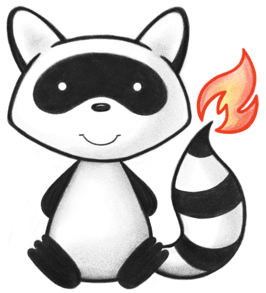
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Block; 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.utilities.Utilities; 044 045/** 046 * Represents a defined collection of entities that may be discussed or acted 047 * upon collectively but which are not expected to act collectively and are not 048 * formally or legally recognized; i.e. a collection of entities that isn't an 049 * Organization. 050 */ 051@ResourceDef(name = "Group", profile = "http://hl7.org/fhir/Profile/Group") 052public class Group extends DomainResource { 053 054 public enum GroupType { 055 /** 056 * Group contains "person" Patient resources 057 */ 058 PERSON, 059 /** 060 * Group contains "animal" Patient resources 061 */ 062 ANIMAL, 063 /** 064 * Group contains healthcare practitioner resources 065 */ 066 PRACTITIONER, 067 /** 068 * Group contains Device resources 069 */ 070 DEVICE, 071 /** 072 * Group contains Medication resources 073 */ 074 MEDICATION, 075 /** 076 * Group contains Substance resources 077 */ 078 SUBSTANCE, 079 /** 080 * added to help the parsers 081 */ 082 NULL; 083 084 public static GroupType fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("person".equals(codeString)) 088 return PERSON; 089 if ("animal".equals(codeString)) 090 return ANIMAL; 091 if ("practitioner".equals(codeString)) 092 return PRACTITIONER; 093 if ("device".equals(codeString)) 094 return DEVICE; 095 if ("medication".equals(codeString)) 096 return MEDICATION; 097 if ("substance".equals(codeString)) 098 return SUBSTANCE; 099 throw new FHIRException("Unknown GroupType code '" + codeString + "'"); 100 } 101 102 public String toCode() { 103 switch (this) { 104 case PERSON: 105 return "person"; 106 case ANIMAL: 107 return "animal"; 108 case PRACTITIONER: 109 return "practitioner"; 110 case DEVICE: 111 return "device"; 112 case MEDICATION: 113 return "medication"; 114 case SUBSTANCE: 115 return "substance"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getSystem() { 124 switch (this) { 125 case PERSON: 126 return "http://hl7.org/fhir/group-type"; 127 case ANIMAL: 128 return "http://hl7.org/fhir/group-type"; 129 case PRACTITIONER: 130 return "http://hl7.org/fhir/group-type"; 131 case DEVICE: 132 return "http://hl7.org/fhir/group-type"; 133 case MEDICATION: 134 return "http://hl7.org/fhir/group-type"; 135 case SUBSTANCE: 136 return "http://hl7.org/fhir/group-type"; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144 public String getDefinition() { 145 switch (this) { 146 case PERSON: 147 return "Group contains \"person\" Patient resources"; 148 case ANIMAL: 149 return "Group contains \"animal\" Patient resources"; 150 case PRACTITIONER: 151 return "Group contains healthcare practitioner resources"; 152 case DEVICE: 153 return "Group contains Device resources"; 154 case MEDICATION: 155 return "Group contains Medication resources"; 156 case SUBSTANCE: 157 return "Group contains Substance resources"; 158 case NULL: 159 return null; 160 default: 161 return "?"; 162 } 163 } 164 165 public String getDisplay() { 166 switch (this) { 167 case PERSON: 168 return "Person"; 169 case ANIMAL: 170 return "Animal"; 171 case PRACTITIONER: 172 return "Practitioner"; 173 case DEVICE: 174 return "Device"; 175 case MEDICATION: 176 return "Medication"; 177 case SUBSTANCE: 178 return "Substance"; 179 case NULL: 180 return null; 181 default: 182 return "?"; 183 } 184 } 185 } 186 187 public static class GroupTypeEnumFactory implements EnumFactory<GroupType> { 188 public GroupType fromCode(String codeString) throws IllegalArgumentException { 189 if (codeString == null || "".equals(codeString)) 190 if (codeString == null || "".equals(codeString)) 191 return null; 192 if ("person".equals(codeString)) 193 return GroupType.PERSON; 194 if ("animal".equals(codeString)) 195 return GroupType.ANIMAL; 196 if ("practitioner".equals(codeString)) 197 return GroupType.PRACTITIONER; 198 if ("device".equals(codeString)) 199 return GroupType.DEVICE; 200 if ("medication".equals(codeString)) 201 return GroupType.MEDICATION; 202 if ("substance".equals(codeString)) 203 return GroupType.SUBSTANCE; 204 throw new IllegalArgumentException("Unknown GroupType code '" + codeString + "'"); 205 } 206 207 public Enumeration<GroupType> fromType(Base code) throws FHIRException { 208 if (code == null || code.isEmpty()) 209 return null; 210 String codeString = ((PrimitiveType) code).asStringValue(); 211 if (codeString == null || "".equals(codeString)) 212 return null; 213 if ("person".equals(codeString)) 214 return new Enumeration<GroupType>(this, GroupType.PERSON); 215 if ("animal".equals(codeString)) 216 return new Enumeration<GroupType>(this, GroupType.ANIMAL); 217 if ("practitioner".equals(codeString)) 218 return new Enumeration<GroupType>(this, GroupType.PRACTITIONER); 219 if ("device".equals(codeString)) 220 return new Enumeration<GroupType>(this, GroupType.DEVICE); 221 if ("medication".equals(codeString)) 222 return new Enumeration<GroupType>(this, GroupType.MEDICATION); 223 if ("substance".equals(codeString)) 224 return new Enumeration<GroupType>(this, GroupType.SUBSTANCE); 225 throw new FHIRException("Unknown GroupType code '" + codeString + "'"); 226 } 227 228 public String toCode(GroupType code) 229 { 230 if (code == GroupType.NULL) 231 return null; 232 if (code == GroupType.PERSON) 233 return "person"; 234 if (code == GroupType.ANIMAL) 235 return "animal"; 236 if (code == GroupType.PRACTITIONER) 237 return "practitioner"; 238 if (code == GroupType.DEVICE) 239 return "device"; 240 if (code == GroupType.MEDICATION) 241 return "medication"; 242 if (code == GroupType.SUBSTANCE) 243 return "substance"; 244 return "?"; 245 } 246 } 247 248 @Block() 249 public static class GroupCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 250 /** 251 * A code that identifies the kind of trait being asserted. 252 */ 253 @Child(name = "code", type = { 254 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 255 @Description(shortDefinition = "Kind of characteristic", formalDefinition = "A code that identifies the kind of trait being asserted.") 256 protected CodeableConcept code; 257 258 /** 259 * The value of the trait that holds (or does not hold - see 'exclude') for 260 * members of the group. 261 */ 262 @Child(name = "value", type = { CodeableConcept.class, BooleanType.class, Quantity.class, 263 Range.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 264 @Description(shortDefinition = "Value held by characteristic", formalDefinition = "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.") 265 protected Type value; 266 267 /** 268 * If true, indicates the characteristic is one that is NOT held by members of 269 * the group. 270 */ 271 @Child(name = "exclude", type = { 272 BooleanType.class }, order = 3, min = 1, max = 1, modifier = true, summary = false) 273 @Description(shortDefinition = "Group includes or excludes", formalDefinition = "If true, indicates the characteristic is one that is NOT held by members of the group.") 274 protected BooleanType exclude; 275 276 /** 277 * The period over which the characteristic is tested; e.g. the patient had an 278 * operation during the month of June. 279 */ 280 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 281 @Description(shortDefinition = "Period over which characteristic is tested", formalDefinition = "The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.") 282 protected Period period; 283 284 private static final long serialVersionUID = -1000688967L; 285 286 /* 287 * Constructor 288 */ 289 public GroupCharacteristicComponent() { 290 super(); 291 } 292 293 /* 294 * Constructor 295 */ 296 public GroupCharacteristicComponent(CodeableConcept code, Type value, BooleanType exclude) { 297 super(); 298 this.code = code; 299 this.value = value; 300 this.exclude = exclude; 301 } 302 303 /** 304 * @return {@link #code} (A code that identifies the kind of trait being 305 * asserted.) 306 */ 307 public CodeableConcept getCode() { 308 if (this.code == null) 309 if (Configuration.errorOnAutoCreate()) 310 throw new Error("Attempt to auto-create GroupCharacteristicComponent.code"); 311 else if (Configuration.doAutoCreate()) 312 this.code = new CodeableConcept(); // cc 313 return this.code; 314 } 315 316 public boolean hasCode() { 317 return this.code != null && !this.code.isEmpty(); 318 } 319 320 /** 321 * @param value {@link #code} (A code that identifies the kind of trait being 322 * asserted.) 323 */ 324 public GroupCharacteristicComponent setCode(CodeableConcept value) { 325 this.code = value; 326 return this; 327 } 328 329 /** 330 * @return {@link #value} (The value of the trait that holds (or does not hold - 331 * see 'exclude') for members of the group.) 332 */ 333 public Type getValue() { 334 return this.value; 335 } 336 337 /** 338 * @return {@link #value} (The value of the trait that holds (or does not hold - 339 * see 'exclude') for members of the group.) 340 */ 341 public CodeableConcept getValueCodeableConcept() throws FHIRException { 342 if (!(this.value instanceof CodeableConcept)) 343 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 344 + this.value.getClass().getName() + " was encountered"); 345 return (CodeableConcept) this.value; 346 } 347 348 public boolean hasValueCodeableConcept() { 349 return this.value instanceof CodeableConcept; 350 } 351 352 /** 353 * @return {@link #value} (The value of the trait that holds (or does not hold - 354 * see 'exclude') for members of the group.) 355 */ 356 public BooleanType getValueBooleanType() throws FHIRException { 357 if (!(this.value instanceof BooleanType)) 358 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 359 + this.value.getClass().getName() + " was encountered"); 360 return (BooleanType) this.value; 361 } 362 363 public boolean hasValueBooleanType() { 364 return this.value instanceof BooleanType; 365 } 366 367 /** 368 * @return {@link #value} (The value of the trait that holds (or does not hold - 369 * see 'exclude') for members of the group.) 370 */ 371 public Quantity getValueQuantity() throws FHIRException { 372 if (!(this.value instanceof Quantity)) 373 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 374 + " was encountered"); 375 return (Quantity) this.value; 376 } 377 378 public boolean hasValueQuantity() { 379 return this.value instanceof Quantity; 380 } 381 382 /** 383 * @return {@link #value} (The value of the trait that holds (or does not hold - 384 * see 'exclude') for members of the group.) 385 */ 386 public Range getValueRange() throws FHIRException { 387 if (!(this.value instanceof Range)) 388 throw new FHIRException( 389 "Type mismatch: the type Range was expected, but " + this.value.getClass().getName() + " was encountered"); 390 return (Range) this.value; 391 } 392 393 public boolean hasValueRange() { 394 return this.value instanceof Range; 395 } 396 397 public boolean hasValue() { 398 return this.value != null && !this.value.isEmpty(); 399 } 400 401 /** 402 * @param value {@link #value} (The value of the trait that holds (or does not 403 * hold - see 'exclude') for members of the group.) 404 */ 405 public GroupCharacteristicComponent setValue(Type value) { 406 this.value = value; 407 return this; 408 } 409 410 /** 411 * @return {@link #exclude} (If true, indicates the characteristic is one that 412 * is NOT held by members of the group.). This is the underlying object 413 * with id, value and extensions. The accessor "getExclude" gives direct 414 * access to the value 415 */ 416 public BooleanType getExcludeElement() { 417 if (this.exclude == null) 418 if (Configuration.errorOnAutoCreate()) 419 throw new Error("Attempt to auto-create GroupCharacteristicComponent.exclude"); 420 else if (Configuration.doAutoCreate()) 421 this.exclude = new BooleanType(); // bb 422 return this.exclude; 423 } 424 425 public boolean hasExcludeElement() { 426 return this.exclude != null && !this.exclude.isEmpty(); 427 } 428 429 public boolean hasExclude() { 430 return this.exclude != null && !this.exclude.isEmpty(); 431 } 432 433 /** 434 * @param value {@link #exclude} (If true, indicates the characteristic is one 435 * that is NOT held by members of the group.). This is the 436 * underlying object with id, value and extensions. The accessor 437 * "getExclude" gives direct access to the value 438 */ 439 public GroupCharacteristicComponent setExcludeElement(BooleanType value) { 440 this.exclude = value; 441 return this; 442 } 443 444 /** 445 * @return If true, indicates the characteristic is one that is NOT held by 446 * members of the group. 447 */ 448 public boolean getExclude() { 449 return this.exclude == null || this.exclude.isEmpty() ? false : this.exclude.getValue(); 450 } 451 452 /** 453 * @param value If true, indicates the characteristic is one that is NOT held by 454 * members of the group. 455 */ 456 public GroupCharacteristicComponent setExclude(boolean value) { 457 if (this.exclude == null) 458 this.exclude = new BooleanType(); 459 this.exclude.setValue(value); 460 return this; 461 } 462 463 /** 464 * @return {@link #period} (The period over which the characteristic is tested; 465 * e.g. the patient had an operation during the month of June.) 466 */ 467 public Period getPeriod() { 468 if (this.period == null) 469 if (Configuration.errorOnAutoCreate()) 470 throw new Error("Attempt to auto-create GroupCharacteristicComponent.period"); 471 else if (Configuration.doAutoCreate()) 472 this.period = new Period(); // cc 473 return this.period; 474 } 475 476 public boolean hasPeriod() { 477 return this.period != null && !this.period.isEmpty(); 478 } 479 480 /** 481 * @param value {@link #period} (The period over which the characteristic is 482 * tested; e.g. the patient had an operation during the month of 483 * June.) 484 */ 485 public GroupCharacteristicComponent setPeriod(Period value) { 486 this.period = value; 487 return this; 488 } 489 490 protected void listChildren(List<Property> childrenList) { 491 super.listChildren(childrenList); 492 childrenList.add(new Property("code", "CodeableConcept", 493 "A code that identifies the kind of trait being asserted.", 0, java.lang.Integer.MAX_VALUE, code)); 494 childrenList.add(new Property("value[x]", "CodeableConcept|boolean|Quantity|Range", 495 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 496 java.lang.Integer.MAX_VALUE, value)); 497 childrenList.add(new Property("exclude", "boolean", 498 "If true, indicates the characteristic is one that is NOT held by members of the group.", 0, 499 java.lang.Integer.MAX_VALUE, exclude)); 500 childrenList.add(new Property("period", "Period", 501 "The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.", 502 0, java.lang.Integer.MAX_VALUE, period)); 503 } 504 505 @Override 506 public void setProperty(String name, Base value) throws FHIRException { 507 if (name.equals("code")) 508 this.code = castToCodeableConcept(value); // CodeableConcept 509 else if (name.equals("value[x]")) 510 this.value = (Type) value; // Type 511 else if (name.equals("exclude")) 512 this.exclude = castToBoolean(value); // BooleanType 513 else if (name.equals("period")) 514 this.period = castToPeriod(value); // Period 515 else 516 super.setProperty(name, value); 517 } 518 519 @Override 520 public Base addChild(String name) throws FHIRException { 521 if (name.equals("code")) { 522 this.code = new CodeableConcept(); 523 return this.code; 524 } else if (name.equals("valueCodeableConcept")) { 525 this.value = new CodeableConcept(); 526 return this.value; 527 } else if (name.equals("valueBoolean")) { 528 this.value = new BooleanType(); 529 return this.value; 530 } else if (name.equals("valueQuantity")) { 531 this.value = new Quantity(); 532 return this.value; 533 } else if (name.equals("valueRange")) { 534 this.value = new Range(); 535 return this.value; 536 } else if (name.equals("exclude")) { 537 throw new FHIRException("Cannot call addChild on a singleton property Group.exclude"); 538 } else if (name.equals("period")) { 539 this.period = new Period(); 540 return this.period; 541 } else 542 return super.addChild(name); 543 } 544 545 public GroupCharacteristicComponent copy() { 546 GroupCharacteristicComponent dst = new GroupCharacteristicComponent(); 547 copyValues(dst); 548 dst.code = code == null ? null : code.copy(); 549 dst.value = value == null ? null : value.copy(); 550 dst.exclude = exclude == null ? null : exclude.copy(); 551 dst.period = period == null ? null : period.copy(); 552 return dst; 553 } 554 555 @Override 556 public boolean equalsDeep(Base other) { 557 if (!super.equalsDeep(other)) 558 return false; 559 if (!(other instanceof GroupCharacteristicComponent)) 560 return false; 561 GroupCharacteristicComponent o = (GroupCharacteristicComponent) other; 562 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) 563 && compareDeep(exclude, o.exclude, true) && compareDeep(period, o.period, true); 564 } 565 566 @Override 567 public boolean equalsShallow(Base other) { 568 if (!super.equalsShallow(other)) 569 return false; 570 if (!(other instanceof GroupCharacteristicComponent)) 571 return false; 572 GroupCharacteristicComponent o = (GroupCharacteristicComponent) other; 573 return compareValues(exclude, o.exclude, true); 574 } 575 576 public boolean isEmpty() { 577 return super.isEmpty() && (code == null || code.isEmpty()) && (value == null || value.isEmpty()) 578 && (exclude == null || exclude.isEmpty()) && (period == null || period.isEmpty()); 579 } 580 581 public String fhirType() { 582 return "Group.characteristic"; 583 584 } 585 586 } 587 588 @Block() 589 public static class GroupMemberComponent extends BackboneElement implements IBaseBackboneElement { 590 /** 591 * A reference to the entity that is a member of the group. Must be consistent 592 * with Group.type. 593 */ 594 @Child(name = "entity", type = { Patient.class, Practitioner.class, Device.class, Medication.class, 595 Substance.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 596 @Description(shortDefinition = "Reference to the group member", formalDefinition = "A reference to the entity that is a member of the group. Must be consistent with Group.type.") 597 protected Reference entity; 598 599 /** 600 * The actual object that is the target of the reference (A reference to the 601 * entity that is a member of the group. Must be consistent with Group.type.) 602 */ 603 protected Resource entityTarget; 604 605 /** 606 * The period that the member was in the group, if known. 607 */ 608 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 609 @Description(shortDefinition = "Period member belonged to the group", formalDefinition = "The period that the member was in the group, if known.") 610 protected Period period; 611 612 /** 613 * A flag to indicate that the member is no longer in the group, but previously 614 * may have been a member. 615 */ 616 @Child(name = "inactive", type = { 617 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 618 @Description(shortDefinition = "If member is no longer in group", formalDefinition = "A flag to indicate that the member is no longer in the group, but previously may have been a member.") 619 protected BooleanType inactive; 620 621 private static final long serialVersionUID = -333869055L; 622 623 /* 624 * Constructor 625 */ 626 public GroupMemberComponent() { 627 super(); 628 } 629 630 /* 631 * Constructor 632 */ 633 public GroupMemberComponent(Reference entity) { 634 super(); 635 this.entity = entity; 636 } 637 638 /** 639 * @return {@link #entity} (A reference to the entity that is a member of the 640 * group. Must be consistent with Group.type.) 641 */ 642 public Reference getEntity() { 643 if (this.entity == null) 644 if (Configuration.errorOnAutoCreate()) 645 throw new Error("Attempt to auto-create GroupMemberComponent.entity"); 646 else if (Configuration.doAutoCreate()) 647 this.entity = new Reference(); // cc 648 return this.entity; 649 } 650 651 public boolean hasEntity() { 652 return this.entity != null && !this.entity.isEmpty(); 653 } 654 655 /** 656 * @param value {@link #entity} (A reference to the entity that is a member of 657 * the group. Must be consistent with Group.type.) 658 */ 659 public GroupMemberComponent setEntity(Reference value) { 660 this.entity = value; 661 return this; 662 } 663 664 /** 665 * @return {@link #entity} The actual object that is the target of the 666 * reference. The reference library doesn't populate this, but you can 667 * use it to hold the resource if you resolve it. (A reference to the 668 * entity that is a member of the group. Must be consistent with 669 * Group.type.) 670 */ 671 public Resource getEntityTarget() { 672 return this.entityTarget; 673 } 674 675 /** 676 * @param value {@link #entity} The actual object that is the target of the 677 * reference. The reference library doesn't use these, but you can 678 * use it to hold the resource if you resolve it. (A reference to 679 * the entity that is a member of the group. Must be consistent 680 * with Group.type.) 681 */ 682 public GroupMemberComponent setEntityTarget(Resource value) { 683 this.entityTarget = value; 684 return this; 685 } 686 687 /** 688 * @return {@link #period} (The period that the member was in the group, if 689 * known.) 690 */ 691 public Period getPeriod() { 692 if (this.period == null) 693 if (Configuration.errorOnAutoCreate()) 694 throw new Error("Attempt to auto-create GroupMemberComponent.period"); 695 else if (Configuration.doAutoCreate()) 696 this.period = new Period(); // cc 697 return this.period; 698 } 699 700 public boolean hasPeriod() { 701 return this.period != null && !this.period.isEmpty(); 702 } 703 704 /** 705 * @param value {@link #period} (The period that the member was in the group, if 706 * known.) 707 */ 708 public GroupMemberComponent setPeriod(Period value) { 709 this.period = value; 710 return this; 711 } 712 713 /** 714 * @return {@link #inactive} (A flag to indicate that the member is no longer in 715 * the group, but previously may have been a member.). This is the 716 * underlying object with id, value and extensions. The accessor 717 * "getInactive" gives direct access to the value 718 */ 719 public BooleanType getInactiveElement() { 720 if (this.inactive == null) 721 if (Configuration.errorOnAutoCreate()) 722 throw new Error("Attempt to auto-create GroupMemberComponent.inactive"); 723 else if (Configuration.doAutoCreate()) 724 this.inactive = new BooleanType(); // bb 725 return this.inactive; 726 } 727 728 public boolean hasInactiveElement() { 729 return this.inactive != null && !this.inactive.isEmpty(); 730 } 731 732 public boolean hasInactive() { 733 return this.inactive != null && !this.inactive.isEmpty(); 734 } 735 736 /** 737 * @param value {@link #inactive} (A flag to indicate that the member is no 738 * longer in the group, but previously may have been a member.). 739 * This is the underlying object with id, value and extensions. The 740 * accessor "getInactive" gives direct access to the value 741 */ 742 public GroupMemberComponent setInactiveElement(BooleanType value) { 743 this.inactive = value; 744 return this; 745 } 746 747 /** 748 * @return A flag to indicate that the member is no longer in the group, but 749 * previously may have been a member. 750 */ 751 public boolean getInactive() { 752 return this.inactive == null || this.inactive.isEmpty() ? false : this.inactive.getValue(); 753 } 754 755 /** 756 * @param value A flag to indicate that the member is no longer in the group, 757 * but previously may have been a member. 758 */ 759 public GroupMemberComponent setInactive(boolean value) { 760 if (this.inactive == null) 761 this.inactive = new BooleanType(); 762 this.inactive.setValue(value); 763 return this; 764 } 765 766 protected void listChildren(List<Property> childrenList) { 767 super.listChildren(childrenList); 768 childrenList.add(new Property("entity", "Reference(Patient|Practitioner|Device|Medication|Substance)", 769 "A reference to the entity that is a member of the group. Must be consistent with Group.type.", 0, 770 java.lang.Integer.MAX_VALUE, entity)); 771 childrenList.add(new Property("period", "Period", "The period that the member was in the group, if known.", 0, 772 java.lang.Integer.MAX_VALUE, period)); 773 childrenList.add(new Property("inactive", "boolean", 774 "A flag to indicate that the member is no longer in the group, but previously may have been a member.", 0, 775 java.lang.Integer.MAX_VALUE, inactive)); 776 } 777 778 @Override 779 public void setProperty(String name, Base value) throws FHIRException { 780 if (name.equals("entity")) 781 this.entity = castToReference(value); // Reference 782 else if (name.equals("period")) 783 this.period = castToPeriod(value); // Period 784 else if (name.equals("inactive")) 785 this.inactive = castToBoolean(value); // BooleanType 786 else 787 super.setProperty(name, value); 788 } 789 790 @Override 791 public Base addChild(String name) throws FHIRException { 792 if (name.equals("entity")) { 793 this.entity = new Reference(); 794 return this.entity; 795 } else if (name.equals("period")) { 796 this.period = new Period(); 797 return this.period; 798 } else if (name.equals("inactive")) { 799 throw new FHIRException("Cannot call addChild on a singleton property Group.inactive"); 800 } else 801 return super.addChild(name); 802 } 803 804 public GroupMemberComponent copy() { 805 GroupMemberComponent dst = new GroupMemberComponent(); 806 copyValues(dst); 807 dst.entity = entity == null ? null : entity.copy(); 808 dst.period = period == null ? null : period.copy(); 809 dst.inactive = inactive == null ? null : inactive.copy(); 810 return dst; 811 } 812 813 @Override 814 public boolean equalsDeep(Base other) { 815 if (!super.equalsDeep(other)) 816 return false; 817 if (!(other instanceof GroupMemberComponent)) 818 return false; 819 GroupMemberComponent o = (GroupMemberComponent) other; 820 return compareDeep(entity, o.entity, true) && compareDeep(period, o.period, true) 821 && compareDeep(inactive, o.inactive, true); 822 } 823 824 @Override 825 public boolean equalsShallow(Base other) { 826 if (!super.equalsShallow(other)) 827 return false; 828 if (!(other instanceof GroupMemberComponent)) 829 return false; 830 GroupMemberComponent o = (GroupMemberComponent) other; 831 return compareValues(inactive, o.inactive, true); 832 } 833 834 public boolean isEmpty() { 835 return super.isEmpty() && (entity == null || entity.isEmpty()) && (period == null || period.isEmpty()) 836 && (inactive == null || inactive.isEmpty()); 837 } 838 839 public String fhirType() { 840 return "Group.member"; 841 842 } 843 844 } 845 846 /** 847 * A unique business identifier for this group. 848 */ 849 @Child(name = "identifier", type = { 850 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 851 @Description(shortDefinition = "Unique id", formalDefinition = "A unique business identifier for this group.") 852 protected List<Identifier> identifier; 853 854 /** 855 * Identifies the broad classification of the kind of resources the group 856 * includes. 857 */ 858 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 859 @Description(shortDefinition = "person | animal | practitioner | device | medication | substance", formalDefinition = "Identifies the broad classification of the kind of resources the group includes.") 860 protected Enumeration<GroupType> type; 861 862 /** 863 * If true, indicates that the resource refers to a specific group of real 864 * individuals. If false, the group defines a set of intended individuals. 865 */ 866 @Child(name = "actual", type = { BooleanType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 867 @Description(shortDefinition = "Descriptive or actual", formalDefinition = "If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals.") 868 protected BooleanType actual; 869 870 /** 871 * Provides a specific type of resource the group includes; e.g. "cow", 872 * "syringe", etc. 873 */ 874 @Child(name = "code", type = { CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 875 @Description(shortDefinition = "Kind of Group members", formalDefinition = "Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc.") 876 protected CodeableConcept code; 877 878 /** 879 * A label assigned to the group for human identification and communication. 880 */ 881 @Child(name = "name", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 882 @Description(shortDefinition = "Label for Group", formalDefinition = "A label assigned to the group for human identification and communication.") 883 protected StringType name; 884 885 /** 886 * A count of the number of resource instances that are part of the group. 887 */ 888 @Child(name = "quantity", type = { 889 UnsignedIntType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 890 @Description(shortDefinition = "Number of members", formalDefinition = "A count of the number of resource instances that are part of the group.") 891 protected UnsignedIntType quantity; 892 893 /** 894 * Identifies the traits shared by members of the group. 895 */ 896 @Child(name = "characteristic", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 897 @Description(shortDefinition = "Trait of group members", formalDefinition = "Identifies the traits shared by members of the group.") 898 protected List<GroupCharacteristicComponent> characteristic; 899 900 /** 901 * Identifies the resource instances that are members of the group. 902 */ 903 @Child(name = "member", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 904 @Description(shortDefinition = "Who or what is in group", formalDefinition = "Identifies the resource instances that are members of the group.") 905 protected List<GroupMemberComponent> member; 906 907 private static final long serialVersionUID = 1401345819L; 908 909 /* 910 * Constructor 911 */ 912 public Group() { 913 super(); 914 } 915 916 /* 917 * Constructor 918 */ 919 public Group(Enumeration<GroupType> type, BooleanType actual) { 920 super(); 921 this.type = type; 922 this.actual = actual; 923 } 924 925 /** 926 * @return {@link #identifier} (A unique business identifier for this group.) 927 */ 928 public List<Identifier> getIdentifier() { 929 if (this.identifier == null) 930 this.identifier = new ArrayList<Identifier>(); 931 return this.identifier; 932 } 933 934 public boolean hasIdentifier() { 935 if (this.identifier == null) 936 return false; 937 for (Identifier item : this.identifier) 938 if (!item.isEmpty()) 939 return true; 940 return false; 941 } 942 943 /** 944 * @return {@link #identifier} (A unique business identifier for this group.) 945 */ 946 // syntactic sugar 947 public Identifier addIdentifier() { // 3 948 Identifier t = new Identifier(); 949 if (this.identifier == null) 950 this.identifier = new ArrayList<Identifier>(); 951 this.identifier.add(t); 952 return t; 953 } 954 955 // syntactic sugar 956 public Group addIdentifier(Identifier t) { // 3 957 if (t == null) 958 return this; 959 if (this.identifier == null) 960 this.identifier = new ArrayList<Identifier>(); 961 this.identifier.add(t); 962 return this; 963 } 964 965 /** 966 * @return {@link #type} (Identifies the broad classification of the kind of 967 * resources the group includes.). This is the underlying object with 968 * id, value and extensions. The accessor "getType" gives direct access 969 * to the value 970 */ 971 public Enumeration<GroupType> getTypeElement() { 972 if (this.type == null) 973 if (Configuration.errorOnAutoCreate()) 974 throw new Error("Attempt to auto-create Group.type"); 975 else if (Configuration.doAutoCreate()) 976 this.type = new Enumeration<GroupType>(new GroupTypeEnumFactory()); // bb 977 return this.type; 978 } 979 980 public boolean hasTypeElement() { 981 return this.type != null && !this.type.isEmpty(); 982 } 983 984 public boolean hasType() { 985 return this.type != null && !this.type.isEmpty(); 986 } 987 988 /** 989 * @param value {@link #type} (Identifies the broad classification of the kind 990 * of resources the group includes.). This is the underlying object 991 * with id, value and extensions. The accessor "getType" gives 992 * direct access to the value 993 */ 994 public Group setTypeElement(Enumeration<GroupType> value) { 995 this.type = value; 996 return this; 997 } 998 999 /** 1000 * @return Identifies the broad classification of the kind of resources the 1001 * group includes. 1002 */ 1003 public GroupType getType() { 1004 return this.type == null ? null : this.type.getValue(); 1005 } 1006 1007 /** 1008 * @param value Identifies the broad classification of the kind of resources the 1009 * group includes. 1010 */ 1011 public Group setType(GroupType value) { 1012 if (this.type == null) 1013 this.type = new Enumeration<GroupType>(new GroupTypeEnumFactory()); 1014 this.type.setValue(value); 1015 return this; 1016 } 1017 1018 /** 1019 * @return {@link #actual} (If true, indicates that the resource refers to a 1020 * specific group of real individuals. If false, the group defines a set 1021 * of intended individuals.). This is the underlying object with id, 1022 * value and extensions. The accessor "getActual" gives direct access to 1023 * the value 1024 */ 1025 public BooleanType getActualElement() { 1026 if (this.actual == null) 1027 if (Configuration.errorOnAutoCreate()) 1028 throw new Error("Attempt to auto-create Group.actual"); 1029 else if (Configuration.doAutoCreate()) 1030 this.actual = new BooleanType(); // bb 1031 return this.actual; 1032 } 1033 1034 public boolean hasActualElement() { 1035 return this.actual != null && !this.actual.isEmpty(); 1036 } 1037 1038 public boolean hasActual() { 1039 return this.actual != null && !this.actual.isEmpty(); 1040 } 1041 1042 /** 1043 * @param value {@link #actual} (If true, indicates that the resource refers to 1044 * a specific group of real individuals. If false, the group 1045 * defines a set of intended individuals.). This is the underlying 1046 * object with id, value and extensions. The accessor "getActual" 1047 * gives direct access to the value 1048 */ 1049 public Group setActualElement(BooleanType value) { 1050 this.actual = value; 1051 return this; 1052 } 1053 1054 /** 1055 * @return If true, indicates that the resource refers to a specific group of 1056 * real individuals. If false, the group defines a set of intended 1057 * individuals. 1058 */ 1059 public boolean getActual() { 1060 return this.actual == null || this.actual.isEmpty() ? false : this.actual.getValue(); 1061 } 1062 1063 /** 1064 * @param value If true, indicates that the resource refers to a specific group 1065 * of real individuals. If false, the group defines a set of 1066 * intended individuals. 1067 */ 1068 public Group setActual(boolean value) { 1069 if (this.actual == null) 1070 this.actual = new BooleanType(); 1071 this.actual.setValue(value); 1072 return this; 1073 } 1074 1075 /** 1076 * @return {@link #code} (Provides a specific type of resource the group 1077 * includes; e.g. "cow", "syringe", etc.) 1078 */ 1079 public CodeableConcept getCode() { 1080 if (this.code == null) 1081 if (Configuration.errorOnAutoCreate()) 1082 throw new Error("Attempt to auto-create Group.code"); 1083 else if (Configuration.doAutoCreate()) 1084 this.code = new CodeableConcept(); // cc 1085 return this.code; 1086 } 1087 1088 public boolean hasCode() { 1089 return this.code != null && !this.code.isEmpty(); 1090 } 1091 1092 /** 1093 * @param value {@link #code} (Provides a specific type of resource the group 1094 * includes; e.g. "cow", "syringe", etc.) 1095 */ 1096 public Group setCode(CodeableConcept value) { 1097 this.code = value; 1098 return this; 1099 } 1100 1101 /** 1102 * @return {@link #name} (A label assigned to the group for human identification 1103 * and communication.). This is the underlying object with id, value and 1104 * extensions. The accessor "getName" gives direct access to the value 1105 */ 1106 public StringType getNameElement() { 1107 if (this.name == null) 1108 if (Configuration.errorOnAutoCreate()) 1109 throw new Error("Attempt to auto-create Group.name"); 1110 else if (Configuration.doAutoCreate()) 1111 this.name = new StringType(); // bb 1112 return this.name; 1113 } 1114 1115 public boolean hasNameElement() { 1116 return this.name != null && !this.name.isEmpty(); 1117 } 1118 1119 public boolean hasName() { 1120 return this.name != null && !this.name.isEmpty(); 1121 } 1122 1123 /** 1124 * @param value {@link #name} (A label assigned to the group for human 1125 * identification and communication.). This is the underlying 1126 * object with id, value and extensions. The accessor "getName" 1127 * gives direct access to the value 1128 */ 1129 public Group setNameElement(StringType value) { 1130 this.name = value; 1131 return this; 1132 } 1133 1134 /** 1135 * @return A label assigned to the group for human identification and 1136 * communication. 1137 */ 1138 public String getName() { 1139 return this.name == null ? null : this.name.getValue(); 1140 } 1141 1142 /** 1143 * @param value A label assigned to the group for human identification and 1144 * communication. 1145 */ 1146 public Group setName(String value) { 1147 if (Utilities.noString(value)) 1148 this.name = null; 1149 else { 1150 if (this.name == null) 1151 this.name = new StringType(); 1152 this.name.setValue(value); 1153 } 1154 return this; 1155 } 1156 1157 /** 1158 * @return {@link #quantity} (A count of the number of resource instances that 1159 * are part of the group.). This is the underlying object with id, value 1160 * and extensions. The accessor "getQuantity" gives direct access to the 1161 * value 1162 */ 1163 public UnsignedIntType getQuantityElement() { 1164 if (this.quantity == null) 1165 if (Configuration.errorOnAutoCreate()) 1166 throw new Error("Attempt to auto-create Group.quantity"); 1167 else if (Configuration.doAutoCreate()) 1168 this.quantity = new UnsignedIntType(); // bb 1169 return this.quantity; 1170 } 1171 1172 public boolean hasQuantityElement() { 1173 return this.quantity != null && !this.quantity.isEmpty(); 1174 } 1175 1176 public boolean hasQuantity() { 1177 return this.quantity != null && !this.quantity.isEmpty(); 1178 } 1179 1180 /** 1181 * @param value {@link #quantity} (A count of the number of resource instances 1182 * that are part of the group.). This is the underlying object with 1183 * id, value and extensions. The accessor "getQuantity" gives 1184 * direct access to the value 1185 */ 1186 public Group setQuantityElement(UnsignedIntType value) { 1187 this.quantity = value; 1188 return this; 1189 } 1190 1191 /** 1192 * @return A count of the number of resource instances that are part of the 1193 * group. 1194 */ 1195 public int getQuantity() { 1196 return this.quantity == null || this.quantity.isEmpty() ? 0 : this.quantity.getValue(); 1197 } 1198 1199 /** 1200 * @param value A count of the number of resource instances that are part of the 1201 * group. 1202 */ 1203 public Group setQuantity(int value) { 1204 if (this.quantity == null) 1205 this.quantity = new UnsignedIntType(); 1206 this.quantity.setValue(value); 1207 return this; 1208 } 1209 1210 /** 1211 * @return {@link #characteristic} (Identifies the traits shared by members of 1212 * the group.) 1213 */ 1214 public List<GroupCharacteristicComponent> getCharacteristic() { 1215 if (this.characteristic == null) 1216 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1217 return this.characteristic; 1218 } 1219 1220 public boolean hasCharacteristic() { 1221 if (this.characteristic == null) 1222 return false; 1223 for (GroupCharacteristicComponent item : this.characteristic) 1224 if (!item.isEmpty()) 1225 return true; 1226 return false; 1227 } 1228 1229 /** 1230 * @return {@link #characteristic} (Identifies the traits shared by members of 1231 * the group.) 1232 */ 1233 // syntactic sugar 1234 public GroupCharacteristicComponent addCharacteristic() { // 3 1235 GroupCharacteristicComponent t = new GroupCharacteristicComponent(); 1236 if (this.characteristic == null) 1237 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1238 this.characteristic.add(t); 1239 return t; 1240 } 1241 1242 // syntactic sugar 1243 public Group addCharacteristic(GroupCharacteristicComponent t) { // 3 1244 if (t == null) 1245 return this; 1246 if (this.characteristic == null) 1247 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1248 this.characteristic.add(t); 1249 return this; 1250 } 1251 1252 /** 1253 * @return {@link #member} (Identifies the resource instances that are members 1254 * of the group.) 1255 */ 1256 public List<GroupMemberComponent> getMember() { 1257 if (this.member == null) 1258 this.member = new ArrayList<GroupMemberComponent>(); 1259 return this.member; 1260 } 1261 1262 public boolean hasMember() { 1263 if (this.member == null) 1264 return false; 1265 for (GroupMemberComponent item : this.member) 1266 if (!item.isEmpty()) 1267 return true; 1268 return false; 1269 } 1270 1271 /** 1272 * @return {@link #member} (Identifies the resource instances that are members 1273 * of the group.) 1274 */ 1275 // syntactic sugar 1276 public GroupMemberComponent addMember() { // 3 1277 GroupMemberComponent t = new GroupMemberComponent(); 1278 if (this.member == null) 1279 this.member = new ArrayList<GroupMemberComponent>(); 1280 this.member.add(t); 1281 return t; 1282 } 1283 1284 // syntactic sugar 1285 public Group addMember(GroupMemberComponent t) { // 3 1286 if (t == null) 1287 return this; 1288 if (this.member == null) 1289 this.member = new ArrayList<GroupMemberComponent>(); 1290 this.member.add(t); 1291 return this; 1292 } 1293 1294 protected void listChildren(List<Property> childrenList) { 1295 super.listChildren(childrenList); 1296 childrenList.add(new Property("identifier", "Identifier", "A unique business identifier for this group.", 0, 1297 java.lang.Integer.MAX_VALUE, identifier)); 1298 childrenList.add( 1299 new Property("type", "code", "Identifies the broad classification of the kind of resources the group includes.", 1300 0, java.lang.Integer.MAX_VALUE, type)); 1301 childrenList.add(new Property("actual", "boolean", 1302 "If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals.", 1303 0, java.lang.Integer.MAX_VALUE, actual)); 1304 childrenList.add(new Property("code", "CodeableConcept", 1305 "Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc.", 0, 1306 java.lang.Integer.MAX_VALUE, code)); 1307 childrenList 1308 .add(new Property("name", "string", "A label assigned to the group for human identification and communication.", 1309 0, java.lang.Integer.MAX_VALUE, name)); 1310 childrenList.add(new Property("quantity", "unsignedInt", 1311 "A count of the number of resource instances that are part of the group.", 0, java.lang.Integer.MAX_VALUE, 1312 quantity)); 1313 childrenList.add(new Property("characteristic", "", "Identifies the traits shared by members of the group.", 0, 1314 java.lang.Integer.MAX_VALUE, characteristic)); 1315 childrenList.add(new Property("member", "", "Identifies the resource instances that are members of the group.", 0, 1316 java.lang.Integer.MAX_VALUE, member)); 1317 } 1318 1319 @Override 1320 public void setProperty(String name, Base value) throws FHIRException { 1321 if (name.equals("identifier")) 1322 this.getIdentifier().add(castToIdentifier(value)); 1323 else if (name.equals("type")) 1324 this.type = new GroupTypeEnumFactory().fromType(value); // Enumeration<GroupType> 1325 else if (name.equals("actual")) 1326 this.actual = castToBoolean(value); // BooleanType 1327 else if (name.equals("code")) 1328 this.code = castToCodeableConcept(value); // CodeableConcept 1329 else if (name.equals("name")) 1330 this.name = castToString(value); // StringType 1331 else if (name.equals("quantity")) 1332 this.quantity = castToUnsignedInt(value); // UnsignedIntType 1333 else if (name.equals("characteristic")) 1334 this.getCharacteristic().add((GroupCharacteristicComponent) value); 1335 else if (name.equals("member")) 1336 this.getMember().add((GroupMemberComponent) value); 1337 else 1338 super.setProperty(name, value); 1339 } 1340 1341 @Override 1342 public Base addChild(String name) throws FHIRException { 1343 if (name.equals("identifier")) { 1344 return addIdentifier(); 1345 } else if (name.equals("type")) { 1346 throw new FHIRException("Cannot call addChild on a singleton property Group.type"); 1347 } else if (name.equals("actual")) { 1348 throw new FHIRException("Cannot call addChild on a singleton property Group.actual"); 1349 } else if (name.equals("code")) { 1350 this.code = new CodeableConcept(); 1351 return this.code; 1352 } else if (name.equals("name")) { 1353 throw new FHIRException("Cannot call addChild on a singleton property Group.name"); 1354 } else if (name.equals("quantity")) { 1355 throw new FHIRException("Cannot call addChild on a singleton property Group.quantity"); 1356 } else if (name.equals("characteristic")) { 1357 return addCharacteristic(); 1358 } else if (name.equals("member")) { 1359 return addMember(); 1360 } else 1361 return super.addChild(name); 1362 } 1363 1364 public String fhirType() { 1365 return "Group"; 1366 1367 } 1368 1369 public Group copy() { 1370 Group dst = new Group(); 1371 copyValues(dst); 1372 if (identifier != null) { 1373 dst.identifier = new ArrayList<Identifier>(); 1374 for (Identifier i : identifier) 1375 dst.identifier.add(i.copy()); 1376 } 1377 ; 1378 dst.type = type == null ? null : type.copy(); 1379 dst.actual = actual == null ? null : actual.copy(); 1380 dst.code = code == null ? null : code.copy(); 1381 dst.name = name == null ? null : name.copy(); 1382 dst.quantity = quantity == null ? null : quantity.copy(); 1383 if (characteristic != null) { 1384 dst.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1385 for (GroupCharacteristicComponent i : characteristic) 1386 dst.characteristic.add(i.copy()); 1387 } 1388 ; 1389 if (member != null) { 1390 dst.member = new ArrayList<GroupMemberComponent>(); 1391 for (GroupMemberComponent i : member) 1392 dst.member.add(i.copy()); 1393 } 1394 ; 1395 return dst; 1396 } 1397 1398 protected Group typedCopy() { 1399 return copy(); 1400 } 1401 1402 @Override 1403 public boolean equalsDeep(Base other) { 1404 if (!super.equalsDeep(other)) 1405 return false; 1406 if (!(other instanceof Group)) 1407 return false; 1408 Group o = (Group) other; 1409 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 1410 && compareDeep(actual, o.actual, true) && compareDeep(code, o.code, true) && compareDeep(name, o.name, true) 1411 && compareDeep(quantity, o.quantity, true) && compareDeep(characteristic, o.characteristic, true) 1412 && compareDeep(member, o.member, true); 1413 } 1414 1415 @Override 1416 public boolean equalsShallow(Base other) { 1417 if (!super.equalsShallow(other)) 1418 return false; 1419 if (!(other instanceof Group)) 1420 return false; 1421 Group o = (Group) other; 1422 return compareValues(type, o.type, true) && compareValues(actual, o.actual, true) 1423 && compareValues(name, o.name, true) && compareValues(quantity, o.quantity, true); 1424 } 1425 1426 public boolean isEmpty() { 1427 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (type == null || type.isEmpty()) 1428 && (actual == null || actual.isEmpty()) && (code == null || code.isEmpty()) && (name == null || name.isEmpty()) 1429 && (quantity == null || quantity.isEmpty()) && (characteristic == null || characteristic.isEmpty()) 1430 && (member == null || member.isEmpty()); 1431 } 1432 1433 @Override 1434 public ResourceType getResourceType() { 1435 return ResourceType.Group; 1436 } 1437 1438 @SearchParamDefinition(name = "actual", path = "Group.actual", description = "Descriptive or actual", type = "token") 1439 public static final String SP_ACTUAL = "actual"; 1440 @SearchParamDefinition(name = "identifier", path = "Group.identifier", description = "Unique id", type = "token") 1441 public static final String SP_IDENTIFIER = "identifier"; 1442 @SearchParamDefinition(name = "characteristic-value", path = "null", description = "A composite of both characteristic and value", type = "composite") 1443 public static final String SP_CHARACTERISTICVALUE = "characteristic-value"; 1444 @SearchParamDefinition(name = "code", path = "Group.code", description = "The kind of resources contained", type = "token") 1445 public static final String SP_CODE = "code"; 1446 @SearchParamDefinition(name = "member", path = "Group.member.entity", description = "Reference to the group member", type = "reference") 1447 public static final String SP_MEMBER = "member"; 1448 @SearchParamDefinition(name = "exclude", path = "Group.characteristic.exclude", description = "Group includes or excludes", type = "token") 1449 public static final String SP_EXCLUDE = "exclude"; 1450 @SearchParamDefinition(name = "type", path = "Group.type", description = "The type of resources the group contains", type = "token") 1451 public static final String SP_TYPE = "type"; 1452 @SearchParamDefinition(name = "value", path = "Group.characteristic.value[x]", description = "Value held by characteristic", type = "token") 1453 public static final String SP_VALUE = "value"; 1454 @SearchParamDefinition(name = "characteristic", path = "Group.characteristic.code", description = "Kind of characteristic", type = "token") 1455 public static final String SP_CHARACTERISTIC = "characteristic"; 1456 1457}