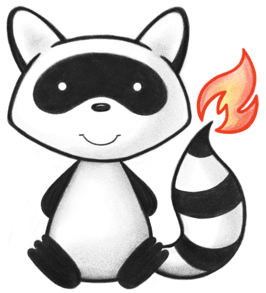
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.exceptions.FHIRException; 042 043/** 044 * This resource provides the insurance enrollment details to the insurer 045 * regarding a specified coverage. 046 */ 047@ResourceDef(name = "EnrollmentRequest", profile = "http://hl7.org/fhir/Profile/EnrollmentRequest") 048public class EnrollmentRequest extends DomainResource { 049 050 /** 051 * The Response business identifier. 052 */ 053 @Child(name = "identifier", type = { 054 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 055 @Description(shortDefinition = "Business Identifier", formalDefinition = "The Response business identifier.") 056 protected List<Identifier> identifier; 057 058 /** 059 * The version of the style of resource contents. This should be mapped to the 060 * allowable profiles for this and supporting resources. 061 */ 062 @Child(name = "ruleset", type = { Coding.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 063 @Description(shortDefinition = "Resource version", formalDefinition = "The version of the style of resource contents. This should be mapped to the allowable profiles for this and supporting resources.") 064 protected Coding ruleset; 065 066 /** 067 * The style (standard) and version of the original material which was converted 068 * into this resource. 069 */ 070 @Child(name = "originalRuleset", type = { 071 Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 072 @Description(shortDefinition = "Original version", formalDefinition = "The style (standard) and version of the original material which was converted into this resource.") 073 protected Coding originalRuleset; 074 075 /** 076 * The date when this resource was created. 077 */ 078 @Child(name = "created", type = { DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 079 @Description(shortDefinition = "Creation date", formalDefinition = "The date when this resource was created.") 080 protected DateTimeType created; 081 082 /** 083 * The Insurer who is target of the request. 084 */ 085 @Child(name = "target", type = { Organization.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 086 @Description(shortDefinition = "Insurer", formalDefinition = "The Insurer who is target of the request.") 087 protected Reference target; 088 089 /** 090 * The actual object that is the target of the reference (The Insurer who is 091 * target of the request.) 092 */ 093 protected Organization targetTarget; 094 095 /** 096 * The practitioner who is responsible for the services rendered to the patient. 097 */ 098 @Child(name = "provider", type = { 099 Practitioner.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 100 @Description(shortDefinition = "Responsible practitioner", formalDefinition = "The practitioner who is responsible for the services rendered to the patient.") 101 protected Reference provider; 102 103 /** 104 * The actual object that is the target of the reference (The practitioner who 105 * is responsible for the services rendered to the patient.) 106 */ 107 protected Practitioner providerTarget; 108 109 /** 110 * The organization which is responsible for the services rendered to the 111 * patient. 112 */ 113 @Child(name = "organization", type = { 114 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 115 @Description(shortDefinition = "Responsible organization", formalDefinition = "The organization which is responsible for the services rendered to the patient.") 116 protected Reference organization; 117 118 /** 119 * The actual object that is the target of the reference (The organization which 120 * is responsible for the services rendered to the patient.) 121 */ 122 protected Organization organizationTarget; 123 124 /** 125 * Patient Resource. 126 */ 127 @Child(name = "subject", type = { Patient.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 128 @Description(shortDefinition = "The subject of the Products and Services", formalDefinition = "Patient Resource.") 129 protected Reference subject; 130 131 /** 132 * The actual object that is the target of the reference (Patient Resource.) 133 */ 134 protected Patient subjectTarget; 135 136 /** 137 * Reference to the program or plan identification, underwriter or payor. 138 */ 139 @Child(name = "coverage", type = { Coverage.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 140 @Description(shortDefinition = "Insurance information", formalDefinition = "Reference to the program or plan identification, underwriter or payor.") 141 protected Reference coverage; 142 143 /** 144 * The actual object that is the target of the reference (Reference to the 145 * program or plan identification, underwriter or payor.) 146 */ 147 protected Coverage coverageTarget; 148 149 /** 150 * The relationship of the patient to the subscriber. 151 */ 152 @Child(name = "relationship", type = { Coding.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 153 @Description(shortDefinition = "Patient relationship to subscriber", formalDefinition = "The relationship of the patient to the subscriber.") 154 protected Coding relationship; 155 156 private static final long serialVersionUID = -1656505325L; 157 158 /* 159 * Constructor 160 */ 161 public EnrollmentRequest() { 162 super(); 163 } 164 165 /* 166 * Constructor 167 */ 168 public EnrollmentRequest(Reference subject, Reference coverage, Coding relationship) { 169 super(); 170 this.subject = subject; 171 this.coverage = coverage; 172 this.relationship = relationship; 173 } 174 175 /** 176 * @return {@link #identifier} (The Response business identifier.) 177 */ 178 public List<Identifier> getIdentifier() { 179 if (this.identifier == null) 180 this.identifier = new ArrayList<Identifier>(); 181 return this.identifier; 182 } 183 184 public boolean hasIdentifier() { 185 if (this.identifier == null) 186 return false; 187 for (Identifier item : this.identifier) 188 if (!item.isEmpty()) 189 return true; 190 return false; 191 } 192 193 /** 194 * @return {@link #identifier} (The Response business identifier.) 195 */ 196 // syntactic sugar 197 public Identifier addIdentifier() { // 3 198 Identifier t = new Identifier(); 199 if (this.identifier == null) 200 this.identifier = new ArrayList<Identifier>(); 201 this.identifier.add(t); 202 return t; 203 } 204 205 // syntactic sugar 206 public EnrollmentRequest addIdentifier(Identifier t) { // 3 207 if (t == null) 208 return this; 209 if (this.identifier == null) 210 this.identifier = new ArrayList<Identifier>(); 211 this.identifier.add(t); 212 return this; 213 } 214 215 /** 216 * @return {@link #ruleset} (The version of the style of resource contents. This 217 * should be mapped to the allowable profiles for this and supporting 218 * resources.) 219 */ 220 public Coding getRuleset() { 221 if (this.ruleset == null) 222 if (Configuration.errorOnAutoCreate()) 223 throw new Error("Attempt to auto-create EnrollmentRequest.ruleset"); 224 else if (Configuration.doAutoCreate()) 225 this.ruleset = new Coding(); // cc 226 return this.ruleset; 227 } 228 229 public boolean hasRuleset() { 230 return this.ruleset != null && !this.ruleset.isEmpty(); 231 } 232 233 /** 234 * @param value {@link #ruleset} (The version of the style of resource contents. 235 * This should be mapped to the allowable profiles for this and 236 * supporting resources.) 237 */ 238 public EnrollmentRequest setRuleset(Coding value) { 239 this.ruleset = value; 240 return this; 241 } 242 243 /** 244 * @return {@link #originalRuleset} (The style (standard) and version of the 245 * original material which was converted into this resource.) 246 */ 247 public Coding getOriginalRuleset() { 248 if (this.originalRuleset == null) 249 if (Configuration.errorOnAutoCreate()) 250 throw new Error("Attempt to auto-create EnrollmentRequest.originalRuleset"); 251 else if (Configuration.doAutoCreate()) 252 this.originalRuleset = new Coding(); // cc 253 return this.originalRuleset; 254 } 255 256 public boolean hasOriginalRuleset() { 257 return this.originalRuleset != null && !this.originalRuleset.isEmpty(); 258 } 259 260 /** 261 * @param value {@link #originalRuleset} (The style (standard) and version of 262 * the original material which was converted into this resource.) 263 */ 264 public EnrollmentRequest setOriginalRuleset(Coding value) { 265 this.originalRuleset = value; 266 return this; 267 } 268 269 /** 270 * @return {@link #created} (The date when this resource was created.). This is 271 * the underlying object with id, value and extensions. The accessor 272 * "getCreated" gives direct access to the value 273 */ 274 public DateTimeType getCreatedElement() { 275 if (this.created == null) 276 if (Configuration.errorOnAutoCreate()) 277 throw new Error("Attempt to auto-create EnrollmentRequest.created"); 278 else if (Configuration.doAutoCreate()) 279 this.created = new DateTimeType(); // bb 280 return this.created; 281 } 282 283 public boolean hasCreatedElement() { 284 return this.created != null && !this.created.isEmpty(); 285 } 286 287 public boolean hasCreated() { 288 return this.created != null && !this.created.isEmpty(); 289 } 290 291 /** 292 * @param value {@link #created} (The date when this resource was created.). 293 * This is the underlying object with id, value and extensions. The 294 * accessor "getCreated" gives direct access to the value 295 */ 296 public EnrollmentRequest setCreatedElement(DateTimeType value) { 297 this.created = value; 298 return this; 299 } 300 301 /** 302 * @return The date when this resource was created. 303 */ 304 public Date getCreated() { 305 return this.created == null ? null : this.created.getValue(); 306 } 307 308 /** 309 * @param value The date when this resource was created. 310 */ 311 public EnrollmentRequest setCreated(Date value) { 312 if (value == null) 313 this.created = null; 314 else { 315 if (this.created == null) 316 this.created = new DateTimeType(); 317 this.created.setValue(value); 318 } 319 return this; 320 } 321 322 /** 323 * @return {@link #target} (The Insurer who is target of the request.) 324 */ 325 public Reference getTarget() { 326 if (this.target == null) 327 if (Configuration.errorOnAutoCreate()) 328 throw new Error("Attempt to auto-create EnrollmentRequest.target"); 329 else if (Configuration.doAutoCreate()) 330 this.target = new Reference(); // cc 331 return this.target; 332 } 333 334 public boolean hasTarget() { 335 return this.target != null && !this.target.isEmpty(); 336 } 337 338 /** 339 * @param value {@link #target} (The Insurer who is target of the request.) 340 */ 341 public EnrollmentRequest setTarget(Reference value) { 342 this.target = value; 343 return this; 344 } 345 346 /** 347 * @return {@link #target} The actual object that is the target of the 348 * reference. The reference library doesn't populate this, but you can 349 * use it to hold the resource if you resolve it. (The Insurer who is 350 * target of the request.) 351 */ 352 public Organization getTargetTarget() { 353 if (this.targetTarget == null) 354 if (Configuration.errorOnAutoCreate()) 355 throw new Error("Attempt to auto-create EnrollmentRequest.target"); 356 else if (Configuration.doAutoCreate()) 357 this.targetTarget = new Organization(); // aa 358 return this.targetTarget; 359 } 360 361 /** 362 * @param value {@link #target} The actual object that is the target of the 363 * reference. The reference library doesn't use these, but you can 364 * use it to hold the resource if you resolve it. (The Insurer who 365 * is target of the request.) 366 */ 367 public EnrollmentRequest setTargetTarget(Organization value) { 368 this.targetTarget = value; 369 return this; 370 } 371 372 /** 373 * @return {@link #provider} (The practitioner who is responsible for the 374 * services rendered to the patient.) 375 */ 376 public Reference getProvider() { 377 if (this.provider == null) 378 if (Configuration.errorOnAutoCreate()) 379 throw new Error("Attempt to auto-create EnrollmentRequest.provider"); 380 else if (Configuration.doAutoCreate()) 381 this.provider = new Reference(); // cc 382 return this.provider; 383 } 384 385 public boolean hasProvider() { 386 return this.provider != null && !this.provider.isEmpty(); 387 } 388 389 /** 390 * @param value {@link #provider} (The practitioner who is responsible for the 391 * services rendered to the patient.) 392 */ 393 public EnrollmentRequest setProvider(Reference value) { 394 this.provider = value; 395 return this; 396 } 397 398 /** 399 * @return {@link #provider} The actual object that is the target of the 400 * reference. The reference library doesn't populate this, but you can 401 * use it to hold the resource if you resolve it. (The practitioner who 402 * is responsible for the services rendered to the patient.) 403 */ 404 public Practitioner getProviderTarget() { 405 if (this.providerTarget == null) 406 if (Configuration.errorOnAutoCreate()) 407 throw new Error("Attempt to auto-create EnrollmentRequest.provider"); 408 else if (Configuration.doAutoCreate()) 409 this.providerTarget = new Practitioner(); // aa 410 return this.providerTarget; 411 } 412 413 /** 414 * @param value {@link #provider} The actual object that is the target of the 415 * reference. The reference library doesn't use these, but you can 416 * use it to hold the resource if you resolve it. (The practitioner 417 * who is responsible for the services rendered to the patient.) 418 */ 419 public EnrollmentRequest setProviderTarget(Practitioner value) { 420 this.providerTarget = value; 421 return this; 422 } 423 424 /** 425 * @return {@link #organization} (The organization which is responsible for the 426 * services rendered to the patient.) 427 */ 428 public Reference getOrganization() { 429 if (this.organization == null) 430 if (Configuration.errorOnAutoCreate()) 431 throw new Error("Attempt to auto-create EnrollmentRequest.organization"); 432 else if (Configuration.doAutoCreate()) 433 this.organization = new Reference(); // cc 434 return this.organization; 435 } 436 437 public boolean hasOrganization() { 438 return this.organization != null && !this.organization.isEmpty(); 439 } 440 441 /** 442 * @param value {@link #organization} (The organization which is responsible for 443 * the services rendered to the patient.) 444 */ 445 public EnrollmentRequest setOrganization(Reference value) { 446 this.organization = value; 447 return this; 448 } 449 450 /** 451 * @return {@link #organization} The actual object that is the target of the 452 * reference. The reference library doesn't populate this, but you can 453 * use it to hold the resource if you resolve it. (The organization 454 * which is responsible for the services rendered to the patient.) 455 */ 456 public Organization getOrganizationTarget() { 457 if (this.organizationTarget == null) 458 if (Configuration.errorOnAutoCreate()) 459 throw new Error("Attempt to auto-create EnrollmentRequest.organization"); 460 else if (Configuration.doAutoCreate()) 461 this.organizationTarget = new Organization(); // aa 462 return this.organizationTarget; 463 } 464 465 /** 466 * @param value {@link #organization} The actual object that is the target of 467 * the reference. The reference library doesn't use these, but you 468 * can use it to hold the resource if you resolve it. (The 469 * organization which is responsible for the services rendered to 470 * the patient.) 471 */ 472 public EnrollmentRequest setOrganizationTarget(Organization value) { 473 this.organizationTarget = value; 474 return this; 475 } 476 477 /** 478 * @return {@link #subject} (Patient Resource.) 479 */ 480 public Reference getSubject() { 481 if (this.subject == null) 482 if (Configuration.errorOnAutoCreate()) 483 throw new Error("Attempt to auto-create EnrollmentRequest.subject"); 484 else if (Configuration.doAutoCreate()) 485 this.subject = new Reference(); // cc 486 return this.subject; 487 } 488 489 public boolean hasSubject() { 490 return this.subject != null && !this.subject.isEmpty(); 491 } 492 493 /** 494 * @param value {@link #subject} (Patient Resource.) 495 */ 496 public EnrollmentRequest setSubject(Reference value) { 497 this.subject = value; 498 return this; 499 } 500 501 /** 502 * @return {@link #subject} The actual object that is the target of the 503 * reference. The reference library doesn't populate this, but you can 504 * use it to hold the resource if you resolve it. (Patient Resource.) 505 */ 506 public Patient getSubjectTarget() { 507 if (this.subjectTarget == null) 508 if (Configuration.errorOnAutoCreate()) 509 throw new Error("Attempt to auto-create EnrollmentRequest.subject"); 510 else if (Configuration.doAutoCreate()) 511 this.subjectTarget = new Patient(); // aa 512 return this.subjectTarget; 513 } 514 515 /** 516 * @param value {@link #subject} The actual object that is the target of the 517 * reference. The reference library doesn't use these, but you can 518 * use it to hold the resource if you resolve it. (Patient 519 * Resource.) 520 */ 521 public EnrollmentRequest setSubjectTarget(Patient value) { 522 this.subjectTarget = value; 523 return this; 524 } 525 526 /** 527 * @return {@link #coverage} (Reference to the program or plan identification, 528 * underwriter or payor.) 529 */ 530 public Reference getCoverage() { 531 if (this.coverage == null) 532 if (Configuration.errorOnAutoCreate()) 533 throw new Error("Attempt to auto-create EnrollmentRequest.coverage"); 534 else if (Configuration.doAutoCreate()) 535 this.coverage = new Reference(); // cc 536 return this.coverage; 537 } 538 539 public boolean hasCoverage() { 540 return this.coverage != null && !this.coverage.isEmpty(); 541 } 542 543 /** 544 * @param value {@link #coverage} (Reference to the program or plan 545 * identification, underwriter or payor.) 546 */ 547 public EnrollmentRequest setCoverage(Reference value) { 548 this.coverage = value; 549 return this; 550 } 551 552 /** 553 * @return {@link #coverage} The actual object that is the target of the 554 * reference. The reference library doesn't populate this, but you can 555 * use it to hold the resource if you resolve it. (Reference to the 556 * program or plan identification, underwriter or payor.) 557 */ 558 public Coverage getCoverageTarget() { 559 if (this.coverageTarget == null) 560 if (Configuration.errorOnAutoCreate()) 561 throw new Error("Attempt to auto-create EnrollmentRequest.coverage"); 562 else if (Configuration.doAutoCreate()) 563 this.coverageTarget = new Coverage(); // aa 564 return this.coverageTarget; 565 } 566 567 /** 568 * @param value {@link #coverage} The actual object that is the target of the 569 * reference. The reference library doesn't use these, but you can 570 * use it to hold the resource if you resolve it. (Reference to the 571 * program or plan identification, underwriter or payor.) 572 */ 573 public EnrollmentRequest setCoverageTarget(Coverage value) { 574 this.coverageTarget = value; 575 return this; 576 } 577 578 /** 579 * @return {@link #relationship} (The relationship of the patient to the 580 * subscriber.) 581 */ 582 public Coding getRelationship() { 583 if (this.relationship == null) 584 if (Configuration.errorOnAutoCreate()) 585 throw new Error("Attempt to auto-create EnrollmentRequest.relationship"); 586 else if (Configuration.doAutoCreate()) 587 this.relationship = new Coding(); // cc 588 return this.relationship; 589 } 590 591 public boolean hasRelationship() { 592 return this.relationship != null && !this.relationship.isEmpty(); 593 } 594 595 /** 596 * @param value {@link #relationship} (The relationship of the patient to the 597 * subscriber.) 598 */ 599 public EnrollmentRequest setRelationship(Coding value) { 600 this.relationship = value; 601 return this; 602 } 603 604 protected void listChildren(List<Property> childrenList) { 605 super.listChildren(childrenList); 606 childrenList.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, 607 java.lang.Integer.MAX_VALUE, identifier)); 608 childrenList.add(new Property("ruleset", "Coding", 609 "The version of the style of resource contents. This should be mapped to the allowable profiles for this and supporting resources.", 610 0, java.lang.Integer.MAX_VALUE, ruleset)); 611 childrenList.add(new Property("originalRuleset", "Coding", 612 "The style (standard) and version of the original material which was converted into this resource.", 0, 613 java.lang.Integer.MAX_VALUE, originalRuleset)); 614 childrenList.add(new Property("created", "dateTime", "The date when this resource was created.", 0, 615 java.lang.Integer.MAX_VALUE, created)); 616 childrenList.add(new Property("target", "Reference(Organization)", "The Insurer who is target of the request.", 0, 617 java.lang.Integer.MAX_VALUE, target)); 618 childrenList.add(new Property("provider", "Reference(Practitioner)", 619 "The practitioner who is responsible for the services rendered to the patient.", 0, java.lang.Integer.MAX_VALUE, 620 provider)); 621 childrenList.add(new Property("organization", "Reference(Organization)", 622 "The organization which is responsible for the services rendered to the patient.", 0, 623 java.lang.Integer.MAX_VALUE, organization)); 624 childrenList.add( 625 new Property("subject", "Reference(Patient)", "Patient Resource.", 0, java.lang.Integer.MAX_VALUE, subject)); 626 childrenList.add(new Property("coverage", "Reference(Coverage)", 627 "Reference to the program or plan identification, underwriter or payor.", 0, java.lang.Integer.MAX_VALUE, 628 coverage)); 629 childrenList.add(new Property("relationship", "Coding", "The relationship of the patient to the subscriber.", 0, 630 java.lang.Integer.MAX_VALUE, relationship)); 631 } 632 633 @Override 634 public void setProperty(String name, Base value) throws FHIRException { 635 if (name.equals("identifier")) 636 this.getIdentifier().add(castToIdentifier(value)); 637 else if (name.equals("ruleset")) 638 this.ruleset = castToCoding(value); // Coding 639 else if (name.equals("originalRuleset")) 640 this.originalRuleset = castToCoding(value); // Coding 641 else if (name.equals("created")) 642 this.created = castToDateTime(value); // DateTimeType 643 else if (name.equals("target")) 644 this.target = castToReference(value); // Reference 645 else if (name.equals("provider")) 646 this.provider = castToReference(value); // Reference 647 else if (name.equals("organization")) 648 this.organization = castToReference(value); // Reference 649 else if (name.equals("subject")) 650 this.subject = castToReference(value); // Reference 651 else if (name.equals("coverage")) 652 this.coverage = castToReference(value); // Reference 653 else if (name.equals("relationship")) 654 this.relationship = castToCoding(value); // Coding 655 else 656 super.setProperty(name, value); 657 } 658 659 @Override 660 public Base addChild(String name) throws FHIRException { 661 if (name.equals("identifier")) { 662 return addIdentifier(); 663 } else if (name.equals("ruleset")) { 664 this.ruleset = new Coding(); 665 return this.ruleset; 666 } else if (name.equals("originalRuleset")) { 667 this.originalRuleset = new Coding(); 668 return this.originalRuleset; 669 } else if (name.equals("created")) { 670 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentRequest.created"); 671 } else if (name.equals("target")) { 672 this.target = new Reference(); 673 return this.target; 674 } else if (name.equals("provider")) { 675 this.provider = new Reference(); 676 return this.provider; 677 } else if (name.equals("organization")) { 678 this.organization = new Reference(); 679 return this.organization; 680 } else if (name.equals("subject")) { 681 this.subject = new Reference(); 682 return this.subject; 683 } else if (name.equals("coverage")) { 684 this.coverage = new Reference(); 685 return this.coverage; 686 } else if (name.equals("relationship")) { 687 this.relationship = new Coding(); 688 return this.relationship; 689 } else 690 return super.addChild(name); 691 } 692 693 public String fhirType() { 694 return "EnrollmentRequest"; 695 696 } 697 698 public EnrollmentRequest copy() { 699 EnrollmentRequest dst = new EnrollmentRequest(); 700 copyValues(dst); 701 if (identifier != null) { 702 dst.identifier = new ArrayList<Identifier>(); 703 for (Identifier i : identifier) 704 dst.identifier.add(i.copy()); 705 } 706 ; 707 dst.ruleset = ruleset == null ? null : ruleset.copy(); 708 dst.originalRuleset = originalRuleset == null ? null : originalRuleset.copy(); 709 dst.created = created == null ? null : created.copy(); 710 dst.target = target == null ? null : target.copy(); 711 dst.provider = provider == null ? null : provider.copy(); 712 dst.organization = organization == null ? null : organization.copy(); 713 dst.subject = subject == null ? null : subject.copy(); 714 dst.coverage = coverage == null ? null : coverage.copy(); 715 dst.relationship = relationship == null ? null : relationship.copy(); 716 return dst; 717 } 718 719 protected EnrollmentRequest typedCopy() { 720 return copy(); 721 } 722 723 @Override 724 public boolean equalsDeep(Base other) { 725 if (!super.equalsDeep(other)) 726 return false; 727 if (!(other instanceof EnrollmentRequest)) 728 return false; 729 EnrollmentRequest o = (EnrollmentRequest) other; 730 return compareDeep(identifier, o.identifier, true) && compareDeep(ruleset, o.ruleset, true) 731 && compareDeep(originalRuleset, o.originalRuleset, true) && compareDeep(created, o.created, true) 732 && compareDeep(target, o.target, true) && compareDeep(provider, o.provider, true) 733 && compareDeep(organization, o.organization, true) && compareDeep(subject, o.subject, true) 734 && compareDeep(coverage, o.coverage, true) && compareDeep(relationship, o.relationship, true); 735 } 736 737 @Override 738 public boolean equalsShallow(Base other) { 739 if (!super.equalsShallow(other)) 740 return false; 741 if (!(other instanceof EnrollmentRequest)) 742 return false; 743 EnrollmentRequest o = (EnrollmentRequest) other; 744 return compareValues(created, o.created, true); 745 } 746 747 public boolean isEmpty() { 748 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (ruleset == null || ruleset.isEmpty()) 749 && (originalRuleset == null || originalRuleset.isEmpty()) && (created == null || created.isEmpty()) 750 && (target == null || target.isEmpty()) && (provider == null || provider.isEmpty()) 751 && (organization == null || organization.isEmpty()) && (subject == null || subject.isEmpty()) 752 && (coverage == null || coverage.isEmpty()) && (relationship == null || relationship.isEmpty()); 753 } 754 755 @Override 756 public ResourceType getResourceType() { 757 return ResourceType.EnrollmentRequest; 758 } 759 760 @SearchParamDefinition(name = "identifier", path = "EnrollmentRequest.identifier", description = "The business identifier of the Enrollment", type = "token") 761 public static final String SP_IDENTIFIER = "identifier"; 762 @SearchParamDefinition(name = "subject", path = "EnrollmentRequest.subject", description = "The party to be enrolled", type = "reference") 763 public static final String SP_SUBJECT = "subject"; 764 @SearchParamDefinition(name = "patient", path = "EnrollmentRequest.subject", description = "The party to be enrolled", type = "reference") 765 public static final String SP_PATIENT = "patient"; 766 767}