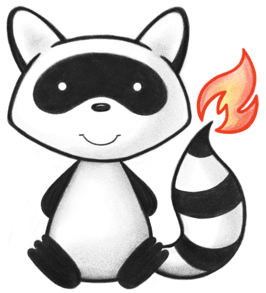
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import org.hl7.fhir.exceptions.FHIRException; 033 034/** 035 * in a language with helper classes, this would be a helper class (at least, 036 * the base exgtension helpers would be) 037 * 038 * @author Grahame 039 * 040 */ 041public class ExtensionHelper { 042 043 /** 044 * @param name the identity of the extension of interest 045 * @return true if the named extension is on this element. Will check modifier 046 * extensions too if appropriate 047 */ 048 public static boolean hasExtension(Element element, String name) { 049 if (element != null && element instanceof BackboneElement) 050 return hasExtension((BackboneElement) element, name); 051 052 if (name == null || element == null || !element.hasExtension()) 053 return false; 054 for (Extension e : element.getExtension()) { 055 if (name.equals(e.getUrl())) 056 return true; 057 } 058 return false; 059 } 060 061 /** 062 * @param name the identity of the extension of interest 063 * @return true if the named extension is on this element. Will check modifier 064 * extensions 065 */ 066 public static boolean hasExtension(BackboneElement element, String name) { 067 if (name == null || element == null || !(element.hasExtension() || element.hasModifierExtension())) 068 return false; 069 for (Extension e : element.getModifierExtension()) { 070 if (name.equals(e.getUrl())) 071 return true; 072 } 073 for (Extension e : element.getExtension()) { 074 if (name.equals(e.getUrl())) 075 return true; 076 } 077 return false; 078 } 079 080 /** 081 * @param name the identity of the extension of interest 082 * @return The extension, if on this element, else null. will check modifier 083 * extensions too, if appropriate 084 */ 085 public static Extension getExtension(Element element, String name) { 086 if (element != null && element instanceof BackboneElement) 087 return getExtension((BackboneElement) element, name); 088 089 if (name == null || element == null || !element.hasExtension()) 090 return null; 091 for (Extension e : element.getExtension()) { 092 if (name.equals(e.getUrl())) 093 return e; 094 } 095 return null; 096 } 097 098 /** 099 * @param name the identity of the extension of interest 100 * @return The extension, if on this element, else null. will check modifier 101 * extensions too 102 */ 103 public static Extension getExtension(BackboneElement element, String name) { 104 if (name == null || element == null || !element.hasExtension()) 105 return null; 106 for (Extension e : element.getModifierExtension()) { 107 if (name.equals(e.getUrl())) 108 return e; 109 } 110 for (Extension e : element.getExtension()) { 111 if (name.equals(e.getUrl())) 112 return e; 113 } 114 return null; 115 } 116 117 /** 118 * set the value of an extension on the element. if value == null, make sure it 119 * doesn't exist 120 * 121 * @param element - the element to act on. Can also be a backbone element 122 * @param modifier - whether this is a modifier. Note that this is a 123 * definitional property of the extension; don't alternate 124 * @param uri - the identifier for the extension 125 * @param value - the value of the extension. Delete if this is null @- if 126 * the modifier logic is incorrect 127 */ 128 public static void setExtension(Element element, boolean modifier, String uri, Type value) throws FHIRException { 129 if (value == null) { 130 // deleting the extension 131 if (element instanceof BackboneElement) 132 for (Extension e : ((BackboneElement) element).getModifierExtension()) { 133 if (uri.equals(e.getUrl())) 134 ((BackboneElement) element).getModifierExtension().remove(e); 135 } 136 for (Extension e : element.getExtension()) { 137 if (uri.equals(e.getUrl())) 138 element.getExtension().remove(e); 139 } 140 } else { 141 // it would probably be easier to delete and then create, but this would 142 // re-order the extensions 143 // not that order matters, but we'll preserve it anyway 144 boolean found = false; 145 if (element instanceof BackboneElement) 146 for (Extension e : ((BackboneElement) element).getModifierExtension()) { 147 if (uri.equals(e.getUrl())) { 148 if (!modifier) 149 throw new FHIRException("Error adding extension \"" + uri 150 + "\": found an existing modifier extension, and the extension is not marked as a modifier"); 151 e.setValue(value); 152 found = true; 153 } 154 } 155 for (Extension e : element.getExtension()) { 156 if (uri.equals(e.getUrl())) { 157 if (modifier) 158 throw new FHIRException("Error adding extension \"" + uri 159 + "\": found an existing extension, and the extension is marked as a modifier"); 160 e.setValue(value); 161 found = true; 162 } 163 } 164 if (!found) { 165 Extension ex = new Extension().setUrl(uri).setValue(value); 166 if (modifier) { 167 if (!(element instanceof BackboneElement)) 168 throw new FHIRException("Error adding extension \"" + uri 169 + "\": extension is marked as a modifier, but element is not a backbone element"); 170 ((BackboneElement) element).getModifierExtension().add(ex); 171 172 } else { 173 element.getExtension().add(ex); 174 } 175 } 176 } 177 } 178 179 public static boolean hasExtensions(Element element) { 180 if (element instanceof BackboneElement) 181 return element.hasExtension() || ((BackboneElement) element).hasModifierExtension(); 182 else 183 return element.hasExtension(); 184 } 185 186}