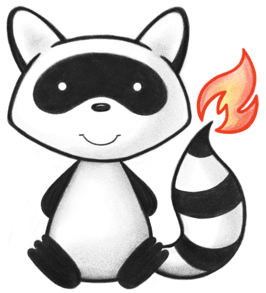
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus; 038import org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatusEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.utilities.Utilities; 047 048/** 049 * A reference to a document . 050 */ 051@ResourceDef(name = "DocumentReference", profile = "http://hl7.org/fhir/Profile/DocumentReference") 052public class DocumentReference extends DomainResource { 053 054 public enum DocumentRelationshipType { 055 /** 056 * This document logically replaces or supersedes the target document. 057 */ 058 REPLACES, 059 /** 060 * This document was generated by transforming the target document (e.g. format 061 * or language conversion). 062 */ 063 TRANSFORMS, 064 /** 065 * This document is a signature of the target document. 066 */ 067 SIGNS, 068 /** 069 * This document adds additional information to the target document. 070 */ 071 APPENDS, 072 /** 073 * added to help the parsers 074 */ 075 NULL; 076 077 public static DocumentRelationshipType fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("replaces".equals(codeString)) 081 return REPLACES; 082 if ("transforms".equals(codeString)) 083 return TRANSFORMS; 084 if ("signs".equals(codeString)) 085 return SIGNS; 086 if ("appends".equals(codeString)) 087 return APPENDS; 088 throw new FHIRException("Unknown DocumentRelationshipType code '" + codeString + "'"); 089 } 090 091 public String toCode() { 092 switch (this) { 093 case REPLACES: 094 return "replaces"; 095 case TRANSFORMS: 096 return "transforms"; 097 case SIGNS: 098 return "signs"; 099 case APPENDS: 100 return "appends"; 101 case NULL: 102 return null; 103 default: 104 return "?"; 105 } 106 } 107 108 public String getSystem() { 109 switch (this) { 110 case REPLACES: 111 return "http://hl7.org/fhir/document-relationship-type"; 112 case TRANSFORMS: 113 return "http://hl7.org/fhir/document-relationship-type"; 114 case SIGNS: 115 return "http://hl7.org/fhir/document-relationship-type"; 116 case APPENDS: 117 return "http://hl7.org/fhir/document-relationship-type"; 118 case NULL: 119 return null; 120 default: 121 return "?"; 122 } 123 } 124 125 public String getDefinition() { 126 switch (this) { 127 case REPLACES: 128 return "This document logically replaces or supersedes the target document."; 129 case TRANSFORMS: 130 return "This document was generated by transforming the target document (e.g. format or language conversion)."; 131 case SIGNS: 132 return "This document is a signature of the target document."; 133 case APPENDS: 134 return "This document adds additional information to the target document."; 135 case NULL: 136 return null; 137 default: 138 return "?"; 139 } 140 } 141 142 public String getDisplay() { 143 switch (this) { 144 case REPLACES: 145 return "Replaces"; 146 case TRANSFORMS: 147 return "Transforms"; 148 case SIGNS: 149 return "Signs"; 150 case APPENDS: 151 return "Appends"; 152 case NULL: 153 return null; 154 default: 155 return "?"; 156 } 157 } 158 } 159 160 public static class DocumentRelationshipTypeEnumFactory implements EnumFactory<DocumentRelationshipType> { 161 public DocumentRelationshipType fromCode(String codeString) throws IllegalArgumentException { 162 if (codeString == null || "".equals(codeString)) 163 if (codeString == null || "".equals(codeString)) 164 return null; 165 if ("replaces".equals(codeString)) 166 return DocumentRelationshipType.REPLACES; 167 if ("transforms".equals(codeString)) 168 return DocumentRelationshipType.TRANSFORMS; 169 if ("signs".equals(codeString)) 170 return DocumentRelationshipType.SIGNS; 171 if ("appends".equals(codeString)) 172 return DocumentRelationshipType.APPENDS; 173 throw new IllegalArgumentException("Unknown DocumentRelationshipType code '" + codeString + "'"); 174 } 175 176 public Enumeration<DocumentRelationshipType> fromType(Base code) throws FHIRException { 177 if (code == null || code.isEmpty()) 178 return null; 179 String codeString = ((PrimitiveType) code).asStringValue(); 180 if (codeString == null || "".equals(codeString)) 181 return null; 182 if ("replaces".equals(codeString)) 183 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.REPLACES); 184 if ("transforms".equals(codeString)) 185 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.TRANSFORMS); 186 if ("signs".equals(codeString)) 187 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.SIGNS); 188 if ("appends".equals(codeString)) 189 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.APPENDS); 190 throw new FHIRException("Unknown DocumentRelationshipType code '" + codeString + "'"); 191 } 192 193 public String toCode(DocumentRelationshipType code) 194 { 195 if (code == DocumentRelationshipType.NULL) 196 return null; 197 if (code == DocumentRelationshipType.REPLACES) 198 return "replaces"; 199 if (code == DocumentRelationshipType.TRANSFORMS) 200 return "transforms"; 201 if (code == DocumentRelationshipType.SIGNS) 202 return "signs"; 203 if (code == DocumentRelationshipType.APPENDS) 204 return "appends"; 205 return "?"; 206 } 207 } 208 209 @Block() 210 public static class DocumentReferenceRelatesToComponent extends BackboneElement implements IBaseBackboneElement { 211 /** 212 * The type of relationship that this document has with anther document. 213 */ 214 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 215 @Description(shortDefinition = "replaces | transforms | signs | appends", formalDefinition = "The type of relationship that this document has with anther document.") 216 protected Enumeration<DocumentRelationshipType> code; 217 218 /** 219 * The target document of this relationship. 220 */ 221 @Child(name = "target", type = { 222 DocumentReference.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 223 @Description(shortDefinition = "Target of the relationship", formalDefinition = "The target document of this relationship.") 224 protected Reference target; 225 226 /** 227 * The actual object that is the target of the reference (The target document of 228 * this relationship.) 229 */ 230 protected DocumentReference targetTarget; 231 232 private static final long serialVersionUID = -347257495L; 233 234 /* 235 * Constructor 236 */ 237 public DocumentReferenceRelatesToComponent() { 238 super(); 239 } 240 241 /* 242 * Constructor 243 */ 244 public DocumentReferenceRelatesToComponent(Enumeration<DocumentRelationshipType> code, Reference target) { 245 super(); 246 this.code = code; 247 this.target = target; 248 } 249 250 /** 251 * @return {@link #code} (The type of relationship that this document has with 252 * anther document.). This is the underlying object with id, value and 253 * extensions. The accessor "getCode" gives direct access to the value 254 */ 255 public Enumeration<DocumentRelationshipType> getCodeElement() { 256 if (this.code == null) 257 if (Configuration.errorOnAutoCreate()) 258 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.code"); 259 else if (Configuration.doAutoCreate()) 260 this.code = new Enumeration<DocumentRelationshipType>(new DocumentRelationshipTypeEnumFactory()); // bb 261 return this.code; 262 } 263 264 public boolean hasCodeElement() { 265 return this.code != null && !this.code.isEmpty(); 266 } 267 268 public boolean hasCode() { 269 return this.code != null && !this.code.isEmpty(); 270 } 271 272 /** 273 * @param value {@link #code} (The type of relationship that this document has 274 * with anther document.). This is the underlying object with id, 275 * value and extensions. The accessor "getCode" gives direct access 276 * to the value 277 */ 278 public DocumentReferenceRelatesToComponent setCodeElement(Enumeration<DocumentRelationshipType> value) { 279 this.code = value; 280 return this; 281 } 282 283 /** 284 * @return The type of relationship that this document has with anther document. 285 */ 286 public DocumentRelationshipType getCode() { 287 return this.code == null ? null : this.code.getValue(); 288 } 289 290 /** 291 * @param value The type of relationship that this document has with anther 292 * document. 293 */ 294 public DocumentReferenceRelatesToComponent setCode(DocumentRelationshipType value) { 295 if (this.code == null) 296 this.code = new Enumeration<DocumentRelationshipType>(new DocumentRelationshipTypeEnumFactory()); 297 this.code.setValue(value); 298 return this; 299 } 300 301 /** 302 * @return {@link #target} (The target document of this relationship.) 303 */ 304 public Reference getTarget() { 305 if (this.target == null) 306 if (Configuration.errorOnAutoCreate()) 307 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.target"); 308 else if (Configuration.doAutoCreate()) 309 this.target = new Reference(); // cc 310 return this.target; 311 } 312 313 public boolean hasTarget() { 314 return this.target != null && !this.target.isEmpty(); 315 } 316 317 /** 318 * @param value {@link #target} (The target document of this relationship.) 319 */ 320 public DocumentReferenceRelatesToComponent setTarget(Reference value) { 321 this.target = value; 322 return this; 323 } 324 325 /** 326 * @return {@link #target} The actual object that is the target of the 327 * reference. The reference library doesn't populate this, but you can 328 * use it to hold the resource if you resolve it. (The target document 329 * of this relationship.) 330 */ 331 public DocumentReference getTargetTarget() { 332 if (this.targetTarget == null) 333 if (Configuration.errorOnAutoCreate()) 334 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.target"); 335 else if (Configuration.doAutoCreate()) 336 this.targetTarget = new DocumentReference(); // aa 337 return this.targetTarget; 338 } 339 340 /** 341 * @param value {@link #target} The actual object that is the target of the 342 * reference. The reference library doesn't use these, but you can 343 * use it to hold the resource if you resolve it. (The target 344 * document of this relationship.) 345 */ 346 public DocumentReferenceRelatesToComponent setTargetTarget(DocumentReference value) { 347 this.targetTarget = value; 348 return this; 349 } 350 351 protected void listChildren(List<Property> childrenList) { 352 super.listChildren(childrenList); 353 childrenList 354 .add(new Property("code", "code", "The type of relationship that this document has with anther document.", 0, 355 java.lang.Integer.MAX_VALUE, code)); 356 childrenList.add(new Property("target", "Reference(DocumentReference)", 357 "The target document of this relationship.", 0, java.lang.Integer.MAX_VALUE, target)); 358 } 359 360 @Override 361 public void setProperty(String name, Base value) throws FHIRException { 362 if (name.equals("code")) 363 this.code = new DocumentRelationshipTypeEnumFactory().fromType(value); // Enumeration<DocumentRelationshipType> 364 else if (name.equals("target")) 365 this.target = castToReference(value); // Reference 366 else 367 super.setProperty(name, value); 368 } 369 370 @Override 371 public Base addChild(String name) throws FHIRException { 372 if (name.equals("code")) { 373 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.code"); 374 } else if (name.equals("target")) { 375 this.target = new Reference(); 376 return this.target; 377 } else 378 return super.addChild(name); 379 } 380 381 public DocumentReferenceRelatesToComponent copy() { 382 DocumentReferenceRelatesToComponent dst = new DocumentReferenceRelatesToComponent(); 383 copyValues(dst); 384 dst.code = code == null ? null : code.copy(); 385 dst.target = target == null ? null : target.copy(); 386 return dst; 387 } 388 389 @Override 390 public boolean equalsDeep(Base other) { 391 if (!super.equalsDeep(other)) 392 return false; 393 if (!(other instanceof DocumentReferenceRelatesToComponent)) 394 return false; 395 DocumentReferenceRelatesToComponent o = (DocumentReferenceRelatesToComponent) other; 396 return compareDeep(code, o.code, true) && compareDeep(target, o.target, true); 397 } 398 399 @Override 400 public boolean equalsShallow(Base other) { 401 if (!super.equalsShallow(other)) 402 return false; 403 if (!(other instanceof DocumentReferenceRelatesToComponent)) 404 return false; 405 DocumentReferenceRelatesToComponent o = (DocumentReferenceRelatesToComponent) other; 406 return compareValues(code, o.code, true); 407 } 408 409 public boolean isEmpty() { 410 return super.isEmpty() && (code == null || code.isEmpty()) && (target == null || target.isEmpty()); 411 } 412 413 public String fhirType() { 414 return "DocumentReference.relatesTo"; 415 416 } 417 418 } 419 420 @Block() 421 public static class DocumentReferenceContentComponent extends BackboneElement implements IBaseBackboneElement { 422 /** 423 * The document or url of the document along with critical metadata to prove 424 * content has integrity. 425 */ 426 @Child(name = "attachment", type = { 427 Attachment.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 428 @Description(shortDefinition = "Where to access the document", formalDefinition = "The document or url of the document along with critical metadata to prove content has integrity.") 429 protected Attachment attachment; 430 431 /** 432 * An identifier of the document encoding, structure, and template that the 433 * document conforms to beyond the base format indicated in the mimeType. 434 */ 435 @Child(name = "format", type = { 436 Coding.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 437 @Description(shortDefinition = "Format/content rules for the document", formalDefinition = "An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.") 438 protected List<Coding> format; 439 440 private static final long serialVersionUID = -1412643085L; 441 442 /* 443 * Constructor 444 */ 445 public DocumentReferenceContentComponent() { 446 super(); 447 } 448 449 /* 450 * Constructor 451 */ 452 public DocumentReferenceContentComponent(Attachment attachment) { 453 super(); 454 this.attachment = attachment; 455 } 456 457 /** 458 * @return {@link #attachment} (The document or url of the document along with 459 * critical metadata to prove content has integrity.) 460 */ 461 public Attachment getAttachment() { 462 if (this.attachment == null) 463 if (Configuration.errorOnAutoCreate()) 464 throw new Error("Attempt to auto-create DocumentReferenceContentComponent.attachment"); 465 else if (Configuration.doAutoCreate()) 466 this.attachment = new Attachment(); // cc 467 return this.attachment; 468 } 469 470 public boolean hasAttachment() { 471 return this.attachment != null && !this.attachment.isEmpty(); 472 } 473 474 /** 475 * @param value {@link #attachment} (The document or url of the document along 476 * with critical metadata to prove content has integrity.) 477 */ 478 public DocumentReferenceContentComponent setAttachment(Attachment value) { 479 this.attachment = value; 480 return this; 481 } 482 483 /** 484 * @return {@link #format} (An identifier of the document encoding, structure, 485 * and template that the document conforms to beyond the base format 486 * indicated in the mimeType.) 487 */ 488 public List<Coding> getFormat() { 489 if (this.format == null) 490 this.format = new ArrayList<Coding>(); 491 return this.format; 492 } 493 494 public boolean hasFormat() { 495 if (this.format == null) 496 return false; 497 for (Coding item : this.format) 498 if (!item.isEmpty()) 499 return true; 500 return false; 501 } 502 503 /** 504 * @return {@link #format} (An identifier of the document encoding, structure, 505 * and template that the document conforms to beyond the base format 506 * indicated in the mimeType.) 507 */ 508 // syntactic sugar 509 public Coding addFormat() { // 3 510 Coding t = new Coding(); 511 if (this.format == null) 512 this.format = new ArrayList<Coding>(); 513 this.format.add(t); 514 return t; 515 } 516 517 // syntactic sugar 518 public DocumentReferenceContentComponent addFormat(Coding t) { // 3 519 if (t == null) 520 return this; 521 if (this.format == null) 522 this.format = new ArrayList<Coding>(); 523 this.format.add(t); 524 return this; 525 } 526 527 protected void listChildren(List<Property> childrenList) { 528 super.listChildren(childrenList); 529 childrenList.add(new Property("attachment", "Attachment", 530 "The document or url of the document along with critical metadata to prove content has integrity.", 0, 531 java.lang.Integer.MAX_VALUE, attachment)); 532 childrenList.add(new Property("format", "Coding", 533 "An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.", 534 0, java.lang.Integer.MAX_VALUE, format)); 535 } 536 537 @Override 538 public void setProperty(String name, Base value) throws FHIRException { 539 if (name.equals("attachment")) 540 this.attachment = castToAttachment(value); // Attachment 541 else if (name.equals("format")) 542 this.getFormat().add(castToCoding(value)); 543 else 544 super.setProperty(name, value); 545 } 546 547 @Override 548 public Base addChild(String name) throws FHIRException { 549 if (name.equals("attachment")) { 550 this.attachment = new Attachment(); 551 return this.attachment; 552 } else if (name.equals("format")) { 553 return addFormat(); 554 } else 555 return super.addChild(name); 556 } 557 558 public DocumentReferenceContentComponent copy() { 559 DocumentReferenceContentComponent dst = new DocumentReferenceContentComponent(); 560 copyValues(dst); 561 dst.attachment = attachment == null ? null : attachment.copy(); 562 if (format != null) { 563 dst.format = new ArrayList<Coding>(); 564 for (Coding i : format) 565 dst.format.add(i.copy()); 566 } 567 ; 568 return dst; 569 } 570 571 @Override 572 public boolean equalsDeep(Base other) { 573 if (!super.equalsDeep(other)) 574 return false; 575 if (!(other instanceof DocumentReferenceContentComponent)) 576 return false; 577 DocumentReferenceContentComponent o = (DocumentReferenceContentComponent) other; 578 return compareDeep(attachment, o.attachment, true) && compareDeep(format, o.format, true); 579 } 580 581 @Override 582 public boolean equalsShallow(Base other) { 583 if (!super.equalsShallow(other)) 584 return false; 585 if (!(other instanceof DocumentReferenceContentComponent)) 586 return false; 587 DocumentReferenceContentComponent o = (DocumentReferenceContentComponent) other; 588 return true; 589 } 590 591 public boolean isEmpty() { 592 return super.isEmpty() && (attachment == null || attachment.isEmpty()) && (format == null || format.isEmpty()); 593 } 594 595 public String fhirType() { 596 return "DocumentReference.content"; 597 598 } 599 600 } 601 602 @Block() 603 public static class DocumentReferenceContextComponent extends BackboneElement implements IBaseBackboneElement { 604 /** 605 * Describes the clinical encounter or type of care that the document content is 606 * associated with. 607 */ 608 @Child(name = "encounter", type = { 609 Encounter.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 610 @Description(shortDefinition = "Context of the document content", formalDefinition = "Describes the clinical encounter or type of care that the document content is associated with.") 611 protected Reference encounter; 612 613 /** 614 * The actual object that is the target of the reference (Describes the clinical 615 * encounter or type of care that the document content is associated with.) 616 */ 617 protected Encounter encounterTarget; 618 619 /** 620 * This list of codes represents the main clinical acts, such as a colonoscopy 621 * or an appendectomy, being documented. In some cases, the event is inherent in 622 * the typeCode, such as a "History and Physical Report" in which the procedure 623 * being documented is necessarily a "History and Physical" act. 624 */ 625 @Child(name = "event", type = { 626 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 627 @Description(shortDefinition = "Main Clinical Acts Documented", formalDefinition = "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.") 628 protected List<CodeableConcept> event; 629 630 /** 631 * The time period over which the service that is described by the document was 632 * provided. 633 */ 634 @Child(name = "period", type = { Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 635 @Description(shortDefinition = "Time of service that is being documented", formalDefinition = "The time period over which the service that is described by the document was provided.") 636 protected Period period; 637 638 /** 639 * The kind of facility where the patient was seen. 640 */ 641 @Child(name = "facilityType", type = { 642 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 643 @Description(shortDefinition = "Kind of facility where patient was seen", formalDefinition = "The kind of facility where the patient was seen.") 644 protected CodeableConcept facilityType; 645 646 /** 647 * This property may convey specifics about the practice setting where the 648 * content was created, often reflecting the clinical specialty. 649 */ 650 @Child(name = "practiceSetting", type = { 651 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 652 @Description(shortDefinition = "Additional details about where the content was created (e.g. clinical specialty)", formalDefinition = "This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.") 653 protected CodeableConcept practiceSetting; 654 655 /** 656 * The Patient Information as known when the document was published. May be a 657 * reference to a version specific, or contained. 658 */ 659 @Child(name = "sourcePatientInfo", type = { 660 Patient.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 661 @Description(shortDefinition = "Patient demographics from source", formalDefinition = "The Patient Information as known when the document was published. May be a reference to a version specific, or contained.") 662 protected Reference sourcePatientInfo; 663 664 /** 665 * The actual object that is the target of the reference (The Patient 666 * Information as known when the document was published. May be a reference to a 667 * version specific, or contained.) 668 */ 669 protected Patient sourcePatientInfoTarget; 670 671 /** 672 * Related identifiers or resources associated with the DocumentReference. 673 */ 674 @Child(name = "related", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 675 @Description(shortDefinition = "Related identifiers or resources", formalDefinition = "Related identifiers or resources associated with the DocumentReference.") 676 protected List<DocumentReferenceContextRelatedComponent> related; 677 678 private static final long serialVersionUID = 994799273L; 679 680 /* 681 * Constructor 682 */ 683 public DocumentReferenceContextComponent() { 684 super(); 685 } 686 687 /** 688 * @return {@link #encounter} (Describes the clinical encounter or type of care 689 * that the document content is associated with.) 690 */ 691 public Reference getEncounter() { 692 if (this.encounter == null) 693 if (Configuration.errorOnAutoCreate()) 694 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.encounter"); 695 else if (Configuration.doAutoCreate()) 696 this.encounter = new Reference(); // cc 697 return this.encounter; 698 } 699 700 public boolean hasEncounter() { 701 return this.encounter != null && !this.encounter.isEmpty(); 702 } 703 704 /** 705 * @param value {@link #encounter} (Describes the clinical encounter or type of 706 * care that the document content is associated with.) 707 */ 708 public DocumentReferenceContextComponent setEncounter(Reference value) { 709 this.encounter = value; 710 return this; 711 } 712 713 /** 714 * @return {@link #encounter} The actual object that is the target of the 715 * reference. The reference library doesn't populate this, but you can 716 * use it to hold the resource if you resolve it. (Describes the 717 * clinical encounter or type of care that the document content is 718 * associated with.) 719 */ 720 public Encounter getEncounterTarget() { 721 if (this.encounterTarget == null) 722 if (Configuration.errorOnAutoCreate()) 723 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.encounter"); 724 else if (Configuration.doAutoCreate()) 725 this.encounterTarget = new Encounter(); // aa 726 return this.encounterTarget; 727 } 728 729 /** 730 * @param value {@link #encounter} The actual object that is the target of the 731 * reference. The reference library doesn't use these, but you can 732 * use it to hold the resource if you resolve it. (Describes the 733 * clinical encounter or type of care that the document content is 734 * associated with.) 735 */ 736 public DocumentReferenceContextComponent setEncounterTarget(Encounter value) { 737 this.encounterTarget = value; 738 return this; 739 } 740 741 /** 742 * @return {@link #event} (This list of codes represents the main clinical acts, 743 * such as a colonoscopy or an appendectomy, being documented. In some 744 * cases, the event is inherent in the typeCode, such as a "History and 745 * Physical Report" in which the procedure being documented is 746 * necessarily a "History and Physical" act.) 747 */ 748 public List<CodeableConcept> getEvent() { 749 if (this.event == null) 750 this.event = new ArrayList<CodeableConcept>(); 751 return this.event; 752 } 753 754 public boolean hasEvent() { 755 if (this.event == null) 756 return false; 757 for (CodeableConcept item : this.event) 758 if (!item.isEmpty()) 759 return true; 760 return false; 761 } 762 763 /** 764 * @return {@link #event} (This list of codes represents the main clinical acts, 765 * such as a colonoscopy or an appendectomy, being documented. In some 766 * cases, the event is inherent in the typeCode, such as a "History and 767 * Physical Report" in which the procedure being documented is 768 * necessarily a "History and Physical" act.) 769 */ 770 // syntactic sugar 771 public CodeableConcept addEvent() { // 3 772 CodeableConcept t = new CodeableConcept(); 773 if (this.event == null) 774 this.event = new ArrayList<CodeableConcept>(); 775 this.event.add(t); 776 return t; 777 } 778 779 // syntactic sugar 780 public DocumentReferenceContextComponent addEvent(CodeableConcept t) { // 3 781 if (t == null) 782 return this; 783 if (this.event == null) 784 this.event = new ArrayList<CodeableConcept>(); 785 this.event.add(t); 786 return this; 787 } 788 789 /** 790 * @return {@link #period} (The time period over which the service that is 791 * described by the document was provided.) 792 */ 793 public Period getPeriod() { 794 if (this.period == null) 795 if (Configuration.errorOnAutoCreate()) 796 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.period"); 797 else if (Configuration.doAutoCreate()) 798 this.period = new Period(); // cc 799 return this.period; 800 } 801 802 public boolean hasPeriod() { 803 return this.period != null && !this.period.isEmpty(); 804 } 805 806 /** 807 * @param value {@link #period} (The time period over which the service that is 808 * described by the document was provided.) 809 */ 810 public DocumentReferenceContextComponent setPeriod(Period value) { 811 this.period = value; 812 return this; 813 } 814 815 /** 816 * @return {@link #facilityType} (The kind of facility where the patient was 817 * seen.) 818 */ 819 public CodeableConcept getFacilityType() { 820 if (this.facilityType == null) 821 if (Configuration.errorOnAutoCreate()) 822 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.facilityType"); 823 else if (Configuration.doAutoCreate()) 824 this.facilityType = new CodeableConcept(); // cc 825 return this.facilityType; 826 } 827 828 public boolean hasFacilityType() { 829 return this.facilityType != null && !this.facilityType.isEmpty(); 830 } 831 832 /** 833 * @param value {@link #facilityType} (The kind of facility where the patient 834 * was seen.) 835 */ 836 public DocumentReferenceContextComponent setFacilityType(CodeableConcept value) { 837 this.facilityType = value; 838 return this; 839 } 840 841 /** 842 * @return {@link #practiceSetting} (This property may convey specifics about 843 * the practice setting where the content was created, often reflecting 844 * the clinical specialty.) 845 */ 846 public CodeableConcept getPracticeSetting() { 847 if (this.practiceSetting == null) 848 if (Configuration.errorOnAutoCreate()) 849 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.practiceSetting"); 850 else if (Configuration.doAutoCreate()) 851 this.practiceSetting = new CodeableConcept(); // cc 852 return this.practiceSetting; 853 } 854 855 public boolean hasPracticeSetting() { 856 return this.practiceSetting != null && !this.practiceSetting.isEmpty(); 857 } 858 859 /** 860 * @param value {@link #practiceSetting} (This property may convey specifics 861 * about the practice setting where the content was created, often 862 * reflecting the clinical specialty.) 863 */ 864 public DocumentReferenceContextComponent setPracticeSetting(CodeableConcept value) { 865 this.practiceSetting = value; 866 return this; 867 } 868 869 /** 870 * @return {@link #sourcePatientInfo} (The Patient Information as known when the 871 * document was published. May be a reference to a version specific, or 872 * contained.) 873 */ 874 public Reference getSourcePatientInfo() { 875 if (this.sourcePatientInfo == null) 876 if (Configuration.errorOnAutoCreate()) 877 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.sourcePatientInfo"); 878 else if (Configuration.doAutoCreate()) 879 this.sourcePatientInfo = new Reference(); // cc 880 return this.sourcePatientInfo; 881 } 882 883 public boolean hasSourcePatientInfo() { 884 return this.sourcePatientInfo != null && !this.sourcePatientInfo.isEmpty(); 885 } 886 887 /** 888 * @param value {@link #sourcePatientInfo} (The Patient Information as known 889 * when the document was published. May be a reference to a version 890 * specific, or contained.) 891 */ 892 public DocumentReferenceContextComponent setSourcePatientInfo(Reference value) { 893 this.sourcePatientInfo = value; 894 return this; 895 } 896 897 /** 898 * @return {@link #sourcePatientInfo} The actual object that is the target of 899 * the reference. The reference library doesn't populate this, but you 900 * can use it to hold the resource if you resolve it. (The Patient 901 * Information as known when the document was published. May be a 902 * reference to a version specific, or contained.) 903 */ 904 public Patient getSourcePatientInfoTarget() { 905 if (this.sourcePatientInfoTarget == null) 906 if (Configuration.errorOnAutoCreate()) 907 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.sourcePatientInfo"); 908 else if (Configuration.doAutoCreate()) 909 this.sourcePatientInfoTarget = new Patient(); // aa 910 return this.sourcePatientInfoTarget; 911 } 912 913 /** 914 * @param value {@link #sourcePatientInfo} The actual object that is the target 915 * of the reference. The reference library doesn't use these, but 916 * you can use it to hold the resource if you resolve it. (The 917 * Patient Information as known when the document was published. 918 * May be a reference to a version specific, or contained.) 919 */ 920 public DocumentReferenceContextComponent setSourcePatientInfoTarget(Patient value) { 921 this.sourcePatientInfoTarget = value; 922 return this; 923 } 924 925 /** 926 * @return {@link #related} (Related identifiers or resources associated with 927 * the DocumentReference.) 928 */ 929 public List<DocumentReferenceContextRelatedComponent> getRelated() { 930 if (this.related == null) 931 this.related = new ArrayList<DocumentReferenceContextRelatedComponent>(); 932 return this.related; 933 } 934 935 public boolean hasRelated() { 936 if (this.related == null) 937 return false; 938 for (DocumentReferenceContextRelatedComponent item : this.related) 939 if (!item.isEmpty()) 940 return true; 941 return false; 942 } 943 944 /** 945 * @return {@link #related} (Related identifiers or resources associated with 946 * the DocumentReference.) 947 */ 948 // syntactic sugar 949 public DocumentReferenceContextRelatedComponent addRelated() { // 3 950 DocumentReferenceContextRelatedComponent t = new DocumentReferenceContextRelatedComponent(); 951 if (this.related == null) 952 this.related = new ArrayList<DocumentReferenceContextRelatedComponent>(); 953 this.related.add(t); 954 return t; 955 } 956 957 // syntactic sugar 958 public DocumentReferenceContextComponent addRelated(DocumentReferenceContextRelatedComponent t) { // 3 959 if (t == null) 960 return this; 961 if (this.related == null) 962 this.related = new ArrayList<DocumentReferenceContextRelatedComponent>(); 963 this.related.add(t); 964 return this; 965 } 966 967 protected void listChildren(List<Property> childrenList) { 968 super.listChildren(childrenList); 969 childrenList.add(new Property("encounter", "Reference(Encounter)", 970 "Describes the clinical encounter or type of care that the document content is associated with.", 0, 971 java.lang.Integer.MAX_VALUE, encounter)); 972 childrenList.add(new Property("event", "CodeableConcept", 973 "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 974 0, java.lang.Integer.MAX_VALUE, event)); 975 childrenList.add(new Property("period", "Period", 976 "The time period over which the service that is described by the document was provided.", 0, 977 java.lang.Integer.MAX_VALUE, period)); 978 childrenList.add(new Property("facilityType", "CodeableConcept", 979 "The kind of facility where the patient was seen.", 0, java.lang.Integer.MAX_VALUE, facilityType)); 980 childrenList.add(new Property("practiceSetting", "CodeableConcept", 981 "This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.", 982 0, java.lang.Integer.MAX_VALUE, practiceSetting)); 983 childrenList.add(new Property("sourcePatientInfo", "Reference(Patient)", 984 "The Patient Information as known when the document was published. May be a reference to a version specific, or contained.", 985 0, java.lang.Integer.MAX_VALUE, sourcePatientInfo)); 986 childrenList 987 .add(new Property("related", "", "Related identifiers or resources associated with the DocumentReference.", 0, 988 java.lang.Integer.MAX_VALUE, related)); 989 } 990 991 @Override 992 public void setProperty(String name, Base value) throws FHIRException { 993 if (name.equals("encounter")) 994 this.encounter = castToReference(value); // Reference 995 else if (name.equals("event")) 996 this.getEvent().add(castToCodeableConcept(value)); 997 else if (name.equals("period")) 998 this.period = castToPeriod(value); // Period 999 else if (name.equals("facilityType")) 1000 this.facilityType = castToCodeableConcept(value); // CodeableConcept 1001 else if (name.equals("practiceSetting")) 1002 this.practiceSetting = castToCodeableConcept(value); // CodeableConcept 1003 else if (name.equals("sourcePatientInfo")) 1004 this.sourcePatientInfo = castToReference(value); // Reference 1005 else if (name.equals("related")) 1006 this.getRelated().add((DocumentReferenceContextRelatedComponent) value); 1007 else 1008 super.setProperty(name, value); 1009 } 1010 1011 @Override 1012 public Base addChild(String name) throws FHIRException { 1013 if (name.equals("encounter")) { 1014 this.encounter = new Reference(); 1015 return this.encounter; 1016 } else if (name.equals("event")) { 1017 return addEvent(); 1018 } else if (name.equals("period")) { 1019 this.period = new Period(); 1020 return this.period; 1021 } else if (name.equals("facilityType")) { 1022 this.facilityType = new CodeableConcept(); 1023 return this.facilityType; 1024 } else if (name.equals("practiceSetting")) { 1025 this.practiceSetting = new CodeableConcept(); 1026 return this.practiceSetting; 1027 } else if (name.equals("sourcePatientInfo")) { 1028 this.sourcePatientInfo = new Reference(); 1029 return this.sourcePatientInfo; 1030 } else if (name.equals("related")) { 1031 return addRelated(); 1032 } else 1033 return super.addChild(name); 1034 } 1035 1036 public DocumentReferenceContextComponent copy() { 1037 DocumentReferenceContextComponent dst = new DocumentReferenceContextComponent(); 1038 copyValues(dst); 1039 dst.encounter = encounter == null ? null : encounter.copy(); 1040 if (event != null) { 1041 dst.event = new ArrayList<CodeableConcept>(); 1042 for (CodeableConcept i : event) 1043 dst.event.add(i.copy()); 1044 } 1045 ; 1046 dst.period = period == null ? null : period.copy(); 1047 dst.facilityType = facilityType == null ? null : facilityType.copy(); 1048 dst.practiceSetting = practiceSetting == null ? null : practiceSetting.copy(); 1049 dst.sourcePatientInfo = sourcePatientInfo == null ? null : sourcePatientInfo.copy(); 1050 if (related != null) { 1051 dst.related = new ArrayList<DocumentReferenceContextRelatedComponent>(); 1052 for (DocumentReferenceContextRelatedComponent i : related) 1053 dst.related.add(i.copy()); 1054 } 1055 ; 1056 return dst; 1057 } 1058 1059 @Override 1060 public boolean equalsDeep(Base other) { 1061 if (!super.equalsDeep(other)) 1062 return false; 1063 if (!(other instanceof DocumentReferenceContextComponent)) 1064 return false; 1065 DocumentReferenceContextComponent o = (DocumentReferenceContextComponent) other; 1066 return compareDeep(encounter, o.encounter, true) && compareDeep(event, o.event, true) 1067 && compareDeep(period, o.period, true) && compareDeep(facilityType, o.facilityType, true) 1068 && compareDeep(practiceSetting, o.practiceSetting, true) 1069 && compareDeep(sourcePatientInfo, o.sourcePatientInfo, true) && compareDeep(related, o.related, true); 1070 } 1071 1072 @Override 1073 public boolean equalsShallow(Base other) { 1074 if (!super.equalsShallow(other)) 1075 return false; 1076 if (!(other instanceof DocumentReferenceContextComponent)) 1077 return false; 1078 DocumentReferenceContextComponent o = (DocumentReferenceContextComponent) other; 1079 return true; 1080 } 1081 1082 public boolean isEmpty() { 1083 return super.isEmpty() && (encounter == null || encounter.isEmpty()) && (event == null || event.isEmpty()) 1084 && (period == null || period.isEmpty()) && (facilityType == null || facilityType.isEmpty()) 1085 && (practiceSetting == null || practiceSetting.isEmpty()) 1086 && (sourcePatientInfo == null || sourcePatientInfo.isEmpty()) && (related == null || related.isEmpty()); 1087 } 1088 1089 public String fhirType() { 1090 return "DocumentReference.context"; 1091 1092 } 1093 1094 } 1095 1096 @Block() 1097 public static class DocumentReferenceContextRelatedComponent extends BackboneElement implements IBaseBackboneElement { 1098 /** 1099 * Related identifier to this DocumentReference. If both id and ref are present 1100 * they shall refer to the same thing. 1101 */ 1102 @Child(name = "identifier", type = { 1103 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1104 @Description(shortDefinition = "Identifier of related objects or events", formalDefinition = "Related identifier to this DocumentReference. If both id and ref are present they shall refer to the same thing.") 1105 protected Identifier identifier; 1106 1107 /** 1108 * Related Resource to this DocumentReference. If both id and ref are present 1109 * they shall refer to the same thing. 1110 */ 1111 @Child(name = "ref", type = {}, order = 2, min = 0, max = 1, modifier = false, summary = true) 1112 @Description(shortDefinition = "Related Resource", formalDefinition = "Related Resource to this DocumentReference. If both id and ref are present they shall refer to the same thing.") 1113 protected Reference ref; 1114 1115 /** 1116 * The actual object that is the target of the reference (Related Resource to 1117 * this DocumentReference. If both id and ref are present they shall refer to 1118 * the same thing.) 1119 */ 1120 protected Resource refTarget; 1121 1122 private static final long serialVersionUID = -1670123330L; 1123 1124 /* 1125 * Constructor 1126 */ 1127 public DocumentReferenceContextRelatedComponent() { 1128 super(); 1129 } 1130 1131 /** 1132 * @return {@link #identifier} (Related identifier to this DocumentReference. If 1133 * both id and ref are present they shall refer to the same thing.) 1134 */ 1135 public Identifier getIdentifier() { 1136 if (this.identifier == null) 1137 if (Configuration.errorOnAutoCreate()) 1138 throw new Error("Attempt to auto-create DocumentReferenceContextRelatedComponent.identifier"); 1139 else if (Configuration.doAutoCreate()) 1140 this.identifier = new Identifier(); // cc 1141 return this.identifier; 1142 } 1143 1144 public boolean hasIdentifier() { 1145 return this.identifier != null && !this.identifier.isEmpty(); 1146 } 1147 1148 /** 1149 * @param value {@link #identifier} (Related identifier to this 1150 * DocumentReference. If both id and ref are present they shall 1151 * refer to the same thing.) 1152 */ 1153 public DocumentReferenceContextRelatedComponent setIdentifier(Identifier value) { 1154 this.identifier = value; 1155 return this; 1156 } 1157 1158 /** 1159 * @return {@link #ref} (Related Resource to this DocumentReference. If both id 1160 * and ref are present they shall refer to the same thing.) 1161 */ 1162 public Reference getRef() { 1163 if (this.ref == null) 1164 if (Configuration.errorOnAutoCreate()) 1165 throw new Error("Attempt to auto-create DocumentReferenceContextRelatedComponent.ref"); 1166 else if (Configuration.doAutoCreate()) 1167 this.ref = new Reference(); // cc 1168 return this.ref; 1169 } 1170 1171 public boolean hasRef() { 1172 return this.ref != null && !this.ref.isEmpty(); 1173 } 1174 1175 /** 1176 * @param value {@link #ref} (Related Resource to this DocumentReference. If 1177 * both id and ref are present they shall refer to the same thing.) 1178 */ 1179 public DocumentReferenceContextRelatedComponent setRef(Reference value) { 1180 this.ref = value; 1181 return this; 1182 } 1183 1184 /** 1185 * @return {@link #ref} The actual object that is the target of the reference. 1186 * The reference library doesn't populate this, but you can use it to 1187 * hold the resource if you resolve it. (Related Resource to this 1188 * DocumentReference. If both id and ref are present they shall refer to 1189 * the same thing.) 1190 */ 1191 public Resource getRefTarget() { 1192 return this.refTarget; 1193 } 1194 1195 /** 1196 * @param value {@link #ref} The actual object that is the target of the 1197 * reference. The reference library doesn't use these, but you can 1198 * use it to hold the resource if you resolve it. (Related Resource 1199 * to this DocumentReference. If both id and ref are present they 1200 * shall refer to the same thing.) 1201 */ 1202 public DocumentReferenceContextRelatedComponent setRefTarget(Resource value) { 1203 this.refTarget = value; 1204 return this; 1205 } 1206 1207 protected void listChildren(List<Property> childrenList) { 1208 super.listChildren(childrenList); 1209 childrenList.add(new Property("identifier", "Identifier", 1210 "Related identifier to this DocumentReference. If both id and ref are present they shall refer to the same thing.", 1211 0, java.lang.Integer.MAX_VALUE, identifier)); 1212 childrenList.add(new Property("ref", "Reference(Any)", 1213 "Related Resource to this DocumentReference. If both id and ref are present they shall refer to the same thing.", 1214 0, java.lang.Integer.MAX_VALUE, ref)); 1215 } 1216 1217 @Override 1218 public void setProperty(String name, Base value) throws FHIRException { 1219 if (name.equals("identifier")) 1220 this.identifier = castToIdentifier(value); // Identifier 1221 else if (name.equals("ref")) 1222 this.ref = castToReference(value); // Reference 1223 else 1224 super.setProperty(name, value); 1225 } 1226 1227 @Override 1228 public Base addChild(String name) throws FHIRException { 1229 if (name.equals("identifier")) { 1230 this.identifier = new Identifier(); 1231 return this.identifier; 1232 } else if (name.equals("ref")) { 1233 this.ref = new Reference(); 1234 return this.ref; 1235 } else 1236 return super.addChild(name); 1237 } 1238 1239 public DocumentReferenceContextRelatedComponent copy() { 1240 DocumentReferenceContextRelatedComponent dst = new DocumentReferenceContextRelatedComponent(); 1241 copyValues(dst); 1242 dst.identifier = identifier == null ? null : identifier.copy(); 1243 dst.ref = ref == null ? null : ref.copy(); 1244 return dst; 1245 } 1246 1247 @Override 1248 public boolean equalsDeep(Base other) { 1249 if (!super.equalsDeep(other)) 1250 return false; 1251 if (!(other instanceof DocumentReferenceContextRelatedComponent)) 1252 return false; 1253 DocumentReferenceContextRelatedComponent o = (DocumentReferenceContextRelatedComponent) other; 1254 return compareDeep(identifier, o.identifier, true) && compareDeep(ref, o.ref, true); 1255 } 1256 1257 @Override 1258 public boolean equalsShallow(Base other) { 1259 if (!super.equalsShallow(other)) 1260 return false; 1261 if (!(other instanceof DocumentReferenceContextRelatedComponent)) 1262 return false; 1263 DocumentReferenceContextRelatedComponent o = (DocumentReferenceContextRelatedComponent) other; 1264 return true; 1265 } 1266 1267 public boolean isEmpty() { 1268 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (ref == null || ref.isEmpty()); 1269 } 1270 1271 public String fhirType() { 1272 return "DocumentReference.context.related"; 1273 1274 } 1275 1276 } 1277 1278 /** 1279 * Document identifier as assigned by the source of the document. This 1280 * identifier is specific to this version of the document. This unique 1281 * identifier may be used elsewhere to identify this version of the document. 1282 */ 1283 @Child(name = "masterIdentifier", type = { 1284 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 1285 @Description(shortDefinition = "Master Version Specific Identifier", formalDefinition = "Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document.") 1286 protected Identifier masterIdentifier; 1287 1288 /** 1289 * Other identifiers associated with the document, including version independent 1290 * identifiers. 1291 */ 1292 @Child(name = "identifier", type = { 1293 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1294 @Description(shortDefinition = "Other identifiers for the document", formalDefinition = "Other identifiers associated with the document, including version independent identifiers.") 1295 protected List<Identifier> identifier; 1296 1297 /** 1298 * Who or what the document is about. The document can be about a person, 1299 * (patient or healthcare practitioner), a device (e.g. a machine) or even a 1300 * group of subjects (such as a document about a herd of farm animals, or a set 1301 * of patients that share a common exposure). 1302 */ 1303 @Child(name = "subject", type = { Patient.class, Practitioner.class, Group.class, 1304 Device.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1305 @Description(shortDefinition = "Who/what is the subject of the document", formalDefinition = "Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).") 1306 protected Reference subject; 1307 1308 /** 1309 * The actual object that is the target of the reference (Who or what the 1310 * document is about. The document can be about a person, (patient or healthcare 1311 * practitioner), a device (e.g. a machine) or even a group of subjects (such as 1312 * a document about a herd of farm animals, or a set of patients that share a 1313 * common exposure).) 1314 */ 1315 protected Resource subjectTarget; 1316 1317 /** 1318 * Specifies the particular kind of document referenced (e.g. History and 1319 * Physical, Discharge Summary, Progress Note). This usually equates to the 1320 * purpose of making the document referenced. 1321 */ 1322 @Child(name = "type", type = { CodeableConcept.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 1323 @Description(shortDefinition = "Kind of document (LOINC if possible)", formalDefinition = "Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.") 1324 protected CodeableConcept type; 1325 1326 /** 1327 * A categorization for the type of document referenced - helps for indexing and 1328 * searching. This may be implied by or derived from the code specified in the 1329 * DocumentReference.type. 1330 */ 1331 @Child(name = "class", type = { 1332 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1333 @Description(shortDefinition = "Categorization of document", formalDefinition = "A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.") 1334 protected CodeableConcept class_; 1335 1336 /** 1337 * Identifies who is responsible for adding the information to the document. 1338 */ 1339 @Child(name = "author", type = { Practitioner.class, Organization.class, Device.class, Patient.class, 1340 RelatedPerson.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1341 @Description(shortDefinition = "Who and/or what authored the document", formalDefinition = "Identifies who is responsible for adding the information to the document.") 1342 protected List<Reference> author; 1343 /** 1344 * The actual objects that are the target of the reference (Identifies who is 1345 * responsible for adding the information to the document.) 1346 */ 1347 protected List<Resource> authorTarget; 1348 1349 /** 1350 * Identifies the organization or group who is responsible for ongoing 1351 * maintenance of and access to the document. 1352 */ 1353 @Child(name = "custodian", type = { 1354 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1355 @Description(shortDefinition = "Organization which maintains the document", formalDefinition = "Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.") 1356 protected Reference custodian; 1357 1358 /** 1359 * The actual object that is the target of the reference (Identifies the 1360 * organization or group who is responsible for ongoing maintenance of and 1361 * access to the document.) 1362 */ 1363 protected Organization custodianTarget; 1364 1365 /** 1366 * Which person or organization authenticates that this document is valid. 1367 */ 1368 @Child(name = "authenticator", type = { Practitioner.class, 1369 Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1370 @Description(shortDefinition = "Who/what authenticated the document", formalDefinition = "Which person or organization authenticates that this document is valid.") 1371 protected Reference authenticator; 1372 1373 /** 1374 * The actual object that is the target of the reference (Which person or 1375 * organization authenticates that this document is valid.) 1376 */ 1377 protected Resource authenticatorTarget; 1378 1379 /** 1380 * When the document was created. 1381 */ 1382 @Child(name = "created", type = { DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1383 @Description(shortDefinition = "Document creation time", formalDefinition = "When the document was created.") 1384 protected DateTimeType created; 1385 1386 /** 1387 * When the document reference was created. 1388 */ 1389 @Child(name = "indexed", type = { InstantType.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 1390 @Description(shortDefinition = "When this document reference created", formalDefinition = "When the document reference was created.") 1391 protected InstantType indexed; 1392 1393 /** 1394 * The status of this document reference. 1395 */ 1396 @Child(name = "status", type = { CodeType.class }, order = 10, min = 1, max = 1, modifier = true, summary = true) 1397 @Description(shortDefinition = "current | superseded | entered-in-error", formalDefinition = "The status of this document reference.") 1398 protected Enumeration<DocumentReferenceStatus> status; 1399 1400 /** 1401 * The status of the underlying document. 1402 */ 1403 @Child(name = "docStatus", type = { 1404 CodeableConcept.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 1405 @Description(shortDefinition = "preliminary | final | appended | amended | entered-in-error", formalDefinition = "The status of the underlying document.") 1406 protected CodeableConcept docStatus; 1407 1408 /** 1409 * Relationships that this document has with other document references that 1410 * already exist. 1411 */ 1412 @Child(name = "relatesTo", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = true, summary = true) 1413 @Description(shortDefinition = "Relationships to other documents", formalDefinition = "Relationships that this document has with other document references that already exist.") 1414 protected List<DocumentReferenceRelatesToComponent> relatesTo; 1415 1416 /** 1417 * Human-readable description of the source document. This is sometimes known as 1418 * the "title". 1419 */ 1420 @Child(name = "description", type = { 1421 StringType.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 1422 @Description(shortDefinition = "Human-readable description (title)", formalDefinition = "Human-readable description of the source document. This is sometimes known as the \"title\".") 1423 protected StringType description; 1424 1425 /** 1426 * A set of Security-Tag codes specifying the level of privacy/security of the 1427 * Document. Note that DocumentReference.meta.security contains the security 1428 * labels of the "reference" to the document, while 1429 * DocumentReference.securityLabel contains a snapshot of the security labels on 1430 * the document the reference refers to. 1431 */ 1432 @Child(name = "securityLabel", type = { 1433 CodeableConcept.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1434 @Description(shortDefinition = "Document security-tags", formalDefinition = "A set of Security-Tag codes specifying the level of privacy/security of the Document. Note that DocumentReference.meta.security contains the security labels of the \"reference\" to the document, while DocumentReference.securityLabel contains a snapshot of the security labels on the document the reference refers to.") 1435 protected List<CodeableConcept> securityLabel; 1436 1437 /** 1438 * The document and format referenced. There may be multiple content element 1439 * repetitions, each with a different format. 1440 */ 1441 @Child(name = "content", type = {}, order = 15, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1442 @Description(shortDefinition = "Document referenced", formalDefinition = "The document and format referenced. There may be multiple content element repetitions, each with a different format.") 1443 protected List<DocumentReferenceContentComponent> content; 1444 1445 /** 1446 * The clinical context in which the document was prepared. 1447 */ 1448 @Child(name = "context", type = {}, order = 16, min = 0, max = 1, modifier = false, summary = true) 1449 @Description(shortDefinition = "Clinical context of document", formalDefinition = "The clinical context in which the document was prepared.") 1450 protected DocumentReferenceContextComponent context; 1451 1452 private static final long serialVersionUID = -1009325322L; 1453 1454 /* 1455 * Constructor 1456 */ 1457 public DocumentReference() { 1458 super(); 1459 } 1460 1461 /* 1462 * Constructor 1463 */ 1464 public DocumentReference(CodeableConcept type, InstantType indexed, Enumeration<DocumentReferenceStatus> status) { 1465 super(); 1466 this.type = type; 1467 this.indexed = indexed; 1468 this.status = status; 1469 } 1470 1471 /** 1472 * @return {@link #masterIdentifier} (Document identifier as assigned by the 1473 * source of the document. This identifier is specific to this version 1474 * of the document. This unique identifier may be used elsewhere to 1475 * identify this version of the document.) 1476 */ 1477 public Identifier getMasterIdentifier() { 1478 if (this.masterIdentifier == null) 1479 if (Configuration.errorOnAutoCreate()) 1480 throw new Error("Attempt to auto-create DocumentReference.masterIdentifier"); 1481 else if (Configuration.doAutoCreate()) 1482 this.masterIdentifier = new Identifier(); // cc 1483 return this.masterIdentifier; 1484 } 1485 1486 public boolean hasMasterIdentifier() { 1487 return this.masterIdentifier != null && !this.masterIdentifier.isEmpty(); 1488 } 1489 1490 /** 1491 * @param value {@link #masterIdentifier} (Document identifier as assigned by 1492 * the source of the document. This identifier is specific to this 1493 * version of the document. This unique identifier may be used 1494 * elsewhere to identify this version of the document.) 1495 */ 1496 public DocumentReference setMasterIdentifier(Identifier value) { 1497 this.masterIdentifier = value; 1498 return this; 1499 } 1500 1501 /** 1502 * @return {@link #identifier} (Other identifiers associated with the document, 1503 * including version independent identifiers.) 1504 */ 1505 public List<Identifier> getIdentifier() { 1506 if (this.identifier == null) 1507 this.identifier = new ArrayList<Identifier>(); 1508 return this.identifier; 1509 } 1510 1511 public boolean hasIdentifier() { 1512 if (this.identifier == null) 1513 return false; 1514 for (Identifier item : this.identifier) 1515 if (!item.isEmpty()) 1516 return true; 1517 return false; 1518 } 1519 1520 /** 1521 * @return {@link #identifier} (Other identifiers associated with the document, 1522 * including version independent identifiers.) 1523 */ 1524 // syntactic sugar 1525 public Identifier addIdentifier() { // 3 1526 Identifier t = new Identifier(); 1527 if (this.identifier == null) 1528 this.identifier = new ArrayList<Identifier>(); 1529 this.identifier.add(t); 1530 return t; 1531 } 1532 1533 // syntactic sugar 1534 public DocumentReference addIdentifier(Identifier t) { // 3 1535 if (t == null) 1536 return this; 1537 if (this.identifier == null) 1538 this.identifier = new ArrayList<Identifier>(); 1539 this.identifier.add(t); 1540 return this; 1541 } 1542 1543 /** 1544 * @return {@link #subject} (Who or what the document is about. The document can 1545 * be about a person, (patient or healthcare practitioner), a device 1546 * (e.g. a machine) or even a group of subjects (such as a document 1547 * about a herd of farm animals, or a set of patients that share a 1548 * common exposure).) 1549 */ 1550 public Reference getSubject() { 1551 if (this.subject == null) 1552 if (Configuration.errorOnAutoCreate()) 1553 throw new Error("Attempt to auto-create DocumentReference.subject"); 1554 else if (Configuration.doAutoCreate()) 1555 this.subject = new Reference(); // cc 1556 return this.subject; 1557 } 1558 1559 public boolean hasSubject() { 1560 return this.subject != null && !this.subject.isEmpty(); 1561 } 1562 1563 /** 1564 * @param value {@link #subject} (Who or what the document is about. The 1565 * document can be about a person, (patient or healthcare 1566 * practitioner), a device (e.g. a machine) or even a group of 1567 * subjects (such as a document about a herd of farm animals, or a 1568 * set of patients that share a common exposure).) 1569 */ 1570 public DocumentReference setSubject(Reference value) { 1571 this.subject = value; 1572 return this; 1573 } 1574 1575 /** 1576 * @return {@link #subject} The actual object that is the target of the 1577 * reference. The reference library doesn't populate this, but you can 1578 * use it to hold the resource if you resolve it. (Who or what the 1579 * document is about. The document can be about a person, (patient or 1580 * healthcare practitioner), a device (e.g. a machine) or even a group 1581 * of subjects (such as a document about a herd of farm animals, or a 1582 * set of patients that share a common exposure).) 1583 */ 1584 public Resource getSubjectTarget() { 1585 return this.subjectTarget; 1586 } 1587 1588 /** 1589 * @param value {@link #subject} The actual object that is the target of the 1590 * reference. The reference library doesn't use these, but you can 1591 * use it to hold the resource if you resolve it. (Who or what the 1592 * document is about. The document can be about a person, (patient 1593 * or healthcare practitioner), a device (e.g. a machine) or even a 1594 * group of subjects (such as a document about a herd of farm 1595 * animals, or a set of patients that share a common exposure).) 1596 */ 1597 public DocumentReference setSubjectTarget(Resource value) { 1598 this.subjectTarget = value; 1599 return this; 1600 } 1601 1602 /** 1603 * @return {@link #type} (Specifies the particular kind of document referenced 1604 * (e.g. History and Physical, Discharge Summary, Progress Note). This 1605 * usually equates to the purpose of making the document referenced.) 1606 */ 1607 public CodeableConcept getType() { 1608 if (this.type == null) 1609 if (Configuration.errorOnAutoCreate()) 1610 throw new Error("Attempt to auto-create DocumentReference.type"); 1611 else if (Configuration.doAutoCreate()) 1612 this.type = new CodeableConcept(); // cc 1613 return this.type; 1614 } 1615 1616 public boolean hasType() { 1617 return this.type != null && !this.type.isEmpty(); 1618 } 1619 1620 /** 1621 * @param value {@link #type} (Specifies the particular kind of document 1622 * referenced (e.g. History and Physical, Discharge Summary, 1623 * Progress Note). This usually equates to the purpose of making 1624 * the document referenced.) 1625 */ 1626 public DocumentReference setType(CodeableConcept value) { 1627 this.type = value; 1628 return this; 1629 } 1630 1631 /** 1632 * @return {@link #class_} (A categorization for the type of document referenced 1633 * - helps for indexing and searching. This may be implied by or derived 1634 * from the code specified in the DocumentReference.type.) 1635 */ 1636 public CodeableConcept getClass_() { 1637 if (this.class_ == null) 1638 if (Configuration.errorOnAutoCreate()) 1639 throw new Error("Attempt to auto-create DocumentReference.class_"); 1640 else if (Configuration.doAutoCreate()) 1641 this.class_ = new CodeableConcept(); // cc 1642 return this.class_; 1643 } 1644 1645 public boolean hasClass_() { 1646 return this.class_ != null && !this.class_.isEmpty(); 1647 } 1648 1649 /** 1650 * @param value {@link #class_} (A categorization for the type of document 1651 * referenced - helps for indexing and searching. This may be 1652 * implied by or derived from the code specified in the 1653 * DocumentReference.type.) 1654 */ 1655 public DocumentReference setClass_(CodeableConcept value) { 1656 this.class_ = value; 1657 return this; 1658 } 1659 1660 /** 1661 * @return {@link #author} (Identifies who is responsible for adding the 1662 * information to the document.) 1663 */ 1664 public List<Reference> getAuthor() { 1665 if (this.author == null) 1666 this.author = new ArrayList<Reference>(); 1667 return this.author; 1668 } 1669 1670 public boolean hasAuthor() { 1671 if (this.author == null) 1672 return false; 1673 for (Reference item : this.author) 1674 if (!item.isEmpty()) 1675 return true; 1676 return false; 1677 } 1678 1679 /** 1680 * @return {@link #author} (Identifies who is responsible for adding the 1681 * information to the document.) 1682 */ 1683 // syntactic sugar 1684 public Reference addAuthor() { // 3 1685 Reference t = new Reference(); 1686 if (this.author == null) 1687 this.author = new ArrayList<Reference>(); 1688 this.author.add(t); 1689 return t; 1690 } 1691 1692 // syntactic sugar 1693 public DocumentReference addAuthor(Reference t) { // 3 1694 if (t == null) 1695 return this; 1696 if (this.author == null) 1697 this.author = new ArrayList<Reference>(); 1698 this.author.add(t); 1699 return this; 1700 } 1701 1702 /** 1703 * @return {@link #author} (The actual objects that are the target of the 1704 * reference. The reference library doesn't populate this, but you can 1705 * use this to hold the resources if you resolvethemt. Identifies who is 1706 * responsible for adding the information to the document.) 1707 */ 1708 public List<Resource> getAuthorTarget() { 1709 if (this.authorTarget == null) 1710 this.authorTarget = new ArrayList<Resource>(); 1711 return this.authorTarget; 1712 } 1713 1714 /** 1715 * @return {@link #custodian} (Identifies the organization or group who is 1716 * responsible for ongoing maintenance of and access to the document.) 1717 */ 1718 public Reference getCustodian() { 1719 if (this.custodian == null) 1720 if (Configuration.errorOnAutoCreate()) 1721 throw new Error("Attempt to auto-create DocumentReference.custodian"); 1722 else if (Configuration.doAutoCreate()) 1723 this.custodian = new Reference(); // cc 1724 return this.custodian; 1725 } 1726 1727 public boolean hasCustodian() { 1728 return this.custodian != null && !this.custodian.isEmpty(); 1729 } 1730 1731 /** 1732 * @param value {@link #custodian} (Identifies the organization or group who is 1733 * responsible for ongoing maintenance of and access to the 1734 * document.) 1735 */ 1736 public DocumentReference setCustodian(Reference value) { 1737 this.custodian = value; 1738 return this; 1739 } 1740 1741 /** 1742 * @return {@link #custodian} The actual object that is the target of the 1743 * reference. The reference library doesn't populate this, but you can 1744 * use it to hold the resource if you resolve it. (Identifies the 1745 * organization or group who is responsible for ongoing maintenance of 1746 * and access to the document.) 1747 */ 1748 public Organization getCustodianTarget() { 1749 if (this.custodianTarget == null) 1750 if (Configuration.errorOnAutoCreate()) 1751 throw new Error("Attempt to auto-create DocumentReference.custodian"); 1752 else if (Configuration.doAutoCreate()) 1753 this.custodianTarget = new Organization(); // aa 1754 return this.custodianTarget; 1755 } 1756 1757 /** 1758 * @param value {@link #custodian} The actual object that is the target of the 1759 * reference. The reference library doesn't use these, but you can 1760 * use it to hold the resource if you resolve it. (Identifies the 1761 * organization or group who is responsible for ongoing maintenance 1762 * of and access to the document.) 1763 */ 1764 public DocumentReference setCustodianTarget(Organization value) { 1765 this.custodianTarget = value; 1766 return this; 1767 } 1768 1769 /** 1770 * @return {@link #authenticator} (Which person or organization authenticates 1771 * that this document is valid.) 1772 */ 1773 public Reference getAuthenticator() { 1774 if (this.authenticator == null) 1775 if (Configuration.errorOnAutoCreate()) 1776 throw new Error("Attempt to auto-create DocumentReference.authenticator"); 1777 else if (Configuration.doAutoCreate()) 1778 this.authenticator = new Reference(); // cc 1779 return this.authenticator; 1780 } 1781 1782 public boolean hasAuthenticator() { 1783 return this.authenticator != null && !this.authenticator.isEmpty(); 1784 } 1785 1786 /** 1787 * @param value {@link #authenticator} (Which person or organization 1788 * authenticates that this document is valid.) 1789 */ 1790 public DocumentReference setAuthenticator(Reference value) { 1791 this.authenticator = value; 1792 return this; 1793 } 1794 1795 /** 1796 * @return {@link #authenticator} The actual object that is the target of the 1797 * reference. The reference library doesn't populate this, but you can 1798 * use it to hold the resource if you resolve it. (Which person or 1799 * organization authenticates that this document is valid.) 1800 */ 1801 public Resource getAuthenticatorTarget() { 1802 return this.authenticatorTarget; 1803 } 1804 1805 /** 1806 * @param value {@link #authenticator} The actual object that is the target of 1807 * the reference. The reference library doesn't use these, but you 1808 * can use it to hold the resource if you resolve it. (Which person 1809 * or organization authenticates that this document is valid.) 1810 */ 1811 public DocumentReference setAuthenticatorTarget(Resource value) { 1812 this.authenticatorTarget = value; 1813 return this; 1814 } 1815 1816 /** 1817 * @return {@link #created} (When the document was created.). This is the 1818 * underlying object with id, value and extensions. The accessor 1819 * "getCreated" gives direct access to the value 1820 */ 1821 public DateTimeType getCreatedElement() { 1822 if (this.created == null) 1823 if (Configuration.errorOnAutoCreate()) 1824 throw new Error("Attempt to auto-create DocumentReference.created"); 1825 else if (Configuration.doAutoCreate()) 1826 this.created = new DateTimeType(); // bb 1827 return this.created; 1828 } 1829 1830 public boolean hasCreatedElement() { 1831 return this.created != null && !this.created.isEmpty(); 1832 } 1833 1834 public boolean hasCreated() { 1835 return this.created != null && !this.created.isEmpty(); 1836 } 1837 1838 /** 1839 * @param value {@link #created} (When the document was created.). This is the 1840 * underlying object with id, value and extensions. The accessor 1841 * "getCreated" gives direct access to the value 1842 */ 1843 public DocumentReference setCreatedElement(DateTimeType value) { 1844 this.created = value; 1845 return this; 1846 } 1847 1848 /** 1849 * @return When the document was created. 1850 */ 1851 public Date getCreated() { 1852 return this.created == null ? null : this.created.getValue(); 1853 } 1854 1855 /** 1856 * @param value When the document was created. 1857 */ 1858 public DocumentReference setCreated(Date value) { 1859 if (value == null) 1860 this.created = null; 1861 else { 1862 if (this.created == null) 1863 this.created = new DateTimeType(); 1864 this.created.setValue(value); 1865 } 1866 return this; 1867 } 1868 1869 /** 1870 * @return {@link #indexed} (When the document reference was created.). This is 1871 * the underlying object with id, value and extensions. The accessor 1872 * "getIndexed" gives direct access to the value 1873 */ 1874 public InstantType getIndexedElement() { 1875 if (this.indexed == null) 1876 if (Configuration.errorOnAutoCreate()) 1877 throw new Error("Attempt to auto-create DocumentReference.indexed"); 1878 else if (Configuration.doAutoCreate()) 1879 this.indexed = new InstantType(); // bb 1880 return this.indexed; 1881 } 1882 1883 public boolean hasIndexedElement() { 1884 return this.indexed != null && !this.indexed.isEmpty(); 1885 } 1886 1887 public boolean hasIndexed() { 1888 return this.indexed != null && !this.indexed.isEmpty(); 1889 } 1890 1891 /** 1892 * @param value {@link #indexed} (When the document reference was created.). 1893 * This is the underlying object with id, value and extensions. The 1894 * accessor "getIndexed" gives direct access to the value 1895 */ 1896 public DocumentReference setIndexedElement(InstantType value) { 1897 this.indexed = value; 1898 return this; 1899 } 1900 1901 /** 1902 * @return When the document reference was created. 1903 */ 1904 public Date getIndexed() { 1905 return this.indexed == null ? null : this.indexed.getValue(); 1906 } 1907 1908 /** 1909 * @param value When the document reference was created. 1910 */ 1911 public DocumentReference setIndexed(Date value) { 1912 if (this.indexed == null) 1913 this.indexed = new InstantType(); 1914 this.indexed.setValue(value); 1915 return this; 1916 } 1917 1918 /** 1919 * @return {@link #status} (The status of this document reference.). This is the 1920 * underlying object with id, value and extensions. The accessor 1921 * "getStatus" gives direct access to the value 1922 */ 1923 public Enumeration<DocumentReferenceStatus> getStatusElement() { 1924 if (this.status == null) 1925 if (Configuration.errorOnAutoCreate()) 1926 throw new Error("Attempt to auto-create DocumentReference.status"); 1927 else if (Configuration.doAutoCreate()) 1928 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); // bb 1929 return this.status; 1930 } 1931 1932 public boolean hasStatusElement() { 1933 return this.status != null && !this.status.isEmpty(); 1934 } 1935 1936 public boolean hasStatus() { 1937 return this.status != null && !this.status.isEmpty(); 1938 } 1939 1940 /** 1941 * @param value {@link #status} (The status of this document reference.). This 1942 * is the underlying object with id, value and extensions. The 1943 * accessor "getStatus" gives direct access to the value 1944 */ 1945 public DocumentReference setStatusElement(Enumeration<DocumentReferenceStatus> value) { 1946 this.status = value; 1947 return this; 1948 } 1949 1950 /** 1951 * @return The status of this document reference. 1952 */ 1953 public DocumentReferenceStatus getStatus() { 1954 return this.status == null ? null : this.status.getValue(); 1955 } 1956 1957 /** 1958 * @param value The status of this document reference. 1959 */ 1960 public DocumentReference setStatus(DocumentReferenceStatus value) { 1961 if (this.status == null) 1962 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); 1963 this.status.setValue(value); 1964 return this; 1965 } 1966 1967 /** 1968 * @return {@link #docStatus} (The status of the underlying document.) 1969 */ 1970 public CodeableConcept getDocStatus() { 1971 if (this.docStatus == null) 1972 if (Configuration.errorOnAutoCreate()) 1973 throw new Error("Attempt to auto-create DocumentReference.docStatus"); 1974 else if (Configuration.doAutoCreate()) 1975 this.docStatus = new CodeableConcept(); // cc 1976 return this.docStatus; 1977 } 1978 1979 public boolean hasDocStatus() { 1980 return this.docStatus != null && !this.docStatus.isEmpty(); 1981 } 1982 1983 /** 1984 * @param value {@link #docStatus} (The status of the underlying document.) 1985 */ 1986 public DocumentReference setDocStatus(CodeableConcept value) { 1987 this.docStatus = value; 1988 return this; 1989 } 1990 1991 /** 1992 * @return {@link #relatesTo} (Relationships that this document has with other 1993 * document references that already exist.) 1994 */ 1995 public List<DocumentReferenceRelatesToComponent> getRelatesTo() { 1996 if (this.relatesTo == null) 1997 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 1998 return this.relatesTo; 1999 } 2000 2001 public boolean hasRelatesTo() { 2002 if (this.relatesTo == null) 2003 return false; 2004 for (DocumentReferenceRelatesToComponent item : this.relatesTo) 2005 if (!item.isEmpty()) 2006 return true; 2007 return false; 2008 } 2009 2010 /** 2011 * @return {@link #relatesTo} (Relationships that this document has with other 2012 * document references that already exist.) 2013 */ 2014 // syntactic sugar 2015 public DocumentReferenceRelatesToComponent addRelatesTo() { // 3 2016 DocumentReferenceRelatesToComponent t = new DocumentReferenceRelatesToComponent(); 2017 if (this.relatesTo == null) 2018 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2019 this.relatesTo.add(t); 2020 return t; 2021 } 2022 2023 // syntactic sugar 2024 public DocumentReference addRelatesTo(DocumentReferenceRelatesToComponent t) { // 3 2025 if (t == null) 2026 return this; 2027 if (this.relatesTo == null) 2028 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2029 this.relatesTo.add(t); 2030 return this; 2031 } 2032 2033 /** 2034 * @return {@link #description} (Human-readable description of the source 2035 * document. This is sometimes known as the "title".). This is the 2036 * underlying object with id, value and extensions. The accessor 2037 * "getDescription" gives direct access to the value 2038 */ 2039 public StringType getDescriptionElement() { 2040 if (this.description == null) 2041 if (Configuration.errorOnAutoCreate()) 2042 throw new Error("Attempt to auto-create DocumentReference.description"); 2043 else if (Configuration.doAutoCreate()) 2044 this.description = new StringType(); // bb 2045 return this.description; 2046 } 2047 2048 public boolean hasDescriptionElement() { 2049 return this.description != null && !this.description.isEmpty(); 2050 } 2051 2052 public boolean hasDescription() { 2053 return this.description != null && !this.description.isEmpty(); 2054 } 2055 2056 /** 2057 * @param value {@link #description} (Human-readable description of the source 2058 * document. This is sometimes known as the "title".). This is the 2059 * underlying object with id, value and extensions. The accessor 2060 * "getDescription" gives direct access to the value 2061 */ 2062 public DocumentReference setDescriptionElement(StringType value) { 2063 this.description = value; 2064 return this; 2065 } 2066 2067 /** 2068 * @return Human-readable description of the source document. This is sometimes 2069 * known as the "title". 2070 */ 2071 public String getDescription() { 2072 return this.description == null ? null : this.description.getValue(); 2073 } 2074 2075 /** 2076 * @param value Human-readable description of the source document. This is 2077 * sometimes known as the "title". 2078 */ 2079 public DocumentReference setDescription(String value) { 2080 if (Utilities.noString(value)) 2081 this.description = null; 2082 else { 2083 if (this.description == null) 2084 this.description = new StringType(); 2085 this.description.setValue(value); 2086 } 2087 return this; 2088 } 2089 2090 /** 2091 * @return {@link #securityLabel} (A set of Security-Tag codes specifying the 2092 * level of privacy/security of the Document. Note that 2093 * DocumentReference.meta.security contains the security labels of the 2094 * "reference" to the document, while DocumentReference.securityLabel 2095 * contains a snapshot of the security labels on the document the 2096 * reference refers to.) 2097 */ 2098 public List<CodeableConcept> getSecurityLabel() { 2099 if (this.securityLabel == null) 2100 this.securityLabel = new ArrayList<CodeableConcept>(); 2101 return this.securityLabel; 2102 } 2103 2104 public boolean hasSecurityLabel() { 2105 if (this.securityLabel == null) 2106 return false; 2107 for (CodeableConcept item : this.securityLabel) 2108 if (!item.isEmpty()) 2109 return true; 2110 return false; 2111 } 2112 2113 /** 2114 * @return {@link #securityLabel} (A set of Security-Tag codes specifying the 2115 * level of privacy/security of the Document. Note that 2116 * DocumentReference.meta.security contains the security labels of the 2117 * "reference" to the document, while DocumentReference.securityLabel 2118 * contains a snapshot of the security labels on the document the 2119 * reference refers to.) 2120 */ 2121 // syntactic sugar 2122 public CodeableConcept addSecurityLabel() { // 3 2123 CodeableConcept t = new CodeableConcept(); 2124 if (this.securityLabel == null) 2125 this.securityLabel = new ArrayList<CodeableConcept>(); 2126 this.securityLabel.add(t); 2127 return t; 2128 } 2129 2130 // syntactic sugar 2131 public DocumentReference addSecurityLabel(CodeableConcept t) { // 3 2132 if (t == null) 2133 return this; 2134 if (this.securityLabel == null) 2135 this.securityLabel = new ArrayList<CodeableConcept>(); 2136 this.securityLabel.add(t); 2137 return this; 2138 } 2139 2140 /** 2141 * @return {@link #content} (The document and format referenced. There may be 2142 * multiple content element repetitions, each with a different format.) 2143 */ 2144 public List<DocumentReferenceContentComponent> getContent() { 2145 if (this.content == null) 2146 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2147 return this.content; 2148 } 2149 2150 public boolean hasContent() { 2151 if (this.content == null) 2152 return false; 2153 for (DocumentReferenceContentComponent item : this.content) 2154 if (!item.isEmpty()) 2155 return true; 2156 return false; 2157 } 2158 2159 /** 2160 * @return {@link #content} (The document and format referenced. There may be 2161 * multiple content element repetitions, each with a different format.) 2162 */ 2163 // syntactic sugar 2164 public DocumentReferenceContentComponent addContent() { // 3 2165 DocumentReferenceContentComponent t = new DocumentReferenceContentComponent(); 2166 if (this.content == null) 2167 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2168 this.content.add(t); 2169 return t; 2170 } 2171 2172 // syntactic sugar 2173 public DocumentReference addContent(DocumentReferenceContentComponent t) { // 3 2174 if (t == null) 2175 return this; 2176 if (this.content == null) 2177 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2178 this.content.add(t); 2179 return this; 2180 } 2181 2182 /** 2183 * @return {@link #context} (The clinical context in which the document was 2184 * prepared.) 2185 */ 2186 public DocumentReferenceContextComponent getContext() { 2187 if (this.context == null) 2188 if (Configuration.errorOnAutoCreate()) 2189 throw new Error("Attempt to auto-create DocumentReference.context"); 2190 else if (Configuration.doAutoCreate()) 2191 this.context = new DocumentReferenceContextComponent(); // cc 2192 return this.context; 2193 } 2194 2195 public boolean hasContext() { 2196 return this.context != null && !this.context.isEmpty(); 2197 } 2198 2199 /** 2200 * @param value {@link #context} (The clinical context in which the document was 2201 * prepared.) 2202 */ 2203 public DocumentReference setContext(DocumentReferenceContextComponent value) { 2204 this.context = value; 2205 return this; 2206 } 2207 2208 protected void listChildren(List<Property> childrenList) { 2209 super.listChildren(childrenList); 2210 childrenList.add(new Property("masterIdentifier", "Identifier", 2211 "Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document.", 2212 0, java.lang.Integer.MAX_VALUE, masterIdentifier)); 2213 childrenList.add(new Property("identifier", "Identifier", 2214 "Other identifiers associated with the document, including version independent identifiers.", 0, 2215 java.lang.Integer.MAX_VALUE, identifier)); 2216 childrenList.add(new Property("subject", "Reference(Patient|Practitioner|Group|Device)", 2217 "Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).", 2218 0, java.lang.Integer.MAX_VALUE, subject)); 2219 childrenList.add(new Property("type", "CodeableConcept", 2220 "Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.", 2221 0, java.lang.Integer.MAX_VALUE, type)); 2222 childrenList.add(new Property("class", "CodeableConcept", 2223 "A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.", 2224 0, java.lang.Integer.MAX_VALUE, class_)); 2225 childrenList.add(new Property("author", "Reference(Practitioner|Organization|Device|Patient|RelatedPerson)", 2226 "Identifies who is responsible for adding the information to the document.", 0, java.lang.Integer.MAX_VALUE, 2227 author)); 2228 childrenList.add(new Property("custodian", "Reference(Organization)", 2229 "Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.", 2230 0, java.lang.Integer.MAX_VALUE, custodian)); 2231 childrenList.add(new Property("authenticator", "Reference(Practitioner|Organization)", 2232 "Which person or organization authenticates that this document is valid.", 0, java.lang.Integer.MAX_VALUE, 2233 authenticator)); 2234 childrenList.add( 2235 new Property("created", "dateTime", "When the document was created.", 0, java.lang.Integer.MAX_VALUE, created)); 2236 childrenList.add(new Property("indexed", "instant", "When the document reference was created.", 0, 2237 java.lang.Integer.MAX_VALUE, indexed)); 2238 childrenList.add(new Property("status", "code", "The status of this document reference.", 0, 2239 java.lang.Integer.MAX_VALUE, status)); 2240 childrenList.add(new Property("docStatus", "CodeableConcept", "The status of the underlying document.", 0, 2241 java.lang.Integer.MAX_VALUE, docStatus)); 2242 childrenList.add(new Property("relatesTo", "", 2243 "Relationships that this document has with other document references that already exist.", 0, 2244 java.lang.Integer.MAX_VALUE, relatesTo)); 2245 childrenList.add(new Property("description", "string", 2246 "Human-readable description of the source document. This is sometimes known as the \"title\".", 0, 2247 java.lang.Integer.MAX_VALUE, description)); 2248 childrenList.add(new Property("securityLabel", "CodeableConcept", 2249 "A set of Security-Tag codes specifying the level of privacy/security of the Document. Note that DocumentReference.meta.security contains the security labels of the \"reference\" to the document, while DocumentReference.securityLabel contains a snapshot of the security labels on the document the reference refers to.", 2250 0, java.lang.Integer.MAX_VALUE, securityLabel)); 2251 childrenList.add(new Property("content", "", 2252 "The document and format referenced. There may be multiple content element repetitions, each with a different format.", 2253 0, java.lang.Integer.MAX_VALUE, content)); 2254 childrenList.add(new Property("context", "", "The clinical context in which the document was prepared.", 0, 2255 java.lang.Integer.MAX_VALUE, context)); 2256 } 2257 2258 @Override 2259 public void setProperty(String name, Base value) throws FHIRException { 2260 if (name.equals("masterIdentifier")) 2261 this.masterIdentifier = castToIdentifier(value); // Identifier 2262 else if (name.equals("identifier")) 2263 this.getIdentifier().add(castToIdentifier(value)); 2264 else if (name.equals("subject")) 2265 this.subject = castToReference(value); // Reference 2266 else if (name.equals("type")) 2267 this.type = castToCodeableConcept(value); // CodeableConcept 2268 else if (name.equals("class")) 2269 this.class_ = castToCodeableConcept(value); // CodeableConcept 2270 else if (name.equals("author")) 2271 this.getAuthor().add(castToReference(value)); 2272 else if (name.equals("custodian")) 2273 this.custodian = castToReference(value); // Reference 2274 else if (name.equals("authenticator")) 2275 this.authenticator = castToReference(value); // Reference 2276 else if (name.equals("created")) 2277 this.created = castToDateTime(value); // DateTimeType 2278 else if (name.equals("indexed")) 2279 this.indexed = castToInstant(value); // InstantType 2280 else if (name.equals("status")) 2281 this.status = new DocumentReferenceStatusEnumFactory().fromType(value); // Enumeration<DocumentReferenceStatus> 2282 else if (name.equals("docStatus")) 2283 this.docStatus = castToCodeableConcept(value); // CodeableConcept 2284 else if (name.equals("relatesTo")) 2285 this.getRelatesTo().add((DocumentReferenceRelatesToComponent) value); 2286 else if (name.equals("description")) 2287 this.description = castToString(value); // StringType 2288 else if (name.equals("securityLabel")) 2289 this.getSecurityLabel().add(castToCodeableConcept(value)); 2290 else if (name.equals("content")) 2291 this.getContent().add((DocumentReferenceContentComponent) value); 2292 else if (name.equals("context")) 2293 this.context = (DocumentReferenceContextComponent) value; // DocumentReferenceContextComponent 2294 else 2295 super.setProperty(name, value); 2296 } 2297 2298 @Override 2299 public Base addChild(String name) throws FHIRException { 2300 if (name.equals("masterIdentifier")) { 2301 this.masterIdentifier = new Identifier(); 2302 return this.masterIdentifier; 2303 } else if (name.equals("identifier")) { 2304 return addIdentifier(); 2305 } else if (name.equals("subject")) { 2306 this.subject = new Reference(); 2307 return this.subject; 2308 } else if (name.equals("type")) { 2309 this.type = new CodeableConcept(); 2310 return this.type; 2311 } else if (name.equals("class")) { 2312 this.class_ = new CodeableConcept(); 2313 return this.class_; 2314 } else if (name.equals("author")) { 2315 return addAuthor(); 2316 } else if (name.equals("custodian")) { 2317 this.custodian = new Reference(); 2318 return this.custodian; 2319 } else if (name.equals("authenticator")) { 2320 this.authenticator = new Reference(); 2321 return this.authenticator; 2322 } else if (name.equals("created")) { 2323 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.created"); 2324 } else if (name.equals("indexed")) { 2325 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.indexed"); 2326 } else if (name.equals("status")) { 2327 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.status"); 2328 } else if (name.equals("docStatus")) { 2329 this.docStatus = new CodeableConcept(); 2330 return this.docStatus; 2331 } else if (name.equals("relatesTo")) { 2332 return addRelatesTo(); 2333 } else if (name.equals("description")) { 2334 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.description"); 2335 } else if (name.equals("securityLabel")) { 2336 return addSecurityLabel(); 2337 } else if (name.equals("content")) { 2338 return addContent(); 2339 } else if (name.equals("context")) { 2340 this.context = new DocumentReferenceContextComponent(); 2341 return this.context; 2342 } else 2343 return super.addChild(name); 2344 } 2345 2346 public String fhirType() { 2347 return "DocumentReference"; 2348 2349 } 2350 2351 public DocumentReference copy() { 2352 DocumentReference dst = new DocumentReference(); 2353 copyValues(dst); 2354 dst.masterIdentifier = masterIdentifier == null ? null : masterIdentifier.copy(); 2355 if (identifier != null) { 2356 dst.identifier = new ArrayList<Identifier>(); 2357 for (Identifier i : identifier) 2358 dst.identifier.add(i.copy()); 2359 } 2360 ; 2361 dst.subject = subject == null ? null : subject.copy(); 2362 dst.type = type == null ? null : type.copy(); 2363 dst.class_ = class_ == null ? null : class_.copy(); 2364 if (author != null) { 2365 dst.author = new ArrayList<Reference>(); 2366 for (Reference i : author) 2367 dst.author.add(i.copy()); 2368 } 2369 ; 2370 dst.custodian = custodian == null ? null : custodian.copy(); 2371 dst.authenticator = authenticator == null ? null : authenticator.copy(); 2372 dst.created = created == null ? null : created.copy(); 2373 dst.indexed = indexed == null ? null : indexed.copy(); 2374 dst.status = status == null ? null : status.copy(); 2375 dst.docStatus = docStatus == null ? null : docStatus.copy(); 2376 if (relatesTo != null) { 2377 dst.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2378 for (DocumentReferenceRelatesToComponent i : relatesTo) 2379 dst.relatesTo.add(i.copy()); 2380 } 2381 ; 2382 dst.description = description == null ? null : description.copy(); 2383 if (securityLabel != null) { 2384 dst.securityLabel = new ArrayList<CodeableConcept>(); 2385 for (CodeableConcept i : securityLabel) 2386 dst.securityLabel.add(i.copy()); 2387 } 2388 ; 2389 if (content != null) { 2390 dst.content = new ArrayList<DocumentReferenceContentComponent>(); 2391 for (DocumentReferenceContentComponent i : content) 2392 dst.content.add(i.copy()); 2393 } 2394 ; 2395 dst.context = context == null ? null : context.copy(); 2396 return dst; 2397 } 2398 2399 protected DocumentReference typedCopy() { 2400 return copy(); 2401 } 2402 2403 @Override 2404 public boolean equalsDeep(Base other) { 2405 if (!super.equalsDeep(other)) 2406 return false; 2407 if (!(other instanceof DocumentReference)) 2408 return false; 2409 DocumentReference o = (DocumentReference) other; 2410 return compareDeep(masterIdentifier, o.masterIdentifier, true) && compareDeep(identifier, o.identifier, true) 2411 && compareDeep(subject, o.subject, true) && compareDeep(type, o.type, true) 2412 && compareDeep(class_, o.class_, true) && compareDeep(author, o.author, true) 2413 && compareDeep(custodian, o.custodian, true) && compareDeep(authenticator, o.authenticator, true) 2414 && compareDeep(created, o.created, true) && compareDeep(indexed, o.indexed, true) 2415 && compareDeep(status, o.status, true) && compareDeep(docStatus, o.docStatus, true) 2416 && compareDeep(relatesTo, o.relatesTo, true) && compareDeep(description, o.description, true) 2417 && compareDeep(securityLabel, o.securityLabel, true) && compareDeep(content, o.content, true) 2418 && compareDeep(context, o.context, true); 2419 } 2420 2421 @Override 2422 public boolean equalsShallow(Base other) { 2423 if (!super.equalsShallow(other)) 2424 return false; 2425 if (!(other instanceof DocumentReference)) 2426 return false; 2427 DocumentReference o = (DocumentReference) other; 2428 return compareValues(created, o.created, true) && compareValues(indexed, o.indexed, true) 2429 && compareValues(status, o.status, true) && compareValues(description, o.description, true); 2430 } 2431 2432 public boolean isEmpty() { 2433 return super.isEmpty() && (masterIdentifier == null || masterIdentifier.isEmpty()) 2434 && (identifier == null || identifier.isEmpty()) && (subject == null || subject.isEmpty()) 2435 && (type == null || type.isEmpty()) && (class_ == null || class_.isEmpty()) 2436 && (author == null || author.isEmpty()) && (custodian == null || custodian.isEmpty()) 2437 && (authenticator == null || authenticator.isEmpty()) && (created == null || created.isEmpty()) 2438 && (indexed == null || indexed.isEmpty()) && (status == null || status.isEmpty()) 2439 && (docStatus == null || docStatus.isEmpty()) && (relatesTo == null || relatesTo.isEmpty()) 2440 && (description == null || description.isEmpty()) && (securityLabel == null || securityLabel.isEmpty()) 2441 && (content == null || content.isEmpty()) && (context == null || context.isEmpty()); 2442 } 2443 2444 @Override 2445 public ResourceType getResourceType() { 2446 return ResourceType.DocumentReference; 2447 } 2448 2449 @SearchParamDefinition(name = "securitylabel", path = "DocumentReference.securityLabel", description = "Document security-tags", type = "token") 2450 public static final String SP_SECURITYLABEL = "securitylabel"; 2451 @SearchParamDefinition(name = "subject", path = "DocumentReference.subject", description = "Who/what is the subject of the document", type = "reference") 2452 public static final String SP_SUBJECT = "subject"; 2453 @SearchParamDefinition(name = "description", path = "DocumentReference.description", description = "Human-readable description (title)", type = "string") 2454 public static final String SP_DESCRIPTION = "description"; 2455 @SearchParamDefinition(name = "language", path = "DocumentReference.content.attachment.language", description = "Human language of the content (BCP-47)", type = "token") 2456 public static final String SP_LANGUAGE = "language"; 2457 @SearchParamDefinition(name = "type", path = "DocumentReference.type", description = "Kind of document (LOINC if possible)", type = "token") 2458 public static final String SP_TYPE = "type"; 2459 @SearchParamDefinition(name = "relation", path = "DocumentReference.relatesTo.code", description = "replaces | transforms | signs | appends", type = "token") 2460 public static final String SP_RELATION = "relation"; 2461 @SearchParamDefinition(name = "setting", path = "DocumentReference.context.practiceSetting", description = "Additional details about where the content was created (e.g. clinical specialty)", type = "token") 2462 public static final String SP_SETTING = "setting"; 2463 @SearchParamDefinition(name = "patient", path = "DocumentReference.subject", description = "Who/what is the subject of the document", type = "reference") 2464 public static final String SP_PATIENT = "patient"; 2465 @SearchParamDefinition(name = "relationship", path = "null", description = "Combination of relation and relatesTo", type = "composite") 2466 public static final String SP_RELATIONSHIP = "relationship"; 2467 @SearchParamDefinition(name = "event", path = "DocumentReference.context.event", description = "Main Clinical Acts Documented", type = "token") 2468 public static final String SP_EVENT = "event"; 2469 @SearchParamDefinition(name = "class", path = "DocumentReference.class", description = "Categorization of document", type = "token") 2470 public static final String SP_CLASS = "class"; 2471 @SearchParamDefinition(name = "authenticator", path = "DocumentReference.authenticator", description = "Who/what authenticated the document", type = "reference") 2472 public static final String SP_AUTHENTICATOR = "authenticator"; 2473 @SearchParamDefinition(name = "identifier", path = "DocumentReference.masterIdentifier | DocumentReference.identifier", description = "Master Version Specific Identifier", type = "token") 2474 public static final String SP_IDENTIFIER = "identifier"; 2475 @SearchParamDefinition(name = "period", path = "DocumentReference.context.period", description = "Time of service that is being documented", type = "date") 2476 public static final String SP_PERIOD = "period"; 2477 @SearchParamDefinition(name = "related-id", path = "DocumentReference.context.related.identifier", description = "Identifier of related objects or events", type = "token") 2478 public static final String SP_RELATEDID = "related-id"; 2479 @SearchParamDefinition(name = "custodian", path = "DocumentReference.custodian", description = "Organization which maintains the document", type = "reference") 2480 public static final String SP_CUSTODIAN = "custodian"; 2481 @SearchParamDefinition(name = "indexed", path = "DocumentReference.indexed", description = "When this document reference created", type = "date") 2482 public static final String SP_INDEXED = "indexed"; 2483 @SearchParamDefinition(name = "author", path = "DocumentReference.author", description = "Who and/or what authored the document", type = "reference") 2484 public static final String SP_AUTHOR = "author"; 2485 @SearchParamDefinition(name = "created", path = "DocumentReference.created", description = "Document creation time", type = "date") 2486 public static final String SP_CREATED = "created"; 2487 @SearchParamDefinition(name = "format", path = "DocumentReference.content.format", description = "Format/content rules for the document", type = "token") 2488 public static final String SP_FORMAT = "format"; 2489 @SearchParamDefinition(name = "encounter", path = "DocumentReference.context.encounter", description = "Context of the document content", type = "reference") 2490 public static final String SP_ENCOUNTER = "encounter"; 2491 @SearchParamDefinition(name = "related-ref", path = "DocumentReference.context.related.ref", description = "Related Resource", type = "reference") 2492 public static final String SP_RELATEDREF = "related-ref"; 2493 @SearchParamDefinition(name = "location", path = "DocumentReference.content.attachment.url", description = "Uri where the data can be found", type = "uri") 2494 public static final String SP_LOCATION = "location"; 2495 @SearchParamDefinition(name = "relatesto", path = "DocumentReference.relatesTo.target", description = "Target of the relationship", type = "reference") 2496 public static final String SP_RELATESTO = "relatesto"; 2497 @SearchParamDefinition(name = "facility", path = "DocumentReference.context.facilityType", description = "Kind of facility where patient was seen", type = "token") 2498 public static final String SP_FACILITY = "facility"; 2499 @SearchParamDefinition(name = "status", path = "DocumentReference.status", description = "current | superseded | entered-in-error", type = "token") 2500 public static final String SP_STATUS = "status"; 2501 2502}