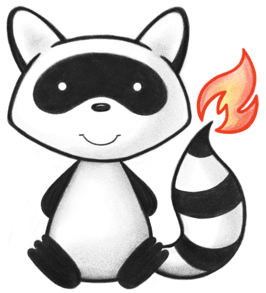
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import org.hl7.fhir.exceptions.FHIRException; 033 034public class Enumerations { 035 036// In here: 037// AdministrativeGender: The gender of a person used for administrative purposes. 038// AgeUnits: A valueSet of UCUM codes for representing age value units. 039// BindingStrength: Indication of the degree of conformance expectations associated with a binding. 040// ConceptMapEquivalence: The degree of equivalence between concepts. 041// ConformanceResourceStatus: The lifecycle status of a Value Set or Concept Map. 042// DataAbsentReason: Used to specify why the normally expected content of the data element is missing. 043// DataType: The type of an element - one of the FHIR data types. 044// DocumentReferenceStatus: The status of the document reference. 045// FHIRDefinedType: Either a resource or a data type. 046// MessageEvent: One of the message events defined as part of FHIR. 047// NoteType: The presentation types of notes. 048// RemittanceOutcome: The outcome of the processing. 049// ResourceType: One of the resource types defined as part of FHIR. 050// SearchParamType: Data types allowed to be used for search parameters. 051// SpecialValues: A set of generally useful codes defined so they can be included in value sets. 052 053 public enum AdministrativeGender { 054 /** 055 * Male 056 */ 057 MALE, 058 /** 059 * Female 060 */ 061 FEMALE, 062 /** 063 * Other 064 */ 065 OTHER, 066 /** 067 * Unknown 068 */ 069 UNKNOWN, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 075 public static AdministrativeGender fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("male".equals(codeString)) 079 return MALE; 080 if ("female".equals(codeString)) 081 return FEMALE; 082 if ("other".equals(codeString)) 083 return OTHER; 084 if ("unknown".equals(codeString)) 085 return UNKNOWN; 086 throw new FHIRException("Unknown AdministrativeGender code '" + codeString + "'"); 087 } 088 089 public String toCode() { 090 switch (this) { 091 case MALE: 092 return "male"; 093 case FEMALE: 094 return "female"; 095 case OTHER: 096 return "other"; 097 case UNKNOWN: 098 return "unknown"; 099 case NULL: 100 return null; 101 default: 102 return "?"; 103 } 104 } 105 106 public String getSystem() { 107 switch (this) { 108 case MALE: 109 return "http://hl7.org/fhir/administrative-gender"; 110 case FEMALE: 111 return "http://hl7.org/fhir/administrative-gender"; 112 case OTHER: 113 return "http://hl7.org/fhir/administrative-gender"; 114 case UNKNOWN: 115 return "http://hl7.org/fhir/administrative-gender"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getDefinition() { 124 switch (this) { 125 case MALE: 126 return "Male"; 127 case FEMALE: 128 return "Female"; 129 case OTHER: 130 return "Other"; 131 case UNKNOWN: 132 return "Unknown"; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getDisplay() { 141 switch (this) { 142 case MALE: 143 return "Male"; 144 case FEMALE: 145 return "Female"; 146 case OTHER: 147 return "Other"; 148 case UNKNOWN: 149 return "Unknown"; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 } 157 158 public static class AdministrativeGenderEnumFactory implements EnumFactory<AdministrativeGender> { 159 public AdministrativeGender fromCode(String codeString) throws IllegalArgumentException { 160 if (codeString == null || "".equals(codeString)) 161 if (codeString == null || "".equals(codeString)) 162 return null; 163 if ("male".equals(codeString)) 164 return AdministrativeGender.MALE; 165 if ("female".equals(codeString)) 166 return AdministrativeGender.FEMALE; 167 if ("other".equals(codeString)) 168 return AdministrativeGender.OTHER; 169 if ("unknown".equals(codeString)) 170 return AdministrativeGender.UNKNOWN; 171 throw new IllegalArgumentException("Unknown AdministrativeGender code '" + codeString + "'"); 172 } 173 174 public Enumeration<AdministrativeGender> fromType(Base code) throws FHIRException { 175 if (code == null || code.isEmpty()) 176 return null; 177 String codeString = ((PrimitiveType) code).asStringValue(); 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("male".equals(codeString)) 181 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.MALE); 182 if ("female".equals(codeString)) 183 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.FEMALE); 184 if ("other".equals(codeString)) 185 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.OTHER); 186 if ("unknown".equals(codeString)) 187 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.UNKNOWN); 188 throw new FHIRException("Unknown AdministrativeGender code '" + codeString + "'"); 189 } 190 191 public String toCode(AdministrativeGender code) 192 { 193 if (code == AdministrativeGender.NULL) 194 return null; 195 if (code == AdministrativeGender.MALE) 196 return "male"; 197 if (code == AdministrativeGender.FEMALE) 198 return "female"; 199 if (code == AdministrativeGender.OTHER) 200 return "other"; 201 if (code == AdministrativeGender.UNKNOWN) 202 return "unknown"; 203 return "?"; 204 } 205 } 206 207 public enum AgeUnits { 208 /** 209 * null 210 */ 211 MIN, 212 /** 213 * null 214 */ 215 H, 216 /** 217 * null 218 */ 219 D, 220 /** 221 * null 222 */ 223 WK, 224 /** 225 * null 226 */ 227 MO, 228 /** 229 * null 230 */ 231 A, 232 /** 233 * added to help the parsers 234 */ 235 NULL; 236 237 public static AgeUnits fromCode(String codeString) throws FHIRException { 238 if (codeString == null || "".equals(codeString)) 239 return null; 240 if ("min".equals(codeString)) 241 return MIN; 242 if ("h".equals(codeString)) 243 return H; 244 if ("d".equals(codeString)) 245 return D; 246 if ("wk".equals(codeString)) 247 return WK; 248 if ("mo".equals(codeString)) 249 return MO; 250 if ("a".equals(codeString)) 251 return A; 252 throw new FHIRException("Unknown AgeUnits code '" + codeString + "'"); 253 } 254 255 public String toCode() { 256 switch (this) { 257 case MIN: 258 return "min"; 259 case H: 260 return "h"; 261 case D: 262 return "d"; 263 case WK: 264 return "wk"; 265 case MO: 266 return "mo"; 267 case A: 268 return "a"; 269 case NULL: 270 return null; 271 default: 272 return "?"; 273 } 274 } 275 276 public String getSystem() { 277 switch (this) { 278 case MIN: 279 return "http://unitsofmeasure.org"; 280 case H: 281 return "http://unitsofmeasure.org"; 282 case D: 283 return "http://unitsofmeasure.org"; 284 case WK: 285 return "http://unitsofmeasure.org"; 286 case MO: 287 return "http://unitsofmeasure.org"; 288 case A: 289 return "http://unitsofmeasure.org"; 290 case NULL: 291 return null; 292 default: 293 return "?"; 294 } 295 } 296 297 public String getDefinition() { 298 switch (this) { 299 case MIN: 300 return ""; 301 case H: 302 return ""; 303 case D: 304 return ""; 305 case WK: 306 return ""; 307 case MO: 308 return ""; 309 case A: 310 return ""; 311 case NULL: 312 return null; 313 default: 314 return "?"; 315 } 316 } 317 318 public String getDisplay() { 319 switch (this) { 320 case MIN: 321 return "Minute"; 322 case H: 323 return "Hour"; 324 case D: 325 return "Day"; 326 case WK: 327 return "Week"; 328 case MO: 329 return "Month"; 330 case A: 331 return "Year"; 332 case NULL: 333 return null; 334 default: 335 return "?"; 336 } 337 } 338 } 339 340 public static class AgeUnitsEnumFactory implements EnumFactory<AgeUnits> { 341 public AgeUnits fromCode(String codeString) throws IllegalArgumentException { 342 if (codeString == null || "".equals(codeString)) 343 if (codeString == null || "".equals(codeString)) 344 return null; 345 if ("min".equals(codeString)) 346 return AgeUnits.MIN; 347 if ("h".equals(codeString)) 348 return AgeUnits.H; 349 if ("d".equals(codeString)) 350 return AgeUnits.D; 351 if ("wk".equals(codeString)) 352 return AgeUnits.WK; 353 if ("mo".equals(codeString)) 354 return AgeUnits.MO; 355 if ("a".equals(codeString)) 356 return AgeUnits.A; 357 throw new IllegalArgumentException("Unknown AgeUnits code '" + codeString + "'"); 358 } 359 360 public Enumeration<AgeUnits> fromType(Base code) throws FHIRException { 361 if (code == null || code.isEmpty()) 362 return null; 363 String codeString = ((PrimitiveType) code).asStringValue(); 364 if (codeString == null || "".equals(codeString)) 365 return null; 366 if ("min".equals(codeString)) 367 return new Enumeration<AgeUnits>(this, AgeUnits.MIN); 368 if ("h".equals(codeString)) 369 return new Enumeration<AgeUnits>(this, AgeUnits.H); 370 if ("d".equals(codeString)) 371 return new Enumeration<AgeUnits>(this, AgeUnits.D); 372 if ("wk".equals(codeString)) 373 return new Enumeration<AgeUnits>(this, AgeUnits.WK); 374 if ("mo".equals(codeString)) 375 return new Enumeration<AgeUnits>(this, AgeUnits.MO); 376 if ("a".equals(codeString)) 377 return new Enumeration<AgeUnits>(this, AgeUnits.A); 378 throw new FHIRException("Unknown AgeUnits code '" + codeString + "'"); 379 } 380 381 public String toCode(AgeUnits code) 382 { 383 if (code == AgeUnits.NULL) 384 return null; 385 if (code == AgeUnits.MIN) 386 return "min"; 387 if (code == AgeUnits.H) 388 return "h"; 389 if (code == AgeUnits.D) 390 return "d"; 391 if (code == AgeUnits.WK) 392 return "wk"; 393 if (code == AgeUnits.MO) 394 return "mo"; 395 if (code == AgeUnits.A) 396 return "a"; 397 return "?"; 398 } 399 } 400 401 public enum BindingStrength { 402 /** 403 * To be conformant, instances of this element SHALL include a code from the 404 * specified value set. 405 */ 406 REQUIRED, 407 /** 408 * To be conformant, instances of this element SHALL include a code from the 409 * specified value set if any of the codes within the value set can apply to the 410 * concept being communicated. If the valueset does not cover the concept (based 411 * on human review), alternate codings (or, data type allowing, text) may be 412 * included instead. 413 */ 414 EXTENSIBLE, 415 /** 416 * Instances are encouraged to draw from the specified codes for 417 * interoperability purposes but are not required to do so to be considered 418 * conformant. 419 */ 420 PREFERRED, 421 /** 422 * Instances are not expected or even encouraged to draw from the specified 423 * value set. The value set merely provides examples of the types of concepts 424 * intended to be included. 425 */ 426 EXAMPLE, 427 /** 428 * added to help the parsers 429 */ 430 NULL; 431 432 public static BindingStrength fromCode(String codeString) throws FHIRException { 433 if (codeString == null || "".equals(codeString)) 434 return null; 435 if ("required".equals(codeString)) 436 return REQUIRED; 437 if ("extensible".equals(codeString)) 438 return EXTENSIBLE; 439 if ("preferred".equals(codeString)) 440 return PREFERRED; 441 if ("example".equals(codeString)) 442 return EXAMPLE; 443 throw new FHIRException("Unknown BindingStrength code '" + codeString + "'"); 444 } 445 446 public String toCode() { 447 switch (this) { 448 case REQUIRED: 449 return "required"; 450 case EXTENSIBLE: 451 return "extensible"; 452 case PREFERRED: 453 return "preferred"; 454 case EXAMPLE: 455 return "example"; 456 case NULL: 457 return null; 458 default: 459 return "?"; 460 } 461 } 462 463 public String getSystem() { 464 switch (this) { 465 case REQUIRED: 466 return "http://hl7.org/fhir/binding-strength"; 467 case EXTENSIBLE: 468 return "http://hl7.org/fhir/binding-strength"; 469 case PREFERRED: 470 return "http://hl7.org/fhir/binding-strength"; 471 case EXAMPLE: 472 return "http://hl7.org/fhir/binding-strength"; 473 case NULL: 474 return null; 475 default: 476 return "?"; 477 } 478 } 479 480 public String getDefinition() { 481 switch (this) { 482 case REQUIRED: 483 return "To be conformant, instances of this element SHALL include a code from the specified value set."; 484 case EXTENSIBLE: 485 return "To be conformant, instances of this element SHALL include a code from the specified value set if any of the codes within the value set can apply to the concept being communicated. If the valueset does not cover the concept (based on human review), alternate codings (or, data type allowing, text) may be included instead."; 486 case PREFERRED: 487 return "Instances are encouraged to draw from the specified codes for interoperability purposes but are not required to do so to be considered conformant."; 488 case EXAMPLE: 489 return "Instances are not expected or even encouraged to draw from the specified value set. The value set merely provides examples of the types of concepts intended to be included."; 490 case NULL: 491 return null; 492 default: 493 return "?"; 494 } 495 } 496 497 public String getDisplay() { 498 switch (this) { 499 case REQUIRED: 500 return "Required"; 501 case EXTENSIBLE: 502 return "Extensible"; 503 case PREFERRED: 504 return "Preferred"; 505 case EXAMPLE: 506 return "Example"; 507 case NULL: 508 return null; 509 default: 510 return "?"; 511 } 512 } 513 } 514 515 public static class BindingStrengthEnumFactory implements EnumFactory<BindingStrength> { 516 public BindingStrength fromCode(String codeString) throws IllegalArgumentException { 517 if (codeString == null || "".equals(codeString)) 518 if (codeString == null || "".equals(codeString)) 519 return null; 520 if ("required".equals(codeString)) 521 return BindingStrength.REQUIRED; 522 if ("extensible".equals(codeString)) 523 return BindingStrength.EXTENSIBLE; 524 if ("preferred".equals(codeString)) 525 return BindingStrength.PREFERRED; 526 if ("example".equals(codeString)) 527 return BindingStrength.EXAMPLE; 528 throw new IllegalArgumentException("Unknown BindingStrength code '" + codeString + "'"); 529 } 530 531 public Enumeration<BindingStrength> fromType(Base code) throws FHIRException { 532 if (code == null || code.isEmpty()) 533 return null; 534 String codeString = ((PrimitiveType) code).asStringValue(); 535 if (codeString == null || "".equals(codeString)) 536 return null; 537 if ("required".equals(codeString)) 538 return new Enumeration<BindingStrength>(this, BindingStrength.REQUIRED); 539 if ("extensible".equals(codeString)) 540 return new Enumeration<BindingStrength>(this, BindingStrength.EXTENSIBLE); 541 if ("preferred".equals(codeString)) 542 return new Enumeration<BindingStrength>(this, BindingStrength.PREFERRED); 543 if ("example".equals(codeString)) 544 return new Enumeration<BindingStrength>(this, BindingStrength.EXAMPLE); 545 throw new FHIRException("Unknown BindingStrength code '" + codeString + "'"); 546 } 547 548 public String toCode(BindingStrength code) 549 { 550 if (code == BindingStrength.NULL) 551 return null; 552 if (code == BindingStrength.REQUIRED) 553 return "required"; 554 if (code == BindingStrength.EXTENSIBLE) 555 return "extensible"; 556 if (code == BindingStrength.PREFERRED) 557 return "preferred"; 558 if (code == BindingStrength.EXAMPLE) 559 return "example"; 560 return "?"; 561 } 562 } 563 564 public enum ConceptMapEquivalence { 565 /** 566 * The definitions of the concepts mean the same thing (including when 567 * structural implications of meaning are considered) (i.e. extensionally 568 * identical). 569 */ 570 EQUIVALENT, 571 /** 572 * The definitions of the concepts are exactly the same (i.e. only grammatical 573 * differences) and structural implications of meaning are identical or 574 * irrelevant (i.e. intentionally identical). 575 */ 576 EQUAL, 577 /** 578 * The target mapping is wider in meaning than the source concept. 579 */ 580 WIDER, 581 /** 582 * The target mapping subsumes the meaning of the source concept (e.g. the 583 * source is-a target). 584 */ 585 SUBSUMES, 586 /** 587 * The target mapping is narrower in meaning that the source concept. The sense 588 * in which the mapping is narrower SHALL be described in the comments in this 589 * case, and applications should be careful when attempting to use these 590 * mappings operationally. 591 */ 592 NARROWER, 593 /** 594 * The target mapping specializes the meaning of the source concept (e.g. the 595 * target is-a source). 596 */ 597 SPECIALIZES, 598 /** 599 * The target mapping overlaps with the source concept, but both source and 600 * target cover additional meaning, or the definitions are imprecise and it is 601 * uncertain whether they have the same boundaries to their meaning. The sense 602 * in which the mapping is narrower SHALL be described in the comments in this 603 * case, and applications should be careful when attempting to use these 604 * mappings operationally. 605 */ 606 INEXACT, 607 /** 608 * There is no match for this concept in the destination concept system. 609 */ 610 UNMATCHED, 611 /** 612 * This is an explicit assertion that there is no mapping between the source and 613 * target concept. 614 */ 615 DISJOINT, 616 /** 617 * added to help the parsers 618 */ 619 NULL; 620 621 public static ConceptMapEquivalence fromCode(String codeString) throws FHIRException { 622 if (codeString == null || "".equals(codeString)) 623 return null; 624 if ("equivalent".equals(codeString)) 625 return EQUIVALENT; 626 if ("equal".equals(codeString)) 627 return EQUAL; 628 if ("wider".equals(codeString)) 629 return WIDER; 630 if ("subsumes".equals(codeString)) 631 return SUBSUMES; 632 if ("narrower".equals(codeString)) 633 return NARROWER; 634 if ("specializes".equals(codeString)) 635 return SPECIALIZES; 636 if ("inexact".equals(codeString)) 637 return INEXACT; 638 if ("unmatched".equals(codeString)) 639 return UNMATCHED; 640 if ("disjoint".equals(codeString)) 641 return DISJOINT; 642 throw new FHIRException("Unknown ConceptMapEquivalence code '" + codeString + "'"); 643 } 644 645 public String toCode() { 646 switch (this) { 647 case EQUIVALENT: 648 return "equivalent"; 649 case EQUAL: 650 return "equal"; 651 case WIDER: 652 return "wider"; 653 case SUBSUMES: 654 return "subsumes"; 655 case NARROWER: 656 return "narrower"; 657 case SPECIALIZES: 658 return "specializes"; 659 case INEXACT: 660 return "inexact"; 661 case UNMATCHED: 662 return "unmatched"; 663 case DISJOINT: 664 return "disjoint"; 665 case NULL: 666 return null; 667 default: 668 return "?"; 669 } 670 } 671 672 public String getSystem() { 673 switch (this) { 674 case EQUIVALENT: 675 return "http://hl7.org/fhir/concept-map-equivalence"; 676 case EQUAL: 677 return "http://hl7.org/fhir/concept-map-equivalence"; 678 case WIDER: 679 return "http://hl7.org/fhir/concept-map-equivalence"; 680 case SUBSUMES: 681 return "http://hl7.org/fhir/concept-map-equivalence"; 682 case NARROWER: 683 return "http://hl7.org/fhir/concept-map-equivalence"; 684 case SPECIALIZES: 685 return "http://hl7.org/fhir/concept-map-equivalence"; 686 case INEXACT: 687 return "http://hl7.org/fhir/concept-map-equivalence"; 688 case UNMATCHED: 689 return "http://hl7.org/fhir/concept-map-equivalence"; 690 case DISJOINT: 691 return "http://hl7.org/fhir/concept-map-equivalence"; 692 case NULL: 693 return null; 694 default: 695 return "?"; 696 } 697 } 698 699 public String getDefinition() { 700 switch (this) { 701 case EQUIVALENT: 702 return "The definitions of the concepts mean the same thing (including when structural implications of meaning are considered) (i.e. extensionally identical)."; 703 case EQUAL: 704 return "The definitions of the concepts are exactly the same (i.e. only grammatical differences) and structural implications of meaning are identical or irrelevant (i.e. intentionally identical)."; 705 case WIDER: 706 return "The target mapping is wider in meaning than the source concept."; 707 case SUBSUMES: 708 return "The target mapping subsumes the meaning of the source concept (e.g. the source is-a target)."; 709 case NARROWER: 710 return "The target mapping is narrower in meaning that the source concept. The sense in which the mapping is narrower SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally."; 711 case SPECIALIZES: 712 return "The target mapping specializes the meaning of the source concept (e.g. the target is-a source)."; 713 case INEXACT: 714 return "The target mapping overlaps with the source concept, but both source and target cover additional meaning, or the definitions are imprecise and it is uncertain whether they have the same boundaries to their meaning. The sense in which the mapping is narrower SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally."; 715 case UNMATCHED: 716 return "There is no match for this concept in the destination concept system."; 717 case DISJOINT: 718 return "This is an explicit assertion that there is no mapping between the source and target concept."; 719 case NULL: 720 return null; 721 default: 722 return "?"; 723 } 724 } 725 726 public String getDisplay() { 727 switch (this) { 728 case EQUIVALENT: 729 return "Equivalent"; 730 case EQUAL: 731 return "Equal"; 732 case WIDER: 733 return "Wider"; 734 case SUBSUMES: 735 return "Subsumes"; 736 case NARROWER: 737 return "Narrower"; 738 case SPECIALIZES: 739 return "Specializes"; 740 case INEXACT: 741 return "Inexact"; 742 case UNMATCHED: 743 return "Unmatched"; 744 case DISJOINT: 745 return "Disjoint"; 746 case NULL: 747 return null; 748 default: 749 return "?"; 750 } 751 } 752 } 753 754 public static class ConceptMapEquivalenceEnumFactory implements EnumFactory<ConceptMapEquivalence> { 755 public ConceptMapEquivalence fromCode(String codeString) throws IllegalArgumentException { 756 if (codeString == null || "".equals(codeString)) 757 if (codeString == null || "".equals(codeString)) 758 return null; 759 if ("equivalent".equals(codeString)) 760 return ConceptMapEquivalence.EQUIVALENT; 761 if ("equal".equals(codeString)) 762 return ConceptMapEquivalence.EQUAL; 763 if ("wider".equals(codeString)) 764 return ConceptMapEquivalence.WIDER; 765 if ("subsumes".equals(codeString)) 766 return ConceptMapEquivalence.SUBSUMES; 767 if ("narrower".equals(codeString)) 768 return ConceptMapEquivalence.NARROWER; 769 if ("specializes".equals(codeString)) 770 return ConceptMapEquivalence.SPECIALIZES; 771 if ("inexact".equals(codeString)) 772 return ConceptMapEquivalence.INEXACT; 773 if ("unmatched".equals(codeString)) 774 return ConceptMapEquivalence.UNMATCHED; 775 if ("disjoint".equals(codeString)) 776 return ConceptMapEquivalence.DISJOINT; 777 throw new IllegalArgumentException("Unknown ConceptMapEquivalence code '" + codeString + "'"); 778 } 779 780 public Enumeration<ConceptMapEquivalence> fromType(Base code) throws FHIRException { 781 if (code == null || code.isEmpty()) 782 return null; 783 String codeString = ((PrimitiveType) code).asStringValue(); 784 if (codeString == null || "".equals(codeString)) 785 return null; 786 if ("equivalent".equals(codeString)) 787 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.EQUIVALENT); 788 if ("equal".equals(codeString)) 789 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.EQUAL); 790 if ("wider".equals(codeString)) 791 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.WIDER); 792 if ("subsumes".equals(codeString)) 793 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.SUBSUMES); 794 if ("narrower".equals(codeString)) 795 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.NARROWER); 796 if ("specializes".equals(codeString)) 797 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.SPECIALIZES); 798 if ("inexact".equals(codeString)) 799 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.INEXACT); 800 if ("unmatched".equals(codeString)) 801 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.UNMATCHED); 802 if ("disjoint".equals(codeString)) 803 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.DISJOINT); 804 throw new FHIRException("Unknown ConceptMapEquivalence code '" + codeString + "'"); 805 } 806 807 public String toCode(ConceptMapEquivalence code) 808 { 809 if (code == ConceptMapEquivalence.NULL) 810 return null; 811 if (code == ConceptMapEquivalence.EQUIVALENT) 812 return "equivalent"; 813 if (code == ConceptMapEquivalence.EQUAL) 814 return "equal"; 815 if (code == ConceptMapEquivalence.WIDER) 816 return "wider"; 817 if (code == ConceptMapEquivalence.SUBSUMES) 818 return "subsumes"; 819 if (code == ConceptMapEquivalence.NARROWER) 820 return "narrower"; 821 if (code == ConceptMapEquivalence.SPECIALIZES) 822 return "specializes"; 823 if (code == ConceptMapEquivalence.INEXACT) 824 return "inexact"; 825 if (code == ConceptMapEquivalence.UNMATCHED) 826 return "unmatched"; 827 if (code == ConceptMapEquivalence.DISJOINT) 828 return "disjoint"; 829 return "?"; 830 } 831 } 832 833 public enum ConformanceResourceStatus { 834 /** 835 * This resource is still under development. 836 */ 837 DRAFT, 838 /** 839 * This resource is ready for normal use. 840 */ 841 ACTIVE, 842 /** 843 * This resource has been withdrawn or superseded and should no longer be used. 844 */ 845 RETIRED, 846 /** 847 * added to help the parsers 848 */ 849 NULL; 850 851 public static ConformanceResourceStatus fromCode(String codeString) throws FHIRException { 852 if (codeString == null || "".equals(codeString)) 853 return null; 854 if ("draft".equals(codeString)) 855 return DRAFT; 856 if ("active".equals(codeString)) 857 return ACTIVE; 858 if ("retired".equals(codeString)) 859 return RETIRED; 860 throw new FHIRException("Unknown ConformanceResourceStatus code '" + codeString + "'"); 861 } 862 863 public String toCode() { 864 switch (this) { 865 case DRAFT: 866 return "draft"; 867 case ACTIVE: 868 return "active"; 869 case RETIRED: 870 return "retired"; 871 case NULL: 872 return null; 873 default: 874 return "?"; 875 } 876 } 877 878 public String getSystem() { 879 switch (this) { 880 case DRAFT: 881 return "http://hl7.org/fhir/conformance-resource-status"; 882 case ACTIVE: 883 return "http://hl7.org/fhir/conformance-resource-status"; 884 case RETIRED: 885 return "http://hl7.org/fhir/conformance-resource-status"; 886 case NULL: 887 return null; 888 default: 889 return "?"; 890 } 891 } 892 893 public String getDefinition() { 894 switch (this) { 895 case DRAFT: 896 return "This resource is still under development."; 897 case ACTIVE: 898 return "This resource is ready for normal use."; 899 case RETIRED: 900 return "This resource has been withdrawn or superseded and should no longer be used."; 901 case NULL: 902 return null; 903 default: 904 return "?"; 905 } 906 } 907 908 public String getDisplay() { 909 switch (this) { 910 case DRAFT: 911 return "Draft"; 912 case ACTIVE: 913 return "Active"; 914 case RETIRED: 915 return "Retired"; 916 case NULL: 917 return null; 918 default: 919 return "?"; 920 } 921 } 922 } 923 924 public static class ConformanceResourceStatusEnumFactory implements EnumFactory<ConformanceResourceStatus> { 925 public ConformanceResourceStatus fromCode(String codeString) throws IllegalArgumentException { 926 if (codeString == null || "".equals(codeString)) 927 if (codeString == null || "".equals(codeString)) 928 return null; 929 if ("draft".equals(codeString)) 930 return ConformanceResourceStatus.DRAFT; 931 if ("active".equals(codeString)) 932 return ConformanceResourceStatus.ACTIVE; 933 if ("retired".equals(codeString)) 934 return ConformanceResourceStatus.RETIRED; 935 throw new IllegalArgumentException("Unknown ConformanceResourceStatus code '" + codeString + "'"); 936 } 937 938 public Enumeration<ConformanceResourceStatus> fromType(Base code) throws FHIRException { 939 if (code == null || code.isEmpty()) 940 return null; 941 String codeString = ((PrimitiveType) code).asStringValue(); 942 if (codeString == null || "".equals(codeString)) 943 return null; 944 if ("draft".equals(codeString)) 945 return new Enumeration<ConformanceResourceStatus>(this, ConformanceResourceStatus.DRAFT); 946 if ("active".equals(codeString)) 947 return new Enumeration<ConformanceResourceStatus>(this, ConformanceResourceStatus.ACTIVE); 948 if ("retired".equals(codeString)) 949 return new Enumeration<ConformanceResourceStatus>(this, ConformanceResourceStatus.RETIRED); 950 throw new FHIRException("Unknown ConformanceResourceStatus code '" + codeString + "'"); 951 } 952 953 public String toCode(ConformanceResourceStatus code) 954 { 955 if (code == ConformanceResourceStatus.NULL) 956 return null; 957 if (code == ConformanceResourceStatus.DRAFT) 958 return "draft"; 959 if (code == ConformanceResourceStatus.ACTIVE) 960 return "active"; 961 if (code == ConformanceResourceStatus.RETIRED) 962 return "retired"; 963 return "?"; 964 } 965 } 966 967 public enum DataAbsentReason { 968 /** 969 * The value is not known. 970 */ 971 UNKNOWN, 972 /** 973 * The source human does not know the value. 974 */ 975 ASKED, 976 /** 977 * There is reason to expect (from the workflow) that the value may become 978 * known. 979 */ 980 TEMP, 981 /** 982 * The workflow didn't lead to this value being known. 983 */ 984 NOTASKED, 985 /** 986 * The information is not available due to security, privacy or related reasons. 987 */ 988 MASKED, 989 /** 990 * The source system wasn't capable of supporting this element. 991 */ 992 UNSUPPORTED, 993 /** 994 * The content of the data is represented in the resource narrative. 995 */ 996 ASTEXT, 997 /** 998 * Some system or workflow process error means that the information is not 999 * available. 1000 */ 1001 ERROR, 1002 /** 1003 * NaN, standing for not a number, is a numeric data type value representing an 1004 * undefined or unrepresentable value. 1005 */ 1006 NAN, 1007 /** 1008 * added to help the parsers 1009 */ 1010 NULL; 1011 1012 public static DataAbsentReason fromCode(String codeString) throws FHIRException { 1013 if (codeString == null || "".equals(codeString)) 1014 return null; 1015 if ("unknown".equals(codeString)) 1016 return UNKNOWN; 1017 if ("asked".equals(codeString)) 1018 return ASKED; 1019 if ("temp".equals(codeString)) 1020 return TEMP; 1021 if ("not-asked".equals(codeString)) 1022 return NOTASKED; 1023 if ("masked".equals(codeString)) 1024 return MASKED; 1025 if ("unsupported".equals(codeString)) 1026 return UNSUPPORTED; 1027 if ("astext".equals(codeString)) 1028 return ASTEXT; 1029 if ("error".equals(codeString)) 1030 return ERROR; 1031 if ("NaN".equals(codeString)) 1032 return NAN; 1033 throw new FHIRException("Unknown DataAbsentReason code '" + codeString + "'"); 1034 } 1035 1036 public String toCode() { 1037 switch (this) { 1038 case UNKNOWN: 1039 return "unknown"; 1040 case ASKED: 1041 return "asked"; 1042 case TEMP: 1043 return "temp"; 1044 case NOTASKED: 1045 return "not-asked"; 1046 case MASKED: 1047 return "masked"; 1048 case UNSUPPORTED: 1049 return "unsupported"; 1050 case ASTEXT: 1051 return "astext"; 1052 case ERROR: 1053 return "error"; 1054 case NAN: 1055 return "NaN"; 1056 case NULL: 1057 return null; 1058 default: 1059 return "?"; 1060 } 1061 } 1062 1063 public String getSystem() { 1064 switch (this) { 1065 case UNKNOWN: 1066 return "http://hl7.org/fhir/data-absent-reason"; 1067 case ASKED: 1068 return "http://hl7.org/fhir/data-absent-reason"; 1069 case TEMP: 1070 return "http://hl7.org/fhir/data-absent-reason"; 1071 case NOTASKED: 1072 return "http://hl7.org/fhir/data-absent-reason"; 1073 case MASKED: 1074 return "http://hl7.org/fhir/data-absent-reason"; 1075 case UNSUPPORTED: 1076 return "http://hl7.org/fhir/data-absent-reason"; 1077 case ASTEXT: 1078 return "http://hl7.org/fhir/data-absent-reason"; 1079 case ERROR: 1080 return "http://hl7.org/fhir/data-absent-reason"; 1081 case NAN: 1082 return "http://hl7.org/fhir/data-absent-reason"; 1083 case NULL: 1084 return null; 1085 default: 1086 return "?"; 1087 } 1088 } 1089 1090 public String getDefinition() { 1091 switch (this) { 1092 case UNKNOWN: 1093 return "The value is not known."; 1094 case ASKED: 1095 return "The source human does not know the value."; 1096 case TEMP: 1097 return "There is reason to expect (from the workflow) that the value may become known."; 1098 case NOTASKED: 1099 return "The workflow didn't lead to this value being known."; 1100 case MASKED: 1101 return "The information is not available due to security, privacy or related reasons."; 1102 case UNSUPPORTED: 1103 return "The source system wasn't capable of supporting this element."; 1104 case ASTEXT: 1105 return "The content of the data is represented in the resource narrative."; 1106 case ERROR: 1107 return "Some system or workflow process error means that the information is not available."; 1108 case NAN: 1109 return "NaN, standing for not a number, is a numeric data type value representing an undefined or unrepresentable value."; 1110 case NULL: 1111 return null; 1112 default: 1113 return "?"; 1114 } 1115 } 1116 1117 public String getDisplay() { 1118 switch (this) { 1119 case UNKNOWN: 1120 return "Unknown"; 1121 case ASKED: 1122 return "Asked"; 1123 case TEMP: 1124 return "Temp"; 1125 case NOTASKED: 1126 return "Not Asked"; 1127 case MASKED: 1128 return "Masked"; 1129 case UNSUPPORTED: 1130 return "Unsupported"; 1131 case ASTEXT: 1132 return "As Text"; 1133 case ERROR: 1134 return "Error"; 1135 case NAN: 1136 return "Not a Number"; 1137 case NULL: 1138 return null; 1139 default: 1140 return "?"; 1141 } 1142 } 1143 } 1144 1145 public static class DataAbsentReasonEnumFactory implements EnumFactory<DataAbsentReason> { 1146 public DataAbsentReason fromCode(String codeString) throws IllegalArgumentException { 1147 if (codeString == null || "".equals(codeString)) 1148 if (codeString == null || "".equals(codeString)) 1149 return null; 1150 if ("unknown".equals(codeString)) 1151 return DataAbsentReason.UNKNOWN; 1152 if ("asked".equals(codeString)) 1153 return DataAbsentReason.ASKED; 1154 if ("temp".equals(codeString)) 1155 return DataAbsentReason.TEMP; 1156 if ("not-asked".equals(codeString)) 1157 return DataAbsentReason.NOTASKED; 1158 if ("masked".equals(codeString)) 1159 return DataAbsentReason.MASKED; 1160 if ("unsupported".equals(codeString)) 1161 return DataAbsentReason.UNSUPPORTED; 1162 if ("astext".equals(codeString)) 1163 return DataAbsentReason.ASTEXT; 1164 if ("error".equals(codeString)) 1165 return DataAbsentReason.ERROR; 1166 if ("NaN".equals(codeString)) 1167 return DataAbsentReason.NAN; 1168 throw new IllegalArgumentException("Unknown DataAbsentReason code '" + codeString + "'"); 1169 } 1170 1171 public Enumeration<DataAbsentReason> fromType(Base code) throws FHIRException { 1172 if (code == null || code.isEmpty()) 1173 return null; 1174 String codeString = ((PrimitiveType) code).asStringValue(); 1175 if (codeString == null || "".equals(codeString)) 1176 return null; 1177 if ("unknown".equals(codeString)) 1178 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.UNKNOWN); 1179 if ("asked".equals(codeString)) 1180 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ASKED); 1181 if ("temp".equals(codeString)) 1182 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.TEMP); 1183 if ("not-asked".equals(codeString)) 1184 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NOTASKED); 1185 if ("masked".equals(codeString)) 1186 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.MASKED); 1187 if ("unsupported".equals(codeString)) 1188 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.UNSUPPORTED); 1189 if ("astext".equals(codeString)) 1190 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ASTEXT); 1191 if ("error".equals(codeString)) 1192 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ERROR); 1193 if ("NaN".equals(codeString)) 1194 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NAN); 1195 throw new FHIRException("Unknown DataAbsentReason code '" + codeString + "'"); 1196 } 1197 1198 public String toCode(DataAbsentReason code) 1199 { 1200 if (code == DataAbsentReason.NULL) 1201 return null; 1202 if (code == DataAbsentReason.UNKNOWN) 1203 return "unknown"; 1204 if (code == DataAbsentReason.ASKED) 1205 return "asked"; 1206 if (code == DataAbsentReason.TEMP) 1207 return "temp"; 1208 if (code == DataAbsentReason.NOTASKED) 1209 return "not-asked"; 1210 if (code == DataAbsentReason.MASKED) 1211 return "masked"; 1212 if (code == DataAbsentReason.UNSUPPORTED) 1213 return "unsupported"; 1214 if (code == DataAbsentReason.ASTEXT) 1215 return "astext"; 1216 if (code == DataAbsentReason.ERROR) 1217 return "error"; 1218 if (code == DataAbsentReason.NAN) 1219 return "NaN"; 1220 return "?"; 1221 } 1222 } 1223 1224 public enum DataType { 1225 /** 1226 * There is a variety of postal address formats defined around the world. This 1227 * format defines a superset that is the basis for all addresses around the 1228 * world. 1229 */ 1230 ADDRESS, 1231 /** 1232 * null 1233 */ 1234 AGE, 1235 /** 1236 * A text note which also contains information about who made the statement and 1237 * when. 1238 */ 1239 ANNOTATION, 1240 /** 1241 * For referring to data content defined in other formats. 1242 */ 1243 ATTACHMENT, 1244 /** 1245 * Base definition for all elements that are defined inside a resource - but not 1246 * those in a data type. 1247 */ 1248 BACKBONEELEMENT, 1249 /** 1250 * A concept that may be defined by a formal reference to a terminology or 1251 * ontology or may be provided by text. 1252 */ 1253 CODEABLECONCEPT, 1254 /** 1255 * A reference to a code defined by a terminology system. 1256 */ 1257 CODING, 1258 /** 1259 * Details for all kinds of technology mediated contact points for a person or 1260 * organization, including telephone, email, etc. 1261 */ 1262 CONTACTPOINT, 1263 /** 1264 * null 1265 */ 1266 COUNT, 1267 /** 1268 * null 1269 */ 1270 DISTANCE, 1271 /** 1272 * null 1273 */ 1274 DURATION, 1275 /** 1276 * Base definition for all elements in a resource. 1277 */ 1278 ELEMENT, 1279 /** 1280 * Captures constraints on each element within the resource, profile, or 1281 * extension. 1282 */ 1283 ELEMENTDEFINITION, 1284 /** 1285 * Optional Extensions Element - found in all resources. 1286 */ 1287 EXTENSION, 1288 /** 1289 * A human's name with the ability to identify parts and usage. 1290 */ 1291 HUMANNAME, 1292 /** 1293 * A technical identifier - identifies some entity uniquely and unambiguously. 1294 */ 1295 IDENTIFIER, 1296 /** 1297 * The metadata about a resource. This is content in the resource that is 1298 * maintained by the infrastructure. Changes to the content may not always be 1299 * associated with version changes to the resource. 1300 */ 1301 META, 1302 /** 1303 * null 1304 */ 1305 MONEY, 1306 /** 1307 * A human-readable formatted text, including images. 1308 */ 1309 NARRATIVE, 1310 /** 1311 * A time period defined by a start and end date and optionally time. 1312 */ 1313 PERIOD, 1314 /** 1315 * A measured amount (or an amount that can potentially be measured). Note that 1316 * measured amounts include amounts that are not precisely quantified, including 1317 * amounts involving arbitrary units and floating currencies. 1318 */ 1319 QUANTITY, 1320 /** 1321 * A set of ordered Quantities defined by a low and high limit. 1322 */ 1323 RANGE, 1324 /** 1325 * A relationship of two Quantity values - expressed as a numerator and a 1326 * denominator. 1327 */ 1328 RATIO, 1329 /** 1330 * A reference from one resource to another. 1331 */ 1332 REFERENCE, 1333 /** 1334 * A series of measurements taken by a device, with upper and lower limits. 1335 * There may be more than one dimension in the data. 1336 */ 1337 SAMPLEDDATA, 1338 /** 1339 * A digital signature along with supporting context. The signature may be 1340 * electronic/cryptographic in nature, or a graphical image representing a 1341 * hand-written signature, or a signature process. Different Signature 1342 * approaches have different utilities. 1343 */ 1344 SIGNATURE, 1345 /** 1346 * null 1347 */ 1348 SIMPLEQUANTITY, 1349 /** 1350 * Specifies an event that may occur multiple times. Timing schedules are used 1351 * to record when things are expected or requested to occur. The most common 1352 * usage is in dosage instructions for medications. They are also used when 1353 * planning care of various kinds. 1354 */ 1355 TIMING, 1356 /** 1357 * A stream of bytes 1358 */ 1359 BASE64BINARY, 1360 /** 1361 * Value of "true" or "false" 1362 */ 1363 BOOLEAN, 1364 /** 1365 * A string which has at least one character and no leading or trailing 1366 * whitespace and where there is no whitespace other than single spaces in the 1367 * contents 1368 */ 1369 CODE, 1370 /** 1371 * A date or partial date (e.g. just year or year + month). There is no time 1372 * zone. The format is a union of the schema types gYear, gYearMonth and date. 1373 * Dates SHALL be valid dates. 1374 */ 1375 DATE, 1376 /** 1377 * A date, date-time or partial date (e.g. just year or year + month). If hours 1378 * and minutes are specified, a time zone SHALL be populated. The format is a 1379 * union of the schema types gYear, gYearMonth, date and dateTime. Seconds must 1380 * be provided due to schema type constraints but may be zero-filled and may be 1381 * ignored. Dates SHALL be valid dates. 1382 */ 1383 DATETIME, 1384 /** 1385 * A rational number with implicit precision 1386 */ 1387 DECIMAL, 1388 /** 1389 * Any combination of letters, numerals, "-" and ".", with a length limit of 64 1390 * characters. (This might be an integer, an unprefixed OID, UUID or any other 1391 * identifier pattern that meets these constraints.) Ids are case-insensitive. 1392 */ 1393 ID, 1394 /** 1395 * An instant in time - known at least to the second 1396 */ 1397 INSTANT, 1398 /** 1399 * A whole number 1400 */ 1401 INTEGER, 1402 /** 1403 * A string that may contain markdown syntax for optional processing by a mark 1404 * down presentation engine 1405 */ 1406 MARKDOWN, 1407 /** 1408 * An oid represented as a URI 1409 */ 1410 OID, 1411 /** 1412 * An integer with a value that is positive (e.g. >0) 1413 */ 1414 POSITIVEINT, 1415 /** 1416 * A sequence of Unicode characters 1417 */ 1418 STRING, 1419 /** 1420 * A time during the day, with no date specified 1421 */ 1422 TIME, 1423 /** 1424 * An integer with a value that is not negative (e.g. >= 0) 1425 */ 1426 UNSIGNEDINT, 1427 /** 1428 * String of characters used to identify a name or a resource 1429 */ 1430 URI, 1431 /** 1432 * A UUID, represented as a URI 1433 */ 1434 UUID, 1435 /** 1436 * XHTML format, as defined by W3C, but restricted usage (mainly, no active 1437 * content) 1438 */ 1439 XHTML, 1440 /** 1441 * added to help the parsers 1442 */ 1443 NULL; 1444 1445 public static DataType fromCode(String codeString) throws FHIRException { 1446 if (codeString == null || "".equals(codeString)) 1447 return null; 1448 if ("Address".equals(codeString)) 1449 return ADDRESS; 1450 if ("Age".equals(codeString)) 1451 return AGE; 1452 if ("Annotation".equals(codeString)) 1453 return ANNOTATION; 1454 if ("Attachment".equals(codeString)) 1455 return ATTACHMENT; 1456 if ("BackboneElement".equals(codeString)) 1457 return BACKBONEELEMENT; 1458 if ("CodeableConcept".equals(codeString)) 1459 return CODEABLECONCEPT; 1460 if ("Coding".equals(codeString)) 1461 return CODING; 1462 if ("ContactPoint".equals(codeString)) 1463 return CONTACTPOINT; 1464 if ("Count".equals(codeString)) 1465 return COUNT; 1466 if ("Distance".equals(codeString)) 1467 return DISTANCE; 1468 if ("Duration".equals(codeString)) 1469 return DURATION; 1470 if ("Element".equals(codeString)) 1471 return ELEMENT; 1472 if ("ElementDefinition".equals(codeString)) 1473 return ELEMENTDEFINITION; 1474 if ("Extension".equals(codeString)) 1475 return EXTENSION; 1476 if ("HumanName".equals(codeString)) 1477 return HUMANNAME; 1478 if ("Identifier".equals(codeString)) 1479 return IDENTIFIER; 1480 if ("Meta".equals(codeString)) 1481 return META; 1482 if ("Money".equals(codeString)) 1483 return MONEY; 1484 if ("Narrative".equals(codeString)) 1485 return NARRATIVE; 1486 if ("Period".equals(codeString)) 1487 return PERIOD; 1488 if ("Quantity".equals(codeString)) 1489 return QUANTITY; 1490 if ("Range".equals(codeString)) 1491 return RANGE; 1492 if ("Ratio".equals(codeString)) 1493 return RATIO; 1494 if ("Reference".equals(codeString)) 1495 return REFERENCE; 1496 if ("SampledData".equals(codeString)) 1497 return SAMPLEDDATA; 1498 if ("Signature".equals(codeString)) 1499 return SIGNATURE; 1500 if ("SimpleQuantity".equals(codeString)) 1501 return SIMPLEQUANTITY; 1502 if ("Timing".equals(codeString)) 1503 return TIMING; 1504 if ("base64Binary".equals(codeString)) 1505 return BASE64BINARY; 1506 if ("boolean".equals(codeString)) 1507 return BOOLEAN; 1508 if ("code".equals(codeString)) 1509 return CODE; 1510 if ("date".equals(codeString)) 1511 return DATE; 1512 if ("dateTime".equals(codeString)) 1513 return DATETIME; 1514 if ("decimal".equals(codeString)) 1515 return DECIMAL; 1516 if ("id".equals(codeString)) 1517 return ID; 1518 if ("instant".equals(codeString)) 1519 return INSTANT; 1520 if ("integer".equals(codeString)) 1521 return INTEGER; 1522 if ("markdown".equals(codeString)) 1523 return MARKDOWN; 1524 if ("oid".equals(codeString)) 1525 return OID; 1526 if ("positiveInt".equals(codeString)) 1527 return POSITIVEINT; 1528 if ("string".equals(codeString)) 1529 return STRING; 1530 if ("time".equals(codeString)) 1531 return TIME; 1532 if ("unsignedInt".equals(codeString)) 1533 return UNSIGNEDINT; 1534 if ("uri".equals(codeString)) 1535 return URI; 1536 if ("uuid".equals(codeString)) 1537 return UUID; 1538 if ("xhtml".equals(codeString)) 1539 return XHTML; 1540 throw new FHIRException("Unknown DataType code '" + codeString + "'"); 1541 } 1542 1543 public String toCode() { 1544 switch (this) { 1545 case ADDRESS: 1546 return "Address"; 1547 case AGE: 1548 return "Age"; 1549 case ANNOTATION: 1550 return "Annotation"; 1551 case ATTACHMENT: 1552 return "Attachment"; 1553 case BACKBONEELEMENT: 1554 return "BackboneElement"; 1555 case CODEABLECONCEPT: 1556 return "CodeableConcept"; 1557 case CODING: 1558 return "Coding"; 1559 case CONTACTPOINT: 1560 return "ContactPoint"; 1561 case COUNT: 1562 return "Count"; 1563 case DISTANCE: 1564 return "Distance"; 1565 case DURATION: 1566 return "Duration"; 1567 case ELEMENT: 1568 return "Element"; 1569 case ELEMENTDEFINITION: 1570 return "ElementDefinition"; 1571 case EXTENSION: 1572 return "Extension"; 1573 case HUMANNAME: 1574 return "HumanName"; 1575 case IDENTIFIER: 1576 return "Identifier"; 1577 case META: 1578 return "Meta"; 1579 case MONEY: 1580 return "Money"; 1581 case NARRATIVE: 1582 return "Narrative"; 1583 case PERIOD: 1584 return "Period"; 1585 case QUANTITY: 1586 return "Quantity"; 1587 case RANGE: 1588 return "Range"; 1589 case RATIO: 1590 return "Ratio"; 1591 case REFERENCE: 1592 return "Reference"; 1593 case SAMPLEDDATA: 1594 return "SampledData"; 1595 case SIGNATURE: 1596 return "Signature"; 1597 case SIMPLEQUANTITY: 1598 return "SimpleQuantity"; 1599 case TIMING: 1600 return "Timing"; 1601 case BASE64BINARY: 1602 return "base64Binary"; 1603 case BOOLEAN: 1604 return "boolean"; 1605 case CODE: 1606 return "code"; 1607 case DATE: 1608 return "date"; 1609 case DATETIME: 1610 return "dateTime"; 1611 case DECIMAL: 1612 return "decimal"; 1613 case ID: 1614 return "id"; 1615 case INSTANT: 1616 return "instant"; 1617 case INTEGER: 1618 return "integer"; 1619 case MARKDOWN: 1620 return "markdown"; 1621 case OID: 1622 return "oid"; 1623 case POSITIVEINT: 1624 return "positiveInt"; 1625 case STRING: 1626 return "string"; 1627 case TIME: 1628 return "time"; 1629 case UNSIGNEDINT: 1630 return "unsignedInt"; 1631 case URI: 1632 return "uri"; 1633 case UUID: 1634 return "uuid"; 1635 case XHTML: 1636 return "xhtml"; 1637 case NULL: 1638 return null; 1639 default: 1640 return "?"; 1641 } 1642 } 1643 1644 public String getSystem() { 1645 switch (this) { 1646 case ADDRESS: 1647 return "http://hl7.org/fhir/data-types"; 1648 case AGE: 1649 return "http://hl7.org/fhir/data-types"; 1650 case ANNOTATION: 1651 return "http://hl7.org/fhir/data-types"; 1652 case ATTACHMENT: 1653 return "http://hl7.org/fhir/data-types"; 1654 case BACKBONEELEMENT: 1655 return "http://hl7.org/fhir/data-types"; 1656 case CODEABLECONCEPT: 1657 return "http://hl7.org/fhir/data-types"; 1658 case CODING: 1659 return "http://hl7.org/fhir/data-types"; 1660 case CONTACTPOINT: 1661 return "http://hl7.org/fhir/data-types"; 1662 case COUNT: 1663 return "http://hl7.org/fhir/data-types"; 1664 case DISTANCE: 1665 return "http://hl7.org/fhir/data-types"; 1666 case DURATION: 1667 return "http://hl7.org/fhir/data-types"; 1668 case ELEMENT: 1669 return "http://hl7.org/fhir/data-types"; 1670 case ELEMENTDEFINITION: 1671 return "http://hl7.org/fhir/data-types"; 1672 case EXTENSION: 1673 return "http://hl7.org/fhir/data-types"; 1674 case HUMANNAME: 1675 return "http://hl7.org/fhir/data-types"; 1676 case IDENTIFIER: 1677 return "http://hl7.org/fhir/data-types"; 1678 case META: 1679 return "http://hl7.org/fhir/data-types"; 1680 case MONEY: 1681 return "http://hl7.org/fhir/data-types"; 1682 case NARRATIVE: 1683 return "http://hl7.org/fhir/data-types"; 1684 case PERIOD: 1685 return "http://hl7.org/fhir/data-types"; 1686 case QUANTITY: 1687 return "http://hl7.org/fhir/data-types"; 1688 case RANGE: 1689 return "http://hl7.org/fhir/data-types"; 1690 case RATIO: 1691 return "http://hl7.org/fhir/data-types"; 1692 case REFERENCE: 1693 return "http://hl7.org/fhir/data-types"; 1694 case SAMPLEDDATA: 1695 return "http://hl7.org/fhir/data-types"; 1696 case SIGNATURE: 1697 return "http://hl7.org/fhir/data-types"; 1698 case SIMPLEQUANTITY: 1699 return "http://hl7.org/fhir/data-types"; 1700 case TIMING: 1701 return "http://hl7.org/fhir/data-types"; 1702 case BASE64BINARY: 1703 return "http://hl7.org/fhir/data-types"; 1704 case BOOLEAN: 1705 return "http://hl7.org/fhir/data-types"; 1706 case CODE: 1707 return "http://hl7.org/fhir/data-types"; 1708 case DATE: 1709 return "http://hl7.org/fhir/data-types"; 1710 case DATETIME: 1711 return "http://hl7.org/fhir/data-types"; 1712 case DECIMAL: 1713 return "http://hl7.org/fhir/data-types"; 1714 case ID: 1715 return "http://hl7.org/fhir/data-types"; 1716 case INSTANT: 1717 return "http://hl7.org/fhir/data-types"; 1718 case INTEGER: 1719 return "http://hl7.org/fhir/data-types"; 1720 case MARKDOWN: 1721 return "http://hl7.org/fhir/data-types"; 1722 case OID: 1723 return "http://hl7.org/fhir/data-types"; 1724 case POSITIVEINT: 1725 return "http://hl7.org/fhir/data-types"; 1726 case STRING: 1727 return "http://hl7.org/fhir/data-types"; 1728 case TIME: 1729 return "http://hl7.org/fhir/data-types"; 1730 case UNSIGNEDINT: 1731 return "http://hl7.org/fhir/data-types"; 1732 case URI: 1733 return "http://hl7.org/fhir/data-types"; 1734 case UUID: 1735 return "http://hl7.org/fhir/data-types"; 1736 case XHTML: 1737 return "http://hl7.org/fhir/data-types"; 1738 case NULL: 1739 return null; 1740 default: 1741 return "?"; 1742 } 1743 } 1744 1745 public String getDefinition() { 1746 switch (this) { 1747 case ADDRESS: 1748 return "There is a variety of postal address formats defined around the world. This format defines a superset that is the basis for all addresses around the world."; 1749 case AGE: 1750 return ""; 1751 case ANNOTATION: 1752 return "A text note which also contains information about who made the statement and when."; 1753 case ATTACHMENT: 1754 return "For referring to data content defined in other formats."; 1755 case BACKBONEELEMENT: 1756 return "Base definition for all elements that are defined inside a resource - but not those in a data type."; 1757 case CODEABLECONCEPT: 1758 return "A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text."; 1759 case CODING: 1760 return "A reference to a code defined by a terminology system."; 1761 case CONTACTPOINT: 1762 return "Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc."; 1763 case COUNT: 1764 return ""; 1765 case DISTANCE: 1766 return ""; 1767 case DURATION: 1768 return ""; 1769 case ELEMENT: 1770 return "Base definition for all elements in a resource."; 1771 case ELEMENTDEFINITION: 1772 return "Captures constraints on each element within the resource, profile, or extension."; 1773 case EXTENSION: 1774 return "Optional Extensions Element - found in all resources."; 1775 case HUMANNAME: 1776 return "A human's name with the ability to identify parts and usage."; 1777 case IDENTIFIER: 1778 return "A technical identifier - identifies some entity uniquely and unambiguously."; 1779 case META: 1780 return "The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource."; 1781 case MONEY: 1782 return ""; 1783 case NARRATIVE: 1784 return "A human-readable formatted text, including images."; 1785 case PERIOD: 1786 return "A time period defined by a start and end date and optionally time."; 1787 case QUANTITY: 1788 return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 1789 case RANGE: 1790 return "A set of ordered Quantities defined by a low and high limit."; 1791 case RATIO: 1792 return "A relationship of two Quantity values - expressed as a numerator and a denominator."; 1793 case REFERENCE: 1794 return "A reference from one resource to another."; 1795 case SAMPLEDDATA: 1796 return "A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data."; 1797 case SIGNATURE: 1798 return "A digital signature along with supporting context. The signature may be electronic/cryptographic in nature, or a graphical image representing a hand-written signature, or a signature process. Different Signature approaches have different utilities."; 1799 case SIMPLEQUANTITY: 1800 return ""; 1801 case TIMING: 1802 return "Specifies an event that may occur multiple times. Timing schedules are used to record when things are expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds."; 1803 case BASE64BINARY: 1804 return "A stream of bytes"; 1805 case BOOLEAN: 1806 return "Value of \"true\" or \"false\""; 1807 case CODE: 1808 return "A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents"; 1809 case DATE: 1810 return "A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates."; 1811 case DATETIME: 1812 return "A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates."; 1813 case DECIMAL: 1814 return "A rational number with implicit precision"; 1815 case ID: 1816 return "Any combination of letters, numerals, \"-\" and \".\", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive."; 1817 case INSTANT: 1818 return "An instant in time - known at least to the second"; 1819 case INTEGER: 1820 return "A whole number"; 1821 case MARKDOWN: 1822 return "A string that may contain markdown syntax for optional processing by a mark down presentation engine"; 1823 case OID: 1824 return "An oid represented as a URI"; 1825 case POSITIVEINT: 1826 return "An integer with a value that is positive (e.g. >0)"; 1827 case STRING: 1828 return "A sequence of Unicode characters"; 1829 case TIME: 1830 return "A time during the day, with no date specified"; 1831 case UNSIGNEDINT: 1832 return "An integer with a value that is not negative (e.g. >= 0)"; 1833 case URI: 1834 return "String of characters used to identify a name or a resource"; 1835 case UUID: 1836 return "A UUID, represented as a URI"; 1837 case XHTML: 1838 return "XHTML format, as defined by W3C, but restricted usage (mainly, no active content)"; 1839 case NULL: 1840 return null; 1841 default: 1842 return "?"; 1843 } 1844 } 1845 1846 public String getDisplay() { 1847 switch (this) { 1848 case ADDRESS: 1849 return "Address"; 1850 case AGE: 1851 return "Age"; 1852 case ANNOTATION: 1853 return "Annotation"; 1854 case ATTACHMENT: 1855 return "Attachment"; 1856 case BACKBONEELEMENT: 1857 return "BackboneElement"; 1858 case CODEABLECONCEPT: 1859 return "CodeableConcept"; 1860 case CODING: 1861 return "Coding"; 1862 case CONTACTPOINT: 1863 return "ContactPoint"; 1864 case COUNT: 1865 return "Count"; 1866 case DISTANCE: 1867 return "Distance"; 1868 case DURATION: 1869 return "Duration"; 1870 case ELEMENT: 1871 return "Element"; 1872 case ELEMENTDEFINITION: 1873 return "ElementDefinition"; 1874 case EXTENSION: 1875 return "Extension"; 1876 case HUMANNAME: 1877 return "HumanName"; 1878 case IDENTIFIER: 1879 return "Identifier"; 1880 case META: 1881 return "Meta"; 1882 case MONEY: 1883 return "Money"; 1884 case NARRATIVE: 1885 return "Narrative"; 1886 case PERIOD: 1887 return "Period"; 1888 case QUANTITY: 1889 return "Quantity"; 1890 case RANGE: 1891 return "Range"; 1892 case RATIO: 1893 return "Ratio"; 1894 case REFERENCE: 1895 return "Reference"; 1896 case SAMPLEDDATA: 1897 return "SampledData"; 1898 case SIGNATURE: 1899 return "Signature"; 1900 case SIMPLEQUANTITY: 1901 return "SimpleQuantity"; 1902 case TIMING: 1903 return "Timing"; 1904 case BASE64BINARY: 1905 return "base64Binary"; 1906 case BOOLEAN: 1907 return "boolean"; 1908 case CODE: 1909 return "code"; 1910 case DATE: 1911 return "date"; 1912 case DATETIME: 1913 return "dateTime"; 1914 case DECIMAL: 1915 return "decimal"; 1916 case ID: 1917 return "id"; 1918 case INSTANT: 1919 return "instant"; 1920 case INTEGER: 1921 return "integer"; 1922 case MARKDOWN: 1923 return "markdown"; 1924 case OID: 1925 return "oid"; 1926 case POSITIVEINT: 1927 return "positiveInt"; 1928 case STRING: 1929 return "string"; 1930 case TIME: 1931 return "time"; 1932 case UNSIGNEDINT: 1933 return "unsignedInt"; 1934 case URI: 1935 return "uri"; 1936 case UUID: 1937 return "uuid"; 1938 case XHTML: 1939 return "XHTML"; 1940 case NULL: 1941 return null; 1942 default: 1943 return "?"; 1944 } 1945 } 1946 } 1947 1948 public static class DataTypeEnumFactory implements EnumFactory<DataType> { 1949 public DataType fromCode(String codeString) throws IllegalArgumentException { 1950 if (codeString == null || "".equals(codeString)) 1951 if (codeString == null || "".equals(codeString)) 1952 return null; 1953 if ("Address".equals(codeString)) 1954 return DataType.ADDRESS; 1955 if ("Age".equals(codeString)) 1956 return DataType.AGE; 1957 if ("Annotation".equals(codeString)) 1958 return DataType.ANNOTATION; 1959 if ("Attachment".equals(codeString)) 1960 return DataType.ATTACHMENT; 1961 if ("BackboneElement".equals(codeString)) 1962 return DataType.BACKBONEELEMENT; 1963 if ("CodeableConcept".equals(codeString)) 1964 return DataType.CODEABLECONCEPT; 1965 if ("Coding".equals(codeString)) 1966 return DataType.CODING; 1967 if ("ContactPoint".equals(codeString)) 1968 return DataType.CONTACTPOINT; 1969 if ("Count".equals(codeString)) 1970 return DataType.COUNT; 1971 if ("Distance".equals(codeString)) 1972 return DataType.DISTANCE; 1973 if ("Duration".equals(codeString)) 1974 return DataType.DURATION; 1975 if ("Element".equals(codeString)) 1976 return DataType.ELEMENT; 1977 if ("ElementDefinition".equals(codeString)) 1978 return DataType.ELEMENTDEFINITION; 1979 if ("Extension".equals(codeString)) 1980 return DataType.EXTENSION; 1981 if ("HumanName".equals(codeString)) 1982 return DataType.HUMANNAME; 1983 if ("Identifier".equals(codeString)) 1984 return DataType.IDENTIFIER; 1985 if ("Meta".equals(codeString)) 1986 return DataType.META; 1987 if ("Money".equals(codeString)) 1988 return DataType.MONEY; 1989 if ("Narrative".equals(codeString)) 1990 return DataType.NARRATIVE; 1991 if ("Period".equals(codeString)) 1992 return DataType.PERIOD; 1993 if ("Quantity".equals(codeString)) 1994 return DataType.QUANTITY; 1995 if ("Range".equals(codeString)) 1996 return DataType.RANGE; 1997 if ("Ratio".equals(codeString)) 1998 return DataType.RATIO; 1999 if ("Reference".equals(codeString)) 2000 return DataType.REFERENCE; 2001 if ("SampledData".equals(codeString)) 2002 return DataType.SAMPLEDDATA; 2003 if ("Signature".equals(codeString)) 2004 return DataType.SIGNATURE; 2005 if ("SimpleQuantity".equals(codeString)) 2006 return DataType.SIMPLEQUANTITY; 2007 if ("Timing".equals(codeString)) 2008 return DataType.TIMING; 2009 if ("base64Binary".equals(codeString)) 2010 return DataType.BASE64BINARY; 2011 if ("boolean".equals(codeString)) 2012 return DataType.BOOLEAN; 2013 if ("code".equals(codeString)) 2014 return DataType.CODE; 2015 if ("date".equals(codeString)) 2016 return DataType.DATE; 2017 if ("dateTime".equals(codeString)) 2018 return DataType.DATETIME; 2019 if ("decimal".equals(codeString)) 2020 return DataType.DECIMAL; 2021 if ("id".equals(codeString)) 2022 return DataType.ID; 2023 if ("instant".equals(codeString)) 2024 return DataType.INSTANT; 2025 if ("integer".equals(codeString)) 2026 return DataType.INTEGER; 2027 if ("markdown".equals(codeString)) 2028 return DataType.MARKDOWN; 2029 if ("oid".equals(codeString)) 2030 return DataType.OID; 2031 if ("positiveInt".equals(codeString)) 2032 return DataType.POSITIVEINT; 2033 if ("string".equals(codeString)) 2034 return DataType.STRING; 2035 if ("time".equals(codeString)) 2036 return DataType.TIME; 2037 if ("unsignedInt".equals(codeString)) 2038 return DataType.UNSIGNEDINT; 2039 if ("uri".equals(codeString)) 2040 return DataType.URI; 2041 if ("uuid".equals(codeString)) 2042 return DataType.UUID; 2043 if ("xhtml".equals(codeString)) 2044 return DataType.XHTML; 2045 throw new IllegalArgumentException("Unknown DataType code '" + codeString + "'"); 2046 } 2047 2048 public Enumeration<DataType> fromType(Base code) throws FHIRException { 2049 if (code == null || code.isEmpty()) 2050 return null; 2051 String codeString = ((PrimitiveType) code).asStringValue(); 2052 if (codeString == null || "".equals(codeString)) 2053 return null; 2054 if ("Address".equals(codeString)) 2055 return new Enumeration<DataType>(this, DataType.ADDRESS); 2056 if ("Age".equals(codeString)) 2057 return new Enumeration<DataType>(this, DataType.AGE); 2058 if ("Annotation".equals(codeString)) 2059 return new Enumeration<DataType>(this, DataType.ANNOTATION); 2060 if ("Attachment".equals(codeString)) 2061 return new Enumeration<DataType>(this, DataType.ATTACHMENT); 2062 if ("BackboneElement".equals(codeString)) 2063 return new Enumeration<DataType>(this, DataType.BACKBONEELEMENT); 2064 if ("CodeableConcept".equals(codeString)) 2065 return new Enumeration<DataType>(this, DataType.CODEABLECONCEPT); 2066 if ("Coding".equals(codeString)) 2067 return new Enumeration<DataType>(this, DataType.CODING); 2068 if ("ContactPoint".equals(codeString)) 2069 return new Enumeration<DataType>(this, DataType.CONTACTPOINT); 2070 if ("Count".equals(codeString)) 2071 return new Enumeration<DataType>(this, DataType.COUNT); 2072 if ("Distance".equals(codeString)) 2073 return new Enumeration<DataType>(this, DataType.DISTANCE); 2074 if ("Duration".equals(codeString)) 2075 return new Enumeration<DataType>(this, DataType.DURATION); 2076 if ("Element".equals(codeString)) 2077 return new Enumeration<DataType>(this, DataType.ELEMENT); 2078 if ("ElementDefinition".equals(codeString)) 2079 return new Enumeration<DataType>(this, DataType.ELEMENTDEFINITION); 2080 if ("Extension".equals(codeString)) 2081 return new Enumeration<DataType>(this, DataType.EXTENSION); 2082 if ("HumanName".equals(codeString)) 2083 return new Enumeration<DataType>(this, DataType.HUMANNAME); 2084 if ("Identifier".equals(codeString)) 2085 return new Enumeration<DataType>(this, DataType.IDENTIFIER); 2086 if ("Meta".equals(codeString)) 2087 return new Enumeration<DataType>(this, DataType.META); 2088 if ("Money".equals(codeString)) 2089 return new Enumeration<DataType>(this, DataType.MONEY); 2090 if ("Narrative".equals(codeString)) 2091 return new Enumeration<DataType>(this, DataType.NARRATIVE); 2092 if ("Period".equals(codeString)) 2093 return new Enumeration<DataType>(this, DataType.PERIOD); 2094 if ("Quantity".equals(codeString)) 2095 return new Enumeration<DataType>(this, DataType.QUANTITY); 2096 if ("Range".equals(codeString)) 2097 return new Enumeration<DataType>(this, DataType.RANGE); 2098 if ("Ratio".equals(codeString)) 2099 return new Enumeration<DataType>(this, DataType.RATIO); 2100 if ("Reference".equals(codeString)) 2101 return new Enumeration<DataType>(this, DataType.REFERENCE); 2102 if ("SampledData".equals(codeString)) 2103 return new Enumeration<DataType>(this, DataType.SAMPLEDDATA); 2104 if ("Signature".equals(codeString)) 2105 return new Enumeration<DataType>(this, DataType.SIGNATURE); 2106 if ("SimpleQuantity".equals(codeString)) 2107 return new Enumeration<DataType>(this, DataType.SIMPLEQUANTITY); 2108 if ("Timing".equals(codeString)) 2109 return new Enumeration<DataType>(this, DataType.TIMING); 2110 if ("base64Binary".equals(codeString)) 2111 return new Enumeration<DataType>(this, DataType.BASE64BINARY); 2112 if ("boolean".equals(codeString)) 2113 return new Enumeration<DataType>(this, DataType.BOOLEAN); 2114 if ("code".equals(codeString)) 2115 return new Enumeration<DataType>(this, DataType.CODE); 2116 if ("date".equals(codeString)) 2117 return new Enumeration<DataType>(this, DataType.DATE); 2118 if ("dateTime".equals(codeString)) 2119 return new Enumeration<DataType>(this, DataType.DATETIME); 2120 if ("decimal".equals(codeString)) 2121 return new Enumeration<DataType>(this, DataType.DECIMAL); 2122 if ("id".equals(codeString)) 2123 return new Enumeration<DataType>(this, DataType.ID); 2124 if ("instant".equals(codeString)) 2125 return new Enumeration<DataType>(this, DataType.INSTANT); 2126 if ("integer".equals(codeString)) 2127 return new Enumeration<DataType>(this, DataType.INTEGER); 2128 if ("markdown".equals(codeString)) 2129 return new Enumeration<DataType>(this, DataType.MARKDOWN); 2130 if ("oid".equals(codeString)) 2131 return new Enumeration<DataType>(this, DataType.OID); 2132 if ("positiveInt".equals(codeString)) 2133 return new Enumeration<DataType>(this, DataType.POSITIVEINT); 2134 if ("string".equals(codeString)) 2135 return new Enumeration<DataType>(this, DataType.STRING); 2136 if ("time".equals(codeString)) 2137 return new Enumeration<DataType>(this, DataType.TIME); 2138 if ("unsignedInt".equals(codeString)) 2139 return new Enumeration<DataType>(this, DataType.UNSIGNEDINT); 2140 if ("uri".equals(codeString)) 2141 return new Enumeration<DataType>(this, DataType.URI); 2142 if ("uuid".equals(codeString)) 2143 return new Enumeration<DataType>(this, DataType.UUID); 2144 if ("xhtml".equals(codeString)) 2145 return new Enumeration<DataType>(this, DataType.XHTML); 2146 throw new FHIRException("Unknown DataType code '" + codeString + "'"); 2147 } 2148 2149 public String toCode(DataType code) 2150 { 2151 if (code == DataType.NULL) 2152 return null; 2153 if (code == DataType.ADDRESS) 2154 return "Address"; 2155 if (code == DataType.AGE) 2156 return "Age"; 2157 if (code == DataType.ANNOTATION) 2158 return "Annotation"; 2159 if (code == DataType.ATTACHMENT) 2160 return "Attachment"; 2161 if (code == DataType.BACKBONEELEMENT) 2162 return "BackboneElement"; 2163 if (code == DataType.CODEABLECONCEPT) 2164 return "CodeableConcept"; 2165 if (code == DataType.CODING) 2166 return "Coding"; 2167 if (code == DataType.CONTACTPOINT) 2168 return "ContactPoint"; 2169 if (code == DataType.COUNT) 2170 return "Count"; 2171 if (code == DataType.DISTANCE) 2172 return "Distance"; 2173 if (code == DataType.DURATION) 2174 return "Duration"; 2175 if (code == DataType.ELEMENT) 2176 return "Element"; 2177 if (code == DataType.ELEMENTDEFINITION) 2178 return "ElementDefinition"; 2179 if (code == DataType.EXTENSION) 2180 return "Extension"; 2181 if (code == DataType.HUMANNAME) 2182 return "HumanName"; 2183 if (code == DataType.IDENTIFIER) 2184 return "Identifier"; 2185 if (code == DataType.META) 2186 return "Meta"; 2187 if (code == DataType.MONEY) 2188 return "Money"; 2189 if (code == DataType.NARRATIVE) 2190 return "Narrative"; 2191 if (code == DataType.PERIOD) 2192 return "Period"; 2193 if (code == DataType.QUANTITY) 2194 return "Quantity"; 2195 if (code == DataType.RANGE) 2196 return "Range"; 2197 if (code == DataType.RATIO) 2198 return "Ratio"; 2199 if (code == DataType.REFERENCE) 2200 return "Reference"; 2201 if (code == DataType.SAMPLEDDATA) 2202 return "SampledData"; 2203 if (code == DataType.SIGNATURE) 2204 return "Signature"; 2205 if (code == DataType.SIMPLEQUANTITY) 2206 return "SimpleQuantity"; 2207 if (code == DataType.TIMING) 2208 return "Timing"; 2209 if (code == DataType.BASE64BINARY) 2210 return "base64Binary"; 2211 if (code == DataType.BOOLEAN) 2212 return "boolean"; 2213 if (code == DataType.CODE) 2214 return "code"; 2215 if (code == DataType.DATE) 2216 return "date"; 2217 if (code == DataType.DATETIME) 2218 return "dateTime"; 2219 if (code == DataType.DECIMAL) 2220 return "decimal"; 2221 if (code == DataType.ID) 2222 return "id"; 2223 if (code == DataType.INSTANT) 2224 return "instant"; 2225 if (code == DataType.INTEGER) 2226 return "integer"; 2227 if (code == DataType.MARKDOWN) 2228 return "markdown"; 2229 if (code == DataType.OID) 2230 return "oid"; 2231 if (code == DataType.POSITIVEINT) 2232 return "positiveInt"; 2233 if (code == DataType.STRING) 2234 return "string"; 2235 if (code == DataType.TIME) 2236 return "time"; 2237 if (code == DataType.UNSIGNEDINT) 2238 return "unsignedInt"; 2239 if (code == DataType.URI) 2240 return "uri"; 2241 if (code == DataType.UUID) 2242 return "uuid"; 2243 if (code == DataType.XHTML) 2244 return "xhtml"; 2245 return "?"; 2246 } 2247 } 2248 2249 public enum DocumentReferenceStatus { 2250 /** 2251 * This is the current reference for this document. 2252 */ 2253 CURRENT, 2254 /** 2255 * This reference has been superseded by another reference. 2256 */ 2257 SUPERSEDED, 2258 /** 2259 * This reference was created in error. 2260 */ 2261 ENTEREDINERROR, 2262 /** 2263 * added to help the parsers 2264 */ 2265 NULL; 2266 2267 public static DocumentReferenceStatus fromCode(String codeString) throws FHIRException { 2268 if (codeString == null || "".equals(codeString)) 2269 return null; 2270 if ("current".equals(codeString)) 2271 return CURRENT; 2272 if ("superseded".equals(codeString)) 2273 return SUPERSEDED; 2274 if ("entered-in-error".equals(codeString)) 2275 return ENTEREDINERROR; 2276 throw new FHIRException("Unknown DocumentReferenceStatus code '" + codeString + "'"); 2277 } 2278 2279 public String toCode() { 2280 switch (this) { 2281 case CURRENT: 2282 return "current"; 2283 case SUPERSEDED: 2284 return "superseded"; 2285 case ENTEREDINERROR: 2286 return "entered-in-error"; 2287 case NULL: 2288 return null; 2289 default: 2290 return "?"; 2291 } 2292 } 2293 2294 public String getSystem() { 2295 switch (this) { 2296 case CURRENT: 2297 return "http://hl7.org/fhir/document-reference-status"; 2298 case SUPERSEDED: 2299 return "http://hl7.org/fhir/document-reference-status"; 2300 case ENTEREDINERROR: 2301 return "http://hl7.org/fhir/document-reference-status"; 2302 case NULL: 2303 return null; 2304 default: 2305 return "?"; 2306 } 2307 } 2308 2309 public String getDefinition() { 2310 switch (this) { 2311 case CURRENT: 2312 return "This is the current reference for this document."; 2313 case SUPERSEDED: 2314 return "This reference has been superseded by another reference."; 2315 case ENTEREDINERROR: 2316 return "This reference was created in error."; 2317 case NULL: 2318 return null; 2319 default: 2320 return "?"; 2321 } 2322 } 2323 2324 public String getDisplay() { 2325 switch (this) { 2326 case CURRENT: 2327 return "Current"; 2328 case SUPERSEDED: 2329 return "Superseded"; 2330 case ENTEREDINERROR: 2331 return "Entered in Error"; 2332 case NULL: 2333 return null; 2334 default: 2335 return "?"; 2336 } 2337 } 2338 } 2339 2340 public static class DocumentReferenceStatusEnumFactory implements EnumFactory<DocumentReferenceStatus> { 2341 public DocumentReferenceStatus fromCode(String codeString) throws IllegalArgumentException { 2342 if (codeString == null || "".equals(codeString)) 2343 if (codeString == null || "".equals(codeString)) 2344 return null; 2345 if ("current".equals(codeString)) 2346 return DocumentReferenceStatus.CURRENT; 2347 if ("superseded".equals(codeString)) 2348 return DocumentReferenceStatus.SUPERSEDED; 2349 if ("entered-in-error".equals(codeString)) 2350 return DocumentReferenceStatus.ENTEREDINERROR; 2351 throw new IllegalArgumentException("Unknown DocumentReferenceStatus code '" + codeString + "'"); 2352 } 2353 2354 public Enumeration<DocumentReferenceStatus> fromType(Base code) throws FHIRException { 2355 if (code == null || code.isEmpty()) 2356 return null; 2357 String codeString = ((PrimitiveType) code).asStringValue(); 2358 if (codeString == null || "".equals(codeString)) 2359 return null; 2360 if ("current".equals(codeString)) 2361 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.CURRENT); 2362 if ("superseded".equals(codeString)) 2363 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.SUPERSEDED); 2364 if ("entered-in-error".equals(codeString)) 2365 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.ENTEREDINERROR); 2366 throw new FHIRException("Unknown DocumentReferenceStatus code '" + codeString + "'"); 2367 } 2368 2369 public String toCode(DocumentReferenceStatus code) 2370 { 2371 if (code == DocumentReferenceStatus.NULL) 2372 return null; 2373 if (code == DocumentReferenceStatus.CURRENT) 2374 return "current"; 2375 if (code == DocumentReferenceStatus.SUPERSEDED) 2376 return "superseded"; 2377 if (code == DocumentReferenceStatus.ENTEREDINERROR) 2378 return "entered-in-error"; 2379 return "?"; 2380 } 2381 } 2382 2383 public enum FHIRDefinedType { 2384 /** 2385 * There is a variety of postal address formats defined around the world. This 2386 * format defines a superset that is the basis for all addresses around the 2387 * world. 2388 */ 2389 ADDRESS, 2390 /** 2391 * null 2392 */ 2393 AGE, 2394 /** 2395 * A text note which also contains information about who made the statement and 2396 * when. 2397 */ 2398 ANNOTATION, 2399 /** 2400 * For referring to data content defined in other formats. 2401 */ 2402 ATTACHMENT, 2403 /** 2404 * Base definition for all elements that are defined inside a resource - but not 2405 * those in a data type. 2406 */ 2407 BACKBONEELEMENT, 2408 /** 2409 * A concept that may be defined by a formal reference to a terminology or 2410 * ontology or may be provided by text. 2411 */ 2412 CODEABLECONCEPT, 2413 /** 2414 * A reference to a code defined by a terminology system. 2415 */ 2416 CODING, 2417 /** 2418 * Details for all kinds of technology mediated contact points for a person or 2419 * organization, including telephone, email, etc. 2420 */ 2421 CONTACTPOINT, 2422 /** 2423 * null 2424 */ 2425 COUNT, 2426 /** 2427 * null 2428 */ 2429 DISTANCE, 2430 /** 2431 * null 2432 */ 2433 DURATION, 2434 /** 2435 * Base definition for all elements in a resource. 2436 */ 2437 ELEMENT, 2438 /** 2439 * Captures constraints on each element within the resource, profile, or 2440 * extension. 2441 */ 2442 ELEMENTDEFINITION, 2443 /** 2444 * Optional Extensions Element - found in all resources. 2445 */ 2446 EXTENSION, 2447 /** 2448 * A human's name with the ability to identify parts and usage. 2449 */ 2450 HUMANNAME, 2451 /** 2452 * A technical identifier - identifies some entity uniquely and unambiguously. 2453 */ 2454 IDENTIFIER, 2455 /** 2456 * The metadata about a resource. This is content in the resource that is 2457 * maintained by the infrastructure. Changes to the content may not always be 2458 * associated with version changes to the resource. 2459 */ 2460 META, 2461 /** 2462 * null 2463 */ 2464 MONEY, 2465 /** 2466 * A human-readable formatted text, including images. 2467 */ 2468 NARRATIVE, 2469 /** 2470 * A time period defined by a start and end date and optionally time. 2471 */ 2472 PERIOD, 2473 /** 2474 * A measured amount (or an amount that can potentially be measured). Note that 2475 * measured amounts include amounts that are not precisely quantified, including 2476 * amounts involving arbitrary units and floating currencies. 2477 */ 2478 QUANTITY, 2479 /** 2480 * A set of ordered Quantities defined by a low and high limit. 2481 */ 2482 RANGE, 2483 /** 2484 * A relationship of two Quantity values - expressed as a numerator and a 2485 * denominator. 2486 */ 2487 RATIO, 2488 /** 2489 * A reference from one resource to another. 2490 */ 2491 REFERENCE, 2492 /** 2493 * A series of measurements taken by a device, with upper and lower limits. 2494 * There may be more than one dimension in the data. 2495 */ 2496 SAMPLEDDATA, 2497 /** 2498 * A digital signature along with supporting context. The signature may be 2499 * electronic/cryptographic in nature, or a graphical image representing a 2500 * hand-written signature, or a signature process. Different Signature 2501 * approaches have different utilities. 2502 */ 2503 SIGNATURE, 2504 /** 2505 * null 2506 */ 2507 SIMPLEQUANTITY, 2508 /** 2509 * Specifies an event that may occur multiple times. Timing schedules are used 2510 * to record when things are expected or requested to occur. The most common 2511 * usage is in dosage instructions for medications. They are also used when 2512 * planning care of various kinds. 2513 */ 2514 TIMING, 2515 /** 2516 * A stream of bytes 2517 */ 2518 BASE64BINARY, 2519 /** 2520 * Value of "true" or "false" 2521 */ 2522 BOOLEAN, 2523 /** 2524 * A string which has at least one character and no leading or trailing 2525 * whitespace and where there is no whitespace other than single spaces in the 2526 * contents 2527 */ 2528 CODE, 2529 /** 2530 * A date or partial date (e.g. just year or year + month). There is no time 2531 * zone. The format is a union of the schema types gYear, gYearMonth and date. 2532 * Dates SHALL be valid dates. 2533 */ 2534 DATE, 2535 /** 2536 * A date, date-time or partial date (e.g. just year or year + month). If hours 2537 * and minutes are specified, a time zone SHALL be populated. The format is a 2538 * union of the schema types gYear, gYearMonth, date and dateTime. Seconds must 2539 * be provided due to schema type constraints but may be zero-filled and may be 2540 * ignored. Dates SHALL be valid dates. 2541 */ 2542 DATETIME, 2543 /** 2544 * A rational number with implicit precision 2545 */ 2546 DECIMAL, 2547 /** 2548 * Any combination of letters, numerals, "-" and ".", with a length limit of 64 2549 * characters. (This might be an integer, an unprefixed OID, UUID or any other 2550 * identifier pattern that meets these constraints.) Ids are case-insensitive. 2551 */ 2552 ID, 2553 /** 2554 * An instant in time - known at least to the second 2555 */ 2556 INSTANT, 2557 /** 2558 * A whole number 2559 */ 2560 INTEGER, 2561 /** 2562 * A string that may contain markdown syntax for optional processing by a mark 2563 * down presentation engine 2564 */ 2565 MARKDOWN, 2566 /** 2567 * An oid represented as a URI 2568 */ 2569 OID, 2570 /** 2571 * An integer with a value that is positive (e.g. >0) 2572 */ 2573 POSITIVEINT, 2574 /** 2575 * A sequence of Unicode characters 2576 */ 2577 STRING, 2578 /** 2579 * A time during the day, with no date specified 2580 */ 2581 TIME, 2582 /** 2583 * An integer with a value that is not negative (e.g. >= 0) 2584 */ 2585 UNSIGNEDINT, 2586 /** 2587 * String of characters used to identify a name or a resource 2588 */ 2589 URI, 2590 /** 2591 * A UUID, represented as a URI 2592 */ 2593 UUID, 2594 /** 2595 * XHTML format, as defined by W3C, but restricted usage (mainly, no active 2596 * content) 2597 */ 2598 XHTML, 2599 /** 2600 * A financial tool for tracking value accrued for a particular purpose. In the 2601 * healthcare field, used to track charges for a patient, cost centres, etc. 2602 */ 2603 ACCOUNT, 2604 /** 2605 * Risk of harmful or undesirable, physiological response which is unique to an 2606 * individual and associated with exposure to a substance. 2607 */ 2608 ALLERGYINTOLERANCE, 2609 /** 2610 * A booking of a healthcare event among patient(s), practitioner(s), related 2611 * person(s) and/or device(s) for a specific date/time. This may result in one 2612 * or more Encounter(s). 2613 */ 2614 APPOINTMENT, 2615 /** 2616 * A reply to an appointment request for a patient and/or practitioner(s), such 2617 * as a confirmation or rejection. 2618 */ 2619 APPOINTMENTRESPONSE, 2620 /** 2621 * A record of an event made for purposes of maintaining a security log. Typical 2622 * uses include detection of intrusion attempts and monitoring for inappropriate 2623 * usage. 2624 */ 2625 AUDITEVENT, 2626 /** 2627 * Basic is used for handling concepts not yet defined in FHIR, narrative-only 2628 * resources that don't map to an existing resource, and custom resources not 2629 * appropriate for inclusion in the FHIR specification. 2630 */ 2631 BASIC, 2632 /** 2633 * A binary resource can contain any content, whether text, image, pdf, zip 2634 * archive, etc. 2635 */ 2636 BINARY, 2637 /** 2638 * Record details about the anatomical location of a specimen or body part. This 2639 * resource may be used when a coded concept does not provide the necessary 2640 * detail needed for the use case. 2641 */ 2642 BODYSITE, 2643 /** 2644 * A container for a collection of resources. 2645 */ 2646 BUNDLE, 2647 /** 2648 * Describes the intention of how one or more practitioners intend to deliver 2649 * care for a particular patient, group or community for a period of time, 2650 * possibly limited to care for a specific condition or set of conditions. 2651 */ 2652 CAREPLAN, 2653 /** 2654 * A provider issued list of services and products provided, or to be provided, 2655 * to a patient which is provided to an insurer for payment recovery. 2656 */ 2657 CLAIM, 2658 /** 2659 * This resource provides the adjudication details from the processing of a 2660 * Claim resource. 2661 */ 2662 CLAIMRESPONSE, 2663 /** 2664 * A record of a clinical assessment performed to determine what problem(s) may 2665 * affect the patient and before planning the treatments or management 2666 * strategies that are best to manage a patient's condition. Assessments are 2667 * often 1:1 with a clinical consultation / encounter, but this varies greatly 2668 * depending on the clinical workflow. This resource is called 2669 * "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with 2670 * the recording of assessment tools such as Apgar score. 2671 */ 2672 CLINICALIMPRESSION, 2673 /** 2674 * An occurrence of information being transmitted; e.g. an alert that was sent 2675 * to a responsible provider, a public health agency was notified about a 2676 * reportable condition. 2677 */ 2678 COMMUNICATION, 2679 /** 2680 * A request to convey information; e.g. the CDS system proposes that an alert 2681 * be sent to a responsible provider, the CDS system proposes that the public 2682 * health agency be notified about a reportable condition. 2683 */ 2684 COMMUNICATIONREQUEST, 2685 /** 2686 * A set of healthcare-related information that is assembled together into a 2687 * single logical document that provides a single coherent statement of meaning, 2688 * establishes its own context and that has clinical attestation with regard to 2689 * who is making the statement. While a Composition defines the structure, it 2690 * does not actually contain the content: rather the full content of a document 2691 * is contained in a Bundle, of which the Composition is the first resource 2692 * contained. 2693 */ 2694 COMPOSITION, 2695 /** 2696 * A statement of relationships from one set of concepts to one or more other 2697 * concepts - either code systems or data elements, or classes in class models. 2698 */ 2699 CONCEPTMAP, 2700 /** 2701 * Use to record detailed information about conditions, problems or diagnoses 2702 * recognized by a clinician. There are many uses including: recording a 2703 * diagnosis during an encounter; populating a problem list or a summary 2704 * statement, such as a discharge summary. 2705 */ 2706 CONDITION, 2707 /** 2708 * A conformance statement is a set of capabilities of a FHIR Server that may be 2709 * used as a statement of actual server functionality or a statement of required 2710 * or desired server implementation. 2711 */ 2712 CONFORMANCE, 2713 /** 2714 * A formal agreement between parties regarding the conduct of business, 2715 * exchange of information or other matters. 2716 */ 2717 CONTRACT, 2718 /** 2719 * Financial instrument which may be used to pay for or reimburse health care 2720 * products and services. 2721 */ 2722 COVERAGE, 2723 /** 2724 * The formal description of a single piece of information that can be gathered 2725 * and reported. 2726 */ 2727 DATAELEMENT, 2728 /** 2729 * Indicates an actual or potential clinical issue with or between one or more 2730 * active or proposed clinical actions for a patient; e.g. Drug-drug 2731 * interaction, Ineffective treatment frequency, Procedure-condition conflict, 2732 * etc. 2733 */ 2734 DETECTEDISSUE, 2735 /** 2736 * This resource identifies an instance of a manufactured item that is used in 2737 * the provision of healthcare without being substantially changed through that 2738 * activity. The device may be a medical or non-medical device. Medical devices 2739 * includes durable (reusable) medical equipment, implantable devices, as well 2740 * as disposable equipment used for diagnostic, treatment, and research for 2741 * healthcare and public health. Non-medical devices may include items such as a 2742 * machine, cellphone, computer, application, etc. 2743 */ 2744 DEVICE, 2745 /** 2746 * Describes the characteristics, operational status and capabilities of a 2747 * medical-related component of a medical device. 2748 */ 2749 DEVICECOMPONENT, 2750 /** 2751 * Describes a measurement, calculation or setting capability of a medical 2752 * device. 2753 */ 2754 DEVICEMETRIC, 2755 /** 2756 * Represents a request for a patient to employ a medical device. The device may 2757 * be an implantable device, or an external assistive device, such as a walker. 2758 */ 2759 DEVICEUSEREQUEST, 2760 /** 2761 * A record of a device being used by a patient where the record is the result 2762 * of a report from the patient or another clinician. 2763 */ 2764 DEVICEUSESTATEMENT, 2765 /** 2766 * A record of a request for a diagnostic investigation service to be performed. 2767 */ 2768 DIAGNOSTICORDER, 2769 /** 2770 * The findings and interpretation of diagnostic tests performed on patients, 2771 * groups of patients, devices, and locations, and/or specimens derived from 2772 * these. The report includes clinical context such as requesting and provider 2773 * information, and some mix of atomic results, images, textual and coded 2774 * interpretations, and formatted representation of diagnostic reports. 2775 */ 2776 DIAGNOSTICREPORT, 2777 /** 2778 * A manifest that defines a set of documents. 2779 */ 2780 DOCUMENTMANIFEST, 2781 /** 2782 * A reference to a document . 2783 */ 2784 DOCUMENTREFERENCE, 2785 /** 2786 * --- Abstract Type! ---A resource that includes narrative, extensions, and 2787 * contained resources. 2788 */ 2789 DOMAINRESOURCE, 2790 /** 2791 * This resource provides the insurance eligibility details from the insurer 2792 * regarding a specified coverage and optionally some class of service. 2793 */ 2794 ELIGIBILITYREQUEST, 2795 /** 2796 * This resource provides eligibility and plan details from the processing of an 2797 * Eligibility resource. 2798 */ 2799 ELIGIBILITYRESPONSE, 2800 /** 2801 * An interaction between a patient and healthcare provider(s) for the purpose 2802 * of providing healthcare service(s) or assessing the health status of a 2803 * patient. 2804 */ 2805 ENCOUNTER, 2806 /** 2807 * This resource provides the insurance enrollment details to the insurer 2808 * regarding a specified coverage. 2809 */ 2810 ENROLLMENTREQUEST, 2811 /** 2812 * This resource provides enrollment and plan details from the processing of an 2813 * Enrollment resource. 2814 */ 2815 ENROLLMENTRESPONSE, 2816 /** 2817 * An association between a patient and an organization / healthcare provider(s) 2818 * during which time encounters may occur. The managing organization assumes a 2819 * level of responsibility for the patient during this time. 2820 */ 2821 EPISODEOFCARE, 2822 /** 2823 * This resource provides: the claim details; adjudication details from the 2824 * processing of a Claim; and optionally account balance information, for 2825 * informing the subscriber of the benefits provided. 2826 */ 2827 EXPLANATIONOFBENEFIT, 2828 /** 2829 * Significant health events and conditions for a person related to the patient 2830 * relevant in the context of care for the patient. 2831 */ 2832 FAMILYMEMBERHISTORY, 2833 /** 2834 * Prospective warnings of potential issues when providing care to the patient. 2835 */ 2836 FLAG, 2837 /** 2838 * Describes the intended objective(s) for a patient, group or organization 2839 * care, for example, weight loss, restoring an activity of daily living, 2840 * obtaining herd immunity via immunization, meeting a process improvement 2841 * objective, etc. 2842 */ 2843 GOAL, 2844 /** 2845 * Represents a defined collection of entities that may be discussed or acted 2846 * upon collectively but which are not expected to act collectively and are not 2847 * formally or legally recognized; i.e. a collection of entities that isn't an 2848 * Organization. 2849 */ 2850 GROUP, 2851 /** 2852 * The details of a healthcare service available at a location. 2853 */ 2854 HEALTHCARESERVICE, 2855 /** 2856 * A manifest of a set of DICOM Service-Object Pair Instances (SOP Instances). 2857 * The referenced SOP Instances (images or other content) are for a single 2858 * patient, and may be from one or more studies. The referenced SOP Instances 2859 * have been selected for a purpose, such as quality assurance, conference, or 2860 * consult. Reflecting that range of purposes, typical ImagingObjectSelection 2861 * resources may include all SOP Instances in a study (perhaps for sharing 2862 * through a Health Information Exchange); key images from multiple studies (for 2863 * reference by a referring or treating physician); a multi-frame ultrasound 2864 * instance ("cine" video clip) and a set of measurements taken from that 2865 * instance (for inclusion in a teaching file); and so on. 2866 */ 2867 IMAGINGOBJECTSELECTION, 2868 /** 2869 * Representation of the content produced in a DICOM imaging study. A study 2870 * comprises a set of series, each of which includes a set of Service-Object 2871 * Pair Instances (SOP Instances - images or other data) acquired or produced in 2872 * a common context. A series is of only one modality (e.g. X-ray, CT, MR, 2873 * ultrasound), but a study may have multiple series of different modalities. 2874 */ 2875 IMAGINGSTUDY, 2876 /** 2877 * Describes the event of a patient being administered a vaccination or a record 2878 * of a vaccination as reported by a patient, a clinician or another party and 2879 * may include vaccine reaction information and what vaccination protocol was 2880 * followed. 2881 */ 2882 IMMUNIZATION, 2883 /** 2884 * A patient's point-in-time immunization and recommendation (i.e. forecasting a 2885 * patient's immunization eligibility according to a published schedule) with 2886 * optional supporting justification. 2887 */ 2888 IMMUNIZATIONRECOMMENDATION, 2889 /** 2890 * A set of rules or how FHIR is used to solve a particular problem. This 2891 * resource is used to gather all the parts of an implementation guide into a 2892 * logical whole, and to publish a computable definition of all the parts. 2893 */ 2894 IMPLEMENTATIONGUIDE, 2895 /** 2896 * A set of information summarized from a list of other resources. 2897 */ 2898 LIST, 2899 /** 2900 * Details and position information for a physical place where services are 2901 * provided and resources and participants may be stored, found, contained or 2902 * accommodated. 2903 */ 2904 LOCATION, 2905 /** 2906 * A photo, video, or audio recording acquired or used in healthcare. The actual 2907 * content may be inline or provided by direct reference. 2908 */ 2909 MEDIA, 2910 /** 2911 * This resource is primarily used for the identification and definition of a 2912 * medication. It covers the ingredients and the packaging for a medication. 2913 */ 2914 MEDICATION, 2915 /** 2916 * Describes the event of a patient consuming or otherwise being administered a 2917 * medication. This may be as simple as swallowing a tablet or it may be a long 2918 * running infusion. Related resources tie this event to the authorizing 2919 * prescription, and the specific encounter between patient and health care 2920 * practitioner. 2921 */ 2922 MEDICATIONADMINISTRATION, 2923 /** 2924 * Indicates that a medication product is to be or has been dispensed for a 2925 * named person/patient. This includes a description of the medication product 2926 * (supply) provided and the instructions for administering the medication. The 2927 * medication dispense is the result of a pharmacy system responding to a 2928 * medication order. 2929 */ 2930 MEDICATIONDISPENSE, 2931 /** 2932 * An order for both supply of the medication and the instructions for 2933 * administration of the medication to a patient. The resource is called 2934 * "MedicationOrder" rather than "MedicationPrescription" to generalize the use 2935 * across inpatient and outpatient settings as well as for care plans, etc. 2936 */ 2937 MEDICATIONORDER, 2938 /** 2939 * A record of a medication that is being consumed by a patient. A 2940 * MedicationStatement may indicate that the patient may be taking the 2941 * medication now, or has taken the medication in the past or will be taking the 2942 * medication in the future. The source of this information can be the patient, 2943 * significant other (such as a family member or spouse), or a clinician. A 2944 * common scenario where this information is captured is during the history 2945 * taking process during a patient visit or stay. The medication information may 2946 * come from e.g. the patient's memory, from a prescription bottle, or from a 2947 * list of medications the patient, clinician or other party maintains 2948 * 2949 * The primary difference between a medication statement and a medication 2950 * administration is that the medication administration has complete 2951 * administration information and is based on actual administration information 2952 * from the person who administered the medication. A medication statement is 2953 * often, if not always, less specific. There is no required date/time when the 2954 * medication was administered, in fact we only know that a source has reported 2955 * the patient is taking this medication, where details such as time, quantity, 2956 * or rate or even medication product may be incomplete or missing or less 2957 * precise. As stated earlier, the medication statement information may come 2958 * from the patient's memory, from a prescription bottle or from a list of 2959 * medications the patient, clinician or other party maintains. Medication 2960 * administration is more formal and is not missing detailed information. 2961 */ 2962 MEDICATIONSTATEMENT, 2963 /** 2964 * The header for a message exchange that is either requesting or responding to 2965 * an action. The reference(s) that are the subject of the action as well as 2966 * other information related to the action are typically transmitted in a bundle 2967 * in which the MessageHeader resource instance is the first resource in the 2968 * bundle. 2969 */ 2970 MESSAGEHEADER, 2971 /** 2972 * A curated namespace that issues unique symbols within that namespace for the 2973 * identification of concepts, people, devices, etc. Represents a "System" used 2974 * within the Identifier and Coding data types. 2975 */ 2976 NAMINGSYSTEM, 2977 /** 2978 * A request to supply a diet, formula feeding (enteral) or oral nutritional 2979 * supplement to a patient/resident. 2980 */ 2981 NUTRITIONORDER, 2982 /** 2983 * Measurements and simple assertions made about a patient, device or other 2984 * subject. 2985 */ 2986 OBSERVATION, 2987 /** 2988 * A formal computable definition of an operation (on the RESTful interface) or 2989 * a named query (using the search interaction). 2990 */ 2991 OPERATIONDEFINITION, 2992 /** 2993 * A collection of error, warning or information messages that result from a 2994 * system action. 2995 */ 2996 OPERATIONOUTCOME, 2997 /** 2998 * A request to perform an action. 2999 */ 3000 ORDER, 3001 /** 3002 * A response to an order. 3003 */ 3004 ORDERRESPONSE, 3005 /** 3006 * A formally or informally recognized grouping of people or organizations 3007 * formed for the purpose of achieving some form of collective action. Includes 3008 * companies, institutions, corporations, departments, community groups, 3009 * healthcare practice groups, etc. 3010 */ 3011 ORGANIZATION, 3012 /** 3013 * This special resource type is used to represent an operation request and 3014 * response (operations.html). It has no other use, and there is no RESTful 3015 * endpoint associated with it. 3016 */ 3017 PARAMETERS, 3018 /** 3019 * Demographics and other administrative information about an individual or 3020 * animal receiving care or other health-related services. 3021 */ 3022 PATIENT, 3023 /** 3024 * This resource provides the status of the payment for goods and services 3025 * rendered, and the request and response resource references. 3026 */ 3027 PAYMENTNOTICE, 3028 /** 3029 * This resource provides payment details and claim references supporting a bulk 3030 * payment. 3031 */ 3032 PAYMENTRECONCILIATION, 3033 /** 3034 * Demographics and administrative information about a person independent of a 3035 * specific health-related context. 3036 */ 3037 PERSON, 3038 /** 3039 * A person who is directly or indirectly involved in the provisioning of 3040 * healthcare. 3041 */ 3042 PRACTITIONER, 3043 /** 3044 * An action that is or was performed on a patient. This can be a physical 3045 * intervention like an operation, or less invasive like counseling or 3046 * hypnotherapy. 3047 */ 3048 PROCEDURE, 3049 /** 3050 * A request for a procedure to be performed. May be a proposal or an order. 3051 */ 3052 PROCEDUREREQUEST, 3053 /** 3054 * This resource provides the target, request and response, and action details 3055 * for an action to be performed by the target on or about existing resources. 3056 */ 3057 PROCESSREQUEST, 3058 /** 3059 * This resource provides processing status, errors and notes from the 3060 * processing of a resource. 3061 */ 3062 PROCESSRESPONSE, 3063 /** 3064 * Provenance of a resource is a record that describes entities and processes 3065 * involved in producing and delivering or otherwise influencing that resource. 3066 * Provenance provides a critical foundation for assessing authenticity, 3067 * enabling trust, and allowing reproducibility. Provenance assertions are a 3068 * form of contextual metadata and can themselves become important records with 3069 * their own provenance. Provenance statement indicates clinical significance in 3070 * terms of confidence in authenticity, reliability, and trustworthiness, 3071 * integrity, and stage in lifecycle (e.g. Document Completion - has the 3072 * artifact been legally authenticated), all of which may impact security, 3073 * privacy, and trust policies. 3074 */ 3075 PROVENANCE, 3076 /** 3077 * A structured set of questions intended to guide the collection of answers. 3078 * The questions are ordered and grouped into coherent subsets, corresponding to 3079 * the structure of the grouping of the underlying questions. 3080 */ 3081 QUESTIONNAIRE, 3082 /** 3083 * A structured set of questions and their answers. The questions are ordered 3084 * and grouped into coherent subsets, corresponding to the structure of the 3085 * grouping of the underlying questions. 3086 */ 3087 QUESTIONNAIRERESPONSE, 3088 /** 3089 * Used to record and send details about a request for referral service or 3090 * transfer of a patient to the care of another provider or provider 3091 * organization. 3092 */ 3093 REFERRALREQUEST, 3094 /** 3095 * Information about a person that is involved in the care for a patient, but 3096 * who is not the target of healthcare, nor has a formal responsibility in the 3097 * care process. 3098 */ 3099 RELATEDPERSON, 3100 /** 3101 * --- Abstract Type! ---This is the base resource type for everything. 3102 */ 3103 RESOURCE, 3104 /** 3105 * An assessment of the likely outcome(s) for a patient or other subject as well 3106 * as the likelihood of each outcome. 3107 */ 3108 RISKASSESSMENT, 3109 /** 3110 * A container for slot(s) of time that may be available for booking 3111 * appointments. 3112 */ 3113 SCHEDULE, 3114 /** 3115 * A search parameter that defines a named search item that can be used to 3116 * search/filter on a resource. 3117 */ 3118 SEARCHPARAMETER, 3119 /** 3120 * A slot of time on a schedule that may be available for booking appointments. 3121 */ 3122 SLOT, 3123 /** 3124 * A sample to be used for analysis. 3125 */ 3126 SPECIMEN, 3127 /** 3128 * A definition of a FHIR structure. This resource is used to describe the 3129 * underlying resources, data types defined in FHIR, and also for describing 3130 * extensions, and constraints on resources and data types. 3131 */ 3132 STRUCTUREDEFINITION, 3133 /** 3134 * The subscription resource is used to define a push based subscription from a 3135 * server to another system. Once a subscription is registered with the server, 3136 * the server checks every resource that is created or updated, and if the 3137 * resource matches the given criteria, it sends a message on the defined 3138 * "channel" so that another system is able to take an appropriate action. 3139 */ 3140 SUBSCRIPTION, 3141 /** 3142 * A homogeneous material with a definite composition. 3143 */ 3144 SUBSTANCE, 3145 /** 3146 * Record of delivery of what is supplied. 3147 */ 3148 SUPPLYDELIVERY, 3149 /** 3150 * A record of a request for a medication, substance or device used in the 3151 * healthcare setting. 3152 */ 3153 SUPPLYREQUEST, 3154 /** 3155 * TestScript is a resource that specifies a suite of tests against a FHIR 3156 * server implementation to determine compliance against the FHIR specification. 3157 */ 3158 TESTSCRIPT, 3159 /** 3160 * A value set specifies a set of codes drawn from one or more code systems. 3161 */ 3162 VALUESET, 3163 /** 3164 * An authorization for the supply of glasses and/or contact lenses to a 3165 * patient. 3166 */ 3167 VISIONPRESCRIPTION, 3168 /** 3169 * added to help the parsers 3170 */ 3171 NULL; 3172 3173 public static FHIRDefinedType fromCode(String codeString) throws FHIRException { 3174 if (codeString == null || "".equals(codeString)) 3175 return null; 3176 if ("Address".equals(codeString)) 3177 return ADDRESS; 3178 if ("Age".equals(codeString)) 3179 return AGE; 3180 if ("Annotation".equals(codeString)) 3181 return ANNOTATION; 3182 if ("Attachment".equals(codeString)) 3183 return ATTACHMENT; 3184 if ("BackboneElement".equals(codeString)) 3185 return BACKBONEELEMENT; 3186 if ("CodeableConcept".equals(codeString)) 3187 return CODEABLECONCEPT; 3188 if ("Coding".equals(codeString)) 3189 return CODING; 3190 if ("ContactPoint".equals(codeString)) 3191 return CONTACTPOINT; 3192 if ("Count".equals(codeString)) 3193 return COUNT; 3194 if ("Distance".equals(codeString)) 3195 return DISTANCE; 3196 if ("Duration".equals(codeString)) 3197 return DURATION; 3198 if ("Element".equals(codeString)) 3199 return ELEMENT; 3200 if ("ElementDefinition".equals(codeString)) 3201 return ELEMENTDEFINITION; 3202 if ("Extension".equals(codeString)) 3203 return EXTENSION; 3204 if ("HumanName".equals(codeString)) 3205 return HUMANNAME; 3206 if ("Identifier".equals(codeString)) 3207 return IDENTIFIER; 3208 if ("Meta".equals(codeString)) 3209 return META; 3210 if ("Money".equals(codeString)) 3211 return MONEY; 3212 if ("Narrative".equals(codeString)) 3213 return NARRATIVE; 3214 if ("Period".equals(codeString)) 3215 return PERIOD; 3216 if ("Quantity".equals(codeString)) 3217 return QUANTITY; 3218 if ("Range".equals(codeString)) 3219 return RANGE; 3220 if ("Ratio".equals(codeString)) 3221 return RATIO; 3222 if ("Reference".equals(codeString)) 3223 return REFERENCE; 3224 if ("SampledData".equals(codeString)) 3225 return SAMPLEDDATA; 3226 if ("Signature".equals(codeString)) 3227 return SIGNATURE; 3228 if ("SimpleQuantity".equals(codeString)) 3229 return SIMPLEQUANTITY; 3230 if ("Timing".equals(codeString)) 3231 return TIMING; 3232 if ("base64Binary".equals(codeString)) 3233 return BASE64BINARY; 3234 if ("boolean".equals(codeString)) 3235 return BOOLEAN; 3236 if ("code".equals(codeString)) 3237 return CODE; 3238 if ("date".equals(codeString)) 3239 return DATE; 3240 if ("dateTime".equals(codeString)) 3241 return DATETIME; 3242 if ("decimal".equals(codeString)) 3243 return DECIMAL; 3244 if ("id".equals(codeString)) 3245 return ID; 3246 if ("instant".equals(codeString)) 3247 return INSTANT; 3248 if ("integer".equals(codeString)) 3249 return INTEGER; 3250 if ("markdown".equals(codeString)) 3251 return MARKDOWN; 3252 if ("oid".equals(codeString)) 3253 return OID; 3254 if ("positiveInt".equals(codeString)) 3255 return POSITIVEINT; 3256 if ("string".equals(codeString)) 3257 return STRING; 3258 if ("time".equals(codeString)) 3259 return TIME; 3260 if ("unsignedInt".equals(codeString)) 3261 return UNSIGNEDINT; 3262 if ("uri".equals(codeString)) 3263 return URI; 3264 if ("uuid".equals(codeString)) 3265 return UUID; 3266 if ("xhtml".equals(codeString)) 3267 return XHTML; 3268 if ("Account".equals(codeString)) 3269 return ACCOUNT; 3270 if ("AllergyIntolerance".equals(codeString)) 3271 return ALLERGYINTOLERANCE; 3272 if ("Appointment".equals(codeString)) 3273 return APPOINTMENT; 3274 if ("AppointmentResponse".equals(codeString)) 3275 return APPOINTMENTRESPONSE; 3276 if ("AuditEvent".equals(codeString)) 3277 return AUDITEVENT; 3278 if ("Basic".equals(codeString)) 3279 return BASIC; 3280 if ("Binary".equals(codeString)) 3281 return BINARY; 3282 if ("BodySite".equals(codeString)) 3283 return BODYSITE; 3284 if ("Bundle".equals(codeString)) 3285 return BUNDLE; 3286 if ("CarePlan".equals(codeString)) 3287 return CAREPLAN; 3288 if ("Claim".equals(codeString)) 3289 return CLAIM; 3290 if ("ClaimResponse".equals(codeString)) 3291 return CLAIMRESPONSE; 3292 if ("ClinicalImpression".equals(codeString)) 3293 return CLINICALIMPRESSION; 3294 if ("Communication".equals(codeString)) 3295 return COMMUNICATION; 3296 if ("CommunicationRequest".equals(codeString)) 3297 return COMMUNICATIONREQUEST; 3298 if ("Composition".equals(codeString)) 3299 return COMPOSITION; 3300 if ("ConceptMap".equals(codeString)) 3301 return CONCEPTMAP; 3302 if ("Condition".equals(codeString)) 3303 return CONDITION; 3304 if ("Conformance".equals(codeString)) 3305 return CONFORMANCE; 3306 if ("Contract".equals(codeString)) 3307 return CONTRACT; 3308 if ("Coverage".equals(codeString)) 3309 return COVERAGE; 3310 if ("DataElement".equals(codeString)) 3311 return DATAELEMENT; 3312 if ("DetectedIssue".equals(codeString)) 3313 return DETECTEDISSUE; 3314 if ("Device".equals(codeString)) 3315 return DEVICE; 3316 if ("DeviceComponent".equals(codeString)) 3317 return DEVICECOMPONENT; 3318 if ("DeviceMetric".equals(codeString)) 3319 return DEVICEMETRIC; 3320 if ("DeviceUseRequest".equals(codeString)) 3321 return DEVICEUSEREQUEST; 3322 if ("DeviceUseStatement".equals(codeString)) 3323 return DEVICEUSESTATEMENT; 3324 if ("DiagnosticOrder".equals(codeString)) 3325 return DIAGNOSTICORDER; 3326 if ("DiagnosticReport".equals(codeString)) 3327 return DIAGNOSTICREPORT; 3328 if ("DocumentManifest".equals(codeString)) 3329 return DOCUMENTMANIFEST; 3330 if ("DocumentReference".equals(codeString)) 3331 return DOCUMENTREFERENCE; 3332 if ("DomainResource".equals(codeString)) 3333 return DOMAINRESOURCE; 3334 if ("EligibilityRequest".equals(codeString)) 3335 return ELIGIBILITYREQUEST; 3336 if ("EligibilityResponse".equals(codeString)) 3337 return ELIGIBILITYRESPONSE; 3338 if ("Encounter".equals(codeString)) 3339 return ENCOUNTER; 3340 if ("EnrollmentRequest".equals(codeString)) 3341 return ENROLLMENTREQUEST; 3342 if ("EnrollmentResponse".equals(codeString)) 3343 return ENROLLMENTRESPONSE; 3344 if ("EpisodeOfCare".equals(codeString)) 3345 return EPISODEOFCARE; 3346 if ("ExplanationOfBenefit".equals(codeString)) 3347 return EXPLANATIONOFBENEFIT; 3348 if ("FamilyMemberHistory".equals(codeString)) 3349 return FAMILYMEMBERHISTORY; 3350 if ("Flag".equals(codeString)) 3351 return FLAG; 3352 if ("Goal".equals(codeString)) 3353 return GOAL; 3354 if ("Group".equals(codeString)) 3355 return GROUP; 3356 if ("HealthcareService".equals(codeString)) 3357 return HEALTHCARESERVICE; 3358 if ("ImagingObjectSelection".equals(codeString)) 3359 return IMAGINGOBJECTSELECTION; 3360 if ("ImagingStudy".equals(codeString)) 3361 return IMAGINGSTUDY; 3362 if ("Immunization".equals(codeString)) 3363 return IMMUNIZATION; 3364 if ("ImmunizationRecommendation".equals(codeString)) 3365 return IMMUNIZATIONRECOMMENDATION; 3366 if ("ImplementationGuide".equals(codeString)) 3367 return IMPLEMENTATIONGUIDE; 3368 if ("List".equals(codeString)) 3369 return LIST; 3370 if ("Location".equals(codeString)) 3371 return LOCATION; 3372 if ("Media".equals(codeString)) 3373 return MEDIA; 3374 if ("Medication".equals(codeString)) 3375 return MEDICATION; 3376 if ("MedicationAdministration".equals(codeString)) 3377 return MEDICATIONADMINISTRATION; 3378 if ("MedicationDispense".equals(codeString)) 3379 return MEDICATIONDISPENSE; 3380 if ("MedicationOrder".equals(codeString)) 3381 return MEDICATIONORDER; 3382 if ("MedicationStatement".equals(codeString)) 3383 return MEDICATIONSTATEMENT; 3384 if ("MessageHeader".equals(codeString)) 3385 return MESSAGEHEADER; 3386 if ("NamingSystem".equals(codeString)) 3387 return NAMINGSYSTEM; 3388 if ("NutritionOrder".equals(codeString)) 3389 return NUTRITIONORDER; 3390 if ("Observation".equals(codeString)) 3391 return OBSERVATION; 3392 if ("OperationDefinition".equals(codeString)) 3393 return OPERATIONDEFINITION; 3394 if ("OperationOutcome".equals(codeString)) 3395 return OPERATIONOUTCOME; 3396 if ("Order".equals(codeString)) 3397 return ORDER; 3398 if ("OrderResponse".equals(codeString)) 3399 return ORDERRESPONSE; 3400 if ("Organization".equals(codeString)) 3401 return ORGANIZATION; 3402 if ("Parameters".equals(codeString)) 3403 return PARAMETERS; 3404 if ("Patient".equals(codeString)) 3405 return PATIENT; 3406 if ("PaymentNotice".equals(codeString)) 3407 return PAYMENTNOTICE; 3408 if ("PaymentReconciliation".equals(codeString)) 3409 return PAYMENTRECONCILIATION; 3410 if ("Person".equals(codeString)) 3411 return PERSON; 3412 if ("Practitioner".equals(codeString)) 3413 return PRACTITIONER; 3414 if ("Procedure".equals(codeString)) 3415 return PROCEDURE; 3416 if ("ProcedureRequest".equals(codeString)) 3417 return PROCEDUREREQUEST; 3418 if ("ProcessRequest".equals(codeString)) 3419 return PROCESSREQUEST; 3420 if ("ProcessResponse".equals(codeString)) 3421 return PROCESSRESPONSE; 3422 if ("Provenance".equals(codeString)) 3423 return PROVENANCE; 3424 if ("Questionnaire".equals(codeString)) 3425 return QUESTIONNAIRE; 3426 if ("QuestionnaireResponse".equals(codeString)) 3427 return QUESTIONNAIRERESPONSE; 3428 if ("ReferralRequest".equals(codeString)) 3429 return REFERRALREQUEST; 3430 if ("RelatedPerson".equals(codeString)) 3431 return RELATEDPERSON; 3432 if ("Resource".equals(codeString)) 3433 return RESOURCE; 3434 if ("RiskAssessment".equals(codeString)) 3435 return RISKASSESSMENT; 3436 if ("Schedule".equals(codeString)) 3437 return SCHEDULE; 3438 if ("SearchParameter".equals(codeString)) 3439 return SEARCHPARAMETER; 3440 if ("Slot".equals(codeString)) 3441 return SLOT; 3442 if ("Specimen".equals(codeString)) 3443 return SPECIMEN; 3444 if ("StructureDefinition".equals(codeString)) 3445 return STRUCTUREDEFINITION; 3446 if ("Subscription".equals(codeString)) 3447 return SUBSCRIPTION; 3448 if ("Substance".equals(codeString)) 3449 return SUBSTANCE; 3450 if ("SupplyDelivery".equals(codeString)) 3451 return SUPPLYDELIVERY; 3452 if ("SupplyRequest".equals(codeString)) 3453 return SUPPLYREQUEST; 3454 if ("TestScript".equals(codeString)) 3455 return TESTSCRIPT; 3456 if ("ValueSet".equals(codeString)) 3457 return VALUESET; 3458 if ("VisionPrescription".equals(codeString)) 3459 return VISIONPRESCRIPTION; 3460 throw new FHIRException("Unknown FHIRDefinedType code '" + codeString + "'"); 3461 } 3462 3463 public String toCode() { 3464 switch (this) { 3465 case ADDRESS: 3466 return "Address"; 3467 case AGE: 3468 return "Age"; 3469 case ANNOTATION: 3470 return "Annotation"; 3471 case ATTACHMENT: 3472 return "Attachment"; 3473 case BACKBONEELEMENT: 3474 return "BackboneElement"; 3475 case CODEABLECONCEPT: 3476 return "CodeableConcept"; 3477 case CODING: 3478 return "Coding"; 3479 case CONTACTPOINT: 3480 return "ContactPoint"; 3481 case COUNT: 3482 return "Count"; 3483 case DISTANCE: 3484 return "Distance"; 3485 case DURATION: 3486 return "Duration"; 3487 case ELEMENT: 3488 return "Element"; 3489 case ELEMENTDEFINITION: 3490 return "ElementDefinition"; 3491 case EXTENSION: 3492 return "Extension"; 3493 case HUMANNAME: 3494 return "HumanName"; 3495 case IDENTIFIER: 3496 return "Identifier"; 3497 case META: 3498 return "Meta"; 3499 case MONEY: 3500 return "Money"; 3501 case NARRATIVE: 3502 return "Narrative"; 3503 case PERIOD: 3504 return "Period"; 3505 case QUANTITY: 3506 return "Quantity"; 3507 case RANGE: 3508 return "Range"; 3509 case RATIO: 3510 return "Ratio"; 3511 case REFERENCE: 3512 return "Reference"; 3513 case SAMPLEDDATA: 3514 return "SampledData"; 3515 case SIGNATURE: 3516 return "Signature"; 3517 case SIMPLEQUANTITY: 3518 return "SimpleQuantity"; 3519 case TIMING: 3520 return "Timing"; 3521 case BASE64BINARY: 3522 return "base64Binary"; 3523 case BOOLEAN: 3524 return "boolean"; 3525 case CODE: 3526 return "code"; 3527 case DATE: 3528 return "date"; 3529 case DATETIME: 3530 return "dateTime"; 3531 case DECIMAL: 3532 return "decimal"; 3533 case ID: 3534 return "id"; 3535 case INSTANT: 3536 return "instant"; 3537 case INTEGER: 3538 return "integer"; 3539 case MARKDOWN: 3540 return "markdown"; 3541 case OID: 3542 return "oid"; 3543 case POSITIVEINT: 3544 return "positiveInt"; 3545 case STRING: 3546 return "string"; 3547 case TIME: 3548 return "time"; 3549 case UNSIGNEDINT: 3550 return "unsignedInt"; 3551 case URI: 3552 return "uri"; 3553 case UUID: 3554 return "uuid"; 3555 case XHTML: 3556 return "xhtml"; 3557 case ACCOUNT: 3558 return "Account"; 3559 case ALLERGYINTOLERANCE: 3560 return "AllergyIntolerance"; 3561 case APPOINTMENT: 3562 return "Appointment"; 3563 case APPOINTMENTRESPONSE: 3564 return "AppointmentResponse"; 3565 case AUDITEVENT: 3566 return "AuditEvent"; 3567 case BASIC: 3568 return "Basic"; 3569 case BINARY: 3570 return "Binary"; 3571 case BODYSITE: 3572 return "BodySite"; 3573 case BUNDLE: 3574 return "Bundle"; 3575 case CAREPLAN: 3576 return "CarePlan"; 3577 case CLAIM: 3578 return "Claim"; 3579 case CLAIMRESPONSE: 3580 return "ClaimResponse"; 3581 case CLINICALIMPRESSION: 3582 return "ClinicalImpression"; 3583 case COMMUNICATION: 3584 return "Communication"; 3585 case COMMUNICATIONREQUEST: 3586 return "CommunicationRequest"; 3587 case COMPOSITION: 3588 return "Composition"; 3589 case CONCEPTMAP: 3590 return "ConceptMap"; 3591 case CONDITION: 3592 return "Condition"; 3593 case CONFORMANCE: 3594 return "Conformance"; 3595 case CONTRACT: 3596 return "Contract"; 3597 case COVERAGE: 3598 return "Coverage"; 3599 case DATAELEMENT: 3600 return "DataElement"; 3601 case DETECTEDISSUE: 3602 return "DetectedIssue"; 3603 case DEVICE: 3604 return "Device"; 3605 case DEVICECOMPONENT: 3606 return "DeviceComponent"; 3607 case DEVICEMETRIC: 3608 return "DeviceMetric"; 3609 case DEVICEUSEREQUEST: 3610 return "DeviceUseRequest"; 3611 case DEVICEUSESTATEMENT: 3612 return "DeviceUseStatement"; 3613 case DIAGNOSTICORDER: 3614 return "DiagnosticOrder"; 3615 case DIAGNOSTICREPORT: 3616 return "DiagnosticReport"; 3617 case DOCUMENTMANIFEST: 3618 return "DocumentManifest"; 3619 case DOCUMENTREFERENCE: 3620 return "DocumentReference"; 3621 case DOMAINRESOURCE: 3622 return "DomainResource"; 3623 case ELIGIBILITYREQUEST: 3624 return "EligibilityRequest"; 3625 case ELIGIBILITYRESPONSE: 3626 return "EligibilityResponse"; 3627 case ENCOUNTER: 3628 return "Encounter"; 3629 case ENROLLMENTREQUEST: 3630 return "EnrollmentRequest"; 3631 case ENROLLMENTRESPONSE: 3632 return "EnrollmentResponse"; 3633 case EPISODEOFCARE: 3634 return "EpisodeOfCare"; 3635 case EXPLANATIONOFBENEFIT: 3636 return "ExplanationOfBenefit"; 3637 case FAMILYMEMBERHISTORY: 3638 return "FamilyMemberHistory"; 3639 case FLAG: 3640 return "Flag"; 3641 case GOAL: 3642 return "Goal"; 3643 case GROUP: 3644 return "Group"; 3645 case HEALTHCARESERVICE: 3646 return "HealthcareService"; 3647 case IMAGINGOBJECTSELECTION: 3648 return "ImagingObjectSelection"; 3649 case IMAGINGSTUDY: 3650 return "ImagingStudy"; 3651 case IMMUNIZATION: 3652 return "Immunization"; 3653 case IMMUNIZATIONRECOMMENDATION: 3654 return "ImmunizationRecommendation"; 3655 case IMPLEMENTATIONGUIDE: 3656 return "ImplementationGuide"; 3657 case LIST: 3658 return "List"; 3659 case LOCATION: 3660 return "Location"; 3661 case MEDIA: 3662 return "Media"; 3663 case MEDICATION: 3664 return "Medication"; 3665 case MEDICATIONADMINISTRATION: 3666 return "MedicationAdministration"; 3667 case MEDICATIONDISPENSE: 3668 return "MedicationDispense"; 3669 case MEDICATIONORDER: 3670 return "MedicationOrder"; 3671 case MEDICATIONSTATEMENT: 3672 return "MedicationStatement"; 3673 case MESSAGEHEADER: 3674 return "MessageHeader"; 3675 case NAMINGSYSTEM: 3676 return "NamingSystem"; 3677 case NUTRITIONORDER: 3678 return "NutritionOrder"; 3679 case OBSERVATION: 3680 return "Observation"; 3681 case OPERATIONDEFINITION: 3682 return "OperationDefinition"; 3683 case OPERATIONOUTCOME: 3684 return "OperationOutcome"; 3685 case ORDER: 3686 return "Order"; 3687 case ORDERRESPONSE: 3688 return "OrderResponse"; 3689 case ORGANIZATION: 3690 return "Organization"; 3691 case PARAMETERS: 3692 return "Parameters"; 3693 case PATIENT: 3694 return "Patient"; 3695 case PAYMENTNOTICE: 3696 return "PaymentNotice"; 3697 case PAYMENTRECONCILIATION: 3698 return "PaymentReconciliation"; 3699 case PERSON: 3700 return "Person"; 3701 case PRACTITIONER: 3702 return "Practitioner"; 3703 case PROCEDURE: 3704 return "Procedure"; 3705 case PROCEDUREREQUEST: 3706 return "ProcedureRequest"; 3707 case PROCESSREQUEST: 3708 return "ProcessRequest"; 3709 case PROCESSRESPONSE: 3710 return "ProcessResponse"; 3711 case PROVENANCE: 3712 return "Provenance"; 3713 case QUESTIONNAIRE: 3714 return "Questionnaire"; 3715 case QUESTIONNAIRERESPONSE: 3716 return "QuestionnaireResponse"; 3717 case REFERRALREQUEST: 3718 return "ReferralRequest"; 3719 case RELATEDPERSON: 3720 return "RelatedPerson"; 3721 case RESOURCE: 3722 return "Resource"; 3723 case RISKASSESSMENT: 3724 return "RiskAssessment"; 3725 case SCHEDULE: 3726 return "Schedule"; 3727 case SEARCHPARAMETER: 3728 return "SearchParameter"; 3729 case SLOT: 3730 return "Slot"; 3731 case SPECIMEN: 3732 return "Specimen"; 3733 case STRUCTUREDEFINITION: 3734 return "StructureDefinition"; 3735 case SUBSCRIPTION: 3736 return "Subscription"; 3737 case SUBSTANCE: 3738 return "Substance"; 3739 case SUPPLYDELIVERY: 3740 return "SupplyDelivery"; 3741 case SUPPLYREQUEST: 3742 return "SupplyRequest"; 3743 case TESTSCRIPT: 3744 return "TestScript"; 3745 case VALUESET: 3746 return "ValueSet"; 3747 case VISIONPRESCRIPTION: 3748 return "VisionPrescription"; 3749 case NULL: 3750 return null; 3751 default: 3752 return "?"; 3753 } 3754 } 3755 3756 public String getSystem() { 3757 switch (this) { 3758 case ADDRESS: 3759 return "http://hl7.org/fhir/data-types"; 3760 case AGE: 3761 return "http://hl7.org/fhir/data-types"; 3762 case ANNOTATION: 3763 return "http://hl7.org/fhir/data-types"; 3764 case ATTACHMENT: 3765 return "http://hl7.org/fhir/data-types"; 3766 case BACKBONEELEMENT: 3767 return "http://hl7.org/fhir/data-types"; 3768 case CODEABLECONCEPT: 3769 return "http://hl7.org/fhir/data-types"; 3770 case CODING: 3771 return "http://hl7.org/fhir/data-types"; 3772 case CONTACTPOINT: 3773 return "http://hl7.org/fhir/data-types"; 3774 case COUNT: 3775 return "http://hl7.org/fhir/data-types"; 3776 case DISTANCE: 3777 return "http://hl7.org/fhir/data-types"; 3778 case DURATION: 3779 return "http://hl7.org/fhir/data-types"; 3780 case ELEMENT: 3781 return "http://hl7.org/fhir/data-types"; 3782 case ELEMENTDEFINITION: 3783 return "http://hl7.org/fhir/data-types"; 3784 case EXTENSION: 3785 return "http://hl7.org/fhir/data-types"; 3786 case HUMANNAME: 3787 return "http://hl7.org/fhir/data-types"; 3788 case IDENTIFIER: 3789 return "http://hl7.org/fhir/data-types"; 3790 case META: 3791 return "http://hl7.org/fhir/data-types"; 3792 case MONEY: 3793 return "http://hl7.org/fhir/data-types"; 3794 case NARRATIVE: 3795 return "http://hl7.org/fhir/data-types"; 3796 case PERIOD: 3797 return "http://hl7.org/fhir/data-types"; 3798 case QUANTITY: 3799 return "http://hl7.org/fhir/data-types"; 3800 case RANGE: 3801 return "http://hl7.org/fhir/data-types"; 3802 case RATIO: 3803 return "http://hl7.org/fhir/data-types"; 3804 case REFERENCE: 3805 return "http://hl7.org/fhir/data-types"; 3806 case SAMPLEDDATA: 3807 return "http://hl7.org/fhir/data-types"; 3808 case SIGNATURE: 3809 return "http://hl7.org/fhir/data-types"; 3810 case SIMPLEQUANTITY: 3811 return "http://hl7.org/fhir/data-types"; 3812 case TIMING: 3813 return "http://hl7.org/fhir/data-types"; 3814 case BASE64BINARY: 3815 return "http://hl7.org/fhir/data-types"; 3816 case BOOLEAN: 3817 return "http://hl7.org/fhir/data-types"; 3818 case CODE: 3819 return "http://hl7.org/fhir/data-types"; 3820 case DATE: 3821 return "http://hl7.org/fhir/data-types"; 3822 case DATETIME: 3823 return "http://hl7.org/fhir/data-types"; 3824 case DECIMAL: 3825 return "http://hl7.org/fhir/data-types"; 3826 case ID: 3827 return "http://hl7.org/fhir/data-types"; 3828 case INSTANT: 3829 return "http://hl7.org/fhir/data-types"; 3830 case INTEGER: 3831 return "http://hl7.org/fhir/data-types"; 3832 case MARKDOWN: 3833 return "http://hl7.org/fhir/data-types"; 3834 case OID: 3835 return "http://hl7.org/fhir/data-types"; 3836 case POSITIVEINT: 3837 return "http://hl7.org/fhir/data-types"; 3838 case STRING: 3839 return "http://hl7.org/fhir/data-types"; 3840 case TIME: 3841 return "http://hl7.org/fhir/data-types"; 3842 case UNSIGNEDINT: 3843 return "http://hl7.org/fhir/data-types"; 3844 case URI: 3845 return "http://hl7.org/fhir/data-types"; 3846 case UUID: 3847 return "http://hl7.org/fhir/data-types"; 3848 case XHTML: 3849 return "http://hl7.org/fhir/data-types"; 3850 case ACCOUNT: 3851 return "http://hl7.org/fhir/resource-types"; 3852 case ALLERGYINTOLERANCE: 3853 return "http://hl7.org/fhir/resource-types"; 3854 case APPOINTMENT: 3855 return "http://hl7.org/fhir/resource-types"; 3856 case APPOINTMENTRESPONSE: 3857 return "http://hl7.org/fhir/resource-types"; 3858 case AUDITEVENT: 3859 return "http://hl7.org/fhir/resource-types"; 3860 case BASIC: 3861 return "http://hl7.org/fhir/resource-types"; 3862 case BINARY: 3863 return "http://hl7.org/fhir/resource-types"; 3864 case BODYSITE: 3865 return "http://hl7.org/fhir/resource-types"; 3866 case BUNDLE: 3867 return "http://hl7.org/fhir/resource-types"; 3868 case CAREPLAN: 3869 return "http://hl7.org/fhir/resource-types"; 3870 case CLAIM: 3871 return "http://hl7.org/fhir/resource-types"; 3872 case CLAIMRESPONSE: 3873 return "http://hl7.org/fhir/resource-types"; 3874 case CLINICALIMPRESSION: 3875 return "http://hl7.org/fhir/resource-types"; 3876 case COMMUNICATION: 3877 return "http://hl7.org/fhir/resource-types"; 3878 case COMMUNICATIONREQUEST: 3879 return "http://hl7.org/fhir/resource-types"; 3880 case COMPOSITION: 3881 return "http://hl7.org/fhir/resource-types"; 3882 case CONCEPTMAP: 3883 return "http://hl7.org/fhir/resource-types"; 3884 case CONDITION: 3885 return "http://hl7.org/fhir/resource-types"; 3886 case CONFORMANCE: 3887 return "http://hl7.org/fhir/resource-types"; 3888 case CONTRACT: 3889 return "http://hl7.org/fhir/resource-types"; 3890 case COVERAGE: 3891 return "http://hl7.org/fhir/resource-types"; 3892 case DATAELEMENT: 3893 return "http://hl7.org/fhir/resource-types"; 3894 case DETECTEDISSUE: 3895 return "http://hl7.org/fhir/resource-types"; 3896 case DEVICE: 3897 return "http://hl7.org/fhir/resource-types"; 3898 case DEVICECOMPONENT: 3899 return "http://hl7.org/fhir/resource-types"; 3900 case DEVICEMETRIC: 3901 return "http://hl7.org/fhir/resource-types"; 3902 case DEVICEUSEREQUEST: 3903 return "http://hl7.org/fhir/resource-types"; 3904 case DEVICEUSESTATEMENT: 3905 return "http://hl7.org/fhir/resource-types"; 3906 case DIAGNOSTICORDER: 3907 return "http://hl7.org/fhir/resource-types"; 3908 case DIAGNOSTICREPORT: 3909 return "http://hl7.org/fhir/resource-types"; 3910 case DOCUMENTMANIFEST: 3911 return "http://hl7.org/fhir/resource-types"; 3912 case DOCUMENTREFERENCE: 3913 return "http://hl7.org/fhir/resource-types"; 3914 case DOMAINRESOURCE: 3915 return "http://hl7.org/fhir/resource-types"; 3916 case ELIGIBILITYREQUEST: 3917 return "http://hl7.org/fhir/resource-types"; 3918 case ELIGIBILITYRESPONSE: 3919 return "http://hl7.org/fhir/resource-types"; 3920 case ENCOUNTER: 3921 return "http://hl7.org/fhir/resource-types"; 3922 case ENROLLMENTREQUEST: 3923 return "http://hl7.org/fhir/resource-types"; 3924 case ENROLLMENTRESPONSE: 3925 return "http://hl7.org/fhir/resource-types"; 3926 case EPISODEOFCARE: 3927 return "http://hl7.org/fhir/resource-types"; 3928 case EXPLANATIONOFBENEFIT: 3929 return "http://hl7.org/fhir/resource-types"; 3930 case FAMILYMEMBERHISTORY: 3931 return "http://hl7.org/fhir/resource-types"; 3932 case FLAG: 3933 return "http://hl7.org/fhir/resource-types"; 3934 case GOAL: 3935 return "http://hl7.org/fhir/resource-types"; 3936 case GROUP: 3937 return "http://hl7.org/fhir/resource-types"; 3938 case HEALTHCARESERVICE: 3939 return "http://hl7.org/fhir/resource-types"; 3940 case IMAGINGOBJECTSELECTION: 3941 return "http://hl7.org/fhir/resource-types"; 3942 case IMAGINGSTUDY: 3943 return "http://hl7.org/fhir/resource-types"; 3944 case IMMUNIZATION: 3945 return "http://hl7.org/fhir/resource-types"; 3946 case IMMUNIZATIONRECOMMENDATION: 3947 return "http://hl7.org/fhir/resource-types"; 3948 case IMPLEMENTATIONGUIDE: 3949 return "http://hl7.org/fhir/resource-types"; 3950 case LIST: 3951 return "http://hl7.org/fhir/resource-types"; 3952 case LOCATION: 3953 return "http://hl7.org/fhir/resource-types"; 3954 case MEDIA: 3955 return "http://hl7.org/fhir/resource-types"; 3956 case MEDICATION: 3957 return "http://hl7.org/fhir/resource-types"; 3958 case MEDICATIONADMINISTRATION: 3959 return "http://hl7.org/fhir/resource-types"; 3960 case MEDICATIONDISPENSE: 3961 return "http://hl7.org/fhir/resource-types"; 3962 case MEDICATIONORDER: 3963 return "http://hl7.org/fhir/resource-types"; 3964 case MEDICATIONSTATEMENT: 3965 return "http://hl7.org/fhir/resource-types"; 3966 case MESSAGEHEADER: 3967 return "http://hl7.org/fhir/resource-types"; 3968 case NAMINGSYSTEM: 3969 return "http://hl7.org/fhir/resource-types"; 3970 case NUTRITIONORDER: 3971 return "http://hl7.org/fhir/resource-types"; 3972 case OBSERVATION: 3973 return "http://hl7.org/fhir/resource-types"; 3974 case OPERATIONDEFINITION: 3975 return "http://hl7.org/fhir/resource-types"; 3976 case OPERATIONOUTCOME: 3977 return "http://hl7.org/fhir/resource-types"; 3978 case ORDER: 3979 return "http://hl7.org/fhir/resource-types"; 3980 case ORDERRESPONSE: 3981 return "http://hl7.org/fhir/resource-types"; 3982 case ORGANIZATION: 3983 return "http://hl7.org/fhir/resource-types"; 3984 case PARAMETERS: 3985 return "http://hl7.org/fhir/resource-types"; 3986 case PATIENT: 3987 return "http://hl7.org/fhir/resource-types"; 3988 case PAYMENTNOTICE: 3989 return "http://hl7.org/fhir/resource-types"; 3990 case PAYMENTRECONCILIATION: 3991 return "http://hl7.org/fhir/resource-types"; 3992 case PERSON: 3993 return "http://hl7.org/fhir/resource-types"; 3994 case PRACTITIONER: 3995 return "http://hl7.org/fhir/resource-types"; 3996 case PROCEDURE: 3997 return "http://hl7.org/fhir/resource-types"; 3998 case PROCEDUREREQUEST: 3999 return "http://hl7.org/fhir/resource-types"; 4000 case PROCESSREQUEST: 4001 return "http://hl7.org/fhir/resource-types"; 4002 case PROCESSRESPONSE: 4003 return "http://hl7.org/fhir/resource-types"; 4004 case PROVENANCE: 4005 return "http://hl7.org/fhir/resource-types"; 4006 case QUESTIONNAIRE: 4007 return "http://hl7.org/fhir/resource-types"; 4008 case QUESTIONNAIRERESPONSE: 4009 return "http://hl7.org/fhir/resource-types"; 4010 case REFERRALREQUEST: 4011 return "http://hl7.org/fhir/resource-types"; 4012 case RELATEDPERSON: 4013 return "http://hl7.org/fhir/resource-types"; 4014 case RESOURCE: 4015 return "http://hl7.org/fhir/resource-types"; 4016 case RISKASSESSMENT: 4017 return "http://hl7.org/fhir/resource-types"; 4018 case SCHEDULE: 4019 return "http://hl7.org/fhir/resource-types"; 4020 case SEARCHPARAMETER: 4021 return "http://hl7.org/fhir/resource-types"; 4022 case SLOT: 4023 return "http://hl7.org/fhir/resource-types"; 4024 case SPECIMEN: 4025 return "http://hl7.org/fhir/resource-types"; 4026 case STRUCTUREDEFINITION: 4027 return "http://hl7.org/fhir/resource-types"; 4028 case SUBSCRIPTION: 4029 return "http://hl7.org/fhir/resource-types"; 4030 case SUBSTANCE: 4031 return "http://hl7.org/fhir/resource-types"; 4032 case SUPPLYDELIVERY: 4033 return "http://hl7.org/fhir/resource-types"; 4034 case SUPPLYREQUEST: 4035 return "http://hl7.org/fhir/resource-types"; 4036 case TESTSCRIPT: 4037 return "http://hl7.org/fhir/resource-types"; 4038 case VALUESET: 4039 return "http://hl7.org/fhir/resource-types"; 4040 case VISIONPRESCRIPTION: 4041 return "http://hl7.org/fhir/resource-types"; 4042 case NULL: 4043 return null; 4044 default: 4045 return "?"; 4046 } 4047 } 4048 4049 public String getDefinition() { 4050 switch (this) { 4051 case ADDRESS: 4052 return "There is a variety of postal address formats defined around the world. This format defines a superset that is the basis for all addresses around the world."; 4053 case AGE: 4054 return ""; 4055 case ANNOTATION: 4056 return "A text note which also contains information about who made the statement and when."; 4057 case ATTACHMENT: 4058 return "For referring to data content defined in other formats."; 4059 case BACKBONEELEMENT: 4060 return "Base definition for all elements that are defined inside a resource - but not those in a data type."; 4061 case CODEABLECONCEPT: 4062 return "A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text."; 4063 case CODING: 4064 return "A reference to a code defined by a terminology system."; 4065 case CONTACTPOINT: 4066 return "Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc."; 4067 case COUNT: 4068 return ""; 4069 case DISTANCE: 4070 return ""; 4071 case DURATION: 4072 return ""; 4073 case ELEMENT: 4074 return "Base definition for all elements in a resource."; 4075 case ELEMENTDEFINITION: 4076 return "Captures constraints on each element within the resource, profile, or extension."; 4077 case EXTENSION: 4078 return "Optional Extensions Element - found in all resources."; 4079 case HUMANNAME: 4080 return "A human's name with the ability to identify parts and usage."; 4081 case IDENTIFIER: 4082 return "A technical identifier - identifies some entity uniquely and unambiguously."; 4083 case META: 4084 return "The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource."; 4085 case MONEY: 4086 return ""; 4087 case NARRATIVE: 4088 return "A human-readable formatted text, including images."; 4089 case PERIOD: 4090 return "A time period defined by a start and end date and optionally time."; 4091 case QUANTITY: 4092 return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 4093 case RANGE: 4094 return "A set of ordered Quantities defined by a low and high limit."; 4095 case RATIO: 4096 return "A relationship of two Quantity values - expressed as a numerator and a denominator."; 4097 case REFERENCE: 4098 return "A reference from one resource to another."; 4099 case SAMPLEDDATA: 4100 return "A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data."; 4101 case SIGNATURE: 4102 return "A digital signature along with supporting context. The signature may be electronic/cryptographic in nature, or a graphical image representing a hand-written signature, or a signature process. Different Signature approaches have different utilities."; 4103 case SIMPLEQUANTITY: 4104 return ""; 4105 case TIMING: 4106 return "Specifies an event that may occur multiple times. Timing schedules are used to record when things are expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds."; 4107 case BASE64BINARY: 4108 return "A stream of bytes"; 4109 case BOOLEAN: 4110 return "Value of \"true\" or \"false\""; 4111 case CODE: 4112 return "A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents"; 4113 case DATE: 4114 return "A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates."; 4115 case DATETIME: 4116 return "A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates."; 4117 case DECIMAL: 4118 return "A rational number with implicit precision"; 4119 case ID: 4120 return "Any combination of letters, numerals, \"-\" and \".\", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive."; 4121 case INSTANT: 4122 return "An instant in time - known at least to the second"; 4123 case INTEGER: 4124 return "A whole number"; 4125 case MARKDOWN: 4126 return "A string that may contain markdown syntax for optional processing by a mark down presentation engine"; 4127 case OID: 4128 return "An oid represented as a URI"; 4129 case POSITIVEINT: 4130 return "An integer with a value that is positive (e.g. >0)"; 4131 case STRING: 4132 return "A sequence of Unicode characters"; 4133 case TIME: 4134 return "A time during the day, with no date specified"; 4135 case UNSIGNEDINT: 4136 return "An integer with a value that is not negative (e.g. >= 0)"; 4137 case URI: 4138 return "String of characters used to identify a name or a resource"; 4139 case UUID: 4140 return "A UUID, represented as a URI"; 4141 case XHTML: 4142 return "XHTML format, as defined by W3C, but restricted usage (mainly, no active content)"; 4143 case ACCOUNT: 4144 return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centres, etc."; 4145 case ALLERGYINTOLERANCE: 4146 return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 4147 case APPOINTMENT: 4148 return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 4149 case APPOINTMENTRESPONSE: 4150 return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 4151 case AUDITEVENT: 4152 return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 4153 case BASIC: 4154 return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 4155 case BINARY: 4156 return "A binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 4157 case BODYSITE: 4158 return "Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 4159 case BUNDLE: 4160 return "A container for a collection of resources."; 4161 case CAREPLAN: 4162 return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 4163 case CLAIM: 4164 return "A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery."; 4165 case CLAIMRESPONSE: 4166 return "This resource provides the adjudication details from the processing of a Claim resource."; 4167 case CLINICALIMPRESSION: 4168 return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 4169 case COMMUNICATION: 4170 return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition."; 4171 case COMMUNICATIONREQUEST: 4172 return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 4173 case COMPOSITION: 4174 return "A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained."; 4175 case CONCEPTMAP: 4176 return "A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models."; 4177 case CONDITION: 4178 return "Use to record detailed information about conditions, problems or diagnoses recognized by a clinician. There are many uses including: recording a diagnosis during an encounter; populating a problem list or a summary statement, such as a discharge summary."; 4179 case CONFORMANCE: 4180 return "A conformance statement is a set of capabilities of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 4181 case CONTRACT: 4182 return "A formal agreement between parties regarding the conduct of business, exchange of information or other matters."; 4183 case COVERAGE: 4184 return "Financial instrument which may be used to pay for or reimburse health care products and services."; 4185 case DATAELEMENT: 4186 return "The formal description of a single piece of information that can be gathered and reported."; 4187 case DETECTEDISSUE: 4188 return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 4189 case DEVICE: 4190 return "This resource identifies an instance of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices includes durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc."; 4191 case DEVICECOMPONENT: 4192 return "Describes the characteristics, operational status and capabilities of a medical-related component of a medical device."; 4193 case DEVICEMETRIC: 4194 return "Describes a measurement, calculation or setting capability of a medical device."; 4195 case DEVICEUSEREQUEST: 4196 return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 4197 case DEVICEUSESTATEMENT: 4198 return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 4199 case DIAGNOSTICORDER: 4200 return "A record of a request for a diagnostic investigation service to be performed."; 4201 case DIAGNOSTICREPORT: 4202 return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 4203 case DOCUMENTMANIFEST: 4204 return "A manifest that defines a set of documents."; 4205 case DOCUMENTREFERENCE: 4206 return "A reference to a document ."; 4207 case DOMAINRESOURCE: 4208 return "--- Abstract Type! ---A resource that includes narrative, extensions, and contained resources."; 4209 case ELIGIBILITYREQUEST: 4210 return "This resource provides the insurance eligibility details from the insurer regarding a specified coverage and optionally some class of service."; 4211 case ELIGIBILITYRESPONSE: 4212 return "This resource provides eligibility and plan details from the processing of an Eligibility resource."; 4213 case ENCOUNTER: 4214 return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 4215 case ENROLLMENTREQUEST: 4216 return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 4217 case ENROLLMENTRESPONSE: 4218 return "This resource provides enrollment and plan details from the processing of an Enrollment resource."; 4219 case EPISODEOFCARE: 4220 return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 4221 case EXPLANATIONOFBENEFIT: 4222 return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 4223 case FAMILYMEMBERHISTORY: 4224 return "Significant health events and conditions for a person related to the patient relevant in the context of care for the patient."; 4225 case FLAG: 4226 return "Prospective warnings of potential issues when providing care to the patient."; 4227 case GOAL: 4228 return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 4229 case GROUP: 4230 return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 4231 case HEALTHCARESERVICE: 4232 return "The details of a healthcare service available at a location."; 4233 case IMAGINGOBJECTSELECTION: 4234 return "A manifest of a set of DICOM Service-Object Pair Instances (SOP Instances). The referenced SOP Instances (images or other content) are for a single patient, and may be from one or more studies. The referenced SOP Instances have been selected for a purpose, such as quality assurance, conference, or consult. Reflecting that range of purposes, typical ImagingObjectSelection resources may include all SOP Instances in a study (perhaps for sharing through a Health Information Exchange); key images from multiple studies (for reference by a referring or treating physician); a multi-frame ultrasound instance (\"cine\" video clip) and a set of measurements taken from that instance (for inclusion in a teaching file); and so on."; 4235 case IMAGINGSTUDY: 4236 return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 4237 case IMMUNIZATION: 4238 return "Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed."; 4239 case IMMUNIZATIONRECOMMENDATION: 4240 return "A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification."; 4241 case IMPLEMENTATIONGUIDE: 4242 return "A set of rules or how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole, and to publish a computable definition of all the parts."; 4243 case LIST: 4244 return "A set of information summarized from a list of other resources."; 4245 case LOCATION: 4246 return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated."; 4247 case MEDIA: 4248 return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 4249 case MEDICATION: 4250 return "This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication."; 4251 case MEDICATIONADMINISTRATION: 4252 return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 4253 case MEDICATIONDISPENSE: 4254 return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 4255 case MEDICATIONORDER: 4256 return "An order for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationOrder\" rather than \"MedicationPrescription\" to generalize the use across inpatient and outpatient settings as well as for care plans, etc."; 4257 case MEDICATIONSTATEMENT: 4258 return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from e.g. the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains \r\rThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 4259 case MESSAGEHEADER: 4260 return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 4261 case NAMINGSYSTEM: 4262 return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 4263 case NUTRITIONORDER: 4264 return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 4265 case OBSERVATION: 4266 return "Measurements and simple assertions made about a patient, device or other subject."; 4267 case OPERATIONDEFINITION: 4268 return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 4269 case OPERATIONOUTCOME: 4270 return "A collection of error, warning or information messages that result from a system action."; 4271 case ORDER: 4272 return "A request to perform an action."; 4273 case ORDERRESPONSE: 4274 return "A response to an order."; 4275 case ORGANIZATION: 4276 return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc."; 4277 case PARAMETERS: 4278 return "This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 4279 case PATIENT: 4280 return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 4281 case PAYMENTNOTICE: 4282 return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 4283 case PAYMENTRECONCILIATION: 4284 return "This resource provides payment details and claim references supporting a bulk payment."; 4285 case PERSON: 4286 return "Demographics and administrative information about a person independent of a specific health-related context."; 4287 case PRACTITIONER: 4288 return "A person who is directly or indirectly involved in the provisioning of healthcare."; 4289 case PROCEDURE: 4290 return "An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy."; 4291 case PROCEDUREREQUEST: 4292 return "A request for a procedure to be performed. May be a proposal or an order."; 4293 case PROCESSREQUEST: 4294 return "This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources."; 4295 case PROCESSRESPONSE: 4296 return "This resource provides processing status, errors and notes from the processing of a resource."; 4297 case PROVENANCE: 4298 return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 4299 case QUESTIONNAIRE: 4300 return "A structured set of questions intended to guide the collection of answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the underlying questions."; 4301 case QUESTIONNAIRERESPONSE: 4302 return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the underlying questions."; 4303 case REFERRALREQUEST: 4304 return "Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization."; 4305 case RELATEDPERSON: 4306 return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 4307 case RESOURCE: 4308 return "--- Abstract Type! ---This is the base resource type for everything."; 4309 case RISKASSESSMENT: 4310 return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 4311 case SCHEDULE: 4312 return "A container for slot(s) of time that may be available for booking appointments."; 4313 case SEARCHPARAMETER: 4314 return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 4315 case SLOT: 4316 return "A slot of time on a schedule that may be available for booking appointments."; 4317 case SPECIMEN: 4318 return "A sample to be used for analysis."; 4319 case STRUCTUREDEFINITION: 4320 return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions, and constraints on resources and data types."; 4321 case SUBSCRIPTION: 4322 return "The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system is able to take an appropriate action."; 4323 case SUBSTANCE: 4324 return "A homogeneous material with a definite composition."; 4325 case SUPPLYDELIVERY: 4326 return "Record of delivery of what is supplied."; 4327 case SUPPLYREQUEST: 4328 return "A record of a request for a medication, substance or device used in the healthcare setting."; 4329 case TESTSCRIPT: 4330 return "TestScript is a resource that specifies a suite of tests against a FHIR server implementation to determine compliance against the FHIR specification."; 4331 case VALUESET: 4332 return "A value set specifies a set of codes drawn from one or more code systems."; 4333 case VISIONPRESCRIPTION: 4334 return "An authorization for the supply of glasses and/or contact lenses to a patient."; 4335 case NULL: 4336 return null; 4337 default: 4338 return "?"; 4339 } 4340 } 4341 4342 public String getDisplay() { 4343 switch (this) { 4344 case ADDRESS: 4345 return "Address"; 4346 case AGE: 4347 return "Age"; 4348 case ANNOTATION: 4349 return "Annotation"; 4350 case ATTACHMENT: 4351 return "Attachment"; 4352 case BACKBONEELEMENT: 4353 return "BackboneElement"; 4354 case CODEABLECONCEPT: 4355 return "CodeableConcept"; 4356 case CODING: 4357 return "Coding"; 4358 case CONTACTPOINT: 4359 return "ContactPoint"; 4360 case COUNT: 4361 return "Count"; 4362 case DISTANCE: 4363 return "Distance"; 4364 case DURATION: 4365 return "Duration"; 4366 case ELEMENT: 4367 return "Element"; 4368 case ELEMENTDEFINITION: 4369 return "ElementDefinition"; 4370 case EXTENSION: 4371 return "Extension"; 4372 case HUMANNAME: 4373 return "HumanName"; 4374 case IDENTIFIER: 4375 return "Identifier"; 4376 case META: 4377 return "Meta"; 4378 case MONEY: 4379 return "Money"; 4380 case NARRATIVE: 4381 return "Narrative"; 4382 case PERIOD: 4383 return "Period"; 4384 case QUANTITY: 4385 return "Quantity"; 4386 case RANGE: 4387 return "Range"; 4388 case RATIO: 4389 return "Ratio"; 4390 case REFERENCE: 4391 return "Reference"; 4392 case SAMPLEDDATA: 4393 return "SampledData"; 4394 case SIGNATURE: 4395 return "Signature"; 4396 case SIMPLEQUANTITY: 4397 return "SimpleQuantity"; 4398 case TIMING: 4399 return "Timing"; 4400 case BASE64BINARY: 4401 return "base64Binary"; 4402 case BOOLEAN: 4403 return "boolean"; 4404 case CODE: 4405 return "code"; 4406 case DATE: 4407 return "date"; 4408 case DATETIME: 4409 return "dateTime"; 4410 case DECIMAL: 4411 return "decimal"; 4412 case ID: 4413 return "id"; 4414 case INSTANT: 4415 return "instant"; 4416 case INTEGER: 4417 return "integer"; 4418 case MARKDOWN: 4419 return "markdown"; 4420 case OID: 4421 return "oid"; 4422 case POSITIVEINT: 4423 return "positiveInt"; 4424 case STRING: 4425 return "string"; 4426 case TIME: 4427 return "time"; 4428 case UNSIGNEDINT: 4429 return "unsignedInt"; 4430 case URI: 4431 return "uri"; 4432 case UUID: 4433 return "uuid"; 4434 case XHTML: 4435 return "XHTML"; 4436 case ACCOUNT: 4437 return "Account"; 4438 case ALLERGYINTOLERANCE: 4439 return "AllergyIntolerance"; 4440 case APPOINTMENT: 4441 return "Appointment"; 4442 case APPOINTMENTRESPONSE: 4443 return "AppointmentResponse"; 4444 case AUDITEVENT: 4445 return "AuditEvent"; 4446 case BASIC: 4447 return "Basic"; 4448 case BINARY: 4449 return "Binary"; 4450 case BODYSITE: 4451 return "BodySite"; 4452 case BUNDLE: 4453 return "Bundle"; 4454 case CAREPLAN: 4455 return "CarePlan"; 4456 case CLAIM: 4457 return "Claim"; 4458 case CLAIMRESPONSE: 4459 return "ClaimResponse"; 4460 case CLINICALIMPRESSION: 4461 return "ClinicalImpression"; 4462 case COMMUNICATION: 4463 return "Communication"; 4464 case COMMUNICATIONREQUEST: 4465 return "CommunicationRequest"; 4466 case COMPOSITION: 4467 return "Composition"; 4468 case CONCEPTMAP: 4469 return "ConceptMap"; 4470 case CONDITION: 4471 return "Condition"; 4472 case CONFORMANCE: 4473 return "Conformance"; 4474 case CONTRACT: 4475 return "Contract"; 4476 case COVERAGE: 4477 return "Coverage"; 4478 case DATAELEMENT: 4479 return "DataElement"; 4480 case DETECTEDISSUE: 4481 return "DetectedIssue"; 4482 case DEVICE: 4483 return "Device"; 4484 case DEVICECOMPONENT: 4485 return "DeviceComponent"; 4486 case DEVICEMETRIC: 4487 return "DeviceMetric"; 4488 case DEVICEUSEREQUEST: 4489 return "DeviceUseRequest"; 4490 case DEVICEUSESTATEMENT: 4491 return "DeviceUseStatement"; 4492 case DIAGNOSTICORDER: 4493 return "DiagnosticOrder"; 4494 case DIAGNOSTICREPORT: 4495 return "DiagnosticReport"; 4496 case DOCUMENTMANIFEST: 4497 return "DocumentManifest"; 4498 case DOCUMENTREFERENCE: 4499 return "DocumentReference"; 4500 case DOMAINRESOURCE: 4501 return "DomainResource"; 4502 case ELIGIBILITYREQUEST: 4503 return "EligibilityRequest"; 4504 case ELIGIBILITYRESPONSE: 4505 return "EligibilityResponse"; 4506 case ENCOUNTER: 4507 return "Encounter"; 4508 case ENROLLMENTREQUEST: 4509 return "EnrollmentRequest"; 4510 case ENROLLMENTRESPONSE: 4511 return "EnrollmentResponse"; 4512 case EPISODEOFCARE: 4513 return "EpisodeOfCare"; 4514 case EXPLANATIONOFBENEFIT: 4515 return "ExplanationOfBenefit"; 4516 case FAMILYMEMBERHISTORY: 4517 return "FamilyMemberHistory"; 4518 case FLAG: 4519 return "Flag"; 4520 case GOAL: 4521 return "Goal"; 4522 case GROUP: 4523 return "Group"; 4524 case HEALTHCARESERVICE: 4525 return "HealthcareService"; 4526 case IMAGINGOBJECTSELECTION: 4527 return "ImagingObjectSelection"; 4528 case IMAGINGSTUDY: 4529 return "ImagingStudy"; 4530 case IMMUNIZATION: 4531 return "Immunization"; 4532 case IMMUNIZATIONRECOMMENDATION: 4533 return "ImmunizationRecommendation"; 4534 case IMPLEMENTATIONGUIDE: 4535 return "ImplementationGuide"; 4536 case LIST: 4537 return "List"; 4538 case LOCATION: 4539 return "Location"; 4540 case MEDIA: 4541 return "Media"; 4542 case MEDICATION: 4543 return "Medication"; 4544 case MEDICATIONADMINISTRATION: 4545 return "MedicationAdministration"; 4546 case MEDICATIONDISPENSE: 4547 return "MedicationDispense"; 4548 case MEDICATIONORDER: 4549 return "MedicationOrder"; 4550 case MEDICATIONSTATEMENT: 4551 return "MedicationStatement"; 4552 case MESSAGEHEADER: 4553 return "MessageHeader"; 4554 case NAMINGSYSTEM: 4555 return "NamingSystem"; 4556 case NUTRITIONORDER: 4557 return "NutritionOrder"; 4558 case OBSERVATION: 4559 return "Observation"; 4560 case OPERATIONDEFINITION: 4561 return "OperationDefinition"; 4562 case OPERATIONOUTCOME: 4563 return "OperationOutcome"; 4564 case ORDER: 4565 return "Order"; 4566 case ORDERRESPONSE: 4567 return "OrderResponse"; 4568 case ORGANIZATION: 4569 return "Organization"; 4570 case PARAMETERS: 4571 return "Parameters"; 4572 case PATIENT: 4573 return "Patient"; 4574 case PAYMENTNOTICE: 4575 return "PaymentNotice"; 4576 case PAYMENTRECONCILIATION: 4577 return "PaymentReconciliation"; 4578 case PERSON: 4579 return "Person"; 4580 case PRACTITIONER: 4581 return "Practitioner"; 4582 case PROCEDURE: 4583 return "Procedure"; 4584 case PROCEDUREREQUEST: 4585 return "ProcedureRequest"; 4586 case PROCESSREQUEST: 4587 return "ProcessRequest"; 4588 case PROCESSRESPONSE: 4589 return "ProcessResponse"; 4590 case PROVENANCE: 4591 return "Provenance"; 4592 case QUESTIONNAIRE: 4593 return "Questionnaire"; 4594 case QUESTIONNAIRERESPONSE: 4595 return "QuestionnaireResponse"; 4596 case REFERRALREQUEST: 4597 return "ReferralRequest"; 4598 case RELATEDPERSON: 4599 return "RelatedPerson"; 4600 case RESOURCE: 4601 return "Resource"; 4602 case RISKASSESSMENT: 4603 return "RiskAssessment"; 4604 case SCHEDULE: 4605 return "Schedule"; 4606 case SEARCHPARAMETER: 4607 return "SearchParameter"; 4608 case SLOT: 4609 return "Slot"; 4610 case SPECIMEN: 4611 return "Specimen"; 4612 case STRUCTUREDEFINITION: 4613 return "StructureDefinition"; 4614 case SUBSCRIPTION: 4615 return "Subscription"; 4616 case SUBSTANCE: 4617 return "Substance"; 4618 case SUPPLYDELIVERY: 4619 return "SupplyDelivery"; 4620 case SUPPLYREQUEST: 4621 return "SupplyRequest"; 4622 case TESTSCRIPT: 4623 return "TestScript"; 4624 case VALUESET: 4625 return "ValueSet"; 4626 case VISIONPRESCRIPTION: 4627 return "VisionPrescription"; 4628 case NULL: 4629 return null; 4630 default: 4631 return "?"; 4632 } 4633 } 4634 } 4635 4636 public static class FHIRDefinedTypeEnumFactory implements EnumFactory<FHIRDefinedType> { 4637 public FHIRDefinedType fromCode(String codeString) throws IllegalArgumentException { 4638 if (codeString == null || "".equals(codeString)) 4639 if (codeString == null || "".equals(codeString)) 4640 return null; 4641 if ("Address".equals(codeString)) 4642 return FHIRDefinedType.ADDRESS; 4643 if ("Age".equals(codeString)) 4644 return FHIRDefinedType.AGE; 4645 if ("Annotation".equals(codeString)) 4646 return FHIRDefinedType.ANNOTATION; 4647 if ("Attachment".equals(codeString)) 4648 return FHIRDefinedType.ATTACHMENT; 4649 if ("BackboneElement".equals(codeString)) 4650 return FHIRDefinedType.BACKBONEELEMENT; 4651 if ("CodeableConcept".equals(codeString)) 4652 return FHIRDefinedType.CODEABLECONCEPT; 4653 if ("Coding".equals(codeString)) 4654 return FHIRDefinedType.CODING; 4655 if ("ContactPoint".equals(codeString)) 4656 return FHIRDefinedType.CONTACTPOINT; 4657 if ("Count".equals(codeString)) 4658 return FHIRDefinedType.COUNT; 4659 if ("Distance".equals(codeString)) 4660 return FHIRDefinedType.DISTANCE; 4661 if ("Duration".equals(codeString)) 4662 return FHIRDefinedType.DURATION; 4663 if ("Element".equals(codeString)) 4664 return FHIRDefinedType.ELEMENT; 4665 if ("ElementDefinition".equals(codeString)) 4666 return FHIRDefinedType.ELEMENTDEFINITION; 4667 if ("Extension".equals(codeString)) 4668 return FHIRDefinedType.EXTENSION; 4669 if ("HumanName".equals(codeString)) 4670 return FHIRDefinedType.HUMANNAME; 4671 if ("Identifier".equals(codeString)) 4672 return FHIRDefinedType.IDENTIFIER; 4673 if ("Meta".equals(codeString)) 4674 return FHIRDefinedType.META; 4675 if ("Money".equals(codeString)) 4676 return FHIRDefinedType.MONEY; 4677 if ("Narrative".equals(codeString)) 4678 return FHIRDefinedType.NARRATIVE; 4679 if ("Period".equals(codeString)) 4680 return FHIRDefinedType.PERIOD; 4681 if ("Quantity".equals(codeString)) 4682 return FHIRDefinedType.QUANTITY; 4683 if ("Range".equals(codeString)) 4684 return FHIRDefinedType.RANGE; 4685 if ("Ratio".equals(codeString)) 4686 return FHIRDefinedType.RATIO; 4687 if ("Reference".equals(codeString)) 4688 return FHIRDefinedType.REFERENCE; 4689 if ("SampledData".equals(codeString)) 4690 return FHIRDefinedType.SAMPLEDDATA; 4691 if ("Signature".equals(codeString)) 4692 return FHIRDefinedType.SIGNATURE; 4693 if ("SimpleQuantity".equals(codeString)) 4694 return FHIRDefinedType.SIMPLEQUANTITY; 4695 if ("Timing".equals(codeString)) 4696 return FHIRDefinedType.TIMING; 4697 if ("base64Binary".equals(codeString)) 4698 return FHIRDefinedType.BASE64BINARY; 4699 if ("boolean".equals(codeString)) 4700 return FHIRDefinedType.BOOLEAN; 4701 if ("code".equals(codeString)) 4702 return FHIRDefinedType.CODE; 4703 if ("date".equals(codeString)) 4704 return FHIRDefinedType.DATE; 4705 if ("dateTime".equals(codeString)) 4706 return FHIRDefinedType.DATETIME; 4707 if ("decimal".equals(codeString)) 4708 return FHIRDefinedType.DECIMAL; 4709 if ("id".equals(codeString)) 4710 return FHIRDefinedType.ID; 4711 if ("instant".equals(codeString)) 4712 return FHIRDefinedType.INSTANT; 4713 if ("integer".equals(codeString)) 4714 return FHIRDefinedType.INTEGER; 4715 if ("markdown".equals(codeString)) 4716 return FHIRDefinedType.MARKDOWN; 4717 if ("oid".equals(codeString)) 4718 return FHIRDefinedType.OID; 4719 if ("positiveInt".equals(codeString)) 4720 return FHIRDefinedType.POSITIVEINT; 4721 if ("string".equals(codeString)) 4722 return FHIRDefinedType.STRING; 4723 if ("time".equals(codeString)) 4724 return FHIRDefinedType.TIME; 4725 if ("unsignedInt".equals(codeString)) 4726 return FHIRDefinedType.UNSIGNEDINT; 4727 if ("uri".equals(codeString)) 4728 return FHIRDefinedType.URI; 4729 if ("uuid".equals(codeString)) 4730 return FHIRDefinedType.UUID; 4731 if ("xhtml".equals(codeString)) 4732 return FHIRDefinedType.XHTML; 4733 if ("Account".equals(codeString)) 4734 return FHIRDefinedType.ACCOUNT; 4735 if ("AllergyIntolerance".equals(codeString)) 4736 return FHIRDefinedType.ALLERGYINTOLERANCE; 4737 if ("Appointment".equals(codeString)) 4738 return FHIRDefinedType.APPOINTMENT; 4739 if ("AppointmentResponse".equals(codeString)) 4740 return FHIRDefinedType.APPOINTMENTRESPONSE; 4741 if ("AuditEvent".equals(codeString)) 4742 return FHIRDefinedType.AUDITEVENT; 4743 if ("Basic".equals(codeString)) 4744 return FHIRDefinedType.BASIC; 4745 if ("Binary".equals(codeString)) 4746 return FHIRDefinedType.BINARY; 4747 if ("BodySite".equals(codeString)) 4748 return FHIRDefinedType.BODYSITE; 4749 if ("Bundle".equals(codeString)) 4750 return FHIRDefinedType.BUNDLE; 4751 if ("CarePlan".equals(codeString)) 4752 return FHIRDefinedType.CAREPLAN; 4753 if ("Claim".equals(codeString)) 4754 return FHIRDefinedType.CLAIM; 4755 if ("ClaimResponse".equals(codeString)) 4756 return FHIRDefinedType.CLAIMRESPONSE; 4757 if ("ClinicalImpression".equals(codeString)) 4758 return FHIRDefinedType.CLINICALIMPRESSION; 4759 if ("Communication".equals(codeString)) 4760 return FHIRDefinedType.COMMUNICATION; 4761 if ("CommunicationRequest".equals(codeString)) 4762 return FHIRDefinedType.COMMUNICATIONREQUEST; 4763 if ("Composition".equals(codeString)) 4764 return FHIRDefinedType.COMPOSITION; 4765 if ("ConceptMap".equals(codeString)) 4766 return FHIRDefinedType.CONCEPTMAP; 4767 if ("Condition".equals(codeString)) 4768 return FHIRDefinedType.CONDITION; 4769 if ("Conformance".equals(codeString)) 4770 return FHIRDefinedType.CONFORMANCE; 4771 if ("Contract".equals(codeString)) 4772 return FHIRDefinedType.CONTRACT; 4773 if ("Coverage".equals(codeString)) 4774 return FHIRDefinedType.COVERAGE; 4775 if ("DataElement".equals(codeString)) 4776 return FHIRDefinedType.DATAELEMENT; 4777 if ("DetectedIssue".equals(codeString)) 4778 return FHIRDefinedType.DETECTEDISSUE; 4779 if ("Device".equals(codeString)) 4780 return FHIRDefinedType.DEVICE; 4781 if ("DeviceComponent".equals(codeString)) 4782 return FHIRDefinedType.DEVICECOMPONENT; 4783 if ("DeviceMetric".equals(codeString)) 4784 return FHIRDefinedType.DEVICEMETRIC; 4785 if ("DeviceUseRequest".equals(codeString)) 4786 return FHIRDefinedType.DEVICEUSEREQUEST; 4787 if ("DeviceUseStatement".equals(codeString)) 4788 return FHIRDefinedType.DEVICEUSESTATEMENT; 4789 if ("DiagnosticOrder".equals(codeString)) 4790 return FHIRDefinedType.DIAGNOSTICORDER; 4791 if ("DiagnosticReport".equals(codeString)) 4792 return FHIRDefinedType.DIAGNOSTICREPORT; 4793 if ("DocumentManifest".equals(codeString)) 4794 return FHIRDefinedType.DOCUMENTMANIFEST; 4795 if ("DocumentReference".equals(codeString)) 4796 return FHIRDefinedType.DOCUMENTREFERENCE; 4797 if ("DomainResource".equals(codeString)) 4798 return FHIRDefinedType.DOMAINRESOURCE; 4799 if ("EligibilityRequest".equals(codeString)) 4800 return FHIRDefinedType.ELIGIBILITYREQUEST; 4801 if ("EligibilityResponse".equals(codeString)) 4802 return FHIRDefinedType.ELIGIBILITYRESPONSE; 4803 if ("Encounter".equals(codeString)) 4804 return FHIRDefinedType.ENCOUNTER; 4805 if ("EnrollmentRequest".equals(codeString)) 4806 return FHIRDefinedType.ENROLLMENTREQUEST; 4807 if ("EnrollmentResponse".equals(codeString)) 4808 return FHIRDefinedType.ENROLLMENTRESPONSE; 4809 if ("EpisodeOfCare".equals(codeString)) 4810 return FHIRDefinedType.EPISODEOFCARE; 4811 if ("ExplanationOfBenefit".equals(codeString)) 4812 return FHIRDefinedType.EXPLANATIONOFBENEFIT; 4813 if ("FamilyMemberHistory".equals(codeString)) 4814 return FHIRDefinedType.FAMILYMEMBERHISTORY; 4815 if ("Flag".equals(codeString)) 4816 return FHIRDefinedType.FLAG; 4817 if ("Goal".equals(codeString)) 4818 return FHIRDefinedType.GOAL; 4819 if ("Group".equals(codeString)) 4820 return FHIRDefinedType.GROUP; 4821 if ("HealthcareService".equals(codeString)) 4822 return FHIRDefinedType.HEALTHCARESERVICE; 4823 if ("ImagingObjectSelection".equals(codeString)) 4824 return FHIRDefinedType.IMAGINGOBJECTSELECTION; 4825 if ("ImagingStudy".equals(codeString)) 4826 return FHIRDefinedType.IMAGINGSTUDY; 4827 if ("Immunization".equals(codeString)) 4828 return FHIRDefinedType.IMMUNIZATION; 4829 if ("ImmunizationRecommendation".equals(codeString)) 4830 return FHIRDefinedType.IMMUNIZATIONRECOMMENDATION; 4831 if ("ImplementationGuide".equals(codeString)) 4832 return FHIRDefinedType.IMPLEMENTATIONGUIDE; 4833 if ("List".equals(codeString)) 4834 return FHIRDefinedType.LIST; 4835 if ("Location".equals(codeString)) 4836 return FHIRDefinedType.LOCATION; 4837 if ("Media".equals(codeString)) 4838 return FHIRDefinedType.MEDIA; 4839 if ("Medication".equals(codeString)) 4840 return FHIRDefinedType.MEDICATION; 4841 if ("MedicationAdministration".equals(codeString)) 4842 return FHIRDefinedType.MEDICATIONADMINISTRATION; 4843 if ("MedicationDispense".equals(codeString)) 4844 return FHIRDefinedType.MEDICATIONDISPENSE; 4845 if ("MedicationOrder".equals(codeString)) 4846 return FHIRDefinedType.MEDICATIONORDER; 4847 if ("MedicationStatement".equals(codeString)) 4848 return FHIRDefinedType.MEDICATIONSTATEMENT; 4849 if ("MessageHeader".equals(codeString)) 4850 return FHIRDefinedType.MESSAGEHEADER; 4851 if ("NamingSystem".equals(codeString)) 4852 return FHIRDefinedType.NAMINGSYSTEM; 4853 if ("NutritionOrder".equals(codeString)) 4854 return FHIRDefinedType.NUTRITIONORDER; 4855 if ("Observation".equals(codeString)) 4856 return FHIRDefinedType.OBSERVATION; 4857 if ("OperationDefinition".equals(codeString)) 4858 return FHIRDefinedType.OPERATIONDEFINITION; 4859 if ("OperationOutcome".equals(codeString)) 4860 return FHIRDefinedType.OPERATIONOUTCOME; 4861 if ("Order".equals(codeString)) 4862 return FHIRDefinedType.ORDER; 4863 if ("OrderResponse".equals(codeString)) 4864 return FHIRDefinedType.ORDERRESPONSE; 4865 if ("Organization".equals(codeString)) 4866 return FHIRDefinedType.ORGANIZATION; 4867 if ("Parameters".equals(codeString)) 4868 return FHIRDefinedType.PARAMETERS; 4869 if ("Patient".equals(codeString)) 4870 return FHIRDefinedType.PATIENT; 4871 if ("PaymentNotice".equals(codeString)) 4872 return FHIRDefinedType.PAYMENTNOTICE; 4873 if ("PaymentReconciliation".equals(codeString)) 4874 return FHIRDefinedType.PAYMENTRECONCILIATION; 4875 if ("Person".equals(codeString)) 4876 return FHIRDefinedType.PERSON; 4877 if ("Practitioner".equals(codeString)) 4878 return FHIRDefinedType.PRACTITIONER; 4879 if ("Procedure".equals(codeString)) 4880 return FHIRDefinedType.PROCEDURE; 4881 if ("ProcedureRequest".equals(codeString)) 4882 return FHIRDefinedType.PROCEDUREREQUEST; 4883 if ("ProcessRequest".equals(codeString)) 4884 return FHIRDefinedType.PROCESSREQUEST; 4885 if ("ProcessResponse".equals(codeString)) 4886 return FHIRDefinedType.PROCESSRESPONSE; 4887 if ("Provenance".equals(codeString)) 4888 return FHIRDefinedType.PROVENANCE; 4889 if ("Questionnaire".equals(codeString)) 4890 return FHIRDefinedType.QUESTIONNAIRE; 4891 if ("QuestionnaireResponse".equals(codeString)) 4892 return FHIRDefinedType.QUESTIONNAIRERESPONSE; 4893 if ("ReferralRequest".equals(codeString)) 4894 return FHIRDefinedType.REFERRALREQUEST; 4895 if ("RelatedPerson".equals(codeString)) 4896 return FHIRDefinedType.RELATEDPERSON; 4897 if ("Resource".equals(codeString)) 4898 return FHIRDefinedType.RESOURCE; 4899 if ("RiskAssessment".equals(codeString)) 4900 return FHIRDefinedType.RISKASSESSMENT; 4901 if ("Schedule".equals(codeString)) 4902 return FHIRDefinedType.SCHEDULE; 4903 if ("SearchParameter".equals(codeString)) 4904 return FHIRDefinedType.SEARCHPARAMETER; 4905 if ("Slot".equals(codeString)) 4906 return FHIRDefinedType.SLOT; 4907 if ("Specimen".equals(codeString)) 4908 return FHIRDefinedType.SPECIMEN; 4909 if ("StructureDefinition".equals(codeString)) 4910 return FHIRDefinedType.STRUCTUREDEFINITION; 4911 if ("Subscription".equals(codeString)) 4912 return FHIRDefinedType.SUBSCRIPTION; 4913 if ("Substance".equals(codeString)) 4914 return FHIRDefinedType.SUBSTANCE; 4915 if ("SupplyDelivery".equals(codeString)) 4916 return FHIRDefinedType.SUPPLYDELIVERY; 4917 if ("SupplyRequest".equals(codeString)) 4918 return FHIRDefinedType.SUPPLYREQUEST; 4919 if ("TestScript".equals(codeString)) 4920 return FHIRDefinedType.TESTSCRIPT; 4921 if ("ValueSet".equals(codeString)) 4922 return FHIRDefinedType.VALUESET; 4923 if ("VisionPrescription".equals(codeString)) 4924 return FHIRDefinedType.VISIONPRESCRIPTION; 4925 throw new IllegalArgumentException("Unknown FHIRDefinedType code '" + codeString + "'"); 4926 } 4927 4928 public Enumeration<FHIRDefinedType> fromType(Base code) throws FHIRException { 4929 if (code == null || code.isEmpty()) 4930 return null; 4931 String codeString = ((PrimitiveType) code).asStringValue(); 4932 if (codeString == null || "".equals(codeString)) 4933 return null; 4934 if ("Address".equals(codeString)) 4935 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ADDRESS); 4936 if ("Age".equals(codeString)) 4937 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.AGE); 4938 if ("Annotation".equals(codeString)) 4939 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ANNOTATION); 4940 if ("Attachment".equals(codeString)) 4941 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ATTACHMENT); 4942 if ("BackboneElement".equals(codeString)) 4943 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BACKBONEELEMENT); 4944 if ("CodeableConcept".equals(codeString)) 4945 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODEABLECONCEPT); 4946 if ("Coding".equals(codeString)) 4947 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODING); 4948 if ("ContactPoint".equals(codeString)) 4949 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTACTPOINT); 4950 if ("Count".equals(codeString)) 4951 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COUNT); 4952 if ("Distance".equals(codeString)) 4953 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DISTANCE); 4954 if ("Duration".equals(codeString)) 4955 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DURATION); 4956 if ("Element".equals(codeString)) 4957 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ELEMENT); 4958 if ("ElementDefinition".equals(codeString)) 4959 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ELEMENTDEFINITION); 4960 if ("Extension".equals(codeString)) 4961 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EXTENSION); 4962 if ("HumanName".equals(codeString)) 4963 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.HUMANNAME); 4964 if ("Identifier".equals(codeString)) 4965 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IDENTIFIER); 4966 if ("Meta".equals(codeString)) 4967 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.META); 4968 if ("Money".equals(codeString)) 4969 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MONEY); 4970 if ("Narrative".equals(codeString)) 4971 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NARRATIVE); 4972 if ("Period".equals(codeString)) 4973 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PERIOD); 4974 if ("Quantity".equals(codeString)) 4975 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.QUANTITY); 4976 if ("Range".equals(codeString)) 4977 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RANGE); 4978 if ("Ratio".equals(codeString)) 4979 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RATIO); 4980 if ("Reference".equals(codeString)) 4981 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.REFERENCE); 4982 if ("SampledData".equals(codeString)) 4983 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SAMPLEDDATA); 4984 if ("Signature".equals(codeString)) 4985 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SIGNATURE); 4986 if ("SimpleQuantity".equals(codeString)) 4987 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SIMPLEQUANTITY); 4988 if ("Timing".equals(codeString)) 4989 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TIMING); 4990 if ("base64Binary".equals(codeString)) 4991 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BASE64BINARY); 4992 if ("boolean".equals(codeString)) 4993 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BOOLEAN); 4994 if ("code".equals(codeString)) 4995 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODE); 4996 if ("date".equals(codeString)) 4997 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATE); 4998 if ("dateTime".equals(codeString)) 4999 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATETIME); 5000 if ("decimal".equals(codeString)) 5001 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DECIMAL); 5002 if ("id".equals(codeString)) 5003 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ID); 5004 if ("instant".equals(codeString)) 5005 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.INSTANT); 5006 if ("integer".equals(codeString)) 5007 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.INTEGER); 5008 if ("markdown".equals(codeString)) 5009 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MARKDOWN); 5010 if ("oid".equals(codeString)) 5011 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OID); 5012 if ("positiveInt".equals(codeString)) 5013 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.POSITIVEINT); 5014 if ("string".equals(codeString)) 5015 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.STRING); 5016 if ("time".equals(codeString)) 5017 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TIME); 5018 if ("unsignedInt".equals(codeString)) 5019 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.UNSIGNEDINT); 5020 if ("uri".equals(codeString)) 5021 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.URI); 5022 if ("uuid".equals(codeString)) 5023 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.UUID); 5024 if ("xhtml".equals(codeString)) 5025 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.XHTML); 5026 if ("Account".equals(codeString)) 5027 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ACCOUNT); 5028 if ("AllergyIntolerance".equals(codeString)) 5029 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ALLERGYINTOLERANCE); 5030 if ("Appointment".equals(codeString)) 5031 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.APPOINTMENT); 5032 if ("AppointmentResponse".equals(codeString)) 5033 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.APPOINTMENTRESPONSE); 5034 if ("AuditEvent".equals(codeString)) 5035 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.AUDITEVENT); 5036 if ("Basic".equals(codeString)) 5037 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BASIC); 5038 if ("Binary".equals(codeString)) 5039 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BINARY); 5040 if ("BodySite".equals(codeString)) 5041 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BODYSITE); 5042 if ("Bundle".equals(codeString)) 5043 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BUNDLE); 5044 if ("CarePlan".equals(codeString)) 5045 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CAREPLAN); 5046 if ("Claim".equals(codeString)) 5047 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CLAIM); 5048 if ("ClaimResponse".equals(codeString)) 5049 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CLAIMRESPONSE); 5050 if ("ClinicalImpression".equals(codeString)) 5051 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CLINICALIMPRESSION); 5052 if ("Communication".equals(codeString)) 5053 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMMUNICATION); 5054 if ("CommunicationRequest".equals(codeString)) 5055 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMMUNICATIONREQUEST); 5056 if ("Composition".equals(codeString)) 5057 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMPOSITION); 5058 if ("ConceptMap".equals(codeString)) 5059 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONCEPTMAP); 5060 if ("Condition".equals(codeString)) 5061 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONDITION); 5062 if ("Conformance".equals(codeString)) 5063 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONFORMANCE); 5064 if ("Contract".equals(codeString)) 5065 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTRACT); 5066 if ("Coverage".equals(codeString)) 5067 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COVERAGE); 5068 if ("DataElement".equals(codeString)) 5069 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATAELEMENT); 5070 if ("DetectedIssue".equals(codeString)) 5071 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DETECTEDISSUE); 5072 if ("Device".equals(codeString)) 5073 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICE); 5074 if ("DeviceComponent".equals(codeString)) 5075 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICECOMPONENT); 5076 if ("DeviceMetric".equals(codeString)) 5077 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEMETRIC); 5078 if ("DeviceUseRequest".equals(codeString)) 5079 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEUSEREQUEST); 5080 if ("DeviceUseStatement".equals(codeString)) 5081 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEUSESTATEMENT); 5082 if ("DiagnosticOrder".equals(codeString)) 5083 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DIAGNOSTICORDER); 5084 if ("DiagnosticReport".equals(codeString)) 5085 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DIAGNOSTICREPORT); 5086 if ("DocumentManifest".equals(codeString)) 5087 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOCUMENTMANIFEST); 5088 if ("DocumentReference".equals(codeString)) 5089 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOCUMENTREFERENCE); 5090 if ("DomainResource".equals(codeString)) 5091 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOMAINRESOURCE); 5092 if ("EligibilityRequest".equals(codeString)) 5093 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ELIGIBILITYREQUEST); 5094 if ("EligibilityResponse".equals(codeString)) 5095 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ELIGIBILITYRESPONSE); 5096 if ("Encounter".equals(codeString)) 5097 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENCOUNTER); 5098 if ("EnrollmentRequest".equals(codeString)) 5099 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENROLLMENTREQUEST); 5100 if ("EnrollmentResponse".equals(codeString)) 5101 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENROLLMENTRESPONSE); 5102 if ("EpisodeOfCare".equals(codeString)) 5103 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EPISODEOFCARE); 5104 if ("ExplanationOfBenefit".equals(codeString)) 5105 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EXPLANATIONOFBENEFIT); 5106 if ("FamilyMemberHistory".equals(codeString)) 5107 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.FAMILYMEMBERHISTORY); 5108 if ("Flag".equals(codeString)) 5109 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.FLAG); 5110 if ("Goal".equals(codeString)) 5111 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GOAL); 5112 if ("Group".equals(codeString)) 5113 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GROUP); 5114 if ("HealthcareService".equals(codeString)) 5115 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.HEALTHCARESERVICE); 5116 if ("ImagingObjectSelection".equals(codeString)) 5117 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMAGINGOBJECTSELECTION); 5118 if ("ImagingStudy".equals(codeString)) 5119 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMAGINGSTUDY); 5120 if ("Immunization".equals(codeString)) 5121 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMMUNIZATION); 5122 if ("ImmunizationRecommendation".equals(codeString)) 5123 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMMUNIZATIONRECOMMENDATION); 5124 if ("ImplementationGuide".equals(codeString)) 5125 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMPLEMENTATIONGUIDE); 5126 if ("List".equals(codeString)) 5127 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LIST); 5128 if ("Location".equals(codeString)) 5129 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LOCATION); 5130 if ("Media".equals(codeString)) 5131 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDIA); 5132 if ("Medication".equals(codeString)) 5133 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATION); 5134 if ("MedicationAdministration".equals(codeString)) 5135 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONADMINISTRATION); 5136 if ("MedicationDispense".equals(codeString)) 5137 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONDISPENSE); 5138 if ("MedicationOrder".equals(codeString)) 5139 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONORDER); 5140 if ("MedicationStatement".equals(codeString)) 5141 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONSTATEMENT); 5142 if ("MessageHeader".equals(codeString)) 5143 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MESSAGEHEADER); 5144 if ("NamingSystem".equals(codeString)) 5145 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NAMINGSYSTEM); 5146 if ("NutritionOrder".equals(codeString)) 5147 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NUTRITIONORDER); 5148 if ("Observation".equals(codeString)) 5149 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OBSERVATION); 5150 if ("OperationDefinition".equals(codeString)) 5151 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OPERATIONDEFINITION); 5152 if ("OperationOutcome".equals(codeString)) 5153 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OPERATIONOUTCOME); 5154 if ("Order".equals(codeString)) 5155 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ORDER); 5156 if ("OrderResponse".equals(codeString)) 5157 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ORDERRESPONSE); 5158 if ("Organization".equals(codeString)) 5159 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ORGANIZATION); 5160 if ("Parameters".equals(codeString)) 5161 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PARAMETERS); 5162 if ("Patient".equals(codeString)) 5163 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PATIENT); 5164 if ("PaymentNotice".equals(codeString)) 5165 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PAYMENTNOTICE); 5166 if ("PaymentReconciliation".equals(codeString)) 5167 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PAYMENTRECONCILIATION); 5168 if ("Person".equals(codeString)) 5169 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PERSON); 5170 if ("Practitioner".equals(codeString)) 5171 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PRACTITIONER); 5172 if ("Procedure".equals(codeString)) 5173 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROCEDURE); 5174 if ("ProcedureRequest".equals(codeString)) 5175 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROCEDUREREQUEST); 5176 if ("ProcessRequest".equals(codeString)) 5177 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROCESSREQUEST); 5178 if ("ProcessResponse".equals(codeString)) 5179 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROCESSRESPONSE); 5180 if ("Provenance".equals(codeString)) 5181 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROVENANCE); 5182 if ("Questionnaire".equals(codeString)) 5183 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.QUESTIONNAIRE); 5184 if ("QuestionnaireResponse".equals(codeString)) 5185 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.QUESTIONNAIRERESPONSE); 5186 if ("ReferralRequest".equals(codeString)) 5187 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.REFERRALREQUEST); 5188 if ("RelatedPerson".equals(codeString)) 5189 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RELATEDPERSON); 5190 if ("Resource".equals(codeString)) 5191 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESOURCE); 5192 if ("RiskAssessment".equals(codeString)) 5193 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RISKASSESSMENT); 5194 if ("Schedule".equals(codeString)) 5195 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SCHEDULE); 5196 if ("SearchParameter".equals(codeString)) 5197 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SEARCHPARAMETER); 5198 if ("Slot".equals(codeString)) 5199 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SLOT); 5200 if ("Specimen".equals(codeString)) 5201 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SPECIMEN); 5202 if ("StructureDefinition".equals(codeString)) 5203 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.STRUCTUREDEFINITION); 5204 if ("Subscription".equals(codeString)) 5205 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSCRIPTION); 5206 if ("Substance".equals(codeString)) 5207 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCE); 5208 if ("SupplyDelivery".equals(codeString)) 5209 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUPPLYDELIVERY); 5210 if ("SupplyRequest".equals(codeString)) 5211 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUPPLYREQUEST); 5212 if ("TestScript".equals(codeString)) 5213 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TESTSCRIPT); 5214 if ("ValueSet".equals(codeString)) 5215 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.VALUESET); 5216 if ("VisionPrescription".equals(codeString)) 5217 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.VISIONPRESCRIPTION); 5218 throw new FHIRException("Unknown FHIRDefinedType code '" + codeString + "'"); 5219 } 5220 5221 public String toCode(FHIRDefinedType code) 5222 { 5223 if (code == FHIRDefinedType.NULL) 5224 return null; 5225 if (code == FHIRDefinedType.ADDRESS) 5226 return "Address"; 5227 if (code == FHIRDefinedType.AGE) 5228 return "Age"; 5229 if (code == FHIRDefinedType.ANNOTATION) 5230 return "Annotation"; 5231 if (code == FHIRDefinedType.ATTACHMENT) 5232 return "Attachment"; 5233 if (code == FHIRDefinedType.BACKBONEELEMENT) 5234 return "BackboneElement"; 5235 if (code == FHIRDefinedType.CODEABLECONCEPT) 5236 return "CodeableConcept"; 5237 if (code == FHIRDefinedType.CODING) 5238 return "Coding"; 5239 if (code == FHIRDefinedType.CONTACTPOINT) 5240 return "ContactPoint"; 5241 if (code == FHIRDefinedType.COUNT) 5242 return "Count"; 5243 if (code == FHIRDefinedType.DISTANCE) 5244 return "Distance"; 5245 if (code == FHIRDefinedType.DURATION) 5246 return "Duration"; 5247 if (code == FHIRDefinedType.ELEMENT) 5248 return "Element"; 5249 if (code == FHIRDefinedType.ELEMENTDEFINITION) 5250 return "ElementDefinition"; 5251 if (code == FHIRDefinedType.EXTENSION) 5252 return "Extension"; 5253 if (code == FHIRDefinedType.HUMANNAME) 5254 return "HumanName"; 5255 if (code == FHIRDefinedType.IDENTIFIER) 5256 return "Identifier"; 5257 if (code == FHIRDefinedType.META) 5258 return "Meta"; 5259 if (code == FHIRDefinedType.MONEY) 5260 return "Money"; 5261 if (code == FHIRDefinedType.NARRATIVE) 5262 return "Narrative"; 5263 if (code == FHIRDefinedType.PERIOD) 5264 return "Period"; 5265 if (code == FHIRDefinedType.QUANTITY) 5266 return "Quantity"; 5267 if (code == FHIRDefinedType.RANGE) 5268 return "Range"; 5269 if (code == FHIRDefinedType.RATIO) 5270 return "Ratio"; 5271 if (code == FHIRDefinedType.REFERENCE) 5272 return "Reference"; 5273 if (code == FHIRDefinedType.SAMPLEDDATA) 5274 return "SampledData"; 5275 if (code == FHIRDefinedType.SIGNATURE) 5276 return "Signature"; 5277 if (code == FHIRDefinedType.SIMPLEQUANTITY) 5278 return "SimpleQuantity"; 5279 if (code == FHIRDefinedType.TIMING) 5280 return "Timing"; 5281 if (code == FHIRDefinedType.BASE64BINARY) 5282 return "base64Binary"; 5283 if (code == FHIRDefinedType.BOOLEAN) 5284 return "boolean"; 5285 if (code == FHIRDefinedType.CODE) 5286 return "code"; 5287 if (code == FHIRDefinedType.DATE) 5288 return "date"; 5289 if (code == FHIRDefinedType.DATETIME) 5290 return "dateTime"; 5291 if (code == FHIRDefinedType.DECIMAL) 5292 return "decimal"; 5293 if (code == FHIRDefinedType.ID) 5294 return "id"; 5295 if (code == FHIRDefinedType.INSTANT) 5296 return "instant"; 5297 if (code == FHIRDefinedType.INTEGER) 5298 return "integer"; 5299 if (code == FHIRDefinedType.MARKDOWN) 5300 return "markdown"; 5301 if (code == FHIRDefinedType.OID) 5302 return "oid"; 5303 if (code == FHIRDefinedType.POSITIVEINT) 5304 return "positiveInt"; 5305 if (code == FHIRDefinedType.STRING) 5306 return "string"; 5307 if (code == FHIRDefinedType.TIME) 5308 return "time"; 5309 if (code == FHIRDefinedType.UNSIGNEDINT) 5310 return "unsignedInt"; 5311 if (code == FHIRDefinedType.URI) 5312 return "uri"; 5313 if (code == FHIRDefinedType.UUID) 5314 return "uuid"; 5315 if (code == FHIRDefinedType.XHTML) 5316 return "xhtml"; 5317 if (code == FHIRDefinedType.ACCOUNT) 5318 return "Account"; 5319 if (code == FHIRDefinedType.ALLERGYINTOLERANCE) 5320 return "AllergyIntolerance"; 5321 if (code == FHIRDefinedType.APPOINTMENT) 5322 return "Appointment"; 5323 if (code == FHIRDefinedType.APPOINTMENTRESPONSE) 5324 return "AppointmentResponse"; 5325 if (code == FHIRDefinedType.AUDITEVENT) 5326 return "AuditEvent"; 5327 if (code == FHIRDefinedType.BASIC) 5328 return "Basic"; 5329 if (code == FHIRDefinedType.BINARY) 5330 return "Binary"; 5331 if (code == FHIRDefinedType.BODYSITE) 5332 return "BodySite"; 5333 if (code == FHIRDefinedType.BUNDLE) 5334 return "Bundle"; 5335 if (code == FHIRDefinedType.CAREPLAN) 5336 return "CarePlan"; 5337 if (code == FHIRDefinedType.CLAIM) 5338 return "Claim"; 5339 if (code == FHIRDefinedType.CLAIMRESPONSE) 5340 return "ClaimResponse"; 5341 if (code == FHIRDefinedType.CLINICALIMPRESSION) 5342 return "ClinicalImpression"; 5343 if (code == FHIRDefinedType.COMMUNICATION) 5344 return "Communication"; 5345 if (code == FHIRDefinedType.COMMUNICATIONREQUEST) 5346 return "CommunicationRequest"; 5347 if (code == FHIRDefinedType.COMPOSITION) 5348 return "Composition"; 5349 if (code == FHIRDefinedType.CONCEPTMAP) 5350 return "ConceptMap"; 5351 if (code == FHIRDefinedType.CONDITION) 5352 return "Condition"; 5353 if (code == FHIRDefinedType.CONFORMANCE) 5354 return "Conformance"; 5355 if (code == FHIRDefinedType.CONTRACT) 5356 return "Contract"; 5357 if (code == FHIRDefinedType.COVERAGE) 5358 return "Coverage"; 5359 if (code == FHIRDefinedType.DATAELEMENT) 5360 return "DataElement"; 5361 if (code == FHIRDefinedType.DETECTEDISSUE) 5362 return "DetectedIssue"; 5363 if (code == FHIRDefinedType.DEVICE) 5364 return "Device"; 5365 if (code == FHIRDefinedType.DEVICECOMPONENT) 5366 return "DeviceComponent"; 5367 if (code == FHIRDefinedType.DEVICEMETRIC) 5368 return "DeviceMetric"; 5369 if (code == FHIRDefinedType.DEVICEUSEREQUEST) 5370 return "DeviceUseRequest"; 5371 if (code == FHIRDefinedType.DEVICEUSESTATEMENT) 5372 return "DeviceUseStatement"; 5373 if (code == FHIRDefinedType.DIAGNOSTICORDER) 5374 return "DiagnosticOrder"; 5375 if (code == FHIRDefinedType.DIAGNOSTICREPORT) 5376 return "DiagnosticReport"; 5377 if (code == FHIRDefinedType.DOCUMENTMANIFEST) 5378 return "DocumentManifest"; 5379 if (code == FHIRDefinedType.DOCUMENTREFERENCE) 5380 return "DocumentReference"; 5381 if (code == FHIRDefinedType.DOMAINRESOURCE) 5382 return "DomainResource"; 5383 if (code == FHIRDefinedType.ELIGIBILITYREQUEST) 5384 return "EligibilityRequest"; 5385 if (code == FHIRDefinedType.ELIGIBILITYRESPONSE) 5386 return "EligibilityResponse"; 5387 if (code == FHIRDefinedType.ENCOUNTER) 5388 return "Encounter"; 5389 if (code == FHIRDefinedType.ENROLLMENTREQUEST) 5390 return "EnrollmentRequest"; 5391 if (code == FHIRDefinedType.ENROLLMENTRESPONSE) 5392 return "EnrollmentResponse"; 5393 if (code == FHIRDefinedType.EPISODEOFCARE) 5394 return "EpisodeOfCare"; 5395 if (code == FHIRDefinedType.EXPLANATIONOFBENEFIT) 5396 return "ExplanationOfBenefit"; 5397 if (code == FHIRDefinedType.FAMILYMEMBERHISTORY) 5398 return "FamilyMemberHistory"; 5399 if (code == FHIRDefinedType.FLAG) 5400 return "Flag"; 5401 if (code == FHIRDefinedType.GOAL) 5402 return "Goal"; 5403 if (code == FHIRDefinedType.GROUP) 5404 return "Group"; 5405 if (code == FHIRDefinedType.HEALTHCARESERVICE) 5406 return "HealthcareService"; 5407 if (code == FHIRDefinedType.IMAGINGOBJECTSELECTION) 5408 return "ImagingObjectSelection"; 5409 if (code == FHIRDefinedType.IMAGINGSTUDY) 5410 return "ImagingStudy"; 5411 if (code == FHIRDefinedType.IMMUNIZATION) 5412 return "Immunization"; 5413 if (code == FHIRDefinedType.IMMUNIZATIONRECOMMENDATION) 5414 return "ImmunizationRecommendation"; 5415 if (code == FHIRDefinedType.IMPLEMENTATIONGUIDE) 5416 return "ImplementationGuide"; 5417 if (code == FHIRDefinedType.LIST) 5418 return "List"; 5419 if (code == FHIRDefinedType.LOCATION) 5420 return "Location"; 5421 if (code == FHIRDefinedType.MEDIA) 5422 return "Media"; 5423 if (code == FHIRDefinedType.MEDICATION) 5424 return "Medication"; 5425 if (code == FHIRDefinedType.MEDICATIONADMINISTRATION) 5426 return "MedicationAdministration"; 5427 if (code == FHIRDefinedType.MEDICATIONDISPENSE) 5428 return "MedicationDispense"; 5429 if (code == FHIRDefinedType.MEDICATIONORDER) 5430 return "MedicationOrder"; 5431 if (code == FHIRDefinedType.MEDICATIONSTATEMENT) 5432 return "MedicationStatement"; 5433 if (code == FHIRDefinedType.MESSAGEHEADER) 5434 return "MessageHeader"; 5435 if (code == FHIRDefinedType.NAMINGSYSTEM) 5436 return "NamingSystem"; 5437 if (code == FHIRDefinedType.NUTRITIONORDER) 5438 return "NutritionOrder"; 5439 if (code == FHIRDefinedType.OBSERVATION) 5440 return "Observation"; 5441 if (code == FHIRDefinedType.OPERATIONDEFINITION) 5442 return "OperationDefinition"; 5443 if (code == FHIRDefinedType.OPERATIONOUTCOME) 5444 return "OperationOutcome"; 5445 if (code == FHIRDefinedType.ORDER) 5446 return "Order"; 5447 if (code == FHIRDefinedType.ORDERRESPONSE) 5448 return "OrderResponse"; 5449 if (code == FHIRDefinedType.ORGANIZATION) 5450 return "Organization"; 5451 if (code == FHIRDefinedType.PARAMETERS) 5452 return "Parameters"; 5453 if (code == FHIRDefinedType.PATIENT) 5454 return "Patient"; 5455 if (code == FHIRDefinedType.PAYMENTNOTICE) 5456 return "PaymentNotice"; 5457 if (code == FHIRDefinedType.PAYMENTRECONCILIATION) 5458 return "PaymentReconciliation"; 5459 if (code == FHIRDefinedType.PERSON) 5460 return "Person"; 5461 if (code == FHIRDefinedType.PRACTITIONER) 5462 return "Practitioner"; 5463 if (code == FHIRDefinedType.PROCEDURE) 5464 return "Procedure"; 5465 if (code == FHIRDefinedType.PROCEDUREREQUEST) 5466 return "ProcedureRequest"; 5467 if (code == FHIRDefinedType.PROCESSREQUEST) 5468 return "ProcessRequest"; 5469 if (code == FHIRDefinedType.PROCESSRESPONSE) 5470 return "ProcessResponse"; 5471 if (code == FHIRDefinedType.PROVENANCE) 5472 return "Provenance"; 5473 if (code == FHIRDefinedType.QUESTIONNAIRE) 5474 return "Questionnaire"; 5475 if (code == FHIRDefinedType.QUESTIONNAIRERESPONSE) 5476 return "QuestionnaireResponse"; 5477 if (code == FHIRDefinedType.REFERRALREQUEST) 5478 return "ReferralRequest"; 5479 if (code == FHIRDefinedType.RELATEDPERSON) 5480 return "RelatedPerson"; 5481 if (code == FHIRDefinedType.RESOURCE) 5482 return "Resource"; 5483 if (code == FHIRDefinedType.RISKASSESSMENT) 5484 return "RiskAssessment"; 5485 if (code == FHIRDefinedType.SCHEDULE) 5486 return "Schedule"; 5487 if (code == FHIRDefinedType.SEARCHPARAMETER) 5488 return "SearchParameter"; 5489 if (code == FHIRDefinedType.SLOT) 5490 return "Slot"; 5491 if (code == FHIRDefinedType.SPECIMEN) 5492 return "Specimen"; 5493 if (code == FHIRDefinedType.STRUCTUREDEFINITION) 5494 return "StructureDefinition"; 5495 if (code == FHIRDefinedType.SUBSCRIPTION) 5496 return "Subscription"; 5497 if (code == FHIRDefinedType.SUBSTANCE) 5498 return "Substance"; 5499 if (code == FHIRDefinedType.SUPPLYDELIVERY) 5500 return "SupplyDelivery"; 5501 if (code == FHIRDefinedType.SUPPLYREQUEST) 5502 return "SupplyRequest"; 5503 if (code == FHIRDefinedType.TESTSCRIPT) 5504 return "TestScript"; 5505 if (code == FHIRDefinedType.VALUESET) 5506 return "ValueSet"; 5507 if (code == FHIRDefinedType.VISIONPRESCRIPTION) 5508 return "VisionPrescription"; 5509 return "?"; 5510 } 5511 } 5512 5513 public enum MessageEvent { 5514 /** 5515 * Change the status of a Medication Administration to show that it is complete. 5516 */ 5517 MEDICATIONADMINISTRATIONCOMPLETE, 5518 /** 5519 * Someone wishes to record that the record of administration of a medication is 5520 * in error and should be ignored. 5521 */ 5522 MEDICATIONADMINISTRATIONNULLIFICATION, 5523 /** 5524 * Indicates that a medication has been recorded against the patient's record. 5525 */ 5526 MEDICATIONADMINISTRATIONRECORDING, 5527 /** 5528 * Update a Medication Administration record. 5529 */ 5530 MEDICATIONADMINISTRATIONUPDATE, 5531 /** 5532 * Notification of a change to an administrative resource (either create or 5533 * update). Note that there is no delete, though some administrative resources 5534 * have status or period elements for this use. 5535 */ 5536 ADMINNOTIFY, 5537 /** 5538 * Provide a diagnostic report, or update a previously provided diagnostic 5539 * report. 5540 */ 5541 DIAGNOSTICREPORTPROVIDE, 5542 /** 5543 * Provide a simple observation or update a previously provided simple 5544 * observation. 5545 */ 5546 OBSERVATIONPROVIDE, 5547 /** 5548 * Notification that two patient records actually identify the same patient. 5549 */ 5550 PATIENTLINK, 5551 /** 5552 * Notification that previous advice that two patient records concern the same 5553 * patient is now considered incorrect. 5554 */ 5555 PATIENTUNLINK, 5556 /** 5557 * The definition of a value set is used to create a simple collection of codes 5558 * suitable for use for data entry or validation. An expanded value set will be 5559 * returned, or an error message. 5560 */ 5561 VALUESETEXPAND, 5562 /** 5563 * added to help the parsers 5564 */ 5565 NULL; 5566 5567 public static MessageEvent fromCode(String codeString) throws FHIRException { 5568 if (codeString == null || "".equals(codeString)) 5569 return null; 5570 if ("MedicationAdministration-Complete".equals(codeString)) 5571 return MEDICATIONADMINISTRATIONCOMPLETE; 5572 if ("MedicationAdministration-Nullification".equals(codeString)) 5573 return MEDICATIONADMINISTRATIONNULLIFICATION; 5574 if ("MedicationAdministration-Recording".equals(codeString)) 5575 return MEDICATIONADMINISTRATIONRECORDING; 5576 if ("MedicationAdministration-Update".equals(codeString)) 5577 return MEDICATIONADMINISTRATIONUPDATE; 5578 if ("admin-notify".equals(codeString)) 5579 return ADMINNOTIFY; 5580 if ("diagnosticreport-provide".equals(codeString)) 5581 return DIAGNOSTICREPORTPROVIDE; 5582 if ("observation-provide".equals(codeString)) 5583 return OBSERVATIONPROVIDE; 5584 if ("patient-link".equals(codeString)) 5585 return PATIENTLINK; 5586 if ("patient-unlink".equals(codeString)) 5587 return PATIENTUNLINK; 5588 if ("valueset-expand".equals(codeString)) 5589 return VALUESETEXPAND; 5590 throw new FHIRException("Unknown MessageEvent code '" + codeString + "'"); 5591 } 5592 5593 public String toCode() { 5594 switch (this) { 5595 case MEDICATIONADMINISTRATIONCOMPLETE: 5596 return "MedicationAdministration-Complete"; 5597 case MEDICATIONADMINISTRATIONNULLIFICATION: 5598 return "MedicationAdministration-Nullification"; 5599 case MEDICATIONADMINISTRATIONRECORDING: 5600 return "MedicationAdministration-Recording"; 5601 case MEDICATIONADMINISTRATIONUPDATE: 5602 return "MedicationAdministration-Update"; 5603 case ADMINNOTIFY: 5604 return "admin-notify"; 5605 case DIAGNOSTICREPORTPROVIDE: 5606 return "diagnosticreport-provide"; 5607 case OBSERVATIONPROVIDE: 5608 return "observation-provide"; 5609 case PATIENTLINK: 5610 return "patient-link"; 5611 case PATIENTUNLINK: 5612 return "patient-unlink"; 5613 case VALUESETEXPAND: 5614 return "valueset-expand"; 5615 case NULL: 5616 return null; 5617 default: 5618 return "?"; 5619 } 5620 } 5621 5622 public String getSystem() { 5623 switch (this) { 5624 case MEDICATIONADMINISTRATIONCOMPLETE: 5625 return "http://hl7.org/fhir/message-events"; 5626 case MEDICATIONADMINISTRATIONNULLIFICATION: 5627 return "http://hl7.org/fhir/message-events"; 5628 case MEDICATIONADMINISTRATIONRECORDING: 5629 return "http://hl7.org/fhir/message-events"; 5630 case MEDICATIONADMINISTRATIONUPDATE: 5631 return "http://hl7.org/fhir/message-events"; 5632 case ADMINNOTIFY: 5633 return "http://hl7.org/fhir/message-events"; 5634 case DIAGNOSTICREPORTPROVIDE: 5635 return "http://hl7.org/fhir/message-events"; 5636 case OBSERVATIONPROVIDE: 5637 return "http://hl7.org/fhir/message-events"; 5638 case PATIENTLINK: 5639 return "http://hl7.org/fhir/message-events"; 5640 case PATIENTUNLINK: 5641 return "http://hl7.org/fhir/message-events"; 5642 case VALUESETEXPAND: 5643 return "http://hl7.org/fhir/message-events"; 5644 case NULL: 5645 return null; 5646 default: 5647 return "?"; 5648 } 5649 } 5650 5651 public String getDefinition() { 5652 switch (this) { 5653 case MEDICATIONADMINISTRATIONCOMPLETE: 5654 return "Change the status of a Medication Administration to show that it is complete."; 5655 case MEDICATIONADMINISTRATIONNULLIFICATION: 5656 return "Someone wishes to record that the record of administration of a medication is in error and should be ignored."; 5657 case MEDICATIONADMINISTRATIONRECORDING: 5658 return "Indicates that a medication has been recorded against the patient's record."; 5659 case MEDICATIONADMINISTRATIONUPDATE: 5660 return "Update a Medication Administration record."; 5661 case ADMINNOTIFY: 5662 return "Notification of a change to an administrative resource (either create or update). Note that there is no delete, though some administrative resources have status or period elements for this use."; 5663 case DIAGNOSTICREPORTPROVIDE: 5664 return "Provide a diagnostic report, or update a previously provided diagnostic report."; 5665 case OBSERVATIONPROVIDE: 5666 return "Provide a simple observation or update a previously provided simple observation."; 5667 case PATIENTLINK: 5668 return "Notification that two patient records actually identify the same patient."; 5669 case PATIENTUNLINK: 5670 return "Notification that previous advice that two patient records concern the same patient is now considered incorrect."; 5671 case VALUESETEXPAND: 5672 return "The definition of a value set is used to create a simple collection of codes suitable for use for data entry or validation. An expanded value set will be returned, or an error message."; 5673 case NULL: 5674 return null; 5675 default: 5676 return "?"; 5677 } 5678 } 5679 5680 public String getDisplay() { 5681 switch (this) { 5682 case MEDICATIONADMINISTRATIONCOMPLETE: 5683 return "MedicationAdministration-Complete"; 5684 case MEDICATIONADMINISTRATIONNULLIFICATION: 5685 return "MedicationAdministration-Nullification"; 5686 case MEDICATIONADMINISTRATIONRECORDING: 5687 return "MedicationAdministration-Recording"; 5688 case MEDICATIONADMINISTRATIONUPDATE: 5689 return "MedicationAdministration-Update"; 5690 case ADMINNOTIFY: 5691 return "admin-notify"; 5692 case DIAGNOSTICREPORTPROVIDE: 5693 return "diagnosticreport-provide"; 5694 case OBSERVATIONPROVIDE: 5695 return "observation-provide"; 5696 case PATIENTLINK: 5697 return "patient-link"; 5698 case PATIENTUNLINK: 5699 return "patient-unlink"; 5700 case VALUESETEXPAND: 5701 return "valueset-expand"; 5702 case NULL: 5703 return null; 5704 default: 5705 return "?"; 5706 } 5707 } 5708 } 5709 5710 public static class MessageEventEnumFactory implements EnumFactory<MessageEvent> { 5711 public MessageEvent fromCode(String codeString) throws IllegalArgumentException { 5712 if (codeString == null || "".equals(codeString)) 5713 if (codeString == null || "".equals(codeString)) 5714 return null; 5715 if ("MedicationAdministration-Complete".equals(codeString)) 5716 return MessageEvent.MEDICATIONADMINISTRATIONCOMPLETE; 5717 if ("MedicationAdministration-Nullification".equals(codeString)) 5718 return MessageEvent.MEDICATIONADMINISTRATIONNULLIFICATION; 5719 if ("MedicationAdministration-Recording".equals(codeString)) 5720 return MessageEvent.MEDICATIONADMINISTRATIONRECORDING; 5721 if ("MedicationAdministration-Update".equals(codeString)) 5722 return MessageEvent.MEDICATIONADMINISTRATIONUPDATE; 5723 if ("admin-notify".equals(codeString)) 5724 return MessageEvent.ADMINNOTIFY; 5725 if ("diagnosticreport-provide".equals(codeString)) 5726 return MessageEvent.DIAGNOSTICREPORTPROVIDE; 5727 if ("observation-provide".equals(codeString)) 5728 return MessageEvent.OBSERVATIONPROVIDE; 5729 if ("patient-link".equals(codeString)) 5730 return MessageEvent.PATIENTLINK; 5731 if ("patient-unlink".equals(codeString)) 5732 return MessageEvent.PATIENTUNLINK; 5733 if ("valueset-expand".equals(codeString)) 5734 return MessageEvent.VALUESETEXPAND; 5735 throw new IllegalArgumentException("Unknown MessageEvent code '" + codeString + "'"); 5736 } 5737 5738 public Enumeration<MessageEvent> fromType(Base code) throws FHIRException { 5739 if (code == null || code.isEmpty()) 5740 return null; 5741 String codeString = ((PrimitiveType) code).asStringValue(); 5742 if (codeString == null || "".equals(codeString)) 5743 return null; 5744 if ("MedicationAdministration-Complete".equals(codeString)) 5745 return new Enumeration<MessageEvent>(this, MessageEvent.MEDICATIONADMINISTRATIONCOMPLETE); 5746 if ("MedicationAdministration-Nullification".equals(codeString)) 5747 return new Enumeration<MessageEvent>(this, MessageEvent.MEDICATIONADMINISTRATIONNULLIFICATION); 5748 if ("MedicationAdministration-Recording".equals(codeString)) 5749 return new Enumeration<MessageEvent>(this, MessageEvent.MEDICATIONADMINISTRATIONRECORDING); 5750 if ("MedicationAdministration-Update".equals(codeString)) 5751 return new Enumeration<MessageEvent>(this, MessageEvent.MEDICATIONADMINISTRATIONUPDATE); 5752 if ("admin-notify".equals(codeString)) 5753 return new Enumeration<MessageEvent>(this, MessageEvent.ADMINNOTIFY); 5754 if ("diagnosticreport-provide".equals(codeString)) 5755 return new Enumeration<MessageEvent>(this, MessageEvent.DIAGNOSTICREPORTPROVIDE); 5756 if ("observation-provide".equals(codeString)) 5757 return new Enumeration<MessageEvent>(this, MessageEvent.OBSERVATIONPROVIDE); 5758 if ("patient-link".equals(codeString)) 5759 return new Enumeration<MessageEvent>(this, MessageEvent.PATIENTLINK); 5760 if ("patient-unlink".equals(codeString)) 5761 return new Enumeration<MessageEvent>(this, MessageEvent.PATIENTUNLINK); 5762 if ("valueset-expand".equals(codeString)) 5763 return new Enumeration<MessageEvent>(this, MessageEvent.VALUESETEXPAND); 5764 throw new FHIRException("Unknown MessageEvent code '" + codeString + "'"); 5765 } 5766 5767 public String toCode(MessageEvent code) 5768 { 5769 if (code == MessageEvent.NULL) 5770 return null; 5771 if (code == MessageEvent.MEDICATIONADMINISTRATIONCOMPLETE) 5772 return "MedicationAdministration-Complete"; 5773 if (code == MessageEvent.MEDICATIONADMINISTRATIONNULLIFICATION) 5774 return "MedicationAdministration-Nullification"; 5775 if (code == MessageEvent.MEDICATIONADMINISTRATIONRECORDING) 5776 return "MedicationAdministration-Recording"; 5777 if (code == MessageEvent.MEDICATIONADMINISTRATIONUPDATE) 5778 return "MedicationAdministration-Update"; 5779 if (code == MessageEvent.ADMINNOTIFY) 5780 return "admin-notify"; 5781 if (code == MessageEvent.DIAGNOSTICREPORTPROVIDE) 5782 return "diagnosticreport-provide"; 5783 if (code == MessageEvent.OBSERVATIONPROVIDE) 5784 return "observation-provide"; 5785 if (code == MessageEvent.PATIENTLINK) 5786 return "patient-link"; 5787 if (code == MessageEvent.PATIENTUNLINK) 5788 return "patient-unlink"; 5789 if (code == MessageEvent.VALUESETEXPAND) 5790 return "valueset-expand"; 5791 return "?"; 5792 } 5793 } 5794 5795 public enum NoteType { 5796 /** 5797 * Display the note. 5798 */ 5799 DISPLAY, 5800 /** 5801 * Print the note on the form. 5802 */ 5803 PRINT, 5804 /** 5805 * Print the note for the operator. 5806 */ 5807 PRINTOPER, 5808 /** 5809 * added to help the parsers 5810 */ 5811 NULL; 5812 5813 public static NoteType fromCode(String codeString) throws FHIRException { 5814 if (codeString == null || "".equals(codeString)) 5815 return null; 5816 if ("display".equals(codeString)) 5817 return DISPLAY; 5818 if ("print".equals(codeString)) 5819 return PRINT; 5820 if ("printoper".equals(codeString)) 5821 return PRINTOPER; 5822 throw new FHIRException("Unknown NoteType code '" + codeString + "'"); 5823 } 5824 5825 public String toCode() { 5826 switch (this) { 5827 case DISPLAY: 5828 return "display"; 5829 case PRINT: 5830 return "print"; 5831 case PRINTOPER: 5832 return "printoper"; 5833 case NULL: 5834 return null; 5835 default: 5836 return "?"; 5837 } 5838 } 5839 5840 public String getSystem() { 5841 switch (this) { 5842 case DISPLAY: 5843 return "http://hl7.org/fhir/note-type"; 5844 case PRINT: 5845 return "http://hl7.org/fhir/note-type"; 5846 case PRINTOPER: 5847 return "http://hl7.org/fhir/note-type"; 5848 case NULL: 5849 return null; 5850 default: 5851 return "?"; 5852 } 5853 } 5854 5855 public String getDefinition() { 5856 switch (this) { 5857 case DISPLAY: 5858 return "Display the note."; 5859 case PRINT: 5860 return "Print the note on the form."; 5861 case PRINTOPER: 5862 return "Print the note for the operator."; 5863 case NULL: 5864 return null; 5865 default: 5866 return "?"; 5867 } 5868 } 5869 5870 public String getDisplay() { 5871 switch (this) { 5872 case DISPLAY: 5873 return "Display"; 5874 case PRINT: 5875 return "Print (Form)"; 5876 case PRINTOPER: 5877 return "Print (Operator)"; 5878 case NULL: 5879 return null; 5880 default: 5881 return "?"; 5882 } 5883 } 5884 } 5885 5886 public static class NoteTypeEnumFactory implements EnumFactory<NoteType> { 5887 public NoteType fromCode(String codeString) throws IllegalArgumentException { 5888 if (codeString == null || "".equals(codeString)) 5889 if (codeString == null || "".equals(codeString)) 5890 return null; 5891 if ("display".equals(codeString)) 5892 return NoteType.DISPLAY; 5893 if ("print".equals(codeString)) 5894 return NoteType.PRINT; 5895 if ("printoper".equals(codeString)) 5896 return NoteType.PRINTOPER; 5897 throw new IllegalArgumentException("Unknown NoteType code '" + codeString + "'"); 5898 } 5899 5900 public Enumeration<NoteType> fromType(Base code) throws FHIRException { 5901 if (code == null || code.isEmpty()) 5902 return null; 5903 String codeString = ((PrimitiveType) code).asStringValue(); 5904 if (codeString == null || "".equals(codeString)) 5905 return null; 5906 if ("display".equals(codeString)) 5907 return new Enumeration<NoteType>(this, NoteType.DISPLAY); 5908 if ("print".equals(codeString)) 5909 return new Enumeration<NoteType>(this, NoteType.PRINT); 5910 if ("printoper".equals(codeString)) 5911 return new Enumeration<NoteType>(this, NoteType.PRINTOPER); 5912 throw new FHIRException("Unknown NoteType code '" + codeString + "'"); 5913 } 5914 5915 public String toCode(NoteType code) 5916 { 5917 if (code == NoteType.NULL) 5918 return null; 5919 if (code == NoteType.DISPLAY) 5920 return "display"; 5921 if (code == NoteType.PRINT) 5922 return "print"; 5923 if (code == NoteType.PRINTOPER) 5924 return "printoper"; 5925 return "?"; 5926 } 5927 } 5928 5929 public enum RemittanceOutcome { 5930 /** 5931 * The processing completed without errors. 5932 */ 5933 COMPLETE, 5934 /** 5935 * The processing identified errors. 5936 */ 5937 ERROR, 5938 /** 5939 * added to help the parsers 5940 */ 5941 NULL; 5942 5943 public static RemittanceOutcome fromCode(String codeString) throws FHIRException { 5944 if (codeString == null || "".equals(codeString)) 5945 return null; 5946 if ("complete".equals(codeString)) 5947 return COMPLETE; 5948 if ("error".equals(codeString)) 5949 return ERROR; 5950 throw new FHIRException("Unknown RemittanceOutcome code '" + codeString + "'"); 5951 } 5952 5953 public String toCode() { 5954 switch (this) { 5955 case COMPLETE: 5956 return "complete"; 5957 case ERROR: 5958 return "error"; 5959 case NULL: 5960 return null; 5961 default: 5962 return "?"; 5963 } 5964 } 5965 5966 public String getSystem() { 5967 switch (this) { 5968 case COMPLETE: 5969 return "http://hl7.org/fhir/remittance-outcome"; 5970 case ERROR: 5971 return "http://hl7.org/fhir/remittance-outcome"; 5972 case NULL: 5973 return null; 5974 default: 5975 return "?"; 5976 } 5977 } 5978 5979 public String getDefinition() { 5980 switch (this) { 5981 case COMPLETE: 5982 return "The processing completed without errors."; 5983 case ERROR: 5984 return "The processing identified errors."; 5985 case NULL: 5986 return null; 5987 default: 5988 return "?"; 5989 } 5990 } 5991 5992 public String getDisplay() { 5993 switch (this) { 5994 case COMPLETE: 5995 return "Complete"; 5996 case ERROR: 5997 return "Error"; 5998 case NULL: 5999 return null; 6000 default: 6001 return "?"; 6002 } 6003 } 6004 } 6005 6006 public static class RemittanceOutcomeEnumFactory implements EnumFactory<RemittanceOutcome> { 6007 public RemittanceOutcome fromCode(String codeString) throws IllegalArgumentException { 6008 if (codeString == null || "".equals(codeString)) 6009 if (codeString == null || "".equals(codeString)) 6010 return null; 6011 if ("complete".equals(codeString)) 6012 return RemittanceOutcome.COMPLETE; 6013 if ("error".equals(codeString)) 6014 return RemittanceOutcome.ERROR; 6015 throw new IllegalArgumentException("Unknown RemittanceOutcome code '" + codeString + "'"); 6016 } 6017 6018 public Enumeration<RemittanceOutcome> fromType(Base code) throws FHIRException { 6019 if (code == null || code.isEmpty()) 6020 return null; 6021 String codeString = ((PrimitiveType) code).asStringValue(); 6022 if (codeString == null || "".equals(codeString)) 6023 return null; 6024 if ("complete".equals(codeString)) 6025 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.COMPLETE); 6026 if ("error".equals(codeString)) 6027 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.ERROR); 6028 throw new FHIRException("Unknown RemittanceOutcome code '" + codeString + "'"); 6029 } 6030 6031 public String toCode(RemittanceOutcome code) 6032 { 6033 if (code == RemittanceOutcome.NULL) 6034 return null; 6035 if (code == RemittanceOutcome.COMPLETE) 6036 return "complete"; 6037 if (code == RemittanceOutcome.ERROR) 6038 return "error"; 6039 return "?"; 6040 } 6041 } 6042 6043 public enum ResourceType { 6044 /** 6045 * A financial tool for tracking value accrued for a particular purpose. In the 6046 * healthcare field, used to track charges for a patient, cost centres, etc. 6047 */ 6048 ACCOUNT, 6049 /** 6050 * Risk of harmful or undesirable, physiological response which is unique to an 6051 * individual and associated with exposure to a substance. 6052 */ 6053 ALLERGYINTOLERANCE, 6054 /** 6055 * A booking of a healthcare event among patient(s), practitioner(s), related 6056 * person(s) and/or device(s) for a specific date/time. This may result in one 6057 * or more Encounter(s). 6058 */ 6059 APPOINTMENT, 6060 /** 6061 * A reply to an appointment request for a patient and/or practitioner(s), such 6062 * as a confirmation or rejection. 6063 */ 6064 APPOINTMENTRESPONSE, 6065 /** 6066 * A record of an event made for purposes of maintaining a security log. Typical 6067 * uses include detection of intrusion attempts and monitoring for inappropriate 6068 * usage. 6069 */ 6070 AUDITEVENT, 6071 /** 6072 * Basic is used for handling concepts not yet defined in FHIR, narrative-only 6073 * resources that don't map to an existing resource, and custom resources not 6074 * appropriate for inclusion in the FHIR specification. 6075 */ 6076 BASIC, 6077 /** 6078 * A binary resource can contain any content, whether text, image, pdf, zip 6079 * archive, etc. 6080 */ 6081 BINARY, 6082 /** 6083 * Record details about the anatomical location of a specimen or body part. This 6084 * resource may be used when a coded concept does not provide the necessary 6085 * detail needed for the use case. 6086 */ 6087 BODYSITE, 6088 /** 6089 * A container for a collection of resources. 6090 */ 6091 BUNDLE, 6092 /** 6093 * Describes the intention of how one or more practitioners intend to deliver 6094 * care for a particular patient, group or community for a period of time, 6095 * possibly limited to care for a specific condition or set of conditions. 6096 */ 6097 CAREPLAN, 6098 /** 6099 * A provider issued list of services and products provided, or to be provided, 6100 * to a patient which is provided to an insurer for payment recovery. 6101 */ 6102 CLAIM, 6103 /** 6104 * This resource provides the adjudication details from the processing of a 6105 * Claim resource. 6106 */ 6107 CLAIMRESPONSE, 6108 /** 6109 * A record of a clinical assessment performed to determine what problem(s) may 6110 * affect the patient and before planning the treatments or management 6111 * strategies that are best to manage a patient's condition. Assessments are 6112 * often 1:1 with a clinical consultation / encounter, but this varies greatly 6113 * depending on the clinical workflow. This resource is called 6114 * "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with 6115 * the recording of assessment tools such as Apgar score. 6116 */ 6117 CLINICALIMPRESSION, 6118 /** 6119 * An occurrence of information being transmitted; e.g. an alert that was sent 6120 * to a responsible provider, a public health agency was notified about a 6121 * reportable condition. 6122 */ 6123 COMMUNICATION, 6124 /** 6125 * A request to convey information; e.g. the CDS system proposes that an alert 6126 * be sent to a responsible provider, the CDS system proposes that the public 6127 * health agency be notified about a reportable condition. 6128 */ 6129 COMMUNICATIONREQUEST, 6130 /** 6131 * A set of healthcare-related information that is assembled together into a 6132 * single logical document that provides a single coherent statement of meaning, 6133 * establishes its own context and that has clinical attestation with regard to 6134 * who is making the statement. While a Composition defines the structure, it 6135 * does not actually contain the content: rather the full content of a document 6136 * is contained in a Bundle, of which the Composition is the first resource 6137 * contained. 6138 */ 6139 COMPOSITION, 6140 /** 6141 * A statement of relationships from one set of concepts to one or more other 6142 * concepts - either code systems or data elements, or classes in class models. 6143 */ 6144 CONCEPTMAP, 6145 /** 6146 * Use to record detailed information about conditions, problems or diagnoses 6147 * recognized by a clinician. There are many uses including: recording a 6148 * diagnosis during an encounter; populating a problem list or a summary 6149 * statement, such as a discharge summary. 6150 */ 6151 CONDITION, 6152 /** 6153 * A conformance statement is a set of capabilities of a FHIR Server that may be 6154 * used as a statement of actual server functionality or a statement of required 6155 * or desired server implementation. 6156 */ 6157 CONFORMANCE, 6158 /** 6159 * A formal agreement between parties regarding the conduct of business, 6160 * exchange of information or other matters. 6161 */ 6162 CONTRACT, 6163 /** 6164 * Financial instrument which may be used to pay for or reimburse health care 6165 * products and services. 6166 */ 6167 COVERAGE, 6168 /** 6169 * The formal description of a single piece of information that can be gathered 6170 * and reported. 6171 */ 6172 DATAELEMENT, 6173 /** 6174 * Indicates an actual or potential clinical issue with or between one or more 6175 * active or proposed clinical actions for a patient; e.g. Drug-drug 6176 * interaction, Ineffective treatment frequency, Procedure-condition conflict, 6177 * etc. 6178 */ 6179 DETECTEDISSUE, 6180 /** 6181 * This resource identifies an instance of a manufactured item that is used in 6182 * the provision of healthcare without being substantially changed through that 6183 * activity. The device may be a medical or non-medical device. Medical devices 6184 * includes durable (reusable) medical equipment, implantable devices, as well 6185 * as disposable equipment used for diagnostic, treatment, and research for 6186 * healthcare and public health. Non-medical devices may include items such as a 6187 * machine, cellphone, computer, application, etc. 6188 */ 6189 DEVICE, 6190 /** 6191 * Describes the characteristics, operational status and capabilities of a 6192 * medical-related component of a medical device. 6193 */ 6194 DEVICECOMPONENT, 6195 /** 6196 * Describes a measurement, calculation or setting capability of a medical 6197 * device. 6198 */ 6199 DEVICEMETRIC, 6200 /** 6201 * Represents a request for a patient to employ a medical device. The device may 6202 * be an implantable device, or an external assistive device, such as a walker. 6203 */ 6204 DEVICEUSEREQUEST, 6205 /** 6206 * A record of a device being used by a patient where the record is the result 6207 * of a report from the patient or another clinician. 6208 */ 6209 DEVICEUSESTATEMENT, 6210 /** 6211 * A record of a request for a diagnostic investigation service to be performed. 6212 */ 6213 DIAGNOSTICORDER, 6214 /** 6215 * The findings and interpretation of diagnostic tests performed on patients, 6216 * groups of patients, devices, and locations, and/or specimens derived from 6217 * these. The report includes clinical context such as requesting and provider 6218 * information, and some mix of atomic results, images, textual and coded 6219 * interpretations, and formatted representation of diagnostic reports. 6220 */ 6221 DIAGNOSTICREPORT, 6222 /** 6223 * A manifest that defines a set of documents. 6224 */ 6225 DOCUMENTMANIFEST, 6226 /** 6227 * A reference to a document . 6228 */ 6229 DOCUMENTREFERENCE, 6230 /** 6231 * --- Abstract Type! ---A resource that includes narrative, extensions, and 6232 * contained resources. 6233 */ 6234 DOMAINRESOURCE, 6235 /** 6236 * This resource provides the insurance eligibility details from the insurer 6237 * regarding a specified coverage and optionally some class of service. 6238 */ 6239 ELIGIBILITYREQUEST, 6240 /** 6241 * This resource provides eligibility and plan details from the processing of an 6242 * Eligibility resource. 6243 */ 6244 ELIGIBILITYRESPONSE, 6245 /** 6246 * An interaction between a patient and healthcare provider(s) for the purpose 6247 * of providing healthcare service(s) or assessing the health status of a 6248 * patient. 6249 */ 6250 ENCOUNTER, 6251 /** 6252 * This resource provides the insurance enrollment details to the insurer 6253 * regarding a specified coverage. 6254 */ 6255 ENROLLMENTREQUEST, 6256 /** 6257 * This resource provides enrollment and plan details from the processing of an 6258 * Enrollment resource. 6259 */ 6260 ENROLLMENTRESPONSE, 6261 /** 6262 * An association between a patient and an organization / healthcare provider(s) 6263 * during which time encounters may occur. The managing organization assumes a 6264 * level of responsibility for the patient during this time. 6265 */ 6266 EPISODEOFCARE, 6267 /** 6268 * This resource provides: the claim details; adjudication details from the 6269 * processing of a Claim; and optionally account balance information, for 6270 * informing the subscriber of the benefits provided. 6271 */ 6272 EXPLANATIONOFBENEFIT, 6273 /** 6274 * Significant health events and conditions for a person related to the patient 6275 * relevant in the context of care for the patient. 6276 */ 6277 FAMILYMEMBERHISTORY, 6278 /** 6279 * Prospective warnings of potential issues when providing care to the patient. 6280 */ 6281 FLAG, 6282 /** 6283 * Describes the intended objective(s) for a patient, group or organization 6284 * care, for example, weight loss, restoring an activity of daily living, 6285 * obtaining herd immunity via immunization, meeting a process improvement 6286 * objective, etc. 6287 */ 6288 GOAL, 6289 /** 6290 * Represents a defined collection of entities that may be discussed or acted 6291 * upon collectively but which are not expected to act collectively and are not 6292 * formally or legally recognized; i.e. a collection of entities that isn't an 6293 * Organization. 6294 */ 6295 GROUP, 6296 /** 6297 * The details of a healthcare service available at a location. 6298 */ 6299 HEALTHCARESERVICE, 6300 /** 6301 * A manifest of a set of DICOM Service-Object Pair Instances (SOP Instances). 6302 * The referenced SOP Instances (images or other content) are for a single 6303 * patient, and may be from one or more studies. The referenced SOP Instances 6304 * have been selected for a purpose, such as quality assurance, conference, or 6305 * consult. Reflecting that range of purposes, typical ImagingObjectSelection 6306 * resources may include all SOP Instances in a study (perhaps for sharing 6307 * through a Health Information Exchange); key images from multiple studies (for 6308 * reference by a referring or treating physician); a multi-frame ultrasound 6309 * instance ("cine" video clip) and a set of measurements taken from that 6310 * instance (for inclusion in a teaching file); and so on. 6311 */ 6312 IMAGINGOBJECTSELECTION, 6313 /** 6314 * Representation of the content produced in a DICOM imaging study. A study 6315 * comprises a set of series, each of which includes a set of Service-Object 6316 * Pair Instances (SOP Instances - images or other data) acquired or produced in 6317 * a common context. A series is of only one modality (e.g. X-ray, CT, MR, 6318 * ultrasound), but a study may have multiple series of different modalities. 6319 */ 6320 IMAGINGSTUDY, 6321 /** 6322 * Describes the event of a patient being administered a vaccination or a record 6323 * of a vaccination as reported by a patient, a clinician or another party and 6324 * may include vaccine reaction information and what vaccination protocol was 6325 * followed. 6326 */ 6327 IMMUNIZATION, 6328 /** 6329 * A patient's point-in-time immunization and recommendation (i.e. forecasting a 6330 * patient's immunization eligibility according to a published schedule) with 6331 * optional supporting justification. 6332 */ 6333 IMMUNIZATIONRECOMMENDATION, 6334 /** 6335 * A set of rules or how FHIR is used to solve a particular problem. This 6336 * resource is used to gather all the parts of an implementation guide into a 6337 * logical whole, and to publish a computable definition of all the parts. 6338 */ 6339 IMPLEMENTATIONGUIDE, 6340 /** 6341 * A set of information summarized from a list of other resources. 6342 */ 6343 LIST, 6344 /** 6345 * Details and position information for a physical place where services are 6346 * provided and resources and participants may be stored, found, contained or 6347 * accommodated. 6348 */ 6349 LOCATION, 6350 /** 6351 * A photo, video, or audio recording acquired or used in healthcare. The actual 6352 * content may be inline or provided by direct reference. 6353 */ 6354 MEDIA, 6355 /** 6356 * This resource is primarily used for the identification and definition of a 6357 * medication. It covers the ingredients and the packaging for a medication. 6358 */ 6359 MEDICATION, 6360 /** 6361 * Describes the event of a patient consuming or otherwise being administered a 6362 * medication. This may be as simple as swallowing a tablet or it may be a long 6363 * running infusion. Related resources tie this event to the authorizing 6364 * prescription, and the specific encounter between patient and health care 6365 * practitioner. 6366 */ 6367 MEDICATIONADMINISTRATION, 6368 /** 6369 * Indicates that a medication product is to be or has been dispensed for a 6370 * named person/patient. This includes a description of the medication product 6371 * (supply) provided and the instructions for administering the medication. The 6372 * medication dispense is the result of a pharmacy system responding to a 6373 * medication order. 6374 */ 6375 MEDICATIONDISPENSE, 6376 /** 6377 * An order for both supply of the medication and the instructions for 6378 * administration of the medication to a patient. The resource is called 6379 * "MedicationOrder" rather than "MedicationPrescription" to generalize the use 6380 * across inpatient and outpatient settings as well as for care plans, etc. 6381 */ 6382 MEDICATIONORDER, 6383 /** 6384 * A record of a medication that is being consumed by a patient. A 6385 * MedicationStatement may indicate that the patient may be taking the 6386 * medication now, or has taken the medication in the past or will be taking the 6387 * medication in the future. The source of this information can be the patient, 6388 * significant other (such as a family member or spouse), or a clinician. A 6389 * common scenario where this information is captured is during the history 6390 * taking process during a patient visit or stay. The medication information may 6391 * come from e.g. the patient's memory, from a prescription bottle, or from a 6392 * list of medications the patient, clinician or other party maintains 6393 * 6394 * The primary difference between a medication statement and a medication 6395 * administration is that the medication administration has complete 6396 * administration information and is based on actual administration information 6397 * from the person who administered the medication. A medication statement is 6398 * often, if not always, less specific. There is no required date/time when the 6399 * medication was administered, in fact we only know that a source has reported 6400 * the patient is taking this medication, where details such as time, quantity, 6401 * or rate or even medication product may be incomplete or missing or less 6402 * precise. As stated earlier, the medication statement information may come 6403 * from the patient's memory, from a prescription bottle or from a list of 6404 * medications the patient, clinician or other party maintains. Medication 6405 * administration is more formal and is not missing detailed information. 6406 */ 6407 MEDICATIONSTATEMENT, 6408 /** 6409 * The header for a message exchange that is either requesting or responding to 6410 * an action. The reference(s) that are the subject of the action as well as 6411 * other information related to the action are typically transmitted in a bundle 6412 * in which the MessageHeader resource instance is the first resource in the 6413 * bundle. 6414 */ 6415 MESSAGEHEADER, 6416 /** 6417 * A curated namespace that issues unique symbols within that namespace for the 6418 * identification of concepts, people, devices, etc. Represents a "System" used 6419 * within the Identifier and Coding data types. 6420 */ 6421 NAMINGSYSTEM, 6422 /** 6423 * A request to supply a diet, formula feeding (enteral) or oral nutritional 6424 * supplement to a patient/resident. 6425 */ 6426 NUTRITIONORDER, 6427 /** 6428 * Measurements and simple assertions made about a patient, device or other 6429 * subject. 6430 */ 6431 OBSERVATION, 6432 /** 6433 * A formal computable definition of an operation (on the RESTful interface) or 6434 * a named query (using the search interaction). 6435 */ 6436 OPERATIONDEFINITION, 6437 /** 6438 * A collection of error, warning or information messages that result from a 6439 * system action. 6440 */ 6441 OPERATIONOUTCOME, 6442 /** 6443 * A request to perform an action. 6444 */ 6445 ORDER, 6446 /** 6447 * A response to an order. 6448 */ 6449 ORDERRESPONSE, 6450 /** 6451 * A formally or informally recognized grouping of people or organizations 6452 * formed for the purpose of achieving some form of collective action. Includes 6453 * companies, institutions, corporations, departments, community groups, 6454 * healthcare practice groups, etc. 6455 */ 6456 ORGANIZATION, 6457 /** 6458 * This special resource type is used to represent an operation request and 6459 * response (operations.html). It has no other use, and there is no RESTful 6460 * endpoint associated with it. 6461 */ 6462 PARAMETERS, 6463 /** 6464 * Demographics and other administrative information about an individual or 6465 * animal receiving care or other health-related services. 6466 */ 6467 PATIENT, 6468 /** 6469 * This resource provides the status of the payment for goods and services 6470 * rendered, and the request and response resource references. 6471 */ 6472 PAYMENTNOTICE, 6473 /** 6474 * This resource provides payment details and claim references supporting a bulk 6475 * payment. 6476 */ 6477 PAYMENTRECONCILIATION, 6478 /** 6479 * Demographics and administrative information about a person independent of a 6480 * specific health-related context. 6481 */ 6482 PERSON, 6483 /** 6484 * A person who is directly or indirectly involved in the provisioning of 6485 * healthcare. 6486 */ 6487 PRACTITIONER, 6488 /** 6489 * An action that is or was performed on a patient. This can be a physical 6490 * intervention like an operation, or less invasive like counseling or 6491 * hypnotherapy. 6492 */ 6493 PROCEDURE, 6494 /** 6495 * A request for a procedure to be performed. May be a proposal or an order. 6496 */ 6497 PROCEDUREREQUEST, 6498 /** 6499 * This resource provides the target, request and response, and action details 6500 * for an action to be performed by the target on or about existing resources. 6501 */ 6502 PROCESSREQUEST, 6503 /** 6504 * This resource provides processing status, errors and notes from the 6505 * processing of a resource. 6506 */ 6507 PROCESSRESPONSE, 6508 /** 6509 * Provenance of a resource is a record that describes entities and processes 6510 * involved in producing and delivering or otherwise influencing that resource. 6511 * Provenance provides a critical foundation for assessing authenticity, 6512 * enabling trust, and allowing reproducibility. Provenance assertions are a 6513 * form of contextual metadata and can themselves become important records with 6514 * their own provenance. Provenance statement indicates clinical significance in 6515 * terms of confidence in authenticity, reliability, and trustworthiness, 6516 * integrity, and stage in lifecycle (e.g. Document Completion - has the 6517 * artifact been legally authenticated), all of which may impact security, 6518 * privacy, and trust policies. 6519 */ 6520 PROVENANCE, 6521 /** 6522 * A structured set of questions intended to guide the collection of answers. 6523 * The questions are ordered and grouped into coherent subsets, corresponding to 6524 * the structure of the grouping of the underlying questions. 6525 */ 6526 QUESTIONNAIRE, 6527 /** 6528 * A structured set of questions and their answers. The questions are ordered 6529 * and grouped into coherent subsets, corresponding to the structure of the 6530 * grouping of the underlying questions. 6531 */ 6532 QUESTIONNAIRERESPONSE, 6533 /** 6534 * Used to record and send details about a request for referral service or 6535 * transfer of a patient to the care of another provider or provider 6536 * organization. 6537 */ 6538 REFERRALREQUEST, 6539 /** 6540 * Information about a person that is involved in the care for a patient, but 6541 * who is not the target of healthcare, nor has a formal responsibility in the 6542 * care process. 6543 */ 6544 RELATEDPERSON, 6545 /** 6546 * --- Abstract Type! ---This is the base resource type for everything. 6547 */ 6548 RESOURCE, 6549 /** 6550 * An assessment of the likely outcome(s) for a patient or other subject as well 6551 * as the likelihood of each outcome. 6552 */ 6553 RISKASSESSMENT, 6554 /** 6555 * A container for slot(s) of time that may be available for booking 6556 * appointments. 6557 */ 6558 SCHEDULE, 6559 /** 6560 * A search parameter that defines a named search item that can be used to 6561 * search/filter on a resource. 6562 */ 6563 SEARCHPARAMETER, 6564 /** 6565 * A slot of time on a schedule that may be available for booking appointments. 6566 */ 6567 SLOT, 6568 /** 6569 * A sample to be used for analysis. 6570 */ 6571 SPECIMEN, 6572 /** 6573 * A definition of a FHIR structure. This resource is used to describe the 6574 * underlying resources, data types defined in FHIR, and also for describing 6575 * extensions, and constraints on resources and data types. 6576 */ 6577 STRUCTUREDEFINITION, 6578 /** 6579 * The subscription resource is used to define a push based subscription from a 6580 * server to another system. Once a subscription is registered with the server, 6581 * the server checks every resource that is created or updated, and if the 6582 * resource matches the given criteria, it sends a message on the defined 6583 * "channel" so that another system is able to take an appropriate action. 6584 */ 6585 SUBSCRIPTION, 6586 /** 6587 * A homogeneous material with a definite composition. 6588 */ 6589 SUBSTANCE, 6590 /** 6591 * Record of delivery of what is supplied. 6592 */ 6593 SUPPLYDELIVERY, 6594 /** 6595 * A record of a request for a medication, substance or device used in the 6596 * healthcare setting. 6597 */ 6598 SUPPLYREQUEST, 6599 /** 6600 * TestScript is a resource that specifies a suite of tests against a FHIR 6601 * server implementation to determine compliance against the FHIR specification. 6602 */ 6603 TESTSCRIPT, 6604 /** 6605 * A value set specifies a set of codes drawn from one or more code systems. 6606 */ 6607 VALUESET, 6608 /** 6609 * An authorization for the supply of glasses and/or contact lenses to a 6610 * patient. 6611 */ 6612 VISIONPRESCRIPTION, 6613 /** 6614 * added to help the parsers 6615 */ 6616 NULL; 6617 6618 public static ResourceType fromCode(String codeString) throws FHIRException { 6619 if (codeString == null || "".equals(codeString)) 6620 return null; 6621 if ("Account".equals(codeString)) 6622 return ACCOUNT; 6623 if ("AllergyIntolerance".equals(codeString)) 6624 return ALLERGYINTOLERANCE; 6625 if ("Appointment".equals(codeString)) 6626 return APPOINTMENT; 6627 if ("AppointmentResponse".equals(codeString)) 6628 return APPOINTMENTRESPONSE; 6629 if ("AuditEvent".equals(codeString)) 6630 return AUDITEVENT; 6631 if ("Basic".equals(codeString)) 6632 return BASIC; 6633 if ("Binary".equals(codeString)) 6634 return BINARY; 6635 if ("BodySite".equals(codeString)) 6636 return BODYSITE; 6637 if ("Bundle".equals(codeString)) 6638 return BUNDLE; 6639 if ("CarePlan".equals(codeString)) 6640 return CAREPLAN; 6641 if ("Claim".equals(codeString)) 6642 return CLAIM; 6643 if ("ClaimResponse".equals(codeString)) 6644 return CLAIMRESPONSE; 6645 if ("ClinicalImpression".equals(codeString)) 6646 return CLINICALIMPRESSION; 6647 if ("Communication".equals(codeString)) 6648 return COMMUNICATION; 6649 if ("CommunicationRequest".equals(codeString)) 6650 return COMMUNICATIONREQUEST; 6651 if ("Composition".equals(codeString)) 6652 return COMPOSITION; 6653 if ("ConceptMap".equals(codeString)) 6654 return CONCEPTMAP; 6655 if ("Condition".equals(codeString)) 6656 return CONDITION; 6657 if ("Conformance".equals(codeString)) 6658 return CONFORMANCE; 6659 if ("Contract".equals(codeString)) 6660 return CONTRACT; 6661 if ("Coverage".equals(codeString)) 6662 return COVERAGE; 6663 if ("DataElement".equals(codeString)) 6664 return DATAELEMENT; 6665 if ("DetectedIssue".equals(codeString)) 6666 return DETECTEDISSUE; 6667 if ("Device".equals(codeString)) 6668 return DEVICE; 6669 if ("DeviceComponent".equals(codeString)) 6670 return DEVICECOMPONENT; 6671 if ("DeviceMetric".equals(codeString)) 6672 return DEVICEMETRIC; 6673 if ("DeviceUseRequest".equals(codeString)) 6674 return DEVICEUSEREQUEST; 6675 if ("DeviceUseStatement".equals(codeString)) 6676 return DEVICEUSESTATEMENT; 6677 if ("DiagnosticOrder".equals(codeString)) 6678 return DIAGNOSTICORDER; 6679 if ("DiagnosticReport".equals(codeString)) 6680 return DIAGNOSTICREPORT; 6681 if ("DocumentManifest".equals(codeString)) 6682 return DOCUMENTMANIFEST; 6683 if ("DocumentReference".equals(codeString)) 6684 return DOCUMENTREFERENCE; 6685 if ("DomainResource".equals(codeString)) 6686 return DOMAINRESOURCE; 6687 if ("EligibilityRequest".equals(codeString)) 6688 return ELIGIBILITYREQUEST; 6689 if ("EligibilityResponse".equals(codeString)) 6690 return ELIGIBILITYRESPONSE; 6691 if ("Encounter".equals(codeString)) 6692 return ENCOUNTER; 6693 if ("EnrollmentRequest".equals(codeString)) 6694 return ENROLLMENTREQUEST; 6695 if ("EnrollmentResponse".equals(codeString)) 6696 return ENROLLMENTRESPONSE; 6697 if ("EpisodeOfCare".equals(codeString)) 6698 return EPISODEOFCARE; 6699 if ("ExplanationOfBenefit".equals(codeString)) 6700 return EXPLANATIONOFBENEFIT; 6701 if ("FamilyMemberHistory".equals(codeString)) 6702 return FAMILYMEMBERHISTORY; 6703 if ("Flag".equals(codeString)) 6704 return FLAG; 6705 if ("Goal".equals(codeString)) 6706 return GOAL; 6707 if ("Group".equals(codeString)) 6708 return GROUP; 6709 if ("HealthcareService".equals(codeString)) 6710 return HEALTHCARESERVICE; 6711 if ("ImagingObjectSelection".equals(codeString)) 6712 return IMAGINGOBJECTSELECTION; 6713 if ("ImagingStudy".equals(codeString)) 6714 return IMAGINGSTUDY; 6715 if ("Immunization".equals(codeString)) 6716 return IMMUNIZATION; 6717 if ("ImmunizationRecommendation".equals(codeString)) 6718 return IMMUNIZATIONRECOMMENDATION; 6719 if ("ImplementationGuide".equals(codeString)) 6720 return IMPLEMENTATIONGUIDE; 6721 if ("List".equals(codeString)) 6722 return LIST; 6723 if ("Location".equals(codeString)) 6724 return LOCATION; 6725 if ("Media".equals(codeString)) 6726 return MEDIA; 6727 if ("Medication".equals(codeString)) 6728 return MEDICATION; 6729 if ("MedicationAdministration".equals(codeString)) 6730 return MEDICATIONADMINISTRATION; 6731 if ("MedicationDispense".equals(codeString)) 6732 return MEDICATIONDISPENSE; 6733 if ("MedicationOrder".equals(codeString)) 6734 return MEDICATIONORDER; 6735 if ("MedicationStatement".equals(codeString)) 6736 return MEDICATIONSTATEMENT; 6737 if ("MessageHeader".equals(codeString)) 6738 return MESSAGEHEADER; 6739 if ("NamingSystem".equals(codeString)) 6740 return NAMINGSYSTEM; 6741 if ("NutritionOrder".equals(codeString)) 6742 return NUTRITIONORDER; 6743 if ("Observation".equals(codeString)) 6744 return OBSERVATION; 6745 if ("OperationDefinition".equals(codeString)) 6746 return OPERATIONDEFINITION; 6747 if ("OperationOutcome".equals(codeString)) 6748 return OPERATIONOUTCOME; 6749 if ("Order".equals(codeString)) 6750 return ORDER; 6751 if ("OrderResponse".equals(codeString)) 6752 return ORDERRESPONSE; 6753 if ("Organization".equals(codeString)) 6754 return ORGANIZATION; 6755 if ("Parameters".equals(codeString)) 6756 return PARAMETERS; 6757 if ("Patient".equals(codeString)) 6758 return PATIENT; 6759 if ("PaymentNotice".equals(codeString)) 6760 return PAYMENTNOTICE; 6761 if ("PaymentReconciliation".equals(codeString)) 6762 return PAYMENTRECONCILIATION; 6763 if ("Person".equals(codeString)) 6764 return PERSON; 6765 if ("Practitioner".equals(codeString)) 6766 return PRACTITIONER; 6767 if ("Procedure".equals(codeString)) 6768 return PROCEDURE; 6769 if ("ProcedureRequest".equals(codeString)) 6770 return PROCEDUREREQUEST; 6771 if ("ProcessRequest".equals(codeString)) 6772 return PROCESSREQUEST; 6773 if ("ProcessResponse".equals(codeString)) 6774 return PROCESSRESPONSE; 6775 if ("Provenance".equals(codeString)) 6776 return PROVENANCE; 6777 if ("Questionnaire".equals(codeString)) 6778 return QUESTIONNAIRE; 6779 if ("QuestionnaireResponse".equals(codeString)) 6780 return QUESTIONNAIRERESPONSE; 6781 if ("ReferralRequest".equals(codeString)) 6782 return REFERRALREQUEST; 6783 if ("RelatedPerson".equals(codeString)) 6784 return RELATEDPERSON; 6785 if ("Resource".equals(codeString)) 6786 return RESOURCE; 6787 if ("RiskAssessment".equals(codeString)) 6788 return RISKASSESSMENT; 6789 if ("Schedule".equals(codeString)) 6790 return SCHEDULE; 6791 if ("SearchParameter".equals(codeString)) 6792 return SEARCHPARAMETER; 6793 if ("Slot".equals(codeString)) 6794 return SLOT; 6795 if ("Specimen".equals(codeString)) 6796 return SPECIMEN; 6797 if ("StructureDefinition".equals(codeString)) 6798 return STRUCTUREDEFINITION; 6799 if ("Subscription".equals(codeString)) 6800 return SUBSCRIPTION; 6801 if ("Substance".equals(codeString)) 6802 return SUBSTANCE; 6803 if ("SupplyDelivery".equals(codeString)) 6804 return SUPPLYDELIVERY; 6805 if ("SupplyRequest".equals(codeString)) 6806 return SUPPLYREQUEST; 6807 if ("TestScript".equals(codeString)) 6808 return TESTSCRIPT; 6809 if ("ValueSet".equals(codeString)) 6810 return VALUESET; 6811 if ("VisionPrescription".equals(codeString)) 6812 return VISIONPRESCRIPTION; 6813 throw new FHIRException("Unknown ResourceType code '" + codeString + "'"); 6814 } 6815 6816 public String toCode() { 6817 switch (this) { 6818 case ACCOUNT: 6819 return "Account"; 6820 case ALLERGYINTOLERANCE: 6821 return "AllergyIntolerance"; 6822 case APPOINTMENT: 6823 return "Appointment"; 6824 case APPOINTMENTRESPONSE: 6825 return "AppointmentResponse"; 6826 case AUDITEVENT: 6827 return "AuditEvent"; 6828 case BASIC: 6829 return "Basic"; 6830 case BINARY: 6831 return "Binary"; 6832 case BODYSITE: 6833 return "BodySite"; 6834 case BUNDLE: 6835 return "Bundle"; 6836 case CAREPLAN: 6837 return "CarePlan"; 6838 case CLAIM: 6839 return "Claim"; 6840 case CLAIMRESPONSE: 6841 return "ClaimResponse"; 6842 case CLINICALIMPRESSION: 6843 return "ClinicalImpression"; 6844 case COMMUNICATION: 6845 return "Communication"; 6846 case COMMUNICATIONREQUEST: 6847 return "CommunicationRequest"; 6848 case COMPOSITION: 6849 return "Composition"; 6850 case CONCEPTMAP: 6851 return "ConceptMap"; 6852 case CONDITION: 6853 return "Condition"; 6854 case CONFORMANCE: 6855 return "Conformance"; 6856 case CONTRACT: 6857 return "Contract"; 6858 case COVERAGE: 6859 return "Coverage"; 6860 case DATAELEMENT: 6861 return "DataElement"; 6862 case DETECTEDISSUE: 6863 return "DetectedIssue"; 6864 case DEVICE: 6865 return "Device"; 6866 case DEVICECOMPONENT: 6867 return "DeviceComponent"; 6868 case DEVICEMETRIC: 6869 return "DeviceMetric"; 6870 case DEVICEUSEREQUEST: 6871 return "DeviceUseRequest"; 6872 case DEVICEUSESTATEMENT: 6873 return "DeviceUseStatement"; 6874 case DIAGNOSTICORDER: 6875 return "DiagnosticOrder"; 6876 case DIAGNOSTICREPORT: 6877 return "DiagnosticReport"; 6878 case DOCUMENTMANIFEST: 6879 return "DocumentManifest"; 6880 case DOCUMENTREFERENCE: 6881 return "DocumentReference"; 6882 case DOMAINRESOURCE: 6883 return "DomainResource"; 6884 case ELIGIBILITYREQUEST: 6885 return "EligibilityRequest"; 6886 case ELIGIBILITYRESPONSE: 6887 return "EligibilityResponse"; 6888 case ENCOUNTER: 6889 return "Encounter"; 6890 case ENROLLMENTREQUEST: 6891 return "EnrollmentRequest"; 6892 case ENROLLMENTRESPONSE: 6893 return "EnrollmentResponse"; 6894 case EPISODEOFCARE: 6895 return "EpisodeOfCare"; 6896 case EXPLANATIONOFBENEFIT: 6897 return "ExplanationOfBenefit"; 6898 case FAMILYMEMBERHISTORY: 6899 return "FamilyMemberHistory"; 6900 case FLAG: 6901 return "Flag"; 6902 case GOAL: 6903 return "Goal"; 6904 case GROUP: 6905 return "Group"; 6906 case HEALTHCARESERVICE: 6907 return "HealthcareService"; 6908 case IMAGINGOBJECTSELECTION: 6909 return "ImagingObjectSelection"; 6910 case IMAGINGSTUDY: 6911 return "ImagingStudy"; 6912 case IMMUNIZATION: 6913 return "Immunization"; 6914 case IMMUNIZATIONRECOMMENDATION: 6915 return "ImmunizationRecommendation"; 6916 case IMPLEMENTATIONGUIDE: 6917 return "ImplementationGuide"; 6918 case LIST: 6919 return "List"; 6920 case LOCATION: 6921 return "Location"; 6922 case MEDIA: 6923 return "Media"; 6924 case MEDICATION: 6925 return "Medication"; 6926 case MEDICATIONADMINISTRATION: 6927 return "MedicationAdministration"; 6928 case MEDICATIONDISPENSE: 6929 return "MedicationDispense"; 6930 case MEDICATIONORDER: 6931 return "MedicationOrder"; 6932 case MEDICATIONSTATEMENT: 6933 return "MedicationStatement"; 6934 case MESSAGEHEADER: 6935 return "MessageHeader"; 6936 case NAMINGSYSTEM: 6937 return "NamingSystem"; 6938 case NUTRITIONORDER: 6939 return "NutritionOrder"; 6940 case OBSERVATION: 6941 return "Observation"; 6942 case OPERATIONDEFINITION: 6943 return "OperationDefinition"; 6944 case OPERATIONOUTCOME: 6945 return "OperationOutcome"; 6946 case ORDER: 6947 return "Order"; 6948 case ORDERRESPONSE: 6949 return "OrderResponse"; 6950 case ORGANIZATION: 6951 return "Organization"; 6952 case PARAMETERS: 6953 return "Parameters"; 6954 case PATIENT: 6955 return "Patient"; 6956 case PAYMENTNOTICE: 6957 return "PaymentNotice"; 6958 case PAYMENTRECONCILIATION: 6959 return "PaymentReconciliation"; 6960 case PERSON: 6961 return "Person"; 6962 case PRACTITIONER: 6963 return "Practitioner"; 6964 case PROCEDURE: 6965 return "Procedure"; 6966 case PROCEDUREREQUEST: 6967 return "ProcedureRequest"; 6968 case PROCESSREQUEST: 6969 return "ProcessRequest"; 6970 case PROCESSRESPONSE: 6971 return "ProcessResponse"; 6972 case PROVENANCE: 6973 return "Provenance"; 6974 case QUESTIONNAIRE: 6975 return "Questionnaire"; 6976 case QUESTIONNAIRERESPONSE: 6977 return "QuestionnaireResponse"; 6978 case REFERRALREQUEST: 6979 return "ReferralRequest"; 6980 case RELATEDPERSON: 6981 return "RelatedPerson"; 6982 case RESOURCE: 6983 return "Resource"; 6984 case RISKASSESSMENT: 6985 return "RiskAssessment"; 6986 case SCHEDULE: 6987 return "Schedule"; 6988 case SEARCHPARAMETER: 6989 return "SearchParameter"; 6990 case SLOT: 6991 return "Slot"; 6992 case SPECIMEN: 6993 return "Specimen"; 6994 case STRUCTUREDEFINITION: 6995 return "StructureDefinition"; 6996 case SUBSCRIPTION: 6997 return "Subscription"; 6998 case SUBSTANCE: 6999 return "Substance"; 7000 case SUPPLYDELIVERY: 7001 return "SupplyDelivery"; 7002 case SUPPLYREQUEST: 7003 return "SupplyRequest"; 7004 case TESTSCRIPT: 7005 return "TestScript"; 7006 case VALUESET: 7007 return "ValueSet"; 7008 case VISIONPRESCRIPTION: 7009 return "VisionPrescription"; 7010 case NULL: 7011 return null; 7012 default: 7013 return "?"; 7014 } 7015 } 7016 7017 public String getSystem() { 7018 switch (this) { 7019 case ACCOUNT: 7020 return "http://hl7.org/fhir/resource-types"; 7021 case ALLERGYINTOLERANCE: 7022 return "http://hl7.org/fhir/resource-types"; 7023 case APPOINTMENT: 7024 return "http://hl7.org/fhir/resource-types"; 7025 case APPOINTMENTRESPONSE: 7026 return "http://hl7.org/fhir/resource-types"; 7027 case AUDITEVENT: 7028 return "http://hl7.org/fhir/resource-types"; 7029 case BASIC: 7030 return "http://hl7.org/fhir/resource-types"; 7031 case BINARY: 7032 return "http://hl7.org/fhir/resource-types"; 7033 case BODYSITE: 7034 return "http://hl7.org/fhir/resource-types"; 7035 case BUNDLE: 7036 return "http://hl7.org/fhir/resource-types"; 7037 case CAREPLAN: 7038 return "http://hl7.org/fhir/resource-types"; 7039 case CLAIM: 7040 return "http://hl7.org/fhir/resource-types"; 7041 case CLAIMRESPONSE: 7042 return "http://hl7.org/fhir/resource-types"; 7043 case CLINICALIMPRESSION: 7044 return "http://hl7.org/fhir/resource-types"; 7045 case COMMUNICATION: 7046 return "http://hl7.org/fhir/resource-types"; 7047 case COMMUNICATIONREQUEST: 7048 return "http://hl7.org/fhir/resource-types"; 7049 case COMPOSITION: 7050 return "http://hl7.org/fhir/resource-types"; 7051 case CONCEPTMAP: 7052 return "http://hl7.org/fhir/resource-types"; 7053 case CONDITION: 7054 return "http://hl7.org/fhir/resource-types"; 7055 case CONFORMANCE: 7056 return "http://hl7.org/fhir/resource-types"; 7057 case CONTRACT: 7058 return "http://hl7.org/fhir/resource-types"; 7059 case COVERAGE: 7060 return "http://hl7.org/fhir/resource-types"; 7061 case DATAELEMENT: 7062 return "http://hl7.org/fhir/resource-types"; 7063 case DETECTEDISSUE: 7064 return "http://hl7.org/fhir/resource-types"; 7065 case DEVICE: 7066 return "http://hl7.org/fhir/resource-types"; 7067 case DEVICECOMPONENT: 7068 return "http://hl7.org/fhir/resource-types"; 7069 case DEVICEMETRIC: 7070 return "http://hl7.org/fhir/resource-types"; 7071 case DEVICEUSEREQUEST: 7072 return "http://hl7.org/fhir/resource-types"; 7073 case DEVICEUSESTATEMENT: 7074 return "http://hl7.org/fhir/resource-types"; 7075 case DIAGNOSTICORDER: 7076 return "http://hl7.org/fhir/resource-types"; 7077 case DIAGNOSTICREPORT: 7078 return "http://hl7.org/fhir/resource-types"; 7079 case DOCUMENTMANIFEST: 7080 return "http://hl7.org/fhir/resource-types"; 7081 case DOCUMENTREFERENCE: 7082 return "http://hl7.org/fhir/resource-types"; 7083 case DOMAINRESOURCE: 7084 return "http://hl7.org/fhir/resource-types"; 7085 case ELIGIBILITYREQUEST: 7086 return "http://hl7.org/fhir/resource-types"; 7087 case ELIGIBILITYRESPONSE: 7088 return "http://hl7.org/fhir/resource-types"; 7089 case ENCOUNTER: 7090 return "http://hl7.org/fhir/resource-types"; 7091 case ENROLLMENTREQUEST: 7092 return "http://hl7.org/fhir/resource-types"; 7093 case ENROLLMENTRESPONSE: 7094 return "http://hl7.org/fhir/resource-types"; 7095 case EPISODEOFCARE: 7096 return "http://hl7.org/fhir/resource-types"; 7097 case EXPLANATIONOFBENEFIT: 7098 return "http://hl7.org/fhir/resource-types"; 7099 case FAMILYMEMBERHISTORY: 7100 return "http://hl7.org/fhir/resource-types"; 7101 case FLAG: 7102 return "http://hl7.org/fhir/resource-types"; 7103 case GOAL: 7104 return "http://hl7.org/fhir/resource-types"; 7105 case GROUP: 7106 return "http://hl7.org/fhir/resource-types"; 7107 case HEALTHCARESERVICE: 7108 return "http://hl7.org/fhir/resource-types"; 7109 case IMAGINGOBJECTSELECTION: 7110 return "http://hl7.org/fhir/resource-types"; 7111 case IMAGINGSTUDY: 7112 return "http://hl7.org/fhir/resource-types"; 7113 case IMMUNIZATION: 7114 return "http://hl7.org/fhir/resource-types"; 7115 case IMMUNIZATIONRECOMMENDATION: 7116 return "http://hl7.org/fhir/resource-types"; 7117 case IMPLEMENTATIONGUIDE: 7118 return "http://hl7.org/fhir/resource-types"; 7119 case LIST: 7120 return "http://hl7.org/fhir/resource-types"; 7121 case LOCATION: 7122 return "http://hl7.org/fhir/resource-types"; 7123 case MEDIA: 7124 return "http://hl7.org/fhir/resource-types"; 7125 case MEDICATION: 7126 return "http://hl7.org/fhir/resource-types"; 7127 case MEDICATIONADMINISTRATION: 7128 return "http://hl7.org/fhir/resource-types"; 7129 case MEDICATIONDISPENSE: 7130 return "http://hl7.org/fhir/resource-types"; 7131 case MEDICATIONORDER: 7132 return "http://hl7.org/fhir/resource-types"; 7133 case MEDICATIONSTATEMENT: 7134 return "http://hl7.org/fhir/resource-types"; 7135 case MESSAGEHEADER: 7136 return "http://hl7.org/fhir/resource-types"; 7137 case NAMINGSYSTEM: 7138 return "http://hl7.org/fhir/resource-types"; 7139 case NUTRITIONORDER: 7140 return "http://hl7.org/fhir/resource-types"; 7141 case OBSERVATION: 7142 return "http://hl7.org/fhir/resource-types"; 7143 case OPERATIONDEFINITION: 7144 return "http://hl7.org/fhir/resource-types"; 7145 case OPERATIONOUTCOME: 7146 return "http://hl7.org/fhir/resource-types"; 7147 case ORDER: 7148 return "http://hl7.org/fhir/resource-types"; 7149 case ORDERRESPONSE: 7150 return "http://hl7.org/fhir/resource-types"; 7151 case ORGANIZATION: 7152 return "http://hl7.org/fhir/resource-types"; 7153 case PARAMETERS: 7154 return "http://hl7.org/fhir/resource-types"; 7155 case PATIENT: 7156 return "http://hl7.org/fhir/resource-types"; 7157 case PAYMENTNOTICE: 7158 return "http://hl7.org/fhir/resource-types"; 7159 case PAYMENTRECONCILIATION: 7160 return "http://hl7.org/fhir/resource-types"; 7161 case PERSON: 7162 return "http://hl7.org/fhir/resource-types"; 7163 case PRACTITIONER: 7164 return "http://hl7.org/fhir/resource-types"; 7165 case PROCEDURE: 7166 return "http://hl7.org/fhir/resource-types"; 7167 case PROCEDUREREQUEST: 7168 return "http://hl7.org/fhir/resource-types"; 7169 case PROCESSREQUEST: 7170 return "http://hl7.org/fhir/resource-types"; 7171 case PROCESSRESPONSE: 7172 return "http://hl7.org/fhir/resource-types"; 7173 case PROVENANCE: 7174 return "http://hl7.org/fhir/resource-types"; 7175 case QUESTIONNAIRE: 7176 return "http://hl7.org/fhir/resource-types"; 7177 case QUESTIONNAIRERESPONSE: 7178 return "http://hl7.org/fhir/resource-types"; 7179 case REFERRALREQUEST: 7180 return "http://hl7.org/fhir/resource-types"; 7181 case RELATEDPERSON: 7182 return "http://hl7.org/fhir/resource-types"; 7183 case RESOURCE: 7184 return "http://hl7.org/fhir/resource-types"; 7185 case RISKASSESSMENT: 7186 return "http://hl7.org/fhir/resource-types"; 7187 case SCHEDULE: 7188 return "http://hl7.org/fhir/resource-types"; 7189 case SEARCHPARAMETER: 7190 return "http://hl7.org/fhir/resource-types"; 7191 case SLOT: 7192 return "http://hl7.org/fhir/resource-types"; 7193 case SPECIMEN: 7194 return "http://hl7.org/fhir/resource-types"; 7195 case STRUCTUREDEFINITION: 7196 return "http://hl7.org/fhir/resource-types"; 7197 case SUBSCRIPTION: 7198 return "http://hl7.org/fhir/resource-types"; 7199 case SUBSTANCE: 7200 return "http://hl7.org/fhir/resource-types"; 7201 case SUPPLYDELIVERY: 7202 return "http://hl7.org/fhir/resource-types"; 7203 case SUPPLYREQUEST: 7204 return "http://hl7.org/fhir/resource-types"; 7205 case TESTSCRIPT: 7206 return "http://hl7.org/fhir/resource-types"; 7207 case VALUESET: 7208 return "http://hl7.org/fhir/resource-types"; 7209 case VISIONPRESCRIPTION: 7210 return "http://hl7.org/fhir/resource-types"; 7211 case NULL: 7212 return null; 7213 default: 7214 return "?"; 7215 } 7216 } 7217 7218 public String getDefinition() { 7219 switch (this) { 7220 case ACCOUNT: 7221 return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centres, etc."; 7222 case ALLERGYINTOLERANCE: 7223 return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 7224 case APPOINTMENT: 7225 return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 7226 case APPOINTMENTRESPONSE: 7227 return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 7228 case AUDITEVENT: 7229 return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 7230 case BASIC: 7231 return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 7232 case BINARY: 7233 return "A binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 7234 case BODYSITE: 7235 return "Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 7236 case BUNDLE: 7237 return "A container for a collection of resources."; 7238 case CAREPLAN: 7239 return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 7240 case CLAIM: 7241 return "A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery."; 7242 case CLAIMRESPONSE: 7243 return "This resource provides the adjudication details from the processing of a Claim resource."; 7244 case CLINICALIMPRESSION: 7245 return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 7246 case COMMUNICATION: 7247 return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition."; 7248 case COMMUNICATIONREQUEST: 7249 return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 7250 case COMPOSITION: 7251 return "A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained."; 7252 case CONCEPTMAP: 7253 return "A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models."; 7254 case CONDITION: 7255 return "Use to record detailed information about conditions, problems or diagnoses recognized by a clinician. There are many uses including: recording a diagnosis during an encounter; populating a problem list or a summary statement, such as a discharge summary."; 7256 case CONFORMANCE: 7257 return "A conformance statement is a set of capabilities of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 7258 case CONTRACT: 7259 return "A formal agreement between parties regarding the conduct of business, exchange of information or other matters."; 7260 case COVERAGE: 7261 return "Financial instrument which may be used to pay for or reimburse health care products and services."; 7262 case DATAELEMENT: 7263 return "The formal description of a single piece of information that can be gathered and reported."; 7264 case DETECTEDISSUE: 7265 return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 7266 case DEVICE: 7267 return "This resource identifies an instance of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices includes durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc."; 7268 case DEVICECOMPONENT: 7269 return "Describes the characteristics, operational status and capabilities of a medical-related component of a medical device."; 7270 case DEVICEMETRIC: 7271 return "Describes a measurement, calculation or setting capability of a medical device."; 7272 case DEVICEUSEREQUEST: 7273 return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 7274 case DEVICEUSESTATEMENT: 7275 return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 7276 case DIAGNOSTICORDER: 7277 return "A record of a request for a diagnostic investigation service to be performed."; 7278 case DIAGNOSTICREPORT: 7279 return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 7280 case DOCUMENTMANIFEST: 7281 return "A manifest that defines a set of documents."; 7282 case DOCUMENTREFERENCE: 7283 return "A reference to a document ."; 7284 case DOMAINRESOURCE: 7285 return "--- Abstract Type! ---A resource that includes narrative, extensions, and contained resources."; 7286 case ELIGIBILITYREQUEST: 7287 return "This resource provides the insurance eligibility details from the insurer regarding a specified coverage and optionally some class of service."; 7288 case ELIGIBILITYRESPONSE: 7289 return "This resource provides eligibility and plan details from the processing of an Eligibility resource."; 7290 case ENCOUNTER: 7291 return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 7292 case ENROLLMENTREQUEST: 7293 return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 7294 case ENROLLMENTRESPONSE: 7295 return "This resource provides enrollment and plan details from the processing of an Enrollment resource."; 7296 case EPISODEOFCARE: 7297 return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 7298 case EXPLANATIONOFBENEFIT: 7299 return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 7300 case FAMILYMEMBERHISTORY: 7301 return "Significant health events and conditions for a person related to the patient relevant in the context of care for the patient."; 7302 case FLAG: 7303 return "Prospective warnings of potential issues when providing care to the patient."; 7304 case GOAL: 7305 return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 7306 case GROUP: 7307 return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 7308 case HEALTHCARESERVICE: 7309 return "The details of a healthcare service available at a location."; 7310 case IMAGINGOBJECTSELECTION: 7311 return "A manifest of a set of DICOM Service-Object Pair Instances (SOP Instances). The referenced SOP Instances (images or other content) are for a single patient, and may be from one or more studies. The referenced SOP Instances have been selected for a purpose, such as quality assurance, conference, or consult. Reflecting that range of purposes, typical ImagingObjectSelection resources may include all SOP Instances in a study (perhaps for sharing through a Health Information Exchange); key images from multiple studies (for reference by a referring or treating physician); a multi-frame ultrasound instance (\"cine\" video clip) and a set of measurements taken from that instance (for inclusion in a teaching file); and so on."; 7312 case IMAGINGSTUDY: 7313 return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 7314 case IMMUNIZATION: 7315 return "Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed."; 7316 case IMMUNIZATIONRECOMMENDATION: 7317 return "A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification."; 7318 case IMPLEMENTATIONGUIDE: 7319 return "A set of rules or how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole, and to publish a computable definition of all the parts."; 7320 case LIST: 7321 return "A set of information summarized from a list of other resources."; 7322 case LOCATION: 7323 return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated."; 7324 case MEDIA: 7325 return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 7326 case MEDICATION: 7327 return "This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication."; 7328 case MEDICATIONADMINISTRATION: 7329 return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 7330 case MEDICATIONDISPENSE: 7331 return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 7332 case MEDICATIONORDER: 7333 return "An order for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationOrder\" rather than \"MedicationPrescription\" to generalize the use across inpatient and outpatient settings as well as for care plans, etc."; 7334 case MEDICATIONSTATEMENT: 7335 return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from e.g. the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains \r\rThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 7336 case MESSAGEHEADER: 7337 return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 7338 case NAMINGSYSTEM: 7339 return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 7340 case NUTRITIONORDER: 7341 return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 7342 case OBSERVATION: 7343 return "Measurements and simple assertions made about a patient, device or other subject."; 7344 case OPERATIONDEFINITION: 7345 return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 7346 case OPERATIONOUTCOME: 7347 return "A collection of error, warning or information messages that result from a system action."; 7348 case ORDER: 7349 return "A request to perform an action."; 7350 case ORDERRESPONSE: 7351 return "A response to an order."; 7352 case ORGANIZATION: 7353 return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc."; 7354 case PARAMETERS: 7355 return "This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 7356 case PATIENT: 7357 return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 7358 case PAYMENTNOTICE: 7359 return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 7360 case PAYMENTRECONCILIATION: 7361 return "This resource provides payment details and claim references supporting a bulk payment."; 7362 case PERSON: 7363 return "Demographics and administrative information about a person independent of a specific health-related context."; 7364 case PRACTITIONER: 7365 return "A person who is directly or indirectly involved in the provisioning of healthcare."; 7366 case PROCEDURE: 7367 return "An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy."; 7368 case PROCEDUREREQUEST: 7369 return "A request for a procedure to be performed. May be a proposal or an order."; 7370 case PROCESSREQUEST: 7371 return "This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources."; 7372 case PROCESSRESPONSE: 7373 return "This resource provides processing status, errors and notes from the processing of a resource."; 7374 case PROVENANCE: 7375 return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 7376 case QUESTIONNAIRE: 7377 return "A structured set of questions intended to guide the collection of answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the underlying questions."; 7378 case QUESTIONNAIRERESPONSE: 7379 return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the underlying questions."; 7380 case REFERRALREQUEST: 7381 return "Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization."; 7382 case RELATEDPERSON: 7383 return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 7384 case RESOURCE: 7385 return "--- Abstract Type! ---This is the base resource type for everything."; 7386 case RISKASSESSMENT: 7387 return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 7388 case SCHEDULE: 7389 return "A container for slot(s) of time that may be available for booking appointments."; 7390 case SEARCHPARAMETER: 7391 return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 7392 case SLOT: 7393 return "A slot of time on a schedule that may be available for booking appointments."; 7394 case SPECIMEN: 7395 return "A sample to be used for analysis."; 7396 case STRUCTUREDEFINITION: 7397 return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions, and constraints on resources and data types."; 7398 case SUBSCRIPTION: 7399 return "The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system is able to take an appropriate action."; 7400 case SUBSTANCE: 7401 return "A homogeneous material with a definite composition."; 7402 case SUPPLYDELIVERY: 7403 return "Record of delivery of what is supplied."; 7404 case SUPPLYREQUEST: 7405 return "A record of a request for a medication, substance or device used in the healthcare setting."; 7406 case TESTSCRIPT: 7407 return "TestScript is a resource that specifies a suite of tests against a FHIR server implementation to determine compliance against the FHIR specification."; 7408 case VALUESET: 7409 return "A value set specifies a set of codes drawn from one or more code systems."; 7410 case VISIONPRESCRIPTION: 7411 return "An authorization for the supply of glasses and/or contact lenses to a patient."; 7412 case NULL: 7413 return null; 7414 default: 7415 return "?"; 7416 } 7417 } 7418 7419 public String getDisplay() { 7420 switch (this) { 7421 case ACCOUNT: 7422 return "Account"; 7423 case ALLERGYINTOLERANCE: 7424 return "AllergyIntolerance"; 7425 case APPOINTMENT: 7426 return "Appointment"; 7427 case APPOINTMENTRESPONSE: 7428 return "AppointmentResponse"; 7429 case AUDITEVENT: 7430 return "AuditEvent"; 7431 case BASIC: 7432 return "Basic"; 7433 case BINARY: 7434 return "Binary"; 7435 case BODYSITE: 7436 return "BodySite"; 7437 case BUNDLE: 7438 return "Bundle"; 7439 case CAREPLAN: 7440 return "CarePlan"; 7441 case CLAIM: 7442 return "Claim"; 7443 case CLAIMRESPONSE: 7444 return "ClaimResponse"; 7445 case CLINICALIMPRESSION: 7446 return "ClinicalImpression"; 7447 case COMMUNICATION: 7448 return "Communication"; 7449 case COMMUNICATIONREQUEST: 7450 return "CommunicationRequest"; 7451 case COMPOSITION: 7452 return "Composition"; 7453 case CONCEPTMAP: 7454 return "ConceptMap"; 7455 case CONDITION: 7456 return "Condition"; 7457 case CONFORMANCE: 7458 return "Conformance"; 7459 case CONTRACT: 7460 return "Contract"; 7461 case COVERAGE: 7462 return "Coverage"; 7463 case DATAELEMENT: 7464 return "DataElement"; 7465 case DETECTEDISSUE: 7466 return "DetectedIssue"; 7467 case DEVICE: 7468 return "Device"; 7469 case DEVICECOMPONENT: 7470 return "DeviceComponent"; 7471 case DEVICEMETRIC: 7472 return "DeviceMetric"; 7473 case DEVICEUSEREQUEST: 7474 return "DeviceUseRequest"; 7475 case DEVICEUSESTATEMENT: 7476 return "DeviceUseStatement"; 7477 case DIAGNOSTICORDER: 7478 return "DiagnosticOrder"; 7479 case DIAGNOSTICREPORT: 7480 return "DiagnosticReport"; 7481 case DOCUMENTMANIFEST: 7482 return "DocumentManifest"; 7483 case DOCUMENTREFERENCE: 7484 return "DocumentReference"; 7485 case DOMAINRESOURCE: 7486 return "DomainResource"; 7487 case ELIGIBILITYREQUEST: 7488 return "EligibilityRequest"; 7489 case ELIGIBILITYRESPONSE: 7490 return "EligibilityResponse"; 7491 case ENCOUNTER: 7492 return "Encounter"; 7493 case ENROLLMENTREQUEST: 7494 return "EnrollmentRequest"; 7495 case ENROLLMENTRESPONSE: 7496 return "EnrollmentResponse"; 7497 case EPISODEOFCARE: 7498 return "EpisodeOfCare"; 7499 case EXPLANATIONOFBENEFIT: 7500 return "ExplanationOfBenefit"; 7501 case FAMILYMEMBERHISTORY: 7502 return "FamilyMemberHistory"; 7503 case FLAG: 7504 return "Flag"; 7505 case GOAL: 7506 return "Goal"; 7507 case GROUP: 7508 return "Group"; 7509 case HEALTHCARESERVICE: 7510 return "HealthcareService"; 7511 case IMAGINGOBJECTSELECTION: 7512 return "ImagingObjectSelection"; 7513 case IMAGINGSTUDY: 7514 return "ImagingStudy"; 7515 case IMMUNIZATION: 7516 return "Immunization"; 7517 case IMMUNIZATIONRECOMMENDATION: 7518 return "ImmunizationRecommendation"; 7519 case IMPLEMENTATIONGUIDE: 7520 return "ImplementationGuide"; 7521 case LIST: 7522 return "List"; 7523 case LOCATION: 7524 return "Location"; 7525 case MEDIA: 7526 return "Media"; 7527 case MEDICATION: 7528 return "Medication"; 7529 case MEDICATIONADMINISTRATION: 7530 return "MedicationAdministration"; 7531 case MEDICATIONDISPENSE: 7532 return "MedicationDispense"; 7533 case MEDICATIONORDER: 7534 return "MedicationOrder"; 7535 case MEDICATIONSTATEMENT: 7536 return "MedicationStatement"; 7537 case MESSAGEHEADER: 7538 return "MessageHeader"; 7539 case NAMINGSYSTEM: 7540 return "NamingSystem"; 7541 case NUTRITIONORDER: 7542 return "NutritionOrder"; 7543 case OBSERVATION: 7544 return "Observation"; 7545 case OPERATIONDEFINITION: 7546 return "OperationDefinition"; 7547 case OPERATIONOUTCOME: 7548 return "OperationOutcome"; 7549 case ORDER: 7550 return "Order"; 7551 case ORDERRESPONSE: 7552 return "OrderResponse"; 7553 case ORGANIZATION: 7554 return "Organization"; 7555 case PARAMETERS: 7556 return "Parameters"; 7557 case PATIENT: 7558 return "Patient"; 7559 case PAYMENTNOTICE: 7560 return "PaymentNotice"; 7561 case PAYMENTRECONCILIATION: 7562 return "PaymentReconciliation"; 7563 case PERSON: 7564 return "Person"; 7565 case PRACTITIONER: 7566 return "Practitioner"; 7567 case PROCEDURE: 7568 return "Procedure"; 7569 case PROCEDUREREQUEST: 7570 return "ProcedureRequest"; 7571 case PROCESSREQUEST: 7572 return "ProcessRequest"; 7573 case PROCESSRESPONSE: 7574 return "ProcessResponse"; 7575 case PROVENANCE: 7576 return "Provenance"; 7577 case QUESTIONNAIRE: 7578 return "Questionnaire"; 7579 case QUESTIONNAIRERESPONSE: 7580 return "QuestionnaireResponse"; 7581 case REFERRALREQUEST: 7582 return "ReferralRequest"; 7583 case RELATEDPERSON: 7584 return "RelatedPerson"; 7585 case RESOURCE: 7586 return "Resource"; 7587 case RISKASSESSMENT: 7588 return "RiskAssessment"; 7589 case SCHEDULE: 7590 return "Schedule"; 7591 case SEARCHPARAMETER: 7592 return "SearchParameter"; 7593 case SLOT: 7594 return "Slot"; 7595 case SPECIMEN: 7596 return "Specimen"; 7597 case STRUCTUREDEFINITION: 7598 return "StructureDefinition"; 7599 case SUBSCRIPTION: 7600 return "Subscription"; 7601 case SUBSTANCE: 7602 return "Substance"; 7603 case SUPPLYDELIVERY: 7604 return "SupplyDelivery"; 7605 case SUPPLYREQUEST: 7606 return "SupplyRequest"; 7607 case TESTSCRIPT: 7608 return "TestScript"; 7609 case VALUESET: 7610 return "ValueSet"; 7611 case VISIONPRESCRIPTION: 7612 return "VisionPrescription"; 7613 case NULL: 7614 return null; 7615 default: 7616 return "?"; 7617 } 7618 } 7619 } 7620 7621 public static class ResourceTypeEnumFactory implements EnumFactory<ResourceType> { 7622 public ResourceType fromCode(String codeString) throws IllegalArgumentException { 7623 if (codeString == null || "".equals(codeString)) 7624 if (codeString == null || "".equals(codeString)) 7625 return null; 7626 if ("Account".equals(codeString)) 7627 return ResourceType.ACCOUNT; 7628 if ("AllergyIntolerance".equals(codeString)) 7629 return ResourceType.ALLERGYINTOLERANCE; 7630 if ("Appointment".equals(codeString)) 7631 return ResourceType.APPOINTMENT; 7632 if ("AppointmentResponse".equals(codeString)) 7633 return ResourceType.APPOINTMENTRESPONSE; 7634 if ("AuditEvent".equals(codeString)) 7635 return ResourceType.AUDITEVENT; 7636 if ("Basic".equals(codeString)) 7637 return ResourceType.BASIC; 7638 if ("Binary".equals(codeString)) 7639 return ResourceType.BINARY; 7640 if ("BodySite".equals(codeString)) 7641 return ResourceType.BODYSITE; 7642 if ("Bundle".equals(codeString)) 7643 return ResourceType.BUNDLE; 7644 if ("CarePlan".equals(codeString)) 7645 return ResourceType.CAREPLAN; 7646 if ("Claim".equals(codeString)) 7647 return ResourceType.CLAIM; 7648 if ("ClaimResponse".equals(codeString)) 7649 return ResourceType.CLAIMRESPONSE; 7650 if ("ClinicalImpression".equals(codeString)) 7651 return ResourceType.CLINICALIMPRESSION; 7652 if ("Communication".equals(codeString)) 7653 return ResourceType.COMMUNICATION; 7654 if ("CommunicationRequest".equals(codeString)) 7655 return ResourceType.COMMUNICATIONREQUEST; 7656 if ("Composition".equals(codeString)) 7657 return ResourceType.COMPOSITION; 7658 if ("ConceptMap".equals(codeString)) 7659 return ResourceType.CONCEPTMAP; 7660 if ("Condition".equals(codeString)) 7661 return ResourceType.CONDITION; 7662 if ("Conformance".equals(codeString)) 7663 return ResourceType.CONFORMANCE; 7664 if ("Contract".equals(codeString)) 7665 return ResourceType.CONTRACT; 7666 if ("Coverage".equals(codeString)) 7667 return ResourceType.COVERAGE; 7668 if ("DataElement".equals(codeString)) 7669 return ResourceType.DATAELEMENT; 7670 if ("DetectedIssue".equals(codeString)) 7671 return ResourceType.DETECTEDISSUE; 7672 if ("Device".equals(codeString)) 7673 return ResourceType.DEVICE; 7674 if ("DeviceComponent".equals(codeString)) 7675 return ResourceType.DEVICECOMPONENT; 7676 if ("DeviceMetric".equals(codeString)) 7677 return ResourceType.DEVICEMETRIC; 7678 if ("DeviceUseRequest".equals(codeString)) 7679 return ResourceType.DEVICEUSEREQUEST; 7680 if ("DeviceUseStatement".equals(codeString)) 7681 return ResourceType.DEVICEUSESTATEMENT; 7682 if ("DiagnosticOrder".equals(codeString)) 7683 return ResourceType.DIAGNOSTICORDER; 7684 if ("DiagnosticReport".equals(codeString)) 7685 return ResourceType.DIAGNOSTICREPORT; 7686 if ("DocumentManifest".equals(codeString)) 7687 return ResourceType.DOCUMENTMANIFEST; 7688 if ("DocumentReference".equals(codeString)) 7689 return ResourceType.DOCUMENTREFERENCE; 7690 if ("DomainResource".equals(codeString)) 7691 return ResourceType.DOMAINRESOURCE; 7692 if ("EligibilityRequest".equals(codeString)) 7693 return ResourceType.ELIGIBILITYREQUEST; 7694 if ("EligibilityResponse".equals(codeString)) 7695 return ResourceType.ELIGIBILITYRESPONSE; 7696 if ("Encounter".equals(codeString)) 7697 return ResourceType.ENCOUNTER; 7698 if ("EnrollmentRequest".equals(codeString)) 7699 return ResourceType.ENROLLMENTREQUEST; 7700 if ("EnrollmentResponse".equals(codeString)) 7701 return ResourceType.ENROLLMENTRESPONSE; 7702 if ("EpisodeOfCare".equals(codeString)) 7703 return ResourceType.EPISODEOFCARE; 7704 if ("ExplanationOfBenefit".equals(codeString)) 7705 return ResourceType.EXPLANATIONOFBENEFIT; 7706 if ("FamilyMemberHistory".equals(codeString)) 7707 return ResourceType.FAMILYMEMBERHISTORY; 7708 if ("Flag".equals(codeString)) 7709 return ResourceType.FLAG; 7710 if ("Goal".equals(codeString)) 7711 return ResourceType.GOAL; 7712 if ("Group".equals(codeString)) 7713 return ResourceType.GROUP; 7714 if ("HealthcareService".equals(codeString)) 7715 return ResourceType.HEALTHCARESERVICE; 7716 if ("ImagingObjectSelection".equals(codeString)) 7717 return ResourceType.IMAGINGOBJECTSELECTION; 7718 if ("ImagingStudy".equals(codeString)) 7719 return ResourceType.IMAGINGSTUDY; 7720 if ("Immunization".equals(codeString)) 7721 return ResourceType.IMMUNIZATION; 7722 if ("ImmunizationRecommendation".equals(codeString)) 7723 return ResourceType.IMMUNIZATIONRECOMMENDATION; 7724 if ("ImplementationGuide".equals(codeString)) 7725 return ResourceType.IMPLEMENTATIONGUIDE; 7726 if ("List".equals(codeString)) 7727 return ResourceType.LIST; 7728 if ("Location".equals(codeString)) 7729 return ResourceType.LOCATION; 7730 if ("Media".equals(codeString)) 7731 return ResourceType.MEDIA; 7732 if ("Medication".equals(codeString)) 7733 return ResourceType.MEDICATION; 7734 if ("MedicationAdministration".equals(codeString)) 7735 return ResourceType.MEDICATIONADMINISTRATION; 7736 if ("MedicationDispense".equals(codeString)) 7737 return ResourceType.MEDICATIONDISPENSE; 7738 if ("MedicationOrder".equals(codeString)) 7739 return ResourceType.MEDICATIONORDER; 7740 if ("MedicationStatement".equals(codeString)) 7741 return ResourceType.MEDICATIONSTATEMENT; 7742 if ("MessageHeader".equals(codeString)) 7743 return ResourceType.MESSAGEHEADER; 7744 if ("NamingSystem".equals(codeString)) 7745 return ResourceType.NAMINGSYSTEM; 7746 if ("NutritionOrder".equals(codeString)) 7747 return ResourceType.NUTRITIONORDER; 7748 if ("Observation".equals(codeString)) 7749 return ResourceType.OBSERVATION; 7750 if ("OperationDefinition".equals(codeString)) 7751 return ResourceType.OPERATIONDEFINITION; 7752 if ("OperationOutcome".equals(codeString)) 7753 return ResourceType.OPERATIONOUTCOME; 7754 if ("Order".equals(codeString)) 7755 return ResourceType.ORDER; 7756 if ("OrderResponse".equals(codeString)) 7757 return ResourceType.ORDERRESPONSE; 7758 if ("Organization".equals(codeString)) 7759 return ResourceType.ORGANIZATION; 7760 if ("Parameters".equals(codeString)) 7761 return ResourceType.PARAMETERS; 7762 if ("Patient".equals(codeString)) 7763 return ResourceType.PATIENT; 7764 if ("PaymentNotice".equals(codeString)) 7765 return ResourceType.PAYMENTNOTICE; 7766 if ("PaymentReconciliation".equals(codeString)) 7767 return ResourceType.PAYMENTRECONCILIATION; 7768 if ("Person".equals(codeString)) 7769 return ResourceType.PERSON; 7770 if ("Practitioner".equals(codeString)) 7771 return ResourceType.PRACTITIONER; 7772 if ("Procedure".equals(codeString)) 7773 return ResourceType.PROCEDURE; 7774 if ("ProcedureRequest".equals(codeString)) 7775 return ResourceType.PROCEDUREREQUEST; 7776 if ("ProcessRequest".equals(codeString)) 7777 return ResourceType.PROCESSREQUEST; 7778 if ("ProcessResponse".equals(codeString)) 7779 return ResourceType.PROCESSRESPONSE; 7780 if ("Provenance".equals(codeString)) 7781 return ResourceType.PROVENANCE; 7782 if ("Questionnaire".equals(codeString)) 7783 return ResourceType.QUESTIONNAIRE; 7784 if ("QuestionnaireResponse".equals(codeString)) 7785 return ResourceType.QUESTIONNAIRERESPONSE; 7786 if ("ReferralRequest".equals(codeString)) 7787 return ResourceType.REFERRALREQUEST; 7788 if ("RelatedPerson".equals(codeString)) 7789 return ResourceType.RELATEDPERSON; 7790 if ("Resource".equals(codeString)) 7791 return ResourceType.RESOURCE; 7792 if ("RiskAssessment".equals(codeString)) 7793 return ResourceType.RISKASSESSMENT; 7794 if ("Schedule".equals(codeString)) 7795 return ResourceType.SCHEDULE; 7796 if ("SearchParameter".equals(codeString)) 7797 return ResourceType.SEARCHPARAMETER; 7798 if ("Slot".equals(codeString)) 7799 return ResourceType.SLOT; 7800 if ("Specimen".equals(codeString)) 7801 return ResourceType.SPECIMEN; 7802 if ("StructureDefinition".equals(codeString)) 7803 return ResourceType.STRUCTUREDEFINITION; 7804 if ("Subscription".equals(codeString)) 7805 return ResourceType.SUBSCRIPTION; 7806 if ("Substance".equals(codeString)) 7807 return ResourceType.SUBSTANCE; 7808 if ("SupplyDelivery".equals(codeString)) 7809 return ResourceType.SUPPLYDELIVERY; 7810 if ("SupplyRequest".equals(codeString)) 7811 return ResourceType.SUPPLYREQUEST; 7812 if ("TestScript".equals(codeString)) 7813 return ResourceType.TESTSCRIPT; 7814 if ("ValueSet".equals(codeString)) 7815 return ResourceType.VALUESET; 7816 if ("VisionPrescription".equals(codeString)) 7817 return ResourceType.VISIONPRESCRIPTION; 7818 throw new IllegalArgumentException("Unknown ResourceType code '" + codeString + "'"); 7819 } 7820 7821 public Enumeration<ResourceType> fromType(Base code) throws FHIRException { 7822 if (code == null || code.isEmpty()) 7823 return null; 7824 String codeString = ((PrimitiveType) code).asStringValue(); 7825 if (codeString == null || "".equals(codeString)) 7826 return null; 7827 if ("Account".equals(codeString)) 7828 return new Enumeration<ResourceType>(this, ResourceType.ACCOUNT); 7829 if ("AllergyIntolerance".equals(codeString)) 7830 return new Enumeration<ResourceType>(this, ResourceType.ALLERGYINTOLERANCE); 7831 if ("Appointment".equals(codeString)) 7832 return new Enumeration<ResourceType>(this, ResourceType.APPOINTMENT); 7833 if ("AppointmentResponse".equals(codeString)) 7834 return new Enumeration<ResourceType>(this, ResourceType.APPOINTMENTRESPONSE); 7835 if ("AuditEvent".equals(codeString)) 7836 return new Enumeration<ResourceType>(this, ResourceType.AUDITEVENT); 7837 if ("Basic".equals(codeString)) 7838 return new Enumeration<ResourceType>(this, ResourceType.BASIC); 7839 if ("Binary".equals(codeString)) 7840 return new Enumeration<ResourceType>(this, ResourceType.BINARY); 7841 if ("BodySite".equals(codeString)) 7842 return new Enumeration<ResourceType>(this, ResourceType.BODYSITE); 7843 if ("Bundle".equals(codeString)) 7844 return new Enumeration<ResourceType>(this, ResourceType.BUNDLE); 7845 if ("CarePlan".equals(codeString)) 7846 return new Enumeration<ResourceType>(this, ResourceType.CAREPLAN); 7847 if ("Claim".equals(codeString)) 7848 return new Enumeration<ResourceType>(this, ResourceType.CLAIM); 7849 if ("ClaimResponse".equals(codeString)) 7850 return new Enumeration<ResourceType>(this, ResourceType.CLAIMRESPONSE); 7851 if ("ClinicalImpression".equals(codeString)) 7852 return new Enumeration<ResourceType>(this, ResourceType.CLINICALIMPRESSION); 7853 if ("Communication".equals(codeString)) 7854 return new Enumeration<ResourceType>(this, ResourceType.COMMUNICATION); 7855 if ("CommunicationRequest".equals(codeString)) 7856 return new Enumeration<ResourceType>(this, ResourceType.COMMUNICATIONREQUEST); 7857 if ("Composition".equals(codeString)) 7858 return new Enumeration<ResourceType>(this, ResourceType.COMPOSITION); 7859 if ("ConceptMap".equals(codeString)) 7860 return new Enumeration<ResourceType>(this, ResourceType.CONCEPTMAP); 7861 if ("Condition".equals(codeString)) 7862 return new Enumeration<ResourceType>(this, ResourceType.CONDITION); 7863 if ("Conformance".equals(codeString)) 7864 return new Enumeration<ResourceType>(this, ResourceType.CONFORMANCE); 7865 if ("Contract".equals(codeString)) 7866 return new Enumeration<ResourceType>(this, ResourceType.CONTRACT); 7867 if ("Coverage".equals(codeString)) 7868 return new Enumeration<ResourceType>(this, ResourceType.COVERAGE); 7869 if ("DataElement".equals(codeString)) 7870 return new Enumeration<ResourceType>(this, ResourceType.DATAELEMENT); 7871 if ("DetectedIssue".equals(codeString)) 7872 return new Enumeration<ResourceType>(this, ResourceType.DETECTEDISSUE); 7873 if ("Device".equals(codeString)) 7874 return new Enumeration<ResourceType>(this, ResourceType.DEVICE); 7875 if ("DeviceComponent".equals(codeString)) 7876 return new Enumeration<ResourceType>(this, ResourceType.DEVICECOMPONENT); 7877 if ("DeviceMetric".equals(codeString)) 7878 return new Enumeration<ResourceType>(this, ResourceType.DEVICEMETRIC); 7879 if ("DeviceUseRequest".equals(codeString)) 7880 return new Enumeration<ResourceType>(this, ResourceType.DEVICEUSEREQUEST); 7881 if ("DeviceUseStatement".equals(codeString)) 7882 return new Enumeration<ResourceType>(this, ResourceType.DEVICEUSESTATEMENT); 7883 if ("DiagnosticOrder".equals(codeString)) 7884 return new Enumeration<ResourceType>(this, ResourceType.DIAGNOSTICORDER); 7885 if ("DiagnosticReport".equals(codeString)) 7886 return new Enumeration<ResourceType>(this, ResourceType.DIAGNOSTICREPORT); 7887 if ("DocumentManifest".equals(codeString)) 7888 return new Enumeration<ResourceType>(this, ResourceType.DOCUMENTMANIFEST); 7889 if ("DocumentReference".equals(codeString)) 7890 return new Enumeration<ResourceType>(this, ResourceType.DOCUMENTREFERENCE); 7891 if ("DomainResource".equals(codeString)) 7892 return new Enumeration<ResourceType>(this, ResourceType.DOMAINRESOURCE); 7893 if ("EligibilityRequest".equals(codeString)) 7894 return new Enumeration<ResourceType>(this, ResourceType.ELIGIBILITYREQUEST); 7895 if ("EligibilityResponse".equals(codeString)) 7896 return new Enumeration<ResourceType>(this, ResourceType.ELIGIBILITYRESPONSE); 7897 if ("Encounter".equals(codeString)) 7898 return new Enumeration<ResourceType>(this, ResourceType.ENCOUNTER); 7899 if ("EnrollmentRequest".equals(codeString)) 7900 return new Enumeration<ResourceType>(this, ResourceType.ENROLLMENTREQUEST); 7901 if ("EnrollmentResponse".equals(codeString)) 7902 return new Enumeration<ResourceType>(this, ResourceType.ENROLLMENTRESPONSE); 7903 if ("EpisodeOfCare".equals(codeString)) 7904 return new Enumeration<ResourceType>(this, ResourceType.EPISODEOFCARE); 7905 if ("ExplanationOfBenefit".equals(codeString)) 7906 return new Enumeration<ResourceType>(this, ResourceType.EXPLANATIONOFBENEFIT); 7907 if ("FamilyMemberHistory".equals(codeString)) 7908 return new Enumeration<ResourceType>(this, ResourceType.FAMILYMEMBERHISTORY); 7909 if ("Flag".equals(codeString)) 7910 return new Enumeration<ResourceType>(this, ResourceType.FLAG); 7911 if ("Goal".equals(codeString)) 7912 return new Enumeration<ResourceType>(this, ResourceType.GOAL); 7913 if ("Group".equals(codeString)) 7914 return new Enumeration<ResourceType>(this, ResourceType.GROUP); 7915 if ("HealthcareService".equals(codeString)) 7916 return new Enumeration<ResourceType>(this, ResourceType.HEALTHCARESERVICE); 7917 if ("ImagingObjectSelection".equals(codeString)) 7918 return new Enumeration<ResourceType>(this, ResourceType.IMAGINGOBJECTSELECTION); 7919 if ("ImagingStudy".equals(codeString)) 7920 return new Enumeration<ResourceType>(this, ResourceType.IMAGINGSTUDY); 7921 if ("Immunization".equals(codeString)) 7922 return new Enumeration<ResourceType>(this, ResourceType.IMMUNIZATION); 7923 if ("ImmunizationRecommendation".equals(codeString)) 7924 return new Enumeration<ResourceType>(this, ResourceType.IMMUNIZATIONRECOMMENDATION); 7925 if ("ImplementationGuide".equals(codeString)) 7926 return new Enumeration<ResourceType>(this, ResourceType.IMPLEMENTATIONGUIDE); 7927 if ("List".equals(codeString)) 7928 return new Enumeration<ResourceType>(this, ResourceType.LIST); 7929 if ("Location".equals(codeString)) 7930 return new Enumeration<ResourceType>(this, ResourceType.LOCATION); 7931 if ("Media".equals(codeString)) 7932 return new Enumeration<ResourceType>(this, ResourceType.MEDIA); 7933 if ("Medication".equals(codeString)) 7934 return new Enumeration<ResourceType>(this, ResourceType.MEDICATION); 7935 if ("MedicationAdministration".equals(codeString)) 7936 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONADMINISTRATION); 7937 if ("MedicationDispense".equals(codeString)) 7938 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONDISPENSE); 7939 if ("MedicationOrder".equals(codeString)) 7940 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONORDER); 7941 if ("MedicationStatement".equals(codeString)) 7942 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONSTATEMENT); 7943 if ("MessageHeader".equals(codeString)) 7944 return new Enumeration<ResourceType>(this, ResourceType.MESSAGEHEADER); 7945 if ("NamingSystem".equals(codeString)) 7946 return new Enumeration<ResourceType>(this, ResourceType.NAMINGSYSTEM); 7947 if ("NutritionOrder".equals(codeString)) 7948 return new Enumeration<ResourceType>(this, ResourceType.NUTRITIONORDER); 7949 if ("Observation".equals(codeString)) 7950 return new Enumeration<ResourceType>(this, ResourceType.OBSERVATION); 7951 if ("OperationDefinition".equals(codeString)) 7952 return new Enumeration<ResourceType>(this, ResourceType.OPERATIONDEFINITION); 7953 if ("OperationOutcome".equals(codeString)) 7954 return new Enumeration<ResourceType>(this, ResourceType.OPERATIONOUTCOME); 7955 if ("Order".equals(codeString)) 7956 return new Enumeration<ResourceType>(this, ResourceType.ORDER); 7957 if ("OrderResponse".equals(codeString)) 7958 return new Enumeration<ResourceType>(this, ResourceType.ORDERRESPONSE); 7959 if ("Organization".equals(codeString)) 7960 return new Enumeration<ResourceType>(this, ResourceType.ORGANIZATION); 7961 if ("Parameters".equals(codeString)) 7962 return new Enumeration<ResourceType>(this, ResourceType.PARAMETERS); 7963 if ("Patient".equals(codeString)) 7964 return new Enumeration<ResourceType>(this, ResourceType.PATIENT); 7965 if ("PaymentNotice".equals(codeString)) 7966 return new Enumeration<ResourceType>(this, ResourceType.PAYMENTNOTICE); 7967 if ("PaymentReconciliation".equals(codeString)) 7968 return new Enumeration<ResourceType>(this, ResourceType.PAYMENTRECONCILIATION); 7969 if ("Person".equals(codeString)) 7970 return new Enumeration<ResourceType>(this, ResourceType.PERSON); 7971 if ("Practitioner".equals(codeString)) 7972 return new Enumeration<ResourceType>(this, ResourceType.PRACTITIONER); 7973 if ("Procedure".equals(codeString)) 7974 return new Enumeration<ResourceType>(this, ResourceType.PROCEDURE); 7975 if ("ProcedureRequest".equals(codeString)) 7976 return new Enumeration<ResourceType>(this, ResourceType.PROCEDUREREQUEST); 7977 if ("ProcessRequest".equals(codeString)) 7978 return new Enumeration<ResourceType>(this, ResourceType.PROCESSREQUEST); 7979 if ("ProcessResponse".equals(codeString)) 7980 return new Enumeration<ResourceType>(this, ResourceType.PROCESSRESPONSE); 7981 if ("Provenance".equals(codeString)) 7982 return new Enumeration<ResourceType>(this, ResourceType.PROVENANCE); 7983 if ("Questionnaire".equals(codeString)) 7984 return new Enumeration<ResourceType>(this, ResourceType.QUESTIONNAIRE); 7985 if ("QuestionnaireResponse".equals(codeString)) 7986 return new Enumeration<ResourceType>(this, ResourceType.QUESTIONNAIRERESPONSE); 7987 if ("ReferralRequest".equals(codeString)) 7988 return new Enumeration<ResourceType>(this, ResourceType.REFERRALREQUEST); 7989 if ("RelatedPerson".equals(codeString)) 7990 return new Enumeration<ResourceType>(this, ResourceType.RELATEDPERSON); 7991 if ("Resource".equals(codeString)) 7992 return new Enumeration<ResourceType>(this, ResourceType.RESOURCE); 7993 if ("RiskAssessment".equals(codeString)) 7994 return new Enumeration<ResourceType>(this, ResourceType.RISKASSESSMENT); 7995 if ("Schedule".equals(codeString)) 7996 return new Enumeration<ResourceType>(this, ResourceType.SCHEDULE); 7997 if ("SearchParameter".equals(codeString)) 7998 return new Enumeration<ResourceType>(this, ResourceType.SEARCHPARAMETER); 7999 if ("Slot".equals(codeString)) 8000 return new Enumeration<ResourceType>(this, ResourceType.SLOT); 8001 if ("Specimen".equals(codeString)) 8002 return new Enumeration<ResourceType>(this, ResourceType.SPECIMEN); 8003 if ("StructureDefinition".equals(codeString)) 8004 return new Enumeration<ResourceType>(this, ResourceType.STRUCTUREDEFINITION); 8005 if ("Subscription".equals(codeString)) 8006 return new Enumeration<ResourceType>(this, ResourceType.SUBSCRIPTION); 8007 if ("Substance".equals(codeString)) 8008 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCE); 8009 if ("SupplyDelivery".equals(codeString)) 8010 return new Enumeration<ResourceType>(this, ResourceType.SUPPLYDELIVERY); 8011 if ("SupplyRequest".equals(codeString)) 8012 return new Enumeration<ResourceType>(this, ResourceType.SUPPLYREQUEST); 8013 if ("TestScript".equals(codeString)) 8014 return new Enumeration<ResourceType>(this, ResourceType.TESTSCRIPT); 8015 if ("ValueSet".equals(codeString)) 8016 return new Enumeration<ResourceType>(this, ResourceType.VALUESET); 8017 if ("VisionPrescription".equals(codeString)) 8018 return new Enumeration<ResourceType>(this, ResourceType.VISIONPRESCRIPTION); 8019 throw new FHIRException("Unknown ResourceType code '" + codeString + "'"); 8020 } 8021 8022 public String toCode(ResourceType code) 8023 { 8024 if (code == ResourceType.NULL) 8025 return null; 8026 if (code == ResourceType.ACCOUNT) 8027 return "Account"; 8028 if (code == ResourceType.ALLERGYINTOLERANCE) 8029 return "AllergyIntolerance"; 8030 if (code == ResourceType.APPOINTMENT) 8031 return "Appointment"; 8032 if (code == ResourceType.APPOINTMENTRESPONSE) 8033 return "AppointmentResponse"; 8034 if (code == ResourceType.AUDITEVENT) 8035 return "AuditEvent"; 8036 if (code == ResourceType.BASIC) 8037 return "Basic"; 8038 if (code == ResourceType.BINARY) 8039 return "Binary"; 8040 if (code == ResourceType.BODYSITE) 8041 return "BodySite"; 8042 if (code == ResourceType.BUNDLE) 8043 return "Bundle"; 8044 if (code == ResourceType.CAREPLAN) 8045 return "CarePlan"; 8046 if (code == ResourceType.CLAIM) 8047 return "Claim"; 8048 if (code == ResourceType.CLAIMRESPONSE) 8049 return "ClaimResponse"; 8050 if (code == ResourceType.CLINICALIMPRESSION) 8051 return "ClinicalImpression"; 8052 if (code == ResourceType.COMMUNICATION) 8053 return "Communication"; 8054 if (code == ResourceType.COMMUNICATIONREQUEST) 8055 return "CommunicationRequest"; 8056 if (code == ResourceType.COMPOSITION) 8057 return "Composition"; 8058 if (code == ResourceType.CONCEPTMAP) 8059 return "ConceptMap"; 8060 if (code == ResourceType.CONDITION) 8061 return "Condition"; 8062 if (code == ResourceType.CONFORMANCE) 8063 return "Conformance"; 8064 if (code == ResourceType.CONTRACT) 8065 return "Contract"; 8066 if (code == ResourceType.COVERAGE) 8067 return "Coverage"; 8068 if (code == ResourceType.DATAELEMENT) 8069 return "DataElement"; 8070 if (code == ResourceType.DETECTEDISSUE) 8071 return "DetectedIssue"; 8072 if (code == ResourceType.DEVICE) 8073 return "Device"; 8074 if (code == ResourceType.DEVICECOMPONENT) 8075 return "DeviceComponent"; 8076 if (code == ResourceType.DEVICEMETRIC) 8077 return "DeviceMetric"; 8078 if (code == ResourceType.DEVICEUSEREQUEST) 8079 return "DeviceUseRequest"; 8080 if (code == ResourceType.DEVICEUSESTATEMENT) 8081 return "DeviceUseStatement"; 8082 if (code == ResourceType.DIAGNOSTICORDER) 8083 return "DiagnosticOrder"; 8084 if (code == ResourceType.DIAGNOSTICREPORT) 8085 return "DiagnosticReport"; 8086 if (code == ResourceType.DOCUMENTMANIFEST) 8087 return "DocumentManifest"; 8088 if (code == ResourceType.DOCUMENTREFERENCE) 8089 return "DocumentReference"; 8090 if (code == ResourceType.DOMAINRESOURCE) 8091 return "DomainResource"; 8092 if (code == ResourceType.ELIGIBILITYREQUEST) 8093 return "EligibilityRequest"; 8094 if (code == ResourceType.ELIGIBILITYRESPONSE) 8095 return "EligibilityResponse"; 8096 if (code == ResourceType.ENCOUNTER) 8097 return "Encounter"; 8098 if (code == ResourceType.ENROLLMENTREQUEST) 8099 return "EnrollmentRequest"; 8100 if (code == ResourceType.ENROLLMENTRESPONSE) 8101 return "EnrollmentResponse"; 8102 if (code == ResourceType.EPISODEOFCARE) 8103 return "EpisodeOfCare"; 8104 if (code == ResourceType.EXPLANATIONOFBENEFIT) 8105 return "ExplanationOfBenefit"; 8106 if (code == ResourceType.FAMILYMEMBERHISTORY) 8107 return "FamilyMemberHistory"; 8108 if (code == ResourceType.FLAG) 8109 return "Flag"; 8110 if (code == ResourceType.GOAL) 8111 return "Goal"; 8112 if (code == ResourceType.GROUP) 8113 return "Group"; 8114 if (code == ResourceType.HEALTHCARESERVICE) 8115 return "HealthcareService"; 8116 if (code == ResourceType.IMAGINGOBJECTSELECTION) 8117 return "ImagingObjectSelection"; 8118 if (code == ResourceType.IMAGINGSTUDY) 8119 return "ImagingStudy"; 8120 if (code == ResourceType.IMMUNIZATION) 8121 return "Immunization"; 8122 if (code == ResourceType.IMMUNIZATIONRECOMMENDATION) 8123 return "ImmunizationRecommendation"; 8124 if (code == ResourceType.IMPLEMENTATIONGUIDE) 8125 return "ImplementationGuide"; 8126 if (code == ResourceType.LIST) 8127 return "List"; 8128 if (code == ResourceType.LOCATION) 8129 return "Location"; 8130 if (code == ResourceType.MEDIA) 8131 return "Media"; 8132 if (code == ResourceType.MEDICATION) 8133 return "Medication"; 8134 if (code == ResourceType.MEDICATIONADMINISTRATION) 8135 return "MedicationAdministration"; 8136 if (code == ResourceType.MEDICATIONDISPENSE) 8137 return "MedicationDispense"; 8138 if (code == ResourceType.MEDICATIONORDER) 8139 return "MedicationOrder"; 8140 if (code == ResourceType.MEDICATIONSTATEMENT) 8141 return "MedicationStatement"; 8142 if (code == ResourceType.MESSAGEHEADER) 8143 return "MessageHeader"; 8144 if (code == ResourceType.NAMINGSYSTEM) 8145 return "NamingSystem"; 8146 if (code == ResourceType.NUTRITIONORDER) 8147 return "NutritionOrder"; 8148 if (code == ResourceType.OBSERVATION) 8149 return "Observation"; 8150 if (code == ResourceType.OPERATIONDEFINITION) 8151 return "OperationDefinition"; 8152 if (code == ResourceType.OPERATIONOUTCOME) 8153 return "OperationOutcome"; 8154 if (code == ResourceType.ORDER) 8155 return "Order"; 8156 if (code == ResourceType.ORDERRESPONSE) 8157 return "OrderResponse"; 8158 if (code == ResourceType.ORGANIZATION) 8159 return "Organization"; 8160 if (code == ResourceType.PARAMETERS) 8161 return "Parameters"; 8162 if (code == ResourceType.PATIENT) 8163 return "Patient"; 8164 if (code == ResourceType.PAYMENTNOTICE) 8165 return "PaymentNotice"; 8166 if (code == ResourceType.PAYMENTRECONCILIATION) 8167 return "PaymentReconciliation"; 8168 if (code == ResourceType.PERSON) 8169 return "Person"; 8170 if (code == ResourceType.PRACTITIONER) 8171 return "Practitioner"; 8172 if (code == ResourceType.PROCEDURE) 8173 return "Procedure"; 8174 if (code == ResourceType.PROCEDUREREQUEST) 8175 return "ProcedureRequest"; 8176 if (code == ResourceType.PROCESSREQUEST) 8177 return "ProcessRequest"; 8178 if (code == ResourceType.PROCESSRESPONSE) 8179 return "ProcessResponse"; 8180 if (code == ResourceType.PROVENANCE) 8181 return "Provenance"; 8182 if (code == ResourceType.QUESTIONNAIRE) 8183 return "Questionnaire"; 8184 if (code == ResourceType.QUESTIONNAIRERESPONSE) 8185 return "QuestionnaireResponse"; 8186 if (code == ResourceType.REFERRALREQUEST) 8187 return "ReferralRequest"; 8188 if (code == ResourceType.RELATEDPERSON) 8189 return "RelatedPerson"; 8190 if (code == ResourceType.RESOURCE) 8191 return "Resource"; 8192 if (code == ResourceType.RISKASSESSMENT) 8193 return "RiskAssessment"; 8194 if (code == ResourceType.SCHEDULE) 8195 return "Schedule"; 8196 if (code == ResourceType.SEARCHPARAMETER) 8197 return "SearchParameter"; 8198 if (code == ResourceType.SLOT) 8199 return "Slot"; 8200 if (code == ResourceType.SPECIMEN) 8201 return "Specimen"; 8202 if (code == ResourceType.STRUCTUREDEFINITION) 8203 return "StructureDefinition"; 8204 if (code == ResourceType.SUBSCRIPTION) 8205 return "Subscription"; 8206 if (code == ResourceType.SUBSTANCE) 8207 return "Substance"; 8208 if (code == ResourceType.SUPPLYDELIVERY) 8209 return "SupplyDelivery"; 8210 if (code == ResourceType.SUPPLYREQUEST) 8211 return "SupplyRequest"; 8212 if (code == ResourceType.TESTSCRIPT) 8213 return "TestScript"; 8214 if (code == ResourceType.VALUESET) 8215 return "ValueSet"; 8216 if (code == ResourceType.VISIONPRESCRIPTION) 8217 return "VisionPrescription"; 8218 return "?"; 8219 } 8220 } 8221 8222 public enum SearchParamType { 8223 /** 8224 * Search parameter SHALL be a number (a whole number, or a decimal). 8225 */ 8226 NUMBER, 8227 /** 8228 * Search parameter is on a date/time. The date format is the standard XML 8229 * format, though other formats may be supported. 8230 */ 8231 DATE, 8232 /** 8233 * Search parameter is a simple string, like a name part. Search is 8234 * case-insensitive and accent-insensitive. May match just the start of a 8235 * string. String parameters may contain spaces. 8236 */ 8237 STRING, 8238 /** 8239 * Search parameter on a coded element or identifier. May be used to search 8240 * through the text, displayname, code and code/codesystem (for codes) and 8241 * label, system and key (for identifier). Its value is either a string or a 8242 * pair of namespace and value, separated by a "|", depending on the modifier 8243 * used. 8244 */ 8245 TOKEN, 8246 /** 8247 * A reference to another resource. 8248 */ 8249 REFERENCE, 8250 /** 8251 * A composite search parameter that combines a search on two values together. 8252 */ 8253 COMPOSITE, 8254 /** 8255 * A search parameter that searches on a quantity. 8256 */ 8257 QUANTITY, 8258 /** 8259 * A search parameter that searches on a URI (RFC 3986). 8260 */ 8261 URI, 8262 /** 8263 * added to help the parsers 8264 */ 8265 NULL; 8266 8267 public static SearchParamType fromCode(String codeString) throws FHIRException { 8268 if (codeString == null || "".equals(codeString)) 8269 return null; 8270 if ("number".equals(codeString)) 8271 return NUMBER; 8272 if ("date".equals(codeString)) 8273 return DATE; 8274 if ("string".equals(codeString)) 8275 return STRING; 8276 if ("token".equals(codeString)) 8277 return TOKEN; 8278 if ("reference".equals(codeString)) 8279 return REFERENCE; 8280 if ("composite".equals(codeString)) 8281 return COMPOSITE; 8282 if ("quantity".equals(codeString)) 8283 return QUANTITY; 8284 if ("uri".equals(codeString)) 8285 return URI; 8286 throw new FHIRException("Unknown SearchParamType code '" + codeString + "'"); 8287 } 8288 8289 public String toCode() { 8290 switch (this) { 8291 case NUMBER: 8292 return "number"; 8293 case DATE: 8294 return "date"; 8295 case STRING: 8296 return "string"; 8297 case TOKEN: 8298 return "token"; 8299 case REFERENCE: 8300 return "reference"; 8301 case COMPOSITE: 8302 return "composite"; 8303 case QUANTITY: 8304 return "quantity"; 8305 case URI: 8306 return "uri"; 8307 case NULL: 8308 return null; 8309 default: 8310 return "?"; 8311 } 8312 } 8313 8314 public String getSystem() { 8315 switch (this) { 8316 case NUMBER: 8317 return "http://hl7.org/fhir/search-param-type"; 8318 case DATE: 8319 return "http://hl7.org/fhir/search-param-type"; 8320 case STRING: 8321 return "http://hl7.org/fhir/search-param-type"; 8322 case TOKEN: 8323 return "http://hl7.org/fhir/search-param-type"; 8324 case REFERENCE: 8325 return "http://hl7.org/fhir/search-param-type"; 8326 case COMPOSITE: 8327 return "http://hl7.org/fhir/search-param-type"; 8328 case QUANTITY: 8329 return "http://hl7.org/fhir/search-param-type"; 8330 case URI: 8331 return "http://hl7.org/fhir/search-param-type"; 8332 case NULL: 8333 return null; 8334 default: 8335 return "?"; 8336 } 8337 } 8338 8339 public String getDefinition() { 8340 switch (this) { 8341 case NUMBER: 8342 return "Search parameter SHALL be a number (a whole number, or a decimal)."; 8343 case DATE: 8344 return "Search parameter is on a date/time. The date format is the standard XML format, though other formats may be supported."; 8345 case STRING: 8346 return "Search parameter is a simple string, like a name part. Search is case-insensitive and accent-insensitive. May match just the start of a string. String parameters may contain spaces."; 8347 case TOKEN: 8348 return "Search parameter on a coded element or identifier. May be used to search through the text, displayname, code and code/codesystem (for codes) and label, system and key (for identifier). Its value is either a string or a pair of namespace and value, separated by a \"|\", depending on the modifier used."; 8349 case REFERENCE: 8350 return "A reference to another resource."; 8351 case COMPOSITE: 8352 return "A composite search parameter that combines a search on two values together."; 8353 case QUANTITY: 8354 return "A search parameter that searches on a quantity."; 8355 case URI: 8356 return "A search parameter that searches on a URI (RFC 3986)."; 8357 case NULL: 8358 return null; 8359 default: 8360 return "?"; 8361 } 8362 } 8363 8364 public String getDisplay() { 8365 switch (this) { 8366 case NUMBER: 8367 return "Number"; 8368 case DATE: 8369 return "Date/DateTime"; 8370 case STRING: 8371 return "String"; 8372 case TOKEN: 8373 return "Token"; 8374 case REFERENCE: 8375 return "Reference"; 8376 case COMPOSITE: 8377 return "Composite"; 8378 case QUANTITY: 8379 return "Quantity"; 8380 case URI: 8381 return "URI"; 8382 case NULL: 8383 return null; 8384 default: 8385 return "?"; 8386 } 8387 } 8388 } 8389 8390 public static class SearchParamTypeEnumFactory implements EnumFactory<SearchParamType> { 8391 public SearchParamType fromCode(String codeString) throws IllegalArgumentException { 8392 if (codeString == null || "".equals(codeString)) 8393 if (codeString == null || "".equals(codeString)) 8394 return null; 8395 if ("number".equals(codeString)) 8396 return SearchParamType.NUMBER; 8397 if ("date".equals(codeString)) 8398 return SearchParamType.DATE; 8399 if ("string".equals(codeString)) 8400 return SearchParamType.STRING; 8401 if ("token".equals(codeString)) 8402 return SearchParamType.TOKEN; 8403 if ("reference".equals(codeString)) 8404 return SearchParamType.REFERENCE; 8405 if ("composite".equals(codeString)) 8406 return SearchParamType.COMPOSITE; 8407 if ("quantity".equals(codeString)) 8408 return SearchParamType.QUANTITY; 8409 if ("uri".equals(codeString)) 8410 return SearchParamType.URI; 8411 throw new IllegalArgumentException("Unknown SearchParamType code '" + codeString + "'"); 8412 } 8413 8414 public Enumeration<SearchParamType> fromType(Base code) throws FHIRException { 8415 if (code == null || code.isEmpty()) 8416 return null; 8417 String codeString = ((PrimitiveType) code).asStringValue(); 8418 if (codeString == null || "".equals(codeString)) 8419 return null; 8420 if ("number".equals(codeString)) 8421 return new Enumeration<SearchParamType>(this, SearchParamType.NUMBER); 8422 if ("date".equals(codeString)) 8423 return new Enumeration<SearchParamType>(this, SearchParamType.DATE); 8424 if ("string".equals(codeString)) 8425 return new Enumeration<SearchParamType>(this, SearchParamType.STRING); 8426 if ("token".equals(codeString)) 8427 return new Enumeration<SearchParamType>(this, SearchParamType.TOKEN); 8428 if ("reference".equals(codeString)) 8429 return new Enumeration<SearchParamType>(this, SearchParamType.REFERENCE); 8430 if ("composite".equals(codeString)) 8431 return new Enumeration<SearchParamType>(this, SearchParamType.COMPOSITE); 8432 if ("quantity".equals(codeString)) 8433 return new Enumeration<SearchParamType>(this, SearchParamType.QUANTITY); 8434 if ("uri".equals(codeString)) 8435 return new Enumeration<SearchParamType>(this, SearchParamType.URI); 8436 throw new FHIRException("Unknown SearchParamType code '" + codeString + "'"); 8437 } 8438 8439 public String toCode(SearchParamType code) 8440 { 8441 if (code == SearchParamType.NULL) 8442 return null; 8443 if (code == SearchParamType.NUMBER) 8444 return "number"; 8445 if (code == SearchParamType.DATE) 8446 return "date"; 8447 if (code == SearchParamType.STRING) 8448 return "string"; 8449 if (code == SearchParamType.TOKEN) 8450 return "token"; 8451 if (code == SearchParamType.REFERENCE) 8452 return "reference"; 8453 if (code == SearchParamType.COMPOSITE) 8454 return "composite"; 8455 if (code == SearchParamType.QUANTITY) 8456 return "quantity"; 8457 if (code == SearchParamType.URI) 8458 return "uri"; 8459 return "?"; 8460 } 8461 } 8462 8463 public enum SpecialValues { 8464 /** 8465 * Boolean true. 8466 */ 8467 TRUE, 8468 /** 8469 * Boolean false. 8470 */ 8471 FALSE, 8472 /** 8473 * The content is greater than zero, but too small to be quantified. 8474 */ 8475 TRACE, 8476 /** 8477 * The specific quantity is not known, but is known to be non-zero and is not 8478 * specified because it makes up the bulk of the material. 8479 */ 8480 SUFFICIENT, 8481 /** 8482 * The value is no longer available. 8483 */ 8484 WITHDRAWN, 8485 /** 8486 * The are no known applicable values in this context. 8487 */ 8488 NILKNOWN, 8489 /** 8490 * added to help the parsers 8491 */ 8492 NULL; 8493 8494 public static SpecialValues fromCode(String codeString) throws FHIRException { 8495 if (codeString == null || "".equals(codeString)) 8496 return null; 8497 if ("true".equals(codeString)) 8498 return TRUE; 8499 if ("false".equals(codeString)) 8500 return FALSE; 8501 if ("trace".equals(codeString)) 8502 return TRACE; 8503 if ("sufficient".equals(codeString)) 8504 return SUFFICIENT; 8505 if ("withdrawn".equals(codeString)) 8506 return WITHDRAWN; 8507 if ("nil-known".equals(codeString)) 8508 return NILKNOWN; 8509 throw new FHIRException("Unknown SpecialValues code '" + codeString + "'"); 8510 } 8511 8512 public String toCode() { 8513 switch (this) { 8514 case TRUE: 8515 return "true"; 8516 case FALSE: 8517 return "false"; 8518 case TRACE: 8519 return "trace"; 8520 case SUFFICIENT: 8521 return "sufficient"; 8522 case WITHDRAWN: 8523 return "withdrawn"; 8524 case NILKNOWN: 8525 return "nil-known"; 8526 case NULL: 8527 return null; 8528 default: 8529 return "?"; 8530 } 8531 } 8532 8533 public String getSystem() { 8534 switch (this) { 8535 case TRUE: 8536 return "http://hl7.org/fhir/special-values"; 8537 case FALSE: 8538 return "http://hl7.org/fhir/special-values"; 8539 case TRACE: 8540 return "http://hl7.org/fhir/special-values"; 8541 case SUFFICIENT: 8542 return "http://hl7.org/fhir/special-values"; 8543 case WITHDRAWN: 8544 return "http://hl7.org/fhir/special-values"; 8545 case NILKNOWN: 8546 return "http://hl7.org/fhir/special-values"; 8547 case NULL: 8548 return null; 8549 default: 8550 return "?"; 8551 } 8552 } 8553 8554 public String getDefinition() { 8555 switch (this) { 8556 case TRUE: 8557 return "Boolean true."; 8558 case FALSE: 8559 return "Boolean false."; 8560 case TRACE: 8561 return "The content is greater than zero, but too small to be quantified."; 8562 case SUFFICIENT: 8563 return "The specific quantity is not known, but is known to be non-zero and is not specified because it makes up the bulk of the material."; 8564 case WITHDRAWN: 8565 return "The value is no longer available."; 8566 case NILKNOWN: 8567 return "The are no known applicable values in this context."; 8568 case NULL: 8569 return null; 8570 default: 8571 return "?"; 8572 } 8573 } 8574 8575 public String getDisplay() { 8576 switch (this) { 8577 case TRUE: 8578 return "true"; 8579 case FALSE: 8580 return "false"; 8581 case TRACE: 8582 return "Trace Amount Detected"; 8583 case SUFFICIENT: 8584 return "Sufficient Quantity"; 8585 case WITHDRAWN: 8586 return "Value Withdrawn"; 8587 case NILKNOWN: 8588 return "Nil Known"; 8589 case NULL: 8590 return null; 8591 default: 8592 return "?"; 8593 } 8594 } 8595 } 8596 8597 public static class SpecialValuesEnumFactory implements EnumFactory<SpecialValues> { 8598 public SpecialValues fromCode(String codeString) throws IllegalArgumentException { 8599 if (codeString == null || "".equals(codeString)) 8600 if (codeString == null || "".equals(codeString)) 8601 return null; 8602 if ("true".equals(codeString)) 8603 return SpecialValues.TRUE; 8604 if ("false".equals(codeString)) 8605 return SpecialValues.FALSE; 8606 if ("trace".equals(codeString)) 8607 return SpecialValues.TRACE; 8608 if ("sufficient".equals(codeString)) 8609 return SpecialValues.SUFFICIENT; 8610 if ("withdrawn".equals(codeString)) 8611 return SpecialValues.WITHDRAWN; 8612 if ("nil-known".equals(codeString)) 8613 return SpecialValues.NILKNOWN; 8614 throw new IllegalArgumentException("Unknown SpecialValues code '" + codeString + "'"); 8615 } 8616 8617 public Enumeration<SpecialValues> fromType(Base code) throws FHIRException { 8618 if (code == null || code.isEmpty()) 8619 return null; 8620 String codeString = ((PrimitiveType) code).asStringValue(); 8621 if (codeString == null || "".equals(codeString)) 8622 return null; 8623 if ("true".equals(codeString)) 8624 return new Enumeration<SpecialValues>(this, SpecialValues.TRUE); 8625 if ("false".equals(codeString)) 8626 return new Enumeration<SpecialValues>(this, SpecialValues.FALSE); 8627 if ("trace".equals(codeString)) 8628 return new Enumeration<SpecialValues>(this, SpecialValues.TRACE); 8629 if ("sufficient".equals(codeString)) 8630 return new Enumeration<SpecialValues>(this, SpecialValues.SUFFICIENT); 8631 if ("withdrawn".equals(codeString)) 8632 return new Enumeration<SpecialValues>(this, SpecialValues.WITHDRAWN); 8633 if ("nil-known".equals(codeString)) 8634 return new Enumeration<SpecialValues>(this, SpecialValues.NILKNOWN); 8635 throw new FHIRException("Unknown SpecialValues code '" + codeString + "'"); 8636 } 8637 8638 public String toCode(SpecialValues code) 8639 { 8640 if (code == SpecialValues.NULL) 8641 return null; 8642 if (code == SpecialValues.TRUE) 8643 return "true"; 8644 if (code == SpecialValues.FALSE) 8645 return "false"; 8646 if (code == SpecialValues.TRACE) 8647 return "trace"; 8648 if (code == SpecialValues.SUFFICIENT) 8649 return "sufficient"; 8650 if (code == SpecialValues.WITHDRAWN) 8651 return "withdrawn"; 8652 if (code == SpecialValues.NILKNOWN) 8653 return "nil-known"; 8654 return "?"; 8655 } 8656 } 8657 8658}