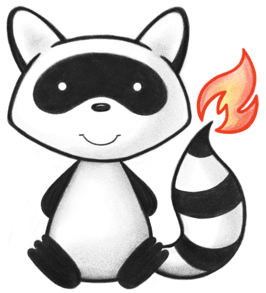
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import ca.uhn.fhir.model.api.annotation.DatatypeDef; 033import org.hl7.fhir.instance.model.api.IBaseEnumFactory; 034import org.hl7.fhir.instance.model.api.IBaseEnumeration; 035 036/* 037Copyright (c) 2011+, HL7, Inc 038All rights reserved. 039 040Redistribution and use in source and binary forms, with or without modification, 041are permitted provided that the following conditions are met: 042 043 * Redistributions of source code must retain the above copyright notice, this 044 list of conditions and the following disclaimer. 045 * Redistributions in binary form must reproduce the above copyright notice, 046 this list of conditions and the following disclaimer in the documentation 047 and/or other materials provided with the distribution. 048 * Neither the name of HL7 nor the names of its contributors may be used to 049 endorse or promote products derived from this software without specific 050 prior written permission. 051 052THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 053ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 054WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 055IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 056INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 057NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 058PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 059WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 060ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 061POSSIBILITY OF SUCH DAMAGE. 062 063*/ 064 065/** 066 * Primitive type "code" in FHIR, where the code is tied to an enumerated list 067 * of possible values 068 * 069 */ 070@DatatypeDef(name = "code", isSpecialization = true) 071public class Enumeration<T extends Enum<?>> extends PrimitiveType<T> implements IBaseEnumeration<T> { 072 073 private static final long serialVersionUID = 1L; 074 private EnumFactory<T> myEnumFactory; 075 076 /** 077 * Constructor 078 */ 079 public Enumeration(EnumFactory<T> theEnumFactory) { 080 if (theEnumFactory == null) 081 throw new IllegalArgumentException("An enumeration factory must be provided"); 082 myEnumFactory = theEnumFactory; 083 } 084 085 /** 086 * Constructor 087 */ 088 public Enumeration(EnumFactory<T> theEnumFactory, T theValue) { 089 if (theEnumFactory == null) 090 throw new IllegalArgumentException("An enumeration factory must be provided"); 091 myEnumFactory = theEnumFactory; 092 setValue(theValue); 093 } 094 095 /** 096 * Constructor 097 */ 098 public Enumeration(EnumFactory<T> theEnumFactory, String theValue) { 099 if (theEnumFactory == null) 100 throw new IllegalArgumentException("An enumeration factory must be provided"); 101 myEnumFactory = theEnumFactory; 102 setValueAsString(theValue); 103 } 104 105 @Override 106 protected T parse(String theValue) { 107 if (myEnumFactory != null) { 108 return myEnumFactory.fromCode(theValue); 109 } 110 return null; 111 } 112 113 @Override 114 protected String encode(T theValue) { 115 return myEnumFactory.toCode(theValue); 116 } 117 118 @Override 119 public Enumeration<T> copy() { 120 return new Enumeration<T>(myEnumFactory, getValue()); 121 } 122 123 public String fhirType() { 124 return "code"; 125 } 126 127 @Override 128 public IBaseEnumFactory<T> getEnumFactory() { 129 return myEnumFactory; 130 } 131}