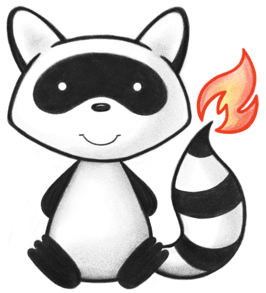
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * The findings and interpretation of diagnostic tests performed on patients, 048 * groups of patients, devices, and locations, and/or specimens derived from 049 * these. The report includes clinical context such as requesting and provider 050 * information, and some mix of atomic results, images, textual and coded 051 * interpretations, and formatted representation of diagnostic reports. 052 */ 053@ResourceDef(name = "DiagnosticReport", profile = "http://hl7.org/fhir/Profile/DiagnosticReport") 054public class DiagnosticReport extends DomainResource { 055 056 public enum DiagnosticReportStatus { 057 /** 058 * The existence of the report is registered, but there is nothing yet 059 * available. 060 */ 061 REGISTERED, 062 /** 063 * This is a partial (e.g. initial, interim or preliminary) report: data in the 064 * report may be incomplete or unverified. 065 */ 066 PARTIAL, 067 /** 068 * The report is complete and verified by an authorized person. 069 */ 070 FINAL, 071 /** 072 * The report has been modified subsequent to being Final, and is complete and 073 * verified by an authorized person. New content has been added, but existing 074 * content hasn't changed 075 */ 076 CORRECTED, 077 /** 078 * The report has been modified subsequent to being Final, and is complete and 079 * verified by an authorized person. New content has been added, but existing 080 * content hasn't changed. 081 */ 082 APPENDED, 083 /** 084 * The report is unavailable because the measurement was not started or not 085 * completed (also sometimes called "aborted"). 086 */ 087 CANCELLED, 088 /** 089 * The report has been withdrawn following a previous final release. 090 */ 091 ENTEREDINERROR, 092 /** 093 * added to help the parsers 094 */ 095 NULL; 096 097 public static DiagnosticReportStatus fromCode(String codeString) throws FHIRException { 098 if (codeString == null || "".equals(codeString)) 099 return null; 100 if ("registered".equals(codeString)) 101 return REGISTERED; 102 if ("partial".equals(codeString)) 103 return PARTIAL; 104 if ("final".equals(codeString)) 105 return FINAL; 106 if ("corrected".equals(codeString)) 107 return CORRECTED; 108 if ("appended".equals(codeString)) 109 return APPENDED; 110 if ("cancelled".equals(codeString)) 111 return CANCELLED; 112 if ("entered-in-error".equals(codeString)) 113 return ENTEREDINERROR; 114 throw new FHIRException("Unknown DiagnosticReportStatus code '" + codeString + "'"); 115 } 116 117 public String toCode() { 118 switch (this) { 119 case REGISTERED: 120 return "registered"; 121 case PARTIAL: 122 return "partial"; 123 case FINAL: 124 return "final"; 125 case CORRECTED: 126 return "corrected"; 127 case APPENDED: 128 return "appended"; 129 case CANCELLED: 130 return "cancelled"; 131 case ENTEREDINERROR: 132 return "entered-in-error"; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getSystem() { 141 switch (this) { 142 case REGISTERED: 143 return "http://hl7.org/fhir/diagnostic-report-status"; 144 case PARTIAL: 145 return "http://hl7.org/fhir/diagnostic-report-status"; 146 case FINAL: 147 return "http://hl7.org/fhir/diagnostic-report-status"; 148 case CORRECTED: 149 return "http://hl7.org/fhir/diagnostic-report-status"; 150 case APPENDED: 151 return "http://hl7.org/fhir/diagnostic-report-status"; 152 case CANCELLED: 153 return "http://hl7.org/fhir/diagnostic-report-status"; 154 case ENTEREDINERROR: 155 return "http://hl7.org/fhir/diagnostic-report-status"; 156 case NULL: 157 return null; 158 default: 159 return "?"; 160 } 161 } 162 163 public String getDefinition() { 164 switch (this) { 165 case REGISTERED: 166 return "The existence of the report is registered, but there is nothing yet available."; 167 case PARTIAL: 168 return "This is a partial (e.g. initial, interim or preliminary) report: data in the report may be incomplete or unverified."; 169 case FINAL: 170 return "The report is complete and verified by an authorized person."; 171 case CORRECTED: 172 return "The report has been modified subsequent to being Final, and is complete and verified by an authorized person. New content has been added, but existing content hasn't changed"; 173 case APPENDED: 174 return "The report has been modified subsequent to being Final, and is complete and verified by an authorized person. New content has been added, but existing content hasn't changed."; 175 case CANCELLED: 176 return "The report is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 177 case ENTEREDINERROR: 178 return "The report has been withdrawn following a previous final release."; 179 case NULL: 180 return null; 181 default: 182 return "?"; 183 } 184 } 185 186 public String getDisplay() { 187 switch (this) { 188 case REGISTERED: 189 return "Registered"; 190 case PARTIAL: 191 return "Partial"; 192 case FINAL: 193 return "Final"; 194 case CORRECTED: 195 return "Corrected"; 196 case APPENDED: 197 return "Appended"; 198 case CANCELLED: 199 return "Cancelled"; 200 case ENTEREDINERROR: 201 return "Entered in Error"; 202 case NULL: 203 return null; 204 default: 205 return "?"; 206 } 207 } 208 } 209 210 public static class DiagnosticReportStatusEnumFactory implements EnumFactory<DiagnosticReportStatus> { 211 public DiagnosticReportStatus fromCode(String codeString) throws IllegalArgumentException { 212 if (codeString == null || "".equals(codeString)) 213 if (codeString == null || "".equals(codeString)) 214 return null; 215 if ("registered".equals(codeString)) 216 return DiagnosticReportStatus.REGISTERED; 217 if ("partial".equals(codeString)) 218 return DiagnosticReportStatus.PARTIAL; 219 if ("final".equals(codeString)) 220 return DiagnosticReportStatus.FINAL; 221 if ("corrected".equals(codeString)) 222 return DiagnosticReportStatus.CORRECTED; 223 if ("appended".equals(codeString)) 224 return DiagnosticReportStatus.APPENDED; 225 if ("cancelled".equals(codeString)) 226 return DiagnosticReportStatus.CANCELLED; 227 if ("entered-in-error".equals(codeString)) 228 return DiagnosticReportStatus.ENTEREDINERROR; 229 throw new IllegalArgumentException("Unknown DiagnosticReportStatus code '" + codeString + "'"); 230 } 231 232 public Enumeration<DiagnosticReportStatus> fromType(Base code) throws FHIRException { 233 if (code == null || code.isEmpty()) 234 return null; 235 String codeString = ((PrimitiveType) code).asStringValue(); 236 if (codeString == null || "".equals(codeString)) 237 return null; 238 if ("registered".equals(codeString)) 239 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.REGISTERED); 240 if ("partial".equals(codeString)) 241 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.PARTIAL); 242 if ("final".equals(codeString)) 243 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.FINAL); 244 if ("corrected".equals(codeString)) 245 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.CORRECTED); 246 if ("appended".equals(codeString)) 247 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.APPENDED); 248 if ("cancelled".equals(codeString)) 249 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.CANCELLED); 250 if ("entered-in-error".equals(codeString)) 251 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.ENTEREDINERROR); 252 throw new FHIRException("Unknown DiagnosticReportStatus code '" + codeString + "'"); 253 } 254 255 public String toCode(DiagnosticReportStatus code) 256 { 257 if (code == DiagnosticReportStatus.NULL) 258 return null; 259 if (code == DiagnosticReportStatus.REGISTERED) 260 return "registered"; 261 if (code == DiagnosticReportStatus.PARTIAL) 262 return "partial"; 263 if (code == DiagnosticReportStatus.FINAL) 264 return "final"; 265 if (code == DiagnosticReportStatus.CORRECTED) 266 return "corrected"; 267 if (code == DiagnosticReportStatus.APPENDED) 268 return "appended"; 269 if (code == DiagnosticReportStatus.CANCELLED) 270 return "cancelled"; 271 if (code == DiagnosticReportStatus.ENTEREDINERROR) 272 return "entered-in-error"; 273 return "?"; 274 } 275 } 276 277 @Block() 278 public static class DiagnosticReportImageComponent extends BackboneElement implements IBaseBackboneElement { 279 /** 280 * A comment about the image. Typically, this is used to provide an explanation 281 * for why the image is included, or to draw the viewer's attention to important 282 * features. 283 */ 284 @Child(name = "comment", type = { 285 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 286 @Description(shortDefinition = "Comment about the image (e.g. explanation)", formalDefinition = "A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features.") 287 protected StringType comment; 288 289 /** 290 * Reference to the image source. 291 */ 292 @Child(name = "link", type = { Media.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 293 @Description(shortDefinition = "Reference to the image source", formalDefinition = "Reference to the image source.") 294 protected Reference link; 295 296 /** 297 * The actual object that is the target of the reference (Reference to the image 298 * source.) 299 */ 300 protected Media linkTarget; 301 302 private static final long serialVersionUID = 935791940L; 303 304 /* 305 * Constructor 306 */ 307 public DiagnosticReportImageComponent() { 308 super(); 309 } 310 311 /* 312 * Constructor 313 */ 314 public DiagnosticReportImageComponent(Reference link) { 315 super(); 316 this.link = link; 317 } 318 319 /** 320 * @return {@link #comment} (A comment about the image. Typically, this is used 321 * to provide an explanation for why the image is included, or to draw 322 * the viewer's attention to important features.). This is the 323 * underlying object with id, value and extensions. The accessor 324 * "getComment" gives direct access to the value 325 */ 326 public StringType getCommentElement() { 327 if (this.comment == null) 328 if (Configuration.errorOnAutoCreate()) 329 throw new Error("Attempt to auto-create DiagnosticReportImageComponent.comment"); 330 else if (Configuration.doAutoCreate()) 331 this.comment = new StringType(); // bb 332 return this.comment; 333 } 334 335 public boolean hasCommentElement() { 336 return this.comment != null && !this.comment.isEmpty(); 337 } 338 339 public boolean hasComment() { 340 return this.comment != null && !this.comment.isEmpty(); 341 } 342 343 /** 344 * @param value {@link #comment} (A comment about the image. Typically, this is 345 * used to provide an explanation for why the image is included, or 346 * to draw the viewer's attention to important features.). This is 347 * the underlying object with id, value and extensions. The 348 * accessor "getComment" gives direct access to the value 349 */ 350 public DiagnosticReportImageComponent setCommentElement(StringType value) { 351 this.comment = value; 352 return this; 353 } 354 355 /** 356 * @return A comment about the image. Typically, this is used to provide an 357 * explanation for why the image is included, or to draw the viewer's 358 * attention to important features. 359 */ 360 public String getComment() { 361 return this.comment == null ? null : this.comment.getValue(); 362 } 363 364 /** 365 * @param value A comment about the image. Typically, this is used to provide an 366 * explanation for why the image is included, or to draw the 367 * viewer's attention to important features. 368 */ 369 public DiagnosticReportImageComponent setComment(String value) { 370 if (Utilities.noString(value)) 371 this.comment = null; 372 else { 373 if (this.comment == null) 374 this.comment = new StringType(); 375 this.comment.setValue(value); 376 } 377 return this; 378 } 379 380 /** 381 * @return {@link #link} (Reference to the image source.) 382 */ 383 public Reference getLink() { 384 if (this.link == null) 385 if (Configuration.errorOnAutoCreate()) 386 throw new Error("Attempt to auto-create DiagnosticReportImageComponent.link"); 387 else if (Configuration.doAutoCreate()) 388 this.link = new Reference(); // cc 389 return this.link; 390 } 391 392 public boolean hasLink() { 393 return this.link != null && !this.link.isEmpty(); 394 } 395 396 /** 397 * @param value {@link #link} (Reference to the image source.) 398 */ 399 public DiagnosticReportImageComponent setLink(Reference value) { 400 this.link = value; 401 return this; 402 } 403 404 /** 405 * @return {@link #link} The actual object that is the target of the reference. 406 * The reference library doesn't populate this, but you can use it to 407 * hold the resource if you resolve it. (Reference to the image source.) 408 */ 409 public Media getLinkTarget() { 410 if (this.linkTarget == null) 411 if (Configuration.errorOnAutoCreate()) 412 throw new Error("Attempt to auto-create DiagnosticReportImageComponent.link"); 413 else if (Configuration.doAutoCreate()) 414 this.linkTarget = new Media(); // aa 415 return this.linkTarget; 416 } 417 418 /** 419 * @param value {@link #link} The actual object that is the target of the 420 * reference. The reference library doesn't use these, but you can 421 * use it to hold the resource if you resolve it. (Reference to the 422 * image source.) 423 */ 424 public DiagnosticReportImageComponent setLinkTarget(Media value) { 425 this.linkTarget = value; 426 return this; 427 } 428 429 protected void listChildren(List<Property> childrenList) { 430 super.listChildren(childrenList); 431 childrenList.add(new Property("comment", "string", 432 "A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features.", 433 0, java.lang.Integer.MAX_VALUE, comment)); 434 childrenList.add(new Property("link", "Reference(Media)", "Reference to the image source.", 0, 435 java.lang.Integer.MAX_VALUE, link)); 436 } 437 438 @Override 439 public void setProperty(String name, Base value) throws FHIRException { 440 if (name.equals("comment")) 441 this.comment = castToString(value); // StringType 442 else if (name.equals("link")) 443 this.link = castToReference(value); // Reference 444 else 445 super.setProperty(name, value); 446 } 447 448 @Override 449 public Base addChild(String name) throws FHIRException { 450 if (name.equals("comment")) { 451 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.comment"); 452 } else if (name.equals("link")) { 453 this.link = new Reference(); 454 return this.link; 455 } else 456 return super.addChild(name); 457 } 458 459 public DiagnosticReportImageComponent copy() { 460 DiagnosticReportImageComponent dst = new DiagnosticReportImageComponent(); 461 copyValues(dst); 462 dst.comment = comment == null ? null : comment.copy(); 463 dst.link = link == null ? null : link.copy(); 464 return dst; 465 } 466 467 @Override 468 public boolean equalsDeep(Base other) { 469 if (!super.equalsDeep(other)) 470 return false; 471 if (!(other instanceof DiagnosticReportImageComponent)) 472 return false; 473 DiagnosticReportImageComponent o = (DiagnosticReportImageComponent) other; 474 return compareDeep(comment, o.comment, true) && compareDeep(link, o.link, true); 475 } 476 477 @Override 478 public boolean equalsShallow(Base other) { 479 if (!super.equalsShallow(other)) 480 return false; 481 if (!(other instanceof DiagnosticReportImageComponent)) 482 return false; 483 DiagnosticReportImageComponent o = (DiagnosticReportImageComponent) other; 484 return compareValues(comment, o.comment, true); 485 } 486 487 public boolean isEmpty() { 488 return super.isEmpty() && (comment == null || comment.isEmpty()) && (link == null || link.isEmpty()); 489 } 490 491 public String fhirType() { 492 return "DiagnosticReport.image"; 493 494 } 495 496 } 497 498 /** 499 * The local ID assigned to the report by the order filler, usually by the 500 * Information System of the diagnostic service provider. 501 */ 502 @Child(name = "identifier", type = { 503 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 504 @Description(shortDefinition = "Id for external references to this report", formalDefinition = "The local ID assigned to the report by the order filler, usually by the Information System of the diagnostic service provider.") 505 protected List<Identifier> identifier; 506 507 /** 508 * The status of the diagnostic report as a whole. 509 */ 510 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 511 @Description(shortDefinition = "registered | partial | final | corrected | appended | cancelled | entered-in-error", formalDefinition = "The status of the diagnostic report as a whole.") 512 protected Enumeration<DiagnosticReportStatus> status; 513 514 /** 515 * A code that classifies the clinical discipline, department or diagnostic 516 * service that created the report (e.g. cardiology, biochemistry, hematology, 517 * MRI). This is used for searching, sorting and display purposes. 518 */ 519 @Child(name = "category", type = { 520 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 521 @Description(shortDefinition = "Service category", formalDefinition = "A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.") 522 protected CodeableConcept category; 523 524 /** 525 * A code or name that describes this diagnostic report. 526 */ 527 @Child(name = "code", type = { CodeableConcept.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 528 @Description(shortDefinition = "Name/Code for this diagnostic report", formalDefinition = "A code or name that describes this diagnostic report.") 529 protected CodeableConcept code; 530 531 /** 532 * The subject of the report. Usually, but not always, this is a patient. 533 * However diagnostic services also perform analyses on specimens collected from 534 * a variety of other sources. 535 */ 536 @Child(name = "subject", type = { Patient.class, Group.class, Device.class, 537 Location.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 538 @Description(shortDefinition = "The subject of the report, usually, but not always, the patient", formalDefinition = "The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources.") 539 protected Reference subject; 540 541 /** 542 * The actual object that is the target of the reference (The subject of the 543 * report. Usually, but not always, this is a patient. However diagnostic 544 * services also perform analyses on specimens collected from a variety of other 545 * sources.) 546 */ 547 protected Resource subjectTarget; 548 549 /** 550 * The link to the health care event (encounter) when the order was made. 551 */ 552 @Child(name = "encounter", type = { Encounter.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 553 @Description(shortDefinition = "Health care event when test ordered", formalDefinition = "The link to the health care event (encounter) when the order was made.") 554 protected Reference encounter; 555 556 /** 557 * The actual object that is the target of the reference (The link to the health 558 * care event (encounter) when the order was made.) 559 */ 560 protected Encounter encounterTarget; 561 562 /** 563 * The time or time-period the observed values are related to. When the subject 564 * of the report is a patient, this is usually either the time of the procedure 565 * or of specimen collection(s), but very often the source of the date/time is 566 * not known, only the date/time itself. 567 */ 568 @Child(name = "effective", type = { DateTimeType.class, 569 Period.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 570 @Description(shortDefinition = "Clinically Relevant time/time-period for report", formalDefinition = "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.") 571 protected Type effective; 572 573 /** 574 * The date and time that this version of the report was released from the 575 * source diagnostic service. 576 */ 577 @Child(name = "issued", type = { InstantType.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 578 @Description(shortDefinition = "DateTime this version was released", formalDefinition = "The date and time that this version of the report was released from the source diagnostic service.") 579 protected InstantType issued; 580 581 /** 582 * The diagnostic service that is responsible for issuing the report. 583 */ 584 @Child(name = "performer", type = { Practitioner.class, 585 Organization.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 586 @Description(shortDefinition = "Responsible Diagnostic Service", formalDefinition = "The diagnostic service that is responsible for issuing the report.") 587 protected Reference performer; 588 589 /** 590 * The actual object that is the target of the reference (The diagnostic service 591 * that is responsible for issuing the report.) 592 */ 593 protected Resource performerTarget; 594 595 /** 596 * Details concerning a test or procedure requested. 597 */ 598 @Child(name = "request", type = { DiagnosticOrder.class, ProcedureRequest.class, 599 ReferralRequest.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 600 @Description(shortDefinition = "What was requested", formalDefinition = "Details concerning a test or procedure requested.") 601 protected List<Reference> request; 602 /** 603 * The actual objects that are the target of the reference (Details concerning a 604 * test or procedure requested.) 605 */ 606 protected List<Resource> requestTarget; 607 608 /** 609 * Details about the specimens on which this diagnostic report is based. 610 */ 611 @Child(name = "specimen", type = { 612 Specimen.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 613 @Description(shortDefinition = "Specimens this report is based on", formalDefinition = "Details about the specimens on which this diagnostic report is based.") 614 protected List<Reference> specimen; 615 /** 616 * The actual objects that are the target of the reference (Details about the 617 * specimens on which this diagnostic report is based.) 618 */ 619 protected List<Specimen> specimenTarget; 620 621 /** 622 * Observations that are part of this diagnostic report. Observations can be 623 * simple name/value pairs (e.g. "atomic" results), or they can be grouping 624 * observations that include references to other members of the group (e.g. 625 * "panels"). 626 */ 627 @Child(name = "result", type = { 628 Observation.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 629 @Description(shortDefinition = "Observations - simple, or complex nested groups", formalDefinition = "Observations that are part of this diagnostic report. Observations can be simple name/value pairs (e.g. \"atomic\" results), or they can be grouping observations that include references to other members of the group (e.g. \"panels\").") 630 protected List<Reference> result; 631 /** 632 * The actual objects that are the target of the reference (Observations that 633 * are part of this diagnostic report. Observations can be simple name/value 634 * pairs (e.g. "atomic" results), or they can be grouping observations that 635 * include references to other members of the group (e.g. "panels").) 636 */ 637 protected List<Observation> resultTarget; 638 639 /** 640 * One or more links to full details of any imaging performed during the 641 * diagnostic investigation. Typically, this is imaging performed by DICOM 642 * enabled modalities, but this is not required. A fully enabled PACS viewer can 643 * use this information to provide views of the source images. 644 */ 645 @Child(name = "imagingStudy", type = { ImagingStudy.class, 646 ImagingObjectSelection.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 647 @Description(shortDefinition = "Reference to full details of imaging associated with the diagnostic report", formalDefinition = "One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images.") 648 protected List<Reference> imagingStudy; 649 /** 650 * The actual objects that are the target of the reference (One or more links to 651 * full details of any imaging performed during the diagnostic investigation. 652 * Typically, this is imaging performed by DICOM enabled modalities, but this is 653 * not required. A fully enabled PACS viewer can use this information to provide 654 * views of the source images.) 655 */ 656 protected List<Resource> imagingStudyTarget; 657 658 /** 659 * A list of key images associated with this report. The images are generally 660 * created during the diagnostic process, and may be directly of the patient, or 661 * of treated specimens (i.e. slides of interest). 662 */ 663 @Child(name = "image", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 664 @Description(shortDefinition = "Key images associated with this report", formalDefinition = "A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).") 665 protected List<DiagnosticReportImageComponent> image; 666 667 /** 668 * Concise and clinically contextualized narrative interpretation of the 669 * diagnostic report. 670 */ 671 @Child(name = "conclusion", type = { 672 StringType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 673 @Description(shortDefinition = "Clinical Interpretation of test results", formalDefinition = "Concise and clinically contextualized narrative interpretation of the diagnostic report.") 674 protected StringType conclusion; 675 676 /** 677 * Codes for the conclusion. 678 */ 679 @Child(name = "codedDiagnosis", type = { 680 CodeableConcept.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 681 @Description(shortDefinition = "Codes for the conclusion", formalDefinition = "Codes for the conclusion.") 682 protected List<CodeableConcept> codedDiagnosis; 683 684 /** 685 * Rich text representation of the entire result as issued by the diagnostic 686 * service. Multiple formats are allowed but they SHALL be semantically 687 * equivalent. 688 */ 689 @Child(name = "presentedForm", type = { 690 Attachment.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 691 @Description(shortDefinition = "Entire report as issued", formalDefinition = "Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.") 692 protected List<Attachment> presentedForm; 693 694 private static final long serialVersionUID = 920334551L; 695 696 /* 697 * Constructor 698 */ 699 public DiagnosticReport() { 700 super(); 701 } 702 703 /* 704 * Constructor 705 */ 706 public DiagnosticReport(Enumeration<DiagnosticReportStatus> status, CodeableConcept code, Reference subject, 707 Type effective, InstantType issued, Reference performer) { 708 super(); 709 this.status = status; 710 this.code = code; 711 this.subject = subject; 712 this.effective = effective; 713 this.issued = issued; 714 this.performer = performer; 715 } 716 717 /** 718 * @return {@link #identifier} (The local ID assigned to the report by the order 719 * filler, usually by the Information System of the diagnostic service 720 * provider.) 721 */ 722 public List<Identifier> getIdentifier() { 723 if (this.identifier == null) 724 this.identifier = new ArrayList<Identifier>(); 725 return this.identifier; 726 } 727 728 public boolean hasIdentifier() { 729 if (this.identifier == null) 730 return false; 731 for (Identifier item : this.identifier) 732 if (!item.isEmpty()) 733 return true; 734 return false; 735 } 736 737 /** 738 * @return {@link #identifier} (The local ID assigned to the report by the order 739 * filler, usually by the Information System of the diagnostic service 740 * provider.) 741 */ 742 // syntactic sugar 743 public Identifier addIdentifier() { // 3 744 Identifier t = new Identifier(); 745 if (this.identifier == null) 746 this.identifier = new ArrayList<Identifier>(); 747 this.identifier.add(t); 748 return t; 749 } 750 751 // syntactic sugar 752 public DiagnosticReport addIdentifier(Identifier t) { // 3 753 if (t == null) 754 return this; 755 if (this.identifier == null) 756 this.identifier = new ArrayList<Identifier>(); 757 this.identifier.add(t); 758 return this; 759 } 760 761 /** 762 * @return {@link #status} (The status of the diagnostic report as a whole.). 763 * This is the underlying object with id, value and extensions. The 764 * accessor "getStatus" gives direct access to the value 765 */ 766 public Enumeration<DiagnosticReportStatus> getStatusElement() { 767 if (this.status == null) 768 if (Configuration.errorOnAutoCreate()) 769 throw new Error("Attempt to auto-create DiagnosticReport.status"); 770 else if (Configuration.doAutoCreate()) 771 this.status = new Enumeration<DiagnosticReportStatus>(new DiagnosticReportStatusEnumFactory()); // bb 772 return this.status; 773 } 774 775 public boolean hasStatusElement() { 776 return this.status != null && !this.status.isEmpty(); 777 } 778 779 public boolean hasStatus() { 780 return this.status != null && !this.status.isEmpty(); 781 } 782 783 /** 784 * @param value {@link #status} (The status of the diagnostic report as a 785 * whole.). This is the underlying object with id, value and 786 * extensions. The accessor "getStatus" gives direct access to the 787 * value 788 */ 789 public DiagnosticReport setStatusElement(Enumeration<DiagnosticReportStatus> value) { 790 this.status = value; 791 return this; 792 } 793 794 /** 795 * @return The status of the diagnostic report as a whole. 796 */ 797 public DiagnosticReportStatus getStatus() { 798 return this.status == null ? null : this.status.getValue(); 799 } 800 801 /** 802 * @param value The status of the diagnostic report as a whole. 803 */ 804 public DiagnosticReport setStatus(DiagnosticReportStatus value) { 805 if (this.status == null) 806 this.status = new Enumeration<DiagnosticReportStatus>(new DiagnosticReportStatusEnumFactory()); 807 this.status.setValue(value); 808 return this; 809 } 810 811 /** 812 * @return {@link #category} (A code that classifies the clinical discipline, 813 * department or diagnostic service that created the report (e.g. 814 * cardiology, biochemistry, hematology, MRI). This is used for 815 * searching, sorting and display purposes.) 816 */ 817 public CodeableConcept getCategory() { 818 if (this.category == null) 819 if (Configuration.errorOnAutoCreate()) 820 throw new Error("Attempt to auto-create DiagnosticReport.category"); 821 else if (Configuration.doAutoCreate()) 822 this.category = new CodeableConcept(); // cc 823 return this.category; 824 } 825 826 public boolean hasCategory() { 827 return this.category != null && !this.category.isEmpty(); 828 } 829 830 /** 831 * @param value {@link #category} (A code that classifies the clinical 832 * discipline, department or diagnostic service that created the 833 * report (e.g. cardiology, biochemistry, hematology, MRI). This is 834 * used for searching, sorting and display purposes.) 835 */ 836 public DiagnosticReport setCategory(CodeableConcept value) { 837 this.category = value; 838 return this; 839 } 840 841 /** 842 * @return {@link #code} (A code or name that describes this diagnostic report.) 843 */ 844 public CodeableConcept getCode() { 845 if (this.code == null) 846 if (Configuration.errorOnAutoCreate()) 847 throw new Error("Attempt to auto-create DiagnosticReport.code"); 848 else if (Configuration.doAutoCreate()) 849 this.code = new CodeableConcept(); // cc 850 return this.code; 851 } 852 853 public boolean hasCode() { 854 return this.code != null && !this.code.isEmpty(); 855 } 856 857 /** 858 * @param value {@link #code} (A code or name that describes this diagnostic 859 * report.) 860 */ 861 public DiagnosticReport setCode(CodeableConcept value) { 862 this.code = value; 863 return this; 864 } 865 866 /** 867 * @return {@link #subject} (The subject of the report. Usually, but not always, 868 * this is a patient. However diagnostic services also perform analyses 869 * on specimens collected from a variety of other sources.) 870 */ 871 public Reference getSubject() { 872 if (this.subject == null) 873 if (Configuration.errorOnAutoCreate()) 874 throw new Error("Attempt to auto-create DiagnosticReport.subject"); 875 else if (Configuration.doAutoCreate()) 876 this.subject = new Reference(); // cc 877 return this.subject; 878 } 879 880 public boolean hasSubject() { 881 return this.subject != null && !this.subject.isEmpty(); 882 } 883 884 /** 885 * @param value {@link #subject} (The subject of the report. Usually, but not 886 * always, this is a patient. However diagnostic services also 887 * perform analyses on specimens collected from a variety of other 888 * sources.) 889 */ 890 public DiagnosticReport setSubject(Reference value) { 891 this.subject = value; 892 return this; 893 } 894 895 /** 896 * @return {@link #subject} The actual object that is the target of the 897 * reference. The reference library doesn't populate this, but you can 898 * use it to hold the resource if you resolve it. (The subject of the 899 * report. Usually, but not always, this is a patient. However 900 * diagnostic services also perform analyses on specimens collected from 901 * a variety of other sources.) 902 */ 903 public Resource getSubjectTarget() { 904 return this.subjectTarget; 905 } 906 907 /** 908 * @param value {@link #subject} The actual object that is the target of the 909 * reference. The reference library doesn't use these, but you can 910 * use it to hold the resource if you resolve it. (The subject of 911 * the report. Usually, but not always, this is a patient. However 912 * diagnostic services also perform analyses on specimens collected 913 * from a variety of other sources.) 914 */ 915 public DiagnosticReport setSubjectTarget(Resource value) { 916 this.subjectTarget = value; 917 return this; 918 } 919 920 /** 921 * @return {@link #encounter} (The link to the health care event (encounter) 922 * when the order was made.) 923 */ 924 public Reference getEncounter() { 925 if (this.encounter == null) 926 if (Configuration.errorOnAutoCreate()) 927 throw new Error("Attempt to auto-create DiagnosticReport.encounter"); 928 else if (Configuration.doAutoCreate()) 929 this.encounter = new Reference(); // cc 930 return this.encounter; 931 } 932 933 public boolean hasEncounter() { 934 return this.encounter != null && !this.encounter.isEmpty(); 935 } 936 937 /** 938 * @param value {@link #encounter} (The link to the health care event 939 * (encounter) when the order was made.) 940 */ 941 public DiagnosticReport setEncounter(Reference value) { 942 this.encounter = value; 943 return this; 944 } 945 946 /** 947 * @return {@link #encounter} The actual object that is the target of the 948 * reference. The reference library doesn't populate this, but you can 949 * use it to hold the resource if you resolve it. (The link to the 950 * health care event (encounter) when the order was made.) 951 */ 952 public Encounter getEncounterTarget() { 953 if (this.encounterTarget == null) 954 if (Configuration.errorOnAutoCreate()) 955 throw new Error("Attempt to auto-create DiagnosticReport.encounter"); 956 else if (Configuration.doAutoCreate()) 957 this.encounterTarget = new Encounter(); // aa 958 return this.encounterTarget; 959 } 960 961 /** 962 * @param value {@link #encounter} The actual object that is the target of the 963 * reference. The reference library doesn't use these, but you can 964 * use it to hold the resource if you resolve it. (The link to the 965 * health care event (encounter) when the order was made.) 966 */ 967 public DiagnosticReport setEncounterTarget(Encounter value) { 968 this.encounterTarget = value; 969 return this; 970 } 971 972 /** 973 * @return {@link #effective} (The time or time-period the observed values are 974 * related to. When the subject of the report is a patient, this is 975 * usually either the time of the procedure or of specimen 976 * collection(s), but very often the source of the date/time is not 977 * known, only the date/time itself.) 978 */ 979 public Type getEffective() { 980 return this.effective; 981 } 982 983 /** 984 * @return {@link #effective} (The time or time-period the observed values are 985 * related to. When the subject of the report is a patient, this is 986 * usually either the time of the procedure or of specimen 987 * collection(s), but very often the source of the date/time is not 988 * known, only the date/time itself.) 989 */ 990 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 991 if (!(this.effective instanceof DateTimeType)) 992 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 993 + this.effective.getClass().getName() + " was encountered"); 994 return (DateTimeType) this.effective; 995 } 996 997 public boolean hasEffectiveDateTimeType() { 998 return this.effective instanceof DateTimeType; 999 } 1000 1001 /** 1002 * @return {@link #effective} (The time or time-period the observed values are 1003 * related to. When the subject of the report is a patient, this is 1004 * usually either the time of the procedure or of specimen 1005 * collection(s), but very often the source of the date/time is not 1006 * known, only the date/time itself.) 1007 */ 1008 public Period getEffectivePeriod() throws FHIRException { 1009 if (!(this.effective instanceof Period)) 1010 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.effective.getClass().getName() 1011 + " was encountered"); 1012 return (Period) this.effective; 1013 } 1014 1015 public boolean hasEffectivePeriod() { 1016 return this.effective instanceof Period; 1017 } 1018 1019 public boolean hasEffective() { 1020 return this.effective != null && !this.effective.isEmpty(); 1021 } 1022 1023 /** 1024 * @param value {@link #effective} (The time or time-period the observed values 1025 * are related to. When the subject of the report is a patient, 1026 * this is usually either the time of the procedure or of specimen 1027 * collection(s), but very often the source of the date/time is not 1028 * known, only the date/time itself.) 1029 */ 1030 public DiagnosticReport setEffective(Type value) { 1031 this.effective = value; 1032 return this; 1033 } 1034 1035 /** 1036 * @return {@link #issued} (The date and time that this version of the report 1037 * was released from the source diagnostic service.). This is the 1038 * underlying object with id, value and extensions. The accessor 1039 * "getIssued" gives direct access to the value 1040 */ 1041 public InstantType getIssuedElement() { 1042 if (this.issued == null) 1043 if (Configuration.errorOnAutoCreate()) 1044 throw new Error("Attempt to auto-create DiagnosticReport.issued"); 1045 else if (Configuration.doAutoCreate()) 1046 this.issued = new InstantType(); // bb 1047 return this.issued; 1048 } 1049 1050 public boolean hasIssuedElement() { 1051 return this.issued != null && !this.issued.isEmpty(); 1052 } 1053 1054 public boolean hasIssued() { 1055 return this.issued != null && !this.issued.isEmpty(); 1056 } 1057 1058 /** 1059 * @param value {@link #issued} (The date and time that this version of the 1060 * report was released from the source diagnostic service.). This 1061 * is the underlying object with id, value and extensions. The 1062 * accessor "getIssued" gives direct access to the value 1063 */ 1064 public DiagnosticReport setIssuedElement(InstantType value) { 1065 this.issued = value; 1066 return this; 1067 } 1068 1069 /** 1070 * @return The date and time that this version of the report was released from 1071 * the source diagnostic service. 1072 */ 1073 public Date getIssued() { 1074 return this.issued == null ? null : this.issued.getValue(); 1075 } 1076 1077 /** 1078 * @param value The date and time that this version of the report was released 1079 * from the source diagnostic service. 1080 */ 1081 public DiagnosticReport setIssued(Date value) { 1082 if (this.issued == null) 1083 this.issued = new InstantType(); 1084 this.issued.setValue(value); 1085 return this; 1086 } 1087 1088 /** 1089 * @return {@link #performer} (The diagnostic service that is responsible for 1090 * issuing the report.) 1091 */ 1092 public Reference getPerformer() { 1093 if (this.performer == null) 1094 if (Configuration.errorOnAutoCreate()) 1095 throw new Error("Attempt to auto-create DiagnosticReport.performer"); 1096 else if (Configuration.doAutoCreate()) 1097 this.performer = new Reference(); // cc 1098 return this.performer; 1099 } 1100 1101 public boolean hasPerformer() { 1102 return this.performer != null && !this.performer.isEmpty(); 1103 } 1104 1105 /** 1106 * @param value {@link #performer} (The diagnostic service that is responsible 1107 * for issuing the report.) 1108 */ 1109 public DiagnosticReport setPerformer(Reference value) { 1110 this.performer = value; 1111 return this; 1112 } 1113 1114 /** 1115 * @return {@link #performer} The actual object that is the target of the 1116 * reference. The reference library doesn't populate this, but you can 1117 * use it to hold the resource if you resolve it. (The diagnostic 1118 * service that is responsible for issuing the report.) 1119 */ 1120 public Resource getPerformerTarget() { 1121 return this.performerTarget; 1122 } 1123 1124 /** 1125 * @param value {@link #performer} The actual object that is the target of the 1126 * reference. The reference library doesn't use these, but you can 1127 * use it to hold the resource if you resolve it. (The diagnostic 1128 * service that is responsible for issuing the report.) 1129 */ 1130 public DiagnosticReport setPerformerTarget(Resource value) { 1131 this.performerTarget = value; 1132 return this; 1133 } 1134 1135 /** 1136 * @return {@link #request} (Details concerning a test or procedure requested.) 1137 */ 1138 public List<Reference> getRequest() { 1139 if (this.request == null) 1140 this.request = new ArrayList<Reference>(); 1141 return this.request; 1142 } 1143 1144 public boolean hasRequest() { 1145 if (this.request == null) 1146 return false; 1147 for (Reference item : this.request) 1148 if (!item.isEmpty()) 1149 return true; 1150 return false; 1151 } 1152 1153 /** 1154 * @return {@link #request} (Details concerning a test or procedure requested.) 1155 */ 1156 // syntactic sugar 1157 public Reference addRequest() { // 3 1158 Reference t = new Reference(); 1159 if (this.request == null) 1160 this.request = new ArrayList<Reference>(); 1161 this.request.add(t); 1162 return t; 1163 } 1164 1165 // syntactic sugar 1166 public DiagnosticReport addRequest(Reference t) { // 3 1167 if (t == null) 1168 return this; 1169 if (this.request == null) 1170 this.request = new ArrayList<Reference>(); 1171 this.request.add(t); 1172 return this; 1173 } 1174 1175 /** 1176 * @return {@link #request} (The actual objects that are the target of the 1177 * reference. The reference library doesn't populate this, but you can 1178 * use this to hold the resources if you resolvethemt. Details 1179 * concerning a test or procedure requested.) 1180 */ 1181 public List<Resource> getRequestTarget() { 1182 if (this.requestTarget == null) 1183 this.requestTarget = new ArrayList<Resource>(); 1184 return this.requestTarget; 1185 } 1186 1187 /** 1188 * @return {@link #specimen} (Details about the specimens on which this 1189 * diagnostic report is based.) 1190 */ 1191 public List<Reference> getSpecimen() { 1192 if (this.specimen == null) 1193 this.specimen = new ArrayList<Reference>(); 1194 return this.specimen; 1195 } 1196 1197 public boolean hasSpecimen() { 1198 if (this.specimen == null) 1199 return false; 1200 for (Reference item : this.specimen) 1201 if (!item.isEmpty()) 1202 return true; 1203 return false; 1204 } 1205 1206 /** 1207 * @return {@link #specimen} (Details about the specimens on which this 1208 * diagnostic report is based.) 1209 */ 1210 // syntactic sugar 1211 public Reference addSpecimen() { // 3 1212 Reference t = new Reference(); 1213 if (this.specimen == null) 1214 this.specimen = new ArrayList<Reference>(); 1215 this.specimen.add(t); 1216 return t; 1217 } 1218 1219 // syntactic sugar 1220 public DiagnosticReport addSpecimen(Reference t) { // 3 1221 if (t == null) 1222 return this; 1223 if (this.specimen == null) 1224 this.specimen = new ArrayList<Reference>(); 1225 this.specimen.add(t); 1226 return this; 1227 } 1228 1229 /** 1230 * @return {@link #specimen} (The actual objects that are the target of the 1231 * reference. The reference library doesn't populate this, but you can 1232 * use this to hold the resources if you resolvethemt. Details about the 1233 * specimens on which this diagnostic report is based.) 1234 */ 1235 public List<Specimen> getSpecimenTarget() { 1236 if (this.specimenTarget == null) 1237 this.specimenTarget = new ArrayList<Specimen>(); 1238 return this.specimenTarget; 1239 } 1240 1241 // syntactic sugar 1242 /** 1243 * @return {@link #specimen} (Add an actual object that is the target of the 1244 * reference. The reference library doesn't use these, but you can use 1245 * this to hold the resources if you resolvethemt. Details about the 1246 * specimens on which this diagnostic report is based.) 1247 */ 1248 public Specimen addSpecimenTarget() { 1249 Specimen r = new Specimen(); 1250 if (this.specimenTarget == null) 1251 this.specimenTarget = new ArrayList<Specimen>(); 1252 this.specimenTarget.add(r); 1253 return r; 1254 } 1255 1256 /** 1257 * @return {@link #result} (Observations that are part of this diagnostic 1258 * report. Observations can be simple name/value pairs (e.g. "atomic" 1259 * results), or they can be grouping observations that include 1260 * references to other members of the group (e.g. "panels").) 1261 */ 1262 public List<Reference> getResult() { 1263 if (this.result == null) 1264 this.result = new ArrayList<Reference>(); 1265 return this.result; 1266 } 1267 1268 public boolean hasResult() { 1269 if (this.result == null) 1270 return false; 1271 for (Reference item : this.result) 1272 if (!item.isEmpty()) 1273 return true; 1274 return false; 1275 } 1276 1277 /** 1278 * @return {@link #result} (Observations that are part of this diagnostic 1279 * report. Observations can be simple name/value pairs (e.g. "atomic" 1280 * results), or they can be grouping observations that include 1281 * references to other members of the group (e.g. "panels").) 1282 */ 1283 // syntactic sugar 1284 public Reference addResult() { // 3 1285 Reference t = new Reference(); 1286 if (this.result == null) 1287 this.result = new ArrayList<Reference>(); 1288 this.result.add(t); 1289 return t; 1290 } 1291 1292 // syntactic sugar 1293 public DiagnosticReport addResult(Reference t) { // 3 1294 if (t == null) 1295 return this; 1296 if (this.result == null) 1297 this.result = new ArrayList<Reference>(); 1298 this.result.add(t); 1299 return this; 1300 } 1301 1302 /** 1303 * @return {@link #result} (The actual objects that are the target of the 1304 * reference. The reference library doesn't populate this, but you can 1305 * use this to hold the resources if you resolvethemt. Observations that 1306 * are part of this diagnostic report. Observations can be simple 1307 * name/value pairs (e.g. "atomic" results), or they can be grouping 1308 * observations that include references to other members of the group 1309 * (e.g. "panels").) 1310 */ 1311 public List<Observation> getResultTarget() { 1312 if (this.resultTarget == null) 1313 this.resultTarget = new ArrayList<Observation>(); 1314 return this.resultTarget; 1315 } 1316 1317 // syntactic sugar 1318 /** 1319 * @return {@link #result} (Add an actual object that is the target of the 1320 * reference. The reference library doesn't use these, but you can use 1321 * this to hold the resources if you resolvethemt. Observations that are 1322 * part of this diagnostic report. Observations can be simple name/value 1323 * pairs (e.g. "atomic" results), or they can be grouping observations 1324 * that include references to other members of the group (e.g. 1325 * "panels").) 1326 */ 1327 public Observation addResultTarget() { 1328 Observation r = new Observation(); 1329 if (this.resultTarget == null) 1330 this.resultTarget = new ArrayList<Observation>(); 1331 this.resultTarget.add(r); 1332 return r; 1333 } 1334 1335 /** 1336 * @return {@link #imagingStudy} (One or more links to full details of any 1337 * imaging performed during the diagnostic investigation. Typically, 1338 * this is imaging performed by DICOM enabled modalities, but this is 1339 * not required. A fully enabled PACS viewer can use this information to 1340 * provide views of the source images.) 1341 */ 1342 public List<Reference> getImagingStudy() { 1343 if (this.imagingStudy == null) 1344 this.imagingStudy = new ArrayList<Reference>(); 1345 return this.imagingStudy; 1346 } 1347 1348 public boolean hasImagingStudy() { 1349 if (this.imagingStudy == null) 1350 return false; 1351 for (Reference item : this.imagingStudy) 1352 if (!item.isEmpty()) 1353 return true; 1354 return false; 1355 } 1356 1357 /** 1358 * @return {@link #imagingStudy} (One or more links to full details of any 1359 * imaging performed during the diagnostic investigation. Typically, 1360 * this is imaging performed by DICOM enabled modalities, but this is 1361 * not required. A fully enabled PACS viewer can use this information to 1362 * provide views of the source images.) 1363 */ 1364 // syntactic sugar 1365 public Reference addImagingStudy() { // 3 1366 Reference t = new Reference(); 1367 if (this.imagingStudy == null) 1368 this.imagingStudy = new ArrayList<Reference>(); 1369 this.imagingStudy.add(t); 1370 return t; 1371 } 1372 1373 // syntactic sugar 1374 public DiagnosticReport addImagingStudy(Reference t) { // 3 1375 if (t == null) 1376 return this; 1377 if (this.imagingStudy == null) 1378 this.imagingStudy = new ArrayList<Reference>(); 1379 this.imagingStudy.add(t); 1380 return this; 1381 } 1382 1383 /** 1384 * @return {@link #imagingStudy} (The actual objects that are the target of the 1385 * reference. The reference library doesn't populate this, but you can 1386 * use this to hold the resources if you resolvethemt. One or more links 1387 * to full details of any imaging performed during the diagnostic 1388 * investigation. Typically, this is imaging performed by DICOM enabled 1389 * modalities, but this is not required. A fully enabled PACS viewer can 1390 * use this information to provide views of the source images.) 1391 */ 1392 public List<Resource> getImagingStudyTarget() { 1393 if (this.imagingStudyTarget == null) 1394 this.imagingStudyTarget = new ArrayList<Resource>(); 1395 return this.imagingStudyTarget; 1396 } 1397 1398 /** 1399 * @return {@link #image} (A list of key images associated with this report. The 1400 * images are generally created during the diagnostic process, and may 1401 * be directly of the patient, or of treated specimens (i.e. slides of 1402 * interest).) 1403 */ 1404 public List<DiagnosticReportImageComponent> getImage() { 1405 if (this.image == null) 1406 this.image = new ArrayList<DiagnosticReportImageComponent>(); 1407 return this.image; 1408 } 1409 1410 public boolean hasImage() { 1411 if (this.image == null) 1412 return false; 1413 for (DiagnosticReportImageComponent item : this.image) 1414 if (!item.isEmpty()) 1415 return true; 1416 return false; 1417 } 1418 1419 /** 1420 * @return {@link #image} (A list of key images associated with this report. The 1421 * images are generally created during the diagnostic process, and may 1422 * be directly of the patient, or of treated specimens (i.e. slides of 1423 * interest).) 1424 */ 1425 // syntactic sugar 1426 public DiagnosticReportImageComponent addImage() { // 3 1427 DiagnosticReportImageComponent t = new DiagnosticReportImageComponent(); 1428 if (this.image == null) 1429 this.image = new ArrayList<DiagnosticReportImageComponent>(); 1430 this.image.add(t); 1431 return t; 1432 } 1433 1434 // syntactic sugar 1435 public DiagnosticReport addImage(DiagnosticReportImageComponent t) { // 3 1436 if (t == null) 1437 return this; 1438 if (this.image == null) 1439 this.image = new ArrayList<DiagnosticReportImageComponent>(); 1440 this.image.add(t); 1441 return this; 1442 } 1443 1444 /** 1445 * @return {@link #conclusion} (Concise and clinically contextualized narrative 1446 * interpretation of the diagnostic report.). This is the underlying 1447 * object with id, value and extensions. The accessor "getConclusion" 1448 * gives direct access to the value 1449 */ 1450 public StringType getConclusionElement() { 1451 if (this.conclusion == null) 1452 if (Configuration.errorOnAutoCreate()) 1453 throw new Error("Attempt to auto-create DiagnosticReport.conclusion"); 1454 else if (Configuration.doAutoCreate()) 1455 this.conclusion = new StringType(); // bb 1456 return this.conclusion; 1457 } 1458 1459 public boolean hasConclusionElement() { 1460 return this.conclusion != null && !this.conclusion.isEmpty(); 1461 } 1462 1463 public boolean hasConclusion() { 1464 return this.conclusion != null && !this.conclusion.isEmpty(); 1465 } 1466 1467 /** 1468 * @param value {@link #conclusion} (Concise and clinically contextualized 1469 * narrative interpretation of the diagnostic report.). This is the 1470 * underlying object with id, value and extensions. The accessor 1471 * "getConclusion" gives direct access to the value 1472 */ 1473 public DiagnosticReport setConclusionElement(StringType value) { 1474 this.conclusion = value; 1475 return this; 1476 } 1477 1478 /** 1479 * @return Concise and clinically contextualized narrative interpretation of the 1480 * diagnostic report. 1481 */ 1482 public String getConclusion() { 1483 return this.conclusion == null ? null : this.conclusion.getValue(); 1484 } 1485 1486 /** 1487 * @param value Concise and clinically contextualized narrative interpretation 1488 * of the diagnostic report. 1489 */ 1490 public DiagnosticReport setConclusion(String value) { 1491 if (Utilities.noString(value)) 1492 this.conclusion = null; 1493 else { 1494 if (this.conclusion == null) 1495 this.conclusion = new StringType(); 1496 this.conclusion.setValue(value); 1497 } 1498 return this; 1499 } 1500 1501 /** 1502 * @return {@link #codedDiagnosis} (Codes for the conclusion.) 1503 */ 1504 public List<CodeableConcept> getCodedDiagnosis() { 1505 if (this.codedDiagnosis == null) 1506 this.codedDiagnosis = new ArrayList<CodeableConcept>(); 1507 return this.codedDiagnosis; 1508 } 1509 1510 public boolean hasCodedDiagnosis() { 1511 if (this.codedDiagnosis == null) 1512 return false; 1513 for (CodeableConcept item : this.codedDiagnosis) 1514 if (!item.isEmpty()) 1515 return true; 1516 return false; 1517 } 1518 1519 /** 1520 * @return {@link #codedDiagnosis} (Codes for the conclusion.) 1521 */ 1522 // syntactic sugar 1523 public CodeableConcept addCodedDiagnosis() { // 3 1524 CodeableConcept t = new CodeableConcept(); 1525 if (this.codedDiagnosis == null) 1526 this.codedDiagnosis = new ArrayList<CodeableConcept>(); 1527 this.codedDiagnosis.add(t); 1528 return t; 1529 } 1530 1531 // syntactic sugar 1532 public DiagnosticReport addCodedDiagnosis(CodeableConcept t) { // 3 1533 if (t == null) 1534 return this; 1535 if (this.codedDiagnosis == null) 1536 this.codedDiagnosis = new ArrayList<CodeableConcept>(); 1537 this.codedDiagnosis.add(t); 1538 return this; 1539 } 1540 1541 /** 1542 * @return {@link #presentedForm} (Rich text representation of the entire result 1543 * as issued by the diagnostic service. Multiple formats are allowed but 1544 * they SHALL be semantically equivalent.) 1545 */ 1546 public List<Attachment> getPresentedForm() { 1547 if (this.presentedForm == null) 1548 this.presentedForm = new ArrayList<Attachment>(); 1549 return this.presentedForm; 1550 } 1551 1552 public boolean hasPresentedForm() { 1553 if (this.presentedForm == null) 1554 return false; 1555 for (Attachment item : this.presentedForm) 1556 if (!item.isEmpty()) 1557 return true; 1558 return false; 1559 } 1560 1561 /** 1562 * @return {@link #presentedForm} (Rich text representation of the entire result 1563 * as issued by the diagnostic service. Multiple formats are allowed but 1564 * they SHALL be semantically equivalent.) 1565 */ 1566 // syntactic sugar 1567 public Attachment addPresentedForm() { // 3 1568 Attachment t = new Attachment(); 1569 if (this.presentedForm == null) 1570 this.presentedForm = new ArrayList<Attachment>(); 1571 this.presentedForm.add(t); 1572 return t; 1573 } 1574 1575 // syntactic sugar 1576 public DiagnosticReport addPresentedForm(Attachment t) { // 3 1577 if (t == null) 1578 return this; 1579 if (this.presentedForm == null) 1580 this.presentedForm = new ArrayList<Attachment>(); 1581 this.presentedForm.add(t); 1582 return this; 1583 } 1584 1585 protected void listChildren(List<Property> childrenList) { 1586 super.listChildren(childrenList); 1587 childrenList.add(new Property("identifier", "Identifier", 1588 "The local ID assigned to the report by the order filler, usually by the Information System of the diagnostic service provider.", 1589 0, java.lang.Integer.MAX_VALUE, identifier)); 1590 childrenList.add(new Property("status", "code", "The status of the diagnostic report as a whole.", 0, 1591 java.lang.Integer.MAX_VALUE, status)); 1592 childrenList.add(new Property("category", "CodeableConcept", 1593 "A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.", 1594 0, java.lang.Integer.MAX_VALUE, category)); 1595 childrenList.add(new Property("code", "CodeableConcept", "A code or name that describes this diagnostic report.", 0, 1596 java.lang.Integer.MAX_VALUE, code)); 1597 childrenList.add(new Property("subject", "Reference(Patient|Group|Device|Location)", 1598 "The subject of the report. Usually, but not always, this is a patient. However diagnostic services also perform analyses on specimens collected from a variety of other sources.", 1599 0, java.lang.Integer.MAX_VALUE, subject)); 1600 childrenList.add(new Property("encounter", "Reference(Encounter)", 1601 "The link to the health care event (encounter) when the order was made.", 0, java.lang.Integer.MAX_VALUE, 1602 encounter)); 1603 childrenList.add(new Property("effective[x]", "dateTime|Period", 1604 "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 1605 0, java.lang.Integer.MAX_VALUE, effective)); 1606 childrenList.add(new Property("issued", "instant", 1607 "The date and time that this version of the report was released from the source diagnostic service.", 0, 1608 java.lang.Integer.MAX_VALUE, issued)); 1609 childrenList.add(new Property("performer", "Reference(Practitioner|Organization)", 1610 "The diagnostic service that is responsible for issuing the report.", 0, java.lang.Integer.MAX_VALUE, 1611 performer)); 1612 childrenList.add(new Property("request", "Reference(DiagnosticOrder|ProcedureRequest|ReferralRequest)", 1613 "Details concerning a test or procedure requested.", 0, java.lang.Integer.MAX_VALUE, request)); 1614 childrenList.add(new Property("specimen", "Reference(Specimen)", 1615 "Details about the specimens on which this diagnostic report is based.", 0, java.lang.Integer.MAX_VALUE, 1616 specimen)); 1617 childrenList.add(new Property("result", "Reference(Observation)", 1618 "Observations that are part of this diagnostic report. Observations can be simple name/value pairs (e.g. \"atomic\" results), or they can be grouping observations that include references to other members of the group (e.g. \"panels\").", 1619 0, java.lang.Integer.MAX_VALUE, result)); 1620 childrenList.add(new Property("imagingStudy", "Reference(ImagingStudy|ImagingObjectSelection)", 1621 "One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images.", 1622 0, java.lang.Integer.MAX_VALUE, imagingStudy)); 1623 childrenList.add(new Property("image", "", 1624 "A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).", 1625 0, java.lang.Integer.MAX_VALUE, image)); 1626 childrenList.add(new Property("conclusion", "string", 1627 "Concise and clinically contextualized narrative interpretation of the diagnostic report.", 0, 1628 java.lang.Integer.MAX_VALUE, conclusion)); 1629 childrenList.add(new Property("codedDiagnosis", "CodeableConcept", "Codes for the conclusion.", 0, 1630 java.lang.Integer.MAX_VALUE, codedDiagnosis)); 1631 childrenList.add(new Property("presentedForm", "Attachment", 1632 "Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.", 1633 0, java.lang.Integer.MAX_VALUE, presentedForm)); 1634 } 1635 1636 @Override 1637 public void setProperty(String name, Base value) throws FHIRException { 1638 if (name.equals("identifier")) 1639 this.getIdentifier().add(castToIdentifier(value)); 1640 else if (name.equals("status")) 1641 this.status = new DiagnosticReportStatusEnumFactory().fromType(value); // Enumeration<DiagnosticReportStatus> 1642 else if (name.equals("category")) 1643 this.category = castToCodeableConcept(value); // CodeableConcept 1644 else if (name.equals("code")) 1645 this.code = castToCodeableConcept(value); // CodeableConcept 1646 else if (name.equals("subject")) 1647 this.subject = castToReference(value); // Reference 1648 else if (name.equals("encounter")) 1649 this.encounter = castToReference(value); // Reference 1650 else if (name.equals("effective[x]")) 1651 this.effective = (Type) value; // Type 1652 else if (name.equals("issued")) 1653 this.issued = castToInstant(value); // InstantType 1654 else if (name.equals("performer")) 1655 this.performer = castToReference(value); // Reference 1656 else if (name.equals("request")) 1657 this.getRequest().add(castToReference(value)); 1658 else if (name.equals("specimen")) 1659 this.getSpecimen().add(castToReference(value)); 1660 else if (name.equals("result")) 1661 this.getResult().add(castToReference(value)); 1662 else if (name.equals("imagingStudy")) 1663 this.getImagingStudy().add(castToReference(value)); 1664 else if (name.equals("image")) 1665 this.getImage().add((DiagnosticReportImageComponent) value); 1666 else if (name.equals("conclusion")) 1667 this.conclusion = castToString(value); // StringType 1668 else if (name.equals("codedDiagnosis")) 1669 this.getCodedDiagnosis().add(castToCodeableConcept(value)); 1670 else if (name.equals("presentedForm")) 1671 this.getPresentedForm().add(castToAttachment(value)); 1672 else 1673 super.setProperty(name, value); 1674 } 1675 1676 @Override 1677 public Base addChild(String name) throws FHIRException { 1678 if (name.equals("identifier")) { 1679 return addIdentifier(); 1680 } else if (name.equals("status")) { 1681 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.status"); 1682 } else if (name.equals("category")) { 1683 this.category = new CodeableConcept(); 1684 return this.category; 1685 } else if (name.equals("code")) { 1686 this.code = new CodeableConcept(); 1687 return this.code; 1688 } else if (name.equals("subject")) { 1689 this.subject = new Reference(); 1690 return this.subject; 1691 } else if (name.equals("encounter")) { 1692 this.encounter = new Reference(); 1693 return this.encounter; 1694 } else if (name.equals("effectiveDateTime")) { 1695 this.effective = new DateTimeType(); 1696 return this.effective; 1697 } else if (name.equals("effectivePeriod")) { 1698 this.effective = new Period(); 1699 return this.effective; 1700 } else if (name.equals("issued")) { 1701 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.issued"); 1702 } else if (name.equals("performer")) { 1703 this.performer = new Reference(); 1704 return this.performer; 1705 } else if (name.equals("request")) { 1706 return addRequest(); 1707 } else if (name.equals("specimen")) { 1708 return addSpecimen(); 1709 } else if (name.equals("result")) { 1710 return addResult(); 1711 } else if (name.equals("imagingStudy")) { 1712 return addImagingStudy(); 1713 } else if (name.equals("image")) { 1714 return addImage(); 1715 } else if (name.equals("conclusion")) { 1716 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.conclusion"); 1717 } else if (name.equals("codedDiagnosis")) { 1718 return addCodedDiagnosis(); 1719 } else if (name.equals("presentedForm")) { 1720 return addPresentedForm(); 1721 } else 1722 return super.addChild(name); 1723 } 1724 1725 public String fhirType() { 1726 return "DiagnosticReport"; 1727 1728 } 1729 1730 public DiagnosticReport copy() { 1731 DiagnosticReport dst = new DiagnosticReport(); 1732 copyValues(dst); 1733 if (identifier != null) { 1734 dst.identifier = new ArrayList<Identifier>(); 1735 for (Identifier i : identifier) 1736 dst.identifier.add(i.copy()); 1737 } 1738 ; 1739 dst.status = status == null ? null : status.copy(); 1740 dst.category = category == null ? null : category.copy(); 1741 dst.code = code == null ? null : code.copy(); 1742 dst.subject = subject == null ? null : subject.copy(); 1743 dst.encounter = encounter == null ? null : encounter.copy(); 1744 dst.effective = effective == null ? null : effective.copy(); 1745 dst.issued = issued == null ? null : issued.copy(); 1746 dst.performer = performer == null ? null : performer.copy(); 1747 if (request != null) { 1748 dst.request = new ArrayList<Reference>(); 1749 for (Reference i : request) 1750 dst.request.add(i.copy()); 1751 } 1752 ; 1753 if (specimen != null) { 1754 dst.specimen = new ArrayList<Reference>(); 1755 for (Reference i : specimen) 1756 dst.specimen.add(i.copy()); 1757 } 1758 ; 1759 if (result != null) { 1760 dst.result = new ArrayList<Reference>(); 1761 for (Reference i : result) 1762 dst.result.add(i.copy()); 1763 } 1764 ; 1765 if (imagingStudy != null) { 1766 dst.imagingStudy = new ArrayList<Reference>(); 1767 for (Reference i : imagingStudy) 1768 dst.imagingStudy.add(i.copy()); 1769 } 1770 ; 1771 if (image != null) { 1772 dst.image = new ArrayList<DiagnosticReportImageComponent>(); 1773 for (DiagnosticReportImageComponent i : image) 1774 dst.image.add(i.copy()); 1775 } 1776 ; 1777 dst.conclusion = conclusion == null ? null : conclusion.copy(); 1778 if (codedDiagnosis != null) { 1779 dst.codedDiagnosis = new ArrayList<CodeableConcept>(); 1780 for (CodeableConcept i : codedDiagnosis) 1781 dst.codedDiagnosis.add(i.copy()); 1782 } 1783 ; 1784 if (presentedForm != null) { 1785 dst.presentedForm = new ArrayList<Attachment>(); 1786 for (Attachment i : presentedForm) 1787 dst.presentedForm.add(i.copy()); 1788 } 1789 ; 1790 return dst; 1791 } 1792 1793 protected DiagnosticReport typedCopy() { 1794 return copy(); 1795 } 1796 1797 @Override 1798 public boolean equalsDeep(Base other) { 1799 if (!super.equalsDeep(other)) 1800 return false; 1801 if (!(other instanceof DiagnosticReport)) 1802 return false; 1803 DiagnosticReport o = (DiagnosticReport) other; 1804 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1805 && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 1806 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 1807 && compareDeep(effective, o.effective, true) && compareDeep(issued, o.issued, true) 1808 && compareDeep(performer, o.performer, true) && compareDeep(request, o.request, true) 1809 && compareDeep(specimen, o.specimen, true) && compareDeep(result, o.result, true) 1810 && compareDeep(imagingStudy, o.imagingStudy, true) && compareDeep(image, o.image, true) 1811 && compareDeep(conclusion, o.conclusion, true) && compareDeep(codedDiagnosis, o.codedDiagnosis, true) 1812 && compareDeep(presentedForm, o.presentedForm, true); 1813 } 1814 1815 @Override 1816 public boolean equalsShallow(Base other) { 1817 if (!super.equalsShallow(other)) 1818 return false; 1819 if (!(other instanceof DiagnosticReport)) 1820 return false; 1821 DiagnosticReport o = (DiagnosticReport) other; 1822 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true) 1823 && compareValues(conclusion, o.conclusion, true); 1824 } 1825 1826 public boolean isEmpty() { 1827 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (status == null || status.isEmpty()) 1828 && (category == null || category.isEmpty()) && (code == null || code.isEmpty()) 1829 && (subject == null || subject.isEmpty()) && (encounter == null || encounter.isEmpty()) 1830 && (effective == null || effective.isEmpty()) && (issued == null || issued.isEmpty()) 1831 && (performer == null || performer.isEmpty()) && (request == null || request.isEmpty()) 1832 && (specimen == null || specimen.isEmpty()) && (result == null || result.isEmpty()) 1833 && (imagingStudy == null || imagingStudy.isEmpty()) && (image == null || image.isEmpty()) 1834 && (conclusion == null || conclusion.isEmpty()) && (codedDiagnosis == null || codedDiagnosis.isEmpty()) 1835 && (presentedForm == null || presentedForm.isEmpty()); 1836 } 1837 1838 @Override 1839 public ResourceType getResourceType() { 1840 return ResourceType.DiagnosticReport; 1841 } 1842 1843 @SearchParamDefinition(name = "date", path = "DiagnosticReport.effective[x]", description = "The clinically relevant time of the report", type = "date") 1844 public static final String SP_DATE = "date"; 1845 @SearchParamDefinition(name = "identifier", path = "DiagnosticReport.identifier", description = "An identifier for the report", type = "token") 1846 public static final String SP_IDENTIFIER = "identifier"; 1847 @SearchParamDefinition(name = "image", path = "DiagnosticReport.image.link", description = "A reference to the image source.", type = "reference") 1848 public static final String SP_IMAGE = "image"; 1849 @SearchParamDefinition(name = "request", path = "DiagnosticReport.request", description = "Reference to the test or procedure request.", type = "reference") 1850 public static final String SP_REQUEST = "request"; 1851 @SearchParamDefinition(name = "performer", path = "DiagnosticReport.performer", description = "Who was the source of the report (organization)", type = "reference") 1852 public static final String SP_PERFORMER = "performer"; 1853 @SearchParamDefinition(name = "code", path = "DiagnosticReport.code", description = "The code for the report as a whole, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result", type = "token") 1854 public static final String SP_CODE = "code"; 1855 @SearchParamDefinition(name = "subject", path = "DiagnosticReport.subject", description = "The subject of the report", type = "reference") 1856 public static final String SP_SUBJECT = "subject"; 1857 @SearchParamDefinition(name = "diagnosis", path = "DiagnosticReport.codedDiagnosis", description = "A coded diagnosis on the report", type = "token") 1858 public static final String SP_DIAGNOSIS = "diagnosis"; 1859 @SearchParamDefinition(name = "encounter", path = "DiagnosticReport.encounter", description = "The Encounter when the order was made", type = "reference") 1860 public static final String SP_ENCOUNTER = "encounter"; 1861 @SearchParamDefinition(name = "result", path = "DiagnosticReport.result", description = "Link to an atomic result (observation resource)", type = "reference") 1862 public static final String SP_RESULT = "result"; 1863 @SearchParamDefinition(name = "patient", path = "DiagnosticReport.subject", description = "The subject of the report if a patient", type = "reference") 1864 public static final String SP_PATIENT = "patient"; 1865 @SearchParamDefinition(name = "specimen", path = "DiagnosticReport.specimen", description = "The specimen details", type = "reference") 1866 public static final String SP_SPECIMEN = "specimen"; 1867 @SearchParamDefinition(name = "issued", path = "DiagnosticReport.issued", description = "When the report was issued", type = "date") 1868 public static final String SP_ISSUED = "issued"; 1869 @SearchParamDefinition(name = "category", path = "DiagnosticReport.category", description = "Which diagnostic discipline/department created the report", type = "token") 1870 public static final String SP_CATEGORY = "category"; 1871 @SearchParamDefinition(name = "status", path = "DiagnosticReport.status", description = "The status of the report", type = "token") 1872 public static final String SP_STATUS = "status"; 1873 1874}