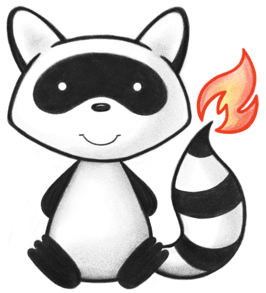
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.exceptions.FHIRException; 042 043/** 044 * Represents a request for a patient to employ a medical device. The device may 045 * be an implantable device, or an external assistive device, such as a walker. 046 */ 047@ResourceDef(name = "DeviceUseRequest", profile = "http://hl7.org/fhir/Profile/DeviceUseRequest") 048public class DeviceUseRequest extends DomainResource { 049 050 public enum DeviceUseRequestStatus { 051 /** 052 * The request has been proposed. 053 */ 054 PROPOSED, 055 /** 056 * The request has been planned. 057 */ 058 PLANNED, 059 /** 060 * The request has been placed. 061 */ 062 REQUESTED, 063 /** 064 * The receiving system has received the request but not yet decided whether it 065 * will be performed. 066 */ 067 RECEIVED, 068 /** 069 * The receiving system has accepted the request but work has not yet commenced. 070 */ 071 ACCEPTED, 072 /** 073 * The work to fulfill the order is happening. 074 */ 075 INPROGRESS, 076 /** 077 * The work has been complete, the report(s) released, and no further work is 078 * planned. 079 */ 080 COMPLETED, 081 /** 082 * The request has been held by originating system/user request. 083 */ 084 SUSPENDED, 085 /** 086 * The receiving system has declined to fulfill the request. 087 */ 088 REJECTED, 089 /** 090 * The request was attempted, but due to some procedural error, it could not be 091 * completed. 092 */ 093 ABORTED, 094 /** 095 * added to help the parsers 096 */ 097 NULL; 098 099 public static DeviceUseRequestStatus fromCode(String codeString) throws FHIRException { 100 if (codeString == null || "".equals(codeString)) 101 return null; 102 if ("proposed".equals(codeString)) 103 return PROPOSED; 104 if ("planned".equals(codeString)) 105 return PLANNED; 106 if ("requested".equals(codeString)) 107 return REQUESTED; 108 if ("received".equals(codeString)) 109 return RECEIVED; 110 if ("accepted".equals(codeString)) 111 return ACCEPTED; 112 if ("in-progress".equals(codeString)) 113 return INPROGRESS; 114 if ("completed".equals(codeString)) 115 return COMPLETED; 116 if ("suspended".equals(codeString)) 117 return SUSPENDED; 118 if ("rejected".equals(codeString)) 119 return REJECTED; 120 if ("aborted".equals(codeString)) 121 return ABORTED; 122 throw new FHIRException("Unknown DeviceUseRequestStatus code '" + codeString + "'"); 123 } 124 125 public String toCode() { 126 switch (this) { 127 case PROPOSED: 128 return "proposed"; 129 case PLANNED: 130 return "planned"; 131 case REQUESTED: 132 return "requested"; 133 case RECEIVED: 134 return "received"; 135 case ACCEPTED: 136 return "accepted"; 137 case INPROGRESS: 138 return "in-progress"; 139 case COMPLETED: 140 return "completed"; 141 case SUSPENDED: 142 return "suspended"; 143 case REJECTED: 144 return "rejected"; 145 case ABORTED: 146 return "aborted"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getSystem() { 155 switch (this) { 156 case PROPOSED: 157 return "http://hl7.org/fhir/device-use-request-status"; 158 case PLANNED: 159 return "http://hl7.org/fhir/device-use-request-status"; 160 case REQUESTED: 161 return "http://hl7.org/fhir/device-use-request-status"; 162 case RECEIVED: 163 return "http://hl7.org/fhir/device-use-request-status"; 164 case ACCEPTED: 165 return "http://hl7.org/fhir/device-use-request-status"; 166 case INPROGRESS: 167 return "http://hl7.org/fhir/device-use-request-status"; 168 case COMPLETED: 169 return "http://hl7.org/fhir/device-use-request-status"; 170 case SUSPENDED: 171 return "http://hl7.org/fhir/device-use-request-status"; 172 case REJECTED: 173 return "http://hl7.org/fhir/device-use-request-status"; 174 case ABORTED: 175 return "http://hl7.org/fhir/device-use-request-status"; 176 case NULL: 177 return null; 178 default: 179 return "?"; 180 } 181 } 182 183 public String getDefinition() { 184 switch (this) { 185 case PROPOSED: 186 return "The request has been proposed."; 187 case PLANNED: 188 return "The request has been planned."; 189 case REQUESTED: 190 return "The request has been placed."; 191 case RECEIVED: 192 return "The receiving system has received the request but not yet decided whether it will be performed."; 193 case ACCEPTED: 194 return "The receiving system has accepted the request but work has not yet commenced."; 195 case INPROGRESS: 196 return "The work to fulfill the order is happening."; 197 case COMPLETED: 198 return "The work has been complete, the report(s) released, and no further work is planned."; 199 case SUSPENDED: 200 return "The request has been held by originating system/user request."; 201 case REJECTED: 202 return "The receiving system has declined to fulfill the request."; 203 case ABORTED: 204 return "The request was attempted, but due to some procedural error, it could not be completed."; 205 case NULL: 206 return null; 207 default: 208 return "?"; 209 } 210 } 211 212 public String getDisplay() { 213 switch (this) { 214 case PROPOSED: 215 return "Proposed"; 216 case PLANNED: 217 return "Planned"; 218 case REQUESTED: 219 return "Requested"; 220 case RECEIVED: 221 return "Received"; 222 case ACCEPTED: 223 return "Accepted"; 224 case INPROGRESS: 225 return "In Progress"; 226 case COMPLETED: 227 return "Completed"; 228 case SUSPENDED: 229 return "Suspended"; 230 case REJECTED: 231 return "Rejected"; 232 case ABORTED: 233 return "Aborted"; 234 case NULL: 235 return null; 236 default: 237 return "?"; 238 } 239 } 240 } 241 242 public static class DeviceUseRequestStatusEnumFactory implements EnumFactory<DeviceUseRequestStatus> { 243 public DeviceUseRequestStatus fromCode(String codeString) throws IllegalArgumentException { 244 if (codeString == null || "".equals(codeString)) 245 if (codeString == null || "".equals(codeString)) 246 return null; 247 if ("proposed".equals(codeString)) 248 return DeviceUseRequestStatus.PROPOSED; 249 if ("planned".equals(codeString)) 250 return DeviceUseRequestStatus.PLANNED; 251 if ("requested".equals(codeString)) 252 return DeviceUseRequestStatus.REQUESTED; 253 if ("received".equals(codeString)) 254 return DeviceUseRequestStatus.RECEIVED; 255 if ("accepted".equals(codeString)) 256 return DeviceUseRequestStatus.ACCEPTED; 257 if ("in-progress".equals(codeString)) 258 return DeviceUseRequestStatus.INPROGRESS; 259 if ("completed".equals(codeString)) 260 return DeviceUseRequestStatus.COMPLETED; 261 if ("suspended".equals(codeString)) 262 return DeviceUseRequestStatus.SUSPENDED; 263 if ("rejected".equals(codeString)) 264 return DeviceUseRequestStatus.REJECTED; 265 if ("aborted".equals(codeString)) 266 return DeviceUseRequestStatus.ABORTED; 267 throw new IllegalArgumentException("Unknown DeviceUseRequestStatus code '" + codeString + "'"); 268 } 269 270 public Enumeration<DeviceUseRequestStatus> fromType(Base code) throws FHIRException { 271 if (code == null || code.isEmpty()) 272 return null; 273 String codeString = ((PrimitiveType) code).asStringValue(); 274 if (codeString == null || "".equals(codeString)) 275 return null; 276 if ("proposed".equals(codeString)) 277 return new Enumeration<DeviceUseRequestStatus>(this, DeviceUseRequestStatus.PROPOSED); 278 if ("planned".equals(codeString)) 279 return new Enumeration<DeviceUseRequestStatus>(this, DeviceUseRequestStatus.PLANNED); 280 if ("requested".equals(codeString)) 281 return new Enumeration<DeviceUseRequestStatus>(this, DeviceUseRequestStatus.REQUESTED); 282 if ("received".equals(codeString)) 283 return new Enumeration<DeviceUseRequestStatus>(this, DeviceUseRequestStatus.RECEIVED); 284 if ("accepted".equals(codeString)) 285 return new Enumeration<DeviceUseRequestStatus>(this, DeviceUseRequestStatus.ACCEPTED); 286 if ("in-progress".equals(codeString)) 287 return new Enumeration<DeviceUseRequestStatus>(this, DeviceUseRequestStatus.INPROGRESS); 288 if ("completed".equals(codeString)) 289 return new Enumeration<DeviceUseRequestStatus>(this, DeviceUseRequestStatus.COMPLETED); 290 if ("suspended".equals(codeString)) 291 return new Enumeration<DeviceUseRequestStatus>(this, DeviceUseRequestStatus.SUSPENDED); 292 if ("rejected".equals(codeString)) 293 return new Enumeration<DeviceUseRequestStatus>(this, DeviceUseRequestStatus.REJECTED); 294 if ("aborted".equals(codeString)) 295 return new Enumeration<DeviceUseRequestStatus>(this, DeviceUseRequestStatus.ABORTED); 296 throw new FHIRException("Unknown DeviceUseRequestStatus code '" + codeString + "'"); 297 } 298 299 public String toCode(DeviceUseRequestStatus code) 300 { 301 if (code == DeviceUseRequestStatus.NULL) 302 return null; 303 if (code == DeviceUseRequestStatus.PROPOSED) 304 return "proposed"; 305 if (code == DeviceUseRequestStatus.PLANNED) 306 return "planned"; 307 if (code == DeviceUseRequestStatus.REQUESTED) 308 return "requested"; 309 if (code == DeviceUseRequestStatus.RECEIVED) 310 return "received"; 311 if (code == DeviceUseRequestStatus.ACCEPTED) 312 return "accepted"; 313 if (code == DeviceUseRequestStatus.INPROGRESS) 314 return "in-progress"; 315 if (code == DeviceUseRequestStatus.COMPLETED) 316 return "completed"; 317 if (code == DeviceUseRequestStatus.SUSPENDED) 318 return "suspended"; 319 if (code == DeviceUseRequestStatus.REJECTED) 320 return "rejected"; 321 if (code == DeviceUseRequestStatus.ABORTED) 322 return "aborted"; 323 return "?"; 324 } 325 } 326 327 public enum DeviceUseRequestPriority { 328 /** 329 * The request has a normal priority. 330 */ 331 ROUTINE, 332 /** 333 * The request should be done urgently. 334 */ 335 URGENT, 336 /** 337 * The request is time-critical. 338 */ 339 STAT, 340 /** 341 * The request should be acted on as soon as possible. 342 */ 343 ASAP, 344 /** 345 * added to help the parsers 346 */ 347 NULL; 348 349 public static DeviceUseRequestPriority fromCode(String codeString) throws FHIRException { 350 if (codeString == null || "".equals(codeString)) 351 return null; 352 if ("routine".equals(codeString)) 353 return ROUTINE; 354 if ("urgent".equals(codeString)) 355 return URGENT; 356 if ("stat".equals(codeString)) 357 return STAT; 358 if ("asap".equals(codeString)) 359 return ASAP; 360 throw new FHIRException("Unknown DeviceUseRequestPriority code '" + codeString + "'"); 361 } 362 363 public String toCode() { 364 switch (this) { 365 case ROUTINE: 366 return "routine"; 367 case URGENT: 368 return "urgent"; 369 case STAT: 370 return "stat"; 371 case ASAP: 372 return "asap"; 373 case NULL: 374 return null; 375 default: 376 return "?"; 377 } 378 } 379 380 public String getSystem() { 381 switch (this) { 382 case ROUTINE: 383 return "http://hl7.org/fhir/device-use-request-priority"; 384 case URGENT: 385 return "http://hl7.org/fhir/device-use-request-priority"; 386 case STAT: 387 return "http://hl7.org/fhir/device-use-request-priority"; 388 case ASAP: 389 return "http://hl7.org/fhir/device-use-request-priority"; 390 case NULL: 391 return null; 392 default: 393 return "?"; 394 } 395 } 396 397 public String getDefinition() { 398 switch (this) { 399 case ROUTINE: 400 return "The request has a normal priority."; 401 case URGENT: 402 return "The request should be done urgently."; 403 case STAT: 404 return "The request is time-critical."; 405 case ASAP: 406 return "The request should be acted on as soon as possible."; 407 case NULL: 408 return null; 409 default: 410 return "?"; 411 } 412 } 413 414 public String getDisplay() { 415 switch (this) { 416 case ROUTINE: 417 return "Routine"; 418 case URGENT: 419 return "Urgent"; 420 case STAT: 421 return "Stat"; 422 case ASAP: 423 return "ASAP"; 424 case NULL: 425 return null; 426 default: 427 return "?"; 428 } 429 } 430 } 431 432 public static class DeviceUseRequestPriorityEnumFactory implements EnumFactory<DeviceUseRequestPriority> { 433 public DeviceUseRequestPriority fromCode(String codeString) throws IllegalArgumentException { 434 if (codeString == null || "".equals(codeString)) 435 if (codeString == null || "".equals(codeString)) 436 return null; 437 if ("routine".equals(codeString)) 438 return DeviceUseRequestPriority.ROUTINE; 439 if ("urgent".equals(codeString)) 440 return DeviceUseRequestPriority.URGENT; 441 if ("stat".equals(codeString)) 442 return DeviceUseRequestPriority.STAT; 443 if ("asap".equals(codeString)) 444 return DeviceUseRequestPriority.ASAP; 445 throw new IllegalArgumentException("Unknown DeviceUseRequestPriority code '" + codeString + "'"); 446 } 447 448 public Enumeration<DeviceUseRequestPriority> fromType(Base code) throws FHIRException { 449 if (code == null || code.isEmpty()) 450 return null; 451 String codeString = ((PrimitiveType) code).asStringValue(); 452 if (codeString == null || "".equals(codeString)) 453 return null; 454 if ("routine".equals(codeString)) 455 return new Enumeration<DeviceUseRequestPriority>(this, DeviceUseRequestPriority.ROUTINE); 456 if ("urgent".equals(codeString)) 457 return new Enumeration<DeviceUseRequestPriority>(this, DeviceUseRequestPriority.URGENT); 458 if ("stat".equals(codeString)) 459 return new Enumeration<DeviceUseRequestPriority>(this, DeviceUseRequestPriority.STAT); 460 if ("asap".equals(codeString)) 461 return new Enumeration<DeviceUseRequestPriority>(this, DeviceUseRequestPriority.ASAP); 462 throw new FHIRException("Unknown DeviceUseRequestPriority code '" + codeString + "'"); 463 } 464 465 public String toCode(DeviceUseRequestPriority code) 466 { 467 if (code == DeviceUseRequestPriority.NULL) 468 return null; 469 if (code == DeviceUseRequestPriority.ROUTINE) 470 return "routine"; 471 if (code == DeviceUseRequestPriority.URGENT) 472 return "urgent"; 473 if (code == DeviceUseRequestPriority.STAT) 474 return "stat"; 475 if (code == DeviceUseRequestPriority.ASAP) 476 return "asap"; 477 return "?"; 478 } 479 } 480 481 /** 482 * Indicates the site on the subject's body where the device should be used ( 483 * i.e. the target site). 484 */ 485 @Child(name = "bodySite", type = { CodeableConcept.class, 486 BodySite.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 487 @Description(shortDefinition = "Target body site", formalDefinition = "Indicates the site on the subject's body where the device should be used ( i.e. the target site).") 488 protected Type bodySite; 489 490 /** 491 * The status of the request. 492 */ 493 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 494 @Description(shortDefinition = "proposed | planned | requested | received | accepted | in-progress | completed | suspended | rejected | aborted", formalDefinition = "The status of the request.") 495 protected Enumeration<DeviceUseRequestStatus> status; 496 497 /** 498 * The details of the device to be used. 499 */ 500 @Child(name = "device", type = { Device.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 501 @Description(shortDefinition = "Device requested", formalDefinition = "The details of the device to be used.") 502 protected Reference device; 503 504 /** 505 * The actual object that is the target of the reference (The details of the 506 * device to be used.) 507 */ 508 protected Device deviceTarget; 509 510 /** 511 * An encounter that provides additional context in which this request is made. 512 */ 513 @Child(name = "encounter", type = { Encounter.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 514 @Description(shortDefinition = "Encounter motivating request", formalDefinition = "An encounter that provides additional context in which this request is made.") 515 protected Reference encounter; 516 517 /** 518 * The actual object that is the target of the reference (An encounter that 519 * provides additional context in which this request is made.) 520 */ 521 protected Encounter encounterTarget; 522 523 /** 524 * Identifiers assigned to this order by the orderer or by the receiver. 525 */ 526 @Child(name = "identifier", type = { 527 Identifier.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 528 @Description(shortDefinition = "Request identifier", formalDefinition = "Identifiers assigned to this order by the orderer or by the receiver.") 529 protected List<Identifier> identifier; 530 531 /** 532 * Reason or justification for the use of this device. 533 */ 534 @Child(name = "indication", type = { 535 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 536 @Description(shortDefinition = "Reason for request", formalDefinition = "Reason or justification for the use of this device.") 537 protected List<CodeableConcept> indication; 538 539 /** 540 * Details about this request that were not represented at all or sufficiently 541 * in one of the attributes provided in a class. These may include for example a 542 * comment, an instruction, or a note associated with the statement. 543 */ 544 @Child(name = "notes", type = { 545 StringType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 546 @Description(shortDefinition = "Notes or comments", formalDefinition = "Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.") 547 protected List<StringType> notes; 548 549 /** 550 * The proposed act must be performed if the indicated conditions occur, e.g.., 551 * shortness of breath, SpO2 less than x%. 552 */ 553 @Child(name = "prnReason", type = { 554 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 555 @Description(shortDefinition = "PRN", formalDefinition = "The proposed act must be performed if the indicated conditions occur, e.g.., shortness of breath, SpO2 less than x%.") 556 protected List<CodeableConcept> prnReason; 557 558 /** 559 * The time when the request was made. 560 */ 561 @Child(name = "orderedOn", type = { 562 DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 563 @Description(shortDefinition = "When ordered", formalDefinition = "The time when the request was made.") 564 protected DateTimeType orderedOn; 565 566 /** 567 * The time at which the request was made/recorded. 568 */ 569 @Child(name = "recordedOn", type = { 570 DateTimeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 571 @Description(shortDefinition = "When recorded", formalDefinition = "The time at which the request was made/recorded.") 572 protected DateTimeType recordedOn; 573 574 /** 575 * The patient who will use the device. 576 */ 577 @Child(name = "subject", type = { Patient.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 578 @Description(shortDefinition = "Focus of request", formalDefinition = "The patient who will use the device.") 579 protected Reference subject; 580 581 /** 582 * The actual object that is the target of the reference (The patient who will 583 * use the device.) 584 */ 585 protected Patient subjectTarget; 586 587 /** 588 * The timing schedule for the use of the device The Schedule data type allows 589 * many different expressions, for example. "Every 8 hours"; "Three times a 590 * day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 591 * 2013, 17 Oct 2013 and 1 Nov 2013". 592 */ 593 @Child(name = "timing", type = { Timing.class, Period.class, 594 DateTimeType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 595 @Description(shortDefinition = "Schedule for use", formalDefinition = "The timing schedule for the use of the device The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".") 596 protected Type timing; 597 598 /** 599 * Characterizes how quickly the use of device must be initiated. Includes 600 * concepts such as stat, urgent, routine. 601 */ 602 @Child(name = "priority", type = { CodeType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 603 @Description(shortDefinition = "routine | urgent | stat | asap", formalDefinition = "Characterizes how quickly the use of device must be initiated. Includes concepts such as stat, urgent, routine.") 604 protected Enumeration<DeviceUseRequestPriority> priority; 605 606 private static final long serialVersionUID = 1208477058L; 607 608 /* 609 * Constructor 610 */ 611 public DeviceUseRequest() { 612 super(); 613 } 614 615 /* 616 * Constructor 617 */ 618 public DeviceUseRequest(Reference device, Reference subject) { 619 super(); 620 this.device = device; 621 this.subject = subject; 622 } 623 624 /** 625 * @return {@link #bodySite} (Indicates the site on the subject's body where the 626 * device should be used ( i.e. the target site).) 627 */ 628 public Type getBodySite() { 629 return this.bodySite; 630 } 631 632 /** 633 * @return {@link #bodySite} (Indicates the site on the subject's body where the 634 * device should be used ( i.e. the target site).) 635 */ 636 public CodeableConcept getBodySiteCodeableConcept() throws FHIRException { 637 if (!(this.bodySite instanceof CodeableConcept)) 638 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 639 + this.bodySite.getClass().getName() + " was encountered"); 640 return (CodeableConcept) this.bodySite; 641 } 642 643 public boolean hasBodySiteCodeableConcept() { 644 return this.bodySite instanceof CodeableConcept; 645 } 646 647 /** 648 * @return {@link #bodySite} (Indicates the site on the subject's body where the 649 * device should be used ( i.e. the target site).) 650 */ 651 public Reference getBodySiteReference() throws FHIRException { 652 if (!(this.bodySite instanceof Reference)) 653 throw new FHIRException("Type mismatch: the type Reference was expected, but " 654 + this.bodySite.getClass().getName() + " was encountered"); 655 return (Reference) this.bodySite; 656 } 657 658 public boolean hasBodySiteReference() { 659 return this.bodySite instanceof Reference; 660 } 661 662 public boolean hasBodySite() { 663 return this.bodySite != null && !this.bodySite.isEmpty(); 664 } 665 666 /** 667 * @param value {@link #bodySite} (Indicates the site on the subject's body 668 * where the device should be used ( i.e. the target site).) 669 */ 670 public DeviceUseRequest setBodySite(Type value) { 671 this.bodySite = value; 672 return this; 673 } 674 675 /** 676 * @return {@link #status} (The status of the request.). This is the underlying 677 * object with id, value and extensions. The accessor "getStatus" gives 678 * direct access to the value 679 */ 680 public Enumeration<DeviceUseRequestStatus> getStatusElement() { 681 if (this.status == null) 682 if (Configuration.errorOnAutoCreate()) 683 throw new Error("Attempt to auto-create DeviceUseRequest.status"); 684 else if (Configuration.doAutoCreate()) 685 this.status = new Enumeration<DeviceUseRequestStatus>(new DeviceUseRequestStatusEnumFactory()); // bb 686 return this.status; 687 } 688 689 public boolean hasStatusElement() { 690 return this.status != null && !this.status.isEmpty(); 691 } 692 693 public boolean hasStatus() { 694 return this.status != null && !this.status.isEmpty(); 695 } 696 697 /** 698 * @param value {@link #status} (The status of the request.). This is the 699 * underlying object with id, value and extensions. The accessor 700 * "getStatus" gives direct access to the value 701 */ 702 public DeviceUseRequest setStatusElement(Enumeration<DeviceUseRequestStatus> value) { 703 this.status = value; 704 return this; 705 } 706 707 /** 708 * @return The status of the request. 709 */ 710 public DeviceUseRequestStatus getStatus() { 711 return this.status == null ? null : this.status.getValue(); 712 } 713 714 /** 715 * @param value The status of the request. 716 */ 717 public DeviceUseRequest setStatus(DeviceUseRequestStatus value) { 718 if (value == null) 719 this.status = null; 720 else { 721 if (this.status == null) 722 this.status = new Enumeration<DeviceUseRequestStatus>(new DeviceUseRequestStatusEnumFactory()); 723 this.status.setValue(value); 724 } 725 return this; 726 } 727 728 /** 729 * @return {@link #device} (The details of the device to be used.) 730 */ 731 public Reference getDevice() { 732 if (this.device == null) 733 if (Configuration.errorOnAutoCreate()) 734 throw new Error("Attempt to auto-create DeviceUseRequest.device"); 735 else if (Configuration.doAutoCreate()) 736 this.device = new Reference(); // cc 737 return this.device; 738 } 739 740 public boolean hasDevice() { 741 return this.device != null && !this.device.isEmpty(); 742 } 743 744 /** 745 * @param value {@link #device} (The details of the device to be used.) 746 */ 747 public DeviceUseRequest setDevice(Reference value) { 748 this.device = value; 749 return this; 750 } 751 752 /** 753 * @return {@link #device} The actual object that is the target of the 754 * reference. The reference library doesn't populate this, but you can 755 * use it to hold the resource if you resolve it. (The details of the 756 * device to be used.) 757 */ 758 public Device getDeviceTarget() { 759 if (this.deviceTarget == null) 760 if (Configuration.errorOnAutoCreate()) 761 throw new Error("Attempt to auto-create DeviceUseRequest.device"); 762 else if (Configuration.doAutoCreate()) 763 this.deviceTarget = new Device(); // aa 764 return this.deviceTarget; 765 } 766 767 /** 768 * @param value {@link #device} The actual object that is the target of the 769 * reference. The reference library doesn't use these, but you can 770 * use it to hold the resource if you resolve it. (The details of 771 * the device to be used.) 772 */ 773 public DeviceUseRequest setDeviceTarget(Device value) { 774 this.deviceTarget = value; 775 return this; 776 } 777 778 /** 779 * @return {@link #encounter} (An encounter that provides additional context in 780 * which this request is made.) 781 */ 782 public Reference getEncounter() { 783 if (this.encounter == null) 784 if (Configuration.errorOnAutoCreate()) 785 throw new Error("Attempt to auto-create DeviceUseRequest.encounter"); 786 else if (Configuration.doAutoCreate()) 787 this.encounter = new Reference(); // cc 788 return this.encounter; 789 } 790 791 public boolean hasEncounter() { 792 return this.encounter != null && !this.encounter.isEmpty(); 793 } 794 795 /** 796 * @param value {@link #encounter} (An encounter that provides additional 797 * context in which this request is made.) 798 */ 799 public DeviceUseRequest setEncounter(Reference value) { 800 this.encounter = value; 801 return this; 802 } 803 804 /** 805 * @return {@link #encounter} The actual object that is the target of the 806 * reference. The reference library doesn't populate this, but you can 807 * use it to hold the resource if you resolve it. (An encounter that 808 * provides additional context in which this request is made.) 809 */ 810 public Encounter getEncounterTarget() { 811 if (this.encounterTarget == null) 812 if (Configuration.errorOnAutoCreate()) 813 throw new Error("Attempt to auto-create DeviceUseRequest.encounter"); 814 else if (Configuration.doAutoCreate()) 815 this.encounterTarget = new Encounter(); // aa 816 return this.encounterTarget; 817 } 818 819 /** 820 * @param value {@link #encounter} The actual object that is the target of the 821 * reference. The reference library doesn't use these, but you can 822 * use it to hold the resource if you resolve it. (An encounter 823 * that provides additional context in which this request is made.) 824 */ 825 public DeviceUseRequest setEncounterTarget(Encounter value) { 826 this.encounterTarget = value; 827 return this; 828 } 829 830 /** 831 * @return {@link #identifier} (Identifiers assigned to this order by the 832 * orderer or by the receiver.) 833 */ 834 public List<Identifier> getIdentifier() { 835 if (this.identifier == null) 836 this.identifier = new ArrayList<Identifier>(); 837 return this.identifier; 838 } 839 840 public boolean hasIdentifier() { 841 if (this.identifier == null) 842 return false; 843 for (Identifier item : this.identifier) 844 if (!item.isEmpty()) 845 return true; 846 return false; 847 } 848 849 /** 850 * @return {@link #identifier} (Identifiers assigned to this order by the 851 * orderer or by the receiver.) 852 */ 853 // syntactic sugar 854 public Identifier addIdentifier() { // 3 855 Identifier t = new Identifier(); 856 if (this.identifier == null) 857 this.identifier = new ArrayList<Identifier>(); 858 this.identifier.add(t); 859 return t; 860 } 861 862 // syntactic sugar 863 public DeviceUseRequest addIdentifier(Identifier t) { // 3 864 if (t == null) 865 return this; 866 if (this.identifier == null) 867 this.identifier = new ArrayList<Identifier>(); 868 this.identifier.add(t); 869 return this; 870 } 871 872 /** 873 * @return {@link #indication} (Reason or justification for the use of this 874 * device.) 875 */ 876 public List<CodeableConcept> getIndication() { 877 if (this.indication == null) 878 this.indication = new ArrayList<CodeableConcept>(); 879 return this.indication; 880 } 881 882 public boolean hasIndication() { 883 if (this.indication == null) 884 return false; 885 for (CodeableConcept item : this.indication) 886 if (!item.isEmpty()) 887 return true; 888 return false; 889 } 890 891 /** 892 * @return {@link #indication} (Reason or justification for the use of this 893 * device.) 894 */ 895 // syntactic sugar 896 public CodeableConcept addIndication() { // 3 897 CodeableConcept t = new CodeableConcept(); 898 if (this.indication == null) 899 this.indication = new ArrayList<CodeableConcept>(); 900 this.indication.add(t); 901 return t; 902 } 903 904 // syntactic sugar 905 public DeviceUseRequest addIndication(CodeableConcept t) { // 3 906 if (t == null) 907 return this; 908 if (this.indication == null) 909 this.indication = new ArrayList<CodeableConcept>(); 910 this.indication.add(t); 911 return this; 912 } 913 914 /** 915 * @return {@link #notes} (Details about this request that were not represented 916 * at all or sufficiently in one of the attributes provided in a class. 917 * These may include for example a comment, an instruction, or a note 918 * associated with the statement.) 919 */ 920 public List<StringType> getNotes() { 921 if (this.notes == null) 922 this.notes = new ArrayList<StringType>(); 923 return this.notes; 924 } 925 926 public boolean hasNotes() { 927 if (this.notes == null) 928 return false; 929 for (StringType item : this.notes) 930 if (!item.isEmpty()) 931 return true; 932 return false; 933 } 934 935 /** 936 * @return {@link #notes} (Details about this request that were not represented 937 * at all or sufficiently in one of the attributes provided in a class. 938 * These may include for example a comment, an instruction, or a note 939 * associated with the statement.) 940 */ 941 // syntactic sugar 942 public StringType addNotesElement() {// 2 943 StringType t = new StringType(); 944 if (this.notes == null) 945 this.notes = new ArrayList<StringType>(); 946 this.notes.add(t); 947 return t; 948 } 949 950 /** 951 * @param value {@link #notes} (Details about this request that were not 952 * represented at all or sufficiently in one of the attributes 953 * provided in a class. These may include for example a comment, an 954 * instruction, or a note associated with the statement.) 955 */ 956 public DeviceUseRequest addNotes(String value) { // 1 957 StringType t = new StringType(); 958 t.setValue(value); 959 if (this.notes == null) 960 this.notes = new ArrayList<StringType>(); 961 this.notes.add(t); 962 return this; 963 } 964 965 /** 966 * @param value {@link #notes} (Details about this request that were not 967 * represented at all or sufficiently in one of the attributes 968 * provided in a class. These may include for example a comment, an 969 * instruction, or a note associated with the statement.) 970 */ 971 public boolean hasNotes(String value) { 972 if (this.notes == null) 973 return false; 974 for (StringType v : this.notes) 975 if (v.equals(value)) // string 976 return true; 977 return false; 978 } 979 980 /** 981 * @return {@link #prnReason} (The proposed act must be performed if the 982 * indicated conditions occur, e.g.., shortness of breath, SpO2 less 983 * than x%.) 984 */ 985 public List<CodeableConcept> getPrnReason() { 986 if (this.prnReason == null) 987 this.prnReason = new ArrayList<CodeableConcept>(); 988 return this.prnReason; 989 } 990 991 public boolean hasPrnReason() { 992 if (this.prnReason == null) 993 return false; 994 for (CodeableConcept item : this.prnReason) 995 if (!item.isEmpty()) 996 return true; 997 return false; 998 } 999 1000 /** 1001 * @return {@link #prnReason} (The proposed act must be performed if the 1002 * indicated conditions occur, e.g.., shortness of breath, SpO2 less 1003 * than x%.) 1004 */ 1005 // syntactic sugar 1006 public CodeableConcept addPrnReason() { // 3 1007 CodeableConcept t = new CodeableConcept(); 1008 if (this.prnReason == null) 1009 this.prnReason = new ArrayList<CodeableConcept>(); 1010 this.prnReason.add(t); 1011 return t; 1012 } 1013 1014 // syntactic sugar 1015 public DeviceUseRequest addPrnReason(CodeableConcept t) { // 3 1016 if (t == null) 1017 return this; 1018 if (this.prnReason == null) 1019 this.prnReason = new ArrayList<CodeableConcept>(); 1020 this.prnReason.add(t); 1021 return this; 1022 } 1023 1024 /** 1025 * @return {@link #orderedOn} (The time when the request was made.). This is the 1026 * underlying object with id, value and extensions. The accessor 1027 * "getOrderedOn" gives direct access to the value 1028 */ 1029 public DateTimeType getOrderedOnElement() { 1030 if (this.orderedOn == null) 1031 if (Configuration.errorOnAutoCreate()) 1032 throw new Error("Attempt to auto-create DeviceUseRequest.orderedOn"); 1033 else if (Configuration.doAutoCreate()) 1034 this.orderedOn = new DateTimeType(); // bb 1035 return this.orderedOn; 1036 } 1037 1038 public boolean hasOrderedOnElement() { 1039 return this.orderedOn != null && !this.orderedOn.isEmpty(); 1040 } 1041 1042 public boolean hasOrderedOn() { 1043 return this.orderedOn != null && !this.orderedOn.isEmpty(); 1044 } 1045 1046 /** 1047 * @param value {@link #orderedOn} (The time when the request was made.). This 1048 * is the underlying object with id, value and extensions. The 1049 * accessor "getOrderedOn" gives direct access to the value 1050 */ 1051 public DeviceUseRequest setOrderedOnElement(DateTimeType value) { 1052 this.orderedOn = value; 1053 return this; 1054 } 1055 1056 /** 1057 * @return The time when the request was made. 1058 */ 1059 public Date getOrderedOn() { 1060 return this.orderedOn == null ? null : this.orderedOn.getValue(); 1061 } 1062 1063 /** 1064 * @param value The time when the request was made. 1065 */ 1066 public DeviceUseRequest setOrderedOn(Date value) { 1067 if (value == null) 1068 this.orderedOn = null; 1069 else { 1070 if (this.orderedOn == null) 1071 this.orderedOn = new DateTimeType(); 1072 this.orderedOn.setValue(value); 1073 } 1074 return this; 1075 } 1076 1077 /** 1078 * @return {@link #recordedOn} (The time at which the request was 1079 * made/recorded.). This is the underlying object with id, value and 1080 * extensions. The accessor "getRecordedOn" gives direct access to the 1081 * value 1082 */ 1083 public DateTimeType getRecordedOnElement() { 1084 if (this.recordedOn == null) 1085 if (Configuration.errorOnAutoCreate()) 1086 throw new Error("Attempt to auto-create DeviceUseRequest.recordedOn"); 1087 else if (Configuration.doAutoCreate()) 1088 this.recordedOn = new DateTimeType(); // bb 1089 return this.recordedOn; 1090 } 1091 1092 public boolean hasRecordedOnElement() { 1093 return this.recordedOn != null && !this.recordedOn.isEmpty(); 1094 } 1095 1096 public boolean hasRecordedOn() { 1097 return this.recordedOn != null && !this.recordedOn.isEmpty(); 1098 } 1099 1100 /** 1101 * @param value {@link #recordedOn} (The time at which the request was 1102 * made/recorded.). This is the underlying object with id, value 1103 * and extensions. The accessor "getRecordedOn" gives direct access 1104 * to the value 1105 */ 1106 public DeviceUseRequest setRecordedOnElement(DateTimeType value) { 1107 this.recordedOn = value; 1108 return this; 1109 } 1110 1111 /** 1112 * @return The time at which the request was made/recorded. 1113 */ 1114 public Date getRecordedOn() { 1115 return this.recordedOn == null ? null : this.recordedOn.getValue(); 1116 } 1117 1118 /** 1119 * @param value The time at which the request was made/recorded. 1120 */ 1121 public DeviceUseRequest setRecordedOn(Date value) { 1122 if (value == null) 1123 this.recordedOn = null; 1124 else { 1125 if (this.recordedOn == null) 1126 this.recordedOn = new DateTimeType(); 1127 this.recordedOn.setValue(value); 1128 } 1129 return this; 1130 } 1131 1132 /** 1133 * @return {@link #subject} (The patient who will use the device.) 1134 */ 1135 public Reference getSubject() { 1136 if (this.subject == null) 1137 if (Configuration.errorOnAutoCreate()) 1138 throw new Error("Attempt to auto-create DeviceUseRequest.subject"); 1139 else if (Configuration.doAutoCreate()) 1140 this.subject = new Reference(); // cc 1141 return this.subject; 1142 } 1143 1144 public boolean hasSubject() { 1145 return this.subject != null && !this.subject.isEmpty(); 1146 } 1147 1148 /** 1149 * @param value {@link #subject} (The patient who will use the device.) 1150 */ 1151 public DeviceUseRequest setSubject(Reference value) { 1152 this.subject = value; 1153 return this; 1154 } 1155 1156 /** 1157 * @return {@link #subject} The actual object that is the target of the 1158 * reference. The reference library doesn't populate this, but you can 1159 * use it to hold the resource if you resolve it. (The patient who will 1160 * use the device.) 1161 */ 1162 public Patient getSubjectTarget() { 1163 if (this.subjectTarget == null) 1164 if (Configuration.errorOnAutoCreate()) 1165 throw new Error("Attempt to auto-create DeviceUseRequest.subject"); 1166 else if (Configuration.doAutoCreate()) 1167 this.subjectTarget = new Patient(); // aa 1168 return this.subjectTarget; 1169 } 1170 1171 /** 1172 * @param value {@link #subject} The actual object that is the target of the 1173 * reference. The reference library doesn't use these, but you can 1174 * use it to hold the resource if you resolve it. (The patient who 1175 * will use the device.) 1176 */ 1177 public DeviceUseRequest setSubjectTarget(Patient value) { 1178 this.subjectTarget = value; 1179 return this; 1180 } 1181 1182 /** 1183 * @return {@link #timing} (The timing schedule for the use of the device The 1184 * Schedule data type allows many different expressions, for example. 1185 * "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast 1186 * for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 1187 * 2013".) 1188 */ 1189 public Type getTiming() { 1190 return this.timing; 1191 } 1192 1193 /** 1194 * @return {@link #timing} (The timing schedule for the use of the device The 1195 * Schedule data type allows many different expressions, for example. 1196 * "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast 1197 * for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 1198 * 2013".) 1199 */ 1200 public Timing getTimingTiming() throws FHIRException { 1201 if (!(this.timing instanceof Timing)) 1202 throw new FHIRException( 1203 "Type mismatch: the type Timing was expected, but " + this.timing.getClass().getName() + " was encountered"); 1204 return (Timing) this.timing; 1205 } 1206 1207 public boolean hasTimingTiming() { 1208 return this.timing instanceof Timing; 1209 } 1210 1211 /** 1212 * @return {@link #timing} (The timing schedule for the use of the device The 1213 * Schedule data type allows many different expressions, for example. 1214 * "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast 1215 * for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 1216 * 2013".) 1217 */ 1218 public Period getTimingPeriod() throws FHIRException { 1219 if (!(this.timing instanceof Period)) 1220 throw new FHIRException( 1221 "Type mismatch: the type Period was expected, but " + this.timing.getClass().getName() + " was encountered"); 1222 return (Period) this.timing; 1223 } 1224 1225 public boolean hasTimingPeriod() { 1226 return this.timing instanceof Period; 1227 } 1228 1229 /** 1230 * @return {@link #timing} (The timing schedule for the use of the device The 1231 * Schedule data type allows many different expressions, for example. 1232 * "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast 1233 * for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 1234 * 2013".) 1235 */ 1236 public DateTimeType getTimingDateTimeType() throws FHIRException { 1237 if (!(this.timing instanceof DateTimeType)) 1238 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1239 + this.timing.getClass().getName() + " was encountered"); 1240 return (DateTimeType) this.timing; 1241 } 1242 1243 public boolean hasTimingDateTimeType() { 1244 return this.timing instanceof DateTimeType; 1245 } 1246 1247 public boolean hasTiming() { 1248 return this.timing != null && !this.timing.isEmpty(); 1249 } 1250 1251 /** 1252 * @param value {@link #timing} (The timing schedule for the use of the device 1253 * The Schedule data type allows many different expressions, for 1254 * example. "Every 8 hours"; "Three times a day"; "1/2 an hour 1255 * before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 1256 * 17 Oct 2013 and 1 Nov 2013".) 1257 */ 1258 public DeviceUseRequest setTiming(Type value) { 1259 this.timing = value; 1260 return this; 1261 } 1262 1263 /** 1264 * @return {@link #priority} (Characterizes how quickly the use of device must 1265 * be initiated. Includes concepts such as stat, urgent, routine.). This 1266 * is the underlying object with id, value and extensions. The accessor 1267 * "getPriority" gives direct access to the value 1268 */ 1269 public Enumeration<DeviceUseRequestPriority> getPriorityElement() { 1270 if (this.priority == null) 1271 if (Configuration.errorOnAutoCreate()) 1272 throw new Error("Attempt to auto-create DeviceUseRequest.priority"); 1273 else if (Configuration.doAutoCreate()) 1274 this.priority = new Enumeration<DeviceUseRequestPriority>(new DeviceUseRequestPriorityEnumFactory()); // bb 1275 return this.priority; 1276 } 1277 1278 public boolean hasPriorityElement() { 1279 return this.priority != null && !this.priority.isEmpty(); 1280 } 1281 1282 public boolean hasPriority() { 1283 return this.priority != null && !this.priority.isEmpty(); 1284 } 1285 1286 /** 1287 * @param value {@link #priority} (Characterizes how quickly the use of device 1288 * must be initiated. Includes concepts such as stat, urgent, 1289 * routine.). This is the underlying object with id, value and 1290 * extensions. The accessor "getPriority" gives direct access to 1291 * the value 1292 */ 1293 public DeviceUseRequest setPriorityElement(Enumeration<DeviceUseRequestPriority> value) { 1294 this.priority = value; 1295 return this; 1296 } 1297 1298 /** 1299 * @return Characterizes how quickly the use of device must be initiated. 1300 * Includes concepts such as stat, urgent, routine. 1301 */ 1302 public DeviceUseRequestPriority getPriority() { 1303 return this.priority == null ? null : this.priority.getValue(); 1304 } 1305 1306 /** 1307 * @param value Characterizes how quickly the use of device must be initiated. 1308 * Includes concepts such as stat, urgent, routine. 1309 */ 1310 public DeviceUseRequest setPriority(DeviceUseRequestPriority value) { 1311 if (value == null) 1312 this.priority = null; 1313 else { 1314 if (this.priority == null) 1315 this.priority = new Enumeration<DeviceUseRequestPriority>(new DeviceUseRequestPriorityEnumFactory()); 1316 this.priority.setValue(value); 1317 } 1318 return this; 1319 } 1320 1321 protected void listChildren(List<Property> childrenList) { 1322 super.listChildren(childrenList); 1323 childrenList.add(new Property("bodySite[x]", "CodeableConcept|Reference(BodySite)", 1324 "Indicates the site on the subject's body where the device should be used ( i.e. the target site).", 0, 1325 java.lang.Integer.MAX_VALUE, bodySite)); 1326 childrenList 1327 .add(new Property("status", "code", "The status of the request.", 0, java.lang.Integer.MAX_VALUE, status)); 1328 childrenList.add(new Property("device", "Reference(Device)", "The details of the device to be used.", 0, 1329 java.lang.Integer.MAX_VALUE, device)); 1330 childrenList.add(new Property("encounter", "Reference(Encounter)", 1331 "An encounter that provides additional context in which this request is made.", 0, java.lang.Integer.MAX_VALUE, 1332 encounter)); 1333 childrenList.add(new Property("identifier", "Identifier", 1334 "Identifiers assigned to this order by the orderer or by the receiver.", 0, java.lang.Integer.MAX_VALUE, 1335 identifier)); 1336 childrenList.add(new Property("indication", "CodeableConcept", 1337 "Reason or justification for the use of this device.", 0, java.lang.Integer.MAX_VALUE, indication)); 1338 childrenList.add(new Property("notes", "string", 1339 "Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 1340 0, java.lang.Integer.MAX_VALUE, notes)); 1341 childrenList.add(new Property("prnReason", "CodeableConcept", 1342 "The proposed act must be performed if the indicated conditions occur, e.g.., shortness of breath, SpO2 less than x%.", 1343 0, java.lang.Integer.MAX_VALUE, prnReason)); 1344 childrenList.add(new Property("orderedOn", "dateTime", "The time when the request was made.", 0, 1345 java.lang.Integer.MAX_VALUE, orderedOn)); 1346 childrenList.add(new Property("recordedOn", "dateTime", "The time at which the request was made/recorded.", 0, 1347 java.lang.Integer.MAX_VALUE, recordedOn)); 1348 childrenList.add(new Property("subject", "Reference(Patient)", "The patient who will use the device.", 0, 1349 java.lang.Integer.MAX_VALUE, subject)); 1350 childrenList.add(new Property("timing[x]", "Timing|Period|dateTime", 1351 "The timing schedule for the use of the device The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 1352 0, java.lang.Integer.MAX_VALUE, timing)); 1353 childrenList.add(new Property("priority", "code", 1354 "Characterizes how quickly the use of device must be initiated. Includes concepts such as stat, urgent, routine.", 1355 0, java.lang.Integer.MAX_VALUE, priority)); 1356 } 1357 1358 @Override 1359 public void setProperty(String name, Base value) throws FHIRException { 1360 if (name.equals("bodySite[x]")) 1361 this.bodySite = (Type) value; // Type 1362 else if (name.equals("status")) 1363 this.status = new DeviceUseRequestStatusEnumFactory().fromType(value); // Enumeration<DeviceUseRequestStatus> 1364 else if (name.equals("device")) 1365 this.device = castToReference(value); // Reference 1366 else if (name.equals("encounter")) 1367 this.encounter = castToReference(value); // Reference 1368 else if (name.equals("identifier")) 1369 this.getIdentifier().add(castToIdentifier(value)); 1370 else if (name.equals("indication")) 1371 this.getIndication().add(castToCodeableConcept(value)); 1372 else if (name.equals("notes")) 1373 this.getNotes().add(castToString(value)); 1374 else if (name.equals("prnReason")) 1375 this.getPrnReason().add(castToCodeableConcept(value)); 1376 else if (name.equals("orderedOn")) 1377 this.orderedOn = castToDateTime(value); // DateTimeType 1378 else if (name.equals("recordedOn")) 1379 this.recordedOn = castToDateTime(value); // DateTimeType 1380 else if (name.equals("subject")) 1381 this.subject = castToReference(value); // Reference 1382 else if (name.equals("timing[x]")) 1383 this.timing = (Type) value; // Type 1384 else if (name.equals("priority")) 1385 this.priority = new DeviceUseRequestPriorityEnumFactory().fromType(value); // Enumeration<DeviceUseRequestPriority> 1386 else 1387 super.setProperty(name, value); 1388 } 1389 1390 @Override 1391 public Base addChild(String name) throws FHIRException { 1392 if (name.equals("bodySiteCodeableConcept")) { 1393 this.bodySite = new CodeableConcept(); 1394 return this.bodySite; 1395 } else if (name.equals("bodySiteReference")) { 1396 this.bodySite = new Reference(); 1397 return this.bodySite; 1398 } else if (name.equals("status")) { 1399 throw new FHIRException("Cannot call addChild on a singleton property DeviceUseRequest.status"); 1400 } else if (name.equals("device")) { 1401 this.device = new Reference(); 1402 return this.device; 1403 } else if (name.equals("encounter")) { 1404 this.encounter = new Reference(); 1405 return this.encounter; 1406 } else if (name.equals("identifier")) { 1407 return addIdentifier(); 1408 } else if (name.equals("indication")) { 1409 return addIndication(); 1410 } else if (name.equals("notes")) { 1411 throw new FHIRException("Cannot call addChild on a singleton property DeviceUseRequest.notes"); 1412 } else if (name.equals("prnReason")) { 1413 return addPrnReason(); 1414 } else if (name.equals("orderedOn")) { 1415 throw new FHIRException("Cannot call addChild on a singleton property DeviceUseRequest.orderedOn"); 1416 } else if (name.equals("recordedOn")) { 1417 throw new FHIRException("Cannot call addChild on a singleton property DeviceUseRequest.recordedOn"); 1418 } else if (name.equals("subject")) { 1419 this.subject = new Reference(); 1420 return this.subject; 1421 } else if (name.equals("timingTiming")) { 1422 this.timing = new Timing(); 1423 return this.timing; 1424 } else if (name.equals("timingPeriod")) { 1425 this.timing = new Period(); 1426 return this.timing; 1427 } else if (name.equals("timingDateTime")) { 1428 this.timing = new DateTimeType(); 1429 return this.timing; 1430 } else if (name.equals("priority")) { 1431 throw new FHIRException("Cannot call addChild on a singleton property DeviceUseRequest.priority"); 1432 } else 1433 return super.addChild(name); 1434 } 1435 1436 public String fhirType() { 1437 return "DeviceUseRequest"; 1438 1439 } 1440 1441 public DeviceUseRequest copy() { 1442 DeviceUseRequest dst = new DeviceUseRequest(); 1443 copyValues(dst); 1444 dst.bodySite = bodySite == null ? null : bodySite.copy(); 1445 dst.status = status == null ? null : status.copy(); 1446 dst.device = device == null ? null : device.copy(); 1447 dst.encounter = encounter == null ? null : encounter.copy(); 1448 if (identifier != null) { 1449 dst.identifier = new ArrayList<Identifier>(); 1450 for (Identifier i : identifier) 1451 dst.identifier.add(i.copy()); 1452 } 1453 ; 1454 if (indication != null) { 1455 dst.indication = new ArrayList<CodeableConcept>(); 1456 for (CodeableConcept i : indication) 1457 dst.indication.add(i.copy()); 1458 } 1459 ; 1460 if (notes != null) { 1461 dst.notes = new ArrayList<StringType>(); 1462 for (StringType i : notes) 1463 dst.notes.add(i.copy()); 1464 } 1465 ; 1466 if (prnReason != null) { 1467 dst.prnReason = new ArrayList<CodeableConcept>(); 1468 for (CodeableConcept i : prnReason) 1469 dst.prnReason.add(i.copy()); 1470 } 1471 ; 1472 dst.orderedOn = orderedOn == null ? null : orderedOn.copy(); 1473 dst.recordedOn = recordedOn == null ? null : recordedOn.copy(); 1474 dst.subject = subject == null ? null : subject.copy(); 1475 dst.timing = timing == null ? null : timing.copy(); 1476 dst.priority = priority == null ? null : priority.copy(); 1477 return dst; 1478 } 1479 1480 protected DeviceUseRequest typedCopy() { 1481 return copy(); 1482 } 1483 1484 @Override 1485 public boolean equalsDeep(Base other) { 1486 if (!super.equalsDeep(other)) 1487 return false; 1488 if (!(other instanceof DeviceUseRequest)) 1489 return false; 1490 DeviceUseRequest o = (DeviceUseRequest) other; 1491 return compareDeep(bodySite, o.bodySite, true) && compareDeep(status, o.status, true) 1492 && compareDeep(device, o.device, true) && compareDeep(encounter, o.encounter, true) 1493 && compareDeep(identifier, o.identifier, true) && compareDeep(indication, o.indication, true) 1494 && compareDeep(notes, o.notes, true) && compareDeep(prnReason, o.prnReason, true) 1495 && compareDeep(orderedOn, o.orderedOn, true) && compareDeep(recordedOn, o.recordedOn, true) 1496 && compareDeep(subject, o.subject, true) && compareDeep(timing, o.timing, true) 1497 && compareDeep(priority, o.priority, true); 1498 } 1499 1500 @Override 1501 public boolean equalsShallow(Base other) { 1502 if (!super.equalsShallow(other)) 1503 return false; 1504 if (!(other instanceof DeviceUseRequest)) 1505 return false; 1506 DeviceUseRequest o = (DeviceUseRequest) other; 1507 return compareValues(status, o.status, true) && compareValues(notes, o.notes, true) 1508 && compareValues(orderedOn, o.orderedOn, true) && compareValues(recordedOn, o.recordedOn, true) 1509 && compareValues(priority, o.priority, true); 1510 } 1511 1512 public boolean isEmpty() { 1513 return super.isEmpty() && (bodySite == null || bodySite.isEmpty()) && (status == null || status.isEmpty()) 1514 && (device == null || device.isEmpty()) && (encounter == null || encounter.isEmpty()) 1515 && (identifier == null || identifier.isEmpty()) && (indication == null || indication.isEmpty()) 1516 && (notes == null || notes.isEmpty()) && (prnReason == null || prnReason.isEmpty()) 1517 && (orderedOn == null || orderedOn.isEmpty()) && (recordedOn == null || recordedOn.isEmpty()) 1518 && (subject == null || subject.isEmpty()) && (timing == null || timing.isEmpty()) 1519 && (priority == null || priority.isEmpty()); 1520 } 1521 1522 @Override 1523 public ResourceType getResourceType() { 1524 return ResourceType.DeviceUseRequest; 1525 } 1526 1527 @SearchParamDefinition(name = "subject", path = "DeviceUseRequest.subject", description = "Search by subject", type = "reference") 1528 public static final String SP_SUBJECT = "subject"; 1529 @SearchParamDefinition(name = "patient", path = "DeviceUseRequest.subject", description = "Search by subject - a patient", type = "reference") 1530 public static final String SP_PATIENT = "patient"; 1531 @SearchParamDefinition(name = "device", path = "DeviceUseRequest.device", description = "Device requested", type = "reference") 1532 public static final String SP_DEVICE = "device"; 1533 1534}