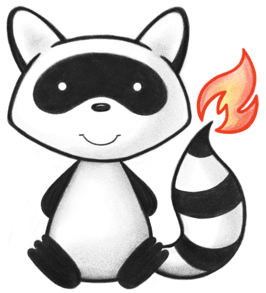
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.dstu2.model.Enumerations.BindingStrength; 037import org.hl7.fhir.dstu2.model.Enumerations.BindingStrengthEnumFactory; 038import ca.uhn.fhir.model.api.annotation.Block; 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.DatatypeDef; 041import ca.uhn.fhir.model.api.annotation.Description; 042import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 043import org.hl7.fhir.instance.model.api.ICompositeType; 044import org.hl7.fhir.exceptions.FHIRException; 045import org.hl7.fhir.utilities.Utilities; 046 047/** 048 * Captures constraints on each element within the resource, profile, or 049 * extension. 050 */ 051@DatatypeDef(name = "ElementDefinition") 052public class ElementDefinition extends Type implements ICompositeType { 053 054 public enum PropertyRepresentation { 055 /** 056 * In XML, this property is represented as an attribute not an element. 057 */ 058 XMLATTR, 059 /** 060 * added to help the parsers 061 */ 062 NULL; 063 064 public static PropertyRepresentation fromCode(String codeString) throws FHIRException { 065 if (codeString == null || "".equals(codeString)) 066 return null; 067 if ("xmlAttr".equals(codeString)) 068 return XMLATTR; 069 throw new FHIRException("Unknown PropertyRepresentation code '" + codeString + "'"); 070 } 071 072 public String toCode() { 073 switch (this) { 074 case XMLATTR: 075 return "xmlAttr"; 076 case NULL: 077 return null; 078 default: 079 return "?"; 080 } 081 } 082 083 public String getSystem() { 084 switch (this) { 085 case XMLATTR: 086 return "http://hl7.org/fhir/property-representation"; 087 case NULL: 088 return null; 089 default: 090 return "?"; 091 } 092 } 093 094 public String getDefinition() { 095 switch (this) { 096 case XMLATTR: 097 return "In XML, this property is represented as an attribute not an element."; 098 case NULL: 099 return null; 100 default: 101 return "?"; 102 } 103 } 104 105 public String getDisplay() { 106 switch (this) { 107 case XMLATTR: 108 return "XML Attribute"; 109 case NULL: 110 return null; 111 default: 112 return "?"; 113 } 114 } 115 } 116 117 public static class PropertyRepresentationEnumFactory implements EnumFactory<PropertyRepresentation> { 118 public PropertyRepresentation fromCode(String codeString) throws IllegalArgumentException { 119 if (codeString == null || "".equals(codeString)) 120 if (codeString == null || "".equals(codeString)) 121 return null; 122 if ("xmlAttr".equals(codeString)) 123 return PropertyRepresentation.XMLATTR; 124 throw new IllegalArgumentException("Unknown PropertyRepresentation code '" + codeString + "'"); 125 } 126 127 public Enumeration<PropertyRepresentation> fromType(Base code) throws FHIRException { 128 if (code == null || code.isEmpty()) 129 return null; 130 String codeString = ((PrimitiveType) code).asStringValue(); 131 if (codeString == null || "".equals(codeString)) 132 return null; 133 if ("xmlAttr".equals(codeString)) 134 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.XMLATTR); 135 throw new FHIRException("Unknown PropertyRepresentation code '" + codeString + "'"); 136 } 137 138 public String toCode(PropertyRepresentation code) 139 { 140 if (code == PropertyRepresentation.NULL) 141 return null; 142 if (code == PropertyRepresentation.XMLATTR) 143 return "xmlAttr"; 144 return "?"; 145 } 146 } 147 148 public enum SlicingRules { 149 /** 150 * No additional content is allowed other than that described by the slices in 151 * this profile. 152 */ 153 CLOSED, 154 /** 155 * Additional content is allowed anywhere in the list. 156 */ 157 OPEN, 158 /** 159 * Additional content is allowed, but only at the end of the list. Note that 160 * using this requires that the slices be ordered, which makes it hard to share 161 * uses. This should only be done where absolutely required. 162 */ 163 OPENATEND, 164 /** 165 * added to help the parsers 166 */ 167 NULL; 168 169 public static SlicingRules fromCode(String codeString) throws FHIRException { 170 if (codeString == null || "".equals(codeString)) 171 return null; 172 if ("closed".equals(codeString)) 173 return CLOSED; 174 if ("open".equals(codeString)) 175 return OPEN; 176 if ("openAtEnd".equals(codeString)) 177 return OPENATEND; 178 throw new FHIRException("Unknown SlicingRules code '" + codeString + "'"); 179 } 180 181 public String toCode() { 182 switch (this) { 183 case CLOSED: 184 return "closed"; 185 case OPEN: 186 return "open"; 187 case OPENATEND: 188 return "openAtEnd"; 189 case NULL: 190 return null; 191 default: 192 return "?"; 193 } 194 } 195 196 public String getSystem() { 197 switch (this) { 198 case CLOSED: 199 return "http://hl7.org/fhir/resource-slicing-rules"; 200 case OPEN: 201 return "http://hl7.org/fhir/resource-slicing-rules"; 202 case OPENATEND: 203 return "http://hl7.org/fhir/resource-slicing-rules"; 204 case NULL: 205 return null; 206 default: 207 return "?"; 208 } 209 } 210 211 public String getDefinition() { 212 switch (this) { 213 case CLOSED: 214 return "No additional content is allowed other than that described by the slices in this profile."; 215 case OPEN: 216 return "Additional content is allowed anywhere in the list."; 217 case OPENATEND: 218 return "Additional content is allowed, but only at the end of the list. Note that using this requires that the slices be ordered, which makes it hard to share uses. This should only be done where absolutely required."; 219 case NULL: 220 return null; 221 default: 222 return "?"; 223 } 224 } 225 226 public String getDisplay() { 227 switch (this) { 228 case CLOSED: 229 return "Closed"; 230 case OPEN: 231 return "Open"; 232 case OPENATEND: 233 return "Open at End"; 234 case NULL: 235 return null; 236 default: 237 return "?"; 238 } 239 } 240 } 241 242 public static class SlicingRulesEnumFactory implements EnumFactory<SlicingRules> { 243 public SlicingRules fromCode(String codeString) throws IllegalArgumentException { 244 if (codeString == null || "".equals(codeString)) 245 if (codeString == null || "".equals(codeString)) 246 return null; 247 if ("closed".equals(codeString)) 248 return SlicingRules.CLOSED; 249 if ("open".equals(codeString)) 250 return SlicingRules.OPEN; 251 if ("openAtEnd".equals(codeString)) 252 return SlicingRules.OPENATEND; 253 throw new IllegalArgumentException("Unknown SlicingRules code '" + codeString + "'"); 254 } 255 256 public Enumeration<SlicingRules> fromType(Base code) throws FHIRException { 257 if (code == null || code.isEmpty()) 258 return null; 259 String codeString = ((PrimitiveType) code).asStringValue(); 260 if (codeString == null || "".equals(codeString)) 261 return null; 262 if ("closed".equals(codeString)) 263 return new Enumeration<SlicingRules>(this, SlicingRules.CLOSED); 264 if ("open".equals(codeString)) 265 return new Enumeration<SlicingRules>(this, SlicingRules.OPEN); 266 if ("openAtEnd".equals(codeString)) 267 return new Enumeration<SlicingRules>(this, SlicingRules.OPENATEND); 268 throw new FHIRException("Unknown SlicingRules code '" + codeString + "'"); 269 } 270 271 public String toCode(SlicingRules code) 272 { 273 if (code == SlicingRules.NULL) 274 return null; 275 if (code == SlicingRules.CLOSED) 276 return "closed"; 277 if (code == SlicingRules.OPEN) 278 return "open"; 279 if (code == SlicingRules.OPENATEND) 280 return "openAtEnd"; 281 return "?"; 282 } 283 } 284 285 public enum AggregationMode { 286 /** 287 * The reference is a local reference to a contained resource. 288 */ 289 CONTAINED, 290 /** 291 * The reference to a resource that has to be resolved externally to the 292 * resource that includes the reference. 293 */ 294 REFERENCED, 295 /** 296 * The resource the reference points to will be found in the same bundle as the 297 * resource that includes the reference. 298 */ 299 BUNDLED, 300 /** 301 * added to help the parsers 302 */ 303 NULL; 304 305 public static AggregationMode fromCode(String codeString) throws FHIRException { 306 if (codeString == null || "".equals(codeString)) 307 return null; 308 if ("contained".equals(codeString)) 309 return CONTAINED; 310 if ("referenced".equals(codeString)) 311 return REFERENCED; 312 if ("bundled".equals(codeString)) 313 return BUNDLED; 314 throw new FHIRException("Unknown AggregationMode code '" + codeString + "'"); 315 } 316 317 public String toCode() { 318 switch (this) { 319 case CONTAINED: 320 return "contained"; 321 case REFERENCED: 322 return "referenced"; 323 case BUNDLED: 324 return "bundled"; 325 case NULL: 326 return null; 327 default: 328 return "?"; 329 } 330 } 331 332 public String getSystem() { 333 switch (this) { 334 case CONTAINED: 335 return "http://hl7.org/fhir/resource-aggregation-mode"; 336 case REFERENCED: 337 return "http://hl7.org/fhir/resource-aggregation-mode"; 338 case BUNDLED: 339 return "http://hl7.org/fhir/resource-aggregation-mode"; 340 case NULL: 341 return null; 342 default: 343 return "?"; 344 } 345 } 346 347 public String getDefinition() { 348 switch (this) { 349 case CONTAINED: 350 return "The reference is a local reference to a contained resource."; 351 case REFERENCED: 352 return "The reference to a resource that has to be resolved externally to the resource that includes the reference."; 353 case BUNDLED: 354 return "The resource the reference points to will be found in the same bundle as the resource that includes the reference."; 355 case NULL: 356 return null; 357 default: 358 return "?"; 359 } 360 } 361 362 public String getDisplay() { 363 switch (this) { 364 case CONTAINED: 365 return "Contained"; 366 case REFERENCED: 367 return "Referenced"; 368 case BUNDLED: 369 return "Bundled"; 370 case NULL: 371 return null; 372 default: 373 return "?"; 374 } 375 } 376 } 377 378 public static class AggregationModeEnumFactory implements EnumFactory<AggregationMode> { 379 public AggregationMode fromCode(String codeString) throws IllegalArgumentException { 380 if (codeString == null || "".equals(codeString)) 381 if (codeString == null || "".equals(codeString)) 382 return null; 383 if ("contained".equals(codeString)) 384 return AggregationMode.CONTAINED; 385 if ("referenced".equals(codeString)) 386 return AggregationMode.REFERENCED; 387 if ("bundled".equals(codeString)) 388 return AggregationMode.BUNDLED; 389 throw new IllegalArgumentException("Unknown AggregationMode code '" + codeString + "'"); 390 } 391 392 public Enumeration<AggregationMode> fromType(Base code) throws FHIRException { 393 if (code == null || code.isEmpty()) 394 return null; 395 String codeString = ((PrimitiveType) code).asStringValue(); 396 if (codeString == null || "".equals(codeString)) 397 return null; 398 if ("contained".equals(codeString)) 399 return new Enumeration<AggregationMode>(this, AggregationMode.CONTAINED); 400 if ("referenced".equals(codeString)) 401 return new Enumeration<AggregationMode>(this, AggregationMode.REFERENCED); 402 if ("bundled".equals(codeString)) 403 return new Enumeration<AggregationMode>(this, AggregationMode.BUNDLED); 404 throw new FHIRException("Unknown AggregationMode code '" + codeString + "'"); 405 } 406 407 public String toCode(AggregationMode code) 408 { 409 if (code == AggregationMode.NULL) 410 return null; 411 if (code == AggregationMode.CONTAINED) 412 return "contained"; 413 if (code == AggregationMode.REFERENCED) 414 return "referenced"; 415 if (code == AggregationMode.BUNDLED) 416 return "bundled"; 417 return "?"; 418 } 419 } 420 421 public enum ConstraintSeverity { 422 /** 423 * If the constraint is violated, the resource is not conformant. 424 */ 425 ERROR, 426 /** 427 * If the constraint is violated, the resource is conformant, but it is not 428 * necessarily following best practice. 429 */ 430 WARNING, 431 /** 432 * added to help the parsers 433 */ 434 NULL; 435 436 public static ConstraintSeverity fromCode(String codeString) throws FHIRException { 437 if (codeString == null || "".equals(codeString)) 438 return null; 439 if ("error".equals(codeString)) 440 return ERROR; 441 if ("warning".equals(codeString)) 442 return WARNING; 443 throw new FHIRException("Unknown ConstraintSeverity code '" + codeString + "'"); 444 } 445 446 public String toCode() { 447 switch (this) { 448 case ERROR: 449 return "error"; 450 case WARNING: 451 return "warning"; 452 case NULL: 453 return null; 454 default: 455 return "?"; 456 } 457 } 458 459 public String getSystem() { 460 switch (this) { 461 case ERROR: 462 return "http://hl7.org/fhir/constraint-severity"; 463 case WARNING: 464 return "http://hl7.org/fhir/constraint-severity"; 465 case NULL: 466 return null; 467 default: 468 return "?"; 469 } 470 } 471 472 public String getDefinition() { 473 switch (this) { 474 case ERROR: 475 return "If the constraint is violated, the resource is not conformant."; 476 case WARNING: 477 return "If the constraint is violated, the resource is conformant, but it is not necessarily following best practice."; 478 case NULL: 479 return null; 480 default: 481 return "?"; 482 } 483 } 484 485 public String getDisplay() { 486 switch (this) { 487 case ERROR: 488 return "Error"; 489 case WARNING: 490 return "Warning"; 491 case NULL: 492 return null; 493 default: 494 return "?"; 495 } 496 } 497 } 498 499 public static class ConstraintSeverityEnumFactory implements EnumFactory<ConstraintSeverity> { 500 public ConstraintSeverity fromCode(String codeString) throws IllegalArgumentException { 501 if (codeString == null || "".equals(codeString)) 502 if (codeString == null || "".equals(codeString)) 503 return null; 504 if ("error".equals(codeString)) 505 return ConstraintSeverity.ERROR; 506 if ("warning".equals(codeString)) 507 return ConstraintSeverity.WARNING; 508 throw new IllegalArgumentException("Unknown ConstraintSeverity code '" + codeString + "'"); 509 } 510 511 public Enumeration<ConstraintSeverity> fromType(Base code) throws FHIRException { 512 if (code == null || code.isEmpty()) 513 return null; 514 String codeString = ((PrimitiveType) code).asStringValue(); 515 if (codeString == null || "".equals(codeString)) 516 return null; 517 if ("error".equals(codeString)) 518 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.ERROR); 519 if ("warning".equals(codeString)) 520 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.WARNING); 521 throw new FHIRException("Unknown ConstraintSeverity code '" + codeString + "'"); 522 } 523 524 public String toCode(ConstraintSeverity code) 525 { 526 if (code == ConstraintSeverity.NULL) 527 return null; 528 if (code == ConstraintSeverity.ERROR) 529 return "error"; 530 if (code == ConstraintSeverity.WARNING) 531 return "warning"; 532 return "?"; 533 } 534 } 535 536 @Block() 537 public static class ElementDefinitionSlicingComponent extends Element implements IBaseDatatypeElement { 538 /** 539 * Designates which child elements are used to discriminate between the slices 540 * when processing an instance. If one or more discriminators are provided, the 541 * value of the child elements in the instance data SHALL completely distinguish 542 * which slice the element in the resource matches based on the allowed values 543 * for those elements in each of the slices. 544 */ 545 @Child(name = "discriminator", type = { 546 StringType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 547 @Description(shortDefinition = "Element values that used to distinguish the slices", formalDefinition = "Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.") 548 protected List<StringType> discriminator; 549 550 /** 551 * A human-readable text description of how the slicing works. If there is no 552 * discriminator, this is required to be present to provide whatever information 553 * is possible about how the slices can be differentiated. 554 */ 555 @Child(name = "description", type = { 556 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 557 @Description(shortDefinition = "Text description of how slicing works (or not)", formalDefinition = "A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.") 558 protected StringType description; 559 560 /** 561 * If the matching elements have to occur in the same order as defined in the 562 * profile. 563 */ 564 @Child(name = "ordered", type = { 565 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 566 @Description(shortDefinition = "If elements must be in same order as slices", formalDefinition = "If the matching elements have to occur in the same order as defined in the profile.") 567 protected BooleanType ordered; 568 569 /** 570 * Whether additional slices are allowed or not. When the slices are ordered, 571 * profile authors can also say that additional slices are only allowed at the 572 * end. 573 */ 574 @Child(name = "rules", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 575 @Description(shortDefinition = "closed | open | openAtEnd", formalDefinition = "Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.") 576 protected Enumeration<SlicingRules> rules; 577 578 private static final long serialVersionUID = 233544215L; 579 580 /* 581 * Constructor 582 */ 583 public ElementDefinitionSlicingComponent() { 584 super(); 585 } 586 587 /* 588 * Constructor 589 */ 590 public ElementDefinitionSlicingComponent(Enumeration<SlicingRules> rules) { 591 super(); 592 this.rules = rules; 593 } 594 595 /** 596 * @return {@link #discriminator} (Designates which child elements are used to 597 * discriminate between the slices when processing an instance. If one 598 * or more discriminators are provided, the value of the child elements 599 * in the instance data SHALL completely distinguish which slice the 600 * element in the resource matches based on the allowed values for those 601 * elements in each of the slices.) 602 */ 603 public List<StringType> getDiscriminator() { 604 if (this.discriminator == null) 605 this.discriminator = new ArrayList<StringType>(); 606 return this.discriminator; 607 } 608 609 public boolean hasDiscriminator() { 610 if (this.discriminator == null) 611 return false; 612 for (StringType item : this.discriminator) 613 if (!item.isEmpty()) 614 return true; 615 return false; 616 } 617 618 /** 619 * @return {@link #discriminator} (Designates which child elements are used to 620 * discriminate between the slices when processing an instance. If one 621 * or more discriminators are provided, the value of the child elements 622 * in the instance data SHALL completely distinguish which slice the 623 * element in the resource matches based on the allowed values for those 624 * elements in each of the slices.) 625 */ 626 // syntactic sugar 627 public StringType addDiscriminatorElement() {// 2 628 StringType t = new StringType(); 629 if (this.discriminator == null) 630 this.discriminator = new ArrayList<StringType>(); 631 this.discriminator.add(t); 632 return t; 633 } 634 635 /** 636 * @param value {@link #discriminator} (Designates which child elements are used 637 * to discriminate between the slices when processing an instance. 638 * If one or more discriminators are provided, the value of the 639 * child elements in the instance data SHALL completely distinguish 640 * which slice the element in the resource matches based on the 641 * allowed values for those elements in each of the slices.) 642 */ 643 public ElementDefinitionSlicingComponent addDiscriminator(String value) { // 1 644 StringType t = new StringType(); 645 t.setValue(value); 646 if (this.discriminator == null) 647 this.discriminator = new ArrayList<StringType>(); 648 this.discriminator.add(t); 649 return this; 650 } 651 652 /** 653 * @param value {@link #discriminator} (Designates which child elements are used 654 * to discriminate between the slices when processing an instance. 655 * If one or more discriminators are provided, the value of the 656 * child elements in the instance data SHALL completely distinguish 657 * which slice the element in the resource matches based on the 658 * allowed values for those elements in each of the slices.) 659 */ 660 public boolean hasDiscriminator(String value) { 661 if (this.discriminator == null) 662 return false; 663 for (StringType v : this.discriminator) 664 if (v.equals(value)) // string 665 return true; 666 return false; 667 } 668 669 /** 670 * @return {@link #description} (A human-readable text description of how the 671 * slicing works. If there is no discriminator, this is required to be 672 * present to provide whatever information is possible about how the 673 * slices can be differentiated.). This is the underlying object with 674 * id, value and extensions. The accessor "getDescription" gives direct 675 * access to the value 676 */ 677 public StringType getDescriptionElement() { 678 if (this.description == null) 679 if (Configuration.errorOnAutoCreate()) 680 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.description"); 681 else if (Configuration.doAutoCreate()) 682 this.description = new StringType(); // bb 683 return this.description; 684 } 685 686 public boolean hasDescriptionElement() { 687 return this.description != null && !this.description.isEmpty(); 688 } 689 690 public boolean hasDescription() { 691 return this.description != null && !this.description.isEmpty(); 692 } 693 694 /** 695 * @param value {@link #description} (A human-readable text description of how 696 * the slicing works. If there is no discriminator, this is 697 * required to be present to provide whatever information is 698 * possible about how the slices can be differentiated.). This is 699 * the underlying object with id, value and extensions. The 700 * accessor "getDescription" gives direct access to the value 701 */ 702 public ElementDefinitionSlicingComponent setDescriptionElement(StringType value) { 703 this.description = value; 704 return this; 705 } 706 707 /** 708 * @return A human-readable text description of how the slicing works. If there 709 * is no discriminator, this is required to be present to provide 710 * whatever information is possible about how the slices can be 711 * differentiated. 712 */ 713 public String getDescription() { 714 return this.description == null ? null : this.description.getValue(); 715 } 716 717 /** 718 * @param value A human-readable text description of how the slicing works. If 719 * there is no discriminator, this is required to be present to 720 * provide whatever information is possible about how the slices 721 * can be differentiated. 722 */ 723 public ElementDefinitionSlicingComponent setDescription(String value) { 724 if (Utilities.noString(value)) 725 this.description = null; 726 else { 727 if (this.description == null) 728 this.description = new StringType(); 729 this.description.setValue(value); 730 } 731 return this; 732 } 733 734 /** 735 * @return {@link #ordered} (If the matching elements have to occur in the same 736 * order as defined in the profile.). This is the underlying object with 737 * id, value and extensions. The accessor "getOrdered" gives direct 738 * access to the value 739 */ 740 public BooleanType getOrderedElement() { 741 if (this.ordered == null) 742 if (Configuration.errorOnAutoCreate()) 743 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.ordered"); 744 else if (Configuration.doAutoCreate()) 745 this.ordered = new BooleanType(); // bb 746 return this.ordered; 747 } 748 749 public boolean hasOrderedElement() { 750 return this.ordered != null && !this.ordered.isEmpty(); 751 } 752 753 public boolean hasOrdered() { 754 return this.ordered != null && !this.ordered.isEmpty(); 755 } 756 757 /** 758 * @param value {@link #ordered} (If the matching elements have to occur in the 759 * same order as defined in the profile.). This is the underlying 760 * object with id, value and extensions. The accessor "getOrdered" 761 * gives direct access to the value 762 */ 763 public ElementDefinitionSlicingComponent setOrderedElement(BooleanType value) { 764 this.ordered = value; 765 return this; 766 } 767 768 /** 769 * @return If the matching elements have to occur in the same order as defined 770 * in the profile. 771 */ 772 public boolean getOrdered() { 773 return this.ordered == null || this.ordered.isEmpty() ? false : this.ordered.getValue(); 774 } 775 776 /** 777 * @param value If the matching elements have to occur in the same order as 778 * defined in the profile. 779 */ 780 public ElementDefinitionSlicingComponent setOrdered(boolean value) { 781 if (this.ordered == null) 782 this.ordered = new BooleanType(); 783 this.ordered.setValue(value); 784 return this; 785 } 786 787 /** 788 * @return {@link #rules} (Whether additional slices are allowed or not. When 789 * the slices are ordered, profile authors can also say that additional 790 * slices are only allowed at the end.). This is the underlying object 791 * with id, value and extensions. The accessor "getRules" gives direct 792 * access to the value 793 */ 794 public Enumeration<SlicingRules> getRulesElement() { 795 if (this.rules == null) 796 if (Configuration.errorOnAutoCreate()) 797 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.rules"); 798 else if (Configuration.doAutoCreate()) 799 this.rules = new Enumeration<SlicingRules>(new SlicingRulesEnumFactory()); // bb 800 return this.rules; 801 } 802 803 public boolean hasRulesElement() { 804 return this.rules != null && !this.rules.isEmpty(); 805 } 806 807 public boolean hasRules() { 808 return this.rules != null && !this.rules.isEmpty(); 809 } 810 811 /** 812 * @param value {@link #rules} (Whether additional slices are allowed or not. 813 * When the slices are ordered, profile authors can also say that 814 * additional slices are only allowed at the end.). This is the 815 * underlying object with id, value and extensions. The accessor 816 * "getRules" gives direct access to the value 817 */ 818 public ElementDefinitionSlicingComponent setRulesElement(Enumeration<SlicingRules> value) { 819 this.rules = value; 820 return this; 821 } 822 823 /** 824 * @return Whether additional slices are allowed or not. When the slices are 825 * ordered, profile authors can also say that additional slices are only 826 * allowed at the end. 827 */ 828 public SlicingRules getRules() { 829 return this.rules == null ? null : this.rules.getValue(); 830 } 831 832 /** 833 * @param value Whether additional slices are allowed or not. When the slices 834 * are ordered, profile authors can also say that additional slices 835 * are only allowed at the end. 836 */ 837 public ElementDefinitionSlicingComponent setRules(SlicingRules value) { 838 if (this.rules == null) 839 this.rules = new Enumeration<SlicingRules>(new SlicingRulesEnumFactory()); 840 this.rules.setValue(value); 841 return this; 842 } 843 844 protected void listChildren(List<Property> childrenList) { 845 super.listChildren(childrenList); 846 childrenList.add(new Property("discriminator", "string", 847 "Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.", 848 0, java.lang.Integer.MAX_VALUE, discriminator)); 849 childrenList.add(new Property("description", "string", 850 "A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.", 851 0, java.lang.Integer.MAX_VALUE, description)); 852 childrenList.add(new Property("ordered", "boolean", 853 "If the matching elements have to occur in the same order as defined in the profile.", 0, 854 java.lang.Integer.MAX_VALUE, ordered)); 855 childrenList.add(new Property("rules", "code", 856 "Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.", 857 0, java.lang.Integer.MAX_VALUE, rules)); 858 } 859 860 @Override 861 public void setProperty(String name, Base value) throws FHIRException { 862 if (name.equals("discriminator")) 863 this.getDiscriminator().add(castToString(value)); 864 else if (name.equals("description")) 865 this.description = castToString(value); // StringType 866 else if (name.equals("ordered")) 867 this.ordered = castToBoolean(value); // BooleanType 868 else if (name.equals("rules")) 869 this.rules = new SlicingRulesEnumFactory().fromType(value); // Enumeration<SlicingRules> 870 else 871 super.setProperty(name, value); 872 } 873 874 @Override 875 public Base addChild(String name) throws FHIRException { 876 if (name.equals("discriminator")) { 877 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.discriminator"); 878 } else if (name.equals("description")) { 879 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.description"); 880 } else if (name.equals("ordered")) { 881 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.ordered"); 882 } else if (name.equals("rules")) { 883 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.rules"); 884 } else 885 return super.addChild(name); 886 } 887 888 public ElementDefinitionSlicingComponent copy() { 889 ElementDefinitionSlicingComponent dst = new ElementDefinitionSlicingComponent(); 890 copyValues(dst); 891 if (discriminator != null) { 892 dst.discriminator = new ArrayList<StringType>(); 893 for (StringType i : discriminator) 894 dst.discriminator.add(i.copy()); 895 } 896 ; 897 dst.description = description == null ? null : description.copy(); 898 dst.ordered = ordered == null ? null : ordered.copy(); 899 dst.rules = rules == null ? null : rules.copy(); 900 return dst; 901 } 902 903 @Override 904 public boolean equalsDeep(Base other) { 905 if (!super.equalsDeep(other)) 906 return false; 907 if (!(other instanceof ElementDefinitionSlicingComponent)) 908 return false; 909 ElementDefinitionSlicingComponent o = (ElementDefinitionSlicingComponent) other; 910 return compareDeep(discriminator, o.discriminator, true) && compareDeep(description, o.description, true) 911 && compareDeep(ordered, o.ordered, true) && compareDeep(rules, o.rules, true); 912 } 913 914 @Override 915 public boolean equalsShallow(Base other) { 916 if (!super.equalsShallow(other)) 917 return false; 918 if (!(other instanceof ElementDefinitionSlicingComponent)) 919 return false; 920 ElementDefinitionSlicingComponent o = (ElementDefinitionSlicingComponent) other; 921 return compareValues(discriminator, o.discriminator, true) && compareValues(description, o.description, true) 922 && compareValues(ordered, o.ordered, true) && compareValues(rules, o.rules, true); 923 } 924 925 public boolean isEmpty() { 926 return super.isEmpty() && (discriminator == null || discriminator.isEmpty()) 927 && (description == null || description.isEmpty()) && (ordered == null || ordered.isEmpty()) 928 && (rules == null || rules.isEmpty()); 929 } 930 931 public String fhirType() { 932 return "ElementDefinition.slicing"; 933 934 } 935 936 } 937 938 @Block() 939 public static class ElementDefinitionBaseComponent extends Element implements IBaseDatatypeElement { 940 /** 941 * The Path that identifies the base element - this matches the 942 * ElementDefinition.path for that element. Across FHIR, there is only one base 943 * definition of any element - that is, an element definition on a 944 * [[[StructureDefinition]]] without a StructureDefinition.base. 945 */ 946 @Child(name = "path", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 947 @Description(shortDefinition = "Path that identifies the base element", formalDefinition = "The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base.") 948 protected StringType path; 949 950 /** 951 * Minimum cardinality of the base element identified by the path. 952 */ 953 @Child(name = "min", type = { IntegerType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 954 @Description(shortDefinition = "Min cardinality of the base element", formalDefinition = "Minimum cardinality of the base element identified by the path.") 955 protected IntegerType min; 956 957 /** 958 * Maximum cardinality of the base element identified by the path. 959 */ 960 @Child(name = "max", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 961 @Description(shortDefinition = "Max cardinality of the base element", formalDefinition = "Maximum cardinality of the base element identified by the path.") 962 protected StringType max; 963 964 private static final long serialVersionUID = 232204455L; 965 966 /* 967 * Constructor 968 */ 969 public ElementDefinitionBaseComponent() { 970 super(); 971 } 972 973 /* 974 * Constructor 975 */ 976 public ElementDefinitionBaseComponent(StringType path, IntegerType min, StringType max) { 977 super(); 978 this.path = path; 979 this.min = min; 980 this.max = max; 981 } 982 983 /** 984 * @return {@link #path} (The Path that identifies the base element - this 985 * matches the ElementDefinition.path for that element. Across FHIR, 986 * there is only one base definition of any element - that is, an 987 * element definition on a [[[StructureDefinition]]] without a 988 * StructureDefinition.base.). This is the underlying object with id, 989 * value and extensions. The accessor "getPath" gives direct access to 990 * the value 991 */ 992 public StringType getPathElement() { 993 if (this.path == null) 994 if (Configuration.errorOnAutoCreate()) 995 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.path"); 996 else if (Configuration.doAutoCreate()) 997 this.path = new StringType(); // bb 998 return this.path; 999 } 1000 1001 public boolean hasPathElement() { 1002 return this.path != null && !this.path.isEmpty(); 1003 } 1004 1005 public boolean hasPath() { 1006 return this.path != null && !this.path.isEmpty(); 1007 } 1008 1009 /** 1010 * @param value {@link #path} (The Path that identifies the base element - this 1011 * matches the ElementDefinition.path for that element. Across 1012 * FHIR, there is only one base definition of any element - that 1013 * is, an element definition on a [[[StructureDefinition]]] without 1014 * a StructureDefinition.base.). This is the underlying object with 1015 * id, value and extensions. The accessor "getPath" gives direct 1016 * access to the value 1017 */ 1018 public ElementDefinitionBaseComponent setPathElement(StringType value) { 1019 this.path = value; 1020 return this; 1021 } 1022 1023 /** 1024 * @return The Path that identifies the base element - this matches the 1025 * ElementDefinition.path for that element. Across FHIR, there is only 1026 * one base definition of any element - that is, an element definition 1027 * on a [[[StructureDefinition]]] without a StructureDefinition.base. 1028 */ 1029 public String getPath() { 1030 return this.path == null ? null : this.path.getValue(); 1031 } 1032 1033 /** 1034 * @param value The Path that identifies the base element - this matches the 1035 * ElementDefinition.path for that element. Across FHIR, there is 1036 * only one base definition of any element - that is, an element 1037 * definition on a [[[StructureDefinition]]] without a 1038 * StructureDefinition.base. 1039 */ 1040 public ElementDefinitionBaseComponent setPath(String value) { 1041 if (this.path == null) 1042 this.path = new StringType(); 1043 this.path.setValue(value); 1044 return this; 1045 } 1046 1047 /** 1048 * @return {@link #min} (Minimum cardinality of the base element identified by 1049 * the path.). This is the underlying object with id, value and 1050 * extensions. The accessor "getMin" gives direct access to the value 1051 */ 1052 public IntegerType getMinElement() { 1053 if (this.min == null) 1054 if (Configuration.errorOnAutoCreate()) 1055 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.min"); 1056 else if (Configuration.doAutoCreate()) 1057 this.min = new IntegerType(); // bb 1058 return this.min; 1059 } 1060 1061 public boolean hasMinElement() { 1062 return this.min != null && !this.min.isEmpty(); 1063 } 1064 1065 public boolean hasMin() { 1066 return this.min != null && !this.min.isEmpty(); 1067 } 1068 1069 /** 1070 * @param value {@link #min} (Minimum cardinality of the base element identified 1071 * by the path.). This is the underlying object with id, value and 1072 * extensions. The accessor "getMin" gives direct access to the 1073 * value 1074 */ 1075 public ElementDefinitionBaseComponent setMinElement(IntegerType value) { 1076 this.min = value; 1077 return this; 1078 } 1079 1080 /** 1081 * @return Minimum cardinality of the base element identified by the path. 1082 */ 1083 public int getMin() { 1084 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 1085 } 1086 1087 /** 1088 * @param value Minimum cardinality of the base element identified by the path. 1089 */ 1090 public ElementDefinitionBaseComponent setMin(int value) { 1091 if (this.min == null) 1092 this.min = new IntegerType(); 1093 this.min.setValue(value); 1094 return this; 1095 } 1096 1097 /** 1098 * @return {@link #max} (Maximum cardinality of the base element identified by 1099 * the path.). This is the underlying object with id, value and 1100 * extensions. The accessor "getMax" gives direct access to the value 1101 */ 1102 public StringType getMaxElement() { 1103 if (this.max == null) 1104 if (Configuration.errorOnAutoCreate()) 1105 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.max"); 1106 else if (Configuration.doAutoCreate()) 1107 this.max = new StringType(); // bb 1108 return this.max; 1109 } 1110 1111 public boolean hasMaxElement() { 1112 return this.max != null && !this.max.isEmpty(); 1113 } 1114 1115 public boolean hasMax() { 1116 return this.max != null && !this.max.isEmpty(); 1117 } 1118 1119 /** 1120 * @param value {@link #max} (Maximum cardinality of the base element identified 1121 * by the path.). This is the underlying object with id, value and 1122 * extensions. The accessor "getMax" gives direct access to the 1123 * value 1124 */ 1125 public ElementDefinitionBaseComponent setMaxElement(StringType value) { 1126 this.max = value; 1127 return this; 1128 } 1129 1130 /** 1131 * @return Maximum cardinality of the base element identified by the path. 1132 */ 1133 public String getMax() { 1134 return this.max == null ? null : this.max.getValue(); 1135 } 1136 1137 /** 1138 * @param value Maximum cardinality of the base element identified by the path. 1139 */ 1140 public ElementDefinitionBaseComponent setMax(String value) { 1141 if (this.max == null) 1142 this.max = new StringType(); 1143 this.max.setValue(value); 1144 return this; 1145 } 1146 1147 protected void listChildren(List<Property> childrenList) { 1148 super.listChildren(childrenList); 1149 childrenList.add(new Property("path", "string", 1150 "The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base.", 1151 0, java.lang.Integer.MAX_VALUE, path)); 1152 childrenList.add(new Property("min", "integer", "Minimum cardinality of the base element identified by the path.", 1153 0, java.lang.Integer.MAX_VALUE, min)); 1154 childrenList.add(new Property("max", "string", "Maximum cardinality of the base element identified by the path.", 1155 0, java.lang.Integer.MAX_VALUE, max)); 1156 } 1157 1158 @Override 1159 public void setProperty(String name, Base value) throws FHIRException { 1160 if (name.equals("path")) 1161 this.path = castToString(value); // StringType 1162 else if (name.equals("min")) 1163 this.min = castToInteger(value); // IntegerType 1164 else if (name.equals("max")) 1165 this.max = castToString(value); // StringType 1166 else 1167 super.setProperty(name, value); 1168 } 1169 1170 @Override 1171 public Base addChild(String name) throws FHIRException { 1172 if (name.equals("path")) { 1173 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.path"); 1174 } else if (name.equals("min")) { 1175 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.min"); 1176 } else if (name.equals("max")) { 1177 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.max"); 1178 } else 1179 return super.addChild(name); 1180 } 1181 1182 public ElementDefinitionBaseComponent copy() { 1183 ElementDefinitionBaseComponent dst = new ElementDefinitionBaseComponent(); 1184 copyValues(dst); 1185 dst.path = path == null ? null : path.copy(); 1186 dst.min = min == null ? null : min.copy(); 1187 dst.max = max == null ? null : max.copy(); 1188 return dst; 1189 } 1190 1191 @Override 1192 public boolean equalsDeep(Base other) { 1193 if (!super.equalsDeep(other)) 1194 return false; 1195 if (!(other instanceof ElementDefinitionBaseComponent)) 1196 return false; 1197 ElementDefinitionBaseComponent o = (ElementDefinitionBaseComponent) other; 1198 return compareDeep(path, o.path, true) && compareDeep(min, o.min, true) && compareDeep(max, o.max, true); 1199 } 1200 1201 @Override 1202 public boolean equalsShallow(Base other) { 1203 if (!super.equalsShallow(other)) 1204 return false; 1205 if (!(other instanceof ElementDefinitionBaseComponent)) 1206 return false; 1207 ElementDefinitionBaseComponent o = (ElementDefinitionBaseComponent) other; 1208 return compareValues(path, o.path, true) && compareValues(min, o.min, true) && compareValues(max, o.max, true); 1209 } 1210 1211 public boolean isEmpty() { 1212 return super.isEmpty() && (path == null || path.isEmpty()) && (min == null || min.isEmpty()) 1213 && (max == null || max.isEmpty()); 1214 } 1215 1216 public String fhirType() { 1217 return "ElementDefinition.base"; 1218 1219 } 1220 1221 } 1222 1223 @Block() 1224 public static class TypeRefComponent extends Element implements IBaseDatatypeElement { 1225 /** 1226 * Name of Data type or Resource that is a(or the) type used for this element. 1227 */ 1228 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1229 @Description(shortDefinition = "Name of Data type or Resource", formalDefinition = "Name of Data type or Resource that is a(or the) type used for this element.") 1230 protected CodeType code; 1231 1232 /** 1233 * Identifies a profile structure or implementation Guide that SHALL hold for 1234 * resources or datatypes referenced as the type of this element. Can be a local 1235 * reference - to another structure in this profile, or a reference to a 1236 * structure in another profile. When more than one profile is specified, the 1237 * content must conform to all of them. When an implementation guide is 1238 * specified, the resource SHALL conform to at least one profile defined in the 1239 * implementation guide. 1240 */ 1241 @Child(name = "profile", type = { 1242 UriType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1243 @Description(shortDefinition = "Profile (StructureDefinition) to apply (or IG)", formalDefinition = "Identifies a profile structure or implementation Guide that SHALL hold for resources or datatypes referenced as the type of this element. Can be a local reference - to another structure in this profile, or a reference to a structure in another profile. When more than one profile is specified, the content must conform to all of them. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide.") 1244 protected List<UriType> profile; 1245 1246 /** 1247 * If the type is a reference to another resource, how the resource is or can be 1248 * aggregated - is it a contained resource, or a reference, and if the context 1249 * is a bundle, is it included in the bundle. 1250 */ 1251 @Child(name = "aggregation", type = { 1252 CodeType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1253 @Description(shortDefinition = "contained | referenced | bundled - how aggregated", formalDefinition = "If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.") 1254 protected List<Enumeration<AggregationMode>> aggregation; 1255 1256 private static final long serialVersionUID = -988693373L; 1257 1258 /* 1259 * Constructor 1260 */ 1261 public TypeRefComponent() { 1262 super(); 1263 } 1264 1265 /* 1266 * Constructor 1267 */ 1268 public TypeRefComponent(CodeType code) { 1269 super(); 1270 this.code = code; 1271 } 1272 1273 /** 1274 * @return {@link #code} (Name of Data type or Resource that is a(or the) type 1275 * used for this element.). This is the underlying object with id, value 1276 * and extensions. The accessor "getCode" gives direct access to the 1277 * value 1278 */ 1279 public CodeType getCodeElement() { 1280 if (this.code == null) 1281 if (Configuration.errorOnAutoCreate()) 1282 throw new Error("Attempt to auto-create TypeRefComponent.code"); 1283 else if (Configuration.doAutoCreate()) 1284 this.code = new CodeType(); // bb 1285 return this.code; 1286 } 1287 1288 public boolean hasCodeElement() { 1289 return this.code != null && !this.code.isEmpty(); 1290 } 1291 1292 public boolean hasCode() { 1293 return this.code != null && !this.code.isEmpty(); 1294 } 1295 1296 /** 1297 * @param value {@link #code} (Name of Data type or Resource that is a(or the) 1298 * type used for this element.). This is the underlying object with 1299 * id, value and extensions. The accessor "getCode" gives direct 1300 * access to the value 1301 */ 1302 public TypeRefComponent setCodeElement(CodeType value) { 1303 this.code = value; 1304 return this; 1305 } 1306 1307 /** 1308 * @return Name of Data type or Resource that is a(or the) type used for this 1309 * element. 1310 */ 1311 public String getCode() { 1312 return this.code == null ? null : this.code.getValue(); 1313 } 1314 1315 /** 1316 * @param value Name of Data type or Resource that is a(or the) type used for 1317 * this element. 1318 */ 1319 public TypeRefComponent setCode(String value) { 1320 if (this.code == null) 1321 this.code = new CodeType(); 1322 this.code.setValue(value); 1323 return this; 1324 } 1325 1326 /** 1327 * @return {@link #profile} (Identifies a profile structure or implementation 1328 * Guide that SHALL hold for resources or datatypes referenced as the 1329 * type of this element. Can be a local reference - to another structure 1330 * in this profile, or a reference to a structure in another profile. 1331 * When more than one profile is specified, the content must conform to 1332 * all of them. When an implementation guide is specified, the resource 1333 * SHALL conform to at least one profile defined in the implementation 1334 * guide.) 1335 */ 1336 public List<UriType> getProfile() { 1337 if (this.profile == null) 1338 this.profile = new ArrayList<UriType>(); 1339 return this.profile; 1340 } 1341 1342 public boolean hasProfile() { 1343 if (this.profile == null) 1344 return false; 1345 for (UriType item : this.profile) 1346 if (!item.isEmpty()) 1347 return true; 1348 return false; 1349 } 1350 1351 /** 1352 * @return {@link #profile} (Identifies a profile structure or implementation 1353 * Guide that SHALL hold for resources or datatypes referenced as the 1354 * type of this element. Can be a local reference - to another structure 1355 * in this profile, or a reference to a structure in another profile. 1356 * When more than one profile is specified, the content must conform to 1357 * all of them. When an implementation guide is specified, the resource 1358 * SHALL conform to at least one profile defined in the implementation 1359 * guide.) 1360 */ 1361 // syntactic sugar 1362 public UriType addProfileElement() {// 2 1363 UriType t = new UriType(); 1364 if (this.profile == null) 1365 this.profile = new ArrayList<UriType>(); 1366 this.profile.add(t); 1367 return t; 1368 } 1369 1370 /** 1371 * @param value {@link #profile} (Identifies a profile structure or 1372 * implementation Guide that SHALL hold for resources or datatypes 1373 * referenced as the type of this element. Can be a local reference 1374 * - to another structure in this profile, or a reference to a 1375 * structure in another profile. When more than one profile is 1376 * specified, the content must conform to all of them. When an 1377 * implementation guide is specified, the resource SHALL conform to 1378 * at least one profile defined in the implementation guide.) 1379 */ 1380 public TypeRefComponent addProfile(String value) { // 1 1381 UriType t = new UriType(); 1382 t.setValue(value); 1383 if (this.profile == null) 1384 this.profile = new ArrayList<UriType>(); 1385 this.profile.add(t); 1386 return this; 1387 } 1388 1389 /** 1390 * @param value {@link #profile} (Identifies a profile structure or 1391 * implementation Guide that SHALL hold for resources or datatypes 1392 * referenced as the type of this element. Can be a local reference 1393 * - to another structure in this profile, or a reference to a 1394 * structure in another profile. When more than one profile is 1395 * specified, the content must conform to all of them. When an 1396 * implementation guide is specified, the resource SHALL conform to 1397 * at least one profile defined in the implementation guide.) 1398 */ 1399 public boolean hasProfile(String value) { 1400 if (this.profile == null) 1401 return false; 1402 for (UriType v : this.profile) 1403 if (v.equals(value)) // uri 1404 return true; 1405 return false; 1406 } 1407 1408 /** 1409 * @return {@link #aggregation} (If the type is a reference to another resource, 1410 * how the resource is or can be aggregated - is it a contained 1411 * resource, or a reference, and if the context is a bundle, is it 1412 * included in the bundle.) 1413 */ 1414 public List<Enumeration<AggregationMode>> getAggregation() { 1415 if (this.aggregation == null) 1416 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 1417 return this.aggregation; 1418 } 1419 1420 public TypeRefComponent setAggregation(List<Enumeration<AggregationMode>> aggregation) { 1421 this.aggregation = aggregation; 1422 return this; 1423 } 1424 1425 public boolean hasAggregation() { 1426 if (this.aggregation == null) 1427 return false; 1428 for (Enumeration<AggregationMode> item : this.aggregation) 1429 if (!item.isEmpty()) 1430 return true; 1431 return false; 1432 } 1433 1434 /** 1435 * @return {@link #aggregation} (If the type is a reference to another resource, 1436 * how the resource is or can be aggregated - is it a contained 1437 * resource, or a reference, and if the context is a bundle, is it 1438 * included in the bundle.) 1439 */ 1440 // syntactic sugar 1441 public Enumeration<AggregationMode> addAggregationElement() {// 2 1442 Enumeration<AggregationMode> t = new Enumeration<AggregationMode>(new AggregationModeEnumFactory()); 1443 if (this.aggregation == null) 1444 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 1445 this.aggregation.add(t); 1446 return t; 1447 } 1448 1449 /** 1450 * @param value {@link #aggregation} (If the type is a reference to another 1451 * resource, how the resource is or can be aggregated - is it a 1452 * contained resource, or a reference, and if the context is a 1453 * bundle, is it included in the bundle.) 1454 */ 1455 public TypeRefComponent addAggregation(AggregationMode value) { // 1 1456 Enumeration<AggregationMode> t = new Enumeration<AggregationMode>(new AggregationModeEnumFactory()); 1457 t.setValue(value); 1458 if (this.aggregation == null) 1459 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 1460 this.aggregation.add(t); 1461 return this; 1462 } 1463 1464 /** 1465 * @param value {@link #aggregation} (If the type is a reference to another 1466 * resource, how the resource is or can be aggregated - is it a 1467 * contained resource, or a reference, and if the context is a 1468 * bundle, is it included in the bundle.) 1469 */ 1470 public boolean hasAggregation(AggregationMode value) { 1471 if (this.aggregation == null) 1472 return false; 1473 for (Enumeration<AggregationMode> v : this.aggregation) 1474 if (v.equals(value)) // code 1475 return true; 1476 return false; 1477 } 1478 1479 protected void listChildren(List<Property> childrenList) { 1480 super.listChildren(childrenList); 1481 childrenList.add( 1482 new Property("code", "code", "Name of Data type or Resource that is a(or the) type used for this element.", 0, 1483 java.lang.Integer.MAX_VALUE, code)); 1484 childrenList.add(new Property("profile", "uri", 1485 "Identifies a profile structure or implementation Guide that SHALL hold for resources or datatypes referenced as the type of this element. Can be a local reference - to another structure in this profile, or a reference to a structure in another profile. When more than one profile is specified, the content must conform to all of them. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide.", 1486 0, java.lang.Integer.MAX_VALUE, profile)); 1487 childrenList.add(new Property("aggregation", "code", 1488 "If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.", 1489 0, java.lang.Integer.MAX_VALUE, aggregation)); 1490 } 1491 1492 @Override 1493 public void setProperty(String name, Base value) throws FHIRException { 1494 if (name.equals("code")) 1495 this.code = castToCode(value); // CodeType 1496 else if (name.equals("profile")) 1497 this.getProfile().add(castToUri(value)); 1498 else if (name.equals("aggregation")) 1499 this.getAggregation().add(new AggregationModeEnumFactory().fromType(value)); 1500 else 1501 super.setProperty(name, value); 1502 } 1503 1504 @Override 1505 public Base addChild(String name) throws FHIRException { 1506 if (name.equals("code")) { 1507 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.code"); 1508 } else if (name.equals("profile")) { 1509 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.profile"); 1510 } else if (name.equals("aggregation")) { 1511 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.aggregation"); 1512 } else 1513 return super.addChild(name); 1514 } 1515 1516 public TypeRefComponent copy() { 1517 TypeRefComponent dst = new TypeRefComponent(); 1518 copyValues(dst); 1519 dst.code = code == null ? null : code.copy(); 1520 if (profile != null) { 1521 dst.profile = new ArrayList<UriType>(); 1522 for (UriType i : profile) 1523 dst.profile.add(i.copy()); 1524 } 1525 ; 1526 if (aggregation != null) { 1527 dst.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 1528 for (Enumeration<AggregationMode> i : aggregation) 1529 dst.aggregation.add(i.copy()); 1530 } 1531 ; 1532 return dst; 1533 } 1534 1535 @Override 1536 public boolean equalsDeep(Base other) { 1537 if (!super.equalsDeep(other)) 1538 return false; 1539 if (!(other instanceof TypeRefComponent)) 1540 return false; 1541 TypeRefComponent o = (TypeRefComponent) other; 1542 return compareDeep(code, o.code, true) && compareDeep(profile, o.profile, true) 1543 && compareDeep(aggregation, o.aggregation, true); 1544 } 1545 1546 @Override 1547 public boolean equalsShallow(Base other) { 1548 if (!super.equalsShallow(other)) 1549 return false; 1550 if (!(other instanceof TypeRefComponent)) 1551 return false; 1552 TypeRefComponent o = (TypeRefComponent) other; 1553 return compareValues(code, o.code, true) && compareValues(profile, o.profile, true) 1554 && compareValues(aggregation, o.aggregation, true); 1555 } 1556 1557 public boolean isEmpty() { 1558 return super.isEmpty() && (code == null || code.isEmpty()) && (profile == null || profile.isEmpty()) 1559 && (aggregation == null || aggregation.isEmpty()); 1560 } 1561 1562 public String fhirType() { 1563 return "ElementDefinition.type"; 1564 1565 } 1566 1567 public boolean hasTarget() { 1568 return Utilities.existsInList(getCode(), "Reference"); 1569 1570 } 1571 1572 } 1573 1574 @Block() 1575 public static class ElementDefinitionConstraintComponent extends Element implements IBaseDatatypeElement { 1576 /** 1577 * Allows identification of which elements have their cardinalities impacted by 1578 * the constraint. Will not be referenced for constraints that do not affect 1579 * cardinality. 1580 */ 1581 @Child(name = "key", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1582 @Description(shortDefinition = "Target of 'condition' reference above", formalDefinition = "Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.") 1583 protected IdType key; 1584 1585 /** 1586 * Description of why this constraint is necessary or appropriate. 1587 */ 1588 @Child(name = "requirements", type = { 1589 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1590 @Description(shortDefinition = "Why this constraint necessary or appropriate", formalDefinition = "Description of why this constraint is necessary or appropriate.") 1591 protected StringType requirements; 1592 1593 /** 1594 * Identifies the impact constraint violation has on the conformance of the 1595 * instance. 1596 */ 1597 @Child(name = "severity", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 1598 @Description(shortDefinition = "error | warning", formalDefinition = "Identifies the impact constraint violation has on the conformance of the instance.") 1599 protected Enumeration<ConstraintSeverity> severity; 1600 1601 /** 1602 * Text that can be used to describe the constraint in messages identifying that 1603 * the constraint has been violated. 1604 */ 1605 @Child(name = "human", type = { StringType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 1606 @Description(shortDefinition = "Human description of constraint", formalDefinition = "Text that can be used to describe the constraint in messages identifying that the constraint has been violated.") 1607 protected StringType human; 1608 1609 /** 1610 * An XPath expression of constraint that can be executed to see if this 1611 * constraint is met. 1612 */ 1613 @Child(name = "xpath", type = { StringType.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 1614 @Description(shortDefinition = "XPath expression of constraint", formalDefinition = "An XPath expression of constraint that can be executed to see if this constraint is met.") 1615 protected StringType xpath; 1616 1617 private static final long serialVersionUID = 854521265L; 1618 1619 /* 1620 * Constructor 1621 */ 1622 public ElementDefinitionConstraintComponent() { 1623 super(); 1624 } 1625 1626 /* 1627 * Constructor 1628 */ 1629 public ElementDefinitionConstraintComponent(IdType key, Enumeration<ConstraintSeverity> severity, StringType human, 1630 StringType xpath) { 1631 super(); 1632 this.key = key; 1633 this.severity = severity; 1634 this.human = human; 1635 this.xpath = xpath; 1636 } 1637 1638 /** 1639 * @return {@link #key} (Allows identification of which elements have their 1640 * cardinalities impacted by the constraint. Will not be referenced for 1641 * constraints that do not affect cardinality.). This is the underlying 1642 * object with id, value and extensions. The accessor "getKey" gives 1643 * direct access to the value 1644 */ 1645 public IdType getKeyElement() { 1646 if (this.key == null) 1647 if (Configuration.errorOnAutoCreate()) 1648 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.key"); 1649 else if (Configuration.doAutoCreate()) 1650 this.key = new IdType(); // bb 1651 return this.key; 1652 } 1653 1654 public boolean hasKeyElement() { 1655 return this.key != null && !this.key.isEmpty(); 1656 } 1657 1658 public boolean hasKey() { 1659 return this.key != null && !this.key.isEmpty(); 1660 } 1661 1662 /** 1663 * @param value {@link #key} (Allows identification of which elements have their 1664 * cardinalities impacted by the constraint. Will not be referenced 1665 * for constraints that do not affect cardinality.). This is the 1666 * underlying object with id, value and extensions. The accessor 1667 * "getKey" gives direct access to the value 1668 */ 1669 public ElementDefinitionConstraintComponent setKeyElement(IdType value) { 1670 this.key = value; 1671 return this; 1672 } 1673 1674 /** 1675 * @return Allows identification of which elements have their cardinalities 1676 * impacted by the constraint. Will not be referenced for constraints 1677 * that do not affect cardinality. 1678 */ 1679 public String getKey() { 1680 return this.key == null ? null : this.key.getValue(); 1681 } 1682 1683 /** 1684 * @param value Allows identification of which elements have their cardinalities 1685 * impacted by the constraint. Will not be referenced for 1686 * constraints that do not affect cardinality. 1687 */ 1688 public ElementDefinitionConstraintComponent setKey(String value) { 1689 if (this.key == null) 1690 this.key = new IdType(); 1691 this.key.setValue(value); 1692 return this; 1693 } 1694 1695 /** 1696 * @return {@link #requirements} (Description of why this constraint is 1697 * necessary or appropriate.). This is the underlying object with id, 1698 * value and extensions. The accessor "getRequirements" gives direct 1699 * access to the value 1700 */ 1701 public StringType getRequirementsElement() { 1702 if (this.requirements == null) 1703 if (Configuration.errorOnAutoCreate()) 1704 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.requirements"); 1705 else if (Configuration.doAutoCreate()) 1706 this.requirements = new StringType(); // bb 1707 return this.requirements; 1708 } 1709 1710 public boolean hasRequirementsElement() { 1711 return this.requirements != null && !this.requirements.isEmpty(); 1712 } 1713 1714 public boolean hasRequirements() { 1715 return this.requirements != null && !this.requirements.isEmpty(); 1716 } 1717 1718 /** 1719 * @param value {@link #requirements} (Description of why this constraint is 1720 * necessary or appropriate.). This is the underlying object with 1721 * id, value and extensions. The accessor "getRequirements" gives 1722 * direct access to the value 1723 */ 1724 public ElementDefinitionConstraintComponent setRequirementsElement(StringType value) { 1725 this.requirements = value; 1726 return this; 1727 } 1728 1729 /** 1730 * @return Description of why this constraint is necessary or appropriate. 1731 */ 1732 public String getRequirements() { 1733 return this.requirements == null ? null : this.requirements.getValue(); 1734 } 1735 1736 /** 1737 * @param value Description of why this constraint is necessary or appropriate. 1738 */ 1739 public ElementDefinitionConstraintComponent setRequirements(String value) { 1740 if (Utilities.noString(value)) 1741 this.requirements = null; 1742 else { 1743 if (this.requirements == null) 1744 this.requirements = new StringType(); 1745 this.requirements.setValue(value); 1746 } 1747 return this; 1748 } 1749 1750 /** 1751 * @return {@link #severity} (Identifies the impact constraint violation has on 1752 * the conformance of the instance.). This is the underlying object with 1753 * id, value and extensions. The accessor "getSeverity" gives direct 1754 * access to the value 1755 */ 1756 public Enumeration<ConstraintSeverity> getSeverityElement() { 1757 if (this.severity == null) 1758 if (Configuration.errorOnAutoCreate()) 1759 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.severity"); 1760 else if (Configuration.doAutoCreate()) 1761 this.severity = new Enumeration<ConstraintSeverity>(new ConstraintSeverityEnumFactory()); // bb 1762 return this.severity; 1763 } 1764 1765 public boolean hasSeverityElement() { 1766 return this.severity != null && !this.severity.isEmpty(); 1767 } 1768 1769 public boolean hasSeverity() { 1770 return this.severity != null && !this.severity.isEmpty(); 1771 } 1772 1773 /** 1774 * @param value {@link #severity} (Identifies the impact constraint violation 1775 * has on the conformance of the instance.). This is the underlying 1776 * object with id, value and extensions. The accessor "getSeverity" 1777 * gives direct access to the value 1778 */ 1779 public ElementDefinitionConstraintComponent setSeverityElement(Enumeration<ConstraintSeverity> value) { 1780 this.severity = value; 1781 return this; 1782 } 1783 1784 /** 1785 * @return Identifies the impact constraint violation has on the conformance of 1786 * the instance. 1787 */ 1788 public ConstraintSeverity getSeverity() { 1789 return this.severity == null ? null : this.severity.getValue(); 1790 } 1791 1792 /** 1793 * @param value Identifies the impact constraint violation has on the 1794 * conformance of the instance. 1795 */ 1796 public ElementDefinitionConstraintComponent setSeverity(ConstraintSeverity value) { 1797 if (this.severity == null) 1798 this.severity = new Enumeration<ConstraintSeverity>(new ConstraintSeverityEnumFactory()); 1799 this.severity.setValue(value); 1800 return this; 1801 } 1802 1803 /** 1804 * @return {@link #human} (Text that can be used to describe the constraint in 1805 * messages identifying that the constraint has been violated.). This is 1806 * the underlying object with id, value and extensions. The accessor 1807 * "getHuman" gives direct access to the value 1808 */ 1809 public StringType getHumanElement() { 1810 if (this.human == null) 1811 if (Configuration.errorOnAutoCreate()) 1812 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.human"); 1813 else if (Configuration.doAutoCreate()) 1814 this.human = new StringType(); // bb 1815 return this.human; 1816 } 1817 1818 public boolean hasHumanElement() { 1819 return this.human != null && !this.human.isEmpty(); 1820 } 1821 1822 public boolean hasHuman() { 1823 return this.human != null && !this.human.isEmpty(); 1824 } 1825 1826 /** 1827 * @param value {@link #human} (Text that can be used to describe the constraint 1828 * in messages identifying that the constraint has been violated.). 1829 * This is the underlying object with id, value and extensions. The 1830 * accessor "getHuman" gives direct access to the value 1831 */ 1832 public ElementDefinitionConstraintComponent setHumanElement(StringType value) { 1833 this.human = value; 1834 return this; 1835 } 1836 1837 /** 1838 * @return Text that can be used to describe the constraint in messages 1839 * identifying that the constraint has been violated. 1840 */ 1841 public String getHuman() { 1842 return this.human == null ? null : this.human.getValue(); 1843 } 1844 1845 /** 1846 * @param value Text that can be used to describe the constraint in messages 1847 * identifying that the constraint has been violated. 1848 */ 1849 public ElementDefinitionConstraintComponent setHuman(String value) { 1850 if (this.human == null) 1851 this.human = new StringType(); 1852 this.human.setValue(value); 1853 return this; 1854 } 1855 1856 /** 1857 * @return {@link #xpath} (An XPath expression of constraint that can be 1858 * executed to see if this constraint is met.). This is the underlying 1859 * object with id, value and extensions. The accessor "getXpath" gives 1860 * direct access to the value 1861 */ 1862 public StringType getXpathElement() { 1863 if (this.xpath == null) 1864 if (Configuration.errorOnAutoCreate()) 1865 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.xpath"); 1866 else if (Configuration.doAutoCreate()) 1867 this.xpath = new StringType(); // bb 1868 return this.xpath; 1869 } 1870 1871 public boolean hasXpathElement() { 1872 return this.xpath != null && !this.xpath.isEmpty(); 1873 } 1874 1875 public boolean hasXpath() { 1876 return this.xpath != null && !this.xpath.isEmpty(); 1877 } 1878 1879 /** 1880 * @param value {@link #xpath} (An XPath expression of constraint that can be 1881 * executed to see if this constraint is met.). This is the 1882 * underlying object with id, value and extensions. The accessor 1883 * "getXpath" gives direct access to the value 1884 */ 1885 public ElementDefinitionConstraintComponent setXpathElement(StringType value) { 1886 this.xpath = value; 1887 return this; 1888 } 1889 1890 /** 1891 * @return An XPath expression of constraint that can be executed to see if this 1892 * constraint is met. 1893 */ 1894 public String getXpath() { 1895 return this.xpath == null ? null : this.xpath.getValue(); 1896 } 1897 1898 /** 1899 * @param value An XPath expression of constraint that can be executed to see if 1900 * this constraint is met. 1901 */ 1902 public ElementDefinitionConstraintComponent setXpath(String value) { 1903 if (this.xpath == null) 1904 this.xpath = new StringType(); 1905 this.xpath.setValue(value); 1906 return this; 1907 } 1908 1909 protected void listChildren(List<Property> childrenList) { 1910 super.listChildren(childrenList); 1911 childrenList.add(new Property("key", "id", 1912 "Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.", 1913 0, java.lang.Integer.MAX_VALUE, key)); 1914 childrenList 1915 .add(new Property("requirements", "string", "Description of why this constraint is necessary or appropriate.", 1916 0, java.lang.Integer.MAX_VALUE, requirements)); 1917 childrenList.add(new Property("severity", "code", 1918 "Identifies the impact constraint violation has on the conformance of the instance.", 0, 1919 java.lang.Integer.MAX_VALUE, severity)); 1920 childrenList.add(new Property("human", "string", 1921 "Text that can be used to describe the constraint in messages identifying that the constraint has been violated.", 1922 0, java.lang.Integer.MAX_VALUE, human)); 1923 childrenList.add(new Property("xpath", "string", 1924 "An XPath expression of constraint that can be executed to see if this constraint is met.", 0, 1925 java.lang.Integer.MAX_VALUE, xpath)); 1926 } 1927 1928 @Override 1929 public void setProperty(String name, Base value) throws FHIRException { 1930 if (name.equals("key")) 1931 this.key = castToId(value); // IdType 1932 else if (name.equals("requirements")) 1933 this.requirements = castToString(value); // StringType 1934 else if (name.equals("severity")) 1935 this.severity = new ConstraintSeverityEnumFactory().fromType(value); // Enumeration<ConstraintSeverity> 1936 else if (name.equals("human")) 1937 this.human = castToString(value); // StringType 1938 else if (name.equals("xpath")) 1939 this.xpath = castToString(value); // StringType 1940 else 1941 super.setProperty(name, value); 1942 } 1943 1944 @Override 1945 public Base addChild(String name) throws FHIRException { 1946 if (name.equals("key")) { 1947 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.key"); 1948 } else if (name.equals("requirements")) { 1949 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.requirements"); 1950 } else if (name.equals("severity")) { 1951 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.severity"); 1952 } else if (name.equals("human")) { 1953 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.human"); 1954 } else if (name.equals("xpath")) { 1955 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.xpath"); 1956 } else 1957 return super.addChild(name); 1958 } 1959 1960 public ElementDefinitionConstraintComponent copy() { 1961 ElementDefinitionConstraintComponent dst = new ElementDefinitionConstraintComponent(); 1962 copyValues(dst); 1963 dst.key = key == null ? null : key.copy(); 1964 dst.requirements = requirements == null ? null : requirements.copy(); 1965 dst.severity = severity == null ? null : severity.copy(); 1966 dst.human = human == null ? null : human.copy(); 1967 dst.xpath = xpath == null ? null : xpath.copy(); 1968 return dst; 1969 } 1970 1971 @Override 1972 public boolean equalsDeep(Base other) { 1973 if (!super.equalsDeep(other)) 1974 return false; 1975 if (!(other instanceof ElementDefinitionConstraintComponent)) 1976 return false; 1977 ElementDefinitionConstraintComponent o = (ElementDefinitionConstraintComponent) other; 1978 return compareDeep(key, o.key, true) && compareDeep(requirements, o.requirements, true) 1979 && compareDeep(severity, o.severity, true) && compareDeep(human, o.human, true) 1980 && compareDeep(xpath, o.xpath, true); 1981 } 1982 1983 @Override 1984 public boolean equalsShallow(Base other) { 1985 if (!super.equalsShallow(other)) 1986 return false; 1987 if (!(other instanceof ElementDefinitionConstraintComponent)) 1988 return false; 1989 ElementDefinitionConstraintComponent o = (ElementDefinitionConstraintComponent) other; 1990 return compareValues(key, o.key, true) && compareValues(requirements, o.requirements, true) 1991 && compareValues(severity, o.severity, true) && compareValues(human, o.human, true) 1992 && compareValues(xpath, o.xpath, true); 1993 } 1994 1995 public boolean isEmpty() { 1996 return super.isEmpty() && (key == null || key.isEmpty()) && (requirements == null || requirements.isEmpty()) 1997 && (severity == null || severity.isEmpty()) && (human == null || human.isEmpty()) 1998 && (xpath == null || xpath.isEmpty()); 1999 } 2000 2001 public String fhirType() { 2002 return "ElementDefinition.constraint"; 2003 2004 } 2005 2006 } 2007 2008 @Block() 2009 public static class ElementDefinitionBindingComponent extends Element implements IBaseDatatypeElement { 2010 /** 2011 * Indicates the degree of conformance expectations associated with this binding 2012 * - that is, the degree to which the provided value set must be adhered to in 2013 * the instances. 2014 */ 2015 @Child(name = "strength", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2016 @Description(shortDefinition = "required | extensible | preferred | example", formalDefinition = "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.") 2017 protected Enumeration<BindingStrength> strength; 2018 2019 /** 2020 * Describes the intended use of this particular set of codes. 2021 */ 2022 @Child(name = "description", type = { 2023 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2024 @Description(shortDefinition = "Human explanation of the value set", formalDefinition = "Describes the intended use of this particular set of codes.") 2025 protected StringType description; 2026 2027 /** 2028 * Points to the value set or external definition (e.g. implicit value set) that 2029 * identifies the set of codes to be used. 2030 */ 2031 @Child(name = "valueSet", type = { UriType.class, 2032 ValueSet.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2033 @Description(shortDefinition = "Source of value set", formalDefinition = "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.") 2034 protected Type valueSet; 2035 2036 private static final long serialVersionUID = 1355538460L; 2037 2038 /* 2039 * Constructor 2040 */ 2041 public ElementDefinitionBindingComponent() { 2042 super(); 2043 } 2044 2045 /* 2046 * Constructor 2047 */ 2048 public ElementDefinitionBindingComponent(Enumeration<BindingStrength> strength) { 2049 super(); 2050 this.strength = strength; 2051 } 2052 2053 /** 2054 * @return {@link #strength} (Indicates the degree of conformance expectations 2055 * associated with this binding - that is, the degree to which the 2056 * provided value set must be adhered to in the instances.). This is the 2057 * underlying object with id, value and extensions. The accessor 2058 * "getStrength" gives direct access to the value 2059 */ 2060 public Enumeration<BindingStrength> getStrengthElement() { 2061 if (this.strength == null) 2062 if (Configuration.errorOnAutoCreate()) 2063 throw new Error("Attempt to auto-create ElementDefinitionBindingComponent.strength"); 2064 else if (Configuration.doAutoCreate()) 2065 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); // bb 2066 return this.strength; 2067 } 2068 2069 public boolean hasStrengthElement() { 2070 return this.strength != null && !this.strength.isEmpty(); 2071 } 2072 2073 public boolean hasStrength() { 2074 return this.strength != null && !this.strength.isEmpty(); 2075 } 2076 2077 /** 2078 * @param value {@link #strength} (Indicates the degree of conformance 2079 * expectations associated with this binding - that is, the degree 2080 * to which the provided value set must be adhered to in the 2081 * instances.). This is the underlying object with id, value and 2082 * extensions. The accessor "getStrength" gives direct access to 2083 * the value 2084 */ 2085 public ElementDefinitionBindingComponent setStrengthElement(Enumeration<BindingStrength> value) { 2086 this.strength = value; 2087 return this; 2088 } 2089 2090 /** 2091 * @return Indicates the degree of conformance expectations associated with this 2092 * binding - that is, the degree to which the provided value set must be 2093 * adhered to in the instances. 2094 */ 2095 public BindingStrength getStrength() { 2096 return this.strength == null ? null : this.strength.getValue(); 2097 } 2098 2099 /** 2100 * @param value Indicates the degree of conformance expectations associated with 2101 * this binding - that is, the degree to which the provided value 2102 * set must be adhered to in the instances. 2103 */ 2104 public ElementDefinitionBindingComponent setStrength(BindingStrength value) { 2105 if (this.strength == null) 2106 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); 2107 this.strength.setValue(value); 2108 return this; 2109 } 2110 2111 /** 2112 * @return {@link #description} (Describes the intended use of this particular 2113 * set of codes.). This is the underlying object with id, value and 2114 * extensions. The accessor "getDescription" gives direct access to the 2115 * value 2116 */ 2117 public StringType getDescriptionElement() { 2118 if (this.description == null) 2119 if (Configuration.errorOnAutoCreate()) 2120 throw new Error("Attempt to auto-create ElementDefinitionBindingComponent.description"); 2121 else if (Configuration.doAutoCreate()) 2122 this.description = new StringType(); // bb 2123 return this.description; 2124 } 2125 2126 public boolean hasDescriptionElement() { 2127 return this.description != null && !this.description.isEmpty(); 2128 } 2129 2130 public boolean hasDescription() { 2131 return this.description != null && !this.description.isEmpty(); 2132 } 2133 2134 /** 2135 * @param value {@link #description} (Describes the intended use of this 2136 * particular set of codes.). This is the underlying object with 2137 * id, value and extensions. The accessor "getDescription" gives 2138 * direct access to the value 2139 */ 2140 public ElementDefinitionBindingComponent setDescriptionElement(StringType value) { 2141 this.description = value; 2142 return this; 2143 } 2144 2145 /** 2146 * @return Describes the intended use of this particular set of codes. 2147 */ 2148 public String getDescription() { 2149 return this.description == null ? null : this.description.getValue(); 2150 } 2151 2152 /** 2153 * @param value Describes the intended use of this particular set of codes. 2154 */ 2155 public ElementDefinitionBindingComponent setDescription(String value) { 2156 if (Utilities.noString(value)) 2157 this.description = null; 2158 else { 2159 if (this.description == null) 2160 this.description = new StringType(); 2161 this.description.setValue(value); 2162 } 2163 return this; 2164 } 2165 2166 /** 2167 * @return {@link #valueSet} (Points to the value set or external definition 2168 * (e.g. implicit value set) that identifies the set of codes to be 2169 * used.) 2170 */ 2171 public Type getValueSet() { 2172 return this.valueSet; 2173 } 2174 2175 /** 2176 * @return {@link #valueSet} (Points to the value set or external definition 2177 * (e.g. implicit value set) that identifies the set of codes to be 2178 * used.) 2179 */ 2180 public UriType getValueSetUriType() throws FHIRException { 2181 if (!(this.valueSet instanceof UriType)) 2182 throw new FHIRException("Type mismatch: the type UriType was expected, but " 2183 + this.valueSet.getClass().getName() + " was encountered"); 2184 return (UriType) this.valueSet; 2185 } 2186 2187 public boolean hasValueSetUriType() { 2188 return this.valueSet instanceof UriType; 2189 } 2190 2191 /** 2192 * @return {@link #valueSet} (Points to the value set or external definition 2193 * (e.g. implicit value set) that identifies the set of codes to be 2194 * used.) 2195 */ 2196 public Reference getValueSetReference() throws FHIRException { 2197 if (!(this.valueSet instanceof Reference)) 2198 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2199 + this.valueSet.getClass().getName() + " was encountered"); 2200 return (Reference) this.valueSet; 2201 } 2202 2203 public boolean hasValueSetReference() { 2204 return this.valueSet instanceof Reference; 2205 } 2206 2207 public boolean hasValueSet() { 2208 return this.valueSet != null && !this.valueSet.isEmpty(); 2209 } 2210 2211 /** 2212 * @param value {@link #valueSet} (Points to the value set or external 2213 * definition (e.g. implicit value set) that identifies the set of 2214 * codes to be used.) 2215 */ 2216 public ElementDefinitionBindingComponent setValueSet(Type value) { 2217 this.valueSet = value; 2218 return this; 2219 } 2220 2221 protected void listChildren(List<Property> childrenList) { 2222 super.listChildren(childrenList); 2223 childrenList.add(new Property("strength", "code", 2224 "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 2225 0, java.lang.Integer.MAX_VALUE, strength)); 2226 childrenList.add(new Property("description", "string", 2227 "Describes the intended use of this particular set of codes.", 0, java.lang.Integer.MAX_VALUE, description)); 2228 childrenList.add(new Property("valueSet[x]", "uri|Reference(ValueSet)", 2229 "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.", 2230 0, java.lang.Integer.MAX_VALUE, valueSet)); 2231 } 2232 2233 @Override 2234 public void setProperty(String name, Base value) throws FHIRException { 2235 if (name.equals("strength")) 2236 this.strength = new BindingStrengthEnumFactory().fromType(value); // Enumeration<BindingStrength> 2237 else if (name.equals("description")) 2238 this.description = castToString(value); // StringType 2239 else if (name.equals("valueSet[x]")) 2240 this.valueSet = (Type) value; // Type 2241 else 2242 super.setProperty(name, value); 2243 } 2244 2245 @Override 2246 public Base addChild(String name) throws FHIRException { 2247 if (name.equals("strength")) { 2248 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.strength"); 2249 } else if (name.equals("description")) { 2250 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.description"); 2251 } else if (name.equals("valueSetUri")) { 2252 this.valueSet = new UriType(); 2253 return this.valueSet; 2254 } else if (name.equals("valueSetReference")) { 2255 this.valueSet = new Reference(); 2256 return this.valueSet; 2257 } else 2258 return super.addChild(name); 2259 } 2260 2261 public ElementDefinitionBindingComponent copy() { 2262 ElementDefinitionBindingComponent dst = new ElementDefinitionBindingComponent(); 2263 copyValues(dst); 2264 dst.strength = strength == null ? null : strength.copy(); 2265 dst.description = description == null ? null : description.copy(); 2266 dst.valueSet = valueSet == null ? null : valueSet.copy(); 2267 return dst; 2268 } 2269 2270 @Override 2271 public boolean equalsDeep(Base other) { 2272 if (!super.equalsDeep(other)) 2273 return false; 2274 if (!(other instanceof ElementDefinitionBindingComponent)) 2275 return false; 2276 ElementDefinitionBindingComponent o = (ElementDefinitionBindingComponent) other; 2277 return compareDeep(strength, o.strength, true) && compareDeep(description, o.description, true) 2278 && compareDeep(valueSet, o.valueSet, true); 2279 } 2280 2281 @Override 2282 public boolean equalsShallow(Base other) { 2283 if (!super.equalsShallow(other)) 2284 return false; 2285 if (!(other instanceof ElementDefinitionBindingComponent)) 2286 return false; 2287 ElementDefinitionBindingComponent o = (ElementDefinitionBindingComponent) other; 2288 return compareValues(strength, o.strength, true) && compareValues(description, o.description, true); 2289 } 2290 2291 public boolean isEmpty() { 2292 return super.isEmpty() && (strength == null || strength.isEmpty()) 2293 && (description == null || description.isEmpty()) && (valueSet == null || valueSet.isEmpty()); 2294 } 2295 2296 public String fhirType() { 2297 return "ElementDefinition.binding"; 2298 2299 } 2300 2301 } 2302 2303 @Block() 2304 public static class ElementDefinitionMappingComponent extends Element implements IBaseDatatypeElement { 2305 /** 2306 * An internal reference to the definition of a mapping. 2307 */ 2308 @Child(name = "identity", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2309 @Description(shortDefinition = "Reference to mapping declaration", formalDefinition = "An internal reference to the definition of a mapping.") 2310 protected IdType identity; 2311 2312 /** 2313 * Identifies the computable language in which mapping.map is expressed. 2314 */ 2315 @Child(name = "language", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2316 @Description(shortDefinition = "Computable language of mapping", formalDefinition = "Identifies the computable language in which mapping.map is expressed.") 2317 protected CodeType language; 2318 2319 /** 2320 * Expresses what part of the target specification corresponds to this element. 2321 */ 2322 @Child(name = "map", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 2323 @Description(shortDefinition = "Details of the mapping", formalDefinition = "Expresses what part of the target specification corresponds to this element.") 2324 protected StringType map; 2325 2326 private static final long serialVersionUID = -669205371L; 2327 2328 /* 2329 * Constructor 2330 */ 2331 public ElementDefinitionMappingComponent() { 2332 super(); 2333 } 2334 2335 /* 2336 * Constructor 2337 */ 2338 public ElementDefinitionMappingComponent(IdType identity, StringType map) { 2339 super(); 2340 this.identity = identity; 2341 this.map = map; 2342 } 2343 2344 /** 2345 * @return {@link #identity} (An internal reference to the definition of a 2346 * mapping.). This is the underlying object with id, value and 2347 * extensions. The accessor "getIdentity" gives direct access to the 2348 * value 2349 */ 2350 public IdType getIdentityElement() { 2351 if (this.identity == null) 2352 if (Configuration.errorOnAutoCreate()) 2353 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.identity"); 2354 else if (Configuration.doAutoCreate()) 2355 this.identity = new IdType(); // bb 2356 return this.identity; 2357 } 2358 2359 public boolean hasIdentityElement() { 2360 return this.identity != null && !this.identity.isEmpty(); 2361 } 2362 2363 public boolean hasIdentity() { 2364 return this.identity != null && !this.identity.isEmpty(); 2365 } 2366 2367 /** 2368 * @param value {@link #identity} (An internal reference to the definition of a 2369 * mapping.). This is the underlying object with id, value and 2370 * extensions. The accessor "getIdentity" gives direct access to 2371 * the value 2372 */ 2373 public ElementDefinitionMappingComponent setIdentityElement(IdType value) { 2374 this.identity = value; 2375 return this; 2376 } 2377 2378 /** 2379 * @return An internal reference to the definition of a mapping. 2380 */ 2381 public String getIdentity() { 2382 return this.identity == null ? null : this.identity.getValue(); 2383 } 2384 2385 /** 2386 * @param value An internal reference to the definition of a mapping. 2387 */ 2388 public ElementDefinitionMappingComponent setIdentity(String value) { 2389 if (this.identity == null) 2390 this.identity = new IdType(); 2391 this.identity.setValue(value); 2392 return this; 2393 } 2394 2395 /** 2396 * @return {@link #language} (Identifies the computable language in which 2397 * mapping.map is expressed.). This is the underlying object with id, 2398 * value and extensions. The accessor "getLanguage" gives direct access 2399 * to the value 2400 */ 2401 public CodeType getLanguageElement() { 2402 if (this.language == null) 2403 if (Configuration.errorOnAutoCreate()) 2404 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.language"); 2405 else if (Configuration.doAutoCreate()) 2406 this.language = new CodeType(); // bb 2407 return this.language; 2408 } 2409 2410 public boolean hasLanguageElement() { 2411 return this.language != null && !this.language.isEmpty(); 2412 } 2413 2414 public boolean hasLanguage() { 2415 return this.language != null && !this.language.isEmpty(); 2416 } 2417 2418 /** 2419 * @param value {@link #language} (Identifies the computable language in which 2420 * mapping.map is expressed.). This is the underlying object with 2421 * id, value and extensions. The accessor "getLanguage" gives 2422 * direct access to the value 2423 */ 2424 public ElementDefinitionMappingComponent setLanguageElement(CodeType value) { 2425 this.language = value; 2426 return this; 2427 } 2428 2429 /** 2430 * @return Identifies the computable language in which mapping.map is expressed. 2431 */ 2432 public String getLanguage() { 2433 return this.language == null ? null : this.language.getValue(); 2434 } 2435 2436 /** 2437 * @param value Identifies the computable language in which mapping.map is 2438 * expressed. 2439 */ 2440 public ElementDefinitionMappingComponent setLanguage(String value) { 2441 if (Utilities.noString(value)) 2442 this.language = null; 2443 else { 2444 if (this.language == null) 2445 this.language = new CodeType(); 2446 this.language.setValue(value); 2447 } 2448 return this; 2449 } 2450 2451 /** 2452 * @return {@link #map} (Expresses what part of the target specification 2453 * corresponds to this element.). This is the underlying object with id, 2454 * value and extensions. The accessor "getMap" gives direct access to 2455 * the value 2456 */ 2457 public StringType getMapElement() { 2458 if (this.map == null) 2459 if (Configuration.errorOnAutoCreate()) 2460 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.map"); 2461 else if (Configuration.doAutoCreate()) 2462 this.map = new StringType(); // bb 2463 return this.map; 2464 } 2465 2466 public boolean hasMapElement() { 2467 return this.map != null && !this.map.isEmpty(); 2468 } 2469 2470 public boolean hasMap() { 2471 return this.map != null && !this.map.isEmpty(); 2472 } 2473 2474 /** 2475 * @param value {@link #map} (Expresses what part of the target specification 2476 * corresponds to this element.). This is the underlying object 2477 * with id, value and extensions. The accessor "getMap" gives 2478 * direct access to the value 2479 */ 2480 public ElementDefinitionMappingComponent setMapElement(StringType value) { 2481 this.map = value; 2482 return this; 2483 } 2484 2485 /** 2486 * @return Expresses what part of the target specification corresponds to this 2487 * element. 2488 */ 2489 public String getMap() { 2490 return this.map == null ? null : this.map.getValue(); 2491 } 2492 2493 /** 2494 * @param value Expresses what part of the target specification corresponds to 2495 * this element. 2496 */ 2497 public ElementDefinitionMappingComponent setMap(String value) { 2498 if (this.map == null) 2499 this.map = new StringType(); 2500 this.map.setValue(value); 2501 return this; 2502 } 2503 2504 protected void listChildren(List<Property> childrenList) { 2505 super.listChildren(childrenList); 2506 childrenList.add(new Property("identity", "id", "An internal reference to the definition of a mapping.", 0, 2507 java.lang.Integer.MAX_VALUE, identity)); 2508 childrenList 2509 .add(new Property("language", "code", "Identifies the computable language in which mapping.map is expressed.", 2510 0, java.lang.Integer.MAX_VALUE, language)); 2511 childrenList.add( 2512 new Property("map", "string", "Expresses what part of the target specification corresponds to this element.", 2513 0, java.lang.Integer.MAX_VALUE, map)); 2514 } 2515 2516 @Override 2517 public void setProperty(String name, Base value) throws FHIRException { 2518 if (name.equals("identity")) 2519 this.identity = castToId(value); // IdType 2520 else if (name.equals("language")) 2521 this.language = castToCode(value); // CodeType 2522 else if (name.equals("map")) 2523 this.map = castToString(value); // StringType 2524 else 2525 super.setProperty(name, value); 2526 } 2527 2528 @Override 2529 public Base addChild(String name) throws FHIRException { 2530 if (name.equals("identity")) { 2531 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.identity"); 2532 } else if (name.equals("language")) { 2533 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.language"); 2534 } else if (name.equals("map")) { 2535 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.map"); 2536 } else 2537 return super.addChild(name); 2538 } 2539 2540 public ElementDefinitionMappingComponent copy() { 2541 ElementDefinitionMappingComponent dst = new ElementDefinitionMappingComponent(); 2542 copyValues(dst); 2543 dst.identity = identity == null ? null : identity.copy(); 2544 dst.language = language == null ? null : language.copy(); 2545 dst.map = map == null ? null : map.copy(); 2546 return dst; 2547 } 2548 2549 @Override 2550 public boolean equalsDeep(Base other) { 2551 if (!super.equalsDeep(other)) 2552 return false; 2553 if (!(other instanceof ElementDefinitionMappingComponent)) 2554 return false; 2555 ElementDefinitionMappingComponent o = (ElementDefinitionMappingComponent) other; 2556 return compareDeep(identity, o.identity, true) && compareDeep(language, o.language, true) 2557 && compareDeep(map, o.map, true); 2558 } 2559 2560 @Override 2561 public boolean equalsShallow(Base other) { 2562 if (!super.equalsShallow(other)) 2563 return false; 2564 if (!(other instanceof ElementDefinitionMappingComponent)) 2565 return false; 2566 ElementDefinitionMappingComponent o = (ElementDefinitionMappingComponent) other; 2567 return compareValues(identity, o.identity, true) && compareValues(language, o.language, true) 2568 && compareValues(map, o.map, true); 2569 } 2570 2571 public boolean isEmpty() { 2572 return super.isEmpty() && (identity == null || identity.isEmpty()) && (language == null || language.isEmpty()) 2573 && (map == null || map.isEmpty()); 2574 } 2575 2576 public String fhirType() { 2577 return "ElementDefinition.mapping"; 2578 2579 } 2580 2581 } 2582 2583 /** 2584 * The path identifies the element and is expressed as a "."-separated list of 2585 * ancestor elements, beginning with the name of the resource or extension. 2586 */ 2587 @Child(name = "path", type = { StringType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 2588 @Description(shortDefinition = "The path of the element (see the Detailed Descriptions)", formalDefinition = "The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension.") 2589 protected StringType path; 2590 2591 /** 2592 * Codes that define how this element is represented in instances, when the 2593 * deviation varies from the normal case. 2594 */ 2595 @Child(name = "representation", type = { 2596 CodeType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2597 @Description(shortDefinition = "How this element is represented in instances", formalDefinition = "Codes that define how this element is represented in instances, when the deviation varies from the normal case.") 2598 protected List<Enumeration<PropertyRepresentation>> representation; 2599 2600 /** 2601 * The name of this element definition (to refer to it from other element 2602 * definitions using ElementDefinition.nameReference). This is a unique name 2603 * referring to a specific set of constraints applied to this element. One use 2604 * of this is to provide a name to different slices of the same element. 2605 */ 2606 @Child(name = "name", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2607 @Description(shortDefinition = "Name for this particular element definition (reference target)", formalDefinition = "The name of this element definition (to refer to it from other element definitions using ElementDefinition.nameReference). This is a unique name referring to a specific set of constraints applied to this element. One use of this is to provide a name to different slices of the same element.") 2608 protected StringType name; 2609 2610 /** 2611 * The text to display beside the element indicating its meaning or to use to 2612 * prompt for the element in a user display or form. 2613 */ 2614 @Child(name = "label", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2615 @Description(shortDefinition = "Name for element to display with or prompt for element", formalDefinition = "The text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.") 2616 protected StringType label; 2617 2618 /** 2619 * A code that provides the meaning for the element according to a particular 2620 * terminology. 2621 */ 2622 @Child(name = "code", type = { 2623 Coding.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2624 @Description(shortDefinition = "Defining code", formalDefinition = "A code that provides the meaning for the element according to a particular terminology.") 2625 protected List<Coding> code; 2626 2627 /** 2628 * Indicates that the element is sliced into a set of alternative definitions 2629 * (i.e. in a structure definition, there are multiple different constraints on 2630 * a single element in the base resource). Slicing can be used in any resource 2631 * that has cardinality ..* on the base resource, or any resource with a choice 2632 * of types. The set of slices is any elements that come after this in the 2633 * element sequence that have the same path, until a shorter path occurs (the 2634 * shorter path terminates the set). 2635 */ 2636 @Child(name = "slicing", type = {}, order = 5, min = 0, max = 1, modifier = false, summary = true) 2637 @Description(shortDefinition = "This element is sliced - slices follow", formalDefinition = "Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).") 2638 protected ElementDefinitionSlicingComponent slicing; 2639 2640 /** 2641 * A concise description of what this element means (e.g. for use in 2642 * autogenerated summaries). 2643 */ 2644 @Child(name = "short", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 2645 @Description(shortDefinition = "Concise definition for xml presentation", formalDefinition = "A concise description of what this element means (e.g. for use in autogenerated summaries).") 2646 protected StringType short_; 2647 2648 /** 2649 * Provides a complete explanation of the meaning of the data element for human 2650 * readability. For the case of elements derived from existing elements (e.g. 2651 * constraints), the definition SHALL be consistent with the base definition, 2652 * but convey the meaning of the element in the particular context of use of the 2653 * resource. 2654 */ 2655 @Child(name = "definition", type = { 2656 MarkdownType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 2657 @Description(shortDefinition = "Full formal definition as narrative text", formalDefinition = "Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource.") 2658 protected MarkdownType definition; 2659 2660 /** 2661 * Explanatory notes and implementation guidance about the data element, 2662 * including notes about how to use the data properly, exceptions to proper use, 2663 * etc. 2664 */ 2665 @Child(name = "comments", type = { 2666 MarkdownType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 2667 @Description(shortDefinition = "Comments about the use of this element", formalDefinition = "Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc.") 2668 protected MarkdownType comments; 2669 2670 /** 2671 * This element is for traceability of why the element was created and why the 2672 * constraints exist as they do. This may be used to point to source materials 2673 * or specifications that drove the structure of this element. 2674 */ 2675 @Child(name = "requirements", type = { 2676 MarkdownType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 2677 @Description(shortDefinition = "Why is this needed?", formalDefinition = "This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.") 2678 protected MarkdownType requirements; 2679 2680 /** 2681 * Identifies additional names by which this element might also be known. 2682 */ 2683 @Child(name = "alias", type = { 2684 StringType.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2685 @Description(shortDefinition = "Other names", formalDefinition = "Identifies additional names by which this element might also be known.") 2686 protected List<StringType> alias; 2687 2688 /** 2689 * The minimum number of times this element SHALL appear in the instance. 2690 */ 2691 @Child(name = "min", type = { IntegerType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 2692 @Description(shortDefinition = "Minimum Cardinality", formalDefinition = "The minimum number of times this element SHALL appear in the instance.") 2693 protected IntegerType min; 2694 2695 /** 2696 * The maximum number of times this element is permitted to appear in the 2697 * instance. 2698 */ 2699 @Child(name = "max", type = { StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 2700 @Description(shortDefinition = "Maximum Cardinality (a number or *)", formalDefinition = "The maximum number of times this element is permitted to appear in the instance.") 2701 protected StringType max; 2702 2703 /** 2704 * Information about the base definition of the element, provided to make it 2705 * unncessary for tools to trace the deviation of the element through the 2706 * derived and related profiles. This information is only provided where the 2707 * element definition represents a constraint on another element definition, and 2708 * must be present if there is a base element definition. 2709 */ 2710 @Child(name = "base", type = {}, order = 13, min = 0, max = 1, modifier = false, summary = true) 2711 @Description(shortDefinition = "Base definition information for tools", formalDefinition = "Information about the base definition of the element, provided to make it unncessary for tools to trace the deviation of the element through the derived and related profiles. This information is only provided where the element definition represents a constraint on another element definition, and must be present if there is a base element definition.") 2712 protected ElementDefinitionBaseComponent base; 2713 2714 /** 2715 * The data type or resource that the value of this element is permitted to be. 2716 */ 2717 @Child(name = "type", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2718 @Description(shortDefinition = "Data type and Profile for this element", formalDefinition = "The data type or resource that the value of this element is permitted to be.") 2719 protected List<TypeRefComponent> type; 2720 2721 /** 2722 * Identifies the name of a slice defined elsewhere in the profile whose 2723 * constraints should be applied to the current element. 2724 */ 2725 @Child(name = "nameReference", type = { 2726 StringType.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 2727 @Description(shortDefinition = "To another element constraint (by element.name)", formalDefinition = "Identifies the name of a slice defined elsewhere in the profile whose constraints should be applied to the current element.") 2728 protected StringType nameReference; 2729 2730 /** 2731 * The value that should be used if there is no value stated in the instance 2732 * (e.g. 'if not otherwise specified, the abstract is false'). 2733 */ 2734 @Child(name = "defaultValue", type = {}, order = 16, min = 0, max = 1, modifier = false, summary = true) 2735 @Description(shortDefinition = "Specified value it missing from instance", formalDefinition = "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').") 2736 protected org.hl7.fhir.dstu2.model.Type defaultValue; 2737 2738 /** 2739 * The Implicit meaning that is to be understood when this element is missing 2740 * (e.g. 'when this element is missing, the period is ongoing'. 2741 */ 2742 @Child(name = "meaningWhenMissing", type = { 2743 MarkdownType.class }, order = 17, min = 0, max = 1, modifier = false, summary = true) 2744 @Description(shortDefinition = "Implicit meaning when this element is missing", formalDefinition = "The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'.") 2745 protected MarkdownType meaningWhenMissing; 2746 2747 /** 2748 * Specifies a value that SHALL be exactly the value for this element in the 2749 * instance. For purposes of comparison, non-significant whitespace is ignored, 2750 * and all values must be an exact match (case and accent sensitive). Missing 2751 * elements/attributes must also be missing. 2752 */ 2753 @Child(name = "fixed", type = {}, order = 18, min = 0, max = 1, modifier = false, summary = true) 2754 @Description(shortDefinition = "Value must be exactly this", formalDefinition = "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.") 2755 protected org.hl7.fhir.dstu2.model.Type fixed; 2756 2757 /** 2758 * Specifies a value that the value in the instance SHALL follow - that is, any 2759 * value in the pattern must be found in the instance. Other additional values 2760 * may be found too. This is effectively constraint by example. The values of 2761 * elements present in the pattern must match exactly (case-sensitive, 2762 * accent-sensitive, etc.). 2763 */ 2764 @Child(name = "pattern", type = {}, order = 19, min = 0, max = 1, modifier = false, summary = true) 2765 @Description(shortDefinition = "Value must have at least these property values", formalDefinition = "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).") 2766 protected org.hl7.fhir.dstu2.model.Type pattern; 2767 2768 /** 2769 * A sample value for this element demonstrating the type of information that 2770 * would typically be captured. 2771 */ 2772 @Child(name = "example", type = {}, order = 20, min = 0, max = 1, modifier = false, summary = true) 2773 @Description(shortDefinition = "Example value: [as defined for type]", formalDefinition = "A sample value for this element demonstrating the type of information that would typically be captured.") 2774 protected org.hl7.fhir.dstu2.model.Type example; 2775 2776 /** 2777 * The minimum allowed value for the element. The value is inclusive. This is 2778 * allowed for the types date, dateTime, instant, time, decimal, integer, and 2779 * Quantity. 2780 */ 2781 @Child(name = "minValue", type = {}, order = 21, min = 0, max = 1, modifier = false, summary = true) 2782 @Description(shortDefinition = "Minimum Allowed Value (for some types)", formalDefinition = "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.") 2783 protected org.hl7.fhir.dstu2.model.Type minValue; 2784 2785 /** 2786 * The maximum allowed value for the element. The value is inclusive. This is 2787 * allowed for the types date, dateTime, instant, time, decimal, integer, and 2788 * Quantity. 2789 */ 2790 @Child(name = "maxValue", type = {}, order = 22, min = 0, max = 1, modifier = false, summary = true) 2791 @Description(shortDefinition = "Maximum Allowed Value (for some types)", formalDefinition = "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.") 2792 protected org.hl7.fhir.dstu2.model.Type maxValue; 2793 2794 /** 2795 * Indicates the maximum length in characters that is permitted to be present in 2796 * conformant instances and which is expected to be supported by conformant 2797 * consumers that support the element. 2798 */ 2799 @Child(name = "maxLength", type = { 2800 IntegerType.class }, order = 23, min = 0, max = 1, modifier = false, summary = true) 2801 @Description(shortDefinition = "Max length for strings", formalDefinition = "Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element.") 2802 protected IntegerType maxLength; 2803 2804 /** 2805 * A reference to an invariant that may make additional statements about the 2806 * cardinality or value in the instance. 2807 */ 2808 @Child(name = "condition", type = { 2809 IdType.class }, order = 24, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2810 @Description(shortDefinition = "Reference to invariant about presence", formalDefinition = "A reference to an invariant that may make additional statements about the cardinality or value in the instance.") 2811 protected List<IdType> condition; 2812 2813 /** 2814 * Formal constraints such as co-occurrence and other constraints that can be 2815 * computationally evaluated within the context of the instance. 2816 */ 2817 @Child(name = "constraint", type = {}, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2818 @Description(shortDefinition = "Condition that must evaluate to true", formalDefinition = "Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.") 2819 protected List<ElementDefinitionConstraintComponent> constraint; 2820 2821 /** 2822 * If true, implementations that produce or consume resources SHALL provide 2823 * "support" for the element in some meaningful way. If false, the element may 2824 * be ignored and not supported. 2825 */ 2826 @Child(name = "mustSupport", type = { 2827 BooleanType.class }, order = 26, min = 0, max = 1, modifier = false, summary = true) 2828 @Description(shortDefinition = "If the element must supported", formalDefinition = "If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. If false, the element may be ignored and not supported.") 2829 protected BooleanType mustSupport; 2830 2831 /** 2832 * If true, the value of this element affects the interpretation of the element 2833 * or resource that contains it, and the value of the element cannot be ignored. 2834 * Typically, this is used for status, negation and qualification codes. The 2835 * effect of this is that the element cannot be ignored by systems: they SHALL 2836 * either recognize the element and process it, and/or a pre-determination has 2837 * been made that it is not relevant to their particular system. 2838 */ 2839 @Child(name = "isModifier", type = { 2840 BooleanType.class }, order = 27, min = 0, max = 1, modifier = false, summary = true) 2841 @Description(shortDefinition = "If this modifies the meaning of other elements", formalDefinition = "If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system.") 2842 protected BooleanType isModifier; 2843 2844 /** 2845 * Whether the element should be included if a client requests a search with the 2846 * parameter _summary=true. 2847 */ 2848 @Child(name = "isSummary", type = { 2849 BooleanType.class }, order = 28, min = 0, max = 1, modifier = false, summary = true) 2850 @Description(shortDefinition = "Include when _summary = true?", formalDefinition = "Whether the element should be included if a client requests a search with the parameter _summary=true.") 2851 protected BooleanType isSummary; 2852 2853 /** 2854 * Binds to a value set if this element is coded (code, Coding, 2855 * CodeableConcept). 2856 */ 2857 @Child(name = "binding", type = {}, order = 29, min = 0, max = 1, modifier = false, summary = true) 2858 @Description(shortDefinition = "ValueSet details if this is coded", formalDefinition = "Binds to a value set if this element is coded (code, Coding, CodeableConcept).") 2859 protected ElementDefinitionBindingComponent binding; 2860 2861 /** 2862 * Identifies a concept from an external specification that roughly corresponds 2863 * to this element. 2864 */ 2865 @Child(name = "mapping", type = {}, order = 30, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2866 @Description(shortDefinition = "Map element to another set of definitions", formalDefinition = "Identifies a concept from an external specification that roughly corresponds to this element.") 2867 protected List<ElementDefinitionMappingComponent> mapping; 2868 2869 private static final long serialVersionUID = -447087484L; 2870 2871 /* 2872 * Constructor 2873 */ 2874 public ElementDefinition() { 2875 super(); 2876 } 2877 2878 /* 2879 * Constructor 2880 */ 2881 public ElementDefinition(StringType path) { 2882 super(); 2883 this.path = path; 2884 } 2885 2886 /** 2887 * @return {@link #path} (The path identifies the element and is expressed as a 2888 * "."-separated list of ancestor elements, beginning with the name of 2889 * the resource or extension.). This is the underlying object with id, 2890 * value and extensions. The accessor "getPath" gives direct access to 2891 * the value 2892 */ 2893 public StringType getPathElement() { 2894 if (this.path == null) 2895 if (Configuration.errorOnAutoCreate()) 2896 throw new Error("Attempt to auto-create ElementDefinition.path"); 2897 else if (Configuration.doAutoCreate()) 2898 this.path = new StringType(); // bb 2899 return this.path; 2900 } 2901 2902 public boolean hasPathElement() { 2903 return this.path != null && !this.path.isEmpty(); 2904 } 2905 2906 public boolean hasPath() { 2907 return this.path != null && !this.path.isEmpty(); 2908 } 2909 2910 /** 2911 * @param value {@link #path} (The path identifies the element and is expressed 2912 * as a "."-separated list of ancestor elements, beginning with the 2913 * name of the resource or extension.). This is the underlying 2914 * object with id, value and extensions. The accessor "getPath" 2915 * gives direct access to the value 2916 */ 2917 public ElementDefinition setPathElement(StringType value) { 2918 this.path = value; 2919 return this; 2920 } 2921 2922 /** 2923 * @return The path identifies the element and is expressed as a "."-separated 2924 * list of ancestor elements, beginning with the name of the resource or 2925 * extension. 2926 */ 2927 public String getPath() { 2928 return this.path == null ? null : this.path.getValue(); 2929 } 2930 2931 /** 2932 * @param value The path identifies the element and is expressed as a 2933 * "."-separated list of ancestor elements, beginning with the name 2934 * of the resource or extension. 2935 */ 2936 public ElementDefinition setPath(String value) { 2937 if (this.path == null) 2938 this.path = new StringType(); 2939 this.path.setValue(value); 2940 return this; 2941 } 2942 2943 /** 2944 * @return {@link #representation} (Codes that define how this element is 2945 * represented in instances, when the deviation varies from the normal 2946 * case.) 2947 */ 2948 public List<Enumeration<PropertyRepresentation>> getRepresentation() { 2949 if (this.representation == null) 2950 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 2951 return this.representation; 2952 } 2953 2954 public ElementDefinition setRepresentation(List<Enumeration<PropertyRepresentation>> representation) { 2955 this.representation = representation; 2956 return this; 2957 } 2958 2959 public boolean hasRepresentation() { 2960 if (this.representation == null) 2961 return false; 2962 for (Enumeration<PropertyRepresentation> item : this.representation) 2963 if (!item.isEmpty()) 2964 return true; 2965 return false; 2966 } 2967 2968 /** 2969 * @return {@link #representation} (Codes that define how this element is 2970 * represented in instances, when the deviation varies from the normal 2971 * case.) 2972 */ 2973 // syntactic sugar 2974 public Enumeration<PropertyRepresentation> addRepresentationElement() {// 2 2975 Enumeration<PropertyRepresentation> t = new Enumeration<PropertyRepresentation>( 2976 new PropertyRepresentationEnumFactory()); 2977 if (this.representation == null) 2978 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 2979 this.representation.add(t); 2980 return t; 2981 } 2982 2983 /** 2984 * @param value {@link #representation} (Codes that define how this element is 2985 * represented in instances, when the deviation varies from the 2986 * normal case.) 2987 */ 2988 public ElementDefinition addRepresentation(PropertyRepresentation value) { // 1 2989 Enumeration<PropertyRepresentation> t = new Enumeration<PropertyRepresentation>( 2990 new PropertyRepresentationEnumFactory()); 2991 t.setValue(value); 2992 if (this.representation == null) 2993 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 2994 this.representation.add(t); 2995 return this; 2996 } 2997 2998 /** 2999 * @param value {@link #representation} (Codes that define how this element is 3000 * represented in instances, when the deviation varies from the 3001 * normal case.) 3002 */ 3003 public boolean hasRepresentation(PropertyRepresentation value) { 3004 if (this.representation == null) 3005 return false; 3006 for (Enumeration<PropertyRepresentation> v : this.representation) 3007 if (v.equals(value)) // code 3008 return true; 3009 return false; 3010 } 3011 3012 /** 3013 * @return {@link #name} (The name of this element definition (to refer to it 3014 * from other element definitions using 3015 * ElementDefinition.nameReference). This is a unique name referring to 3016 * a specific set of constraints applied to this element. One use of 3017 * this is to provide a name to different slices of the same element.). 3018 * This is the underlying object with id, value and extensions. The 3019 * accessor "getName" gives direct access to the value 3020 */ 3021 public StringType getNameElement() { 3022 if (this.name == null) 3023 if (Configuration.errorOnAutoCreate()) 3024 throw new Error("Attempt to auto-create ElementDefinition.name"); 3025 else if (Configuration.doAutoCreate()) 3026 this.name = new StringType(); // bb 3027 return this.name; 3028 } 3029 3030 public boolean hasNameElement() { 3031 return this.name != null && !this.name.isEmpty(); 3032 } 3033 3034 public boolean hasName() { 3035 return this.name != null && !this.name.isEmpty(); 3036 } 3037 3038 /** 3039 * @param value {@link #name} (The name of this element definition (to refer to 3040 * it from other element definitions using 3041 * ElementDefinition.nameReference). This is a unique name 3042 * referring to a specific set of constraints applied to this 3043 * element. One use of this is to provide a name to different 3044 * slices of the same element.). This is the underlying object with 3045 * id, value and extensions. The accessor "getName" gives direct 3046 * access to the value 3047 */ 3048 public ElementDefinition setNameElement(StringType value) { 3049 this.name = value; 3050 return this; 3051 } 3052 3053 /** 3054 * @return The name of this element definition (to refer to it from other 3055 * element definitions using ElementDefinition.nameReference). This is a 3056 * unique name referring to a specific set of constraints applied to 3057 * this element. One use of this is to provide a name to different 3058 * slices of the same element. 3059 */ 3060 public String getName() { 3061 return this.name == null ? null : this.name.getValue(); 3062 } 3063 3064 /** 3065 * @param value The name of this element definition (to refer to it from other 3066 * element definitions using ElementDefinition.nameReference). This 3067 * is a unique name referring to a specific set of constraints 3068 * applied to this element. One use of this is to provide a name to 3069 * different slices of the same element. 3070 */ 3071 public ElementDefinition setName(String value) { 3072 if (Utilities.noString(value)) 3073 this.name = null; 3074 else { 3075 if (this.name == null) 3076 this.name = new StringType(); 3077 this.name.setValue(value); 3078 } 3079 return this; 3080 } 3081 3082 /** 3083 * @return {@link #label} (The text to display beside the element indicating its 3084 * meaning or to use to prompt for the element in a user display or 3085 * form.). This is the underlying object with id, value and extensions. 3086 * The accessor "getLabel" gives direct access to the value 3087 */ 3088 public StringType getLabelElement() { 3089 if (this.label == null) 3090 if (Configuration.errorOnAutoCreate()) 3091 throw new Error("Attempt to auto-create ElementDefinition.label"); 3092 else if (Configuration.doAutoCreate()) 3093 this.label = new StringType(); // bb 3094 return this.label; 3095 } 3096 3097 public boolean hasLabelElement() { 3098 return this.label != null && !this.label.isEmpty(); 3099 } 3100 3101 public boolean hasLabel() { 3102 return this.label != null && !this.label.isEmpty(); 3103 } 3104 3105 /** 3106 * @param value {@link #label} (The text to display beside the element 3107 * indicating its meaning or to use to prompt for the element in a 3108 * user display or form.). This is the underlying object with id, 3109 * value and extensions. The accessor "getLabel" gives direct 3110 * access to the value 3111 */ 3112 public ElementDefinition setLabelElement(StringType value) { 3113 this.label = value; 3114 return this; 3115 } 3116 3117 /** 3118 * @return The text to display beside the element indicating its meaning or to 3119 * use to prompt for the element in a user display or form. 3120 */ 3121 public String getLabel() { 3122 return this.label == null ? null : this.label.getValue(); 3123 } 3124 3125 /** 3126 * @param value The text to display beside the element indicating its meaning or 3127 * to use to prompt for the element in a user display or form. 3128 */ 3129 public ElementDefinition setLabel(String value) { 3130 if (Utilities.noString(value)) 3131 this.label = null; 3132 else { 3133 if (this.label == null) 3134 this.label = new StringType(); 3135 this.label.setValue(value); 3136 } 3137 return this; 3138 } 3139 3140 /** 3141 * @return {@link #code} (A code that provides the meaning for the element 3142 * according to a particular terminology.) 3143 */ 3144 public List<Coding> getCode() { 3145 if (this.code == null) 3146 this.code = new ArrayList<Coding>(); 3147 return this.code; 3148 } 3149 3150 public boolean hasCode() { 3151 if (this.code == null) 3152 return false; 3153 for (Coding item : this.code) 3154 if (!item.isEmpty()) 3155 return true; 3156 return false; 3157 } 3158 3159 /** 3160 * @return {@link #code} (A code that provides the meaning for the element 3161 * according to a particular terminology.) 3162 */ 3163 // syntactic sugar 3164 public Coding addCode() { // 3 3165 Coding t = new Coding(); 3166 if (this.code == null) 3167 this.code = new ArrayList<Coding>(); 3168 this.code.add(t); 3169 return t; 3170 } 3171 3172 // syntactic sugar 3173 public ElementDefinition addCode(Coding t) { // 3 3174 if (t == null) 3175 return this; 3176 if (this.code == null) 3177 this.code = new ArrayList<Coding>(); 3178 this.code.add(t); 3179 return this; 3180 } 3181 3182 /** 3183 * @return {@link #slicing} (Indicates that the element is sliced into a set of 3184 * alternative definitions (i.e. in a structure definition, there are 3185 * multiple different constraints on a single element in the base 3186 * resource). Slicing can be used in any resource that has cardinality 3187 * ..* on the base resource, or any resource with a choice of types. The 3188 * set of slices is any elements that come after this in the element 3189 * sequence that have the same path, until a shorter path occurs (the 3190 * shorter path terminates the set).) 3191 */ 3192 public ElementDefinitionSlicingComponent getSlicing() { 3193 if (this.slicing == null) 3194 if (Configuration.errorOnAutoCreate()) 3195 throw new Error("Attempt to auto-create ElementDefinition.slicing"); 3196 else if (Configuration.doAutoCreate()) 3197 this.slicing = new ElementDefinitionSlicingComponent(); // cc 3198 return this.slicing; 3199 } 3200 3201 public boolean hasSlicing() { 3202 return this.slicing != null && !this.slicing.isEmpty(); 3203 } 3204 3205 /** 3206 * @param value {@link #slicing} (Indicates that the element is sliced into a 3207 * set of alternative definitions (i.e. in a structure definition, 3208 * there are multiple different constraints on a single element in 3209 * the base resource). Slicing can be used in any resource that has 3210 * cardinality ..* on the base resource, or any resource with a 3211 * choice of types. The set of slices is any elements that come 3212 * after this in the element sequence that have the same path, 3213 * until a shorter path occurs (the shorter path terminates the 3214 * set).) 3215 */ 3216 public ElementDefinition setSlicing(ElementDefinitionSlicingComponent value) { 3217 this.slicing = value; 3218 return this; 3219 } 3220 3221 /** 3222 * @return {@link #short_} (A concise description of what this element means 3223 * (e.g. for use in autogenerated summaries).). This is the underlying 3224 * object with id, value and extensions. The accessor "getShort" gives 3225 * direct access to the value 3226 */ 3227 public StringType getShortElement() { 3228 if (this.short_ == null) 3229 if (Configuration.errorOnAutoCreate()) 3230 throw new Error("Attempt to auto-create ElementDefinition.short_"); 3231 else if (Configuration.doAutoCreate()) 3232 this.short_ = new StringType(); // bb 3233 return this.short_; 3234 } 3235 3236 public boolean hasShortElement() { 3237 return this.short_ != null && !this.short_.isEmpty(); 3238 } 3239 3240 public boolean hasShort() { 3241 return this.short_ != null && !this.short_.isEmpty(); 3242 } 3243 3244 /** 3245 * @param value {@link #short_} (A concise description of what this element 3246 * means (e.g. for use in autogenerated summaries).). This is the 3247 * underlying object with id, value and extensions. The accessor 3248 * "getShort" gives direct access to the value 3249 */ 3250 public ElementDefinition setShortElement(StringType value) { 3251 this.short_ = value; 3252 return this; 3253 } 3254 3255 /** 3256 * @return A concise description of what this element means (e.g. for use in 3257 * autogenerated summaries). 3258 */ 3259 public String getShort() { 3260 return this.short_ == null ? null : this.short_.getValue(); 3261 } 3262 3263 /** 3264 * @param value A concise description of what this element means (e.g. for use 3265 * in autogenerated summaries). 3266 */ 3267 public ElementDefinition setShort(String value) { 3268 if (Utilities.noString(value)) 3269 this.short_ = null; 3270 else { 3271 if (this.short_ == null) 3272 this.short_ = new StringType(); 3273 this.short_.setValue(value); 3274 } 3275 return this; 3276 } 3277 3278 /** 3279 * @return {@link #definition} (Provides a complete explanation of the meaning 3280 * of the data element for human readability. For the case of elements 3281 * derived from existing elements (e.g. constraints), the definition 3282 * SHALL be consistent with the base definition, but convey the meaning 3283 * of the element in the particular context of use of the resource.). 3284 * This is the underlying object with id, value and extensions. The 3285 * accessor "getDefinition" gives direct access to the value 3286 */ 3287 public MarkdownType getDefinitionElement() { 3288 if (this.definition == null) 3289 if (Configuration.errorOnAutoCreate()) 3290 throw new Error("Attempt to auto-create ElementDefinition.definition"); 3291 else if (Configuration.doAutoCreate()) 3292 this.definition = new MarkdownType(); // bb 3293 return this.definition; 3294 } 3295 3296 public boolean hasDefinitionElement() { 3297 return this.definition != null && !this.definition.isEmpty(); 3298 } 3299 3300 public boolean hasDefinition() { 3301 return this.definition != null && !this.definition.isEmpty(); 3302 } 3303 3304 /** 3305 * @param value {@link #definition} (Provides a complete explanation of the 3306 * meaning of the data element for human readability. For the case 3307 * of elements derived from existing elements (e.g. constraints), 3308 * the definition SHALL be consistent with the base definition, but 3309 * convey the meaning of the element in the particular context of 3310 * use of the resource.). This is the underlying object with id, 3311 * value and extensions. The accessor "getDefinition" gives direct 3312 * access to the value 3313 */ 3314 public ElementDefinition setDefinitionElement(MarkdownType value) { 3315 this.definition = value; 3316 return this; 3317 } 3318 3319 /** 3320 * @return Provides a complete explanation of the meaning of the data element 3321 * for human readability. For the case of elements derived from existing 3322 * elements (e.g. constraints), the definition SHALL be consistent with 3323 * the base definition, but convey the meaning of the element in the 3324 * particular context of use of the resource. 3325 */ 3326 public String getDefinition() { 3327 return this.definition == null ? null : this.definition.getValue(); 3328 } 3329 3330 /** 3331 * @param value Provides a complete explanation of the meaning of the data 3332 * element for human readability. For the case of elements derived 3333 * from existing elements (e.g. constraints), the definition SHALL 3334 * be consistent with the base definition, but convey the meaning 3335 * of the element in the particular context of use of the resource. 3336 */ 3337 public ElementDefinition setDefinition(String value) { 3338 if (value == null) 3339 this.definition = null; 3340 else { 3341 if (this.definition == null) 3342 this.definition = new MarkdownType(); 3343 this.definition.setValue(value); 3344 } 3345 return this; 3346 } 3347 3348 /** 3349 * @return {@link #comments} (Explanatory notes and implementation guidance 3350 * about the data element, including notes about how to use the data 3351 * properly, exceptions to proper use, etc.). This is the underlying 3352 * object with id, value and extensions. The accessor "getComments" 3353 * gives direct access to the value 3354 */ 3355 public MarkdownType getCommentsElement() { 3356 if (this.comments == null) 3357 if (Configuration.errorOnAutoCreate()) 3358 throw new Error("Attempt to auto-create ElementDefinition.comments"); 3359 else if (Configuration.doAutoCreate()) 3360 this.comments = new MarkdownType(); // bb 3361 return this.comments; 3362 } 3363 3364 public boolean hasCommentsElement() { 3365 return this.comments != null && !this.comments.isEmpty(); 3366 } 3367 3368 public boolean hasComments() { 3369 return this.comments != null && !this.comments.isEmpty(); 3370 } 3371 3372 /** 3373 * @param value {@link #comments} (Explanatory notes and implementation guidance 3374 * about the data element, including notes about how to use the 3375 * data properly, exceptions to proper use, etc.). This is the 3376 * underlying object with id, value and extensions. The accessor 3377 * "getComments" gives direct access to the value 3378 */ 3379 public ElementDefinition setCommentsElement(MarkdownType value) { 3380 this.comments = value; 3381 return this; 3382 } 3383 3384 /** 3385 * @return Explanatory notes and implementation guidance about the data element, 3386 * including notes about how to use the data properly, exceptions to 3387 * proper use, etc. 3388 */ 3389 public String getComments() { 3390 return this.comments == null ? null : this.comments.getValue(); 3391 } 3392 3393 /** 3394 * @param value Explanatory notes and implementation guidance about the data 3395 * element, including notes about how to use the data properly, 3396 * exceptions to proper use, etc. 3397 */ 3398 public ElementDefinition setComments(String value) { 3399 if (value == null) 3400 this.comments = null; 3401 else { 3402 if (this.comments == null) 3403 this.comments = new MarkdownType(); 3404 this.comments.setValue(value); 3405 } 3406 return this; 3407 } 3408 3409 /** 3410 * @return {@link #requirements} (This element is for traceability of why the 3411 * element was created and why the constraints exist as they do. This 3412 * may be used to point to source materials or specifications that drove 3413 * the structure of this element.). This is the underlying object with 3414 * id, value and extensions. The accessor "getRequirements" gives direct 3415 * access to the value 3416 */ 3417 public MarkdownType getRequirementsElement() { 3418 if (this.requirements == null) 3419 if (Configuration.errorOnAutoCreate()) 3420 throw new Error("Attempt to auto-create ElementDefinition.requirements"); 3421 else if (Configuration.doAutoCreate()) 3422 this.requirements = new MarkdownType(); // bb 3423 return this.requirements; 3424 } 3425 3426 public boolean hasRequirementsElement() { 3427 return this.requirements != null && !this.requirements.isEmpty(); 3428 } 3429 3430 public boolean hasRequirements() { 3431 return this.requirements != null && !this.requirements.isEmpty(); 3432 } 3433 3434 /** 3435 * @param value {@link #requirements} (This element is for traceability of why 3436 * the element was created and why the constraints exist as they 3437 * do. This may be used to point to source materials or 3438 * specifications that drove the structure of this element.). This 3439 * is the underlying object with id, value and extensions. The 3440 * accessor "getRequirements" gives direct access to the value 3441 */ 3442 public ElementDefinition setRequirementsElement(MarkdownType value) { 3443 this.requirements = value; 3444 return this; 3445 } 3446 3447 /** 3448 * @return This element is for traceability of why the element was created and 3449 * why the constraints exist as they do. This may be used to point to 3450 * source materials or specifications that drove the structure of this 3451 * element. 3452 */ 3453 public String getRequirements() { 3454 return this.requirements == null ? null : this.requirements.getValue(); 3455 } 3456 3457 /** 3458 * @param value This element is for traceability of why the element was created 3459 * and why the constraints exist as they do. This may be used to 3460 * point to source materials or specifications that drove the 3461 * structure of this element. 3462 */ 3463 public ElementDefinition setRequirements(String value) { 3464 if (value == null) 3465 this.requirements = null; 3466 else { 3467 if (this.requirements == null) 3468 this.requirements = new MarkdownType(); 3469 this.requirements.setValue(value); 3470 } 3471 return this; 3472 } 3473 3474 /** 3475 * @return {@link #alias} (Identifies additional names by which this element 3476 * might also be known.) 3477 */ 3478 public List<StringType> getAlias() { 3479 if (this.alias == null) 3480 this.alias = new ArrayList<StringType>(); 3481 return this.alias; 3482 } 3483 3484 public boolean hasAlias() { 3485 if (this.alias == null) 3486 return false; 3487 for (StringType item : this.alias) 3488 if (!item.isEmpty()) 3489 return true; 3490 return false; 3491 } 3492 3493 /** 3494 * @return {@link #alias} (Identifies additional names by which this element 3495 * might also be known.) 3496 */ 3497 // syntactic sugar 3498 public StringType addAliasElement() {// 2 3499 StringType t = new StringType(); 3500 if (this.alias == null) 3501 this.alias = new ArrayList<StringType>(); 3502 this.alias.add(t); 3503 return t; 3504 } 3505 3506 /** 3507 * @param value {@link #alias} (Identifies additional names by which this 3508 * element might also be known.) 3509 */ 3510 public ElementDefinition addAlias(String value) { // 1 3511 StringType t = new StringType(); 3512 t.setValue(value); 3513 if (this.alias == null) 3514 this.alias = new ArrayList<StringType>(); 3515 this.alias.add(t); 3516 return this; 3517 } 3518 3519 /** 3520 * @param value {@link #alias} (Identifies additional names by which this 3521 * element might also be known.) 3522 */ 3523 public boolean hasAlias(String value) { 3524 if (this.alias == null) 3525 return false; 3526 for (StringType v : this.alias) 3527 if (v.equals(value)) // string 3528 return true; 3529 return false; 3530 } 3531 3532 /** 3533 * @return {@link #min} (The minimum number of times this element SHALL appear 3534 * in the instance.). This is the underlying object with id, value and 3535 * extensions. The accessor "getMin" gives direct access to the value 3536 */ 3537 public IntegerType getMinElement() { 3538 if (this.min == null) 3539 if (Configuration.errorOnAutoCreate()) 3540 throw new Error("Attempt to auto-create ElementDefinition.min"); 3541 else if (Configuration.doAutoCreate()) 3542 this.min = new IntegerType(); // bb 3543 return this.min; 3544 } 3545 3546 public boolean hasMinElement() { 3547 return this.min != null && !this.min.isEmpty(); 3548 } 3549 3550 public boolean hasMin() { 3551 return this.min != null && !this.min.isEmpty(); 3552 } 3553 3554 /** 3555 * @param value {@link #min} (The minimum number of times this element SHALL 3556 * appear in the instance.). This is the underlying object with id, 3557 * value and extensions. The accessor "getMin" gives direct access 3558 * to the value 3559 */ 3560 public ElementDefinition setMinElement(IntegerType value) { 3561 this.min = value; 3562 return this; 3563 } 3564 3565 /** 3566 * @return The minimum number of times this element SHALL appear in the 3567 * instance. 3568 */ 3569 public int getMin() { 3570 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 3571 } 3572 3573 /** 3574 * @param value The minimum number of times this element SHALL appear in the 3575 * instance. 3576 */ 3577 public ElementDefinition setMin(int value) { 3578 if (this.min == null) 3579 this.min = new IntegerType(); 3580 this.min.setValue(value); 3581 return this; 3582 } 3583 3584 /** 3585 * @return {@link #max} (The maximum number of times this element is permitted 3586 * to appear in the instance.). This is the underlying object with id, 3587 * value and extensions. The accessor "getMax" gives direct access to 3588 * the value 3589 */ 3590 public StringType getMaxElement() { 3591 if (this.max == null) 3592 if (Configuration.errorOnAutoCreate()) 3593 throw new Error("Attempt to auto-create ElementDefinition.max"); 3594 else if (Configuration.doAutoCreate()) 3595 this.max = new StringType(); // bb 3596 return this.max; 3597 } 3598 3599 public boolean hasMaxElement() { 3600 return this.max != null && !this.max.isEmpty(); 3601 } 3602 3603 public boolean hasMax() { 3604 return this.max != null && !this.max.isEmpty(); 3605 } 3606 3607 /** 3608 * @param value {@link #max} (The maximum number of times this element is 3609 * permitted to appear in the instance.). This is the underlying 3610 * object with id, value and extensions. The accessor "getMax" 3611 * gives direct access to the value 3612 */ 3613 public ElementDefinition setMaxElement(StringType value) { 3614 this.max = value; 3615 return this; 3616 } 3617 3618 /** 3619 * @return The maximum number of times this element is permitted to appear in 3620 * the instance. 3621 */ 3622 public String getMax() { 3623 return this.max == null ? null : this.max.getValue(); 3624 } 3625 3626 /** 3627 * @param value The maximum number of times this element is permitted to appear 3628 * in the instance. 3629 */ 3630 public ElementDefinition setMax(String value) { 3631 if (Utilities.noString(value)) 3632 this.max = null; 3633 else { 3634 if (this.max == null) 3635 this.max = new StringType(); 3636 this.max.setValue(value); 3637 } 3638 return this; 3639 } 3640 3641 /** 3642 * @return {@link #base} (Information about the base definition of the element, 3643 * provided to make it unncessary for tools to trace the deviation of 3644 * the element through the derived and related profiles. This 3645 * information is only provided where the element definition represents 3646 * a constraint on another element definition, and must be present if 3647 * there is a base element definition.) 3648 */ 3649 public ElementDefinitionBaseComponent getBase() { 3650 if (this.base == null) 3651 if (Configuration.errorOnAutoCreate()) 3652 throw new Error("Attempt to auto-create ElementDefinition.base"); 3653 else if (Configuration.doAutoCreate()) 3654 this.base = new ElementDefinitionBaseComponent(); // cc 3655 return this.base; 3656 } 3657 3658 public boolean hasBase() { 3659 return this.base != null && !this.base.isEmpty(); 3660 } 3661 3662 /** 3663 * @param value {@link #base} (Information about the base definition of the 3664 * element, provided to make it unncessary for tools to trace the 3665 * deviation of the element through the derived and related 3666 * profiles. This information is only provided where the element 3667 * definition represents a constraint on another element 3668 * definition, and must be present if there is a base element 3669 * definition.) 3670 */ 3671 public ElementDefinition setBase(ElementDefinitionBaseComponent value) { 3672 this.base = value; 3673 return this; 3674 } 3675 3676 /** 3677 * @return {@link #type} (The data type or resource that the value of this 3678 * element is permitted to be.) 3679 */ 3680 public List<TypeRefComponent> getType() { 3681 if (this.type == null) 3682 this.type = new ArrayList<TypeRefComponent>(); 3683 return this.type; 3684 } 3685 3686 public boolean hasType() { 3687 if (this.type == null) 3688 return false; 3689 for (TypeRefComponent item : this.type) 3690 if (!item.isEmpty()) 3691 return true; 3692 return false; 3693 } 3694 3695 /** 3696 * @return {@link #type} (The data type or resource that the value of this 3697 * element is permitted to be.) 3698 */ 3699 // syntactic sugar 3700 public TypeRefComponent addType() { // 3 3701 TypeRefComponent t = new TypeRefComponent(); 3702 if (this.type == null) 3703 this.type = new ArrayList<TypeRefComponent>(); 3704 this.type.add(t); 3705 return t; 3706 } 3707 3708 // syntactic sugar 3709 public ElementDefinition addType(TypeRefComponent t) { // 3 3710 if (t == null) 3711 return this; 3712 if (this.type == null) 3713 this.type = new ArrayList<TypeRefComponent>(); 3714 this.type.add(t); 3715 return this; 3716 } 3717 3718 /** 3719 * @return {@link #nameReference} (Identifies the name of a slice defined 3720 * elsewhere in the profile whose constraints should be applied to the 3721 * current element.). This is the underlying object with id, value and 3722 * extensions. The accessor "getNameReference" gives direct access to 3723 * the value 3724 */ 3725 public StringType getNameReferenceElement() { 3726 if (this.nameReference == null) 3727 if (Configuration.errorOnAutoCreate()) 3728 throw new Error("Attempt to auto-create ElementDefinition.nameReference"); 3729 else if (Configuration.doAutoCreate()) 3730 this.nameReference = new StringType(); // bb 3731 return this.nameReference; 3732 } 3733 3734 public boolean hasNameReferenceElement() { 3735 return this.nameReference != null && !this.nameReference.isEmpty(); 3736 } 3737 3738 public boolean hasNameReference() { 3739 return this.nameReference != null && !this.nameReference.isEmpty(); 3740 } 3741 3742 /** 3743 * @param value {@link #nameReference} (Identifies the name of a slice defined 3744 * elsewhere in the profile whose constraints should be applied to 3745 * the current element.). This is the underlying object with id, 3746 * value and extensions. The accessor "getNameReference" gives 3747 * direct access to the value 3748 */ 3749 public ElementDefinition setNameReferenceElement(StringType value) { 3750 this.nameReference = value; 3751 return this; 3752 } 3753 3754 /** 3755 * @return Identifies the name of a slice defined elsewhere in the profile whose 3756 * constraints should be applied to the current element. 3757 */ 3758 public String getNameReference() { 3759 return this.nameReference == null ? null : this.nameReference.getValue(); 3760 } 3761 3762 /** 3763 * @param value Identifies the name of a slice defined elsewhere in the profile 3764 * whose constraints should be applied to the current element. 3765 */ 3766 public ElementDefinition setNameReference(String value) { 3767 if (Utilities.noString(value)) 3768 this.nameReference = null; 3769 else { 3770 if (this.nameReference == null) 3771 this.nameReference = new StringType(); 3772 this.nameReference.setValue(value); 3773 } 3774 return this; 3775 } 3776 3777 /** 3778 * @return {@link #defaultValue} (The value that should be used if there is no 3779 * value stated in the instance (e.g. 'if not otherwise specified, the 3780 * abstract is false').) 3781 */ 3782 public org.hl7.fhir.dstu2.model.Type getDefaultValue() { 3783 return this.defaultValue; 3784 } 3785 3786 public boolean hasDefaultValue() { 3787 return this.defaultValue != null && !this.defaultValue.isEmpty(); 3788 } 3789 3790 /** 3791 * @param value {@link #defaultValue} (The value that should be used if there is 3792 * no value stated in the instance (e.g. 'if not otherwise 3793 * specified, the abstract is false').) 3794 */ 3795 public ElementDefinition setDefaultValue(org.hl7.fhir.dstu2.model.Type value) { 3796 this.defaultValue = value; 3797 return this; 3798 } 3799 3800 /** 3801 * @return {@link #meaningWhenMissing} (The Implicit meaning that is to be 3802 * understood when this element is missing (e.g. 'when this element is 3803 * missing, the period is ongoing'.). This is the underlying object with 3804 * id, value and extensions. The accessor "getMeaningWhenMissing" gives 3805 * direct access to the value 3806 */ 3807 public MarkdownType getMeaningWhenMissingElement() { 3808 if (this.meaningWhenMissing == null) 3809 if (Configuration.errorOnAutoCreate()) 3810 throw new Error("Attempt to auto-create ElementDefinition.meaningWhenMissing"); 3811 else if (Configuration.doAutoCreate()) 3812 this.meaningWhenMissing = new MarkdownType(); // bb 3813 return this.meaningWhenMissing; 3814 } 3815 3816 public boolean hasMeaningWhenMissingElement() { 3817 return this.meaningWhenMissing != null && !this.meaningWhenMissing.isEmpty(); 3818 } 3819 3820 public boolean hasMeaningWhenMissing() { 3821 return this.meaningWhenMissing != null && !this.meaningWhenMissing.isEmpty(); 3822 } 3823 3824 /** 3825 * @param value {@link #meaningWhenMissing} (The Implicit meaning that is to be 3826 * understood when this element is missing (e.g. 'when this element 3827 * is missing, the period is ongoing'.). This is the underlying 3828 * object with id, value and extensions. The accessor 3829 * "getMeaningWhenMissing" gives direct access to the value 3830 */ 3831 public ElementDefinition setMeaningWhenMissingElement(MarkdownType value) { 3832 this.meaningWhenMissing = value; 3833 return this; 3834 } 3835 3836 /** 3837 * @return The Implicit meaning that is to be understood when this element is 3838 * missing (e.g. 'when this element is missing, the period is ongoing'. 3839 */ 3840 public String getMeaningWhenMissing() { 3841 return this.meaningWhenMissing == null ? null : this.meaningWhenMissing.getValue(); 3842 } 3843 3844 /** 3845 * @param value The Implicit meaning that is to be understood when this element 3846 * is missing (e.g. 'when this element is missing, the period is 3847 * ongoing'. 3848 */ 3849 public ElementDefinition setMeaningWhenMissing(String value) { 3850 if (value == null) 3851 this.meaningWhenMissing = null; 3852 else { 3853 if (this.meaningWhenMissing == null) 3854 this.meaningWhenMissing = new MarkdownType(); 3855 this.meaningWhenMissing.setValue(value); 3856 } 3857 return this; 3858 } 3859 3860 /** 3861 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for 3862 * this element in the instance. For purposes of comparison, 3863 * non-significant whitespace is ignored, and all values must be an 3864 * exact match (case and accent sensitive). Missing elements/attributes 3865 * must also be missing.) 3866 */ 3867 public org.hl7.fhir.dstu2.model.Type getFixed() { 3868 return this.fixed; 3869 } 3870 3871 public boolean hasFixed() { 3872 return this.fixed != null && !this.fixed.isEmpty(); 3873 } 3874 3875 /** 3876 * @param value {@link #fixed} (Specifies a value that SHALL be exactly the 3877 * value for this element in the instance. For purposes of 3878 * comparison, non-significant whitespace is ignored, and all 3879 * values must be an exact match (case and accent sensitive). 3880 * Missing elements/attributes must also be missing.) 3881 */ 3882 public ElementDefinition setFixed(org.hl7.fhir.dstu2.model.Type value) { 3883 this.fixed = value; 3884 return this; 3885 } 3886 3887 /** 3888 * @return {@link #pattern} (Specifies a value that the value in the instance 3889 * SHALL follow - that is, any value in the pattern must be found in the 3890 * instance. Other additional values may be found too. This is 3891 * effectively constraint by example. The values of elements present in 3892 * the pattern must match exactly (case-sensitive, accent-sensitive, 3893 * etc.).) 3894 */ 3895 public org.hl7.fhir.dstu2.model.Type getPattern() { 3896 return this.pattern; 3897 } 3898 3899 public boolean hasPattern() { 3900 return this.pattern != null && !this.pattern.isEmpty(); 3901 } 3902 3903 /** 3904 * @param value {@link #pattern} (Specifies a value that the value in the 3905 * instance SHALL follow - that is, any value in the pattern must 3906 * be found in the instance. Other additional values may be found 3907 * too. This is effectively constraint by example. The values of 3908 * elements present in the pattern must match exactly 3909 * (case-sensitive, accent-sensitive, etc.).) 3910 */ 3911 public ElementDefinition setPattern(org.hl7.fhir.dstu2.model.Type value) { 3912 this.pattern = value; 3913 return this; 3914 } 3915 3916 /** 3917 * @return {@link #example} (A sample value for this element demonstrating the 3918 * type of information that would typically be captured.) 3919 */ 3920 public org.hl7.fhir.dstu2.model.Type getExample() { 3921 return this.example; 3922 } 3923 3924 public boolean hasExample() { 3925 return this.example != null && !this.example.isEmpty(); 3926 } 3927 3928 /** 3929 * @param value {@link #example} (A sample value for this element demonstrating 3930 * the type of information that would typically be captured.) 3931 */ 3932 public ElementDefinition setExample(org.hl7.fhir.dstu2.model.Type value) { 3933 this.example = value; 3934 return this; 3935 } 3936 3937 /** 3938 * @return {@link #minValue} (The minimum allowed value for the element. The 3939 * value is inclusive. This is allowed for the types date, dateTime, 3940 * instant, time, decimal, integer, and Quantity.) 3941 */ 3942 public org.hl7.fhir.dstu2.model.Type getMinValue() { 3943 return this.minValue; 3944 } 3945 3946 public boolean hasMinValue() { 3947 return this.minValue != null && !this.minValue.isEmpty(); 3948 } 3949 3950 /** 3951 * @param value {@link #minValue} (The minimum allowed value for the element. 3952 * The value is inclusive. This is allowed for the types date, 3953 * dateTime, instant, time, decimal, integer, and Quantity.) 3954 */ 3955 public ElementDefinition setMinValue(org.hl7.fhir.dstu2.model.Type value) { 3956 this.minValue = value; 3957 return this; 3958 } 3959 3960 /** 3961 * @return {@link #maxValue} (The maximum allowed value for the element. The 3962 * value is inclusive. This is allowed for the types date, dateTime, 3963 * instant, time, decimal, integer, and Quantity.) 3964 */ 3965 public org.hl7.fhir.dstu2.model.Type getMaxValue() { 3966 return this.maxValue; 3967 } 3968 3969 public boolean hasMaxValue() { 3970 return this.maxValue != null && !this.maxValue.isEmpty(); 3971 } 3972 3973 /** 3974 * @param value {@link #maxValue} (The maximum allowed value for the element. 3975 * The value is inclusive. This is allowed for the types date, 3976 * dateTime, instant, time, decimal, integer, and Quantity.) 3977 */ 3978 public ElementDefinition setMaxValue(org.hl7.fhir.dstu2.model.Type value) { 3979 this.maxValue = value; 3980 return this; 3981 } 3982 3983 /** 3984 * @return {@link #maxLength} (Indicates the maximum length in characters that 3985 * is permitted to be present in conformant instances and which is 3986 * expected to be supported by conformant consumers that support the 3987 * element.). This is the underlying object with id, value and 3988 * extensions. The accessor "getMaxLength" gives direct access to the 3989 * value 3990 */ 3991 public IntegerType getMaxLengthElement() { 3992 if (this.maxLength == null) 3993 if (Configuration.errorOnAutoCreate()) 3994 throw new Error("Attempt to auto-create ElementDefinition.maxLength"); 3995 else if (Configuration.doAutoCreate()) 3996 this.maxLength = new IntegerType(); // bb 3997 return this.maxLength; 3998 } 3999 4000 public boolean hasMaxLengthElement() { 4001 return this.maxLength != null && !this.maxLength.isEmpty(); 4002 } 4003 4004 public boolean hasMaxLength() { 4005 return this.maxLength != null && !this.maxLength.isEmpty(); 4006 } 4007 4008 /** 4009 * @param value {@link #maxLength} (Indicates the maximum length in characters 4010 * that is permitted to be present in conformant instances and 4011 * which is expected to be supported by conformant consumers that 4012 * support the element.). This is the underlying object with id, 4013 * value and extensions. The accessor "getMaxLength" gives direct 4014 * access to the value 4015 */ 4016 public ElementDefinition setMaxLengthElement(IntegerType value) { 4017 this.maxLength = value; 4018 return this; 4019 } 4020 4021 /** 4022 * @return Indicates the maximum length in characters that is permitted to be 4023 * present in conformant instances and which is expected to be supported 4024 * by conformant consumers that support the element. 4025 */ 4026 public int getMaxLength() { 4027 return this.maxLength == null || this.maxLength.isEmpty() ? 0 : this.maxLength.getValue(); 4028 } 4029 4030 /** 4031 * @param value Indicates the maximum length in characters that is permitted to 4032 * be present in conformant instances and which is expected to be 4033 * supported by conformant consumers that support the element. 4034 */ 4035 public ElementDefinition setMaxLength(int value) { 4036 if (this.maxLength == null) 4037 this.maxLength = new IntegerType(); 4038 this.maxLength.setValue(value); 4039 return this; 4040 } 4041 4042 /** 4043 * @return {@link #condition} (A reference to an invariant that may make 4044 * additional statements about the cardinality or value in the 4045 * instance.) 4046 */ 4047 public List<IdType> getCondition() { 4048 if (this.condition == null) 4049 this.condition = new ArrayList<IdType>(); 4050 return this.condition; 4051 } 4052 4053 public boolean hasCondition() { 4054 if (this.condition == null) 4055 return false; 4056 for (IdType item : this.condition) 4057 if (!item.isEmpty()) 4058 return true; 4059 return false; 4060 } 4061 4062 /** 4063 * @return {@link #condition} (A reference to an invariant that may make 4064 * additional statements about the cardinality or value in the 4065 * instance.) 4066 */ 4067 // syntactic sugar 4068 public IdType addConditionElement() {// 2 4069 IdType t = new IdType(); 4070 if (this.condition == null) 4071 this.condition = new ArrayList<IdType>(); 4072 this.condition.add(t); 4073 return t; 4074 } 4075 4076 /** 4077 * @param value {@link #condition} (A reference to an invariant that may make 4078 * additional statements about the cardinality or value in the 4079 * instance.) 4080 */ 4081 public ElementDefinition addCondition(String value) { // 1 4082 IdType t = new IdType(); 4083 t.setValue(value); 4084 if (this.condition == null) 4085 this.condition = new ArrayList<IdType>(); 4086 this.condition.add(t); 4087 return this; 4088 } 4089 4090 /** 4091 * @param value {@link #condition} (A reference to an invariant that may make 4092 * additional statements about the cardinality or value in the 4093 * instance.) 4094 */ 4095 public boolean hasCondition(String value) { 4096 if (this.condition == null) 4097 return false; 4098 for (IdType v : this.condition) 4099 if (v.equals(value)) // id 4100 return true; 4101 return false; 4102 } 4103 4104 /** 4105 * @return {@link #constraint} (Formal constraints such as co-occurrence and 4106 * other constraints that can be computationally evaluated within the 4107 * context of the instance.) 4108 */ 4109 public List<ElementDefinitionConstraintComponent> getConstraint() { 4110 if (this.constraint == null) 4111 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 4112 return this.constraint; 4113 } 4114 4115 public boolean hasConstraint() { 4116 if (this.constraint == null) 4117 return false; 4118 for (ElementDefinitionConstraintComponent item : this.constraint) 4119 if (!item.isEmpty()) 4120 return true; 4121 return false; 4122 } 4123 4124 /** 4125 * @return {@link #constraint} (Formal constraints such as co-occurrence and 4126 * other constraints that can be computationally evaluated within the 4127 * context of the instance.) 4128 */ 4129 // syntactic sugar 4130 public ElementDefinitionConstraintComponent addConstraint() { // 3 4131 ElementDefinitionConstraintComponent t = new ElementDefinitionConstraintComponent(); 4132 if (this.constraint == null) 4133 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 4134 this.constraint.add(t); 4135 return t; 4136 } 4137 4138 // syntactic sugar 4139 public ElementDefinition addConstraint(ElementDefinitionConstraintComponent t) { // 3 4140 if (t == null) 4141 return this; 4142 if (this.constraint == null) 4143 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 4144 this.constraint.add(t); 4145 return this; 4146 } 4147 4148 /** 4149 * @return {@link #mustSupport} (If true, implementations that produce or 4150 * consume resources SHALL provide "support" for the element in some 4151 * meaningful way. If false, the element may be ignored and not 4152 * supported.). This is the underlying object with id, value and 4153 * extensions. The accessor "getMustSupport" gives direct access to the 4154 * value 4155 */ 4156 public BooleanType getMustSupportElement() { 4157 if (this.mustSupport == null) 4158 if (Configuration.errorOnAutoCreate()) 4159 throw new Error("Attempt to auto-create ElementDefinition.mustSupport"); 4160 else if (Configuration.doAutoCreate()) 4161 this.mustSupport = new BooleanType(); // bb 4162 return this.mustSupport; 4163 } 4164 4165 public boolean hasMustSupportElement() { 4166 return this.mustSupport != null && !this.mustSupport.isEmpty(); 4167 } 4168 4169 public boolean hasMustSupport() { 4170 return this.mustSupport != null && !this.mustSupport.isEmpty(); 4171 } 4172 4173 /** 4174 * @param value {@link #mustSupport} (If true, implementations that produce or 4175 * consume resources SHALL provide "support" for the element in 4176 * some meaningful way. If false, the element may be ignored and 4177 * not supported.). This is the underlying object with id, value 4178 * and extensions. The accessor "getMustSupport" gives direct 4179 * access to the value 4180 */ 4181 public ElementDefinition setMustSupportElement(BooleanType value) { 4182 this.mustSupport = value; 4183 return this; 4184 } 4185 4186 /** 4187 * @return If true, implementations that produce or consume resources SHALL 4188 * provide "support" for the element in some meaningful way. If false, 4189 * the element may be ignored and not supported. 4190 */ 4191 public boolean getMustSupport() { 4192 return this.mustSupport == null || this.mustSupport.isEmpty() ? false : this.mustSupport.getValue(); 4193 } 4194 4195 /** 4196 * @param value If true, implementations that produce or consume resources SHALL 4197 * provide "support" for the element in some meaningful way. If 4198 * false, the element may be ignored and not supported. 4199 */ 4200 public ElementDefinition setMustSupport(boolean value) { 4201 if (this.mustSupport == null) 4202 this.mustSupport = new BooleanType(); 4203 this.mustSupport.setValue(value); 4204 return this; 4205 } 4206 4207 /** 4208 * @return {@link #isModifier} (If true, the value of this element affects the 4209 * interpretation of the element or resource that contains it, and the 4210 * value of the element cannot be ignored. Typically, this is used for 4211 * status, negation and qualification codes. The effect of this is that 4212 * the element cannot be ignored by systems: they SHALL either recognize 4213 * the element and process it, and/or a pre-determination has been made 4214 * that it is not relevant to their particular system.). This is the 4215 * underlying object with id, value and extensions. The accessor 4216 * "getIsModifier" gives direct access to the value 4217 */ 4218 public BooleanType getIsModifierElement() { 4219 if (this.isModifier == null) 4220 if (Configuration.errorOnAutoCreate()) 4221 throw new Error("Attempt to auto-create ElementDefinition.isModifier"); 4222 else if (Configuration.doAutoCreate()) 4223 this.isModifier = new BooleanType(); // bb 4224 return this.isModifier; 4225 } 4226 4227 public boolean hasIsModifierElement() { 4228 return this.isModifier != null && !this.isModifier.isEmpty(); 4229 } 4230 4231 public boolean hasIsModifier() { 4232 return this.isModifier != null && !this.isModifier.isEmpty(); 4233 } 4234 4235 /** 4236 * @param value {@link #isModifier} (If true, the value of this element affects 4237 * the interpretation of the element or resource that contains it, 4238 * and the value of the element cannot be ignored. Typically, this 4239 * is used for status, negation and qualification codes. The effect 4240 * of this is that the element cannot be ignored by systems: they 4241 * SHALL either recognize the element and process it, and/or a 4242 * pre-determination has been made that it is not relevant to their 4243 * particular system.). This is the underlying object with id, 4244 * value and extensions. The accessor "getIsModifier" gives direct 4245 * access to the value 4246 */ 4247 public ElementDefinition setIsModifierElement(BooleanType value) { 4248 this.isModifier = value; 4249 return this; 4250 } 4251 4252 /** 4253 * @return If true, the value of this element affects the interpretation of the 4254 * element or resource that contains it, and the value of the element 4255 * cannot be ignored. Typically, this is used for status, negation and 4256 * qualification codes. The effect of this is that the element cannot be 4257 * ignored by systems: they SHALL either recognize the element and 4258 * process it, and/or a pre-determination has been made that it is not 4259 * relevant to their particular system. 4260 */ 4261 public boolean getIsModifier() { 4262 return this.isModifier == null || this.isModifier.isEmpty() ? false : this.isModifier.getValue(); 4263 } 4264 4265 /** 4266 * @param value If true, the value of this element affects the interpretation of 4267 * the element or resource that contains it, and the value of the 4268 * element cannot be ignored. Typically, this is used for status, 4269 * negation and qualification codes. The effect of this is that the 4270 * element cannot be ignored by systems: they SHALL either 4271 * recognize the element and process it, and/or a pre-determination 4272 * has been made that it is not relevant to their particular 4273 * system. 4274 */ 4275 public ElementDefinition setIsModifier(boolean value) { 4276 if (this.isModifier == null) 4277 this.isModifier = new BooleanType(); 4278 this.isModifier.setValue(value); 4279 return this; 4280 } 4281 4282 /** 4283 * @return {@link #isSummary} (Whether the element should be included if a 4284 * client requests a search with the parameter _summary=true.). This is 4285 * the underlying object with id, value and extensions. The accessor 4286 * "getIsSummary" gives direct access to the value 4287 */ 4288 public BooleanType getIsSummaryElement() { 4289 if (this.isSummary == null) 4290 if (Configuration.errorOnAutoCreate()) 4291 throw new Error("Attempt to auto-create ElementDefinition.isSummary"); 4292 else if (Configuration.doAutoCreate()) 4293 this.isSummary = new BooleanType(); // bb 4294 return this.isSummary; 4295 } 4296 4297 public boolean hasIsSummaryElement() { 4298 return this.isSummary != null && !this.isSummary.isEmpty(); 4299 } 4300 4301 public boolean hasIsSummary() { 4302 return this.isSummary != null && !this.isSummary.isEmpty(); 4303 } 4304 4305 /** 4306 * @param value {@link #isSummary} (Whether the element should be included if a 4307 * client requests a search with the parameter _summary=true.). 4308 * This is the underlying object with id, value and extensions. The 4309 * accessor "getIsSummary" gives direct access to the value 4310 */ 4311 public ElementDefinition setIsSummaryElement(BooleanType value) { 4312 this.isSummary = value; 4313 return this; 4314 } 4315 4316 /** 4317 * @return Whether the element should be included if a client requests a search 4318 * with the parameter _summary=true. 4319 */ 4320 public boolean getIsSummary() { 4321 return this.isSummary == null || this.isSummary.isEmpty() ? false : this.isSummary.getValue(); 4322 } 4323 4324 /** 4325 * @param value Whether the element should be included if a client requests a 4326 * search with the parameter _summary=true. 4327 */ 4328 public ElementDefinition setIsSummary(boolean value) { 4329 if (this.isSummary == null) 4330 this.isSummary = new BooleanType(); 4331 this.isSummary.setValue(value); 4332 return this; 4333 } 4334 4335 /** 4336 * @return {@link #binding} (Binds to a value set if this element is coded 4337 * (code, Coding, CodeableConcept).) 4338 */ 4339 public ElementDefinitionBindingComponent getBinding() { 4340 if (this.binding == null) 4341 if (Configuration.errorOnAutoCreate()) 4342 throw new Error("Attempt to auto-create ElementDefinition.binding"); 4343 else if (Configuration.doAutoCreate()) 4344 this.binding = new ElementDefinitionBindingComponent(); // cc 4345 return this.binding; 4346 } 4347 4348 public boolean hasBinding() { 4349 return this.binding != null && !this.binding.isEmpty(); 4350 } 4351 4352 /** 4353 * @param value {@link #binding} (Binds to a value set if this element is coded 4354 * (code, Coding, CodeableConcept).) 4355 */ 4356 public ElementDefinition setBinding(ElementDefinitionBindingComponent value) { 4357 this.binding = value; 4358 return this; 4359 } 4360 4361 /** 4362 * @return {@link #mapping} (Identifies a concept from an external specification 4363 * that roughly corresponds to this element.) 4364 */ 4365 public List<ElementDefinitionMappingComponent> getMapping() { 4366 if (this.mapping == null) 4367 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 4368 return this.mapping; 4369 } 4370 4371 public boolean hasMapping() { 4372 if (this.mapping == null) 4373 return false; 4374 for (ElementDefinitionMappingComponent item : this.mapping) 4375 if (!item.isEmpty()) 4376 return true; 4377 return false; 4378 } 4379 4380 /** 4381 * @return {@link #mapping} (Identifies a concept from an external specification 4382 * that roughly corresponds to this element.) 4383 */ 4384 // syntactic sugar 4385 public ElementDefinitionMappingComponent addMapping() { // 3 4386 ElementDefinitionMappingComponent t = new ElementDefinitionMappingComponent(); 4387 if (this.mapping == null) 4388 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 4389 this.mapping.add(t); 4390 return t; 4391 } 4392 4393 // syntactic sugar 4394 public ElementDefinition addMapping(ElementDefinitionMappingComponent t) { // 3 4395 if (t == null) 4396 return this; 4397 if (this.mapping == null) 4398 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 4399 this.mapping.add(t); 4400 return this; 4401 } 4402 4403 protected void listChildren(List<Property> childrenList) { 4404 super.listChildren(childrenList); 4405 childrenList.add(new Property("path", "string", 4406 "The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension.", 4407 0, java.lang.Integer.MAX_VALUE, path)); 4408 childrenList.add(new Property("representation", "code", 4409 "Codes that define how this element is represented in instances, when the deviation varies from the normal case.", 4410 0, java.lang.Integer.MAX_VALUE, representation)); 4411 childrenList.add(new Property("name", "string", 4412 "The name of this element definition (to refer to it from other element definitions using ElementDefinition.nameReference). This is a unique name referring to a specific set of constraints applied to this element. One use of this is to provide a name to different slices of the same element.", 4413 0, java.lang.Integer.MAX_VALUE, name)); 4414 childrenList.add(new Property("label", "string", 4415 "The text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.", 4416 0, java.lang.Integer.MAX_VALUE, label)); 4417 childrenList.add(new Property("code", "Coding", 4418 "A code that provides the meaning for the element according to a particular terminology.", 0, 4419 java.lang.Integer.MAX_VALUE, code)); 4420 childrenList.add(new Property("slicing", "", 4421 "Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).", 4422 0, java.lang.Integer.MAX_VALUE, slicing)); 4423 childrenList.add(new Property("short", "string", 4424 "A concise description of what this element means (e.g. for use in autogenerated summaries).", 0, 4425 java.lang.Integer.MAX_VALUE, short_)); 4426 childrenList.add(new Property("definition", "markdown", 4427 "Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource.", 4428 0, java.lang.Integer.MAX_VALUE, definition)); 4429 childrenList.add(new Property("comments", "markdown", 4430 "Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc.", 4431 0, java.lang.Integer.MAX_VALUE, comments)); 4432 childrenList.add(new Property("requirements", "markdown", 4433 "This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.", 4434 0, java.lang.Integer.MAX_VALUE, requirements)); 4435 childrenList 4436 .add(new Property("alias", "string", "Identifies additional names by which this element might also be known.", 4437 0, java.lang.Integer.MAX_VALUE, alias)); 4438 childrenList.add(new Property("min", "integer", 4439 "The minimum number of times this element SHALL appear in the instance.", 0, java.lang.Integer.MAX_VALUE, min)); 4440 childrenList.add(new Property("max", "string", 4441 "The maximum number of times this element is permitted to appear in the instance.", 0, 4442 java.lang.Integer.MAX_VALUE, max)); 4443 childrenList.add(new Property("base", "", 4444 "Information about the base definition of the element, provided to make it unncessary for tools to trace the deviation of the element through the derived and related profiles. This information is only provided where the element definition represents a constraint on another element definition, and must be present if there is a base element definition.", 4445 0, java.lang.Integer.MAX_VALUE, base)); 4446 childrenList 4447 .add(new Property("type", "", "The data type or resource that the value of this element is permitted to be.", 0, 4448 java.lang.Integer.MAX_VALUE, type)); 4449 childrenList.add(new Property("nameReference", "string", 4450 "Identifies the name of a slice defined elsewhere in the profile whose constraints should be applied to the current element.", 4451 0, java.lang.Integer.MAX_VALUE, nameReference)); 4452 childrenList.add(new Property("defaultValue[x]", "*", 4453 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 4454 0, java.lang.Integer.MAX_VALUE, defaultValue)); 4455 childrenList.add(new Property("meaningWhenMissing", "markdown", 4456 "The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'.", 4457 0, java.lang.Integer.MAX_VALUE, meaningWhenMissing)); 4458 childrenList.add(new Property("fixed[x]", "*", 4459 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 4460 0, java.lang.Integer.MAX_VALUE, fixed)); 4461 childrenList.add(new Property("pattern[x]", "*", 4462 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 4463 0, java.lang.Integer.MAX_VALUE, pattern)); 4464 childrenList.add(new Property("example[x]", "*", 4465 "A sample value for this element demonstrating the type of information that would typically be captured.", 0, 4466 java.lang.Integer.MAX_VALUE, example)); 4467 childrenList.add(new Property("minValue[x]", "*", 4468 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 4469 0, java.lang.Integer.MAX_VALUE, minValue)); 4470 childrenList.add(new Property("maxValue[x]", "*", 4471 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 4472 0, java.lang.Integer.MAX_VALUE, maxValue)); 4473 childrenList.add(new Property("maxLength", "integer", 4474 "Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element.", 4475 0, java.lang.Integer.MAX_VALUE, maxLength)); 4476 childrenList.add(new Property("condition", "id", 4477 "A reference to an invariant that may make additional statements about the cardinality or value in the instance.", 4478 0, java.lang.Integer.MAX_VALUE, condition)); 4479 childrenList.add(new Property("constraint", "", 4480 "Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.", 4481 0, java.lang.Integer.MAX_VALUE, constraint)); 4482 childrenList.add(new Property("mustSupport", "boolean", 4483 "If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. If false, the element may be ignored and not supported.", 4484 0, java.lang.Integer.MAX_VALUE, mustSupport)); 4485 childrenList.add(new Property("isModifier", "boolean", 4486 "If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system.", 4487 0, java.lang.Integer.MAX_VALUE, isModifier)); 4488 childrenList.add(new Property("isSummary", "boolean", 4489 "Whether the element should be included if a client requests a search with the parameter _summary=true.", 0, 4490 java.lang.Integer.MAX_VALUE, isSummary)); 4491 childrenList.add( 4492 new Property("binding", "", "Binds to a value set if this element is coded (code, Coding, CodeableConcept).", 0, 4493 java.lang.Integer.MAX_VALUE, binding)); 4494 childrenList.add(new Property("mapping", "", 4495 "Identifies a concept from an external specification that roughly corresponds to this element.", 0, 4496 java.lang.Integer.MAX_VALUE, mapping)); 4497 } 4498 4499 @Override 4500 public void setProperty(String name, Base value) throws FHIRException { 4501 if (name.equals("path")) 4502 this.path = castToString(value); // StringType 4503 else if (name.equals("representation")) 4504 this.getRepresentation().add(new PropertyRepresentationEnumFactory().fromType(value)); 4505 else if (name.equals("name")) 4506 this.name = castToString(value); // StringType 4507 else if (name.equals("label")) 4508 this.label = castToString(value); // StringType 4509 else if (name.equals("code")) 4510 this.getCode().add(castToCoding(value)); 4511 else if (name.equals("slicing")) 4512 this.slicing = (ElementDefinitionSlicingComponent) value; // ElementDefinitionSlicingComponent 4513 else if (name.equals("short")) 4514 this.short_ = castToString(value); // StringType 4515 else if (name.equals("definition")) 4516 this.definition = castToMarkdown(value); // MarkdownType 4517 else if (name.equals("comments")) 4518 this.comments = castToMarkdown(value); // MarkdownType 4519 else if (name.equals("requirements")) 4520 this.requirements = castToMarkdown(value); // MarkdownType 4521 else if (name.equals("alias")) 4522 this.getAlias().add(castToString(value)); 4523 else if (name.equals("min")) 4524 this.min = castToInteger(value); // IntegerType 4525 else if (name.equals("max")) 4526 this.max = castToString(value); // StringType 4527 else if (name.equals("base")) 4528 this.base = (ElementDefinitionBaseComponent) value; // ElementDefinitionBaseComponent 4529 else if (name.equals("type")) 4530 this.getType().add((TypeRefComponent) value); 4531 else if (name.equals("nameReference")) 4532 this.nameReference = castToString(value); // StringType 4533 else if (name.equals("defaultValue[x]")) 4534 this.defaultValue = (org.hl7.fhir.dstu2.model.Type) value; // org.hl7.fhir.dstu2.model.Type 4535 else if (name.equals("meaningWhenMissing")) 4536 this.meaningWhenMissing = castToMarkdown(value); // MarkdownType 4537 else if (name.equals("fixed[x]")) 4538 this.fixed = (org.hl7.fhir.dstu2.model.Type) value; // org.hl7.fhir.dstu2.model.Type 4539 else if (name.equals("pattern[x]")) 4540 this.pattern = (org.hl7.fhir.dstu2.model.Type) value; // org.hl7.fhir.dstu2.model.Type 4541 else if (name.equals("example[x]")) 4542 this.example = (org.hl7.fhir.dstu2.model.Type) value; // org.hl7.fhir.dstu2.model.Type 4543 else if (name.equals("minValue[x]")) 4544 this.minValue = (org.hl7.fhir.dstu2.model.Type) value; // org.hl7.fhir.dstu2.model.Type 4545 else if (name.equals("maxValue[x]")) 4546 this.maxValue = (org.hl7.fhir.dstu2.model.Type) value; // org.hl7.fhir.dstu2.model.Type 4547 else if (name.equals("maxLength")) 4548 this.maxLength = castToInteger(value); // IntegerType 4549 else if (name.equals("condition")) 4550 this.getCondition().add(castToId(value)); 4551 else if (name.equals("constraint")) 4552 this.getConstraint().add((ElementDefinitionConstraintComponent) value); 4553 else if (name.equals("mustSupport")) 4554 this.mustSupport = castToBoolean(value); // BooleanType 4555 else if (name.equals("isModifier")) 4556 this.isModifier = castToBoolean(value); // BooleanType 4557 else if (name.equals("isSummary")) 4558 this.isSummary = castToBoolean(value); // BooleanType 4559 else if (name.equals("binding")) 4560 this.binding = (ElementDefinitionBindingComponent) value; // ElementDefinitionBindingComponent 4561 else if (name.equals("mapping")) 4562 this.getMapping().add((ElementDefinitionMappingComponent) value); 4563 else 4564 super.setProperty(name, value); 4565 } 4566 4567 @Override 4568 public Base addChild(String name) throws FHIRException { 4569 if (name.equals("path")) { 4570 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.path"); 4571 } else if (name.equals("representation")) { 4572 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.representation"); 4573 } else if (name.equals("name")) { 4574 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.name"); 4575 } else if (name.equals("label")) { 4576 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.label"); 4577 } else if (name.equals("code")) { 4578 return addCode(); 4579 } else if (name.equals("slicing")) { 4580 this.slicing = new ElementDefinitionSlicingComponent(); 4581 return this.slicing; 4582 } else if (name.equals("short")) { 4583 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.short"); 4584 } else if (name.equals("definition")) { 4585 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.definition"); 4586 } else if (name.equals("comments")) { 4587 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.comments"); 4588 } else if (name.equals("requirements")) { 4589 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.requirements"); 4590 } else if (name.equals("alias")) { 4591 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.alias"); 4592 } else if (name.equals("min")) { 4593 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.min"); 4594 } else if (name.equals("max")) { 4595 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.max"); 4596 } else if (name.equals("base")) { 4597 this.base = new ElementDefinitionBaseComponent(); 4598 return this.base; 4599 } else if (name.equals("type")) { 4600 return addType(); 4601 } else if (name.equals("nameReference")) { 4602 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.nameReference"); 4603 } else if (name.equals("defaultValueBoolean")) { 4604 this.defaultValue = new BooleanType(); 4605 return this.defaultValue; 4606 } else if (name.equals("defaultValueInteger")) { 4607 this.defaultValue = new IntegerType(); 4608 return this.defaultValue; 4609 } else if (name.equals("defaultValueDecimal")) { 4610 this.defaultValue = new DecimalType(); 4611 return this.defaultValue; 4612 } else if (name.equals("defaultValueBase64Binary")) { 4613 this.defaultValue = new Base64BinaryType(); 4614 return this.defaultValue; 4615 } else if (name.equals("defaultValueInstant")) { 4616 this.defaultValue = new InstantType(); 4617 return this.defaultValue; 4618 } else if (name.equals("defaultValueString")) { 4619 this.defaultValue = new StringType(); 4620 return this.defaultValue; 4621 } else if (name.equals("defaultValueUri")) { 4622 this.defaultValue = new UriType(); 4623 return this.defaultValue; 4624 } else if (name.equals("defaultValueDate")) { 4625 this.defaultValue = new DateType(); 4626 return this.defaultValue; 4627 } else if (name.equals("defaultValueDateTime")) { 4628 this.defaultValue = new DateTimeType(); 4629 return this.defaultValue; 4630 } else if (name.equals("defaultValueTime")) { 4631 this.defaultValue = new TimeType(); 4632 return this.defaultValue; 4633 } else if (name.equals("defaultValueCode")) { 4634 this.defaultValue = new CodeType(); 4635 return this.defaultValue; 4636 } else if (name.equals("defaultValueOid")) { 4637 this.defaultValue = new OidType(); 4638 return this.defaultValue; 4639 } else if (name.equals("defaultValueId")) { 4640 this.defaultValue = new IdType(); 4641 return this.defaultValue; 4642 } else if (name.equals("defaultValueUnsignedInt")) { 4643 this.defaultValue = new UnsignedIntType(); 4644 return this.defaultValue; 4645 } else if (name.equals("defaultValuePositiveInt")) { 4646 this.defaultValue = new PositiveIntType(); 4647 return this.defaultValue; 4648 } else if (name.equals("defaultValueMarkdown")) { 4649 this.defaultValue = new MarkdownType(); 4650 return this.defaultValue; 4651 } else if (name.equals("defaultValueAnnotation")) { 4652 this.defaultValue = new Annotation(); 4653 return this.defaultValue; 4654 } else if (name.equals("defaultValueAttachment")) { 4655 this.defaultValue = new Attachment(); 4656 return this.defaultValue; 4657 } else if (name.equals("defaultValueIdentifier")) { 4658 this.defaultValue = new Identifier(); 4659 return this.defaultValue; 4660 } else if (name.equals("defaultValueCodeableConcept")) { 4661 this.defaultValue = new CodeableConcept(); 4662 return this.defaultValue; 4663 } else if (name.equals("defaultValueCoding")) { 4664 this.defaultValue = new Coding(); 4665 return this.defaultValue; 4666 } else if (name.equals("defaultValueQuantity")) { 4667 this.defaultValue = new Quantity(); 4668 return this.defaultValue; 4669 } else if (name.equals("defaultValueRange")) { 4670 this.defaultValue = new Range(); 4671 return this.defaultValue; 4672 } else if (name.equals("defaultValuePeriod")) { 4673 this.defaultValue = new Period(); 4674 return this.defaultValue; 4675 } else if (name.equals("defaultValueRatio")) { 4676 this.defaultValue = new Ratio(); 4677 return this.defaultValue; 4678 } else if (name.equals("defaultValueSampledData")) { 4679 this.defaultValue = new SampledData(); 4680 return this.defaultValue; 4681 } else if (name.equals("defaultValueSignature")) { 4682 this.defaultValue = new Signature(); 4683 return this.defaultValue; 4684 } else if (name.equals("defaultValueHumanName")) { 4685 this.defaultValue = new HumanName(); 4686 return this.defaultValue; 4687 } else if (name.equals("defaultValueAddress")) { 4688 this.defaultValue = new Address(); 4689 return this.defaultValue; 4690 } else if (name.equals("defaultValueContactPoint")) { 4691 this.defaultValue = new ContactPoint(); 4692 return this.defaultValue; 4693 } else if (name.equals("defaultValueTiming")) { 4694 this.defaultValue = new Timing(); 4695 return this.defaultValue; 4696 } else if (name.equals("defaultValueReference")) { 4697 this.defaultValue = new Reference(); 4698 return this.defaultValue; 4699 } else if (name.equals("defaultValueMeta")) { 4700 this.defaultValue = new Meta(); 4701 return this.defaultValue; 4702 } else if (name.equals("meaningWhenMissing")) { 4703 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.meaningWhenMissing"); 4704 } else if (name.equals("fixedBoolean")) { 4705 this.fixed = new BooleanType(); 4706 return this.fixed; 4707 } else if (name.equals("fixedInteger")) { 4708 this.fixed = new IntegerType(); 4709 return this.fixed; 4710 } else if (name.equals("fixedDecimal")) { 4711 this.fixed = new DecimalType(); 4712 return this.fixed; 4713 } else if (name.equals("fixedBase64Binary")) { 4714 this.fixed = new Base64BinaryType(); 4715 return this.fixed; 4716 } else if (name.equals("fixedInstant")) { 4717 this.fixed = new InstantType(); 4718 return this.fixed; 4719 } else if (name.equals("fixedString")) { 4720 this.fixed = new StringType(); 4721 return this.fixed; 4722 } else if (name.equals("fixedUri")) { 4723 this.fixed = new UriType(); 4724 return this.fixed; 4725 } else if (name.equals("fixedDate")) { 4726 this.fixed = new DateType(); 4727 return this.fixed; 4728 } else if (name.equals("fixedDateTime")) { 4729 this.fixed = new DateTimeType(); 4730 return this.fixed; 4731 } else if (name.equals("fixedTime")) { 4732 this.fixed = new TimeType(); 4733 return this.fixed; 4734 } else if (name.equals("fixedCode")) { 4735 this.fixed = new CodeType(); 4736 return this.fixed; 4737 } else if (name.equals("fixedOid")) { 4738 this.fixed = new OidType(); 4739 return this.fixed; 4740 } else if (name.equals("fixedId")) { 4741 this.fixed = new IdType(); 4742 return this.fixed; 4743 } else if (name.equals("fixedUnsignedInt")) { 4744 this.fixed = new UnsignedIntType(); 4745 return this.fixed; 4746 } else if (name.equals("fixedPositiveInt")) { 4747 this.fixed = new PositiveIntType(); 4748 return this.fixed; 4749 } else if (name.equals("fixedMarkdown")) { 4750 this.fixed = new MarkdownType(); 4751 return this.fixed; 4752 } else if (name.equals("fixedAnnotation")) { 4753 this.fixed = new Annotation(); 4754 return this.fixed; 4755 } else if (name.equals("fixedAttachment")) { 4756 this.fixed = new Attachment(); 4757 return this.fixed; 4758 } else if (name.equals("fixedIdentifier")) { 4759 this.fixed = new Identifier(); 4760 return this.fixed; 4761 } else if (name.equals("fixedCodeableConcept")) { 4762 this.fixed = new CodeableConcept(); 4763 return this.fixed; 4764 } else if (name.equals("fixedCoding")) { 4765 this.fixed = new Coding(); 4766 return this.fixed; 4767 } else if (name.equals("fixedQuantity")) { 4768 this.fixed = new Quantity(); 4769 return this.fixed; 4770 } else if (name.equals("fixedRange")) { 4771 this.fixed = new Range(); 4772 return this.fixed; 4773 } else if (name.equals("fixedPeriod")) { 4774 this.fixed = new Period(); 4775 return this.fixed; 4776 } else if (name.equals("fixedRatio")) { 4777 this.fixed = new Ratio(); 4778 return this.fixed; 4779 } else if (name.equals("fixedSampledData")) { 4780 this.fixed = new SampledData(); 4781 return this.fixed; 4782 } else if (name.equals("fixedSignature")) { 4783 this.fixed = new Signature(); 4784 return this.fixed; 4785 } else if (name.equals("fixedHumanName")) { 4786 this.fixed = new HumanName(); 4787 return this.fixed; 4788 } else if (name.equals("fixedAddress")) { 4789 this.fixed = new Address(); 4790 return this.fixed; 4791 } else if (name.equals("fixedContactPoint")) { 4792 this.fixed = new ContactPoint(); 4793 return this.fixed; 4794 } else if (name.equals("fixedTiming")) { 4795 this.fixed = new Timing(); 4796 return this.fixed; 4797 } else if (name.equals("fixedReference")) { 4798 this.fixed = new Reference(); 4799 return this.fixed; 4800 } else if (name.equals("fixedMeta")) { 4801 this.fixed = new Meta(); 4802 return this.fixed; 4803 } else if (name.equals("patternBoolean")) { 4804 this.pattern = new BooleanType(); 4805 return this.pattern; 4806 } else if (name.equals("patternInteger")) { 4807 this.pattern = new IntegerType(); 4808 return this.pattern; 4809 } else if (name.equals("patternDecimal")) { 4810 this.pattern = new DecimalType(); 4811 return this.pattern; 4812 } else if (name.equals("patternBase64Binary")) { 4813 this.pattern = new Base64BinaryType(); 4814 return this.pattern; 4815 } else if (name.equals("patternInstant")) { 4816 this.pattern = new InstantType(); 4817 return this.pattern; 4818 } else if (name.equals("patternString")) { 4819 this.pattern = new StringType(); 4820 return this.pattern; 4821 } else if (name.equals("patternUri")) { 4822 this.pattern = new UriType(); 4823 return this.pattern; 4824 } else if (name.equals("patternDate")) { 4825 this.pattern = new DateType(); 4826 return this.pattern; 4827 } else if (name.equals("patternDateTime")) { 4828 this.pattern = new DateTimeType(); 4829 return this.pattern; 4830 } else if (name.equals("patternTime")) { 4831 this.pattern = new TimeType(); 4832 return this.pattern; 4833 } else if (name.equals("patternCode")) { 4834 this.pattern = new CodeType(); 4835 return this.pattern; 4836 } else if (name.equals("patternOid")) { 4837 this.pattern = new OidType(); 4838 return this.pattern; 4839 } else if (name.equals("patternId")) { 4840 this.pattern = new IdType(); 4841 return this.pattern; 4842 } else if (name.equals("patternUnsignedInt")) { 4843 this.pattern = new UnsignedIntType(); 4844 return this.pattern; 4845 } else if (name.equals("patternPositiveInt")) { 4846 this.pattern = new PositiveIntType(); 4847 return this.pattern; 4848 } else if (name.equals("patternMarkdown")) { 4849 this.pattern = new MarkdownType(); 4850 return this.pattern; 4851 } else if (name.equals("patternAnnotation")) { 4852 this.pattern = new Annotation(); 4853 return this.pattern; 4854 } else if (name.equals("patternAttachment")) { 4855 this.pattern = new Attachment(); 4856 return this.pattern; 4857 } else if (name.equals("patternIdentifier")) { 4858 this.pattern = new Identifier(); 4859 return this.pattern; 4860 } else if (name.equals("patternCodeableConcept")) { 4861 this.pattern = new CodeableConcept(); 4862 return this.pattern; 4863 } else if (name.equals("patternCoding")) { 4864 this.pattern = new Coding(); 4865 return this.pattern; 4866 } else if (name.equals("patternQuantity")) { 4867 this.pattern = new Quantity(); 4868 return this.pattern; 4869 } else if (name.equals("patternRange")) { 4870 this.pattern = new Range(); 4871 return this.pattern; 4872 } else if (name.equals("patternPeriod")) { 4873 this.pattern = new Period(); 4874 return this.pattern; 4875 } else if (name.equals("patternRatio")) { 4876 this.pattern = new Ratio(); 4877 return this.pattern; 4878 } else if (name.equals("patternSampledData")) { 4879 this.pattern = new SampledData(); 4880 return this.pattern; 4881 } else if (name.equals("patternSignature")) { 4882 this.pattern = new Signature(); 4883 return this.pattern; 4884 } else if (name.equals("patternHumanName")) { 4885 this.pattern = new HumanName(); 4886 return this.pattern; 4887 } else if (name.equals("patternAddress")) { 4888 this.pattern = new Address(); 4889 return this.pattern; 4890 } else if (name.equals("patternContactPoint")) { 4891 this.pattern = new ContactPoint(); 4892 return this.pattern; 4893 } else if (name.equals("patternTiming")) { 4894 this.pattern = new Timing(); 4895 return this.pattern; 4896 } else if (name.equals("patternReference")) { 4897 this.pattern = new Reference(); 4898 return this.pattern; 4899 } else if (name.equals("patternMeta")) { 4900 this.pattern = new Meta(); 4901 return this.pattern; 4902 } else if (name.equals("exampleBoolean")) { 4903 this.example = new BooleanType(); 4904 return this.example; 4905 } else if (name.equals("exampleInteger")) { 4906 this.example = new IntegerType(); 4907 return this.example; 4908 } else if (name.equals("exampleDecimal")) { 4909 this.example = new DecimalType(); 4910 return this.example; 4911 } else if (name.equals("exampleBase64Binary")) { 4912 this.example = new Base64BinaryType(); 4913 return this.example; 4914 } else if (name.equals("exampleInstant")) { 4915 this.example = new InstantType(); 4916 return this.example; 4917 } else if (name.equals("exampleString")) { 4918 this.example = new StringType(); 4919 return this.example; 4920 } else if (name.equals("exampleUri")) { 4921 this.example = new UriType(); 4922 return this.example; 4923 } else if (name.equals("exampleDate")) { 4924 this.example = new DateType(); 4925 return this.example; 4926 } else if (name.equals("exampleDateTime")) { 4927 this.example = new DateTimeType(); 4928 return this.example; 4929 } else if (name.equals("exampleTime")) { 4930 this.example = new TimeType(); 4931 return this.example; 4932 } else if (name.equals("exampleCode")) { 4933 this.example = new CodeType(); 4934 return this.example; 4935 } else if (name.equals("exampleOid")) { 4936 this.example = new OidType(); 4937 return this.example; 4938 } else if (name.equals("exampleId")) { 4939 this.example = new IdType(); 4940 return this.example; 4941 } else if (name.equals("exampleUnsignedInt")) { 4942 this.example = new UnsignedIntType(); 4943 return this.example; 4944 } else if (name.equals("examplePositiveInt")) { 4945 this.example = new PositiveIntType(); 4946 return this.example; 4947 } else if (name.equals("exampleMarkdown")) { 4948 this.example = new MarkdownType(); 4949 return this.example; 4950 } else if (name.equals("exampleAnnotation")) { 4951 this.example = new Annotation(); 4952 return this.example; 4953 } else if (name.equals("exampleAttachment")) { 4954 this.example = new Attachment(); 4955 return this.example; 4956 } else if (name.equals("exampleIdentifier")) { 4957 this.example = new Identifier(); 4958 return this.example; 4959 } else if (name.equals("exampleCodeableConcept")) { 4960 this.example = new CodeableConcept(); 4961 return this.example; 4962 } else if (name.equals("exampleCoding")) { 4963 this.example = new Coding(); 4964 return this.example; 4965 } else if (name.equals("exampleQuantity")) { 4966 this.example = new Quantity(); 4967 return this.example; 4968 } else if (name.equals("exampleRange")) { 4969 this.example = new Range(); 4970 return this.example; 4971 } else if (name.equals("examplePeriod")) { 4972 this.example = new Period(); 4973 return this.example; 4974 } else if (name.equals("exampleRatio")) { 4975 this.example = new Ratio(); 4976 return this.example; 4977 } else if (name.equals("exampleSampledData")) { 4978 this.example = new SampledData(); 4979 return this.example; 4980 } else if (name.equals("exampleSignature")) { 4981 this.example = new Signature(); 4982 return this.example; 4983 } else if (name.equals("exampleHumanName")) { 4984 this.example = new HumanName(); 4985 return this.example; 4986 } else if (name.equals("exampleAddress")) { 4987 this.example = new Address(); 4988 return this.example; 4989 } else if (name.equals("exampleContactPoint")) { 4990 this.example = new ContactPoint(); 4991 return this.example; 4992 } else if (name.equals("exampleTiming")) { 4993 this.example = new Timing(); 4994 return this.example; 4995 } else if (name.equals("exampleReference")) { 4996 this.example = new Reference(); 4997 return this.example; 4998 } else if (name.equals("exampleMeta")) { 4999 this.example = new Meta(); 5000 return this.example; 5001 } else if (name.equals("minValueBoolean")) { 5002 this.minValue = new BooleanType(); 5003 return this.minValue; 5004 } else if (name.equals("minValueInteger")) { 5005 this.minValue = new IntegerType(); 5006 return this.minValue; 5007 } else if (name.equals("minValueDecimal")) { 5008 this.minValue = new DecimalType(); 5009 return this.minValue; 5010 } else if (name.equals("minValueBase64Binary")) { 5011 this.minValue = new Base64BinaryType(); 5012 return this.minValue; 5013 } else if (name.equals("minValueInstant")) { 5014 this.minValue = new InstantType(); 5015 return this.minValue; 5016 } else if (name.equals("minValueString")) { 5017 this.minValue = new StringType(); 5018 return this.minValue; 5019 } else if (name.equals("minValueUri")) { 5020 this.minValue = new UriType(); 5021 return this.minValue; 5022 } else if (name.equals("minValueDate")) { 5023 this.minValue = new DateType(); 5024 return this.minValue; 5025 } else if (name.equals("minValueDateTime")) { 5026 this.minValue = new DateTimeType(); 5027 return this.minValue; 5028 } else if (name.equals("minValueTime")) { 5029 this.minValue = new TimeType(); 5030 return this.minValue; 5031 } else if (name.equals("minValueCode")) { 5032 this.minValue = new CodeType(); 5033 return this.minValue; 5034 } else if (name.equals("minValueOid")) { 5035 this.minValue = new OidType(); 5036 return this.minValue; 5037 } else if (name.equals("minValueId")) { 5038 this.minValue = new IdType(); 5039 return this.minValue; 5040 } else if (name.equals("minValueUnsignedInt")) { 5041 this.minValue = new UnsignedIntType(); 5042 return this.minValue; 5043 } else if (name.equals("minValuePositiveInt")) { 5044 this.minValue = new PositiveIntType(); 5045 return this.minValue; 5046 } else if (name.equals("minValueMarkdown")) { 5047 this.minValue = new MarkdownType(); 5048 return this.minValue; 5049 } else if (name.equals("minValueAnnotation")) { 5050 this.minValue = new Annotation(); 5051 return this.minValue; 5052 } else if (name.equals("minValueAttachment")) { 5053 this.minValue = new Attachment(); 5054 return this.minValue; 5055 } else if (name.equals("minValueIdentifier")) { 5056 this.minValue = new Identifier(); 5057 return this.minValue; 5058 } else if (name.equals("minValueCodeableConcept")) { 5059 this.minValue = new CodeableConcept(); 5060 return this.minValue; 5061 } else if (name.equals("minValueCoding")) { 5062 this.minValue = new Coding(); 5063 return this.minValue; 5064 } else if (name.equals("minValueQuantity")) { 5065 this.minValue = new Quantity(); 5066 return this.minValue; 5067 } else if (name.equals("minValueRange")) { 5068 this.minValue = new Range(); 5069 return this.minValue; 5070 } else if (name.equals("minValuePeriod")) { 5071 this.minValue = new Period(); 5072 return this.minValue; 5073 } else if (name.equals("minValueRatio")) { 5074 this.minValue = new Ratio(); 5075 return this.minValue; 5076 } else if (name.equals("minValueSampledData")) { 5077 this.minValue = new SampledData(); 5078 return this.minValue; 5079 } else if (name.equals("minValueSignature")) { 5080 this.minValue = new Signature(); 5081 return this.minValue; 5082 } else if (name.equals("minValueHumanName")) { 5083 this.minValue = new HumanName(); 5084 return this.minValue; 5085 } else if (name.equals("minValueAddress")) { 5086 this.minValue = new Address(); 5087 return this.minValue; 5088 } else if (name.equals("minValueContactPoint")) { 5089 this.minValue = new ContactPoint(); 5090 return this.minValue; 5091 } else if (name.equals("minValueTiming")) { 5092 this.minValue = new Timing(); 5093 return this.minValue; 5094 } else if (name.equals("minValueReference")) { 5095 this.minValue = new Reference(); 5096 return this.minValue; 5097 } else if (name.equals("minValueMeta")) { 5098 this.minValue = new Meta(); 5099 return this.minValue; 5100 } else if (name.equals("maxValueBoolean")) { 5101 this.maxValue = new BooleanType(); 5102 return this.maxValue; 5103 } else if (name.equals("maxValueInteger")) { 5104 this.maxValue = new IntegerType(); 5105 return this.maxValue; 5106 } else if (name.equals("maxValueDecimal")) { 5107 this.maxValue = new DecimalType(); 5108 return this.maxValue; 5109 } else if (name.equals("maxValueBase64Binary")) { 5110 this.maxValue = new Base64BinaryType(); 5111 return this.maxValue; 5112 } else if (name.equals("maxValueInstant")) { 5113 this.maxValue = new InstantType(); 5114 return this.maxValue; 5115 } else if (name.equals("maxValueString")) { 5116 this.maxValue = new StringType(); 5117 return this.maxValue; 5118 } else if (name.equals("maxValueUri")) { 5119 this.maxValue = new UriType(); 5120 return this.maxValue; 5121 } else if (name.equals("maxValueDate")) { 5122 this.maxValue = new DateType(); 5123 return this.maxValue; 5124 } else if (name.equals("maxValueDateTime")) { 5125 this.maxValue = new DateTimeType(); 5126 return this.maxValue; 5127 } else if (name.equals("maxValueTime")) { 5128 this.maxValue = new TimeType(); 5129 return this.maxValue; 5130 } else if (name.equals("maxValueCode")) { 5131 this.maxValue = new CodeType(); 5132 return this.maxValue; 5133 } else if (name.equals("maxValueOid")) { 5134 this.maxValue = new OidType(); 5135 return this.maxValue; 5136 } else if (name.equals("maxValueId")) { 5137 this.maxValue = new IdType(); 5138 return this.maxValue; 5139 } else if (name.equals("maxValueUnsignedInt")) { 5140 this.maxValue = new UnsignedIntType(); 5141 return this.maxValue; 5142 } else if (name.equals("maxValuePositiveInt")) { 5143 this.maxValue = new PositiveIntType(); 5144 return this.maxValue; 5145 } else if (name.equals("maxValueMarkdown")) { 5146 this.maxValue = new MarkdownType(); 5147 return this.maxValue; 5148 } else if (name.equals("maxValueAnnotation")) { 5149 this.maxValue = new Annotation(); 5150 return this.maxValue; 5151 } else if (name.equals("maxValueAttachment")) { 5152 this.maxValue = new Attachment(); 5153 return this.maxValue; 5154 } else if (name.equals("maxValueIdentifier")) { 5155 this.maxValue = new Identifier(); 5156 return this.maxValue; 5157 } else if (name.equals("maxValueCodeableConcept")) { 5158 this.maxValue = new CodeableConcept(); 5159 return this.maxValue; 5160 } else if (name.equals("maxValueCoding")) { 5161 this.maxValue = new Coding(); 5162 return this.maxValue; 5163 } else if (name.equals("maxValueQuantity")) { 5164 this.maxValue = new Quantity(); 5165 return this.maxValue; 5166 } else if (name.equals("maxValueRange")) { 5167 this.maxValue = new Range(); 5168 return this.maxValue; 5169 } else if (name.equals("maxValuePeriod")) { 5170 this.maxValue = new Period(); 5171 return this.maxValue; 5172 } else if (name.equals("maxValueRatio")) { 5173 this.maxValue = new Ratio(); 5174 return this.maxValue; 5175 } else if (name.equals("maxValueSampledData")) { 5176 this.maxValue = new SampledData(); 5177 return this.maxValue; 5178 } else if (name.equals("maxValueSignature")) { 5179 this.maxValue = new Signature(); 5180 return this.maxValue; 5181 } else if (name.equals("maxValueHumanName")) { 5182 this.maxValue = new HumanName(); 5183 return this.maxValue; 5184 } else if (name.equals("maxValueAddress")) { 5185 this.maxValue = new Address(); 5186 return this.maxValue; 5187 } else if (name.equals("maxValueContactPoint")) { 5188 this.maxValue = new ContactPoint(); 5189 return this.maxValue; 5190 } else if (name.equals("maxValueTiming")) { 5191 this.maxValue = new Timing(); 5192 return this.maxValue; 5193 } else if (name.equals("maxValueReference")) { 5194 this.maxValue = new Reference(); 5195 return this.maxValue; 5196 } else if (name.equals("maxValueMeta")) { 5197 this.maxValue = new Meta(); 5198 return this.maxValue; 5199 } else if (name.equals("maxLength")) { 5200 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.maxLength"); 5201 } else if (name.equals("condition")) { 5202 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.condition"); 5203 } else if (name.equals("constraint")) { 5204 return addConstraint(); 5205 } else if (name.equals("mustSupport")) { 5206 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.mustSupport"); 5207 } else if (name.equals("isModifier")) { 5208 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.isModifier"); 5209 } else if (name.equals("isSummary")) { 5210 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.isSummary"); 5211 } else if (name.equals("binding")) { 5212 this.binding = new ElementDefinitionBindingComponent(); 5213 return this.binding; 5214 } else if (name.equals("mapping")) { 5215 return addMapping(); 5216 } else 5217 return super.addChild(name); 5218 } 5219 5220 public String fhirType() { 5221 return "ElementDefinition"; 5222 5223 } 5224 5225 public ElementDefinition copy() { 5226 ElementDefinition dst = new ElementDefinition(); 5227 copyValues(dst); 5228 dst.path = path == null ? null : path.copy(); 5229 if (representation != null) { 5230 dst.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 5231 for (Enumeration<PropertyRepresentation> i : representation) 5232 dst.representation.add(i.copy()); 5233 } 5234 ; 5235 dst.name = name == null ? null : name.copy(); 5236 dst.label = label == null ? null : label.copy(); 5237 if (code != null) { 5238 dst.code = new ArrayList<Coding>(); 5239 for (Coding i : code) 5240 dst.code.add(i.copy()); 5241 } 5242 ; 5243 dst.slicing = slicing == null ? null : slicing.copy(); 5244 dst.short_ = short_ == null ? null : short_.copy(); 5245 dst.definition = definition == null ? null : definition.copy(); 5246 dst.comments = comments == null ? null : comments.copy(); 5247 dst.requirements = requirements == null ? null : requirements.copy(); 5248 if (alias != null) { 5249 dst.alias = new ArrayList<StringType>(); 5250 for (StringType i : alias) 5251 dst.alias.add(i.copy()); 5252 } 5253 ; 5254 dst.min = min == null ? null : min.copy(); 5255 dst.max = max == null ? null : max.copy(); 5256 dst.base = base == null ? null : base.copy(); 5257 if (type != null) { 5258 dst.type = new ArrayList<TypeRefComponent>(); 5259 for (TypeRefComponent i : type) 5260 dst.type.add(i.copy()); 5261 } 5262 ; 5263 dst.nameReference = nameReference == null ? null : nameReference.copy(); 5264 dst.defaultValue = defaultValue == null ? null : defaultValue.copy(); 5265 dst.meaningWhenMissing = meaningWhenMissing == null ? null : meaningWhenMissing.copy(); 5266 dst.fixed = fixed == null ? null : fixed.copy(); 5267 dst.pattern = pattern == null ? null : pattern.copy(); 5268 dst.example = example == null ? null : example.copy(); 5269 dst.minValue = minValue == null ? null : minValue.copy(); 5270 dst.maxValue = maxValue == null ? null : maxValue.copy(); 5271 dst.maxLength = maxLength == null ? null : maxLength.copy(); 5272 if (condition != null) { 5273 dst.condition = new ArrayList<IdType>(); 5274 for (IdType i : condition) 5275 dst.condition.add(i.copy()); 5276 } 5277 ; 5278 if (constraint != null) { 5279 dst.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 5280 for (ElementDefinitionConstraintComponent i : constraint) 5281 dst.constraint.add(i.copy()); 5282 } 5283 ; 5284 dst.mustSupport = mustSupport == null ? null : mustSupport.copy(); 5285 dst.isModifier = isModifier == null ? null : isModifier.copy(); 5286 dst.isSummary = isSummary == null ? null : isSummary.copy(); 5287 dst.binding = binding == null ? null : binding.copy(); 5288 if (mapping != null) { 5289 dst.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 5290 for (ElementDefinitionMappingComponent i : mapping) 5291 dst.mapping.add(i.copy()); 5292 } 5293 ; 5294 return dst; 5295 } 5296 5297 protected ElementDefinition typedCopy() { 5298 return copy(); 5299 } 5300 5301 @Override 5302 public boolean equalsDeep(Base other) { 5303 if (!super.equalsDeep(other)) 5304 return false; 5305 if (!(other instanceof ElementDefinition)) 5306 return false; 5307 ElementDefinition o = (ElementDefinition) other; 5308 return compareDeep(path, o.path, true) && compareDeep(representation, o.representation, true) 5309 && compareDeep(name, o.name, true) && compareDeep(label, o.label, true) && compareDeep(code, o.code, true) 5310 && compareDeep(slicing, o.slicing, true) && compareDeep(short_, o.short_, true) 5311 && compareDeep(definition, o.definition, true) && compareDeep(comments, o.comments, true) 5312 && compareDeep(requirements, o.requirements, true) && compareDeep(alias, o.alias, true) 5313 && compareDeep(min, o.min, true) && compareDeep(max, o.max, true) && compareDeep(base, o.base, true) 5314 && compareDeep(type, o.type, true) && compareDeep(nameReference, o.nameReference, true) 5315 && compareDeep(defaultValue, o.defaultValue, true) 5316 && compareDeep(meaningWhenMissing, o.meaningWhenMissing, true) && compareDeep(fixed, o.fixed, true) 5317 && compareDeep(pattern, o.pattern, true) && compareDeep(example, o.example, true) 5318 && compareDeep(minValue, o.minValue, true) && compareDeep(maxValue, o.maxValue, true) 5319 && compareDeep(maxLength, o.maxLength, true) && compareDeep(condition, o.condition, true) 5320 && compareDeep(constraint, o.constraint, true) && compareDeep(mustSupport, o.mustSupport, true) 5321 && compareDeep(isModifier, o.isModifier, true) && compareDeep(isSummary, o.isSummary, true) 5322 && compareDeep(binding, o.binding, true) && compareDeep(mapping, o.mapping, true); 5323 } 5324 5325 @Override 5326 public boolean equalsShallow(Base other) { 5327 if (!super.equalsShallow(other)) 5328 return false; 5329 if (!(other instanceof ElementDefinition)) 5330 return false; 5331 ElementDefinition o = (ElementDefinition) other; 5332 return compareValues(path, o.path, true) && compareValues(representation, o.representation, true) 5333 && compareValues(name, o.name, true) && compareValues(label, o.label, true) 5334 && compareValues(short_, o.short_, true) && compareValues(definition, o.definition, true) 5335 && compareValues(comments, o.comments, true) && compareValues(requirements, o.requirements, true) 5336 && compareValues(alias, o.alias, true) && compareValues(min, o.min, true) && compareValues(max, o.max, true) 5337 && compareValues(nameReference, o.nameReference, true) 5338 && compareValues(meaningWhenMissing, o.meaningWhenMissing, true) && compareValues(maxLength, o.maxLength, true) 5339 && compareValues(condition, o.condition, true) && compareValues(mustSupport, o.mustSupport, true) 5340 && compareValues(isModifier, o.isModifier, true) && compareValues(isSummary, o.isSummary, true); 5341 } 5342 5343 public boolean isEmpty() { 5344 return super.isEmpty() && (path == null || path.isEmpty()) && (representation == null || representation.isEmpty()) 5345 && (name == null || name.isEmpty()) && (label == null || label.isEmpty()) && (code == null || code.isEmpty()) 5346 && (slicing == null || slicing.isEmpty()) && (short_ == null || short_.isEmpty()) 5347 && (definition == null || definition.isEmpty()) && (comments == null || comments.isEmpty()) 5348 && (requirements == null || requirements.isEmpty()) && (alias == null || alias.isEmpty()) 5349 && (min == null || min.isEmpty()) && (max == null || max.isEmpty()) && (base == null || base.isEmpty()) 5350 && (type == null || type.isEmpty()) && (nameReference == null || nameReference.isEmpty()) 5351 && (defaultValue == null || defaultValue.isEmpty()) 5352 && (meaningWhenMissing == null || meaningWhenMissing.isEmpty()) && (fixed == null || fixed.isEmpty()) 5353 && (pattern == null || pattern.isEmpty()) && (example == null || example.isEmpty()) 5354 && (minValue == null || minValue.isEmpty()) && (maxValue == null || maxValue.isEmpty()) 5355 && (maxLength == null || maxLength.isEmpty()) && (condition == null || condition.isEmpty()) 5356 && (constraint == null || constraint.isEmpty()) && (mustSupport == null || mustSupport.isEmpty()) 5357 && (isModifier == null || isModifier.isEmpty()) && (isSummary == null || isSummary.isEmpty()) 5358 && (binding == null || binding.isEmpty()) && (mapping == null || mapping.isEmpty()); 5359 } 5360 5361}