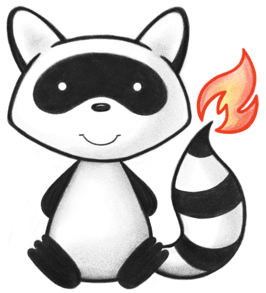
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Block; 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.exceptions.FHIRException; 043 044/** 045 * An association between a patient and an organization / healthcare provider(s) 046 * during which time encounters may occur. The managing organization assumes a 047 * level of responsibility for the patient during this time. 048 */ 049@ResourceDef(name = "EpisodeOfCare", profile = "http://hl7.org/fhir/Profile/EpisodeOfCare") 050public class EpisodeOfCare extends DomainResource { 051 052 public enum EpisodeOfCareStatus { 053 /** 054 * This episode of care is planned to start at the date specified in the 055 * period.start. During this status an organization may perform assessments to 056 * determine if they are eligible to receive services, or be organizing to make 057 * resources available to provide care services. 058 */ 059 PLANNED, 060 /** 061 * This episode has been placed on a waitlist, pending the episode being made 062 * active (or cancelled). 063 */ 064 WAITLIST, 065 /** 066 * This episode of care is current. 067 */ 068 ACTIVE, 069 /** 070 * This episode of care is on hold, the organization has limited responsibility 071 * for the patient (such as while on respite). 072 */ 073 ONHOLD, 074 /** 075 * This episode of care is finished at the organization is not expecting to be 076 * providing care to the patient. Can also be known as "closed", "completed" or 077 * other similar terms. 078 */ 079 FINISHED, 080 /** 081 * The episode of care was cancelled, or withdrawn from service, often selected 082 * during the planned stage as the patient may have gone elsewhere, or the 083 * circumstances have changed and the organization is unable to provide the 084 * care. It indicates that services terminated outside the planned/expected 085 * workflow. 086 */ 087 CANCELLED, 088 /** 089 * added to help the parsers 090 */ 091 NULL; 092 093 public static EpisodeOfCareStatus fromCode(String codeString) throws FHIRException { 094 if (codeString == null || "".equals(codeString)) 095 return null; 096 if ("planned".equals(codeString)) 097 return PLANNED; 098 if ("waitlist".equals(codeString)) 099 return WAITLIST; 100 if ("active".equals(codeString)) 101 return ACTIVE; 102 if ("onhold".equals(codeString)) 103 return ONHOLD; 104 if ("finished".equals(codeString)) 105 return FINISHED; 106 if ("cancelled".equals(codeString)) 107 return CANCELLED; 108 throw new FHIRException("Unknown EpisodeOfCareStatus code '" + codeString + "'"); 109 } 110 111 public String toCode() { 112 switch (this) { 113 case PLANNED: 114 return "planned"; 115 case WAITLIST: 116 return "waitlist"; 117 case ACTIVE: 118 return "active"; 119 case ONHOLD: 120 return "onhold"; 121 case FINISHED: 122 return "finished"; 123 case CANCELLED: 124 return "cancelled"; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 132 public String getSystem() { 133 switch (this) { 134 case PLANNED: 135 return "http://hl7.org/fhir/episode-of-care-status"; 136 case WAITLIST: 137 return "http://hl7.org/fhir/episode-of-care-status"; 138 case ACTIVE: 139 return "http://hl7.org/fhir/episode-of-care-status"; 140 case ONHOLD: 141 return "http://hl7.org/fhir/episode-of-care-status"; 142 case FINISHED: 143 return "http://hl7.org/fhir/episode-of-care-status"; 144 case CANCELLED: 145 return "http://hl7.org/fhir/episode-of-care-status"; 146 case NULL: 147 return null; 148 default: 149 return "?"; 150 } 151 } 152 153 public String getDefinition() { 154 switch (this) { 155 case PLANNED: 156 return "This episode of care is planned to start at the date specified in the period.start. During this status an organization may perform assessments to determine if they are eligible to receive services, or be organizing to make resources available to provide care services."; 157 case WAITLIST: 158 return "This episode has been placed on a waitlist, pending the episode being made active (or cancelled)."; 159 case ACTIVE: 160 return "This episode of care is current."; 161 case ONHOLD: 162 return "This episode of care is on hold, the organization has limited responsibility for the patient (such as while on respite)."; 163 case FINISHED: 164 return "This episode of care is finished at the organization is not expecting to be providing care to the patient. Can also be known as \"closed\", \"completed\" or other similar terms."; 165 case CANCELLED: 166 return "The episode of care was cancelled, or withdrawn from service, often selected during the planned stage as the patient may have gone elsewhere, or the circumstances have changed and the organization is unable to provide the care. It indicates that services terminated outside the planned/expected workflow."; 167 case NULL: 168 return null; 169 default: 170 return "?"; 171 } 172 } 173 174 public String getDisplay() { 175 switch (this) { 176 case PLANNED: 177 return "Planned"; 178 case WAITLIST: 179 return "Waitlist"; 180 case ACTIVE: 181 return "Active"; 182 case ONHOLD: 183 return "On Hold"; 184 case FINISHED: 185 return "Finished"; 186 case CANCELLED: 187 return "Cancelled"; 188 case NULL: 189 return null; 190 default: 191 return "?"; 192 } 193 } 194 } 195 196 public static class EpisodeOfCareStatusEnumFactory implements EnumFactory<EpisodeOfCareStatus> { 197 public EpisodeOfCareStatus fromCode(String codeString) throws IllegalArgumentException { 198 if (codeString == null || "".equals(codeString)) 199 if (codeString == null || "".equals(codeString)) 200 return null; 201 if ("planned".equals(codeString)) 202 return EpisodeOfCareStatus.PLANNED; 203 if ("waitlist".equals(codeString)) 204 return EpisodeOfCareStatus.WAITLIST; 205 if ("active".equals(codeString)) 206 return EpisodeOfCareStatus.ACTIVE; 207 if ("onhold".equals(codeString)) 208 return EpisodeOfCareStatus.ONHOLD; 209 if ("finished".equals(codeString)) 210 return EpisodeOfCareStatus.FINISHED; 211 if ("cancelled".equals(codeString)) 212 return EpisodeOfCareStatus.CANCELLED; 213 throw new IllegalArgumentException("Unknown EpisodeOfCareStatus code '" + codeString + "'"); 214 } 215 216 public Enumeration<EpisodeOfCareStatus> fromType(Base code) throws FHIRException { 217 if (code == null || code.isEmpty()) 218 return null; 219 String codeString = ((PrimitiveType) code).asStringValue(); 220 if (codeString == null || "".equals(codeString)) 221 return null; 222 if ("planned".equals(codeString)) 223 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.PLANNED); 224 if ("waitlist".equals(codeString)) 225 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.WAITLIST); 226 if ("active".equals(codeString)) 227 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.ACTIVE); 228 if ("onhold".equals(codeString)) 229 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.ONHOLD); 230 if ("finished".equals(codeString)) 231 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.FINISHED); 232 if ("cancelled".equals(codeString)) 233 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.CANCELLED); 234 throw new FHIRException("Unknown EpisodeOfCareStatus code '" + codeString + "'"); 235 } 236 237 public String toCode(EpisodeOfCareStatus code) 238 { 239 if (code == EpisodeOfCareStatus.NULL) 240 return null; 241 if (code == EpisodeOfCareStatus.PLANNED) 242 return "planned"; 243 if (code == EpisodeOfCareStatus.WAITLIST) 244 return "waitlist"; 245 if (code == EpisodeOfCareStatus.ACTIVE) 246 return "active"; 247 if (code == EpisodeOfCareStatus.ONHOLD) 248 return "onhold"; 249 if (code == EpisodeOfCareStatus.FINISHED) 250 return "finished"; 251 if (code == EpisodeOfCareStatus.CANCELLED) 252 return "cancelled"; 253 return "?"; 254 } 255 } 256 257 @Block() 258 public static class EpisodeOfCareStatusHistoryComponent extends BackboneElement implements IBaseBackboneElement { 259 /** 260 * planned | waitlist | active | onhold | finished | cancelled. 261 */ 262 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 263 @Description(shortDefinition = "planned | waitlist | active | onhold | finished | cancelled", formalDefinition = "planned | waitlist | active | onhold | finished | cancelled.") 264 protected Enumeration<EpisodeOfCareStatus> status; 265 266 /** 267 * The period during this EpisodeOfCare that the specific status applied. 268 */ 269 @Child(name = "period", type = { Period.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 270 @Description(shortDefinition = "Period for the status", formalDefinition = "The period during this EpisodeOfCare that the specific status applied.") 271 protected Period period; 272 273 private static final long serialVersionUID = -1192432864L; 274 275 /* 276 * Constructor 277 */ 278 public EpisodeOfCareStatusHistoryComponent() { 279 super(); 280 } 281 282 /* 283 * Constructor 284 */ 285 public EpisodeOfCareStatusHistoryComponent(Enumeration<EpisodeOfCareStatus> status, Period period) { 286 super(); 287 this.status = status; 288 this.period = period; 289 } 290 291 /** 292 * @return {@link #status} (planned | waitlist | active | onhold | finished | 293 * cancelled.). This is the underlying object with id, value and 294 * extensions. The accessor "getStatus" gives direct access to the value 295 */ 296 public Enumeration<EpisodeOfCareStatus> getStatusElement() { 297 if (this.status == null) 298 if (Configuration.errorOnAutoCreate()) 299 throw new Error("Attempt to auto-create EpisodeOfCareStatusHistoryComponent.status"); 300 else if (Configuration.doAutoCreate()) 301 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); // bb 302 return this.status; 303 } 304 305 public boolean hasStatusElement() { 306 return this.status != null && !this.status.isEmpty(); 307 } 308 309 public boolean hasStatus() { 310 return this.status != null && !this.status.isEmpty(); 311 } 312 313 /** 314 * @param value {@link #status} (planned | waitlist | active | onhold | finished 315 * | cancelled.). This is the underlying object with id, value and 316 * extensions. The accessor "getStatus" gives direct access to the 317 * value 318 */ 319 public EpisodeOfCareStatusHistoryComponent setStatusElement(Enumeration<EpisodeOfCareStatus> value) { 320 this.status = value; 321 return this; 322 } 323 324 /** 325 * @return planned | waitlist | active | onhold | finished | cancelled. 326 */ 327 public EpisodeOfCareStatus getStatus() { 328 return this.status == null ? null : this.status.getValue(); 329 } 330 331 /** 332 * @param value planned | waitlist | active | onhold | finished | cancelled. 333 */ 334 public EpisodeOfCareStatusHistoryComponent setStatus(EpisodeOfCareStatus value) { 335 if (this.status == null) 336 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); 337 this.status.setValue(value); 338 return this; 339 } 340 341 /** 342 * @return {@link #period} (The period during this EpisodeOfCare that the 343 * specific status applied.) 344 */ 345 public Period getPeriod() { 346 if (this.period == null) 347 if (Configuration.errorOnAutoCreate()) 348 throw new Error("Attempt to auto-create EpisodeOfCareStatusHistoryComponent.period"); 349 else if (Configuration.doAutoCreate()) 350 this.period = new Period(); // cc 351 return this.period; 352 } 353 354 public boolean hasPeriod() { 355 return this.period != null && !this.period.isEmpty(); 356 } 357 358 /** 359 * @param value {@link #period} (The period during this EpisodeOfCare that the 360 * specific status applied.) 361 */ 362 public EpisodeOfCareStatusHistoryComponent setPeriod(Period value) { 363 this.period = value; 364 return this; 365 } 366 367 protected void listChildren(List<Property> childrenList) { 368 super.listChildren(childrenList); 369 childrenList.add(new Property("status", "code", "planned | waitlist | active | onhold | finished | cancelled.", 0, 370 java.lang.Integer.MAX_VALUE, status)); 371 childrenList.add( 372 new Property("period", "Period", "The period during this EpisodeOfCare that the specific status applied.", 0, 373 java.lang.Integer.MAX_VALUE, period)); 374 } 375 376 @Override 377 public void setProperty(String name, Base value) throws FHIRException { 378 if (name.equals("status")) 379 this.status = new EpisodeOfCareStatusEnumFactory().fromType(value); // Enumeration<EpisodeOfCareStatus> 380 else if (name.equals("period")) 381 this.period = castToPeriod(value); // Period 382 else 383 super.setProperty(name, value); 384 } 385 386 @Override 387 public Base addChild(String name) throws FHIRException { 388 if (name.equals("status")) { 389 throw new FHIRException("Cannot call addChild on a singleton property EpisodeOfCare.status"); 390 } else if (name.equals("period")) { 391 this.period = new Period(); 392 return this.period; 393 } else 394 return super.addChild(name); 395 } 396 397 public EpisodeOfCareStatusHistoryComponent copy() { 398 EpisodeOfCareStatusHistoryComponent dst = new EpisodeOfCareStatusHistoryComponent(); 399 copyValues(dst); 400 dst.status = status == null ? null : status.copy(); 401 dst.period = period == null ? null : period.copy(); 402 return dst; 403 } 404 405 @Override 406 public boolean equalsDeep(Base other) { 407 if (!super.equalsDeep(other)) 408 return false; 409 if (!(other instanceof EpisodeOfCareStatusHistoryComponent)) 410 return false; 411 EpisodeOfCareStatusHistoryComponent o = (EpisodeOfCareStatusHistoryComponent) other; 412 return compareDeep(status, o.status, true) && compareDeep(period, o.period, true); 413 } 414 415 @Override 416 public boolean equalsShallow(Base other) { 417 if (!super.equalsShallow(other)) 418 return false; 419 if (!(other instanceof EpisodeOfCareStatusHistoryComponent)) 420 return false; 421 EpisodeOfCareStatusHistoryComponent o = (EpisodeOfCareStatusHistoryComponent) other; 422 return compareValues(status, o.status, true); 423 } 424 425 public boolean isEmpty() { 426 return super.isEmpty() && (status == null || status.isEmpty()) && (period == null || period.isEmpty()); 427 } 428 429 public String fhirType() { 430 return "EpisodeOfCare.statusHistory"; 431 432 } 433 434 } 435 436 @Block() 437 public static class EpisodeOfCareCareTeamComponent extends BackboneElement implements IBaseBackboneElement { 438 /** 439 * The role this team member is taking within this episode of care. 440 */ 441 @Child(name = "role", type = { 442 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 443 @Description(shortDefinition = "Role taken by this team member", formalDefinition = "The role this team member is taking within this episode of care.") 444 protected List<CodeableConcept> role; 445 446 /** 447 * The period of time this practitioner is performing some role within the 448 * episode of care. 449 */ 450 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 451 @Description(shortDefinition = "Period of time for this role", formalDefinition = "The period of time this practitioner is performing some role within the episode of care.") 452 protected Period period; 453 454 /** 455 * The practitioner (or Organization) within the team. 456 */ 457 @Child(name = "member", type = { Practitioner.class, 458 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 459 @Description(shortDefinition = "The practitioner (or Organization) within the team", formalDefinition = "The practitioner (or Organization) within the team.") 460 protected Reference member; 461 462 /** 463 * The actual object that is the target of the reference (The practitioner (or 464 * Organization) within the team.) 465 */ 466 protected Resource memberTarget; 467 468 private static final long serialVersionUID = -437303089L; 469 470 /* 471 * Constructor 472 */ 473 public EpisodeOfCareCareTeamComponent() { 474 super(); 475 } 476 477 /** 478 * @return {@link #role} (The role this team member is taking within this 479 * episode of care.) 480 */ 481 public List<CodeableConcept> getRole() { 482 if (this.role == null) 483 this.role = new ArrayList<CodeableConcept>(); 484 return this.role; 485 } 486 487 public boolean hasRole() { 488 if (this.role == null) 489 return false; 490 for (CodeableConcept item : this.role) 491 if (!item.isEmpty()) 492 return true; 493 return false; 494 } 495 496 /** 497 * @return {@link #role} (The role this team member is taking within this 498 * episode of care.) 499 */ 500 // syntactic sugar 501 public CodeableConcept addRole() { // 3 502 CodeableConcept t = new CodeableConcept(); 503 if (this.role == null) 504 this.role = new ArrayList<CodeableConcept>(); 505 this.role.add(t); 506 return t; 507 } 508 509 // syntactic sugar 510 public EpisodeOfCareCareTeamComponent addRole(CodeableConcept t) { // 3 511 if (t == null) 512 return this; 513 if (this.role == null) 514 this.role = new ArrayList<CodeableConcept>(); 515 this.role.add(t); 516 return this; 517 } 518 519 /** 520 * @return {@link #period} (The period of time this practitioner is performing 521 * some role within the episode of care.) 522 */ 523 public Period getPeriod() { 524 if (this.period == null) 525 if (Configuration.errorOnAutoCreate()) 526 throw new Error("Attempt to auto-create EpisodeOfCareCareTeamComponent.period"); 527 else if (Configuration.doAutoCreate()) 528 this.period = new Period(); // cc 529 return this.period; 530 } 531 532 public boolean hasPeriod() { 533 return this.period != null && !this.period.isEmpty(); 534 } 535 536 /** 537 * @param value {@link #period} (The period of time this practitioner is 538 * performing some role within the episode of care.) 539 */ 540 public EpisodeOfCareCareTeamComponent setPeriod(Period value) { 541 this.period = value; 542 return this; 543 } 544 545 /** 546 * @return {@link #member} (The practitioner (or Organization) within the team.) 547 */ 548 public Reference getMember() { 549 if (this.member == null) 550 if (Configuration.errorOnAutoCreate()) 551 throw new Error("Attempt to auto-create EpisodeOfCareCareTeamComponent.member"); 552 else if (Configuration.doAutoCreate()) 553 this.member = new Reference(); // cc 554 return this.member; 555 } 556 557 public boolean hasMember() { 558 return this.member != null && !this.member.isEmpty(); 559 } 560 561 /** 562 * @param value {@link #member} (The practitioner (or Organization) within the 563 * team.) 564 */ 565 public EpisodeOfCareCareTeamComponent setMember(Reference value) { 566 this.member = value; 567 return this; 568 } 569 570 /** 571 * @return {@link #member} The actual object that is the target of the 572 * reference. The reference library doesn't populate this, but you can 573 * use it to hold the resource if you resolve it. (The practitioner (or 574 * Organization) within the team.) 575 */ 576 public Resource getMemberTarget() { 577 return this.memberTarget; 578 } 579 580 /** 581 * @param value {@link #member} The actual object that is the target of the 582 * reference. The reference library doesn't use these, but you can 583 * use it to hold the resource if you resolve it. (The practitioner 584 * (or Organization) within the team.) 585 */ 586 public EpisodeOfCareCareTeamComponent setMemberTarget(Resource value) { 587 this.memberTarget = value; 588 return this; 589 } 590 591 protected void listChildren(List<Property> childrenList) { 592 super.listChildren(childrenList); 593 childrenList.add(new Property("role", "CodeableConcept", 594 "The role this team member is taking within this episode of care.", 0, java.lang.Integer.MAX_VALUE, role)); 595 childrenList.add(new Property("period", "Period", 596 "The period of time this practitioner is performing some role within the episode of care.", 0, 597 java.lang.Integer.MAX_VALUE, period)); 598 childrenList.add(new Property("member", "Reference(Practitioner|Organization)", 599 "The practitioner (or Organization) within the team.", 0, java.lang.Integer.MAX_VALUE, member)); 600 } 601 602 @Override 603 public void setProperty(String name, Base value) throws FHIRException { 604 if (name.equals("role")) 605 this.getRole().add(castToCodeableConcept(value)); 606 else if (name.equals("period")) 607 this.period = castToPeriod(value); // Period 608 else if (name.equals("member")) 609 this.member = castToReference(value); // Reference 610 else 611 super.setProperty(name, value); 612 } 613 614 @Override 615 public Base addChild(String name) throws FHIRException { 616 if (name.equals("role")) { 617 return addRole(); 618 } else if (name.equals("period")) { 619 this.period = new Period(); 620 return this.period; 621 } else if (name.equals("member")) { 622 this.member = new Reference(); 623 return this.member; 624 } else 625 return super.addChild(name); 626 } 627 628 public EpisodeOfCareCareTeamComponent copy() { 629 EpisodeOfCareCareTeamComponent dst = new EpisodeOfCareCareTeamComponent(); 630 copyValues(dst); 631 if (role != null) { 632 dst.role = new ArrayList<CodeableConcept>(); 633 for (CodeableConcept i : role) 634 dst.role.add(i.copy()); 635 } 636 ; 637 dst.period = period == null ? null : period.copy(); 638 dst.member = member == null ? null : member.copy(); 639 return dst; 640 } 641 642 @Override 643 public boolean equalsDeep(Base other) { 644 if (!super.equalsDeep(other)) 645 return false; 646 if (!(other instanceof EpisodeOfCareCareTeamComponent)) 647 return false; 648 EpisodeOfCareCareTeamComponent o = (EpisodeOfCareCareTeamComponent) other; 649 return compareDeep(role, o.role, true) && compareDeep(period, o.period, true) 650 && compareDeep(member, o.member, true); 651 } 652 653 @Override 654 public boolean equalsShallow(Base other) { 655 if (!super.equalsShallow(other)) 656 return false; 657 if (!(other instanceof EpisodeOfCareCareTeamComponent)) 658 return false; 659 EpisodeOfCareCareTeamComponent o = (EpisodeOfCareCareTeamComponent) other; 660 return true; 661 } 662 663 public boolean isEmpty() { 664 return super.isEmpty() && (role == null || role.isEmpty()) && (period == null || period.isEmpty()) 665 && (member == null || member.isEmpty()); 666 } 667 668 public String fhirType() { 669 return "EpisodeOfCare.careTeam"; 670 671 } 672 673 } 674 675 /** 676 * Identifier(s) by which this EpisodeOfCare is known. 677 */ 678 @Child(name = "identifier", type = { 679 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 680 @Description(shortDefinition = "Identifier(s) for the EpisodeOfCare", formalDefinition = "Identifier(s) by which this EpisodeOfCare is known.") 681 protected List<Identifier> identifier; 682 683 /** 684 * planned | waitlist | active | onhold | finished | cancelled. 685 */ 686 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 687 @Description(shortDefinition = "planned | waitlist | active | onhold | finished | cancelled", formalDefinition = "planned | waitlist | active | onhold | finished | cancelled.") 688 protected Enumeration<EpisodeOfCareStatus> status; 689 690 /** 691 * The history of statuses that the EpisodeOfCare has been through (without 692 * requiring processing the history of the resource). 693 */ 694 @Child(name = "statusHistory", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 695 @Description(shortDefinition = "Past list of status codes", formalDefinition = "The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource).") 696 protected List<EpisodeOfCareStatusHistoryComponent> statusHistory; 697 698 /** 699 * A classification of the type of encounter; e.g. specialist referral, disease 700 * management, type of funded care. 701 */ 702 @Child(name = "type", type = { 703 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 704 @Description(shortDefinition = "Type/class - e.g. specialist referral, disease management", formalDefinition = "A classification of the type of encounter; e.g. specialist referral, disease management, type of funded care.") 705 protected List<CodeableConcept> type; 706 707 /** 708 * A list of conditions/problems/diagnoses that this episode of care is intended 709 * to be providing care for. 710 */ 711 @Child(name = "condition", type = { 712 Condition.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 713 @Description(shortDefinition = "Conditions/problems/diagnoses this episode of care is for", formalDefinition = "A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for.") 714 protected List<Reference> condition; 715 /** 716 * The actual objects that are the target of the reference (A list of 717 * conditions/problems/diagnoses that this episode of care is intended to be 718 * providing care for.) 719 */ 720 protected List<Condition> conditionTarget; 721 722 /** 723 * The patient that this EpisodeOfCare applies to. 724 */ 725 @Child(name = "patient", type = { Patient.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 726 @Description(shortDefinition = "Patient for this episode of care", formalDefinition = "The patient that this EpisodeOfCare applies to.") 727 protected Reference patient; 728 729 /** 730 * The actual object that is the target of the reference (The patient that this 731 * EpisodeOfCare applies to.) 732 */ 733 protected Patient patientTarget; 734 735 /** 736 * The organization that has assumed the specific responsibilities for the 737 * specified duration. 738 */ 739 @Child(name = "managingOrganization", type = { 740 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 741 @Description(shortDefinition = "Organization that assumes care", formalDefinition = "The organization that has assumed the specific responsibilities for the specified duration.") 742 protected Reference managingOrganization; 743 744 /** 745 * The actual object that is the target of the reference (The organization that 746 * has assumed the specific responsibilities for the specified duration.) 747 */ 748 protected Organization managingOrganizationTarget; 749 750 /** 751 * The interval during which the managing organization assumes the defined 752 * responsibility. 753 */ 754 @Child(name = "period", type = { Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 755 @Description(shortDefinition = "Interval during responsibility is assumed", formalDefinition = "The interval during which the managing organization assumes the defined responsibility.") 756 protected Period period; 757 758 /** 759 * Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming 760 * referrals. 761 */ 762 @Child(name = "referralRequest", type = { 763 ReferralRequest.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 764 @Description(shortDefinition = "Originating Referral Request(s)", formalDefinition = "Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals.") 765 protected List<Reference> referralRequest; 766 /** 767 * The actual objects that are the target of the reference (Referral Request(s) 768 * that are fulfilled by this EpisodeOfCare, incoming referrals.) 769 */ 770 protected List<ReferralRequest> referralRequestTarget; 771 772 /** 773 * The practitioner that is the care manager/care co-ordinator for this patient. 774 */ 775 @Child(name = "careManager", type = { 776 Practitioner.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 777 @Description(shortDefinition = "Care manager/care co-ordinator for the patient", formalDefinition = "The practitioner that is the care manager/care co-ordinator for this patient.") 778 protected Reference careManager; 779 780 /** 781 * The actual object that is the target of the reference (The practitioner that 782 * is the care manager/care co-ordinator for this patient.) 783 */ 784 protected Practitioner careManagerTarget; 785 786 /** 787 * The list of practitioners that may be facilitating this episode of care for 788 * specific purposes. 789 */ 790 @Child(name = "careTeam", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 791 @Description(shortDefinition = "Other practitioners facilitating this episode of care", formalDefinition = "The list of practitioners that may be facilitating this episode of care for specific purposes.") 792 protected List<EpisodeOfCareCareTeamComponent> careTeam; 793 794 private static final long serialVersionUID = 1652653406L; 795 796 /* 797 * Constructor 798 */ 799 public EpisodeOfCare() { 800 super(); 801 } 802 803 /* 804 * Constructor 805 */ 806 public EpisodeOfCare(Enumeration<EpisodeOfCareStatus> status, Reference patient) { 807 super(); 808 this.status = status; 809 this.patient = patient; 810 } 811 812 /** 813 * @return {@link #identifier} (Identifier(s) by which this EpisodeOfCare is 814 * known.) 815 */ 816 public List<Identifier> getIdentifier() { 817 if (this.identifier == null) 818 this.identifier = new ArrayList<Identifier>(); 819 return this.identifier; 820 } 821 822 public boolean hasIdentifier() { 823 if (this.identifier == null) 824 return false; 825 for (Identifier item : this.identifier) 826 if (!item.isEmpty()) 827 return true; 828 return false; 829 } 830 831 /** 832 * @return {@link #identifier} (Identifier(s) by which this EpisodeOfCare is 833 * known.) 834 */ 835 // syntactic sugar 836 public Identifier addIdentifier() { // 3 837 Identifier t = new Identifier(); 838 if (this.identifier == null) 839 this.identifier = new ArrayList<Identifier>(); 840 this.identifier.add(t); 841 return t; 842 } 843 844 // syntactic sugar 845 public EpisodeOfCare addIdentifier(Identifier t) { // 3 846 if (t == null) 847 return this; 848 if (this.identifier == null) 849 this.identifier = new ArrayList<Identifier>(); 850 this.identifier.add(t); 851 return this; 852 } 853 854 /** 855 * @return {@link #status} (planned | waitlist | active | onhold | finished | 856 * cancelled.). This is the underlying object with id, value and 857 * extensions. The accessor "getStatus" gives direct access to the value 858 */ 859 public Enumeration<EpisodeOfCareStatus> getStatusElement() { 860 if (this.status == null) 861 if (Configuration.errorOnAutoCreate()) 862 throw new Error("Attempt to auto-create EpisodeOfCare.status"); 863 else if (Configuration.doAutoCreate()) 864 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); // bb 865 return this.status; 866 } 867 868 public boolean hasStatusElement() { 869 return this.status != null && !this.status.isEmpty(); 870 } 871 872 public boolean hasStatus() { 873 return this.status != null && !this.status.isEmpty(); 874 } 875 876 /** 877 * @param value {@link #status} (planned | waitlist | active | onhold | finished 878 * | cancelled.). This is the underlying object with id, value and 879 * extensions. The accessor "getStatus" gives direct access to the 880 * value 881 */ 882 public EpisodeOfCare setStatusElement(Enumeration<EpisodeOfCareStatus> value) { 883 this.status = value; 884 return this; 885 } 886 887 /** 888 * @return planned | waitlist | active | onhold | finished | cancelled. 889 */ 890 public EpisodeOfCareStatus getStatus() { 891 return this.status == null ? null : this.status.getValue(); 892 } 893 894 /** 895 * @param value planned | waitlist | active | onhold | finished | cancelled. 896 */ 897 public EpisodeOfCare setStatus(EpisodeOfCareStatus value) { 898 if (this.status == null) 899 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); 900 this.status.setValue(value); 901 return this; 902 } 903 904 /** 905 * @return {@link #statusHistory} (The history of statuses that the 906 * EpisodeOfCare has been through (without requiring processing the 907 * history of the resource).) 908 */ 909 public List<EpisodeOfCareStatusHistoryComponent> getStatusHistory() { 910 if (this.statusHistory == null) 911 this.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 912 return this.statusHistory; 913 } 914 915 public boolean hasStatusHistory() { 916 if (this.statusHistory == null) 917 return false; 918 for (EpisodeOfCareStatusHistoryComponent item : this.statusHistory) 919 if (!item.isEmpty()) 920 return true; 921 return false; 922 } 923 924 /** 925 * @return {@link #statusHistory} (The history of statuses that the 926 * EpisodeOfCare has been through (without requiring processing the 927 * history of the resource).) 928 */ 929 // syntactic sugar 930 public EpisodeOfCareStatusHistoryComponent addStatusHistory() { // 3 931 EpisodeOfCareStatusHistoryComponent t = new EpisodeOfCareStatusHistoryComponent(); 932 if (this.statusHistory == null) 933 this.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 934 this.statusHistory.add(t); 935 return t; 936 } 937 938 // syntactic sugar 939 public EpisodeOfCare addStatusHistory(EpisodeOfCareStatusHistoryComponent t) { // 3 940 if (t == null) 941 return this; 942 if (this.statusHistory == null) 943 this.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 944 this.statusHistory.add(t); 945 return this; 946 } 947 948 /** 949 * @return {@link #type} (A classification of the type of encounter; e.g. 950 * specialist referral, disease management, type of funded care.) 951 */ 952 public List<CodeableConcept> getType() { 953 if (this.type == null) 954 this.type = new ArrayList<CodeableConcept>(); 955 return this.type; 956 } 957 958 public boolean hasType() { 959 if (this.type == null) 960 return false; 961 for (CodeableConcept item : this.type) 962 if (!item.isEmpty()) 963 return true; 964 return false; 965 } 966 967 /** 968 * @return {@link #type} (A classification of the type of encounter; e.g. 969 * specialist referral, disease management, type of funded care.) 970 */ 971 // syntactic sugar 972 public CodeableConcept addType() { // 3 973 CodeableConcept t = new CodeableConcept(); 974 if (this.type == null) 975 this.type = new ArrayList<CodeableConcept>(); 976 this.type.add(t); 977 return t; 978 } 979 980 // syntactic sugar 981 public EpisodeOfCare addType(CodeableConcept t) { // 3 982 if (t == null) 983 return this; 984 if (this.type == null) 985 this.type = new ArrayList<CodeableConcept>(); 986 this.type.add(t); 987 return this; 988 } 989 990 /** 991 * @return {@link #condition} (A list of conditions/problems/diagnoses that this 992 * episode of care is intended to be providing care for.) 993 */ 994 public List<Reference> getCondition() { 995 if (this.condition == null) 996 this.condition = new ArrayList<Reference>(); 997 return this.condition; 998 } 999 1000 public boolean hasCondition() { 1001 if (this.condition == null) 1002 return false; 1003 for (Reference item : this.condition) 1004 if (!item.isEmpty()) 1005 return true; 1006 return false; 1007 } 1008 1009 /** 1010 * @return {@link #condition} (A list of conditions/problems/diagnoses that this 1011 * episode of care is intended to be providing care for.) 1012 */ 1013 // syntactic sugar 1014 public Reference addCondition() { // 3 1015 Reference t = new Reference(); 1016 if (this.condition == null) 1017 this.condition = new ArrayList<Reference>(); 1018 this.condition.add(t); 1019 return t; 1020 } 1021 1022 // syntactic sugar 1023 public EpisodeOfCare addCondition(Reference t) { // 3 1024 if (t == null) 1025 return this; 1026 if (this.condition == null) 1027 this.condition = new ArrayList<Reference>(); 1028 this.condition.add(t); 1029 return this; 1030 } 1031 1032 /** 1033 * @return {@link #condition} (The actual objects that are the target of the 1034 * reference. The reference library doesn't populate this, but you can 1035 * use this to hold the resources if you resolvethemt. A list of 1036 * conditions/problems/diagnoses that this episode of care is intended 1037 * to be providing care for.) 1038 */ 1039 public List<Condition> getConditionTarget() { 1040 if (this.conditionTarget == null) 1041 this.conditionTarget = new ArrayList<Condition>(); 1042 return this.conditionTarget; 1043 } 1044 1045 // syntactic sugar 1046 /** 1047 * @return {@link #condition} (Add an actual object that is the target of the 1048 * reference. The reference library doesn't use these, but you can use 1049 * this to hold the resources if you resolvethemt. A list of 1050 * conditions/problems/diagnoses that this episode of care is intended 1051 * to be providing care for.) 1052 */ 1053 public Condition addConditionTarget() { 1054 Condition r = new Condition(); 1055 if (this.conditionTarget == null) 1056 this.conditionTarget = new ArrayList<Condition>(); 1057 this.conditionTarget.add(r); 1058 return r; 1059 } 1060 1061 /** 1062 * @return {@link #patient} (The patient that this EpisodeOfCare applies to.) 1063 */ 1064 public Reference getPatient() { 1065 if (this.patient == null) 1066 if (Configuration.errorOnAutoCreate()) 1067 throw new Error("Attempt to auto-create EpisodeOfCare.patient"); 1068 else if (Configuration.doAutoCreate()) 1069 this.patient = new Reference(); // cc 1070 return this.patient; 1071 } 1072 1073 public boolean hasPatient() { 1074 return this.patient != null && !this.patient.isEmpty(); 1075 } 1076 1077 /** 1078 * @param value {@link #patient} (The patient that this EpisodeOfCare applies 1079 * to.) 1080 */ 1081 public EpisodeOfCare setPatient(Reference value) { 1082 this.patient = value; 1083 return this; 1084 } 1085 1086 /** 1087 * @return {@link #patient} The actual object that is the target of the 1088 * reference. The reference library doesn't populate this, but you can 1089 * use it to hold the resource if you resolve it. (The patient that this 1090 * EpisodeOfCare applies to.) 1091 */ 1092 public Patient getPatientTarget() { 1093 if (this.patientTarget == null) 1094 if (Configuration.errorOnAutoCreate()) 1095 throw new Error("Attempt to auto-create EpisodeOfCare.patient"); 1096 else if (Configuration.doAutoCreate()) 1097 this.patientTarget = new Patient(); // aa 1098 return this.patientTarget; 1099 } 1100 1101 /** 1102 * @param value {@link #patient} The actual object that is the target of the 1103 * reference. The reference library doesn't use these, but you can 1104 * use it to hold the resource if you resolve it. (The patient that 1105 * this EpisodeOfCare applies to.) 1106 */ 1107 public EpisodeOfCare setPatientTarget(Patient value) { 1108 this.patientTarget = value; 1109 return this; 1110 } 1111 1112 /** 1113 * @return {@link #managingOrganization} (The organization that has assumed the 1114 * specific responsibilities for the specified duration.) 1115 */ 1116 public Reference getManagingOrganization() { 1117 if (this.managingOrganization == null) 1118 if (Configuration.errorOnAutoCreate()) 1119 throw new Error("Attempt to auto-create EpisodeOfCare.managingOrganization"); 1120 else if (Configuration.doAutoCreate()) 1121 this.managingOrganization = new Reference(); // cc 1122 return this.managingOrganization; 1123 } 1124 1125 public boolean hasManagingOrganization() { 1126 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 1127 } 1128 1129 /** 1130 * @param value {@link #managingOrganization} (The organization that has assumed 1131 * the specific responsibilities for the specified duration.) 1132 */ 1133 public EpisodeOfCare setManagingOrganization(Reference value) { 1134 this.managingOrganization = value; 1135 return this; 1136 } 1137 1138 /** 1139 * @return {@link #managingOrganization} The actual object that is the target of 1140 * the reference. The reference library doesn't populate this, but you 1141 * can use it to hold the resource if you resolve it. (The organization 1142 * that has assumed the specific responsibilities for the specified 1143 * duration.) 1144 */ 1145 public Organization getManagingOrganizationTarget() { 1146 if (this.managingOrganizationTarget == null) 1147 if (Configuration.errorOnAutoCreate()) 1148 throw new Error("Attempt to auto-create EpisodeOfCare.managingOrganization"); 1149 else if (Configuration.doAutoCreate()) 1150 this.managingOrganizationTarget = new Organization(); // aa 1151 return this.managingOrganizationTarget; 1152 } 1153 1154 /** 1155 * @param value {@link #managingOrganization} The actual object that is the 1156 * target of the reference. The reference library doesn't use 1157 * these, but you can use it to hold the resource if you resolve 1158 * it. (The organization that has assumed the specific 1159 * responsibilities for the specified duration.) 1160 */ 1161 public EpisodeOfCare setManagingOrganizationTarget(Organization value) { 1162 this.managingOrganizationTarget = value; 1163 return this; 1164 } 1165 1166 /** 1167 * @return {@link #period} (The interval during which the managing organization 1168 * assumes the defined responsibility.) 1169 */ 1170 public Period getPeriod() { 1171 if (this.period == null) 1172 if (Configuration.errorOnAutoCreate()) 1173 throw new Error("Attempt to auto-create EpisodeOfCare.period"); 1174 else if (Configuration.doAutoCreate()) 1175 this.period = new Period(); // cc 1176 return this.period; 1177 } 1178 1179 public boolean hasPeriod() { 1180 return this.period != null && !this.period.isEmpty(); 1181 } 1182 1183 /** 1184 * @param value {@link #period} (The interval during which the managing 1185 * organization assumes the defined responsibility.) 1186 */ 1187 public EpisodeOfCare setPeriod(Period value) { 1188 this.period = value; 1189 return this; 1190 } 1191 1192 /** 1193 * @return {@link #referralRequest} (Referral Request(s) that are fulfilled by 1194 * this EpisodeOfCare, incoming referrals.) 1195 */ 1196 public List<Reference> getReferralRequest() { 1197 if (this.referralRequest == null) 1198 this.referralRequest = new ArrayList<Reference>(); 1199 return this.referralRequest; 1200 } 1201 1202 public boolean hasReferralRequest() { 1203 if (this.referralRequest == null) 1204 return false; 1205 for (Reference item : this.referralRequest) 1206 if (!item.isEmpty()) 1207 return true; 1208 return false; 1209 } 1210 1211 /** 1212 * @return {@link #referralRequest} (Referral Request(s) that are fulfilled by 1213 * this EpisodeOfCare, incoming referrals.) 1214 */ 1215 // syntactic sugar 1216 public Reference addReferralRequest() { // 3 1217 Reference t = new Reference(); 1218 if (this.referralRequest == null) 1219 this.referralRequest = new ArrayList<Reference>(); 1220 this.referralRequest.add(t); 1221 return t; 1222 } 1223 1224 // syntactic sugar 1225 public EpisodeOfCare addReferralRequest(Reference t) { // 3 1226 if (t == null) 1227 return this; 1228 if (this.referralRequest == null) 1229 this.referralRequest = new ArrayList<Reference>(); 1230 this.referralRequest.add(t); 1231 return this; 1232 } 1233 1234 /** 1235 * @return {@link #referralRequest} (The actual objects that are the target of 1236 * the reference. The reference library doesn't populate this, but you 1237 * can use this to hold the resources if you resolvethemt. Referral 1238 * Request(s) that are fulfilled by this EpisodeOfCare, incoming 1239 * referrals.) 1240 */ 1241 public List<ReferralRequest> getReferralRequestTarget() { 1242 if (this.referralRequestTarget == null) 1243 this.referralRequestTarget = new ArrayList<ReferralRequest>(); 1244 return this.referralRequestTarget; 1245 } 1246 1247 // syntactic sugar 1248 /** 1249 * @return {@link #referralRequest} (Add an actual object that is the target of 1250 * the reference. The reference library doesn't use these, but you can 1251 * use this to hold the resources if you resolvethemt. Referral 1252 * Request(s) that are fulfilled by this EpisodeOfCare, incoming 1253 * referrals.) 1254 */ 1255 public ReferralRequest addReferralRequestTarget() { 1256 ReferralRequest r = new ReferralRequest(); 1257 if (this.referralRequestTarget == null) 1258 this.referralRequestTarget = new ArrayList<ReferralRequest>(); 1259 this.referralRequestTarget.add(r); 1260 return r; 1261 } 1262 1263 /** 1264 * @return {@link #careManager} (The practitioner that is the care manager/care 1265 * co-ordinator for this patient.) 1266 */ 1267 public Reference getCareManager() { 1268 if (this.careManager == null) 1269 if (Configuration.errorOnAutoCreate()) 1270 throw new Error("Attempt to auto-create EpisodeOfCare.careManager"); 1271 else if (Configuration.doAutoCreate()) 1272 this.careManager = new Reference(); // cc 1273 return this.careManager; 1274 } 1275 1276 public boolean hasCareManager() { 1277 return this.careManager != null && !this.careManager.isEmpty(); 1278 } 1279 1280 /** 1281 * @param value {@link #careManager} (The practitioner that is the care 1282 * manager/care co-ordinator for this patient.) 1283 */ 1284 public EpisodeOfCare setCareManager(Reference value) { 1285 this.careManager = value; 1286 return this; 1287 } 1288 1289 /** 1290 * @return {@link #careManager} The actual object that is the target of the 1291 * reference. The reference library doesn't populate this, but you can 1292 * use it to hold the resource if you resolve it. (The practitioner that 1293 * is the care manager/care co-ordinator for this patient.) 1294 */ 1295 public Practitioner getCareManagerTarget() { 1296 if (this.careManagerTarget == null) 1297 if (Configuration.errorOnAutoCreate()) 1298 throw new Error("Attempt to auto-create EpisodeOfCare.careManager"); 1299 else if (Configuration.doAutoCreate()) 1300 this.careManagerTarget = new Practitioner(); // aa 1301 return this.careManagerTarget; 1302 } 1303 1304 /** 1305 * @param value {@link #careManager} The actual object that is the target of the 1306 * reference. The reference library doesn't use these, but you can 1307 * use it to hold the resource if you resolve it. (The practitioner 1308 * that is the care manager/care co-ordinator for this patient.) 1309 */ 1310 public EpisodeOfCare setCareManagerTarget(Practitioner value) { 1311 this.careManagerTarget = value; 1312 return this; 1313 } 1314 1315 /** 1316 * @return {@link #careTeam} (The list of practitioners that may be facilitating 1317 * this episode of care for specific purposes.) 1318 */ 1319 public List<EpisodeOfCareCareTeamComponent> getCareTeam() { 1320 if (this.careTeam == null) 1321 this.careTeam = new ArrayList<EpisodeOfCareCareTeamComponent>(); 1322 return this.careTeam; 1323 } 1324 1325 public boolean hasCareTeam() { 1326 if (this.careTeam == null) 1327 return false; 1328 for (EpisodeOfCareCareTeamComponent item : this.careTeam) 1329 if (!item.isEmpty()) 1330 return true; 1331 return false; 1332 } 1333 1334 /** 1335 * @return {@link #careTeam} (The list of practitioners that may be facilitating 1336 * this episode of care for specific purposes.) 1337 */ 1338 // syntactic sugar 1339 public EpisodeOfCareCareTeamComponent addCareTeam() { // 3 1340 EpisodeOfCareCareTeamComponent t = new EpisodeOfCareCareTeamComponent(); 1341 if (this.careTeam == null) 1342 this.careTeam = new ArrayList<EpisodeOfCareCareTeamComponent>(); 1343 this.careTeam.add(t); 1344 return t; 1345 } 1346 1347 // syntactic sugar 1348 public EpisodeOfCare addCareTeam(EpisodeOfCareCareTeamComponent t) { // 3 1349 if (t == null) 1350 return this; 1351 if (this.careTeam == null) 1352 this.careTeam = new ArrayList<EpisodeOfCareCareTeamComponent>(); 1353 this.careTeam.add(t); 1354 return this; 1355 } 1356 1357 protected void listChildren(List<Property> childrenList) { 1358 super.listChildren(childrenList); 1359 childrenList.add(new Property("identifier", "Identifier", "Identifier(s) by which this EpisodeOfCare is known.", 0, 1360 java.lang.Integer.MAX_VALUE, identifier)); 1361 childrenList.add(new Property("status", "code", "planned | waitlist | active | onhold | finished | cancelled.", 0, 1362 java.lang.Integer.MAX_VALUE, status)); 1363 childrenList.add(new Property("statusHistory", "", 1364 "The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource).", 1365 0, java.lang.Integer.MAX_VALUE, statusHistory)); 1366 childrenList.add(new Property("type", "CodeableConcept", 1367 "A classification of the type of encounter; e.g. specialist referral, disease management, type of funded care.", 1368 0, java.lang.Integer.MAX_VALUE, type)); 1369 childrenList.add(new Property("condition", "Reference(Condition)", 1370 "A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for.", 0, 1371 java.lang.Integer.MAX_VALUE, condition)); 1372 childrenList.add(new Property("patient", "Reference(Patient)", "The patient that this EpisodeOfCare applies to.", 0, 1373 java.lang.Integer.MAX_VALUE, patient)); 1374 childrenList.add(new Property("managingOrganization", "Reference(Organization)", 1375 "The organization that has assumed the specific responsibilities for the specified duration.", 0, 1376 java.lang.Integer.MAX_VALUE, managingOrganization)); 1377 childrenList.add(new Property("period", "Period", 1378 "The interval during which the managing organization assumes the defined responsibility.", 0, 1379 java.lang.Integer.MAX_VALUE, period)); 1380 childrenList.add(new Property("referralRequest", "Reference(ReferralRequest)", 1381 "Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals.", 0, 1382 java.lang.Integer.MAX_VALUE, referralRequest)); 1383 childrenList.add(new Property("careManager", "Reference(Practitioner)", 1384 "The practitioner that is the care manager/care co-ordinator for this patient.", 0, java.lang.Integer.MAX_VALUE, 1385 careManager)); 1386 childrenList.add(new Property("careTeam", "", 1387 "The list of practitioners that may be facilitating this episode of care for specific purposes.", 0, 1388 java.lang.Integer.MAX_VALUE, careTeam)); 1389 } 1390 1391 @Override 1392 public void setProperty(String name, Base value) throws FHIRException { 1393 if (name.equals("identifier")) 1394 this.getIdentifier().add(castToIdentifier(value)); 1395 else if (name.equals("status")) 1396 this.status = new EpisodeOfCareStatusEnumFactory().fromType(value); // Enumeration<EpisodeOfCareStatus> 1397 else if (name.equals("statusHistory")) 1398 this.getStatusHistory().add((EpisodeOfCareStatusHistoryComponent) value); 1399 else if (name.equals("type")) 1400 this.getType().add(castToCodeableConcept(value)); 1401 else if (name.equals("condition")) 1402 this.getCondition().add(castToReference(value)); 1403 else if (name.equals("patient")) 1404 this.patient = castToReference(value); // Reference 1405 else if (name.equals("managingOrganization")) 1406 this.managingOrganization = castToReference(value); // Reference 1407 else if (name.equals("period")) 1408 this.period = castToPeriod(value); // Period 1409 else if (name.equals("referralRequest")) 1410 this.getReferralRequest().add(castToReference(value)); 1411 else if (name.equals("careManager")) 1412 this.careManager = castToReference(value); // Reference 1413 else if (name.equals("careTeam")) 1414 this.getCareTeam().add((EpisodeOfCareCareTeamComponent) value); 1415 else 1416 super.setProperty(name, value); 1417 } 1418 1419 @Override 1420 public Base addChild(String name) throws FHIRException { 1421 if (name.equals("identifier")) { 1422 return addIdentifier(); 1423 } else if (name.equals("status")) { 1424 throw new FHIRException("Cannot call addChild on a singleton property EpisodeOfCare.status"); 1425 } else if (name.equals("statusHistory")) { 1426 return addStatusHistory(); 1427 } else if (name.equals("type")) { 1428 return addType(); 1429 } else if (name.equals("condition")) { 1430 return addCondition(); 1431 } else if (name.equals("patient")) { 1432 this.patient = new Reference(); 1433 return this.patient; 1434 } else if (name.equals("managingOrganization")) { 1435 this.managingOrganization = new Reference(); 1436 return this.managingOrganization; 1437 } else if (name.equals("period")) { 1438 this.period = new Period(); 1439 return this.period; 1440 } else if (name.equals("referralRequest")) { 1441 return addReferralRequest(); 1442 } else if (name.equals("careManager")) { 1443 this.careManager = new Reference(); 1444 return this.careManager; 1445 } else if (name.equals("careTeam")) { 1446 return addCareTeam(); 1447 } else 1448 return super.addChild(name); 1449 } 1450 1451 public String fhirType() { 1452 return "EpisodeOfCare"; 1453 1454 } 1455 1456 public EpisodeOfCare copy() { 1457 EpisodeOfCare dst = new EpisodeOfCare(); 1458 copyValues(dst); 1459 if (identifier != null) { 1460 dst.identifier = new ArrayList<Identifier>(); 1461 for (Identifier i : identifier) 1462 dst.identifier.add(i.copy()); 1463 } 1464 ; 1465 dst.status = status == null ? null : status.copy(); 1466 if (statusHistory != null) { 1467 dst.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 1468 for (EpisodeOfCareStatusHistoryComponent i : statusHistory) 1469 dst.statusHistory.add(i.copy()); 1470 } 1471 ; 1472 if (type != null) { 1473 dst.type = new ArrayList<CodeableConcept>(); 1474 for (CodeableConcept i : type) 1475 dst.type.add(i.copy()); 1476 } 1477 ; 1478 if (condition != null) { 1479 dst.condition = new ArrayList<Reference>(); 1480 for (Reference i : condition) 1481 dst.condition.add(i.copy()); 1482 } 1483 ; 1484 dst.patient = patient == null ? null : patient.copy(); 1485 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 1486 dst.period = period == null ? null : period.copy(); 1487 if (referralRequest != null) { 1488 dst.referralRequest = new ArrayList<Reference>(); 1489 for (Reference i : referralRequest) 1490 dst.referralRequest.add(i.copy()); 1491 } 1492 ; 1493 dst.careManager = careManager == null ? null : careManager.copy(); 1494 if (careTeam != null) { 1495 dst.careTeam = new ArrayList<EpisodeOfCareCareTeamComponent>(); 1496 for (EpisodeOfCareCareTeamComponent i : careTeam) 1497 dst.careTeam.add(i.copy()); 1498 } 1499 ; 1500 return dst; 1501 } 1502 1503 protected EpisodeOfCare typedCopy() { 1504 return copy(); 1505 } 1506 1507 @Override 1508 public boolean equalsDeep(Base other) { 1509 if (!super.equalsDeep(other)) 1510 return false; 1511 if (!(other instanceof EpisodeOfCare)) 1512 return false; 1513 EpisodeOfCare o = (EpisodeOfCare) other; 1514 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1515 && compareDeep(statusHistory, o.statusHistory, true) && compareDeep(type, o.type, true) 1516 && compareDeep(condition, o.condition, true) && compareDeep(patient, o.patient, true) 1517 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(period, o.period, true) 1518 && compareDeep(referralRequest, o.referralRequest, true) && compareDeep(careManager, o.careManager, true) 1519 && compareDeep(careTeam, o.careTeam, true); 1520 } 1521 1522 @Override 1523 public boolean equalsShallow(Base other) { 1524 if (!super.equalsShallow(other)) 1525 return false; 1526 if (!(other instanceof EpisodeOfCare)) 1527 return false; 1528 EpisodeOfCare o = (EpisodeOfCare) other; 1529 return compareValues(status, o.status, true); 1530 } 1531 1532 public boolean isEmpty() { 1533 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (status == null || status.isEmpty()) 1534 && (statusHistory == null || statusHistory.isEmpty()) && (type == null || type.isEmpty()) 1535 && (condition == null || condition.isEmpty()) && (patient == null || patient.isEmpty()) 1536 && (managingOrganization == null || managingOrganization.isEmpty()) && (period == null || period.isEmpty()) 1537 && (referralRequest == null || referralRequest.isEmpty()) && (careManager == null || careManager.isEmpty()) 1538 && (careTeam == null || careTeam.isEmpty()); 1539 } 1540 1541 @Override 1542 public ResourceType getResourceType() { 1543 return ResourceType.EpisodeOfCare; 1544 } 1545 1546 @SearchParamDefinition(name = "date", path = "EpisodeOfCare.period", description = "The provided date search value falls within the episode of care's period", type = "date") 1547 public static final String SP_DATE = "date"; 1548 @SearchParamDefinition(name = "identifier", path = "EpisodeOfCare.identifier", description = "Identifier(s) for the EpisodeOfCare", type = "token") 1549 public static final String SP_IDENTIFIER = "identifier"; 1550 @SearchParamDefinition(name = "condition", path = "EpisodeOfCare.condition", description = "Conditions/problems/diagnoses this episode of care is for", type = "reference") 1551 public static final String SP_CONDITION = "condition"; 1552 @SearchParamDefinition(name = "incomingreferral", path = "EpisodeOfCare.referralRequest", description = "Incoming Referral Request", type = "reference") 1553 public static final String SP_INCOMINGREFERRAL = "incomingreferral"; 1554 @SearchParamDefinition(name = "patient", path = "EpisodeOfCare.patient", description = "Patient for this episode of care", type = "reference") 1555 public static final String SP_PATIENT = "patient"; 1556 @SearchParamDefinition(name = "organization", path = "EpisodeOfCare.managingOrganization", description = "The organization that has assumed the specific responsibilities of this EpisodeOfCare", type = "reference") 1557 public static final String SP_ORGANIZATION = "organization"; 1558 @SearchParamDefinition(name = "team-member", path = "EpisodeOfCare.careTeam.member", description = "A Practitioner or Organization allocated to the care team for this EpisodeOfCare", type = "reference") 1559 public static final String SP_TEAMMEMBER = "team-member"; 1560 @SearchParamDefinition(name = "type", path = "EpisodeOfCare.type", description = "Type/class - e.g. specialist referral, disease management", type = "token") 1561 public static final String SP_TYPE = "type"; 1562 @SearchParamDefinition(name = "care-manager", path = "EpisodeOfCare.careManager", description = "Care manager/care co-ordinator for the patient", type = "reference") 1563 public static final String SP_CAREMANAGER = "care-manager"; 1564 @SearchParamDefinition(name = "status", path = "EpisodeOfCare.status", description = "The current status of the Episode of Care as provided (does not check the status history collection)", type = "token") 1565 public static final String SP_STATUS = "status"; 1566 1567}