * Identifies how precise the data element is in its definition. 914 */ 915 @Child(name = "stringency", type = { CodeType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 916 @Description(shortDefinition = "comparable | fully-specified | equivalent | convertable | scaleable | flexible", formalDefinition = "Identifies how precise the data element is in its definition.") 917 protected Enumeration<DataElementStringency> stringency; 918 919 /** 920 * Identifies a specification (other than a terminology) that the elements which 921 * make up the DataElement have some correspondence with. 922 */ 923 @Child(name = "mapping", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 924 @Description(shortDefinition = "External specification mapped to", formalDefinition = "Identifies a specification (other than a terminology) that the elements which make up the DataElement have some correspondence with.") 925 protected List<DataElementMappingComponent> mapping; 926 927 /** 928 * Defines the structure, type, allowed values and other constraining 929 * characteristics of the data element. 930 */ 931 @Child(name = "element", type = { 932 ElementDefinition.class }, order = 13, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 933 @Description(shortDefinition = "Definition of element", formalDefinition = "Defines the structure, type, allowed values and other constraining characteristics of the data element.") 934 protected List<ElementDefinition> element; 935 936 private static final long serialVersionUID = 2017352331L; 937 938 /* 939 * Constructor 940 */ 941 public DataElement() { 942 super(); 943 } 944 945 /* 946 * Constructor 947 */ 948 public DataElement(Enumeration<ConformanceResourceStatus> status) { 949 super(); 950 this.status = status; 951 } 952 953 /** 954 * @return {@link #url} (An absolute URL that is used to identify this data 955 * element when it is referenced in a specification, model, design or an 956 * instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD 957 * be an address at which this data element is (or will be) published.). 958 * This is the underlying object with id, value and extensions. The 959 * accessor "getUrl" gives direct access to the value 960 */ 961 public UriType getUrlElement() { 962 if (this.url == null) 963 if (Configuration.errorOnAutoCreate()) 964 throw new Error("Attempt to auto-create DataElement.url"); 965 else if (Configuration.doAutoCreate()) 966 this.url = new UriType(); // bb 967 return this.url; 968 } 969 970 public boolean hasUrlElement() { 971 return this.url != null && !this.url.isEmpty(); 972 } 973 974 public boolean hasUrl() { 975 return this.url != null && !this.url.isEmpty(); 976 } 977 978 /** 979 * @param value {@link #url} (An absolute URL that is used to identify this data 980 * element when it is referenced in a specification, model, design 981 * or an instance. This SHALL be a URL, SHOULD be globally unique, 982 * and SHOULD be an address at which this data element is (or will 983 * be) published.). This is the underlying object with id, value 984 * and extensions. The accessor "getUrl" gives direct access to the 985 * value 986 */ 987 public DataElement setUrlElement(UriType value) { 988 this.url = value; 989 return this; 990 } 991 992 /** 993 * @return An absolute URL that is used to identify this data element when it is 994 * referenced in a specification, model, design or an instance. This 995 * SHALL be a URL, SHOULD be globally unique, and SHOULD be an address 996 * at which this data element is (or will be) published. 997 */ 998 public String getUrl() { 999 return this.url == null ? null : this.url.getValue(); 1000 } 1001 1002 /** 1003 * @param value An absolute URL that is used to identify this data element when 1004 * it is referenced in a specification, model, design or an 1005 * instance. This SHALL be a URL, SHOULD be globally unique, and 1006 * SHOULD be an address at which this data element is (or will be) 1007 * published. 1008 */ 1009 public DataElement setUrl(String value) { 1010 if (Utilities.noString(value)) 1011 this.url = null; 1012 else { 1013 if (this.url == null) 1014 this.url = new UriType(); 1015 this.url.setValue(value); 1016 } 1017 return this; 1018 } 1019 1020 /** 1021 * @return {@link #identifier} (Formal identifier that is used to identify this 1022 * data element when it is represented in other formats, or referenced 1023 * in a specification, model, design or an instance.) 1024 */ 1025 public List<Identifier> getIdentifier() { 1026 if (this.identifier == null) 1027 this.identifier = new ArrayList<Identifier>(); 1028 return this.identifier; 1029 } 1030 1031 public boolean hasIdentifier() { 1032 if (this.identifier == null) 1033 return false; 1034 for (Identifier item : this.identifier) 1035 if (!item.isEmpty()) 1036 return true; 1037 return false; 1038 } 1039 1040 /** 1041 * @return {@link #identifier} (Formal identifier that is used to identify this 1042 * data element when it is represented in other formats, or referenced 1043 * in a specification, model, design or an instance.) 1044 */ 1045 // syntactic sugar 1046 public Identifier addIdentifier() { // 3 1047 Identifier t = new Identifier(); 1048 if (this.identifier == null) 1049 this.identifier = new ArrayList<Identifier>(); 1050 this.identifier.add(t); 1051 return t; 1052 } 1053 1054 // syntactic sugar 1055 public DataElement addIdentifier(Identifier t) { // 3 1056 if (t == null) 1057 return this; 1058 if (this.identifier == null) 1059 this.identifier = new ArrayList<Identifier>(); 1060 this.identifier.add(t); 1061 return this; 1062 } 1063 1064 /** 1065 * @return {@link #version} (The identifier that is used to identify this 1066 * version of the data element when it is referenced in a 1067 * StructureDefinition, Questionnaire or instance. This is an arbitrary 1068 * value managed by the definition author manually.). This is the 1069 * underlying object with id, value and extensions. The accessor 1070 * "getVersion" gives direct access to the value 1071 */ 1072 public StringType getVersionElement() { 1073 if (this.version == null) 1074 if (Configuration.errorOnAutoCreate()) 1075 throw new Error("Attempt to auto-create DataElement.version"); 1076 else if (Configuration.doAutoCreate()) 1077 this.version = new StringType(); // bb 1078 return this.version; 1079 } 1080 1081 public boolean hasVersionElement() { 1082 return this.version != null && !this.version.isEmpty(); 1083 } 1084 1085 public boolean hasVersion() { 1086 return this.version != null && !this.version.isEmpty(); 1087 } 1088 1089 /** 1090 * @param value {@link #version} (The identifier that is used to identify this 1091 * version of the data element when it is referenced in a 1092 * StructureDefinition, Questionnaire or instance. This is an 1093 * arbitrary value managed by the definition author manually.). 1094 * This is the underlying object with id, value and extensions. The 1095 * accessor "getVersion" gives direct access to the value 1096 */ 1097 public DataElement setVersionElement(StringType value) { 1098 this.version = value; 1099 return this; 1100 } 1101 1102 /** 1103 * @return The identifier that is used to identify this version of the data 1104 * element when it is referenced in a StructureDefinition, Questionnaire 1105 * or instance. This is an arbitrary value managed by the definition 1106 * author manually. 1107 */ 1108 public String getVersion() { 1109 return this.version == null ? null : this.version.getValue(); 1110 } 1111 1112 /** 1113 * @param value The identifier that is used to identify this version of the data 1114 * element when it is referenced in a StructureDefinition, 1115 * Questionnaire or instance. This is an arbitrary value managed by 1116 * the definition author manually. 1117 */ 1118 public DataElement setVersion(String value) { 1119 if (Utilities.noString(value)) 1120 this.version = null; 1121 else { 1122 if (this.version == null) 1123 this.version = new StringType(); 1124 this.version.setValue(value); 1125 } 1126 return this; 1127 } 1128 1129 /** 1130 * @return {@link #name} (The term used by humans to refer to the data element. 1131 * Should ideally be unique within the context in which the data element 1132 * is expected to be used.). This is the underlying object with id, 1133 * value and extensions. The accessor "getName" gives direct access to 1134 * the value 1135 */ 1136 public StringType getNameElement() { 1137 if (this.name == null) 1138 if (Configuration.errorOnAutoCreate()) 1139 throw new Error("Attempt to auto-create DataElement.name"); 1140 else if (Configuration.doAutoCreate()) 1141 this.name = new StringType(); // bb 1142 return this.name; 1143 } 1144 1145 public boolean hasNameElement() { 1146 return this.name != null && !this.name.isEmpty(); 1147 } 1148 1149 public boolean hasName() { 1150 return this.name != null && !this.name.isEmpty(); 1151 } 1152 1153 /** 1154 * @param value {@link #name} (The term used by humans to refer to the data 1155 * element. Should ideally be unique within the context in which 1156 * the data element is expected to be used.). This is the 1157 * underlying object with id, value and extensions. The accessor 1158 * "getName" gives direct access to the value 1159 */ 1160 public DataElement setNameElement(StringType value) { 1161 this.name = value; 1162 return this; 1163 } 1164 1165 /** 1166 * @return The term used by humans to refer to the data element. Should ideally 1167 * be unique within the context in which the data element is expected to 1168 * be used. 1169 */ 1170 public String getName() { 1171 return this.name == null ? null : this.name.getValue(); 1172 } 1173 1174 /** 1175 * @param value The term used by humans to refer to the data element. Should 1176 * ideally be unique within the context in which the data element 1177 * is expected to be used. 1178 */ 1179 public DataElement setName(String value) { 1180 if (Utilities.noString(value)) 1181 this.name = null; 1182 else { 1183 if (this.name == null) 1184 this.name = new StringType(); 1185 this.name.setValue(value); 1186 } 1187 return this; 1188 } 1189 1190 /** 1191 * @return {@link #status} (The status of the data element.). This is the 1192 * underlying object with id, value and extensions. The accessor 1193 * "getStatus" gives direct access to the value 1194 */ 1195 public Enumeration<ConformanceResourceStatus> getStatusElement() { 1196 if (this.status == null) 1197 if (Configuration.errorOnAutoCreate()) 1198 throw new Error("Attempt to auto-create DataElement.status"); 1199 else if (Configuration.doAutoCreate()) 1200 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); // bb 1201 return this.status; 1202 } 1203 1204 public boolean hasStatusElement() { 1205 return this.status != null && !this.status.isEmpty(); 1206 } 1207 1208 public boolean hasStatus() { 1209 return this.status != null && !this.status.isEmpty(); 1210 } 1211 1212 /** 1213 * @param value {@link #status} (The status of the data element.). This is the 1214 * underlying object with id, value and extensions. The accessor 1215 * "getStatus" gives direct access to the value 1216 */ 1217 public DataElement setStatusElement(Enumeration<ConformanceResourceStatus> value) { 1218 this.status = value; 1219 return this; 1220 } 1221 1222 /** 1223 * @return The status of the data element. 1224 */ 1225 public ConformanceResourceStatus getStatus() { 1226 return this.status == null ? null : this.status.getValue(); 1227 } 1228 1229 /** 1230 * @param value The status of the data element. 1231 */ 1232 public DataElement setStatus(ConformanceResourceStatus value) { 1233 if (this.status == null) 1234 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); 1235 this.status.setValue(value); 1236 return this; 1237 } 1238 1239 /** 1240 * @return {@link #experimental} (A flag to indicate that this search data 1241 * element definition is authored for testing purposes (or 1242 * education/evaluation/marketing), and is not intended to be used for 1243 * genuine usage.). This is the underlying object with id, value and 1244 * extensions. The accessor "getExperimental" gives direct access to the 1245 * value 1246 */ 1247 public BooleanType getExperimentalElement() { 1248 if (this.experimental == null) 1249 if (Configuration.errorOnAutoCreate()) 1250 throw new Error("Attempt to auto-create DataElement.experimental"); 1251 else if (Configuration.doAutoCreate()) 1252 this.experimental = new BooleanType(); // bb 1253 return this.experimental; 1254 } 1255 1256 public boolean hasExperimentalElement() { 1257 return this.experimental != null && !this.experimental.isEmpty(); 1258 } 1259 1260 public boolean hasExperimental() { 1261 return this.experimental != null && !this.experimental.isEmpty(); 1262 } 1263 1264 /** 1265 * @param value {@link #experimental} (A flag to indicate that this search data 1266 * element definition is authored for testing purposes (or 1267 * education/evaluation/marketing), and is not intended to be used 1268 * for genuine usage.). This is the underlying object with id, 1269 * value and extensions. The accessor "getExperimental" gives 1270 * direct access to the value 1271 */ 1272 public DataElement setExperimentalElement(BooleanType value) { 1273 this.experimental = value; 1274 return this; 1275 } 1276 1277 /** 1278 * @return A flag to indicate that this search data element definition is 1279 * authored for testing purposes (or education/evaluation/marketing), 1280 * and is not intended to be used for genuine usage. 1281 */ 1282 public boolean getExperimental() { 1283 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1284 } 1285 1286 /** 1287 * @param value A flag to indicate that this search data element definition is 1288 * authored for testing purposes (or 1289 * education/evaluation/marketing), and is not intended to be used 1290 * for genuine usage. 1291 */ 1292 public DataElement setExperimental(boolean value) { 1293 if (this.experimental == null) 1294 this.experimental = new BooleanType(); 1295 this.experimental.setValue(value); 1296 return this; 1297 } 1298 1299 /** 1300 * @return {@link #publisher} (The name of the individual or organization that 1301 * published the data element.). This is the underlying object with id, 1302 * value and extensions. The accessor "getPublisher" gives direct access 1303 * to the value 1304 */ 1305 public StringType getPublisherElement() { 1306 if (this.publisher == null) 1307 if (Configuration.errorOnAutoCreate()) 1308 throw new Error("Attempt to auto-create DataElement.publisher"); 1309 else if (Configuration.doAutoCreate()) 1310 this.publisher = new StringType(); // bb 1311 return this.publisher; 1312 } 1313 1314 public boolean hasPublisherElement() { 1315 return this.publisher != null && !this.publisher.isEmpty(); 1316 } 1317 1318 public boolean hasPublisher() { 1319 return this.publisher != null && !this.publisher.isEmpty(); 1320 } 1321 1322 /** 1323 * @param value {@link #publisher} (The name of the individual or organization 1324 * that published the data element.). This is the underlying object 1325 * with id, value and extensions. The accessor "getPublisher" gives 1326 * direct access to the value 1327 */ 1328 public DataElement setPublisherElement(StringType value) { 1329 this.publisher = value; 1330 return this; 1331 } 1332 1333 /** 1334 * @return The name of the individual or organization that published the data 1335 * element. 1336 */ 1337 public String getPublisher() { 1338 return this.publisher == null ? null : this.publisher.getValue(); 1339 } 1340 1341 /** 1342 * @param value The name of the individual or organization that published the 1343 * data element. 1344 */ 1345 public DataElement setPublisher(String value) { 1346 if (Utilities.noString(value)) 1347 this.publisher = null; 1348 else { 1349 if (this.publisher == null) 1350 this.publisher = new StringType(); 1351 this.publisher.setValue(value); 1352 } 1353 return this; 1354 } 1355 1356 /** 1357 * @return {@link #contact} (Contacts to assist a user in finding and 1358 * communicating with the publisher.) 1359 */ 1360 public List<DataElementContactComponent> getContact() { 1361 if (this.contact == null) 1362 this.contact = new ArrayList<DataElementContactComponent>(); 1363 return this.contact; 1364 } 1365 1366 public boolean hasContact() { 1367 if (this.contact == null) 1368 return false; 1369 for (DataElementContactComponent item : this.contact) 1370 if (!item.isEmpty()) 1371 return true; 1372 return false; 1373 } 1374 1375 /** 1376 * @return {@link #contact} (Contacts to assist a user in finding and 1377 * communicating with the publisher.) 1378 */ 1379 // syntactic sugar 1380 public DataElementContactComponent addContact() { // 3 1381 DataElementContactComponent t = new DataElementContactComponent(); 1382 if (this.contact == null) 1383 this.contact = new ArrayList<DataElementContactComponent>(); 1384 this.contact.add(t); 1385 return t; 1386 } 1387 1388 // syntactic sugar 1389 public DataElement addContact(DataElementContactComponent t) { // 3 1390 if (t == null) 1391 return this; 1392 if (this.contact == null) 1393 this.contact = new ArrayList<DataElementContactComponent>(); 1394 this.contact.add(t); 1395 return this; 1396 } 1397 1398 /** 1399 * @return {@link #date} (The date this version of the data element was 1400 * published. The date must change when the business version changes, if 1401 * it does, and it must change if the status code changes. In addition, 1402 * it should change when the substantive content of the data element 1403 * changes.). This is the underlying object with id, value and 1404 * extensions. The accessor "getDate" gives direct access to the value 1405 */ 1406 public DateTimeType getDateElement() { 1407 if (this.date == null) 1408 if (Configuration.errorOnAutoCreate()) 1409 throw new Error("Attempt to auto-create DataElement.date"); 1410 else if (Configuration.doAutoCreate()) 1411 this.date = new DateTimeType(); // bb 1412 return this.date; 1413 } 1414 1415 public boolean hasDateElement() { 1416 return this.date != null && !this.date.isEmpty(); 1417 } 1418 1419 public boolean hasDate() { 1420 return this.date != null && !this.date.isEmpty(); 1421 } 1422 1423 /** 1424 * @param value {@link #date} (The date this version of the data element was 1425 * published. The date must change when the business version 1426 * changes, if it does, and it must change if the status code 1427 * changes. In addition, it should change when the substantive 1428 * content of the data element changes.). This is the underlying 1429 * object with id, value and extensions. The accessor "getDate" 1430 * gives direct access to the value 1431 */ 1432 public DataElement setDateElement(DateTimeType value) { 1433 this.date = value; 1434 return this; 1435 } 1436 1437 /** 1438 * @return The date this version of the data element was published. The date 1439 * must change when the business version changes, if it does, and it 1440 * must change if the status code changes. In addition, it should change 1441 * when the substantive content of the data element changes. 1442 */ 1443 public Date getDate() { 1444 return this.date == null ? null : this.date.getValue(); 1445 } 1446 1447 /** 1448 * @param value The date this version of the data element was published. The 1449 * date must change when the business version changes, if it does, 1450 * and it must change if the status code changes. In addition, it 1451 * should change when the substantive content of the data element 1452 * changes. 1453 */ 1454 public DataElement setDate(Date value) { 1455 if (value == null) 1456 this.date = null; 1457 else { 1458 if (this.date == null) 1459 this.date = new DateTimeType(); 1460 this.date.setValue(value); 1461 } 1462 return this; 1463 } 1464 1465 /** 1466 * @return {@link #useContext} (The content was developed with a focus and 1467 * intent of supporting the contexts that are listed. These terms may be 1468 * used to assist with indexing and searching of data element 1469 * definitions.) 1470 */ 1471 public List<CodeableConcept> getUseContext() { 1472 if (this.useContext == null) 1473 this.useContext = new ArrayList<CodeableConcept>(); 1474 return this.useContext; 1475 } 1476 1477 public boolean hasUseContext() { 1478 if (this.useContext == null) 1479 return false; 1480 for (CodeableConcept item : this.useContext) 1481 if (!item.isEmpty()) 1482 return true; 1483 return false; 1484 } 1485 1486 /** 1487 * @return {@link #useContext} (The content was developed with a focus and 1488 * intent of supporting the contexts that are listed. These terms may be 1489 * used to assist with indexing and searching of data element 1490 * definitions.) 1491 */ 1492 // syntactic sugar 1493 public CodeableConcept addUseContext() { // 3 1494 CodeableConcept t = new CodeableConcept(); 1495 if (this.useContext == null) 1496 this.useContext = new ArrayList<CodeableConcept>(); 1497 this.useContext.add(t); 1498 return t; 1499 } 1500 1501 // syntactic sugar 1502 public DataElement addUseContext(CodeableConcept t) { // 3 1503 if (t == null) 1504 return this; 1505 if (this.useContext == null) 1506 this.useContext = new ArrayList<CodeableConcept>(); 1507 this.useContext.add(t); 1508 return this; 1509 } 1510 1511 /** 1512 * @return {@link #copyright} (A copyright statement relating to the definition 1513 * of the data element. Copyright statements are generally legal 1514 * restrictions on the use and publishing of the details of the 1515 * definition of the data element.). This is the underlying object with 1516 * id, value and extensions. The accessor "getCopyright" gives direct 1517 * access to the value 1518 */ 1519 public StringType getCopyrightElement() { 1520 if (this.copyright == null) 1521 if (Configuration.errorOnAutoCreate()) 1522 throw new Error("Attempt to auto-create DataElement.copyright"); 1523 else if (Configuration.doAutoCreate()) 1524 this.copyright = new StringType(); // bb 1525 return this.copyright; 1526 } 1527 1528 public boolean hasCopyrightElement() { 1529 return this.copyright != null && !this.copyright.isEmpty(); 1530 } 1531 1532 public boolean hasCopyright() { 1533 return this.copyright != null && !this.copyright.isEmpty(); 1534 } 1535 1536 /** 1537 * @param value {@link #copyright} (A copyright statement relating to the 1538 * definition of the data element. Copyright statements are 1539 * generally legal restrictions on the use and publishing of the 1540 * details of the definition of the data element.). This is the 1541 * underlying object with id, value and extensions. The accessor 1542 * "getCopyright" gives direct access to the value 1543 */ 1544 public DataElement setCopyrightElement(StringType value) { 1545 this.copyright = value; 1546 return this; 1547 } 1548 1549 /** 1550 * @return A copyright statement relating to the definition of the data element. 1551 * Copyright statements are generally legal restrictions on the use and 1552 * publishing of the details of the definition of the data element. 1553 */ 1554 public String getCopyright() { 1555 return this.copyright == null ? null : this.copyright.getValue(); 1556 } 1557 1558 /** 1559 * @param value A copyright statement relating to the definition of the data 1560 * element. Copyright statements are generally legal restrictions 1561 * on the use and publishing of the details of the definition of 1562 * the data element. 1563 */ 1564 public DataElement setCopyright(String value) { 1565 if (Utilities.noString(value)) 1566 this.copyright = null; 1567 else { 1568 if (this.copyright == null) 1569 this.copyright = new StringType(); 1570 this.copyright.setValue(value); 1571 } 1572 return this; 1573 } 1574 1575 /** 1576 * @return {@link #stringency} (Identifies how precise the data element is in 1577 * its definition.). This is the underlying object with id, value and 1578 * extensions. The accessor "getStringency" gives direct access to the 1579 * value 1580 */ 1581 public Enumeration<DataElementStringency> getStringencyElement() { 1582 if (this.stringency == null) 1583 if (Configuration.errorOnAutoCreate()) 1584 throw new Error("Attempt to auto-create DataElement.stringency"); 1585 else if (Configuration.doAutoCreate()) 1586 this.stringency = new Enumeration<DataElementStringency>(new DataElementStringencyEnumFactory()); // bb 1587 return this.stringency; 1588 } 1589 1590 public boolean hasStringencyElement() { 1591 return this.stringency != null && !this.stringency.isEmpty(); 1592 } 1593 1594 public boolean hasStringency() { 1595 return this.stringency != null && !this.stringency.isEmpty(); 1596 } 1597 1598 /** 1599 * @param value {@link #stringency} (Identifies how precise the data element is 1600 * in its definition.). This is the underlying object with id, 1601 * value and extensions. The accessor "getStringency" gives direct 1602 * access to the value 1603 */ 1604 public DataElement setStringencyElement(Enumeration<DataElementStringency> value) { 1605 this.stringency = value; 1606 return this; 1607 } 1608 1609 /** 1610 * @return Identifies how precise the data element is in its definition. 1611 */ 1612 public DataElementStringency getStringency() { 1613 return this.stringency == null ? null : this.stringency.getValue(); 1614 } 1615 1616 /** 1617 * @param value Identifies how precise the data element is in its definition. 1618 */ 1619 public DataElement setStringency(DataElementStringency value) { 1620 if (value == null) 1621 this.stringency = null; 1622 else { 1623 if (this.stringency == null) 1624 this.stringency = new Enumeration<DataElementStringency>(new DataElementStringencyEnumFactory()); 1625 this.stringency.setValue(value); 1626 } 1627 return this; 1628 } 1629 1630 /** 1631 * @return {@link #mapping} (Identifies a specification (other than a 1632 * terminology) that the elements which make up the DataElement have 1633 * some correspondence with.) 1634 */ 1635 public List<DataElementMappingComponent> getMapping() { 1636 if (this.mapping == null) 1637 this.mapping = new ArrayList<DataElementMappingComponent>(); 1638 return this.mapping; 1639 } 1640 1641 public boolean hasMapping() { 1642 if (this.mapping == null) 1643 return false; 1644 for (DataElementMappingComponent item : this.mapping) 1645 if (!item.isEmpty()) 1646 return true; 1647 return false; 1648 } 1649 1650 /** 1651 * @return {@link #mapping} (Identifies a specification (other than a 1652 * terminology) that the elements which make up the DataElement have 1653 * some correspondence with.) 1654 */ 1655 // syntactic sugar 1656 public DataElementMappingComponent addMapping() { // 3 1657 DataElementMappingComponent t = new DataElementMappingComponent(); 1658 if (this.mapping == null) 1659 this.mapping = new ArrayList<DataElementMappingComponent>(); 1660 this.mapping.add(t); 1661 return t; 1662 } 1663 1664 // syntactic sugar 1665 public DataElement addMapping(DataElementMappingComponent t) { // 3 1666 if (t == null) 1667 return this; 1668 if (this.mapping == null) 1669 this.mapping = new ArrayList<DataElementMappingComponent>(); 1670 this.mapping.add(t); 1671 return this; 1672 } 1673 1674 /** 1675 * @return {@link #element} (Defines the structure, type, allowed values and 1676 * other constraining characteristics of the data element.) 1677 */ 1678 public List<ElementDefinition> getElement() { 1679 if (this.element == null) 1680 this.element = new ArrayList<ElementDefinition>(); 1681 return this.element; 1682 } 1683 1684 public boolean hasElement() { 1685 if (this.element == null) 1686 return false; 1687 for (ElementDefinition item : this.element) 1688 if (!item.isEmpty()) 1689 return true; 1690 return false; 1691 } 1692 1693 /** 1694 * @return {@link #element} (Defines the structure, type, allowed values and 1695 * other constraining characteristics of the data element.) 1696 */ 1697 // syntactic sugar 1698 public ElementDefinition addElement() { // 3 1699 ElementDefinition t = new ElementDefinition(); 1700 if (this.element == null) 1701 this.element = new ArrayList<ElementDefinition>(); 1702 this.element.add(t); 1703 return t; 1704 } 1705 1706 // syntactic sugar 1707 public DataElement addElement(ElementDefinition t) { // 3 1708 if (t == null) 1709 return this; 1710 if (this.element == null) 1711 this.element = new ArrayList<ElementDefinition>(); 1712 this.element.add(t); 1713 return this; 1714 } 1715 1716 protected void listChildren(List<Property> childrenList) { 1717 super.listChildren(childrenList); 1718 childrenList.add(new Property("url", "uri", 1719 "An absolute URL that is used to identify this data element when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this data element is (or will be) published.", 1720 0, java.lang.Integer.MAX_VALUE, url)); 1721 childrenList.add(new Property("identifier", "Identifier", 1722 "Formal identifier that is used to identify this data element when it is represented in other formats, or referenced in a specification, model, design or an instance.", 1723 0, java.lang.Integer.MAX_VALUE, identifier)); 1724 childrenList.add(new Property("version", "string", 1725 "The identifier that is used to identify this version of the data element when it is referenced in a StructureDefinition, Questionnaire or instance. This is an arbitrary value managed by the definition author manually.", 1726 0, java.lang.Integer.MAX_VALUE, version)); 1727 childrenList.add(new Property("name", "string", 1728 "The term used by humans to refer to the data element. Should ideally be unique within the context in which the data element is expected to be used.", 1729 0, java.lang.Integer.MAX_VALUE, name)); 1730 childrenList 1731 .add(new Property("status", "code", "The status of the data element.", 0, java.lang.Integer.MAX_VALUE, status)); 1732 childrenList.add(new Property("experimental", "boolean", 1733 "A flag to indicate that this search data element definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 1734 0, java.lang.Integer.MAX_VALUE, experimental)); 1735 childrenList.add(new Property("publisher", "string", 1736 "The name of the individual or organization that published the data element.", 0, java.lang.Integer.MAX_VALUE, 1737 publisher)); 1738 childrenList 1739 .add(new Property("contact", "", "Contacts to assist a user in finding and communicating with the publisher.", 1740 0, java.lang.Integer.MAX_VALUE, contact)); 1741 childrenList.add(new Property("date", "dateTime", 1742 "The date this version of the data element was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the data element changes.", 1743 0, java.lang.Integer.MAX_VALUE, date)); 1744 childrenList.add(new Property("useContext", "CodeableConcept", 1745 "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of data element definitions.", 1746 0, java.lang.Integer.MAX_VALUE, useContext)); 1747 childrenList.add(new Property("copyright", "string", 1748 "A copyright statement relating to the definition of the data element. Copyright statements are generally legal restrictions on the use and publishing of the details of the definition of the data element.", 1749 0, java.lang.Integer.MAX_VALUE, copyright)); 1750 childrenList.add(new Property("stringency", "code", "Identifies how precise the data element is in its definition.", 1751 0, java.lang.Integer.MAX_VALUE, stringency)); 1752 childrenList.add(new Property("mapping", "", 1753 "Identifies a specification (other than a terminology) that the elements which make up the DataElement have some correspondence with.", 1754 0, java.lang.Integer.MAX_VALUE, mapping)); 1755 childrenList.add(new Property("element", "ElementDefinition", 1756 "Defines the structure, type, allowed values and other constraining characteristics of the data element.", 0, 1757 java.lang.Integer.MAX_VALUE, element)); 1758 } 1759 1760 @Override 1761 public void setProperty(String name, Base value) throws FHIRException { 1762 if (name.equals("url")) 1763 this.url = castToUri(value); // UriType 1764 else if (name.equals("identifier")) 1765 this.getIdentifier().add(castToIdentifier(value)); 1766 else if (name.equals("version")) 1767 this.version = castToString(value); // StringType 1768 else if (name.equals("name")) 1769 this.name = castToString(value); // StringType 1770 else if (name.equals("status")) 1771 this.status = new ConformanceResourceStatusEnumFactory().fromType(value); // Enumeration<ConformanceResourceStatus> 1772 else if (name.equals("experimental")) 1773 this.experimental = castToBoolean(value); // BooleanType 1774 else if (name.equals("publisher")) 1775 this.publisher = castToString(value); // StringType 1776 else if (name.equals("contact")) 1777 this.getContact().add((DataElementContactComponent) value); 1778 else if (name.equals("date")) 1779 this.date = castToDateTime(value); // DateTimeType 1780 else if (name.equals("useContext")) 1781 this.getUseContext().add(castToCodeableConcept(value)); 1782 else if (name.equals("copyright")) 1783 this.copyright = castToString(value); // StringType 1784 else if (name.equals("stringency")) 1785 this.stringency = new DataElementStringencyEnumFactory().fromType(value); // Enumeration<DataElementStringency> 1786 else if (name.equals("mapping")) 1787 this.getMapping().add((DataElementMappingComponent) value); 1788 else if (name.equals("element")) 1789 this.getElement().add(castToElementDefinition(value)); 1790 else 1791 super.setProperty(name, value); 1792 } 1793 1794 @Override 1795 public Base addChild(String name) throws FHIRException { 1796 if (name.equals("url")) { 1797 throw new FHIRException("Cannot call addChild on a singleton property DataElement.url"); 1798 } else if (name.equals("identifier")) { 1799 return addIdentifier(); 1800 } else if (name.equals("version")) { 1801 throw new FHIRException("Cannot call addChild on a singleton property DataElement.version"); 1802 } else if (name.equals("name")) { 1803 throw new FHIRException("Cannot call addChild on a singleton property DataElement.name"); 1804 } else if (name.equals("status")) { 1805 throw new FHIRException("Cannot call addChild on a singleton property DataElement.status"); 1806 } else if (name.equals("experimental")) { 1807 throw new FHIRException("Cannot call addChild on a singleton property DataElement.experimental"); 1808 } else if (name.equals("publisher")) { 1809 throw new FHIRException("Cannot call addChild on a singleton property DataElement.publisher"); 1810 } else if (name.equals("contact")) { 1811 return addContact(); 1812 } else if (name.equals("date")) { 1813 throw new FHIRException("Cannot call addChild on a singleton property DataElement.date"); 1814 } else if (name.equals("useContext")) { 1815 return addUseContext(); 1816 } else if (name.equals("copyright")) { 1817 throw new FHIRException("Cannot call addChild on a singleton property DataElement.copyright"); 1818 } else if (name.equals("stringency")) { 1819 throw new FHIRException("Cannot call addChild on a singleton property DataElement.stringency"); 1820 } else if (name.equals("mapping")) { 1821 return addMapping(); 1822 } else if (name.equals("element")) { 1823 return addElement(); 1824 } else 1825 return super.addChild(name); 1826 } 1827 1828 public String fhirType() { 1829 return "DataElement"; 1830 1831 } 1832 1833 public DataElement copy() { 1834 DataElement dst = new DataElement(); 1835 copyValues(dst); 1836 dst.url = url == null ? null : url.copy(); 1837 if (identifier != null) { 1838 dst.identifier = new ArrayList<Identifier>(); 1839 for (Identifier i : identifier) 1840 dst.identifier.add(i.copy()); 1841 } 1842 ; 1843 dst.version = version == null ? null : version.copy(); 1844 dst.name = name == null ? null : name.copy(); 1845 dst.status = status == null ? null : status.copy(); 1846 dst.experimental = experimental == null ? null : experimental.copy(); 1847 dst.publisher = publisher == null ? null : publisher.copy(); 1848 if (contact != null) { 1849 dst.contact = new ArrayList<DataElementContactComponent>(); 1850 for (DataElementContactComponent i : contact) 1851 dst.contact.add(i.copy()); 1852 } 1853 ; 1854 dst.date = date == null ? null : date.copy(); 1855 if (useContext != null) { 1856 dst.useContext = new ArrayList<CodeableConcept>(); 1857 for (CodeableConcept i : useContext) 1858 dst.useContext.add(i.copy()); 1859 } 1860 ; 1861 dst.copyright = copyright == null ? null : copyright.copy(); 1862 dst.stringency = stringency == null ? null : stringency.copy(); 1863 if (mapping != null) { 1864 dst.mapping = new ArrayList<DataElementMappingComponent>(); 1865 for (DataElementMappingComponent i : mapping) 1866 dst.mapping.add(i.copy()); 1867 } 1868 ; 1869 if (element != null) { 1870 dst.element = new ArrayList<ElementDefinition>(); 1871 for (ElementDefinition i : element) 1872 dst.element.add(i.copy()); 1873 } 1874 ; 1875 return dst; 1876 } 1877 1878 protected DataElement typedCopy() { 1879 return copy(); 1880 } 1881 1882 @Override 1883 public boolean equalsDeep(Base other) { 1884 if (!super.equalsDeep(other)) 1885 return false; 1886 if (!(other instanceof DataElement)) 1887 return false; 1888 DataElement o = (DataElement) other; 1889 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) 1890 && compareDeep(version, o.version, true) && compareDeep(name, o.name, true) 1891 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 1892 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 1893 && compareDeep(date, o.date, true) && compareDeep(useContext, o.useContext, true) 1894 && compareDeep(copyright, o.copyright, true) && compareDeep(stringency, o.stringency, true) 1895 && compareDeep(mapping, o.mapping, true) && compareDeep(element, o.element, true); 1896 } 1897 1898 @Override 1899 public boolean equalsShallow(Base other) { 1900 if (!super.equalsShallow(other)) 1901 return false; 1902 if (!(other instanceof DataElement)) 1903 return false; 1904 DataElement o = (DataElement) other; 1905 return compareValues(url, o.url, true) && compareValues(version, o.version, true) 1906 && compareValues(name, o.name, true) && compareValues(status, o.status, true) 1907 && compareValues(experimental, o.experimental, true) && compareValues(publisher, o.publisher, true) 1908 && compareValues(date, o.date, true) && compareValues(copyright, o.copyright, true) 1909 && compareValues(stringency, o.stringency, true); 1910 } 1911 1912 public boolean isEmpty() { 1913 return super.isEmpty() && (url == null || url.isEmpty()) && (identifier == null || identifier.isEmpty()) 1914 && (version == null || version.isEmpty()) && (name == null || name.isEmpty()) 1915 && (status == null || status.isEmpty()) && (experimental == null || experimental.isEmpty()) 1916 && (publisher == null || publisher.isEmpty()) && (contact == null || contact.isEmpty()) 1917 && (date == null || date.isEmpty()) && (useContext == null || useContext.isEmpty()) 1918 && (copyright == null || copyright.isEmpty()) && (stringency == null || stringency.isEmpty()) 1919 && (mapping == null || mapping.isEmpty()) && (element == null || element.isEmpty()); 1920 } 1921 1922 @Override 1923 public ResourceType getResourceType() { 1924 return ResourceType.DataElement; 1925 } 1926 1927 @SearchParamDefinition(name = "date", path = "DataElement.date", description = "The data element publication date", type = "date") 1928 public static final String SP_DATE = "date"; 1929 @SearchParamDefinition(name = "identifier", path = "DataElement.identifier", description = "The identifier of the data element", type = "token") 1930 public static final String SP_IDENTIFIER = "identifier"; 1931 @SearchParamDefinition(name = "code", path = "DataElement.element.code", description = "A code for the data element (server may choose to do subsumption)", type = "token") 1932 public static final String SP_CODE = "code"; 1933 @SearchParamDefinition(name = "stringency", path = "DataElement.stringency", description = "The stringency of the data element definition", type = "token") 1934 public static final String SP_STRINGENCY = "stringency"; 1935 @SearchParamDefinition(name = "name", path = "DataElement.name", description = "Name of the data element", type = "string") 1936 public static final String SP_NAME = "name"; 1937 @SearchParamDefinition(name = "context", path = "DataElement.useContext", description = "A use context assigned to the data element", type = "token") 1938 public static final String SP_CONTEXT = "context"; 1939 @SearchParamDefinition(name = "publisher", path = "DataElement.publisher", description = "Name of the publisher of the data element", type = "string") 1940 public static final String SP_PUBLISHER = "publisher"; 1941 @SearchParamDefinition(name = "description", path = "DataElement.element.definition", description = "Text search in the description of the data element. This corresponds to the definition of the first DataElement.element.", type = "string") 1942 public static final String SP_DESCRIPTION = "description"; 1943 @SearchParamDefinition(name = "version", path = "DataElement.version", description = "The version identifier of the data element", type = "string") 1944 public static final String SP_VERSION = "version"; 1945 @SearchParamDefinition(name = "url", path = "DataElement.url", description = "The official URL for the data element", type = "uri") 1946 public static final String SP_URL = "url"; 1947 @SearchParamDefinition(name = "status", path = "DataElement.status", description = "The current status of the data element", type = "token") 1948 public static final String SP_STATUS = "status"; 1949 1950}