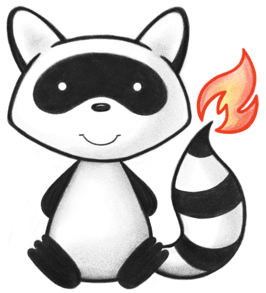
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * Describes the characteristics, operational status and capabilities of a 048 * medical-related component of a medical device. 049 */ 050@ResourceDef(name = "DeviceComponent", profile = "http://hl7.org/fhir/Profile/DeviceComponent") 051public class DeviceComponent extends DomainResource { 052 053 public enum MeasmntPrinciple { 054 /** 055 * Measurement principle isn't in the list. 056 */ 057 OTHER, 058 /** 059 * Measurement is done using the chemical principle. 060 */ 061 CHEMICAL, 062 /** 063 * Measurement is done using the electrical principle. 064 */ 065 ELECTRICAL, 066 /** 067 * Measurement is done using the impedance principle. 068 */ 069 IMPEDANCE, 070 /** 071 * Measurement is done using the nuclear principle. 072 */ 073 NUCLEAR, 074 /** 075 * Measurement is done using the optical principle. 076 */ 077 OPTICAL, 078 /** 079 * Measurement is done using the thermal principle. 080 */ 081 THERMAL, 082 /** 083 * Measurement is done using the biological principle. 084 */ 085 BIOLOGICAL, 086 /** 087 * Measurement is done using the mechanical principle. 088 */ 089 MECHANICAL, 090 /** 091 * Measurement is done using the acoustical principle. 092 */ 093 ACOUSTICAL, 094 /** 095 * Measurement is done using the manual principle. 096 */ 097 MANUAL, 098 /** 099 * added to help the parsers 100 */ 101 NULL; 102 103 public static MeasmntPrinciple fromCode(String codeString) throws FHIRException { 104 if (codeString == null || "".equals(codeString)) 105 return null; 106 if ("other".equals(codeString)) 107 return OTHER; 108 if ("chemical".equals(codeString)) 109 return CHEMICAL; 110 if ("electrical".equals(codeString)) 111 return ELECTRICAL; 112 if ("impedance".equals(codeString)) 113 return IMPEDANCE; 114 if ("nuclear".equals(codeString)) 115 return NUCLEAR; 116 if ("optical".equals(codeString)) 117 return OPTICAL; 118 if ("thermal".equals(codeString)) 119 return THERMAL; 120 if ("biological".equals(codeString)) 121 return BIOLOGICAL; 122 if ("mechanical".equals(codeString)) 123 return MECHANICAL; 124 if ("acoustical".equals(codeString)) 125 return ACOUSTICAL; 126 if ("manual".equals(codeString)) 127 return MANUAL; 128 throw new FHIRException("Unknown MeasmntPrinciple code '" + codeString + "'"); 129 } 130 131 public String toCode() { 132 switch (this) { 133 case OTHER: 134 return "other"; 135 case CHEMICAL: 136 return "chemical"; 137 case ELECTRICAL: 138 return "electrical"; 139 case IMPEDANCE: 140 return "impedance"; 141 case NUCLEAR: 142 return "nuclear"; 143 case OPTICAL: 144 return "optical"; 145 case THERMAL: 146 return "thermal"; 147 case BIOLOGICAL: 148 return "biological"; 149 case MECHANICAL: 150 return "mechanical"; 151 case ACOUSTICAL: 152 return "acoustical"; 153 case MANUAL: 154 return "manual"; 155 case NULL: 156 return null; 157 default: 158 return "?"; 159 } 160 } 161 162 public String getSystem() { 163 switch (this) { 164 case OTHER: 165 return "http://hl7.org/fhir/measurement-principle"; 166 case CHEMICAL: 167 return "http://hl7.org/fhir/measurement-principle"; 168 case ELECTRICAL: 169 return "http://hl7.org/fhir/measurement-principle"; 170 case IMPEDANCE: 171 return "http://hl7.org/fhir/measurement-principle"; 172 case NUCLEAR: 173 return "http://hl7.org/fhir/measurement-principle"; 174 case OPTICAL: 175 return "http://hl7.org/fhir/measurement-principle"; 176 case THERMAL: 177 return "http://hl7.org/fhir/measurement-principle"; 178 case BIOLOGICAL: 179 return "http://hl7.org/fhir/measurement-principle"; 180 case MECHANICAL: 181 return "http://hl7.org/fhir/measurement-principle"; 182 case ACOUSTICAL: 183 return "http://hl7.org/fhir/measurement-principle"; 184 case MANUAL: 185 return "http://hl7.org/fhir/measurement-principle"; 186 case NULL: 187 return null; 188 default: 189 return "?"; 190 } 191 } 192 193 public String getDefinition() { 194 switch (this) { 195 case OTHER: 196 return "Measurement principle isn't in the list."; 197 case CHEMICAL: 198 return "Measurement is done using the chemical principle."; 199 case ELECTRICAL: 200 return "Measurement is done using the electrical principle."; 201 case IMPEDANCE: 202 return "Measurement is done using the impedance principle."; 203 case NUCLEAR: 204 return "Measurement is done using the nuclear principle."; 205 case OPTICAL: 206 return "Measurement is done using the optical principle."; 207 case THERMAL: 208 return "Measurement is done using the thermal principle."; 209 case BIOLOGICAL: 210 return "Measurement is done using the biological principle."; 211 case MECHANICAL: 212 return "Measurement is done using the mechanical principle."; 213 case ACOUSTICAL: 214 return "Measurement is done using the acoustical principle."; 215 case MANUAL: 216 return "Measurement is done using the manual principle."; 217 case NULL: 218 return null; 219 default: 220 return "?"; 221 } 222 } 223 224 public String getDisplay() { 225 switch (this) { 226 case OTHER: 227 return "MSP Other"; 228 case CHEMICAL: 229 return "MSP Chemical"; 230 case ELECTRICAL: 231 return "MSP Electrical"; 232 case IMPEDANCE: 233 return "MSP Impedance"; 234 case NUCLEAR: 235 return "MSP Nuclear"; 236 case OPTICAL: 237 return "MSP Optical"; 238 case THERMAL: 239 return "MSP Thermal"; 240 case BIOLOGICAL: 241 return "MSP Biological"; 242 case MECHANICAL: 243 return "MSP Mechanical"; 244 case ACOUSTICAL: 245 return "MSP Acoustical"; 246 case MANUAL: 247 return "MSP Manual"; 248 case NULL: 249 return null; 250 default: 251 return "?"; 252 } 253 } 254 } 255 256 public static class MeasmntPrincipleEnumFactory implements EnumFactory<MeasmntPrinciple> { 257 public MeasmntPrinciple fromCode(String codeString) throws IllegalArgumentException { 258 if (codeString == null || "".equals(codeString)) 259 if (codeString == null || "".equals(codeString)) 260 return null; 261 if ("other".equals(codeString)) 262 return MeasmntPrinciple.OTHER; 263 if ("chemical".equals(codeString)) 264 return MeasmntPrinciple.CHEMICAL; 265 if ("electrical".equals(codeString)) 266 return MeasmntPrinciple.ELECTRICAL; 267 if ("impedance".equals(codeString)) 268 return MeasmntPrinciple.IMPEDANCE; 269 if ("nuclear".equals(codeString)) 270 return MeasmntPrinciple.NUCLEAR; 271 if ("optical".equals(codeString)) 272 return MeasmntPrinciple.OPTICAL; 273 if ("thermal".equals(codeString)) 274 return MeasmntPrinciple.THERMAL; 275 if ("biological".equals(codeString)) 276 return MeasmntPrinciple.BIOLOGICAL; 277 if ("mechanical".equals(codeString)) 278 return MeasmntPrinciple.MECHANICAL; 279 if ("acoustical".equals(codeString)) 280 return MeasmntPrinciple.ACOUSTICAL; 281 if ("manual".equals(codeString)) 282 return MeasmntPrinciple.MANUAL; 283 throw new IllegalArgumentException("Unknown MeasmntPrinciple code '" + codeString + "'"); 284 } 285 286 public Enumeration<MeasmntPrinciple> fromType(Base code) throws FHIRException { 287 if (code == null || code.isEmpty()) 288 return null; 289 String codeString = ((PrimitiveType) code).asStringValue(); 290 if (codeString == null || "".equals(codeString)) 291 return null; 292 if ("other".equals(codeString)) 293 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.OTHER); 294 if ("chemical".equals(codeString)) 295 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.CHEMICAL); 296 if ("electrical".equals(codeString)) 297 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.ELECTRICAL); 298 if ("impedance".equals(codeString)) 299 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.IMPEDANCE); 300 if ("nuclear".equals(codeString)) 301 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.NUCLEAR); 302 if ("optical".equals(codeString)) 303 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.OPTICAL); 304 if ("thermal".equals(codeString)) 305 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.THERMAL); 306 if ("biological".equals(codeString)) 307 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.BIOLOGICAL); 308 if ("mechanical".equals(codeString)) 309 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.MECHANICAL); 310 if ("acoustical".equals(codeString)) 311 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.ACOUSTICAL); 312 if ("manual".equals(codeString)) 313 return new Enumeration<MeasmntPrinciple>(this, MeasmntPrinciple.MANUAL); 314 throw new FHIRException("Unknown MeasmntPrinciple code '" + codeString + "'"); 315 } 316 317 public String toCode(MeasmntPrinciple code) 318 { 319 if (code == MeasmntPrinciple.NULL) 320 return null; 321 if (code == MeasmntPrinciple.OTHER) 322 return "other"; 323 if (code == MeasmntPrinciple.CHEMICAL) 324 return "chemical"; 325 if (code == MeasmntPrinciple.ELECTRICAL) 326 return "electrical"; 327 if (code == MeasmntPrinciple.IMPEDANCE) 328 return "impedance"; 329 if (code == MeasmntPrinciple.NUCLEAR) 330 return "nuclear"; 331 if (code == MeasmntPrinciple.OPTICAL) 332 return "optical"; 333 if (code == MeasmntPrinciple.THERMAL) 334 return "thermal"; 335 if (code == MeasmntPrinciple.BIOLOGICAL) 336 return "biological"; 337 if (code == MeasmntPrinciple.MECHANICAL) 338 return "mechanical"; 339 if (code == MeasmntPrinciple.ACOUSTICAL) 340 return "acoustical"; 341 if (code == MeasmntPrinciple.MANUAL) 342 return "manual"; 343 return "?"; 344 } 345 } 346 347 @Block() 348 public static class DeviceComponentProductionSpecificationComponent extends BackboneElement 349 implements IBaseBackboneElement { 350 /** 351 * Describes the specification type, such as, serial number, part number, 352 * hardware revision, software revision, etc. 353 */ 354 @Child(name = "specType", type = { 355 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 356 @Description(shortDefinition = "Specification type", formalDefinition = "Describes the specification type, such as, serial number, part number, hardware revision, software revision, etc.") 357 protected CodeableConcept specType; 358 359 /** 360 * Describes the internal component unique identification. This is a provision 361 * for manufacture specific standard components using a private OID. 11073-10101 362 * has a partition for private OID semantic that the manufacture can make use 363 * of. 364 */ 365 @Child(name = "componentId", type = { 366 Identifier.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 367 @Description(shortDefinition = "Internal component unique identification", formalDefinition = "Describes the internal component unique identification. This is a provision for manufacture specific standard components using a private OID. 11073-10101 has a partition for private OID semantic that the manufacture can make use of.") 368 protected Identifier componentId; 369 370 /** 371 * Describes the printable string defining the component. 372 */ 373 @Child(name = "productionSpec", type = { 374 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 375 @Description(shortDefinition = "A printable string defining the component", formalDefinition = "Describes the printable string defining the component.") 376 protected StringType productionSpec; 377 378 private static final long serialVersionUID = -1476597516L; 379 380 /* 381 * Constructor 382 */ 383 public DeviceComponentProductionSpecificationComponent() { 384 super(); 385 } 386 387 /** 388 * @return {@link #specType} (Describes the specification type, such as, serial 389 * number, part number, hardware revision, software revision, etc.) 390 */ 391 public CodeableConcept getSpecType() { 392 if (this.specType == null) 393 if (Configuration.errorOnAutoCreate()) 394 throw new Error("Attempt to auto-create DeviceComponentProductionSpecificationComponent.specType"); 395 else if (Configuration.doAutoCreate()) 396 this.specType = new CodeableConcept(); // cc 397 return this.specType; 398 } 399 400 public boolean hasSpecType() { 401 return this.specType != null && !this.specType.isEmpty(); 402 } 403 404 /** 405 * @param value {@link #specType} (Describes the specification type, such as, 406 * serial number, part number, hardware revision, software 407 * revision, etc.) 408 */ 409 public DeviceComponentProductionSpecificationComponent setSpecType(CodeableConcept value) { 410 this.specType = value; 411 return this; 412 } 413 414 /** 415 * @return {@link #componentId} (Describes the internal component unique 416 * identification. This is a provision for manufacture specific standard 417 * components using a private OID. 11073-10101 has a partition for 418 * private OID semantic that the manufacture can make use of.) 419 */ 420 public Identifier getComponentId() { 421 if (this.componentId == null) 422 if (Configuration.errorOnAutoCreate()) 423 throw new Error("Attempt to auto-create DeviceComponentProductionSpecificationComponent.componentId"); 424 else if (Configuration.doAutoCreate()) 425 this.componentId = new Identifier(); // cc 426 return this.componentId; 427 } 428 429 public boolean hasComponentId() { 430 return this.componentId != null && !this.componentId.isEmpty(); 431 } 432 433 /** 434 * @param value {@link #componentId} (Describes the internal component unique 435 * identification. This is a provision for manufacture specific 436 * standard components using a private OID. 11073-10101 has a 437 * partition for private OID semantic that the manufacture can make 438 * use of.) 439 */ 440 public DeviceComponentProductionSpecificationComponent setComponentId(Identifier value) { 441 this.componentId = value; 442 return this; 443 } 444 445 /** 446 * @return {@link #productionSpec} (Describes the printable string defining the 447 * component.). This is the underlying object with id, value and 448 * extensions. The accessor "getProductionSpec" gives direct access to 449 * the value 450 */ 451 public StringType getProductionSpecElement() { 452 if (this.productionSpec == null) 453 if (Configuration.errorOnAutoCreate()) 454 throw new Error("Attempt to auto-create DeviceComponentProductionSpecificationComponent.productionSpec"); 455 else if (Configuration.doAutoCreate()) 456 this.productionSpec = new StringType(); // bb 457 return this.productionSpec; 458 } 459 460 public boolean hasProductionSpecElement() { 461 return this.productionSpec != null && !this.productionSpec.isEmpty(); 462 } 463 464 public boolean hasProductionSpec() { 465 return this.productionSpec != null && !this.productionSpec.isEmpty(); 466 } 467 468 /** 469 * @param value {@link #productionSpec} (Describes the printable string defining 470 * the component.). This is the underlying object with id, value 471 * and extensions. The accessor "getProductionSpec" gives direct 472 * access to the value 473 */ 474 public DeviceComponentProductionSpecificationComponent setProductionSpecElement(StringType value) { 475 this.productionSpec = value; 476 return this; 477 } 478 479 /** 480 * @return Describes the printable string defining the component. 481 */ 482 public String getProductionSpec() { 483 return this.productionSpec == null ? null : this.productionSpec.getValue(); 484 } 485 486 /** 487 * @param value Describes the printable string defining the component. 488 */ 489 public DeviceComponentProductionSpecificationComponent setProductionSpec(String value) { 490 if (Utilities.noString(value)) 491 this.productionSpec = null; 492 else { 493 if (this.productionSpec == null) 494 this.productionSpec = new StringType(); 495 this.productionSpec.setValue(value); 496 } 497 return this; 498 } 499 500 protected void listChildren(List<Property> childrenList) { 501 super.listChildren(childrenList); 502 childrenList.add(new Property("specType", "CodeableConcept", 503 "Describes the specification type, such as, serial number, part number, hardware revision, software revision, etc.", 504 0, java.lang.Integer.MAX_VALUE, specType)); 505 childrenList.add(new Property("componentId", "Identifier", 506 "Describes the internal component unique identification. This is a provision for manufacture specific standard components using a private OID. 11073-10101 has a partition for private OID semantic that the manufacture can make use of.", 507 0, java.lang.Integer.MAX_VALUE, componentId)); 508 childrenList.add(new Property("productionSpec", "string", 509 "Describes the printable string defining the component.", 0, java.lang.Integer.MAX_VALUE, productionSpec)); 510 } 511 512 @Override 513 public void setProperty(String name, Base value) throws FHIRException { 514 if (name.equals("specType")) 515 this.specType = castToCodeableConcept(value); // CodeableConcept 516 else if (name.equals("componentId")) 517 this.componentId = castToIdentifier(value); // Identifier 518 else if (name.equals("productionSpec")) 519 this.productionSpec = castToString(value); // StringType 520 else 521 super.setProperty(name, value); 522 } 523 524 @Override 525 public Base addChild(String name) throws FHIRException { 526 if (name.equals("specType")) { 527 this.specType = new CodeableConcept(); 528 return this.specType; 529 } else if (name.equals("componentId")) { 530 this.componentId = new Identifier(); 531 return this.componentId; 532 } else if (name.equals("productionSpec")) { 533 throw new FHIRException("Cannot call addChild on a singleton property DeviceComponent.productionSpec"); 534 } else 535 return super.addChild(name); 536 } 537 538 public DeviceComponentProductionSpecificationComponent copy() { 539 DeviceComponentProductionSpecificationComponent dst = new DeviceComponentProductionSpecificationComponent(); 540 copyValues(dst); 541 dst.specType = specType == null ? null : specType.copy(); 542 dst.componentId = componentId == null ? null : componentId.copy(); 543 dst.productionSpec = productionSpec == null ? null : productionSpec.copy(); 544 return dst; 545 } 546 547 @Override 548 public boolean equalsDeep(Base other) { 549 if (!super.equalsDeep(other)) 550 return false; 551 if (!(other instanceof DeviceComponentProductionSpecificationComponent)) 552 return false; 553 DeviceComponentProductionSpecificationComponent o = (DeviceComponentProductionSpecificationComponent) other; 554 return compareDeep(specType, o.specType, true) && compareDeep(componentId, o.componentId, true) 555 && compareDeep(productionSpec, o.productionSpec, true); 556 } 557 558 @Override 559 public boolean equalsShallow(Base other) { 560 if (!super.equalsShallow(other)) 561 return false; 562 if (!(other instanceof DeviceComponentProductionSpecificationComponent)) 563 return false; 564 DeviceComponentProductionSpecificationComponent o = (DeviceComponentProductionSpecificationComponent) other; 565 return compareValues(productionSpec, o.productionSpec, true); 566 } 567 568 public boolean isEmpty() { 569 return super.isEmpty() && (specType == null || specType.isEmpty()) 570 && (componentId == null || componentId.isEmpty()) && (productionSpec == null || productionSpec.isEmpty()); 571 } 572 573 public String fhirType() { 574 return "DeviceComponent.productionSpecification"; 575 576 } 577 578 } 579 580 /** 581 * Describes the specific component type as defined in the object-oriented or 582 * metric nomenclature partition. 583 */ 584 @Child(name = "type", type = { CodeableConcept.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 585 @Description(shortDefinition = "What kind of component it is", formalDefinition = "Describes the specific component type as defined in the object-oriented or metric nomenclature partition.") 586 protected CodeableConcept type; 587 588 /** 589 * Describes the local assigned unique identification by the software. For 590 * example: handle ID. 591 */ 592 @Child(name = "identifier", type = { 593 Identifier.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 594 @Description(shortDefinition = "Instance id assigned by the software stack", formalDefinition = "Describes the local assigned unique identification by the software. For example: handle ID.") 595 protected Identifier identifier; 596 597 /** 598 * Describes the timestamp for the most recent system change which includes 599 * device configuration or setting change. 600 */ 601 @Child(name = "lastSystemChange", type = { 602 InstantType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 603 @Description(shortDefinition = "Recent system change timestamp", formalDefinition = "Describes the timestamp for the most recent system change which includes device configuration or setting change.") 604 protected InstantType lastSystemChange; 605 606 /** 607 * Describes the link to the source Device that contains administrative device 608 * information such as manufacture, serial number, etc. 609 */ 610 @Child(name = "source", type = { Device.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 611 @Description(shortDefinition = "A source device of this component", formalDefinition = "Describes the link to the source Device that contains administrative device information such as manufacture, serial number, etc.") 612 protected Reference source; 613 614 /** 615 * The actual object that is the target of the reference (Describes the link to 616 * the source Device that contains administrative device information such as 617 * manufacture, serial number, etc.) 618 */ 619 protected Device sourceTarget; 620 621 /** 622 * Describes the link to the parent resource. For example: Channel is linked to 623 * its VMD parent. 624 */ 625 @Child(name = "parent", type = { 626 DeviceComponent.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 627 @Description(shortDefinition = "Parent resource link", formalDefinition = "Describes the link to the parent resource. For example: Channel is linked to its VMD parent.") 628 protected Reference parent; 629 630 /** 631 * The actual object that is the target of the reference (Describes the link to 632 * the parent resource. For example: Channel is linked to its VMD parent.) 633 */ 634 protected DeviceComponent parentTarget; 635 636 /** 637 * Indicates current operational status of the device. For example: On, Off, 638 * Standby, etc. 639 */ 640 @Child(name = "operationalStatus", type = { 641 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 642 @Description(shortDefinition = "Component operational status", formalDefinition = "Indicates current operational status of the device. For example: On, Off, Standby, etc.") 643 protected List<CodeableConcept> operationalStatus; 644 645 /** 646 * Describes the parameter group supported by the current device component that 647 * is based on some nomenclature, e.g. cardiovascular. 648 */ 649 @Child(name = "parameterGroup", type = { 650 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 651 @Description(shortDefinition = "Current supported parameter group", formalDefinition = "Describes the parameter group supported by the current device component that is based on some nomenclature, e.g. cardiovascular.") 652 protected CodeableConcept parameterGroup; 653 654 /** 655 * Describes the physical principle of the measurement. For example: thermal, 656 * chemical, acoustical, etc. 657 */ 658 @Child(name = "measurementPrinciple", type = { 659 CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 660 @Description(shortDefinition = "other | chemical | electrical | impedance | nuclear | optical | thermal | biological | mechanical | acoustical | manual+", formalDefinition = "Describes the physical principle of the measurement. For example: thermal, chemical, acoustical, etc.") 661 protected Enumeration<MeasmntPrinciple> measurementPrinciple; 662 663 /** 664 * Describes the production specification such as component revision, serial 665 * number, etc. 666 */ 667 @Child(name = "productionSpecification", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 668 @Description(shortDefinition = "Production specification of the component", formalDefinition = "Describes the production specification such as component revision, serial number, etc.") 669 protected List<DeviceComponentProductionSpecificationComponent> productionSpecification; 670 671 /** 672 * Describes the language code for the human-readable text string produced by 673 * the device. This language code will follow the IETF language tag. Example: 674 * en-US. 675 */ 676 @Child(name = "languageCode", type = { 677 CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 678 @Description(shortDefinition = "Language code for the human-readable text strings produced by the device", formalDefinition = "Describes the language code for the human-readable text string produced by the device. This language code will follow the IETF language tag. Example: en-US.") 679 protected CodeableConcept languageCode; 680 681 private static final long serialVersionUID = -1742890034L; 682 683 /* 684 * Constructor 685 */ 686 public DeviceComponent() { 687 super(); 688 } 689 690 /* 691 * Constructor 692 */ 693 public DeviceComponent(CodeableConcept type, Identifier identifier, InstantType lastSystemChange) { 694 super(); 695 this.type = type; 696 this.identifier = identifier; 697 this.lastSystemChange = lastSystemChange; 698 } 699 700 /** 701 * @return {@link #type} (Describes the specific component type as defined in 702 * the object-oriented or metric nomenclature partition.) 703 */ 704 public CodeableConcept getType() { 705 if (this.type == null) 706 if (Configuration.errorOnAutoCreate()) 707 throw new Error("Attempt to auto-create DeviceComponent.type"); 708 else if (Configuration.doAutoCreate()) 709 this.type = new CodeableConcept(); // cc 710 return this.type; 711 } 712 713 public boolean hasType() { 714 return this.type != null && !this.type.isEmpty(); 715 } 716 717 /** 718 * @param value {@link #type} (Describes the specific component type as defined 719 * in the object-oriented or metric nomenclature partition.) 720 */ 721 public DeviceComponent setType(CodeableConcept value) { 722 this.type = value; 723 return this; 724 } 725 726 /** 727 * @return {@link #identifier} (Describes the local assigned unique 728 * identification by the software. For example: handle ID.) 729 */ 730 public Identifier getIdentifier() { 731 if (this.identifier == null) 732 if (Configuration.errorOnAutoCreate()) 733 throw new Error("Attempt to auto-create DeviceComponent.identifier"); 734 else if (Configuration.doAutoCreate()) 735 this.identifier = new Identifier(); // cc 736 return this.identifier; 737 } 738 739 public boolean hasIdentifier() { 740 return this.identifier != null && !this.identifier.isEmpty(); 741 } 742 743 /** 744 * @param value {@link #identifier} (Describes the local assigned unique 745 * identification by the software. For example: handle ID.) 746 */ 747 public DeviceComponent setIdentifier(Identifier value) { 748 this.identifier = value; 749 return this; 750 } 751 752 /** 753 * @return {@link #lastSystemChange} (Describes the timestamp for the most 754 * recent system change which includes device configuration or setting 755 * change.). This is the underlying object with id, value and 756 * extensions. The accessor "getLastSystemChange" gives direct access to 757 * the value 758 */ 759 public InstantType getLastSystemChangeElement() { 760 if (this.lastSystemChange == null) 761 if (Configuration.errorOnAutoCreate()) 762 throw new Error("Attempt to auto-create DeviceComponent.lastSystemChange"); 763 else if (Configuration.doAutoCreate()) 764 this.lastSystemChange = new InstantType(); // bb 765 return this.lastSystemChange; 766 } 767 768 public boolean hasLastSystemChangeElement() { 769 return this.lastSystemChange != null && !this.lastSystemChange.isEmpty(); 770 } 771 772 public boolean hasLastSystemChange() { 773 return this.lastSystemChange != null && !this.lastSystemChange.isEmpty(); 774 } 775 776 /** 777 * @param value {@link #lastSystemChange} (Describes the timestamp for the most 778 * recent system change which includes device configuration or 779 * setting change.). This is the underlying object with id, value 780 * and extensions. The accessor "getLastSystemChange" gives direct 781 * access to the value 782 */ 783 public DeviceComponent setLastSystemChangeElement(InstantType value) { 784 this.lastSystemChange = value; 785 return this; 786 } 787 788 /** 789 * @return Describes the timestamp for the most recent system change which 790 * includes device configuration or setting change. 791 */ 792 public Date getLastSystemChange() { 793 return this.lastSystemChange == null ? null : this.lastSystemChange.getValue(); 794 } 795 796 /** 797 * @param value Describes the timestamp for the most recent system change which 798 * includes device configuration or setting change. 799 */ 800 public DeviceComponent setLastSystemChange(Date value) { 801 if (this.lastSystemChange == null) 802 this.lastSystemChange = new InstantType(); 803 this.lastSystemChange.setValue(value); 804 return this; 805 } 806 807 /** 808 * @return {@link #source} (Describes the link to the source Device that 809 * contains administrative device information such as manufacture, 810 * serial number, etc.) 811 */ 812 public Reference getSource() { 813 if (this.source == null) 814 if (Configuration.errorOnAutoCreate()) 815 throw new Error("Attempt to auto-create DeviceComponent.source"); 816 else if (Configuration.doAutoCreate()) 817 this.source = new Reference(); // cc 818 return this.source; 819 } 820 821 public boolean hasSource() { 822 return this.source != null && !this.source.isEmpty(); 823 } 824 825 /** 826 * @param value {@link #source} (Describes the link to the source Device that 827 * contains administrative device information such as manufacture, 828 * serial number, etc.) 829 */ 830 public DeviceComponent setSource(Reference value) { 831 this.source = value; 832 return this; 833 } 834 835 /** 836 * @return {@link #source} The actual object that is the target of the 837 * reference. The reference library doesn't populate this, but you can 838 * use it to hold the resource if you resolve it. (Describes the link to 839 * the source Device that contains administrative device information 840 * such as manufacture, serial number, etc.) 841 */ 842 public Device getSourceTarget() { 843 if (this.sourceTarget == null) 844 if (Configuration.errorOnAutoCreate()) 845 throw new Error("Attempt to auto-create DeviceComponent.source"); 846 else if (Configuration.doAutoCreate()) 847 this.sourceTarget = new Device(); // aa 848 return this.sourceTarget; 849 } 850 851 /** 852 * @param value {@link #source} The actual object that is the target of the 853 * reference. The reference library doesn't use these, but you can 854 * use it to hold the resource if you resolve it. (Describes the 855 * link to the source Device that contains administrative device 856 * information such as manufacture, serial number, etc.) 857 */ 858 public DeviceComponent setSourceTarget(Device value) { 859 this.sourceTarget = value; 860 return this; 861 } 862 863 /** 864 * @return {@link #parent} (Describes the link to the parent resource. For 865 * example: Channel is linked to its VMD parent.) 866 */ 867 public Reference getParent() { 868 if (this.parent == null) 869 if (Configuration.errorOnAutoCreate()) 870 throw new Error("Attempt to auto-create DeviceComponent.parent"); 871 else if (Configuration.doAutoCreate()) 872 this.parent = new Reference(); // cc 873 return this.parent; 874 } 875 876 public boolean hasParent() { 877 return this.parent != null && !this.parent.isEmpty(); 878 } 879 880 /** 881 * @param value {@link #parent} (Describes the link to the parent resource. For 882 * example: Channel is linked to its VMD parent.) 883 */ 884 public DeviceComponent setParent(Reference value) { 885 this.parent = value; 886 return this; 887 } 888 889 /** 890 * @return {@link #parent} The actual object that is the target of the 891 * reference. The reference library doesn't populate this, but you can 892 * use it to hold the resource if you resolve it. (Describes the link to 893 * the parent resource. For example: Channel is linked to its VMD 894 * parent.) 895 */ 896 public DeviceComponent getParentTarget() { 897 if (this.parentTarget == null) 898 if (Configuration.errorOnAutoCreate()) 899 throw new Error("Attempt to auto-create DeviceComponent.parent"); 900 else if (Configuration.doAutoCreate()) 901 this.parentTarget = new DeviceComponent(); // aa 902 return this.parentTarget; 903 } 904 905 /** 906 * @param value {@link #parent} The actual object that is the target of the 907 * reference. The reference library doesn't use these, but you can 908 * use it to hold the resource if you resolve it. (Describes the 909 * link to the parent resource. For example: Channel is linked to 910 * its VMD parent.) 911 */ 912 public DeviceComponent setParentTarget(DeviceComponent value) { 913 this.parentTarget = value; 914 return this; 915 } 916 917 /** 918 * @return {@link #operationalStatus} (Indicates current operational status of 919 * the device. For example: On, Off, Standby, etc.) 920 */ 921 public List<CodeableConcept> getOperationalStatus() { 922 if (this.operationalStatus == null) 923 this.operationalStatus = new ArrayList<CodeableConcept>(); 924 return this.operationalStatus; 925 } 926 927 public boolean hasOperationalStatus() { 928 if (this.operationalStatus == null) 929 return false; 930 for (CodeableConcept item : this.operationalStatus) 931 if (!item.isEmpty()) 932 return true; 933 return false; 934 } 935 936 /** 937 * @return {@link #operationalStatus} (Indicates current operational status of 938 * the device. For example: On, Off, Standby, etc.) 939 */ 940 // syntactic sugar 941 public CodeableConcept addOperationalStatus() { // 3 942 CodeableConcept t = new CodeableConcept(); 943 if (this.operationalStatus == null) 944 this.operationalStatus = new ArrayList<CodeableConcept>(); 945 this.operationalStatus.add(t); 946 return t; 947 } 948 949 // syntactic sugar 950 public DeviceComponent addOperationalStatus(CodeableConcept t) { // 3 951 if (t == null) 952 return this; 953 if (this.operationalStatus == null) 954 this.operationalStatus = new ArrayList<CodeableConcept>(); 955 this.operationalStatus.add(t); 956 return this; 957 } 958 959 /** 960 * @return {@link #parameterGroup} (Describes the parameter group supported by 961 * the current device component that is based on some nomenclature, e.g. 962 * cardiovascular.) 963 */ 964 public CodeableConcept getParameterGroup() { 965 if (this.parameterGroup == null) 966 if (Configuration.errorOnAutoCreate()) 967 throw new Error("Attempt to auto-create DeviceComponent.parameterGroup"); 968 else if (Configuration.doAutoCreate()) 969 this.parameterGroup = new CodeableConcept(); // cc 970 return this.parameterGroup; 971 } 972 973 public boolean hasParameterGroup() { 974 return this.parameterGroup != null && !this.parameterGroup.isEmpty(); 975 } 976 977 /** 978 * @param value {@link #parameterGroup} (Describes the parameter group supported 979 * by the current device component that is based on some 980 * nomenclature, e.g. cardiovascular.) 981 */ 982 public DeviceComponent setParameterGroup(CodeableConcept value) { 983 this.parameterGroup = value; 984 return this; 985 } 986 987 /** 988 * @return {@link #measurementPrinciple} (Describes the physical principle of 989 * the measurement. For example: thermal, chemical, acoustical, etc.). 990 * This is the underlying object with id, value and extensions. The 991 * accessor "getMeasurementPrinciple" gives direct access to the value 992 */ 993 public Enumeration<MeasmntPrinciple> getMeasurementPrincipleElement() { 994 if (this.measurementPrinciple == null) 995 if (Configuration.errorOnAutoCreate()) 996 throw new Error("Attempt to auto-create DeviceComponent.measurementPrinciple"); 997 else if (Configuration.doAutoCreate()) 998 this.measurementPrinciple = new Enumeration<MeasmntPrinciple>(new MeasmntPrincipleEnumFactory()); // bb 999 return this.measurementPrinciple; 1000 } 1001 1002 public boolean hasMeasurementPrincipleElement() { 1003 return this.measurementPrinciple != null && !this.measurementPrinciple.isEmpty(); 1004 } 1005 1006 public boolean hasMeasurementPrinciple() { 1007 return this.measurementPrinciple != null && !this.measurementPrinciple.isEmpty(); 1008 } 1009 1010 /** 1011 * @param value {@link #measurementPrinciple} (Describes the physical principle 1012 * of the measurement. For example: thermal, chemical, acoustical, 1013 * etc.). This is the underlying object with id, value and 1014 * extensions. The accessor "getMeasurementPrinciple" gives direct 1015 * access to the value 1016 */ 1017 public DeviceComponent setMeasurementPrincipleElement(Enumeration<MeasmntPrinciple> value) { 1018 this.measurementPrinciple = value; 1019 return this; 1020 } 1021 1022 /** 1023 * @return Describes the physical principle of the measurement. For example: 1024 * thermal, chemical, acoustical, etc. 1025 */ 1026 public MeasmntPrinciple getMeasurementPrinciple() { 1027 return this.measurementPrinciple == null ? null : this.measurementPrinciple.getValue(); 1028 } 1029 1030 /** 1031 * @param value Describes the physical principle of the measurement. For 1032 * example: thermal, chemical, acoustical, etc. 1033 */ 1034 public DeviceComponent setMeasurementPrinciple(MeasmntPrinciple value) { 1035 if (value == null) 1036 this.measurementPrinciple = null; 1037 else { 1038 if (this.measurementPrinciple == null) 1039 this.measurementPrinciple = new Enumeration<MeasmntPrinciple>(new MeasmntPrincipleEnumFactory()); 1040 this.measurementPrinciple.setValue(value); 1041 } 1042 return this; 1043 } 1044 1045 /** 1046 * @return {@link #productionSpecification} (Describes the production 1047 * specification such as component revision, serial number, etc.) 1048 */ 1049 public List<DeviceComponentProductionSpecificationComponent> getProductionSpecification() { 1050 if (this.productionSpecification == null) 1051 this.productionSpecification = new ArrayList<DeviceComponentProductionSpecificationComponent>(); 1052 return this.productionSpecification; 1053 } 1054 1055 public boolean hasProductionSpecification() { 1056 if (this.productionSpecification == null) 1057 return false; 1058 for (DeviceComponentProductionSpecificationComponent item : this.productionSpecification) 1059 if (!item.isEmpty()) 1060 return true; 1061 return false; 1062 } 1063 1064 /** 1065 * @return {@link #productionSpecification} (Describes the production 1066 * specification such as component revision, serial number, etc.) 1067 */ 1068 // syntactic sugar 1069 public DeviceComponentProductionSpecificationComponent addProductionSpecification() { // 3 1070 DeviceComponentProductionSpecificationComponent t = new DeviceComponentProductionSpecificationComponent(); 1071 if (this.productionSpecification == null) 1072 this.productionSpecification = new ArrayList<DeviceComponentProductionSpecificationComponent>(); 1073 this.productionSpecification.add(t); 1074 return t; 1075 } 1076 1077 // syntactic sugar 1078 public DeviceComponent addProductionSpecification(DeviceComponentProductionSpecificationComponent t) { // 3 1079 if (t == null) 1080 return this; 1081 if (this.productionSpecification == null) 1082 this.productionSpecification = new ArrayList<DeviceComponentProductionSpecificationComponent>(); 1083 this.productionSpecification.add(t); 1084 return this; 1085 } 1086 1087 /** 1088 * @return {@link #languageCode} (Describes the language code for the 1089 * human-readable text string produced by the device. This language code 1090 * will follow the IETF language tag. Example: en-US.) 1091 */ 1092 public CodeableConcept getLanguageCode() { 1093 if (this.languageCode == null) 1094 if (Configuration.errorOnAutoCreate()) 1095 throw new Error("Attempt to auto-create DeviceComponent.languageCode"); 1096 else if (Configuration.doAutoCreate()) 1097 this.languageCode = new CodeableConcept(); // cc 1098 return this.languageCode; 1099 } 1100 1101 public boolean hasLanguageCode() { 1102 return this.languageCode != null && !this.languageCode.isEmpty(); 1103 } 1104 1105 /** 1106 * @param value {@link #languageCode} (Describes the language code for the 1107 * human-readable text string produced by the device. This language 1108 * code will follow the IETF language tag. Example: en-US.) 1109 */ 1110 public DeviceComponent setLanguageCode(CodeableConcept value) { 1111 this.languageCode = value; 1112 return this; 1113 } 1114 1115 protected void listChildren(List<Property> childrenList) { 1116 super.listChildren(childrenList); 1117 childrenList.add(new Property("type", "CodeableConcept", 1118 "Describes the specific component type as defined in the object-oriented or metric nomenclature partition.", 0, 1119 java.lang.Integer.MAX_VALUE, type)); 1120 childrenList.add(new Property("identifier", "Identifier", 1121 "Describes the local assigned unique identification by the software. For example: handle ID.", 0, 1122 java.lang.Integer.MAX_VALUE, identifier)); 1123 childrenList.add(new Property("lastSystemChange", "instant", 1124 "Describes the timestamp for the most recent system change which includes device configuration or setting change.", 1125 0, java.lang.Integer.MAX_VALUE, lastSystemChange)); 1126 childrenList.add(new Property("source", "Reference(Device)", 1127 "Describes the link to the source Device that contains administrative device information such as manufacture, serial number, etc.", 1128 0, java.lang.Integer.MAX_VALUE, source)); 1129 childrenList.add(new Property("parent", "Reference(DeviceComponent)", 1130 "Describes the link to the parent resource. For example: Channel is linked to its VMD parent.", 0, 1131 java.lang.Integer.MAX_VALUE, parent)); 1132 childrenList.add(new Property("operationalStatus", "CodeableConcept", 1133 "Indicates current operational status of the device. For example: On, Off, Standby, etc.", 0, 1134 java.lang.Integer.MAX_VALUE, operationalStatus)); 1135 childrenList.add(new Property("parameterGroup", "CodeableConcept", 1136 "Describes the parameter group supported by the current device component that is based on some nomenclature, e.g. cardiovascular.", 1137 0, java.lang.Integer.MAX_VALUE, parameterGroup)); 1138 childrenList.add(new Property("measurementPrinciple", "code", 1139 "Describes the physical principle of the measurement. For example: thermal, chemical, acoustical, etc.", 0, 1140 java.lang.Integer.MAX_VALUE, measurementPrinciple)); 1141 childrenList.add(new Property("productionSpecification", "", 1142 "Describes the production specification such as component revision, serial number, etc.", 0, 1143 java.lang.Integer.MAX_VALUE, productionSpecification)); 1144 childrenList.add(new Property("languageCode", "CodeableConcept", 1145 "Describes the language code for the human-readable text string produced by the device. This language code will follow the IETF language tag. Example: en-US.", 1146 0, java.lang.Integer.MAX_VALUE, languageCode)); 1147 } 1148 1149 @Override 1150 public void setProperty(String name, Base value) throws FHIRException { 1151 if (name.equals("type")) 1152 this.type = castToCodeableConcept(value); // CodeableConcept 1153 else if (name.equals("identifier")) 1154 this.identifier = castToIdentifier(value); // Identifier 1155 else if (name.equals("lastSystemChange")) 1156 this.lastSystemChange = castToInstant(value); // InstantType 1157 else if (name.equals("source")) 1158 this.source = castToReference(value); // Reference 1159 else if (name.equals("parent")) 1160 this.parent = castToReference(value); // Reference 1161 else if (name.equals("operationalStatus")) 1162 this.getOperationalStatus().add(castToCodeableConcept(value)); 1163 else if (name.equals("parameterGroup")) 1164 this.parameterGroup = castToCodeableConcept(value); // CodeableConcept 1165 else if (name.equals("measurementPrinciple")) 1166 this.measurementPrinciple = new MeasmntPrincipleEnumFactory().fromType(value); // Enumeration<MeasmntPrinciple> 1167 else if (name.equals("productionSpecification")) 1168 this.getProductionSpecification().add((DeviceComponentProductionSpecificationComponent) value); 1169 else if (name.equals("languageCode")) 1170 this.languageCode = castToCodeableConcept(value); // CodeableConcept 1171 else 1172 super.setProperty(name, value); 1173 } 1174 1175 @Override 1176 public Base addChild(String name) throws FHIRException { 1177 if (name.equals("type")) { 1178 this.type = new CodeableConcept(); 1179 return this.type; 1180 } else if (name.equals("identifier")) { 1181 this.identifier = new Identifier(); 1182 return this.identifier; 1183 } else if (name.equals("lastSystemChange")) { 1184 throw new FHIRException("Cannot call addChild on a singleton property DeviceComponent.lastSystemChange"); 1185 } else if (name.equals("source")) { 1186 this.source = new Reference(); 1187 return this.source; 1188 } else if (name.equals("parent")) { 1189 this.parent = new Reference(); 1190 return this.parent; 1191 } else if (name.equals("operationalStatus")) { 1192 return addOperationalStatus(); 1193 } else if (name.equals("parameterGroup")) { 1194 this.parameterGroup = new CodeableConcept(); 1195 return this.parameterGroup; 1196 } else if (name.equals("measurementPrinciple")) { 1197 throw new FHIRException("Cannot call addChild on a singleton property DeviceComponent.measurementPrinciple"); 1198 } else if (name.equals("productionSpecification")) { 1199 return addProductionSpecification(); 1200 } else if (name.equals("languageCode")) { 1201 this.languageCode = new CodeableConcept(); 1202 return this.languageCode; 1203 } else 1204 return super.addChild(name); 1205 } 1206 1207 public String fhirType() { 1208 return "DeviceComponent"; 1209 1210 } 1211 1212 public DeviceComponent copy() { 1213 DeviceComponent dst = new DeviceComponent(); 1214 copyValues(dst); 1215 dst.type = type == null ? null : type.copy(); 1216 dst.identifier = identifier == null ? null : identifier.copy(); 1217 dst.lastSystemChange = lastSystemChange == null ? null : lastSystemChange.copy(); 1218 dst.source = source == null ? null : source.copy(); 1219 dst.parent = parent == null ? null : parent.copy(); 1220 if (operationalStatus != null) { 1221 dst.operationalStatus = new ArrayList<CodeableConcept>(); 1222 for (CodeableConcept i : operationalStatus) 1223 dst.operationalStatus.add(i.copy()); 1224 } 1225 ; 1226 dst.parameterGroup = parameterGroup == null ? null : parameterGroup.copy(); 1227 dst.measurementPrinciple = measurementPrinciple == null ? null : measurementPrinciple.copy(); 1228 if (productionSpecification != null) { 1229 dst.productionSpecification = new ArrayList<DeviceComponentProductionSpecificationComponent>(); 1230 for (DeviceComponentProductionSpecificationComponent i : productionSpecification) 1231 dst.productionSpecification.add(i.copy()); 1232 } 1233 ; 1234 dst.languageCode = languageCode == null ? null : languageCode.copy(); 1235 return dst; 1236 } 1237 1238 protected DeviceComponent typedCopy() { 1239 return copy(); 1240 } 1241 1242 @Override 1243 public boolean equalsDeep(Base other) { 1244 if (!super.equalsDeep(other)) 1245 return false; 1246 if (!(other instanceof DeviceComponent)) 1247 return false; 1248 DeviceComponent o = (DeviceComponent) other; 1249 return compareDeep(type, o.type, true) && compareDeep(identifier, o.identifier, true) 1250 && compareDeep(lastSystemChange, o.lastSystemChange, true) && compareDeep(source, o.source, true) 1251 && compareDeep(parent, o.parent, true) && compareDeep(operationalStatus, o.operationalStatus, true) 1252 && compareDeep(parameterGroup, o.parameterGroup, true) 1253 && compareDeep(measurementPrinciple, o.measurementPrinciple, true) 1254 && compareDeep(productionSpecification, o.productionSpecification, true) 1255 && compareDeep(languageCode, o.languageCode, true); 1256 } 1257 1258 @Override 1259 public boolean equalsShallow(Base other) { 1260 if (!super.equalsShallow(other)) 1261 return false; 1262 if (!(other instanceof DeviceComponent)) 1263 return false; 1264 DeviceComponent o = (DeviceComponent) other; 1265 return compareValues(lastSystemChange, o.lastSystemChange, true) 1266 && compareValues(measurementPrinciple, o.measurementPrinciple, true); 1267 } 1268 1269 public boolean isEmpty() { 1270 return super.isEmpty() && (type == null || type.isEmpty()) && (identifier == null || identifier.isEmpty()) 1271 && (lastSystemChange == null || lastSystemChange.isEmpty()) && (source == null || source.isEmpty()) 1272 && (parent == null || parent.isEmpty()) && (operationalStatus == null || operationalStatus.isEmpty()) 1273 && (parameterGroup == null || parameterGroup.isEmpty()) 1274 && (measurementPrinciple == null || measurementPrinciple.isEmpty()) 1275 && (productionSpecification == null || productionSpecification.isEmpty()) 1276 && (languageCode == null || languageCode.isEmpty()); 1277 } 1278 1279 @Override 1280 public ResourceType getResourceType() { 1281 return ResourceType.DeviceComponent; 1282 } 1283 1284 @SearchParamDefinition(name = "parent", path = "DeviceComponent.parent", description = "The parent DeviceComponent resource", type = "reference") 1285 public static final String SP_PARENT = "parent"; 1286 @SearchParamDefinition(name = "source", path = "DeviceComponent.source", description = "The device source", type = "reference") 1287 public static final String SP_SOURCE = "source"; 1288 @SearchParamDefinition(name = "type", path = "DeviceComponent.type", description = "The device component type", type = "token") 1289 public static final String SP_TYPE = "type"; 1290 1291}