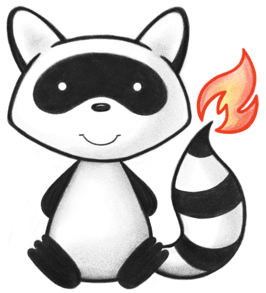
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender; 038import org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGenderEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.utilities.Utilities; 047 048/** 049 * Significant health events and conditions for a person related to the patient 050 * relevant in the context of care for the patient. 051 */ 052@ResourceDef(name = "FamilyMemberHistory", profile = "http://hl7.org/fhir/Profile/FamilyMemberHistory") 053public class FamilyMemberHistory extends DomainResource { 054 055 public enum FamilyHistoryStatus { 056 /** 057 * Some health information is known and captured, but not complete - see notes 058 * for details. 059 */ 060 PARTIAL, 061 /** 062 * All relevant health information is known and captured. 063 */ 064 COMPLETED, 065 /** 066 * This instance should not have been part of this patient's medical record. 067 */ 068 ENTEREDINERROR, 069 /** 070 * Health information for this individual is unavailable/unknown. 071 */ 072 HEALTHUNKNOWN, 073 /** 074 * added to help the parsers 075 */ 076 NULL; 077 078 public static FamilyHistoryStatus fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("partial".equals(codeString)) 082 return PARTIAL; 083 if ("completed".equals(codeString)) 084 return COMPLETED; 085 if ("entered-in-error".equals(codeString)) 086 return ENTEREDINERROR; 087 if ("health-unknown".equals(codeString)) 088 return HEALTHUNKNOWN; 089 throw new FHIRException("Unknown FamilyHistoryStatus code '" + codeString + "'"); 090 } 091 092 public String toCode() { 093 switch (this) { 094 case PARTIAL: 095 return "partial"; 096 case COMPLETED: 097 return "completed"; 098 case ENTEREDINERROR: 099 return "entered-in-error"; 100 case HEALTHUNKNOWN: 101 return "health-unknown"; 102 case NULL: 103 return null; 104 default: 105 return "?"; 106 } 107 } 108 109 public String getSystem() { 110 switch (this) { 111 case PARTIAL: 112 return "http://hl7.org/fhir/history-status"; 113 case COMPLETED: 114 return "http://hl7.org/fhir/history-status"; 115 case ENTEREDINERROR: 116 return "http://hl7.org/fhir/history-status"; 117 case HEALTHUNKNOWN: 118 return "http://hl7.org/fhir/history-status"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getDefinition() { 127 switch (this) { 128 case PARTIAL: 129 return "Some health information is known and captured, but not complete - see notes for details."; 130 case COMPLETED: 131 return "All relevant health information is known and captured."; 132 case ENTEREDINERROR: 133 return "This instance should not have been part of this patient's medical record."; 134 case HEALTHUNKNOWN: 135 return "Health information for this individual is unavailable/unknown."; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getDisplay() { 144 switch (this) { 145 case PARTIAL: 146 return "Partial"; 147 case COMPLETED: 148 return "Completed"; 149 case ENTEREDINERROR: 150 return "Entered in error"; 151 case HEALTHUNKNOWN: 152 return "Health unknown"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 } 160 161 public static class FamilyHistoryStatusEnumFactory implements EnumFactory<FamilyHistoryStatus> { 162 public FamilyHistoryStatus fromCode(String codeString) throws IllegalArgumentException { 163 if (codeString == null || "".equals(codeString)) 164 if (codeString == null || "".equals(codeString)) 165 return null; 166 if ("partial".equals(codeString)) 167 return FamilyHistoryStatus.PARTIAL; 168 if ("completed".equals(codeString)) 169 return FamilyHistoryStatus.COMPLETED; 170 if ("entered-in-error".equals(codeString)) 171 return FamilyHistoryStatus.ENTEREDINERROR; 172 if ("health-unknown".equals(codeString)) 173 return FamilyHistoryStatus.HEALTHUNKNOWN; 174 throw new IllegalArgumentException("Unknown FamilyHistoryStatus code '" + codeString + "'"); 175 } 176 177 public Enumeration<FamilyHistoryStatus> fromType(Base code) throws FHIRException { 178 if (code == null || code.isEmpty()) 179 return null; 180 String codeString = ((PrimitiveType) code).asStringValue(); 181 if (codeString == null || "".equals(codeString)) 182 return null; 183 if ("partial".equals(codeString)) 184 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.PARTIAL); 185 if ("completed".equals(codeString)) 186 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.COMPLETED); 187 if ("entered-in-error".equals(codeString)) 188 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.ENTEREDINERROR); 189 if ("health-unknown".equals(codeString)) 190 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.HEALTHUNKNOWN); 191 throw new FHIRException("Unknown FamilyHistoryStatus code '" + codeString + "'"); 192 } 193 194 public String toCode(FamilyHistoryStatus code) { 195 if (code == FamilyHistoryStatus.PARTIAL) 196 return "partial"; 197 if (code == FamilyHistoryStatus.COMPLETED) 198 return "completed"; 199 if (code == FamilyHistoryStatus.ENTEREDINERROR) 200 return "entered-in-error"; 201 if (code == FamilyHistoryStatus.HEALTHUNKNOWN) 202 return "health-unknown"; 203 return "?"; 204 } 205 } 206 207 @Block() 208 public static class FamilyMemberHistoryConditionComponent extends BackboneElement implements IBaseBackboneElement { 209 /** 210 * The actual condition specified. Could be a coded condition (like MI or 211 * Diabetes) or a less specific string like 'cancer' depending on how much is 212 * known about the condition and the capabilities of the creating system. 213 */ 214 @Child(name = "code", type = { 215 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 216 @Description(shortDefinition = "Condition suffered by relation", formalDefinition = "The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system.") 217 protected CodeableConcept code; 218 219 /** 220 * Indicates what happened as a result of this condition. If the condition 221 * resulted in death, deceased date is captured on the relation. 222 */ 223 @Child(name = "outcome", type = { 224 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 225 @Description(shortDefinition = "deceased | permanent disability | etc.", formalDefinition = "Indicates what happened as a result of this condition. If the condition resulted in death, deceased date is captured on the relation.") 226 protected CodeableConcept outcome; 227 228 /** 229 * Either the age of onset, range of approximate age or descriptive string can 230 * be recorded. For conditions with multiple occurrences, this describes the 231 * first known occurrence. 232 */ 233 @Child(name = "onset", type = { Age.class, Range.class, Period.class, 234 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 235 @Description(shortDefinition = "When condition first manifested", formalDefinition = "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.") 236 protected Type onset; 237 238 /** 239 * An area where general notes can be placed about this specific condition. 240 */ 241 @Child(name = "note", type = { Annotation.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 242 @Description(shortDefinition = "Extra information about condition", formalDefinition = "An area where general notes can be placed about this specific condition.") 243 protected Annotation note; 244 245 private static final long serialVersionUID = -1221569121L; 246 247 /* 248 * Constructor 249 */ 250 public FamilyMemberHistoryConditionComponent() { 251 super(); 252 } 253 254 /* 255 * Constructor 256 */ 257 public FamilyMemberHistoryConditionComponent(CodeableConcept code) { 258 super(); 259 this.code = code; 260 } 261 262 /** 263 * @return {@link #code} (The actual condition specified. Could be a coded 264 * condition (like MI or Diabetes) or a less specific string like 265 * 'cancer' depending on how much is known about the condition and the 266 * capabilities of the creating system.) 267 */ 268 public CodeableConcept getCode() { 269 if (this.code == null) 270 if (Configuration.errorOnAutoCreate()) 271 throw new Error("Attempt to auto-create FamilyMemberHistoryConditionComponent.code"); 272 else if (Configuration.doAutoCreate()) 273 this.code = new CodeableConcept(); // cc 274 return this.code; 275 } 276 277 public boolean hasCode() { 278 return this.code != null && !this.code.isEmpty(); 279 } 280 281 /** 282 * @param value {@link #code} (The actual condition specified. Could be a coded 283 * condition (like MI or Diabetes) or a less specific string like 284 * 'cancer' depending on how much is known about the condition and 285 * the capabilities of the creating system.) 286 */ 287 public FamilyMemberHistoryConditionComponent setCode(CodeableConcept value) { 288 this.code = value; 289 return this; 290 } 291 292 /** 293 * @return {@link #outcome} (Indicates what happened as a result of this 294 * condition. If the condition resulted in death, deceased date is 295 * captured on the relation.) 296 */ 297 public CodeableConcept getOutcome() { 298 if (this.outcome == null) 299 if (Configuration.errorOnAutoCreate()) 300 throw new Error("Attempt to auto-create FamilyMemberHistoryConditionComponent.outcome"); 301 else if (Configuration.doAutoCreate()) 302 this.outcome = new CodeableConcept(); // cc 303 return this.outcome; 304 } 305 306 public boolean hasOutcome() { 307 return this.outcome != null && !this.outcome.isEmpty(); 308 } 309 310 /** 311 * @param value {@link #outcome} (Indicates what happened as a result of this 312 * condition. If the condition resulted in death, deceased date is 313 * captured on the relation.) 314 */ 315 public FamilyMemberHistoryConditionComponent setOutcome(CodeableConcept value) { 316 this.outcome = value; 317 return this; 318 } 319 320 /** 321 * @return {@link #onset} (Either the age of onset, range of approximate age or 322 * descriptive string can be recorded. For conditions with multiple 323 * occurrences, this describes the first known occurrence.) 324 */ 325 public Type getOnset() { 326 return this.onset; 327 } 328 329 /** 330 * @return {@link #onset} (Either the age of onset, range of approximate age or 331 * descriptive string can be recorded. For conditions with multiple 332 * occurrences, this describes the first known occurrence.) 333 */ 334 public Age getOnsetAge() throws FHIRException { 335 if (!(this.onset instanceof Age)) 336 throw new FHIRException( 337 "Type mismatch: the type Age was expected, but " + this.onset.getClass().getName() + " was encountered"); 338 return (Age) this.onset; 339 } 340 341 public boolean hasOnsetAge() { 342 return this.onset instanceof Age; 343 } 344 345 /** 346 * @return {@link #onset} (Either the age of onset, range of approximate age or 347 * descriptive string can be recorded. For conditions with multiple 348 * occurrences, this describes the first known occurrence.) 349 */ 350 public Range getOnsetRange() throws FHIRException { 351 if (!(this.onset instanceof Range)) 352 throw new FHIRException( 353 "Type mismatch: the type Range was expected, but " + this.onset.getClass().getName() + " was encountered"); 354 return (Range) this.onset; 355 } 356 357 public boolean hasOnsetRange() { 358 return this.onset instanceof Range; 359 } 360 361 /** 362 * @return {@link #onset} (Either the age of onset, range of approximate age or 363 * descriptive string can be recorded. For conditions with multiple 364 * occurrences, this describes the first known occurrence.) 365 */ 366 public Period getOnsetPeriod() throws FHIRException { 367 if (!(this.onset instanceof Period)) 368 throw new FHIRException( 369 "Type mismatch: the type Period was expected, but " + this.onset.getClass().getName() + " was encountered"); 370 return (Period) this.onset; 371 } 372 373 public boolean hasOnsetPeriod() { 374 return this.onset instanceof Period; 375 } 376 377 /** 378 * @return {@link #onset} (Either the age of onset, range of approximate age or 379 * descriptive string can be recorded. For conditions with multiple 380 * occurrences, this describes the first known occurrence.) 381 */ 382 public StringType getOnsetStringType() throws FHIRException { 383 if (!(this.onset instanceof StringType)) 384 throw new FHIRException("Type mismatch: the type StringType was expected, but " 385 + this.onset.getClass().getName() + " was encountered"); 386 return (StringType) this.onset; 387 } 388 389 public boolean hasOnsetStringType() { 390 return this.onset instanceof StringType; 391 } 392 393 public boolean hasOnset() { 394 return this.onset != null && !this.onset.isEmpty(); 395 } 396 397 /** 398 * @param value {@link #onset} (Either the age of onset, range of approximate 399 * age or descriptive string can be recorded. For conditions with 400 * multiple occurrences, this describes the first known 401 * occurrence.) 402 */ 403 public FamilyMemberHistoryConditionComponent setOnset(Type value) { 404 this.onset = value; 405 return this; 406 } 407 408 /** 409 * @return {@link #note} (An area where general notes can be placed about this 410 * specific condition.) 411 */ 412 public Annotation getNote() { 413 if (this.note == null) 414 if (Configuration.errorOnAutoCreate()) 415 throw new Error("Attempt to auto-create FamilyMemberHistoryConditionComponent.note"); 416 else if (Configuration.doAutoCreate()) 417 this.note = new Annotation(); // cc 418 return this.note; 419 } 420 421 public boolean hasNote() { 422 return this.note != null && !this.note.isEmpty(); 423 } 424 425 /** 426 * @param value {@link #note} (An area where general notes can be placed about 427 * this specific condition.) 428 */ 429 public FamilyMemberHistoryConditionComponent setNote(Annotation value) { 430 this.note = value; 431 return this; 432 } 433 434 protected void listChildren(List<Property> childrenList) { 435 super.listChildren(childrenList); 436 childrenList.add(new Property("code", "CodeableConcept", 437 "The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system.", 438 0, java.lang.Integer.MAX_VALUE, code)); 439 childrenList.add(new Property("outcome", "CodeableConcept", 440 "Indicates what happened as a result of this condition. If the condition resulted in death, deceased date is captured on the relation.", 441 0, java.lang.Integer.MAX_VALUE, outcome)); 442 childrenList.add(new Property("onset[x]", "Age|Range|Period|string", 443 "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 444 0, java.lang.Integer.MAX_VALUE, onset)); 445 childrenList.add( 446 new Property("note", "Annotation", "An area where general notes can be placed about this specific condition.", 447 0, java.lang.Integer.MAX_VALUE, note)); 448 } 449 450 @Override 451 public void setProperty(String name, Base value) throws FHIRException { 452 if (name.equals("code")) 453 this.code = castToCodeableConcept(value); // CodeableConcept 454 else if (name.equals("outcome")) 455 this.outcome = castToCodeableConcept(value); // CodeableConcept 456 else if (name.equals("onset[x]")) 457 this.onset = (Type) value; // Type 458 else if (name.equals("note")) 459 this.note = castToAnnotation(value); // Annotation 460 else 461 super.setProperty(name, value); 462 } 463 464 @Override 465 public Base addChild(String name) throws FHIRException { 466 if (name.equals("code")) { 467 this.code = new CodeableConcept(); 468 return this.code; 469 } else if (name.equals("outcome")) { 470 this.outcome = new CodeableConcept(); 471 return this.outcome; 472 } else if (name.equals("onsetAge")) { 473 this.onset = new Age(); 474 return this.onset; 475 } else if (name.equals("onsetRange")) { 476 this.onset = new Range(); 477 return this.onset; 478 } else if (name.equals("onsetPeriod")) { 479 this.onset = new Period(); 480 return this.onset; 481 } else if (name.equals("onsetString")) { 482 this.onset = new StringType(); 483 return this.onset; 484 } else if (name.equals("note")) { 485 this.note = new Annotation(); 486 return this.note; 487 } else 488 return super.addChild(name); 489 } 490 491 public FamilyMemberHistoryConditionComponent copy() { 492 FamilyMemberHistoryConditionComponent dst = new FamilyMemberHistoryConditionComponent(); 493 copyValues(dst); 494 dst.code = code == null ? null : code.copy(); 495 dst.outcome = outcome == null ? null : outcome.copy(); 496 dst.onset = onset == null ? null : onset.copy(); 497 dst.note = note == null ? null : note.copy(); 498 return dst; 499 } 500 501 @Override 502 public boolean equalsDeep(Base other) { 503 if (!super.equalsDeep(other)) 504 return false; 505 if (!(other instanceof FamilyMemberHistoryConditionComponent)) 506 return false; 507 FamilyMemberHistoryConditionComponent o = (FamilyMemberHistoryConditionComponent) other; 508 return compareDeep(code, o.code, true) && compareDeep(outcome, o.outcome, true) 509 && compareDeep(onset, o.onset, true) && compareDeep(note, o.note, true); 510 } 511 512 @Override 513 public boolean equalsShallow(Base other) { 514 if (!super.equalsShallow(other)) 515 return false; 516 if (!(other instanceof FamilyMemberHistoryConditionComponent)) 517 return false; 518 FamilyMemberHistoryConditionComponent o = (FamilyMemberHistoryConditionComponent) other; 519 return true; 520 } 521 522 public boolean isEmpty() { 523 return super.isEmpty() && (code == null || code.isEmpty()) && (outcome == null || outcome.isEmpty()) 524 && (onset == null || onset.isEmpty()) && (note == null || note.isEmpty()); 525 } 526 527 public String fhirType() { 528 return "FamilyMemberHistory.condition"; 529 530 } 531 532 } 533 534 /** 535 * This records identifiers associated with this family member history record 536 * that are defined by business processes and/ or used to refer to it when a 537 * direct URL reference to the resource itself is not appropriate (e.g. in CDA 538 * documents, or in written / printed documentation). 539 */ 540 @Child(name = "identifier", type = { 541 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 542 @Description(shortDefinition = "External Id(s) for this record", formalDefinition = "This records identifiers associated with this family member history record that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).") 543 protected List<Identifier> identifier; 544 545 /** 546 * The person who this history concerns. 547 */ 548 @Child(name = "patient", type = { Patient.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 549 @Description(shortDefinition = "Patient history is about", formalDefinition = "The person who this history concerns.") 550 protected Reference patient; 551 552 /** 553 * The actual object that is the target of the reference (The person who this 554 * history concerns.) 555 */ 556 protected Patient patientTarget; 557 558 /** 559 * The date (and possibly time) when the family member history was taken. 560 */ 561 @Child(name = "date", type = { DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 562 @Description(shortDefinition = "When history was captured/updated", formalDefinition = "The date (and possibly time) when the family member history was taken.") 563 protected DateTimeType date; 564 565 /** 566 * A code specifying a state of a Family Member History record. 567 */ 568 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = true) 569 @Description(shortDefinition = "partial | completed | entered-in-error | health-unknown", formalDefinition = "A code specifying a state of a Family Member History record.") 570 protected Enumeration<FamilyHistoryStatus> status; 571 572 /** 573 * This will either be a name or a description; e.g. "Aunt Susan", "my cousin 574 * with the red hair". 575 */ 576 @Child(name = "name", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 577 @Description(shortDefinition = "The family member described", formalDefinition = "This will either be a name or a description; e.g. \"Aunt Susan\", \"my cousin with the red hair\".") 578 protected StringType name; 579 580 /** 581 * The type of relationship this person has to the patient (father, mother, 582 * brother etc.). 583 */ 584 @Child(name = "relationship", type = { 585 CodeableConcept.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 586 @Description(shortDefinition = "Relationship to the subject", formalDefinition = "The type of relationship this person has to the patient (father, mother, brother etc.).") 587 protected CodeableConcept relationship; 588 589 /** 590 * Administrative Gender - the gender that the relative is considered to have 591 * for administration and record keeping purposes. 592 */ 593 @Child(name = "gender", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 594 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender - the gender that the relative is considered to have for administration and record keeping purposes.") 595 protected Enumeration<AdministrativeGender> gender; 596 597 /** 598 * The actual or approximate date of birth of the relative. 599 */ 600 @Child(name = "born", type = { Period.class, DateType.class, 601 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 602 @Description(shortDefinition = "(approximate) date of birth", formalDefinition = "The actual or approximate date of birth of the relative.") 603 protected Type born; 604 605 /** 606 * The actual or approximate age of the relative at the time the family member 607 * history is recorded. 608 */ 609 @Child(name = "age", type = { Age.class, Range.class, 610 StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 611 @Description(shortDefinition = "(approximate) age", formalDefinition = "The actual or approximate age of the relative at the time the family member history is recorded.") 612 protected Type age; 613 614 /** 615 * Deceased flag or the actual or approximate age of the relative at the time of 616 * death for the family member history record. 617 */ 618 @Child(name = "deceased", type = { BooleanType.class, Age.class, Range.class, DateType.class, 619 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 620 @Description(shortDefinition = "Dead? How old/when?", formalDefinition = "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.") 621 protected Type deceased; 622 623 /** 624 * This property allows a non condition-specific note to the made about the 625 * related person. Ideally, the note would be in the condition property, but 626 * this is not always possible. 627 */ 628 @Child(name = "note", type = { Annotation.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 629 @Description(shortDefinition = "General note about related person", formalDefinition = "This property allows a non condition-specific note to the made about the related person. Ideally, the note would be in the condition property, but this is not always possible.") 630 protected Annotation note; 631 632 /** 633 * The significant Conditions (or condition) that the family member had. This is 634 * a repeating section to allow a system to represent more than one condition 635 * per resource, though there is nothing stopping multiple resources - one per 636 * condition. 637 */ 638 @Child(name = "condition", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 639 @Description(shortDefinition = "Condition that the related person had", formalDefinition = "The significant Conditions (or condition) that the family member had. This is a repeating section to allow a system to represent more than one condition per resource, though there is nothing stopping multiple resources - one per condition.") 640 protected List<FamilyMemberHistoryConditionComponent> condition; 641 642 private static final long serialVersionUID = -1799103041L; 643 644 /* 645 * Constructor 646 */ 647 public FamilyMemberHistory() { 648 super(); 649 } 650 651 /* 652 * Constructor 653 */ 654 public FamilyMemberHistory(Reference patient, Enumeration<FamilyHistoryStatus> status, CodeableConcept relationship) { 655 super(); 656 this.patient = patient; 657 this.status = status; 658 this.relationship = relationship; 659 } 660 661 /** 662 * @return {@link #identifier} (This records identifiers associated with this 663 * family member history record that are defined by business processes 664 * and/ or used to refer to it when a direct URL reference to the 665 * resource itself is not appropriate (e.g. in CDA documents, or in 666 * written / printed documentation).) 667 */ 668 public List<Identifier> getIdentifier() { 669 if (this.identifier == null) 670 this.identifier = new ArrayList<Identifier>(); 671 return this.identifier; 672 } 673 674 public boolean hasIdentifier() { 675 if (this.identifier == null) 676 return false; 677 for (Identifier item : this.identifier) 678 if (!item.isEmpty()) 679 return true; 680 return false; 681 } 682 683 /** 684 * @return {@link #identifier} (This records identifiers associated with this 685 * family member history record that are defined by business processes 686 * and/ or used to refer to it when a direct URL reference to the 687 * resource itself is not appropriate (e.g. in CDA documents, or in 688 * written / printed documentation).) 689 */ 690 // syntactic sugar 691 public Identifier addIdentifier() { // 3 692 Identifier t = new Identifier(); 693 if (this.identifier == null) 694 this.identifier = new ArrayList<Identifier>(); 695 this.identifier.add(t); 696 return t; 697 } 698 699 // syntactic sugar 700 public FamilyMemberHistory addIdentifier(Identifier t) { // 3 701 if (t == null) 702 return this; 703 if (this.identifier == null) 704 this.identifier = new ArrayList<Identifier>(); 705 this.identifier.add(t); 706 return this; 707 } 708 709 /** 710 * @return {@link #patient} (The person who this history concerns.) 711 */ 712 public Reference getPatient() { 713 if (this.patient == null) 714 if (Configuration.errorOnAutoCreate()) 715 throw new Error("Attempt to auto-create FamilyMemberHistory.patient"); 716 else if (Configuration.doAutoCreate()) 717 this.patient = new Reference(); // cc 718 return this.patient; 719 } 720 721 public boolean hasPatient() { 722 return this.patient != null && !this.patient.isEmpty(); 723 } 724 725 /** 726 * @param value {@link #patient} (The person who this history concerns.) 727 */ 728 public FamilyMemberHistory setPatient(Reference value) { 729 this.patient = value; 730 return this; 731 } 732 733 /** 734 * @return {@link #patient} The actual object that is the target of the 735 * reference. The reference library doesn't populate this, but you can 736 * use it to hold the resource if you resolve it. (The person who this 737 * history concerns.) 738 */ 739 public Patient getPatientTarget() { 740 if (this.patientTarget == null) 741 if (Configuration.errorOnAutoCreate()) 742 throw new Error("Attempt to auto-create FamilyMemberHistory.patient"); 743 else if (Configuration.doAutoCreate()) 744 this.patientTarget = new Patient(); // aa 745 return this.patientTarget; 746 } 747 748 /** 749 * @param value {@link #patient} The actual object that is the target of the 750 * reference. The reference library doesn't use these, but you can 751 * use it to hold the resource if you resolve it. (The person who 752 * this history concerns.) 753 */ 754 public FamilyMemberHistory setPatientTarget(Patient value) { 755 this.patientTarget = value; 756 return this; 757 } 758 759 /** 760 * @return {@link #date} (The date (and possibly time) when the family member 761 * history was taken.). This is the underlying object with id, value and 762 * extensions. The accessor "getDate" gives direct access to the value 763 */ 764 public DateTimeType getDateElement() { 765 if (this.date == null) 766 if (Configuration.errorOnAutoCreate()) 767 throw new Error("Attempt to auto-create FamilyMemberHistory.date"); 768 else if (Configuration.doAutoCreate()) 769 this.date = new DateTimeType(); // bb 770 return this.date; 771 } 772 773 public boolean hasDateElement() { 774 return this.date != null && !this.date.isEmpty(); 775 } 776 777 public boolean hasDate() { 778 return this.date != null && !this.date.isEmpty(); 779 } 780 781 /** 782 * @param value {@link #date} (The date (and possibly time) when the family 783 * member history was taken.). This is the underlying object with 784 * id, value and extensions. The accessor "getDate" gives direct 785 * access to the value 786 */ 787 public FamilyMemberHistory setDateElement(DateTimeType value) { 788 this.date = value; 789 return this; 790 } 791 792 /** 793 * @return The date (and possibly time) when the family member history was 794 * taken. 795 */ 796 public Date getDate() { 797 return this.date == null ? null : this.date.getValue(); 798 } 799 800 /** 801 * @param value The date (and possibly time) when the family member history was 802 * taken. 803 */ 804 public FamilyMemberHistory setDate(Date value) { 805 if (value == null) 806 this.date = null; 807 else { 808 if (this.date == null) 809 this.date = new DateTimeType(); 810 this.date.setValue(value); 811 } 812 return this; 813 } 814 815 /** 816 * @return {@link #status} (A code specifying a state of a Family Member History 817 * record.). This is the underlying object with id, value and 818 * extensions. The accessor "getStatus" gives direct access to the value 819 */ 820 public Enumeration<FamilyHistoryStatus> getStatusElement() { 821 if (this.status == null) 822 if (Configuration.errorOnAutoCreate()) 823 throw new Error("Attempt to auto-create FamilyMemberHistory.status"); 824 else if (Configuration.doAutoCreate()) 825 this.status = new Enumeration<FamilyHistoryStatus>(new FamilyHistoryStatusEnumFactory()); // bb 826 return this.status; 827 } 828 829 public boolean hasStatusElement() { 830 return this.status != null && !this.status.isEmpty(); 831 } 832 833 public boolean hasStatus() { 834 return this.status != null && !this.status.isEmpty(); 835 } 836 837 /** 838 * @param value {@link #status} (A code specifying a state of a Family Member 839 * History record.). This is the underlying object with id, value 840 * and extensions. The accessor "getStatus" gives direct access to 841 * the value 842 */ 843 public FamilyMemberHistory setStatusElement(Enumeration<FamilyHistoryStatus> value) { 844 this.status = value; 845 return this; 846 } 847 848 /** 849 * @return A code specifying a state of a Family Member History record. 850 */ 851 public FamilyHistoryStatus getStatus() { 852 return this.status == null ? null : this.status.getValue(); 853 } 854 855 /** 856 * @param value A code specifying a state of a Family Member History record. 857 */ 858 public FamilyMemberHistory setStatus(FamilyHistoryStatus value) { 859 if (this.status == null) 860 this.status = new Enumeration<FamilyHistoryStatus>(new FamilyHistoryStatusEnumFactory()); 861 this.status.setValue(value); 862 return this; 863 } 864 865 /** 866 * @return {@link #name} (This will either be a name or a description; e.g. 867 * "Aunt Susan", "my cousin with the red hair".). This is the underlying 868 * object with id, value and extensions. The accessor "getName" gives 869 * direct access to the value 870 */ 871 public StringType getNameElement() { 872 if (this.name == null) 873 if (Configuration.errorOnAutoCreate()) 874 throw new Error("Attempt to auto-create FamilyMemberHistory.name"); 875 else if (Configuration.doAutoCreate()) 876 this.name = new StringType(); // bb 877 return this.name; 878 } 879 880 public boolean hasNameElement() { 881 return this.name != null && !this.name.isEmpty(); 882 } 883 884 public boolean hasName() { 885 return this.name != null && !this.name.isEmpty(); 886 } 887 888 /** 889 * @param value {@link #name} (This will either be a name or a description; e.g. 890 * "Aunt Susan", "my cousin with the red hair".). This is the 891 * underlying object with id, value and extensions. The accessor 892 * "getName" gives direct access to the value 893 */ 894 public FamilyMemberHistory setNameElement(StringType value) { 895 this.name = value; 896 return this; 897 } 898 899 /** 900 * @return This will either be a name or a description; e.g. "Aunt Susan", "my 901 * cousin with the red hair". 902 */ 903 public String getName() { 904 return this.name == null ? null : this.name.getValue(); 905 } 906 907 /** 908 * @param value This will either be a name or a description; e.g. "Aunt Susan", 909 * "my cousin with the red hair". 910 */ 911 public FamilyMemberHistory setName(String value) { 912 if (Utilities.noString(value)) 913 this.name = null; 914 else { 915 if (this.name == null) 916 this.name = new StringType(); 917 this.name.setValue(value); 918 } 919 return this; 920 } 921 922 /** 923 * @return {@link #relationship} (The type of relationship this person has to 924 * the patient (father, mother, brother etc.).) 925 */ 926 public CodeableConcept getRelationship() { 927 if (this.relationship == null) 928 if (Configuration.errorOnAutoCreate()) 929 throw new Error("Attempt to auto-create FamilyMemberHistory.relationship"); 930 else if (Configuration.doAutoCreate()) 931 this.relationship = new CodeableConcept(); // cc 932 return this.relationship; 933 } 934 935 public boolean hasRelationship() { 936 return this.relationship != null && !this.relationship.isEmpty(); 937 } 938 939 /** 940 * @param value {@link #relationship} (The type of relationship this person has 941 * to the patient (father, mother, brother etc.).) 942 */ 943 public FamilyMemberHistory setRelationship(CodeableConcept value) { 944 this.relationship = value; 945 return this; 946 } 947 948 /** 949 * @return {@link #gender} (Administrative Gender - the gender that the relative 950 * is considered to have for administration and record keeping 951 * purposes.). This is the underlying object with id, value and 952 * extensions. The accessor "getGender" gives direct access to the value 953 */ 954 public Enumeration<AdministrativeGender> getGenderElement() { 955 if (this.gender == null) 956 if (Configuration.errorOnAutoCreate()) 957 throw new Error("Attempt to auto-create FamilyMemberHistory.gender"); 958 else if (Configuration.doAutoCreate()) 959 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 960 return this.gender; 961 } 962 963 public boolean hasGenderElement() { 964 return this.gender != null && !this.gender.isEmpty(); 965 } 966 967 public boolean hasGender() { 968 return this.gender != null && !this.gender.isEmpty(); 969 } 970 971 /** 972 * @param value {@link #gender} (Administrative Gender - the gender that the 973 * relative is considered to have for administration and record 974 * keeping purposes.). This is the underlying object with id, value 975 * and extensions. The accessor "getGender" gives direct access to 976 * the value 977 */ 978 public FamilyMemberHistory setGenderElement(Enumeration<AdministrativeGender> value) { 979 this.gender = value; 980 return this; 981 } 982 983 /** 984 * @return Administrative Gender - the gender that the relative is considered to 985 * have for administration and record keeping purposes. 986 */ 987 public AdministrativeGender getGender() { 988 return this.gender == null ? null : this.gender.getValue(); 989 } 990 991 /** 992 * @param value Administrative Gender - the gender that the relative is 993 * considered to have for administration and record keeping 994 * purposes. 995 */ 996 public FamilyMemberHistory setGender(AdministrativeGender value) { 997 if (value == null) 998 this.gender = null; 999 else { 1000 if (this.gender == null) 1001 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 1002 this.gender.setValue(value); 1003 } 1004 return this; 1005 } 1006 1007 /** 1008 * @return {@link #born} (The actual or approximate date of birth of the 1009 * relative.) 1010 */ 1011 public Type getBorn() { 1012 return this.born; 1013 } 1014 1015 /** 1016 * @return {@link #born} (The actual or approximate date of birth of the 1017 * relative.) 1018 */ 1019 public Period getBornPeriod() throws FHIRException { 1020 if (!(this.born instanceof Period)) 1021 throw new FHIRException( 1022 "Type mismatch: the type Period was expected, but " + this.born.getClass().getName() + " was encountered"); 1023 return (Period) this.born; 1024 } 1025 1026 public boolean hasBornPeriod() { 1027 return this.born instanceof Period; 1028 } 1029 1030 /** 1031 * @return {@link #born} (The actual or approximate date of birth of the 1032 * relative.) 1033 */ 1034 public DateType getBornDateType() throws FHIRException { 1035 if (!(this.born instanceof DateType)) 1036 throw new FHIRException( 1037 "Type mismatch: the type DateType was expected, but " + this.born.getClass().getName() + " was encountered"); 1038 return (DateType) this.born; 1039 } 1040 1041 public boolean hasBornDateType() { 1042 return this.born instanceof DateType; 1043 } 1044 1045 /** 1046 * @return {@link #born} (The actual or approximate date of birth of the 1047 * relative.) 1048 */ 1049 public StringType getBornStringType() throws FHIRException { 1050 if (!(this.born instanceof StringType)) 1051 throw new FHIRException("Type mismatch: the type StringType was expected, but " + this.born.getClass().getName() 1052 + " was encountered"); 1053 return (StringType) this.born; 1054 } 1055 1056 public boolean hasBornStringType() { 1057 return this.born instanceof StringType; 1058 } 1059 1060 public boolean hasBorn() { 1061 return this.born != null && !this.born.isEmpty(); 1062 } 1063 1064 /** 1065 * @param value {@link #born} (The actual or approximate date of birth of the 1066 * relative.) 1067 */ 1068 public FamilyMemberHistory setBorn(Type value) { 1069 this.born = value; 1070 return this; 1071 } 1072 1073 /** 1074 * @return {@link #age} (The actual or approximate age of the relative at the 1075 * time the family member history is recorded.) 1076 */ 1077 public Type getAge() { 1078 return this.age; 1079 } 1080 1081 /** 1082 * @return {@link #age} (The actual or approximate age of the relative at the 1083 * time the family member history is recorded.) 1084 */ 1085 public Age getAgeAge() throws FHIRException { 1086 if (!(this.age instanceof Age)) 1087 throw new FHIRException( 1088 "Type mismatch: the type Age was expected, but " + this.age.getClass().getName() + " was encountered"); 1089 return (Age) this.age; 1090 } 1091 1092 public boolean hasAgeAge() { 1093 return this.age instanceof Age; 1094 } 1095 1096 /** 1097 * @return {@link #age} (The actual or approximate age of the relative at the 1098 * time the family member history is recorded.) 1099 */ 1100 public Range getAgeRange() throws FHIRException { 1101 if (!(this.age instanceof Range)) 1102 throw new FHIRException( 1103 "Type mismatch: the type Range was expected, but " + this.age.getClass().getName() + " was encountered"); 1104 return (Range) this.age; 1105 } 1106 1107 public boolean hasAgeRange() { 1108 return this.age instanceof Range; 1109 } 1110 1111 /** 1112 * @return {@link #age} (The actual or approximate age of the relative at the 1113 * time the family member history is recorded.) 1114 */ 1115 public StringType getAgeStringType() throws FHIRException { 1116 if (!(this.age instanceof StringType)) 1117 throw new FHIRException( 1118 "Type mismatch: the type StringType was expected, but " + this.age.getClass().getName() + " was encountered"); 1119 return (StringType) this.age; 1120 } 1121 1122 public boolean hasAgeStringType() { 1123 return this.age instanceof StringType; 1124 } 1125 1126 public boolean hasAge() { 1127 return this.age != null && !this.age.isEmpty(); 1128 } 1129 1130 /** 1131 * @param value {@link #age} (The actual or approximate age of the relative at 1132 * the time the family member history is recorded.) 1133 */ 1134 public FamilyMemberHistory setAge(Type value) { 1135 this.age = value; 1136 return this; 1137 } 1138 1139 /** 1140 * @return {@link #deceased} (Deceased flag or the actual or approximate age of 1141 * the relative at the time of death for the family member history 1142 * record.) 1143 */ 1144 public Type getDeceased() { 1145 return this.deceased; 1146 } 1147 1148 /** 1149 * @return {@link #deceased} (Deceased flag or the actual or approximate age of 1150 * the relative at the time of death for the family member history 1151 * record.) 1152 */ 1153 public BooleanType getDeceasedBooleanType() throws FHIRException { 1154 if (!(this.deceased instanceof BooleanType)) 1155 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1156 + this.deceased.getClass().getName() + " was encountered"); 1157 return (BooleanType) this.deceased; 1158 } 1159 1160 public boolean hasDeceasedBooleanType() { 1161 return this.deceased instanceof BooleanType; 1162 } 1163 1164 /** 1165 * @return {@link #deceased} (Deceased flag or the actual or approximate age of 1166 * the relative at the time of death for the family member history 1167 * record.) 1168 */ 1169 public Age getDeceasedAge() throws FHIRException { 1170 if (!(this.deceased instanceof Age)) 1171 throw new FHIRException( 1172 "Type mismatch: the type Age was expected, but " + this.deceased.getClass().getName() + " was encountered"); 1173 return (Age) this.deceased; 1174 } 1175 1176 public boolean hasDeceasedAge() { 1177 return this.deceased instanceof Age; 1178 } 1179 1180 /** 1181 * @return {@link #deceased} (Deceased flag or the actual or approximate age of 1182 * the relative at the time of death for the family member history 1183 * record.) 1184 */ 1185 public Range getDeceasedRange() throws FHIRException { 1186 if (!(this.deceased instanceof Range)) 1187 throw new FHIRException( 1188 "Type mismatch: the type Range was expected, but " + this.deceased.getClass().getName() + " was encountered"); 1189 return (Range) this.deceased; 1190 } 1191 1192 public boolean hasDeceasedRange() { 1193 return this.deceased instanceof Range; 1194 } 1195 1196 /** 1197 * @return {@link #deceased} (Deceased flag or the actual or approximate age of 1198 * the relative at the time of death for the family member history 1199 * record.) 1200 */ 1201 public DateType getDeceasedDateType() throws FHIRException { 1202 if (!(this.deceased instanceof DateType)) 1203 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.deceased.getClass().getName() 1204 + " was encountered"); 1205 return (DateType) this.deceased; 1206 } 1207 1208 public boolean hasDeceasedDateType() { 1209 return this.deceased instanceof DateType; 1210 } 1211 1212 /** 1213 * @return {@link #deceased} (Deceased flag or the actual or approximate age of 1214 * the relative at the time of death for the family member history 1215 * record.) 1216 */ 1217 public StringType getDeceasedStringType() throws FHIRException { 1218 if (!(this.deceased instanceof StringType)) 1219 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1220 + this.deceased.getClass().getName() + " was encountered"); 1221 return (StringType) this.deceased; 1222 } 1223 1224 public boolean hasDeceasedStringType() { 1225 return this.deceased instanceof StringType; 1226 } 1227 1228 public boolean hasDeceased() { 1229 return this.deceased != null && !this.deceased.isEmpty(); 1230 } 1231 1232 /** 1233 * @param value {@link #deceased} (Deceased flag or the actual or approximate 1234 * age of the relative at the time of death for the family member 1235 * history record.) 1236 */ 1237 public FamilyMemberHistory setDeceased(Type value) { 1238 this.deceased = value; 1239 return this; 1240 } 1241 1242 /** 1243 * @return {@link #note} (This property allows a non condition-specific note to 1244 * the made about the related person. Ideally, the note would be in the 1245 * condition property, but this is not always possible.) 1246 */ 1247 public Annotation getNote() { 1248 if (this.note == null) 1249 if (Configuration.errorOnAutoCreate()) 1250 throw new Error("Attempt to auto-create FamilyMemberHistory.note"); 1251 else if (Configuration.doAutoCreate()) 1252 this.note = new Annotation(); // cc 1253 return this.note; 1254 } 1255 1256 public boolean hasNote() { 1257 return this.note != null && !this.note.isEmpty(); 1258 } 1259 1260 /** 1261 * @param value {@link #note} (This property allows a non condition-specific 1262 * note to the made about the related person. Ideally, the note 1263 * would be in the condition property, but this is not always 1264 * possible.) 1265 */ 1266 public FamilyMemberHistory setNote(Annotation value) { 1267 this.note = value; 1268 return this; 1269 } 1270 1271 /** 1272 * @return {@link #condition} (The significant Conditions (or condition) that 1273 * the family member had. This is a repeating section to allow a system 1274 * to represent more than one condition per resource, though there is 1275 * nothing stopping multiple resources - one per condition.) 1276 */ 1277 public List<FamilyMemberHistoryConditionComponent> getCondition() { 1278 if (this.condition == null) 1279 this.condition = new ArrayList<FamilyMemberHistoryConditionComponent>(); 1280 return this.condition; 1281 } 1282 1283 public boolean hasCondition() { 1284 if (this.condition == null) 1285 return false; 1286 for (FamilyMemberHistoryConditionComponent item : this.condition) 1287 if (!item.isEmpty()) 1288 return true; 1289 return false; 1290 } 1291 1292 /** 1293 * @return {@link #condition} (The significant Conditions (or condition) that 1294 * the family member had. This is a repeating section to allow a system 1295 * to represent more than one condition per resource, though there is 1296 * nothing stopping multiple resources - one per condition.) 1297 */ 1298 // syntactic sugar 1299 public FamilyMemberHistoryConditionComponent addCondition() { // 3 1300 FamilyMemberHistoryConditionComponent t = new FamilyMemberHistoryConditionComponent(); 1301 if (this.condition == null) 1302 this.condition = new ArrayList<FamilyMemberHistoryConditionComponent>(); 1303 this.condition.add(t); 1304 return t; 1305 } 1306 1307 // syntactic sugar 1308 public FamilyMemberHistory addCondition(FamilyMemberHistoryConditionComponent t) { // 3 1309 if (t == null) 1310 return this; 1311 if (this.condition == null) 1312 this.condition = new ArrayList<FamilyMemberHistoryConditionComponent>(); 1313 this.condition.add(t); 1314 return this; 1315 } 1316 1317 protected void listChildren(List<Property> childrenList) { 1318 super.listChildren(childrenList); 1319 childrenList.add(new Property("identifier", "Identifier", 1320 "This records identifiers associated with this family member history record that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 1321 0, java.lang.Integer.MAX_VALUE, identifier)); 1322 childrenList.add(new Property("patient", "Reference(Patient)", "The person who this history concerns.", 0, 1323 java.lang.Integer.MAX_VALUE, patient)); 1324 childrenList 1325 .add(new Property("date", "dateTime", "The date (and possibly time) when the family member history was taken.", 1326 0, java.lang.Integer.MAX_VALUE, date)); 1327 childrenList.add(new Property("status", "code", "A code specifying a state of a Family Member History record.", 0, 1328 java.lang.Integer.MAX_VALUE, status)); 1329 childrenList.add(new Property("name", "string", 1330 "This will either be a name or a description; e.g. \"Aunt Susan\", \"my cousin with the red hair\".", 0, 1331 java.lang.Integer.MAX_VALUE, name)); 1332 childrenList.add(new Property("relationship", "CodeableConcept", 1333 "The type of relationship this person has to the patient (father, mother, brother etc.).", 0, 1334 java.lang.Integer.MAX_VALUE, relationship)); 1335 childrenList.add(new Property("gender", "code", 1336 "Administrative Gender - the gender that the relative is considered to have for administration and record keeping purposes.", 1337 0, java.lang.Integer.MAX_VALUE, gender)); 1338 childrenList.add(new Property("born[x]", "Period|date|string", 1339 "The actual or approximate date of birth of the relative.", 0, java.lang.Integer.MAX_VALUE, born)); 1340 childrenList.add(new Property("age[x]", "Age|Range|string", 1341 "The actual or approximate age of the relative at the time the family member history is recorded.", 0, 1342 java.lang.Integer.MAX_VALUE, age)); 1343 childrenList.add(new Property("deceased[x]", "boolean|Age|Range|date|string", 1344 "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 1345 0, java.lang.Integer.MAX_VALUE, deceased)); 1346 childrenList.add(new Property("note", "Annotation", 1347 "This property allows a non condition-specific note to the made about the related person. Ideally, the note would be in the condition property, but this is not always possible.", 1348 0, java.lang.Integer.MAX_VALUE, note)); 1349 childrenList.add(new Property("condition", "", 1350 "The significant Conditions (or condition) that the family member had. This is a repeating section to allow a system to represent more than one condition per resource, though there is nothing stopping multiple resources - one per condition.", 1351 0, java.lang.Integer.MAX_VALUE, condition)); 1352 } 1353 1354 @Override 1355 public void setProperty(String name, Base value) throws FHIRException { 1356 if (name.equals("identifier")) 1357 this.getIdentifier().add(castToIdentifier(value)); 1358 else if (name.equals("patient")) 1359 this.patient = castToReference(value); // Reference 1360 else if (name.equals("date")) 1361 this.date = castToDateTime(value); // DateTimeType 1362 else if (name.equals("status")) 1363 this.status = new FamilyHistoryStatusEnumFactory().fromType(value); // Enumeration<FamilyHistoryStatus> 1364 else if (name.equals("name")) 1365 this.name = castToString(value); // StringType 1366 else if (name.equals("relationship")) 1367 this.relationship = castToCodeableConcept(value); // CodeableConcept 1368 else if (name.equals("gender")) 1369 this.gender = new AdministrativeGenderEnumFactory().fromType(value); // Enumeration<AdministrativeGender> 1370 else if (name.equals("born[x]")) 1371 this.born = (Type) value; // Type 1372 else if (name.equals("age[x]")) 1373 this.age = (Type) value; // Type 1374 else if (name.equals("deceased[x]")) 1375 this.deceased = (Type) value; // Type 1376 else if (name.equals("note")) 1377 this.note = castToAnnotation(value); // Annotation 1378 else if (name.equals("condition")) 1379 this.getCondition().add((FamilyMemberHistoryConditionComponent) value); 1380 else 1381 super.setProperty(name, value); 1382 } 1383 1384 @Override 1385 public Base addChild(String name) throws FHIRException { 1386 if (name.equals("identifier")) { 1387 return addIdentifier(); 1388 } else if (name.equals("patient")) { 1389 this.patient = new Reference(); 1390 return this.patient; 1391 } else if (name.equals("date")) { 1392 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.date"); 1393 } else if (name.equals("status")) { 1394 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.status"); 1395 } else if (name.equals("name")) { 1396 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.name"); 1397 } else if (name.equals("relationship")) { 1398 this.relationship = new CodeableConcept(); 1399 return this.relationship; 1400 } else if (name.equals("gender")) { 1401 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.gender"); 1402 } else if (name.equals("bornPeriod")) { 1403 this.born = new Period(); 1404 return this.born; 1405 } else if (name.equals("bornDate")) { 1406 this.born = new DateType(); 1407 return this.born; 1408 } else if (name.equals("bornString")) { 1409 this.born = new StringType(); 1410 return this.born; 1411 } else if (name.equals("ageAge")) { 1412 this.age = new Age(); 1413 return this.age; 1414 } else if (name.equals("ageRange")) { 1415 this.age = new Range(); 1416 return this.age; 1417 } else if (name.equals("ageString")) { 1418 this.age = new StringType(); 1419 return this.age; 1420 } else if (name.equals("deceasedBoolean")) { 1421 this.deceased = new BooleanType(); 1422 return this.deceased; 1423 } else if (name.equals("deceasedAge")) { 1424 this.deceased = new Age(); 1425 return this.deceased; 1426 } else if (name.equals("deceasedRange")) { 1427 this.deceased = new Range(); 1428 return this.deceased; 1429 } else if (name.equals("deceasedDate")) { 1430 this.deceased = new DateType(); 1431 return this.deceased; 1432 } else if (name.equals("deceasedString")) { 1433 this.deceased = new StringType(); 1434 return this.deceased; 1435 } else if (name.equals("note")) { 1436 this.note = new Annotation(); 1437 return this.note; 1438 } else if (name.equals("condition")) { 1439 return addCondition(); 1440 } else 1441 return super.addChild(name); 1442 } 1443 1444 public String fhirType() { 1445 return "FamilyMemberHistory"; 1446 1447 } 1448 1449 public FamilyMemberHistory copy() { 1450 FamilyMemberHistory dst = new FamilyMemberHistory(); 1451 copyValues(dst); 1452 if (identifier != null) { 1453 dst.identifier = new ArrayList<Identifier>(); 1454 for (Identifier i : identifier) 1455 dst.identifier.add(i.copy()); 1456 } 1457 ; 1458 dst.patient = patient == null ? null : patient.copy(); 1459 dst.date = date == null ? null : date.copy(); 1460 dst.status = status == null ? null : status.copy(); 1461 dst.name = name == null ? null : name.copy(); 1462 dst.relationship = relationship == null ? null : relationship.copy(); 1463 dst.gender = gender == null ? null : gender.copy(); 1464 dst.born = born == null ? null : born.copy(); 1465 dst.age = age == null ? null : age.copy(); 1466 dst.deceased = deceased == null ? null : deceased.copy(); 1467 dst.note = note == null ? null : note.copy(); 1468 if (condition != null) { 1469 dst.condition = new ArrayList<FamilyMemberHistoryConditionComponent>(); 1470 for (FamilyMemberHistoryConditionComponent i : condition) 1471 dst.condition.add(i.copy()); 1472 } 1473 ; 1474 return dst; 1475 } 1476 1477 protected FamilyMemberHistory typedCopy() { 1478 return copy(); 1479 } 1480 1481 @Override 1482 public boolean equalsDeep(Base other) { 1483 if (!super.equalsDeep(other)) 1484 return false; 1485 if (!(other instanceof FamilyMemberHistory)) 1486 return false; 1487 FamilyMemberHistory o = (FamilyMemberHistory) other; 1488 return compareDeep(identifier, o.identifier, true) && compareDeep(patient, o.patient, true) 1489 && compareDeep(date, o.date, true) && compareDeep(status, o.status, true) && compareDeep(name, o.name, true) 1490 && compareDeep(relationship, o.relationship, true) && compareDeep(gender, o.gender, true) 1491 && compareDeep(born, o.born, true) && compareDeep(age, o.age, true) && compareDeep(deceased, o.deceased, true) 1492 && compareDeep(note, o.note, true) && compareDeep(condition, o.condition, true); 1493 } 1494 1495 @Override 1496 public boolean equalsShallow(Base other) { 1497 if (!super.equalsShallow(other)) 1498 return false; 1499 if (!(other instanceof FamilyMemberHistory)) 1500 return false; 1501 FamilyMemberHistory o = (FamilyMemberHistory) other; 1502 return compareValues(date, o.date, true) && compareValues(status, o.status, true) 1503 && compareValues(name, o.name, true) && compareValues(gender, o.gender, true); 1504 } 1505 1506 public boolean isEmpty() { 1507 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (patient == null || patient.isEmpty()) 1508 && (date == null || date.isEmpty()) && (status == null || status.isEmpty()) && (name == null || name.isEmpty()) 1509 && (relationship == null || relationship.isEmpty()) && (gender == null || gender.isEmpty()) 1510 && (born == null || born.isEmpty()) && (age == null || age.isEmpty()) 1511 && (deceased == null || deceased.isEmpty()) && (note == null || note.isEmpty()) 1512 && (condition == null || condition.isEmpty()); 1513 } 1514 1515 @Override 1516 public ResourceType getResourceType() { 1517 return ResourceType.FamilyMemberHistory; 1518 } 1519 1520 @SearchParamDefinition(name = "date", path = "FamilyMemberHistory.date", description = "When history was captured/updated", type = "date") 1521 public static final String SP_DATE = "date"; 1522 @SearchParamDefinition(name = "identifier", path = "FamilyMemberHistory.identifier", description = "A search by a record identifier", type = "token") 1523 public static final String SP_IDENTIFIER = "identifier"; 1524 @SearchParamDefinition(name = "code", path = "FamilyMemberHistory.condition.code", description = "A search by a condition code", type = "token") 1525 public static final String SP_CODE = "code"; 1526 @SearchParamDefinition(name = "gender", path = "FamilyMemberHistory.gender", description = "A search by a gender code of a family member", type = "token") 1527 public static final String SP_GENDER = "gender"; 1528 @SearchParamDefinition(name = "patient", path = "FamilyMemberHistory.patient", description = "The identity of a subject to list family member history items for", type = "reference") 1529 public static final String SP_PATIENT = "patient"; 1530 @SearchParamDefinition(name = "relationship", path = "FamilyMemberHistory.relationship", description = "A search by a relationship type", type = "token") 1531 public static final String SP_RELATIONSHIP = "relationship"; 1532 1533}