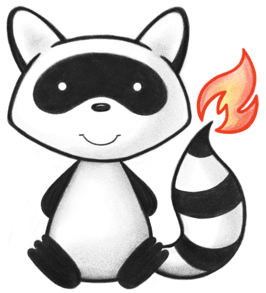
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.utilities.Utilities; 043 044/** 045 * This resource identifies an instance of a manufactured item that is used in 046 * the provision of healthcare without being substantially changed through that 047 * activity. The device may be a medical or non-medical device. Medical devices 048 * includes durable (reusable) medical equipment, implantable devices, as well 049 * as disposable equipment used for diagnostic, treatment, and research for 050 * healthcare and public health. Non-medical devices may include items such as a 051 * machine, cellphone, computer, application, etc. 052 */ 053@ResourceDef(name = "Device", profile = "http://hl7.org/fhir/Profile/Device") 054public class Device extends DomainResource { 055 056 public enum DeviceStatus { 057 /** 058 * The Device is available for use. 059 */ 060 AVAILABLE, 061 /** 062 * The Device is no longer available for use (e.g. lost, expired, damaged). 063 */ 064 NOTAVAILABLE, 065 /** 066 * The Device was entered in error and voided. 067 */ 068 ENTEREDINERROR, 069 /** 070 * added to help the parsers 071 */ 072 NULL; 073 074 public static DeviceStatus fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("available".equals(codeString)) 078 return AVAILABLE; 079 if ("not-available".equals(codeString)) 080 return NOTAVAILABLE; 081 if ("entered-in-error".equals(codeString)) 082 return ENTEREDINERROR; 083 throw new FHIRException("Unknown DeviceStatus code '" + codeString + "'"); 084 } 085 086 public String toCode() { 087 switch (this) { 088 case AVAILABLE: 089 return "available"; 090 case NOTAVAILABLE: 091 return "not-available"; 092 case ENTEREDINERROR: 093 return "entered-in-error"; 094 case NULL: 095 return null; 096 default: 097 return "?"; 098 } 099 } 100 101 public String getSystem() { 102 switch (this) { 103 case AVAILABLE: 104 return "http://hl7.org/fhir/devicestatus"; 105 case NOTAVAILABLE: 106 return "http://hl7.org/fhir/devicestatus"; 107 case ENTEREDINERROR: 108 return "http://hl7.org/fhir/devicestatus"; 109 case NULL: 110 return null; 111 default: 112 return "?"; 113 } 114 } 115 116 public String getDefinition() { 117 switch (this) { 118 case AVAILABLE: 119 return "The Device is available for use."; 120 case NOTAVAILABLE: 121 return "The Device is no longer available for use (e.g. lost, expired, damaged)."; 122 case ENTEREDINERROR: 123 return "The Device was entered in error and voided."; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 131 public String getDisplay() { 132 switch (this) { 133 case AVAILABLE: 134 return "Available"; 135 case NOTAVAILABLE: 136 return "Not Available"; 137 case ENTEREDINERROR: 138 return "Entered in Error"; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 } 146 147 public static class DeviceStatusEnumFactory implements EnumFactory<DeviceStatus> { 148 public DeviceStatus fromCode(String codeString) throws IllegalArgumentException { 149 if (codeString == null || "".equals(codeString)) 150 if (codeString == null || "".equals(codeString)) 151 return null; 152 if ("available".equals(codeString)) 153 return DeviceStatus.AVAILABLE; 154 if ("not-available".equals(codeString)) 155 return DeviceStatus.NOTAVAILABLE; 156 if ("entered-in-error".equals(codeString)) 157 return DeviceStatus.ENTEREDINERROR; 158 throw new IllegalArgumentException("Unknown DeviceStatus code '" + codeString + "'"); 159 } 160 161 public Enumeration<DeviceStatus> fromType(Base code) throws FHIRException { 162 if (code == null || code.isEmpty()) 163 return null; 164 String codeString = ((PrimitiveType) code).asStringValue(); 165 if (codeString == null || "".equals(codeString)) 166 return null; 167 if ("available".equals(codeString)) 168 return new Enumeration<DeviceStatus>(this, DeviceStatus.AVAILABLE); 169 if ("not-available".equals(codeString)) 170 return new Enumeration<DeviceStatus>(this, DeviceStatus.NOTAVAILABLE); 171 if ("entered-in-error".equals(codeString)) 172 return new Enumeration<DeviceStatus>(this, DeviceStatus.ENTEREDINERROR); 173 throw new FHIRException("Unknown DeviceStatus code '" + codeString + "'"); 174 } 175 176 public String toCode(DeviceStatus code) 177 { 178 if (code == DeviceStatus.NULL) 179 return null; 180 if (code == DeviceStatus.AVAILABLE) 181 return "available"; 182 if (code == DeviceStatus.NOTAVAILABLE) 183 return "not-available"; 184 if (code == DeviceStatus.ENTEREDINERROR) 185 return "entered-in-error"; 186 return "?"; 187 } 188 } 189 190 /** 191 * Unique instance identifiers assigned to a device by organizations like 192 * manufacturers or owners. If the identifier identifies the type of device, 193 * Device.type should be used. 194 */ 195 @Child(name = "identifier", type = { 196 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 197 @Description(shortDefinition = "Instance id from manufacturer, owner, and others", formalDefinition = "Unique instance identifiers assigned to a device by organizations like manufacturers or owners. If the identifier identifies the type of device, Device.type should be used.") 198 protected List<Identifier> identifier; 199 200 /** 201 * Code or identifier to identify a kind of device. 202 */ 203 @Child(name = "type", type = { 204 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 205 @Description(shortDefinition = "What kind of device this is", formalDefinition = "Code or identifier to identify a kind of device.") 206 protected CodeableConcept type; 207 208 /** 209 * Descriptive information, usage information or implantation information that 210 * is not captured in an existing element. 211 */ 212 @Child(name = "note", type = { 213 Annotation.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 214 @Description(shortDefinition = "Device notes and comments", formalDefinition = "Descriptive information, usage information or implantation information that is not captured in an existing element.") 215 protected List<Annotation> note; 216 217 /** 218 * Status of the Device availability. 219 */ 220 @Child(name = "status", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = true, summary = true) 221 @Description(shortDefinition = "available | not-available | entered-in-error", formalDefinition = "Status of the Device availability.") 222 protected Enumeration<DeviceStatus> status; 223 224 /** 225 * A name of the manufacturer. 226 */ 227 @Child(name = "manufacturer", type = { 228 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 229 @Description(shortDefinition = "Name of device manufacturer", formalDefinition = "A name of the manufacturer.") 230 protected StringType manufacturer; 231 232 /** 233 * The "model" is an identifier assigned by the manufacturer to identify the 234 * product by its type. This number is shared by the all devices sold as the 235 * same type. 236 */ 237 @Child(name = "model", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 238 @Description(shortDefinition = "Model id assigned by the manufacturer", formalDefinition = "The \"model\" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type.") 239 protected StringType model; 240 241 /** 242 * The version of the device, if the device has multiple releases under the same 243 * model, or if the device is software or carries firmware. 244 */ 245 @Child(name = "version", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 246 @Description(shortDefinition = "Version number (i.e. software)", formalDefinition = "The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware.") 247 protected StringType version; 248 249 /** 250 * The date and time when the device was manufactured. 251 */ 252 @Child(name = "manufactureDate", type = { 253 DateTimeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 254 @Description(shortDefinition = "Manufacture date", formalDefinition = "The date and time when the device was manufactured.") 255 protected DateTimeType manufactureDate; 256 257 /** 258 * The date and time beyond which this device is no longer valid or should not 259 * be used (if applicable). 260 */ 261 @Child(name = "expiry", type = { DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 262 @Description(shortDefinition = "Date and time of expiry of this device (if applicable)", formalDefinition = "The date and time beyond which this device is no longer valid or should not be used (if applicable).") 263 protected DateTimeType expiry; 264 265 /** 266 * United States Food and Drug Administration mandated Unique Device Identifier 267 * (UDI). Use the human readable information (the content that the user sees, 268 * which is sometimes different to the exact syntax represented in the barcode) 269 * - see 270 * http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/default.htm. 271 */ 272 @Child(name = "udi", type = { StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 273 @Description(shortDefinition = "FDA mandated Unique Device Identifier", formalDefinition = "United States Food and Drug Administration mandated Unique Device Identifier (UDI). Use the human readable information (the content that the user sees, which is sometimes different to the exact syntax represented in the barcode) - see http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/default.htm.") 274 protected StringType udi; 275 276 /** 277 * Lot number assigned by the manufacturer. 278 */ 279 @Child(name = "lotNumber", type = { 280 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 281 @Description(shortDefinition = "Lot number of manufacture", formalDefinition = "Lot number assigned by the manufacturer.") 282 protected StringType lotNumber; 283 284 /** 285 * An organization that is responsible for the provision and ongoing maintenance 286 * of the device. 287 */ 288 @Child(name = "owner", type = { Organization.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 289 @Description(shortDefinition = "Organization responsible for device", formalDefinition = "An organization that is responsible for the provision and ongoing maintenance of the device.") 290 protected Reference owner; 291 292 /** 293 * The actual object that is the target of the reference (An organization that 294 * is responsible for the provision and ongoing maintenance of the device.) 295 */ 296 protected Organization ownerTarget; 297 298 /** 299 * The place where the device can be found. 300 */ 301 @Child(name = "location", type = { Location.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 302 @Description(shortDefinition = "Where the resource is found", formalDefinition = "The place where the device can be found.") 303 protected Reference location; 304 305 /** 306 * The actual object that is the target of the reference (The place where the 307 * device can be found.) 308 */ 309 protected Location locationTarget; 310 311 /** 312 * Patient information, if the resource is affixed to a person. 313 */ 314 @Child(name = "patient", type = { Patient.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 315 @Description(shortDefinition = "If the resource is affixed to a person", formalDefinition = "Patient information, if the resource is affixed to a person.") 316 protected Reference patient; 317 318 /** 319 * The actual object that is the target of the reference (Patient information, 320 * if the resource is affixed to a person.) 321 */ 322 protected Patient patientTarget; 323 324 /** 325 * Contact details for an organization or a particular human that is responsible 326 * for the device. 327 */ 328 @Child(name = "contact", type = { 329 ContactPoint.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 330 @Description(shortDefinition = "Details for human/organization for support", formalDefinition = "Contact details for an organization or a particular human that is responsible for the device.") 331 protected List<ContactPoint> contact; 332 333 /** 334 * A network address on which the device may be contacted directly. 335 */ 336 @Child(name = "url", type = { UriType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 337 @Description(shortDefinition = "Network address to contact device", formalDefinition = "A network address on which the device may be contacted directly.") 338 protected UriType url; 339 340 private static final long serialVersionUID = 366690094L; 341 342 /* 343 * Constructor 344 */ 345 public Device() { 346 super(); 347 } 348 349 /* 350 * Constructor 351 */ 352 public Device(CodeableConcept type) { 353 super(); 354 this.type = type; 355 } 356 357 /** 358 * @return {@link #identifier} (Unique instance identifiers assigned to a device 359 * by organizations like manufacturers or owners. If the identifier 360 * identifies the type of device, Device.type should be used.) 361 */ 362 public List<Identifier> getIdentifier() { 363 if (this.identifier == null) 364 this.identifier = new ArrayList<Identifier>(); 365 return this.identifier; 366 } 367 368 public boolean hasIdentifier() { 369 if (this.identifier == null) 370 return false; 371 for (Identifier item : this.identifier) 372 if (!item.isEmpty()) 373 return true; 374 return false; 375 } 376 377 /** 378 * @return {@link #identifier} (Unique instance identifiers assigned to a device 379 * by organizations like manufacturers or owners. If the identifier 380 * identifies the type of device, Device.type should be used.) 381 */ 382 // syntactic sugar 383 public Identifier addIdentifier() { // 3 384 Identifier t = new Identifier(); 385 if (this.identifier == null) 386 this.identifier = new ArrayList<Identifier>(); 387 this.identifier.add(t); 388 return t; 389 } 390 391 // syntactic sugar 392 public Device addIdentifier(Identifier t) { // 3 393 if (t == null) 394 return this; 395 if (this.identifier == null) 396 this.identifier = new ArrayList<Identifier>(); 397 this.identifier.add(t); 398 return this; 399 } 400 401 /** 402 * @return {@link #type} (Code or identifier to identify a kind of device.) 403 */ 404 public CodeableConcept getType() { 405 if (this.type == null) 406 if (Configuration.errorOnAutoCreate()) 407 throw new Error("Attempt to auto-create Device.type"); 408 else if (Configuration.doAutoCreate()) 409 this.type = new CodeableConcept(); // cc 410 return this.type; 411 } 412 413 public boolean hasType() { 414 return this.type != null && !this.type.isEmpty(); 415 } 416 417 /** 418 * @param value {@link #type} (Code or identifier to identify a kind of device.) 419 */ 420 public Device setType(CodeableConcept value) { 421 this.type = value; 422 return this; 423 } 424 425 /** 426 * @return {@link #note} (Descriptive information, usage information or 427 * implantation information that is not captured in an existing 428 * element.) 429 */ 430 public List<Annotation> getNote() { 431 if (this.note == null) 432 this.note = new ArrayList<Annotation>(); 433 return this.note; 434 } 435 436 public boolean hasNote() { 437 if (this.note == null) 438 return false; 439 for (Annotation item : this.note) 440 if (!item.isEmpty()) 441 return true; 442 return false; 443 } 444 445 /** 446 * @return {@link #note} (Descriptive information, usage information or 447 * implantation information that is not captured in an existing 448 * element.) 449 */ 450 // syntactic sugar 451 public Annotation addNote() { // 3 452 Annotation t = new Annotation(); 453 if (this.note == null) 454 this.note = new ArrayList<Annotation>(); 455 this.note.add(t); 456 return t; 457 } 458 459 // syntactic sugar 460 public Device addNote(Annotation t) { // 3 461 if (t == null) 462 return this; 463 if (this.note == null) 464 this.note = new ArrayList<Annotation>(); 465 this.note.add(t); 466 return this; 467 } 468 469 /** 470 * @return {@link #status} (Status of the Device availability.). This is the 471 * underlying object with id, value and extensions. The accessor 472 * "getStatus" gives direct access to the value 473 */ 474 public Enumeration<DeviceStatus> getStatusElement() { 475 if (this.status == null) 476 if (Configuration.errorOnAutoCreate()) 477 throw new Error("Attempt to auto-create Device.status"); 478 else if (Configuration.doAutoCreate()) 479 this.status = new Enumeration<DeviceStatus>(new DeviceStatusEnumFactory()); // bb 480 return this.status; 481 } 482 483 public boolean hasStatusElement() { 484 return this.status != null && !this.status.isEmpty(); 485 } 486 487 public boolean hasStatus() { 488 return this.status != null && !this.status.isEmpty(); 489 } 490 491 /** 492 * @param value {@link #status} (Status of the Device availability.). This is 493 * the underlying object with id, value and extensions. The 494 * accessor "getStatus" gives direct access to the value 495 */ 496 public Device setStatusElement(Enumeration<DeviceStatus> value) { 497 this.status = value; 498 return this; 499 } 500 501 /** 502 * @return Status of the Device availability. 503 */ 504 public DeviceStatus getStatus() { 505 return this.status == null ? null : this.status.getValue(); 506 } 507 508 /** 509 * @param value Status of the Device availability. 510 */ 511 public Device setStatus(DeviceStatus value) { 512 if (value == null) 513 this.status = null; 514 else { 515 if (this.status == null) 516 this.status = new Enumeration<DeviceStatus>(new DeviceStatusEnumFactory()); 517 this.status.setValue(value); 518 } 519 return this; 520 } 521 522 /** 523 * @return {@link #manufacturer} (A name of the manufacturer.). This is the 524 * underlying object with id, value and extensions. The accessor 525 * "getManufacturer" gives direct access to the value 526 */ 527 public StringType getManufacturerElement() { 528 if (this.manufacturer == null) 529 if (Configuration.errorOnAutoCreate()) 530 throw new Error("Attempt to auto-create Device.manufacturer"); 531 else if (Configuration.doAutoCreate()) 532 this.manufacturer = new StringType(); // bb 533 return this.manufacturer; 534 } 535 536 public boolean hasManufacturerElement() { 537 return this.manufacturer != null && !this.manufacturer.isEmpty(); 538 } 539 540 public boolean hasManufacturer() { 541 return this.manufacturer != null && !this.manufacturer.isEmpty(); 542 } 543 544 /** 545 * @param value {@link #manufacturer} (A name of the manufacturer.). This is the 546 * underlying object with id, value and extensions. The accessor 547 * "getManufacturer" gives direct access to the value 548 */ 549 public Device setManufacturerElement(StringType value) { 550 this.manufacturer = value; 551 return this; 552 } 553 554 /** 555 * @return A name of the manufacturer. 556 */ 557 public String getManufacturer() { 558 return this.manufacturer == null ? null : this.manufacturer.getValue(); 559 } 560 561 /** 562 * @param value A name of the manufacturer. 563 */ 564 public Device setManufacturer(String value) { 565 if (Utilities.noString(value)) 566 this.manufacturer = null; 567 else { 568 if (this.manufacturer == null) 569 this.manufacturer = new StringType(); 570 this.manufacturer.setValue(value); 571 } 572 return this; 573 } 574 575 /** 576 * @return {@link #model} (The "model" is an identifier assigned by the 577 * manufacturer to identify the product by its type. This number is 578 * shared by the all devices sold as the same type.). This is the 579 * underlying object with id, value and extensions. The accessor 580 * "getModel" gives direct access to the value 581 */ 582 public StringType getModelElement() { 583 if (this.model == null) 584 if (Configuration.errorOnAutoCreate()) 585 throw new Error("Attempt to auto-create Device.model"); 586 else if (Configuration.doAutoCreate()) 587 this.model = new StringType(); // bb 588 return this.model; 589 } 590 591 public boolean hasModelElement() { 592 return this.model != null && !this.model.isEmpty(); 593 } 594 595 public boolean hasModel() { 596 return this.model != null && !this.model.isEmpty(); 597 } 598 599 /** 600 * @param value {@link #model} (The "model" is an identifier assigned by the 601 * manufacturer to identify the product by its type. This number is 602 * shared by the all devices sold as the same type.). This is the 603 * underlying object with id, value and extensions. The accessor 604 * "getModel" gives direct access to the value 605 */ 606 public Device setModelElement(StringType value) { 607 this.model = value; 608 return this; 609 } 610 611 /** 612 * @return The "model" is an identifier assigned by the manufacturer to identify 613 * the product by its type. This number is shared by the all devices 614 * sold as the same type. 615 */ 616 public String getModel() { 617 return this.model == null ? null : this.model.getValue(); 618 } 619 620 /** 621 * @param value The "model" is an identifier assigned by the manufacturer to 622 * identify the product by its type. This number is shared by the 623 * all devices sold as the same type. 624 */ 625 public Device setModel(String value) { 626 if (Utilities.noString(value)) 627 this.model = null; 628 else { 629 if (this.model == null) 630 this.model = new StringType(); 631 this.model.setValue(value); 632 } 633 return this; 634 } 635 636 /** 637 * @return {@link #version} (The version of the device, if the device has 638 * multiple releases under the same model, or if the device is software 639 * or carries firmware.). This is the underlying object with id, value 640 * and extensions. The accessor "getVersion" gives direct access to the 641 * value 642 */ 643 public StringType getVersionElement() { 644 if (this.version == null) 645 if (Configuration.errorOnAutoCreate()) 646 throw new Error("Attempt to auto-create Device.version"); 647 else if (Configuration.doAutoCreate()) 648 this.version = new StringType(); // bb 649 return this.version; 650 } 651 652 public boolean hasVersionElement() { 653 return this.version != null && !this.version.isEmpty(); 654 } 655 656 public boolean hasVersion() { 657 return this.version != null && !this.version.isEmpty(); 658 } 659 660 /** 661 * @param value {@link #version} (The version of the device, if the device has 662 * multiple releases under the same model, or if the device is 663 * software or carries firmware.). This is the underlying object 664 * with id, value and extensions. The accessor "getVersion" gives 665 * direct access to the value 666 */ 667 public Device setVersionElement(StringType value) { 668 this.version = value; 669 return this; 670 } 671 672 /** 673 * @return The version of the device, if the device has multiple releases under 674 * the same model, or if the device is software or carries firmware. 675 */ 676 public String getVersion() { 677 return this.version == null ? null : this.version.getValue(); 678 } 679 680 /** 681 * @param value The version of the device, if the device has multiple releases 682 * under the same model, or if the device is software or carries 683 * firmware. 684 */ 685 public Device setVersion(String value) { 686 if (Utilities.noString(value)) 687 this.version = null; 688 else { 689 if (this.version == null) 690 this.version = new StringType(); 691 this.version.setValue(value); 692 } 693 return this; 694 } 695 696 /** 697 * @return {@link #manufactureDate} (The date and time when the device was 698 * manufactured.). This is the underlying object with id, value and 699 * extensions. The accessor "getManufactureDate" gives direct access to 700 * the value 701 */ 702 public DateTimeType getManufactureDateElement() { 703 if (this.manufactureDate == null) 704 if (Configuration.errorOnAutoCreate()) 705 throw new Error("Attempt to auto-create Device.manufactureDate"); 706 else if (Configuration.doAutoCreate()) 707 this.manufactureDate = new DateTimeType(); // bb 708 return this.manufactureDate; 709 } 710 711 public boolean hasManufactureDateElement() { 712 return this.manufactureDate != null && !this.manufactureDate.isEmpty(); 713 } 714 715 public boolean hasManufactureDate() { 716 return this.manufactureDate != null && !this.manufactureDate.isEmpty(); 717 } 718 719 /** 720 * @param value {@link #manufactureDate} (The date and time when the device was 721 * manufactured.). This is the underlying object with id, value and 722 * extensions. The accessor "getManufactureDate" gives direct 723 * access to the value 724 */ 725 public Device setManufactureDateElement(DateTimeType value) { 726 this.manufactureDate = value; 727 return this; 728 } 729 730 /** 731 * @return The date and time when the device was manufactured. 732 */ 733 public Date getManufactureDate() { 734 return this.manufactureDate == null ? null : this.manufactureDate.getValue(); 735 } 736 737 /** 738 * @param value The date and time when the device was manufactured. 739 */ 740 public Device setManufactureDate(Date value) { 741 if (value == null) 742 this.manufactureDate = null; 743 else { 744 if (this.manufactureDate == null) 745 this.manufactureDate = new DateTimeType(); 746 this.manufactureDate.setValue(value); 747 } 748 return this; 749 } 750 751 /** 752 * @return {@link #expiry} (The date and time beyond which this device is no 753 * longer valid or should not be used (if applicable).). This is the 754 * underlying object with id, value and extensions. The accessor 755 * "getExpiry" gives direct access to the value 756 */ 757 public DateTimeType getExpiryElement() { 758 if (this.expiry == null) 759 if (Configuration.errorOnAutoCreate()) 760 throw new Error("Attempt to auto-create Device.expiry"); 761 else if (Configuration.doAutoCreate()) 762 this.expiry = new DateTimeType(); // bb 763 return this.expiry; 764 } 765 766 public boolean hasExpiryElement() { 767 return this.expiry != null && !this.expiry.isEmpty(); 768 } 769 770 public boolean hasExpiry() { 771 return this.expiry != null && !this.expiry.isEmpty(); 772 } 773 774 /** 775 * @param value {@link #expiry} (The date and time beyond which this device is 776 * no longer valid or should not be used (if applicable).). This is 777 * the underlying object with id, value and extensions. The 778 * accessor "getExpiry" gives direct access to the value 779 */ 780 public Device setExpiryElement(DateTimeType value) { 781 this.expiry = value; 782 return this; 783 } 784 785 /** 786 * @return The date and time beyond which this device is no longer valid or 787 * should not be used (if applicable). 788 */ 789 public Date getExpiry() { 790 return this.expiry == null ? null : this.expiry.getValue(); 791 } 792 793 /** 794 * @param value The date and time beyond which this device is no longer valid or 795 * should not be used (if applicable). 796 */ 797 public Device setExpiry(Date value) { 798 if (value == null) 799 this.expiry = null; 800 else { 801 if (this.expiry == null) 802 this.expiry = new DateTimeType(); 803 this.expiry.setValue(value); 804 } 805 return this; 806 } 807 808 /** 809 * @return {@link #udi} (United States Food and Drug Administration mandated 810 * Unique Device Identifier (UDI). Use the human readable information 811 * (the content that the user sees, which is sometimes different to the 812 * exact syntax represented in the barcode) - see 813 * http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/default.htm.). 814 * This is the underlying object with id, value and extensions. The 815 * accessor "getUdi" gives direct access to the value 816 */ 817 public StringType getUdiElement() { 818 if (this.udi == null) 819 if (Configuration.errorOnAutoCreate()) 820 throw new Error("Attempt to auto-create Device.udi"); 821 else if (Configuration.doAutoCreate()) 822 this.udi = new StringType(); // bb 823 return this.udi; 824 } 825 826 public boolean hasUdiElement() { 827 return this.udi != null && !this.udi.isEmpty(); 828 } 829 830 public boolean hasUdi() { 831 return this.udi != null && !this.udi.isEmpty(); 832 } 833 834 /** 835 * @param value {@link #udi} (United States Food and Drug Administration 836 * mandated Unique Device Identifier (UDI). Use the human readable 837 * information (the content that the user sees, which is sometimes 838 * different to the exact syntax represented in the barcode) - see 839 * http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/default.htm.). 840 * This is the underlying object with id, value and extensions. The 841 * accessor "getUdi" gives direct access to the value 842 */ 843 public Device setUdiElement(StringType value) { 844 this.udi = value; 845 return this; 846 } 847 848 /** 849 * @return United States Food and Drug Administration mandated Unique Device 850 * Identifier (UDI). Use the human readable information (the content 851 * that the user sees, which is sometimes different to the exact syntax 852 * represented in the barcode) - see 853 * http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/default.htm. 854 */ 855 public String getUdi() { 856 return this.udi == null ? null : this.udi.getValue(); 857 } 858 859 /** 860 * @param value United States Food and Drug Administration mandated Unique 861 * Device Identifier (UDI). Use the human readable information (the 862 * content that the user sees, which is sometimes different to the 863 * exact syntax represented in the barcode) - see 864 * http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/default.htm. 865 */ 866 public Device setUdi(String value) { 867 if (Utilities.noString(value)) 868 this.udi = null; 869 else { 870 if (this.udi == null) 871 this.udi = new StringType(); 872 this.udi.setValue(value); 873 } 874 return this; 875 } 876 877 /** 878 * @return {@link #lotNumber} (Lot number assigned by the manufacturer.). This 879 * is the underlying object with id, value and extensions. The accessor 880 * "getLotNumber" gives direct access to the value 881 */ 882 public StringType getLotNumberElement() { 883 if (this.lotNumber == null) 884 if (Configuration.errorOnAutoCreate()) 885 throw new Error("Attempt to auto-create Device.lotNumber"); 886 else if (Configuration.doAutoCreate()) 887 this.lotNumber = new StringType(); // bb 888 return this.lotNumber; 889 } 890 891 public boolean hasLotNumberElement() { 892 return this.lotNumber != null && !this.lotNumber.isEmpty(); 893 } 894 895 public boolean hasLotNumber() { 896 return this.lotNumber != null && !this.lotNumber.isEmpty(); 897 } 898 899 /** 900 * @param value {@link #lotNumber} (Lot number assigned by the manufacturer.). 901 * This is the underlying object with id, value and extensions. The 902 * accessor "getLotNumber" gives direct access to the value 903 */ 904 public Device setLotNumberElement(StringType value) { 905 this.lotNumber = value; 906 return this; 907 } 908 909 /** 910 * @return Lot number assigned by the manufacturer. 911 */ 912 public String getLotNumber() { 913 return this.lotNumber == null ? null : this.lotNumber.getValue(); 914 } 915 916 /** 917 * @param value Lot number assigned by the manufacturer. 918 */ 919 public Device setLotNumber(String value) { 920 if (Utilities.noString(value)) 921 this.lotNumber = null; 922 else { 923 if (this.lotNumber == null) 924 this.lotNumber = new StringType(); 925 this.lotNumber.setValue(value); 926 } 927 return this; 928 } 929 930 /** 931 * @return {@link #owner} (An organization that is responsible for the provision 932 * and ongoing maintenance of the device.) 933 */ 934 public Reference getOwner() { 935 if (this.owner == null) 936 if (Configuration.errorOnAutoCreate()) 937 throw new Error("Attempt to auto-create Device.owner"); 938 else if (Configuration.doAutoCreate()) 939 this.owner = new Reference(); // cc 940 return this.owner; 941 } 942 943 public boolean hasOwner() { 944 return this.owner != null && !this.owner.isEmpty(); 945 } 946 947 /** 948 * @param value {@link #owner} (An organization that is responsible for the 949 * provision and ongoing maintenance of the device.) 950 */ 951 public Device setOwner(Reference value) { 952 this.owner = value; 953 return this; 954 } 955 956 /** 957 * @return {@link #owner} The actual object that is the target of the reference. 958 * The reference library doesn't populate this, but you can use it to 959 * hold the resource if you resolve it. (An organization that is 960 * responsible for the provision and ongoing maintenance of the device.) 961 */ 962 public Organization getOwnerTarget() { 963 if (this.ownerTarget == null) 964 if (Configuration.errorOnAutoCreate()) 965 throw new Error("Attempt to auto-create Device.owner"); 966 else if (Configuration.doAutoCreate()) 967 this.ownerTarget = new Organization(); // aa 968 return this.ownerTarget; 969 } 970 971 /** 972 * @param value {@link #owner} The actual object that is the target of the 973 * reference. The reference library doesn't use these, but you can 974 * use it to hold the resource if you resolve it. (An organization 975 * that is responsible for the provision and ongoing maintenance of 976 * the device.) 977 */ 978 public Device setOwnerTarget(Organization value) { 979 this.ownerTarget = value; 980 return this; 981 } 982 983 /** 984 * @return {@link #location} (The place where the device can be found.) 985 */ 986 public Reference getLocation() { 987 if (this.location == null) 988 if (Configuration.errorOnAutoCreate()) 989 throw new Error("Attempt to auto-create Device.location"); 990 else if (Configuration.doAutoCreate()) 991 this.location = new Reference(); // cc 992 return this.location; 993 } 994 995 public boolean hasLocation() { 996 return this.location != null && !this.location.isEmpty(); 997 } 998 999 /** 1000 * @param value {@link #location} (The place where the device can be found.) 1001 */ 1002 public Device setLocation(Reference value) { 1003 this.location = value; 1004 return this; 1005 } 1006 1007 /** 1008 * @return {@link #location} The actual object that is the target of the 1009 * reference. The reference library doesn't populate this, but you can 1010 * use it to hold the resource if you resolve it. (The place where the 1011 * device can be found.) 1012 */ 1013 public Location getLocationTarget() { 1014 if (this.locationTarget == null) 1015 if (Configuration.errorOnAutoCreate()) 1016 throw new Error("Attempt to auto-create Device.location"); 1017 else if (Configuration.doAutoCreate()) 1018 this.locationTarget = new Location(); // aa 1019 return this.locationTarget; 1020 } 1021 1022 /** 1023 * @param value {@link #location} The actual object that is the target of the 1024 * reference. The reference library doesn't use these, but you can 1025 * use it to hold the resource if you resolve it. (The place where 1026 * the device can be found.) 1027 */ 1028 public Device setLocationTarget(Location value) { 1029 this.locationTarget = value; 1030 return this; 1031 } 1032 1033 /** 1034 * @return {@link #patient} (Patient information, if the resource is affixed to 1035 * a person.) 1036 */ 1037 public Reference getPatient() { 1038 if (this.patient == null) 1039 if (Configuration.errorOnAutoCreate()) 1040 throw new Error("Attempt to auto-create Device.patient"); 1041 else if (Configuration.doAutoCreate()) 1042 this.patient = new Reference(); // cc 1043 return this.patient; 1044 } 1045 1046 public boolean hasPatient() { 1047 return this.patient != null && !this.patient.isEmpty(); 1048 } 1049 1050 /** 1051 * @param value {@link #patient} (Patient information, if the resource is 1052 * affixed to a person.) 1053 */ 1054 public Device setPatient(Reference value) { 1055 this.patient = value; 1056 return this; 1057 } 1058 1059 /** 1060 * @return {@link #patient} The actual object that is the target of the 1061 * reference. The reference library doesn't populate this, but you can 1062 * use it to hold the resource if you resolve it. (Patient information, 1063 * if the resource is affixed to a person.) 1064 */ 1065 public Patient getPatientTarget() { 1066 if (this.patientTarget == null) 1067 if (Configuration.errorOnAutoCreate()) 1068 throw new Error("Attempt to auto-create Device.patient"); 1069 else if (Configuration.doAutoCreate()) 1070 this.patientTarget = new Patient(); // aa 1071 return this.patientTarget; 1072 } 1073 1074 /** 1075 * @param value {@link #patient} The actual object that is the target of the 1076 * reference. The reference library doesn't use these, but you can 1077 * use it to hold the resource if you resolve it. (Patient 1078 * information, if the resource is affixed to a person.) 1079 */ 1080 public Device setPatientTarget(Patient value) { 1081 this.patientTarget = value; 1082 return this; 1083 } 1084 1085 /** 1086 * @return {@link #contact} (Contact details for an organization or a particular 1087 * human that is responsible for the device.) 1088 */ 1089 public List<ContactPoint> getContact() { 1090 if (this.contact == null) 1091 this.contact = new ArrayList<ContactPoint>(); 1092 return this.contact; 1093 } 1094 1095 public boolean hasContact() { 1096 if (this.contact == null) 1097 return false; 1098 for (ContactPoint item : this.contact) 1099 if (!item.isEmpty()) 1100 return true; 1101 return false; 1102 } 1103 1104 /** 1105 * @return {@link #contact} (Contact details for an organization or a particular 1106 * human that is responsible for the device.) 1107 */ 1108 // syntactic sugar 1109 public ContactPoint addContact() { // 3 1110 ContactPoint t = new ContactPoint(); 1111 if (this.contact == null) 1112 this.contact = new ArrayList<ContactPoint>(); 1113 this.contact.add(t); 1114 return t; 1115 } 1116 1117 // syntactic sugar 1118 public Device addContact(ContactPoint t) { // 3 1119 if (t == null) 1120 return this; 1121 if (this.contact == null) 1122 this.contact = new ArrayList<ContactPoint>(); 1123 this.contact.add(t); 1124 return this; 1125 } 1126 1127 /** 1128 * @return {@link #url} (A network address on which the device may be contacted 1129 * directly.). This is the underlying object with id, value and 1130 * extensions. The accessor "getUrl" gives direct access to the value 1131 */ 1132 public UriType getUrlElement() { 1133 if (this.url == null) 1134 if (Configuration.errorOnAutoCreate()) 1135 throw new Error("Attempt to auto-create Device.url"); 1136 else if (Configuration.doAutoCreate()) 1137 this.url = new UriType(); // bb 1138 return this.url; 1139 } 1140 1141 public boolean hasUrlElement() { 1142 return this.url != null && !this.url.isEmpty(); 1143 } 1144 1145 public boolean hasUrl() { 1146 return this.url != null && !this.url.isEmpty(); 1147 } 1148 1149 /** 1150 * @param value {@link #url} (A network address on which the device may be 1151 * contacted directly.). This is the underlying object with id, 1152 * value and extensions. The accessor "getUrl" gives direct access 1153 * to the value 1154 */ 1155 public Device setUrlElement(UriType value) { 1156 this.url = value; 1157 return this; 1158 } 1159 1160 /** 1161 * @return A network address on which the device may be contacted directly. 1162 */ 1163 public String getUrl() { 1164 return this.url == null ? null : this.url.getValue(); 1165 } 1166 1167 /** 1168 * @param value A network address on which the device may be contacted directly. 1169 */ 1170 public Device setUrl(String value) { 1171 if (Utilities.noString(value)) 1172 this.url = null; 1173 else { 1174 if (this.url == null) 1175 this.url = new UriType(); 1176 this.url.setValue(value); 1177 } 1178 return this; 1179 } 1180 1181 protected void listChildren(List<Property> childrenList) { 1182 super.listChildren(childrenList); 1183 childrenList.add(new Property("identifier", "Identifier", 1184 "Unique instance identifiers assigned to a device by organizations like manufacturers or owners. If the identifier identifies the type of device, Device.type should be used.", 1185 0, java.lang.Integer.MAX_VALUE, identifier)); 1186 childrenList.add(new Property("type", "CodeableConcept", "Code or identifier to identify a kind of device.", 0, 1187 java.lang.Integer.MAX_VALUE, type)); 1188 childrenList.add(new Property("note", "Annotation", 1189 "Descriptive information, usage information or implantation information that is not captured in an existing element.", 1190 0, java.lang.Integer.MAX_VALUE, note)); 1191 childrenList.add( 1192 new Property("status", "code", "Status of the Device availability.", 0, java.lang.Integer.MAX_VALUE, status)); 1193 childrenList.add(new Property("manufacturer", "string", "A name of the manufacturer.", 0, 1194 java.lang.Integer.MAX_VALUE, manufacturer)); 1195 childrenList.add(new Property("model", "string", 1196 "The \"model\" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type.", 1197 0, java.lang.Integer.MAX_VALUE, model)); 1198 childrenList.add(new Property("version", "string", 1199 "The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware.", 1200 0, java.lang.Integer.MAX_VALUE, version)); 1201 childrenList.add(new Property("manufactureDate", "dateTime", "The date and time when the device was manufactured.", 1202 0, java.lang.Integer.MAX_VALUE, manufactureDate)); 1203 childrenList.add(new Property("expiry", "dateTime", 1204 "The date and time beyond which this device is no longer valid or should not be used (if applicable).", 0, 1205 java.lang.Integer.MAX_VALUE, expiry)); 1206 childrenList.add(new Property("udi", "string", 1207 "United States Food and Drug Administration mandated Unique Device Identifier (UDI). Use the human readable information (the content that the user sees, which is sometimes different to the exact syntax represented in the barcode) - see http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/default.htm.", 1208 0, java.lang.Integer.MAX_VALUE, udi)); 1209 childrenList.add(new Property("lotNumber", "string", "Lot number assigned by the manufacturer.", 0, 1210 java.lang.Integer.MAX_VALUE, lotNumber)); 1211 childrenList.add(new Property("owner", "Reference(Organization)", 1212 "An organization that is responsible for the provision and ongoing maintenance of the device.", 0, 1213 java.lang.Integer.MAX_VALUE, owner)); 1214 childrenList.add(new Property("location", "Reference(Location)", "The place where the device can be found.", 0, 1215 java.lang.Integer.MAX_VALUE, location)); 1216 childrenList.add(new Property("patient", "Reference(Patient)", 1217 "Patient information, if the resource is affixed to a person.", 0, java.lang.Integer.MAX_VALUE, patient)); 1218 childrenList.add(new Property("contact", "ContactPoint", 1219 "Contact details for an organization or a particular human that is responsible for the device.", 0, 1220 java.lang.Integer.MAX_VALUE, contact)); 1221 childrenList.add(new Property("url", "uri", "A network address on which the device may be contacted directly.", 0, 1222 java.lang.Integer.MAX_VALUE, url)); 1223 } 1224 1225 @Override 1226 public void setProperty(String name, Base value) throws FHIRException { 1227 if (name.equals("identifier")) 1228 this.getIdentifier().add(castToIdentifier(value)); 1229 else if (name.equals("type")) 1230 this.type = castToCodeableConcept(value); // CodeableConcept 1231 else if (name.equals("note")) 1232 this.getNote().add(castToAnnotation(value)); 1233 else if (name.equals("status")) 1234 this.status = new DeviceStatusEnumFactory().fromType(value); // Enumeration<DeviceStatus> 1235 else if (name.equals("manufacturer")) 1236 this.manufacturer = castToString(value); // StringType 1237 else if (name.equals("model")) 1238 this.model = castToString(value); // StringType 1239 else if (name.equals("version")) 1240 this.version = castToString(value); // StringType 1241 else if (name.equals("manufactureDate")) 1242 this.manufactureDate = castToDateTime(value); // DateTimeType 1243 else if (name.equals("expiry")) 1244 this.expiry = castToDateTime(value); // DateTimeType 1245 else if (name.equals("udi")) 1246 this.udi = castToString(value); // StringType 1247 else if (name.equals("lotNumber")) 1248 this.lotNumber = castToString(value); // StringType 1249 else if (name.equals("owner")) 1250 this.owner = castToReference(value); // Reference 1251 else if (name.equals("location")) 1252 this.location = castToReference(value); // Reference 1253 else if (name.equals("patient")) 1254 this.patient = castToReference(value); // Reference 1255 else if (name.equals("contact")) 1256 this.getContact().add(castToContactPoint(value)); 1257 else if (name.equals("url")) 1258 this.url = castToUri(value); // UriType 1259 else 1260 super.setProperty(name, value); 1261 } 1262 1263 @Override 1264 public Base addChild(String name) throws FHIRException { 1265 if (name.equals("identifier")) { 1266 return addIdentifier(); 1267 } else if (name.equals("type")) { 1268 this.type = new CodeableConcept(); 1269 return this.type; 1270 } else if (name.equals("note")) { 1271 return addNote(); 1272 } else if (name.equals("status")) { 1273 throw new FHIRException("Cannot call addChild on a singleton property Device.status"); 1274 } else if (name.equals("manufacturer")) { 1275 throw new FHIRException("Cannot call addChild on a singleton property Device.manufacturer"); 1276 } else if (name.equals("model")) { 1277 throw new FHIRException("Cannot call addChild on a singleton property Device.model"); 1278 } else if (name.equals("version")) { 1279 throw new FHIRException("Cannot call addChild on a singleton property Device.version"); 1280 } else if (name.equals("manufactureDate")) { 1281 throw new FHIRException("Cannot call addChild on a singleton property Device.manufactureDate"); 1282 } else if (name.equals("expiry")) { 1283 throw new FHIRException("Cannot call addChild on a singleton property Device.expiry"); 1284 } else if (name.equals("udi")) { 1285 throw new FHIRException("Cannot call addChild on a singleton property Device.udi"); 1286 } else if (name.equals("lotNumber")) { 1287 throw new FHIRException("Cannot call addChild on a singleton property Device.lotNumber"); 1288 } else if (name.equals("owner")) { 1289 this.owner = new Reference(); 1290 return this.owner; 1291 } else if (name.equals("location")) { 1292 this.location = new Reference(); 1293 return this.location; 1294 } else if (name.equals("patient")) { 1295 this.patient = new Reference(); 1296 return this.patient; 1297 } else if (name.equals("contact")) { 1298 return addContact(); 1299 } else if (name.equals("url")) { 1300 throw new FHIRException("Cannot call addChild on a singleton property Device.url"); 1301 } else 1302 return super.addChild(name); 1303 } 1304 1305 public String fhirType() { 1306 return "Device"; 1307 1308 } 1309 1310 public Device copy() { 1311 Device dst = new Device(); 1312 copyValues(dst); 1313 if (identifier != null) { 1314 dst.identifier = new ArrayList<Identifier>(); 1315 for (Identifier i : identifier) 1316 dst.identifier.add(i.copy()); 1317 } 1318 ; 1319 dst.type = type == null ? null : type.copy(); 1320 if (note != null) { 1321 dst.note = new ArrayList<Annotation>(); 1322 for (Annotation i : note) 1323 dst.note.add(i.copy()); 1324 } 1325 ; 1326 dst.status = status == null ? null : status.copy(); 1327 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 1328 dst.model = model == null ? null : model.copy(); 1329 dst.version = version == null ? null : version.copy(); 1330 dst.manufactureDate = manufactureDate == null ? null : manufactureDate.copy(); 1331 dst.expiry = expiry == null ? null : expiry.copy(); 1332 dst.udi = udi == null ? null : udi.copy(); 1333 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 1334 dst.owner = owner == null ? null : owner.copy(); 1335 dst.location = location == null ? null : location.copy(); 1336 dst.patient = patient == null ? null : patient.copy(); 1337 if (contact != null) { 1338 dst.contact = new ArrayList<ContactPoint>(); 1339 for (ContactPoint i : contact) 1340 dst.contact.add(i.copy()); 1341 } 1342 ; 1343 dst.url = url == null ? null : url.copy(); 1344 return dst; 1345 } 1346 1347 protected Device typedCopy() { 1348 return copy(); 1349 } 1350 1351 @Override 1352 public boolean equalsDeep(Base other) { 1353 if (!super.equalsDeep(other)) 1354 return false; 1355 if (!(other instanceof Device)) 1356 return false; 1357 Device o = (Device) other; 1358 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 1359 && compareDeep(note, o.note, true) && compareDeep(status, o.status, true) 1360 && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(model, o.model, true) 1361 && compareDeep(version, o.version, true) && compareDeep(manufactureDate, o.manufactureDate, true) 1362 && compareDeep(expiry, o.expiry, true) && compareDeep(udi, o.udi, true) 1363 && compareDeep(lotNumber, o.lotNumber, true) && compareDeep(owner, o.owner, true) 1364 && compareDeep(location, o.location, true) && compareDeep(patient, o.patient, true) 1365 && compareDeep(contact, o.contact, true) && compareDeep(url, o.url, true); 1366 } 1367 1368 @Override 1369 public boolean equalsShallow(Base other) { 1370 if (!super.equalsShallow(other)) 1371 return false; 1372 if (!(other instanceof Device)) 1373 return false; 1374 Device o = (Device) other; 1375 return compareValues(status, o.status, true) && compareValues(manufacturer, o.manufacturer, true) 1376 && compareValues(model, o.model, true) && compareValues(version, o.version, true) 1377 && compareValues(manufactureDate, o.manufactureDate, true) && compareValues(expiry, o.expiry, true) 1378 && compareValues(udi, o.udi, true) && compareValues(lotNumber, o.lotNumber, true) 1379 && compareValues(url, o.url, true); 1380 } 1381 1382 public boolean isEmpty() { 1383 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (type == null || type.isEmpty()) 1384 && (note == null || note.isEmpty()) && (status == null || status.isEmpty()) 1385 && (manufacturer == null || manufacturer.isEmpty()) && (model == null || model.isEmpty()) 1386 && (version == null || version.isEmpty()) && (manufactureDate == null || manufactureDate.isEmpty()) 1387 && (expiry == null || expiry.isEmpty()) && (udi == null || udi.isEmpty()) 1388 && (lotNumber == null || lotNumber.isEmpty()) && (owner == null || owner.isEmpty()) 1389 && (location == null || location.isEmpty()) && (patient == null || patient.isEmpty()) 1390 && (contact == null || contact.isEmpty()) && (url == null || url.isEmpty()); 1391 } 1392 1393 @Override 1394 public ResourceType getResourceType() { 1395 return ResourceType.Device; 1396 } 1397 1398 @SearchParamDefinition(name = "identifier", path = "Device.identifier", description = "Instance id from manufacturer, owner, and others", type = "token") 1399 public static final String SP_IDENTIFIER = "identifier"; 1400 @SearchParamDefinition(name = "patient", path = "Device.patient", description = "Patient information, if the resource is affixed to a person", type = "reference") 1401 public static final String SP_PATIENT = "patient"; 1402 @SearchParamDefinition(name = "organization", path = "Device.owner", description = "The organization responsible for the device", type = "reference") 1403 public static final String SP_ORGANIZATION = "organization"; 1404 @SearchParamDefinition(name = "model", path = "Device.model", description = "The model of the device", type = "string") 1405 public static final String SP_MODEL = "model"; 1406 @SearchParamDefinition(name = "location", path = "Device.location", description = "A location, where the resource is found", type = "reference") 1407 public static final String SP_LOCATION = "location"; 1408 @SearchParamDefinition(name = "udi", path = "Device.udi", description = "FDA mandated Unique Device Identifier", type = "string") 1409 public static final String SP_UDI = "udi"; 1410 @SearchParamDefinition(name = "type", path = "Device.type", description = "The type of the device", type = "token") 1411 public static final String SP_TYPE = "type"; 1412 @SearchParamDefinition(name = "url", path = "Device.url", description = "Network address to contact device", type = "uri") 1413 public static final String SP_URL = "url"; 1414 @SearchParamDefinition(name = "manufacturer", path = "Device.manufacturer", description = "The manufacturer of the device", type = "string") 1415 public static final String SP_MANUFACTURER = "manufacturer"; 1416 1417}