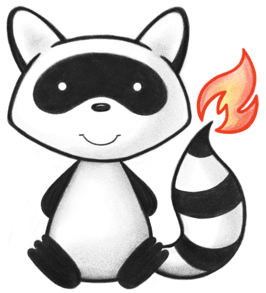
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Child; 037import ca.uhn.fhir.model.api.annotation.Description; 038import ca.uhn.fhir.model.api.annotation.ResourceDef; 039import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 040import org.hl7.fhir.exceptions.FHIRException; 041 042/** 043 * Prospective warnings of potential issues when providing care to the patient. 044 */ 045@ResourceDef(name = "Flag", profile = "http://hl7.org/fhir/Profile/Flag") 046public class Flag extends DomainResource { 047 048 public enum FlagStatus { 049 /** 050 * A current flag that should be displayed to a user. A system may use the 051 * category to determine which roles should view the flag. 052 */ 053 ACTIVE, 054 /** 055 * The flag does not need to be displayed any more. 056 */ 057 INACTIVE, 058 /** 059 * The flag was added in error, and should no longer be displayed. 060 */ 061 ENTEREDINERROR, 062 /** 063 * added to help the parsers 064 */ 065 NULL; 066 067 public static FlagStatus fromCode(String codeString) throws FHIRException { 068 if (codeString == null || "".equals(codeString)) 069 return null; 070 if ("active".equals(codeString)) 071 return ACTIVE; 072 if ("inactive".equals(codeString)) 073 return INACTIVE; 074 if ("entered-in-error".equals(codeString)) 075 return ENTEREDINERROR; 076 throw new FHIRException("Unknown FlagStatus code '" + codeString + "'"); 077 } 078 079 public String toCode() { 080 switch (this) { 081 case ACTIVE: 082 return "active"; 083 case INACTIVE: 084 return "inactive"; 085 case ENTEREDINERROR: 086 return "entered-in-error"; 087 case NULL: 088 return null; 089 default: 090 return "?"; 091 } 092 } 093 094 public String getSystem() { 095 switch (this) { 096 case ACTIVE: 097 return "http://hl7.org/fhir/flag-status"; 098 case INACTIVE: 099 return "http://hl7.org/fhir/flag-status"; 100 case ENTEREDINERROR: 101 return "http://hl7.org/fhir/flag-status"; 102 case NULL: 103 return null; 104 default: 105 return "?"; 106 } 107 } 108 109 public String getDefinition() { 110 switch (this) { 111 case ACTIVE: 112 return "A current flag that should be displayed to a user. A system may use the category to determine which roles should view the flag."; 113 case INACTIVE: 114 return "The flag does not need to be displayed any more."; 115 case ENTEREDINERROR: 116 return "The flag was added in error, and should no longer be displayed."; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getDisplay() { 125 switch (this) { 126 case ACTIVE: 127 return "Active"; 128 case INACTIVE: 129 return "Inactive"; 130 case ENTEREDINERROR: 131 return "Entered in Error"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 } 139 140 public static class FlagStatusEnumFactory implements EnumFactory<FlagStatus> { 141 public FlagStatus fromCode(String codeString) throws IllegalArgumentException { 142 if (codeString == null || "".equals(codeString)) 143 if (codeString == null || "".equals(codeString)) 144 return null; 145 if ("active".equals(codeString)) 146 return FlagStatus.ACTIVE; 147 if ("inactive".equals(codeString)) 148 return FlagStatus.INACTIVE; 149 if ("entered-in-error".equals(codeString)) 150 return FlagStatus.ENTEREDINERROR; 151 throw new IllegalArgumentException("Unknown FlagStatus code '" + codeString + "'"); 152 } 153 154 public Enumeration<FlagStatus> fromType(Base code) throws FHIRException { 155 if (code == null || code.isEmpty()) 156 return null; 157 String codeString = ((PrimitiveType) code).asStringValue(); 158 if (codeString == null || "".equals(codeString)) 159 return null; 160 if ("active".equals(codeString)) 161 return new Enumeration<FlagStatus>(this, FlagStatus.ACTIVE); 162 if ("inactive".equals(codeString)) 163 return new Enumeration<FlagStatus>(this, FlagStatus.INACTIVE); 164 if ("entered-in-error".equals(codeString)) 165 return new Enumeration<FlagStatus>(this, FlagStatus.ENTEREDINERROR); 166 throw new FHIRException("Unknown FlagStatus code '" + codeString + "'"); 167 } 168 169 public String toCode(FlagStatus code) 170 { 171 if (code == FlagStatus.NULL) 172 return null; 173 if (code == FlagStatus.ACTIVE) 174 return "active"; 175 if (code == FlagStatus.INACTIVE) 176 return "inactive"; 177 if (code == FlagStatus.ENTEREDINERROR) 178 return "entered-in-error"; 179 return "?"; 180 } 181 } 182 183 /** 184 * Identifier assigned to the flag for external use (outside the FHIR 185 * environment). 186 */ 187 @Child(name = "identifier", type = { 188 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 189 @Description(shortDefinition = "Business identifier", formalDefinition = "Identifier assigned to the flag for external use (outside the FHIR environment).") 190 protected List<Identifier> identifier; 191 192 /** 193 * Allows an flag to be divided into different categories like clinical, 194 * administrative etc. Intended to be used as a means of filtering which flags 195 * are displayed to particular user or in a given context. 196 */ 197 @Child(name = "category", type = { 198 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 199 @Description(shortDefinition = "Clinical, administrative, etc.", formalDefinition = "Allows an flag to be divided into different categories like clinical, administrative etc. Intended to be used as a means of filtering which flags are displayed to particular user or in a given context.") 200 protected CodeableConcept category; 201 202 /** 203 * Supports basic workflow. 204 */ 205 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 206 @Description(shortDefinition = "active | inactive | entered-in-error", formalDefinition = "Supports basic workflow.") 207 protected Enumeration<FlagStatus> status; 208 209 /** 210 * The period of time from the activation of the flag to inactivation of the 211 * flag. If the flag is active, the end of the period should be unspecified. 212 */ 213 @Child(name = "period", type = { Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 214 @Description(shortDefinition = "Time period when flag is active", formalDefinition = "The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified.") 215 protected Period period; 216 217 /** 218 * The patient, location, group , organization , or practitioner this is about 219 * record this flag is associated with. 220 */ 221 @Child(name = "subject", type = { Patient.class, Location.class, Group.class, Organization.class, 222 Practitioner.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 223 @Description(shortDefinition = "Who/What is flag about?", formalDefinition = "The patient, location, group , organization , or practitioner this is about record this flag is associated with.") 224 protected Reference subject; 225 226 /** 227 * The actual object that is the target of the reference (The patient, location, 228 * group , organization , or practitioner this is about record this flag is 229 * associated with.) 230 */ 231 protected Resource subjectTarget; 232 233 /** 234 * This alert is only relevant during the encounter. 235 */ 236 @Child(name = "encounter", type = { Encounter.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 237 @Description(shortDefinition = "Alert relevant during encounter", formalDefinition = "This alert is only relevant during the encounter.") 238 protected Reference encounter; 239 240 /** 241 * The actual object that is the target of the reference (This alert is only 242 * relevant during the encounter.) 243 */ 244 protected Encounter encounterTarget; 245 246 /** 247 * The person, organization or device that created the flag. 248 */ 249 @Child(name = "author", type = { Device.class, Organization.class, Patient.class, 250 Practitioner.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 251 @Description(shortDefinition = "Flag creator", formalDefinition = "The person, organization or device that created the flag.") 252 protected Reference author; 253 254 /** 255 * The actual object that is the target of the reference (The person, 256 * organization or device that created the flag.) 257 */ 258 protected Resource authorTarget; 259 260 /** 261 * The coded value or textual component of the flag to display to the user. 262 */ 263 @Child(name = "code", type = { CodeableConcept.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 264 @Description(shortDefinition = "Partially deaf, Requires easy open caps, No permanent address, etc.", formalDefinition = "The coded value or textual component of the flag to display to the user.") 265 protected CodeableConcept code; 266 267 private static final long serialVersionUID = 701147751L; 268 269 /* 270 * Constructor 271 */ 272 public Flag() { 273 super(); 274 } 275 276 /* 277 * Constructor 278 */ 279 public Flag(Enumeration<FlagStatus> status, Reference subject, CodeableConcept code) { 280 super(); 281 this.status = status; 282 this.subject = subject; 283 this.code = code; 284 } 285 286 /** 287 * @return {@link #identifier} (Identifier assigned to the flag for external use 288 * (outside the FHIR environment).) 289 */ 290 public List<Identifier> getIdentifier() { 291 if (this.identifier == null) 292 this.identifier = new ArrayList<Identifier>(); 293 return this.identifier; 294 } 295 296 public boolean hasIdentifier() { 297 if (this.identifier == null) 298 return false; 299 for (Identifier item : this.identifier) 300 if (!item.isEmpty()) 301 return true; 302 return false; 303 } 304 305 /** 306 * @return {@link #identifier} (Identifier assigned to the flag for external use 307 * (outside the FHIR environment).) 308 */ 309 // syntactic sugar 310 public Identifier addIdentifier() { // 3 311 Identifier t = new Identifier(); 312 if (this.identifier == null) 313 this.identifier = new ArrayList<Identifier>(); 314 this.identifier.add(t); 315 return t; 316 } 317 318 // syntactic sugar 319 public Flag addIdentifier(Identifier t) { // 3 320 if (t == null) 321 return this; 322 if (this.identifier == null) 323 this.identifier = new ArrayList<Identifier>(); 324 this.identifier.add(t); 325 return this; 326 } 327 328 /** 329 * @return {@link #category} (Allows an flag to be divided into different 330 * categories like clinical, administrative etc. Intended to be used as 331 * a means of filtering which flags are displayed to particular user or 332 * in a given context.) 333 */ 334 public CodeableConcept getCategory() { 335 if (this.category == null) 336 if (Configuration.errorOnAutoCreate()) 337 throw new Error("Attempt to auto-create Flag.category"); 338 else if (Configuration.doAutoCreate()) 339 this.category = new CodeableConcept(); // cc 340 return this.category; 341 } 342 343 public boolean hasCategory() { 344 return this.category != null && !this.category.isEmpty(); 345 } 346 347 /** 348 * @param value {@link #category} (Allows an flag to be divided into different 349 * categories like clinical, administrative etc. Intended to be 350 * used as a means of filtering which flags are displayed to 351 * particular user or in a given context.) 352 */ 353 public Flag setCategory(CodeableConcept value) { 354 this.category = value; 355 return this; 356 } 357 358 /** 359 * @return {@link #status} (Supports basic workflow.). This is the underlying 360 * object with id, value and extensions. The accessor "getStatus" gives 361 * direct access to the value 362 */ 363 public Enumeration<FlagStatus> getStatusElement() { 364 if (this.status == null) 365 if (Configuration.errorOnAutoCreate()) 366 throw new Error("Attempt to auto-create Flag.status"); 367 else if (Configuration.doAutoCreate()) 368 this.status = new Enumeration<FlagStatus>(new FlagStatusEnumFactory()); // bb 369 return this.status; 370 } 371 372 public boolean hasStatusElement() { 373 return this.status != null && !this.status.isEmpty(); 374 } 375 376 public boolean hasStatus() { 377 return this.status != null && !this.status.isEmpty(); 378 } 379 380 /** 381 * @param value {@link #status} (Supports basic workflow.). This is the 382 * underlying object with id, value and extensions. The accessor 383 * "getStatus" gives direct access to the value 384 */ 385 public Flag setStatusElement(Enumeration<FlagStatus> value) { 386 this.status = value; 387 return this; 388 } 389 390 /** 391 * @return Supports basic workflow. 392 */ 393 public FlagStatus getStatus() { 394 return this.status == null ? null : this.status.getValue(); 395 } 396 397 /** 398 * @param value Supports basic workflow. 399 */ 400 public Flag setStatus(FlagStatus value) { 401 if (this.status == null) 402 this.status = new Enumeration<FlagStatus>(new FlagStatusEnumFactory()); 403 this.status.setValue(value); 404 return this; 405 } 406 407 /** 408 * @return {@link #period} (The period of time from the activation of the flag 409 * to inactivation of the flag. If the flag is active, the end of the 410 * period should be unspecified.) 411 */ 412 public Period getPeriod() { 413 if (this.period == null) 414 if (Configuration.errorOnAutoCreate()) 415 throw new Error("Attempt to auto-create Flag.period"); 416 else if (Configuration.doAutoCreate()) 417 this.period = new Period(); // cc 418 return this.period; 419 } 420 421 public boolean hasPeriod() { 422 return this.period != null && !this.period.isEmpty(); 423 } 424 425 /** 426 * @param value {@link #period} (The period of time from the activation of the 427 * flag to inactivation of the flag. If the flag is active, the end 428 * of the period should be unspecified.) 429 */ 430 public Flag setPeriod(Period value) { 431 this.period = value; 432 return this; 433 } 434 435 /** 436 * @return {@link #subject} (The patient, location, group , organization , or 437 * practitioner this is about record this flag is associated with.) 438 */ 439 public Reference getSubject() { 440 if (this.subject == null) 441 if (Configuration.errorOnAutoCreate()) 442 throw new Error("Attempt to auto-create Flag.subject"); 443 else if (Configuration.doAutoCreate()) 444 this.subject = new Reference(); // cc 445 return this.subject; 446 } 447 448 public boolean hasSubject() { 449 return this.subject != null && !this.subject.isEmpty(); 450 } 451 452 /** 453 * @param value {@link #subject} (The patient, location, group , organization , 454 * or practitioner this is about record this flag is associated 455 * with.) 456 */ 457 public Flag setSubject(Reference value) { 458 this.subject = value; 459 return this; 460 } 461 462 /** 463 * @return {@link #subject} The actual object that is the target of the 464 * reference. The reference library doesn't populate this, but you can 465 * use it to hold the resource if you resolve it. (The patient, 466 * location, group , organization , or practitioner this is about record 467 * this flag is associated with.) 468 */ 469 public Resource getSubjectTarget() { 470 return this.subjectTarget; 471 } 472 473 /** 474 * @param value {@link #subject} The actual object that is the target of the 475 * reference. The reference library doesn't use these, but you can 476 * use it to hold the resource if you resolve it. (The patient, 477 * location, group , organization , or practitioner this is about 478 * record this flag is associated with.) 479 */ 480 public Flag setSubjectTarget(Resource value) { 481 this.subjectTarget = value; 482 return this; 483 } 484 485 /** 486 * @return {@link #encounter} (This alert is only relevant during the 487 * encounter.) 488 */ 489 public Reference getEncounter() { 490 if (this.encounter == null) 491 if (Configuration.errorOnAutoCreate()) 492 throw new Error("Attempt to auto-create Flag.encounter"); 493 else if (Configuration.doAutoCreate()) 494 this.encounter = new Reference(); // cc 495 return this.encounter; 496 } 497 498 public boolean hasEncounter() { 499 return this.encounter != null && !this.encounter.isEmpty(); 500 } 501 502 /** 503 * @param value {@link #encounter} (This alert is only relevant during the 504 * encounter.) 505 */ 506 public Flag setEncounter(Reference value) { 507 this.encounter = value; 508 return this; 509 } 510 511 /** 512 * @return {@link #encounter} The actual object that is the target of the 513 * reference. The reference library doesn't populate this, but you can 514 * use it to hold the resource if you resolve it. (This alert is only 515 * relevant during the encounter.) 516 */ 517 public Encounter getEncounterTarget() { 518 if (this.encounterTarget == null) 519 if (Configuration.errorOnAutoCreate()) 520 throw new Error("Attempt to auto-create Flag.encounter"); 521 else if (Configuration.doAutoCreate()) 522 this.encounterTarget = new Encounter(); // aa 523 return this.encounterTarget; 524 } 525 526 /** 527 * @param value {@link #encounter} The actual object that is the target of the 528 * reference. The reference library doesn't use these, but you can 529 * use it to hold the resource if you resolve it. (This alert is 530 * only relevant during the encounter.) 531 */ 532 public Flag setEncounterTarget(Encounter value) { 533 this.encounterTarget = value; 534 return this; 535 } 536 537 /** 538 * @return {@link #author} (The person, organization or device that created the 539 * flag.) 540 */ 541 public Reference getAuthor() { 542 if (this.author == null) 543 if (Configuration.errorOnAutoCreate()) 544 throw new Error("Attempt to auto-create Flag.author"); 545 else if (Configuration.doAutoCreate()) 546 this.author = new Reference(); // cc 547 return this.author; 548 } 549 550 public boolean hasAuthor() { 551 return this.author != null && !this.author.isEmpty(); 552 } 553 554 /** 555 * @param value {@link #author} (The person, organization or device that created 556 * the flag.) 557 */ 558 public Flag setAuthor(Reference value) { 559 this.author = value; 560 return this; 561 } 562 563 /** 564 * @return {@link #author} The actual object that is the target of the 565 * reference. The reference library doesn't populate this, but you can 566 * use it to hold the resource if you resolve it. (The person, 567 * organization or device that created the flag.) 568 */ 569 public Resource getAuthorTarget() { 570 return this.authorTarget; 571 } 572 573 /** 574 * @param value {@link #author} The actual object that is the target of the 575 * reference. The reference library doesn't use these, but you can 576 * use it to hold the resource if you resolve it. (The person, 577 * organization or device that created the flag.) 578 */ 579 public Flag setAuthorTarget(Resource value) { 580 this.authorTarget = value; 581 return this; 582 } 583 584 /** 585 * @return {@link #code} (The coded value or textual component of the flag to 586 * display to the user.) 587 */ 588 public CodeableConcept getCode() { 589 if (this.code == null) 590 if (Configuration.errorOnAutoCreate()) 591 throw new Error("Attempt to auto-create Flag.code"); 592 else if (Configuration.doAutoCreate()) 593 this.code = new CodeableConcept(); // cc 594 return this.code; 595 } 596 597 public boolean hasCode() { 598 return this.code != null && !this.code.isEmpty(); 599 } 600 601 /** 602 * @param value {@link #code} (The coded value or textual component of the flag 603 * to display to the user.) 604 */ 605 public Flag setCode(CodeableConcept value) { 606 this.code = value; 607 return this; 608 } 609 610 protected void listChildren(List<Property> childrenList) { 611 super.listChildren(childrenList); 612 childrenList.add(new Property("identifier", "Identifier", 613 "Identifier assigned to the flag for external use (outside the FHIR environment).", 0, 614 java.lang.Integer.MAX_VALUE, identifier)); 615 childrenList.add(new Property("category", "CodeableConcept", 616 "Allows an flag to be divided into different categories like clinical, administrative etc. Intended to be used as a means of filtering which flags are displayed to particular user or in a given context.", 617 0, java.lang.Integer.MAX_VALUE, category)); 618 childrenList 619 .add(new Property("status", "code", "Supports basic workflow.", 0, java.lang.Integer.MAX_VALUE, status)); 620 childrenList.add(new Property("period", "Period", 621 "The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified.", 622 0, java.lang.Integer.MAX_VALUE, period)); 623 childrenList.add(new Property("subject", "Reference(Patient|Location|Group|Organization|Practitioner)", 624 "The patient, location, group , organization , or practitioner this is about record this flag is associated with.", 625 0, java.lang.Integer.MAX_VALUE, subject)); 626 childrenList.add(new Property("encounter", "Reference(Encounter)", 627 "This alert is only relevant during the encounter.", 0, java.lang.Integer.MAX_VALUE, encounter)); 628 childrenList.add(new Property("author", "Reference(Device|Organization|Patient|Practitioner)", 629 "The person, organization or device that created the flag.", 0, java.lang.Integer.MAX_VALUE, author)); 630 childrenList.add(new Property("code", "CodeableConcept", 631 "The coded value or textual component of the flag to display to the user.", 0, java.lang.Integer.MAX_VALUE, 632 code)); 633 } 634 635 @Override 636 public void setProperty(String name, Base value) throws FHIRException { 637 if (name.equals("identifier")) 638 this.getIdentifier().add(castToIdentifier(value)); 639 else if (name.equals("category")) 640 this.category = castToCodeableConcept(value); // CodeableConcept 641 else if (name.equals("status")) 642 this.status = new FlagStatusEnumFactory().fromType(value); // Enumeration<FlagStatus> 643 else if (name.equals("period")) 644 this.period = castToPeriod(value); // Period 645 else if (name.equals("subject")) 646 this.subject = castToReference(value); // Reference 647 else if (name.equals("encounter")) 648 this.encounter = castToReference(value); // Reference 649 else if (name.equals("author")) 650 this.author = castToReference(value); // Reference 651 else if (name.equals("code")) 652 this.code = castToCodeableConcept(value); // CodeableConcept 653 else 654 super.setProperty(name, value); 655 } 656 657 @Override 658 public Base addChild(String name) throws FHIRException { 659 if (name.equals("identifier")) { 660 return addIdentifier(); 661 } else if (name.equals("category")) { 662 this.category = new CodeableConcept(); 663 return this.category; 664 } else if (name.equals("status")) { 665 throw new FHIRException("Cannot call addChild on a singleton property Flag.status"); 666 } else if (name.equals("period")) { 667 this.period = new Period(); 668 return this.period; 669 } else if (name.equals("subject")) { 670 this.subject = new Reference(); 671 return this.subject; 672 } else if (name.equals("encounter")) { 673 this.encounter = new Reference(); 674 return this.encounter; 675 } else if (name.equals("author")) { 676 this.author = new Reference(); 677 return this.author; 678 } else if (name.equals("code")) { 679 this.code = new CodeableConcept(); 680 return this.code; 681 } else 682 return super.addChild(name); 683 } 684 685 public String fhirType() { 686 return "Flag"; 687 688 } 689 690 public Flag copy() { 691 Flag dst = new Flag(); 692 copyValues(dst); 693 if (identifier != null) { 694 dst.identifier = new ArrayList<Identifier>(); 695 for (Identifier i : identifier) 696 dst.identifier.add(i.copy()); 697 } 698 ; 699 dst.category = category == null ? null : category.copy(); 700 dst.status = status == null ? null : status.copy(); 701 dst.period = period == null ? null : period.copy(); 702 dst.subject = subject == null ? null : subject.copy(); 703 dst.encounter = encounter == null ? null : encounter.copy(); 704 dst.author = author == null ? null : author.copy(); 705 dst.code = code == null ? null : code.copy(); 706 return dst; 707 } 708 709 protected Flag typedCopy() { 710 return copy(); 711 } 712 713 @Override 714 public boolean equalsDeep(Base other) { 715 if (!super.equalsDeep(other)) 716 return false; 717 if (!(other instanceof Flag)) 718 return false; 719 Flag o = (Flag) other; 720 return compareDeep(identifier, o.identifier, true) && compareDeep(category, o.category, true) 721 && compareDeep(status, o.status, true) && compareDeep(period, o.period, true) 722 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 723 && compareDeep(author, o.author, true) && compareDeep(code, o.code, true); 724 } 725 726 @Override 727 public boolean equalsShallow(Base other) { 728 if (!super.equalsShallow(other)) 729 return false; 730 if (!(other instanceof Flag)) 731 return false; 732 Flag o = (Flag) other; 733 return compareValues(status, o.status, true); 734 } 735 736 public boolean isEmpty() { 737 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (category == null || category.isEmpty()) 738 && (status == null || status.isEmpty()) && (period == null || period.isEmpty()) 739 && (subject == null || subject.isEmpty()) && (encounter == null || encounter.isEmpty()) 740 && (author == null || author.isEmpty()) && (code == null || code.isEmpty()); 741 } 742 743 @Override 744 public ResourceType getResourceType() { 745 return ResourceType.Flag; 746 } 747 748 @SearchParamDefinition(name = "date", path = "Flag.period", description = "Time period when flag is active", type = "date") 749 public static final String SP_DATE = "date"; 750 @SearchParamDefinition(name = "subject", path = "Flag.subject", description = "The identity of a subject to list flags for", type = "reference") 751 public static final String SP_SUBJECT = "subject"; 752 @SearchParamDefinition(name = "patient", path = "Flag.subject", description = "The identity of a subject to list flags for", type = "reference") 753 public static final String SP_PATIENT = "patient"; 754 @SearchParamDefinition(name = "author", path = "Flag.author", description = "Flag creator", type = "reference") 755 public static final String SP_AUTHOR = "author"; 756 @SearchParamDefinition(name = "encounter", path = "Flag.encounter", description = "Alert relevant during encounter", type = "reference") 757 public static final String SP_ENCOUNTER = "encounter"; 758 759}