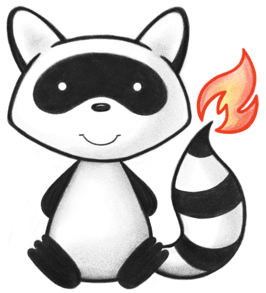
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033 034import ca.uhn.fhir.model.api.annotation.Child; 035import ca.uhn.fhir.model.api.annotation.DatatypeDef; 036import ca.uhn.fhir.model.api.annotation.Description; 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseExtension; 039import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 040 041import java.util.List; 042 043/** 044 * Optional Extensions Element - found in all resources. 045 */ 046@DatatypeDef(name = "Extension") 047public class Extension extends BaseExtension implements IBaseExtension<Extension, Type>, IBaseHasExtensions { 048 049 /** 050 * Source of the definition for the extension code - a logical name or a URL. 051 */ 052 @Child(name = "url", type = { UriType.class }, order = 0, min = 1, max = 1, modifier = false, summary = false) 053 @Description(shortDefinition = "identifies the meaning of the extension", formalDefinition = "Source of the definition for the extension code - a logical name or a URL.") 054 protected UriType url; 055 056 /** 057 * Value of extension - may be a resource or one of a constrained set of the 058 * data types (see Extensibility in the spec for list). 059 */ 060 @Child(name = "value", type = {}, order = 1, min = 0, max = 1, modifier = false, summary = false) 061 @Description(shortDefinition = "Value of extension", formalDefinition = "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).") 062 protected org.hl7.fhir.dstu2.model.Type value; 063 064 private static final long serialVersionUID = 1029480965L; 065 066 /* 067 * Constructor 068 */ 069 public Extension() { 070 super(); 071 } 072 073 /* 074 * Constructor 075 */ 076 public Extension(UriType url) { 077 super(); 078 this.url = url; 079 } 080 081 /** 082 * @return {@link #url} (Source of the definition for the extension code - a 083 * logical name or a URL.). This is the underlying object with id, value 084 * and extensions. The accessor "getUrl" gives direct access to the 085 * value 086 */ 087 public UriType getUrlElement() { 088 if (this.url == null) 089 if (Configuration.errorOnAutoCreate()) 090 throw new Error("Attempt to auto-create Extension.url"); 091 else if (Configuration.doAutoCreate()) 092 this.url = new UriType(); // bb 093 return this.url; 094 } 095 096 public boolean hasUrlElement() { 097 return this.url != null && !this.url.isEmpty(); 098 } 099 100 public boolean hasUrl() { 101 return this.url != null && !this.url.isEmpty(); 102 } 103 104 /** 105 * @param value {@link #url} (Source of the definition for the extension code - 106 * a logical name or a URL.). This is the underlying object with 107 * id, value and extensions. The accessor "getUrl" gives direct 108 * access to the value 109 */ 110 public Extension setUrlElement(UriType value) { 111 this.url = value; 112 return this; 113 } 114 115 /** 116 * @return Source of the definition for the extension code - a logical name or a 117 * URL. 118 */ 119 public String getUrl() { 120 return this.url == null ? null : this.url.getValue(); 121 } 122 123 /** 124 * @param value Source of the definition for the extension code - a logical name 125 * or a URL. 126 */ 127 public Extension setUrl(String value) { 128 if (this.url == null) 129 this.url = new UriType(); 130 this.url.setValue(value); 131 return this; 132 } 133 134 /** 135 * @return {@link #value} (Value of extension - may be a resource or one of a 136 * constrained set of the data types (see Extensibility in the spec for 137 * list).) 138 */ 139 public org.hl7.fhir.dstu2.model.Type getValue() { 140 return this.value; 141 } 142 143 public boolean hasValue() { 144 return this.value != null && !this.value.isEmpty(); 145 } 146 147 /** 148 * @param value {@link #value} (Value of extension - may be a resource or one of 149 * a constrained set of the data types (see Extensibility in the 150 * spec for list).) 151 */ 152 public Extension setValue(org.hl7.fhir.dstu2.model.Type value) { 153 this.value = value; 154 return this; 155 } 156 157 protected void listChildren(List<Property> childrenList) { 158 super.listChildren(childrenList); 159 childrenList 160 .add(new Property("url", "uri", "Source of the definition for the extension code - a logical name or a URL.", 0, 161 java.lang.Integer.MAX_VALUE, url)); 162 childrenList.add(new Property("value[x]", "*", 163 "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 164 0, java.lang.Integer.MAX_VALUE, value)); 165 } 166 167 @Override 168 public void setProperty(String name, Base value) throws FHIRException { 169 if (name.equals("url")) 170 this.url = castToUri(value); // UriType 171 else if (name.equals("value[x]")) 172 this.value = (org.hl7.fhir.dstu2.model.Type) value; // org.hl7.fhir.dstu2.model.Type 173 else 174 super.setProperty(name, value); 175 } 176 177 @Override 178 public Base addChild(String name) throws FHIRException { 179 if (name.equals("url")) { 180 throw new FHIRException("Cannot call addChild on a singleton property Extension.url"); 181 } else if (name.equals("valueBoolean")) { 182 this.value = new BooleanType(); 183 return this.value; 184 } else if (name.equals("valueInteger")) { 185 this.value = new IntegerType(); 186 return this.value; 187 } else if (name.equals("valueDecimal")) { 188 this.value = new DecimalType(); 189 return this.value; 190 } else if (name.equals("valueBase64Binary")) { 191 this.value = new Base64BinaryType(); 192 return this.value; 193 } else if (name.equals("valueInstant")) { 194 this.value = new InstantType(); 195 return this.value; 196 } else if (name.equals("valueString")) { 197 this.value = new StringType(); 198 return this.value; 199 } else if (name.equals("valueUri")) { 200 this.value = new UriType(); 201 return this.value; 202 } else if (name.equals("valueDate")) { 203 this.value = new DateType(); 204 return this.value; 205 } else if (name.equals("valueDateTime")) { 206 this.value = new DateTimeType(); 207 return this.value; 208 } else if (name.equals("valueTime")) { 209 this.value = new TimeType(); 210 return this.value; 211 } else if (name.equals("valueCode")) { 212 this.value = new CodeType(); 213 return this.value; 214 } else if (name.equals("valueOid")) { 215 this.value = new OidType(); 216 return this.value; 217 } else if (name.equals("valueId")) { 218 this.value = new IdType(); 219 return this.value; 220 } else if (name.equals("valueUnsignedInt")) { 221 this.value = new UnsignedIntType(); 222 return this.value; 223 } else if (name.equals("valuePositiveInt")) { 224 this.value = new PositiveIntType(); 225 return this.value; 226 } else if (name.equals("valueMarkdown")) { 227 this.value = new MarkdownType(); 228 return this.value; 229 } else if (name.equals("valueAnnotation")) { 230 this.value = new Annotation(); 231 return this.value; 232 } else if (name.equals("valueAttachment")) { 233 this.value = new Attachment(); 234 return this.value; 235 } else if (name.equals("valueIdentifier")) { 236 this.value = new Identifier(); 237 return this.value; 238 } else if (name.equals("valueCodeableConcept")) { 239 this.value = new CodeableConcept(); 240 return this.value; 241 } else if (name.equals("valueCoding")) { 242 this.value = new Coding(); 243 return this.value; 244 } else if (name.equals("valueQuantity")) { 245 this.value = new Quantity(); 246 return this.value; 247 } else if (name.equals("valueRange")) { 248 this.value = new Range(); 249 return this.value; 250 } else if (name.equals("valuePeriod")) { 251 this.value = new Period(); 252 return this.value; 253 } else if (name.equals("valueRatio")) { 254 this.value = new Ratio(); 255 return this.value; 256 } else if (name.equals("valueSampledData")) { 257 this.value = new SampledData(); 258 return this.value; 259 } else if (name.equals("valueSignature")) { 260 this.value = new Signature(); 261 return this.value; 262 } else if (name.equals("valueHumanName")) { 263 this.value = new HumanName(); 264 return this.value; 265 } else if (name.equals("valueAddress")) { 266 this.value = new Address(); 267 return this.value; 268 } else if (name.equals("valueContactPoint")) { 269 this.value = new ContactPoint(); 270 return this.value; 271 } else if (name.equals("valueTiming")) { 272 this.value = new Timing(); 273 return this.value; 274 } else if (name.equals("valueReference")) { 275 this.value = new Reference(); 276 return this.value; 277 } else if (name.equals("valueMeta")) { 278 this.value = new Meta(); 279 return this.value; 280 } else 281 return super.addChild(name); 282 } 283 284 public String fhirType() { 285 return "Extension"; 286 287 } 288 289 public Extension copy() { 290 Extension dst = new Extension(); 291 copyValues(dst); 292 dst.url = url == null ? null : url.copy(); 293 dst.value = value == null ? null : value.copy(); 294 return dst; 295 } 296 297 protected Extension typedCopy() { 298 return copy(); 299 } 300 301 @Override 302 public boolean equalsDeep(Base other) { 303 if (!super.equalsDeep(other)) 304 return false; 305 if (!(other instanceof Extension)) 306 return false; 307 Extension o = (Extension) other; 308 return compareDeep(url, o.url, true) && compareDeep(value, o.value, true); 309 } 310 311 @Override 312 public boolean equalsShallow(Base other) { 313 if (!super.equalsShallow(other)) 314 return false; 315 if (!(other instanceof Extension)) 316 return false; 317 Extension o = (Extension) other; 318 return compareValues(url, o.url, true); 319 } 320 321 public boolean isEmpty() { 322 return super.isEmpty() && (url == null || url.isEmpty()) && (value == null || value.isEmpty()); 323 } 324 325}