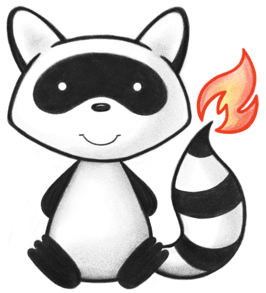
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.io.IOException; 033import java.net.URISyntaxException; 034import java.text.ParseException; 035import java.util.UUID; 036 037import org.hl7.fhir.dstu2.model.ContactPoint.ContactPointSystem; 038import org.hl7.fhir.dstu2.model.Narrative.NarrativeStatus; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.utilities.Utilities; 041import org.hl7.fhir.utilities.xhtml.XhtmlParser; 042 043/* 044Copyright (c) 2011+, HL7, Inc 045All rights reserved. 046 047Redistribution and use in source and binary forms, with or without modification, 048are permitted provided that the following conditions are met: 049 050 * Redistributions of source code must retain the above copyright notice, this 051 list of conditions and the following disclaimer. 052 * Redistributions in binary form must reproduce the above copyright notice, 053 this list of conditions and the following disclaimer in the documentation 054 and/or other materials provided with the distribution. 055 * Neither the name of HL7 nor the names of its contributors may be used to 056 endorse or promote products derived from this software without specific 057 prior written permission. 058 059THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 060ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 061WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 062IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 063INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 064NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 065PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 066WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 067ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 068POSSIBILITY OF SUCH DAMAGE. 069 070*/ 071 072public class Factory { 073 074 public static IdType newId(String value) { 075 if (value == null) 076 return null; 077 IdType res = new IdType(); 078 res.setValue(value); 079 return res; 080 } 081 082 public static StringType newString_(String value) { 083 if (value == null) 084 return null; 085 StringType res = new StringType(); 086 res.setValue(value); 087 return res; 088 } 089 090 public static UriType newUri(String value) throws URISyntaxException { 091 if (value == null) 092 return null; 093 UriType res = new UriType(); 094 res.setValue(value); 095 return res; 096 } 097 098 public static DateTimeType newDateTime(String value) throws ParseException { 099 if (value == null) 100 return null; 101 return new DateTimeType(value); 102 } 103 104 public static DateType newDate(String value) throws ParseException { 105 if (value == null) 106 return null; 107 return new DateType(value); 108 } 109 110 public static CodeType newCode(String value) { 111 if (value == null) 112 return null; 113 CodeType res = new CodeType(); 114 res.setValue(value); 115 return res; 116 } 117 118 public static IntegerType newInteger(int value) { 119 IntegerType res = new IntegerType(); 120 res.setValue(value); 121 return res; 122 } 123 124 public static IntegerType newInteger(java.lang.Integer value) { 125 if (value == null) 126 return null; 127 IntegerType res = new IntegerType(); 128 res.setValue(value); 129 return res; 130 } 131 132 public static BooleanType newBoolean(boolean value) { 133 BooleanType res = new BooleanType(); 134 res.setValue(value); 135 return res; 136 } 137 138 public static ContactPoint newContactPoint(ContactPointSystem system, String value) { 139 ContactPoint res = new ContactPoint(); 140 res.setSystem(system); 141 res.setValue(value); 142 return res; 143 } 144 145 public static Extension newExtension(String uri, Type value, boolean evenIfNull) { 146 if (!evenIfNull && (value == null || value.isEmpty())) 147 return null; 148 Extension e = new Extension(); 149 e.setUrl(uri); 150 e.setValue(value); 151 return e; 152 } 153 154 public static CodeableConcept newCodeableConcept(String code, String system, String display) { 155 CodeableConcept cc = new CodeableConcept(); 156 Coding c = new Coding(); 157 c.setCode(code); 158 c.setSystem(system); 159 c.setDisplay(display); 160 cc.getCoding().add(c); 161 return cc; 162 } 163 164 public static Reference makeReference(String url) { 165 Reference rr = new Reference(); 166 rr.setReference(url); 167 return rr; 168 } 169 170 public static Narrative newNarrative(NarrativeStatus status, String html) throws IOException, FHIRException { 171 Narrative n = new Narrative(); 172 n.setStatus(status); 173 n.setDiv(new XhtmlParser().parseFragment("<div>" + Utilities.escapeXml(html) + "</div>")); 174 return n; 175 } 176 177 public static Coding makeCoding(String code) throws FHIRException { 178 String[] parts = code.split("\\|"); 179 Coding c = new Coding(); 180 if (parts.length == 2) { 181 c.setSystem(parts[0]); 182 c.setCode(parts[1]); 183 } else if (parts.length == 3) { 184 c.setSystem(parts[0]); 185 c.setCode(parts[1]); 186 c.setDisplay(parts[2]); 187 } else 188 throw new FHIRException("Unable to understand the code '" + code + "'. Use the format system|code(|display)"); 189 return c; 190 } 191 192 public static Reference makeReference(String url, String text) { 193 Reference rr = new Reference(); 194 rr.setReference(url); 195 if (!Utilities.noString(text)) 196 rr.setDisplay(text); 197 return rr; 198 } 199 200 public static String createUUID() { 201 return "urn:uuid:" + UUID.randomUUID().toString().toLowerCase(); 202 } 203 204 public Type create(String name) throws FHIRException { 205 if (name.equals("boolean")) 206 return new BooleanType(); 207 else if (name.equals("integer")) 208 return new IntegerType(); 209 else if (name.equals("decimal")) 210 return new DecimalType(); 211 else if (name.equals("base64Binary")) 212 return new Base64BinaryType(); 213 else if (name.equals("instant")) 214 return new InstantType(); 215 else if (name.equals("string")) 216 return new StringType(); 217 else if (name.equals("uri")) 218 return new UriType(); 219 else if (name.equals("date")) 220 return new DateType(); 221 else if (name.equals("dateTime")) 222 return new DateTimeType(); 223 else if (name.equals("time")) 224 return new TimeType(); 225 else if (name.equals("code")) 226 return new CodeType(); 227 else if (name.equals("oid")) 228 return new OidType(); 229 else if (name.equals("id")) 230 return new IdType(); 231 else if (name.equals("unsignedInt")) 232 return new UnsignedIntType(); 233 else if (name.equals("positiveInt")) 234 return new PositiveIntType(); 235 else if (name.equals("markdown")) 236 return new MarkdownType(); 237 else if (name.equals("Annotation")) 238 return new Annotation(); 239 else if (name.equals("Attachment")) 240 return new Attachment(); 241 else if (name.equals("Identifier")) 242 return new Identifier(); 243 else if (name.equals("CodeableConcept")) 244 return new CodeableConcept(); 245 else if (name.equals("Coding")) 246 return new Coding(); 247 else if (name.equals("Quantity")) 248 return new Quantity(); 249 else if (name.equals("Range")) 250 return new Range(); 251 else if (name.equals("Period")) 252 return new Period(); 253 else if (name.equals("Ratio")) 254 return new Ratio(); 255 else if (name.equals("SampledData")) 256 return new SampledData(); 257 else if (name.equals("Signature")) 258 return new Signature(); 259 else if (name.equals("HumanName")) 260 return new HumanName(); 261 else if (name.equals("Address")) 262 return new Address(); 263 else if (name.equals("ContactPoint")) 264 return new ContactPoint(); 265 else if (name.equals("Timing")) 266 return new Timing(); 267 else if (name.equals("Reference")) 268 return new Reference(); 269 else if (name.equals("Meta")) 270 return new Meta(); 271 else 272 throw new FHIRException("Unknown data type name " + name); 273 } 274}