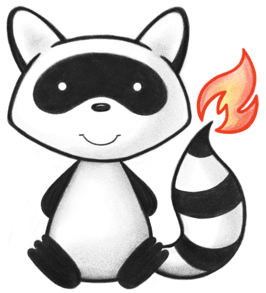
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044 045/** 046 * Describes the intended objective(s) for a patient, group or organization 047 * care, for example, weight loss, restoring an activity of daily living, 048 * obtaining herd immunity via immunization, meeting a process improvement 049 * objective, etc. 050 */ 051@ResourceDef(name = "Goal", profile = "http://hl7.org/fhir/Profile/Goal") 052public class Goal extends DomainResource { 053 054 public enum GoalStatus { 055 /** 056 * A goal is proposed for this patient 057 */ 058 PROPOSED, 059 /** 060 * A goal is planned for this patient 061 */ 062 PLANNED, 063 /** 064 * A proposed goal was accepted 065 */ 066 ACCEPTED, 067 /** 068 * A proposed goal was rejected 069 */ 070 REJECTED, 071 /** 072 * The goal is being sought but has not yet been reached. (Also applies if goal 073 * was reached in the past but there has been regression and goal is being 074 * sought again) 075 */ 076 INPROGRESS, 077 /** 078 * The goal has been met and no further action is needed 079 */ 080 ACHIEVED, 081 /** 082 * The goal has been met, but ongoing activity is needed to sustain the goal 083 * objective 084 */ 085 SUSTAINING, 086 /** 087 * The goal remains a long term objective but is no longer being actively 088 * pursued for a temporary period of time. 089 */ 090 ONHOLD, 091 /** 092 * The goal is no longer being sought 093 */ 094 CANCELLED, 095 /** 096 * added to help the parsers 097 */ 098 NULL; 099 100 public static GoalStatus fromCode(String codeString) throws FHIRException { 101 if (codeString == null || "".equals(codeString)) 102 return null; 103 if ("proposed".equals(codeString)) 104 return PROPOSED; 105 if ("planned".equals(codeString)) 106 return PLANNED; 107 if ("accepted".equals(codeString)) 108 return ACCEPTED; 109 if ("rejected".equals(codeString)) 110 return REJECTED; 111 if ("in-progress".equals(codeString)) 112 return INPROGRESS; 113 if ("achieved".equals(codeString)) 114 return ACHIEVED; 115 if ("sustaining".equals(codeString)) 116 return SUSTAINING; 117 if ("on-hold".equals(codeString)) 118 return ONHOLD; 119 if ("cancelled".equals(codeString)) 120 return CANCELLED; 121 throw new FHIRException("Unknown GoalStatus code '" + codeString + "'"); 122 } 123 124 public String toCode() { 125 switch (this) { 126 case PROPOSED: 127 return "proposed"; 128 case PLANNED: 129 return "planned"; 130 case ACCEPTED: 131 return "accepted"; 132 case REJECTED: 133 return "rejected"; 134 case INPROGRESS: 135 return "in-progress"; 136 case ACHIEVED: 137 return "achieved"; 138 case SUSTAINING: 139 return "sustaining"; 140 case ONHOLD: 141 return "on-hold"; 142 case CANCELLED: 143 return "cancelled"; 144 case NULL: 145 return null; 146 default: 147 return "?"; 148 } 149 } 150 151 public String getSystem() { 152 switch (this) { 153 case PROPOSED: 154 return "http://hl7.org/fhir/goal-status"; 155 case PLANNED: 156 return "http://hl7.org/fhir/goal-status"; 157 case ACCEPTED: 158 return "http://hl7.org/fhir/goal-status"; 159 case REJECTED: 160 return "http://hl7.org/fhir/goal-status"; 161 case INPROGRESS: 162 return "http://hl7.org/fhir/goal-status"; 163 case ACHIEVED: 164 return "http://hl7.org/fhir/goal-status"; 165 case SUSTAINING: 166 return "http://hl7.org/fhir/goal-status"; 167 case ONHOLD: 168 return "http://hl7.org/fhir/goal-status"; 169 case CANCELLED: 170 return "http://hl7.org/fhir/goal-status"; 171 case NULL: 172 return null; 173 default: 174 return "?"; 175 } 176 } 177 178 public String getDefinition() { 179 switch (this) { 180 case PROPOSED: 181 return "A goal is proposed for this patient"; 182 case PLANNED: 183 return "A goal is planned for this patient"; 184 case ACCEPTED: 185 return "A proposed goal was accepted"; 186 case REJECTED: 187 return "A proposed goal was rejected"; 188 case INPROGRESS: 189 return "The goal is being sought but has not yet been reached. (Also applies if goal was reached in the past but there has been regression and goal is being sought again)"; 190 case ACHIEVED: 191 return "The goal has been met and no further action is needed"; 192 case SUSTAINING: 193 return "The goal has been met, but ongoing activity is needed to sustain the goal objective"; 194 case ONHOLD: 195 return "The goal remains a long term objective but is no longer being actively pursued for a temporary period of time."; 196 case CANCELLED: 197 return "The goal is no longer being sought"; 198 case NULL: 199 return null; 200 default: 201 return "?"; 202 } 203 } 204 205 public String getDisplay() { 206 switch (this) { 207 case PROPOSED: 208 return "Proposed"; 209 case PLANNED: 210 return "Planned"; 211 case ACCEPTED: 212 return "Accepted"; 213 case REJECTED: 214 return "Rejected"; 215 case INPROGRESS: 216 return "In Progress"; 217 case ACHIEVED: 218 return "Achieved"; 219 case SUSTAINING: 220 return "Sustaining"; 221 case ONHOLD: 222 return "On Hold"; 223 case CANCELLED: 224 return "Cancelled"; 225 case NULL: 226 return null; 227 default: 228 return "?"; 229 } 230 } 231 } 232 233 public static class GoalStatusEnumFactory implements EnumFactory<GoalStatus> { 234 public GoalStatus fromCode(String codeString) throws IllegalArgumentException { 235 if (codeString == null || "".equals(codeString)) 236 if (codeString == null || "".equals(codeString)) 237 return null; 238 if ("proposed".equals(codeString)) 239 return GoalStatus.PROPOSED; 240 if ("planned".equals(codeString)) 241 return GoalStatus.PLANNED; 242 if ("accepted".equals(codeString)) 243 return GoalStatus.ACCEPTED; 244 if ("rejected".equals(codeString)) 245 return GoalStatus.REJECTED; 246 if ("in-progress".equals(codeString)) 247 return GoalStatus.INPROGRESS; 248 if ("achieved".equals(codeString)) 249 return GoalStatus.ACHIEVED; 250 if ("sustaining".equals(codeString)) 251 return GoalStatus.SUSTAINING; 252 if ("on-hold".equals(codeString)) 253 return GoalStatus.ONHOLD; 254 if ("cancelled".equals(codeString)) 255 return GoalStatus.CANCELLED; 256 throw new IllegalArgumentException("Unknown GoalStatus code '" + codeString + "'"); 257 } 258 259 public Enumeration<GoalStatus> fromType(Base code) throws FHIRException { 260 if (code == null || code.isEmpty()) 261 return null; 262 String codeString = ((PrimitiveType) code).asStringValue(); 263 if (codeString == null || "".equals(codeString)) 264 return null; 265 if ("proposed".equals(codeString)) 266 return new Enumeration<GoalStatus>(this, GoalStatus.PROPOSED); 267 if ("planned".equals(codeString)) 268 return new Enumeration<GoalStatus>(this, GoalStatus.PLANNED); 269 if ("accepted".equals(codeString)) 270 return new Enumeration<GoalStatus>(this, GoalStatus.ACCEPTED); 271 if ("rejected".equals(codeString)) 272 return new Enumeration<GoalStatus>(this, GoalStatus.REJECTED); 273 if ("in-progress".equals(codeString)) 274 return new Enumeration<GoalStatus>(this, GoalStatus.INPROGRESS); 275 if ("achieved".equals(codeString)) 276 return new Enumeration<GoalStatus>(this, GoalStatus.ACHIEVED); 277 if ("sustaining".equals(codeString)) 278 return new Enumeration<GoalStatus>(this, GoalStatus.SUSTAINING); 279 if ("on-hold".equals(codeString)) 280 return new Enumeration<GoalStatus>(this, GoalStatus.ONHOLD); 281 if ("cancelled".equals(codeString)) 282 return new Enumeration<GoalStatus>(this, GoalStatus.CANCELLED); 283 throw new FHIRException("Unknown GoalStatus code '" + codeString + "'"); 284 } 285 286 public String toCode(GoalStatus code) 287 { 288 if (code == GoalStatus.NULL) 289 return null; 290 if (code == GoalStatus.PROPOSED) 291 return "proposed"; 292 if (code == GoalStatus.PLANNED) 293 return "planned"; 294 if (code == GoalStatus.ACCEPTED) 295 return "accepted"; 296 if (code == GoalStatus.REJECTED) 297 return "rejected"; 298 if (code == GoalStatus.INPROGRESS) 299 return "in-progress"; 300 if (code == GoalStatus.ACHIEVED) 301 return "achieved"; 302 if (code == GoalStatus.SUSTAINING) 303 return "sustaining"; 304 if (code == GoalStatus.ONHOLD) 305 return "on-hold"; 306 if (code == GoalStatus.CANCELLED) 307 return "cancelled"; 308 return "?"; 309 } 310 } 311 312 @Block() 313 public static class GoalOutcomeComponent extends BackboneElement implements IBaseBackboneElement { 314 /** 315 * Details of what's changed (or not changed). 316 */ 317 @Child(name = "result", type = { CodeableConcept.class, 318 Observation.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 319 @Description(shortDefinition = "Code or observation that resulted from goal", formalDefinition = "Details of what's changed (or not changed).") 320 protected Type result; 321 322 private static final long serialVersionUID = 1994317639L; 323 324 /* 325 * Constructor 326 */ 327 public GoalOutcomeComponent() { 328 super(); 329 } 330 331 /** 332 * @return {@link #result} (Details of what's changed (or not changed).) 333 */ 334 public Type getResult() { 335 return this.result; 336 } 337 338 /** 339 * @return {@link #result} (Details of what's changed (or not changed).) 340 */ 341 public CodeableConcept getResultCodeableConcept() throws FHIRException { 342 if (!(this.result instanceof CodeableConcept)) 343 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 344 + this.result.getClass().getName() + " was encountered"); 345 return (CodeableConcept) this.result; 346 } 347 348 public boolean hasResultCodeableConcept() { 349 return this.result instanceof CodeableConcept; 350 } 351 352 /** 353 * @return {@link #result} (Details of what's changed (or not changed).) 354 */ 355 public Reference getResultReference() throws FHIRException { 356 if (!(this.result instanceof Reference)) 357 throw new FHIRException("Type mismatch: the type Reference was expected, but " 358 + this.result.getClass().getName() + " was encountered"); 359 return (Reference) this.result; 360 } 361 362 public boolean hasResultReference() { 363 return this.result instanceof Reference; 364 } 365 366 public boolean hasResult() { 367 return this.result != null && !this.result.isEmpty(); 368 } 369 370 /** 371 * @param value {@link #result} (Details of what's changed (or not changed).) 372 */ 373 public GoalOutcomeComponent setResult(Type value) { 374 this.result = value; 375 return this; 376 } 377 378 protected void listChildren(List<Property> childrenList) { 379 super.listChildren(childrenList); 380 childrenList.add(new Property("result[x]", "CodeableConcept|Reference(Observation)", 381 "Details of what's changed (or not changed).", 0, java.lang.Integer.MAX_VALUE, result)); 382 } 383 384 @Override 385 public void setProperty(String name, Base value) throws FHIRException { 386 if (name.equals("result[x]")) 387 this.result = (Type) value; // Type 388 else 389 super.setProperty(name, value); 390 } 391 392 @Override 393 public Base addChild(String name) throws FHIRException { 394 if (name.equals("resultCodeableConcept")) { 395 this.result = new CodeableConcept(); 396 return this.result; 397 } else if (name.equals("resultReference")) { 398 this.result = new Reference(); 399 return this.result; 400 } else 401 return super.addChild(name); 402 } 403 404 public GoalOutcomeComponent copy() { 405 GoalOutcomeComponent dst = new GoalOutcomeComponent(); 406 copyValues(dst); 407 dst.result = result == null ? null : result.copy(); 408 return dst; 409 } 410 411 @Override 412 public boolean equalsDeep(Base other) { 413 if (!super.equalsDeep(other)) 414 return false; 415 if (!(other instanceof GoalOutcomeComponent)) 416 return false; 417 GoalOutcomeComponent o = (GoalOutcomeComponent) other; 418 return compareDeep(result, o.result, true); 419 } 420 421 @Override 422 public boolean equalsShallow(Base other) { 423 if (!super.equalsShallow(other)) 424 return false; 425 if (!(other instanceof GoalOutcomeComponent)) 426 return false; 427 GoalOutcomeComponent o = (GoalOutcomeComponent) other; 428 return true; 429 } 430 431 public boolean isEmpty() { 432 return super.isEmpty() && (result == null || result.isEmpty()); 433 } 434 435 public String fhirType() { 436 return "Goal.outcome"; 437 438 } 439 440 } 441 442 /** 443 * This records identifiers associated with this care plan that are defined by 444 * business processes and/or used to refer to it when a direct URL reference to 445 * the resource itself is not appropriate (e.g. in CDA documents, or in written 446 * / printed documentation). 447 */ 448 @Child(name = "identifier", type = { 449 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 450 @Description(shortDefinition = "External Ids for this goal", formalDefinition = "This records identifiers associated with this care plan that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).") 451 protected List<Identifier> identifier; 452 453 /** 454 * Identifies the patient, group or organization for whom the goal is being 455 * established. 456 */ 457 @Child(name = "subject", type = { Patient.class, Group.class, 458 Organization.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 459 @Description(shortDefinition = "Who this goal is intended for", formalDefinition = "Identifies the patient, group or organization for whom the goal is being established.") 460 protected Reference subject; 461 462 /** 463 * The actual object that is the target of the reference (Identifies the 464 * patient, group or organization for whom the goal is being established.) 465 */ 466 protected Resource subjectTarget; 467 468 /** 469 * The date or event after which the goal should begin being pursued. 470 */ 471 @Child(name = "start", type = { DateType.class, 472 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 473 @Description(shortDefinition = "When goal pursuit begins", formalDefinition = "The date or event after which the goal should begin being pursued.") 474 protected Type start; 475 476 /** 477 * Indicates either the date or the duration after start by which the goal 478 * should be met. 479 */ 480 @Child(name = "target", type = { DateType.class, 481 Duration.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 482 @Description(shortDefinition = "Reach goal on or before", formalDefinition = "Indicates either the date or the duration after start by which the goal should be met.") 483 protected Type target; 484 485 /** 486 * Indicates a category the goal falls within. 487 */ 488 @Child(name = "category", type = { 489 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 490 @Description(shortDefinition = "E.g. Treatment, dietary, behavioral, etc.", formalDefinition = "Indicates a category the goal falls within.") 491 protected List<CodeableConcept> category; 492 493 /** 494 * Human-readable description of a specific desired objective of care. 495 */ 496 @Child(name = "description", type = { 497 StringType.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 498 @Description(shortDefinition = "What's the desired outcome?", formalDefinition = "Human-readable description of a specific desired objective of care.") 499 protected StringType description; 500 501 /** 502 * Indicates whether the goal has been reached and is still considered relevant. 503 */ 504 @Child(name = "status", type = { CodeType.class }, order = 6, min = 1, max = 1, modifier = true, summary = true) 505 @Description(shortDefinition = "proposed | planned | accepted | rejected | in-progress | achieved | sustaining | on-hold | cancelled", formalDefinition = "Indicates whether the goal has been reached and is still considered relevant.") 506 protected Enumeration<GoalStatus> status; 507 508 /** 509 * Identifies when the current status. I.e. When initially created, when 510 * achieved, when cancelled, etc. 511 */ 512 @Child(name = "statusDate", type = { DateType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 513 @Description(shortDefinition = "When goal status took effect", formalDefinition = "Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc.") 514 protected DateType statusDate; 515 516 /** 517 * Captures the reason for the current status. 518 */ 519 @Child(name = "statusReason", type = { 520 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 521 @Description(shortDefinition = "Reason for current status", formalDefinition = "Captures the reason for the current status.") 522 protected CodeableConcept statusReason; 523 524 /** 525 * Indicates whose goal this is - patient goal, practitioner goal, etc. 526 */ 527 @Child(name = "author", type = { Patient.class, Practitioner.class, 528 RelatedPerson.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 529 @Description(shortDefinition = "Who's responsible for creating Goal?", formalDefinition = "Indicates whose goal this is - patient goal, practitioner goal, etc.") 530 protected Reference author; 531 532 /** 533 * The actual object that is the target of the reference (Indicates whose goal 534 * this is - patient goal, practitioner goal, etc.) 535 */ 536 protected Resource authorTarget; 537 538 /** 539 * Identifies the mutually agreed level of importance associated with 540 * reaching/sustaining the goal. 541 */ 542 @Child(name = "priority", type = { 543 CodeableConcept.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 544 @Description(shortDefinition = "high | medium |low", formalDefinition = "Identifies the mutually agreed level of importance associated with reaching/sustaining the goal.") 545 protected CodeableConcept priority; 546 547 /** 548 * The identified conditions and other health record elements that are intended 549 * to be addressed by the goal. 550 */ 551 @Child(name = "addresses", type = { Condition.class, Observation.class, MedicationStatement.class, 552 NutritionOrder.class, ProcedureRequest.class, 553 RiskAssessment.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 554 @Description(shortDefinition = "Issues addressed by this goal", formalDefinition = "The identified conditions and other health record elements that are intended to be addressed by the goal.") 555 protected List<Reference> addresses; 556 /** 557 * The actual objects that are the target of the reference (The identified 558 * conditions and other health record elements that are intended to be addressed 559 * by the goal.) 560 */ 561 protected List<Resource> addressesTarget; 562 563 /** 564 * Any comments related to the goal. 565 */ 566 @Child(name = "note", type = { 567 Annotation.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 568 @Description(shortDefinition = "Comments about the goal", formalDefinition = "Any comments related to the goal.") 569 protected List<Annotation> note; 570 571 /** 572 * Identifies the change (or lack of change) at the point where the goal was 573 * deepmed to be cancelled or achieved. 574 */ 575 @Child(name = "outcome", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 576 @Description(shortDefinition = "What was end result of goal?", formalDefinition = "Identifies the change (or lack of change) at the point where the goal was deepmed to be cancelled or achieved.") 577 protected List<GoalOutcomeComponent> outcome; 578 579 private static final long serialVersionUID = 2029459056L; 580 581 /* 582 * Constructor 583 */ 584 public Goal() { 585 super(); 586 } 587 588 /* 589 * Constructor 590 */ 591 public Goal(StringType description, Enumeration<GoalStatus> status) { 592 super(); 593 this.description = description; 594 this.status = status; 595 } 596 597 /** 598 * @return {@link #identifier} (This records identifiers associated with this 599 * care plan that are defined by business processes and/or used to refer 600 * to it when a direct URL reference to the resource itself is not 601 * appropriate (e.g. in CDA documents, or in written / printed 602 * documentation).) 603 */ 604 public List<Identifier> getIdentifier() { 605 if (this.identifier == null) 606 this.identifier = new ArrayList<Identifier>(); 607 return this.identifier; 608 } 609 610 public boolean hasIdentifier() { 611 if (this.identifier == null) 612 return false; 613 for (Identifier item : this.identifier) 614 if (!item.isEmpty()) 615 return true; 616 return false; 617 } 618 619 /** 620 * @return {@link #identifier} (This records identifiers associated with this 621 * care plan that are defined by business processes and/or used to refer 622 * to it when a direct URL reference to the resource itself is not 623 * appropriate (e.g. in CDA documents, or in written / printed 624 * documentation).) 625 */ 626 // syntactic sugar 627 public Identifier addIdentifier() { // 3 628 Identifier t = new Identifier(); 629 if (this.identifier == null) 630 this.identifier = new ArrayList<Identifier>(); 631 this.identifier.add(t); 632 return t; 633 } 634 635 // syntactic sugar 636 public Goal addIdentifier(Identifier t) { // 3 637 if (t == null) 638 return this; 639 if (this.identifier == null) 640 this.identifier = new ArrayList<Identifier>(); 641 this.identifier.add(t); 642 return this; 643 } 644 645 /** 646 * @return {@link #subject} (Identifies the patient, group or organization for 647 * whom the goal is being established.) 648 */ 649 public Reference getSubject() { 650 if (this.subject == null) 651 if (Configuration.errorOnAutoCreate()) 652 throw new Error("Attempt to auto-create Goal.subject"); 653 else if (Configuration.doAutoCreate()) 654 this.subject = new Reference(); // cc 655 return this.subject; 656 } 657 658 public boolean hasSubject() { 659 return this.subject != null && !this.subject.isEmpty(); 660 } 661 662 /** 663 * @param value {@link #subject} (Identifies the patient, group or organization 664 * for whom the goal is being established.) 665 */ 666 public Goal setSubject(Reference value) { 667 this.subject = value; 668 return this; 669 } 670 671 /** 672 * @return {@link #subject} The actual object that is the target of the 673 * reference. The reference library doesn't populate this, but you can 674 * use it to hold the resource if you resolve it. (Identifies the 675 * patient, group or organization for whom the goal is being 676 * established.) 677 */ 678 public Resource getSubjectTarget() { 679 return this.subjectTarget; 680 } 681 682 /** 683 * @param value {@link #subject} The actual object that is the target of the 684 * reference. The reference library doesn't use these, but you can 685 * use it to hold the resource if you resolve it. (Identifies the 686 * patient, group or organization for whom the goal is being 687 * established.) 688 */ 689 public Goal setSubjectTarget(Resource value) { 690 this.subjectTarget = value; 691 return this; 692 } 693 694 /** 695 * @return {@link #start} (The date or event after which the goal should begin 696 * being pursued.) 697 */ 698 public Type getStart() { 699 return this.start; 700 } 701 702 /** 703 * @return {@link #start} (The date or event after which the goal should begin 704 * being pursued.) 705 */ 706 public DateType getStartDateType() throws FHIRException { 707 if (!(this.start instanceof DateType)) 708 throw new FHIRException( 709 "Type mismatch: the type DateType was expected, but " + this.start.getClass().getName() + " was encountered"); 710 return (DateType) this.start; 711 } 712 713 public boolean hasStartDateType() { 714 return this.start instanceof DateType; 715 } 716 717 /** 718 * @return {@link #start} (The date or event after which the goal should begin 719 * being pursued.) 720 */ 721 public CodeableConcept getStartCodeableConcept() throws FHIRException { 722 if (!(this.start instanceof CodeableConcept)) 723 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 724 + this.start.getClass().getName() + " was encountered"); 725 return (CodeableConcept) this.start; 726 } 727 728 public boolean hasStartCodeableConcept() { 729 return this.start instanceof CodeableConcept; 730 } 731 732 public boolean hasStart() { 733 return this.start != null && !this.start.isEmpty(); 734 } 735 736 /** 737 * @param value {@link #start} (The date or event after which the goal should 738 * begin being pursued.) 739 */ 740 public Goal setStart(Type value) { 741 this.start = value; 742 return this; 743 } 744 745 /** 746 * @return {@link #target} (Indicates either the date or the duration after 747 * start by which the goal should be met.) 748 */ 749 public Type getTarget() { 750 return this.target; 751 } 752 753 /** 754 * @return {@link #target} (Indicates either the date or the duration after 755 * start by which the goal should be met.) 756 */ 757 public DateType getTargetDateType() throws FHIRException { 758 if (!(this.target instanceof DateType)) 759 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.target.getClass().getName() 760 + " was encountered"); 761 return (DateType) this.target; 762 } 763 764 public boolean hasTargetDateType() { 765 return this.target instanceof DateType; 766 } 767 768 /** 769 * @return {@link #target} (Indicates either the date or the duration after 770 * start by which the goal should be met.) 771 */ 772 public Duration getTargetDuration() throws FHIRException { 773 if (!(this.target instanceof Duration)) 774 throw new FHIRException("Type mismatch: the type Duration was expected, but " + this.target.getClass().getName() 775 + " was encountered"); 776 return (Duration) this.target; 777 } 778 779 public boolean hasTargetDuration() { 780 return this.target instanceof Duration; 781 } 782 783 public boolean hasTarget() { 784 return this.target != null && !this.target.isEmpty(); 785 } 786 787 /** 788 * @param value {@link #target} (Indicates either the date or the duration after 789 * start by which the goal should be met.) 790 */ 791 public Goal setTarget(Type value) { 792 this.target = value; 793 return this; 794 } 795 796 /** 797 * @return {@link #category} (Indicates a category the goal falls within.) 798 */ 799 public List<CodeableConcept> getCategory() { 800 if (this.category == null) 801 this.category = new ArrayList<CodeableConcept>(); 802 return this.category; 803 } 804 805 public boolean hasCategory() { 806 if (this.category == null) 807 return false; 808 for (CodeableConcept item : this.category) 809 if (!item.isEmpty()) 810 return true; 811 return false; 812 } 813 814 /** 815 * @return {@link #category} (Indicates a category the goal falls within.) 816 */ 817 // syntactic sugar 818 public CodeableConcept addCategory() { // 3 819 CodeableConcept t = new CodeableConcept(); 820 if (this.category == null) 821 this.category = new ArrayList<CodeableConcept>(); 822 this.category.add(t); 823 return t; 824 } 825 826 // syntactic sugar 827 public Goal addCategory(CodeableConcept t) { // 3 828 if (t == null) 829 return this; 830 if (this.category == null) 831 this.category = new ArrayList<CodeableConcept>(); 832 this.category.add(t); 833 return this; 834 } 835 836 /** 837 * @return {@link #description} (Human-readable description of a specific 838 * desired objective of care.). This is the underlying object with id, 839 * value and extensions. The accessor "getDescription" gives direct 840 * access to the value 841 */ 842 public StringType getDescriptionElement() { 843 if (this.description == null) 844 if (Configuration.errorOnAutoCreate()) 845 throw new Error("Attempt to auto-create Goal.description"); 846 else if (Configuration.doAutoCreate()) 847 this.description = new StringType(); // bb 848 return this.description; 849 } 850 851 public boolean hasDescriptionElement() { 852 return this.description != null && !this.description.isEmpty(); 853 } 854 855 public boolean hasDescription() { 856 return this.description != null && !this.description.isEmpty(); 857 } 858 859 /** 860 * @param value {@link #description} (Human-readable description of a specific 861 * desired objective of care.). This is the underlying object with 862 * id, value and extensions. The accessor "getDescription" gives 863 * direct access to the value 864 */ 865 public Goal setDescriptionElement(StringType value) { 866 this.description = value; 867 return this; 868 } 869 870 /** 871 * @return Human-readable description of a specific desired objective of care. 872 */ 873 public String getDescription() { 874 return this.description == null ? null : this.description.getValue(); 875 } 876 877 /** 878 * @param value Human-readable description of a specific desired objective of 879 * care. 880 */ 881 public Goal setDescription(String value) { 882 if (this.description == null) 883 this.description = new StringType(); 884 this.description.setValue(value); 885 return this; 886 } 887 888 /** 889 * @return {@link #status} (Indicates whether the goal has been reached and is 890 * still considered relevant.). This is the underlying object with id, 891 * value and extensions. The accessor "getStatus" gives direct access to 892 * the value 893 */ 894 public Enumeration<GoalStatus> getStatusElement() { 895 if (this.status == null) 896 if (Configuration.errorOnAutoCreate()) 897 throw new Error("Attempt to auto-create Goal.status"); 898 else if (Configuration.doAutoCreate()) 899 this.status = new Enumeration<GoalStatus>(new GoalStatusEnumFactory()); // bb 900 return this.status; 901 } 902 903 public boolean hasStatusElement() { 904 return this.status != null && !this.status.isEmpty(); 905 } 906 907 public boolean hasStatus() { 908 return this.status != null && !this.status.isEmpty(); 909 } 910 911 /** 912 * @param value {@link #status} (Indicates whether the goal has been reached and 913 * is still considered relevant.). This is the underlying object 914 * with id, value and extensions. The accessor "getStatus" gives 915 * direct access to the value 916 */ 917 public Goal setStatusElement(Enumeration<GoalStatus> value) { 918 this.status = value; 919 return this; 920 } 921 922 /** 923 * @return Indicates whether the goal has been reached and is still considered 924 * relevant. 925 */ 926 public GoalStatus getStatus() { 927 return this.status == null ? null : this.status.getValue(); 928 } 929 930 /** 931 * @param value Indicates whether the goal has been reached and is still 932 * considered relevant. 933 */ 934 public Goal setStatus(GoalStatus value) { 935 if (this.status == null) 936 this.status = new Enumeration<GoalStatus>(new GoalStatusEnumFactory()); 937 this.status.setValue(value); 938 return this; 939 } 940 941 /** 942 * @return {@link #statusDate} (Identifies when the current status. I.e. When 943 * initially created, when achieved, when cancelled, etc.). This is the 944 * underlying object with id, value and extensions. The accessor 945 * "getStatusDate" gives direct access to the value 946 */ 947 public DateType getStatusDateElement() { 948 if (this.statusDate == null) 949 if (Configuration.errorOnAutoCreate()) 950 throw new Error("Attempt to auto-create Goal.statusDate"); 951 else if (Configuration.doAutoCreate()) 952 this.statusDate = new DateType(); // bb 953 return this.statusDate; 954 } 955 956 public boolean hasStatusDateElement() { 957 return this.statusDate != null && !this.statusDate.isEmpty(); 958 } 959 960 public boolean hasStatusDate() { 961 return this.statusDate != null && !this.statusDate.isEmpty(); 962 } 963 964 /** 965 * @param value {@link #statusDate} (Identifies when the current status. I.e. 966 * When initially created, when achieved, when cancelled, etc.). 967 * This is the underlying object with id, value and extensions. The 968 * accessor "getStatusDate" gives direct access to the value 969 */ 970 public Goal setStatusDateElement(DateType value) { 971 this.statusDate = value; 972 return this; 973 } 974 975 /** 976 * @return Identifies when the current status. I.e. When initially created, when 977 * achieved, when cancelled, etc. 978 */ 979 public Date getStatusDate() { 980 return this.statusDate == null ? null : this.statusDate.getValue(); 981 } 982 983 /** 984 * @param value Identifies when the current status. I.e. When initially created, 985 * when achieved, when cancelled, etc. 986 */ 987 public Goal setStatusDate(Date value) { 988 if (value == null) 989 this.statusDate = null; 990 else { 991 if (this.statusDate == null) 992 this.statusDate = new DateType(); 993 this.statusDate.setValue(value); 994 } 995 return this; 996 } 997 998 /** 999 * @return {@link #statusReason} (Captures the reason for the current status.) 1000 */ 1001 public CodeableConcept getStatusReason() { 1002 if (this.statusReason == null) 1003 if (Configuration.errorOnAutoCreate()) 1004 throw new Error("Attempt to auto-create Goal.statusReason"); 1005 else if (Configuration.doAutoCreate()) 1006 this.statusReason = new CodeableConcept(); // cc 1007 return this.statusReason; 1008 } 1009 1010 public boolean hasStatusReason() { 1011 return this.statusReason != null && !this.statusReason.isEmpty(); 1012 } 1013 1014 /** 1015 * @param value {@link #statusReason} (Captures the reason for the current 1016 * status.) 1017 */ 1018 public Goal setStatusReason(CodeableConcept value) { 1019 this.statusReason = value; 1020 return this; 1021 } 1022 1023 /** 1024 * @return {@link #author} (Indicates whose goal this is - patient goal, 1025 * practitioner goal, etc.) 1026 */ 1027 public Reference getAuthor() { 1028 if (this.author == null) 1029 if (Configuration.errorOnAutoCreate()) 1030 throw new Error("Attempt to auto-create Goal.author"); 1031 else if (Configuration.doAutoCreate()) 1032 this.author = new Reference(); // cc 1033 return this.author; 1034 } 1035 1036 public boolean hasAuthor() { 1037 return this.author != null && !this.author.isEmpty(); 1038 } 1039 1040 /** 1041 * @param value {@link #author} (Indicates whose goal this is - patient goal, 1042 * practitioner goal, etc.) 1043 */ 1044 public Goal setAuthor(Reference value) { 1045 this.author = value; 1046 return this; 1047 } 1048 1049 /** 1050 * @return {@link #author} The actual object that is the target of the 1051 * reference. The reference library doesn't populate this, but you can 1052 * use it to hold the resource if you resolve it. (Indicates whose goal 1053 * this is - patient goal, practitioner goal, etc.) 1054 */ 1055 public Resource getAuthorTarget() { 1056 return this.authorTarget; 1057 } 1058 1059 /** 1060 * @param value {@link #author} The actual object that is the target of the 1061 * reference. The reference library doesn't use these, but you can 1062 * use it to hold the resource if you resolve it. (Indicates whose 1063 * goal this is - patient goal, practitioner goal, etc.) 1064 */ 1065 public Goal setAuthorTarget(Resource value) { 1066 this.authorTarget = value; 1067 return this; 1068 } 1069 1070 /** 1071 * @return {@link #priority} (Identifies the mutually agreed level of importance 1072 * associated with reaching/sustaining the goal.) 1073 */ 1074 public CodeableConcept getPriority() { 1075 if (this.priority == null) 1076 if (Configuration.errorOnAutoCreate()) 1077 throw new Error("Attempt to auto-create Goal.priority"); 1078 else if (Configuration.doAutoCreate()) 1079 this.priority = new CodeableConcept(); // cc 1080 return this.priority; 1081 } 1082 1083 public boolean hasPriority() { 1084 return this.priority != null && !this.priority.isEmpty(); 1085 } 1086 1087 /** 1088 * @param value {@link #priority} (Identifies the mutually agreed level of 1089 * importance associated with reaching/sustaining the goal.) 1090 */ 1091 public Goal setPriority(CodeableConcept value) { 1092 this.priority = value; 1093 return this; 1094 } 1095 1096 /** 1097 * @return {@link #addresses} (The identified conditions and other health record 1098 * elements that are intended to be addressed by the goal.) 1099 */ 1100 public List<Reference> getAddresses() { 1101 if (this.addresses == null) 1102 this.addresses = new ArrayList<Reference>(); 1103 return this.addresses; 1104 } 1105 1106 public boolean hasAddresses() { 1107 if (this.addresses == null) 1108 return false; 1109 for (Reference item : this.addresses) 1110 if (!item.isEmpty()) 1111 return true; 1112 return false; 1113 } 1114 1115 /** 1116 * @return {@link #addresses} (The identified conditions and other health record 1117 * elements that are intended to be addressed by the goal.) 1118 */ 1119 // syntactic sugar 1120 public Reference addAddresses() { // 3 1121 Reference t = new Reference(); 1122 if (this.addresses == null) 1123 this.addresses = new ArrayList<Reference>(); 1124 this.addresses.add(t); 1125 return t; 1126 } 1127 1128 // syntactic sugar 1129 public Goal addAddresses(Reference t) { // 3 1130 if (t == null) 1131 return this; 1132 if (this.addresses == null) 1133 this.addresses = new ArrayList<Reference>(); 1134 this.addresses.add(t); 1135 return this; 1136 } 1137 1138 /** 1139 * @return {@link #addresses} (The actual objects that are the target of the 1140 * reference. The reference library doesn't populate this, but you can 1141 * use this to hold the resources if you resolvethemt. The identified 1142 * conditions and other health record elements that are intended to be 1143 * addressed by the goal.) 1144 */ 1145 public List<Resource> getAddressesTarget() { 1146 if (this.addressesTarget == null) 1147 this.addressesTarget = new ArrayList<Resource>(); 1148 return this.addressesTarget; 1149 } 1150 1151 /** 1152 * @return {@link #note} (Any comments related to the goal.) 1153 */ 1154 public List<Annotation> getNote() { 1155 if (this.note == null) 1156 this.note = new ArrayList<Annotation>(); 1157 return this.note; 1158 } 1159 1160 public boolean hasNote() { 1161 if (this.note == null) 1162 return false; 1163 for (Annotation item : this.note) 1164 if (!item.isEmpty()) 1165 return true; 1166 return false; 1167 } 1168 1169 /** 1170 * @return {@link #note} (Any comments related to the goal.) 1171 */ 1172 // syntactic sugar 1173 public Annotation addNote() { // 3 1174 Annotation t = new Annotation(); 1175 if (this.note == null) 1176 this.note = new ArrayList<Annotation>(); 1177 this.note.add(t); 1178 return t; 1179 } 1180 1181 // syntactic sugar 1182 public Goal addNote(Annotation t) { // 3 1183 if (t == null) 1184 return this; 1185 if (this.note == null) 1186 this.note = new ArrayList<Annotation>(); 1187 this.note.add(t); 1188 return this; 1189 } 1190 1191 /** 1192 * @return {@link #outcome} (Identifies the change (or lack of change) at the 1193 * point where the goal was deepmed to be cancelled or achieved.) 1194 */ 1195 public List<GoalOutcomeComponent> getOutcome() { 1196 if (this.outcome == null) 1197 this.outcome = new ArrayList<GoalOutcomeComponent>(); 1198 return this.outcome; 1199 } 1200 1201 public boolean hasOutcome() { 1202 if (this.outcome == null) 1203 return false; 1204 for (GoalOutcomeComponent item : this.outcome) 1205 if (!item.isEmpty()) 1206 return true; 1207 return false; 1208 } 1209 1210 /** 1211 * @return {@link #outcome} (Identifies the change (or lack of change) at the 1212 * point where the goal was deepmed to be cancelled or achieved.) 1213 */ 1214 // syntactic sugar 1215 public GoalOutcomeComponent addOutcome() { // 3 1216 GoalOutcomeComponent t = new GoalOutcomeComponent(); 1217 if (this.outcome == null) 1218 this.outcome = new ArrayList<GoalOutcomeComponent>(); 1219 this.outcome.add(t); 1220 return t; 1221 } 1222 1223 // syntactic sugar 1224 public Goal addOutcome(GoalOutcomeComponent t) { // 3 1225 if (t == null) 1226 return this; 1227 if (this.outcome == null) 1228 this.outcome = new ArrayList<GoalOutcomeComponent>(); 1229 this.outcome.add(t); 1230 return this; 1231 } 1232 1233 protected void listChildren(List<Property> childrenList) { 1234 super.listChildren(childrenList); 1235 childrenList.add(new Property("identifier", "Identifier", 1236 "This records identifiers associated with this care plan that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 1237 0, java.lang.Integer.MAX_VALUE, identifier)); 1238 childrenList.add(new Property("subject", "Reference(Patient|Group|Organization)", 1239 "Identifies the patient, group or organization for whom the goal is being established.", 0, 1240 java.lang.Integer.MAX_VALUE, subject)); 1241 childrenList.add(new Property("start[x]", "date|CodeableConcept", 1242 "The date or event after which the goal should begin being pursued.", 0, java.lang.Integer.MAX_VALUE, start)); 1243 childrenList.add(new Property("target[x]", "date|Duration", 1244 "Indicates either the date or the duration after start by which the goal should be met.", 0, 1245 java.lang.Integer.MAX_VALUE, target)); 1246 childrenList.add(new Property("category", "CodeableConcept", "Indicates a category the goal falls within.", 0, 1247 java.lang.Integer.MAX_VALUE, category)); 1248 childrenList.add( 1249 new Property("description", "string", "Human-readable description of a specific desired objective of care.", 0, 1250 java.lang.Integer.MAX_VALUE, description)); 1251 childrenList.add( 1252 new Property("status", "code", "Indicates whether the goal has been reached and is still considered relevant.", 1253 0, java.lang.Integer.MAX_VALUE, status)); 1254 childrenList.add(new Property("statusDate", "date", 1255 "Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc.", 0, 1256 java.lang.Integer.MAX_VALUE, statusDate)); 1257 childrenList.add(new Property("statusReason", "CodeableConcept", "Captures the reason for the current status.", 0, 1258 java.lang.Integer.MAX_VALUE, statusReason)); 1259 childrenList.add(new Property("author", "Reference(Patient|Practitioner|RelatedPerson)", 1260 "Indicates whose goal this is - patient goal, practitioner goal, etc.", 0, java.lang.Integer.MAX_VALUE, 1261 author)); 1262 childrenList.add(new Property("priority", "CodeableConcept", 1263 "Identifies the mutually agreed level of importance associated with reaching/sustaining the goal.", 0, 1264 java.lang.Integer.MAX_VALUE, priority)); 1265 childrenList.add(new Property("addresses", 1266 "Reference(Condition|Observation|MedicationStatement|NutritionOrder|ProcedureRequest|RiskAssessment)", 1267 "The identified conditions and other health record elements that are intended to be addressed by the goal.", 0, 1268 java.lang.Integer.MAX_VALUE, addresses)); 1269 childrenList.add( 1270 new Property("note", "Annotation", "Any comments related to the goal.", 0, java.lang.Integer.MAX_VALUE, note)); 1271 childrenList.add(new Property("outcome", "", 1272 "Identifies the change (or lack of change) at the point where the goal was deepmed to be cancelled or achieved.", 1273 0, java.lang.Integer.MAX_VALUE, outcome)); 1274 } 1275 1276 @Override 1277 public void setProperty(String name, Base value) throws FHIRException { 1278 if (name.equals("identifier")) 1279 this.getIdentifier().add(castToIdentifier(value)); 1280 else if (name.equals("subject")) 1281 this.subject = castToReference(value); // Reference 1282 else if (name.equals("start[x]")) 1283 this.start = (Type) value; // Type 1284 else if (name.equals("target[x]")) 1285 this.target = (Type) value; // Type 1286 else if (name.equals("category")) 1287 this.getCategory().add(castToCodeableConcept(value)); 1288 else if (name.equals("description")) 1289 this.description = castToString(value); // StringType 1290 else if (name.equals("status")) 1291 this.status = new GoalStatusEnumFactory().fromType(value); // Enumeration<GoalStatus> 1292 else if (name.equals("statusDate")) 1293 this.statusDate = castToDate(value); // DateType 1294 else if (name.equals("statusReason")) 1295 this.statusReason = castToCodeableConcept(value); // CodeableConcept 1296 else if (name.equals("author")) 1297 this.author = castToReference(value); // Reference 1298 else if (name.equals("priority")) 1299 this.priority = castToCodeableConcept(value); // CodeableConcept 1300 else if (name.equals("addresses")) 1301 this.getAddresses().add(castToReference(value)); 1302 else if (name.equals("note")) 1303 this.getNote().add(castToAnnotation(value)); 1304 else if (name.equals("outcome")) 1305 this.getOutcome().add((GoalOutcomeComponent) value); 1306 else 1307 super.setProperty(name, value); 1308 } 1309 1310 @Override 1311 public Base addChild(String name) throws FHIRException { 1312 if (name.equals("identifier")) { 1313 return addIdentifier(); 1314 } else if (name.equals("subject")) { 1315 this.subject = new Reference(); 1316 return this.subject; 1317 } else if (name.equals("startDate")) { 1318 this.start = new DateType(); 1319 return this.start; 1320 } else if (name.equals("startCodeableConcept")) { 1321 this.start = new CodeableConcept(); 1322 return this.start; 1323 } else if (name.equals("targetDate")) { 1324 this.target = new DateType(); 1325 return this.target; 1326 } else if (name.equals("targetDuration")) { 1327 this.target = new Duration(); 1328 return this.target; 1329 } else if (name.equals("category")) { 1330 return addCategory(); 1331 } else if (name.equals("description")) { 1332 throw new FHIRException("Cannot call addChild on a singleton property Goal.description"); 1333 } else if (name.equals("status")) { 1334 throw new FHIRException("Cannot call addChild on a singleton property Goal.status"); 1335 } else if (name.equals("statusDate")) { 1336 throw new FHIRException("Cannot call addChild on a singleton property Goal.statusDate"); 1337 } else if (name.equals("statusReason")) { 1338 this.statusReason = new CodeableConcept(); 1339 return this.statusReason; 1340 } else if (name.equals("author")) { 1341 this.author = new Reference(); 1342 return this.author; 1343 } else if (name.equals("priority")) { 1344 this.priority = new CodeableConcept(); 1345 return this.priority; 1346 } else if (name.equals("addresses")) { 1347 return addAddresses(); 1348 } else if (name.equals("note")) { 1349 return addNote(); 1350 } else if (name.equals("outcome")) { 1351 return addOutcome(); 1352 } else 1353 return super.addChild(name); 1354 } 1355 1356 public String fhirType() { 1357 return "Goal"; 1358 1359 } 1360 1361 public Goal copy() { 1362 Goal dst = new Goal(); 1363 copyValues(dst); 1364 if (identifier != null) { 1365 dst.identifier = new ArrayList<Identifier>(); 1366 for (Identifier i : identifier) 1367 dst.identifier.add(i.copy()); 1368 } 1369 ; 1370 dst.subject = subject == null ? null : subject.copy(); 1371 dst.start = start == null ? null : start.copy(); 1372 dst.target = target == null ? null : target.copy(); 1373 if (category != null) { 1374 dst.category = new ArrayList<CodeableConcept>(); 1375 for (CodeableConcept i : category) 1376 dst.category.add(i.copy()); 1377 } 1378 ; 1379 dst.description = description == null ? null : description.copy(); 1380 dst.status = status == null ? null : status.copy(); 1381 dst.statusDate = statusDate == null ? null : statusDate.copy(); 1382 dst.statusReason = statusReason == null ? null : statusReason.copy(); 1383 dst.author = author == null ? null : author.copy(); 1384 dst.priority = priority == null ? null : priority.copy(); 1385 if (addresses != null) { 1386 dst.addresses = new ArrayList<Reference>(); 1387 for (Reference i : addresses) 1388 dst.addresses.add(i.copy()); 1389 } 1390 ; 1391 if (note != null) { 1392 dst.note = new ArrayList<Annotation>(); 1393 for (Annotation i : note) 1394 dst.note.add(i.copy()); 1395 } 1396 ; 1397 if (outcome != null) { 1398 dst.outcome = new ArrayList<GoalOutcomeComponent>(); 1399 for (GoalOutcomeComponent i : outcome) 1400 dst.outcome.add(i.copy()); 1401 } 1402 ; 1403 return dst; 1404 } 1405 1406 protected Goal typedCopy() { 1407 return copy(); 1408 } 1409 1410 @Override 1411 public boolean equalsDeep(Base other) { 1412 if (!super.equalsDeep(other)) 1413 return false; 1414 if (!(other instanceof Goal)) 1415 return false; 1416 Goal o = (Goal) other; 1417 return compareDeep(identifier, o.identifier, true) && compareDeep(subject, o.subject, true) 1418 && compareDeep(start, o.start, true) && compareDeep(target, o.target, true) 1419 && compareDeep(category, o.category, true) && compareDeep(description, o.description, true) 1420 && compareDeep(status, o.status, true) && compareDeep(statusDate, o.statusDate, true) 1421 && compareDeep(statusReason, o.statusReason, true) && compareDeep(author, o.author, true) 1422 && compareDeep(priority, o.priority, true) && compareDeep(addresses, o.addresses, true) 1423 && compareDeep(note, o.note, true) && compareDeep(outcome, o.outcome, true); 1424 } 1425 1426 @Override 1427 public boolean equalsShallow(Base other) { 1428 if (!super.equalsShallow(other)) 1429 return false; 1430 if (!(other instanceof Goal)) 1431 return false; 1432 Goal o = (Goal) other; 1433 return compareValues(description, o.description, true) && compareValues(status, o.status, true) 1434 && compareValues(statusDate, o.statusDate, true); 1435 } 1436 1437 public boolean isEmpty() { 1438 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (subject == null || subject.isEmpty()) 1439 && (start == null || start.isEmpty()) && (target == null || target.isEmpty()) 1440 && (category == null || category.isEmpty()) && (description == null || description.isEmpty()) 1441 && (status == null || status.isEmpty()) && (statusDate == null || statusDate.isEmpty()) 1442 && (statusReason == null || statusReason.isEmpty()) && (author == null || author.isEmpty()) 1443 && (priority == null || priority.isEmpty()) && (addresses == null || addresses.isEmpty()) 1444 && (note == null || note.isEmpty()) && (outcome == null || outcome.isEmpty()); 1445 } 1446 1447 @Override 1448 public ResourceType getResourceType() { 1449 return ResourceType.Goal; 1450 } 1451 1452 @SearchParamDefinition(name = "identifier", path = "Goal.identifier", description = "External Ids for this goal", type = "token") 1453 public static final String SP_IDENTIFIER = "identifier"; 1454 @SearchParamDefinition(name = "patient", path = "Goal.subject", description = "Who this goal is intended for", type = "reference") 1455 public static final String SP_PATIENT = "patient"; 1456 @SearchParamDefinition(name = "subject", path = "Goal.subject", description = "Who this goal is intended for", type = "reference") 1457 public static final String SP_SUBJECT = "subject"; 1458 @SearchParamDefinition(name = "targetdate", path = "Goal.targetDate", description = "Reach goal on or before", type = "date") 1459 public static final String SP_TARGETDATE = "targetdate"; 1460 @SearchParamDefinition(name = "category", path = "Goal.category", description = "E.g. Treatment, dietary, behavioral, etc.", type = "token") 1461 public static final String SP_CATEGORY = "category"; 1462 @SearchParamDefinition(name = "status", path = "Goal.status", description = "proposed | planned | accepted | rejected | in-progress | achieved | sustaining | on-hold | cancelled", type = "token") 1463 public static final String SP_STATUS = "status"; 1464 1465}