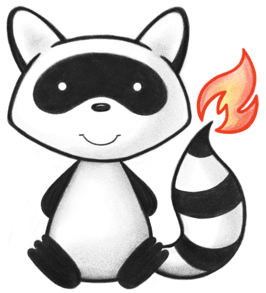
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus; 038import org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatusEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.utilities.Utilities; 047 048/** 049 * A manifest that defines a set of documents. 050 */ 051@ResourceDef(name = "DocumentManifest", profile = "http://hl7.org/fhir/Profile/DocumentManifest") 052public class DocumentManifest extends DomainResource { 053 054 @Block() 055 public static class DocumentManifestContentComponent extends BackboneElement implements IBaseBackboneElement { 056 /** 057 * The list of references to document content, or Attachment that consist of the 058 * parts of this document manifest. Usually, these would be document references, 059 * but direct references to Media or Attachments are also allowed. 060 */ 061 @Child(name = "p", type = { Attachment.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 062 @Description(shortDefinition = "Contents of this set of documents", formalDefinition = "The list of references to document content, or Attachment that consist of the parts of this document manifest. Usually, these would be document references, but direct references to Media or Attachments are also allowed.") 063 protected Type p; 064 065 private static final long serialVersionUID = -347538500L; 066 067 /* 068 * Constructor 069 */ 070 public DocumentManifestContentComponent() { 071 super(); 072 } 073 074 /* 075 * Constructor 076 */ 077 public DocumentManifestContentComponent(Type p) { 078 super(); 079 this.p = p; 080 } 081 082 /** 083 * @return {@link #p} (The list of references to document content, or Attachment 084 * that consist of the parts of this document manifest. Usually, these 085 * would be document references, but direct references to Media or 086 * Attachments are also allowed.) 087 */ 088 public Type getP() { 089 return this.p; 090 } 091 092 /** 093 * @return {@link #p} (The list of references to document content, or Attachment 094 * that consist of the parts of this document manifest. Usually, these 095 * would be document references, but direct references to Media or 096 * Attachments are also allowed.) 097 */ 098 public Attachment getPAttachment() throws FHIRException { 099 if (!(this.p instanceof Attachment)) 100 throw new FHIRException( 101 "Type mismatch: the type Attachment was expected, but " + this.p.getClass().getName() + " was encountered"); 102 return (Attachment) this.p; 103 } 104 105 public boolean hasPAttachment() { 106 return this.p instanceof Attachment; 107 } 108 109 /** 110 * @return {@link #p} (The list of references to document content, or Attachment 111 * that consist of the parts of this document manifest. Usually, these 112 * would be document references, but direct references to Media or 113 * Attachments are also allowed.) 114 */ 115 public Reference getPReference() throws FHIRException { 116 if (!(this.p instanceof Reference)) 117 throw new FHIRException( 118 "Type mismatch: the type Reference was expected, but " + this.p.getClass().getName() + " was encountered"); 119 return (Reference) this.p; 120 } 121 122 public boolean hasPReference() { 123 return this.p instanceof Reference; 124 } 125 126 public boolean hasP() { 127 return this.p != null && !this.p.isEmpty(); 128 } 129 130 /** 131 * @param value {@link #p} (The list of references to document content, or 132 * Attachment that consist of the parts of this document manifest. 133 * Usually, these would be document references, but direct 134 * references to Media or Attachments are also allowed.) 135 */ 136 public DocumentManifestContentComponent setP(Type value) { 137 this.p = value; 138 return this; 139 } 140 141 protected void listChildren(List<Property> childrenList) { 142 super.listChildren(childrenList); 143 childrenList.add(new Property("p[x]", "Attachment|Reference(Any)", 144 "The list of references to document content, or Attachment that consist of the parts of this document manifest. Usually, these would be document references, but direct references to Media or Attachments are also allowed.", 145 0, java.lang.Integer.MAX_VALUE, p)); 146 } 147 148 @Override 149 public void setProperty(String name, Base value) throws FHIRException { 150 if (name.equals("p[x]")) 151 this.p = (Type) value; // Type 152 else 153 super.setProperty(name, value); 154 } 155 156 @Override 157 public Base addChild(String name) throws FHIRException { 158 if (name.equals("pAttachment")) { 159 this.p = new Attachment(); 160 return this.p; 161 } else if (name.equals("pReference")) { 162 this.p = new Reference(); 163 return this.p; 164 } else 165 return super.addChild(name); 166 } 167 168 public DocumentManifestContentComponent copy() { 169 DocumentManifestContentComponent dst = new DocumentManifestContentComponent(); 170 copyValues(dst); 171 dst.p = p == null ? null : p.copy(); 172 return dst; 173 } 174 175 @Override 176 public boolean equalsDeep(Base other) { 177 if (!super.equalsDeep(other)) 178 return false; 179 if (!(other instanceof DocumentManifestContentComponent)) 180 return false; 181 DocumentManifestContentComponent o = (DocumentManifestContentComponent) other; 182 return compareDeep(p, o.p, true); 183 } 184 185 @Override 186 public boolean equalsShallow(Base other) { 187 if (!super.equalsShallow(other)) 188 return false; 189 if (!(other instanceof DocumentManifestContentComponent)) 190 return false; 191 DocumentManifestContentComponent o = (DocumentManifestContentComponent) other; 192 return true; 193 } 194 195 public boolean isEmpty() { 196 return super.isEmpty() && (p == null || p.isEmpty()); 197 } 198 199 public String fhirType() { 200 return "DocumentManifest.content"; 201 202 } 203 204 } 205 206 @Block() 207 public static class DocumentManifestRelatedComponent extends BackboneElement implements IBaseBackboneElement { 208 /** 209 * Related identifier to this DocumentManifest. For example, Order numbers, 210 * accession numbers, XDW workflow numbers. 211 */ 212 @Child(name = "identifier", type = { 213 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 214 @Description(shortDefinition = "Identifiers of things that are related", formalDefinition = "Related identifier to this DocumentManifest. For example, Order numbers, accession numbers, XDW workflow numbers.") 215 protected Identifier identifier; 216 217 /** 218 * Related Resource to this DocumentManifest. For example, Order, 219 * DiagnosticOrder, Procedure, EligibilityRequest, etc. 220 */ 221 @Child(name = "ref", type = {}, order = 2, min = 0, max = 1, modifier = false, summary = true) 222 @Description(shortDefinition = "Related Resource", formalDefinition = "Related Resource to this DocumentManifest. For example, Order, DiagnosticOrder, Procedure, EligibilityRequest, etc.") 223 protected Reference ref; 224 225 /** 226 * The actual object that is the target of the reference (Related Resource to 227 * this DocumentManifest. For example, Order, DiagnosticOrder, Procedure, 228 * EligibilityRequest, etc.) 229 */ 230 protected Resource refTarget; 231 232 private static final long serialVersionUID = -1670123330L; 233 234 /* 235 * Constructor 236 */ 237 public DocumentManifestRelatedComponent() { 238 super(); 239 } 240 241 /** 242 * @return {@link #identifier} (Related identifier to this DocumentManifest. For 243 * example, Order numbers, accession numbers, XDW workflow numbers.) 244 */ 245 public Identifier getIdentifier() { 246 if (this.identifier == null) 247 if (Configuration.errorOnAutoCreate()) 248 throw new Error("Attempt to auto-create DocumentManifestRelatedComponent.identifier"); 249 else if (Configuration.doAutoCreate()) 250 this.identifier = new Identifier(); // cc 251 return this.identifier; 252 } 253 254 public boolean hasIdentifier() { 255 return this.identifier != null && !this.identifier.isEmpty(); 256 } 257 258 /** 259 * @param value {@link #identifier} (Related identifier to this 260 * DocumentManifest. For example, Order numbers, accession numbers, 261 * XDW workflow numbers.) 262 */ 263 public DocumentManifestRelatedComponent setIdentifier(Identifier value) { 264 this.identifier = value; 265 return this; 266 } 267 268 /** 269 * @return {@link #ref} (Related Resource to this DocumentManifest. For example, 270 * Order, DiagnosticOrder, Procedure, EligibilityRequest, etc.) 271 */ 272 public Reference getRef() { 273 if (this.ref == null) 274 if (Configuration.errorOnAutoCreate()) 275 throw new Error("Attempt to auto-create DocumentManifestRelatedComponent.ref"); 276 else if (Configuration.doAutoCreate()) 277 this.ref = new Reference(); // cc 278 return this.ref; 279 } 280 281 public boolean hasRef() { 282 return this.ref != null && !this.ref.isEmpty(); 283 } 284 285 /** 286 * @param value {@link #ref} (Related Resource to this DocumentManifest. For 287 * example, Order, DiagnosticOrder, Procedure, EligibilityRequest, 288 * etc.) 289 */ 290 public DocumentManifestRelatedComponent setRef(Reference value) { 291 this.ref = value; 292 return this; 293 } 294 295 /** 296 * @return {@link #ref} The actual object that is the target of the reference. 297 * The reference library doesn't populate this, but you can use it to 298 * hold the resource if you resolve it. (Related Resource to this 299 * DocumentManifest. For example, Order, DiagnosticOrder, Procedure, 300 * EligibilityRequest, etc.) 301 */ 302 public Resource getRefTarget() { 303 return this.refTarget; 304 } 305 306 /** 307 * @param value {@link #ref} The actual object that is the target of the 308 * reference. The reference library doesn't use these, but you can 309 * use it to hold the resource if you resolve it. (Related Resource 310 * to this DocumentManifest. For example, Order, DiagnosticOrder, 311 * Procedure, EligibilityRequest, etc.) 312 */ 313 public DocumentManifestRelatedComponent setRefTarget(Resource value) { 314 this.refTarget = value; 315 return this; 316 } 317 318 protected void listChildren(List<Property> childrenList) { 319 super.listChildren(childrenList); 320 childrenList.add(new Property("identifier", "Identifier", 321 "Related identifier to this DocumentManifest. For example, Order numbers, accession numbers, XDW workflow numbers.", 322 0, java.lang.Integer.MAX_VALUE, identifier)); 323 childrenList.add(new Property("ref", "Reference(Any)", 324 "Related Resource to this DocumentManifest. For example, Order, DiagnosticOrder, Procedure, EligibilityRequest, etc.", 325 0, java.lang.Integer.MAX_VALUE, ref)); 326 } 327 328 @Override 329 public void setProperty(String name, Base value) throws FHIRException { 330 if (name.equals("identifier")) 331 this.identifier = castToIdentifier(value); // Identifier 332 else if (name.equals("ref")) 333 this.ref = castToReference(value); // Reference 334 else 335 super.setProperty(name, value); 336 } 337 338 @Override 339 public Base addChild(String name) throws FHIRException { 340 if (name.equals("identifier")) { 341 this.identifier = new Identifier(); 342 return this.identifier; 343 } else if (name.equals("ref")) { 344 this.ref = new Reference(); 345 return this.ref; 346 } else 347 return super.addChild(name); 348 } 349 350 public DocumentManifestRelatedComponent copy() { 351 DocumentManifestRelatedComponent dst = new DocumentManifestRelatedComponent(); 352 copyValues(dst); 353 dst.identifier = identifier == null ? null : identifier.copy(); 354 dst.ref = ref == null ? null : ref.copy(); 355 return dst; 356 } 357 358 @Override 359 public boolean equalsDeep(Base other) { 360 if (!super.equalsDeep(other)) 361 return false; 362 if (!(other instanceof DocumentManifestRelatedComponent)) 363 return false; 364 DocumentManifestRelatedComponent o = (DocumentManifestRelatedComponent) other; 365 return compareDeep(identifier, o.identifier, true) && compareDeep(ref, o.ref, true); 366 } 367 368 @Override 369 public boolean equalsShallow(Base other) { 370 if (!super.equalsShallow(other)) 371 return false; 372 if (!(other instanceof DocumentManifestRelatedComponent)) 373 return false; 374 DocumentManifestRelatedComponent o = (DocumentManifestRelatedComponent) other; 375 return true; 376 } 377 378 public boolean isEmpty() { 379 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (ref == null || ref.isEmpty()); 380 } 381 382 public String fhirType() { 383 return "DocumentManifest.related"; 384 385 } 386 387 } 388 389 /** 390 * A single identifier that uniquely identifies this manifest. Principally used 391 * to refer to the manifest in non-FHIR contexts. 392 */ 393 @Child(name = "masterIdentifier", type = { 394 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 395 @Description(shortDefinition = "Unique Identifier for the set of documents", formalDefinition = "A single identifier that uniquely identifies this manifest. Principally used to refer to the manifest in non-FHIR contexts.") 396 protected Identifier masterIdentifier; 397 398 /** 399 * Other identifiers associated with the document manifest, including version 400 * independent identifiers. 401 */ 402 @Child(name = "identifier", type = { 403 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 404 @Description(shortDefinition = "Other identifiers for the manifest", formalDefinition = "Other identifiers associated with the document manifest, including version independent identifiers.") 405 protected List<Identifier> identifier; 406 407 /** 408 * Who or what the set of documents is about. The documents can be about a 409 * person, (patient or healthcare practitioner), a device (i.e. machine) or even 410 * a group of subjects (such as a document about a herd of farm animals, or a 411 * set of patients that share a common exposure). If the documents cross more 412 * than one subject, then more than one subject is allowed here (unusual use 413 * case). 414 */ 415 @Child(name = "subject", type = { Patient.class, Practitioner.class, Group.class, 416 Device.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 417 @Description(shortDefinition = "The subject of the set of documents", formalDefinition = "Who or what the set of documents is about. The documents can be about a person, (patient or healthcare practitioner), a device (i.e. machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure). If the documents cross more than one subject, then more than one subject is allowed here (unusual use case).") 418 protected Reference subject; 419 420 /** 421 * The actual object that is the target of the reference (Who or what the set of 422 * documents is about. The documents can be about a person, (patient or 423 * healthcare practitioner), a device (i.e. machine) or even a group of subjects 424 * (such as a document about a herd of farm animals, or a set of patients that 425 * share a common exposure). If the documents cross more than one subject, then 426 * more than one subject is allowed here (unusual use case).) 427 */ 428 protected Resource subjectTarget; 429 430 /** 431 * A patient, practitioner, or organization for which this set of documents is 432 * intended. 433 */ 434 @Child(name = "recipient", type = { Patient.class, Practitioner.class, RelatedPerson.class, 435 Organization.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 436 @Description(shortDefinition = "Intended to get notified about this set of documents", formalDefinition = "A patient, practitioner, or organization for which this set of documents is intended.") 437 protected List<Reference> recipient; 438 /** 439 * The actual objects that are the target of the reference (A patient, 440 * practitioner, or organization for which this set of documents is intended.) 441 */ 442 protected List<Resource> recipientTarget; 443 444 /** 445 * Specifies the kind of this set of documents (e.g. Patient Summary, Discharge 446 * Summary, Prescription, etc.). The type of a set of documents may be the same 447 * as one of the documents in it - especially if there is only one - but it may 448 * be wider. 449 */ 450 @Child(name = "type", type = { CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 451 @Description(shortDefinition = "Kind of document set", formalDefinition = "Specifies the kind of this set of documents (e.g. Patient Summary, Discharge Summary, Prescription, etc.). The type of a set of documents may be the same as one of the documents in it - especially if there is only one - but it may be wider.") 452 protected CodeableConcept type; 453 454 /** 455 * Identifies who is responsible for creating the manifest, and adding documents 456 * to it. 457 */ 458 @Child(name = "author", type = { Practitioner.class, Organization.class, Device.class, Patient.class, 459 RelatedPerson.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 460 @Description(shortDefinition = "Who and/or what authored the manifest", formalDefinition = "Identifies who is responsible for creating the manifest, and adding documents to it.") 461 protected List<Reference> author; 462 /** 463 * The actual objects that are the target of the reference (Identifies who is 464 * responsible for creating the manifest, and adding documents to it.) 465 */ 466 protected List<Resource> authorTarget; 467 468 /** 469 * When the document manifest was created for submission to the server (not 470 * necessarily the same thing as the actual resource last modified time, since 471 * it may be modified, replicated, etc.). 472 */ 473 @Child(name = "created", type = { DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 474 @Description(shortDefinition = "When this document manifest created", formalDefinition = "When the document manifest was created for submission to the server (not necessarily the same thing as the actual resource last modified time, since it may be modified, replicated, etc.).") 475 protected DateTimeType created; 476 477 /** 478 * Identifies the source system, application, or software that produced the 479 * document manifest. 480 */ 481 @Child(name = "source", type = { UriType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 482 @Description(shortDefinition = "The source system/application/software", formalDefinition = "Identifies the source system, application, or software that produced the document manifest.") 483 protected UriType source; 484 485 /** 486 * The status of this document manifest. 487 */ 488 @Child(name = "status", type = { CodeType.class }, order = 8, min = 1, max = 1, modifier = true, summary = true) 489 @Description(shortDefinition = "current | superseded | entered-in-error", formalDefinition = "The status of this document manifest.") 490 protected Enumeration<DocumentReferenceStatus> status; 491 492 /** 493 * Human-readable description of the source document. This is sometimes known as 494 * the "title". 495 */ 496 @Child(name = "description", type = { 497 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 498 @Description(shortDefinition = "Human-readable description (title)", formalDefinition = "Human-readable description of the source document. This is sometimes known as the \"title\".") 499 protected StringType description; 500 501 /** 502 * The list of Documents included in the manifest. 503 */ 504 @Child(name = "content", type = {}, order = 10, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 505 @Description(shortDefinition = "The items included", formalDefinition = "The list of Documents included in the manifest.") 506 protected List<DocumentManifestContentComponent> content; 507 508 /** 509 * Related identifiers or resources associated with the DocumentManifest. 510 */ 511 @Child(name = "related", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 512 @Description(shortDefinition = "Related things", formalDefinition = "Related identifiers or resources associated with the DocumentManifest.") 513 protected List<DocumentManifestRelatedComponent> related; 514 515 private static final long serialVersionUID = -2056683927L; 516 517 /* 518 * Constructor 519 */ 520 public DocumentManifest() { 521 super(); 522 } 523 524 /* 525 * Constructor 526 */ 527 public DocumentManifest(Enumeration<DocumentReferenceStatus> status) { 528 super(); 529 this.status = status; 530 } 531 532 /** 533 * @return {@link #masterIdentifier} (A single identifier that uniquely 534 * identifies this manifest. Principally used to refer to the manifest 535 * in non-FHIR contexts.) 536 */ 537 public Identifier getMasterIdentifier() { 538 if (this.masterIdentifier == null) 539 if (Configuration.errorOnAutoCreate()) 540 throw new Error("Attempt to auto-create DocumentManifest.masterIdentifier"); 541 else if (Configuration.doAutoCreate()) 542 this.masterIdentifier = new Identifier(); // cc 543 return this.masterIdentifier; 544 } 545 546 public boolean hasMasterIdentifier() { 547 return this.masterIdentifier != null && !this.masterIdentifier.isEmpty(); 548 } 549 550 /** 551 * @param value {@link #masterIdentifier} (A single identifier that uniquely 552 * identifies this manifest. Principally used to refer to the 553 * manifest in non-FHIR contexts.) 554 */ 555 public DocumentManifest setMasterIdentifier(Identifier value) { 556 this.masterIdentifier = value; 557 return this; 558 } 559 560 /** 561 * @return {@link #identifier} (Other identifiers associated with the document 562 * manifest, including version independent identifiers.) 563 */ 564 public List<Identifier> getIdentifier() { 565 if (this.identifier == null) 566 this.identifier = new ArrayList<Identifier>(); 567 return this.identifier; 568 } 569 570 public boolean hasIdentifier() { 571 if (this.identifier == null) 572 return false; 573 for (Identifier item : this.identifier) 574 if (!item.isEmpty()) 575 return true; 576 return false; 577 } 578 579 /** 580 * @return {@link #identifier} (Other identifiers associated with the document 581 * manifest, including version independent identifiers.) 582 */ 583 // syntactic sugar 584 public Identifier addIdentifier() { // 3 585 Identifier t = new Identifier(); 586 if (this.identifier == null) 587 this.identifier = new ArrayList<Identifier>(); 588 this.identifier.add(t); 589 return t; 590 } 591 592 // syntactic sugar 593 public DocumentManifest addIdentifier(Identifier t) { // 3 594 if (t == null) 595 return this; 596 if (this.identifier == null) 597 this.identifier = new ArrayList<Identifier>(); 598 this.identifier.add(t); 599 return this; 600 } 601 602 /** 603 * @return {@link #subject} (Who or what the set of documents is about. The 604 * documents can be about a person, (patient or healthcare 605 * practitioner), a device (i.e. machine) or even a group of subjects 606 * (such as a document about a herd of farm animals, or a set of 607 * patients that share a common exposure). If the documents cross more 608 * than one subject, then more than one subject is allowed here (unusual 609 * use case).) 610 */ 611 public Reference getSubject() { 612 if (this.subject == null) 613 if (Configuration.errorOnAutoCreate()) 614 throw new Error("Attempt to auto-create DocumentManifest.subject"); 615 else if (Configuration.doAutoCreate()) 616 this.subject = new Reference(); // cc 617 return this.subject; 618 } 619 620 public boolean hasSubject() { 621 return this.subject != null && !this.subject.isEmpty(); 622 } 623 624 /** 625 * @param value {@link #subject} (Who or what the set of documents is about. The 626 * documents can be about a person, (patient or healthcare 627 * practitioner), a device (i.e. machine) or even a group of 628 * subjects (such as a document about a herd of farm animals, or a 629 * set of patients that share a common exposure). If the documents 630 * cross more than one subject, then more than one subject is 631 * allowed here (unusual use case).) 632 */ 633 public DocumentManifest setSubject(Reference value) { 634 this.subject = value; 635 return this; 636 } 637 638 /** 639 * @return {@link #subject} The actual object that is the target of the 640 * reference. The reference library doesn't populate this, but you can 641 * use it to hold the resource if you resolve it. (Who or what the set 642 * of documents is about. The documents can be about a person, (patient 643 * or healthcare practitioner), a device (i.e. machine) or even a group 644 * of subjects (such as a document about a herd of farm animals, or a 645 * set of patients that share a common exposure). If the documents cross 646 * more than one subject, then more than one subject is allowed here 647 * (unusual use case).) 648 */ 649 public Resource getSubjectTarget() { 650 return this.subjectTarget; 651 } 652 653 /** 654 * @param value {@link #subject} The actual object that is the target of the 655 * reference. The reference library doesn't use these, but you can 656 * use it to hold the resource if you resolve it. (Who or what the 657 * set of documents is about. The documents can be about a person, 658 * (patient or healthcare practitioner), a device (i.e. machine) or 659 * even a group of subjects (such as a document about a herd of 660 * farm animals, or a set of patients that share a common 661 * exposure). If the documents cross more than one subject, then 662 * more than one subject is allowed here (unusual use case).) 663 */ 664 public DocumentManifest setSubjectTarget(Resource value) { 665 this.subjectTarget = value; 666 return this; 667 } 668 669 /** 670 * @return {@link #recipient} (A patient, practitioner, or organization for 671 * which this set of documents is intended.) 672 */ 673 public List<Reference> getRecipient() { 674 if (this.recipient == null) 675 this.recipient = new ArrayList<Reference>(); 676 return this.recipient; 677 } 678 679 public boolean hasRecipient() { 680 if (this.recipient == null) 681 return false; 682 for (Reference item : this.recipient) 683 if (!item.isEmpty()) 684 return true; 685 return false; 686 } 687 688 /** 689 * @return {@link #recipient} (A patient, practitioner, or organization for 690 * which this set of documents is intended.) 691 */ 692 // syntactic sugar 693 public Reference addRecipient() { // 3 694 Reference t = new Reference(); 695 if (this.recipient == null) 696 this.recipient = new ArrayList<Reference>(); 697 this.recipient.add(t); 698 return t; 699 } 700 701 // syntactic sugar 702 public DocumentManifest addRecipient(Reference t) { // 3 703 if (t == null) 704 return this; 705 if (this.recipient == null) 706 this.recipient = new ArrayList<Reference>(); 707 this.recipient.add(t); 708 return this; 709 } 710 711 /** 712 * @return {@link #recipient} (The actual objects that are the target of the 713 * reference. The reference library doesn't populate this, but you can 714 * use this to hold the resources if you resolvethemt. A patient, 715 * practitioner, or organization for which this set of documents is 716 * intended.) 717 */ 718 public List<Resource> getRecipientTarget() { 719 if (this.recipientTarget == null) 720 this.recipientTarget = new ArrayList<Resource>(); 721 return this.recipientTarget; 722 } 723 724 /** 725 * @return {@link #type} (Specifies the kind of this set of documents (e.g. 726 * Patient Summary, Discharge Summary, Prescription, etc.). The type of 727 * a set of documents may be the same as one of the documents in it - 728 * especially if there is only one - but it may be wider.) 729 */ 730 public CodeableConcept getType() { 731 if (this.type == null) 732 if (Configuration.errorOnAutoCreate()) 733 throw new Error("Attempt to auto-create DocumentManifest.type"); 734 else if (Configuration.doAutoCreate()) 735 this.type = new CodeableConcept(); // cc 736 return this.type; 737 } 738 739 public boolean hasType() { 740 return this.type != null && !this.type.isEmpty(); 741 } 742 743 /** 744 * @param value {@link #type} (Specifies the kind of this set of documents (e.g. 745 * Patient Summary, Discharge Summary, Prescription, etc.). The 746 * type of a set of documents may be the same as one of the 747 * documents in it - especially if there is only one - but it may 748 * be wider.) 749 */ 750 public DocumentManifest setType(CodeableConcept value) { 751 this.type = value; 752 return this; 753 } 754 755 /** 756 * @return {@link #author} (Identifies who is responsible for creating the 757 * manifest, and adding documents to it.) 758 */ 759 public List<Reference> getAuthor() { 760 if (this.author == null) 761 this.author = new ArrayList<Reference>(); 762 return this.author; 763 } 764 765 public boolean hasAuthor() { 766 if (this.author == null) 767 return false; 768 for (Reference item : this.author) 769 if (!item.isEmpty()) 770 return true; 771 return false; 772 } 773 774 /** 775 * @return {@link #author} (Identifies who is responsible for creating the 776 * manifest, and adding documents to it.) 777 */ 778 // syntactic sugar 779 public Reference addAuthor() { // 3 780 Reference t = new Reference(); 781 if (this.author == null) 782 this.author = new ArrayList<Reference>(); 783 this.author.add(t); 784 return t; 785 } 786 787 // syntactic sugar 788 public DocumentManifest addAuthor(Reference t) { // 3 789 if (t == null) 790 return this; 791 if (this.author == null) 792 this.author = new ArrayList<Reference>(); 793 this.author.add(t); 794 return this; 795 } 796 797 /** 798 * @return {@link #author} (The actual objects that are the target of the 799 * reference. The reference library doesn't populate this, but you can 800 * use this to hold the resources if you resolvethemt. Identifies who is 801 * responsible for creating the manifest, and adding documents to it.) 802 */ 803 public List<Resource> getAuthorTarget() { 804 if (this.authorTarget == null) 805 this.authorTarget = new ArrayList<Resource>(); 806 return this.authorTarget; 807 } 808 809 /** 810 * @return {@link #created} (When the document manifest was created for 811 * submission to the server (not necessarily the same thing as the 812 * actual resource last modified time, since it may be modified, 813 * replicated, etc.).). This is the underlying object with id, value and 814 * extensions. The accessor "getCreated" gives direct access to the 815 * value 816 */ 817 public DateTimeType getCreatedElement() { 818 if (this.created == null) 819 if (Configuration.errorOnAutoCreate()) 820 throw new Error("Attempt to auto-create DocumentManifest.created"); 821 else if (Configuration.doAutoCreate()) 822 this.created = new DateTimeType(); // bb 823 return this.created; 824 } 825 826 public boolean hasCreatedElement() { 827 return this.created != null && !this.created.isEmpty(); 828 } 829 830 public boolean hasCreated() { 831 return this.created != null && !this.created.isEmpty(); 832 } 833 834 /** 835 * @param value {@link #created} (When the document manifest was created for 836 * submission to the server (not necessarily the same thing as the 837 * actual resource last modified time, since it may be modified, 838 * replicated, etc.).). This is the underlying object with id, 839 * value and extensions. The accessor "getCreated" gives direct 840 * access to the value 841 */ 842 public DocumentManifest setCreatedElement(DateTimeType value) { 843 this.created = value; 844 return this; 845 } 846 847 /** 848 * @return When the document manifest was created for submission to the server 849 * (not necessarily the same thing as the actual resource last modified 850 * time, since it may be modified, replicated, etc.). 851 */ 852 public Date getCreated() { 853 return this.created == null ? null : this.created.getValue(); 854 } 855 856 /** 857 * @param value When the document manifest was created for submission to the 858 * server (not necessarily the same thing as the actual resource 859 * last modified time, since it may be modified, replicated, etc.). 860 */ 861 public DocumentManifest setCreated(Date value) { 862 if (value == null) 863 this.created = null; 864 else { 865 if (this.created == null) 866 this.created = new DateTimeType(); 867 this.created.setValue(value); 868 } 869 return this; 870 } 871 872 /** 873 * @return {@link #source} (Identifies the source system, application, or 874 * software that produced the document manifest.). This is the 875 * underlying object with id, value and extensions. The accessor 876 * "getSource" gives direct access to the value 877 */ 878 public UriType getSourceElement() { 879 if (this.source == null) 880 if (Configuration.errorOnAutoCreate()) 881 throw new Error("Attempt to auto-create DocumentManifest.source"); 882 else if (Configuration.doAutoCreate()) 883 this.source = new UriType(); // bb 884 return this.source; 885 } 886 887 public boolean hasSourceElement() { 888 return this.source != null && !this.source.isEmpty(); 889 } 890 891 public boolean hasSource() { 892 return this.source != null && !this.source.isEmpty(); 893 } 894 895 /** 896 * @param value {@link #source} (Identifies the source system, application, or 897 * software that produced the document manifest.). This is the 898 * underlying object with id, value and extensions. The accessor 899 * "getSource" gives direct access to the value 900 */ 901 public DocumentManifest setSourceElement(UriType value) { 902 this.source = value; 903 return this; 904 } 905 906 /** 907 * @return Identifies the source system, application, or software that produced 908 * the document manifest. 909 */ 910 public String getSource() { 911 return this.source == null ? null : this.source.getValue(); 912 } 913 914 /** 915 * @param value Identifies the source system, application, or software that 916 * produced the document manifest. 917 */ 918 public DocumentManifest setSource(String value) { 919 if (Utilities.noString(value)) 920 this.source = null; 921 else { 922 if (this.source == null) 923 this.source = new UriType(); 924 this.source.setValue(value); 925 } 926 return this; 927 } 928 929 /** 930 * @return {@link #status} (The status of this document manifest.). This is the 931 * underlying object with id, value and extensions. The accessor 932 * "getStatus" gives direct access to the value 933 */ 934 public Enumeration<DocumentReferenceStatus> getStatusElement() { 935 if (this.status == null) 936 if (Configuration.errorOnAutoCreate()) 937 throw new Error("Attempt to auto-create DocumentManifest.status"); 938 else if (Configuration.doAutoCreate()) 939 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); // bb 940 return this.status; 941 } 942 943 public boolean hasStatusElement() { 944 return this.status != null && !this.status.isEmpty(); 945 } 946 947 public boolean hasStatus() { 948 return this.status != null && !this.status.isEmpty(); 949 } 950 951 /** 952 * @param value {@link #status} (The status of this document manifest.). This is 953 * the underlying object with id, value and extensions. The 954 * accessor "getStatus" gives direct access to the value 955 */ 956 public DocumentManifest setStatusElement(Enumeration<DocumentReferenceStatus> value) { 957 this.status = value; 958 return this; 959 } 960 961 /** 962 * @return The status of this document manifest. 963 */ 964 public DocumentReferenceStatus getStatus() { 965 return this.status == null ? null : this.status.getValue(); 966 } 967 968 /** 969 * @param value The status of this document manifest. 970 */ 971 public DocumentManifest setStatus(DocumentReferenceStatus value) { 972 if (this.status == null) 973 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); 974 this.status.setValue(value); 975 return this; 976 } 977 978 /** 979 * @return {@link #description} (Human-readable description of the source 980 * document. This is sometimes known as the "title".). This is the 981 * underlying object with id, value and extensions. The accessor 982 * "getDescription" gives direct access to the value 983 */ 984 public StringType getDescriptionElement() { 985 if (this.description == null) 986 if (Configuration.errorOnAutoCreate()) 987 throw new Error("Attempt to auto-create DocumentManifest.description"); 988 else if (Configuration.doAutoCreate()) 989 this.description = new StringType(); // bb 990 return this.description; 991 } 992 993 public boolean hasDescriptionElement() { 994 return this.description != null && !this.description.isEmpty(); 995 } 996 997 public boolean hasDescription() { 998 return this.description != null && !this.description.isEmpty(); 999 } 1000 1001 /** 1002 * @param value {@link #description} (Human-readable description of the source 1003 * document. This is sometimes known as the "title".). This is the 1004 * underlying object with id, value and extensions. The accessor 1005 * "getDescription" gives direct access to the value 1006 */ 1007 public DocumentManifest setDescriptionElement(StringType value) { 1008 this.description = value; 1009 return this; 1010 } 1011 1012 /** 1013 * @return Human-readable description of the source document. This is sometimes 1014 * known as the "title". 1015 */ 1016 public String getDescription() { 1017 return this.description == null ? null : this.description.getValue(); 1018 } 1019 1020 /** 1021 * @param value Human-readable description of the source document. This is 1022 * sometimes known as the "title". 1023 */ 1024 public DocumentManifest setDescription(String value) { 1025 if (Utilities.noString(value)) 1026 this.description = null; 1027 else { 1028 if (this.description == null) 1029 this.description = new StringType(); 1030 this.description.setValue(value); 1031 } 1032 return this; 1033 } 1034 1035 /** 1036 * @return {@link #content} (The list of Documents included in the manifest.) 1037 */ 1038 public List<DocumentManifestContentComponent> getContent() { 1039 if (this.content == null) 1040 this.content = new ArrayList<DocumentManifestContentComponent>(); 1041 return this.content; 1042 } 1043 1044 public boolean hasContent() { 1045 if (this.content == null) 1046 return false; 1047 for (DocumentManifestContentComponent item : this.content) 1048 if (!item.isEmpty()) 1049 return true; 1050 return false; 1051 } 1052 1053 /** 1054 * @return {@link #content} (The list of Documents included in the manifest.) 1055 */ 1056 // syntactic sugar 1057 public DocumentManifestContentComponent addContent() { // 3 1058 DocumentManifestContentComponent t = new DocumentManifestContentComponent(); 1059 if (this.content == null) 1060 this.content = new ArrayList<DocumentManifestContentComponent>(); 1061 this.content.add(t); 1062 return t; 1063 } 1064 1065 // syntactic sugar 1066 public DocumentManifest addContent(DocumentManifestContentComponent t) { // 3 1067 if (t == null) 1068 return this; 1069 if (this.content == null) 1070 this.content = new ArrayList<DocumentManifestContentComponent>(); 1071 this.content.add(t); 1072 return this; 1073 } 1074 1075 /** 1076 * @return {@link #related} (Related identifiers or resources associated with 1077 * the DocumentManifest.) 1078 */ 1079 public List<DocumentManifestRelatedComponent> getRelated() { 1080 if (this.related == null) 1081 this.related = new ArrayList<DocumentManifestRelatedComponent>(); 1082 return this.related; 1083 } 1084 1085 public boolean hasRelated() { 1086 if (this.related == null) 1087 return false; 1088 for (DocumentManifestRelatedComponent item : this.related) 1089 if (!item.isEmpty()) 1090 return true; 1091 return false; 1092 } 1093 1094 /** 1095 * @return {@link #related} (Related identifiers or resources associated with 1096 * the DocumentManifest.) 1097 */ 1098 // syntactic sugar 1099 public DocumentManifestRelatedComponent addRelated() { // 3 1100 DocumentManifestRelatedComponent t = new DocumentManifestRelatedComponent(); 1101 if (this.related == null) 1102 this.related = new ArrayList<DocumentManifestRelatedComponent>(); 1103 this.related.add(t); 1104 return t; 1105 } 1106 1107 // syntactic sugar 1108 public DocumentManifest addRelated(DocumentManifestRelatedComponent t) { // 3 1109 if (t == null) 1110 return this; 1111 if (this.related == null) 1112 this.related = new ArrayList<DocumentManifestRelatedComponent>(); 1113 this.related.add(t); 1114 return this; 1115 } 1116 1117 protected void listChildren(List<Property> childrenList) { 1118 super.listChildren(childrenList); 1119 childrenList.add(new Property("masterIdentifier", "Identifier", 1120 "A single identifier that uniquely identifies this manifest. Principally used to refer to the manifest in non-FHIR contexts.", 1121 0, java.lang.Integer.MAX_VALUE, masterIdentifier)); 1122 childrenList.add(new Property("identifier", "Identifier", 1123 "Other identifiers associated with the document manifest, including version independent identifiers.", 0, 1124 java.lang.Integer.MAX_VALUE, identifier)); 1125 childrenList.add(new Property("subject", "Reference(Patient|Practitioner|Group|Device)", 1126 "Who or what the set of documents is about. The documents can be about a person, (patient or healthcare practitioner), a device (i.e. machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure). If the documents cross more than one subject, then more than one subject is allowed here (unusual use case).", 1127 0, java.lang.Integer.MAX_VALUE, subject)); 1128 childrenList.add(new Property("recipient", "Reference(Patient|Practitioner|RelatedPerson|Organization)", 1129 "A patient, practitioner, or organization for which this set of documents is intended.", 0, 1130 java.lang.Integer.MAX_VALUE, recipient)); 1131 childrenList.add(new Property("type", "CodeableConcept", 1132 "Specifies the kind of this set of documents (e.g. Patient Summary, Discharge Summary, Prescription, etc.). The type of a set of documents may be the same as one of the documents in it - especially if there is only one - but it may be wider.", 1133 0, java.lang.Integer.MAX_VALUE, type)); 1134 childrenList.add(new Property("author", "Reference(Practitioner|Organization|Device|Patient|RelatedPerson)", 1135 "Identifies who is responsible for creating the manifest, and adding documents to it.", 0, 1136 java.lang.Integer.MAX_VALUE, author)); 1137 childrenList.add(new Property("created", "dateTime", 1138 "When the document manifest was created for submission to the server (not necessarily the same thing as the actual resource last modified time, since it may be modified, replicated, etc.).", 1139 0, java.lang.Integer.MAX_VALUE, created)); 1140 childrenList.add(new Property("source", "uri", 1141 "Identifies the source system, application, or software that produced the document manifest.", 0, 1142 java.lang.Integer.MAX_VALUE, source)); 1143 childrenList.add(new Property("status", "code", "The status of this document manifest.", 0, 1144 java.lang.Integer.MAX_VALUE, status)); 1145 childrenList.add(new Property("description", "string", 1146 "Human-readable description of the source document. This is sometimes known as the \"title\".", 0, 1147 java.lang.Integer.MAX_VALUE, description)); 1148 childrenList.add(new Property("content", "", "The list of Documents included in the manifest.", 0, 1149 java.lang.Integer.MAX_VALUE, content)); 1150 childrenList 1151 .add(new Property("related", "", "Related identifiers or resources associated with the DocumentManifest.", 0, 1152 java.lang.Integer.MAX_VALUE, related)); 1153 } 1154 1155 @Override 1156 public void setProperty(String name, Base value) throws FHIRException { 1157 if (name.equals("masterIdentifier")) 1158 this.masterIdentifier = castToIdentifier(value); // Identifier 1159 else if (name.equals("identifier")) 1160 this.getIdentifier().add(castToIdentifier(value)); 1161 else if (name.equals("subject")) 1162 this.subject = castToReference(value); // Reference 1163 else if (name.equals("recipient")) 1164 this.getRecipient().add(castToReference(value)); 1165 else if (name.equals("type")) 1166 this.type = castToCodeableConcept(value); // CodeableConcept 1167 else if (name.equals("author")) 1168 this.getAuthor().add(castToReference(value)); 1169 else if (name.equals("created")) 1170 this.created = castToDateTime(value); // DateTimeType 1171 else if (name.equals("source")) 1172 this.source = castToUri(value); // UriType 1173 else if (name.equals("status")) 1174 this.status = new DocumentReferenceStatusEnumFactory().fromType(value); // Enumeration<DocumentReferenceStatus> 1175 else if (name.equals("description")) 1176 this.description = castToString(value); // StringType 1177 else if (name.equals("content")) 1178 this.getContent().add((DocumentManifestContentComponent) value); 1179 else if (name.equals("related")) 1180 this.getRelated().add((DocumentManifestRelatedComponent) value); 1181 else 1182 super.setProperty(name, value); 1183 } 1184 1185 @Override 1186 public Base addChild(String name) throws FHIRException { 1187 if (name.equals("masterIdentifier")) { 1188 this.masterIdentifier = new Identifier(); 1189 return this.masterIdentifier; 1190 } else if (name.equals("identifier")) { 1191 return addIdentifier(); 1192 } else if (name.equals("subject")) { 1193 this.subject = new Reference(); 1194 return this.subject; 1195 } else if (name.equals("recipient")) { 1196 return addRecipient(); 1197 } else if (name.equals("type")) { 1198 this.type = new CodeableConcept(); 1199 return this.type; 1200 } else if (name.equals("author")) { 1201 return addAuthor(); 1202 } else if (name.equals("created")) { 1203 throw new FHIRException("Cannot call addChild on a singleton property DocumentManifest.created"); 1204 } else if (name.equals("source")) { 1205 throw new FHIRException("Cannot call addChild on a singleton property DocumentManifest.source"); 1206 } else if (name.equals("status")) { 1207 throw new FHIRException("Cannot call addChild on a singleton property DocumentManifest.status"); 1208 } else if (name.equals("description")) { 1209 throw new FHIRException("Cannot call addChild on a singleton property DocumentManifest.description"); 1210 } else if (name.equals("content")) { 1211 return addContent(); 1212 } else if (name.equals("related")) { 1213 return addRelated(); 1214 } else 1215 return super.addChild(name); 1216 } 1217 1218 public String fhirType() { 1219 return "DocumentManifest"; 1220 1221 } 1222 1223 public DocumentManifest copy() { 1224 DocumentManifest dst = new DocumentManifest(); 1225 copyValues(dst); 1226 dst.masterIdentifier = masterIdentifier == null ? null : masterIdentifier.copy(); 1227 if (identifier != null) { 1228 dst.identifier = new ArrayList<Identifier>(); 1229 for (Identifier i : identifier) 1230 dst.identifier.add(i.copy()); 1231 } 1232 ; 1233 dst.subject = subject == null ? null : subject.copy(); 1234 if (recipient != null) { 1235 dst.recipient = new ArrayList<Reference>(); 1236 for (Reference i : recipient) 1237 dst.recipient.add(i.copy()); 1238 } 1239 ; 1240 dst.type = type == null ? null : type.copy(); 1241 if (author != null) { 1242 dst.author = new ArrayList<Reference>(); 1243 for (Reference i : author) 1244 dst.author.add(i.copy()); 1245 } 1246 ; 1247 dst.created = created == null ? null : created.copy(); 1248 dst.source = source == null ? null : source.copy(); 1249 dst.status = status == null ? null : status.copy(); 1250 dst.description = description == null ? null : description.copy(); 1251 if (content != null) { 1252 dst.content = new ArrayList<DocumentManifestContentComponent>(); 1253 for (DocumentManifestContentComponent i : content) 1254 dst.content.add(i.copy()); 1255 } 1256 ; 1257 if (related != null) { 1258 dst.related = new ArrayList<DocumentManifestRelatedComponent>(); 1259 for (DocumentManifestRelatedComponent i : related) 1260 dst.related.add(i.copy()); 1261 } 1262 ; 1263 return dst; 1264 } 1265 1266 protected DocumentManifest typedCopy() { 1267 return copy(); 1268 } 1269 1270 @Override 1271 public boolean equalsDeep(Base other) { 1272 if (!super.equalsDeep(other)) 1273 return false; 1274 if (!(other instanceof DocumentManifest)) 1275 return false; 1276 DocumentManifest o = (DocumentManifest) other; 1277 return compareDeep(masterIdentifier, o.masterIdentifier, true) && compareDeep(identifier, o.identifier, true) 1278 && compareDeep(subject, o.subject, true) && compareDeep(recipient, o.recipient, true) 1279 && compareDeep(type, o.type, true) && compareDeep(author, o.author, true) 1280 && compareDeep(created, o.created, true) && compareDeep(source, o.source, true) 1281 && compareDeep(status, o.status, true) && compareDeep(description, o.description, true) 1282 && compareDeep(content, o.content, true) && compareDeep(related, o.related, true); 1283 } 1284 1285 @Override 1286 public boolean equalsShallow(Base other) { 1287 if (!super.equalsShallow(other)) 1288 return false; 1289 if (!(other instanceof DocumentManifest)) 1290 return false; 1291 DocumentManifest o = (DocumentManifest) other; 1292 return compareValues(created, o.created, true) && compareValues(source, o.source, true) 1293 && compareValues(status, o.status, true) && compareValues(description, o.description, true); 1294 } 1295 1296 public boolean isEmpty() { 1297 return super.isEmpty() && (masterIdentifier == null || masterIdentifier.isEmpty()) 1298 && (identifier == null || identifier.isEmpty()) && (subject == null || subject.isEmpty()) 1299 && (recipient == null || recipient.isEmpty()) && (type == null || type.isEmpty()) 1300 && (author == null || author.isEmpty()) && (created == null || created.isEmpty()) 1301 && (source == null || source.isEmpty()) && (status == null || status.isEmpty()) 1302 && (description == null || description.isEmpty()) && (content == null || content.isEmpty()) 1303 && (related == null || related.isEmpty()); 1304 } 1305 1306 @Override 1307 public ResourceType getResourceType() { 1308 return ResourceType.DocumentManifest; 1309 } 1310 1311 @SearchParamDefinition(name = "identifier", path = "DocumentManifest.masterIdentifier | DocumentManifest.identifier", description = "Unique Identifier for the set of documents", type = "token") 1312 public static final String SP_IDENTIFIER = "identifier"; 1313 @SearchParamDefinition(name = "related-id", path = "DocumentManifest.related.identifier", description = "Identifiers of things that are related", type = "token") 1314 public static final String SP_RELATEDID = "related-id"; 1315 @SearchParamDefinition(name = "content-ref", path = "DocumentManifest.content.pReference", description = "Contents of this set of documents", type = "reference") 1316 public static final String SP_CONTENTREF = "content-ref"; 1317 @SearchParamDefinition(name = "subject", path = "DocumentManifest.subject", description = "The subject of the set of documents", type = "reference") 1318 public static final String SP_SUBJECT = "subject"; 1319 @SearchParamDefinition(name = "author", path = "DocumentManifest.author", description = "Who and/or what authored the manifest", type = "reference") 1320 public static final String SP_AUTHOR = "author"; 1321 @SearchParamDefinition(name = "created", path = "DocumentManifest.created", description = "When this document manifest created", type = "date") 1322 public static final String SP_CREATED = "created"; 1323 @SearchParamDefinition(name = "description", path = "DocumentManifest.description", description = "Human-readable description (title)", type = "string") 1324 public static final String SP_DESCRIPTION = "description"; 1325 @SearchParamDefinition(name = "source", path = "DocumentManifest.source", description = "The source system/application/software", type = "uri") 1326 public static final String SP_SOURCE = "source"; 1327 @SearchParamDefinition(name = "type", path = "DocumentManifest.type", description = "Kind of document set", type = "token") 1328 public static final String SP_TYPE = "type"; 1329 @SearchParamDefinition(name = "related-ref", path = "DocumentManifest.related.ref", description = "Related Resource", type = "reference") 1330 public static final String SP_RELATEDREF = "related-ref"; 1331 @SearchParamDefinition(name = "patient", path = "DocumentManifest.subject", description = "The subject of the set of documents", type = "reference") 1332 public static final String SP_PATIENT = "patient"; 1333 @SearchParamDefinition(name = "recipient", path = "DocumentManifest.recipient", description = "Intended to get notified about this set of documents", type = "reference") 1334 public static final String SP_RECIPIENT = "recipient"; 1335 @SearchParamDefinition(name = "status", path = "DocumentManifest.status", description = "current | superseded | entered-in-error", type = "token") 1336 public static final String SP_STATUS = "status"; 1337 1338}