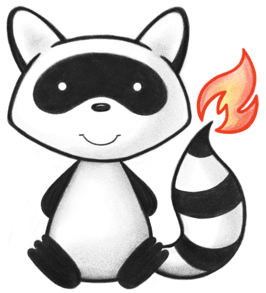
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Child; 037import ca.uhn.fhir.model.api.annotation.Description; 038import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 039import org.hl7.fhir.instance.model.api.IBaseHasModifierExtensions; 040import org.hl7.fhir.instance.model.api.IDomainResource; 041import org.hl7.fhir.exceptions.FHIRException; 042 043/** 044 * A resource that includes narrative, extensions, and contained resources. 045 */ 046public abstract class DomainResource extends Resource 047 implements IBaseHasExtensions, IBaseHasModifierExtensions, IDomainResource { 048 049 /** 050 * A human-readable narrative that contains a summary of the resource, and may 051 * be used to represent the content of the resource to a human. The narrative 052 * need not encode all the structured data, but is required to contain 053 * sufficient detail to make it "clinically safe" for a human to just read the 054 * narrative. Resource definitions may define what content should be represented 055 * in the narrative to ensure clinical safety. 056 */ 057 @Child(name = "text", type = { Narrative.class }, order = 0, min = 0, max = 1, modifier = false, summary = false) 058 @Description(shortDefinition = "Text summary of the resource, for human interpretation", formalDefinition = "A human-readable narrative that contains a summary of the resource, and may be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety.") 059 protected Narrative text; 060 061 /** 062 * These resources do not have an independent existence apart from the resource 063 * that contains them - they cannot be identified independently, and nor can 064 * they have their own independent transaction scope. 065 */ 066 @Child(name = "contained", type = { 067 Resource.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 068 @Description(shortDefinition = "Contained, inline Resources", formalDefinition = "These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, and nor can they have their own independent transaction scope.") 069 protected List<Resource> contained; 070 071 /** 072 * May be used to represent additional information that is not part of the basic 073 * definition of the resource. In order to make the use of extensions safe and 074 * manageable, there is a strict set of governance applied to the definition and 075 * use of extensions. Though any implementer is allowed to define an extension, 076 * there is a set of requirements that SHALL be met as part of the definition of 077 * the extension. 078 */ 079 @Child(name = "extension", type = { 080 Extension.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 081 @Description(shortDefinition = "Additional Content defined by implementations", formalDefinition = "May be used to represent additional information that is not part of the basic definition of the resource. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.") 082 protected List<Extension> extension; 083 084 /** 085 * May be used to represent additional information that is not part of the basic 086 * definition of the resource, and that modifies the understanding of the 087 * element that contains it. Usually modifier elements provide negation or 088 * qualification. In order to make the use of extensions safe and manageable, 089 * there is a strict set of governance applied to the definition and use of 090 * extensions. Though any implementer is allowed to define an extension, there 091 * is a set of requirements that SHALL be met as part of the definition of the 092 * extension. Applications processing a resource are required to check for 093 * modifier extensions. 094 */ 095 @Child(name = "modifierExtension", type = { 096 Extension.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = true, summary = false) 097 @Description(shortDefinition = "Extensions that cannot be ignored", formalDefinition = "May be used to represent additional information that is not part of the basic definition of the resource, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.") 098 protected List<Extension> modifierExtension; 099 100 private static final long serialVersionUID = -970285559L; 101 102 /* 103 * Constructor 104 */ 105 public DomainResource() { 106 super(); 107 } 108 109 /** 110 * @return {@link #text} (A human-readable narrative that contains a summary of 111 * the resource, and may be used to represent the content of the 112 * resource to a human. The narrative need not encode all the structured 113 * data, but is required to contain sufficient detail to make it 114 * "clinically safe" for a human to just read the narrative. Resource 115 * definitions may define what content should be represented in the 116 * narrative to ensure clinical safety.) 117 */ 118 public Narrative getText() { 119 if (this.text == null) 120 if (Configuration.errorOnAutoCreate()) 121 throw new Error("Attempt to auto-create DomainResource.text"); 122 else if (Configuration.doAutoCreate()) 123 this.text = new Narrative(); // cc 124 return this.text; 125 } 126 127 public boolean hasText() { 128 return this.text != null && !this.text.isEmpty(); 129 } 130 131 /** 132 * @param value {@link #text} (A human-readable narrative that contains a 133 * summary of the resource, and may be used to represent the 134 * content of the resource to a human. The narrative need not 135 * encode all the structured data, but is required to contain 136 * sufficient detail to make it "clinically safe" for a human to 137 * just read the narrative. Resource definitions may define what 138 * content should be represented in the narrative to ensure 139 * clinical safety.) 140 */ 141 public DomainResource setText(Narrative value) { 142 this.text = value; 143 return this; 144 } 145 146 /** 147 * @return {@link #contained} (These resources do not have an independent 148 * existence apart from the resource that contains them - they cannot be 149 * identified independently, and nor can they have their own independent 150 * transaction scope.) 151 */ 152 public List<Resource> getContained() { 153 if (this.contained == null) 154 this.contained = new ArrayList<Resource>(); 155 return this.contained; 156 } 157 158 public boolean hasContained() { 159 if (this.contained == null) 160 return false; 161 for (Resource item : this.contained) 162 if (!item.isEmpty()) 163 return true; 164 return false; 165 } 166 167 // syntactic sugar 168 public DomainResource addContained(Resource t) { // 3 169 if (t == null) 170 return this; 171 if (this.contained == null) 172 this.contained = new ArrayList<Resource>(); 173 this.contained.add(t); 174 return this; 175 } 176 177 /** 178 * @return {@link #contained} (These resources do not have an independent 179 * existence apart from the resource that contains them - they cannot be 180 * identified independently, and nor can they have their own independent 181 * transaction scope.) 182 */ 183 /** 184 * @return {@link #extension} (May be used to represent additional information 185 * that is not part of the basic definition of the resource. In order to 186 * make the use of extensions safe and manageable, there is a strict set 187 * of governance applied to the definition and use of extensions. Though 188 * any implementer is allowed to define an extension, there is a set of 189 * requirements that SHALL be met as part of the definition of the 190 * extension.) 191 */ 192 public List<Extension> getExtension() { 193 if (this.extension == null) 194 this.extension = new ArrayList<Extension>(); 195 return this.extension; 196 } 197 198 public boolean hasExtension() { 199 if (this.extension == null) 200 return false; 201 for (Extension item : this.extension) 202 if (!item.isEmpty()) 203 return true; 204 return false; 205 } 206 207 /** 208 * @return {@link #extension} (May be used to represent additional information 209 * that is not part of the basic definition of the resource. In order to 210 * make the use of extensions safe and manageable, there is a strict set 211 * of governance applied to the definition and use of extensions. Though 212 * any implementer is allowed to define an extension, there is a set of 213 * requirements that SHALL be met as part of the definition of the 214 * extension.) 215 */ 216 // syntactic sugar 217 public Extension addExtension() { // 3 218 Extension t = new Extension(); 219 if (this.extension == null) 220 this.extension = new ArrayList<Extension>(); 221 this.extension.add(t); 222 return t; 223 } 224 225 // syntactic sugar 226 public DomainResource addExtension(Extension t) { // 3 227 if (t == null) 228 return this; 229 if (this.extension == null) 230 this.extension = new ArrayList<Extension>(); 231 this.extension.add(t); 232 return this; 233 } 234 235 /** 236 * @return {@link #modifierExtension} (May be used to represent additional 237 * information that is not part of the basic definition of the resource, 238 * and that modifies the understanding of the element that contains it. 239 * Usually modifier elements provide negation or qualification. In order 240 * to make the use of extensions safe and manageable, there is a strict 241 * set of governance applied to the definition and use of extensions. 242 * Though any implementer is allowed to define an extension, there is a 243 * set of requirements that SHALL be met as part of the definition of 244 * the extension. Applications processing a resource are required to 245 * check for modifier extensions.) 246 */ 247 public List<Extension> getModifierExtension() { 248 if (this.modifierExtension == null) 249 this.modifierExtension = new ArrayList<Extension>(); 250 return this.modifierExtension; 251 } 252 253 public boolean hasModifierExtension() { 254 if (this.modifierExtension == null) 255 return false; 256 for (Extension item : this.modifierExtension) 257 if (!item.isEmpty()) 258 return true; 259 return false; 260 } 261 262 /** 263 * @return {@link #modifierExtension} (May be used to represent additional 264 * information that is not part of the basic definition of the resource, 265 * and that modifies the understanding of the element that contains it. 266 * Usually modifier elements provide negation or qualification. In order 267 * to make the use of extensions safe and manageable, there is a strict 268 * set of governance applied to the definition and use of extensions. 269 * Though any implementer is allowed to define an extension, there is a 270 * set of requirements that SHALL be met as part of the definition of 271 * the extension. Applications processing a resource are required to 272 * check for modifier extensions.) 273 */ 274 // syntactic sugar 275 public Extension addModifierExtension() { // 3 276 Extension t = new Extension(); 277 if (this.modifierExtension == null) 278 this.modifierExtension = new ArrayList<Extension>(); 279 this.modifierExtension.add(t); 280 return t; 281 } 282 283 // syntactic sugar 284 public DomainResource addModifierExtension(Extension t) { // 3 285 if (t == null) 286 return this; 287 if (this.modifierExtension == null) 288 this.modifierExtension = new ArrayList<Extension>(); 289 this.modifierExtension.add(t); 290 return this; 291 } 292 293 protected void listChildren(List<Property> childrenList) { 294 childrenList.add(new Property("text", "Narrative", 295 "A human-readable narrative that contains a summary of the resource, and may be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety.", 296 0, java.lang.Integer.MAX_VALUE, text)); 297 childrenList.add(new Property("contained", "Resource", 298 "These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, and nor can they have their own independent transaction scope.", 299 0, java.lang.Integer.MAX_VALUE, contained)); 300 childrenList.add(new Property("extension", "Extension", 301 "May be used to represent additional information that is not part of the basic definition of the resource. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 302 0, java.lang.Integer.MAX_VALUE, extension)); 303 childrenList.add(new Property("modifierExtension", "Extension", 304 "May be used to represent additional information that is not part of the basic definition of the resource, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.", 305 0, java.lang.Integer.MAX_VALUE, modifierExtension)); 306 } 307 308 @Override 309 public void setProperty(String name, Base value) throws FHIRException { 310 if (name.equals("text")) 311 this.text = castToNarrative(value); // Narrative 312 else if (name.equals("contained")) 313 this.getContained().add(castToResource(value)); 314 else if (name.equals("extension")) 315 this.getExtension().add(castToExtension(value)); 316 else if (name.equals("modifierExtension")) 317 this.getModifierExtension().add(castToExtension(value)); 318 else 319 super.setProperty(name, value); 320 } 321 322 @Override 323 public Base addChild(String name) throws FHIRException { 324 if (name.equals("text")) { 325 this.text = new Narrative(); 326 return this.text; 327 } else if (name.equals("contained")) { 328 throw new FHIRException("Cannot call addChild on an abstract type DomainResource.contained"); 329 } else if (name.equals("extension")) { 330 return addExtension(); 331 } else if (name.equals("modifierExtension")) { 332 return addModifierExtension(); 333 } else 334 return super.addChild(name); 335 } 336 337 public String fhirType() { 338 return "DomainResource"; 339 340 } 341 342 public abstract DomainResource copy(); 343 344 public void copyValues(DomainResource dst) { 345 super.copyValues(dst); 346 dst.text = text == null ? null : text.copy(); 347 if (contained != null) { 348 dst.contained = new ArrayList<Resource>(); 349 for (Resource i : contained) 350 dst.contained.add(i.copy()); 351 } 352 ; 353 if (extension != null) { 354 dst.extension = new ArrayList<Extension>(); 355 for (Extension i : extension) 356 dst.extension.add(i.copy()); 357 } 358 ; 359 if (modifierExtension != null) { 360 dst.modifierExtension = new ArrayList<Extension>(); 361 for (Extension i : modifierExtension) 362 dst.modifierExtension.add(i.copy()); 363 } 364 ; 365 } 366 367 @Override 368 public boolean equalsDeep(Base other) { 369 if (!super.equalsDeep(other)) 370 return false; 371 if (!(other instanceof DomainResource)) 372 return false; 373 DomainResource o = (DomainResource) other; 374 return compareDeep(text, o.text, true) && compareDeep(contained, o.contained, true) 375 && compareDeep(extension, o.extension, true) && compareDeep(modifierExtension, o.modifierExtension, true); 376 } 377 378 @Override 379 public boolean equalsShallow(Base other) { 380 if (!super.equalsShallow(other)) 381 return false; 382 if (!(other instanceof DomainResource)) 383 return false; 384 DomainResource o = (DomainResource) other; 385 return true; 386 } 387 388 public boolean isEmpty() { 389 return super.isEmpty() && (text == null || text.isEmpty()) && (contained == null || contained.isEmpty()) 390 && (extension == null || extension.isEmpty()) && (modifierExtension == null || modifierExtension.isEmpty()); 391 } 392 393 public boolean hasExtension(String url) { 394 for (Extension e : getExtension()) 395 if (url.equals(e.getUrl())) 396 return true; 397 return false; 398 } 399 400 public Extension getExtensionByUrl(String theUrl) { 401 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 402 ArrayList<Extension> retVal = new ArrayList<Extension>(); 403 for (Extension next : getExtension()) { 404 if (theUrl.equals(next.getUrl())) { 405 retVal.add(next); 406 } 407 } 408 if (retVal.size() == 0) 409 return null; 410 else { 411 org.apache.commons.lang3.Validate.isTrue(retVal.size() == 1, "Url " + theUrl + " must have only one match"); 412 return retVal.get(0); 413 } 414 } 415}