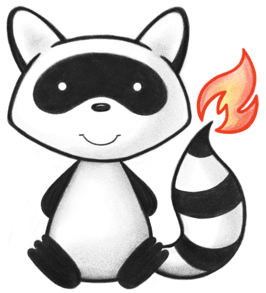
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.RemittanceOutcome; 038import org.hl7.fhir.dstu2.model.Enumerations.RemittanceOutcomeEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.Description; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * This resource provides eligibility and plan details from the processing of an 048 * Eligibility resource. 049 */ 050@ResourceDef(name = "EligibilityResponse", profile = "http://hl7.org/fhir/Profile/EligibilityResponse") 051public class EligibilityResponse extends DomainResource { 052 053 /** 054 * The Response business identifier. 055 */ 056 @Child(name = "identifier", type = { 057 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 058 @Description(shortDefinition = "Business Identifier", formalDefinition = "The Response business identifier.") 059 protected List<Identifier> identifier; 060 061 /** 062 * Original request resource reference. 063 */ 064 @Child(name = "request", type = { 065 EligibilityRequest.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 066 @Description(shortDefinition = "Claim reference", formalDefinition = "Original request resource reference.") 067 protected Reference request; 068 069 /** 070 * The actual object that is the target of the reference (Original request 071 * resource reference.) 072 */ 073 protected EligibilityRequest requestTarget; 074 075 /** 076 * Transaction status: error, complete. 077 */ 078 @Child(name = "outcome", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 079 @Description(shortDefinition = "complete | error", formalDefinition = "Transaction status: error, complete.") 080 protected Enumeration<RemittanceOutcome> outcome; 081 082 /** 083 * A description of the status of the adjudication. 084 */ 085 @Child(name = "disposition", type = { 086 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 087 @Description(shortDefinition = "Disposition Message", formalDefinition = "A description of the status of the adjudication.") 088 protected StringType disposition; 089 090 /** 091 * The version of the style of resource contents. This should be mapped to the 092 * allowable profiles for this and supporting resources. 093 */ 094 @Child(name = "ruleset", type = { Coding.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 095 @Description(shortDefinition = "Resource version", formalDefinition = "The version of the style of resource contents. This should be mapped to the allowable profiles for this and supporting resources.") 096 protected Coding ruleset; 097 098 /** 099 * The style (standard) and version of the original material which was converted 100 * into this resource. 101 */ 102 @Child(name = "originalRuleset", type = { 103 Coding.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 104 @Description(shortDefinition = "Original version", formalDefinition = "The style (standard) and version of the original material which was converted into this resource.") 105 protected Coding originalRuleset; 106 107 /** 108 * The date when the enclosed suite of services were performed or completed. 109 */ 110 @Child(name = "created", type = { DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 111 @Description(shortDefinition = "Creation date", formalDefinition = "The date when the enclosed suite of services were performed or completed.") 112 protected DateTimeType created; 113 114 /** 115 * The Insurer who produced this adjudicated response. 116 */ 117 @Child(name = "organization", type = { 118 Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 119 @Description(shortDefinition = "Insurer", formalDefinition = "The Insurer who produced this adjudicated response.") 120 protected Reference organization; 121 122 /** 123 * The actual object that is the target of the reference (The Insurer who 124 * produced this adjudicated response.) 125 */ 126 protected Organization organizationTarget; 127 128 /** 129 * The practitioner who is responsible for the services rendered to the patient. 130 */ 131 @Child(name = "requestProvider", type = { 132 Practitioner.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 133 @Description(shortDefinition = "Responsible practitioner", formalDefinition = "The practitioner who is responsible for the services rendered to the patient.") 134 protected Reference requestProvider; 135 136 /** 137 * The actual object that is the target of the reference (The practitioner who 138 * is responsible for the services rendered to the patient.) 139 */ 140 protected Practitioner requestProviderTarget; 141 142 /** 143 * The organization which is responsible for the services rendered to the 144 * patient. 145 */ 146 @Child(name = "requestOrganization", type = { 147 Organization.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 148 @Description(shortDefinition = "Responsible organization", formalDefinition = "The organization which is responsible for the services rendered to the patient.") 149 protected Reference requestOrganization; 150 151 /** 152 * The actual object that is the target of the reference (The organization which 153 * is responsible for the services rendered to the patient.) 154 */ 155 protected Organization requestOrganizationTarget; 156 157 private static final long serialVersionUID = -299931023L; 158 159 /* 160 * Constructor 161 */ 162 public EligibilityResponse() { 163 super(); 164 } 165 166 /** 167 * @return {@link #identifier} (The Response business identifier.) 168 */ 169 public List<Identifier> getIdentifier() { 170 if (this.identifier == null) 171 this.identifier = new ArrayList<Identifier>(); 172 return this.identifier; 173 } 174 175 public boolean hasIdentifier() { 176 if (this.identifier == null) 177 return false; 178 for (Identifier item : this.identifier) 179 if (!item.isEmpty()) 180 return true; 181 return false; 182 } 183 184 /** 185 * @return {@link #identifier} (The Response business identifier.) 186 */ 187 // syntactic sugar 188 public Identifier addIdentifier() { // 3 189 Identifier t = new Identifier(); 190 if (this.identifier == null) 191 this.identifier = new ArrayList<Identifier>(); 192 this.identifier.add(t); 193 return t; 194 } 195 196 // syntactic sugar 197 public EligibilityResponse addIdentifier(Identifier t) { // 3 198 if (t == null) 199 return this; 200 if (this.identifier == null) 201 this.identifier = new ArrayList<Identifier>(); 202 this.identifier.add(t); 203 return this; 204 } 205 206 /** 207 * @return {@link #request} (Original request resource reference.) 208 */ 209 public Reference getRequest() { 210 if (this.request == null) 211 if (Configuration.errorOnAutoCreate()) 212 throw new Error("Attempt to auto-create EligibilityResponse.request"); 213 else if (Configuration.doAutoCreate()) 214 this.request = new Reference(); // cc 215 return this.request; 216 } 217 218 public boolean hasRequest() { 219 return this.request != null && !this.request.isEmpty(); 220 } 221 222 /** 223 * @param value {@link #request} (Original request resource reference.) 224 */ 225 public EligibilityResponse setRequest(Reference value) { 226 this.request = value; 227 return this; 228 } 229 230 /** 231 * @return {@link #request} The actual object that is the target of the 232 * reference. The reference library doesn't populate this, but you can 233 * use it to hold the resource if you resolve it. (Original request 234 * resource reference.) 235 */ 236 public EligibilityRequest getRequestTarget() { 237 if (this.requestTarget == null) 238 if (Configuration.errorOnAutoCreate()) 239 throw new Error("Attempt to auto-create EligibilityResponse.request"); 240 else if (Configuration.doAutoCreate()) 241 this.requestTarget = new EligibilityRequest(); // aa 242 return this.requestTarget; 243 } 244 245 /** 246 * @param value {@link #request} The actual object that is the target of the 247 * reference. The reference library doesn't use these, but you can 248 * use it to hold the resource if you resolve it. (Original request 249 * resource reference.) 250 */ 251 public EligibilityResponse setRequestTarget(EligibilityRequest value) { 252 this.requestTarget = value; 253 return this; 254 } 255 256 /** 257 * @return {@link #outcome} (Transaction status: error, complete.). This is the 258 * underlying object with id, value and extensions. The accessor 259 * "getOutcome" gives direct access to the value 260 */ 261 public Enumeration<RemittanceOutcome> getOutcomeElement() { 262 if (this.outcome == null) 263 if (Configuration.errorOnAutoCreate()) 264 throw new Error("Attempt to auto-create EligibilityResponse.outcome"); 265 else if (Configuration.doAutoCreate()) 266 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); // bb 267 return this.outcome; 268 } 269 270 public boolean hasOutcomeElement() { 271 return this.outcome != null && !this.outcome.isEmpty(); 272 } 273 274 public boolean hasOutcome() { 275 return this.outcome != null && !this.outcome.isEmpty(); 276 } 277 278 /** 279 * @param value {@link #outcome} (Transaction status: error, complete.). This is 280 * the underlying object with id, value and extensions. The 281 * accessor "getOutcome" gives direct access to the value 282 */ 283 public EligibilityResponse setOutcomeElement(Enumeration<RemittanceOutcome> value) { 284 this.outcome = value; 285 return this; 286 } 287 288 /** 289 * @return Transaction status: error, complete. 290 */ 291 public RemittanceOutcome getOutcome() { 292 return this.outcome == null ? null : this.outcome.getValue(); 293 } 294 295 /** 296 * @param value Transaction status: error, complete. 297 */ 298 public EligibilityResponse setOutcome(RemittanceOutcome value) { 299 if (value == null) 300 this.outcome = null; 301 else { 302 if (this.outcome == null) 303 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); 304 this.outcome.setValue(value); 305 } 306 return this; 307 } 308 309 /** 310 * @return {@link #disposition} (A description of the status of the 311 * adjudication.). This is the underlying object with id, value and 312 * extensions. The accessor "getDisposition" gives direct access to the 313 * value 314 */ 315 public StringType getDispositionElement() { 316 if (this.disposition == null) 317 if (Configuration.errorOnAutoCreate()) 318 throw new Error("Attempt to auto-create EligibilityResponse.disposition"); 319 else if (Configuration.doAutoCreate()) 320 this.disposition = new StringType(); // bb 321 return this.disposition; 322 } 323 324 public boolean hasDispositionElement() { 325 return this.disposition != null && !this.disposition.isEmpty(); 326 } 327 328 public boolean hasDisposition() { 329 return this.disposition != null && !this.disposition.isEmpty(); 330 } 331 332 /** 333 * @param value {@link #disposition} (A description of the status of the 334 * adjudication.). This is the underlying object with id, value and 335 * extensions. The accessor "getDisposition" gives direct access to 336 * the value 337 */ 338 public EligibilityResponse setDispositionElement(StringType value) { 339 this.disposition = value; 340 return this; 341 } 342 343 /** 344 * @return A description of the status of the adjudication. 345 */ 346 public String getDisposition() { 347 return this.disposition == null ? null : this.disposition.getValue(); 348 } 349 350 /** 351 * @param value A description of the status of the adjudication. 352 */ 353 public EligibilityResponse setDisposition(String value) { 354 if (Utilities.noString(value)) 355 this.disposition = null; 356 else { 357 if (this.disposition == null) 358 this.disposition = new StringType(); 359 this.disposition.setValue(value); 360 } 361 return this; 362 } 363 364 /** 365 * @return {@link #ruleset} (The version of the style of resource contents. This 366 * should be mapped to the allowable profiles for this and supporting 367 * resources.) 368 */ 369 public Coding getRuleset() { 370 if (this.ruleset == null) 371 if (Configuration.errorOnAutoCreate()) 372 throw new Error("Attempt to auto-create EligibilityResponse.ruleset"); 373 else if (Configuration.doAutoCreate()) 374 this.ruleset = new Coding(); // cc 375 return this.ruleset; 376 } 377 378 public boolean hasRuleset() { 379 return this.ruleset != null && !this.ruleset.isEmpty(); 380 } 381 382 /** 383 * @param value {@link #ruleset} (The version of the style of resource contents. 384 * This should be mapped to the allowable profiles for this and 385 * supporting resources.) 386 */ 387 public EligibilityResponse setRuleset(Coding value) { 388 this.ruleset = value; 389 return this; 390 } 391 392 /** 393 * @return {@link #originalRuleset} (The style (standard) and version of the 394 * original material which was converted into this resource.) 395 */ 396 public Coding getOriginalRuleset() { 397 if (this.originalRuleset == null) 398 if (Configuration.errorOnAutoCreate()) 399 throw new Error("Attempt to auto-create EligibilityResponse.originalRuleset"); 400 else if (Configuration.doAutoCreate()) 401 this.originalRuleset = new Coding(); // cc 402 return this.originalRuleset; 403 } 404 405 public boolean hasOriginalRuleset() { 406 return this.originalRuleset != null && !this.originalRuleset.isEmpty(); 407 } 408 409 /** 410 * @param value {@link #originalRuleset} (The style (standard) and version of 411 * the original material which was converted into this resource.) 412 */ 413 public EligibilityResponse setOriginalRuleset(Coding value) { 414 this.originalRuleset = value; 415 return this; 416 } 417 418 /** 419 * @return {@link #created} (The date when the enclosed suite of services were 420 * performed or completed.). This is the underlying object with id, 421 * value and extensions. The accessor "getCreated" gives direct access 422 * to the value 423 */ 424 public DateTimeType getCreatedElement() { 425 if (this.created == null) 426 if (Configuration.errorOnAutoCreate()) 427 throw new Error("Attempt to auto-create EligibilityResponse.created"); 428 else if (Configuration.doAutoCreate()) 429 this.created = new DateTimeType(); // bb 430 return this.created; 431 } 432 433 public boolean hasCreatedElement() { 434 return this.created != null && !this.created.isEmpty(); 435 } 436 437 public boolean hasCreated() { 438 return this.created != null && !this.created.isEmpty(); 439 } 440 441 /** 442 * @param value {@link #created} (The date when the enclosed suite of services 443 * were performed or completed.). This is the underlying object 444 * with id, value and extensions. The accessor "getCreated" gives 445 * direct access to the value 446 */ 447 public EligibilityResponse setCreatedElement(DateTimeType value) { 448 this.created = value; 449 return this; 450 } 451 452 /** 453 * @return The date when the enclosed suite of services were performed or 454 * completed. 455 */ 456 public Date getCreated() { 457 return this.created == null ? null : this.created.getValue(); 458 } 459 460 /** 461 * @param value The date when the enclosed suite of services were performed or 462 * completed. 463 */ 464 public EligibilityResponse setCreated(Date value) { 465 if (value == null) 466 this.created = null; 467 else { 468 if (this.created == null) 469 this.created = new DateTimeType(); 470 this.created.setValue(value); 471 } 472 return this; 473 } 474 475 /** 476 * @return {@link #organization} (The Insurer who produced this adjudicated 477 * response.) 478 */ 479 public Reference getOrganization() { 480 if (this.organization == null) 481 if (Configuration.errorOnAutoCreate()) 482 throw new Error("Attempt to auto-create EligibilityResponse.organization"); 483 else if (Configuration.doAutoCreate()) 484 this.organization = new Reference(); // cc 485 return this.organization; 486 } 487 488 public boolean hasOrganization() { 489 return this.organization != null && !this.organization.isEmpty(); 490 } 491 492 /** 493 * @param value {@link #organization} (The Insurer who produced this adjudicated 494 * response.) 495 */ 496 public EligibilityResponse setOrganization(Reference value) { 497 this.organization = value; 498 return this; 499 } 500 501 /** 502 * @return {@link #organization} The actual object that is the target of the 503 * reference. The reference library doesn't populate this, but you can 504 * use it to hold the resource if you resolve it. (The Insurer who 505 * produced this adjudicated response.) 506 */ 507 public Organization getOrganizationTarget() { 508 if (this.organizationTarget == null) 509 if (Configuration.errorOnAutoCreate()) 510 throw new Error("Attempt to auto-create EligibilityResponse.organization"); 511 else if (Configuration.doAutoCreate()) 512 this.organizationTarget = new Organization(); // aa 513 return this.organizationTarget; 514 } 515 516 /** 517 * @param value {@link #organization} The actual object that is the target of 518 * the reference. The reference library doesn't use these, but you 519 * can use it to hold the resource if you resolve it. (The Insurer 520 * who produced this adjudicated response.) 521 */ 522 public EligibilityResponse setOrganizationTarget(Organization value) { 523 this.organizationTarget = value; 524 return this; 525 } 526 527 /** 528 * @return {@link #requestProvider} (The practitioner who is responsible for the 529 * services rendered to the patient.) 530 */ 531 public Reference getRequestProvider() { 532 if (this.requestProvider == null) 533 if (Configuration.errorOnAutoCreate()) 534 throw new Error("Attempt to auto-create EligibilityResponse.requestProvider"); 535 else if (Configuration.doAutoCreate()) 536 this.requestProvider = new Reference(); // cc 537 return this.requestProvider; 538 } 539 540 public boolean hasRequestProvider() { 541 return this.requestProvider != null && !this.requestProvider.isEmpty(); 542 } 543 544 /** 545 * @param value {@link #requestProvider} (The practitioner who is responsible 546 * for the services rendered to the patient.) 547 */ 548 public EligibilityResponse setRequestProvider(Reference value) { 549 this.requestProvider = value; 550 return this; 551 } 552 553 /** 554 * @return {@link #requestProvider} The actual object that is the target of the 555 * reference. The reference library doesn't populate this, but you can 556 * use it to hold the resource if you resolve it. (The practitioner who 557 * is responsible for the services rendered to the patient.) 558 */ 559 public Practitioner getRequestProviderTarget() { 560 if (this.requestProviderTarget == null) 561 if (Configuration.errorOnAutoCreate()) 562 throw new Error("Attempt to auto-create EligibilityResponse.requestProvider"); 563 else if (Configuration.doAutoCreate()) 564 this.requestProviderTarget = new Practitioner(); // aa 565 return this.requestProviderTarget; 566 } 567 568 /** 569 * @param value {@link #requestProvider} The actual object that is the target of 570 * the reference. The reference library doesn't use these, but you 571 * can use it to hold the resource if you resolve it. (The 572 * practitioner who is responsible for the services rendered to the 573 * patient.) 574 */ 575 public EligibilityResponse setRequestProviderTarget(Practitioner value) { 576 this.requestProviderTarget = value; 577 return this; 578 } 579 580 /** 581 * @return {@link #requestOrganization} (The organization which is responsible 582 * for the services rendered to the patient.) 583 */ 584 public Reference getRequestOrganization() { 585 if (this.requestOrganization == null) 586 if (Configuration.errorOnAutoCreate()) 587 throw new Error("Attempt to auto-create EligibilityResponse.requestOrganization"); 588 else if (Configuration.doAutoCreate()) 589 this.requestOrganization = new Reference(); // cc 590 return this.requestOrganization; 591 } 592 593 public boolean hasRequestOrganization() { 594 return this.requestOrganization != null && !this.requestOrganization.isEmpty(); 595 } 596 597 /** 598 * @param value {@link #requestOrganization} (The organization which is 599 * responsible for the services rendered to the patient.) 600 */ 601 public EligibilityResponse setRequestOrganization(Reference value) { 602 this.requestOrganization = value; 603 return this; 604 } 605 606 /** 607 * @return {@link #requestOrganization} The actual object that is the target of 608 * the reference. The reference library doesn't populate this, but you 609 * can use it to hold the resource if you resolve it. (The organization 610 * which is responsible for the services rendered to the patient.) 611 */ 612 public Organization getRequestOrganizationTarget() { 613 if (this.requestOrganizationTarget == null) 614 if (Configuration.errorOnAutoCreate()) 615 throw new Error("Attempt to auto-create EligibilityResponse.requestOrganization"); 616 else if (Configuration.doAutoCreate()) 617 this.requestOrganizationTarget = new Organization(); // aa 618 return this.requestOrganizationTarget; 619 } 620 621 /** 622 * @param value {@link #requestOrganization} The actual object that is the 623 * target of the reference. The reference library doesn't use 624 * these, but you can use it to hold the resource if you resolve 625 * it. (The organization which is responsible for the services 626 * rendered to the patient.) 627 */ 628 public EligibilityResponse setRequestOrganizationTarget(Organization value) { 629 this.requestOrganizationTarget = value; 630 return this; 631 } 632 633 protected void listChildren(List<Property> childrenList) { 634 super.listChildren(childrenList); 635 childrenList.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, 636 java.lang.Integer.MAX_VALUE, identifier)); 637 childrenList.add(new Property("request", "Reference(EligibilityRequest)", "Original request resource reference.", 0, 638 java.lang.Integer.MAX_VALUE, request)); 639 childrenList.add(new Property("outcome", "code", "Transaction status: error, complete.", 0, 640 java.lang.Integer.MAX_VALUE, outcome)); 641 childrenList.add(new Property("disposition", "string", "A description of the status of the adjudication.", 0, 642 java.lang.Integer.MAX_VALUE, disposition)); 643 childrenList.add(new Property("ruleset", "Coding", 644 "The version of the style of resource contents. This should be mapped to the allowable profiles for this and supporting resources.", 645 0, java.lang.Integer.MAX_VALUE, ruleset)); 646 childrenList.add(new Property("originalRuleset", "Coding", 647 "The style (standard) and version of the original material which was converted into this resource.", 0, 648 java.lang.Integer.MAX_VALUE, originalRuleset)); 649 childrenList.add( 650 new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 651 0, java.lang.Integer.MAX_VALUE, created)); 652 childrenList.add(new Property("organization", "Reference(Organization)", 653 "The Insurer who produced this adjudicated response.", 0, java.lang.Integer.MAX_VALUE, organization)); 654 childrenList.add(new Property("requestProvider", "Reference(Practitioner)", 655 "The practitioner who is responsible for the services rendered to the patient.", 0, java.lang.Integer.MAX_VALUE, 656 requestProvider)); 657 childrenList.add(new Property("requestOrganization", "Reference(Organization)", 658 "The organization which is responsible for the services rendered to the patient.", 0, 659 java.lang.Integer.MAX_VALUE, requestOrganization)); 660 } 661 662 @Override 663 public void setProperty(String name, Base value) throws FHIRException { 664 if (name.equals("identifier")) 665 this.getIdentifier().add(castToIdentifier(value)); 666 else if (name.equals("request")) 667 this.request = castToReference(value); // Reference 668 else if (name.equals("outcome")) 669 this.outcome = new RemittanceOutcomeEnumFactory().fromType(value); // Enumeration<RemittanceOutcome> 670 else if (name.equals("disposition")) 671 this.disposition = castToString(value); // StringType 672 else if (name.equals("ruleset")) 673 this.ruleset = castToCoding(value); // Coding 674 else if (name.equals("originalRuleset")) 675 this.originalRuleset = castToCoding(value); // Coding 676 else if (name.equals("created")) 677 this.created = castToDateTime(value); // DateTimeType 678 else if (name.equals("organization")) 679 this.organization = castToReference(value); // Reference 680 else if (name.equals("requestProvider")) 681 this.requestProvider = castToReference(value); // Reference 682 else if (name.equals("requestOrganization")) 683 this.requestOrganization = castToReference(value); // Reference 684 else 685 super.setProperty(name, value); 686 } 687 688 @Override 689 public Base addChild(String name) throws FHIRException { 690 if (name.equals("identifier")) { 691 return addIdentifier(); 692 } else if (name.equals("request")) { 693 this.request = new Reference(); 694 return this.request; 695 } else if (name.equals("outcome")) { 696 throw new FHIRException("Cannot call addChild on a singleton property EligibilityResponse.outcome"); 697 } else if (name.equals("disposition")) { 698 throw new FHIRException("Cannot call addChild on a singleton property EligibilityResponse.disposition"); 699 } else if (name.equals("ruleset")) { 700 this.ruleset = new Coding(); 701 return this.ruleset; 702 } else if (name.equals("originalRuleset")) { 703 this.originalRuleset = new Coding(); 704 return this.originalRuleset; 705 } else if (name.equals("created")) { 706 throw new FHIRException("Cannot call addChild on a singleton property EligibilityResponse.created"); 707 } else if (name.equals("organization")) { 708 this.organization = new Reference(); 709 return this.organization; 710 } else if (name.equals("requestProvider")) { 711 this.requestProvider = new Reference(); 712 return this.requestProvider; 713 } else if (name.equals("requestOrganization")) { 714 this.requestOrganization = new Reference(); 715 return this.requestOrganization; 716 } else 717 return super.addChild(name); 718 } 719 720 public String fhirType() { 721 return "EligibilityResponse"; 722 723 } 724 725 public EligibilityResponse copy() { 726 EligibilityResponse dst = new EligibilityResponse(); 727 copyValues(dst); 728 if (identifier != null) { 729 dst.identifier = new ArrayList<Identifier>(); 730 for (Identifier i : identifier) 731 dst.identifier.add(i.copy()); 732 } 733 ; 734 dst.request = request == null ? null : request.copy(); 735 dst.outcome = outcome == null ? null : outcome.copy(); 736 dst.disposition = disposition == null ? null : disposition.copy(); 737 dst.ruleset = ruleset == null ? null : ruleset.copy(); 738 dst.originalRuleset = originalRuleset == null ? null : originalRuleset.copy(); 739 dst.created = created == null ? null : created.copy(); 740 dst.organization = organization == null ? null : organization.copy(); 741 dst.requestProvider = requestProvider == null ? null : requestProvider.copy(); 742 dst.requestOrganization = requestOrganization == null ? null : requestOrganization.copy(); 743 return dst; 744 } 745 746 protected EligibilityResponse typedCopy() { 747 return copy(); 748 } 749 750 @Override 751 public boolean equalsDeep(Base other) { 752 if (!super.equalsDeep(other)) 753 return false; 754 if (!(other instanceof EligibilityResponse)) 755 return false; 756 EligibilityResponse o = (EligibilityResponse) other; 757 return compareDeep(identifier, o.identifier, true) && compareDeep(request, o.request, true) 758 && compareDeep(outcome, o.outcome, true) && compareDeep(disposition, o.disposition, true) 759 && compareDeep(ruleset, o.ruleset, true) && compareDeep(originalRuleset, o.originalRuleset, true) 760 && compareDeep(created, o.created, true) && compareDeep(organization, o.organization, true) 761 && compareDeep(requestProvider, o.requestProvider, true) 762 && compareDeep(requestOrganization, o.requestOrganization, true); 763 } 764 765 @Override 766 public boolean equalsShallow(Base other) { 767 if (!super.equalsShallow(other)) 768 return false; 769 if (!(other instanceof EligibilityResponse)) 770 return false; 771 EligibilityResponse o = (EligibilityResponse) other; 772 return compareValues(outcome, o.outcome, true) && compareValues(disposition, o.disposition, true) 773 && compareValues(created, o.created, true); 774 } 775 776 public boolean isEmpty() { 777 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (request == null || request.isEmpty()) 778 && (outcome == null || outcome.isEmpty()) && (disposition == null || disposition.isEmpty()) 779 && (ruleset == null || ruleset.isEmpty()) && (originalRuleset == null || originalRuleset.isEmpty()) 780 && (created == null || created.isEmpty()) && (organization == null || organization.isEmpty()) 781 && (requestProvider == null || requestProvider.isEmpty()) 782 && (requestOrganization == null || requestOrganization.isEmpty()); 783 } 784 785 @Override 786 public ResourceType getResourceType() { 787 return ResourceType.EligibilityResponse; 788 } 789 790 @SearchParamDefinition(name = "identifier", path = "EligibilityResponse.identifier", description = "The business identifier of the Explanation of Benefit", type = "token") 791 public static final String SP_IDENTIFIER = "identifier"; 792 793}