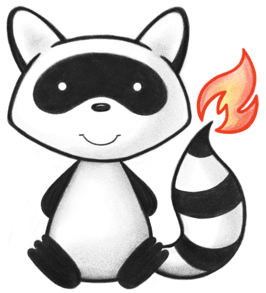
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.exceptions.FHIRException; 042 043/** 044 * This resource provides the insurance eligibility details from the insurer 045 * regarding a specified coverage and optionally some class of service. 046 */ 047@ResourceDef(name = "EligibilityRequest", profile = "http://hl7.org/fhir/Profile/EligibilityRequest") 048public class EligibilityRequest extends DomainResource { 049 050 /** 051 * The Response business identifier. 052 */ 053 @Child(name = "identifier", type = { 054 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 055 @Description(shortDefinition = "Business Identifier", formalDefinition = "The Response business identifier.") 056 protected List<Identifier> identifier; 057 058 /** 059 * The version of the style of resource contents. This should be mapped to the 060 * allowable profiles for this and supporting resources. 061 */ 062 @Child(name = "ruleset", type = { Coding.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 063 @Description(shortDefinition = "Resource version", formalDefinition = "The version of the style of resource contents. This should be mapped to the allowable profiles for this and supporting resources.") 064 protected Coding ruleset; 065 066 /** 067 * The style (standard) and version of the original material which was converted 068 * into this resource. 069 */ 070 @Child(name = "originalRuleset", type = { 071 Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 072 @Description(shortDefinition = "Original version", formalDefinition = "The style (standard) and version of the original material which was converted into this resource.") 073 protected Coding originalRuleset; 074 075 /** 076 * The date when this resource was created. 077 */ 078 @Child(name = "created", type = { DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 079 @Description(shortDefinition = "Creation date", formalDefinition = "The date when this resource was created.") 080 protected DateTimeType created; 081 082 /** 083 * The Insurer who is target of the request. 084 */ 085 @Child(name = "target", type = { Organization.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 086 @Description(shortDefinition = "Insurer", formalDefinition = "The Insurer who is target of the request.") 087 protected Reference target; 088 089 /** 090 * The actual object that is the target of the reference (The Insurer who is 091 * target of the request.) 092 */ 093 protected Organization targetTarget; 094 095 /** 096 * The practitioner who is responsible for the services rendered to the patient. 097 */ 098 @Child(name = "provider", type = { 099 Practitioner.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 100 @Description(shortDefinition = "Responsible practitioner", formalDefinition = "The practitioner who is responsible for the services rendered to the patient.") 101 protected Reference provider; 102 103 /** 104 * The actual object that is the target of the reference (The practitioner who 105 * is responsible for the services rendered to the patient.) 106 */ 107 protected Practitioner providerTarget; 108 109 /** 110 * The organization which is responsible for the services rendered to the 111 * patient. 112 */ 113 @Child(name = "organization", type = { 114 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 115 @Description(shortDefinition = "Responsible organization", formalDefinition = "The organization which is responsible for the services rendered to the patient.") 116 protected Reference organization; 117 118 /** 119 * The actual object that is the target of the reference (The organization which 120 * is responsible for the services rendered to the patient.) 121 */ 122 protected Organization organizationTarget; 123 124 private static final long serialVersionUID = 1836339504L; 125 126 /* 127 * Constructor 128 */ 129 public EligibilityRequest() { 130 super(); 131 } 132 133 /** 134 * @return {@link #identifier} (The Response business identifier.) 135 */ 136 public List<Identifier> getIdentifier() { 137 if (this.identifier == null) 138 this.identifier = new ArrayList<Identifier>(); 139 return this.identifier; 140 } 141 142 public boolean hasIdentifier() { 143 if (this.identifier == null) 144 return false; 145 for (Identifier item : this.identifier) 146 if (!item.isEmpty()) 147 return true; 148 return false; 149 } 150 151 /** 152 * @return {@link #identifier} (The Response business identifier.) 153 */ 154 // syntactic sugar 155 public Identifier addIdentifier() { // 3 156 Identifier t = new Identifier(); 157 if (this.identifier == null) 158 this.identifier = new ArrayList<Identifier>(); 159 this.identifier.add(t); 160 return t; 161 } 162 163 // syntactic sugar 164 public EligibilityRequest addIdentifier(Identifier t) { // 3 165 if (t == null) 166 return this; 167 if (this.identifier == null) 168 this.identifier = new ArrayList<Identifier>(); 169 this.identifier.add(t); 170 return this; 171 } 172 173 /** 174 * @return {@link #ruleset} (The version of the style of resource contents. This 175 * should be mapped to the allowable profiles for this and supporting 176 * resources.) 177 */ 178 public Coding getRuleset() { 179 if (this.ruleset == null) 180 if (Configuration.errorOnAutoCreate()) 181 throw new Error("Attempt to auto-create EligibilityRequest.ruleset"); 182 else if (Configuration.doAutoCreate()) 183 this.ruleset = new Coding(); // cc 184 return this.ruleset; 185 } 186 187 public boolean hasRuleset() { 188 return this.ruleset != null && !this.ruleset.isEmpty(); 189 } 190 191 /** 192 * @param value {@link #ruleset} (The version of the style of resource contents. 193 * This should be mapped to the allowable profiles for this and 194 * supporting resources.) 195 */ 196 public EligibilityRequest setRuleset(Coding value) { 197 this.ruleset = value; 198 return this; 199 } 200 201 /** 202 * @return {@link #originalRuleset} (The style (standard) and version of the 203 * original material which was converted into this resource.) 204 */ 205 public Coding getOriginalRuleset() { 206 if (this.originalRuleset == null) 207 if (Configuration.errorOnAutoCreate()) 208 throw new Error("Attempt to auto-create EligibilityRequest.originalRuleset"); 209 else if (Configuration.doAutoCreate()) 210 this.originalRuleset = new Coding(); // cc 211 return this.originalRuleset; 212 } 213 214 public boolean hasOriginalRuleset() { 215 return this.originalRuleset != null && !this.originalRuleset.isEmpty(); 216 } 217 218 /** 219 * @param value {@link #originalRuleset} (The style (standard) and version of 220 * the original material which was converted into this resource.) 221 */ 222 public EligibilityRequest setOriginalRuleset(Coding value) { 223 this.originalRuleset = value; 224 return this; 225 } 226 227 /** 228 * @return {@link #created} (The date when this resource was created.). This is 229 * the underlying object with id, value and extensions. The accessor 230 * "getCreated" gives direct access to the value 231 */ 232 public DateTimeType getCreatedElement() { 233 if (this.created == null) 234 if (Configuration.errorOnAutoCreate()) 235 throw new Error("Attempt to auto-create EligibilityRequest.created"); 236 else if (Configuration.doAutoCreate()) 237 this.created = new DateTimeType(); // bb 238 return this.created; 239 } 240 241 public boolean hasCreatedElement() { 242 return this.created != null && !this.created.isEmpty(); 243 } 244 245 public boolean hasCreated() { 246 return this.created != null && !this.created.isEmpty(); 247 } 248 249 /** 250 * @param value {@link #created} (The date when this resource was created.). 251 * This is the underlying object with id, value and extensions. The 252 * accessor "getCreated" gives direct access to the value 253 */ 254 public EligibilityRequest setCreatedElement(DateTimeType value) { 255 this.created = value; 256 return this; 257 } 258 259 /** 260 * @return The date when this resource was created. 261 */ 262 public Date getCreated() { 263 return this.created == null ? null : this.created.getValue(); 264 } 265 266 /** 267 * @param value The date when this resource was created. 268 */ 269 public EligibilityRequest setCreated(Date value) { 270 if (value == null) 271 this.created = null; 272 else { 273 if (this.created == null) 274 this.created = new DateTimeType(); 275 this.created.setValue(value); 276 } 277 return this; 278 } 279 280 /** 281 * @return {@link #target} (The Insurer who is target of the request.) 282 */ 283 public Reference getTarget() { 284 if (this.target == null) 285 if (Configuration.errorOnAutoCreate()) 286 throw new Error("Attempt to auto-create EligibilityRequest.target"); 287 else if (Configuration.doAutoCreate()) 288 this.target = new Reference(); // cc 289 return this.target; 290 } 291 292 public boolean hasTarget() { 293 return this.target != null && !this.target.isEmpty(); 294 } 295 296 /** 297 * @param value {@link #target} (The Insurer who is target of the request.) 298 */ 299 public EligibilityRequest setTarget(Reference value) { 300 this.target = value; 301 return this; 302 } 303 304 /** 305 * @return {@link #target} The actual object that is the target of the 306 * reference. The reference library doesn't populate this, but you can 307 * use it to hold the resource if you resolve it. (The Insurer who is 308 * target of the request.) 309 */ 310 public Organization getTargetTarget() { 311 if (this.targetTarget == null) 312 if (Configuration.errorOnAutoCreate()) 313 throw new Error("Attempt to auto-create EligibilityRequest.target"); 314 else if (Configuration.doAutoCreate()) 315 this.targetTarget = new Organization(); // aa 316 return this.targetTarget; 317 } 318 319 /** 320 * @param value {@link #target} The actual object that is the target of the 321 * reference. The reference library doesn't use these, but you can 322 * use it to hold the resource if you resolve it. (The Insurer who 323 * is target of the request.) 324 */ 325 public EligibilityRequest setTargetTarget(Organization value) { 326 this.targetTarget = value; 327 return this; 328 } 329 330 /** 331 * @return {@link #provider} (The practitioner who is responsible for the 332 * services rendered to the patient.) 333 */ 334 public Reference getProvider() { 335 if (this.provider == null) 336 if (Configuration.errorOnAutoCreate()) 337 throw new Error("Attempt to auto-create EligibilityRequest.provider"); 338 else if (Configuration.doAutoCreate()) 339 this.provider = new Reference(); // cc 340 return this.provider; 341 } 342 343 public boolean hasProvider() { 344 return this.provider != null && !this.provider.isEmpty(); 345 } 346 347 /** 348 * @param value {@link #provider} (The practitioner who is responsible for the 349 * services rendered to the patient.) 350 */ 351 public EligibilityRequest setProvider(Reference value) { 352 this.provider = value; 353 return this; 354 } 355 356 /** 357 * @return {@link #provider} The actual object that is the target of the 358 * reference. The reference library doesn't populate this, but you can 359 * use it to hold the resource if you resolve it. (The practitioner who 360 * is responsible for the services rendered to the patient.) 361 */ 362 public Practitioner getProviderTarget() { 363 if (this.providerTarget == null) 364 if (Configuration.errorOnAutoCreate()) 365 throw new Error("Attempt to auto-create EligibilityRequest.provider"); 366 else if (Configuration.doAutoCreate()) 367 this.providerTarget = new Practitioner(); // aa 368 return this.providerTarget; 369 } 370 371 /** 372 * @param value {@link #provider} The actual object that is the target of the 373 * reference. The reference library doesn't use these, but you can 374 * use it to hold the resource if you resolve it. (The practitioner 375 * who is responsible for the services rendered to the patient.) 376 */ 377 public EligibilityRequest setProviderTarget(Practitioner value) { 378 this.providerTarget = value; 379 return this; 380 } 381 382 /** 383 * @return {@link #organization} (The organization which is responsible for the 384 * services rendered to the patient.) 385 */ 386 public Reference getOrganization() { 387 if (this.organization == null) 388 if (Configuration.errorOnAutoCreate()) 389 throw new Error("Attempt to auto-create EligibilityRequest.organization"); 390 else if (Configuration.doAutoCreate()) 391 this.organization = new Reference(); // cc 392 return this.organization; 393 } 394 395 public boolean hasOrganization() { 396 return this.organization != null && !this.organization.isEmpty(); 397 } 398 399 /** 400 * @param value {@link #organization} (The organization which is responsible for 401 * the services rendered to the patient.) 402 */ 403 public EligibilityRequest setOrganization(Reference value) { 404 this.organization = value; 405 return this; 406 } 407 408 /** 409 * @return {@link #organization} The actual object that is the target of the 410 * reference. The reference library doesn't populate this, but you can 411 * use it to hold the resource if you resolve it. (The organization 412 * which is responsible for the services rendered to the patient.) 413 */ 414 public Organization getOrganizationTarget() { 415 if (this.organizationTarget == null) 416 if (Configuration.errorOnAutoCreate()) 417 throw new Error("Attempt to auto-create EligibilityRequest.organization"); 418 else if (Configuration.doAutoCreate()) 419 this.organizationTarget = new Organization(); // aa 420 return this.organizationTarget; 421 } 422 423 /** 424 * @param value {@link #organization} The actual object that is the target of 425 * the reference. The reference library doesn't use these, but you 426 * can use it to hold the resource if you resolve it. (The 427 * organization which is responsible for the services rendered to 428 * the patient.) 429 */ 430 public EligibilityRequest setOrganizationTarget(Organization value) { 431 this.organizationTarget = value; 432 return this; 433 } 434 435 protected void listChildren(List<Property> childrenList) { 436 super.listChildren(childrenList); 437 childrenList.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, 438 java.lang.Integer.MAX_VALUE, identifier)); 439 childrenList.add(new Property("ruleset", "Coding", 440 "The version of the style of resource contents. This should be mapped to the allowable profiles for this and supporting resources.", 441 0, java.lang.Integer.MAX_VALUE, ruleset)); 442 childrenList.add(new Property("originalRuleset", "Coding", 443 "The style (standard) and version of the original material which was converted into this resource.", 0, 444 java.lang.Integer.MAX_VALUE, originalRuleset)); 445 childrenList.add(new Property("created", "dateTime", "The date when this resource was created.", 0, 446 java.lang.Integer.MAX_VALUE, created)); 447 childrenList.add(new Property("target", "Reference(Organization)", "The Insurer who is target of the request.", 0, 448 java.lang.Integer.MAX_VALUE, target)); 449 childrenList.add(new Property("provider", "Reference(Practitioner)", 450 "The practitioner who is responsible for the services rendered to the patient.", 0, java.lang.Integer.MAX_VALUE, 451 provider)); 452 childrenList.add(new Property("organization", "Reference(Organization)", 453 "The organization which is responsible for the services rendered to the patient.", 0, 454 java.lang.Integer.MAX_VALUE, organization)); 455 } 456 457 @Override 458 public void setProperty(String name, Base value) throws FHIRException { 459 if (name.equals("identifier")) 460 this.getIdentifier().add(castToIdentifier(value)); 461 else if (name.equals("ruleset")) 462 this.ruleset = castToCoding(value); // Coding 463 else if (name.equals("originalRuleset")) 464 this.originalRuleset = castToCoding(value); // Coding 465 else if (name.equals("created")) 466 this.created = castToDateTime(value); // DateTimeType 467 else if (name.equals("target")) 468 this.target = castToReference(value); // Reference 469 else if (name.equals("provider")) 470 this.provider = castToReference(value); // Reference 471 else if (name.equals("organization")) 472 this.organization = castToReference(value); // Reference 473 else 474 super.setProperty(name, value); 475 } 476 477 @Override 478 public Base addChild(String name) throws FHIRException { 479 if (name.equals("identifier")) { 480 return addIdentifier(); 481 } else if (name.equals("ruleset")) { 482 this.ruleset = new Coding(); 483 return this.ruleset; 484 } else if (name.equals("originalRuleset")) { 485 this.originalRuleset = new Coding(); 486 return this.originalRuleset; 487 } else if (name.equals("created")) { 488 throw new FHIRException("Cannot call addChild on a singleton property EligibilityRequest.created"); 489 } else if (name.equals("target")) { 490 this.target = new Reference(); 491 return this.target; 492 } else if (name.equals("provider")) { 493 this.provider = new Reference(); 494 return this.provider; 495 } else if (name.equals("organization")) { 496 this.organization = new Reference(); 497 return this.organization; 498 } else 499 return super.addChild(name); 500 } 501 502 public String fhirType() { 503 return "EligibilityRequest"; 504 505 } 506 507 public EligibilityRequest copy() { 508 EligibilityRequest dst = new EligibilityRequest(); 509 copyValues(dst); 510 if (identifier != null) { 511 dst.identifier = new ArrayList<Identifier>(); 512 for (Identifier i : identifier) 513 dst.identifier.add(i.copy()); 514 } 515 ; 516 dst.ruleset = ruleset == null ? null : ruleset.copy(); 517 dst.originalRuleset = originalRuleset == null ? null : originalRuleset.copy(); 518 dst.created = created == null ? null : created.copy(); 519 dst.target = target == null ? null : target.copy(); 520 dst.provider = provider == null ? null : provider.copy(); 521 dst.organization = organization == null ? null : organization.copy(); 522 return dst; 523 } 524 525 protected EligibilityRequest typedCopy() { 526 return copy(); 527 } 528 529 @Override 530 public boolean equalsDeep(Base other) { 531 if (!super.equalsDeep(other)) 532 return false; 533 if (!(other instanceof EligibilityRequest)) 534 return false; 535 EligibilityRequest o = (EligibilityRequest) other; 536 return compareDeep(identifier, o.identifier, true) && compareDeep(ruleset, o.ruleset, true) 537 && compareDeep(originalRuleset, o.originalRuleset, true) && compareDeep(created, o.created, true) 538 && compareDeep(target, o.target, true) && compareDeep(provider, o.provider, true) 539 && compareDeep(organization, o.organization, true); 540 } 541 542 @Override 543 public boolean equalsShallow(Base other) { 544 if (!super.equalsShallow(other)) 545 return false; 546 if (!(other instanceof EligibilityRequest)) 547 return false; 548 EligibilityRequest o = (EligibilityRequest) other; 549 return compareValues(created, o.created, true); 550 } 551 552 public boolean isEmpty() { 553 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (ruleset == null || ruleset.isEmpty()) 554 && (originalRuleset == null || originalRuleset.isEmpty()) && (created == null || created.isEmpty()) 555 && (target == null || target.isEmpty()) && (provider == null || provider.isEmpty()) 556 && (organization == null || organization.isEmpty()); 557 } 558 559 @Override 560 public ResourceType getResourceType() { 561 return ResourceType.EligibilityRequest; 562 } 563 564 @SearchParamDefinition(name = "identifier", path = "EligibilityRequest.identifier", description = "The business identifier of the Eligibility", type = "token") 565 public static final String SP_IDENTIFIER = "identifier"; 566 567}