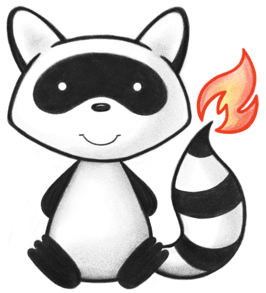
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Block; 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.exceptions.FHIRException; 043 044/** 045 * An interaction between a patient and healthcare provider(s) for the purpose 046 * of providing healthcare service(s) or assessing the health status of a 047 * patient. 048 */ 049@ResourceDef(name = "Encounter", profile = "http://hl7.org/fhir/Profile/Encounter") 050public class Encounter extends DomainResource { 051 052 public enum EncounterState { 053 /** 054 * The Encounter has not yet started. 055 */ 056 PLANNED, 057 /** 058 * The Patient is present for the encounter, however is not currently meeting 059 * with a practitioner. 060 */ 061 ARRIVED, 062 /** 063 * The Encounter has begun and the patient is present / the practitioner and the 064 * patient are meeting. 065 */ 066 INPROGRESS, 067 /** 068 * The Encounter has begun, but the patient is temporarily on leave. 069 */ 070 ONLEAVE, 071 /** 072 * The Encounter has ended. 073 */ 074 FINISHED, 075 /** 076 * The Encounter has ended before it has begun. 077 */ 078 CANCELLED, 079 /** 080 * added to help the parsers 081 */ 082 NULL; 083 084 public static EncounterState fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("planned".equals(codeString)) 088 return PLANNED; 089 if ("arrived".equals(codeString)) 090 return ARRIVED; 091 if ("in-progress".equals(codeString)) 092 return INPROGRESS; 093 if ("onleave".equals(codeString)) 094 return ONLEAVE; 095 if ("finished".equals(codeString)) 096 return FINISHED; 097 if ("cancelled".equals(codeString)) 098 return CANCELLED; 099 throw new FHIRException("Unknown EncounterState code '" + codeString + "'"); 100 } 101 102 public String toCode() { 103 switch (this) { 104 case PLANNED: 105 return "planned"; 106 case ARRIVED: 107 return "arrived"; 108 case INPROGRESS: 109 return "in-progress"; 110 case ONLEAVE: 111 return "onleave"; 112 case FINISHED: 113 return "finished"; 114 case CANCELLED: 115 return "cancelled"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getSystem() { 124 switch (this) { 125 case PLANNED: 126 return "http://hl7.org/fhir/encounter-state"; 127 case ARRIVED: 128 return "http://hl7.org/fhir/encounter-state"; 129 case INPROGRESS: 130 return "http://hl7.org/fhir/encounter-state"; 131 case ONLEAVE: 132 return "http://hl7.org/fhir/encounter-state"; 133 case FINISHED: 134 return "http://hl7.org/fhir/encounter-state"; 135 case CANCELLED: 136 return "http://hl7.org/fhir/encounter-state"; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144 public String getDefinition() { 145 switch (this) { 146 case PLANNED: 147 return "The Encounter has not yet started."; 148 case ARRIVED: 149 return "The Patient is present for the encounter, however is not currently meeting with a practitioner."; 150 case INPROGRESS: 151 return "The Encounter has begun and the patient is present / the practitioner and the patient are meeting."; 152 case ONLEAVE: 153 return "The Encounter has begun, but the patient is temporarily on leave."; 154 case FINISHED: 155 return "The Encounter has ended."; 156 case CANCELLED: 157 return "The Encounter has ended before it has begun."; 158 case NULL: 159 return null; 160 default: 161 return "?"; 162 } 163 } 164 165 public String getDisplay() { 166 switch (this) { 167 case PLANNED: 168 return "Planned"; 169 case ARRIVED: 170 return "Arrived"; 171 case INPROGRESS: 172 return "in Progress"; 173 case ONLEAVE: 174 return "On Leave"; 175 case FINISHED: 176 return "Finished"; 177 case CANCELLED: 178 return "Cancelled"; 179 case NULL: 180 return null; 181 default: 182 return "?"; 183 } 184 } 185 } 186 187 public static class EncounterStateEnumFactory implements EnumFactory<EncounterState> { 188 public EncounterState fromCode(String codeString) throws IllegalArgumentException { 189 if (codeString == null || "".equals(codeString)) 190 if (codeString == null || "".equals(codeString)) 191 return null; 192 if ("planned".equals(codeString)) 193 return EncounterState.PLANNED; 194 if ("arrived".equals(codeString)) 195 return EncounterState.ARRIVED; 196 if ("in-progress".equals(codeString)) 197 return EncounterState.INPROGRESS; 198 if ("onleave".equals(codeString)) 199 return EncounterState.ONLEAVE; 200 if ("finished".equals(codeString)) 201 return EncounterState.FINISHED; 202 if ("cancelled".equals(codeString)) 203 return EncounterState.CANCELLED; 204 throw new IllegalArgumentException("Unknown EncounterState code '" + codeString + "'"); 205 } 206 207 public Enumeration<EncounterState> fromType(Base code) throws FHIRException { 208 if (code == null || code.isEmpty()) 209 return null; 210 String codeString = ((PrimitiveType) code).asStringValue(); 211 if (codeString == null || "".equals(codeString)) 212 return null; 213 if ("planned".equals(codeString)) 214 return new Enumeration<EncounterState>(this, EncounterState.PLANNED); 215 if ("arrived".equals(codeString)) 216 return new Enumeration<EncounterState>(this, EncounterState.ARRIVED); 217 if ("in-progress".equals(codeString)) 218 return new Enumeration<EncounterState>(this, EncounterState.INPROGRESS); 219 if ("onleave".equals(codeString)) 220 return new Enumeration<EncounterState>(this, EncounterState.ONLEAVE); 221 if ("finished".equals(codeString)) 222 return new Enumeration<EncounterState>(this, EncounterState.FINISHED); 223 if ("cancelled".equals(codeString)) 224 return new Enumeration<EncounterState>(this, EncounterState.CANCELLED); 225 throw new FHIRException("Unknown EncounterState code '" + codeString + "'"); 226 } 227 228 public String toCode(EncounterState code) { 229 if (code == EncounterState.PLANNED) 230 return "planned"; 231 if (code == EncounterState.ARRIVED) 232 return "arrived"; 233 if (code == EncounterState.INPROGRESS) 234 return "in-progress"; 235 if (code == EncounterState.ONLEAVE) 236 return "onleave"; 237 if (code == EncounterState.FINISHED) 238 return "finished"; 239 if (code == EncounterState.CANCELLED) 240 return "cancelled"; 241 return "?"; 242 } 243 } 244 245 public enum EncounterClass { 246 /** 247 * An encounter during which the patient is hospitalized and stays overnight. 248 */ 249 INPATIENT, 250 /** 251 * An encounter during which the patient is not hospitalized overnight. 252 */ 253 OUTPATIENT, 254 /** 255 * An encounter where the patient visits the practitioner in his/her office, 256 * e.g. a G.P. visit. 257 */ 258 AMBULATORY, 259 /** 260 * An encounter in the Emergency Care Department. 261 */ 262 EMERGENCY, 263 /** 264 * An encounter where the practitioner visits the patient at his/her home. 265 */ 266 HOME, 267 /** 268 * An encounter taking place outside the regular environment for giving care. 269 */ 270 FIELD, 271 /** 272 * An encounter where the patient needs more prolonged treatment or 273 * investigations than outpatients, but who do not need to stay in the hospital 274 * overnight. 275 */ 276 DAYTIME, 277 /** 278 * An encounter that takes place where the patient and practitioner do not 279 * physically meet but use electronic means for contact. 280 */ 281 VIRTUAL, 282 /** 283 * Any other encounter type that is not described by one of the other values. 284 * Where this is used it is expected that an implementer will include an 285 * extension value to define what the actual other type is. 286 */ 287 OTHER, 288 /** 289 * added to help the parsers 290 */ 291 NULL; 292 293 public static EncounterClass fromCode(String codeString) throws FHIRException { 294 if (codeString == null || "".equals(codeString)) 295 return null; 296 if ("inpatient".equals(codeString)) 297 return INPATIENT; 298 if ("outpatient".equals(codeString)) 299 return OUTPATIENT; 300 if ("ambulatory".equals(codeString)) 301 return AMBULATORY; 302 if ("emergency".equals(codeString)) 303 return EMERGENCY; 304 if ("home".equals(codeString)) 305 return HOME; 306 if ("field".equals(codeString)) 307 return FIELD; 308 if ("daytime".equals(codeString)) 309 return DAYTIME; 310 if ("virtual".equals(codeString)) 311 return VIRTUAL; 312 if ("other".equals(codeString)) 313 return OTHER; 314 throw new FHIRException("Unknown EncounterClass code '" + codeString + "'"); 315 } 316 317 public String toCode() { 318 switch (this) { 319 case INPATIENT: 320 return "inpatient"; 321 case OUTPATIENT: 322 return "outpatient"; 323 case AMBULATORY: 324 return "ambulatory"; 325 case EMERGENCY: 326 return "emergency"; 327 case HOME: 328 return "home"; 329 case FIELD: 330 return "field"; 331 case DAYTIME: 332 return "daytime"; 333 case VIRTUAL: 334 return "virtual"; 335 case OTHER: 336 return "other"; 337 case NULL: 338 return null; 339 default: 340 return "?"; 341 } 342 } 343 344 public String getSystem() { 345 switch (this) { 346 case INPATIENT: 347 return "http://hl7.org/fhir/encounter-class"; 348 case OUTPATIENT: 349 return "http://hl7.org/fhir/encounter-class"; 350 case AMBULATORY: 351 return "http://hl7.org/fhir/encounter-class"; 352 case EMERGENCY: 353 return "http://hl7.org/fhir/encounter-class"; 354 case HOME: 355 return "http://hl7.org/fhir/encounter-class"; 356 case FIELD: 357 return "http://hl7.org/fhir/encounter-class"; 358 case DAYTIME: 359 return "http://hl7.org/fhir/encounter-class"; 360 case VIRTUAL: 361 return "http://hl7.org/fhir/encounter-class"; 362 case OTHER: 363 return "http://hl7.org/fhir/encounter-class"; 364 case NULL: 365 return null; 366 default: 367 return "?"; 368 } 369 } 370 371 public String getDefinition() { 372 switch (this) { 373 case INPATIENT: 374 return "An encounter during which the patient is hospitalized and stays overnight."; 375 case OUTPATIENT: 376 return "An encounter during which the patient is not hospitalized overnight."; 377 case AMBULATORY: 378 return "An encounter where the patient visits the practitioner in his/her office, e.g. a G.P. visit."; 379 case EMERGENCY: 380 return "An encounter in the Emergency Care Department."; 381 case HOME: 382 return "An encounter where the practitioner visits the patient at his/her home."; 383 case FIELD: 384 return "An encounter taking place outside the regular environment for giving care."; 385 case DAYTIME: 386 return "An encounter where the patient needs more prolonged treatment or investigations than outpatients, but who do not need to stay in the hospital overnight."; 387 case VIRTUAL: 388 return "An encounter that takes place where the patient and practitioner do not physically meet but use electronic means for contact."; 389 case OTHER: 390 return "Any other encounter type that is not described by one of the other values. Where this is used it is expected that an implementer will include an extension value to define what the actual other type is."; 391 case NULL: 392 return null; 393 default: 394 return "?"; 395 } 396 } 397 398 public String getDisplay() { 399 switch (this) { 400 case INPATIENT: 401 return "Inpatient"; 402 case OUTPATIENT: 403 return "Outpatient"; 404 case AMBULATORY: 405 return "Ambulatory"; 406 case EMERGENCY: 407 return "Emergency"; 408 case HOME: 409 return "Home"; 410 case FIELD: 411 return "Field"; 412 case DAYTIME: 413 return "Daytime"; 414 case VIRTUAL: 415 return "Virtual"; 416 case OTHER: 417 return "Other"; 418 case NULL: 419 return null; 420 default: 421 return "?"; 422 } 423 } 424 } 425 426 public static class EncounterClassEnumFactory implements EnumFactory<EncounterClass> { 427 public EncounterClass fromCode(String codeString) throws IllegalArgumentException { 428 if (codeString == null || "".equals(codeString)) 429 if (codeString == null || "".equals(codeString)) 430 return null; 431 if ("inpatient".equals(codeString)) 432 return EncounterClass.INPATIENT; 433 if ("outpatient".equals(codeString)) 434 return EncounterClass.OUTPATIENT; 435 if ("ambulatory".equals(codeString)) 436 return EncounterClass.AMBULATORY; 437 if ("emergency".equals(codeString)) 438 return EncounterClass.EMERGENCY; 439 if ("home".equals(codeString)) 440 return EncounterClass.HOME; 441 if ("field".equals(codeString)) 442 return EncounterClass.FIELD; 443 if ("daytime".equals(codeString)) 444 return EncounterClass.DAYTIME; 445 if ("virtual".equals(codeString)) 446 return EncounterClass.VIRTUAL; 447 if ("other".equals(codeString)) 448 return EncounterClass.OTHER; 449 throw new IllegalArgumentException("Unknown EncounterClass code '" + codeString + "'"); 450 } 451 452 public Enumeration<EncounterClass> fromType(Base code) throws FHIRException { 453 if (code == null || code.isEmpty()) 454 return null; 455 String codeString = ((PrimitiveType) code).asStringValue(); 456 if (codeString == null || "".equals(codeString)) 457 return null; 458 if ("inpatient".equals(codeString)) 459 return new Enumeration<EncounterClass>(this, EncounterClass.INPATIENT); 460 if ("outpatient".equals(codeString)) 461 return new Enumeration<EncounterClass>(this, EncounterClass.OUTPATIENT); 462 if ("ambulatory".equals(codeString)) 463 return new Enumeration<EncounterClass>(this, EncounterClass.AMBULATORY); 464 if ("emergency".equals(codeString)) 465 return new Enumeration<EncounterClass>(this, EncounterClass.EMERGENCY); 466 if ("home".equals(codeString)) 467 return new Enumeration<EncounterClass>(this, EncounterClass.HOME); 468 if ("field".equals(codeString)) 469 return new Enumeration<EncounterClass>(this, EncounterClass.FIELD); 470 if ("daytime".equals(codeString)) 471 return new Enumeration<EncounterClass>(this, EncounterClass.DAYTIME); 472 if ("virtual".equals(codeString)) 473 return new Enumeration<EncounterClass>(this, EncounterClass.VIRTUAL); 474 if ("other".equals(codeString)) 475 return new Enumeration<EncounterClass>(this, EncounterClass.OTHER); 476 throw new FHIRException("Unknown EncounterClass code '" + codeString + "'"); 477 } 478 479 public String toCode(EncounterClass code) { 480 if (code == EncounterClass.INPATIENT) 481 return "inpatient"; 482 if (code == EncounterClass.OUTPATIENT) 483 return "outpatient"; 484 if (code == EncounterClass.AMBULATORY) 485 return "ambulatory"; 486 if (code == EncounterClass.EMERGENCY) 487 return "emergency"; 488 if (code == EncounterClass.HOME) 489 return "home"; 490 if (code == EncounterClass.FIELD) 491 return "field"; 492 if (code == EncounterClass.DAYTIME) 493 return "daytime"; 494 if (code == EncounterClass.VIRTUAL) 495 return "virtual"; 496 if (code == EncounterClass.OTHER) 497 return "other"; 498 return "?"; 499 } 500 } 501 502 public enum EncounterLocationStatus { 503 /** 504 * The patient is planned to be moved to this location at some point in the 505 * future. 506 */ 507 PLANNED, 508 /** 509 * The patient is currently at this location, or was between the period 510 * specified. 511 * 512 * A system may update these records when the patient leaves the location to 513 * either reserved, or completed 514 */ 515 ACTIVE, 516 /** 517 * This location is held empty for this patient. 518 */ 519 RESERVED, 520 /** 521 * The patient was at this location during the period specified. 522 * 523 * Not to be used when the patient is currently at the location 524 */ 525 COMPLETED, 526 /** 527 * added to help the parsers 528 */ 529 NULL; 530 531 public static EncounterLocationStatus fromCode(String codeString) throws FHIRException { 532 if (codeString == null || "".equals(codeString)) 533 return null; 534 if ("planned".equals(codeString)) 535 return PLANNED; 536 if ("active".equals(codeString)) 537 return ACTIVE; 538 if ("reserved".equals(codeString)) 539 return RESERVED; 540 if ("completed".equals(codeString)) 541 return COMPLETED; 542 throw new FHIRException("Unknown EncounterLocationStatus code '" + codeString + "'"); 543 } 544 545 public String toCode() { 546 switch (this) { 547 case PLANNED: 548 return "planned"; 549 case ACTIVE: 550 return "active"; 551 case RESERVED: 552 return "reserved"; 553 case COMPLETED: 554 return "completed"; 555 case NULL: 556 return null; 557 default: 558 return "?"; 559 } 560 } 561 562 public String getSystem() { 563 switch (this) { 564 case PLANNED: 565 return "http://hl7.org/fhir/encounter-location-status"; 566 case ACTIVE: 567 return "http://hl7.org/fhir/encounter-location-status"; 568 case RESERVED: 569 return "http://hl7.org/fhir/encounter-location-status"; 570 case COMPLETED: 571 return "http://hl7.org/fhir/encounter-location-status"; 572 case NULL: 573 return null; 574 default: 575 return "?"; 576 } 577 } 578 579 public String getDefinition() { 580 switch (this) { 581 case PLANNED: 582 return "The patient is planned to be moved to this location at some point in the future."; 583 case ACTIVE: 584 return "The patient is currently at this location, or was between the period specified.\n\nA system may update these records when the patient leaves the location to either reserved, or completed"; 585 case RESERVED: 586 return "This location is held empty for this patient."; 587 case COMPLETED: 588 return "The patient was at this location during the period specified.\n\nNot to be used when the patient is currently at the location"; 589 case NULL: 590 return null; 591 default: 592 return "?"; 593 } 594 } 595 596 public String getDisplay() { 597 switch (this) { 598 case PLANNED: 599 return "Planned"; 600 case ACTIVE: 601 return "Active"; 602 case RESERVED: 603 return "Reserved"; 604 case COMPLETED: 605 return "Completed"; 606 case NULL: 607 return null; 608 default: 609 return "?"; 610 } 611 } 612 } 613 614 public static class EncounterLocationStatusEnumFactory implements EnumFactory<EncounterLocationStatus> { 615 public EncounterLocationStatus fromCode(String codeString) throws IllegalArgumentException { 616 if (codeString == null || "".equals(codeString)) 617 if (codeString == null || "".equals(codeString)) 618 return null; 619 if ("planned".equals(codeString)) 620 return EncounterLocationStatus.PLANNED; 621 if ("active".equals(codeString)) 622 return EncounterLocationStatus.ACTIVE; 623 if ("reserved".equals(codeString)) 624 return EncounterLocationStatus.RESERVED; 625 if ("completed".equals(codeString)) 626 return EncounterLocationStatus.COMPLETED; 627 throw new IllegalArgumentException("Unknown EncounterLocationStatus code '" + codeString + "'"); 628 } 629 630 public Enumeration<EncounterLocationStatus> fromType(Base code) throws FHIRException { 631 if (code == null || code.isEmpty()) 632 return null; 633 String codeString = ((PrimitiveType) code).asStringValue(); 634 if (codeString == null || "".equals(codeString)) 635 return null; 636 if ("planned".equals(codeString)) 637 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.PLANNED); 638 if ("active".equals(codeString)) 639 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.ACTIVE); 640 if ("reserved".equals(codeString)) 641 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.RESERVED); 642 if ("completed".equals(codeString)) 643 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.COMPLETED); 644 throw new FHIRException("Unknown EncounterLocationStatus code '" + codeString + "'"); 645 } 646 647 public String toCode(EncounterLocationStatus code) { 648 if (code == EncounterLocationStatus.PLANNED) 649 return "planned"; 650 if (code == EncounterLocationStatus.ACTIVE) 651 return "active"; 652 if (code == EncounterLocationStatus.RESERVED) 653 return "reserved"; 654 if (code == EncounterLocationStatus.COMPLETED) 655 return "completed"; 656 return "?"; 657 } 658 } 659 660 @Block() 661 public static class EncounterStatusHistoryComponent extends BackboneElement implements IBaseBackboneElement { 662 /** 663 * planned | arrived | in-progress | onleave | finished | cancelled. 664 */ 665 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 666 @Description(shortDefinition = "planned | arrived | in-progress | onleave | finished | cancelled", formalDefinition = "planned | arrived | in-progress | onleave | finished | cancelled.") 667 protected Enumeration<EncounterState> status; 668 669 /** 670 * The time that the episode was in the specified status. 671 */ 672 @Child(name = "period", type = { Period.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 673 @Description(shortDefinition = "The time that the episode was in the specified status", formalDefinition = "The time that the episode was in the specified status.") 674 protected Period period; 675 676 private static final long serialVersionUID = 919229161L; 677 678 /* 679 * Constructor 680 */ 681 public EncounterStatusHistoryComponent() { 682 super(); 683 } 684 685 /* 686 * Constructor 687 */ 688 public EncounterStatusHistoryComponent(Enumeration<EncounterState> status, Period period) { 689 super(); 690 this.status = status; 691 this.period = period; 692 } 693 694 /** 695 * @return {@link #status} (planned | arrived | in-progress | onleave | finished 696 * | cancelled.). This is the underlying object with id, value and 697 * extensions. The accessor "getStatus" gives direct access to the value 698 */ 699 public Enumeration<EncounterState> getStatusElement() { 700 if (this.status == null) 701 if (Configuration.errorOnAutoCreate()) 702 throw new Error("Attempt to auto-create EncounterStatusHistoryComponent.status"); 703 else if (Configuration.doAutoCreate()) 704 this.status = new Enumeration<EncounterState>(new EncounterStateEnumFactory()); // bb 705 return this.status; 706 } 707 708 public boolean hasStatusElement() { 709 return this.status != null && !this.status.isEmpty(); 710 } 711 712 public boolean hasStatus() { 713 return this.status != null && !this.status.isEmpty(); 714 } 715 716 /** 717 * @param value {@link #status} (planned | arrived | in-progress | onleave | 718 * finished | cancelled.). This is the underlying object with id, 719 * value and extensions. The accessor "getStatus" gives direct 720 * access to the value 721 */ 722 public EncounterStatusHistoryComponent setStatusElement(Enumeration<EncounterState> value) { 723 this.status = value; 724 return this; 725 } 726 727 /** 728 * @return planned | arrived | in-progress | onleave | finished | cancelled. 729 */ 730 public EncounterState getStatus() { 731 return this.status == null ? null : this.status.getValue(); 732 } 733 734 /** 735 * @param value planned | arrived | in-progress | onleave | finished | 736 * cancelled. 737 */ 738 public EncounterStatusHistoryComponent setStatus(EncounterState value) { 739 if (this.status == null) 740 this.status = new Enumeration<EncounterState>(new EncounterStateEnumFactory()); 741 this.status.setValue(value); 742 return this; 743 } 744 745 /** 746 * @return {@link #period} (The time that the episode was in the specified 747 * status.) 748 */ 749 public Period getPeriod() { 750 if (this.period == null) 751 if (Configuration.errorOnAutoCreate()) 752 throw new Error("Attempt to auto-create EncounterStatusHistoryComponent.period"); 753 else if (Configuration.doAutoCreate()) 754 this.period = new Period(); // cc 755 return this.period; 756 } 757 758 public boolean hasPeriod() { 759 return this.period != null && !this.period.isEmpty(); 760 } 761 762 /** 763 * @param value {@link #period} (The time that the episode was in the specified 764 * status.) 765 */ 766 public EncounterStatusHistoryComponent setPeriod(Period value) { 767 this.period = value; 768 return this; 769 } 770 771 protected void listChildren(List<Property> childrenList) { 772 super.listChildren(childrenList); 773 childrenList.add(new Property("status", "code", 774 "planned | arrived | in-progress | onleave | finished | cancelled.", 0, java.lang.Integer.MAX_VALUE, status)); 775 childrenList.add(new Property("period", "Period", "The time that the episode was in the specified status.", 0, 776 java.lang.Integer.MAX_VALUE, period)); 777 } 778 779 @Override 780 public void setProperty(String name, Base value) throws FHIRException { 781 if (name.equals("status")) 782 this.status = new EncounterStateEnumFactory().fromType(value); // Enumeration<EncounterState> 783 else if (name.equals("period")) 784 this.period = castToPeriod(value); // Period 785 else 786 super.setProperty(name, value); 787 } 788 789 @Override 790 public Base addChild(String name) throws FHIRException { 791 if (name.equals("status")) { 792 throw new FHIRException("Cannot call addChild on a singleton property Encounter.status"); 793 } else if (name.equals("period")) { 794 this.period = new Period(); 795 return this.period; 796 } else 797 return super.addChild(name); 798 } 799 800 public EncounterStatusHistoryComponent copy() { 801 EncounterStatusHistoryComponent dst = new EncounterStatusHistoryComponent(); 802 copyValues(dst); 803 dst.status = status == null ? null : status.copy(); 804 dst.period = period == null ? null : period.copy(); 805 return dst; 806 } 807 808 @Override 809 public boolean equalsDeep(Base other) { 810 if (!super.equalsDeep(other)) 811 return false; 812 if (!(other instanceof EncounterStatusHistoryComponent)) 813 return false; 814 EncounterStatusHistoryComponent o = (EncounterStatusHistoryComponent) other; 815 return compareDeep(status, o.status, true) && compareDeep(period, o.period, true); 816 } 817 818 @Override 819 public boolean equalsShallow(Base other) { 820 if (!super.equalsShallow(other)) 821 return false; 822 if (!(other instanceof EncounterStatusHistoryComponent)) 823 return false; 824 EncounterStatusHistoryComponent o = (EncounterStatusHistoryComponent) other; 825 return compareValues(status, o.status, true); 826 } 827 828 public boolean isEmpty() { 829 return super.isEmpty() && (status == null || status.isEmpty()) && (period == null || period.isEmpty()); 830 } 831 832 public String fhirType() { 833 return "Encounter.statusHistory"; 834 835 } 836 837 } 838 839 @Block() 840 public static class EncounterParticipantComponent extends BackboneElement implements IBaseBackboneElement { 841 /** 842 * Role of participant in encounter. 843 */ 844 @Child(name = "type", type = { 845 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 846 @Description(shortDefinition = "Role of participant in encounter", formalDefinition = "Role of participant in encounter.") 847 protected List<CodeableConcept> type; 848 849 /** 850 * The period of time that the specified participant was present during the 851 * encounter. These can overlap or be sub-sets of the overall encounters period. 852 */ 853 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 854 @Description(shortDefinition = "Period of time during the encounter participant was present", formalDefinition = "The period of time that the specified participant was present during the encounter. These can overlap or be sub-sets of the overall encounters period.") 855 protected Period period; 856 857 /** 858 * Persons involved in the encounter other than the patient. 859 */ 860 @Child(name = "individual", type = { Practitioner.class, 861 RelatedPerson.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 862 @Description(shortDefinition = "Persons involved in the encounter other than the patient", formalDefinition = "Persons involved in the encounter other than the patient.") 863 protected Reference individual; 864 865 /** 866 * The actual object that is the target of the reference (Persons involved in 867 * the encounter other than the patient.) 868 */ 869 protected Resource individualTarget; 870 871 private static final long serialVersionUID = 317095765L; 872 873 /* 874 * Constructor 875 */ 876 public EncounterParticipantComponent() { 877 super(); 878 } 879 880 /** 881 * @return {@link #type} (Role of participant in encounter.) 882 */ 883 public List<CodeableConcept> getType() { 884 if (this.type == null) 885 this.type = new ArrayList<CodeableConcept>(); 886 return this.type; 887 } 888 889 public boolean hasType() { 890 if (this.type == null) 891 return false; 892 for (CodeableConcept item : this.type) 893 if (!item.isEmpty()) 894 return true; 895 return false; 896 } 897 898 /** 899 * @return {@link #type} (Role of participant in encounter.) 900 */ 901 // syntactic sugar 902 public CodeableConcept addType() { // 3 903 CodeableConcept t = new CodeableConcept(); 904 if (this.type == null) 905 this.type = new ArrayList<CodeableConcept>(); 906 this.type.add(t); 907 return t; 908 } 909 910 // syntactic sugar 911 public EncounterParticipantComponent addType(CodeableConcept t) { // 3 912 if (t == null) 913 return this; 914 if (this.type == null) 915 this.type = new ArrayList<CodeableConcept>(); 916 this.type.add(t); 917 return this; 918 } 919 920 /** 921 * @return {@link #period} (The period of time that the specified participant 922 * was present during the encounter. These can overlap or be sub-sets of 923 * the overall encounters period.) 924 */ 925 public Period getPeriod() { 926 if (this.period == null) 927 if (Configuration.errorOnAutoCreate()) 928 throw new Error("Attempt to auto-create EncounterParticipantComponent.period"); 929 else if (Configuration.doAutoCreate()) 930 this.period = new Period(); // cc 931 return this.period; 932 } 933 934 public boolean hasPeriod() { 935 return this.period != null && !this.period.isEmpty(); 936 } 937 938 /** 939 * @param value {@link #period} (The period of time that the specified 940 * participant was present during the encounter. These can overlap 941 * or be sub-sets of the overall encounters period.) 942 */ 943 public EncounterParticipantComponent setPeriod(Period value) { 944 this.period = value; 945 return this; 946 } 947 948 /** 949 * @return {@link #individual} (Persons involved in the encounter other than the 950 * patient.) 951 */ 952 public Reference getIndividual() { 953 if (this.individual == null) 954 if (Configuration.errorOnAutoCreate()) 955 throw new Error("Attempt to auto-create EncounterParticipantComponent.individual"); 956 else if (Configuration.doAutoCreate()) 957 this.individual = new Reference(); // cc 958 return this.individual; 959 } 960 961 public boolean hasIndividual() { 962 return this.individual != null && !this.individual.isEmpty(); 963 } 964 965 /** 966 * @param value {@link #individual} (Persons involved in the encounter other 967 * than the patient.) 968 */ 969 public EncounterParticipantComponent setIndividual(Reference value) { 970 this.individual = value; 971 return this; 972 } 973 974 /** 975 * @return {@link #individual} The actual object that is the target of the 976 * reference. The reference library doesn't populate this, but you can 977 * use it to hold the resource if you resolve it. (Persons involved in 978 * the encounter other than the patient.) 979 */ 980 public Resource getIndividualTarget() { 981 return this.individualTarget; 982 } 983 984 /** 985 * @param value {@link #individual} The actual object that is the target of the 986 * reference. The reference library doesn't use these, but you can 987 * use it to hold the resource if you resolve it. (Persons involved 988 * in the encounter other than the patient.) 989 */ 990 public EncounterParticipantComponent setIndividualTarget(Resource value) { 991 this.individualTarget = value; 992 return this; 993 } 994 995 protected void listChildren(List<Property> childrenList) { 996 super.listChildren(childrenList); 997 childrenList.add(new Property("type", "CodeableConcept", "Role of participant in encounter.", 0, 998 java.lang.Integer.MAX_VALUE, type)); 999 childrenList.add(new Property("period", "Period", 1000 "The period of time that the specified participant was present during the encounter. These can overlap or be sub-sets of the overall encounters period.", 1001 0, java.lang.Integer.MAX_VALUE, period)); 1002 childrenList.add(new Property("individual", "Reference(Practitioner|RelatedPerson)", 1003 "Persons involved in the encounter other than the patient.", 0, java.lang.Integer.MAX_VALUE, individual)); 1004 } 1005 1006 @Override 1007 public void setProperty(String name, Base value) throws FHIRException { 1008 if (name.equals("type")) 1009 this.getType().add(castToCodeableConcept(value)); 1010 else if (name.equals("period")) 1011 this.period = castToPeriod(value); // Period 1012 else if (name.equals("individual")) 1013 this.individual = castToReference(value); // Reference 1014 else 1015 super.setProperty(name, value); 1016 } 1017 1018 @Override 1019 public Base addChild(String name) throws FHIRException { 1020 if (name.equals("type")) { 1021 return addType(); 1022 } else if (name.equals("period")) { 1023 this.period = new Period(); 1024 return this.period; 1025 } else if (name.equals("individual")) { 1026 this.individual = new Reference(); 1027 return this.individual; 1028 } else 1029 return super.addChild(name); 1030 } 1031 1032 public EncounterParticipantComponent copy() { 1033 EncounterParticipantComponent dst = new EncounterParticipantComponent(); 1034 copyValues(dst); 1035 if (type != null) { 1036 dst.type = new ArrayList<CodeableConcept>(); 1037 for (CodeableConcept i : type) 1038 dst.type.add(i.copy()); 1039 } 1040 ; 1041 dst.period = period == null ? null : period.copy(); 1042 dst.individual = individual == null ? null : individual.copy(); 1043 return dst; 1044 } 1045 1046 @Override 1047 public boolean equalsDeep(Base other) { 1048 if (!super.equalsDeep(other)) 1049 return false; 1050 if (!(other instanceof EncounterParticipantComponent)) 1051 return false; 1052 EncounterParticipantComponent o = (EncounterParticipantComponent) other; 1053 return compareDeep(type, o.type, true) && compareDeep(period, o.period, true) 1054 && compareDeep(individual, o.individual, true); 1055 } 1056 1057 @Override 1058 public boolean equalsShallow(Base other) { 1059 if (!super.equalsShallow(other)) 1060 return false; 1061 if (!(other instanceof EncounterParticipantComponent)) 1062 return false; 1063 EncounterParticipantComponent o = (EncounterParticipantComponent) other; 1064 return true; 1065 } 1066 1067 public boolean isEmpty() { 1068 return super.isEmpty() && (type == null || type.isEmpty()) && (period == null || period.isEmpty()) 1069 && (individual == null || individual.isEmpty()); 1070 } 1071 1072 public String fhirType() { 1073 return "Encounter.participant"; 1074 1075 } 1076 1077 } 1078 1079 @Block() 1080 public static class EncounterHospitalizationComponent extends BackboneElement implements IBaseBackboneElement { 1081 /** 1082 * Pre-admission identifier. 1083 */ 1084 @Child(name = "preAdmissionIdentifier", type = { 1085 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1086 @Description(shortDefinition = "Pre-admission identifier", formalDefinition = "Pre-admission identifier.") 1087 protected Identifier preAdmissionIdentifier; 1088 1089 /** 1090 * The location from which the patient came before admission. 1091 */ 1092 @Child(name = "origin", type = { Location.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1093 @Description(shortDefinition = "The location from which the patient came before admission", formalDefinition = "The location from which the patient came before admission.") 1094 protected Reference origin; 1095 1096 /** 1097 * The actual object that is the target of the reference (The location from 1098 * which the patient came before admission.) 1099 */ 1100 protected Location originTarget; 1101 1102 /** 1103 * From where patient was admitted (physician referral, transfer). 1104 */ 1105 @Child(name = "admitSource", type = { 1106 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1107 @Description(shortDefinition = "From where patient was admitted (physician referral, transfer)", formalDefinition = "From where patient was admitted (physician referral, transfer).") 1108 protected CodeableConcept admitSource; 1109 1110 /** 1111 * The admitting diagnosis field is used to record the diagnosis codes as 1112 * reported by admitting practitioner. This could be different or in addition to 1113 * the conditions reported as reason-condition(s) for the encounter. 1114 */ 1115 @Child(name = "admittingDiagnosis", type = { 1116 Condition.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1117 @Description(shortDefinition = "The admitting diagnosis as reported by admitting practitioner", formalDefinition = "The admitting diagnosis field is used to record the diagnosis codes as reported by admitting practitioner. This could be different or in addition to the conditions reported as reason-condition(s) for the encounter.") 1118 protected List<Reference> admittingDiagnosis; 1119 /** 1120 * The actual objects that are the target of the reference (The admitting 1121 * diagnosis field is used to record the diagnosis codes as reported by 1122 * admitting practitioner. This could be different or in addition to the 1123 * conditions reported as reason-condition(s) for the encounter.) 1124 */ 1125 protected List<Condition> admittingDiagnosisTarget; 1126 1127 /** 1128 * Whether this hospitalization is a readmission and why if known. 1129 */ 1130 @Child(name = "reAdmission", type = { 1131 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1132 @Description(shortDefinition = "The type of hospital re-admission that has occurred (if any). If the value is absent, then this is not identified as a readmission", formalDefinition = "Whether this hospitalization is a readmission and why if known.") 1133 protected CodeableConcept reAdmission; 1134 1135 /** 1136 * Diet preferences reported by the patient. 1137 */ 1138 @Child(name = "dietPreference", type = { 1139 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1140 @Description(shortDefinition = "Diet preferences reported by the patient", formalDefinition = "Diet preferences reported by the patient.") 1141 protected List<CodeableConcept> dietPreference; 1142 1143 /** 1144 * Special courtesies (VIP, board member). 1145 */ 1146 @Child(name = "specialCourtesy", type = { 1147 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1148 @Description(shortDefinition = "Special courtesies (VIP, board member)", formalDefinition = "Special courtesies (VIP, board member).") 1149 protected List<CodeableConcept> specialCourtesy; 1150 1151 /** 1152 * Wheelchair, translator, stretcher, etc. 1153 */ 1154 @Child(name = "specialArrangement", type = { 1155 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1156 @Description(shortDefinition = "Wheelchair, translator, stretcher, etc.", formalDefinition = "Wheelchair, translator, stretcher, etc.") 1157 protected List<CodeableConcept> specialArrangement; 1158 1159 /** 1160 * Location to which the patient is discharged. 1161 */ 1162 @Child(name = "destination", type = { 1163 Location.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1164 @Description(shortDefinition = "Location to which the patient is discharged", formalDefinition = "Location to which the patient is discharged.") 1165 protected Reference destination; 1166 1167 /** 1168 * The actual object that is the target of the reference (Location to which the 1169 * patient is discharged.) 1170 */ 1171 protected Location destinationTarget; 1172 1173 /** 1174 * Category or kind of location after discharge. 1175 */ 1176 @Child(name = "dischargeDisposition", type = { 1177 CodeableConcept.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1178 @Description(shortDefinition = "Category or kind of location after discharge", formalDefinition = "Category or kind of location after discharge.") 1179 protected CodeableConcept dischargeDisposition; 1180 1181 /** 1182 * The final diagnosis given a patient before release from the hospital after 1183 * all testing, surgery, and workup are complete. 1184 */ 1185 @Child(name = "dischargeDiagnosis", type = { 1186 Condition.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1187 @Description(shortDefinition = "The final diagnosis given a patient before release from the hospital after all testing, surgery, and workup are complete", formalDefinition = "The final diagnosis given a patient before release from the hospital after all testing, surgery, and workup are complete.") 1188 protected List<Reference> dischargeDiagnosis; 1189 /** 1190 * The actual objects that are the target of the reference (The final diagnosis 1191 * given a patient before release from the hospital after all testing, surgery, 1192 * and workup are complete.) 1193 */ 1194 protected List<Condition> dischargeDiagnosisTarget; 1195 1196 private static final long serialVersionUID = 164618034L; 1197 1198 /* 1199 * Constructor 1200 */ 1201 public EncounterHospitalizationComponent() { 1202 super(); 1203 } 1204 1205 /** 1206 * @return {@link #preAdmissionIdentifier} (Pre-admission identifier.) 1207 */ 1208 public Identifier getPreAdmissionIdentifier() { 1209 if (this.preAdmissionIdentifier == null) 1210 if (Configuration.errorOnAutoCreate()) 1211 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.preAdmissionIdentifier"); 1212 else if (Configuration.doAutoCreate()) 1213 this.preAdmissionIdentifier = new Identifier(); // cc 1214 return this.preAdmissionIdentifier; 1215 } 1216 1217 public boolean hasPreAdmissionIdentifier() { 1218 return this.preAdmissionIdentifier != null && !this.preAdmissionIdentifier.isEmpty(); 1219 } 1220 1221 /** 1222 * @param value {@link #preAdmissionIdentifier} (Pre-admission identifier.) 1223 */ 1224 public EncounterHospitalizationComponent setPreAdmissionIdentifier(Identifier value) { 1225 this.preAdmissionIdentifier = value; 1226 return this; 1227 } 1228 1229 /** 1230 * @return {@link #origin} (The location from which the patient came before 1231 * admission.) 1232 */ 1233 public Reference getOrigin() { 1234 if (this.origin == null) 1235 if (Configuration.errorOnAutoCreate()) 1236 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.origin"); 1237 else if (Configuration.doAutoCreate()) 1238 this.origin = new Reference(); // cc 1239 return this.origin; 1240 } 1241 1242 public boolean hasOrigin() { 1243 return this.origin != null && !this.origin.isEmpty(); 1244 } 1245 1246 /** 1247 * @param value {@link #origin} (The location from which the patient came before 1248 * admission.) 1249 */ 1250 public EncounterHospitalizationComponent setOrigin(Reference value) { 1251 this.origin = value; 1252 return this; 1253 } 1254 1255 /** 1256 * @return {@link #origin} The actual object that is the target of the 1257 * reference. The reference library doesn't populate this, but you can 1258 * use it to hold the resource if you resolve it. (The location from 1259 * which the patient came before admission.) 1260 */ 1261 public Location getOriginTarget() { 1262 if (this.originTarget == null) 1263 if (Configuration.errorOnAutoCreate()) 1264 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.origin"); 1265 else if (Configuration.doAutoCreate()) 1266 this.originTarget = new Location(); // aa 1267 return this.originTarget; 1268 } 1269 1270 /** 1271 * @param value {@link #origin} The actual object that is the target of the 1272 * reference. The reference library doesn't use these, but you can 1273 * use it to hold the resource if you resolve it. (The location 1274 * from which the patient came before admission.) 1275 */ 1276 public EncounterHospitalizationComponent setOriginTarget(Location value) { 1277 this.originTarget = value; 1278 return this; 1279 } 1280 1281 /** 1282 * @return {@link #admitSource} (From where patient was admitted (physician 1283 * referral, transfer).) 1284 */ 1285 public CodeableConcept getAdmitSource() { 1286 if (this.admitSource == null) 1287 if (Configuration.errorOnAutoCreate()) 1288 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.admitSource"); 1289 else if (Configuration.doAutoCreate()) 1290 this.admitSource = new CodeableConcept(); // cc 1291 return this.admitSource; 1292 } 1293 1294 public boolean hasAdmitSource() { 1295 return this.admitSource != null && !this.admitSource.isEmpty(); 1296 } 1297 1298 /** 1299 * @param value {@link #admitSource} (From where patient was admitted (physician 1300 * referral, transfer).) 1301 */ 1302 public EncounterHospitalizationComponent setAdmitSource(CodeableConcept value) { 1303 this.admitSource = value; 1304 return this; 1305 } 1306 1307 /** 1308 * @return {@link #admittingDiagnosis} (The admitting diagnosis field is used to 1309 * record the diagnosis codes as reported by admitting practitioner. 1310 * This could be different or in addition to the conditions reported as 1311 * reason-condition(s) for the encounter.) 1312 */ 1313 public List<Reference> getAdmittingDiagnosis() { 1314 if (this.admittingDiagnosis == null) 1315 this.admittingDiagnosis = new ArrayList<Reference>(); 1316 return this.admittingDiagnosis; 1317 } 1318 1319 public boolean hasAdmittingDiagnosis() { 1320 if (this.admittingDiagnosis == null) 1321 return false; 1322 for (Reference item : this.admittingDiagnosis) 1323 if (!item.isEmpty()) 1324 return true; 1325 return false; 1326 } 1327 1328 /** 1329 * @return {@link #admittingDiagnosis} (The admitting diagnosis field is used to 1330 * record the diagnosis codes as reported by admitting practitioner. 1331 * This could be different or in addition to the conditions reported as 1332 * reason-condition(s) for the encounter.) 1333 */ 1334 // syntactic sugar 1335 public Reference addAdmittingDiagnosis() { // 3 1336 Reference t = new Reference(); 1337 if (this.admittingDiagnosis == null) 1338 this.admittingDiagnosis = new ArrayList<Reference>(); 1339 this.admittingDiagnosis.add(t); 1340 return t; 1341 } 1342 1343 // syntactic sugar 1344 public EncounterHospitalizationComponent addAdmittingDiagnosis(Reference t) { // 3 1345 if (t == null) 1346 return this; 1347 if (this.admittingDiagnosis == null) 1348 this.admittingDiagnosis = new ArrayList<Reference>(); 1349 this.admittingDiagnosis.add(t); 1350 return this; 1351 } 1352 1353 /** 1354 * @return {@link #admittingDiagnosis} (The actual objects that are the target 1355 * of the reference. The reference library doesn't populate this, but 1356 * you can use this to hold the resources if you resolvethemt. The 1357 * admitting diagnosis field is used to record the diagnosis codes as 1358 * reported by admitting practitioner. This could be different or in 1359 * addition to the conditions reported as reason-condition(s) for the 1360 * encounter.) 1361 */ 1362 public List<Condition> getAdmittingDiagnosisTarget() { 1363 if (this.admittingDiagnosisTarget == null) 1364 this.admittingDiagnosisTarget = new ArrayList<Condition>(); 1365 return this.admittingDiagnosisTarget; 1366 } 1367 1368 // syntactic sugar 1369 /** 1370 * @return {@link #admittingDiagnosis} (Add an actual object that is the target 1371 * of the reference. The reference library doesn't use these, but you 1372 * can use this to hold the resources if you resolvethemt. The admitting 1373 * diagnosis field is used to record the diagnosis codes as reported by 1374 * admitting practitioner. This could be different or in addition to the 1375 * conditions reported as reason-condition(s) for the encounter.) 1376 */ 1377 public Condition addAdmittingDiagnosisTarget() { 1378 Condition r = new Condition(); 1379 if (this.admittingDiagnosisTarget == null) 1380 this.admittingDiagnosisTarget = new ArrayList<Condition>(); 1381 this.admittingDiagnosisTarget.add(r); 1382 return r; 1383 } 1384 1385 /** 1386 * @return {@link #reAdmission} (Whether this hospitalization is a readmission 1387 * and why if known.) 1388 */ 1389 public CodeableConcept getReAdmission() { 1390 if (this.reAdmission == null) 1391 if (Configuration.errorOnAutoCreate()) 1392 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.reAdmission"); 1393 else if (Configuration.doAutoCreate()) 1394 this.reAdmission = new CodeableConcept(); // cc 1395 return this.reAdmission; 1396 } 1397 1398 public boolean hasReAdmission() { 1399 return this.reAdmission != null && !this.reAdmission.isEmpty(); 1400 } 1401 1402 /** 1403 * @param value {@link #reAdmission} (Whether this hospitalization is a 1404 * readmission and why if known.) 1405 */ 1406 public EncounterHospitalizationComponent setReAdmission(CodeableConcept value) { 1407 this.reAdmission = value; 1408 return this; 1409 } 1410 1411 /** 1412 * @return {@link #dietPreference} (Diet preferences reported by the patient.) 1413 */ 1414 public List<CodeableConcept> getDietPreference() { 1415 if (this.dietPreference == null) 1416 this.dietPreference = new ArrayList<CodeableConcept>(); 1417 return this.dietPreference; 1418 } 1419 1420 public boolean hasDietPreference() { 1421 if (this.dietPreference == null) 1422 return false; 1423 for (CodeableConcept item : this.dietPreference) 1424 if (!item.isEmpty()) 1425 return true; 1426 return false; 1427 } 1428 1429 /** 1430 * @return {@link #dietPreference} (Diet preferences reported by the patient.) 1431 */ 1432 // syntactic sugar 1433 public CodeableConcept addDietPreference() { // 3 1434 CodeableConcept t = new CodeableConcept(); 1435 if (this.dietPreference == null) 1436 this.dietPreference = new ArrayList<CodeableConcept>(); 1437 this.dietPreference.add(t); 1438 return t; 1439 } 1440 1441 // syntactic sugar 1442 public EncounterHospitalizationComponent addDietPreference(CodeableConcept t) { // 3 1443 if (t == null) 1444 return this; 1445 if (this.dietPreference == null) 1446 this.dietPreference = new ArrayList<CodeableConcept>(); 1447 this.dietPreference.add(t); 1448 return this; 1449 } 1450 1451 /** 1452 * @return {@link #specialCourtesy} (Special courtesies (VIP, board member).) 1453 */ 1454 public List<CodeableConcept> getSpecialCourtesy() { 1455 if (this.specialCourtesy == null) 1456 this.specialCourtesy = new ArrayList<CodeableConcept>(); 1457 return this.specialCourtesy; 1458 } 1459 1460 public boolean hasSpecialCourtesy() { 1461 if (this.specialCourtesy == null) 1462 return false; 1463 for (CodeableConcept item : this.specialCourtesy) 1464 if (!item.isEmpty()) 1465 return true; 1466 return false; 1467 } 1468 1469 /** 1470 * @return {@link #specialCourtesy} (Special courtesies (VIP, board member).) 1471 */ 1472 // syntactic sugar 1473 public CodeableConcept addSpecialCourtesy() { // 3 1474 CodeableConcept t = new CodeableConcept(); 1475 if (this.specialCourtesy == null) 1476 this.specialCourtesy = new ArrayList<CodeableConcept>(); 1477 this.specialCourtesy.add(t); 1478 return t; 1479 } 1480 1481 // syntactic sugar 1482 public EncounterHospitalizationComponent addSpecialCourtesy(CodeableConcept t) { // 3 1483 if (t == null) 1484 return this; 1485 if (this.specialCourtesy == null) 1486 this.specialCourtesy = new ArrayList<CodeableConcept>(); 1487 this.specialCourtesy.add(t); 1488 return this; 1489 } 1490 1491 /** 1492 * @return {@link #specialArrangement} (Wheelchair, translator, stretcher, etc.) 1493 */ 1494 public List<CodeableConcept> getSpecialArrangement() { 1495 if (this.specialArrangement == null) 1496 this.specialArrangement = new ArrayList<CodeableConcept>(); 1497 return this.specialArrangement; 1498 } 1499 1500 public boolean hasSpecialArrangement() { 1501 if (this.specialArrangement == null) 1502 return false; 1503 for (CodeableConcept item : this.specialArrangement) 1504 if (!item.isEmpty()) 1505 return true; 1506 return false; 1507 } 1508 1509 /** 1510 * @return {@link #specialArrangement} (Wheelchair, translator, stretcher, etc.) 1511 */ 1512 // syntactic sugar 1513 public CodeableConcept addSpecialArrangement() { // 3 1514 CodeableConcept t = new CodeableConcept(); 1515 if (this.specialArrangement == null) 1516 this.specialArrangement = new ArrayList<CodeableConcept>(); 1517 this.specialArrangement.add(t); 1518 return t; 1519 } 1520 1521 // syntactic sugar 1522 public EncounterHospitalizationComponent addSpecialArrangement(CodeableConcept t) { // 3 1523 if (t == null) 1524 return this; 1525 if (this.specialArrangement == null) 1526 this.specialArrangement = new ArrayList<CodeableConcept>(); 1527 this.specialArrangement.add(t); 1528 return this; 1529 } 1530 1531 /** 1532 * @return {@link #destination} (Location to which the patient is discharged.) 1533 */ 1534 public Reference getDestination() { 1535 if (this.destination == null) 1536 if (Configuration.errorOnAutoCreate()) 1537 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.destination"); 1538 else if (Configuration.doAutoCreate()) 1539 this.destination = new Reference(); // cc 1540 return this.destination; 1541 } 1542 1543 public boolean hasDestination() { 1544 return this.destination != null && !this.destination.isEmpty(); 1545 } 1546 1547 /** 1548 * @param value {@link #destination} (Location to which the patient is 1549 * discharged.) 1550 */ 1551 public EncounterHospitalizationComponent setDestination(Reference value) { 1552 this.destination = value; 1553 return this; 1554 } 1555 1556 /** 1557 * @return {@link #destination} The actual object that is the target of the 1558 * reference. The reference library doesn't populate this, but you can 1559 * use it to hold the resource if you resolve it. (Location to which the 1560 * patient is discharged.) 1561 */ 1562 public Location getDestinationTarget() { 1563 if (this.destinationTarget == null) 1564 if (Configuration.errorOnAutoCreate()) 1565 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.destination"); 1566 else if (Configuration.doAutoCreate()) 1567 this.destinationTarget = new Location(); // aa 1568 return this.destinationTarget; 1569 } 1570 1571 /** 1572 * @param value {@link #destination} The actual object that is the target of the 1573 * reference. The reference library doesn't use these, but you can 1574 * use it to hold the resource if you resolve it. (Location to 1575 * which the patient is discharged.) 1576 */ 1577 public EncounterHospitalizationComponent setDestinationTarget(Location value) { 1578 this.destinationTarget = value; 1579 return this; 1580 } 1581 1582 /** 1583 * @return {@link #dischargeDisposition} (Category or kind of location after 1584 * discharge.) 1585 */ 1586 public CodeableConcept getDischargeDisposition() { 1587 if (this.dischargeDisposition == null) 1588 if (Configuration.errorOnAutoCreate()) 1589 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.dischargeDisposition"); 1590 else if (Configuration.doAutoCreate()) 1591 this.dischargeDisposition = new CodeableConcept(); // cc 1592 return this.dischargeDisposition; 1593 } 1594 1595 public boolean hasDischargeDisposition() { 1596 return this.dischargeDisposition != null && !this.dischargeDisposition.isEmpty(); 1597 } 1598 1599 /** 1600 * @param value {@link #dischargeDisposition} (Category or kind of location 1601 * after discharge.) 1602 */ 1603 public EncounterHospitalizationComponent setDischargeDisposition(CodeableConcept value) { 1604 this.dischargeDisposition = value; 1605 return this; 1606 } 1607 1608 /** 1609 * @return {@link #dischargeDiagnosis} (The final diagnosis given a patient 1610 * before release from the hospital after all testing, surgery, and 1611 * workup are complete.) 1612 */ 1613 public List<Reference> getDischargeDiagnosis() { 1614 if (this.dischargeDiagnosis == null) 1615 this.dischargeDiagnosis = new ArrayList<Reference>(); 1616 return this.dischargeDiagnosis; 1617 } 1618 1619 public boolean hasDischargeDiagnosis() { 1620 if (this.dischargeDiagnosis == null) 1621 return false; 1622 for (Reference item : this.dischargeDiagnosis) 1623 if (!item.isEmpty()) 1624 return true; 1625 return false; 1626 } 1627 1628 /** 1629 * @return {@link #dischargeDiagnosis} (The final diagnosis given a patient 1630 * before release from the hospital after all testing, surgery, and 1631 * workup are complete.) 1632 */ 1633 // syntactic sugar 1634 public Reference addDischargeDiagnosis() { // 3 1635 Reference t = new Reference(); 1636 if (this.dischargeDiagnosis == null) 1637 this.dischargeDiagnosis = new ArrayList<Reference>(); 1638 this.dischargeDiagnosis.add(t); 1639 return t; 1640 } 1641 1642 // syntactic sugar 1643 public EncounterHospitalizationComponent addDischargeDiagnosis(Reference t) { // 3 1644 if (t == null) 1645 return this; 1646 if (this.dischargeDiagnosis == null) 1647 this.dischargeDiagnosis = new ArrayList<Reference>(); 1648 this.dischargeDiagnosis.add(t); 1649 return this; 1650 } 1651 1652 /** 1653 * @return {@link #dischargeDiagnosis} (The actual objects that are the target 1654 * of the reference. The reference library doesn't populate this, but 1655 * you can use this to hold the resources if you resolvethemt. The final 1656 * diagnosis given a patient before release from the hospital after all 1657 * testing, surgery, and workup are complete.) 1658 */ 1659 public List<Condition> getDischargeDiagnosisTarget() { 1660 if (this.dischargeDiagnosisTarget == null) 1661 this.dischargeDiagnosisTarget = new ArrayList<Condition>(); 1662 return this.dischargeDiagnosisTarget; 1663 } 1664 1665 // syntactic sugar 1666 /** 1667 * @return {@link #dischargeDiagnosis} (Add an actual object that is the target 1668 * of the reference. The reference library doesn't use these, but you 1669 * can use this to hold the resources if you resolvethemt. The final 1670 * diagnosis given a patient before release from the hospital after all 1671 * testing, surgery, and workup are complete.) 1672 */ 1673 public Condition addDischargeDiagnosisTarget() { 1674 Condition r = new Condition(); 1675 if (this.dischargeDiagnosisTarget == null) 1676 this.dischargeDiagnosisTarget = new ArrayList<Condition>(); 1677 this.dischargeDiagnosisTarget.add(r); 1678 return r; 1679 } 1680 1681 protected void listChildren(List<Property> childrenList) { 1682 super.listChildren(childrenList); 1683 childrenList.add(new Property("preAdmissionIdentifier", "Identifier", "Pre-admission identifier.", 0, 1684 java.lang.Integer.MAX_VALUE, preAdmissionIdentifier)); 1685 childrenList.add(new Property("origin", "Reference(Location)", 1686 "The location from which the patient came before admission.", 0, java.lang.Integer.MAX_VALUE, origin)); 1687 childrenList.add(new Property("admitSource", "CodeableConcept", 1688 "From where patient was admitted (physician referral, transfer).", 0, java.lang.Integer.MAX_VALUE, 1689 admitSource)); 1690 childrenList.add(new Property("admittingDiagnosis", "Reference(Condition)", 1691 "The admitting diagnosis field is used to record the diagnosis codes as reported by admitting practitioner. This could be different or in addition to the conditions reported as reason-condition(s) for the encounter.", 1692 0, java.lang.Integer.MAX_VALUE, admittingDiagnosis)); 1693 childrenList.add(new Property("reAdmission", "CodeableConcept", 1694 "Whether this hospitalization is a readmission and why if known.", 0, java.lang.Integer.MAX_VALUE, 1695 reAdmission)); 1696 childrenList.add(new Property("dietPreference", "CodeableConcept", "Diet preferences reported by the patient.", 0, 1697 java.lang.Integer.MAX_VALUE, dietPreference)); 1698 childrenList.add(new Property("specialCourtesy", "CodeableConcept", "Special courtesies (VIP, board member).", 0, 1699 java.lang.Integer.MAX_VALUE, specialCourtesy)); 1700 childrenList.add(new Property("specialArrangement", "CodeableConcept", "Wheelchair, translator, stretcher, etc.", 1701 0, java.lang.Integer.MAX_VALUE, specialArrangement)); 1702 childrenList.add(new Property("destination", "Reference(Location)", 1703 "Location to which the patient is discharged.", 0, java.lang.Integer.MAX_VALUE, destination)); 1704 childrenList.add(new Property("dischargeDisposition", "CodeableConcept", 1705 "Category or kind of location after discharge.", 0, java.lang.Integer.MAX_VALUE, dischargeDisposition)); 1706 childrenList.add(new Property("dischargeDiagnosis", "Reference(Condition)", 1707 "The final diagnosis given a patient before release from the hospital after all testing, surgery, and workup are complete.", 1708 0, java.lang.Integer.MAX_VALUE, dischargeDiagnosis)); 1709 } 1710 1711 @Override 1712 public void setProperty(String name, Base value) throws FHIRException { 1713 if (name.equals("preAdmissionIdentifier")) 1714 this.preAdmissionIdentifier = castToIdentifier(value); // Identifier 1715 else if (name.equals("origin")) 1716 this.origin = castToReference(value); // Reference 1717 else if (name.equals("admitSource")) 1718 this.admitSource = castToCodeableConcept(value); // CodeableConcept 1719 else if (name.equals("admittingDiagnosis")) 1720 this.getAdmittingDiagnosis().add(castToReference(value)); 1721 else if (name.equals("reAdmission")) 1722 this.reAdmission = castToCodeableConcept(value); // CodeableConcept 1723 else if (name.equals("dietPreference")) 1724 this.getDietPreference().add(castToCodeableConcept(value)); 1725 else if (name.equals("specialCourtesy")) 1726 this.getSpecialCourtesy().add(castToCodeableConcept(value)); 1727 else if (name.equals("specialArrangement")) 1728 this.getSpecialArrangement().add(castToCodeableConcept(value)); 1729 else if (name.equals("destination")) 1730 this.destination = castToReference(value); // Reference 1731 else if (name.equals("dischargeDisposition")) 1732 this.dischargeDisposition = castToCodeableConcept(value); // CodeableConcept 1733 else if (name.equals("dischargeDiagnosis")) 1734 this.getDischargeDiagnosis().add(castToReference(value)); 1735 else 1736 super.setProperty(name, value); 1737 } 1738 1739 @Override 1740 public Base addChild(String name) throws FHIRException { 1741 if (name.equals("preAdmissionIdentifier")) { 1742 this.preAdmissionIdentifier = new Identifier(); 1743 return this.preAdmissionIdentifier; 1744 } else if (name.equals("origin")) { 1745 this.origin = new Reference(); 1746 return this.origin; 1747 } else if (name.equals("admitSource")) { 1748 this.admitSource = new CodeableConcept(); 1749 return this.admitSource; 1750 } else if (name.equals("admittingDiagnosis")) { 1751 return addAdmittingDiagnosis(); 1752 } else if (name.equals("reAdmission")) { 1753 this.reAdmission = new CodeableConcept(); 1754 return this.reAdmission; 1755 } else if (name.equals("dietPreference")) { 1756 return addDietPreference(); 1757 } else if (name.equals("specialCourtesy")) { 1758 return addSpecialCourtesy(); 1759 } else if (name.equals("specialArrangement")) { 1760 return addSpecialArrangement(); 1761 } else if (name.equals("destination")) { 1762 this.destination = new Reference(); 1763 return this.destination; 1764 } else if (name.equals("dischargeDisposition")) { 1765 this.dischargeDisposition = new CodeableConcept(); 1766 return this.dischargeDisposition; 1767 } else if (name.equals("dischargeDiagnosis")) { 1768 return addDischargeDiagnosis(); 1769 } else 1770 return super.addChild(name); 1771 } 1772 1773 public EncounterHospitalizationComponent copy() { 1774 EncounterHospitalizationComponent dst = new EncounterHospitalizationComponent(); 1775 copyValues(dst); 1776 dst.preAdmissionIdentifier = preAdmissionIdentifier == null ? null : preAdmissionIdentifier.copy(); 1777 dst.origin = origin == null ? null : origin.copy(); 1778 dst.admitSource = admitSource == null ? null : admitSource.copy(); 1779 if (admittingDiagnosis != null) { 1780 dst.admittingDiagnosis = new ArrayList<Reference>(); 1781 for (Reference i : admittingDiagnosis) 1782 dst.admittingDiagnosis.add(i.copy()); 1783 } 1784 ; 1785 dst.reAdmission = reAdmission == null ? null : reAdmission.copy(); 1786 if (dietPreference != null) { 1787 dst.dietPreference = new ArrayList<CodeableConcept>(); 1788 for (CodeableConcept i : dietPreference) 1789 dst.dietPreference.add(i.copy()); 1790 } 1791 ; 1792 if (specialCourtesy != null) { 1793 dst.specialCourtesy = new ArrayList<CodeableConcept>(); 1794 for (CodeableConcept i : specialCourtesy) 1795 dst.specialCourtesy.add(i.copy()); 1796 } 1797 ; 1798 if (specialArrangement != null) { 1799 dst.specialArrangement = new ArrayList<CodeableConcept>(); 1800 for (CodeableConcept i : specialArrangement) 1801 dst.specialArrangement.add(i.copy()); 1802 } 1803 ; 1804 dst.destination = destination == null ? null : destination.copy(); 1805 dst.dischargeDisposition = dischargeDisposition == null ? null : dischargeDisposition.copy(); 1806 if (dischargeDiagnosis != null) { 1807 dst.dischargeDiagnosis = new ArrayList<Reference>(); 1808 for (Reference i : dischargeDiagnosis) 1809 dst.dischargeDiagnosis.add(i.copy()); 1810 } 1811 ; 1812 return dst; 1813 } 1814 1815 @Override 1816 public boolean equalsDeep(Base other) { 1817 if (!super.equalsDeep(other)) 1818 return false; 1819 if (!(other instanceof EncounterHospitalizationComponent)) 1820 return false; 1821 EncounterHospitalizationComponent o = (EncounterHospitalizationComponent) other; 1822 return compareDeep(preAdmissionIdentifier, o.preAdmissionIdentifier, true) && compareDeep(origin, o.origin, true) 1823 && compareDeep(admitSource, o.admitSource, true) 1824 && compareDeep(admittingDiagnosis, o.admittingDiagnosis, true) 1825 && compareDeep(reAdmission, o.reAdmission, true) && compareDeep(dietPreference, o.dietPreference, true) 1826 && compareDeep(specialCourtesy, o.specialCourtesy, true) 1827 && compareDeep(specialArrangement, o.specialArrangement, true) 1828 && compareDeep(destination, o.destination, true) 1829 && compareDeep(dischargeDisposition, o.dischargeDisposition, true) 1830 && compareDeep(dischargeDiagnosis, o.dischargeDiagnosis, true); 1831 } 1832 1833 @Override 1834 public boolean equalsShallow(Base other) { 1835 if (!super.equalsShallow(other)) 1836 return false; 1837 if (!(other instanceof EncounterHospitalizationComponent)) 1838 return false; 1839 EncounterHospitalizationComponent o = (EncounterHospitalizationComponent) other; 1840 return true; 1841 } 1842 1843 public boolean isEmpty() { 1844 return super.isEmpty() && (preAdmissionIdentifier == null || preAdmissionIdentifier.isEmpty()) 1845 && (origin == null || origin.isEmpty()) && (admitSource == null || admitSource.isEmpty()) 1846 && (admittingDiagnosis == null || admittingDiagnosis.isEmpty()) 1847 && (reAdmission == null || reAdmission.isEmpty()) && (dietPreference == null || dietPreference.isEmpty()) 1848 && (specialCourtesy == null || specialCourtesy.isEmpty()) 1849 && (specialArrangement == null || specialArrangement.isEmpty()) 1850 && (destination == null || destination.isEmpty()) 1851 && (dischargeDisposition == null || dischargeDisposition.isEmpty()) 1852 && (dischargeDiagnosis == null || dischargeDiagnosis.isEmpty()); 1853 } 1854 1855 public String fhirType() { 1856 return "Encounter.hospitalization"; 1857 1858 } 1859 1860 } 1861 1862 @Block() 1863 public static class EncounterLocationComponent extends BackboneElement implements IBaseBackboneElement { 1864 /** 1865 * The location where the encounter takes place. 1866 */ 1867 @Child(name = "location", type = { Location.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1868 @Description(shortDefinition = "Location the encounter takes place", formalDefinition = "The location where the encounter takes place.") 1869 protected Reference location; 1870 1871 /** 1872 * The actual object that is the target of the reference (The location where the 1873 * encounter takes place.) 1874 */ 1875 protected Location locationTarget; 1876 1877 /** 1878 * The status of the participants' presence at the specified location during the 1879 * period specified. If the participant is is no longer at the location, then 1880 * the period will have an end date/time. 1881 */ 1882 @Child(name = "status", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1883 @Description(shortDefinition = "planned | active | reserved | completed", formalDefinition = "The status of the participants' presence at the specified location during the period specified. If the participant is is no longer at the location, then the period will have an end date/time.") 1884 protected Enumeration<EncounterLocationStatus> status; 1885 1886 /** 1887 * Time period during which the patient was present at the location. 1888 */ 1889 @Child(name = "period", type = { Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1890 @Description(shortDefinition = "Time period during which the patient was present at the location", formalDefinition = "Time period during which the patient was present at the location.") 1891 protected Period period; 1892 1893 private static final long serialVersionUID = -322984880L; 1894 1895 /* 1896 * Constructor 1897 */ 1898 public EncounterLocationComponent() { 1899 super(); 1900 } 1901 1902 /* 1903 * Constructor 1904 */ 1905 public EncounterLocationComponent(Reference location) { 1906 super(); 1907 this.location = location; 1908 } 1909 1910 /** 1911 * @return {@link #location} (The location where the encounter takes place.) 1912 */ 1913 public Reference getLocation() { 1914 if (this.location == null) 1915 if (Configuration.errorOnAutoCreate()) 1916 throw new Error("Attempt to auto-create EncounterLocationComponent.location"); 1917 else if (Configuration.doAutoCreate()) 1918 this.location = new Reference(); // cc 1919 return this.location; 1920 } 1921 1922 public boolean hasLocation() { 1923 return this.location != null && !this.location.isEmpty(); 1924 } 1925 1926 /** 1927 * @param value {@link #location} (The location where the encounter takes 1928 * place.) 1929 */ 1930 public EncounterLocationComponent setLocation(Reference value) { 1931 this.location = value; 1932 return this; 1933 } 1934 1935 /** 1936 * @return {@link #location} The actual object that is the target of the 1937 * reference. The reference library doesn't populate this, but you can 1938 * use it to hold the resource if you resolve it. (The location where 1939 * the encounter takes place.) 1940 */ 1941 public Location getLocationTarget() { 1942 if (this.locationTarget == null) 1943 if (Configuration.errorOnAutoCreate()) 1944 throw new Error("Attempt to auto-create EncounterLocationComponent.location"); 1945 else if (Configuration.doAutoCreate()) 1946 this.locationTarget = new Location(); // aa 1947 return this.locationTarget; 1948 } 1949 1950 /** 1951 * @param value {@link #location} The actual object that is the target of the 1952 * reference. The reference library doesn't use these, but you can 1953 * use it to hold the resource if you resolve it. (The location 1954 * where the encounter takes place.) 1955 */ 1956 public EncounterLocationComponent setLocationTarget(Location value) { 1957 this.locationTarget = value; 1958 return this; 1959 } 1960 1961 /** 1962 * @return {@link #status} (The status of the participants' presence at the 1963 * specified location during the period specified. If the participant is 1964 * is no longer at the location, then the period will have an end 1965 * date/time.). This is the underlying object with id, value and 1966 * extensions. The accessor "getStatus" gives direct access to the value 1967 */ 1968 public Enumeration<EncounterLocationStatus> getStatusElement() { 1969 if (this.status == null) 1970 if (Configuration.errorOnAutoCreate()) 1971 throw new Error("Attempt to auto-create EncounterLocationComponent.status"); 1972 else if (Configuration.doAutoCreate()) 1973 this.status = new Enumeration<EncounterLocationStatus>(new EncounterLocationStatusEnumFactory()); // bb 1974 return this.status; 1975 } 1976 1977 public boolean hasStatusElement() { 1978 return this.status != null && !this.status.isEmpty(); 1979 } 1980 1981 public boolean hasStatus() { 1982 return this.status != null && !this.status.isEmpty(); 1983 } 1984 1985 /** 1986 * @param value {@link #status} (The status of the participants' presence at the 1987 * specified location during the period specified. If the 1988 * participant is is no longer at the location, then the period 1989 * will have an end date/time.). This is the underlying object with 1990 * id, value and extensions. The accessor "getStatus" gives direct 1991 * access to the value 1992 */ 1993 public EncounterLocationComponent setStatusElement(Enumeration<EncounterLocationStatus> value) { 1994 this.status = value; 1995 return this; 1996 } 1997 1998 /** 1999 * @return The status of the participants' presence at the specified location 2000 * during the period specified. If the participant is is no longer at 2001 * the location, then the period will have an end date/time. 2002 */ 2003 public EncounterLocationStatus getStatus() { 2004 return this.status == null ? null : this.status.getValue(); 2005 } 2006 2007 /** 2008 * @param value The status of the participants' presence at the specified 2009 * location during the period specified. If the participant is is 2010 * no longer at the location, then the period will have an end 2011 * date/time. 2012 */ 2013 public EncounterLocationComponent setStatus(EncounterLocationStatus value) { 2014 if (value == null) 2015 this.status = null; 2016 else { 2017 if (this.status == null) 2018 this.status = new Enumeration<EncounterLocationStatus>(new EncounterLocationStatusEnumFactory()); 2019 this.status.setValue(value); 2020 } 2021 return this; 2022 } 2023 2024 /** 2025 * @return {@link #period} (Time period during which the patient was present at 2026 * the location.) 2027 */ 2028 public Period getPeriod() { 2029 if (this.period == null) 2030 if (Configuration.errorOnAutoCreate()) 2031 throw new Error("Attempt to auto-create EncounterLocationComponent.period"); 2032 else if (Configuration.doAutoCreate()) 2033 this.period = new Period(); // cc 2034 return this.period; 2035 } 2036 2037 public boolean hasPeriod() { 2038 return this.period != null && !this.period.isEmpty(); 2039 } 2040 2041 /** 2042 * @param value {@link #period} (Time period during which the patient was 2043 * present at the location.) 2044 */ 2045 public EncounterLocationComponent setPeriod(Period value) { 2046 this.period = value; 2047 return this; 2048 } 2049 2050 protected void listChildren(List<Property> childrenList) { 2051 super.listChildren(childrenList); 2052 childrenList.add(new Property("location", "Reference(Location)", "The location where the encounter takes place.", 2053 0, java.lang.Integer.MAX_VALUE, location)); 2054 childrenList.add(new Property("status", "code", 2055 "The status of the participants' presence at the specified location during the period specified. If the participant is is no longer at the location, then the period will have an end date/time.", 2056 0, java.lang.Integer.MAX_VALUE, status)); 2057 childrenList.add(new Property("period", "Period", 2058 "Time period during which the patient was present at the location.", 0, java.lang.Integer.MAX_VALUE, period)); 2059 } 2060 2061 @Override 2062 public void setProperty(String name, Base value) throws FHIRException { 2063 if (name.equals("location")) 2064 this.location = castToReference(value); // Reference 2065 else if (name.equals("status")) 2066 this.status = new EncounterLocationStatusEnumFactory().fromType(value); // Enumeration<EncounterLocationStatus> 2067 else if (name.equals("period")) 2068 this.period = castToPeriod(value); // Period 2069 else 2070 super.setProperty(name, value); 2071 } 2072 2073 @Override 2074 public Base addChild(String name) throws FHIRException { 2075 if (name.equals("location")) { 2076 this.location = new Reference(); 2077 return this.location; 2078 } else if (name.equals("status")) { 2079 throw new FHIRException("Cannot call addChild on a singleton property Encounter.status"); 2080 } else if (name.equals("period")) { 2081 this.period = new Period(); 2082 return this.period; 2083 } else 2084 return super.addChild(name); 2085 } 2086 2087 public EncounterLocationComponent copy() { 2088 EncounterLocationComponent dst = new EncounterLocationComponent(); 2089 copyValues(dst); 2090 dst.location = location == null ? null : location.copy(); 2091 dst.status = status == null ? null : status.copy(); 2092 dst.period = period == null ? null : period.copy(); 2093 return dst; 2094 } 2095 2096 @Override 2097 public boolean equalsDeep(Base other) { 2098 if (!super.equalsDeep(other)) 2099 return false; 2100 if (!(other instanceof EncounterLocationComponent)) 2101 return false; 2102 EncounterLocationComponent o = (EncounterLocationComponent) other; 2103 return compareDeep(location, o.location, true) && compareDeep(status, o.status, true) 2104 && compareDeep(period, o.period, true); 2105 } 2106 2107 @Override 2108 public boolean equalsShallow(Base other) { 2109 if (!super.equalsShallow(other)) 2110 return false; 2111 if (!(other instanceof EncounterLocationComponent)) 2112 return false; 2113 EncounterLocationComponent o = (EncounterLocationComponent) other; 2114 return compareValues(status, o.status, true); 2115 } 2116 2117 public boolean isEmpty() { 2118 return super.isEmpty() && (location == null || location.isEmpty()) && (status == null || status.isEmpty()) 2119 && (period == null || period.isEmpty()); 2120 } 2121 2122 public String fhirType() { 2123 return "Encounter.location"; 2124 2125 } 2126 2127 } 2128 2129 /** 2130 * Identifier(s) by which this encounter is known. 2131 */ 2132 @Child(name = "identifier", type = { 2133 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2134 @Description(shortDefinition = "Identifier(s) by which this encounter is known", formalDefinition = "Identifier(s) by which this encounter is known.") 2135 protected List<Identifier> identifier; 2136 2137 /** 2138 * planned | arrived | in-progress | onleave | finished | cancelled. 2139 */ 2140 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 2141 @Description(shortDefinition = "planned | arrived | in-progress | onleave | finished | cancelled", formalDefinition = "planned | arrived | in-progress | onleave | finished | cancelled.") 2142 protected Enumeration<EncounterState> status; 2143 2144 /** 2145 * The status history permits the encounter resource to contain the status 2146 * history without needing to read through the historical versions of the 2147 * resource, or even have the server store them. 2148 */ 2149 @Child(name = "statusHistory", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2150 @Description(shortDefinition = "List of past encounter statuses", formalDefinition = "The status history permits the encounter resource to contain the status history without needing to read through the historical versions of the resource, or even have the server store them.") 2151 protected List<EncounterStatusHistoryComponent> statusHistory; 2152 2153 /** 2154 * inpatient | outpatient | ambulatory | emergency +. 2155 */ 2156 @Child(name = "class", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2157 @Description(shortDefinition = "inpatient | outpatient | ambulatory | emergency +", formalDefinition = "inpatient | outpatient | ambulatory | emergency +.") 2158 protected Enumeration<EncounterClass> class_; 2159 2160 /** 2161 * Specific type of encounter (e.g. e-mail consultation, surgical day-care, 2162 * skilled nursing, rehabilitation). 2163 */ 2164 @Child(name = "type", type = { 2165 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2166 @Description(shortDefinition = "Specific type of encounter", formalDefinition = "Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).") 2167 protected List<CodeableConcept> type; 2168 2169 /** 2170 * Indicates the urgency of the encounter. 2171 */ 2172 @Child(name = "priority", type = { 2173 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2174 @Description(shortDefinition = "Indicates the urgency of the encounter", formalDefinition = "Indicates the urgency of the encounter.") 2175 protected CodeableConcept priority; 2176 2177 /** 2178 * The patient present at the encounter. 2179 */ 2180 @Child(name = "patient", type = { Patient.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 2181 @Description(shortDefinition = "The patient present at the encounter", formalDefinition = "The patient present at the encounter.") 2182 protected Reference patient; 2183 2184 /** 2185 * The actual object that is the target of the reference (The patient present at 2186 * the encounter.) 2187 */ 2188 protected Patient patientTarget; 2189 2190 /** 2191 * Where a specific encounter should be classified as a part of a specific 2192 * episode(s) of care this field should be used. This association can facilitate 2193 * grouping of related encounters together for a specific purpose, such as 2194 * government reporting, issue tracking, association via a common problem. The 2195 * association is recorded on the encounter as these are typically created after 2196 * the episode of care, and grouped on entry rather than editing the episode of 2197 * care to append another encounter to it (the episode of care could span 2198 * years). 2199 */ 2200 @Child(name = "episodeOfCare", type = { 2201 EpisodeOfCare.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2202 @Description(shortDefinition = "Episode(s) of care that this encounter should be recorded against", formalDefinition = "Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care, and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years).") 2203 protected List<Reference> episodeOfCare; 2204 /** 2205 * The actual objects that are the target of the reference (Where a specific 2206 * encounter should be classified as a part of a specific episode(s) of care 2207 * this field should be used. This association can facilitate grouping of 2208 * related encounters together for a specific purpose, such as government 2209 * reporting, issue tracking, association via a common problem. The association 2210 * is recorded on the encounter as these are typically created after the episode 2211 * of care, and grouped on entry rather than editing the episode of care to 2212 * append another encounter to it (the episode of care could span years).) 2213 */ 2214 protected List<EpisodeOfCare> episodeOfCareTarget; 2215 2216 /** 2217 * The referral request this encounter satisfies (incoming referral). 2218 */ 2219 @Child(name = "incomingReferral", type = { 2220 ReferralRequest.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2221 @Description(shortDefinition = "The ReferralRequest that initiated this encounter", formalDefinition = "The referral request this encounter satisfies (incoming referral).") 2222 protected List<Reference> incomingReferral; 2223 /** 2224 * The actual objects that are the target of the reference (The referral request 2225 * this encounter satisfies (incoming referral).) 2226 */ 2227 protected List<ReferralRequest> incomingReferralTarget; 2228 2229 /** 2230 * The list of people responsible for providing the service. 2231 */ 2232 @Child(name = "participant", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2233 @Description(shortDefinition = "List of participants involved in the encounter", formalDefinition = "The list of people responsible for providing the service.") 2234 protected List<EncounterParticipantComponent> participant; 2235 2236 /** 2237 * The appointment that scheduled this encounter. 2238 */ 2239 @Child(name = "appointment", type = { 2240 Appointment.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 2241 @Description(shortDefinition = "The appointment that scheduled this encounter", formalDefinition = "The appointment that scheduled this encounter.") 2242 protected Reference appointment; 2243 2244 /** 2245 * The actual object that is the target of the reference (The appointment that 2246 * scheduled this encounter.) 2247 */ 2248 protected Appointment appointmentTarget; 2249 2250 /** 2251 * The start and end time of the encounter. 2252 */ 2253 @Child(name = "period", type = { Period.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 2254 @Description(shortDefinition = "The start and end time of the encounter", formalDefinition = "The start and end time of the encounter.") 2255 protected Period period; 2256 2257 /** 2258 * Quantity of time the encounter lasted. This excludes the time during leaves 2259 * of absence. 2260 */ 2261 @Child(name = "length", type = { Duration.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 2262 @Description(shortDefinition = "Quantity of time the encounter lasted (less time absent)", formalDefinition = "Quantity of time the encounter lasted. This excludes the time during leaves of absence.") 2263 protected Duration length; 2264 2265 /** 2266 * Reason the encounter takes place, expressed as a code. For admissions, this 2267 * can be used for a coded admission diagnosis. 2268 */ 2269 @Child(name = "reason", type = { 2270 CodeableConcept.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2271 @Description(shortDefinition = "Reason the encounter takes place (code)", formalDefinition = "Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.") 2272 protected List<CodeableConcept> reason; 2273 2274 /** 2275 * Reason the encounter takes place, as specified using information from another 2276 * resource. For admissions, this is the admission diagnosis. The indication 2277 * will typically be a Condition (with other resources referenced in the 2278 * evidence.detail), or a Procedure. 2279 */ 2280 @Child(name = "indication", type = { Condition.class, 2281 Procedure.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2282 @Description(shortDefinition = "Reason the encounter takes place (resource)", formalDefinition = "Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.") 2283 protected List<Reference> indication; 2284 /** 2285 * The actual objects that are the target of the reference (Reason the encounter 2286 * takes place, as specified using information from another resource. For 2287 * admissions, this is the admission diagnosis. The indication will typically be 2288 * a Condition (with other resources referenced in the evidence.detail), or a 2289 * Procedure.) 2290 */ 2291 protected List<Resource> indicationTarget; 2292 2293 /** 2294 * Details about the admission to a healthcare service. 2295 */ 2296 @Child(name = "hospitalization", type = {}, order = 15, min = 0, max = 1, modifier = false, summary = false) 2297 @Description(shortDefinition = "Details about the admission to a healthcare service", formalDefinition = "Details about the admission to a healthcare service.") 2298 protected EncounterHospitalizationComponent hospitalization; 2299 2300 /** 2301 * List of locations where the patient has been during this encounter. 2302 */ 2303 @Child(name = "location", type = {}, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2304 @Description(shortDefinition = "List of locations where the patient has been", formalDefinition = "List of locations where the patient has been during this encounter.") 2305 protected List<EncounterLocationComponent> location; 2306 2307 /** 2308 * An organization that is in charge of maintaining the information of this 2309 * Encounter (e.g. who maintains the report or the master service catalog item, 2310 * etc.). This MAY be the same as the organization on the Patient record, 2311 * however it could be different. This MAY not be not the Service Delivery 2312 * Location's Organization. 2313 */ 2314 @Child(name = "serviceProvider", type = { 2315 Organization.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 2316 @Description(shortDefinition = "The custodian organization of this Encounter record", formalDefinition = "An organization that is in charge of maintaining the information of this Encounter (e.g. who maintains the report or the master service catalog item, etc.). This MAY be the same as the organization on the Patient record, however it could be different. This MAY not be not the Service Delivery Location's Organization.") 2317 protected Reference serviceProvider; 2318 2319 /** 2320 * The actual object that is the target of the reference (An organization that 2321 * is in charge of maintaining the information of this Encounter (e.g. who 2322 * maintains the report or the master service catalog item, etc.). This MAY be 2323 * the same as the organization on the Patient record, however it could be 2324 * different. This MAY not be not the Service Delivery Location's Organization.) 2325 */ 2326 protected Organization serviceProviderTarget; 2327 2328 /** 2329 * Another Encounter of which this encounter is a part of (administratively or 2330 * in time). 2331 */ 2332 @Child(name = "partOf", type = { Encounter.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 2333 @Description(shortDefinition = "Another Encounter this encounter is part of", formalDefinition = "Another Encounter of which this encounter is a part of (administratively or in time).") 2334 protected Reference partOf; 2335 2336 /** 2337 * The actual object that is the target of the reference (Another Encounter of 2338 * which this encounter is a part of (administratively or in time).) 2339 */ 2340 protected Encounter partOfTarget; 2341 2342 private static final long serialVersionUID = 929562300L; 2343 2344 /* 2345 * Constructor 2346 */ 2347 public Encounter() { 2348 super(); 2349 } 2350 2351 /* 2352 * Constructor 2353 */ 2354 public Encounter(Enumeration<EncounterState> status) { 2355 super(); 2356 this.status = status; 2357 } 2358 2359 /** 2360 * @return {@link #identifier} (Identifier(s) by which this encounter is known.) 2361 */ 2362 public List<Identifier> getIdentifier() { 2363 if (this.identifier == null) 2364 this.identifier = new ArrayList<Identifier>(); 2365 return this.identifier; 2366 } 2367 2368 public boolean hasIdentifier() { 2369 if (this.identifier == null) 2370 return false; 2371 for (Identifier item : this.identifier) 2372 if (!item.isEmpty()) 2373 return true; 2374 return false; 2375 } 2376 2377 /** 2378 * @return {@link #identifier} (Identifier(s) by which this encounter is known.) 2379 */ 2380 // syntactic sugar 2381 public Identifier addIdentifier() { // 3 2382 Identifier t = new Identifier(); 2383 if (this.identifier == null) 2384 this.identifier = new ArrayList<Identifier>(); 2385 this.identifier.add(t); 2386 return t; 2387 } 2388 2389 // syntactic sugar 2390 public Encounter addIdentifier(Identifier t) { // 3 2391 if (t == null) 2392 return this; 2393 if (this.identifier == null) 2394 this.identifier = new ArrayList<Identifier>(); 2395 this.identifier.add(t); 2396 return this; 2397 } 2398 2399 /** 2400 * @return {@link #status} (planned | arrived | in-progress | onleave | finished 2401 * | cancelled.). This is the underlying object with id, value and 2402 * extensions. The accessor "getStatus" gives direct access to the value 2403 */ 2404 public Enumeration<EncounterState> getStatusElement() { 2405 if (this.status == null) 2406 if (Configuration.errorOnAutoCreate()) 2407 throw new Error("Attempt to auto-create Encounter.status"); 2408 else if (Configuration.doAutoCreate()) 2409 this.status = new Enumeration<EncounterState>(new EncounterStateEnumFactory()); // bb 2410 return this.status; 2411 } 2412 2413 public boolean hasStatusElement() { 2414 return this.status != null && !this.status.isEmpty(); 2415 } 2416 2417 public boolean hasStatus() { 2418 return this.status != null && !this.status.isEmpty(); 2419 } 2420 2421 /** 2422 * @param value {@link #status} (planned | arrived | in-progress | onleave | 2423 * finished | cancelled.). This is the underlying object with id, 2424 * value and extensions. The accessor "getStatus" gives direct 2425 * access to the value 2426 */ 2427 public Encounter setStatusElement(Enumeration<EncounterState> value) { 2428 this.status = value; 2429 return this; 2430 } 2431 2432 /** 2433 * @return planned | arrived | in-progress | onleave | finished | cancelled. 2434 */ 2435 public EncounterState getStatus() { 2436 return this.status == null ? null : this.status.getValue(); 2437 } 2438 2439 /** 2440 * @param value planned | arrived | in-progress | onleave | finished | 2441 * cancelled. 2442 */ 2443 public Encounter setStatus(EncounterState value) { 2444 if (this.status == null) 2445 this.status = new Enumeration<EncounterState>(new EncounterStateEnumFactory()); 2446 this.status.setValue(value); 2447 return this; 2448 } 2449 2450 /** 2451 * @return {@link #statusHistory} (The status history permits the encounter 2452 * resource to contain the status history without needing to read 2453 * through the historical versions of the resource, or even have the 2454 * server store them.) 2455 */ 2456 public List<EncounterStatusHistoryComponent> getStatusHistory() { 2457 if (this.statusHistory == null) 2458 this.statusHistory = new ArrayList<EncounterStatusHistoryComponent>(); 2459 return this.statusHistory; 2460 } 2461 2462 public boolean hasStatusHistory() { 2463 if (this.statusHistory == null) 2464 return false; 2465 for (EncounterStatusHistoryComponent item : this.statusHistory) 2466 if (!item.isEmpty()) 2467 return true; 2468 return false; 2469 } 2470 2471 /** 2472 * @return {@link #statusHistory} (The status history permits the encounter 2473 * resource to contain the status history without needing to read 2474 * through the historical versions of the resource, or even have the 2475 * server store them.) 2476 */ 2477 // syntactic sugar 2478 public EncounterStatusHistoryComponent addStatusHistory() { // 3 2479 EncounterStatusHistoryComponent t = new EncounterStatusHistoryComponent(); 2480 if (this.statusHistory == null) 2481 this.statusHistory = new ArrayList<EncounterStatusHistoryComponent>(); 2482 this.statusHistory.add(t); 2483 return t; 2484 } 2485 2486 // syntactic sugar 2487 public Encounter addStatusHistory(EncounterStatusHistoryComponent t) { // 3 2488 if (t == null) 2489 return this; 2490 if (this.statusHistory == null) 2491 this.statusHistory = new ArrayList<EncounterStatusHistoryComponent>(); 2492 this.statusHistory.add(t); 2493 return this; 2494 } 2495 2496 /** 2497 * @return {@link #class_} (inpatient | outpatient | ambulatory | emergency +.). 2498 * This is the underlying object with id, value and extensions. The 2499 * accessor "getClass_" gives direct access to the value 2500 */ 2501 public Enumeration<EncounterClass> getClass_Element() { 2502 if (this.class_ == null) 2503 if (Configuration.errorOnAutoCreate()) 2504 throw new Error("Attempt to auto-create Encounter.class_"); 2505 else if (Configuration.doAutoCreate()) 2506 this.class_ = new Enumeration<EncounterClass>(new EncounterClassEnumFactory()); // bb 2507 return this.class_; 2508 } 2509 2510 public boolean hasClass_Element() { 2511 return this.class_ != null && !this.class_.isEmpty(); 2512 } 2513 2514 public boolean hasClass_() { 2515 return this.class_ != null && !this.class_.isEmpty(); 2516 } 2517 2518 /** 2519 * @param value {@link #class_} (inpatient | outpatient | ambulatory | emergency 2520 * +.). This is the underlying object with id, value and 2521 * extensions. The accessor "getClass_" gives direct access to the 2522 * value 2523 */ 2524 public Encounter setClass_Element(Enumeration<EncounterClass> value) { 2525 this.class_ = value; 2526 return this; 2527 } 2528 2529 /** 2530 * @return inpatient | outpatient | ambulatory | emergency +. 2531 */ 2532 public EncounterClass getClass_() { 2533 return this.class_ == null ? null : this.class_.getValue(); 2534 } 2535 2536 /** 2537 * @param value inpatient | outpatient | ambulatory | emergency +. 2538 */ 2539 public Encounter setClass_(EncounterClass value) { 2540 if (value == null) 2541 this.class_ = null; 2542 else { 2543 if (this.class_ == null) 2544 this.class_ = new Enumeration<EncounterClass>(new EncounterClassEnumFactory()); 2545 this.class_.setValue(value); 2546 } 2547 return this; 2548 } 2549 2550 /** 2551 * @return {@link #type} (Specific type of encounter (e.g. e-mail consultation, 2552 * surgical day-care, skilled nursing, rehabilitation).) 2553 */ 2554 public List<CodeableConcept> getType() { 2555 if (this.type == null) 2556 this.type = new ArrayList<CodeableConcept>(); 2557 return this.type; 2558 } 2559 2560 public boolean hasType() { 2561 if (this.type == null) 2562 return false; 2563 for (CodeableConcept item : this.type) 2564 if (!item.isEmpty()) 2565 return true; 2566 return false; 2567 } 2568 2569 /** 2570 * @return {@link #type} (Specific type of encounter (e.g. e-mail consultation, 2571 * surgical day-care, skilled nursing, rehabilitation).) 2572 */ 2573 // syntactic sugar 2574 public CodeableConcept addType() { // 3 2575 CodeableConcept t = new CodeableConcept(); 2576 if (this.type == null) 2577 this.type = new ArrayList<CodeableConcept>(); 2578 this.type.add(t); 2579 return t; 2580 } 2581 2582 // syntactic sugar 2583 public Encounter addType(CodeableConcept t) { // 3 2584 if (t == null) 2585 return this; 2586 if (this.type == null) 2587 this.type = new ArrayList<CodeableConcept>(); 2588 this.type.add(t); 2589 return this; 2590 } 2591 2592 /** 2593 * @return {@link #priority} (Indicates the urgency of the encounter.) 2594 */ 2595 public CodeableConcept getPriority() { 2596 if (this.priority == null) 2597 if (Configuration.errorOnAutoCreate()) 2598 throw new Error("Attempt to auto-create Encounter.priority"); 2599 else if (Configuration.doAutoCreate()) 2600 this.priority = new CodeableConcept(); // cc 2601 return this.priority; 2602 } 2603 2604 public boolean hasPriority() { 2605 return this.priority != null && !this.priority.isEmpty(); 2606 } 2607 2608 /** 2609 * @param value {@link #priority} (Indicates the urgency of the encounter.) 2610 */ 2611 public Encounter setPriority(CodeableConcept value) { 2612 this.priority = value; 2613 return this; 2614 } 2615 2616 /** 2617 * @return {@link #patient} (The patient present at the encounter.) 2618 */ 2619 public Reference getPatient() { 2620 if (this.patient == null) 2621 if (Configuration.errorOnAutoCreate()) 2622 throw new Error("Attempt to auto-create Encounter.patient"); 2623 else if (Configuration.doAutoCreate()) 2624 this.patient = new Reference(); // cc 2625 return this.patient; 2626 } 2627 2628 public boolean hasPatient() { 2629 return this.patient != null && !this.patient.isEmpty(); 2630 } 2631 2632 /** 2633 * @param value {@link #patient} (The patient present at the encounter.) 2634 */ 2635 public Encounter setPatient(Reference value) { 2636 this.patient = value; 2637 return this; 2638 } 2639 2640 /** 2641 * @return {@link #patient} The actual object that is the target of the 2642 * reference. The reference library doesn't populate this, but you can 2643 * use it to hold the resource if you resolve it. (The patient present 2644 * at the encounter.) 2645 */ 2646 public Patient getPatientTarget() { 2647 if (this.patientTarget == null) 2648 if (Configuration.errorOnAutoCreate()) 2649 throw new Error("Attempt to auto-create Encounter.patient"); 2650 else if (Configuration.doAutoCreate()) 2651 this.patientTarget = new Patient(); // aa 2652 return this.patientTarget; 2653 } 2654 2655 /** 2656 * @param value {@link #patient} The actual object that is the target of the 2657 * reference. The reference library doesn't use these, but you can 2658 * use it to hold the resource if you resolve it. (The patient 2659 * present at the encounter.) 2660 */ 2661 public Encounter setPatientTarget(Patient value) { 2662 this.patientTarget = value; 2663 return this; 2664 } 2665 2666 /** 2667 * @return {@link #episodeOfCare} (Where a specific encounter should be 2668 * classified as a part of a specific episode(s) of care this field 2669 * should be used. This association can facilitate grouping of related 2670 * encounters together for a specific purpose, such as government 2671 * reporting, issue tracking, association via a common problem. The 2672 * association is recorded on the encounter as these are typically 2673 * created after the episode of care, and grouped on entry rather than 2674 * editing the episode of care to append another encounter to it (the 2675 * episode of care could span years).) 2676 */ 2677 public List<Reference> getEpisodeOfCare() { 2678 if (this.episodeOfCare == null) 2679 this.episodeOfCare = new ArrayList<Reference>(); 2680 return this.episodeOfCare; 2681 } 2682 2683 public boolean hasEpisodeOfCare() { 2684 if (this.episodeOfCare == null) 2685 return false; 2686 for (Reference item : this.episodeOfCare) 2687 if (!item.isEmpty()) 2688 return true; 2689 return false; 2690 } 2691 2692 /** 2693 * @return {@link #episodeOfCare} (Where a specific encounter should be 2694 * classified as a part of a specific episode(s) of care this field 2695 * should be used. This association can facilitate grouping of related 2696 * encounters together for a specific purpose, such as government 2697 * reporting, issue tracking, association via a common problem. The 2698 * association is recorded on the encounter as these are typically 2699 * created after the episode of care, and grouped on entry rather than 2700 * editing the episode of care to append another encounter to it (the 2701 * episode of care could span years).) 2702 */ 2703 // syntactic sugar 2704 public Reference addEpisodeOfCare() { // 3 2705 Reference t = new Reference(); 2706 if (this.episodeOfCare == null) 2707 this.episodeOfCare = new ArrayList<Reference>(); 2708 this.episodeOfCare.add(t); 2709 return t; 2710 } 2711 2712 // syntactic sugar 2713 public Encounter addEpisodeOfCare(Reference t) { // 3 2714 if (t == null) 2715 return this; 2716 if (this.episodeOfCare == null) 2717 this.episodeOfCare = new ArrayList<Reference>(); 2718 this.episodeOfCare.add(t); 2719 return this; 2720 } 2721 2722 /** 2723 * @return {@link #episodeOfCare} (The actual objects that are the target of the 2724 * reference. The reference library doesn't populate this, but you can 2725 * use this to hold the resources if you resolvethemt. Where a specific 2726 * encounter should be classified as a part of a specific episode(s) of 2727 * care this field should be used. This association can facilitate 2728 * grouping of related encounters together for a specific purpose, such 2729 * as government reporting, issue tracking, association via a common 2730 * problem. The association is recorded on the encounter as these are 2731 * typically created after the episode of care, and grouped on entry 2732 * rather than editing the episode of care to append another encounter 2733 * to it (the episode of care could span years).) 2734 */ 2735 public List<EpisodeOfCare> getEpisodeOfCareTarget() { 2736 if (this.episodeOfCareTarget == null) 2737 this.episodeOfCareTarget = new ArrayList<EpisodeOfCare>(); 2738 return this.episodeOfCareTarget; 2739 } 2740 2741 // syntactic sugar 2742 /** 2743 * @return {@link #episodeOfCare} (Add an actual object that is the target of 2744 * the reference. The reference library doesn't use these, but you can 2745 * use this to hold the resources if you resolvethemt. Where a specific 2746 * encounter should be classified as a part of a specific episode(s) of 2747 * care this field should be used. This association can facilitate 2748 * grouping of related encounters together for a specific purpose, such 2749 * as government reporting, issue tracking, association via a common 2750 * problem. The association is recorded on the encounter as these are 2751 * typically created after the episode of care, and grouped on entry 2752 * rather than editing the episode of care to append another encounter 2753 * to it (the episode of care could span years).) 2754 */ 2755 public EpisodeOfCare addEpisodeOfCareTarget() { 2756 EpisodeOfCare r = new EpisodeOfCare(); 2757 if (this.episodeOfCareTarget == null) 2758 this.episodeOfCareTarget = new ArrayList<EpisodeOfCare>(); 2759 this.episodeOfCareTarget.add(r); 2760 return r; 2761 } 2762 2763 /** 2764 * @return {@link #incomingReferral} (The referral request this encounter 2765 * satisfies (incoming referral).) 2766 */ 2767 public List<Reference> getIncomingReferral() { 2768 if (this.incomingReferral == null) 2769 this.incomingReferral = new ArrayList<Reference>(); 2770 return this.incomingReferral; 2771 } 2772 2773 public boolean hasIncomingReferral() { 2774 if (this.incomingReferral == null) 2775 return false; 2776 for (Reference item : this.incomingReferral) 2777 if (!item.isEmpty()) 2778 return true; 2779 return false; 2780 } 2781 2782 /** 2783 * @return {@link #incomingReferral} (The referral request this encounter 2784 * satisfies (incoming referral).) 2785 */ 2786 // syntactic sugar 2787 public Reference addIncomingReferral() { // 3 2788 Reference t = new Reference(); 2789 if (this.incomingReferral == null) 2790 this.incomingReferral = new ArrayList<Reference>(); 2791 this.incomingReferral.add(t); 2792 return t; 2793 } 2794 2795 // syntactic sugar 2796 public Encounter addIncomingReferral(Reference t) { // 3 2797 if (t == null) 2798 return this; 2799 if (this.incomingReferral == null) 2800 this.incomingReferral = new ArrayList<Reference>(); 2801 this.incomingReferral.add(t); 2802 return this; 2803 } 2804 2805 /** 2806 * @return {@link #incomingReferral} (The actual objects that are the target of 2807 * the reference. The reference library doesn't populate this, but you 2808 * can use this to hold the resources if you resolvethemt. The referral 2809 * request this encounter satisfies (incoming referral).) 2810 */ 2811 public List<ReferralRequest> getIncomingReferralTarget() { 2812 if (this.incomingReferralTarget == null) 2813 this.incomingReferralTarget = new ArrayList<ReferralRequest>(); 2814 return this.incomingReferralTarget; 2815 } 2816 2817 // syntactic sugar 2818 /** 2819 * @return {@link #incomingReferral} (Add an actual object that is the target of 2820 * the reference. The reference library doesn't use these, but you can 2821 * use this to hold the resources if you resolvethemt. The referral 2822 * request this encounter satisfies (incoming referral).) 2823 */ 2824 public ReferralRequest addIncomingReferralTarget() { 2825 ReferralRequest r = new ReferralRequest(); 2826 if (this.incomingReferralTarget == null) 2827 this.incomingReferralTarget = new ArrayList<ReferralRequest>(); 2828 this.incomingReferralTarget.add(r); 2829 return r; 2830 } 2831 2832 /** 2833 * @return {@link #participant} (The list of people responsible for providing 2834 * the service.) 2835 */ 2836 public List<EncounterParticipantComponent> getParticipant() { 2837 if (this.participant == null) 2838 this.participant = new ArrayList<EncounterParticipantComponent>(); 2839 return this.participant; 2840 } 2841 2842 public boolean hasParticipant() { 2843 if (this.participant == null) 2844 return false; 2845 for (EncounterParticipantComponent item : this.participant) 2846 if (!item.isEmpty()) 2847 return true; 2848 return false; 2849 } 2850 2851 /** 2852 * @return {@link #participant} (The list of people responsible for providing 2853 * the service.) 2854 */ 2855 // syntactic sugar 2856 public EncounterParticipantComponent addParticipant() { // 3 2857 EncounterParticipantComponent t = new EncounterParticipantComponent(); 2858 if (this.participant == null) 2859 this.participant = new ArrayList<EncounterParticipantComponent>(); 2860 this.participant.add(t); 2861 return t; 2862 } 2863 2864 // syntactic sugar 2865 public Encounter addParticipant(EncounterParticipantComponent t) { // 3 2866 if (t == null) 2867 return this; 2868 if (this.participant == null) 2869 this.participant = new ArrayList<EncounterParticipantComponent>(); 2870 this.participant.add(t); 2871 return this; 2872 } 2873 2874 /** 2875 * @return {@link #appointment} (The appointment that scheduled this encounter.) 2876 */ 2877 public Reference getAppointment() { 2878 if (this.appointment == null) 2879 if (Configuration.errorOnAutoCreate()) 2880 throw new Error("Attempt to auto-create Encounter.appointment"); 2881 else if (Configuration.doAutoCreate()) 2882 this.appointment = new Reference(); // cc 2883 return this.appointment; 2884 } 2885 2886 public boolean hasAppointment() { 2887 return this.appointment != null && !this.appointment.isEmpty(); 2888 } 2889 2890 /** 2891 * @param value {@link #appointment} (The appointment that scheduled this 2892 * encounter.) 2893 */ 2894 public Encounter setAppointment(Reference value) { 2895 this.appointment = value; 2896 return this; 2897 } 2898 2899 /** 2900 * @return {@link #appointment} The actual object that is the target of the 2901 * reference. The reference library doesn't populate this, but you can 2902 * use it to hold the resource if you resolve it. (The appointment that 2903 * scheduled this encounter.) 2904 */ 2905 public Appointment getAppointmentTarget() { 2906 if (this.appointmentTarget == null) 2907 if (Configuration.errorOnAutoCreate()) 2908 throw new Error("Attempt to auto-create Encounter.appointment"); 2909 else if (Configuration.doAutoCreate()) 2910 this.appointmentTarget = new Appointment(); // aa 2911 return this.appointmentTarget; 2912 } 2913 2914 /** 2915 * @param value {@link #appointment} The actual object that is the target of the 2916 * reference. The reference library doesn't use these, but you can 2917 * use it to hold the resource if you resolve it. (The appointment 2918 * that scheduled this encounter.) 2919 */ 2920 public Encounter setAppointmentTarget(Appointment value) { 2921 this.appointmentTarget = value; 2922 return this; 2923 } 2924 2925 /** 2926 * @return {@link #period} (The start and end time of the encounter.) 2927 */ 2928 public Period getPeriod() { 2929 if (this.period == null) 2930 if (Configuration.errorOnAutoCreate()) 2931 throw new Error("Attempt to auto-create Encounter.period"); 2932 else if (Configuration.doAutoCreate()) 2933 this.period = new Period(); // cc 2934 return this.period; 2935 } 2936 2937 public boolean hasPeriod() { 2938 return this.period != null && !this.period.isEmpty(); 2939 } 2940 2941 /** 2942 * @param value {@link #period} (The start and end time of the encounter.) 2943 */ 2944 public Encounter setPeriod(Period value) { 2945 this.period = value; 2946 return this; 2947 } 2948 2949 /** 2950 * @return {@link #length} (Quantity of time the encounter lasted. This excludes 2951 * the time during leaves of absence.) 2952 */ 2953 public Duration getLength() { 2954 if (this.length == null) 2955 if (Configuration.errorOnAutoCreate()) 2956 throw new Error("Attempt to auto-create Encounter.length"); 2957 else if (Configuration.doAutoCreate()) 2958 this.length = new Duration(); // cc 2959 return this.length; 2960 } 2961 2962 public boolean hasLength() { 2963 return this.length != null && !this.length.isEmpty(); 2964 } 2965 2966 /** 2967 * @param value {@link #length} (Quantity of time the encounter lasted. This 2968 * excludes the time during leaves of absence.) 2969 */ 2970 public Encounter setLength(Duration value) { 2971 this.length = value; 2972 return this; 2973 } 2974 2975 /** 2976 * @return {@link #reason} (Reason the encounter takes place, expressed as a 2977 * code. For admissions, this can be used for a coded admission 2978 * diagnosis.) 2979 */ 2980 public List<CodeableConcept> getReason() { 2981 if (this.reason == null) 2982 this.reason = new ArrayList<CodeableConcept>(); 2983 return this.reason; 2984 } 2985 2986 public boolean hasReason() { 2987 if (this.reason == null) 2988 return false; 2989 for (CodeableConcept item : this.reason) 2990 if (!item.isEmpty()) 2991 return true; 2992 return false; 2993 } 2994 2995 /** 2996 * @return {@link #reason} (Reason the encounter takes place, expressed as a 2997 * code. For admissions, this can be used for a coded admission 2998 * diagnosis.) 2999 */ 3000 // syntactic sugar 3001 public CodeableConcept addReason() { // 3 3002 CodeableConcept t = new CodeableConcept(); 3003 if (this.reason == null) 3004 this.reason = new ArrayList<CodeableConcept>(); 3005 this.reason.add(t); 3006 return t; 3007 } 3008 3009 // syntactic sugar 3010 public Encounter addReason(CodeableConcept t) { // 3 3011 if (t == null) 3012 return this; 3013 if (this.reason == null) 3014 this.reason = new ArrayList<CodeableConcept>(); 3015 this.reason.add(t); 3016 return this; 3017 } 3018 3019 /** 3020 * @return {@link #indication} (Reason the encounter takes place, as specified 3021 * using information from another resource. For admissions, this is the 3022 * admission diagnosis. The indication will typically be a Condition 3023 * (with other resources referenced in the evidence.detail), or a 3024 * Procedure.) 3025 */ 3026 public List<Reference> getIndication() { 3027 if (this.indication == null) 3028 this.indication = new ArrayList<Reference>(); 3029 return this.indication; 3030 } 3031 3032 public boolean hasIndication() { 3033 if (this.indication == null) 3034 return false; 3035 for (Reference item : this.indication) 3036 if (!item.isEmpty()) 3037 return true; 3038 return false; 3039 } 3040 3041 /** 3042 * @return {@link #indication} (Reason the encounter takes place, as specified 3043 * using information from another resource. For admissions, this is the 3044 * admission diagnosis. The indication will typically be a Condition 3045 * (with other resources referenced in the evidence.detail), or a 3046 * Procedure.) 3047 */ 3048 // syntactic sugar 3049 public Reference addIndication() { // 3 3050 Reference t = new Reference(); 3051 if (this.indication == null) 3052 this.indication = new ArrayList<Reference>(); 3053 this.indication.add(t); 3054 return t; 3055 } 3056 3057 // syntactic sugar 3058 public Encounter addIndication(Reference t) { // 3 3059 if (t == null) 3060 return this; 3061 if (this.indication == null) 3062 this.indication = new ArrayList<Reference>(); 3063 this.indication.add(t); 3064 return this; 3065 } 3066 3067 /** 3068 * @return {@link #indication} (The actual objects that are the target of the 3069 * reference. The reference library doesn't populate this, but you can 3070 * use this to hold the resources if you resolvethemt. Reason the 3071 * encounter takes place, as specified using information from another 3072 * resource. For admissions, this is the admission diagnosis. The 3073 * indication will typically be a Condition (with other resources 3074 * referenced in the evidence.detail), or a Procedure.) 3075 */ 3076 public List<Resource> getIndicationTarget() { 3077 if (this.indicationTarget == null) 3078 this.indicationTarget = new ArrayList<Resource>(); 3079 return this.indicationTarget; 3080 } 3081 3082 /** 3083 * @return {@link #hospitalization} (Details about the admission to a healthcare 3084 * service.) 3085 */ 3086 public EncounterHospitalizationComponent getHospitalization() { 3087 if (this.hospitalization == null) 3088 if (Configuration.errorOnAutoCreate()) 3089 throw new Error("Attempt to auto-create Encounter.hospitalization"); 3090 else if (Configuration.doAutoCreate()) 3091 this.hospitalization = new EncounterHospitalizationComponent(); // cc 3092 return this.hospitalization; 3093 } 3094 3095 public boolean hasHospitalization() { 3096 return this.hospitalization != null && !this.hospitalization.isEmpty(); 3097 } 3098 3099 /** 3100 * @param value {@link #hospitalization} (Details about the admission to a 3101 * healthcare service.) 3102 */ 3103 public Encounter setHospitalization(EncounterHospitalizationComponent value) { 3104 this.hospitalization = value; 3105 return this; 3106 } 3107 3108 /** 3109 * @return {@link #location} (List of locations where the patient has been 3110 * during this encounter.) 3111 */ 3112 public List<EncounterLocationComponent> getLocation() { 3113 if (this.location == null) 3114 this.location = new ArrayList<EncounterLocationComponent>(); 3115 return this.location; 3116 } 3117 3118 public boolean hasLocation() { 3119 if (this.location == null) 3120 return false; 3121 for (EncounterLocationComponent item : this.location) 3122 if (!item.isEmpty()) 3123 return true; 3124 return false; 3125 } 3126 3127 /** 3128 * @return {@link #location} (List of locations where the patient has been 3129 * during this encounter.) 3130 */ 3131 // syntactic sugar 3132 public EncounterLocationComponent addLocation() { // 3 3133 EncounterLocationComponent t = new EncounterLocationComponent(); 3134 if (this.location == null) 3135 this.location = new ArrayList<EncounterLocationComponent>(); 3136 this.location.add(t); 3137 return t; 3138 } 3139 3140 // syntactic sugar 3141 public Encounter addLocation(EncounterLocationComponent t) { // 3 3142 if (t == null) 3143 return this; 3144 if (this.location == null) 3145 this.location = new ArrayList<EncounterLocationComponent>(); 3146 this.location.add(t); 3147 return this; 3148 } 3149 3150 /** 3151 * @return {@link #serviceProvider} (An organization that is in charge of 3152 * maintaining the information of this Encounter (e.g. who maintains the 3153 * report or the master service catalog item, etc.). This MAY be the 3154 * same as the organization on the Patient record, however it could be 3155 * different. This MAY not be not the Service Delivery Location's 3156 * Organization.) 3157 */ 3158 public Reference getServiceProvider() { 3159 if (this.serviceProvider == null) 3160 if (Configuration.errorOnAutoCreate()) 3161 throw new Error("Attempt to auto-create Encounter.serviceProvider"); 3162 else if (Configuration.doAutoCreate()) 3163 this.serviceProvider = new Reference(); // cc 3164 return this.serviceProvider; 3165 } 3166 3167 public boolean hasServiceProvider() { 3168 return this.serviceProvider != null && !this.serviceProvider.isEmpty(); 3169 } 3170 3171 /** 3172 * @param value {@link #serviceProvider} (An organization that is in charge of 3173 * maintaining the information of this Encounter (e.g. who 3174 * maintains the report or the master service catalog item, etc.). 3175 * This MAY be the same as the organization on the Patient record, 3176 * however it could be different. This MAY not be not the Service 3177 * Delivery Location's Organization.) 3178 */ 3179 public Encounter setServiceProvider(Reference value) { 3180 this.serviceProvider = value; 3181 return this; 3182 } 3183 3184 /** 3185 * @return {@link #serviceProvider} The actual object that is the target of the 3186 * reference. The reference library doesn't populate this, but you can 3187 * use it to hold the resource if you resolve it. (An organization that 3188 * is in charge of maintaining the information of this Encounter (e.g. 3189 * who maintains the report or the master service catalog item, etc.). 3190 * This MAY be the same as the organization on the Patient record, 3191 * however it could be different. This MAY not be not the Service 3192 * Delivery Location's Organization.) 3193 */ 3194 public Organization getServiceProviderTarget() { 3195 if (this.serviceProviderTarget == null) 3196 if (Configuration.errorOnAutoCreate()) 3197 throw new Error("Attempt to auto-create Encounter.serviceProvider"); 3198 else if (Configuration.doAutoCreate()) 3199 this.serviceProviderTarget = new Organization(); // aa 3200 return this.serviceProviderTarget; 3201 } 3202 3203 /** 3204 * @param value {@link #serviceProvider} The actual object that is the target of 3205 * the reference. The reference library doesn't use these, but you 3206 * can use it to hold the resource if you resolve it. (An 3207 * organization that is in charge of maintaining the information of 3208 * this Encounter (e.g. who maintains the report or the master 3209 * service catalog item, etc.). This MAY be the same as the 3210 * organization on the Patient record, however it could be 3211 * different. This MAY not be not the Service Delivery Location's 3212 * Organization.) 3213 */ 3214 public Encounter setServiceProviderTarget(Organization value) { 3215 this.serviceProviderTarget = value; 3216 return this; 3217 } 3218 3219 /** 3220 * @return {@link #partOf} (Another Encounter of which this encounter is a part 3221 * of (administratively or in time).) 3222 */ 3223 public Reference getPartOf() { 3224 if (this.partOf == null) 3225 if (Configuration.errorOnAutoCreate()) 3226 throw new Error("Attempt to auto-create Encounter.partOf"); 3227 else if (Configuration.doAutoCreate()) 3228 this.partOf = new Reference(); // cc 3229 return this.partOf; 3230 } 3231 3232 public boolean hasPartOf() { 3233 return this.partOf != null && !this.partOf.isEmpty(); 3234 } 3235 3236 /** 3237 * @param value {@link #partOf} (Another Encounter of which this encounter is a 3238 * part of (administratively or in time).) 3239 */ 3240 public Encounter setPartOf(Reference value) { 3241 this.partOf = value; 3242 return this; 3243 } 3244 3245 /** 3246 * @return {@link #partOf} The actual object that is the target of the 3247 * reference. The reference library doesn't populate this, but you can 3248 * use it to hold the resource if you resolve it. (Another Encounter of 3249 * which this encounter is a part of (administratively or in time).) 3250 */ 3251 public Encounter getPartOfTarget() { 3252 if (this.partOfTarget == null) 3253 if (Configuration.errorOnAutoCreate()) 3254 throw new Error("Attempt to auto-create Encounter.partOf"); 3255 else if (Configuration.doAutoCreate()) 3256 this.partOfTarget = new Encounter(); // aa 3257 return this.partOfTarget; 3258 } 3259 3260 /** 3261 * @param value {@link #partOf} The actual object that is the target of the 3262 * reference. The reference library doesn't use these, but you can 3263 * use it to hold the resource if you resolve it. (Another 3264 * Encounter of which this encounter is a part of (administratively 3265 * or in time).) 3266 */ 3267 public Encounter setPartOfTarget(Encounter value) { 3268 this.partOfTarget = value; 3269 return this; 3270 } 3271 3272 protected void listChildren(List<Property> childrenList) { 3273 super.listChildren(childrenList); 3274 childrenList.add(new Property("identifier", "Identifier", "Identifier(s) by which this encounter is known.", 0, 3275 java.lang.Integer.MAX_VALUE, identifier)); 3276 childrenList.add(new Property("status", "code", "planned | arrived | in-progress | onleave | finished | cancelled.", 3277 0, java.lang.Integer.MAX_VALUE, status)); 3278 childrenList.add(new Property("statusHistory", "", 3279 "The status history permits the encounter resource to contain the status history without needing to read through the historical versions of the resource, or even have the server store them.", 3280 0, java.lang.Integer.MAX_VALUE, statusHistory)); 3281 childrenList.add(new Property("class", "code", "inpatient | outpatient | ambulatory | emergency +.", 0, 3282 java.lang.Integer.MAX_VALUE, class_)); 3283 childrenList.add(new Property("type", "CodeableConcept", 3284 "Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).", 0, 3285 java.lang.Integer.MAX_VALUE, type)); 3286 childrenList.add(new Property("priority", "CodeableConcept", "Indicates the urgency of the encounter.", 0, 3287 java.lang.Integer.MAX_VALUE, priority)); 3288 childrenList.add(new Property("patient", "Reference(Patient)", "The patient present at the encounter.", 0, 3289 java.lang.Integer.MAX_VALUE, patient)); 3290 childrenList.add(new Property("episodeOfCare", "Reference(EpisodeOfCare)", 3291 "Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care, and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years).", 3292 0, java.lang.Integer.MAX_VALUE, episodeOfCare)); 3293 childrenList.add(new Property("incomingReferral", "Reference(ReferralRequest)", 3294 "The referral request this encounter satisfies (incoming referral).", 0, java.lang.Integer.MAX_VALUE, 3295 incomingReferral)); 3296 childrenList.add(new Property("participant", "", "The list of people responsible for providing the service.", 0, 3297 java.lang.Integer.MAX_VALUE, participant)); 3298 childrenList.add(new Property("appointment", "Reference(Appointment)", 3299 "The appointment that scheduled this encounter.", 0, java.lang.Integer.MAX_VALUE, appointment)); 3300 childrenList.add(new Property("period", "Period", "The start and end time of the encounter.", 0, 3301 java.lang.Integer.MAX_VALUE, period)); 3302 childrenList.add(new Property("length", "Duration", 3303 "Quantity of time the encounter lasted. This excludes the time during leaves of absence.", 0, 3304 java.lang.Integer.MAX_VALUE, length)); 3305 childrenList.add(new Property("reason", "CodeableConcept", 3306 "Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.", 3307 0, java.lang.Integer.MAX_VALUE, reason)); 3308 childrenList.add(new Property("indication", "Reference(Condition|Procedure)", 3309 "Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.", 3310 0, java.lang.Integer.MAX_VALUE, indication)); 3311 childrenList.add(new Property("hospitalization", "", "Details about the admission to a healthcare service.", 0, 3312 java.lang.Integer.MAX_VALUE, hospitalization)); 3313 childrenList 3314 .add(new Property("location", "", "List of locations where the patient has been during this encounter.", 0, 3315 java.lang.Integer.MAX_VALUE, location)); 3316 childrenList.add(new Property("serviceProvider", "Reference(Organization)", 3317 "An organization that is in charge of maintaining the information of this Encounter (e.g. who maintains the report or the master service catalog item, etc.). This MAY be the same as the organization on the Patient record, however it could be different. This MAY not be not the Service Delivery Location's Organization.", 3318 0, java.lang.Integer.MAX_VALUE, serviceProvider)); 3319 childrenList.add(new Property("partOf", "Reference(Encounter)", 3320 "Another Encounter of which this encounter is a part of (administratively or in time).", 0, 3321 java.lang.Integer.MAX_VALUE, partOf)); 3322 } 3323 3324 @Override 3325 public void setProperty(String name, Base value) throws FHIRException { 3326 if (name.equals("identifier")) 3327 this.getIdentifier().add(castToIdentifier(value)); 3328 else if (name.equals("status")) 3329 this.status = new EncounterStateEnumFactory().fromType(value); // Enumeration<EncounterState> 3330 else if (name.equals("statusHistory")) 3331 this.getStatusHistory().add((EncounterStatusHistoryComponent) value); 3332 else if (name.equals("class")) 3333 this.class_ = new EncounterClassEnumFactory().fromType(value); // Enumeration<EncounterClass> 3334 else if (name.equals("type")) 3335 this.getType().add(castToCodeableConcept(value)); 3336 else if (name.equals("priority")) 3337 this.priority = castToCodeableConcept(value); // CodeableConcept 3338 else if (name.equals("patient")) 3339 this.patient = castToReference(value); // Reference 3340 else if (name.equals("episodeOfCare")) 3341 this.getEpisodeOfCare().add(castToReference(value)); 3342 else if (name.equals("incomingReferral")) 3343 this.getIncomingReferral().add(castToReference(value)); 3344 else if (name.equals("participant")) 3345 this.getParticipant().add((EncounterParticipantComponent) value); 3346 else if (name.equals("appointment")) 3347 this.appointment = castToReference(value); // Reference 3348 else if (name.equals("period")) 3349 this.period = castToPeriod(value); // Period 3350 else if (name.equals("length")) 3351 this.length = castToDuration(value); // Duration 3352 else if (name.equals("reason")) 3353 this.getReason().add(castToCodeableConcept(value)); 3354 else if (name.equals("indication")) 3355 this.getIndication().add(castToReference(value)); 3356 else if (name.equals("hospitalization")) 3357 this.hospitalization = (EncounterHospitalizationComponent) value; // EncounterHospitalizationComponent 3358 else if (name.equals("location")) 3359 this.getLocation().add((EncounterLocationComponent) value); 3360 else if (name.equals("serviceProvider")) 3361 this.serviceProvider = castToReference(value); // Reference 3362 else if (name.equals("partOf")) 3363 this.partOf = castToReference(value); // Reference 3364 else 3365 super.setProperty(name, value); 3366 } 3367 3368 @Override 3369 public Base addChild(String name) throws FHIRException { 3370 if (name.equals("identifier")) { 3371 return addIdentifier(); 3372 } else if (name.equals("status")) { 3373 throw new FHIRException("Cannot call addChild on a singleton property Encounter.status"); 3374 } else if (name.equals("statusHistory")) { 3375 return addStatusHistory(); 3376 } else if (name.equals("class")) { 3377 throw new FHIRException("Cannot call addChild on a singleton property Encounter.class"); 3378 } else if (name.equals("type")) { 3379 return addType(); 3380 } else if (name.equals("priority")) { 3381 this.priority = new CodeableConcept(); 3382 return this.priority; 3383 } else if (name.equals("patient")) { 3384 this.patient = new Reference(); 3385 return this.patient; 3386 } else if (name.equals("episodeOfCare")) { 3387 return addEpisodeOfCare(); 3388 } else if (name.equals("incomingReferral")) { 3389 return addIncomingReferral(); 3390 } else if (name.equals("participant")) { 3391 return addParticipant(); 3392 } else if (name.equals("appointment")) { 3393 this.appointment = new Reference(); 3394 return this.appointment; 3395 } else if (name.equals("period")) { 3396 this.period = new Period(); 3397 return this.period; 3398 } else if (name.equals("length")) { 3399 this.length = new Duration(); 3400 return this.length; 3401 } else if (name.equals("reason")) { 3402 return addReason(); 3403 } else if (name.equals("indication")) { 3404 return addIndication(); 3405 } else if (name.equals("hospitalization")) { 3406 this.hospitalization = new EncounterHospitalizationComponent(); 3407 return this.hospitalization; 3408 } else if (name.equals("location")) { 3409 return addLocation(); 3410 } else if (name.equals("serviceProvider")) { 3411 this.serviceProvider = new Reference(); 3412 return this.serviceProvider; 3413 } else if (name.equals("partOf")) { 3414 this.partOf = new Reference(); 3415 return this.partOf; 3416 } else 3417 return super.addChild(name); 3418 } 3419 3420 public String fhirType() { 3421 return "Encounter"; 3422 3423 } 3424 3425 public Encounter copy() { 3426 Encounter dst = new Encounter(); 3427 copyValues(dst); 3428 if (identifier != null) { 3429 dst.identifier = new ArrayList<Identifier>(); 3430 for (Identifier i : identifier) 3431 dst.identifier.add(i.copy()); 3432 } 3433 ; 3434 dst.status = status == null ? null : status.copy(); 3435 if (statusHistory != null) { 3436 dst.statusHistory = new ArrayList<EncounterStatusHistoryComponent>(); 3437 for (EncounterStatusHistoryComponent i : statusHistory) 3438 dst.statusHistory.add(i.copy()); 3439 } 3440 ; 3441 dst.class_ = class_ == null ? null : class_.copy(); 3442 if (type != null) { 3443 dst.type = new ArrayList<CodeableConcept>(); 3444 for (CodeableConcept i : type) 3445 dst.type.add(i.copy()); 3446 } 3447 ; 3448 dst.priority = priority == null ? null : priority.copy(); 3449 dst.patient = patient == null ? null : patient.copy(); 3450 if (episodeOfCare != null) { 3451 dst.episodeOfCare = new ArrayList<Reference>(); 3452 for (Reference i : episodeOfCare) 3453 dst.episodeOfCare.add(i.copy()); 3454 } 3455 ; 3456 if (incomingReferral != null) { 3457 dst.incomingReferral = new ArrayList<Reference>(); 3458 for (Reference i : incomingReferral) 3459 dst.incomingReferral.add(i.copy()); 3460 } 3461 ; 3462 if (participant != null) { 3463 dst.participant = new ArrayList<EncounterParticipantComponent>(); 3464 for (EncounterParticipantComponent i : participant) 3465 dst.participant.add(i.copy()); 3466 } 3467 ; 3468 dst.appointment = appointment == null ? null : appointment.copy(); 3469 dst.period = period == null ? null : period.copy(); 3470 dst.length = length == null ? null : length.copy(); 3471 if (reason != null) { 3472 dst.reason = new ArrayList<CodeableConcept>(); 3473 for (CodeableConcept i : reason) 3474 dst.reason.add(i.copy()); 3475 } 3476 ; 3477 if (indication != null) { 3478 dst.indication = new ArrayList<Reference>(); 3479 for (Reference i : indication) 3480 dst.indication.add(i.copy()); 3481 } 3482 ; 3483 dst.hospitalization = hospitalization == null ? null : hospitalization.copy(); 3484 if (location != null) { 3485 dst.location = new ArrayList<EncounterLocationComponent>(); 3486 for (EncounterLocationComponent i : location) 3487 dst.location.add(i.copy()); 3488 } 3489 ; 3490 dst.serviceProvider = serviceProvider == null ? null : serviceProvider.copy(); 3491 dst.partOf = partOf == null ? null : partOf.copy(); 3492 return dst; 3493 } 3494 3495 protected Encounter typedCopy() { 3496 return copy(); 3497 } 3498 3499 @Override 3500 public boolean equalsDeep(Base other) { 3501 if (!super.equalsDeep(other)) 3502 return false; 3503 if (!(other instanceof Encounter)) 3504 return false; 3505 Encounter o = (Encounter) other; 3506 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 3507 && compareDeep(statusHistory, o.statusHistory, true) && compareDeep(class_, o.class_, true) 3508 && compareDeep(type, o.type, true) && compareDeep(priority, o.priority, true) 3509 && compareDeep(patient, o.patient, true) && compareDeep(episodeOfCare, o.episodeOfCare, true) 3510 && compareDeep(incomingReferral, o.incomingReferral, true) && compareDeep(participant, o.participant, true) 3511 && compareDeep(appointment, o.appointment, true) && compareDeep(period, o.period, true) 3512 && compareDeep(length, o.length, true) && compareDeep(reason, o.reason, true) 3513 && compareDeep(indication, o.indication, true) && compareDeep(hospitalization, o.hospitalization, true) 3514 && compareDeep(location, o.location, true) && compareDeep(serviceProvider, o.serviceProvider, true) 3515 && compareDeep(partOf, o.partOf, true); 3516 } 3517 3518 @Override 3519 public boolean equalsShallow(Base other) { 3520 if (!super.equalsShallow(other)) 3521 return false; 3522 if (!(other instanceof Encounter)) 3523 return false; 3524 Encounter o = (Encounter) other; 3525 return compareValues(status, o.status, true) && compareValues(class_, o.class_, true); 3526 } 3527 3528 public boolean isEmpty() { 3529 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (status == null || status.isEmpty()) 3530 && (statusHistory == null || statusHistory.isEmpty()) && (class_ == null || class_.isEmpty()) 3531 && (type == null || type.isEmpty()) && (priority == null || priority.isEmpty()) 3532 && (patient == null || patient.isEmpty()) && (episodeOfCare == null || episodeOfCare.isEmpty()) 3533 && (incomingReferral == null || incomingReferral.isEmpty()) && (participant == null || participant.isEmpty()) 3534 && (appointment == null || appointment.isEmpty()) && (period == null || period.isEmpty()) 3535 && (length == null || length.isEmpty()) && (reason == null || reason.isEmpty()) 3536 && (indication == null || indication.isEmpty()) && (hospitalization == null || hospitalization.isEmpty()) 3537 && (location == null || location.isEmpty()) && (serviceProvider == null || serviceProvider.isEmpty()) 3538 && (partOf == null || partOf.isEmpty()); 3539 } 3540 3541 @Override 3542 public ResourceType getResourceType() { 3543 return ResourceType.Encounter; 3544 } 3545 3546 @SearchParamDefinition(name = "date", path = "Encounter.period", description = "A date within the period the Encounter lasted", type = "date") 3547 public static final String SP_DATE = "date"; 3548 @SearchParamDefinition(name = "identifier", path = "Encounter.identifier", description = "Identifier(s) by which this encounter is known", type = "token") 3549 public static final String SP_IDENTIFIER = "identifier"; 3550 @SearchParamDefinition(name = "reason", path = "Encounter.reason", description = "Reason the encounter takes place (code)", type = "token") 3551 public static final String SP_REASON = "reason"; 3552 @SearchParamDefinition(name = "episodeofcare", path = "Encounter.episodeOfCare", description = "Episode(s) of care that this encounter should be recorded against", type = "reference") 3553 public static final String SP_EPISODEOFCARE = "episodeofcare"; 3554 @SearchParamDefinition(name = "participant-type", path = "Encounter.participant.type", description = "Role of participant in encounter", type = "token") 3555 public static final String SP_PARTICIPANTTYPE = "participant-type"; 3556 @SearchParamDefinition(name = "incomingreferral", path = "Encounter.incomingReferral", description = "The ReferralRequest that initiated this encounter", type = "reference") 3557 public static final String SP_INCOMINGREFERRAL = "incomingreferral"; 3558 @SearchParamDefinition(name = "practitioner", path = "Encounter.participant.individual", description = "Persons involved in the encounter other than the patient", type = "reference") 3559 public static final String SP_PRACTITIONER = "practitioner"; 3560 @SearchParamDefinition(name = "length", path = "Encounter.length", description = "Length of encounter in days", type = "number") 3561 public static final String SP_LENGTH = "length"; 3562 @SearchParamDefinition(name = "appointment", path = "Encounter.appointment", description = "The appointment that scheduled this encounter", type = "reference") 3563 public static final String SP_APPOINTMENT = "appointment"; 3564 @SearchParamDefinition(name = "part-of", path = "Encounter.partOf", description = "Another Encounter this encounter is part of", type = "reference") 3565 public static final String SP_PARTOF = "part-of"; 3566 @SearchParamDefinition(name = "procedure", path = "Encounter.indication", description = "Reason the encounter takes place (resource)", type = "reference") 3567 public static final String SP_PROCEDURE = "procedure"; 3568 @SearchParamDefinition(name = "type", path = "Encounter.type", description = "Specific type of encounter", type = "token") 3569 public static final String SP_TYPE = "type"; 3570 @SearchParamDefinition(name = "participant", path = "Encounter.participant.individual", description = "Persons involved in the encounter other than the patient", type = "reference") 3571 public static final String SP_PARTICIPANT = "participant"; 3572 @SearchParamDefinition(name = "condition", path = "Encounter.indication", description = "Reason the encounter takes place (resource)", type = "reference") 3573 public static final String SP_CONDITION = "condition"; 3574 @SearchParamDefinition(name = "patient", path = "Encounter.patient", description = "The patient present at the encounter", type = "reference") 3575 public static final String SP_PATIENT = "patient"; 3576 @SearchParamDefinition(name = "location-period", path = "Encounter.location.period", description = "Time period during which the patient was present at the location", type = "date") 3577 public static final String SP_LOCATIONPERIOD = "location-period"; 3578 @SearchParamDefinition(name = "location", path = "Encounter.location.location", description = "Location the encounter takes place", type = "reference") 3579 public static final String SP_LOCATION = "location"; 3580 @SearchParamDefinition(name = "indication", path = "Encounter.indication", description = "Reason the encounter takes place (resource)", type = "reference") 3581 public static final String SP_INDICATION = "indication"; 3582 @SearchParamDefinition(name = "special-arrangement", path = "Encounter.hospitalization.specialArrangement", description = "Wheelchair, translator, stretcher, etc.", type = "token") 3583 public static final String SP_SPECIALARRANGEMENT = "special-arrangement"; 3584 @SearchParamDefinition(name = "status", path = "Encounter.status", description = "planned | arrived | in-progress | onleave | finished | cancelled", type = "token") 3585 public static final String SP_STATUS = "status"; 3586 3587}