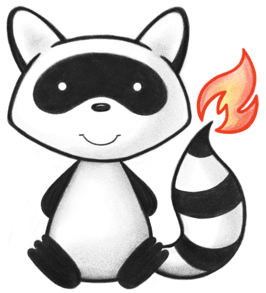
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044 045/** 046 * Describes a measurement, calculation or setting capability of a medical 047 * device. 048 */ 049@ResourceDef(name = "DeviceMetric", profile = "http://hl7.org/fhir/Profile/DeviceMetric") 050public class DeviceMetric extends DomainResource { 051 052 public enum DeviceMetricOperationalStatus { 053 /** 054 * The DeviceMetric is operating and will generate DeviceObservations. 055 */ 056 ON, 057 /** 058 * The DeviceMetric is not operating. 059 */ 060 OFF, 061 /** 062 * The DeviceMetric is operating, but will not generate any DeviceObservations. 063 */ 064 STANDBY, 065 /** 066 * added to help the parsers 067 */ 068 NULL; 069 070 public static DeviceMetricOperationalStatus fromCode(String codeString) throws FHIRException { 071 if (codeString == null || "".equals(codeString)) 072 return null; 073 if ("on".equals(codeString)) 074 return ON; 075 if ("off".equals(codeString)) 076 return OFF; 077 if ("standby".equals(codeString)) 078 return STANDBY; 079 throw new FHIRException("Unknown DeviceMetricOperationalStatus code '" + codeString + "'"); 080 } 081 082 public String toCode() { 083 switch (this) { 084 case ON: 085 return "on"; 086 case OFF: 087 return "off"; 088 case STANDBY: 089 return "standby"; 090 case NULL: 091 return null; 092 default: 093 return "?"; 094 } 095 } 096 097 public String getSystem() { 098 switch (this) { 099 case ON: 100 return "http://hl7.org/fhir/metric-operational-status"; 101 case OFF: 102 return "http://hl7.org/fhir/metric-operational-status"; 103 case STANDBY: 104 return "http://hl7.org/fhir/metric-operational-status"; 105 case NULL: 106 return null; 107 default: 108 return "?"; 109 } 110 } 111 112 public String getDefinition() { 113 switch (this) { 114 case ON: 115 return "The DeviceMetric is operating and will generate DeviceObservations."; 116 case OFF: 117 return "The DeviceMetric is not operating."; 118 case STANDBY: 119 return "The DeviceMetric is operating, but will not generate any DeviceObservations."; 120 case NULL: 121 return null; 122 default: 123 return "?"; 124 } 125 } 126 127 public String getDisplay() { 128 switch (this) { 129 case ON: 130 return "On"; 131 case OFF: 132 return "Off"; 133 case STANDBY: 134 return "Standby"; 135 case NULL: 136 return null; 137 default: 138 return "?"; 139 } 140 } 141 } 142 143 public static class DeviceMetricOperationalStatusEnumFactory implements EnumFactory<DeviceMetricOperationalStatus> { 144 public DeviceMetricOperationalStatus fromCode(String codeString) throws IllegalArgumentException { 145 if (codeString == null || "".equals(codeString)) 146 if (codeString == null || "".equals(codeString)) 147 return null; 148 if ("on".equals(codeString)) 149 return DeviceMetricOperationalStatus.ON; 150 if ("off".equals(codeString)) 151 return DeviceMetricOperationalStatus.OFF; 152 if ("standby".equals(codeString)) 153 return DeviceMetricOperationalStatus.STANDBY; 154 throw new IllegalArgumentException("Unknown DeviceMetricOperationalStatus code '" + codeString + "'"); 155 } 156 157 public Enumeration<DeviceMetricOperationalStatus> fromType(Base code) throws FHIRException { 158 if (code == null || code.isEmpty()) 159 return null; 160 String codeString = ((PrimitiveType) code).asStringValue(); 161 if (codeString == null || "".equals(codeString)) 162 return null; 163 if ("on".equals(codeString)) 164 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.ON); 165 if ("off".equals(codeString)) 166 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.OFF); 167 if ("standby".equals(codeString)) 168 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.STANDBY); 169 throw new FHIRException("Unknown DeviceMetricOperationalStatus code '" + codeString + "'"); 170 } 171 172 public String toCode(DeviceMetricOperationalStatus code) 173 { 174 if (code == DeviceMetricOperationalStatus.NULL) 175 return null; 176 if (code == DeviceMetricOperationalStatus.ON) 177 return "on"; 178 if (code == DeviceMetricOperationalStatus.OFF) 179 return "off"; 180 if (code == DeviceMetricOperationalStatus.STANDBY) 181 return "standby"; 182 return "?"; 183 } 184 } 185 186 public enum DeviceMetricColor { 187 /** 188 * Color for representation - black. 189 */ 190 BLACK, 191 /** 192 * Color for representation - red. 193 */ 194 RED, 195 /** 196 * Color for representation - green. 197 */ 198 GREEN, 199 /** 200 * Color for representation - yellow. 201 */ 202 YELLOW, 203 /** 204 * Color for representation - blue. 205 */ 206 BLUE, 207 /** 208 * Color for representation - magenta. 209 */ 210 MAGENTA, 211 /** 212 * Color for representation - cyan. 213 */ 214 CYAN, 215 /** 216 * Color for representation - white. 217 */ 218 WHITE, 219 /** 220 * added to help the parsers 221 */ 222 NULL; 223 224 public static DeviceMetricColor fromCode(String codeString) throws FHIRException { 225 if (codeString == null || "".equals(codeString)) 226 return null; 227 if ("black".equals(codeString)) 228 return BLACK; 229 if ("red".equals(codeString)) 230 return RED; 231 if ("green".equals(codeString)) 232 return GREEN; 233 if ("yellow".equals(codeString)) 234 return YELLOW; 235 if ("blue".equals(codeString)) 236 return BLUE; 237 if ("magenta".equals(codeString)) 238 return MAGENTA; 239 if ("cyan".equals(codeString)) 240 return CYAN; 241 if ("white".equals(codeString)) 242 return WHITE; 243 throw new FHIRException("Unknown DeviceMetricColor code '" + codeString + "'"); 244 } 245 246 public String toCode() { 247 switch (this) { 248 case BLACK: 249 return "black"; 250 case RED: 251 return "red"; 252 case GREEN: 253 return "green"; 254 case YELLOW: 255 return "yellow"; 256 case BLUE: 257 return "blue"; 258 case MAGENTA: 259 return "magenta"; 260 case CYAN: 261 return "cyan"; 262 case WHITE: 263 return "white"; 264 case NULL: 265 return null; 266 default: 267 return "?"; 268 } 269 } 270 271 public String getSystem() { 272 switch (this) { 273 case BLACK: 274 return "http://hl7.org/fhir/metric-color"; 275 case RED: 276 return "http://hl7.org/fhir/metric-color"; 277 case GREEN: 278 return "http://hl7.org/fhir/metric-color"; 279 case YELLOW: 280 return "http://hl7.org/fhir/metric-color"; 281 case BLUE: 282 return "http://hl7.org/fhir/metric-color"; 283 case MAGENTA: 284 return "http://hl7.org/fhir/metric-color"; 285 case CYAN: 286 return "http://hl7.org/fhir/metric-color"; 287 case WHITE: 288 return "http://hl7.org/fhir/metric-color"; 289 case NULL: 290 return null; 291 default: 292 return "?"; 293 } 294 } 295 296 public String getDefinition() { 297 switch (this) { 298 case BLACK: 299 return "Color for representation - black."; 300 case RED: 301 return "Color for representation - red."; 302 case GREEN: 303 return "Color for representation - green."; 304 case YELLOW: 305 return "Color for representation - yellow."; 306 case BLUE: 307 return "Color for representation - blue."; 308 case MAGENTA: 309 return "Color for representation - magenta."; 310 case CYAN: 311 return "Color for representation - cyan."; 312 case WHITE: 313 return "Color for representation - white."; 314 case NULL: 315 return null; 316 default: 317 return "?"; 318 } 319 } 320 321 public String getDisplay() { 322 switch (this) { 323 case BLACK: 324 return "Color Black"; 325 case RED: 326 return "Color Red"; 327 case GREEN: 328 return "Color Green"; 329 case YELLOW: 330 return "Color Yellow"; 331 case BLUE: 332 return "Color Blue"; 333 case MAGENTA: 334 return "Color Magenta"; 335 case CYAN: 336 return "Color Cyan"; 337 case WHITE: 338 return "Color White"; 339 case NULL: 340 return null; 341 default: 342 return "?"; 343 } 344 } 345 } 346 347 public static class DeviceMetricColorEnumFactory implements EnumFactory<DeviceMetricColor> { 348 public DeviceMetricColor fromCode(String codeString) throws IllegalArgumentException { 349 if (codeString == null || "".equals(codeString)) 350 if (codeString == null || "".equals(codeString)) 351 return null; 352 if ("black".equals(codeString)) 353 return DeviceMetricColor.BLACK; 354 if ("red".equals(codeString)) 355 return DeviceMetricColor.RED; 356 if ("green".equals(codeString)) 357 return DeviceMetricColor.GREEN; 358 if ("yellow".equals(codeString)) 359 return DeviceMetricColor.YELLOW; 360 if ("blue".equals(codeString)) 361 return DeviceMetricColor.BLUE; 362 if ("magenta".equals(codeString)) 363 return DeviceMetricColor.MAGENTA; 364 if ("cyan".equals(codeString)) 365 return DeviceMetricColor.CYAN; 366 if ("white".equals(codeString)) 367 return DeviceMetricColor.WHITE; 368 throw new IllegalArgumentException("Unknown DeviceMetricColor code '" + codeString + "'"); 369 } 370 371 public Enumeration<DeviceMetricColor> fromType(Base code) throws FHIRException { 372 if (code == null || code.isEmpty()) 373 return null; 374 String codeString = ((PrimitiveType) code).asStringValue(); 375 if (codeString == null || "".equals(codeString)) 376 return null; 377 if ("black".equals(codeString)) 378 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.BLACK); 379 if ("red".equals(codeString)) 380 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.RED); 381 if ("green".equals(codeString)) 382 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.GREEN); 383 if ("yellow".equals(codeString)) 384 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.YELLOW); 385 if ("blue".equals(codeString)) 386 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.BLUE); 387 if ("magenta".equals(codeString)) 388 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.MAGENTA); 389 if ("cyan".equals(codeString)) 390 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.CYAN); 391 if ("white".equals(codeString)) 392 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.WHITE); 393 throw new FHIRException("Unknown DeviceMetricColor code '" + codeString + "'"); 394 } 395 396 public String toCode(DeviceMetricColor code) 397 { 398 if (code == DeviceMetricColor.NULL) 399 return null; 400 if (code == DeviceMetricColor.BLACK) 401 return "black"; 402 if (code == DeviceMetricColor.RED) 403 return "red"; 404 if (code == DeviceMetricColor.GREEN) 405 return "green"; 406 if (code == DeviceMetricColor.YELLOW) 407 return "yellow"; 408 if (code == DeviceMetricColor.BLUE) 409 return "blue"; 410 if (code == DeviceMetricColor.MAGENTA) 411 return "magenta"; 412 if (code == DeviceMetricColor.CYAN) 413 return "cyan"; 414 if (code == DeviceMetricColor.WHITE) 415 return "white"; 416 return "?"; 417 } 418 } 419 420 public enum DeviceMetricCategory { 421 /** 422 * DeviceObservations generated for this DeviceMetric are measured. 423 */ 424 MEASUREMENT, 425 /** 426 * DeviceObservations generated for this DeviceMetric is a setting that will 427 * influence the behavior of the Device. 428 */ 429 SETTING, 430 /** 431 * DeviceObservations generated for this DeviceMetric are calculated. 432 */ 433 CALCULATION, 434 /** 435 * The category of this DeviceMetric is unspecified. 436 */ 437 UNSPECIFIED, 438 /** 439 * added to help the parsers 440 */ 441 NULL; 442 443 public static DeviceMetricCategory fromCode(String codeString) throws FHIRException { 444 if (codeString == null || "".equals(codeString)) 445 return null; 446 if ("measurement".equals(codeString)) 447 return MEASUREMENT; 448 if ("setting".equals(codeString)) 449 return SETTING; 450 if ("calculation".equals(codeString)) 451 return CALCULATION; 452 if ("unspecified".equals(codeString)) 453 return UNSPECIFIED; 454 throw new FHIRException("Unknown DeviceMetricCategory code '" + codeString + "'"); 455 } 456 457 public String toCode() { 458 switch (this) { 459 case MEASUREMENT: 460 return "measurement"; 461 case SETTING: 462 return "setting"; 463 case CALCULATION: 464 return "calculation"; 465 case UNSPECIFIED: 466 return "unspecified"; 467 case NULL: 468 return null; 469 default: 470 return "?"; 471 } 472 } 473 474 public String getSystem() { 475 switch (this) { 476 case MEASUREMENT: 477 return "http://hl7.org/fhir/metric-category"; 478 case SETTING: 479 return "http://hl7.org/fhir/metric-category"; 480 case CALCULATION: 481 return "http://hl7.org/fhir/metric-category"; 482 case UNSPECIFIED: 483 return "http://hl7.org/fhir/metric-category"; 484 case NULL: 485 return null; 486 default: 487 return "?"; 488 } 489 } 490 491 public String getDefinition() { 492 switch (this) { 493 case MEASUREMENT: 494 return "DeviceObservations generated for this DeviceMetric are measured."; 495 case SETTING: 496 return "DeviceObservations generated for this DeviceMetric is a setting that will influence the behavior of the Device."; 497 case CALCULATION: 498 return "DeviceObservations generated for this DeviceMetric are calculated."; 499 case UNSPECIFIED: 500 return "The category of this DeviceMetric is unspecified."; 501 case NULL: 502 return null; 503 default: 504 return "?"; 505 } 506 } 507 508 public String getDisplay() { 509 switch (this) { 510 case MEASUREMENT: 511 return "Measurement"; 512 case SETTING: 513 return "Setting"; 514 case CALCULATION: 515 return "Calculation"; 516 case UNSPECIFIED: 517 return "Unspecified"; 518 case NULL: 519 return null; 520 default: 521 return "?"; 522 } 523 } 524 } 525 526 public static class DeviceMetricCategoryEnumFactory implements EnumFactory<DeviceMetricCategory> { 527 public DeviceMetricCategory fromCode(String codeString) throws IllegalArgumentException { 528 if (codeString == null || "".equals(codeString)) 529 if (codeString == null || "".equals(codeString)) 530 return null; 531 if ("measurement".equals(codeString)) 532 return DeviceMetricCategory.MEASUREMENT; 533 if ("setting".equals(codeString)) 534 return DeviceMetricCategory.SETTING; 535 if ("calculation".equals(codeString)) 536 return DeviceMetricCategory.CALCULATION; 537 if ("unspecified".equals(codeString)) 538 return DeviceMetricCategory.UNSPECIFIED; 539 throw new IllegalArgumentException("Unknown DeviceMetricCategory code '" + codeString + "'"); 540 } 541 542 public Enumeration<DeviceMetricCategory> fromType(Base code) throws FHIRException { 543 if (code == null || code.isEmpty()) 544 return null; 545 String codeString = ((PrimitiveType) code).asStringValue(); 546 if (codeString == null || "".equals(codeString)) 547 return null; 548 if ("measurement".equals(codeString)) 549 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.MEASUREMENT); 550 if ("setting".equals(codeString)) 551 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.SETTING); 552 if ("calculation".equals(codeString)) 553 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.CALCULATION); 554 if ("unspecified".equals(codeString)) 555 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.UNSPECIFIED); 556 throw new FHIRException("Unknown DeviceMetricCategory code '" + codeString + "'"); 557 } 558 559 public String toCode(DeviceMetricCategory code) 560 { 561 if (code == DeviceMetricCategory.NULL) 562 return null; 563 if (code == DeviceMetricCategory.MEASUREMENT) 564 return "measurement"; 565 if (code == DeviceMetricCategory.SETTING) 566 return "setting"; 567 if (code == DeviceMetricCategory.CALCULATION) 568 return "calculation"; 569 if (code == DeviceMetricCategory.UNSPECIFIED) 570 return "unspecified"; 571 return "?"; 572 } 573 } 574 575 public enum DeviceMetricCalibrationType { 576 /** 577 * TODO 578 */ 579 UNSPECIFIED, 580 /** 581 * TODO 582 */ 583 OFFSET, 584 /** 585 * TODO 586 */ 587 GAIN, 588 /** 589 * TODO 590 */ 591 TWOPOINT, 592 /** 593 * added to help the parsers 594 */ 595 NULL; 596 597 public static DeviceMetricCalibrationType fromCode(String codeString) throws FHIRException { 598 if (codeString == null || "".equals(codeString)) 599 return null; 600 if ("unspecified".equals(codeString)) 601 return UNSPECIFIED; 602 if ("offset".equals(codeString)) 603 return OFFSET; 604 if ("gain".equals(codeString)) 605 return GAIN; 606 if ("two-point".equals(codeString)) 607 return TWOPOINT; 608 throw new FHIRException("Unknown DeviceMetricCalibrationType code '" + codeString + "'"); 609 } 610 611 public String toCode() { 612 switch (this) { 613 case UNSPECIFIED: 614 return "unspecified"; 615 case OFFSET: 616 return "offset"; 617 case GAIN: 618 return "gain"; 619 case TWOPOINT: 620 return "two-point"; 621 case NULL: 622 return null; 623 default: 624 return "?"; 625 } 626 } 627 628 public String getSystem() { 629 switch (this) { 630 case UNSPECIFIED: 631 return "http://hl7.org/fhir/metric-calibration-type"; 632 case OFFSET: 633 return "http://hl7.org/fhir/metric-calibration-type"; 634 case GAIN: 635 return "http://hl7.org/fhir/metric-calibration-type"; 636 case TWOPOINT: 637 return "http://hl7.org/fhir/metric-calibration-type"; 638 case NULL: 639 return null; 640 default: 641 return "?"; 642 } 643 } 644 645 public String getDefinition() { 646 switch (this) { 647 case UNSPECIFIED: 648 return "TODO"; 649 case OFFSET: 650 return "TODO"; 651 case GAIN: 652 return "TODO"; 653 case TWOPOINT: 654 return "TODO"; 655 case NULL: 656 return null; 657 default: 658 return "?"; 659 } 660 } 661 662 public String getDisplay() { 663 switch (this) { 664 case UNSPECIFIED: 665 return "Unspecified"; 666 case OFFSET: 667 return "Offset"; 668 case GAIN: 669 return "Gain"; 670 case TWOPOINT: 671 return "Two Point"; 672 case NULL: 673 return null; 674 default: 675 return "?"; 676 } 677 } 678 } 679 680 public static class DeviceMetricCalibrationTypeEnumFactory implements EnumFactory<DeviceMetricCalibrationType> { 681 public DeviceMetricCalibrationType fromCode(String codeString) throws IllegalArgumentException { 682 if (codeString == null || "".equals(codeString)) 683 if (codeString == null || "".equals(codeString)) 684 return null; 685 if ("unspecified".equals(codeString)) 686 return DeviceMetricCalibrationType.UNSPECIFIED; 687 if ("offset".equals(codeString)) 688 return DeviceMetricCalibrationType.OFFSET; 689 if ("gain".equals(codeString)) 690 return DeviceMetricCalibrationType.GAIN; 691 if ("two-point".equals(codeString)) 692 return DeviceMetricCalibrationType.TWOPOINT; 693 throw new IllegalArgumentException("Unknown DeviceMetricCalibrationType code '" + codeString + "'"); 694 } 695 696 public Enumeration<DeviceMetricCalibrationType> fromType(Base code) throws FHIRException { 697 if (code == null || code.isEmpty()) 698 return null; 699 String codeString = ((PrimitiveType) code).asStringValue(); 700 if (codeString == null || "".equals(codeString)) 701 return null; 702 if ("unspecified".equals(codeString)) 703 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.UNSPECIFIED); 704 if ("offset".equals(codeString)) 705 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.OFFSET); 706 if ("gain".equals(codeString)) 707 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.GAIN); 708 if ("two-point".equals(codeString)) 709 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.TWOPOINT); 710 throw new FHIRException("Unknown DeviceMetricCalibrationType code '" + codeString + "'"); 711 } 712 713 public String toCode(DeviceMetricCalibrationType code) 714 { 715 if (code == DeviceMetricCalibrationType.NULL) 716 return null; 717 if (code == DeviceMetricCalibrationType.UNSPECIFIED) 718 return "unspecified"; 719 if (code == DeviceMetricCalibrationType.OFFSET) 720 return "offset"; 721 if (code == DeviceMetricCalibrationType.GAIN) 722 return "gain"; 723 if (code == DeviceMetricCalibrationType.TWOPOINT) 724 return "two-point"; 725 return "?"; 726 } 727 } 728 729 public enum DeviceMetricCalibrationState { 730 /** 731 * The metric has not been calibrated. 732 */ 733 NOTCALIBRATED, 734 /** 735 * The metric needs to be calibrated. 736 */ 737 CALIBRATIONREQUIRED, 738 /** 739 * The metric has been calibrated. 740 */ 741 CALIBRATED, 742 /** 743 * The state of calibration of this metric is unspecified. 744 */ 745 UNSPECIFIED, 746 /** 747 * added to help the parsers 748 */ 749 NULL; 750 751 public static DeviceMetricCalibrationState fromCode(String codeString) throws FHIRException { 752 if (codeString == null || "".equals(codeString)) 753 return null; 754 if ("not-calibrated".equals(codeString)) 755 return NOTCALIBRATED; 756 if ("calibration-required".equals(codeString)) 757 return CALIBRATIONREQUIRED; 758 if ("calibrated".equals(codeString)) 759 return CALIBRATED; 760 if ("unspecified".equals(codeString)) 761 return UNSPECIFIED; 762 throw new FHIRException("Unknown DeviceMetricCalibrationState code '" + codeString + "'"); 763 } 764 765 public String toCode() { 766 switch (this) { 767 case NOTCALIBRATED: 768 return "not-calibrated"; 769 case CALIBRATIONREQUIRED: 770 return "calibration-required"; 771 case CALIBRATED: 772 return "calibrated"; 773 case UNSPECIFIED: 774 return "unspecified"; 775 case NULL: 776 return null; 777 default: 778 return "?"; 779 } 780 } 781 782 public String getSystem() { 783 switch (this) { 784 case NOTCALIBRATED: 785 return "http://hl7.org/fhir/metric-calibration-state"; 786 case CALIBRATIONREQUIRED: 787 return "http://hl7.org/fhir/metric-calibration-state"; 788 case CALIBRATED: 789 return "http://hl7.org/fhir/metric-calibration-state"; 790 case UNSPECIFIED: 791 return "http://hl7.org/fhir/metric-calibration-state"; 792 case NULL: 793 return null; 794 default: 795 return "?"; 796 } 797 } 798 799 public String getDefinition() { 800 switch (this) { 801 case NOTCALIBRATED: 802 return "The metric has not been calibrated."; 803 case CALIBRATIONREQUIRED: 804 return "The metric needs to be calibrated."; 805 case CALIBRATED: 806 return "The metric has been calibrated."; 807 case UNSPECIFIED: 808 return "The state of calibration of this metric is unspecified."; 809 case NULL: 810 return null; 811 default: 812 return "?"; 813 } 814 } 815 816 public String getDisplay() { 817 switch (this) { 818 case NOTCALIBRATED: 819 return "Not Calibrated"; 820 case CALIBRATIONREQUIRED: 821 return "Calibration Required"; 822 case CALIBRATED: 823 return "Calibrated"; 824 case UNSPECIFIED: 825 return "Unspecified"; 826 case NULL: 827 return null; 828 default: 829 return "?"; 830 } 831 } 832 } 833 834 public static class DeviceMetricCalibrationStateEnumFactory implements EnumFactory<DeviceMetricCalibrationState> { 835 public DeviceMetricCalibrationState fromCode(String codeString) throws IllegalArgumentException { 836 if (codeString == null || "".equals(codeString)) 837 if (codeString == null || "".equals(codeString)) 838 return null; 839 if ("not-calibrated".equals(codeString)) 840 return DeviceMetricCalibrationState.NOTCALIBRATED; 841 if ("calibration-required".equals(codeString)) 842 return DeviceMetricCalibrationState.CALIBRATIONREQUIRED; 843 if ("calibrated".equals(codeString)) 844 return DeviceMetricCalibrationState.CALIBRATED; 845 if ("unspecified".equals(codeString)) 846 return DeviceMetricCalibrationState.UNSPECIFIED; 847 throw new IllegalArgumentException("Unknown DeviceMetricCalibrationState code '" + codeString + "'"); 848 } 849 850 public Enumeration<DeviceMetricCalibrationState> fromType(Base code) throws FHIRException { 851 if (code == null || code.isEmpty()) 852 return null; 853 String codeString = ((PrimitiveType) code).asStringValue(); 854 if (codeString == null || "".equals(codeString)) 855 return null; 856 if ("not-calibrated".equals(codeString)) 857 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.NOTCALIBRATED); 858 if ("calibration-required".equals(codeString)) 859 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.CALIBRATIONREQUIRED); 860 if ("calibrated".equals(codeString)) 861 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.CALIBRATED); 862 if ("unspecified".equals(codeString)) 863 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.UNSPECIFIED); 864 throw new FHIRException("Unknown DeviceMetricCalibrationState code '" + codeString + "'"); 865 } 866 867 public String toCode(DeviceMetricCalibrationState code) 868 { 869 if (code == DeviceMetricCalibrationState.NULL) 870 return null; 871 if (code == DeviceMetricCalibrationState.NOTCALIBRATED) 872 return "not-calibrated"; 873 if (code == DeviceMetricCalibrationState.CALIBRATIONREQUIRED) 874 return "calibration-required"; 875 if (code == DeviceMetricCalibrationState.CALIBRATED) 876 return "calibrated"; 877 if (code == DeviceMetricCalibrationState.UNSPECIFIED) 878 return "unspecified"; 879 return "?"; 880 } 881 } 882 883 @Block() 884 public static class DeviceMetricCalibrationComponent extends BackboneElement implements IBaseBackboneElement { 885 /** 886 * Describes the type of the calibration method. 887 */ 888 @Child(name = "type", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 889 @Description(shortDefinition = "unspecified | offset | gain | two-point", formalDefinition = "Describes the type of the calibration method.") 890 protected Enumeration<DeviceMetricCalibrationType> type; 891 892 /** 893 * Describes the state of the calibration. 894 */ 895 @Child(name = "state", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 896 @Description(shortDefinition = "not-calibrated | calibration-required | calibrated | unspecified", formalDefinition = "Describes the state of the calibration.") 897 protected Enumeration<DeviceMetricCalibrationState> state; 898 899 /** 900 * Describes the time last calibration has been performed. 901 */ 902 @Child(name = "time", type = { InstantType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 903 @Description(shortDefinition = "Describes the time last calibration has been performed", formalDefinition = "Describes the time last calibration has been performed.") 904 protected InstantType time; 905 906 private static final long serialVersionUID = 1163986578L; 907 908 /* 909 * Constructor 910 */ 911 public DeviceMetricCalibrationComponent() { 912 super(); 913 } 914 915 /** 916 * @return {@link #type} (Describes the type of the calibration method.). This 917 * is the underlying object with id, value and extensions. The accessor 918 * "getType" gives direct access to the value 919 */ 920 public Enumeration<DeviceMetricCalibrationType> getTypeElement() { 921 if (this.type == null) 922 if (Configuration.errorOnAutoCreate()) 923 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.type"); 924 else if (Configuration.doAutoCreate()) 925 this.type = new Enumeration<DeviceMetricCalibrationType>(new DeviceMetricCalibrationTypeEnumFactory()); // bb 926 return this.type; 927 } 928 929 public boolean hasTypeElement() { 930 return this.type != null && !this.type.isEmpty(); 931 } 932 933 public boolean hasType() { 934 return this.type != null && !this.type.isEmpty(); 935 } 936 937 /** 938 * @param value {@link #type} (Describes the type of the calibration method.). 939 * This is the underlying object with id, value and extensions. The 940 * accessor "getType" gives direct access to the value 941 */ 942 public DeviceMetricCalibrationComponent setTypeElement(Enumeration<DeviceMetricCalibrationType> value) { 943 this.type = value; 944 return this; 945 } 946 947 /** 948 * @return Describes the type of the calibration method. 949 */ 950 public DeviceMetricCalibrationType getType() { 951 return this.type == null ? null : this.type.getValue(); 952 } 953 954 /** 955 * @param value Describes the type of the calibration method. 956 */ 957 public DeviceMetricCalibrationComponent setType(DeviceMetricCalibrationType value) { 958 if (value == null) 959 this.type = null; 960 else { 961 if (this.type == null) 962 this.type = new Enumeration<DeviceMetricCalibrationType>(new DeviceMetricCalibrationTypeEnumFactory()); 963 this.type.setValue(value); 964 } 965 return this; 966 } 967 968 /** 969 * @return {@link #state} (Describes the state of the calibration.). This is the 970 * underlying object with id, value and extensions. The accessor 971 * "getState" gives direct access to the value 972 */ 973 public Enumeration<DeviceMetricCalibrationState> getStateElement() { 974 if (this.state == null) 975 if (Configuration.errorOnAutoCreate()) 976 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.state"); 977 else if (Configuration.doAutoCreate()) 978 this.state = new Enumeration<DeviceMetricCalibrationState>(new DeviceMetricCalibrationStateEnumFactory()); // bb 979 return this.state; 980 } 981 982 public boolean hasStateElement() { 983 return this.state != null && !this.state.isEmpty(); 984 } 985 986 public boolean hasState() { 987 return this.state != null && !this.state.isEmpty(); 988 } 989 990 /** 991 * @param value {@link #state} (Describes the state of the calibration.). This 992 * is the underlying object with id, value and extensions. The 993 * accessor "getState" gives direct access to the value 994 */ 995 public DeviceMetricCalibrationComponent setStateElement(Enumeration<DeviceMetricCalibrationState> value) { 996 this.state = value; 997 return this; 998 } 999 1000 /** 1001 * @return Describes the state of the calibration. 1002 */ 1003 public DeviceMetricCalibrationState getState() { 1004 return this.state == null ? null : this.state.getValue(); 1005 } 1006 1007 /** 1008 * @param value Describes the state of the calibration. 1009 */ 1010 public DeviceMetricCalibrationComponent setState(DeviceMetricCalibrationState value) { 1011 if (value == null) 1012 this.state = null; 1013 else { 1014 if (this.state == null) 1015 this.state = new Enumeration<DeviceMetricCalibrationState>(new DeviceMetricCalibrationStateEnumFactory()); 1016 this.state.setValue(value); 1017 } 1018 return this; 1019 } 1020 1021 /** 1022 * @return {@link #time} (Describes the time last calibration has been 1023 * performed.). This is the underlying object with id, value and 1024 * extensions. The accessor "getTime" gives direct access to the value 1025 */ 1026 public InstantType getTimeElement() { 1027 if (this.time == null) 1028 if (Configuration.errorOnAutoCreate()) 1029 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.time"); 1030 else if (Configuration.doAutoCreate()) 1031 this.time = new InstantType(); // bb 1032 return this.time; 1033 } 1034 1035 public boolean hasTimeElement() { 1036 return this.time != null && !this.time.isEmpty(); 1037 } 1038 1039 public boolean hasTime() { 1040 return this.time != null && !this.time.isEmpty(); 1041 } 1042 1043 /** 1044 * @param value {@link #time} (Describes the time last calibration has been 1045 * performed.). This is the underlying object with id, value and 1046 * extensions. The accessor "getTime" gives direct access to the 1047 * value 1048 */ 1049 public DeviceMetricCalibrationComponent setTimeElement(InstantType value) { 1050 this.time = value; 1051 return this; 1052 } 1053 1054 /** 1055 * @return Describes the time last calibration has been performed. 1056 */ 1057 public Date getTime() { 1058 return this.time == null ? null : this.time.getValue(); 1059 } 1060 1061 /** 1062 * @param value Describes the time last calibration has been performed. 1063 */ 1064 public DeviceMetricCalibrationComponent setTime(Date value) { 1065 if (value == null) 1066 this.time = null; 1067 else { 1068 if (this.time == null) 1069 this.time = new InstantType(); 1070 this.time.setValue(value); 1071 } 1072 return this; 1073 } 1074 1075 protected void listChildren(List<Property> childrenList) { 1076 super.listChildren(childrenList); 1077 childrenList.add(new Property("type", "code", "Describes the type of the calibration method.", 0, 1078 java.lang.Integer.MAX_VALUE, type)); 1079 childrenList.add(new Property("state", "code", "Describes the state of the calibration.", 0, 1080 java.lang.Integer.MAX_VALUE, state)); 1081 childrenList.add(new Property("time", "instant", "Describes the time last calibration has been performed.", 0, 1082 java.lang.Integer.MAX_VALUE, time)); 1083 } 1084 1085 @Override 1086 public void setProperty(String name, Base value) throws FHIRException { 1087 if (name.equals("type")) 1088 this.type = new DeviceMetricCalibrationTypeEnumFactory().fromType(value); // Enumeration<DeviceMetricCalibrationType> 1089 else if (name.equals("state")) 1090 this.state = new DeviceMetricCalibrationStateEnumFactory().fromType(value); // Enumeration<DeviceMetricCalibrationState> 1091 else if (name.equals("time")) 1092 this.time = castToInstant(value); // InstantType 1093 else 1094 super.setProperty(name, value); 1095 } 1096 1097 @Override 1098 public Base addChild(String name) throws FHIRException { 1099 if (name.equals("type")) { 1100 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.type"); 1101 } else if (name.equals("state")) { 1102 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.state"); 1103 } else if (name.equals("time")) { 1104 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.time"); 1105 } else 1106 return super.addChild(name); 1107 } 1108 1109 public DeviceMetricCalibrationComponent copy() { 1110 DeviceMetricCalibrationComponent dst = new DeviceMetricCalibrationComponent(); 1111 copyValues(dst); 1112 dst.type = type == null ? null : type.copy(); 1113 dst.state = state == null ? null : state.copy(); 1114 dst.time = time == null ? null : time.copy(); 1115 return dst; 1116 } 1117 1118 @Override 1119 public boolean equalsDeep(Base other) { 1120 if (!super.equalsDeep(other)) 1121 return false; 1122 if (!(other instanceof DeviceMetricCalibrationComponent)) 1123 return false; 1124 DeviceMetricCalibrationComponent o = (DeviceMetricCalibrationComponent) other; 1125 return compareDeep(type, o.type, true) && compareDeep(state, o.state, true) && compareDeep(time, o.time, true); 1126 } 1127 1128 @Override 1129 public boolean equalsShallow(Base other) { 1130 if (!super.equalsShallow(other)) 1131 return false; 1132 if (!(other instanceof DeviceMetricCalibrationComponent)) 1133 return false; 1134 DeviceMetricCalibrationComponent o = (DeviceMetricCalibrationComponent) other; 1135 return compareValues(type, o.type, true) && compareValues(state, o.state, true) 1136 && compareValues(time, o.time, true); 1137 } 1138 1139 public boolean isEmpty() { 1140 return super.isEmpty() && (type == null || type.isEmpty()) && (state == null || state.isEmpty()) 1141 && (time == null || time.isEmpty()); 1142 } 1143 1144 public String fhirType() { 1145 return "DeviceMetric.calibration"; 1146 1147 } 1148 1149 } 1150 1151 /** 1152 * Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc. 1153 */ 1154 @Child(name = "type", type = { CodeableConcept.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 1155 @Description(shortDefinition = "Type of metric", formalDefinition = "Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.") 1156 protected CodeableConcept type; 1157 1158 /** 1159 * Describes the unique identification of this metric that has been assigned by 1160 * the device or gateway software. For example: handle ID. It should be noted 1161 * that in order to make the identifier unique, the system element of the 1162 * identifier should be set to the unique identifier of the device. 1163 */ 1164 @Child(name = "identifier", type = { 1165 Identifier.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1166 @Description(shortDefinition = "Unique identifier of this DeviceMetric", formalDefinition = "Describes the unique identification of this metric that has been assigned by the device or gateway software. For example: handle ID. It should be noted that in order to make the identifier unique, the system element of the identifier should be set to the unique identifier of the device.") 1167 protected Identifier identifier; 1168 1169 /** 1170 * Describes the unit that an observed value determined for this metric will 1171 * have. For example: Percent, Seconds, etc. 1172 */ 1173 @Child(name = "unit", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1174 @Description(shortDefinition = "Unit of metric", formalDefinition = "Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.") 1175 protected CodeableConcept unit; 1176 1177 /** 1178 * Describes the link to the Device that this DeviceMetric belongs to and that 1179 * contains administrative device information such as manufacture, serial 1180 * number, etc. 1181 */ 1182 @Child(name = "source", type = { Device.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1183 @Description(shortDefinition = "Describes the link to the source Device", formalDefinition = "Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacture, serial number, etc.") 1184 protected Reference source; 1185 1186 /** 1187 * The actual object that is the target of the reference (Describes the link to 1188 * the Device that this DeviceMetric belongs to and that contains administrative 1189 * device information such as manufacture, serial number, etc.) 1190 */ 1191 protected Device sourceTarget; 1192 1193 /** 1194 * Describes the link to the DeviceComponent that this DeviceMetric belongs to 1195 * and that provide information about the location of this DeviceMetric in the 1196 * containment structure of the parent Device. An example would be a 1197 * DeviceComponent that represents a Channel. This reference can be used by a 1198 * client application to distinguish DeviceMetrics that have the same type, but 1199 * should be interpreted based on their containment location. 1200 */ 1201 @Child(name = "parent", type = { 1202 DeviceComponent.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1203 @Description(shortDefinition = "Describes the link to the parent DeviceComponent", formalDefinition = "Describes the link to the DeviceComponent that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a DeviceComponent that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location.") 1204 protected Reference parent; 1205 1206 /** 1207 * The actual object that is the target of the reference (Describes the link to 1208 * the DeviceComponent that this DeviceMetric belongs to and that provide 1209 * information about the location of this DeviceMetric in the containment 1210 * structure of the parent Device. An example would be a DeviceComponent that 1211 * represents a Channel. This reference can be used by a client application to 1212 * distinguish DeviceMetrics that have the same type, but should be interpreted 1213 * based on their containment location.) 1214 */ 1215 protected DeviceComponent parentTarget; 1216 1217 /** 1218 * Indicates current operational state of the device. For example: On, Off, 1219 * Standby, etc. 1220 */ 1221 @Child(name = "operationalStatus", type = { 1222 CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1223 @Description(shortDefinition = "on | off | standby", formalDefinition = "Indicates current operational state of the device. For example: On, Off, Standby, etc.") 1224 protected Enumeration<DeviceMetricOperationalStatus> operationalStatus; 1225 1226 /** 1227 * Describes the color representation for the metric. This is often used to aid 1228 * clinicians to track and identify parameter types by color. In practice, 1229 * consider a Patient Monitor that has ECG/HR and Pleth for example; the 1230 * parameters are displayed in different characteristic colors, such as HR-blue, 1231 * BP-green, and PR and SpO2- magenta. 1232 */ 1233 @Child(name = "color", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1234 @Description(shortDefinition = "black | red | green | yellow | blue | magenta | cyan | white", formalDefinition = "Describes the color representation for the metric. This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth for example; the parameters are displayed in different characteristic colors, such as HR-blue, BP-green, and PR and SpO2- magenta.") 1235 protected Enumeration<DeviceMetricColor> color; 1236 1237 /** 1238 * Indicates the category of the observation generation process. A DeviceMetric 1239 * can be for example a setting, measurement, or calculation. 1240 */ 1241 @Child(name = "category", type = { CodeType.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 1242 @Description(shortDefinition = "measurement | setting | calculation | unspecified", formalDefinition = "Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.") 1243 protected Enumeration<DeviceMetricCategory> category; 1244 1245 /** 1246 * Describes the measurement repetition time. This is not necessarily the same 1247 * as the update period. The measurement repetition time can range from 1248 * milliseconds up to hours. An example for a measurement repetition time in the 1249 * range of milliseconds is the sampling rate of an ECG. An example for a 1250 * measurement repetition time in the range of hours is a NIBP that is triggered 1251 * automatically every hour. The update period may be different than the 1252 * measurement repetition time, if the device does not update the published 1253 * observed value with the same frequency as it was measured. 1254 */ 1255 @Child(name = "measurementPeriod", type = { 1256 Timing.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1257 @Description(shortDefinition = "Describes the measurement repetition time", formalDefinition = "Describes the measurement repetition time. This is not necessarily the same as the update period. The measurement repetition time can range from milliseconds up to hours. An example for a measurement repetition time in the range of milliseconds is the sampling rate of an ECG. An example for a measurement repetition time in the range of hours is a NIBP that is triggered automatically every hour. The update period may be different than the measurement repetition time, if the device does not update the published observed value with the same frequency as it was measured.") 1258 protected Timing measurementPeriod; 1259 1260 /** 1261 * Describes the calibrations that have been performed or that are required to 1262 * be performed. 1263 */ 1264 @Child(name = "calibration", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1265 @Description(shortDefinition = "Describes the calibrations that have been performed or that are required to be performed", formalDefinition = "Describes the calibrations that have been performed or that are required to be performed.") 1266 protected List<DeviceMetricCalibrationComponent> calibration; 1267 1268 private static final long serialVersionUID = 1786401018L; 1269 1270 /* 1271 * Constructor 1272 */ 1273 public DeviceMetric() { 1274 super(); 1275 } 1276 1277 /* 1278 * Constructor 1279 */ 1280 public DeviceMetric(CodeableConcept type, Identifier identifier, Enumeration<DeviceMetricCategory> category) { 1281 super(); 1282 this.type = type; 1283 this.identifier = identifier; 1284 this.category = category; 1285 } 1286 1287 /** 1288 * @return {@link #type} (Describes the type of the metric. For example: Heart 1289 * Rate, PEEP Setting, etc.) 1290 */ 1291 public CodeableConcept getType() { 1292 if (this.type == null) 1293 if (Configuration.errorOnAutoCreate()) 1294 throw new Error("Attempt to auto-create DeviceMetric.type"); 1295 else if (Configuration.doAutoCreate()) 1296 this.type = new CodeableConcept(); // cc 1297 return this.type; 1298 } 1299 1300 public boolean hasType() { 1301 return this.type != null && !this.type.isEmpty(); 1302 } 1303 1304 /** 1305 * @param value {@link #type} (Describes the type of the metric. For example: 1306 * Heart Rate, PEEP Setting, etc.) 1307 */ 1308 public DeviceMetric setType(CodeableConcept value) { 1309 this.type = value; 1310 return this; 1311 } 1312 1313 /** 1314 * @return {@link #identifier} (Describes the unique identification of this 1315 * metric that has been assigned by the device or gateway software. For 1316 * example: handle ID. It should be noted that in order to make the 1317 * identifier unique, the system element of the identifier should be set 1318 * to the unique identifier of the device.) 1319 */ 1320 public Identifier getIdentifier() { 1321 if (this.identifier == null) 1322 if (Configuration.errorOnAutoCreate()) 1323 throw new Error("Attempt to auto-create DeviceMetric.identifier"); 1324 else if (Configuration.doAutoCreate()) 1325 this.identifier = new Identifier(); // cc 1326 return this.identifier; 1327 } 1328 1329 public boolean hasIdentifier() { 1330 return this.identifier != null && !this.identifier.isEmpty(); 1331 } 1332 1333 /** 1334 * @param value {@link #identifier} (Describes the unique identification of this 1335 * metric that has been assigned by the device or gateway software. 1336 * For example: handle ID. It should be noted that in order to make 1337 * the identifier unique, the system element of the identifier 1338 * should be set to the unique identifier of the device.) 1339 */ 1340 public DeviceMetric setIdentifier(Identifier value) { 1341 this.identifier = value; 1342 return this; 1343 } 1344 1345 /** 1346 * @return {@link #unit} (Describes the unit that an observed value determined 1347 * for this metric will have. For example: Percent, Seconds, etc.) 1348 */ 1349 public CodeableConcept getUnit() { 1350 if (this.unit == null) 1351 if (Configuration.errorOnAutoCreate()) 1352 throw new Error("Attempt to auto-create DeviceMetric.unit"); 1353 else if (Configuration.doAutoCreate()) 1354 this.unit = new CodeableConcept(); // cc 1355 return this.unit; 1356 } 1357 1358 public boolean hasUnit() { 1359 return this.unit != null && !this.unit.isEmpty(); 1360 } 1361 1362 /** 1363 * @param value {@link #unit} (Describes the unit that an observed value 1364 * determined for this metric will have. For example: Percent, 1365 * Seconds, etc.) 1366 */ 1367 public DeviceMetric setUnit(CodeableConcept value) { 1368 this.unit = value; 1369 return this; 1370 } 1371 1372 /** 1373 * @return {@link #source} (Describes the link to the Device that this 1374 * DeviceMetric belongs to and that contains administrative device 1375 * information such as manufacture, serial number, etc.) 1376 */ 1377 public Reference getSource() { 1378 if (this.source == null) 1379 if (Configuration.errorOnAutoCreate()) 1380 throw new Error("Attempt to auto-create DeviceMetric.source"); 1381 else if (Configuration.doAutoCreate()) 1382 this.source = new Reference(); // cc 1383 return this.source; 1384 } 1385 1386 public boolean hasSource() { 1387 return this.source != null && !this.source.isEmpty(); 1388 } 1389 1390 /** 1391 * @param value {@link #source} (Describes the link to the Device that this 1392 * DeviceMetric belongs to and that contains administrative device 1393 * information such as manufacture, serial number, etc.) 1394 */ 1395 public DeviceMetric setSource(Reference value) { 1396 this.source = value; 1397 return this; 1398 } 1399 1400 /** 1401 * @return {@link #source} The actual object that is the target of the 1402 * reference. The reference library doesn't populate this, but you can 1403 * use it to hold the resource if you resolve it. (Describes the link to 1404 * the Device that this DeviceMetric belongs to and that contains 1405 * administrative device information such as manufacture, serial number, 1406 * etc.) 1407 */ 1408 public Device getSourceTarget() { 1409 if (this.sourceTarget == null) 1410 if (Configuration.errorOnAutoCreate()) 1411 throw new Error("Attempt to auto-create DeviceMetric.source"); 1412 else if (Configuration.doAutoCreate()) 1413 this.sourceTarget = new Device(); // aa 1414 return this.sourceTarget; 1415 } 1416 1417 /** 1418 * @param value {@link #source} The actual object that is the target of the 1419 * reference. The reference library doesn't use these, but you can 1420 * use it to hold the resource if you resolve it. (Describes the 1421 * link to the Device that this DeviceMetric belongs to and that 1422 * contains administrative device information such as manufacture, 1423 * serial number, etc.) 1424 */ 1425 public DeviceMetric setSourceTarget(Device value) { 1426 this.sourceTarget = value; 1427 return this; 1428 } 1429 1430 /** 1431 * @return {@link #parent} (Describes the link to the DeviceComponent that this 1432 * DeviceMetric belongs to and that provide information about the 1433 * location of this DeviceMetric in the containment structure of the 1434 * parent Device. An example would be a DeviceComponent that represents 1435 * a Channel. This reference can be used by a client application to 1436 * distinguish DeviceMetrics that have the same type, but should be 1437 * interpreted based on their containment location.) 1438 */ 1439 public Reference getParent() { 1440 if (this.parent == null) 1441 if (Configuration.errorOnAutoCreate()) 1442 throw new Error("Attempt to auto-create DeviceMetric.parent"); 1443 else if (Configuration.doAutoCreate()) 1444 this.parent = new Reference(); // cc 1445 return this.parent; 1446 } 1447 1448 public boolean hasParent() { 1449 return this.parent != null && !this.parent.isEmpty(); 1450 } 1451 1452 /** 1453 * @param value {@link #parent} (Describes the link to the DeviceComponent that 1454 * this DeviceMetric belongs to and that provide information about 1455 * the location of this DeviceMetric in the containment structure 1456 * of the parent Device. An example would be a DeviceComponent that 1457 * represents a Channel. This reference can be used by a client 1458 * application to distinguish DeviceMetrics that have the same 1459 * type, but should be interpreted based on their containment 1460 * location.) 1461 */ 1462 public DeviceMetric setParent(Reference value) { 1463 this.parent = value; 1464 return this; 1465 } 1466 1467 /** 1468 * @return {@link #parent} The actual object that is the target of the 1469 * reference. The reference library doesn't populate this, but you can 1470 * use it to hold the resource if you resolve it. (Describes the link to 1471 * the DeviceComponent that this DeviceMetric belongs to and that 1472 * provide information about the location of this DeviceMetric in the 1473 * containment structure of the parent Device. An example would be a 1474 * DeviceComponent that represents a Channel. This reference can be used 1475 * by a client application to distinguish DeviceMetrics that have the 1476 * same type, but should be interpreted based on their containment 1477 * location.) 1478 */ 1479 public DeviceComponent getParentTarget() { 1480 if (this.parentTarget == null) 1481 if (Configuration.errorOnAutoCreate()) 1482 throw new Error("Attempt to auto-create DeviceMetric.parent"); 1483 else if (Configuration.doAutoCreate()) 1484 this.parentTarget = new DeviceComponent(); // aa 1485 return this.parentTarget; 1486 } 1487 1488 /** 1489 * @param value {@link #parent} The actual object that is the target of the 1490 * reference. The reference library doesn't use these, but you can 1491 * use it to hold the resource if you resolve it. (Describes the 1492 * link to the DeviceComponent that this DeviceMetric belongs to 1493 * and that provide information about the location of this 1494 * DeviceMetric in the containment structure of the parent Device. 1495 * An example would be a DeviceComponent that represents a Channel. 1496 * This reference can be used by a client application to 1497 * distinguish DeviceMetrics that have the same type, but should be 1498 * interpreted based on their containment location.) 1499 */ 1500 public DeviceMetric setParentTarget(DeviceComponent value) { 1501 this.parentTarget = value; 1502 return this; 1503 } 1504 1505 /** 1506 * @return {@link #operationalStatus} (Indicates current operational state of 1507 * the device. For example: On, Off, Standby, etc.). This is the 1508 * underlying object with id, value and extensions. The accessor 1509 * "getOperationalStatus" gives direct access to the value 1510 */ 1511 public Enumeration<DeviceMetricOperationalStatus> getOperationalStatusElement() { 1512 if (this.operationalStatus == null) 1513 if (Configuration.errorOnAutoCreate()) 1514 throw new Error("Attempt to auto-create DeviceMetric.operationalStatus"); 1515 else if (Configuration.doAutoCreate()) 1516 this.operationalStatus = new Enumeration<DeviceMetricOperationalStatus>( 1517 new DeviceMetricOperationalStatusEnumFactory()); // bb 1518 return this.operationalStatus; 1519 } 1520 1521 public boolean hasOperationalStatusElement() { 1522 return this.operationalStatus != null && !this.operationalStatus.isEmpty(); 1523 } 1524 1525 public boolean hasOperationalStatus() { 1526 return this.operationalStatus != null && !this.operationalStatus.isEmpty(); 1527 } 1528 1529 /** 1530 * @param value {@link #operationalStatus} (Indicates current operational state 1531 * of the device. For example: On, Off, Standby, etc.). This is the 1532 * underlying object with id, value and extensions. The accessor 1533 * "getOperationalStatus" gives direct access to the value 1534 */ 1535 public DeviceMetric setOperationalStatusElement(Enumeration<DeviceMetricOperationalStatus> value) { 1536 this.operationalStatus = value; 1537 return this; 1538 } 1539 1540 /** 1541 * @return Indicates current operational state of the device. For example: On, 1542 * Off, Standby, etc. 1543 */ 1544 public DeviceMetricOperationalStatus getOperationalStatus() { 1545 return this.operationalStatus == null ? null : this.operationalStatus.getValue(); 1546 } 1547 1548 /** 1549 * @param value Indicates current operational state of the device. For example: 1550 * On, Off, Standby, etc. 1551 */ 1552 public DeviceMetric setOperationalStatus(DeviceMetricOperationalStatus value) { 1553 if (value == null) 1554 this.operationalStatus = null; 1555 else { 1556 if (this.operationalStatus == null) 1557 this.operationalStatus = new Enumeration<DeviceMetricOperationalStatus>( 1558 new DeviceMetricOperationalStatusEnumFactory()); 1559 this.operationalStatus.setValue(value); 1560 } 1561 return this; 1562 } 1563 1564 /** 1565 * @return {@link #color} (Describes the color representation for the metric. 1566 * This is often used to aid clinicians to track and identify parameter 1567 * types by color. In practice, consider a Patient Monitor that has 1568 * ECG/HR and Pleth for example; the parameters are displayed in 1569 * different characteristic colors, such as HR-blue, BP-green, and PR 1570 * and SpO2- magenta.). This is the underlying object with id, value and 1571 * extensions. The accessor "getColor" gives direct access to the value 1572 */ 1573 public Enumeration<DeviceMetricColor> getColorElement() { 1574 if (this.color == null) 1575 if (Configuration.errorOnAutoCreate()) 1576 throw new Error("Attempt to auto-create DeviceMetric.color"); 1577 else if (Configuration.doAutoCreate()) 1578 this.color = new Enumeration<DeviceMetricColor>(new DeviceMetricColorEnumFactory()); // bb 1579 return this.color; 1580 } 1581 1582 public boolean hasColorElement() { 1583 return this.color != null && !this.color.isEmpty(); 1584 } 1585 1586 public boolean hasColor() { 1587 return this.color != null && !this.color.isEmpty(); 1588 } 1589 1590 /** 1591 * @param value {@link #color} (Describes the color representation for the 1592 * metric. This is often used to aid clinicians to track and 1593 * identify parameter types by color. In practice, consider a 1594 * Patient Monitor that has ECG/HR and Pleth for example; the 1595 * parameters are displayed in different characteristic colors, 1596 * such as HR-blue, BP-green, and PR and SpO2- magenta.). This is 1597 * the underlying object with id, value and extensions. The 1598 * accessor "getColor" gives direct access to the value 1599 */ 1600 public DeviceMetric setColorElement(Enumeration<DeviceMetricColor> value) { 1601 this.color = value; 1602 return this; 1603 } 1604 1605 /** 1606 * @return Describes the color representation for the metric. This is often used 1607 * to aid clinicians to track and identify parameter types by color. In 1608 * practice, consider a Patient Monitor that has ECG/HR and Pleth for 1609 * example; the parameters are displayed in different characteristic 1610 * colors, such as HR-blue, BP-green, and PR and SpO2- magenta. 1611 */ 1612 public DeviceMetricColor getColor() { 1613 return this.color == null ? null : this.color.getValue(); 1614 } 1615 1616 /** 1617 * @param value Describes the color representation for the metric. This is often 1618 * used to aid clinicians to track and identify parameter types by 1619 * color. In practice, consider a Patient Monitor that has ECG/HR 1620 * and Pleth for example; the parameters are displayed in different 1621 * characteristic colors, such as HR-blue, BP-green, and PR and 1622 * SpO2- magenta. 1623 */ 1624 public DeviceMetric setColor(DeviceMetricColor value) { 1625 if (value == null) 1626 this.color = null; 1627 else { 1628 if (this.color == null) 1629 this.color = new Enumeration<DeviceMetricColor>(new DeviceMetricColorEnumFactory()); 1630 this.color.setValue(value); 1631 } 1632 return this; 1633 } 1634 1635 /** 1636 * @return {@link #category} (Indicates the category of the observation 1637 * generation process. A DeviceMetric can be for example a setting, 1638 * measurement, or calculation.). This is the underlying object with id, 1639 * value and extensions. The accessor "getCategory" gives direct access 1640 * to the value 1641 */ 1642 public Enumeration<DeviceMetricCategory> getCategoryElement() { 1643 if (this.category == null) 1644 if (Configuration.errorOnAutoCreate()) 1645 throw new Error("Attempt to auto-create DeviceMetric.category"); 1646 else if (Configuration.doAutoCreate()) 1647 this.category = new Enumeration<DeviceMetricCategory>(new DeviceMetricCategoryEnumFactory()); // bb 1648 return this.category; 1649 } 1650 1651 public boolean hasCategoryElement() { 1652 return this.category != null && !this.category.isEmpty(); 1653 } 1654 1655 public boolean hasCategory() { 1656 return this.category != null && !this.category.isEmpty(); 1657 } 1658 1659 /** 1660 * @param value {@link #category} (Indicates the category of the observation 1661 * generation process. A DeviceMetric can be for example a setting, 1662 * measurement, or calculation.). This is the underlying object 1663 * with id, value and extensions. The accessor "getCategory" gives 1664 * direct access to the value 1665 */ 1666 public DeviceMetric setCategoryElement(Enumeration<DeviceMetricCategory> value) { 1667 this.category = value; 1668 return this; 1669 } 1670 1671 /** 1672 * @return Indicates the category of the observation generation process. A 1673 * DeviceMetric can be for example a setting, measurement, or 1674 * calculation. 1675 */ 1676 public DeviceMetricCategory getCategory() { 1677 return this.category == null ? null : this.category.getValue(); 1678 } 1679 1680 /** 1681 * @param value Indicates the category of the observation generation process. A 1682 * DeviceMetric can be for example a setting, measurement, or 1683 * calculation. 1684 */ 1685 public DeviceMetric setCategory(DeviceMetricCategory value) { 1686 if (this.category == null) 1687 this.category = new Enumeration<DeviceMetricCategory>(new DeviceMetricCategoryEnumFactory()); 1688 this.category.setValue(value); 1689 return this; 1690 } 1691 1692 /** 1693 * @return {@link #measurementPeriod} (Describes the measurement repetition 1694 * time. This is not necessarily the same as the update period. The 1695 * measurement repetition time can range from milliseconds up to hours. 1696 * An example for a measurement repetition time in the range of 1697 * milliseconds is the sampling rate of an ECG. An example for a 1698 * measurement repetition time in the range of hours is a NIBP that is 1699 * triggered automatically every hour. The update period may be 1700 * different than the measurement repetition time, if the device does 1701 * not update the published observed value with the same frequency as it 1702 * was measured.) 1703 */ 1704 public Timing getMeasurementPeriod() { 1705 if (this.measurementPeriod == null) 1706 if (Configuration.errorOnAutoCreate()) 1707 throw new Error("Attempt to auto-create DeviceMetric.measurementPeriod"); 1708 else if (Configuration.doAutoCreate()) 1709 this.measurementPeriod = new Timing(); // cc 1710 return this.measurementPeriod; 1711 } 1712 1713 public boolean hasMeasurementPeriod() { 1714 return this.measurementPeriod != null && !this.measurementPeriod.isEmpty(); 1715 } 1716 1717 /** 1718 * @param value {@link #measurementPeriod} (Describes the measurement repetition 1719 * time. This is not necessarily the same as the update period. The 1720 * measurement repetition time can range from milliseconds up to 1721 * hours. An example for a measurement repetition time in the range 1722 * of milliseconds is the sampling rate of an ECG. An example for a 1723 * measurement repetition time in the range of hours is a NIBP that 1724 * is triggered automatically every hour. The update period may be 1725 * different than the measurement repetition time, if the device 1726 * does not update the published observed value with the same 1727 * frequency as it was measured.) 1728 */ 1729 public DeviceMetric setMeasurementPeriod(Timing value) { 1730 this.measurementPeriod = value; 1731 return this; 1732 } 1733 1734 /** 1735 * @return {@link #calibration} (Describes the calibrations that have been 1736 * performed or that are required to be performed.) 1737 */ 1738 public List<DeviceMetricCalibrationComponent> getCalibration() { 1739 if (this.calibration == null) 1740 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1741 return this.calibration; 1742 } 1743 1744 public boolean hasCalibration() { 1745 if (this.calibration == null) 1746 return false; 1747 for (DeviceMetricCalibrationComponent item : this.calibration) 1748 if (!item.isEmpty()) 1749 return true; 1750 return false; 1751 } 1752 1753 /** 1754 * @return {@link #calibration} (Describes the calibrations that have been 1755 * performed or that are required to be performed.) 1756 */ 1757 // syntactic sugar 1758 public DeviceMetricCalibrationComponent addCalibration() { // 3 1759 DeviceMetricCalibrationComponent t = new DeviceMetricCalibrationComponent(); 1760 if (this.calibration == null) 1761 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1762 this.calibration.add(t); 1763 return t; 1764 } 1765 1766 // syntactic sugar 1767 public DeviceMetric addCalibration(DeviceMetricCalibrationComponent t) { // 3 1768 if (t == null) 1769 return this; 1770 if (this.calibration == null) 1771 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1772 this.calibration.add(t); 1773 return this; 1774 } 1775 1776 protected void listChildren(List<Property> childrenList) { 1777 super.listChildren(childrenList); 1778 childrenList.add(new Property("type", "CodeableConcept", 1779 "Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.", 0, java.lang.Integer.MAX_VALUE, 1780 type)); 1781 childrenList.add(new Property("identifier", "Identifier", 1782 "Describes the unique identification of this metric that has been assigned by the device or gateway software. For example: handle ID. It should be noted that in order to make the identifier unique, the system element of the identifier should be set to the unique identifier of the device.", 1783 0, java.lang.Integer.MAX_VALUE, identifier)); 1784 childrenList.add(new Property("unit", "CodeableConcept", 1785 "Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.", 1786 0, java.lang.Integer.MAX_VALUE, unit)); 1787 childrenList.add(new Property("source", "Reference(Device)", 1788 "Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacture, serial number, etc.", 1789 0, java.lang.Integer.MAX_VALUE, source)); 1790 childrenList.add(new Property("parent", "Reference(DeviceComponent)", 1791 "Describes the link to the DeviceComponent that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a DeviceComponent that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location.", 1792 0, java.lang.Integer.MAX_VALUE, parent)); 1793 childrenList.add(new Property("operationalStatus", "code", 1794 "Indicates current operational state of the device. For example: On, Off, Standby, etc.", 0, 1795 java.lang.Integer.MAX_VALUE, operationalStatus)); 1796 childrenList.add(new Property("color", "code", 1797 "Describes the color representation for the metric. This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth for example; the parameters are displayed in different characteristic colors, such as HR-blue, BP-green, and PR and SpO2- magenta.", 1798 0, java.lang.Integer.MAX_VALUE, color)); 1799 childrenList.add(new Property("category", "code", 1800 "Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.", 1801 0, java.lang.Integer.MAX_VALUE, category)); 1802 childrenList.add(new Property("measurementPeriod", "Timing", 1803 "Describes the measurement repetition time. This is not necessarily the same as the update period. The measurement repetition time can range from milliseconds up to hours. An example for a measurement repetition time in the range of milliseconds is the sampling rate of an ECG. An example for a measurement repetition time in the range of hours is a NIBP that is triggered automatically every hour. The update period may be different than the measurement repetition time, if the device does not update the published observed value with the same frequency as it was measured.", 1804 0, java.lang.Integer.MAX_VALUE, measurementPeriod)); 1805 childrenList.add(new Property("calibration", "", 1806 "Describes the calibrations that have been performed or that are required to be performed.", 0, 1807 java.lang.Integer.MAX_VALUE, calibration)); 1808 } 1809 1810 @Override 1811 public void setProperty(String name, Base value) throws FHIRException { 1812 if (name.equals("type")) 1813 this.type = castToCodeableConcept(value); // CodeableConcept 1814 else if (name.equals("identifier")) 1815 this.identifier = castToIdentifier(value); // Identifier 1816 else if (name.equals("unit")) 1817 this.unit = castToCodeableConcept(value); // CodeableConcept 1818 else if (name.equals("source")) 1819 this.source = castToReference(value); // Reference 1820 else if (name.equals("parent")) 1821 this.parent = castToReference(value); // Reference 1822 else if (name.equals("operationalStatus")) 1823 this.operationalStatus = new DeviceMetricOperationalStatusEnumFactory().fromType(value); // Enumeration<DeviceMetricOperationalStatus> 1824 else if (name.equals("color")) 1825 this.color = new DeviceMetricColorEnumFactory().fromType(value); // Enumeration<DeviceMetricColor> 1826 else if (name.equals("category")) 1827 this.category = new DeviceMetricCategoryEnumFactory().fromType(value); // Enumeration<DeviceMetricCategory> 1828 else if (name.equals("measurementPeriod")) 1829 this.measurementPeriod = castToTiming(value); // Timing 1830 else if (name.equals("calibration")) 1831 this.getCalibration().add((DeviceMetricCalibrationComponent) value); 1832 else 1833 super.setProperty(name, value); 1834 } 1835 1836 @Override 1837 public Base addChild(String name) throws FHIRException { 1838 if (name.equals("type")) { 1839 this.type = new CodeableConcept(); 1840 return this.type; 1841 } else if (name.equals("identifier")) { 1842 this.identifier = new Identifier(); 1843 return this.identifier; 1844 } else if (name.equals("unit")) { 1845 this.unit = new CodeableConcept(); 1846 return this.unit; 1847 } else if (name.equals("source")) { 1848 this.source = new Reference(); 1849 return this.source; 1850 } else if (name.equals("parent")) { 1851 this.parent = new Reference(); 1852 return this.parent; 1853 } else if (name.equals("operationalStatus")) { 1854 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.operationalStatus"); 1855 } else if (name.equals("color")) { 1856 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.color"); 1857 } else if (name.equals("category")) { 1858 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.category"); 1859 } else if (name.equals("measurementPeriod")) { 1860 this.measurementPeriod = new Timing(); 1861 return this.measurementPeriod; 1862 } else if (name.equals("calibration")) { 1863 return addCalibration(); 1864 } else 1865 return super.addChild(name); 1866 } 1867 1868 public String fhirType() { 1869 return "DeviceMetric"; 1870 1871 } 1872 1873 public DeviceMetric copy() { 1874 DeviceMetric dst = new DeviceMetric(); 1875 copyValues(dst); 1876 dst.type = type == null ? null : type.copy(); 1877 dst.identifier = identifier == null ? null : identifier.copy(); 1878 dst.unit = unit == null ? null : unit.copy(); 1879 dst.source = source == null ? null : source.copy(); 1880 dst.parent = parent == null ? null : parent.copy(); 1881 dst.operationalStatus = operationalStatus == null ? null : operationalStatus.copy(); 1882 dst.color = color == null ? null : color.copy(); 1883 dst.category = category == null ? null : category.copy(); 1884 dst.measurementPeriod = measurementPeriod == null ? null : measurementPeriod.copy(); 1885 if (calibration != null) { 1886 dst.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1887 for (DeviceMetricCalibrationComponent i : calibration) 1888 dst.calibration.add(i.copy()); 1889 } 1890 ; 1891 return dst; 1892 } 1893 1894 protected DeviceMetric typedCopy() { 1895 return copy(); 1896 } 1897 1898 @Override 1899 public boolean equalsDeep(Base other) { 1900 if (!super.equalsDeep(other)) 1901 return false; 1902 if (!(other instanceof DeviceMetric)) 1903 return false; 1904 DeviceMetric o = (DeviceMetric) other; 1905 return compareDeep(type, o.type, true) && compareDeep(identifier, o.identifier, true) 1906 && compareDeep(unit, o.unit, true) && compareDeep(source, o.source, true) && compareDeep(parent, o.parent, true) 1907 && compareDeep(operationalStatus, o.operationalStatus, true) && compareDeep(color, o.color, true) 1908 && compareDeep(category, o.category, true) && compareDeep(measurementPeriod, o.measurementPeriod, true) 1909 && compareDeep(calibration, o.calibration, true); 1910 } 1911 1912 @Override 1913 public boolean equalsShallow(Base other) { 1914 if (!super.equalsShallow(other)) 1915 return false; 1916 if (!(other instanceof DeviceMetric)) 1917 return false; 1918 DeviceMetric o = (DeviceMetric) other; 1919 return compareValues(operationalStatus, o.operationalStatus, true) && compareValues(color, o.color, true) 1920 && compareValues(category, o.category, true); 1921 } 1922 1923 public boolean isEmpty() { 1924 return super.isEmpty() && (type == null || type.isEmpty()) && (identifier == null || identifier.isEmpty()) 1925 && (unit == null || unit.isEmpty()) && (source == null || source.isEmpty()) 1926 && (parent == null || parent.isEmpty()) && (operationalStatus == null || operationalStatus.isEmpty()) 1927 && (color == null || color.isEmpty()) && (category == null || category.isEmpty()) 1928 && (measurementPeriod == null || measurementPeriod.isEmpty()) && (calibration == null || calibration.isEmpty()); 1929 } 1930 1931 @Override 1932 public ResourceType getResourceType() { 1933 return ResourceType.DeviceMetric; 1934 } 1935 1936 @SearchParamDefinition(name = "parent", path = "DeviceMetric.parent", description = "The parent DeviceMetric resource", type = "reference") 1937 public static final String SP_PARENT = "parent"; 1938 @SearchParamDefinition(name = "identifier", path = "DeviceMetric.identifier", description = "The identifier of the metric", type = "token") 1939 public static final String SP_IDENTIFIER = "identifier"; 1940 @SearchParamDefinition(name = "source", path = "DeviceMetric.source", description = "The device resource", type = "reference") 1941 public static final String SP_SOURCE = "source"; 1942 @SearchParamDefinition(name = "type", path = "DeviceMetric.type", description = "The component type", type = "token") 1943 public static final String SP_TYPE = "type"; 1944 @SearchParamDefinition(name = "category", path = "DeviceMetric.category", description = "The category of the metric", type = "token") 1945 public static final String SP_CATEGORY = "category"; 1946 1947}