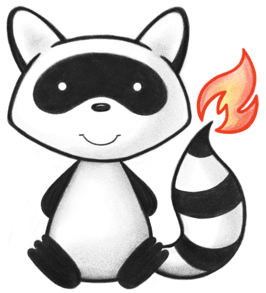
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.exceptions.FHIRException; 042 043/** 044 * A record of a device being used by a patient where the record is the result 045 * of a report from the patient or another clinician. 046 */ 047@ResourceDef(name = "DeviceUseStatement", profile = "http://hl7.org/fhir/Profile/DeviceUseStatement") 048public class DeviceUseStatement extends DomainResource { 049 050 /** 051 * Indicates the site on the subject's body where the device was used ( i.e. the 052 * target site). 053 */ 054 @Child(name = "bodySite", type = { CodeableConcept.class, 055 BodySite.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 056 @Description(shortDefinition = "Target body site", formalDefinition = "Indicates the site on the subject's body where the device was used ( i.e. the target site).") 057 protected Type bodySite; 058 059 /** 060 * The time period over which the device was used. 061 */ 062 @Child(name = "whenUsed", type = { Period.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 063 @Description(shortDefinition = "", formalDefinition = "The time period over which the device was used.") 064 protected Period whenUsed; 065 066 /** 067 * The details of the device used. 068 */ 069 @Child(name = "device", type = { Device.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 070 @Description(shortDefinition = "", formalDefinition = "The details of the device used.") 071 protected Reference device; 072 073 /** 074 * The actual object that is the target of the reference (The details of the 075 * device used.) 076 */ 077 protected Device deviceTarget; 078 079 /** 080 * An external identifier for this statement such as an IRI. 081 */ 082 @Child(name = "identifier", type = { 083 Identifier.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 084 @Description(shortDefinition = "", formalDefinition = "An external identifier for this statement such as an IRI.") 085 protected List<Identifier> identifier; 086 087 /** 088 * Reason or justification for the use of the device. 089 */ 090 @Child(name = "indication", type = { 091 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 092 @Description(shortDefinition = "", formalDefinition = "Reason or justification for the use of the device.") 093 protected List<CodeableConcept> indication; 094 095 /** 096 * Details about the device statement that were not represented at all or 097 * sufficiently in one of the attributes provided in a class. These may include 098 * for example a comment, an instruction, or a note associated with the 099 * statement. 100 */ 101 @Child(name = "notes", type = { 102 StringType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 103 @Description(shortDefinition = "", formalDefinition = "Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.") 104 protected List<StringType> notes; 105 106 /** 107 * The time at which the statement was made/recorded. 108 */ 109 @Child(name = "recordedOn", type = { 110 DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 111 @Description(shortDefinition = "", formalDefinition = "The time at which the statement was made/recorded.") 112 protected DateTimeType recordedOn; 113 114 /** 115 * The patient who used the device. 116 */ 117 @Child(name = "subject", type = { Patient.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 118 @Description(shortDefinition = "", formalDefinition = "The patient who used the device.") 119 protected Reference subject; 120 121 /** 122 * The actual object that is the target of the reference (The patient who used 123 * the device.) 124 */ 125 protected Patient subjectTarget; 126 127 /** 128 * How often the device was used. 129 */ 130 @Child(name = "timing", type = { Timing.class, Period.class, 131 DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 132 @Description(shortDefinition = "", formalDefinition = "How often the device was used.") 133 protected Type timing; 134 135 private static final long serialVersionUID = -1668571635L; 136 137 /* 138 * Constructor 139 */ 140 public DeviceUseStatement() { 141 super(); 142 } 143 144 /* 145 * Constructor 146 */ 147 public DeviceUseStatement(Reference device, Reference subject) { 148 super(); 149 this.device = device; 150 this.subject = subject; 151 } 152 153 /** 154 * @return {@link #bodySite} (Indicates the site on the subject's body where the 155 * device was used ( i.e. the target site).) 156 */ 157 public Type getBodySite() { 158 return this.bodySite; 159 } 160 161 /** 162 * @return {@link #bodySite} (Indicates the site on the subject's body where the 163 * device was used ( i.e. the target site).) 164 */ 165 public CodeableConcept getBodySiteCodeableConcept() throws FHIRException { 166 if (!(this.bodySite instanceof CodeableConcept)) 167 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 168 + this.bodySite.getClass().getName() + " was encountered"); 169 return (CodeableConcept) this.bodySite; 170 } 171 172 public boolean hasBodySiteCodeableConcept() { 173 return this.bodySite instanceof CodeableConcept; 174 } 175 176 /** 177 * @return {@link #bodySite} (Indicates the site on the subject's body where the 178 * device was used ( i.e. the target site).) 179 */ 180 public Reference getBodySiteReference() throws FHIRException { 181 if (!(this.bodySite instanceof Reference)) 182 throw new FHIRException("Type mismatch: the type Reference was expected, but " 183 + this.bodySite.getClass().getName() + " was encountered"); 184 return (Reference) this.bodySite; 185 } 186 187 public boolean hasBodySiteReference() { 188 return this.bodySite instanceof Reference; 189 } 190 191 public boolean hasBodySite() { 192 return this.bodySite != null && !this.bodySite.isEmpty(); 193 } 194 195 /** 196 * @param value {@link #bodySite} (Indicates the site on the subject's body 197 * where the device was used ( i.e. the target site).) 198 */ 199 public DeviceUseStatement setBodySite(Type value) { 200 this.bodySite = value; 201 return this; 202 } 203 204 /** 205 * @return {@link #whenUsed} (The time period over which the device was used.) 206 */ 207 public Period getWhenUsed() { 208 if (this.whenUsed == null) 209 if (Configuration.errorOnAutoCreate()) 210 throw new Error("Attempt to auto-create DeviceUseStatement.whenUsed"); 211 else if (Configuration.doAutoCreate()) 212 this.whenUsed = new Period(); // cc 213 return this.whenUsed; 214 } 215 216 public boolean hasWhenUsed() { 217 return this.whenUsed != null && !this.whenUsed.isEmpty(); 218 } 219 220 /** 221 * @param value {@link #whenUsed} (The time period over which the device was 222 * used.) 223 */ 224 public DeviceUseStatement setWhenUsed(Period value) { 225 this.whenUsed = value; 226 return this; 227 } 228 229 /** 230 * @return {@link #device} (The details of the device used.) 231 */ 232 public Reference getDevice() { 233 if (this.device == null) 234 if (Configuration.errorOnAutoCreate()) 235 throw new Error("Attempt to auto-create DeviceUseStatement.device"); 236 else if (Configuration.doAutoCreate()) 237 this.device = new Reference(); // cc 238 return this.device; 239 } 240 241 public boolean hasDevice() { 242 return this.device != null && !this.device.isEmpty(); 243 } 244 245 /** 246 * @param value {@link #device} (The details of the device used.) 247 */ 248 public DeviceUseStatement setDevice(Reference value) { 249 this.device = value; 250 return this; 251 } 252 253 /** 254 * @return {@link #device} The actual object that is the target of the 255 * reference. The reference library doesn't populate this, but you can 256 * use it to hold the resource if you resolve it. (The details of the 257 * device used.) 258 */ 259 public Device getDeviceTarget() { 260 if (this.deviceTarget == null) 261 if (Configuration.errorOnAutoCreate()) 262 throw new Error("Attempt to auto-create DeviceUseStatement.device"); 263 else if (Configuration.doAutoCreate()) 264 this.deviceTarget = new Device(); // aa 265 return this.deviceTarget; 266 } 267 268 /** 269 * @param value {@link #device} The actual object that is the target of the 270 * reference. The reference library doesn't use these, but you can 271 * use it to hold the resource if you resolve it. (The details of 272 * the device used.) 273 */ 274 public DeviceUseStatement setDeviceTarget(Device value) { 275 this.deviceTarget = value; 276 return this; 277 } 278 279 /** 280 * @return {@link #identifier} (An external identifier for this statement such 281 * as an IRI.) 282 */ 283 public List<Identifier> getIdentifier() { 284 if (this.identifier == null) 285 this.identifier = new ArrayList<Identifier>(); 286 return this.identifier; 287 } 288 289 public boolean hasIdentifier() { 290 if (this.identifier == null) 291 return false; 292 for (Identifier item : this.identifier) 293 if (!item.isEmpty()) 294 return true; 295 return false; 296 } 297 298 /** 299 * @return {@link #identifier} (An external identifier for this statement such 300 * as an IRI.) 301 */ 302 // syntactic sugar 303 public Identifier addIdentifier() { // 3 304 Identifier t = new Identifier(); 305 if (this.identifier == null) 306 this.identifier = new ArrayList<Identifier>(); 307 this.identifier.add(t); 308 return t; 309 } 310 311 // syntactic sugar 312 public DeviceUseStatement addIdentifier(Identifier t) { // 3 313 if (t == null) 314 return this; 315 if (this.identifier == null) 316 this.identifier = new ArrayList<Identifier>(); 317 this.identifier.add(t); 318 return this; 319 } 320 321 /** 322 * @return {@link #indication} (Reason or justification for the use of the 323 * device.) 324 */ 325 public List<CodeableConcept> getIndication() { 326 if (this.indication == null) 327 this.indication = new ArrayList<CodeableConcept>(); 328 return this.indication; 329 } 330 331 public boolean hasIndication() { 332 if (this.indication == null) 333 return false; 334 for (CodeableConcept item : this.indication) 335 if (!item.isEmpty()) 336 return true; 337 return false; 338 } 339 340 /** 341 * @return {@link #indication} (Reason or justification for the use of the 342 * device.) 343 */ 344 // syntactic sugar 345 public CodeableConcept addIndication() { // 3 346 CodeableConcept t = new CodeableConcept(); 347 if (this.indication == null) 348 this.indication = new ArrayList<CodeableConcept>(); 349 this.indication.add(t); 350 return t; 351 } 352 353 // syntactic sugar 354 public DeviceUseStatement addIndication(CodeableConcept t) { // 3 355 if (t == null) 356 return this; 357 if (this.indication == null) 358 this.indication = new ArrayList<CodeableConcept>(); 359 this.indication.add(t); 360 return this; 361 } 362 363 /** 364 * @return {@link #notes} (Details about the device statement that were not 365 * represented at all or sufficiently in one of the attributes provided 366 * in a class. These may include for example a comment, an instruction, 367 * or a note associated with the statement.) 368 */ 369 public List<StringType> getNotes() { 370 if (this.notes == null) 371 this.notes = new ArrayList<StringType>(); 372 return this.notes; 373 } 374 375 public boolean hasNotes() { 376 if (this.notes == null) 377 return false; 378 for (StringType item : this.notes) 379 if (!item.isEmpty()) 380 return true; 381 return false; 382 } 383 384 /** 385 * @return {@link #notes} (Details about the device statement that were not 386 * represented at all or sufficiently in one of the attributes provided 387 * in a class. These may include for example a comment, an instruction, 388 * or a note associated with the statement.) 389 */ 390 // syntactic sugar 391 public StringType addNotesElement() {// 2 392 StringType t = new StringType(); 393 if (this.notes == null) 394 this.notes = new ArrayList<StringType>(); 395 this.notes.add(t); 396 return t; 397 } 398 399 /** 400 * @param value {@link #notes} (Details about the device statement that were not 401 * represented at all or sufficiently in one of the attributes 402 * provided in a class. These may include for example a comment, an 403 * instruction, or a note associated with the statement.) 404 */ 405 public DeviceUseStatement addNotes(String value) { // 1 406 StringType t = new StringType(); 407 t.setValue(value); 408 if (this.notes == null) 409 this.notes = new ArrayList<StringType>(); 410 this.notes.add(t); 411 return this; 412 } 413 414 /** 415 * @param value {@link #notes} (Details about the device statement that were not 416 * represented at all or sufficiently in one of the attributes 417 * provided in a class. These may include for example a comment, an 418 * instruction, or a note associated with the statement.) 419 */ 420 public boolean hasNotes(String value) { 421 if (this.notes == null) 422 return false; 423 for (StringType v : this.notes) 424 if (v.equals(value)) // string 425 return true; 426 return false; 427 } 428 429 /** 430 * @return {@link #recordedOn} (The time at which the statement was 431 * made/recorded.). This is the underlying object with id, value and 432 * extensions. The accessor "getRecordedOn" gives direct access to the 433 * value 434 */ 435 public DateTimeType getRecordedOnElement() { 436 if (this.recordedOn == null) 437 if (Configuration.errorOnAutoCreate()) 438 throw new Error("Attempt to auto-create DeviceUseStatement.recordedOn"); 439 else if (Configuration.doAutoCreate()) 440 this.recordedOn = new DateTimeType(); // bb 441 return this.recordedOn; 442 } 443 444 public boolean hasRecordedOnElement() { 445 return this.recordedOn != null && !this.recordedOn.isEmpty(); 446 } 447 448 public boolean hasRecordedOn() { 449 return this.recordedOn != null && !this.recordedOn.isEmpty(); 450 } 451 452 /** 453 * @param value {@link #recordedOn} (The time at which the statement was 454 * made/recorded.). This is the underlying object with id, value 455 * and extensions. The accessor "getRecordedOn" gives direct access 456 * to the value 457 */ 458 public DeviceUseStatement setRecordedOnElement(DateTimeType value) { 459 this.recordedOn = value; 460 return this; 461 } 462 463 /** 464 * @return The time at which the statement was made/recorded. 465 */ 466 public Date getRecordedOn() { 467 return this.recordedOn == null ? null : this.recordedOn.getValue(); 468 } 469 470 /** 471 * @param value The time at which the statement was made/recorded. 472 */ 473 public DeviceUseStatement setRecordedOn(Date value) { 474 if (value == null) 475 this.recordedOn = null; 476 else { 477 if (this.recordedOn == null) 478 this.recordedOn = new DateTimeType(); 479 this.recordedOn.setValue(value); 480 } 481 return this; 482 } 483 484 /** 485 * @return {@link #subject} (The patient who used the device.) 486 */ 487 public Reference getSubject() { 488 if (this.subject == null) 489 if (Configuration.errorOnAutoCreate()) 490 throw new Error("Attempt to auto-create DeviceUseStatement.subject"); 491 else if (Configuration.doAutoCreate()) 492 this.subject = new Reference(); // cc 493 return this.subject; 494 } 495 496 public boolean hasSubject() { 497 return this.subject != null && !this.subject.isEmpty(); 498 } 499 500 /** 501 * @param value {@link #subject} (The patient who used the device.) 502 */ 503 public DeviceUseStatement setSubject(Reference value) { 504 this.subject = value; 505 return this; 506 } 507 508 /** 509 * @return {@link #subject} The actual object that is the target of the 510 * reference. The reference library doesn't populate this, but you can 511 * use it to hold the resource if you resolve it. (The patient who used 512 * the device.) 513 */ 514 public Patient getSubjectTarget() { 515 if (this.subjectTarget == null) 516 if (Configuration.errorOnAutoCreate()) 517 throw new Error("Attempt to auto-create DeviceUseStatement.subject"); 518 else if (Configuration.doAutoCreate()) 519 this.subjectTarget = new Patient(); // aa 520 return this.subjectTarget; 521 } 522 523 /** 524 * @param value {@link #subject} The actual object that is the target of the 525 * reference. The reference library doesn't use these, but you can 526 * use it to hold the resource if you resolve it. (The patient who 527 * used the device.) 528 */ 529 public DeviceUseStatement setSubjectTarget(Patient value) { 530 this.subjectTarget = value; 531 return this; 532 } 533 534 /** 535 * @return {@link #timing} (How often the device was used.) 536 */ 537 public Type getTiming() { 538 return this.timing; 539 } 540 541 /** 542 * @return {@link #timing} (How often the device was used.) 543 */ 544 public Timing getTimingTiming() throws FHIRException { 545 if (!(this.timing instanceof Timing)) 546 throw new FHIRException( 547 "Type mismatch: the type Timing was expected, but " + this.timing.getClass().getName() + " was encountered"); 548 return (Timing) this.timing; 549 } 550 551 public boolean hasTimingTiming() { 552 return this.timing instanceof Timing; 553 } 554 555 /** 556 * @return {@link #timing} (How often the device was used.) 557 */ 558 public Period getTimingPeriod() throws FHIRException { 559 if (!(this.timing instanceof Period)) 560 throw new FHIRException( 561 "Type mismatch: the type Period was expected, but " + this.timing.getClass().getName() + " was encountered"); 562 return (Period) this.timing; 563 } 564 565 public boolean hasTimingPeriod() { 566 return this.timing instanceof Period; 567 } 568 569 /** 570 * @return {@link #timing} (How often the device was used.) 571 */ 572 public DateTimeType getTimingDateTimeType() throws FHIRException { 573 if (!(this.timing instanceof DateTimeType)) 574 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 575 + this.timing.getClass().getName() + " was encountered"); 576 return (DateTimeType) this.timing; 577 } 578 579 public boolean hasTimingDateTimeType() { 580 return this.timing instanceof DateTimeType; 581 } 582 583 public boolean hasTiming() { 584 return this.timing != null && !this.timing.isEmpty(); 585 } 586 587 /** 588 * @param value {@link #timing} (How often the device was used.) 589 */ 590 public DeviceUseStatement setTiming(Type value) { 591 this.timing = value; 592 return this; 593 } 594 595 protected void listChildren(List<Property> childrenList) { 596 super.listChildren(childrenList); 597 childrenList.add(new Property("bodySite[x]", "CodeableConcept|Reference(BodySite)", 598 "Indicates the site on the subject's body where the device was used ( i.e. the target site).", 0, 599 java.lang.Integer.MAX_VALUE, bodySite)); 600 childrenList.add(new Property("whenUsed", "Period", "The time period over which the device was used.", 0, 601 java.lang.Integer.MAX_VALUE, whenUsed)); 602 childrenList.add(new Property("device", "Reference(Device)", "The details of the device used.", 0, 603 java.lang.Integer.MAX_VALUE, device)); 604 childrenList.add(new Property("identifier", "Identifier", 605 "An external identifier for this statement such as an IRI.", 0, java.lang.Integer.MAX_VALUE, identifier)); 606 childrenList.add(new Property("indication", "CodeableConcept", "Reason or justification for the use of the device.", 607 0, java.lang.Integer.MAX_VALUE, indication)); 608 childrenList.add(new Property("notes", "string", 609 "Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 610 0, java.lang.Integer.MAX_VALUE, notes)); 611 childrenList.add(new Property("recordedOn", "dateTime", "The time at which the statement was made/recorded.", 0, 612 java.lang.Integer.MAX_VALUE, recordedOn)); 613 childrenList.add(new Property("subject", "Reference(Patient)", "The patient who used the device.", 0, 614 java.lang.Integer.MAX_VALUE, subject)); 615 childrenList.add(new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 616 java.lang.Integer.MAX_VALUE, timing)); 617 } 618 619 @Override 620 public void setProperty(String name, Base value) throws FHIRException { 621 if (name.equals("bodySite[x]")) 622 this.bodySite = (Type) value; // Type 623 else if (name.equals("whenUsed")) 624 this.whenUsed = castToPeriod(value); // Period 625 else if (name.equals("device")) 626 this.device = castToReference(value); // Reference 627 else if (name.equals("identifier")) 628 this.getIdentifier().add(castToIdentifier(value)); 629 else if (name.equals("indication")) 630 this.getIndication().add(castToCodeableConcept(value)); 631 else if (name.equals("notes")) 632 this.getNotes().add(castToString(value)); 633 else if (name.equals("recordedOn")) 634 this.recordedOn = castToDateTime(value); // DateTimeType 635 else if (name.equals("subject")) 636 this.subject = castToReference(value); // Reference 637 else if (name.equals("timing[x]")) 638 this.timing = (Type) value; // Type 639 else 640 super.setProperty(name, value); 641 } 642 643 @Override 644 public Base addChild(String name) throws FHIRException { 645 if (name.equals("bodySiteCodeableConcept")) { 646 this.bodySite = new CodeableConcept(); 647 return this.bodySite; 648 } else if (name.equals("bodySiteReference")) { 649 this.bodySite = new Reference(); 650 return this.bodySite; 651 } else if (name.equals("whenUsed")) { 652 this.whenUsed = new Period(); 653 return this.whenUsed; 654 } else if (name.equals("device")) { 655 this.device = new Reference(); 656 return this.device; 657 } else if (name.equals("identifier")) { 658 return addIdentifier(); 659 } else if (name.equals("indication")) { 660 return addIndication(); 661 } else if (name.equals("notes")) { 662 throw new FHIRException("Cannot call addChild on a singleton property DeviceUseStatement.notes"); 663 } else if (name.equals("recordedOn")) { 664 throw new FHIRException("Cannot call addChild on a singleton property DeviceUseStatement.recordedOn"); 665 } else if (name.equals("subject")) { 666 this.subject = new Reference(); 667 return this.subject; 668 } else if (name.equals("timingTiming")) { 669 this.timing = new Timing(); 670 return this.timing; 671 } else if (name.equals("timingPeriod")) { 672 this.timing = new Period(); 673 return this.timing; 674 } else if (name.equals("timingDateTime")) { 675 this.timing = new DateTimeType(); 676 return this.timing; 677 } else 678 return super.addChild(name); 679 } 680 681 public String fhirType() { 682 return "DeviceUseStatement"; 683 684 } 685 686 public DeviceUseStatement copy() { 687 DeviceUseStatement dst = new DeviceUseStatement(); 688 copyValues(dst); 689 dst.bodySite = bodySite == null ? null : bodySite.copy(); 690 dst.whenUsed = whenUsed == null ? null : whenUsed.copy(); 691 dst.device = device == null ? null : device.copy(); 692 if (identifier != null) { 693 dst.identifier = new ArrayList<Identifier>(); 694 for (Identifier i : identifier) 695 dst.identifier.add(i.copy()); 696 } 697 ; 698 if (indication != null) { 699 dst.indication = new ArrayList<CodeableConcept>(); 700 for (CodeableConcept i : indication) 701 dst.indication.add(i.copy()); 702 } 703 ; 704 if (notes != null) { 705 dst.notes = new ArrayList<StringType>(); 706 for (StringType i : notes) 707 dst.notes.add(i.copy()); 708 } 709 ; 710 dst.recordedOn = recordedOn == null ? null : recordedOn.copy(); 711 dst.subject = subject == null ? null : subject.copy(); 712 dst.timing = timing == null ? null : timing.copy(); 713 return dst; 714 } 715 716 protected DeviceUseStatement typedCopy() { 717 return copy(); 718 } 719 720 @Override 721 public boolean equalsDeep(Base other) { 722 if (!super.equalsDeep(other)) 723 return false; 724 if (!(other instanceof DeviceUseStatement)) 725 return false; 726 DeviceUseStatement o = (DeviceUseStatement) other; 727 return compareDeep(bodySite, o.bodySite, true) && compareDeep(whenUsed, o.whenUsed, true) 728 && compareDeep(device, o.device, true) && compareDeep(identifier, o.identifier, true) 729 && compareDeep(indication, o.indication, true) && compareDeep(notes, o.notes, true) 730 && compareDeep(recordedOn, o.recordedOn, true) && compareDeep(subject, o.subject, true) 731 && compareDeep(timing, o.timing, true); 732 } 733 734 @Override 735 public boolean equalsShallow(Base other) { 736 if (!super.equalsShallow(other)) 737 return false; 738 if (!(other instanceof DeviceUseStatement)) 739 return false; 740 DeviceUseStatement o = (DeviceUseStatement) other; 741 return compareValues(notes, o.notes, true) && compareValues(recordedOn, o.recordedOn, true); 742 } 743 744 public boolean isEmpty() { 745 return super.isEmpty() && (bodySite == null || bodySite.isEmpty()) && (whenUsed == null || whenUsed.isEmpty()) 746 && (device == null || device.isEmpty()) && (identifier == null || identifier.isEmpty()) 747 && (indication == null || indication.isEmpty()) && (notes == null || notes.isEmpty()) 748 && (recordedOn == null || recordedOn.isEmpty()) && (subject == null || subject.isEmpty()) 749 && (timing == null || timing.isEmpty()); 750 } 751 752 @Override 753 public ResourceType getResourceType() { 754 return ResourceType.DeviceUseStatement; 755 } 756 757 @SearchParamDefinition(name = "subject", path = "DeviceUseStatement.subject", description = "Search by subject", type = "reference") 758 public static final String SP_SUBJECT = "subject"; 759 @SearchParamDefinition(name = "patient", path = "DeviceUseStatement.subject", description = "Search by subject - a patient", type = "reference") 760 public static final String SP_PATIENT = "patient"; 761 @SearchParamDefinition(name = "device", path = "DeviceUseStatement.device", description = "Search by device", type = "reference") 762 public static final String SP_DEVICE = "device"; 763 764}