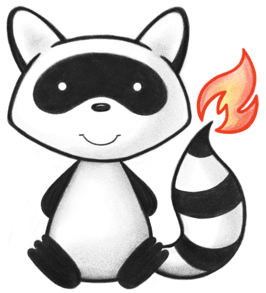
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044 045/** 046 * A record of a request for a diagnostic investigation service to be performed. 047 */ 048@ResourceDef(name = "DiagnosticOrder", profile = "http://hl7.org/fhir/Profile/DiagnosticOrder") 049public class DiagnosticOrder extends DomainResource { 050 051 public enum DiagnosticOrderStatus { 052 /** 053 * The request has been proposed. 054 */ 055 PROPOSED, 056 /** 057 * The request is in preliminary form prior to being sent. 058 */ 059 DRAFT, 060 /** 061 * The request has been planned. 062 */ 063 PLANNED, 064 /** 065 * The request has been placed. 066 */ 067 REQUESTED, 068 /** 069 * The receiving system has received the order, but not yet decided whether it 070 * will be performed. 071 */ 072 RECEIVED, 073 /** 074 * The receiving system has accepted the order, but work has not yet commenced. 075 */ 076 ACCEPTED, 077 /** 078 * The work to fulfill the order is happening. 079 */ 080 INPROGRESS, 081 /** 082 * The work is complete, and the outcomes are being reviewed for approval. 083 */ 084 REVIEW, 085 /** 086 * The work has been completed, the report(s) released, and no further work is 087 * planned. 088 */ 089 COMPLETED, 090 /** 091 * The request has been withdrawn. 092 */ 093 CANCELLED, 094 /** 095 * The request has been held by originating system/user request. 096 */ 097 SUSPENDED, 098 /** 099 * The receiving system has declined to fulfill the request. 100 */ 101 REJECTED, 102 /** 103 * The diagnostic investigation was attempted, but due to some procedural error, 104 * it could not be completed. 105 */ 106 FAILED, 107 /** 108 * added to help the parsers 109 */ 110 NULL; 111 112 public static DiagnosticOrderStatus fromCode(String codeString) throws FHIRException { 113 if (codeString == null || "".equals(codeString)) 114 return null; 115 if ("proposed".equals(codeString)) 116 return PROPOSED; 117 if ("draft".equals(codeString)) 118 return DRAFT; 119 if ("planned".equals(codeString)) 120 return PLANNED; 121 if ("requested".equals(codeString)) 122 return REQUESTED; 123 if ("received".equals(codeString)) 124 return RECEIVED; 125 if ("accepted".equals(codeString)) 126 return ACCEPTED; 127 if ("in-progress".equals(codeString)) 128 return INPROGRESS; 129 if ("review".equals(codeString)) 130 return REVIEW; 131 if ("completed".equals(codeString)) 132 return COMPLETED; 133 if ("cancelled".equals(codeString)) 134 return CANCELLED; 135 if ("suspended".equals(codeString)) 136 return SUSPENDED; 137 if ("rejected".equals(codeString)) 138 return REJECTED; 139 if ("failed".equals(codeString)) 140 return FAILED; 141 throw new FHIRException("Unknown DiagnosticOrderStatus code '" + codeString + "'"); 142 } 143 144 public String toCode() { 145 switch (this) { 146 case PROPOSED: 147 return "proposed"; 148 case DRAFT: 149 return "draft"; 150 case PLANNED: 151 return "planned"; 152 case REQUESTED: 153 return "requested"; 154 case RECEIVED: 155 return "received"; 156 case ACCEPTED: 157 return "accepted"; 158 case INPROGRESS: 159 return "in-progress"; 160 case REVIEW: 161 return "review"; 162 case COMPLETED: 163 return "completed"; 164 case CANCELLED: 165 return "cancelled"; 166 case SUSPENDED: 167 return "suspended"; 168 case REJECTED: 169 return "rejected"; 170 case FAILED: 171 return "failed"; 172 case NULL: 173 return null; 174 default: 175 return "?"; 176 } 177 } 178 179 public String getSystem() { 180 switch (this) { 181 case PROPOSED: 182 return "http://hl7.org/fhir/diagnostic-order-status"; 183 case DRAFT: 184 return "http://hl7.org/fhir/diagnostic-order-status"; 185 case PLANNED: 186 return "http://hl7.org/fhir/diagnostic-order-status"; 187 case REQUESTED: 188 return "http://hl7.org/fhir/diagnostic-order-status"; 189 case RECEIVED: 190 return "http://hl7.org/fhir/diagnostic-order-status"; 191 case ACCEPTED: 192 return "http://hl7.org/fhir/diagnostic-order-status"; 193 case INPROGRESS: 194 return "http://hl7.org/fhir/diagnostic-order-status"; 195 case REVIEW: 196 return "http://hl7.org/fhir/diagnostic-order-status"; 197 case COMPLETED: 198 return "http://hl7.org/fhir/diagnostic-order-status"; 199 case CANCELLED: 200 return "http://hl7.org/fhir/diagnostic-order-status"; 201 case SUSPENDED: 202 return "http://hl7.org/fhir/diagnostic-order-status"; 203 case REJECTED: 204 return "http://hl7.org/fhir/diagnostic-order-status"; 205 case FAILED: 206 return "http://hl7.org/fhir/diagnostic-order-status"; 207 case NULL: 208 return null; 209 default: 210 return "?"; 211 } 212 } 213 214 public String getDefinition() { 215 switch (this) { 216 case PROPOSED: 217 return "The request has been proposed."; 218 case DRAFT: 219 return "The request is in preliminary form prior to being sent."; 220 case PLANNED: 221 return "The request has been planned."; 222 case REQUESTED: 223 return "The request has been placed."; 224 case RECEIVED: 225 return "The receiving system has received the order, but not yet decided whether it will be performed."; 226 case ACCEPTED: 227 return "The receiving system has accepted the order, but work has not yet commenced."; 228 case INPROGRESS: 229 return "The work to fulfill the order is happening."; 230 case REVIEW: 231 return "The work is complete, and the outcomes are being reviewed for approval."; 232 case COMPLETED: 233 return "The work has been completed, the report(s) released, and no further work is planned."; 234 case CANCELLED: 235 return "The request has been withdrawn."; 236 case SUSPENDED: 237 return "The request has been held by originating system/user request."; 238 case REJECTED: 239 return "The receiving system has declined to fulfill the request."; 240 case FAILED: 241 return "The diagnostic investigation was attempted, but due to some procedural error, it could not be completed."; 242 case NULL: 243 return null; 244 default: 245 return "?"; 246 } 247 } 248 249 public String getDisplay() { 250 switch (this) { 251 case PROPOSED: 252 return "Proposed"; 253 case DRAFT: 254 return "Draft"; 255 case PLANNED: 256 return "Planned"; 257 case REQUESTED: 258 return "Requested"; 259 case RECEIVED: 260 return "Received"; 261 case ACCEPTED: 262 return "Accepted"; 263 case INPROGRESS: 264 return "In-Progress"; 265 case REVIEW: 266 return "Review"; 267 case COMPLETED: 268 return "Completed"; 269 case CANCELLED: 270 return "Cancelled"; 271 case SUSPENDED: 272 return "Suspended"; 273 case REJECTED: 274 return "Rejected"; 275 case FAILED: 276 return "Failed"; 277 case NULL: 278 return null; 279 default: 280 return "?"; 281 } 282 } 283 } 284 285 public static class DiagnosticOrderStatusEnumFactory implements EnumFactory<DiagnosticOrderStatus> { 286 public DiagnosticOrderStatus fromCode(String codeString) throws IllegalArgumentException { 287 if (codeString == null || "".equals(codeString)) 288 if (codeString == null || "".equals(codeString)) 289 return null; 290 if ("proposed".equals(codeString)) 291 return DiagnosticOrderStatus.PROPOSED; 292 if ("draft".equals(codeString)) 293 return DiagnosticOrderStatus.DRAFT; 294 if ("planned".equals(codeString)) 295 return DiagnosticOrderStatus.PLANNED; 296 if ("requested".equals(codeString)) 297 return DiagnosticOrderStatus.REQUESTED; 298 if ("received".equals(codeString)) 299 return DiagnosticOrderStatus.RECEIVED; 300 if ("accepted".equals(codeString)) 301 return DiagnosticOrderStatus.ACCEPTED; 302 if ("in-progress".equals(codeString)) 303 return DiagnosticOrderStatus.INPROGRESS; 304 if ("review".equals(codeString)) 305 return DiagnosticOrderStatus.REVIEW; 306 if ("completed".equals(codeString)) 307 return DiagnosticOrderStatus.COMPLETED; 308 if ("cancelled".equals(codeString)) 309 return DiagnosticOrderStatus.CANCELLED; 310 if ("suspended".equals(codeString)) 311 return DiagnosticOrderStatus.SUSPENDED; 312 if ("rejected".equals(codeString)) 313 return DiagnosticOrderStatus.REJECTED; 314 if ("failed".equals(codeString)) 315 return DiagnosticOrderStatus.FAILED; 316 throw new IllegalArgumentException("Unknown DiagnosticOrderStatus code '" + codeString + "'"); 317 } 318 319 public Enumeration<DiagnosticOrderStatus> fromType(Base code) throws FHIRException { 320 if (code == null || code.isEmpty()) 321 return null; 322 String codeString = ((PrimitiveType) code).asStringValue(); 323 if (codeString == null || "".equals(codeString)) 324 return null; 325 if ("proposed".equals(codeString)) 326 return new Enumeration<DiagnosticOrderStatus>(this, DiagnosticOrderStatus.PROPOSED); 327 if ("draft".equals(codeString)) 328 return new Enumeration<DiagnosticOrderStatus>(this, DiagnosticOrderStatus.DRAFT); 329 if ("planned".equals(codeString)) 330 return new Enumeration<DiagnosticOrderStatus>(this, DiagnosticOrderStatus.PLANNED); 331 if ("requested".equals(codeString)) 332 return new Enumeration<DiagnosticOrderStatus>(this, DiagnosticOrderStatus.REQUESTED); 333 if ("received".equals(codeString)) 334 return new Enumeration<DiagnosticOrderStatus>(this, DiagnosticOrderStatus.RECEIVED); 335 if ("accepted".equals(codeString)) 336 return new Enumeration<DiagnosticOrderStatus>(this, DiagnosticOrderStatus.ACCEPTED); 337 if ("in-progress".equals(codeString)) 338 return new Enumeration<DiagnosticOrderStatus>(this, DiagnosticOrderStatus.INPROGRESS); 339 if ("review".equals(codeString)) 340 return new Enumeration<DiagnosticOrderStatus>(this, DiagnosticOrderStatus.REVIEW); 341 if ("completed".equals(codeString)) 342 return new Enumeration<DiagnosticOrderStatus>(this, DiagnosticOrderStatus.COMPLETED); 343 if ("cancelled".equals(codeString)) 344 return new Enumeration<DiagnosticOrderStatus>(this, DiagnosticOrderStatus.CANCELLED); 345 if ("suspended".equals(codeString)) 346 return new Enumeration<DiagnosticOrderStatus>(this, DiagnosticOrderStatus.SUSPENDED); 347 if ("rejected".equals(codeString)) 348 return new Enumeration<DiagnosticOrderStatus>(this, DiagnosticOrderStatus.REJECTED); 349 if ("failed".equals(codeString)) 350 return new Enumeration<DiagnosticOrderStatus>(this, DiagnosticOrderStatus.FAILED); 351 throw new FHIRException("Unknown DiagnosticOrderStatus code '" + codeString + "'"); 352 } 353 354 public String toCode(DiagnosticOrderStatus code) 355 { 356 if (code == DiagnosticOrderStatus.NULL) 357 return null; 358 if (code == DiagnosticOrderStatus.PROPOSED) 359 return "proposed"; 360 if (code == DiagnosticOrderStatus.DRAFT) 361 return "draft"; 362 if (code == DiagnosticOrderStatus.PLANNED) 363 return "planned"; 364 if (code == DiagnosticOrderStatus.REQUESTED) 365 return "requested"; 366 if (code == DiagnosticOrderStatus.RECEIVED) 367 return "received"; 368 if (code == DiagnosticOrderStatus.ACCEPTED) 369 return "accepted"; 370 if (code == DiagnosticOrderStatus.INPROGRESS) 371 return "in-progress"; 372 if (code == DiagnosticOrderStatus.REVIEW) 373 return "review"; 374 if (code == DiagnosticOrderStatus.COMPLETED) 375 return "completed"; 376 if (code == DiagnosticOrderStatus.CANCELLED) 377 return "cancelled"; 378 if (code == DiagnosticOrderStatus.SUSPENDED) 379 return "suspended"; 380 if (code == DiagnosticOrderStatus.REJECTED) 381 return "rejected"; 382 if (code == DiagnosticOrderStatus.FAILED) 383 return "failed"; 384 return "?"; 385 } 386 } 387 388 public enum DiagnosticOrderPriority { 389 /** 390 * The order has a normal priority . 391 */ 392 ROUTINE, 393 /** 394 * The order should be urgently. 395 */ 396 URGENT, 397 /** 398 * The order is time-critical. 399 */ 400 STAT, 401 /** 402 * The order should be acted on as soon as possible. 403 */ 404 ASAP, 405 /** 406 * added to help the parsers 407 */ 408 NULL; 409 410 public static DiagnosticOrderPriority fromCode(String codeString) throws FHIRException { 411 if (codeString == null || "".equals(codeString)) 412 return null; 413 if ("routine".equals(codeString)) 414 return ROUTINE; 415 if ("urgent".equals(codeString)) 416 return URGENT; 417 if ("stat".equals(codeString)) 418 return STAT; 419 if ("asap".equals(codeString)) 420 return ASAP; 421 throw new FHIRException("Unknown DiagnosticOrderPriority code '" + codeString + "'"); 422 } 423 424 public String toCode() { 425 switch (this) { 426 case ROUTINE: 427 return "routine"; 428 case URGENT: 429 return "urgent"; 430 case STAT: 431 return "stat"; 432 case ASAP: 433 return "asap"; 434 case NULL: 435 return null; 436 default: 437 return "?"; 438 } 439 } 440 441 public String getSystem() { 442 switch (this) { 443 case ROUTINE: 444 return "http://hl7.org/fhir/diagnostic-order-priority"; 445 case URGENT: 446 return "http://hl7.org/fhir/diagnostic-order-priority"; 447 case STAT: 448 return "http://hl7.org/fhir/diagnostic-order-priority"; 449 case ASAP: 450 return "http://hl7.org/fhir/diagnostic-order-priority"; 451 case NULL: 452 return null; 453 default: 454 return "?"; 455 } 456 } 457 458 public String getDefinition() { 459 switch (this) { 460 case ROUTINE: 461 return "The order has a normal priority ."; 462 case URGENT: 463 return "The order should be urgently."; 464 case STAT: 465 return "The order is time-critical."; 466 case ASAP: 467 return "The order should be acted on as soon as possible."; 468 case NULL: 469 return null; 470 default: 471 return "?"; 472 } 473 } 474 475 public String getDisplay() { 476 switch (this) { 477 case ROUTINE: 478 return "Routine"; 479 case URGENT: 480 return "Urgent"; 481 case STAT: 482 return "Stat"; 483 case ASAP: 484 return "ASAP"; 485 case NULL: 486 return null; 487 default: 488 return "?"; 489 } 490 } 491 } 492 493 public static class DiagnosticOrderPriorityEnumFactory implements EnumFactory<DiagnosticOrderPriority> { 494 public DiagnosticOrderPriority fromCode(String codeString) throws IllegalArgumentException { 495 if (codeString == null || "".equals(codeString)) 496 if (codeString == null || "".equals(codeString)) 497 return null; 498 if ("routine".equals(codeString)) 499 return DiagnosticOrderPriority.ROUTINE; 500 if ("urgent".equals(codeString)) 501 return DiagnosticOrderPriority.URGENT; 502 if ("stat".equals(codeString)) 503 return DiagnosticOrderPriority.STAT; 504 if ("asap".equals(codeString)) 505 return DiagnosticOrderPriority.ASAP; 506 throw new IllegalArgumentException("Unknown DiagnosticOrderPriority code '" + codeString + "'"); 507 } 508 509 public Enumeration<DiagnosticOrderPriority> fromType(Base code) throws FHIRException { 510 if (code == null || code.isEmpty()) 511 return null; 512 String codeString = ((PrimitiveType) code).asStringValue(); 513 if (codeString == null || "".equals(codeString)) 514 return null; 515 if ("routine".equals(codeString)) 516 return new Enumeration<DiagnosticOrderPriority>(this, DiagnosticOrderPriority.ROUTINE); 517 if ("urgent".equals(codeString)) 518 return new Enumeration<DiagnosticOrderPriority>(this, DiagnosticOrderPriority.URGENT); 519 if ("stat".equals(codeString)) 520 return new Enumeration<DiagnosticOrderPriority>(this, DiagnosticOrderPriority.STAT); 521 if ("asap".equals(codeString)) 522 return new Enumeration<DiagnosticOrderPriority>(this, DiagnosticOrderPriority.ASAP); 523 throw new FHIRException("Unknown DiagnosticOrderPriority code '" + codeString + "'"); 524 } 525 526 public String toCode(DiagnosticOrderPriority code) 527 { 528 if (code == DiagnosticOrderPriority.NULL) 529 return null; 530 if (code == DiagnosticOrderPriority.ROUTINE) 531 return "routine"; 532 if (code == DiagnosticOrderPriority.URGENT) 533 return "urgent"; 534 if (code == DiagnosticOrderPriority.STAT) 535 return "stat"; 536 if (code == DiagnosticOrderPriority.ASAP) 537 return "asap"; 538 return "?"; 539 } 540 } 541 542 @Block() 543 public static class DiagnosticOrderEventComponent extends BackboneElement implements IBaseBackboneElement { 544 /** 545 * The status for the event. 546 */ 547 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 548 @Description(shortDefinition = "proposed | draft | planned | requested | received | accepted | in-progress | review | completed | cancelled | suspended | rejected | failed", formalDefinition = "The status for the event.") 549 protected Enumeration<DiagnosticOrderStatus> status; 550 551 /** 552 * Additional information about the event that occurred - e.g. if the status 553 * remained unchanged. 554 */ 555 @Child(name = "description", type = { 556 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 557 @Description(shortDefinition = "More information about the event and its context", formalDefinition = "Additional information about the event that occurred - e.g. if the status remained unchanged.") 558 protected CodeableConcept description; 559 560 /** 561 * The date/time at which the event occurred. 562 */ 563 @Child(name = "dateTime", type = { 564 DateTimeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 565 @Description(shortDefinition = "The date at which the event happened", formalDefinition = "The date/time at which the event occurred.") 566 protected DateTimeType dateTime; 567 568 /** 569 * The person responsible for performing or recording the action. 570 */ 571 @Child(name = "actor", type = { Practitioner.class, 572 Device.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 573 @Description(shortDefinition = "Who recorded or did this", formalDefinition = "The person responsible for performing or recording the action.") 574 protected Reference actor; 575 576 /** 577 * The actual object that is the target of the reference (The person responsible 578 * for performing or recording the action.) 579 */ 580 protected Resource actorTarget; 581 582 private static final long serialVersionUID = -370793723L; 583 584 /* 585 * Constructor 586 */ 587 public DiagnosticOrderEventComponent() { 588 super(); 589 } 590 591 /* 592 * Constructor 593 */ 594 public DiagnosticOrderEventComponent(Enumeration<DiagnosticOrderStatus> status, DateTimeType dateTime) { 595 super(); 596 this.status = status; 597 this.dateTime = dateTime; 598 } 599 600 /** 601 * @return {@link #status} (The status for the event.). This is the underlying 602 * object with id, value and extensions. The accessor "getStatus" gives 603 * direct access to the value 604 */ 605 public Enumeration<DiagnosticOrderStatus> getStatusElement() { 606 if (this.status == null) 607 if (Configuration.errorOnAutoCreate()) 608 throw new Error("Attempt to auto-create DiagnosticOrderEventComponent.status"); 609 else if (Configuration.doAutoCreate()) 610 this.status = new Enumeration<DiagnosticOrderStatus>(new DiagnosticOrderStatusEnumFactory()); // bb 611 return this.status; 612 } 613 614 public boolean hasStatusElement() { 615 return this.status != null && !this.status.isEmpty(); 616 } 617 618 public boolean hasStatus() { 619 return this.status != null && !this.status.isEmpty(); 620 } 621 622 /** 623 * @param value {@link #status} (The status for the event.). This is the 624 * underlying object with id, value and extensions. The accessor 625 * "getStatus" gives direct access to the value 626 */ 627 public DiagnosticOrderEventComponent setStatusElement(Enumeration<DiagnosticOrderStatus> value) { 628 this.status = value; 629 return this; 630 } 631 632 /** 633 * @return The status for the event. 634 */ 635 public DiagnosticOrderStatus getStatus() { 636 return this.status == null ? null : this.status.getValue(); 637 } 638 639 /** 640 * @param value The status for the event. 641 */ 642 public DiagnosticOrderEventComponent setStatus(DiagnosticOrderStatus value) { 643 if (this.status == null) 644 this.status = new Enumeration<DiagnosticOrderStatus>(new DiagnosticOrderStatusEnumFactory()); 645 this.status.setValue(value); 646 return this; 647 } 648 649 /** 650 * @return {@link #description} (Additional information about the event that 651 * occurred - e.g. if the status remained unchanged.) 652 */ 653 public CodeableConcept getDescription() { 654 if (this.description == null) 655 if (Configuration.errorOnAutoCreate()) 656 throw new Error("Attempt to auto-create DiagnosticOrderEventComponent.description"); 657 else if (Configuration.doAutoCreate()) 658 this.description = new CodeableConcept(); // cc 659 return this.description; 660 } 661 662 public boolean hasDescription() { 663 return this.description != null && !this.description.isEmpty(); 664 } 665 666 /** 667 * @param value {@link #description} (Additional information about the event 668 * that occurred - e.g. if the status remained unchanged.) 669 */ 670 public DiagnosticOrderEventComponent setDescription(CodeableConcept value) { 671 this.description = value; 672 return this; 673 } 674 675 /** 676 * @return {@link #dateTime} (The date/time at which the event occurred.). This 677 * is the underlying object with id, value and extensions. The accessor 678 * "getDateTime" gives direct access to the value 679 */ 680 public DateTimeType getDateTimeElement() { 681 if (this.dateTime == null) 682 if (Configuration.errorOnAutoCreate()) 683 throw new Error("Attempt to auto-create DiagnosticOrderEventComponent.dateTime"); 684 else if (Configuration.doAutoCreate()) 685 this.dateTime = new DateTimeType(); // bb 686 return this.dateTime; 687 } 688 689 public boolean hasDateTimeElement() { 690 return this.dateTime != null && !this.dateTime.isEmpty(); 691 } 692 693 public boolean hasDateTime() { 694 return this.dateTime != null && !this.dateTime.isEmpty(); 695 } 696 697 /** 698 * @param value {@link #dateTime} (The date/time at which the event occurred.). 699 * This is the underlying object with id, value and extensions. The 700 * accessor "getDateTime" gives direct access to the value 701 */ 702 public DiagnosticOrderEventComponent setDateTimeElement(DateTimeType value) { 703 this.dateTime = value; 704 return this; 705 } 706 707 /** 708 * @return The date/time at which the event occurred. 709 */ 710 public Date getDateTime() { 711 return this.dateTime == null ? null : this.dateTime.getValue(); 712 } 713 714 /** 715 * @param value The date/time at which the event occurred. 716 */ 717 public DiagnosticOrderEventComponent setDateTime(Date value) { 718 if (this.dateTime == null) 719 this.dateTime = new DateTimeType(); 720 this.dateTime.setValue(value); 721 return this; 722 } 723 724 /** 725 * @return {@link #actor} (The person responsible for performing or recording 726 * the action.) 727 */ 728 public Reference getActor() { 729 if (this.actor == null) 730 if (Configuration.errorOnAutoCreate()) 731 throw new Error("Attempt to auto-create DiagnosticOrderEventComponent.actor"); 732 else if (Configuration.doAutoCreate()) 733 this.actor = new Reference(); // cc 734 return this.actor; 735 } 736 737 public boolean hasActor() { 738 return this.actor != null && !this.actor.isEmpty(); 739 } 740 741 /** 742 * @param value {@link #actor} (The person responsible for performing or 743 * recording the action.) 744 */ 745 public DiagnosticOrderEventComponent setActor(Reference value) { 746 this.actor = value; 747 return this; 748 } 749 750 /** 751 * @return {@link #actor} The actual object that is the target of the reference. 752 * The reference library doesn't populate this, but you can use it to 753 * hold the resource if you resolve it. (The person responsible for 754 * performing or recording the action.) 755 */ 756 public Resource getActorTarget() { 757 return this.actorTarget; 758 } 759 760 /** 761 * @param value {@link #actor} The actual object that is the target of the 762 * reference. The reference library doesn't use these, but you can 763 * use it to hold the resource if you resolve it. (The person 764 * responsible for performing or recording the action.) 765 */ 766 public DiagnosticOrderEventComponent setActorTarget(Resource value) { 767 this.actorTarget = value; 768 return this; 769 } 770 771 protected void listChildren(List<Property> childrenList) { 772 super.listChildren(childrenList); 773 childrenList 774 .add(new Property("status", "code", "The status for the event.", 0, java.lang.Integer.MAX_VALUE, status)); 775 childrenList.add(new Property("description", "CodeableConcept", 776 "Additional information about the event that occurred - e.g. if the status remained unchanged.", 0, 777 java.lang.Integer.MAX_VALUE, description)); 778 childrenList.add(new Property("dateTime", "dateTime", "The date/time at which the event occurred.", 0, 779 java.lang.Integer.MAX_VALUE, dateTime)); 780 childrenList.add(new Property("actor", "Reference(Practitioner|Device)", 781 "The person responsible for performing or recording the action.", 0, java.lang.Integer.MAX_VALUE, actor)); 782 } 783 784 @Override 785 public void setProperty(String name, Base value) throws FHIRException { 786 if (name.equals("status")) 787 this.status = new DiagnosticOrderStatusEnumFactory().fromType(value); // Enumeration<DiagnosticOrderStatus> 788 else if (name.equals("description")) 789 this.description = castToCodeableConcept(value); // CodeableConcept 790 else if (name.equals("dateTime")) 791 this.dateTime = castToDateTime(value); // DateTimeType 792 else if (name.equals("actor")) 793 this.actor = castToReference(value); // Reference 794 else 795 super.setProperty(name, value); 796 } 797 798 @Override 799 public Base addChild(String name) throws FHIRException { 800 if (name.equals("status")) { 801 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticOrder.status"); 802 } else if (name.equals("description")) { 803 this.description = new CodeableConcept(); 804 return this.description; 805 } else if (name.equals("dateTime")) { 806 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticOrder.dateTime"); 807 } else if (name.equals("actor")) { 808 this.actor = new Reference(); 809 return this.actor; 810 } else 811 return super.addChild(name); 812 } 813 814 public DiagnosticOrderEventComponent copy() { 815 DiagnosticOrderEventComponent dst = new DiagnosticOrderEventComponent(); 816 copyValues(dst); 817 dst.status = status == null ? null : status.copy(); 818 dst.description = description == null ? null : description.copy(); 819 dst.dateTime = dateTime == null ? null : dateTime.copy(); 820 dst.actor = actor == null ? null : actor.copy(); 821 return dst; 822 } 823 824 @Override 825 public boolean equalsDeep(Base other) { 826 if (!super.equalsDeep(other)) 827 return false; 828 if (!(other instanceof DiagnosticOrderEventComponent)) 829 return false; 830 DiagnosticOrderEventComponent o = (DiagnosticOrderEventComponent) other; 831 return compareDeep(status, o.status, true) && compareDeep(description, o.description, true) 832 && compareDeep(dateTime, o.dateTime, true) && compareDeep(actor, o.actor, true); 833 } 834 835 @Override 836 public boolean equalsShallow(Base other) { 837 if (!super.equalsShallow(other)) 838 return false; 839 if (!(other instanceof DiagnosticOrderEventComponent)) 840 return false; 841 DiagnosticOrderEventComponent o = (DiagnosticOrderEventComponent) other; 842 return compareValues(status, o.status, true) && compareValues(dateTime, o.dateTime, true); 843 } 844 845 public boolean isEmpty() { 846 return super.isEmpty() && (status == null || status.isEmpty()) && (description == null || description.isEmpty()) 847 && (dateTime == null || dateTime.isEmpty()) && (actor == null || actor.isEmpty()); 848 } 849 850 public String fhirType() { 851 return "DiagnosticOrder.event"; 852 853 } 854 855 } 856 857 @Block() 858 public static class DiagnosticOrderItemComponent extends BackboneElement implements IBaseBackboneElement { 859 /** 860 * A code that identifies a particular diagnostic investigation, or panel of 861 * investigations, that have been requested. 862 */ 863 @Child(name = "code", type = { 864 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 865 @Description(shortDefinition = "Code to indicate the item (test or panel) being ordered", formalDefinition = "A code that identifies a particular diagnostic investigation, or panel of investigations, that have been requested.") 866 protected CodeableConcept code; 867 868 /** 869 * If the item is related to a specific specimen. 870 */ 871 @Child(name = "specimen", type = { 872 Specimen.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 873 @Description(shortDefinition = "If this item relates to specific specimens", formalDefinition = "If the item is related to a specific specimen.") 874 protected List<Reference> specimen; 875 /** 876 * The actual objects that are the target of the reference (If the item is 877 * related to a specific specimen.) 878 */ 879 protected List<Specimen> specimenTarget; 880 881 /** 882 * Anatomical location where the request test should be performed. This is the 883 * target site. 884 */ 885 @Child(name = "bodySite", type = { 886 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 887 @Description(shortDefinition = "Location of requested test (if applicable)", formalDefinition = "Anatomical location where the request test should be performed. This is the target site.") 888 protected CodeableConcept bodySite; 889 890 /** 891 * The status of this individual item within the order. 892 */ 893 @Child(name = "status", type = { CodeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 894 @Description(shortDefinition = "proposed | draft | planned | requested | received | accepted | in-progress | review | completed | cancelled | suspended | rejected | failed", formalDefinition = "The status of this individual item within the order.") 895 protected Enumeration<DiagnosticOrderStatus> status; 896 897 /** 898 * A summary of the events of interest that have occurred as this item of the 899 * request is processed. 900 */ 901 @Child(name = "event", type = { 902 DiagnosticOrderEventComponent.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 903 @Description(shortDefinition = "Events specific to this item", formalDefinition = "A summary of the events of interest that have occurred as this item of the request is processed.") 904 protected List<DiagnosticOrderEventComponent> event; 905 906 private static final long serialVersionUID = 381238192L; 907 908 /* 909 * Constructor 910 */ 911 public DiagnosticOrderItemComponent() { 912 super(); 913 } 914 915 /* 916 * Constructor 917 */ 918 public DiagnosticOrderItemComponent(CodeableConcept code) { 919 super(); 920 this.code = code; 921 } 922 923 /** 924 * @return {@link #code} (A code that identifies a particular diagnostic 925 * investigation, or panel of investigations, that have been requested.) 926 */ 927 public CodeableConcept getCode() { 928 if (this.code == null) 929 if (Configuration.errorOnAutoCreate()) 930 throw new Error("Attempt to auto-create DiagnosticOrderItemComponent.code"); 931 else if (Configuration.doAutoCreate()) 932 this.code = new CodeableConcept(); // cc 933 return this.code; 934 } 935 936 public boolean hasCode() { 937 return this.code != null && !this.code.isEmpty(); 938 } 939 940 /** 941 * @param value {@link #code} (A code that identifies a particular diagnostic 942 * investigation, or panel of investigations, that have been 943 * requested.) 944 */ 945 public DiagnosticOrderItemComponent setCode(CodeableConcept value) { 946 this.code = value; 947 return this; 948 } 949 950 /** 951 * @return {@link #specimen} (If the item is related to a specific specimen.) 952 */ 953 public List<Reference> getSpecimen() { 954 if (this.specimen == null) 955 this.specimen = new ArrayList<Reference>(); 956 return this.specimen; 957 } 958 959 public boolean hasSpecimen() { 960 if (this.specimen == null) 961 return false; 962 for (Reference item : this.specimen) 963 if (!item.isEmpty()) 964 return true; 965 return false; 966 } 967 968 /** 969 * @return {@link #specimen} (If the item is related to a specific specimen.) 970 */ 971 // syntactic sugar 972 public Reference addSpecimen() { // 3 973 Reference t = new Reference(); 974 if (this.specimen == null) 975 this.specimen = new ArrayList<Reference>(); 976 this.specimen.add(t); 977 return t; 978 } 979 980 // syntactic sugar 981 public DiagnosticOrderItemComponent addSpecimen(Reference t) { // 3 982 if (t == null) 983 return this; 984 if (this.specimen == null) 985 this.specimen = new ArrayList<Reference>(); 986 this.specimen.add(t); 987 return this; 988 } 989 990 /** 991 * @return {@link #specimen} (The actual objects that are the target of the 992 * reference. The reference library doesn't populate this, but you can 993 * use this to hold the resources if you resolvethemt. If the item is 994 * related to a specific specimen.) 995 */ 996 public List<Specimen> getSpecimenTarget() { 997 if (this.specimenTarget == null) 998 this.specimenTarget = new ArrayList<Specimen>(); 999 return this.specimenTarget; 1000 } 1001 1002 // syntactic sugar 1003 /** 1004 * @return {@link #specimen} (Add an actual object that is the target of the 1005 * reference. The reference library doesn't use these, but you can use 1006 * this to hold the resources if you resolvethemt. If the item is 1007 * related to a specific specimen.) 1008 */ 1009 public Specimen addSpecimenTarget() { 1010 Specimen r = new Specimen(); 1011 if (this.specimenTarget == null) 1012 this.specimenTarget = new ArrayList<Specimen>(); 1013 this.specimenTarget.add(r); 1014 return r; 1015 } 1016 1017 /** 1018 * @return {@link #bodySite} (Anatomical location where the request test should 1019 * be performed. This is the target site.) 1020 */ 1021 public CodeableConcept getBodySite() { 1022 if (this.bodySite == null) 1023 if (Configuration.errorOnAutoCreate()) 1024 throw new Error("Attempt to auto-create DiagnosticOrderItemComponent.bodySite"); 1025 else if (Configuration.doAutoCreate()) 1026 this.bodySite = new CodeableConcept(); // cc 1027 return this.bodySite; 1028 } 1029 1030 public boolean hasBodySite() { 1031 return this.bodySite != null && !this.bodySite.isEmpty(); 1032 } 1033 1034 /** 1035 * @param value {@link #bodySite} (Anatomical location where the request test 1036 * should be performed. This is the target site.) 1037 */ 1038 public DiagnosticOrderItemComponent setBodySite(CodeableConcept value) { 1039 this.bodySite = value; 1040 return this; 1041 } 1042 1043 /** 1044 * @return {@link #status} (The status of this individual item within the 1045 * order.). This is the underlying object with id, value and extensions. 1046 * The accessor "getStatus" gives direct access to the value 1047 */ 1048 public Enumeration<DiagnosticOrderStatus> getStatusElement() { 1049 if (this.status == null) 1050 if (Configuration.errorOnAutoCreate()) 1051 throw new Error("Attempt to auto-create DiagnosticOrderItemComponent.status"); 1052 else if (Configuration.doAutoCreate()) 1053 this.status = new Enumeration<DiagnosticOrderStatus>(new DiagnosticOrderStatusEnumFactory()); // bb 1054 return this.status; 1055 } 1056 1057 public boolean hasStatusElement() { 1058 return this.status != null && !this.status.isEmpty(); 1059 } 1060 1061 public boolean hasStatus() { 1062 return this.status != null && !this.status.isEmpty(); 1063 } 1064 1065 /** 1066 * @param value {@link #status} (The status of this individual item within the 1067 * order.). This is the underlying object with id, value and 1068 * extensions. The accessor "getStatus" gives direct access to the 1069 * value 1070 */ 1071 public DiagnosticOrderItemComponent setStatusElement(Enumeration<DiagnosticOrderStatus> value) { 1072 this.status = value; 1073 return this; 1074 } 1075 1076 /** 1077 * @return The status of this individual item within the order. 1078 */ 1079 public DiagnosticOrderStatus getStatus() { 1080 return this.status == null ? null : this.status.getValue(); 1081 } 1082 1083 /** 1084 * @param value The status of this individual item within the order. 1085 */ 1086 public DiagnosticOrderItemComponent setStatus(DiagnosticOrderStatus value) { 1087 if (value == null) 1088 this.status = null; 1089 else { 1090 if (this.status == null) 1091 this.status = new Enumeration<DiagnosticOrderStatus>(new DiagnosticOrderStatusEnumFactory()); 1092 this.status.setValue(value); 1093 } 1094 return this; 1095 } 1096 1097 /** 1098 * @return {@link #event} (A summary of the events of interest that have 1099 * occurred as this item of the request is processed.) 1100 */ 1101 public List<DiagnosticOrderEventComponent> getEvent() { 1102 if (this.event == null) 1103 this.event = new ArrayList<DiagnosticOrderEventComponent>(); 1104 return this.event; 1105 } 1106 1107 public boolean hasEvent() { 1108 if (this.event == null) 1109 return false; 1110 for (DiagnosticOrderEventComponent item : this.event) 1111 if (!item.isEmpty()) 1112 return true; 1113 return false; 1114 } 1115 1116 /** 1117 * @return {@link #event} (A summary of the events of interest that have 1118 * occurred as this item of the request is processed.) 1119 */ 1120 // syntactic sugar 1121 public DiagnosticOrderEventComponent addEvent() { // 3 1122 DiagnosticOrderEventComponent t = new DiagnosticOrderEventComponent(); 1123 if (this.event == null) 1124 this.event = new ArrayList<DiagnosticOrderEventComponent>(); 1125 this.event.add(t); 1126 return t; 1127 } 1128 1129 // syntactic sugar 1130 public DiagnosticOrderItemComponent addEvent(DiagnosticOrderEventComponent t) { // 3 1131 if (t == null) 1132 return this; 1133 if (this.event == null) 1134 this.event = new ArrayList<DiagnosticOrderEventComponent>(); 1135 this.event.add(t); 1136 return this; 1137 } 1138 1139 protected void listChildren(List<Property> childrenList) { 1140 super.listChildren(childrenList); 1141 childrenList.add(new Property("code", "CodeableConcept", 1142 "A code that identifies a particular diagnostic investigation, or panel of investigations, that have been requested.", 1143 0, java.lang.Integer.MAX_VALUE, code)); 1144 childrenList.add(new Property("specimen", "Reference(Specimen)", "If the item is related to a specific specimen.", 1145 0, java.lang.Integer.MAX_VALUE, specimen)); 1146 childrenList.add(new Property("bodySite", "CodeableConcept", 1147 "Anatomical location where the request test should be performed. This is the target site.", 0, 1148 java.lang.Integer.MAX_VALUE, bodySite)); 1149 childrenList.add(new Property("status", "code", "The status of this individual item within the order.", 0, 1150 java.lang.Integer.MAX_VALUE, status)); 1151 childrenList.add(new Property("event", "@DiagnosticOrder.event", 1152 "A summary of the events of interest that have occurred as this item of the request is processed.", 0, 1153 java.lang.Integer.MAX_VALUE, event)); 1154 } 1155 1156 @Override 1157 public void setProperty(String name, Base value) throws FHIRException { 1158 if (name.equals("code")) 1159 this.code = castToCodeableConcept(value); // CodeableConcept 1160 else if (name.equals("specimen")) 1161 this.getSpecimen().add(castToReference(value)); 1162 else if (name.equals("bodySite")) 1163 this.bodySite = castToCodeableConcept(value); // CodeableConcept 1164 else if (name.equals("status")) 1165 this.status = new DiagnosticOrderStatusEnumFactory().fromType(value); // Enumeration<DiagnosticOrderStatus> 1166 else if (name.equals("event")) 1167 this.getEvent().add((DiagnosticOrderEventComponent) value); 1168 else 1169 super.setProperty(name, value); 1170 } 1171 1172 @Override 1173 public Base addChild(String name) throws FHIRException { 1174 if (name.equals("code")) { 1175 this.code = new CodeableConcept(); 1176 return this.code; 1177 } else if (name.equals("specimen")) { 1178 return addSpecimen(); 1179 } else if (name.equals("bodySite")) { 1180 this.bodySite = new CodeableConcept(); 1181 return this.bodySite; 1182 } else if (name.equals("status")) { 1183 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticOrder.status"); 1184 } else if (name.equals("event")) { 1185 return addEvent(); 1186 } else 1187 return super.addChild(name); 1188 } 1189 1190 public DiagnosticOrderItemComponent copy() { 1191 DiagnosticOrderItemComponent dst = new DiagnosticOrderItemComponent(); 1192 copyValues(dst); 1193 dst.code = code == null ? null : code.copy(); 1194 if (specimen != null) { 1195 dst.specimen = new ArrayList<Reference>(); 1196 for (Reference i : specimen) 1197 dst.specimen.add(i.copy()); 1198 } 1199 ; 1200 dst.bodySite = bodySite == null ? null : bodySite.copy(); 1201 dst.status = status == null ? null : status.copy(); 1202 if (event != null) { 1203 dst.event = new ArrayList<DiagnosticOrderEventComponent>(); 1204 for (DiagnosticOrderEventComponent i : event) 1205 dst.event.add(i.copy()); 1206 } 1207 ; 1208 return dst; 1209 } 1210 1211 @Override 1212 public boolean equalsDeep(Base other) { 1213 if (!super.equalsDeep(other)) 1214 return false; 1215 if (!(other instanceof DiagnosticOrderItemComponent)) 1216 return false; 1217 DiagnosticOrderItemComponent o = (DiagnosticOrderItemComponent) other; 1218 return compareDeep(code, o.code, true) && compareDeep(specimen, o.specimen, true) 1219 && compareDeep(bodySite, o.bodySite, true) && compareDeep(status, o.status, true) 1220 && compareDeep(event, o.event, true); 1221 } 1222 1223 @Override 1224 public boolean equalsShallow(Base other) { 1225 if (!super.equalsShallow(other)) 1226 return false; 1227 if (!(other instanceof DiagnosticOrderItemComponent)) 1228 return false; 1229 DiagnosticOrderItemComponent o = (DiagnosticOrderItemComponent) other; 1230 return compareValues(status, o.status, true); 1231 } 1232 1233 public boolean isEmpty() { 1234 return super.isEmpty() && (code == null || code.isEmpty()) && (specimen == null || specimen.isEmpty()) 1235 && (bodySite == null || bodySite.isEmpty()) && (status == null || status.isEmpty()) 1236 && (event == null || event.isEmpty()); 1237 } 1238 1239 public String fhirType() { 1240 return "DiagnosticOrder.item"; 1241 1242 } 1243 1244 } 1245 1246 /** 1247 * Who or what the investigation is to be performed on. This is usually a human 1248 * patient, but diagnostic tests can also be requested on animals, groups of 1249 * humans or animals, devices such as dialysis machines, or even locations 1250 * (typically for environmental scans). 1251 */ 1252 @Child(name = "subject", type = { Patient.class, Group.class, Location.class, 1253 Device.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 1254 @Description(shortDefinition = "Who and/or what test is about", formalDefinition = "Who or what the investigation is to be performed on. This is usually a human patient, but diagnostic tests can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).") 1255 protected Reference subject; 1256 1257 /** 1258 * The actual object that is the target of the reference (Who or what the 1259 * investigation is to be performed on. This is usually a human patient, but 1260 * diagnostic tests can also be requested on animals, groups of humans or 1261 * animals, devices such as dialysis machines, or even locations (typically for 1262 * environmental scans).) 1263 */ 1264 protected Resource subjectTarget; 1265 1266 /** 1267 * The practitioner that holds legal responsibility for ordering the 1268 * investigation. 1269 */ 1270 @Child(name = "orderer", type = { Practitioner.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1271 @Description(shortDefinition = "Who ordered the test", formalDefinition = "The practitioner that holds legal responsibility for ordering the investigation.") 1272 protected Reference orderer; 1273 1274 /** 1275 * The actual object that is the target of the reference (The practitioner that 1276 * holds legal responsibility for ordering the investigation.) 1277 */ 1278 protected Practitioner ordererTarget; 1279 1280 /** 1281 * Identifiers assigned to this order instance by the orderer and/or the 1282 * receiver and/or order fulfiller. 1283 */ 1284 @Child(name = "identifier", type = { 1285 Identifier.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1286 @Description(shortDefinition = "Identifiers assigned to this order", formalDefinition = "Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller.") 1287 protected List<Identifier> identifier; 1288 1289 /** 1290 * An encounter that provides additional information about the healthcare 1291 * context in which this request is made. 1292 */ 1293 @Child(name = "encounter", type = { Encounter.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1294 @Description(shortDefinition = "The encounter that this diagnostic order is associated with", formalDefinition = "An encounter that provides additional information about the healthcare context in which this request is made.") 1295 protected Reference encounter; 1296 1297 /** 1298 * The actual object that is the target of the reference (An encounter that 1299 * provides additional information about the healthcare context in which this 1300 * request is made.) 1301 */ 1302 protected Encounter encounterTarget; 1303 1304 /** 1305 * An explanation or justification for why this diagnostic investigation is 1306 * being requested. This is often for billing purposes. May relate to the 1307 * resources referred to in supportingInformation. 1308 */ 1309 @Child(name = "reason", type = { 1310 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1311 @Description(shortDefinition = "Explanation/Justification for test", formalDefinition = "An explanation or justification for why this diagnostic investigation is being requested. This is often for billing purposes. May relate to the resources referred to in supportingInformation.") 1312 protected List<CodeableConcept> reason; 1313 1314 /** 1315 * Additional clinical information about the patient or specimen that may 1316 * influence test interpretations. This includes observations explicitly 1317 * requested by the producer(filler) to provide context or supporting 1318 * information needed to complete the order. 1319 */ 1320 @Child(name = "supportingInformation", type = { Observation.class, Condition.class, 1321 DocumentReference.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1322 @Description(shortDefinition = "Additional clinical information", formalDefinition = "Additional clinical information about the patient or specimen that may influence test interpretations. This includes observations explicitly requested by the producer(filler) to provide context or supporting information needed to complete the order.") 1323 protected List<Reference> supportingInformation; 1324 /** 1325 * The actual objects that are the target of the reference (Additional clinical 1326 * information about the patient or specimen that may influence test 1327 * interpretations. This includes observations explicitly requested by the 1328 * producer(filler) to provide context or supporting information needed to 1329 * complete the order.) 1330 */ 1331 protected List<Resource> supportingInformationTarget; 1332 1333 /** 1334 * One or more specimens that the diagnostic investigation is about. 1335 */ 1336 @Child(name = "specimen", type = { 1337 Specimen.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1338 @Description(shortDefinition = "If the whole order relates to specific specimens", formalDefinition = "One or more specimens that the diagnostic investigation is about.") 1339 protected List<Reference> specimen; 1340 /** 1341 * The actual objects that are the target of the reference (One or more 1342 * specimens that the diagnostic investigation is about.) 1343 */ 1344 protected List<Specimen> specimenTarget; 1345 1346 /** 1347 * The status of the order. 1348 */ 1349 @Child(name = "status", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = true, summary = true) 1350 @Description(shortDefinition = "proposed | draft | planned | requested | received | accepted | in-progress | review | completed | cancelled | suspended | rejected | failed", formalDefinition = "The status of the order.") 1351 protected Enumeration<DiagnosticOrderStatus> status; 1352 1353 /** 1354 * The clinical priority associated with this order. 1355 */ 1356 @Child(name = "priority", type = { CodeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1357 @Description(shortDefinition = "routine | urgent | stat | asap", formalDefinition = "The clinical priority associated with this order.") 1358 protected Enumeration<DiagnosticOrderPriority> priority; 1359 1360 /** 1361 * A summary of the events of interest that have occurred as the request is 1362 * processed; e.g. when the order was made, various processing steps (specimens 1363 * received), when it was completed. 1364 */ 1365 @Child(name = "event", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1366 @Description(shortDefinition = "A list of events of interest in the lifecycle", formalDefinition = "A summary of the events of interest that have occurred as the request is processed; e.g. when the order was made, various processing steps (specimens received), when it was completed.") 1367 protected List<DiagnosticOrderEventComponent> event; 1368 1369 /** 1370 * The specific diagnostic investigations that are requested as part of this 1371 * request. Sometimes, there can only be one item per request, but in most 1372 * contexts, more than one investigation can be requested. 1373 */ 1374 @Child(name = "item", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1375 @Description(shortDefinition = "The items the orderer requested", formalDefinition = "The specific diagnostic investigations that are requested as part of this request. Sometimes, there can only be one item per request, but in most contexts, more than one investigation can be requested.") 1376 protected List<DiagnosticOrderItemComponent> item; 1377 1378 /** 1379 * Any other notes associated with this patient, specimen or order (e.g. 1380 * "patient hates needles"). 1381 */ 1382 @Child(name = "note", type = { 1383 Annotation.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1384 @Description(shortDefinition = "Other notes and comments", formalDefinition = "Any other notes associated with this patient, specimen or order (e.g. \"patient hates needles\").") 1385 protected List<Annotation> note; 1386 1387 private static final long serialVersionUID = 700891227L; 1388 1389 /* 1390 * Constructor 1391 */ 1392 public DiagnosticOrder() { 1393 super(); 1394 } 1395 1396 /* 1397 * Constructor 1398 */ 1399 public DiagnosticOrder(Reference subject) { 1400 super(); 1401 this.subject = subject; 1402 } 1403 1404 /** 1405 * @return {@link #subject} (Who or what the investigation is to be performed 1406 * on. This is usually a human patient, but diagnostic tests can also be 1407 * requested on animals, groups of humans or animals, devices such as 1408 * dialysis machines, or even locations (typically for environmental 1409 * scans).) 1410 */ 1411 public Reference getSubject() { 1412 if (this.subject == null) 1413 if (Configuration.errorOnAutoCreate()) 1414 throw new Error("Attempt to auto-create DiagnosticOrder.subject"); 1415 else if (Configuration.doAutoCreate()) 1416 this.subject = new Reference(); // cc 1417 return this.subject; 1418 } 1419 1420 public boolean hasSubject() { 1421 return this.subject != null && !this.subject.isEmpty(); 1422 } 1423 1424 /** 1425 * @param value {@link #subject} (Who or what the investigation is to be 1426 * performed on. This is usually a human patient, but diagnostic 1427 * tests can also be requested on animals, groups of humans or 1428 * animals, devices such as dialysis machines, or even locations 1429 * (typically for environmental scans).) 1430 */ 1431 public DiagnosticOrder setSubject(Reference value) { 1432 this.subject = value; 1433 return this; 1434 } 1435 1436 /** 1437 * @return {@link #subject} The actual object that is the target of the 1438 * reference. The reference library doesn't populate this, but you can 1439 * use it to hold the resource if you resolve it. (Who or what the 1440 * investigation is to be performed on. This is usually a human patient, 1441 * but diagnostic tests can also be requested on animals, groups of 1442 * humans or animals, devices such as dialysis machines, or even 1443 * locations (typically for environmental scans).) 1444 */ 1445 public Resource getSubjectTarget() { 1446 return this.subjectTarget; 1447 } 1448 1449 /** 1450 * @param value {@link #subject} The actual object that is the target of the 1451 * reference. The reference library doesn't use these, but you can 1452 * use it to hold the resource if you resolve it. (Who or what the 1453 * investigation is to be performed on. This is usually a human 1454 * patient, but diagnostic tests can also be requested on animals, 1455 * groups of humans or animals, devices such as dialysis machines, 1456 * or even locations (typically for environmental scans).) 1457 */ 1458 public DiagnosticOrder setSubjectTarget(Resource value) { 1459 this.subjectTarget = value; 1460 return this; 1461 } 1462 1463 /** 1464 * @return {@link #orderer} (The practitioner that holds legal responsibility 1465 * for ordering the investigation.) 1466 */ 1467 public Reference getOrderer() { 1468 if (this.orderer == null) 1469 if (Configuration.errorOnAutoCreate()) 1470 throw new Error("Attempt to auto-create DiagnosticOrder.orderer"); 1471 else if (Configuration.doAutoCreate()) 1472 this.orderer = new Reference(); // cc 1473 return this.orderer; 1474 } 1475 1476 public boolean hasOrderer() { 1477 return this.orderer != null && !this.orderer.isEmpty(); 1478 } 1479 1480 /** 1481 * @param value {@link #orderer} (The practitioner that holds legal 1482 * responsibility for ordering the investigation.) 1483 */ 1484 public DiagnosticOrder setOrderer(Reference value) { 1485 this.orderer = value; 1486 return this; 1487 } 1488 1489 /** 1490 * @return {@link #orderer} The actual object that is the target of the 1491 * reference. The reference library doesn't populate this, but you can 1492 * use it to hold the resource if you resolve it. (The practitioner that 1493 * holds legal responsibility for ordering the investigation.) 1494 */ 1495 public Practitioner getOrdererTarget() { 1496 if (this.ordererTarget == null) 1497 if (Configuration.errorOnAutoCreate()) 1498 throw new Error("Attempt to auto-create DiagnosticOrder.orderer"); 1499 else if (Configuration.doAutoCreate()) 1500 this.ordererTarget = new Practitioner(); // aa 1501 return this.ordererTarget; 1502 } 1503 1504 /** 1505 * @param value {@link #orderer} The actual object that is the target of the 1506 * reference. The reference library doesn't use these, but you can 1507 * use it to hold the resource if you resolve it. (The practitioner 1508 * that holds legal responsibility for ordering the investigation.) 1509 */ 1510 public DiagnosticOrder setOrdererTarget(Practitioner value) { 1511 this.ordererTarget = value; 1512 return this; 1513 } 1514 1515 /** 1516 * @return {@link #identifier} (Identifiers assigned to this order instance by 1517 * the orderer and/or the receiver and/or order fulfiller.) 1518 */ 1519 public List<Identifier> getIdentifier() { 1520 if (this.identifier == null) 1521 this.identifier = new ArrayList<Identifier>(); 1522 return this.identifier; 1523 } 1524 1525 public boolean hasIdentifier() { 1526 if (this.identifier == null) 1527 return false; 1528 for (Identifier item : this.identifier) 1529 if (!item.isEmpty()) 1530 return true; 1531 return false; 1532 } 1533 1534 /** 1535 * @return {@link #identifier} (Identifiers assigned to this order instance by 1536 * the orderer and/or the receiver and/or order fulfiller.) 1537 */ 1538 // syntactic sugar 1539 public Identifier addIdentifier() { // 3 1540 Identifier t = new Identifier(); 1541 if (this.identifier == null) 1542 this.identifier = new ArrayList<Identifier>(); 1543 this.identifier.add(t); 1544 return t; 1545 } 1546 1547 // syntactic sugar 1548 public DiagnosticOrder addIdentifier(Identifier t) { // 3 1549 if (t == null) 1550 return this; 1551 if (this.identifier == null) 1552 this.identifier = new ArrayList<Identifier>(); 1553 this.identifier.add(t); 1554 return this; 1555 } 1556 1557 /** 1558 * @return {@link #encounter} (An encounter that provides additional information 1559 * about the healthcare context in which this request is made.) 1560 */ 1561 public Reference getEncounter() { 1562 if (this.encounter == null) 1563 if (Configuration.errorOnAutoCreate()) 1564 throw new Error("Attempt to auto-create DiagnosticOrder.encounter"); 1565 else if (Configuration.doAutoCreate()) 1566 this.encounter = new Reference(); // cc 1567 return this.encounter; 1568 } 1569 1570 public boolean hasEncounter() { 1571 return this.encounter != null && !this.encounter.isEmpty(); 1572 } 1573 1574 /** 1575 * @param value {@link #encounter} (An encounter that provides additional 1576 * information about the healthcare context in which this request 1577 * is made.) 1578 */ 1579 public DiagnosticOrder setEncounter(Reference value) { 1580 this.encounter = value; 1581 return this; 1582 } 1583 1584 /** 1585 * @return {@link #encounter} The actual object that is the target of the 1586 * reference. The reference library doesn't populate this, but you can 1587 * use it to hold the resource if you resolve it. (An encounter that 1588 * provides additional information about the healthcare context in which 1589 * this request is made.) 1590 */ 1591 public Encounter getEncounterTarget() { 1592 if (this.encounterTarget == null) 1593 if (Configuration.errorOnAutoCreate()) 1594 throw new Error("Attempt to auto-create DiagnosticOrder.encounter"); 1595 else if (Configuration.doAutoCreate()) 1596 this.encounterTarget = new Encounter(); // aa 1597 return this.encounterTarget; 1598 } 1599 1600 /** 1601 * @param value {@link #encounter} The actual object that is the target of the 1602 * reference. The reference library doesn't use these, but you can 1603 * use it to hold the resource if you resolve it. (An encounter 1604 * that provides additional information about the healthcare 1605 * context in which this request is made.) 1606 */ 1607 public DiagnosticOrder setEncounterTarget(Encounter value) { 1608 this.encounterTarget = value; 1609 return this; 1610 } 1611 1612 /** 1613 * @return {@link #reason} (An explanation or justification for why this 1614 * diagnostic investigation is being requested. This is often for 1615 * billing purposes. May relate to the resources referred to in 1616 * supportingInformation.) 1617 */ 1618 public List<CodeableConcept> getReason() { 1619 if (this.reason == null) 1620 this.reason = new ArrayList<CodeableConcept>(); 1621 return this.reason; 1622 } 1623 1624 public boolean hasReason() { 1625 if (this.reason == null) 1626 return false; 1627 for (CodeableConcept item : this.reason) 1628 if (!item.isEmpty()) 1629 return true; 1630 return false; 1631 } 1632 1633 /** 1634 * @return {@link #reason} (An explanation or justification for why this 1635 * diagnostic investigation is being requested. This is often for 1636 * billing purposes. May relate to the resources referred to in 1637 * supportingInformation.) 1638 */ 1639 // syntactic sugar 1640 public CodeableConcept addReason() { // 3 1641 CodeableConcept t = new CodeableConcept(); 1642 if (this.reason == null) 1643 this.reason = new ArrayList<CodeableConcept>(); 1644 this.reason.add(t); 1645 return t; 1646 } 1647 1648 // syntactic sugar 1649 public DiagnosticOrder addReason(CodeableConcept t) { // 3 1650 if (t == null) 1651 return this; 1652 if (this.reason == null) 1653 this.reason = new ArrayList<CodeableConcept>(); 1654 this.reason.add(t); 1655 return this; 1656 } 1657 1658 /** 1659 * @return {@link #supportingInformation} (Additional clinical information about 1660 * the patient or specimen that may influence test interpretations. This 1661 * includes observations explicitly requested by the producer(filler) to 1662 * provide context or supporting information needed to complete the 1663 * order.) 1664 */ 1665 public List<Reference> getSupportingInformation() { 1666 if (this.supportingInformation == null) 1667 this.supportingInformation = new ArrayList<Reference>(); 1668 return this.supportingInformation; 1669 } 1670 1671 public boolean hasSupportingInformation() { 1672 if (this.supportingInformation == null) 1673 return false; 1674 for (Reference item : this.supportingInformation) 1675 if (!item.isEmpty()) 1676 return true; 1677 return false; 1678 } 1679 1680 /** 1681 * @return {@link #supportingInformation} (Additional clinical information about 1682 * the patient or specimen that may influence test interpretations. This 1683 * includes observations explicitly requested by the producer(filler) to 1684 * provide context or supporting information needed to complete the 1685 * order.) 1686 */ 1687 // syntactic sugar 1688 public Reference addSupportingInformation() { // 3 1689 Reference t = new Reference(); 1690 if (this.supportingInformation == null) 1691 this.supportingInformation = new ArrayList<Reference>(); 1692 this.supportingInformation.add(t); 1693 return t; 1694 } 1695 1696 // syntactic sugar 1697 public DiagnosticOrder addSupportingInformation(Reference t) { // 3 1698 if (t == null) 1699 return this; 1700 if (this.supportingInformation == null) 1701 this.supportingInformation = new ArrayList<Reference>(); 1702 this.supportingInformation.add(t); 1703 return this; 1704 } 1705 1706 /** 1707 * @return {@link #supportingInformation} (The actual objects that are the 1708 * target of the reference. The reference library doesn't populate this, 1709 * but you can use this to hold the resources if you resolvethemt. 1710 * Additional clinical information about the patient or specimen that 1711 * may influence test interpretations. This includes observations 1712 * explicitly requested by the producer(filler) to provide context or 1713 * supporting information needed to complete the order.) 1714 */ 1715 public List<Resource> getSupportingInformationTarget() { 1716 if (this.supportingInformationTarget == null) 1717 this.supportingInformationTarget = new ArrayList<Resource>(); 1718 return this.supportingInformationTarget; 1719 } 1720 1721 /** 1722 * @return {@link #specimen} (One or more specimens that the diagnostic 1723 * investigation is about.) 1724 */ 1725 public List<Reference> getSpecimen() { 1726 if (this.specimen == null) 1727 this.specimen = new ArrayList<Reference>(); 1728 return this.specimen; 1729 } 1730 1731 public boolean hasSpecimen() { 1732 if (this.specimen == null) 1733 return false; 1734 for (Reference item : this.specimen) 1735 if (!item.isEmpty()) 1736 return true; 1737 return false; 1738 } 1739 1740 /** 1741 * @return {@link #specimen} (One or more specimens that the diagnostic 1742 * investigation is about.) 1743 */ 1744 // syntactic sugar 1745 public Reference addSpecimen() { // 3 1746 Reference t = new Reference(); 1747 if (this.specimen == null) 1748 this.specimen = new ArrayList<Reference>(); 1749 this.specimen.add(t); 1750 return t; 1751 } 1752 1753 // syntactic sugar 1754 public DiagnosticOrder addSpecimen(Reference t) { // 3 1755 if (t == null) 1756 return this; 1757 if (this.specimen == null) 1758 this.specimen = new ArrayList<Reference>(); 1759 this.specimen.add(t); 1760 return this; 1761 } 1762 1763 /** 1764 * @return {@link #specimen} (The actual objects that are the target of the 1765 * reference. The reference library doesn't populate this, but you can 1766 * use this to hold the resources if you resolvethemt. One or more 1767 * specimens that the diagnostic investigation is about.) 1768 */ 1769 public List<Specimen> getSpecimenTarget() { 1770 if (this.specimenTarget == null) 1771 this.specimenTarget = new ArrayList<Specimen>(); 1772 return this.specimenTarget; 1773 } 1774 1775 // syntactic sugar 1776 /** 1777 * @return {@link #specimen} (Add an actual object that is the target of the 1778 * reference. The reference library doesn't use these, but you can use 1779 * this to hold the resources if you resolvethemt. One or more specimens 1780 * that the diagnostic investigation is about.) 1781 */ 1782 public Specimen addSpecimenTarget() { 1783 Specimen r = new Specimen(); 1784 if (this.specimenTarget == null) 1785 this.specimenTarget = new ArrayList<Specimen>(); 1786 this.specimenTarget.add(r); 1787 return r; 1788 } 1789 1790 /** 1791 * @return {@link #status} (The status of the order.). This is the underlying 1792 * object with id, value and extensions. The accessor "getStatus" gives 1793 * direct access to the value 1794 */ 1795 public Enumeration<DiagnosticOrderStatus> getStatusElement() { 1796 if (this.status == null) 1797 if (Configuration.errorOnAutoCreate()) 1798 throw new Error("Attempt to auto-create DiagnosticOrder.status"); 1799 else if (Configuration.doAutoCreate()) 1800 this.status = new Enumeration<DiagnosticOrderStatus>(new DiagnosticOrderStatusEnumFactory()); // bb 1801 return this.status; 1802 } 1803 1804 public boolean hasStatusElement() { 1805 return this.status != null && !this.status.isEmpty(); 1806 } 1807 1808 public boolean hasStatus() { 1809 return this.status != null && !this.status.isEmpty(); 1810 } 1811 1812 /** 1813 * @param value {@link #status} (The status of the order.). This is the 1814 * underlying object with id, value and extensions. The accessor 1815 * "getStatus" gives direct access to the value 1816 */ 1817 public DiagnosticOrder setStatusElement(Enumeration<DiagnosticOrderStatus> value) { 1818 this.status = value; 1819 return this; 1820 } 1821 1822 /** 1823 * @return The status of the order. 1824 */ 1825 public DiagnosticOrderStatus getStatus() { 1826 return this.status == null ? null : this.status.getValue(); 1827 } 1828 1829 /** 1830 * @param value The status of the order. 1831 */ 1832 public DiagnosticOrder setStatus(DiagnosticOrderStatus value) { 1833 if (value == null) 1834 this.status = null; 1835 else { 1836 if (this.status == null) 1837 this.status = new Enumeration<DiagnosticOrderStatus>(new DiagnosticOrderStatusEnumFactory()); 1838 this.status.setValue(value); 1839 } 1840 return this; 1841 } 1842 1843 /** 1844 * @return {@link #priority} (The clinical priority associated with this 1845 * order.). This is the underlying object with id, value and extensions. 1846 * The accessor "getPriority" gives direct access to the value 1847 */ 1848 public Enumeration<DiagnosticOrderPriority> getPriorityElement() { 1849 if (this.priority == null) 1850 if (Configuration.errorOnAutoCreate()) 1851 throw new Error("Attempt to auto-create DiagnosticOrder.priority"); 1852 else if (Configuration.doAutoCreate()) 1853 this.priority = new Enumeration<DiagnosticOrderPriority>(new DiagnosticOrderPriorityEnumFactory()); // bb 1854 return this.priority; 1855 } 1856 1857 public boolean hasPriorityElement() { 1858 return this.priority != null && !this.priority.isEmpty(); 1859 } 1860 1861 public boolean hasPriority() { 1862 return this.priority != null && !this.priority.isEmpty(); 1863 } 1864 1865 /** 1866 * @param value {@link #priority} (The clinical priority associated with this 1867 * order.). This is the underlying object with id, value and 1868 * extensions. The accessor "getPriority" gives direct access to 1869 * the value 1870 */ 1871 public DiagnosticOrder setPriorityElement(Enumeration<DiagnosticOrderPriority> value) { 1872 this.priority = value; 1873 return this; 1874 } 1875 1876 /** 1877 * @return The clinical priority associated with this order. 1878 */ 1879 public DiagnosticOrderPriority getPriority() { 1880 return this.priority == null ? null : this.priority.getValue(); 1881 } 1882 1883 /** 1884 * @param value The clinical priority associated with this order. 1885 */ 1886 public DiagnosticOrder setPriority(DiagnosticOrderPriority value) { 1887 if (value == null) 1888 this.priority = null; 1889 else { 1890 if (this.priority == null) 1891 this.priority = new Enumeration<DiagnosticOrderPriority>(new DiagnosticOrderPriorityEnumFactory()); 1892 this.priority.setValue(value); 1893 } 1894 return this; 1895 } 1896 1897 /** 1898 * @return {@link #event} (A summary of the events of interest that have 1899 * occurred as the request is processed; e.g. when the order was made, 1900 * various processing steps (specimens received), when it was 1901 * completed.) 1902 */ 1903 public List<DiagnosticOrderEventComponent> getEvent() { 1904 if (this.event == null) 1905 this.event = new ArrayList<DiagnosticOrderEventComponent>(); 1906 return this.event; 1907 } 1908 1909 public boolean hasEvent() { 1910 if (this.event == null) 1911 return false; 1912 for (DiagnosticOrderEventComponent item : this.event) 1913 if (!item.isEmpty()) 1914 return true; 1915 return false; 1916 } 1917 1918 /** 1919 * @return {@link #event} (A summary of the events of interest that have 1920 * occurred as the request is processed; e.g. when the order was made, 1921 * various processing steps (specimens received), when it was 1922 * completed.) 1923 */ 1924 // syntactic sugar 1925 public DiagnosticOrderEventComponent addEvent() { // 3 1926 DiagnosticOrderEventComponent t = new DiagnosticOrderEventComponent(); 1927 if (this.event == null) 1928 this.event = new ArrayList<DiagnosticOrderEventComponent>(); 1929 this.event.add(t); 1930 return t; 1931 } 1932 1933 // syntactic sugar 1934 public DiagnosticOrder addEvent(DiagnosticOrderEventComponent t) { // 3 1935 if (t == null) 1936 return this; 1937 if (this.event == null) 1938 this.event = new ArrayList<DiagnosticOrderEventComponent>(); 1939 this.event.add(t); 1940 return this; 1941 } 1942 1943 /** 1944 * @return {@link #item} (The specific diagnostic investigations that are 1945 * requested as part of this request. Sometimes, there can only be one 1946 * item per request, but in most contexts, more than one investigation 1947 * can be requested.) 1948 */ 1949 public List<DiagnosticOrderItemComponent> getItem() { 1950 if (this.item == null) 1951 this.item = new ArrayList<DiagnosticOrderItemComponent>(); 1952 return this.item; 1953 } 1954 1955 public boolean hasItem() { 1956 if (this.item == null) 1957 return false; 1958 for (DiagnosticOrderItemComponent item : this.item) 1959 if (!item.isEmpty()) 1960 return true; 1961 return false; 1962 } 1963 1964 /** 1965 * @return {@link #item} (The specific diagnostic investigations that are 1966 * requested as part of this request. Sometimes, there can only be one 1967 * item per request, but in most contexts, more than one investigation 1968 * can be requested.) 1969 */ 1970 // syntactic sugar 1971 public DiagnosticOrderItemComponent addItem() { // 3 1972 DiagnosticOrderItemComponent t = new DiagnosticOrderItemComponent(); 1973 if (this.item == null) 1974 this.item = new ArrayList<DiagnosticOrderItemComponent>(); 1975 this.item.add(t); 1976 return t; 1977 } 1978 1979 // syntactic sugar 1980 public DiagnosticOrder addItem(DiagnosticOrderItemComponent t) { // 3 1981 if (t == null) 1982 return this; 1983 if (this.item == null) 1984 this.item = new ArrayList<DiagnosticOrderItemComponent>(); 1985 this.item.add(t); 1986 return this; 1987 } 1988 1989 /** 1990 * @return {@link #note} (Any other notes associated with this patient, specimen 1991 * or order (e.g. "patient hates needles").) 1992 */ 1993 public List<Annotation> getNote() { 1994 if (this.note == null) 1995 this.note = new ArrayList<Annotation>(); 1996 return this.note; 1997 } 1998 1999 public boolean hasNote() { 2000 if (this.note == null) 2001 return false; 2002 for (Annotation item : this.note) 2003 if (!item.isEmpty()) 2004 return true; 2005 return false; 2006 } 2007 2008 /** 2009 * @return {@link #note} (Any other notes associated with this patient, specimen 2010 * or order (e.g. "patient hates needles").) 2011 */ 2012 // syntactic sugar 2013 public Annotation addNote() { // 3 2014 Annotation t = new Annotation(); 2015 if (this.note == null) 2016 this.note = new ArrayList<Annotation>(); 2017 this.note.add(t); 2018 return t; 2019 } 2020 2021 // syntactic sugar 2022 public DiagnosticOrder addNote(Annotation t) { // 3 2023 if (t == null) 2024 return this; 2025 if (this.note == null) 2026 this.note = new ArrayList<Annotation>(); 2027 this.note.add(t); 2028 return this; 2029 } 2030 2031 protected void listChildren(List<Property> childrenList) { 2032 super.listChildren(childrenList); 2033 childrenList.add(new Property("subject", "Reference(Patient|Group|Location|Device)", 2034 "Who or what the investigation is to be performed on. This is usually a human patient, but diagnostic tests can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).", 2035 0, java.lang.Integer.MAX_VALUE, subject)); 2036 childrenList.add(new Property("orderer", "Reference(Practitioner)", 2037 "The practitioner that holds legal responsibility for ordering the investigation.", 0, 2038 java.lang.Integer.MAX_VALUE, orderer)); 2039 childrenList.add(new Property("identifier", "Identifier", 2040 "Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller.", 0, 2041 java.lang.Integer.MAX_VALUE, identifier)); 2042 childrenList.add(new Property("encounter", "Reference(Encounter)", 2043 "An encounter that provides additional information about the healthcare context in which this request is made.", 2044 0, java.lang.Integer.MAX_VALUE, encounter)); 2045 childrenList.add(new Property("reason", "CodeableConcept", 2046 "An explanation or justification for why this diagnostic investigation is being requested. This is often for billing purposes. May relate to the resources referred to in supportingInformation.", 2047 0, java.lang.Integer.MAX_VALUE, reason)); 2048 childrenList.add(new Property("supportingInformation", "Reference(Observation|Condition|DocumentReference)", 2049 "Additional clinical information about the patient or specimen that may influence test interpretations. This includes observations explicitly requested by the producer(filler) to provide context or supporting information needed to complete the order.", 2050 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 2051 childrenList.add(new Property("specimen", "Reference(Specimen)", 2052 "One or more specimens that the diagnostic investigation is about.", 0, java.lang.Integer.MAX_VALUE, specimen)); 2053 childrenList 2054 .add(new Property("status", "code", "The status of the order.", 0, java.lang.Integer.MAX_VALUE, status)); 2055 childrenList.add(new Property("priority", "code", "The clinical priority associated with this order.", 0, 2056 java.lang.Integer.MAX_VALUE, priority)); 2057 childrenList.add(new Property("event", "", 2058 "A summary of the events of interest that have occurred as the request is processed; e.g. when the order was made, various processing steps (specimens received), when it was completed.", 2059 0, java.lang.Integer.MAX_VALUE, event)); 2060 childrenList.add(new Property("item", "", 2061 "The specific diagnostic investigations that are requested as part of this request. Sometimes, there can only be one item per request, but in most contexts, more than one investigation can be requested.", 2062 0, java.lang.Integer.MAX_VALUE, item)); 2063 childrenList.add(new Property("note", "Annotation", 2064 "Any other notes associated with this patient, specimen or order (e.g. \"patient hates needles\").", 0, 2065 java.lang.Integer.MAX_VALUE, note)); 2066 } 2067 2068 @Override 2069 public void setProperty(String name, Base value) throws FHIRException { 2070 if (name.equals("subject")) 2071 this.subject = castToReference(value); // Reference 2072 else if (name.equals("orderer")) 2073 this.orderer = castToReference(value); // Reference 2074 else if (name.equals("identifier")) 2075 this.getIdentifier().add(castToIdentifier(value)); 2076 else if (name.equals("encounter")) 2077 this.encounter = castToReference(value); // Reference 2078 else if (name.equals("reason")) 2079 this.getReason().add(castToCodeableConcept(value)); 2080 else if (name.equals("supportingInformation")) 2081 this.getSupportingInformation().add(castToReference(value)); 2082 else if (name.equals("specimen")) 2083 this.getSpecimen().add(castToReference(value)); 2084 else if (name.equals("status")) 2085 this.status = new DiagnosticOrderStatusEnumFactory().fromType(value); // Enumeration<DiagnosticOrderStatus> 2086 else if (name.equals("priority")) 2087 this.priority = new DiagnosticOrderPriorityEnumFactory().fromType(value); // Enumeration<DiagnosticOrderPriority> 2088 else if (name.equals("event")) 2089 this.getEvent().add((DiagnosticOrderEventComponent) value); 2090 else if (name.equals("item")) 2091 this.getItem().add((DiagnosticOrderItemComponent) value); 2092 else if (name.equals("note")) 2093 this.getNote().add(castToAnnotation(value)); 2094 else 2095 super.setProperty(name, value); 2096 } 2097 2098 @Override 2099 public Base addChild(String name) throws FHIRException { 2100 if (name.equals("subject")) { 2101 this.subject = new Reference(); 2102 return this.subject; 2103 } else if (name.equals("orderer")) { 2104 this.orderer = new Reference(); 2105 return this.orderer; 2106 } else if (name.equals("identifier")) { 2107 return addIdentifier(); 2108 } else if (name.equals("encounter")) { 2109 this.encounter = new Reference(); 2110 return this.encounter; 2111 } else if (name.equals("reason")) { 2112 return addReason(); 2113 } else if (name.equals("supportingInformation")) { 2114 return addSupportingInformation(); 2115 } else if (name.equals("specimen")) { 2116 return addSpecimen(); 2117 } else if (name.equals("status")) { 2118 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticOrder.status"); 2119 } else if (name.equals("priority")) { 2120 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticOrder.priority"); 2121 } else if (name.equals("event")) { 2122 return addEvent(); 2123 } else if (name.equals("item")) { 2124 return addItem(); 2125 } else if (name.equals("note")) { 2126 return addNote(); 2127 } else 2128 return super.addChild(name); 2129 } 2130 2131 public String fhirType() { 2132 return "DiagnosticOrder"; 2133 2134 } 2135 2136 public DiagnosticOrder copy() { 2137 DiagnosticOrder dst = new DiagnosticOrder(); 2138 copyValues(dst); 2139 dst.subject = subject == null ? null : subject.copy(); 2140 dst.orderer = orderer == null ? null : orderer.copy(); 2141 if (identifier != null) { 2142 dst.identifier = new ArrayList<Identifier>(); 2143 for (Identifier i : identifier) 2144 dst.identifier.add(i.copy()); 2145 } 2146 ; 2147 dst.encounter = encounter == null ? null : encounter.copy(); 2148 if (reason != null) { 2149 dst.reason = new ArrayList<CodeableConcept>(); 2150 for (CodeableConcept i : reason) 2151 dst.reason.add(i.copy()); 2152 } 2153 ; 2154 if (supportingInformation != null) { 2155 dst.supportingInformation = new ArrayList<Reference>(); 2156 for (Reference i : supportingInformation) 2157 dst.supportingInformation.add(i.copy()); 2158 } 2159 ; 2160 if (specimen != null) { 2161 dst.specimen = new ArrayList<Reference>(); 2162 for (Reference i : specimen) 2163 dst.specimen.add(i.copy()); 2164 } 2165 ; 2166 dst.status = status == null ? null : status.copy(); 2167 dst.priority = priority == null ? null : priority.copy(); 2168 if (event != null) { 2169 dst.event = new ArrayList<DiagnosticOrderEventComponent>(); 2170 for (DiagnosticOrderEventComponent i : event) 2171 dst.event.add(i.copy()); 2172 } 2173 ; 2174 if (item != null) { 2175 dst.item = new ArrayList<DiagnosticOrderItemComponent>(); 2176 for (DiagnosticOrderItemComponent i : item) 2177 dst.item.add(i.copy()); 2178 } 2179 ; 2180 if (note != null) { 2181 dst.note = new ArrayList<Annotation>(); 2182 for (Annotation i : note) 2183 dst.note.add(i.copy()); 2184 } 2185 ; 2186 return dst; 2187 } 2188 2189 protected DiagnosticOrder typedCopy() { 2190 return copy(); 2191 } 2192 2193 @Override 2194 public boolean equalsDeep(Base other) { 2195 if (!super.equalsDeep(other)) 2196 return false; 2197 if (!(other instanceof DiagnosticOrder)) 2198 return false; 2199 DiagnosticOrder o = (DiagnosticOrder) other; 2200 return compareDeep(subject, o.subject, true) && compareDeep(orderer, o.orderer, true) 2201 && compareDeep(identifier, o.identifier, true) && compareDeep(encounter, o.encounter, true) 2202 && compareDeep(reason, o.reason, true) && compareDeep(supportingInformation, o.supportingInformation, true) 2203 && compareDeep(specimen, o.specimen, true) && compareDeep(status, o.status, true) 2204 && compareDeep(priority, o.priority, true) && compareDeep(event, o.event, true) 2205 && compareDeep(item, o.item, true) && compareDeep(note, o.note, true); 2206 } 2207 2208 @Override 2209 public boolean equalsShallow(Base other) { 2210 if (!super.equalsShallow(other)) 2211 return false; 2212 if (!(other instanceof DiagnosticOrder)) 2213 return false; 2214 DiagnosticOrder o = (DiagnosticOrder) other; 2215 return compareValues(status, o.status, true) && compareValues(priority, o.priority, true); 2216 } 2217 2218 public boolean isEmpty() { 2219 return super.isEmpty() && (subject == null || subject.isEmpty()) && (orderer == null || orderer.isEmpty()) 2220 && (identifier == null || identifier.isEmpty()) && (encounter == null || encounter.isEmpty()) 2221 && (reason == null || reason.isEmpty()) && (supportingInformation == null || supportingInformation.isEmpty()) 2222 && (specimen == null || specimen.isEmpty()) && (status == null || status.isEmpty()) 2223 && (priority == null || priority.isEmpty()) && (event == null || event.isEmpty()) 2224 && (item == null || item.isEmpty()) && (note == null || note.isEmpty()); 2225 } 2226 2227 @Override 2228 public ResourceType getResourceType() { 2229 return ResourceType.DiagnosticOrder; 2230 } 2231 2232 @SearchParamDefinition(name = "item-past-status", path = "DiagnosticOrder.item.event.status", description = "proposed | draft | planned | requested | received | accepted | in-progress | review | completed | cancelled | suspended | rejected | failed", type = "token") 2233 public static final String SP_ITEMPASTSTATUS = "item-past-status"; 2234 @SearchParamDefinition(name = "identifier", path = "DiagnosticOrder.identifier", description = "Identifiers assigned to this order", type = "token") 2235 public static final String SP_IDENTIFIER = "identifier"; 2236 @SearchParamDefinition(name = "bodysite", path = "DiagnosticOrder.item.bodySite", description = "Location of requested test (if applicable)", type = "token") 2237 public static final String SP_BODYSITE = "bodysite"; 2238 @SearchParamDefinition(name = "code", path = "DiagnosticOrder.item.code", description = "Code to indicate the item (test or panel) being ordered", type = "token") 2239 public static final String SP_CODE = "code"; 2240 @SearchParamDefinition(name = "event-date", path = "DiagnosticOrder.event.dateTime", description = "The date at which the event happened", type = "date") 2241 public static final String SP_EVENTDATE = "event-date"; 2242 @SearchParamDefinition(name = "event-status-date", path = "null", description = "A combination of past-status and date", type = "composite") 2243 public static final String SP_EVENTSTATUSDATE = "event-status-date"; 2244 @SearchParamDefinition(name = "subject", path = "DiagnosticOrder.subject", description = "Who and/or what test is about", type = "reference") 2245 public static final String SP_SUBJECT = "subject"; 2246 @SearchParamDefinition(name = "encounter", path = "DiagnosticOrder.encounter", description = "The encounter that this diagnostic order is associated with", type = "reference") 2247 public static final String SP_ENCOUNTER = "encounter"; 2248 @SearchParamDefinition(name = "actor", path = "DiagnosticOrder.event.actor | DiagnosticOrder.item.event.actor", description = "Who recorded or did this", type = "reference") 2249 public static final String SP_ACTOR = "actor"; 2250 @SearchParamDefinition(name = "item-date", path = "DiagnosticOrder.item.event.dateTime", description = "The date at which the event happened", type = "date") 2251 public static final String SP_ITEMDATE = "item-date"; 2252 @SearchParamDefinition(name = "item-status-date", path = "null", description = "A combination of item-past-status and item-date", type = "composite") 2253 public static final String SP_ITEMSTATUSDATE = "item-status-date"; 2254 @SearchParamDefinition(name = "event-status", path = "DiagnosticOrder.event.status", description = "proposed | draft | planned | requested | received | accepted | in-progress | review | completed | cancelled | suspended | rejected | failed", type = "token") 2255 public static final String SP_EVENTSTATUS = "event-status"; 2256 @SearchParamDefinition(name = "item-status", path = "DiagnosticOrder.item.status", description = "proposed | draft | planned | requested | received | accepted | in-progress | review | completed | cancelled | suspended | rejected | failed", type = "token") 2257 public static final String SP_ITEMSTATUS = "item-status"; 2258 @SearchParamDefinition(name = "patient", path = "DiagnosticOrder.subject", description = "Who and/or what test is about", type = "reference") 2259 public static final String SP_PATIENT = "patient"; 2260 @SearchParamDefinition(name = "orderer", path = "DiagnosticOrder.orderer", description = "Who ordered the test", type = "reference") 2261 public static final String SP_ORDERER = "orderer"; 2262 @SearchParamDefinition(name = "specimen", path = "DiagnosticOrder.specimen | DiagnosticOrder.item.specimen", description = "If the whole order relates to specific specimens", type = "reference") 2263 public static final String SP_SPECIMEN = "specimen"; 2264 @SearchParamDefinition(name = "status", path = "DiagnosticOrder.status", description = "proposed | draft | planned | requested | received | accepted | in-progress | review | completed | cancelled | suspended | rejected | failed", type = "token") 2265 public static final String SP_STATUS = "status"; 2266 2267}