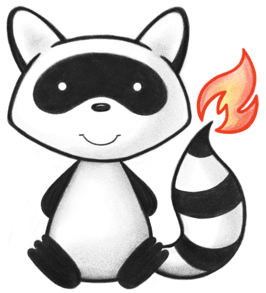
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Child; 037import ca.uhn.fhir.model.api.annotation.Description; 038import org.hl7.fhir.instance.model.api.IBaseElement; 039import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.utilities.Utilities; 042 043/** 044 * Base definition for all elements in a resource. 045 */ 046public abstract class Element extends Base implements IBaseHasExtensions, IBaseElement { 047 048 /** 049 * unique id for the element within a resource (for internal references). 050 */ 051 @Child(name = "id", type = { IdType.class }, order = 0, min = 0, max = 1, modifier = false, summary = false) 052 @Description(shortDefinition = "xml:id (or equivalent in JSON)", formalDefinition = "unique id for the element within a resource (for internal references).") 053 protected IdType id; 054 055 /** 056 * May be used to represent additional information that is not part of the basic 057 * definition of the element. In order to make the use of extensions safe and 058 * manageable, there is a strict set of governance applied to the definition and 059 * use of extensions. Though any implementer is allowed to define an extension, 060 * there is a set of requirements that SHALL be met as part of the definition of 061 * the extension. 062 */ 063 @Child(name = "extension", type = { 064 Extension.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 065 @Description(shortDefinition = "Additional Content defined by implementations", formalDefinition = "May be used to represent additional information that is not part of the basic definition of the element. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.") 066 protected List<Extension> extension; 067 068 private static final long serialVersionUID = -158027598L; 069 070 /* 071 * Constructor 072 */ 073 public Element() { 074 super(); 075 } 076 077 /** 078 * @return {@link #id} (unique id for the element within a resource (for 079 * internal references).). This is the underlying object with id, value 080 * and extensions. The accessor "getId" gives direct access to the value 081 */ 082 public IdType getIdElement() { 083 if (this.id == null) 084 if (Configuration.errorOnAutoCreate()) 085 throw new Error("Attempt to auto-create Element.id"); 086 else if (Configuration.doAutoCreate()) 087 this.id = new IdType(); // bb 088 return this.id; 089 } 090 091 public boolean hasIdElement() { 092 return this.id != null && !this.id.isEmpty(); 093 } 094 095 public boolean hasId() { 096 return this.id != null && !this.id.isEmpty(); 097 } 098 099 /** 100 * @param value {@link #id} (unique id for the element within a resource (for 101 * internal references).). This is the underlying object with id, 102 * value and extensions. The accessor "getId" gives direct access 103 * to the value 104 */ 105 public Element setIdElement(IdType value) { 106 this.id = value; 107 return this; 108 } 109 110 /** 111 * @return unique id for the element within a resource (for internal 112 * references). 113 */ 114 public String getId() { 115 return this.id == null ? null : this.id.getValue(); 116 } 117 118 /** 119 * @param value unique id for the element within a resource (for internal 120 * references). 121 */ 122 public Element setId(String value) { 123 if (Utilities.noString(value)) 124 this.id = null; 125 else { 126 if (this.id == null) 127 this.id = new IdType(); 128 this.id.setValue(value); 129 } 130 return this; 131 } 132 133 /** 134 * @return {@link #extension} (May be used to represent additional information 135 * that is not part of the basic definition of the element. In order to 136 * make the use of extensions safe and manageable, there is a strict set 137 * of governance applied to the definition and use of extensions. Though 138 * any implementer is allowed to define an extension, there is a set of 139 * requirements that SHALL be met as part of the definition of the 140 * extension.) 141 */ 142 public List<Extension> getExtension() { 143 if (this.extension == null) 144 this.extension = new ArrayList<Extension>(); 145 return this.extension; 146 } 147 148 public boolean hasExtension() { 149 if (this.extension == null) 150 return false; 151 for (Extension item : this.extension) 152 if (!item.isEmpty()) 153 return true; 154 return false; 155 } 156 157 /** 158 * @return {@link #extension} (May be used to represent additional information 159 * that is not part of the basic definition of the element. In order to 160 * make the use of extensions safe and manageable, there is a strict set 161 * of governance applied to the definition and use of extensions. Though 162 * any implementer is allowed to define an extension, there is a set of 163 * requirements that SHALL be met as part of the definition of the 164 * extension.) 165 */ 166 // syntactic sugar 167 public Extension addExtension() { // 3 168 Extension t = new Extension(); 169 if (this.extension == null) 170 this.extension = new ArrayList<Extension>(); 171 this.extension.add(t); 172 return t; 173 } 174 175 // syntactic sugar 176 public Element addExtension(Extension t) { // 3 177 if (t == null) 178 return this; 179 if (this.extension == null) 180 this.extension = new ArrayList<Extension>(); 181 this.extension.add(t); 182 return this; 183 } 184 185 /** 186 * Returns an unmodifiable list containing all extensions on this element which 187 * match the given URL. 188 * 189 * @param theUrl The URL. Must not be blank or null. 190 * @return an unmodifiable list containing all extensions on this element which 191 * match the given URL 192 */ 193 public List<Extension> getExtensionsByUrl(String theUrl) { 194 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 195 ArrayList<Extension> retVal = new ArrayList<Extension>(); 196 for (Extension next : getExtension()) { 197 if (theUrl.equals(next.getUrl())) { 198 retVal.add(next); 199 } 200 } 201 return java.util.Collections.unmodifiableList(retVal); 202 } 203 204 public Extension getExtensionByUrl(String theUrl) { 205 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 206 ArrayList<Extension> retVal = new ArrayList<Extension>(); 207 for (Extension next : getExtension()) { 208 if (theUrl.equals(next.getUrl())) { 209 retVal.add(next); 210 } 211 } 212 if (retVal.size() == 0) 213 return null; 214 else { 215 org.apache.commons.lang3.Validate.isTrue(retVal.size() == 1, "Url " + theUrl + " must have only one match"); 216 return retVal.get(0); 217 } 218 } 219 220 public void addExtension(String url, Type value) { 221 Extension ex = new Extension(); 222 ex.setUrl(url); 223 ex.setValue(value); 224 getExtension().add(ex); 225 } 226 227 public boolean hasExtension(String theUrl) { 228 return !getExtensionsByUrl(theUrl).isEmpty(); 229 } 230 231 public String getExtensionString(String theUrl) throws FHIRException { 232 List<Extension> ext = getExtensionsByUrl(theUrl); 233 if (ext.isEmpty()) 234 return null; 235 if (ext.size() > 1) 236 throw new FHIRException("Multiple matching extensions found"); 237 if (!ext.get(0).getValue().isPrimitive()) 238 throw new FHIRException("Extension could not be converted to a string"); 239 return ext.get(0).getValue().primitiveValue(); 240 } 241 242 protected void listChildren(List<Property> childrenList) { 243 childrenList.add(new Property("id", "id", "unique id for the element within a resource (for internal references).", 244 0, java.lang.Integer.MAX_VALUE, id)); 245 childrenList.add(new Property("extension", "Extension", 246 "May be used to represent additional information that is not part of the basic definition of the element. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 247 0, java.lang.Integer.MAX_VALUE, extension)); 248 } 249 250 @Override 251 public void setProperty(String name, Base value) throws FHIRException { 252 if (name.equals("id")) 253 this.id = castToId(value); // IdType 254 else if (name.equals("extension")) 255 this.getExtension().add(castToExtension(value)); 256 else 257 super.setProperty(name, value); 258 } 259 260 @Override 261 public Base addChild(String name) throws FHIRException { 262 if (name.equals("id")) { 263 throw new FHIRException("Cannot call addChild on a singleton property Element.id"); 264 } else if (name.equals("extension")) { 265 return addExtension(); 266 } else 267 return super.addChild(name); 268 } 269 270 public String fhirType() { 271 return "Element"; 272 273 } 274 275 public abstract Element copy(); 276 277 public void copyValues(Element dst) { 278 dst.id = id == null ? null : id.copy(); 279 if (extension != null) { 280 dst.extension = new ArrayList<Extension>(); 281 for (Extension i : extension) 282 dst.extension.add(i.copy()); 283 } 284 ; 285 } 286 287 @Override 288 public boolean equalsDeep(Base other) { 289 if (!super.equalsDeep(other)) 290 return false; 291 if (!(other instanceof Element)) 292 return false; 293 Element o = (Element) other; 294 return compareDeep(id, o.id, true) && compareDeep(extension, o.extension, true); 295 } 296 297 @Override 298 public boolean equalsShallow(Base other) { 299 if (!super.equalsShallow(other)) 300 return false; 301 if (!(other instanceof Element)) 302 return false; 303 Element o = (Element) other; 304 return compareValues(id, o.id, true); 305 } 306 307 public boolean isEmpty() { 308 return super.isEmpty() && (id == null || id.isEmpty()) && (extension == null || extension.isEmpty()); 309 } 310 311}