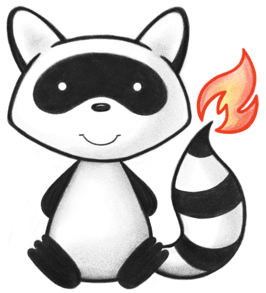
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus; 038import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.utilities.Utilities; 047 048/** 049 * The formal description of a single piece of information that can be gathered 050 * and reported. 051 */ 052@ResourceDef(name = "DataElement", profile = "http://hl7.org/fhir/Profile/DataElement") 053public class DataElement extends DomainResource { 054 055 public enum DataElementStringency { 056 /** 057 * The data element is sufficiently well-constrained that multiple pieces of 058 * data captured according to the constraints of the data element will be 059 * comparable (though in some cases, a degree of automated 060 * conversion/normalization may be required). 061 */ 062 COMPARABLE, 063 /** 064 * The data element is fully specified down to a single value set, single unit 065 * of measure, single data type, etc. Multiple pieces of data associated with 066 * this data element are fully comparable. 067 */ 068 FULLYSPECIFIED, 069 /** 070 * The data element allows multiple units of measure having equivalent meaning; 071 * e.g. "cc" (cubic centimeter) and "mL" (milliliter). 072 */ 073 EQUIVALENT, 074 /** 075 * The data element allows multiple units of measure that are convertable 076 * between each other (e.g. inches and centimeters) and/or allows data to be 077 * captured in multiple value sets for which a known mapping exists allowing 078 * conversion of meaning. 079 */ 080 CONVERTABLE, 081 /** 082 * A convertable data element where unit conversions are different only by a 083 * power of 10; e.g. g, mg, kg. 084 */ 085 SCALEABLE, 086 /** 087 * The data element is unconstrained in units, choice of data types and/or 088 * choice of vocabulary such that automated comparison of data captured using 089 * the data element is not possible. 090 */ 091 FLEXIBLE, 092 /** 093 * added to help the parsers 094 */ 095 NULL; 096 097 public static DataElementStringency fromCode(String codeString) throws FHIRException { 098 if (codeString == null || "".equals(codeString)) 099 return null; 100 if ("comparable".equals(codeString)) 101 return COMPARABLE; 102 if ("fully-specified".equals(codeString)) 103 return FULLYSPECIFIED; 104 if ("equivalent".equals(codeString)) 105 return EQUIVALENT; 106 if ("convertable".equals(codeString)) 107 return CONVERTABLE; 108 if ("scaleable".equals(codeString)) 109 return SCALEABLE; 110 if ("flexible".equals(codeString)) 111 return FLEXIBLE; 112 throw new FHIRException("Unknown DataElementStringency code '" + codeString + "'"); 113 } 114 115 public String toCode() { 116 switch (this) { 117 case COMPARABLE: 118 return "comparable"; 119 case FULLYSPECIFIED: 120 return "fully-specified"; 121 case EQUIVALENT: 122 return "equivalent"; 123 case CONVERTABLE: 124 return "convertable"; 125 case SCALEABLE: 126 return "scaleable"; 127 case FLEXIBLE: 128 return "flexible"; 129 case NULL: 130 return null; 131 default: 132 return "?"; 133 } 134 } 135 136 public String getSystem() { 137 switch (this) { 138 case COMPARABLE: 139 return "http://hl7.org/fhir/dataelement-stringency"; 140 case FULLYSPECIFIED: 141 return "http://hl7.org/fhir/dataelement-stringency"; 142 case EQUIVALENT: 143 return "http://hl7.org/fhir/dataelement-stringency"; 144 case CONVERTABLE: 145 return "http://hl7.org/fhir/dataelement-stringency"; 146 case SCALEABLE: 147 return "http://hl7.org/fhir/dataelement-stringency"; 148 case FLEXIBLE: 149 return "http://hl7.org/fhir/dataelement-stringency"; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 157 public String getDefinition() { 158 switch (this) { 159 case COMPARABLE: 160 return "The data element is sufficiently well-constrained that multiple pieces of data captured according to the constraints of the data element will be comparable (though in some cases, a degree of automated conversion/normalization may be required)."; 161 case FULLYSPECIFIED: 162 return "The data element is fully specified down to a single value set, single unit of measure, single data type, etc. Multiple pieces of data associated with this data element are fully comparable."; 163 case EQUIVALENT: 164 return "The data element allows multiple units of measure having equivalent meaning; e.g. \"cc\" (cubic centimeter) and \"mL\" (milliliter)."; 165 case CONVERTABLE: 166 return "The data element allows multiple units of measure that are convertable between each other (e.g. inches and centimeters) and/or allows data to be captured in multiple value sets for which a known mapping exists allowing conversion of meaning."; 167 case SCALEABLE: 168 return "A convertable data element where unit conversions are different only by a power of 10; e.g. g, mg, kg."; 169 case FLEXIBLE: 170 return "The data element is unconstrained in units, choice of data types and/or choice of vocabulary such that automated comparison of data captured using the data element is not possible."; 171 case NULL: 172 return null; 173 default: 174 return "?"; 175 } 176 } 177 178 public String getDisplay() { 179 switch (this) { 180 case COMPARABLE: 181 return "Comparable"; 182 case FULLYSPECIFIED: 183 return "Fully Specified"; 184 case EQUIVALENT: 185 return "Equivalent"; 186 case CONVERTABLE: 187 return "Convertable"; 188 case SCALEABLE: 189 return "Scaleable"; 190 case FLEXIBLE: 191 return "Flexible"; 192 case NULL: 193 return null; 194 default: 195 return "?"; 196 } 197 } 198 } 199 200 public static class DataElementStringencyEnumFactory implements EnumFactory<DataElementStringency> { 201 public DataElementStringency fromCode(String codeString) throws IllegalArgumentException { 202 if (codeString == null || "".equals(codeString)) 203 if (codeString == null || "".equals(codeString)) 204 return null; 205 if ("comparable".equals(codeString)) 206 return DataElementStringency.COMPARABLE; 207 if ("fully-specified".equals(codeString)) 208 return DataElementStringency.FULLYSPECIFIED; 209 if ("equivalent".equals(codeString)) 210 return DataElementStringency.EQUIVALENT; 211 if ("convertable".equals(codeString)) 212 return DataElementStringency.CONVERTABLE; 213 if ("scaleable".equals(codeString)) 214 return DataElementStringency.SCALEABLE; 215 if ("flexible".equals(codeString)) 216 return DataElementStringency.FLEXIBLE; 217 throw new IllegalArgumentException("Unknown DataElementStringency code '" + codeString + "'"); 218 } 219 220 public Enumeration<DataElementStringency> fromType(Base code) throws FHIRException { 221 if (code == null || code.isEmpty()) 222 return null; 223 String codeString = ((PrimitiveType) code).asStringValue(); 224 if (codeString == null || "".equals(codeString)) 225 return null; 226 if ("comparable".equals(codeString)) 227 return new Enumeration<DataElementStringency>(this, DataElementStringency.COMPARABLE); 228 if ("fully-specified".equals(codeString)) 229 return new Enumeration<DataElementStringency>(this, DataElementStringency.FULLYSPECIFIED); 230 if ("equivalent".equals(codeString)) 231 return new Enumeration<DataElementStringency>(this, DataElementStringency.EQUIVALENT); 232 if ("convertable".equals(codeString)) 233 return new Enumeration<DataElementStringency>(this, DataElementStringency.CONVERTABLE); 234 if ("scaleable".equals(codeString)) 235 return new Enumeration<DataElementStringency>(this, DataElementStringency.SCALEABLE); 236 if ("flexible".equals(codeString)) 237 return new Enumeration<DataElementStringency>(this, DataElementStringency.FLEXIBLE); 238 throw new FHIRException("Unknown DataElementStringency code '" + codeString + "'"); 239 } 240 241 public String toCode(DataElementStringency code) 242 { 243 if (code == DataElementStringency.NULL) 244 return null; 245 if (code == DataElementStringency.COMPARABLE) 246 return "comparable"; 247 if (code == DataElementStringency.FULLYSPECIFIED) 248 return "fully-specified"; 249 if (code == DataElementStringency.EQUIVALENT) 250 return "equivalent"; 251 if (code == DataElementStringency.CONVERTABLE) 252 return "convertable"; 253 if (code == DataElementStringency.SCALEABLE) 254 return "scaleable"; 255 if (code == DataElementStringency.FLEXIBLE) 256 return "flexible"; 257 return "?"; 258 } 259 } 260 261 @Block() 262 public static class DataElementContactComponent extends BackboneElement implements IBaseBackboneElement { 263 /** 264 * The name of an individual to contact regarding the data element. 265 */ 266 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 267 @Description(shortDefinition = "Name of a individual to contact", formalDefinition = "The name of an individual to contact regarding the data element.") 268 protected StringType name; 269 270 /** 271 * Contact details for individual (if a name was provided) or the publisher. 272 */ 273 @Child(name = "telecom", type = { 274 ContactPoint.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 275 @Description(shortDefinition = "Contact details for individual or publisher", formalDefinition = "Contact details for individual (if a name was provided) or the publisher.") 276 protected List<ContactPoint> telecom; 277 278 private static final long serialVersionUID = -1179697803L; 279 280 /* 281 * Constructor 282 */ 283 public DataElementContactComponent() { 284 super(); 285 } 286 287 /** 288 * @return {@link #name} (The name of an individual to contact regarding the 289 * data element.). This is the underlying object with id, value and 290 * extensions. The accessor "getName" gives direct access to the value 291 */ 292 public StringType getNameElement() { 293 if (this.name == null) 294 if (Configuration.errorOnAutoCreate()) 295 throw new Error("Attempt to auto-create DataElementContactComponent.name"); 296 else if (Configuration.doAutoCreate()) 297 this.name = new StringType(); // bb 298 return this.name; 299 } 300 301 public boolean hasNameElement() { 302 return this.name != null && !this.name.isEmpty(); 303 } 304 305 public boolean hasName() { 306 return this.name != null && !this.name.isEmpty(); 307 } 308 309 /** 310 * @param value {@link #name} (The name of an individual to contact regarding 311 * the data element.). This is the underlying object with id, value 312 * and extensions. The accessor "getName" gives direct access to 313 * the value 314 */ 315 public DataElementContactComponent setNameElement(StringType value) { 316 this.name = value; 317 return this; 318 } 319 320 /** 321 * @return The name of an individual to contact regarding the data element. 322 */ 323 public String getName() { 324 return this.name == null ? null : this.name.getValue(); 325 } 326 327 /** 328 * @param value The name of an individual to contact regarding the data element. 329 */ 330 public DataElementContactComponent setName(String value) { 331 if (Utilities.noString(value)) 332 this.name = null; 333 else { 334 if (this.name == null) 335 this.name = new StringType(); 336 this.name.setValue(value); 337 } 338 return this; 339 } 340 341 /** 342 * @return {@link #telecom} (Contact details for individual (if a name was 343 * provided) or the publisher.) 344 */ 345 public List<ContactPoint> getTelecom() { 346 if (this.telecom == null) 347 this.telecom = new ArrayList<ContactPoint>(); 348 return this.telecom; 349 } 350 351 public boolean hasTelecom() { 352 if (this.telecom == null) 353 return false; 354 for (ContactPoint item : this.telecom) 355 if (!item.isEmpty()) 356 return true; 357 return false; 358 } 359 360 /** 361 * @return {@link #telecom} (Contact details for individual (if a name was 362 * provided) or the publisher.) 363 */ 364 // syntactic sugar 365 public ContactPoint addTelecom() { // 3 366 ContactPoint t = new ContactPoint(); 367 if (this.telecom == null) 368 this.telecom = new ArrayList<ContactPoint>(); 369 this.telecom.add(t); 370 return t; 371 } 372 373 // syntactic sugar 374 public DataElementContactComponent addTelecom(ContactPoint t) { // 3 375 if (t == null) 376 return this; 377 if (this.telecom == null) 378 this.telecom = new ArrayList<ContactPoint>(); 379 this.telecom.add(t); 380 return this; 381 } 382 383 protected void listChildren(List<Property> childrenList) { 384 super.listChildren(childrenList); 385 childrenList.add(new Property("name", "string", 386 "The name of an individual to contact regarding the data element.", 0, java.lang.Integer.MAX_VALUE, name)); 387 childrenList.add(new Property("telecom", "ContactPoint", 388 "Contact details for individual (if a name was provided) or the publisher.", 0, java.lang.Integer.MAX_VALUE, 389 telecom)); 390 } 391 392 @Override 393 public void setProperty(String name, Base value) throws FHIRException { 394 if (name.equals("name")) 395 this.name = castToString(value); // StringType 396 else if (name.equals("telecom")) 397 this.getTelecom().add(castToContactPoint(value)); 398 else 399 super.setProperty(name, value); 400 } 401 402 @Override 403 public Base addChild(String name) throws FHIRException { 404 if (name.equals("name")) { 405 throw new FHIRException("Cannot call addChild on a singleton property DataElement.name"); 406 } else if (name.equals("telecom")) { 407 return addTelecom(); 408 } else 409 return super.addChild(name); 410 } 411 412 public DataElementContactComponent copy() { 413 DataElementContactComponent dst = new DataElementContactComponent(); 414 copyValues(dst); 415 dst.name = name == null ? null : name.copy(); 416 if (telecom != null) { 417 dst.telecom = new ArrayList<ContactPoint>(); 418 for (ContactPoint i : telecom) 419 dst.telecom.add(i.copy()); 420 } 421 ; 422 return dst; 423 } 424 425 @Override 426 public boolean equalsDeep(Base other) { 427 if (!super.equalsDeep(other)) 428 return false; 429 if (!(other instanceof DataElementContactComponent)) 430 return false; 431 DataElementContactComponent o = (DataElementContactComponent) other; 432 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 433 } 434 435 @Override 436 public boolean equalsShallow(Base other) { 437 if (!super.equalsShallow(other)) 438 return false; 439 if (!(other instanceof DataElementContactComponent)) 440 return false; 441 DataElementContactComponent o = (DataElementContactComponent) other; 442 return compareValues(name, o.name, true); 443 } 444 445 public boolean isEmpty() { 446 return super.isEmpty() && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()); 447 } 448 449 public String fhirType() { 450 return "DataElement.contact"; 451 452 } 453 454 } 455 456 @Block() 457 public static class DataElementMappingComponent extends BackboneElement implements IBaseBackboneElement { 458 /** 459 * An internal id that is used to identify this mapping set when specific 460 * mappings are made on a per-element basis. 461 */ 462 @Child(name = "identity", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 463 @Description(shortDefinition = "Internal id when this mapping is used", formalDefinition = "An internal id that is used to identify this mapping set when specific mappings are made on a per-element basis.") 464 protected IdType identity; 465 466 /** 467 * An absolute URI that identifies the specification that this mapping is 468 * expressed to. 469 */ 470 @Child(name = "uri", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 471 @Description(shortDefinition = "Identifies what this mapping refers to", formalDefinition = "An absolute URI that identifies the specification that this mapping is expressed to.") 472 protected UriType uri; 473 474 /** 475 * A name for the specification that is being mapped to. 476 */ 477 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 478 @Description(shortDefinition = "Names what this mapping refers to", formalDefinition = "A name for the specification that is being mapped to.") 479 protected StringType name; 480 481 /** 482 * Comments about this mapping, including version notes, issues, scope 483 * limitations, and other important notes for usage. 484 */ 485 @Child(name = "comments", type = { 486 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 487 @Description(shortDefinition = "Versions, Issues, Scope limitations etc.", formalDefinition = "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.") 488 protected StringType comments; 489 490 private static final long serialVersionUID = 299630820L; 491 492 /* 493 * Constructor 494 */ 495 public DataElementMappingComponent() { 496 super(); 497 } 498 499 /* 500 * Constructor 501 */ 502 public DataElementMappingComponent(IdType identity) { 503 super(); 504 this.identity = identity; 505 } 506 507 /** 508 * @return {@link #identity} (An internal id that is used to identify this 509 * mapping set when specific mappings are made on a per-element basis.). 510 * This is the underlying object with id, value and extensions. The 511 * accessor "getIdentity" gives direct access to the value 512 */ 513 public IdType getIdentityElement() { 514 if (this.identity == null) 515 if (Configuration.errorOnAutoCreate()) 516 throw new Error("Attempt to auto-create DataElementMappingComponent.identity"); 517 else if (Configuration.doAutoCreate()) 518 this.identity = new IdType(); // bb 519 return this.identity; 520 } 521 522 public boolean hasIdentityElement() { 523 return this.identity != null && !this.identity.isEmpty(); 524 } 525 526 public boolean hasIdentity() { 527 return this.identity != null && !this.identity.isEmpty(); 528 } 529 530 /** 531 * @param value {@link #identity} (An internal id that is used to identify this 532 * mapping set when specific mappings are made on a per-element 533 * basis.). This is the underlying object with id, value and 534 * extensions. The accessor "getIdentity" gives direct access to 535 * the value 536 */ 537 public DataElementMappingComponent setIdentityElement(IdType value) { 538 this.identity = value; 539 return this; 540 } 541 542 /** 543 * @return An internal id that is used to identify this mapping set when 544 * specific mappings are made on a per-element basis. 545 */ 546 public String getIdentity() { 547 return this.identity == null ? null : this.identity.getValue(); 548 } 549 550 /** 551 * @param value An internal id that is used to identify this mapping set when 552 * specific mappings are made on a per-element basis. 553 */ 554 public DataElementMappingComponent setIdentity(String value) { 555 if (this.identity == null) 556 this.identity = new IdType(); 557 this.identity.setValue(value); 558 return this; 559 } 560 561 /** 562 * @return {@link #uri} (An absolute URI that identifies the specification that 563 * this mapping is expressed to.). This is the underlying object with 564 * id, value and extensions. The accessor "getUri" gives direct access 565 * to the value 566 */ 567 public UriType getUriElement() { 568 if (this.uri == null) 569 if (Configuration.errorOnAutoCreate()) 570 throw new Error("Attempt to auto-create DataElementMappingComponent.uri"); 571 else if (Configuration.doAutoCreate()) 572 this.uri = new UriType(); // bb 573 return this.uri; 574 } 575 576 public boolean hasUriElement() { 577 return this.uri != null && !this.uri.isEmpty(); 578 } 579 580 public boolean hasUri() { 581 return this.uri != null && !this.uri.isEmpty(); 582 } 583 584 /** 585 * @param value {@link #uri} (An absolute URI that identifies the specification 586 * that this mapping is expressed to.). This is the underlying 587 * object with id, value and extensions. The accessor "getUri" 588 * gives direct access to the value 589 */ 590 public DataElementMappingComponent setUriElement(UriType value) { 591 this.uri = value; 592 return this; 593 } 594 595 /** 596 * @return An absolute URI that identifies the specification that this mapping 597 * is expressed to. 598 */ 599 public String getUri() { 600 return this.uri == null ? null : this.uri.getValue(); 601 } 602 603 /** 604 * @param value An absolute URI that identifies the specification that this 605 * mapping is expressed to. 606 */ 607 public DataElementMappingComponent setUri(String value) { 608 if (Utilities.noString(value)) 609 this.uri = null; 610 else { 611 if (this.uri == null) 612 this.uri = new UriType(); 613 this.uri.setValue(value); 614 } 615 return this; 616 } 617 618 /** 619 * @return {@link #name} (A name for the specification that is being mapped 620 * to.). This is the underlying object with id, value and extensions. 621 * The accessor "getName" gives direct access to the value 622 */ 623 public StringType getNameElement() { 624 if (this.name == null) 625 if (Configuration.errorOnAutoCreate()) 626 throw new Error("Attempt to auto-create DataElementMappingComponent.name"); 627 else if (Configuration.doAutoCreate()) 628 this.name = new StringType(); // bb 629 return this.name; 630 } 631 632 public boolean hasNameElement() { 633 return this.name != null && !this.name.isEmpty(); 634 } 635 636 public boolean hasName() { 637 return this.name != null && !this.name.isEmpty(); 638 } 639 640 /** 641 * @param value {@link #name} (A name for the specification that is being mapped 642 * to.). This is the underlying object with id, value and 643 * extensions. The accessor "getName" gives direct access to the 644 * value 645 */ 646 public DataElementMappingComponent setNameElement(StringType value) { 647 this.name = value; 648 return this; 649 } 650 651 /** 652 * @return A name for the specification that is being mapped to. 653 */ 654 public String getName() { 655 return this.name == null ? null : this.name.getValue(); 656 } 657 658 /** 659 * @param value A name for the specification that is being mapped to. 660 */ 661 public DataElementMappingComponent setName(String value) { 662 if (Utilities.noString(value)) 663 this.name = null; 664 else { 665 if (this.name == null) 666 this.name = new StringType(); 667 this.name.setValue(value); 668 } 669 return this; 670 } 671 672 /** 673 * @return {@link #comments} (Comments about this mapping, including version 674 * notes, issues, scope limitations, and other important notes for 675 * usage.). This is the underlying object with id, value and extensions. 676 * The accessor "getComments" gives direct access to the value 677 */ 678 public StringType getCommentsElement() { 679 if (this.comments == null) 680 if (Configuration.errorOnAutoCreate()) 681 throw new Error("Attempt to auto-create DataElementMappingComponent.comments"); 682 else if (Configuration.doAutoCreate()) 683 this.comments = new StringType(); // bb 684 return this.comments; 685 } 686 687 public boolean hasCommentsElement() { 688 return this.comments != null && !this.comments.isEmpty(); 689 } 690 691 public boolean hasComments() { 692 return this.comments != null && !this.comments.isEmpty(); 693 } 694 695 /** 696 * @param value {@link #comments} (Comments about this mapping, including 697 * version notes, issues, scope limitations, and other important 698 * notes for usage.). This is the underlying object with id, value 699 * and extensions. The accessor "getComments" gives direct access 700 * to the value 701 */ 702 public DataElementMappingComponent setCommentsElement(StringType value) { 703 this.comments = value; 704 return this; 705 } 706 707 /** 708 * @return Comments about this mapping, including version notes, issues, scope 709 * limitations, and other important notes for usage. 710 */ 711 public String getComments() { 712 return this.comments == null ? null : this.comments.getValue(); 713 } 714 715 /** 716 * @param value Comments about this mapping, including version notes, issues, 717 * scope limitations, and other important notes for usage. 718 */ 719 public DataElementMappingComponent setComments(String value) { 720 if (Utilities.noString(value)) 721 this.comments = null; 722 else { 723 if (this.comments == null) 724 this.comments = new StringType(); 725 this.comments.setValue(value); 726 } 727 return this; 728 } 729 730 protected void listChildren(List<Property> childrenList) { 731 super.listChildren(childrenList); 732 childrenList.add(new Property("identity", "id", 733 "An internal id that is used to identify this mapping set when specific mappings are made on a per-element basis.", 734 0, java.lang.Integer.MAX_VALUE, identity)); 735 childrenList.add(new Property("uri", "uri", 736 "An absolute URI that identifies the specification that this mapping is expressed to.", 0, 737 java.lang.Integer.MAX_VALUE, uri)); 738 childrenList.add(new Property("name", "string", "A name for the specification that is being mapped to.", 0, 739 java.lang.Integer.MAX_VALUE, name)); 740 childrenList.add(new Property("comments", "string", 741 "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.", 742 0, java.lang.Integer.MAX_VALUE, comments)); 743 } 744 745 @Override 746 public void setProperty(String name, Base value) throws FHIRException { 747 if (name.equals("identity")) 748 this.identity = castToId(value); // IdType 749 else if (name.equals("uri")) 750 this.uri = castToUri(value); // UriType 751 else if (name.equals("name")) 752 this.name = castToString(value); // StringType 753 else if (name.equals("comments")) 754 this.comments = castToString(value); // StringType 755 else 756 super.setProperty(name, value); 757 } 758 759 @Override 760 public Base addChild(String name) throws FHIRException { 761 if (name.equals("identity")) { 762 throw new FHIRException("Cannot call addChild on a singleton property DataElement.identity"); 763 } else if (name.equals("uri")) { 764 throw new FHIRException("Cannot call addChild on a singleton property DataElement.uri"); 765 } else if (name.equals("name")) { 766 throw new FHIRException("Cannot call addChild on a singleton property DataElement.name"); 767 } else if (name.equals("comments")) { 768 throw new FHIRException("Cannot call addChild on a singleton property DataElement.comments"); 769 } else 770 return super.addChild(name); 771 } 772 773 public DataElementMappingComponent copy() { 774 DataElementMappingComponent dst = new DataElementMappingComponent(); 775 copyValues(dst); 776 dst.identity = identity == null ? null : identity.copy(); 777 dst.uri = uri == null ? null : uri.copy(); 778 dst.name = name == null ? null : name.copy(); 779 dst.comments = comments == null ? null : comments.copy(); 780 return dst; 781 } 782 783 @Override 784 public boolean equalsDeep(Base other) { 785 if (!super.equalsDeep(other)) 786 return false; 787 if (!(other instanceof DataElementMappingComponent)) 788 return false; 789 DataElementMappingComponent o = (DataElementMappingComponent) other; 790 return compareDeep(identity, o.identity, true) && compareDeep(uri, o.uri, true) && compareDeep(name, o.name, true) 791 && compareDeep(comments, o.comments, true); 792 } 793 794 @Override 795 public boolean equalsShallow(Base other) { 796 if (!super.equalsShallow(other)) 797 return false; 798 if (!(other instanceof DataElementMappingComponent)) 799 return false; 800 DataElementMappingComponent o = (DataElementMappingComponent) other; 801 return compareValues(identity, o.identity, true) && compareValues(uri, o.uri, true) 802 && compareValues(name, o.name, true) && compareValues(comments, o.comments, true); 803 } 804 805 public boolean isEmpty() { 806 return super.isEmpty() && (identity == null || identity.isEmpty()) && (uri == null || uri.isEmpty()) 807 && (name == null || name.isEmpty()) && (comments == null || comments.isEmpty()); 808 } 809 810 public String fhirType() { 811 return "DataElement.mapping"; 812 813 } 814 815 } 816 817 /** 818 * An absolute URL that is used to identify this data element when it is 819 * referenced in a specification, model, design or an instance. This SHALL be a 820 * URL, SHOULD be globally unique, and SHOULD be an address at which this data 821 * element is (or will be) published. 822 */ 823 @Child(name = "url", type = { UriType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 824 @Description(shortDefinition = "Globally unique logical id for data element", formalDefinition = "An absolute URL that is used to identify this data element when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this data element is (or will be) published.") 825 protected UriType url; 826 827 /** 828 * Formal identifier that is used to identify this data element when it is 829 * represented in other formats, or referenced in a specification, model, design 830 * or an instance. 831 */ 832 @Child(name = "identifier", type = { 833 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 834 @Description(shortDefinition = "Logical id to reference this data element", formalDefinition = "Formal identifier that is used to identify this data element when it is represented in other formats, or referenced in a specification, model, design or an instance.") 835 protected List<Identifier> identifier; 836 837 /** 838 * The identifier that is used to identify this version of the data element when 839 * it is referenced in a StructureDefinition, Questionnaire or instance. This is 840 * an arbitrary value managed by the definition author manually. 841 */ 842 @Child(name = "version", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 843 @Description(shortDefinition = "Logical id for this version of the data element", formalDefinition = "The identifier that is used to identify this version of the data element when it is referenced in a StructureDefinition, Questionnaire or instance. This is an arbitrary value managed by the definition author manually.") 844 protected StringType version; 845 846 /** 847 * The term used by humans to refer to the data element. Should ideally be 848 * unique within the context in which the data element is expected to be used. 849 */ 850 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 851 @Description(shortDefinition = "Descriptive label for this element definition", formalDefinition = "The term used by humans to refer to the data element. Should ideally be unique within the context in which the data element is expected to be used.") 852 protected StringType name; 853 854 /** 855 * The status of the data element. 856 */ 857 @Child(name = "status", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = true, summary = true) 858 @Description(shortDefinition = "draft | active | retired", formalDefinition = "The status of the data element.") 859 protected Enumeration<ConformanceResourceStatus> status; 860 861 /** 862 * A flag to indicate that this search data element definition is authored for 863 * testing purposes (or education/evaluation/marketing), and is not intended to 864 * be used for genuine usage. 865 */ 866 @Child(name = "experimental", type = { 867 BooleanType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 868 @Description(shortDefinition = "If for testing purposes, not real usage", formalDefinition = "A flag to indicate that this search data element definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.") 869 protected BooleanType experimental; 870 871 /** 872 * The name of the individual or organization that published the data element. 873 */ 874 @Child(name = "publisher", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 875 @Description(shortDefinition = "Name of the publisher (Organization or individual)", formalDefinition = "The name of the individual or organization that published the data element.") 876 protected StringType publisher; 877 878 /** 879 * Contacts to assist a user in finding and communicating with the publisher. 880 */ 881 @Child(name = "contact", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 882 @Description(shortDefinition = "Contact details of the publisher", formalDefinition = "Contacts to assist a user in finding and communicating with the publisher.") 883 protected List<DataElementContactComponent> contact; 884 885 /** 886 * The date this version of the data element was published. The date must change 887 * when the business version changes, if it does, and it must change if the 888 * status code changes. In addition, it should change when the substantive 889 * content of the data element changes. 890 */ 891 @Child(name = "date", type = { DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 892 @Description(shortDefinition = "Date for this version of the data element", formalDefinition = "The date this version of the data element was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the data element changes.") 893 protected DateTimeType date; 894 895 /** 896 * The content was developed with a focus and intent of supporting the contexts 897 * that are listed. These terms may be used to assist with indexing and 898 * searching of data element definitions. 899 */ 900 @Child(name = "useContext", type = { 901 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 902 @Description(shortDefinition = "Content intends to support these contexts", formalDefinition = "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of data element definitions.") 903 protected List<CodeableConcept> useContext; 904 905 /** 906 * A copyright statement relating to the definition of the data element. 907 * Copyright statements are generally legal restrictions on the use and 908 * publishing of the details of the definition of the data element. 909 */ 910 @Child(name = "copyright", type = { 911 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 912 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the definition of the data element. Copyright statements are generally legal restrictions on the use and publishing of the details of the definition of the data element.") 913 protected StringType copyright; 914 915 /** 916 * Identifies how precise the data element is in its definition. 917 */ 918 @Child(name = "stringency", type = { CodeType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 919 @Description(shortDefinition = "comparable | fully-specified | equivalent | convertable | scaleable | flexible", formalDefinition = "Identifies how precise the data element is in its definition.") 920 protected Enumeration<DataElementStringency> stringency; 921 922 /** 923 * Identifies a specification (other than a terminology) that the elements which 924 * make up the DataElement have some correspondence with. 925 */ 926 @Child(name = "mapping", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 927 @Description(shortDefinition = "External specification mapped to", formalDefinition = "Identifies a specification (other than a terminology) that the elements which make up the DataElement have some correspondence with.") 928 protected List<DataElementMappingComponent> mapping; 929 930 /** 931 * Defines the structure, type, allowed values and other constraining 932 * characteristics of the data element. 933 */ 934 @Child(name = "element", type = { 935 ElementDefinition.class }, order = 13, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 936 @Description(shortDefinition = "Definition of element", formalDefinition = "Defines the structure, type, allowed values and other constraining characteristics of the data element.") 937 protected List<ElementDefinition> element; 938 939 private static final long serialVersionUID = 2017352331L; 940 941 /* 942 * Constructor 943 */ 944 public DataElement() { 945 super(); 946 } 947 948 /* 949 * Constructor 950 */ 951 public DataElement(Enumeration<ConformanceResourceStatus> status) { 952 super(); 953 this.status = status; 954 } 955 956 /** 957 * @return {@link #url} (An absolute URL that is used to identify this data 958 * element when it is referenced in a specification, model, design or an 959 * instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD 960 * be an address at which this data element is (or will be) published.). 961 * This is the underlying object with id, value and extensions. The 962 * accessor "getUrl" gives direct access to the value 963 */ 964 public UriType getUrlElement() { 965 if (this.url == null) 966 if (Configuration.errorOnAutoCreate()) 967 throw new Error("Attempt to auto-create DataElement.url"); 968 else if (Configuration.doAutoCreate()) 969 this.url = new UriType(); // bb 970 return this.url; 971 } 972 973 public boolean hasUrlElement() { 974 return this.url != null && !this.url.isEmpty(); 975 } 976 977 public boolean hasUrl() { 978 return this.url != null && !this.url.isEmpty(); 979 } 980 981 /** 982 * @param value {@link #url} (An absolute URL that is used to identify this data 983 * element when it is referenced in a specification, model, design 984 * or an instance. This SHALL be a URL, SHOULD be globally unique, 985 * and SHOULD be an address at which this data element is (or will 986 * be) published.). This is the underlying object with id, value 987 * and extensions. The accessor "getUrl" gives direct access to the 988 * value 989 */ 990 public DataElement setUrlElement(UriType value) { 991 this.url = value; 992 return this; 993 } 994 995 /** 996 * @return An absolute URL that is used to identify this data element when it is 997 * referenced in a specification, model, design or an instance. This 998 * SHALL be a URL, SHOULD be globally unique, and SHOULD be an address 999 * at which this data element is (or will be) published. 1000 */ 1001 public String getUrl() { 1002 return this.url == null ? null : this.url.getValue(); 1003 } 1004 1005 /** 1006 * @param value An absolute URL that is used to identify this data element when 1007 * it is referenced in a specification, model, design or an 1008 * instance. This SHALL be a URL, SHOULD be globally unique, and 1009 * SHOULD be an address at which this data element is (or will be) 1010 * published. 1011 */ 1012 public DataElement setUrl(String value) { 1013 if (Utilities.noString(value)) 1014 this.url = null; 1015 else { 1016 if (this.url == null) 1017 this.url = new UriType(); 1018 this.url.setValue(value); 1019 } 1020 return this; 1021 } 1022 1023 /** 1024 * @return {@link #identifier} (Formal identifier that is used to identify this 1025 * data element when it is represented in other formats, or referenced 1026 * in a specification, model, design or an instance.) 1027 */ 1028 public List<Identifier> getIdentifier() { 1029 if (this.identifier == null) 1030 this.identifier = new ArrayList<Identifier>(); 1031 return this.identifier; 1032 } 1033 1034 public boolean hasIdentifier() { 1035 if (this.identifier == null) 1036 return false; 1037 for (Identifier item : this.identifier) 1038 if (!item.isEmpty()) 1039 return true; 1040 return false; 1041 } 1042 1043 /** 1044 * @return {@link #identifier} (Formal identifier that is used to identify this 1045 * data element when it is represented in other formats, or referenced 1046 * in a specification, model, design or an instance.) 1047 */ 1048 // syntactic sugar 1049 public Identifier addIdentifier() { // 3 1050 Identifier t = new Identifier(); 1051 if (this.identifier == null) 1052 this.identifier = new ArrayList<Identifier>(); 1053 this.identifier.add(t); 1054 return t; 1055 } 1056 1057 // syntactic sugar 1058 public DataElement addIdentifier(Identifier t) { // 3 1059 if (t == null) 1060 return this; 1061 if (this.identifier == null) 1062 this.identifier = new ArrayList<Identifier>(); 1063 this.identifier.add(t); 1064 return this; 1065 } 1066 1067 /** 1068 * @return {@link #version} (The identifier that is used to identify this 1069 * version of the data element when it is referenced in a 1070 * StructureDefinition, Questionnaire or instance. This is an arbitrary 1071 * value managed by the definition author manually.). This is the 1072 * underlying object with id, value and extensions. The accessor 1073 * "getVersion" gives direct access to the value 1074 */ 1075 public StringType getVersionElement() { 1076 if (this.version == null) 1077 if (Configuration.errorOnAutoCreate()) 1078 throw new Error("Attempt to auto-create DataElement.version"); 1079 else if (Configuration.doAutoCreate()) 1080 this.version = new StringType(); // bb 1081 return this.version; 1082 } 1083 1084 public boolean hasVersionElement() { 1085 return this.version != null && !this.version.isEmpty(); 1086 } 1087 1088 public boolean hasVersion() { 1089 return this.version != null && !this.version.isEmpty(); 1090 } 1091 1092 /** 1093 * @param value {@link #version} (The identifier that is used to identify this 1094 * version of the data element when it is referenced in a 1095 * StructureDefinition, Questionnaire or instance. This is an 1096 * arbitrary value managed by the definition author manually.). 1097 * This is the underlying object with id, value and extensions. The 1098 * accessor "getVersion" gives direct access to the value 1099 */ 1100 public DataElement setVersionElement(StringType value) { 1101 this.version = value; 1102 return this; 1103 } 1104 1105 /** 1106 * @return The identifier that is used to identify this version of the data 1107 * element when it is referenced in a StructureDefinition, Questionnaire 1108 * or instance. This is an arbitrary value managed by the definition 1109 * author manually. 1110 */ 1111 public String getVersion() { 1112 return this.version == null ? null : this.version.getValue(); 1113 } 1114 1115 /** 1116 * @param value The identifier that is used to identify this version of the data 1117 * element when it is referenced in a StructureDefinition, 1118 * Questionnaire or instance. This is an arbitrary value managed by 1119 * the definition author manually. 1120 */ 1121 public DataElement setVersion(String value) { 1122 if (Utilities.noString(value)) 1123 this.version = null; 1124 else { 1125 if (this.version == null) 1126 this.version = new StringType(); 1127 this.version.setValue(value); 1128 } 1129 return this; 1130 } 1131 1132 /** 1133 * @return {@link #name} (The term used by humans to refer to the data element. 1134 * Should ideally be unique within the context in which the data element 1135 * is expected to be used.). This is the underlying object with id, 1136 * value and extensions. The accessor "getName" gives direct access to 1137 * the value 1138 */ 1139 public StringType getNameElement() { 1140 if (this.name == null) 1141 if (Configuration.errorOnAutoCreate()) 1142 throw new Error("Attempt to auto-create DataElement.name"); 1143 else if (Configuration.doAutoCreate()) 1144 this.name = new StringType(); // bb 1145 return this.name; 1146 } 1147 1148 public boolean hasNameElement() { 1149 return this.name != null && !this.name.isEmpty(); 1150 } 1151 1152 public boolean hasName() { 1153 return this.name != null && !this.name.isEmpty(); 1154 } 1155 1156 /** 1157 * @param value {@link #name} (The term used by humans to refer to the data 1158 * element. Should ideally be unique within the context in which 1159 * the data element is expected to be used.). This is the 1160 * underlying object with id, value and extensions. The accessor 1161 * "getName" gives direct access to the value 1162 */ 1163 public DataElement setNameElement(StringType value) { 1164 this.name = value; 1165 return this; 1166 } 1167 1168 /** 1169 * @return The term used by humans to refer to the data element. Should ideally 1170 * be unique within the context in which the data element is expected to 1171 * be used. 1172 */ 1173 public String getName() { 1174 return this.name == null ? null : this.name.getValue(); 1175 } 1176 1177 /** 1178 * @param value The term used by humans to refer to the data element. Should 1179 * ideally be unique within the context in which the data element 1180 * is expected to be used. 1181 */ 1182 public DataElement setName(String value) { 1183 if (Utilities.noString(value)) 1184 this.name = null; 1185 else { 1186 if (this.name == null) 1187 this.name = new StringType(); 1188 this.name.setValue(value); 1189 } 1190 return this; 1191 } 1192 1193 /** 1194 * @return {@link #status} (The status of the data element.). This is the 1195 * underlying object with id, value and extensions. The accessor 1196 * "getStatus" gives direct access to the value 1197 */ 1198 public Enumeration<ConformanceResourceStatus> getStatusElement() { 1199 if (this.status == null) 1200 if (Configuration.errorOnAutoCreate()) 1201 throw new Error("Attempt to auto-create DataElement.status"); 1202 else if (Configuration.doAutoCreate()) 1203 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); // bb 1204 return this.status; 1205 } 1206 1207 public boolean hasStatusElement() { 1208 return this.status != null && !this.status.isEmpty(); 1209 } 1210 1211 public boolean hasStatus() { 1212 return this.status != null && !this.status.isEmpty(); 1213 } 1214 1215 /** 1216 * @param value {@link #status} (The status of the data element.). This is the 1217 * underlying object with id, value and extensions. The accessor 1218 * "getStatus" gives direct access to the value 1219 */ 1220 public DataElement setStatusElement(Enumeration<ConformanceResourceStatus> value) { 1221 this.status = value; 1222 return this; 1223 } 1224 1225 /** 1226 * @return The status of the data element. 1227 */ 1228 public ConformanceResourceStatus getStatus() { 1229 return this.status == null ? null : this.status.getValue(); 1230 } 1231 1232 /** 1233 * @param value The status of the data element. 1234 */ 1235 public DataElement setStatus(ConformanceResourceStatus value) { 1236 if (this.status == null) 1237 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); 1238 this.status.setValue(value); 1239 return this; 1240 } 1241 1242 /** 1243 * @return {@link #experimental} (A flag to indicate that this search data 1244 * element definition is authored for testing purposes (or 1245 * education/evaluation/marketing), and is not intended to be used for 1246 * genuine usage.). This is the underlying object with id, value and 1247 * extensions. The accessor "getExperimental" gives direct access to the 1248 * value 1249 */ 1250 public BooleanType getExperimentalElement() { 1251 if (this.experimental == null) 1252 if (Configuration.errorOnAutoCreate()) 1253 throw new Error("Attempt to auto-create DataElement.experimental"); 1254 else if (Configuration.doAutoCreate()) 1255 this.experimental = new BooleanType(); // bb 1256 return this.experimental; 1257 } 1258 1259 public boolean hasExperimentalElement() { 1260 return this.experimental != null && !this.experimental.isEmpty(); 1261 } 1262 1263 public boolean hasExperimental() { 1264 return this.experimental != null && !this.experimental.isEmpty(); 1265 } 1266 1267 /** 1268 * @param value {@link #experimental} (A flag to indicate that this search data 1269 * element definition is authored for testing purposes (or 1270 * education/evaluation/marketing), and is not intended to be used 1271 * for genuine usage.). This is the underlying object with id, 1272 * value and extensions. The accessor "getExperimental" gives 1273 * direct access to the value 1274 */ 1275 public DataElement setExperimentalElement(BooleanType value) { 1276 this.experimental = value; 1277 return this; 1278 } 1279 1280 /** 1281 * @return A flag to indicate that this search data element definition is 1282 * authored for testing purposes (or education/evaluation/marketing), 1283 * and is not intended to be used for genuine usage. 1284 */ 1285 public boolean getExperimental() { 1286 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1287 } 1288 1289 /** 1290 * @param value A flag to indicate that this search data element definition is 1291 * authored for testing purposes (or 1292 * education/evaluation/marketing), and is not intended to be used 1293 * for genuine usage. 1294 */ 1295 public DataElement setExperimental(boolean value) { 1296 if (this.experimental == null) 1297 this.experimental = new BooleanType(); 1298 this.experimental.setValue(value); 1299 return this; 1300 } 1301 1302 /** 1303 * @return {@link #publisher} (The name of the individual or organization that 1304 * published the data element.). This is the underlying object with id, 1305 * value and extensions. The accessor "getPublisher" gives direct access 1306 * to the value 1307 */ 1308 public StringType getPublisherElement() { 1309 if (this.publisher == null) 1310 if (Configuration.errorOnAutoCreate()) 1311 throw new Error("Attempt to auto-create DataElement.publisher"); 1312 else if (Configuration.doAutoCreate()) 1313 this.publisher = new StringType(); // bb 1314 return this.publisher; 1315 } 1316 1317 public boolean hasPublisherElement() { 1318 return this.publisher != null && !this.publisher.isEmpty(); 1319 } 1320 1321 public boolean hasPublisher() { 1322 return this.publisher != null && !this.publisher.isEmpty(); 1323 } 1324 1325 /** 1326 * @param value {@link #publisher} (The name of the individual or organization 1327 * that published the data element.). This is the underlying object 1328 * with id, value and extensions. The accessor "getPublisher" gives 1329 * direct access to the value 1330 */ 1331 public DataElement setPublisherElement(StringType value) { 1332 this.publisher = value; 1333 return this; 1334 } 1335 1336 /** 1337 * @return The name of the individual or organization that published the data 1338 * element. 1339 */ 1340 public String getPublisher() { 1341 return this.publisher == null ? null : this.publisher.getValue(); 1342 } 1343 1344 /** 1345 * @param value The name of the individual or organization that published the 1346 * data element. 1347 */ 1348 public DataElement setPublisher(String value) { 1349 if (Utilities.noString(value)) 1350 this.publisher = null; 1351 else { 1352 if (this.publisher == null) 1353 this.publisher = new StringType(); 1354 this.publisher.setValue(value); 1355 } 1356 return this; 1357 } 1358 1359 /** 1360 * @return {@link #contact} (Contacts to assist a user in finding and 1361 * communicating with the publisher.) 1362 */ 1363 public List<DataElementContactComponent> getContact() { 1364 if (this.contact == null) 1365 this.contact = new ArrayList<DataElementContactComponent>(); 1366 return this.contact; 1367 } 1368 1369 public boolean hasContact() { 1370 if (this.contact == null) 1371 return false; 1372 for (DataElementContactComponent item : this.contact) 1373 if (!item.isEmpty()) 1374 return true; 1375 return false; 1376 } 1377 1378 /** 1379 * @return {@link #contact} (Contacts to assist a user in finding and 1380 * communicating with the publisher.) 1381 */ 1382 // syntactic sugar 1383 public DataElementContactComponent addContact() { // 3 1384 DataElementContactComponent t = new DataElementContactComponent(); 1385 if (this.contact == null) 1386 this.contact = new ArrayList<DataElementContactComponent>(); 1387 this.contact.add(t); 1388 return t; 1389 } 1390 1391 // syntactic sugar 1392 public DataElement addContact(DataElementContactComponent t) { // 3 1393 if (t == null) 1394 return this; 1395 if (this.contact == null) 1396 this.contact = new ArrayList<DataElementContactComponent>(); 1397 this.contact.add(t); 1398 return this; 1399 } 1400 1401 /** 1402 * @return {@link #date} (The date this version of the data element was 1403 * published. The date must change when the business version changes, if 1404 * it does, and it must change if the status code changes. In addition, 1405 * it should change when the substantive content of the data element 1406 * changes.). This is the underlying object with id, value and 1407 * extensions. The accessor "getDate" gives direct access to the value 1408 */ 1409 public DateTimeType getDateElement() { 1410 if (this.date == null) 1411 if (Configuration.errorOnAutoCreate()) 1412 throw new Error("Attempt to auto-create DataElement.date"); 1413 else if (Configuration.doAutoCreate()) 1414 this.date = new DateTimeType(); // bb 1415 return this.date; 1416 } 1417 1418 public boolean hasDateElement() { 1419 return this.date != null && !this.date.isEmpty(); 1420 } 1421 1422 public boolean hasDate() { 1423 return this.date != null && !this.date.isEmpty(); 1424 } 1425 1426 /** 1427 * @param value {@link #date} (The date this version of the data element was 1428 * published. The date must change when the business version 1429 * changes, if it does, and it must change if the status code 1430 * changes. In addition, it should change when the substantive 1431 * content of the data element changes.). This is the underlying 1432 * object with id, value and extensions. The accessor "getDate" 1433 * gives direct access to the value 1434 */ 1435 public DataElement setDateElement(DateTimeType value) { 1436 this.date = value; 1437 return this; 1438 } 1439 1440 /** 1441 * @return The date this version of the data element was published. The date 1442 * must change when the business version changes, if it does, and it 1443 * must change if the status code changes. In addition, it should change 1444 * when the substantive content of the data element changes. 1445 */ 1446 public Date getDate() { 1447 return this.date == null ? null : this.date.getValue(); 1448 } 1449 1450 /** 1451 * @param value The date this version of the data element was published. The 1452 * date must change when the business version changes, if it does, 1453 * and it must change if the status code changes. In addition, it 1454 * should change when the substantive content of the data element 1455 * changes. 1456 */ 1457 public DataElement setDate(Date value) { 1458 if (value == null) 1459 this.date = null; 1460 else { 1461 if (this.date == null) 1462 this.date = new DateTimeType(); 1463 this.date.setValue(value); 1464 } 1465 return this; 1466 } 1467 1468 /** 1469 * @return {@link #useContext} (The content was developed with a focus and 1470 * intent of supporting the contexts that are listed. These terms may be 1471 * used to assist with indexing and searching of data element 1472 * definitions.) 1473 */ 1474 public List<CodeableConcept> getUseContext() { 1475 if (this.useContext == null) 1476 this.useContext = new ArrayList<CodeableConcept>(); 1477 return this.useContext; 1478 } 1479 1480 public boolean hasUseContext() { 1481 if (this.useContext == null) 1482 return false; 1483 for (CodeableConcept item : this.useContext) 1484 if (!item.isEmpty()) 1485 return true; 1486 return false; 1487 } 1488 1489 /** 1490 * @return {@link #useContext} (The content was developed with a focus and 1491 * intent of supporting the contexts that are listed. These terms may be 1492 * used to assist with indexing and searching of data element 1493 * definitions.) 1494 */ 1495 // syntactic sugar 1496 public CodeableConcept addUseContext() { // 3 1497 CodeableConcept t = new CodeableConcept(); 1498 if (this.useContext == null) 1499 this.useContext = new ArrayList<CodeableConcept>(); 1500 this.useContext.add(t); 1501 return t; 1502 } 1503 1504 // syntactic sugar 1505 public DataElement addUseContext(CodeableConcept t) { // 3 1506 if (t == null) 1507 return this; 1508 if (this.useContext == null) 1509 this.useContext = new ArrayList<CodeableConcept>(); 1510 this.useContext.add(t); 1511 return this; 1512 } 1513 1514 /** 1515 * @return {@link #copyright} (A copyright statement relating to the definition 1516 * of the data element. Copyright statements are generally legal 1517 * restrictions on the use and publishing of the details of the 1518 * definition of the data element.). This is the underlying object with 1519 * id, value and extensions. The accessor "getCopyright" gives direct 1520 * access to the value 1521 */ 1522 public StringType getCopyrightElement() { 1523 if (this.copyright == null) 1524 if (Configuration.errorOnAutoCreate()) 1525 throw new Error("Attempt to auto-create DataElement.copyright"); 1526 else if (Configuration.doAutoCreate()) 1527 this.copyright = new StringType(); // bb 1528 return this.copyright; 1529 } 1530 1531 public boolean hasCopyrightElement() { 1532 return this.copyright != null && !this.copyright.isEmpty(); 1533 } 1534 1535 public boolean hasCopyright() { 1536 return this.copyright != null && !this.copyright.isEmpty(); 1537 } 1538 1539 /** 1540 * @param value {@link #copyright} (A copyright statement relating to the 1541 * definition of the data element. Copyright statements are 1542 * generally legal restrictions on the use and publishing of the 1543 * details of the definition of the data element.). This is the 1544 * underlying object with id, value and extensions. The accessor 1545 * "getCopyright" gives direct access to the value 1546 */ 1547 public DataElement setCopyrightElement(StringType value) { 1548 this.copyright = value; 1549 return this; 1550 } 1551 1552 /** 1553 * @return A copyright statement relating to the definition of the data element. 1554 * Copyright statements are generally legal restrictions on the use and 1555 * publishing of the details of the definition of the data element. 1556 */ 1557 public String getCopyright() { 1558 return this.copyright == null ? null : this.copyright.getValue(); 1559 } 1560 1561 /** 1562 * @param value A copyright statement relating to the definition of the data 1563 * element. Copyright statements are generally legal restrictions 1564 * on the use and publishing of the details of the definition of 1565 * the data element. 1566 */ 1567 public DataElement setCopyright(String value) { 1568 if (Utilities.noString(value)) 1569 this.copyright = null; 1570 else { 1571 if (this.copyright == null) 1572 this.copyright = new StringType(); 1573 this.copyright.setValue(value); 1574 } 1575 return this; 1576 } 1577 1578 /** 1579 * @return {@link #stringency} (Identifies how precise the data element is in 1580 * its definition.). This is the underlying object with id, value and 1581 * extensions. The accessor "getStringency" gives direct access to the 1582 * value 1583 */ 1584 public Enumeration<DataElementStringency> getStringencyElement() { 1585 if (this.stringency == null) 1586 if (Configuration.errorOnAutoCreate()) 1587 throw new Error("Attempt to auto-create DataElement.stringency"); 1588 else if (Configuration.doAutoCreate()) 1589 this.stringency = new Enumeration<DataElementStringency>(new DataElementStringencyEnumFactory()); // bb 1590 return this.stringency; 1591 } 1592 1593 public boolean hasStringencyElement() { 1594 return this.stringency != null && !this.stringency.isEmpty(); 1595 } 1596 1597 public boolean hasStringency() { 1598 return this.stringency != null && !this.stringency.isEmpty(); 1599 } 1600 1601 /** 1602 * @param value {@link #stringency} (Identifies how precise the data element is 1603 * in its definition.). This is the underlying object with id, 1604 * value and extensions. The accessor "getStringency" gives direct 1605 * access to the value 1606 */ 1607 public DataElement setStringencyElement(Enumeration<DataElementStringency> value) { 1608 this.stringency = value; 1609 return this; 1610 } 1611 1612 /** 1613 * @return Identifies how precise the data element is in its definition. 1614 */ 1615 public DataElementStringency getStringency() { 1616 return this.stringency == null ? null : this.stringency.getValue(); 1617 } 1618 1619 /** 1620 * @param value Identifies how precise the data element is in its definition. 1621 */ 1622 public DataElement setStringency(DataElementStringency value) { 1623 if (value == null) 1624 this.stringency = null; 1625 else { 1626 if (this.stringency == null) 1627 this.stringency = new Enumeration<DataElementStringency>(new DataElementStringencyEnumFactory()); 1628 this.stringency.setValue(value); 1629 } 1630 return this; 1631 } 1632 1633 /** 1634 * @return {@link #mapping} (Identifies a specification (other than a 1635 * terminology) that the elements which make up the DataElement have 1636 * some correspondence with.) 1637 */ 1638 public List<DataElementMappingComponent> getMapping() { 1639 if (this.mapping == null) 1640 this.mapping = new ArrayList<DataElementMappingComponent>(); 1641 return this.mapping; 1642 } 1643 1644 public boolean hasMapping() { 1645 if (this.mapping == null) 1646 return false; 1647 for (DataElementMappingComponent item : this.mapping) 1648 if (!item.isEmpty()) 1649 return true; 1650 return false; 1651 } 1652 1653 /** 1654 * @return {@link #mapping} (Identifies a specification (other than a 1655 * terminology) that the elements which make up the DataElement have 1656 * some correspondence with.) 1657 */ 1658 // syntactic sugar 1659 public DataElementMappingComponent addMapping() { // 3 1660 DataElementMappingComponent t = new DataElementMappingComponent(); 1661 if (this.mapping == null) 1662 this.mapping = new ArrayList<DataElementMappingComponent>(); 1663 this.mapping.add(t); 1664 return t; 1665 } 1666 1667 // syntactic sugar 1668 public DataElement addMapping(DataElementMappingComponent t) { // 3 1669 if (t == null) 1670 return this; 1671 if (this.mapping == null) 1672 this.mapping = new ArrayList<DataElementMappingComponent>(); 1673 this.mapping.add(t); 1674 return this; 1675 } 1676 1677 /** 1678 * @return {@link #element} (Defines the structure, type, allowed values and 1679 * other constraining characteristics of the data element.) 1680 */ 1681 public List<ElementDefinition> getElement() { 1682 if (this.element == null) 1683 this.element = new ArrayList<ElementDefinition>(); 1684 return this.element; 1685 } 1686 1687 public boolean hasElement() { 1688 if (this.element == null) 1689 return false; 1690 for (ElementDefinition item : this.element) 1691 if (!item.isEmpty()) 1692 return true; 1693 return false; 1694 } 1695 1696 /** 1697 * @return {@link #element} (Defines the structure, type, allowed values and 1698 * other constraining characteristics of the data element.) 1699 */ 1700 // syntactic sugar 1701 public ElementDefinition addElement() { // 3 1702 ElementDefinition t = new ElementDefinition(); 1703 if (this.element == null) 1704 this.element = new ArrayList<ElementDefinition>(); 1705 this.element.add(t); 1706 return t; 1707 } 1708 1709 // syntactic sugar 1710 public DataElement addElement(ElementDefinition t) { // 3 1711 if (t == null) 1712 return this; 1713 if (this.element == null) 1714 this.element = new ArrayList<ElementDefinition>(); 1715 this.element.add(t); 1716 return this; 1717 } 1718 1719 protected void listChildren(List<Property> childrenList) { 1720 super.listChildren(childrenList); 1721 childrenList.add(new Property("url", "uri", 1722 "An absolute URL that is used to identify this data element when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this data element is (or will be) published.", 1723 0, java.lang.Integer.MAX_VALUE, url)); 1724 childrenList.add(new Property("identifier", "Identifier", 1725 "Formal identifier that is used to identify this data element when it is represented in other formats, or referenced in a specification, model, design or an instance.", 1726 0, java.lang.Integer.MAX_VALUE, identifier)); 1727 childrenList.add(new Property("version", "string", 1728 "The identifier that is used to identify this version of the data element when it is referenced in a StructureDefinition, Questionnaire or instance. This is an arbitrary value managed by the definition author manually.", 1729 0, java.lang.Integer.MAX_VALUE, version)); 1730 childrenList.add(new Property("name", "string", 1731 "The term used by humans to refer to the data element. Should ideally be unique within the context in which the data element is expected to be used.", 1732 0, java.lang.Integer.MAX_VALUE, name)); 1733 childrenList 1734 .add(new Property("status", "code", "The status of the data element.", 0, java.lang.Integer.MAX_VALUE, status)); 1735 childrenList.add(new Property("experimental", "boolean", 1736 "A flag to indicate that this search data element definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 1737 0, java.lang.Integer.MAX_VALUE, experimental)); 1738 childrenList.add(new Property("publisher", "string", 1739 "The name of the individual or organization that published the data element.", 0, java.lang.Integer.MAX_VALUE, 1740 publisher)); 1741 childrenList 1742 .add(new Property("contact", "", "Contacts to assist a user in finding and communicating with the publisher.", 1743 0, java.lang.Integer.MAX_VALUE, contact)); 1744 childrenList.add(new Property("date", "dateTime", 1745 "The date this version of the data element was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the data element changes.", 1746 0, java.lang.Integer.MAX_VALUE, date)); 1747 childrenList.add(new Property("useContext", "CodeableConcept", 1748 "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of data element definitions.", 1749 0, java.lang.Integer.MAX_VALUE, useContext)); 1750 childrenList.add(new Property("copyright", "string", 1751 "A copyright statement relating to the definition of the data element. Copyright statements are generally legal restrictions on the use and publishing of the details of the definition of the data element.", 1752 0, java.lang.Integer.MAX_VALUE, copyright)); 1753 childrenList.add(new Property("stringency", "code", "Identifies how precise the data element is in its definition.", 1754 0, java.lang.Integer.MAX_VALUE, stringency)); 1755 childrenList.add(new Property("mapping", "", 1756 "Identifies a specification (other than a terminology) that the elements which make up the DataElement have some correspondence with.", 1757 0, java.lang.Integer.MAX_VALUE, mapping)); 1758 childrenList.add(new Property("element", "ElementDefinition", 1759 "Defines the structure, type, allowed values and other constraining characteristics of the data element.", 0, 1760 java.lang.Integer.MAX_VALUE, element)); 1761 } 1762 1763 @Override 1764 public void setProperty(String name, Base value) throws FHIRException { 1765 if (name.equals("url")) 1766 this.url = castToUri(value); // UriType 1767 else if (name.equals("identifier")) 1768 this.getIdentifier().add(castToIdentifier(value)); 1769 else if (name.equals("version")) 1770 this.version = castToString(value); // StringType 1771 else if (name.equals("name")) 1772 this.name = castToString(value); // StringType 1773 else if (name.equals("status")) 1774 this.status = new ConformanceResourceStatusEnumFactory().fromType(value); // Enumeration<ConformanceResourceStatus> 1775 else if (name.equals("experimental")) 1776 this.experimental = castToBoolean(value); // BooleanType 1777 else if (name.equals("publisher")) 1778 this.publisher = castToString(value); // StringType 1779 else if (name.equals("contact")) 1780 this.getContact().add((DataElementContactComponent) value); 1781 else if (name.equals("date")) 1782 this.date = castToDateTime(value); // DateTimeType 1783 else if (name.equals("useContext")) 1784 this.getUseContext().add(castToCodeableConcept(value)); 1785 else if (name.equals("copyright")) 1786 this.copyright = castToString(value); // StringType 1787 else if (name.equals("stringency")) 1788 this.stringency = new DataElementStringencyEnumFactory().fromType(value); // Enumeration<DataElementStringency> 1789 else if (name.equals("mapping")) 1790 this.getMapping().add((DataElementMappingComponent) value); 1791 else if (name.equals("element")) 1792 this.getElement().add(castToElementDefinition(value)); 1793 else 1794 super.setProperty(name, value); 1795 } 1796 1797 @Override 1798 public Base addChild(String name) throws FHIRException { 1799 if (name.equals("url")) { 1800 throw new FHIRException("Cannot call addChild on a singleton property DataElement.url"); 1801 } else if (name.equals("identifier")) { 1802 return addIdentifier(); 1803 } else if (name.equals("version")) { 1804 throw new FHIRException("Cannot call addChild on a singleton property DataElement.version"); 1805 } else if (name.equals("name")) { 1806 throw new FHIRException("Cannot call addChild on a singleton property DataElement.name"); 1807 } else if (name.equals("status")) { 1808 throw new FHIRException("Cannot call addChild on a singleton property DataElement.status"); 1809 } else if (name.equals("experimental")) { 1810 throw new FHIRException("Cannot call addChild on a singleton property DataElement.experimental"); 1811 } else if (name.equals("publisher")) { 1812 throw new FHIRException("Cannot call addChild on a singleton property DataElement.publisher"); 1813 } else if (name.equals("contact")) { 1814 return addContact(); 1815 } else if (name.equals("date")) { 1816 throw new FHIRException("Cannot call addChild on a singleton property DataElement.date"); 1817 } else if (name.equals("useContext")) { 1818 return addUseContext(); 1819 } else if (name.equals("copyright")) { 1820 throw new FHIRException("Cannot call addChild on a singleton property DataElement.copyright"); 1821 } else if (name.equals("stringency")) { 1822 throw new FHIRException("Cannot call addChild on a singleton property DataElement.stringency"); 1823 } else if (name.equals("mapping")) { 1824 return addMapping(); 1825 } else if (name.equals("element")) { 1826 return addElement(); 1827 } else 1828 return super.addChild(name); 1829 } 1830 1831 public String fhirType() { 1832 return "DataElement"; 1833 1834 } 1835 1836 public DataElement copy() { 1837 DataElement dst = new DataElement(); 1838 copyValues(dst); 1839 dst.url = url == null ? null : url.copy(); 1840 if (identifier != null) { 1841 dst.identifier = new ArrayList<Identifier>(); 1842 for (Identifier i : identifier) 1843 dst.identifier.add(i.copy()); 1844 } 1845 ; 1846 dst.version = version == null ? null : version.copy(); 1847 dst.name = name == null ? null : name.copy(); 1848 dst.status = status == null ? null : status.copy(); 1849 dst.experimental = experimental == null ? null : experimental.copy(); 1850 dst.publisher = publisher == null ? null : publisher.copy(); 1851 if (contact != null) { 1852 dst.contact = new ArrayList<DataElementContactComponent>(); 1853 for (DataElementContactComponent i : contact) 1854 dst.contact.add(i.copy()); 1855 } 1856 ; 1857 dst.date = date == null ? null : date.copy(); 1858 if (useContext != null) { 1859 dst.useContext = new ArrayList<CodeableConcept>(); 1860 for (CodeableConcept i : useContext) 1861 dst.useContext.add(i.copy()); 1862 } 1863 ; 1864 dst.copyright = copyright == null ? null : copyright.copy(); 1865 dst.stringency = stringency == null ? null : stringency.copy(); 1866 if (mapping != null) { 1867 dst.mapping = new ArrayList<DataElementMappingComponent>(); 1868 for (DataElementMappingComponent i : mapping) 1869 dst.mapping.add(i.copy()); 1870 } 1871 ; 1872 if (element != null) { 1873 dst.element = new ArrayList<ElementDefinition>(); 1874 for (ElementDefinition i : element) 1875 dst.element.add(i.copy()); 1876 } 1877 ; 1878 return dst; 1879 } 1880 1881 protected DataElement typedCopy() { 1882 return copy(); 1883 } 1884 1885 @Override 1886 public boolean equalsDeep(Base other) { 1887 if (!super.equalsDeep(other)) 1888 return false; 1889 if (!(other instanceof DataElement)) 1890 return false; 1891 DataElement o = (DataElement) other; 1892 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) 1893 && compareDeep(version, o.version, true) && compareDeep(name, o.name, true) 1894 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 1895 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 1896 && compareDeep(date, o.date, true) && compareDeep(useContext, o.useContext, true) 1897 && compareDeep(copyright, o.copyright, true) && compareDeep(stringency, o.stringency, true) 1898 && compareDeep(mapping, o.mapping, true) && compareDeep(element, o.element, true); 1899 } 1900 1901 @Override 1902 public boolean equalsShallow(Base other) { 1903 if (!super.equalsShallow(other)) 1904 return false; 1905 if (!(other instanceof DataElement)) 1906 return false; 1907 DataElement o = (DataElement) other; 1908 return compareValues(url, o.url, true) && compareValues(version, o.version, true) 1909 && compareValues(name, o.name, true) && compareValues(status, o.status, true) 1910 && compareValues(experimental, o.experimental, true) && compareValues(publisher, o.publisher, true) 1911 && compareValues(date, o.date, true) && compareValues(copyright, o.copyright, true) 1912 && compareValues(stringency, o.stringency, true); 1913 } 1914 1915 public boolean isEmpty() { 1916 return super.isEmpty() && (url == null || url.isEmpty()) && (identifier == null || identifier.isEmpty()) 1917 && (version == null || version.isEmpty()) && (name == null || name.isEmpty()) 1918 && (status == null || status.isEmpty()) && (experimental == null || experimental.isEmpty()) 1919 && (publisher == null || publisher.isEmpty()) && (contact == null || contact.isEmpty()) 1920 && (date == null || date.isEmpty()) && (useContext == null || useContext.isEmpty()) 1921 && (copyright == null || copyright.isEmpty()) && (stringency == null || stringency.isEmpty()) 1922 && (mapping == null || mapping.isEmpty()) && (element == null || element.isEmpty()); 1923 } 1924 1925 @Override 1926 public ResourceType getResourceType() { 1927 return ResourceType.DataElement; 1928 } 1929 1930 @SearchParamDefinition(name = "date", path = "DataElement.date", description = "The data element publication date", type = "date") 1931 public static final String SP_DATE = "date"; 1932 @SearchParamDefinition(name = "identifier", path = "DataElement.identifier", description = "The identifier of the data element", type = "token") 1933 public static final String SP_IDENTIFIER = "identifier"; 1934 @SearchParamDefinition(name = "code", path = "DataElement.element.code", description = "A code for the data element (server may choose to do subsumption)", type = "token") 1935 public static final String SP_CODE = "code"; 1936 @SearchParamDefinition(name = "stringency", path = "DataElement.stringency", description = "The stringency of the data element definition", type = "token") 1937 public static final String SP_STRINGENCY = "stringency"; 1938 @SearchParamDefinition(name = "name", path = "DataElement.name", description = "Name of the data element", type = "string") 1939 public static final String SP_NAME = "name"; 1940 @SearchParamDefinition(name = "context", path = "DataElement.useContext", description = "A use context assigned to the data element", type = "token") 1941 public static final String SP_CONTEXT = "context"; 1942 @SearchParamDefinition(name = "publisher", path = "DataElement.publisher", description = "Name of the publisher of the data element", type = "string") 1943 public static final String SP_PUBLISHER = "publisher"; 1944 @SearchParamDefinition(name = "description", path = "DataElement.element.definition", description = "Text search in the description of the data element. This corresponds to the definition of the first DataElement.element.", type = "string") 1945 public static final String SP_DESCRIPTION = "description"; 1946 @SearchParamDefinition(name = "version", path = "DataElement.version", description = "The version identifier of the data element", type = "string") 1947 public static final String SP_VERSION = "version"; 1948 @SearchParamDefinition(name = "url", path = "DataElement.url", description = "The official URL for the data element", type = "uri") 1949 public static final String SP_URL = "url"; 1950 @SearchParamDefinition(name = "status", path = "DataElement.status", description = "The current status of the data element", type = "token") 1951 public static final String SP_STATUS = "status"; 1952 1953}