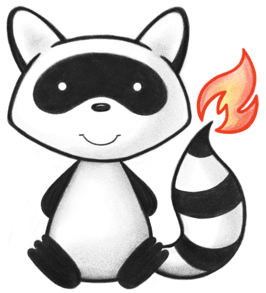
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * Indicates an actual or potential clinical issue with or between one or more 048 * active or proposed clinical actions for a patient; e.g. Drug-drug 049 * interaction, Ineffective treatment frequency, Procedure-condition conflict, 050 * etc. 051 */ 052@ResourceDef(name = "DetectedIssue", profile = "http://hl7.org/fhir/Profile/DetectedIssue") 053public class DetectedIssue extends DomainResource { 054 055 public enum DetectedIssueSeverity { 056 /** 057 * Indicates the issue may be life-threatening or has the potential to cause 058 * permanent injury. 059 */ 060 HIGH, 061 /** 062 * Indicates the issue may result in noticeable adverse consequences but is 063 * unlikely to be life-threatening or cause permanent injury. 064 */ 065 MODERATE, 066 /** 067 * Indicates the issue may result in some adverse consequences but is unlikely 068 * to substantially affect the situation of the subject. 069 */ 070 LOW, 071 /** 072 * added to help the parsers 073 */ 074 NULL; 075 076 public static DetectedIssueSeverity fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("high".equals(codeString)) 080 return HIGH; 081 if ("moderate".equals(codeString)) 082 return MODERATE; 083 if ("low".equals(codeString)) 084 return LOW; 085 throw new FHIRException("Unknown DetectedIssueSeverity code '" + codeString + "'"); 086 } 087 088 public String toCode() { 089 switch (this) { 090 case HIGH: 091 return "high"; 092 case MODERATE: 093 return "moderate"; 094 case LOW: 095 return "low"; 096 case NULL: 097 return null; 098 default: 099 return "?"; 100 } 101 } 102 103 public String getSystem() { 104 switch (this) { 105 case HIGH: 106 return "http://hl7.org/fhir/detectedissue-severity"; 107 case MODERATE: 108 return "http://hl7.org/fhir/detectedissue-severity"; 109 case LOW: 110 return "http://hl7.org/fhir/detectedissue-severity"; 111 case NULL: 112 return null; 113 default: 114 return "?"; 115 } 116 } 117 118 public String getDefinition() { 119 switch (this) { 120 case HIGH: 121 return "Indicates the issue may be life-threatening or has the potential to cause permanent injury."; 122 case MODERATE: 123 return "Indicates the issue may result in noticeable adverse consequences but is unlikely to be life-threatening or cause permanent injury."; 124 case LOW: 125 return "Indicates the issue may result in some adverse consequences but is unlikely to substantially affect the situation of the subject."; 126 case NULL: 127 return null; 128 default: 129 return "?"; 130 } 131 } 132 133 public String getDisplay() { 134 switch (this) { 135 case HIGH: 136 return "High"; 137 case MODERATE: 138 return "Moderate"; 139 case LOW: 140 return "Low"; 141 case NULL: 142 return null; 143 default: 144 return "?"; 145 } 146 } 147 } 148 149 public static class DetectedIssueSeverityEnumFactory implements EnumFactory<DetectedIssueSeverity> { 150 public DetectedIssueSeverity fromCode(String codeString) throws IllegalArgumentException { 151 if (codeString == null || "".equals(codeString)) 152 if (codeString == null || "".equals(codeString)) 153 return null; 154 if ("high".equals(codeString)) 155 return DetectedIssueSeverity.HIGH; 156 if ("moderate".equals(codeString)) 157 return DetectedIssueSeverity.MODERATE; 158 if ("low".equals(codeString)) 159 return DetectedIssueSeverity.LOW; 160 throw new IllegalArgumentException("Unknown DetectedIssueSeverity code '" + codeString + "'"); 161 } 162 163 public Enumeration<DetectedIssueSeverity> fromType(Base code) throws FHIRException { 164 if (code == null || code.isEmpty()) 165 return null; 166 String codeString = ((PrimitiveType) code).asStringValue(); 167 if (codeString == null || "".equals(codeString)) 168 return null; 169 if ("high".equals(codeString)) 170 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.HIGH); 171 if ("moderate".equals(codeString)) 172 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.MODERATE); 173 if ("low".equals(codeString)) 174 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.LOW); 175 throw new FHIRException("Unknown DetectedIssueSeverity code '" + codeString + "'"); 176 } 177 178 public String toCode(DetectedIssueSeverity code) 179 { 180 if (code == DetectedIssueSeverity.NULL) 181 return null; 182 if (code == DetectedIssueSeverity.HIGH) 183 return "high"; 184 if (code == DetectedIssueSeverity.MODERATE) 185 return "moderate"; 186 if (code == DetectedIssueSeverity.LOW) 187 return "low"; 188 return "?"; 189 } 190 } 191 192 @Block() 193 public static class DetectedIssueMitigationComponent extends BackboneElement implements IBaseBackboneElement { 194 /** 195 * Describes the action that was taken or the observation that was made that 196 * reduces/eliminates the risk associated with the identified issue. 197 */ 198 @Child(name = "action", type = { 199 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 200 @Description(shortDefinition = "What mitigation?", formalDefinition = "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.") 201 protected CodeableConcept action; 202 203 /** 204 * Indicates when the mitigating action was documented. 205 */ 206 @Child(name = "date", type = { DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 207 @Description(shortDefinition = "Date committed", formalDefinition = "Indicates when the mitigating action was documented.") 208 protected DateTimeType date; 209 210 /** 211 * Identifies the practitioner who determined the mitigation and takes 212 * responsibility for the mitigation step occurring. 213 */ 214 @Child(name = "author", type = { 215 Practitioner.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 216 @Description(shortDefinition = "Who is committing?", formalDefinition = "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.") 217 protected Reference author; 218 219 /** 220 * The actual object that is the target of the reference (Identifies the 221 * practitioner who determined the mitigation and takes responsibility for the 222 * mitigation step occurring.) 223 */ 224 protected Practitioner authorTarget; 225 226 private static final long serialVersionUID = -1994768436L; 227 228 /* 229 * Constructor 230 */ 231 public DetectedIssueMitigationComponent() { 232 super(); 233 } 234 235 /* 236 * Constructor 237 */ 238 public DetectedIssueMitigationComponent(CodeableConcept action) { 239 super(); 240 this.action = action; 241 } 242 243 /** 244 * @return {@link #action} (Describes the action that was taken or the 245 * observation that was made that reduces/eliminates the risk associated 246 * with the identified issue.) 247 */ 248 public CodeableConcept getAction() { 249 if (this.action == null) 250 if (Configuration.errorOnAutoCreate()) 251 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.action"); 252 else if (Configuration.doAutoCreate()) 253 this.action = new CodeableConcept(); // cc 254 return this.action; 255 } 256 257 public boolean hasAction() { 258 return this.action != null && !this.action.isEmpty(); 259 } 260 261 /** 262 * @param value {@link #action} (Describes the action that was taken or the 263 * observation that was made that reduces/eliminates the risk 264 * associated with the identified issue.) 265 */ 266 public DetectedIssueMitigationComponent setAction(CodeableConcept value) { 267 this.action = value; 268 return this; 269 } 270 271 /** 272 * @return {@link #date} (Indicates when the mitigating action was documented.). 273 * This is the underlying object with id, value and extensions. The 274 * accessor "getDate" gives direct access to the value 275 */ 276 public DateTimeType getDateElement() { 277 if (this.date == null) 278 if (Configuration.errorOnAutoCreate()) 279 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.date"); 280 else if (Configuration.doAutoCreate()) 281 this.date = new DateTimeType(); // bb 282 return this.date; 283 } 284 285 public boolean hasDateElement() { 286 return this.date != null && !this.date.isEmpty(); 287 } 288 289 public boolean hasDate() { 290 return this.date != null && !this.date.isEmpty(); 291 } 292 293 /** 294 * @param value {@link #date} (Indicates when the mitigating action was 295 * documented.). This is the underlying object with id, value and 296 * extensions. The accessor "getDate" gives direct access to the 297 * value 298 */ 299 public DetectedIssueMitigationComponent setDateElement(DateTimeType value) { 300 this.date = value; 301 return this; 302 } 303 304 /** 305 * @return Indicates when the mitigating action was documented. 306 */ 307 public Date getDate() { 308 return this.date == null ? null : this.date.getValue(); 309 } 310 311 /** 312 * @param value Indicates when the mitigating action was documented. 313 */ 314 public DetectedIssueMitigationComponent setDate(Date value) { 315 if (value == null) 316 this.date = null; 317 else { 318 if (this.date == null) 319 this.date = new DateTimeType(); 320 this.date.setValue(value); 321 } 322 return this; 323 } 324 325 /** 326 * @return {@link #author} (Identifies the practitioner who determined the 327 * mitigation and takes responsibility for the mitigation step 328 * occurring.) 329 */ 330 public Reference getAuthor() { 331 if (this.author == null) 332 if (Configuration.errorOnAutoCreate()) 333 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.author"); 334 else if (Configuration.doAutoCreate()) 335 this.author = new Reference(); // cc 336 return this.author; 337 } 338 339 public boolean hasAuthor() { 340 return this.author != null && !this.author.isEmpty(); 341 } 342 343 /** 344 * @param value {@link #author} (Identifies the practitioner who determined the 345 * mitigation and takes responsibility for the mitigation step 346 * occurring.) 347 */ 348 public DetectedIssueMitigationComponent setAuthor(Reference value) { 349 this.author = value; 350 return this; 351 } 352 353 /** 354 * @return {@link #author} The actual object that is the target of the 355 * reference. The reference library doesn't populate this, but you can 356 * use it to hold the resource if you resolve it. (Identifies the 357 * practitioner who determined the mitigation and takes responsibility 358 * for the mitigation step occurring.) 359 */ 360 public Practitioner getAuthorTarget() { 361 if (this.authorTarget == null) 362 if (Configuration.errorOnAutoCreate()) 363 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.author"); 364 else if (Configuration.doAutoCreate()) 365 this.authorTarget = new Practitioner(); // aa 366 return this.authorTarget; 367 } 368 369 /** 370 * @param value {@link #author} The actual object that is the target of the 371 * reference. The reference library doesn't use these, but you can 372 * use it to hold the resource if you resolve it. (Identifies the 373 * practitioner who determined the mitigation and takes 374 * responsibility for the mitigation step occurring.) 375 */ 376 public DetectedIssueMitigationComponent setAuthorTarget(Practitioner value) { 377 this.authorTarget = value; 378 return this; 379 } 380 381 protected void listChildren(List<Property> childrenList) { 382 super.listChildren(childrenList); 383 childrenList.add(new Property("action", "CodeableConcept", 384 "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.", 385 0, java.lang.Integer.MAX_VALUE, action)); 386 childrenList.add(new Property("date", "dateTime", "Indicates when the mitigating action was documented.", 0, 387 java.lang.Integer.MAX_VALUE, date)); 388 childrenList.add(new Property("author", "Reference(Practitioner)", 389 "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.", 390 0, java.lang.Integer.MAX_VALUE, author)); 391 } 392 393 @Override 394 public void setProperty(String name, Base value) throws FHIRException { 395 if (name.equals("action")) 396 this.action = castToCodeableConcept(value); // CodeableConcept 397 else if (name.equals("date")) 398 this.date = castToDateTime(value); // DateTimeType 399 else if (name.equals("author")) 400 this.author = castToReference(value); // Reference 401 else 402 super.setProperty(name, value); 403 } 404 405 @Override 406 public Base addChild(String name) throws FHIRException { 407 if (name.equals("action")) { 408 this.action = new CodeableConcept(); 409 return this.action; 410 } else if (name.equals("date")) { 411 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.date"); 412 } else if (name.equals("author")) { 413 this.author = new Reference(); 414 return this.author; 415 } else 416 return super.addChild(name); 417 } 418 419 public DetectedIssueMitigationComponent copy() { 420 DetectedIssueMitigationComponent dst = new DetectedIssueMitigationComponent(); 421 copyValues(dst); 422 dst.action = action == null ? null : action.copy(); 423 dst.date = date == null ? null : date.copy(); 424 dst.author = author == null ? null : author.copy(); 425 return dst; 426 } 427 428 @Override 429 public boolean equalsDeep(Base other) { 430 if (!super.equalsDeep(other)) 431 return false; 432 if (!(other instanceof DetectedIssueMitigationComponent)) 433 return false; 434 DetectedIssueMitigationComponent o = (DetectedIssueMitigationComponent) other; 435 return compareDeep(action, o.action, true) && compareDeep(date, o.date, true) 436 && compareDeep(author, o.author, true); 437 } 438 439 @Override 440 public boolean equalsShallow(Base other) { 441 if (!super.equalsShallow(other)) 442 return false; 443 if (!(other instanceof DetectedIssueMitigationComponent)) 444 return false; 445 DetectedIssueMitigationComponent o = (DetectedIssueMitigationComponent) other; 446 return compareValues(date, o.date, true); 447 } 448 449 public boolean isEmpty() { 450 return super.isEmpty() && (action == null || action.isEmpty()) && (date == null || date.isEmpty()) 451 && (author == null || author.isEmpty()); 452 } 453 454 public String fhirType() { 455 return "DetectedIssue.mitigation"; 456 457 } 458 459 } 460 461 /** 462 * Indicates the patient whose record the detected issue is associated with. 463 */ 464 @Child(name = "patient", type = { Patient.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 465 @Description(shortDefinition = "Associated patient", formalDefinition = "Indicates the patient whose record the detected issue is associated with.") 466 protected Reference patient; 467 468 /** 469 * The actual object that is the target of the reference (Indicates the patient 470 * whose record the detected issue is associated with.) 471 */ 472 protected Patient patientTarget; 473 474 /** 475 * Identifies the general type of issue identified. 476 */ 477 @Child(name = "category", type = { 478 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 479 @Description(shortDefinition = "Issue Category, e.g. drug-drug, duplicate therapy, etc.", formalDefinition = "Identifies the general type of issue identified.") 480 protected CodeableConcept category; 481 482 /** 483 * Indicates the degree of importance associated with the identified issue based 484 * on the potential impact on the patient. 485 */ 486 @Child(name = "severity", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 487 @Description(shortDefinition = "high | moderate | low", formalDefinition = "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.") 488 protected Enumeration<DetectedIssueSeverity> severity; 489 490 /** 491 * Indicates the resource representing the current activity or proposed activity 492 * that is potentially problematic. 493 */ 494 @Child(name = "implicated", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 495 @Description(shortDefinition = "Problem resource", formalDefinition = "Indicates the resource representing the current activity or proposed activity that is potentially problematic.") 496 protected List<Reference> implicated; 497 /** 498 * The actual objects that are the target of the reference (Indicates the 499 * resource representing the current activity or proposed activity that is 500 * potentially problematic.) 501 */ 502 protected List<Resource> implicatedTarget; 503 504 /** 505 * A textual explanation of the detected issue. 506 */ 507 @Child(name = "detail", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 508 @Description(shortDefinition = "Description and context", formalDefinition = "A textual explanation of the detected issue.") 509 protected StringType detail; 510 511 /** 512 * The date or date-time when the detected issue was initially identified. 513 */ 514 @Child(name = "date", type = { DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 515 @Description(shortDefinition = "When identified", formalDefinition = "The date or date-time when the detected issue was initially identified.") 516 protected DateTimeType date; 517 518 /** 519 * Individual or device responsible for the issue being raised. For example, a 520 * decision support application or a pharmacist conducting a medication review. 521 */ 522 @Child(name = "author", type = { Practitioner.class, 523 Device.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 524 @Description(shortDefinition = "The provider or device that identified the issue", formalDefinition = "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.") 525 protected Reference author; 526 527 /** 528 * The actual object that is the target of the reference (Individual or device 529 * responsible for the issue being raised. For example, a decision support 530 * application or a pharmacist conducting a medication review.) 531 */ 532 protected Resource authorTarget; 533 534 /** 535 * Business identifier associated with the detected issue record. 536 */ 537 @Child(name = "identifier", type = { 538 Identifier.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 539 @Description(shortDefinition = "Unique id for the detected issue", formalDefinition = "Business identifier associated with the detected issue record.") 540 protected Identifier identifier; 541 542 /** 543 * The literature, knowledge-base or similar reference that describes the 544 * propensity for the detected issue identified. 545 */ 546 @Child(name = "reference", type = { UriType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 547 @Description(shortDefinition = "Authority for issue", formalDefinition = "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.") 548 protected UriType reference; 549 550 /** 551 * Indicates an action that has been taken or is committed to to reduce or 552 * eliminate the likelihood of the risk identified by the detected issue from 553 * manifesting. Can also reflect an observation of known mitigating factors that 554 * may reduce/eliminate the need for any action. 555 */ 556 @Child(name = "mitigation", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 557 @Description(shortDefinition = "Step taken to address", formalDefinition = "Indicates an action that has been taken or is committed to to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.") 558 protected List<DetectedIssueMitigationComponent> mitigation; 559 560 private static final long serialVersionUID = -403732234L; 561 562 /* 563 * Constructor 564 */ 565 public DetectedIssue() { 566 super(); 567 } 568 569 /** 570 * @return {@link #patient} (Indicates the patient whose record the detected 571 * issue is associated with.) 572 */ 573 public Reference getPatient() { 574 if (this.patient == null) 575 if (Configuration.errorOnAutoCreate()) 576 throw new Error("Attempt to auto-create DetectedIssue.patient"); 577 else if (Configuration.doAutoCreate()) 578 this.patient = new Reference(); // cc 579 return this.patient; 580 } 581 582 public boolean hasPatient() { 583 return this.patient != null && !this.patient.isEmpty(); 584 } 585 586 /** 587 * @param value {@link #patient} (Indicates the patient whose record the 588 * detected issue is associated with.) 589 */ 590 public DetectedIssue setPatient(Reference value) { 591 this.patient = value; 592 return this; 593 } 594 595 /** 596 * @return {@link #patient} The actual object that is the target of the 597 * reference. The reference library doesn't populate this, but you can 598 * use it to hold the resource if you resolve it. (Indicates the patient 599 * whose record the detected issue is associated with.) 600 */ 601 public Patient getPatientTarget() { 602 if (this.patientTarget == null) 603 if (Configuration.errorOnAutoCreate()) 604 throw new Error("Attempt to auto-create DetectedIssue.patient"); 605 else if (Configuration.doAutoCreate()) 606 this.patientTarget = new Patient(); // aa 607 return this.patientTarget; 608 } 609 610 /** 611 * @param value {@link #patient} The actual object that is the target of the 612 * reference. The reference library doesn't use these, but you can 613 * use it to hold the resource if you resolve it. (Indicates the 614 * patient whose record the detected issue is associated with.) 615 */ 616 public DetectedIssue setPatientTarget(Patient value) { 617 this.patientTarget = value; 618 return this; 619 } 620 621 /** 622 * @return {@link #category} (Identifies the general type of issue identified.) 623 */ 624 public CodeableConcept getCategory() { 625 if (this.category == null) 626 if (Configuration.errorOnAutoCreate()) 627 throw new Error("Attempt to auto-create DetectedIssue.category"); 628 else if (Configuration.doAutoCreate()) 629 this.category = new CodeableConcept(); // cc 630 return this.category; 631 } 632 633 public boolean hasCategory() { 634 return this.category != null && !this.category.isEmpty(); 635 } 636 637 /** 638 * @param value {@link #category} (Identifies the general type of issue 639 * identified.) 640 */ 641 public DetectedIssue setCategory(CodeableConcept value) { 642 this.category = value; 643 return this; 644 } 645 646 /** 647 * @return {@link #severity} (Indicates the degree of importance associated with 648 * the identified issue based on the potential impact on the patient.). 649 * This is the underlying object with id, value and extensions. The 650 * accessor "getSeverity" gives direct access to the value 651 */ 652 public Enumeration<DetectedIssueSeverity> getSeverityElement() { 653 if (this.severity == null) 654 if (Configuration.errorOnAutoCreate()) 655 throw new Error("Attempt to auto-create DetectedIssue.severity"); 656 else if (Configuration.doAutoCreate()) 657 this.severity = new Enumeration<DetectedIssueSeverity>(new DetectedIssueSeverityEnumFactory()); // bb 658 return this.severity; 659 } 660 661 public boolean hasSeverityElement() { 662 return this.severity != null && !this.severity.isEmpty(); 663 } 664 665 public boolean hasSeverity() { 666 return this.severity != null && !this.severity.isEmpty(); 667 } 668 669 /** 670 * @param value {@link #severity} (Indicates the degree of importance associated 671 * with the identified issue based on the potential impact on the 672 * patient.). This is the underlying object with id, value and 673 * extensions. The accessor "getSeverity" gives direct access to 674 * the value 675 */ 676 public DetectedIssue setSeverityElement(Enumeration<DetectedIssueSeverity> value) { 677 this.severity = value; 678 return this; 679 } 680 681 /** 682 * @return Indicates the degree of importance associated with the identified 683 * issue based on the potential impact on the patient. 684 */ 685 public DetectedIssueSeverity getSeverity() { 686 return this.severity == null ? null : this.severity.getValue(); 687 } 688 689 /** 690 * @param value Indicates the degree of importance associated with the 691 * identified issue based on the potential impact on the patient. 692 */ 693 public DetectedIssue setSeverity(DetectedIssueSeverity value) { 694 if (value == null) 695 this.severity = null; 696 else { 697 if (this.severity == null) 698 this.severity = new Enumeration<DetectedIssueSeverity>(new DetectedIssueSeverityEnumFactory()); 699 this.severity.setValue(value); 700 } 701 return this; 702 } 703 704 /** 705 * @return {@link #implicated} (Indicates the resource representing the current 706 * activity or proposed activity that is potentially problematic.) 707 */ 708 public List<Reference> getImplicated() { 709 if (this.implicated == null) 710 this.implicated = new ArrayList<Reference>(); 711 return this.implicated; 712 } 713 714 public boolean hasImplicated() { 715 if (this.implicated == null) 716 return false; 717 for (Reference item : this.implicated) 718 if (!item.isEmpty()) 719 return true; 720 return false; 721 } 722 723 /** 724 * @return {@link #implicated} (Indicates the resource representing the current 725 * activity or proposed activity that is potentially problematic.) 726 */ 727 // syntactic sugar 728 public Reference addImplicated() { // 3 729 Reference t = new Reference(); 730 if (this.implicated == null) 731 this.implicated = new ArrayList<Reference>(); 732 this.implicated.add(t); 733 return t; 734 } 735 736 // syntactic sugar 737 public DetectedIssue addImplicated(Reference t) { // 3 738 if (t == null) 739 return this; 740 if (this.implicated == null) 741 this.implicated = new ArrayList<Reference>(); 742 this.implicated.add(t); 743 return this; 744 } 745 746 /** 747 * @return {@link #implicated} (The actual objects that are the target of the 748 * reference. The reference library doesn't populate this, but you can 749 * use this to hold the resources if you resolvethemt. Indicates the 750 * resource representing the current activity or proposed activity that 751 * is potentially problematic.) 752 */ 753 public List<Resource> getImplicatedTarget() { 754 if (this.implicatedTarget == null) 755 this.implicatedTarget = new ArrayList<Resource>(); 756 return this.implicatedTarget; 757 } 758 759 /** 760 * @return {@link #detail} (A textual explanation of the detected issue.). This 761 * is the underlying object with id, value and extensions. The accessor 762 * "getDetail" gives direct access to the value 763 */ 764 public StringType getDetailElement() { 765 if (this.detail == null) 766 if (Configuration.errorOnAutoCreate()) 767 throw new Error("Attempt to auto-create DetectedIssue.detail"); 768 else if (Configuration.doAutoCreate()) 769 this.detail = new StringType(); // bb 770 return this.detail; 771 } 772 773 public boolean hasDetailElement() { 774 return this.detail != null && !this.detail.isEmpty(); 775 } 776 777 public boolean hasDetail() { 778 return this.detail != null && !this.detail.isEmpty(); 779 } 780 781 /** 782 * @param value {@link #detail} (A textual explanation of the detected issue.). 783 * This is the underlying object with id, value and extensions. The 784 * accessor "getDetail" gives direct access to the value 785 */ 786 public DetectedIssue setDetailElement(StringType value) { 787 this.detail = value; 788 return this; 789 } 790 791 /** 792 * @return A textual explanation of the detected issue. 793 */ 794 public String getDetail() { 795 return this.detail == null ? null : this.detail.getValue(); 796 } 797 798 /** 799 * @param value A textual explanation of the detected issue. 800 */ 801 public DetectedIssue setDetail(String value) { 802 if (Utilities.noString(value)) 803 this.detail = null; 804 else { 805 if (this.detail == null) 806 this.detail = new StringType(); 807 this.detail.setValue(value); 808 } 809 return this; 810 } 811 812 /** 813 * @return {@link #date} (The date or date-time when the detected issue was 814 * initially identified.). This is the underlying object with id, value 815 * and extensions. The accessor "getDate" gives direct access to the 816 * value 817 */ 818 public DateTimeType getDateElement() { 819 if (this.date == null) 820 if (Configuration.errorOnAutoCreate()) 821 throw new Error("Attempt to auto-create DetectedIssue.date"); 822 else if (Configuration.doAutoCreate()) 823 this.date = new DateTimeType(); // bb 824 return this.date; 825 } 826 827 public boolean hasDateElement() { 828 return this.date != null && !this.date.isEmpty(); 829 } 830 831 public boolean hasDate() { 832 return this.date != null && !this.date.isEmpty(); 833 } 834 835 /** 836 * @param value {@link #date} (The date or date-time when the detected issue was 837 * initially identified.). This is the underlying object with id, 838 * value and extensions. The accessor "getDate" gives direct access 839 * to the value 840 */ 841 public DetectedIssue setDateElement(DateTimeType value) { 842 this.date = value; 843 return this; 844 } 845 846 /** 847 * @return The date or date-time when the detected issue was initially 848 * identified. 849 */ 850 public Date getDate() { 851 return this.date == null ? null : this.date.getValue(); 852 } 853 854 /** 855 * @param value The date or date-time when the detected issue was initially 856 * identified. 857 */ 858 public DetectedIssue setDate(Date value) { 859 if (value == null) 860 this.date = null; 861 else { 862 if (this.date == null) 863 this.date = new DateTimeType(); 864 this.date.setValue(value); 865 } 866 return this; 867 } 868 869 /** 870 * @return {@link #author} (Individual or device responsible for the issue being 871 * raised. For example, a decision support application or a pharmacist 872 * conducting a medication review.) 873 */ 874 public Reference getAuthor() { 875 if (this.author == null) 876 if (Configuration.errorOnAutoCreate()) 877 throw new Error("Attempt to auto-create DetectedIssue.author"); 878 else if (Configuration.doAutoCreate()) 879 this.author = new Reference(); // cc 880 return this.author; 881 } 882 883 public boolean hasAuthor() { 884 return this.author != null && !this.author.isEmpty(); 885 } 886 887 /** 888 * @param value {@link #author} (Individual or device responsible for the issue 889 * being raised. For example, a decision support application or a 890 * pharmacist conducting a medication review.) 891 */ 892 public DetectedIssue setAuthor(Reference value) { 893 this.author = value; 894 return this; 895 } 896 897 /** 898 * @return {@link #author} The actual object that is the target of the 899 * reference. The reference library doesn't populate this, but you can 900 * use it to hold the resource if you resolve it. (Individual or device 901 * responsible for the issue being raised. For example, a decision 902 * support application or a pharmacist conducting a medication review.) 903 */ 904 public Resource getAuthorTarget() { 905 return this.authorTarget; 906 } 907 908 /** 909 * @param value {@link #author} The actual object that is the target of the 910 * reference. The reference library doesn't use these, but you can 911 * use it to hold the resource if you resolve it. (Individual or 912 * device responsible for the issue being raised. For example, a 913 * decision support application or a pharmacist conducting a 914 * medication review.) 915 */ 916 public DetectedIssue setAuthorTarget(Resource value) { 917 this.authorTarget = value; 918 return this; 919 } 920 921 /** 922 * @return {@link #identifier} (Business identifier associated with the detected 923 * issue record.) 924 */ 925 public Identifier getIdentifier() { 926 if (this.identifier == null) 927 if (Configuration.errorOnAutoCreate()) 928 throw new Error("Attempt to auto-create DetectedIssue.identifier"); 929 else if (Configuration.doAutoCreate()) 930 this.identifier = new Identifier(); // cc 931 return this.identifier; 932 } 933 934 public boolean hasIdentifier() { 935 return this.identifier != null && !this.identifier.isEmpty(); 936 } 937 938 /** 939 * @param value {@link #identifier} (Business identifier associated with the 940 * detected issue record.) 941 */ 942 public DetectedIssue setIdentifier(Identifier value) { 943 this.identifier = value; 944 return this; 945 } 946 947 /** 948 * @return {@link #reference} (The literature, knowledge-base or similar 949 * reference that describes the propensity for the detected issue 950 * identified.). This is the underlying object with id, value and 951 * extensions. The accessor "getReference" gives direct access to the 952 * value 953 */ 954 public UriType getReferenceElement() { 955 if (this.reference == null) 956 if (Configuration.errorOnAutoCreate()) 957 throw new Error("Attempt to auto-create DetectedIssue.reference"); 958 else if (Configuration.doAutoCreate()) 959 this.reference = new UriType(); // bb 960 return this.reference; 961 } 962 963 public boolean hasReferenceElement() { 964 return this.reference != null && !this.reference.isEmpty(); 965 } 966 967 public boolean hasReference() { 968 return this.reference != null && !this.reference.isEmpty(); 969 } 970 971 /** 972 * @param value {@link #reference} (The literature, knowledge-base or similar 973 * reference that describes the propensity for the detected issue 974 * identified.). This is the underlying object with id, value and 975 * extensions. The accessor "getReference" gives direct access to 976 * the value 977 */ 978 public DetectedIssue setReferenceElement(UriType value) { 979 this.reference = value; 980 return this; 981 } 982 983 /** 984 * @return The literature, knowledge-base or similar reference that describes 985 * the propensity for the detected issue identified. 986 */ 987 public String getReference() { 988 return this.reference == null ? null : this.reference.getValue(); 989 } 990 991 /** 992 * @param value The literature, knowledge-base or similar reference that 993 * describes the propensity for the detected issue identified. 994 */ 995 public DetectedIssue setReference(String value) { 996 if (Utilities.noString(value)) 997 this.reference = null; 998 else { 999 if (this.reference == null) 1000 this.reference = new UriType(); 1001 this.reference.setValue(value); 1002 } 1003 return this; 1004 } 1005 1006 /** 1007 * @return {@link #mitigation} (Indicates an action that has been taken or is 1008 * committed to to reduce or eliminate the likelihood of the risk 1009 * identified by the detected issue from manifesting. Can also reflect 1010 * an observation of known mitigating factors that may reduce/eliminate 1011 * the need for any action.) 1012 */ 1013 public List<DetectedIssueMitigationComponent> getMitigation() { 1014 if (this.mitigation == null) 1015 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1016 return this.mitigation; 1017 } 1018 1019 public boolean hasMitigation() { 1020 if (this.mitigation == null) 1021 return false; 1022 for (DetectedIssueMitigationComponent item : this.mitigation) 1023 if (!item.isEmpty()) 1024 return true; 1025 return false; 1026 } 1027 1028 /** 1029 * @return {@link #mitigation} (Indicates an action that has been taken or is 1030 * committed to to reduce or eliminate the likelihood of the risk 1031 * identified by the detected issue from manifesting. Can also reflect 1032 * an observation of known mitigating factors that may reduce/eliminate 1033 * the need for any action.) 1034 */ 1035 // syntactic sugar 1036 public DetectedIssueMitigationComponent addMitigation() { // 3 1037 DetectedIssueMitigationComponent t = new DetectedIssueMitigationComponent(); 1038 if (this.mitigation == null) 1039 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1040 this.mitigation.add(t); 1041 return t; 1042 } 1043 1044 // syntactic sugar 1045 public DetectedIssue addMitigation(DetectedIssueMitigationComponent t) { // 3 1046 if (t == null) 1047 return this; 1048 if (this.mitigation == null) 1049 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1050 this.mitigation.add(t); 1051 return this; 1052 } 1053 1054 protected void listChildren(List<Property> childrenList) { 1055 super.listChildren(childrenList); 1056 childrenList.add(new Property("patient", "Reference(Patient)", 1057 "Indicates the patient whose record the detected issue is associated with.", 0, java.lang.Integer.MAX_VALUE, 1058 patient)); 1059 childrenList.add(new Property("category", "CodeableConcept", "Identifies the general type of issue identified.", 0, 1060 java.lang.Integer.MAX_VALUE, category)); 1061 childrenList.add(new Property("severity", "code", 1062 "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.", 1063 0, java.lang.Integer.MAX_VALUE, severity)); 1064 childrenList.add(new Property("implicated", "Reference(Any)", 1065 "Indicates the resource representing the current activity or proposed activity that is potentially problematic.", 1066 0, java.lang.Integer.MAX_VALUE, implicated)); 1067 childrenList.add(new Property("detail", "string", "A textual explanation of the detected issue.", 0, 1068 java.lang.Integer.MAX_VALUE, detail)); 1069 childrenList 1070 .add(new Property("date", "dateTime", "The date or date-time when the detected issue was initially identified.", 1071 0, java.lang.Integer.MAX_VALUE, date)); 1072 childrenList.add(new Property("author", "Reference(Practitioner|Device)", 1073 "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.", 1074 0, java.lang.Integer.MAX_VALUE, author)); 1075 childrenList.add(new Property("identifier", "Identifier", 1076 "Business identifier associated with the detected issue record.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1077 childrenList.add(new Property("reference", "uri", 1078 "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.", 1079 0, java.lang.Integer.MAX_VALUE, reference)); 1080 childrenList.add(new Property("mitigation", "", 1081 "Indicates an action that has been taken or is committed to to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.", 1082 0, java.lang.Integer.MAX_VALUE, mitigation)); 1083 } 1084 1085 @Override 1086 public void setProperty(String name, Base value) throws FHIRException { 1087 if (name.equals("patient")) 1088 this.patient = castToReference(value); // Reference 1089 else if (name.equals("category")) 1090 this.category = castToCodeableConcept(value); // CodeableConcept 1091 else if (name.equals("severity")) 1092 this.severity = new DetectedIssueSeverityEnumFactory().fromType(value); // Enumeration<DetectedIssueSeverity> 1093 else if (name.equals("implicated")) 1094 this.getImplicated().add(castToReference(value)); 1095 else if (name.equals("detail")) 1096 this.detail = castToString(value); // StringType 1097 else if (name.equals("date")) 1098 this.date = castToDateTime(value); // DateTimeType 1099 else if (name.equals("author")) 1100 this.author = castToReference(value); // Reference 1101 else if (name.equals("identifier")) 1102 this.identifier = castToIdentifier(value); // Identifier 1103 else if (name.equals("reference")) 1104 this.reference = castToUri(value); // UriType 1105 else if (name.equals("mitigation")) 1106 this.getMitigation().add((DetectedIssueMitigationComponent) value); 1107 else 1108 super.setProperty(name, value); 1109 } 1110 1111 @Override 1112 public Base addChild(String name) throws FHIRException { 1113 if (name.equals("patient")) { 1114 this.patient = new Reference(); 1115 return this.patient; 1116 } else if (name.equals("category")) { 1117 this.category = new CodeableConcept(); 1118 return this.category; 1119 } else if (name.equals("severity")) { 1120 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.severity"); 1121 } else if (name.equals("implicated")) { 1122 return addImplicated(); 1123 } else if (name.equals("detail")) { 1124 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.detail"); 1125 } else if (name.equals("date")) { 1126 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.date"); 1127 } else if (name.equals("author")) { 1128 this.author = new Reference(); 1129 return this.author; 1130 } else if (name.equals("identifier")) { 1131 this.identifier = new Identifier(); 1132 return this.identifier; 1133 } else if (name.equals("reference")) { 1134 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.reference"); 1135 } else if (name.equals("mitigation")) { 1136 return addMitigation(); 1137 } else 1138 return super.addChild(name); 1139 } 1140 1141 public String fhirType() { 1142 return "DetectedIssue"; 1143 1144 } 1145 1146 public DetectedIssue copy() { 1147 DetectedIssue dst = new DetectedIssue(); 1148 copyValues(dst); 1149 dst.patient = patient == null ? null : patient.copy(); 1150 dst.category = category == null ? null : category.copy(); 1151 dst.severity = severity == null ? null : severity.copy(); 1152 if (implicated != null) { 1153 dst.implicated = new ArrayList<Reference>(); 1154 for (Reference i : implicated) 1155 dst.implicated.add(i.copy()); 1156 } 1157 ; 1158 dst.detail = detail == null ? null : detail.copy(); 1159 dst.date = date == null ? null : date.copy(); 1160 dst.author = author == null ? null : author.copy(); 1161 dst.identifier = identifier == null ? null : identifier.copy(); 1162 dst.reference = reference == null ? null : reference.copy(); 1163 if (mitigation != null) { 1164 dst.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1165 for (DetectedIssueMitigationComponent i : mitigation) 1166 dst.mitigation.add(i.copy()); 1167 } 1168 ; 1169 return dst; 1170 } 1171 1172 protected DetectedIssue typedCopy() { 1173 return copy(); 1174 } 1175 1176 @Override 1177 public boolean equalsDeep(Base other) { 1178 if (!super.equalsDeep(other)) 1179 return false; 1180 if (!(other instanceof DetectedIssue)) 1181 return false; 1182 DetectedIssue o = (DetectedIssue) other; 1183 return compareDeep(patient, o.patient, true) && compareDeep(category, o.category, true) 1184 && compareDeep(severity, o.severity, true) && compareDeep(implicated, o.implicated, true) 1185 && compareDeep(detail, o.detail, true) && compareDeep(date, o.date, true) && compareDeep(author, o.author, true) 1186 && compareDeep(identifier, o.identifier, true) && compareDeep(reference, o.reference, true) 1187 && compareDeep(mitigation, o.mitigation, true); 1188 } 1189 1190 @Override 1191 public boolean equalsShallow(Base other) { 1192 if (!super.equalsShallow(other)) 1193 return false; 1194 if (!(other instanceof DetectedIssue)) 1195 return false; 1196 DetectedIssue o = (DetectedIssue) other; 1197 return compareValues(severity, o.severity, true) && compareValues(detail, o.detail, true) 1198 && compareValues(date, o.date, true) && compareValues(reference, o.reference, true); 1199 } 1200 1201 public boolean isEmpty() { 1202 return super.isEmpty() && (patient == null || patient.isEmpty()) && (category == null || category.isEmpty()) 1203 && (severity == null || severity.isEmpty()) && (implicated == null || implicated.isEmpty()) 1204 && (detail == null || detail.isEmpty()) && (date == null || date.isEmpty()) 1205 && (author == null || author.isEmpty()) && (identifier == null || identifier.isEmpty()) 1206 && (reference == null || reference.isEmpty()) && (mitigation == null || mitigation.isEmpty()); 1207 } 1208 1209 @Override 1210 public ResourceType getResourceType() { 1211 return ResourceType.DetectedIssue; 1212 } 1213 1214 @SearchParamDefinition(name = "date", path = "DetectedIssue.date", description = "When identified", type = "date") 1215 public static final String SP_DATE = "date"; 1216 @SearchParamDefinition(name = "identifier", path = "DetectedIssue.identifier", description = "Unique id for the detected issue", type = "token") 1217 public static final String SP_IDENTIFIER = "identifier"; 1218 @SearchParamDefinition(name = "patient", path = "DetectedIssue.patient", description = "Associated patient", type = "reference") 1219 public static final String SP_PATIENT = "patient"; 1220 @SearchParamDefinition(name = "author", path = "DetectedIssue.author", description = "The provider or device that identified the issue", type = "reference") 1221 public static final String SP_AUTHOR = "author"; 1222 @SearchParamDefinition(name = "implicated", path = "DetectedIssue.implicated", description = "Problem resource", type = "reference") 1223 public static final String SP_IMPLICATED = "implicated"; 1224 @SearchParamDefinition(name = "category", path = "DetectedIssue.category", description = "Issue Category, e.g. drug-drug, duplicate therapy, etc.", type = "token") 1225 public static final String SP_CATEGORY = "category"; 1226 1227}