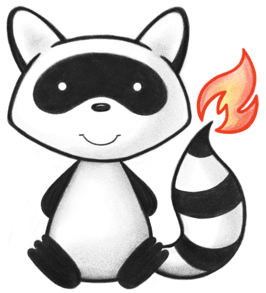
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Block; 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.utilities.Utilities; 044 045/** 046 * The details of a healthcare service available at a location. 047 */ 048@ResourceDef(name = "HealthcareService", profile = "http://hl7.org/fhir/Profile/HealthcareService") 049public class HealthcareService extends DomainResource { 050 051 public enum DaysOfWeek { 052 /** 053 * Monday 054 */ 055 MON, 056 /** 057 * Tuesday 058 */ 059 TUE, 060 /** 061 * Wednesday 062 */ 063 WED, 064 /** 065 * Thursday 066 */ 067 THU, 068 /** 069 * Friday 070 */ 071 FRI, 072 /** 073 * Saturday 074 */ 075 SAT, 076 /** 077 * Sunday 078 */ 079 SUN, 080 /** 081 * added to help the parsers 082 */ 083 NULL; 084 085 public static DaysOfWeek fromCode(String codeString) throws FHIRException { 086 if (codeString == null || "".equals(codeString)) 087 return null; 088 if ("mon".equals(codeString)) 089 return MON; 090 if ("tue".equals(codeString)) 091 return TUE; 092 if ("wed".equals(codeString)) 093 return WED; 094 if ("thu".equals(codeString)) 095 return THU; 096 if ("fri".equals(codeString)) 097 return FRI; 098 if ("sat".equals(codeString)) 099 return SAT; 100 if ("sun".equals(codeString)) 101 return SUN; 102 throw new FHIRException("Unknown DaysOfWeek code '" + codeString + "'"); 103 } 104 105 public String toCode() { 106 switch (this) { 107 case MON: 108 return "mon"; 109 case TUE: 110 return "tue"; 111 case WED: 112 return "wed"; 113 case THU: 114 return "thu"; 115 case FRI: 116 return "fri"; 117 case SAT: 118 return "sat"; 119 case SUN: 120 return "sun"; 121 case NULL: 122 return null; 123 default: 124 return "?"; 125 } 126 } 127 128 public String getSystem() { 129 switch (this) { 130 case MON: 131 return "http://hl7.org/fhir/days-of-week"; 132 case TUE: 133 return "http://hl7.org/fhir/days-of-week"; 134 case WED: 135 return "http://hl7.org/fhir/days-of-week"; 136 case THU: 137 return "http://hl7.org/fhir/days-of-week"; 138 case FRI: 139 return "http://hl7.org/fhir/days-of-week"; 140 case SAT: 141 return "http://hl7.org/fhir/days-of-week"; 142 case SUN: 143 return "http://hl7.org/fhir/days-of-week"; 144 case NULL: 145 return null; 146 default: 147 return "?"; 148 } 149 } 150 151 public String getDefinition() { 152 switch (this) { 153 case MON: 154 return "Monday"; 155 case TUE: 156 return "Tuesday"; 157 case WED: 158 return "Wednesday"; 159 case THU: 160 return "Thursday"; 161 case FRI: 162 return "Friday"; 163 case SAT: 164 return "Saturday"; 165 case SUN: 166 return "Sunday"; 167 case NULL: 168 return null; 169 default: 170 return "?"; 171 } 172 } 173 174 public String getDisplay() { 175 switch (this) { 176 case MON: 177 return "Monday"; 178 case TUE: 179 return "Tuesday"; 180 case WED: 181 return "Wednesday"; 182 case THU: 183 return "Thursday"; 184 case FRI: 185 return "Friday"; 186 case SAT: 187 return "Saturday"; 188 case SUN: 189 return "Sunday"; 190 case NULL: 191 return null; 192 default: 193 return "?"; 194 } 195 } 196 } 197 198 public static class DaysOfWeekEnumFactory implements EnumFactory<DaysOfWeek> { 199 public DaysOfWeek fromCode(String codeString) throws IllegalArgumentException { 200 if (codeString == null || "".equals(codeString)) 201 if (codeString == null || "".equals(codeString)) 202 return null; 203 if ("mon".equals(codeString)) 204 return DaysOfWeek.MON; 205 if ("tue".equals(codeString)) 206 return DaysOfWeek.TUE; 207 if ("wed".equals(codeString)) 208 return DaysOfWeek.WED; 209 if ("thu".equals(codeString)) 210 return DaysOfWeek.THU; 211 if ("fri".equals(codeString)) 212 return DaysOfWeek.FRI; 213 if ("sat".equals(codeString)) 214 return DaysOfWeek.SAT; 215 if ("sun".equals(codeString)) 216 return DaysOfWeek.SUN; 217 throw new IllegalArgumentException("Unknown DaysOfWeek code '" + codeString + "'"); 218 } 219 220 public Enumeration<DaysOfWeek> fromType(Base code) throws FHIRException { 221 if (code == null || code.isEmpty()) 222 return null; 223 String codeString = ((PrimitiveType) code).asStringValue(); 224 if (codeString == null || "".equals(codeString)) 225 return null; 226 if ("mon".equals(codeString)) 227 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.MON); 228 if ("tue".equals(codeString)) 229 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.TUE); 230 if ("wed".equals(codeString)) 231 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.WED); 232 if ("thu".equals(codeString)) 233 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.THU); 234 if ("fri".equals(codeString)) 235 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.FRI); 236 if ("sat".equals(codeString)) 237 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SAT); 238 if ("sun".equals(codeString)) 239 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SUN); 240 throw new FHIRException("Unknown DaysOfWeek code '" + codeString + "'"); 241 } 242 243 public String toCode(DaysOfWeek code) { 244 if (code == DaysOfWeek.MON) 245 return "mon"; 246 if (code == DaysOfWeek.TUE) 247 return "tue"; 248 if (code == DaysOfWeek.WED) 249 return "wed"; 250 if (code == DaysOfWeek.THU) 251 return "thu"; 252 if (code == DaysOfWeek.FRI) 253 return "fri"; 254 if (code == DaysOfWeek.SAT) 255 return "sat"; 256 if (code == DaysOfWeek.SUN) 257 return "sun"; 258 return "?"; 259 } 260 } 261 262 @Block() 263 public static class ServiceTypeComponent extends BackboneElement implements IBaseBackboneElement { 264 /** 265 * The specific type of service being delivered or performed. 266 */ 267 @Child(name = "type", type = { 268 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 269 @Description(shortDefinition = "Type of service delivered or performed", formalDefinition = "The specific type of service being delivered or performed.") 270 protected CodeableConcept type; 271 272 /** 273 * Collection of specialties handled by the service site. This is more of a 274 * medical term. 275 */ 276 @Child(name = "specialty", type = { 277 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 278 @Description(shortDefinition = "Specialties handled by the Service Site", formalDefinition = "Collection of specialties handled by the service site. This is more of a medical term.") 279 protected List<CodeableConcept> specialty; 280 281 private static final long serialVersionUID = 1703986174L; 282 283 /* 284 * Constructor 285 */ 286 public ServiceTypeComponent() { 287 super(); 288 } 289 290 /* 291 * Constructor 292 */ 293 public ServiceTypeComponent(CodeableConcept type) { 294 super(); 295 this.type = type; 296 } 297 298 /** 299 * @return {@link #type} (The specific type of service being delivered or 300 * performed.) 301 */ 302 public CodeableConcept getType() { 303 if (this.type == null) 304 if (Configuration.errorOnAutoCreate()) 305 throw new Error("Attempt to auto-create ServiceTypeComponent.type"); 306 else if (Configuration.doAutoCreate()) 307 this.type = new CodeableConcept(); // cc 308 return this.type; 309 } 310 311 public boolean hasType() { 312 return this.type != null && !this.type.isEmpty(); 313 } 314 315 /** 316 * @param value {@link #type} (The specific type of service being delivered or 317 * performed.) 318 */ 319 public ServiceTypeComponent setType(CodeableConcept value) { 320 this.type = value; 321 return this; 322 } 323 324 /** 325 * @return {@link #specialty} (Collection of specialties handled by the service 326 * site. This is more of a medical term.) 327 */ 328 public List<CodeableConcept> getSpecialty() { 329 if (this.specialty == null) 330 this.specialty = new ArrayList<CodeableConcept>(); 331 return this.specialty; 332 } 333 334 public boolean hasSpecialty() { 335 if (this.specialty == null) 336 return false; 337 for (CodeableConcept item : this.specialty) 338 if (!item.isEmpty()) 339 return true; 340 return false; 341 } 342 343 /** 344 * @return {@link #specialty} (Collection of specialties handled by the service 345 * site. This is more of a medical term.) 346 */ 347 // syntactic sugar 348 public CodeableConcept addSpecialty() { // 3 349 CodeableConcept t = new CodeableConcept(); 350 if (this.specialty == null) 351 this.specialty = new ArrayList<CodeableConcept>(); 352 this.specialty.add(t); 353 return t; 354 } 355 356 // syntactic sugar 357 public ServiceTypeComponent addSpecialty(CodeableConcept t) { // 3 358 if (t == null) 359 return this; 360 if (this.specialty == null) 361 this.specialty = new ArrayList<CodeableConcept>(); 362 this.specialty.add(t); 363 return this; 364 } 365 366 protected void listChildren(List<Property> childrenList) { 367 super.listChildren(childrenList); 368 childrenList.add(new Property("type", "CodeableConcept", 369 "The specific type of service being delivered or performed.", 0, java.lang.Integer.MAX_VALUE, type)); 370 childrenList.add(new Property("specialty", "CodeableConcept", 371 "Collection of specialties handled by the service site. This is more of a medical term.", 0, 372 java.lang.Integer.MAX_VALUE, specialty)); 373 } 374 375 @Override 376 public void setProperty(String name, Base value) throws FHIRException { 377 if (name.equals("type")) 378 this.type = castToCodeableConcept(value); // CodeableConcept 379 else if (name.equals("specialty")) 380 this.getSpecialty().add(castToCodeableConcept(value)); 381 else 382 super.setProperty(name, value); 383 } 384 385 @Override 386 public Base addChild(String name) throws FHIRException { 387 if (name.equals("type")) { 388 this.type = new CodeableConcept(); 389 return this.type; 390 } else if (name.equals("specialty")) { 391 return addSpecialty(); 392 } else 393 return super.addChild(name); 394 } 395 396 public ServiceTypeComponent copy() { 397 ServiceTypeComponent dst = new ServiceTypeComponent(); 398 copyValues(dst); 399 dst.type = type == null ? null : type.copy(); 400 if (specialty != null) { 401 dst.specialty = new ArrayList<CodeableConcept>(); 402 for (CodeableConcept i : specialty) 403 dst.specialty.add(i.copy()); 404 } 405 ; 406 return dst; 407 } 408 409 @Override 410 public boolean equalsDeep(Base other) { 411 if (!super.equalsDeep(other)) 412 return false; 413 if (!(other instanceof ServiceTypeComponent)) 414 return false; 415 ServiceTypeComponent o = (ServiceTypeComponent) other; 416 return compareDeep(type, o.type, true) && compareDeep(specialty, o.specialty, true); 417 } 418 419 @Override 420 public boolean equalsShallow(Base other) { 421 if (!super.equalsShallow(other)) 422 return false; 423 if (!(other instanceof ServiceTypeComponent)) 424 return false; 425 ServiceTypeComponent o = (ServiceTypeComponent) other; 426 return true; 427 } 428 429 public boolean isEmpty() { 430 return super.isEmpty() && (type == null || type.isEmpty()) && (specialty == null || specialty.isEmpty()); 431 } 432 433 public String fhirType() { 434 return "HealthcareService.serviceType"; 435 436 } 437 438 } 439 440 @Block() 441 public static class HealthcareServiceAvailableTimeComponent extends BackboneElement implements IBaseBackboneElement { 442 /** 443 * Indicates which days of the week are available between the start and end 444 * Times. 445 */ 446 @Child(name = "daysOfWeek", type = { 447 CodeType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 448 @Description(shortDefinition = "mon | tue | wed | thu | fri | sat | sun", formalDefinition = "Indicates which days of the week are available between the start and end Times.") 449 protected List<Enumeration<DaysOfWeek>> daysOfWeek; 450 451 /** 452 * Is this always available? (hence times are irrelevant) e.g. 24 hour service. 453 */ 454 @Child(name = "allDay", type = { 455 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 456 @Description(shortDefinition = "Always available? e.g. 24 hour service", formalDefinition = "Is this always available? (hence times are irrelevant) e.g. 24 hour service.") 457 protected BooleanType allDay; 458 459 /** 460 * The opening time of day. Note: If the AllDay flag is set, then this time is 461 * ignored. 462 */ 463 @Child(name = "availableStartTime", type = { 464 TimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 465 @Description(shortDefinition = "Opening time of day (ignored if allDay = true)", formalDefinition = "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.") 466 protected TimeType availableStartTime; 467 468 /** 469 * The closing time of day. Note: If the AllDay flag is set, then this time is 470 * ignored. 471 */ 472 @Child(name = "availableEndTime", type = { 473 TimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 474 @Description(shortDefinition = "Closing time of day (ignored if allDay = true)", formalDefinition = "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.") 475 protected TimeType availableEndTime; 476 477 private static final long serialVersionUID = -2139510127L; 478 479 /* 480 * Constructor 481 */ 482 public HealthcareServiceAvailableTimeComponent() { 483 super(); 484 } 485 486 /** 487 * @return {@link #daysOfWeek} (Indicates which days of the week are available 488 * between the start and end Times.) 489 */ 490 public List<Enumeration<DaysOfWeek>> getDaysOfWeek() { 491 if (this.daysOfWeek == null) 492 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 493 return this.daysOfWeek; 494 } 495 496 public boolean hasDaysOfWeek() { 497 if (this.daysOfWeek == null) 498 return false; 499 for (Enumeration<DaysOfWeek> item : this.daysOfWeek) 500 if (!item.isEmpty()) 501 return true; 502 return false; 503 } 504 505 /** 506 * @return Returns a reference to <code>this</code> for easy method chaining 507 */ 508 public HealthcareServiceAvailableTimeComponent setDaysOfWeek(List<Enumeration<DaysOfWeek>> daysOfWeek) { 509 this.daysOfWeek = daysOfWeek; 510 return this; 511 } 512 513 /** 514 * @return {@link #daysOfWeek} (Indicates which days of the week are available 515 * between the start and end Times.) 516 */ 517 // syntactic sugar 518 public Enumeration<DaysOfWeek> addDaysOfWeekElement() {// 2 519 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 520 if (this.daysOfWeek == null) 521 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 522 this.daysOfWeek.add(t); 523 return t; 524 } 525 526 /** 527 * @param value {@link #daysOfWeek} (Indicates which days of the week are 528 * available between the start and end Times.) 529 */ 530 public HealthcareServiceAvailableTimeComponent addDaysOfWeek(DaysOfWeek value) { // 1 531 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 532 t.setValue(value); 533 if (this.daysOfWeek == null) 534 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 535 this.daysOfWeek.add(t); 536 return this; 537 } 538 539 /** 540 * @param value {@link #daysOfWeek} (Indicates which days of the week are 541 * available between the start and end Times.) 542 */ 543 public boolean hasDaysOfWeek(DaysOfWeek value) { 544 if (this.daysOfWeek == null) 545 return false; 546 for (Enumeration<DaysOfWeek> v : this.daysOfWeek) 547 if (v.equals(value)) // code 548 return true; 549 return false; 550 } 551 552 /** 553 * @return {@link #allDay} (Is this always available? (hence times are 554 * irrelevant) e.g. 24 hour service.). This is the underlying object 555 * with id, value and extensions. The accessor "getAllDay" gives direct 556 * access to the value 557 */ 558 public BooleanType getAllDayElement() { 559 if (this.allDay == null) 560 if (Configuration.errorOnAutoCreate()) 561 throw new Error("Attempt to auto-create HealthcareServiceAvailableTimeComponent.allDay"); 562 else if (Configuration.doAutoCreate()) 563 this.allDay = new BooleanType(); // bb 564 return this.allDay; 565 } 566 567 public boolean hasAllDayElement() { 568 return this.allDay != null && !this.allDay.isEmpty(); 569 } 570 571 public boolean hasAllDay() { 572 return this.allDay != null && !this.allDay.isEmpty(); 573 } 574 575 /** 576 * @param value {@link #allDay} (Is this always available? (hence times are 577 * irrelevant) e.g. 24 hour service.). This is the underlying 578 * object with id, value and extensions. The accessor "getAllDay" 579 * gives direct access to the value 580 */ 581 public HealthcareServiceAvailableTimeComponent setAllDayElement(BooleanType value) { 582 this.allDay = value; 583 return this; 584 } 585 586 /** 587 * @return Is this always available? (hence times are irrelevant) e.g. 24 hour 588 * service. 589 */ 590 public boolean getAllDay() { 591 return this.allDay == null || this.allDay.isEmpty() ? false : this.allDay.getValue(); 592 } 593 594 /** 595 * @param value Is this always available? (hence times are irrelevant) e.g. 24 596 * hour service. 597 */ 598 public HealthcareServiceAvailableTimeComponent setAllDay(boolean value) { 599 if (this.allDay == null) 600 this.allDay = new BooleanType(); 601 this.allDay.setValue(value); 602 return this; 603 } 604 605 /** 606 * @return {@link #availableStartTime} (The opening time of day. Note: If the 607 * AllDay flag is set, then this time is ignored.). This is the 608 * underlying object with id, value and extensions. The accessor 609 * "getAvailableStartTime" gives direct access to the value 610 */ 611 public TimeType getAvailableStartTimeElement() { 612 if (this.availableStartTime == null) 613 if (Configuration.errorOnAutoCreate()) 614 throw new Error("Attempt to auto-create HealthcareServiceAvailableTimeComponent.availableStartTime"); 615 else if (Configuration.doAutoCreate()) 616 this.availableStartTime = new TimeType(); // bb 617 return this.availableStartTime; 618 } 619 620 public boolean hasAvailableStartTimeElement() { 621 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 622 } 623 624 public boolean hasAvailableStartTime() { 625 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 626 } 627 628 /** 629 * @param value {@link #availableStartTime} (The opening time of day. Note: If 630 * the AllDay flag is set, then this time is ignored.). This is the 631 * underlying object with id, value and extensions. The accessor 632 * "getAvailableStartTime" gives direct access to the value 633 */ 634 public HealthcareServiceAvailableTimeComponent setAvailableStartTimeElement(TimeType value) { 635 this.availableStartTime = value; 636 return this; 637 } 638 639 /** 640 * @return The opening time of day. Note: If the AllDay flag is set, then this 641 * time is ignored. 642 */ 643 public String getAvailableStartTime() { 644 return this.availableStartTime == null ? null : this.availableStartTime.getValue(); 645 } 646 647 /** 648 * @param value The opening time of day. Note: If the AllDay flag is set, then 649 * this time is ignored. 650 */ 651 public HealthcareServiceAvailableTimeComponent setAvailableStartTime(String value) { 652 if (value == null) 653 this.availableStartTime = null; 654 else { 655 if (this.availableStartTime == null) 656 this.availableStartTime = new TimeType(); 657 this.availableStartTime.setValue(value); 658 } 659 return this; 660 } 661 662 /** 663 * @return {@link #availableEndTime} (The closing time of day. Note: If the 664 * AllDay flag is set, then this time is ignored.). This is the 665 * underlying object with id, value and extensions. The accessor 666 * "getAvailableEndTime" gives direct access to the value 667 */ 668 public TimeType getAvailableEndTimeElement() { 669 if (this.availableEndTime == null) 670 if (Configuration.errorOnAutoCreate()) 671 throw new Error("Attempt to auto-create HealthcareServiceAvailableTimeComponent.availableEndTime"); 672 else if (Configuration.doAutoCreate()) 673 this.availableEndTime = new TimeType(); // bb 674 return this.availableEndTime; 675 } 676 677 public boolean hasAvailableEndTimeElement() { 678 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 679 } 680 681 public boolean hasAvailableEndTime() { 682 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 683 } 684 685 /** 686 * @param value {@link #availableEndTime} (The closing time of day. Note: If the 687 * AllDay flag is set, then this time is ignored.). This is the 688 * underlying object with id, value and extensions. The accessor 689 * "getAvailableEndTime" gives direct access to the value 690 */ 691 public HealthcareServiceAvailableTimeComponent setAvailableEndTimeElement(TimeType value) { 692 this.availableEndTime = value; 693 return this; 694 } 695 696 /** 697 * @return The closing time of day. Note: If the AllDay flag is set, then this 698 * time is ignored. 699 */ 700 public String getAvailableEndTime() { 701 return this.availableEndTime == null ? null : this.availableEndTime.getValue(); 702 } 703 704 /** 705 * @param value The closing time of day. Note: If the AllDay flag is set, then 706 * this time is ignored. 707 */ 708 public HealthcareServiceAvailableTimeComponent setAvailableEndTime(String value) { 709 if (value == null) 710 this.availableEndTime = null; 711 else { 712 if (this.availableEndTime == null) 713 this.availableEndTime = new TimeType(); 714 this.availableEndTime.setValue(value); 715 } 716 return this; 717 } 718 719 protected void listChildren(List<Property> childrenList) { 720 super.listChildren(childrenList); 721 childrenList.add(new Property("daysOfWeek", "code", 722 "Indicates which days of the week are available between the start and end Times.", 0, 723 java.lang.Integer.MAX_VALUE, daysOfWeek)); 724 childrenList.add(new Property("allDay", "boolean", 725 "Is this always available? (hence times are irrelevant) e.g. 24 hour service.", 0, 726 java.lang.Integer.MAX_VALUE, allDay)); 727 childrenList.add(new Property("availableStartTime", "time", 728 "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 729 java.lang.Integer.MAX_VALUE, availableStartTime)); 730 childrenList.add(new Property("availableEndTime", "time", 731 "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 732 java.lang.Integer.MAX_VALUE, availableEndTime)); 733 } 734 735 @Override 736 public void setProperty(String name, Base value) throws FHIRException { 737 if (name.equals("daysOfWeek")) 738 this.getDaysOfWeek().add(new DaysOfWeekEnumFactory().fromType(value)); 739 else if (name.equals("allDay")) 740 this.allDay = castToBoolean(value); // BooleanType 741 else if (name.equals("availableStartTime")) 742 this.availableStartTime = castToTime(value); // TimeType 743 else if (name.equals("availableEndTime")) 744 this.availableEndTime = castToTime(value); // TimeType 745 else 746 super.setProperty(name, value); 747 } 748 749 @Override 750 public Base addChild(String name) throws FHIRException { 751 if (name.equals("daysOfWeek")) { 752 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.daysOfWeek"); 753 } else if (name.equals("allDay")) { 754 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.allDay"); 755 } else if (name.equals("availableStartTime")) { 756 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.availableStartTime"); 757 } else if (name.equals("availableEndTime")) { 758 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.availableEndTime"); 759 } else 760 return super.addChild(name); 761 } 762 763 public HealthcareServiceAvailableTimeComponent copy() { 764 HealthcareServiceAvailableTimeComponent dst = new HealthcareServiceAvailableTimeComponent(); 765 copyValues(dst); 766 if (daysOfWeek != null) { 767 dst.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 768 for (Enumeration<DaysOfWeek> i : daysOfWeek) 769 dst.daysOfWeek.add(i.copy()); 770 } 771 ; 772 dst.allDay = allDay == null ? null : allDay.copy(); 773 dst.availableStartTime = availableStartTime == null ? null : availableStartTime.copy(); 774 dst.availableEndTime = availableEndTime == null ? null : availableEndTime.copy(); 775 return dst; 776 } 777 778 @Override 779 public boolean equalsDeep(Base other) { 780 if (!super.equalsDeep(other)) 781 return false; 782 if (!(other instanceof HealthcareServiceAvailableTimeComponent)) 783 return false; 784 HealthcareServiceAvailableTimeComponent o = (HealthcareServiceAvailableTimeComponent) other; 785 return compareDeep(daysOfWeek, o.daysOfWeek, true) && compareDeep(allDay, o.allDay, true) 786 && compareDeep(availableStartTime, o.availableStartTime, true) 787 && compareDeep(availableEndTime, o.availableEndTime, true); 788 } 789 790 @Override 791 public boolean equalsShallow(Base other) { 792 if (!super.equalsShallow(other)) 793 return false; 794 if (!(other instanceof HealthcareServiceAvailableTimeComponent)) 795 return false; 796 HealthcareServiceAvailableTimeComponent o = (HealthcareServiceAvailableTimeComponent) other; 797 return compareValues(daysOfWeek, o.daysOfWeek, true) && compareValues(allDay, o.allDay, true) 798 && compareValues(availableStartTime, o.availableStartTime, true) 799 && compareValues(availableEndTime, o.availableEndTime, true); 800 } 801 802 public boolean isEmpty() { 803 return super.isEmpty() && (daysOfWeek == null || daysOfWeek.isEmpty()) && (allDay == null || allDay.isEmpty()) 804 && (availableStartTime == null || availableStartTime.isEmpty()) 805 && (availableEndTime == null || availableEndTime.isEmpty()); 806 } 807 808 public String fhirType() { 809 return "HealthcareService.availableTime"; 810 811 } 812 813 } 814 815 @Block() 816 public static class HealthcareServiceNotAvailableComponent extends BackboneElement implements IBaseBackboneElement { 817 /** 818 * The reason that can be presented to the user as to why this time is not 819 * available. 820 */ 821 @Child(name = "description", type = { 822 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 823 @Description(shortDefinition = "Reason presented to the user explaining why time not available", formalDefinition = "The reason that can be presented to the user as to why this time is not available.") 824 protected StringType description; 825 826 /** 827 * Service is not available (seasonally or for a public holiday) from this date. 828 */ 829 @Child(name = "during", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 830 @Description(shortDefinition = "Service not availablefrom this date", formalDefinition = "Service is not available (seasonally or for a public holiday) from this date.") 831 protected Period during; 832 833 private static final long serialVersionUID = 310849929L; 834 835 /* 836 * Constructor 837 */ 838 public HealthcareServiceNotAvailableComponent() { 839 super(); 840 } 841 842 /* 843 * Constructor 844 */ 845 public HealthcareServiceNotAvailableComponent(StringType description) { 846 super(); 847 this.description = description; 848 } 849 850 /** 851 * @return {@link #description} (The reason that can be presented to the user as 852 * to why this time is not available.). This is the underlying object 853 * with id, value and extensions. The accessor "getDescription" gives 854 * direct access to the value 855 */ 856 public StringType getDescriptionElement() { 857 if (this.description == null) 858 if (Configuration.errorOnAutoCreate()) 859 throw new Error("Attempt to auto-create HealthcareServiceNotAvailableComponent.description"); 860 else if (Configuration.doAutoCreate()) 861 this.description = new StringType(); // bb 862 return this.description; 863 } 864 865 public boolean hasDescriptionElement() { 866 return this.description != null && !this.description.isEmpty(); 867 } 868 869 public boolean hasDescription() { 870 return this.description != null && !this.description.isEmpty(); 871 } 872 873 /** 874 * @param value {@link #description} (The reason that can be presented to the 875 * user as to why this time is not available.). This is the 876 * underlying object with id, value and extensions. The accessor 877 * "getDescription" gives direct access to the value 878 */ 879 public HealthcareServiceNotAvailableComponent setDescriptionElement(StringType value) { 880 this.description = value; 881 return this; 882 } 883 884 /** 885 * @return The reason that can be presented to the user as to why this time is 886 * not available. 887 */ 888 public String getDescription() { 889 return this.description == null ? null : this.description.getValue(); 890 } 891 892 /** 893 * @param value The reason that can be presented to the user as to why this time 894 * is not available. 895 */ 896 public HealthcareServiceNotAvailableComponent setDescription(String value) { 897 if (this.description == null) 898 this.description = new StringType(); 899 this.description.setValue(value); 900 return this; 901 } 902 903 /** 904 * @return {@link #during} (Service is not available (seasonally or for a public 905 * holiday) from this date.) 906 */ 907 public Period getDuring() { 908 if (this.during == null) 909 if (Configuration.errorOnAutoCreate()) 910 throw new Error("Attempt to auto-create HealthcareServiceNotAvailableComponent.during"); 911 else if (Configuration.doAutoCreate()) 912 this.during = new Period(); // cc 913 return this.during; 914 } 915 916 public boolean hasDuring() { 917 return this.during != null && !this.during.isEmpty(); 918 } 919 920 /** 921 * @param value {@link #during} (Service is not available (seasonally or for a 922 * public holiday) from this date.) 923 */ 924 public HealthcareServiceNotAvailableComponent setDuring(Period value) { 925 this.during = value; 926 return this; 927 } 928 929 protected void listChildren(List<Property> childrenList) { 930 super.listChildren(childrenList); 931 childrenList.add(new Property("description", "string", 932 "The reason that can be presented to the user as to why this time is not available.", 0, 933 java.lang.Integer.MAX_VALUE, description)); 934 childrenList.add(new Property("during", "Period", 935 "Service is not available (seasonally or for a public holiday) from this date.", 0, 936 java.lang.Integer.MAX_VALUE, during)); 937 } 938 939 @Override 940 public void setProperty(String name, Base value) throws FHIRException { 941 if (name.equals("description")) 942 this.description = castToString(value); // StringType 943 else if (name.equals("during")) 944 this.during = castToPeriod(value); // Period 945 else 946 super.setProperty(name, value); 947 } 948 949 @Override 950 public Base addChild(String name) throws FHIRException { 951 if (name.equals("description")) { 952 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.description"); 953 } else if (name.equals("during")) { 954 this.during = new Period(); 955 return this.during; 956 } else 957 return super.addChild(name); 958 } 959 960 public HealthcareServiceNotAvailableComponent copy() { 961 HealthcareServiceNotAvailableComponent dst = new HealthcareServiceNotAvailableComponent(); 962 copyValues(dst); 963 dst.description = description == null ? null : description.copy(); 964 dst.during = during == null ? null : during.copy(); 965 return dst; 966 } 967 968 @Override 969 public boolean equalsDeep(Base other) { 970 if (!super.equalsDeep(other)) 971 return false; 972 if (!(other instanceof HealthcareServiceNotAvailableComponent)) 973 return false; 974 HealthcareServiceNotAvailableComponent o = (HealthcareServiceNotAvailableComponent) other; 975 return compareDeep(description, o.description, true) && compareDeep(during, o.during, true); 976 } 977 978 @Override 979 public boolean equalsShallow(Base other) { 980 if (!super.equalsShallow(other)) 981 return false; 982 if (!(other instanceof HealthcareServiceNotAvailableComponent)) 983 return false; 984 HealthcareServiceNotAvailableComponent o = (HealthcareServiceNotAvailableComponent) other; 985 return compareValues(description, o.description, true); 986 } 987 988 public boolean isEmpty() { 989 return super.isEmpty() && (description == null || description.isEmpty()) && (during == null || during.isEmpty()); 990 } 991 992 public String fhirType() { 993 return "HealthcareService.notAvailable"; 994 995 } 996 997 } 998 999 /** 1000 * External identifiers for this item. 1001 */ 1002 @Child(name = "identifier", type = { 1003 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1004 @Description(shortDefinition = "External identifiers for this item", formalDefinition = "External identifiers for this item.") 1005 protected List<Identifier> identifier; 1006 1007 /** 1008 * The organization that provides this healthcare service. 1009 */ 1010 @Child(name = "providedBy", type = { 1011 Organization.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1012 @Description(shortDefinition = "Organization that provides this service", formalDefinition = "The organization that provides this healthcare service.") 1013 protected Reference providedBy; 1014 1015 /** 1016 * The actual object that is the target of the reference (The organization that 1017 * provides this healthcare service.) 1018 */ 1019 protected Organization providedByTarget; 1020 1021 /** 1022 * Identifies the broad category of service being performed or delivered. 1023 */ 1024 @Child(name = "serviceCategory", type = { 1025 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1026 @Description(shortDefinition = "Broad category of service being performed or delivered", formalDefinition = "Identifies the broad category of service being performed or delivered.") 1027 protected CodeableConcept serviceCategory; 1028 1029 /** 1030 * A specific type of service that may be delivered or performed. 1031 */ 1032 @Child(name = "serviceType", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1033 @Description(shortDefinition = "Specific service delivered or performed", formalDefinition = "A specific type of service that may be delivered or performed.") 1034 protected List<ServiceTypeComponent> serviceType; 1035 1036 /** 1037 * The location where this healthcare service may be provided. 1038 */ 1039 @Child(name = "location", type = { Location.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 1040 @Description(shortDefinition = "Location where service may be provided", formalDefinition = "The location where this healthcare service may be provided.") 1041 protected Reference location; 1042 1043 /** 1044 * The actual object that is the target of the reference (The location where 1045 * this healthcare service may be provided.) 1046 */ 1047 protected Location locationTarget; 1048 1049 /** 1050 * Further description of the service as it would be presented to a consumer 1051 * while searching. 1052 */ 1053 @Child(name = "serviceName", type = { 1054 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1055 @Description(shortDefinition = "Description of service as presented to a consumer while searching", formalDefinition = "Further description of the service as it would be presented to a consumer while searching.") 1056 protected StringType serviceName; 1057 1058 /** 1059 * Any additional description of the service and/or any specific issues not 1060 * covered by the other attributes, which can be displayed as further detail 1061 * under the serviceName. 1062 */ 1063 @Child(name = "comment", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1064 @Description(shortDefinition = "Additional description and/or any specific issues not covered elsewhere", formalDefinition = "Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName.") 1065 protected StringType comment; 1066 1067 /** 1068 * Extra details about the service that can't be placed in the other fields. 1069 */ 1070 @Child(name = "extraDetails", type = { 1071 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1072 @Description(shortDefinition = "Extra details about the service that can't be placed in the other fields", formalDefinition = "Extra details about the service that can't be placed in the other fields.") 1073 protected StringType extraDetails; 1074 1075 /** 1076 * If there is a photo/symbol associated with this HealthcareService, it may be 1077 * included here to facilitate quick identification of the service in a list. 1078 */ 1079 @Child(name = "photo", type = { Attachment.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1080 @Description(shortDefinition = "Facilitates quick identification of the service", formalDefinition = "If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list.") 1081 protected Attachment photo; 1082 1083 /** 1084 * List of contacts related to this specific healthcare service. 1085 */ 1086 @Child(name = "telecom", type = { 1087 ContactPoint.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1088 @Description(shortDefinition = "Contacts related to the healthcare service", formalDefinition = "List of contacts related to this specific healthcare service.") 1089 protected List<ContactPoint> telecom; 1090 1091 /** 1092 * The location(s) that this service is available to (not where the service is 1093 * provided). 1094 */ 1095 @Child(name = "coverageArea", type = { 1096 Location.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1097 @Description(shortDefinition = "Location(s) service is inteded for/available to", formalDefinition = "The location(s) that this service is available to (not where the service is provided).") 1098 protected List<Reference> coverageArea; 1099 /** 1100 * The actual objects that are the target of the reference (The location(s) that 1101 * this service is available to (not where the service is provided).) 1102 */ 1103 protected List<Location> coverageAreaTarget; 1104 1105 /** 1106 * The code(s) that detail the conditions under which the healthcare service is 1107 * available/offered. 1108 */ 1109 @Child(name = "serviceProvisionCode", type = { 1110 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1111 @Description(shortDefinition = "Conditions under which service is available/offered", formalDefinition = "The code(s) that detail the conditions under which the healthcare service is available/offered.") 1112 protected List<CodeableConcept> serviceProvisionCode; 1113 1114 /** 1115 * Does this service have specific eligibility requirements that need to be met 1116 * in order to use the service? 1117 */ 1118 @Child(name = "eligibility", type = { 1119 CodeableConcept.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1120 @Description(shortDefinition = "Specific eligibility requirements required to use the service", formalDefinition = "Does this service have specific eligibility requirements that need to be met in order to use the service?") 1121 protected CodeableConcept eligibility; 1122 1123 /** 1124 * Describes the eligibility conditions for the service. 1125 */ 1126 @Child(name = "eligibilityNote", type = { 1127 StringType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1128 @Description(shortDefinition = "Describes the eligibility conditions for the service", formalDefinition = "Describes the eligibility conditions for the service.") 1129 protected StringType eligibilityNote; 1130 1131 /** 1132 * Program Names that can be used to categorize the service. 1133 */ 1134 @Child(name = "programName", type = { 1135 StringType.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1136 @Description(shortDefinition = "Program Names that categorize the service", formalDefinition = "Program Names that can be used to categorize the service.") 1137 protected List<StringType> programName; 1138 1139 /** 1140 * Collection of characteristics (attributes). 1141 */ 1142 @Child(name = "characteristic", type = { 1143 CodeableConcept.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1144 @Description(shortDefinition = "Collection of characteristics (attributes)", formalDefinition = "Collection of characteristics (attributes).") 1145 protected List<CodeableConcept> characteristic; 1146 1147 /** 1148 * Ways that the service accepts referrals, if this is not provided then it is 1149 * implied that no referral is required. 1150 */ 1151 @Child(name = "referralMethod", type = { 1152 CodeableConcept.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1153 @Description(shortDefinition = "Ways that the service accepts referrals", formalDefinition = "Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required.") 1154 protected List<CodeableConcept> referralMethod; 1155 1156 /** 1157 * The public part of the 'keys' allocated to an Organization by an accredited 1158 * body to support secure exchange of data over the internet. To be provided by 1159 * the Organization, where available. 1160 */ 1161 @Child(name = "publicKey", type = { 1162 StringType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 1163 @Description(shortDefinition = "PKI Public keys to support secure communications", formalDefinition = "The public part of the 'keys' allocated to an Organization by an accredited body to support secure exchange of data over the internet. To be provided by the Organization, where available.") 1164 protected StringType publicKey; 1165 1166 /** 1167 * Indicates whether or not a prospective consumer will require an appointment 1168 * for a particular service at a site to be provided by the Organization. 1169 * Indicates if an appointment is required for access to this service. 1170 */ 1171 @Child(name = "appointmentRequired", type = { 1172 BooleanType.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 1173 @Description(shortDefinition = "If an appointment is required for access to this service", formalDefinition = "Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service.") 1174 protected BooleanType appointmentRequired; 1175 1176 /** 1177 * A collection of times that the Service Site is available. 1178 */ 1179 @Child(name = "availableTime", type = {}, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1180 @Description(shortDefinition = "Times the Service Site is available", formalDefinition = "A collection of times that the Service Site is available.") 1181 protected List<HealthcareServiceAvailableTimeComponent> availableTime; 1182 1183 /** 1184 * The HealthcareService is not available during this period of time due to the 1185 * provided reason. 1186 */ 1187 @Child(name = "notAvailable", type = {}, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1188 @Description(shortDefinition = "Not available during this time due to provided reason", formalDefinition = "The HealthcareService is not available during this period of time due to the provided reason.") 1189 protected List<HealthcareServiceNotAvailableComponent> notAvailable; 1190 1191 /** 1192 * A description of site availability exceptions, e.g. public holiday 1193 * availability. Succinctly describing all possible exceptions to normal site 1194 * availability as details in the available Times and not available Times. 1195 */ 1196 @Child(name = "availabilityExceptions", type = { 1197 StringType.class }, order = 21, min = 0, max = 1, modifier = false, summary = false) 1198 @Description(shortDefinition = "Description of availability exceptions", formalDefinition = "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.") 1199 protected StringType availabilityExceptions; 1200 1201 private static final long serialVersionUID = 683771126L; 1202 1203 /* 1204 * Constructor 1205 */ 1206 public HealthcareService() { 1207 super(); 1208 } 1209 1210 /* 1211 * Constructor 1212 */ 1213 public HealthcareService(Reference location) { 1214 super(); 1215 this.location = location; 1216 } 1217 1218 /** 1219 * @return {@link #identifier} (External identifiers for this item.) 1220 */ 1221 public List<Identifier> getIdentifier() { 1222 if (this.identifier == null) 1223 this.identifier = new ArrayList<Identifier>(); 1224 return this.identifier; 1225 } 1226 1227 public boolean hasIdentifier() { 1228 if (this.identifier == null) 1229 return false; 1230 for (Identifier item : this.identifier) 1231 if (!item.isEmpty()) 1232 return true; 1233 return false; 1234 } 1235 1236 /** 1237 * @return {@link #identifier} (External identifiers for this item.) 1238 */ 1239 // syntactic sugar 1240 public Identifier addIdentifier() { // 3 1241 Identifier t = new Identifier(); 1242 if (this.identifier == null) 1243 this.identifier = new ArrayList<Identifier>(); 1244 this.identifier.add(t); 1245 return t; 1246 } 1247 1248 // syntactic sugar 1249 public HealthcareService addIdentifier(Identifier t) { // 3 1250 if (t == null) 1251 return this; 1252 if (this.identifier == null) 1253 this.identifier = new ArrayList<Identifier>(); 1254 this.identifier.add(t); 1255 return this; 1256 } 1257 1258 /** 1259 * @return {@link #providedBy} (The organization that provides this healthcare 1260 * service.) 1261 */ 1262 public Reference getProvidedBy() { 1263 if (this.providedBy == null) 1264 if (Configuration.errorOnAutoCreate()) 1265 throw new Error("Attempt to auto-create HealthcareService.providedBy"); 1266 else if (Configuration.doAutoCreate()) 1267 this.providedBy = new Reference(); // cc 1268 return this.providedBy; 1269 } 1270 1271 public boolean hasProvidedBy() { 1272 return this.providedBy != null && !this.providedBy.isEmpty(); 1273 } 1274 1275 /** 1276 * @param value {@link #providedBy} (The organization that provides this 1277 * healthcare service.) 1278 */ 1279 public HealthcareService setProvidedBy(Reference value) { 1280 this.providedBy = value; 1281 return this; 1282 } 1283 1284 /** 1285 * @return {@link #providedBy} The actual object that is the target of the 1286 * reference. The reference library doesn't populate this, but you can 1287 * use it to hold the resource if you resolve it. (The organization that 1288 * provides this healthcare service.) 1289 */ 1290 public Organization getProvidedByTarget() { 1291 if (this.providedByTarget == null) 1292 if (Configuration.errorOnAutoCreate()) 1293 throw new Error("Attempt to auto-create HealthcareService.providedBy"); 1294 else if (Configuration.doAutoCreate()) 1295 this.providedByTarget = new Organization(); // aa 1296 return this.providedByTarget; 1297 } 1298 1299 /** 1300 * @param value {@link #providedBy} The actual object that is the target of the 1301 * reference. The reference library doesn't use these, but you can 1302 * use it to hold the resource if you resolve it. (The organization 1303 * that provides this healthcare service.) 1304 */ 1305 public HealthcareService setProvidedByTarget(Organization value) { 1306 this.providedByTarget = value; 1307 return this; 1308 } 1309 1310 /** 1311 * @return {@link #serviceCategory} (Identifies the broad category of service 1312 * being performed or delivered.) 1313 */ 1314 public CodeableConcept getServiceCategory() { 1315 if (this.serviceCategory == null) 1316 if (Configuration.errorOnAutoCreate()) 1317 throw new Error("Attempt to auto-create HealthcareService.serviceCategory"); 1318 else if (Configuration.doAutoCreate()) 1319 this.serviceCategory = new CodeableConcept(); // cc 1320 return this.serviceCategory; 1321 } 1322 1323 public boolean hasServiceCategory() { 1324 return this.serviceCategory != null && !this.serviceCategory.isEmpty(); 1325 } 1326 1327 /** 1328 * @param value {@link #serviceCategory} (Identifies the broad category of 1329 * service being performed or delivered.) 1330 */ 1331 public HealthcareService setServiceCategory(CodeableConcept value) { 1332 this.serviceCategory = value; 1333 return this; 1334 } 1335 1336 /** 1337 * @return {@link #serviceType} (A specific type of service that may be 1338 * delivered or performed.) 1339 */ 1340 public List<ServiceTypeComponent> getServiceType() { 1341 if (this.serviceType == null) 1342 this.serviceType = new ArrayList<ServiceTypeComponent>(); 1343 return this.serviceType; 1344 } 1345 1346 public boolean hasServiceType() { 1347 if (this.serviceType == null) 1348 return false; 1349 for (ServiceTypeComponent item : this.serviceType) 1350 if (!item.isEmpty()) 1351 return true; 1352 return false; 1353 } 1354 1355 /** 1356 * @return {@link #serviceType} (A specific type of service that may be 1357 * delivered or performed.) 1358 */ 1359 // syntactic sugar 1360 public ServiceTypeComponent addServiceType() { // 3 1361 ServiceTypeComponent t = new ServiceTypeComponent(); 1362 if (this.serviceType == null) 1363 this.serviceType = new ArrayList<ServiceTypeComponent>(); 1364 this.serviceType.add(t); 1365 return t; 1366 } 1367 1368 // syntactic sugar 1369 public HealthcareService addServiceType(ServiceTypeComponent t) { // 3 1370 if (t == null) 1371 return this; 1372 if (this.serviceType == null) 1373 this.serviceType = new ArrayList<ServiceTypeComponent>(); 1374 this.serviceType.add(t); 1375 return this; 1376 } 1377 1378 /** 1379 * @return {@link #location} (The location where this healthcare service may be 1380 * provided.) 1381 */ 1382 public Reference getLocation() { 1383 if (this.location == null) 1384 if (Configuration.errorOnAutoCreate()) 1385 throw new Error("Attempt to auto-create HealthcareService.location"); 1386 else if (Configuration.doAutoCreate()) 1387 this.location = new Reference(); // cc 1388 return this.location; 1389 } 1390 1391 public boolean hasLocation() { 1392 return this.location != null && !this.location.isEmpty(); 1393 } 1394 1395 /** 1396 * @param value {@link #location} (The location where this healthcare service 1397 * may be provided.) 1398 */ 1399 public HealthcareService setLocation(Reference value) { 1400 this.location = value; 1401 return this; 1402 } 1403 1404 /** 1405 * @return {@link #location} The actual object that is the target of the 1406 * reference. The reference library doesn't populate this, but you can 1407 * use it to hold the resource if you resolve it. (The location where 1408 * this healthcare service may be provided.) 1409 */ 1410 public Location getLocationTarget() { 1411 if (this.locationTarget == null) 1412 if (Configuration.errorOnAutoCreate()) 1413 throw new Error("Attempt to auto-create HealthcareService.location"); 1414 else if (Configuration.doAutoCreate()) 1415 this.locationTarget = new Location(); // aa 1416 return this.locationTarget; 1417 } 1418 1419 /** 1420 * @param value {@link #location} The actual object that is the target of the 1421 * reference. The reference library doesn't use these, but you can 1422 * use it to hold the resource if you resolve it. (The location 1423 * where this healthcare service may be provided.) 1424 */ 1425 public HealthcareService setLocationTarget(Location value) { 1426 this.locationTarget = value; 1427 return this; 1428 } 1429 1430 /** 1431 * @return {@link #serviceName} (Further description of the service as it would 1432 * be presented to a consumer while searching.). This is the underlying 1433 * object with id, value and extensions. The accessor "getServiceName" 1434 * gives direct access to the value 1435 */ 1436 public StringType getServiceNameElement() { 1437 if (this.serviceName == null) 1438 if (Configuration.errorOnAutoCreate()) 1439 throw new Error("Attempt to auto-create HealthcareService.serviceName"); 1440 else if (Configuration.doAutoCreate()) 1441 this.serviceName = new StringType(); // bb 1442 return this.serviceName; 1443 } 1444 1445 public boolean hasServiceNameElement() { 1446 return this.serviceName != null && !this.serviceName.isEmpty(); 1447 } 1448 1449 public boolean hasServiceName() { 1450 return this.serviceName != null && !this.serviceName.isEmpty(); 1451 } 1452 1453 /** 1454 * @param value {@link #serviceName} (Further description of the service as it 1455 * would be presented to a consumer while searching.). This is the 1456 * underlying object with id, value and extensions. The accessor 1457 * "getServiceName" gives direct access to the value 1458 */ 1459 public HealthcareService setServiceNameElement(StringType value) { 1460 this.serviceName = value; 1461 return this; 1462 } 1463 1464 /** 1465 * @return Further description of the service as it would be presented to a 1466 * consumer while searching. 1467 */ 1468 public String getServiceName() { 1469 return this.serviceName == null ? null : this.serviceName.getValue(); 1470 } 1471 1472 /** 1473 * @param value Further description of the service as it would be presented to a 1474 * consumer while searching. 1475 */ 1476 public HealthcareService setServiceName(String value) { 1477 if (Utilities.noString(value)) 1478 this.serviceName = null; 1479 else { 1480 if (this.serviceName == null) 1481 this.serviceName = new StringType(); 1482 this.serviceName.setValue(value); 1483 } 1484 return this; 1485 } 1486 1487 /** 1488 * @return {@link #comment} (Any additional description of the service and/or 1489 * any specific issues not covered by the other attributes, which can be 1490 * displayed as further detail under the serviceName.). This is the 1491 * underlying object with id, value and extensions. The accessor 1492 * "getComment" gives direct access to the value 1493 */ 1494 public StringType getCommentElement() { 1495 if (this.comment == null) 1496 if (Configuration.errorOnAutoCreate()) 1497 throw new Error("Attempt to auto-create HealthcareService.comment"); 1498 else if (Configuration.doAutoCreate()) 1499 this.comment = new StringType(); // bb 1500 return this.comment; 1501 } 1502 1503 public boolean hasCommentElement() { 1504 return this.comment != null && !this.comment.isEmpty(); 1505 } 1506 1507 public boolean hasComment() { 1508 return this.comment != null && !this.comment.isEmpty(); 1509 } 1510 1511 /** 1512 * @param value {@link #comment} (Any additional description of the service 1513 * and/or any specific issues not covered by the other attributes, 1514 * which can be displayed as further detail under the 1515 * serviceName.). This is the underlying object with id, value and 1516 * extensions. The accessor "getComment" gives direct access to the 1517 * value 1518 */ 1519 public HealthcareService setCommentElement(StringType value) { 1520 this.comment = value; 1521 return this; 1522 } 1523 1524 /** 1525 * @return Any additional description of the service and/or any specific issues 1526 * not covered by the other attributes, which can be displayed as 1527 * further detail under the serviceName. 1528 */ 1529 public String getComment() { 1530 return this.comment == null ? null : this.comment.getValue(); 1531 } 1532 1533 /** 1534 * @param value Any additional description of the service and/or any specific 1535 * issues not covered by the other attributes, which can be 1536 * displayed as further detail under the serviceName. 1537 */ 1538 public HealthcareService setComment(String value) { 1539 if (Utilities.noString(value)) 1540 this.comment = null; 1541 else { 1542 if (this.comment == null) 1543 this.comment = new StringType(); 1544 this.comment.setValue(value); 1545 } 1546 return this; 1547 } 1548 1549 /** 1550 * @return {@link #extraDetails} (Extra details about the service that can't be 1551 * placed in the other fields.). This is the underlying object with id, 1552 * value and extensions. The accessor "getExtraDetails" gives direct 1553 * access to the value 1554 */ 1555 public StringType getExtraDetailsElement() { 1556 if (this.extraDetails == null) 1557 if (Configuration.errorOnAutoCreate()) 1558 throw new Error("Attempt to auto-create HealthcareService.extraDetails"); 1559 else if (Configuration.doAutoCreate()) 1560 this.extraDetails = new StringType(); // bb 1561 return this.extraDetails; 1562 } 1563 1564 public boolean hasExtraDetailsElement() { 1565 return this.extraDetails != null && !this.extraDetails.isEmpty(); 1566 } 1567 1568 public boolean hasExtraDetails() { 1569 return this.extraDetails != null && !this.extraDetails.isEmpty(); 1570 } 1571 1572 /** 1573 * @param value {@link #extraDetails} (Extra details about the service that 1574 * can't be placed in the other fields.). This is the underlying 1575 * object with id, value and extensions. The accessor 1576 * "getExtraDetails" gives direct access to the value 1577 */ 1578 public HealthcareService setExtraDetailsElement(StringType value) { 1579 this.extraDetails = value; 1580 return this; 1581 } 1582 1583 /** 1584 * @return Extra details about the service that can't be placed in the other 1585 * fields. 1586 */ 1587 public String getExtraDetails() { 1588 return this.extraDetails == null ? null : this.extraDetails.getValue(); 1589 } 1590 1591 /** 1592 * @param value Extra details about the service that can't be placed in the 1593 * other fields. 1594 */ 1595 public HealthcareService setExtraDetails(String value) { 1596 if (Utilities.noString(value)) 1597 this.extraDetails = null; 1598 else { 1599 if (this.extraDetails == null) 1600 this.extraDetails = new StringType(); 1601 this.extraDetails.setValue(value); 1602 } 1603 return this; 1604 } 1605 1606 /** 1607 * @return {@link #photo} (If there is a photo/symbol associated with this 1608 * HealthcareService, it may be included here to facilitate quick 1609 * identification of the service in a list.) 1610 */ 1611 public Attachment getPhoto() { 1612 if (this.photo == null) 1613 if (Configuration.errorOnAutoCreate()) 1614 throw new Error("Attempt to auto-create HealthcareService.photo"); 1615 else if (Configuration.doAutoCreate()) 1616 this.photo = new Attachment(); // cc 1617 return this.photo; 1618 } 1619 1620 public boolean hasPhoto() { 1621 return this.photo != null && !this.photo.isEmpty(); 1622 } 1623 1624 /** 1625 * @param value {@link #photo} (If there is a photo/symbol associated with this 1626 * HealthcareService, it may be included here to facilitate quick 1627 * identification of the service in a list.) 1628 */ 1629 public HealthcareService setPhoto(Attachment value) { 1630 this.photo = value; 1631 return this; 1632 } 1633 1634 /** 1635 * @return {@link #telecom} (List of contacts related to this specific 1636 * healthcare service.) 1637 */ 1638 public List<ContactPoint> getTelecom() { 1639 if (this.telecom == null) 1640 this.telecom = new ArrayList<ContactPoint>(); 1641 return this.telecom; 1642 } 1643 1644 public boolean hasTelecom() { 1645 if (this.telecom == null) 1646 return false; 1647 for (ContactPoint item : this.telecom) 1648 if (!item.isEmpty()) 1649 return true; 1650 return false; 1651 } 1652 1653 /** 1654 * @return {@link #telecom} (List of contacts related to this specific 1655 * healthcare service.) 1656 */ 1657 // syntactic sugar 1658 public ContactPoint addTelecom() { // 3 1659 ContactPoint t = new ContactPoint(); 1660 if (this.telecom == null) 1661 this.telecom = new ArrayList<ContactPoint>(); 1662 this.telecom.add(t); 1663 return t; 1664 } 1665 1666 // syntactic sugar 1667 public HealthcareService addTelecom(ContactPoint t) { // 3 1668 if (t == null) 1669 return this; 1670 if (this.telecom == null) 1671 this.telecom = new ArrayList<ContactPoint>(); 1672 this.telecom.add(t); 1673 return this; 1674 } 1675 1676 /** 1677 * @return {@link #coverageArea} (The location(s) that this service is available 1678 * to (not where the service is provided).) 1679 */ 1680 public List<Reference> getCoverageArea() { 1681 if (this.coverageArea == null) 1682 this.coverageArea = new ArrayList<Reference>(); 1683 return this.coverageArea; 1684 } 1685 1686 public boolean hasCoverageArea() { 1687 if (this.coverageArea == null) 1688 return false; 1689 for (Reference item : this.coverageArea) 1690 if (!item.isEmpty()) 1691 return true; 1692 return false; 1693 } 1694 1695 /** 1696 * @return {@link #coverageArea} (The location(s) that this service is available 1697 * to (not where the service is provided).) 1698 */ 1699 // syntactic sugar 1700 public Reference addCoverageArea() { // 3 1701 Reference t = new Reference(); 1702 if (this.coverageArea == null) 1703 this.coverageArea = new ArrayList<Reference>(); 1704 this.coverageArea.add(t); 1705 return t; 1706 } 1707 1708 // syntactic sugar 1709 public HealthcareService addCoverageArea(Reference t) { // 3 1710 if (t == null) 1711 return this; 1712 if (this.coverageArea == null) 1713 this.coverageArea = new ArrayList<Reference>(); 1714 this.coverageArea.add(t); 1715 return this; 1716 } 1717 1718 /** 1719 * @return {@link #coverageArea} (The actual objects that are the target of the 1720 * reference. The reference library doesn't populate this, but you can 1721 * use this to hold the resources if you resolvethemt. The location(s) 1722 * that this service is available to (not where the service is 1723 * provided).) 1724 */ 1725 public List<Location> getCoverageAreaTarget() { 1726 if (this.coverageAreaTarget == null) 1727 this.coverageAreaTarget = new ArrayList<Location>(); 1728 return this.coverageAreaTarget; 1729 } 1730 1731 // syntactic sugar 1732 /** 1733 * @return {@link #coverageArea} (Add an actual object that is the target of the 1734 * reference. The reference library doesn't use these, but you can use 1735 * this to hold the resources if you resolvethemt. The location(s) that 1736 * this service is available to (not where the service is provided).) 1737 */ 1738 public Location addCoverageAreaTarget() { 1739 Location r = new Location(); 1740 if (this.coverageAreaTarget == null) 1741 this.coverageAreaTarget = new ArrayList<Location>(); 1742 this.coverageAreaTarget.add(r); 1743 return r; 1744 } 1745 1746 /** 1747 * @return {@link #serviceProvisionCode} (The code(s) that detail the conditions 1748 * under which the healthcare service is available/offered.) 1749 */ 1750 public List<CodeableConcept> getServiceProvisionCode() { 1751 if (this.serviceProvisionCode == null) 1752 this.serviceProvisionCode = new ArrayList<CodeableConcept>(); 1753 return this.serviceProvisionCode; 1754 } 1755 1756 public boolean hasServiceProvisionCode() { 1757 if (this.serviceProvisionCode == null) 1758 return false; 1759 for (CodeableConcept item : this.serviceProvisionCode) 1760 if (!item.isEmpty()) 1761 return true; 1762 return false; 1763 } 1764 1765 /** 1766 * @return {@link #serviceProvisionCode} (The code(s) that detail the conditions 1767 * under which the healthcare service is available/offered.) 1768 */ 1769 // syntactic sugar 1770 public CodeableConcept addServiceProvisionCode() { // 3 1771 CodeableConcept t = new CodeableConcept(); 1772 if (this.serviceProvisionCode == null) 1773 this.serviceProvisionCode = new ArrayList<CodeableConcept>(); 1774 this.serviceProvisionCode.add(t); 1775 return t; 1776 } 1777 1778 // syntactic sugar 1779 public HealthcareService addServiceProvisionCode(CodeableConcept t) { // 3 1780 if (t == null) 1781 return this; 1782 if (this.serviceProvisionCode == null) 1783 this.serviceProvisionCode = new ArrayList<CodeableConcept>(); 1784 this.serviceProvisionCode.add(t); 1785 return this; 1786 } 1787 1788 /** 1789 * @return {@link #eligibility} (Does this service have specific eligibility 1790 * requirements that need to be met in order to use the service?) 1791 */ 1792 public CodeableConcept getEligibility() { 1793 if (this.eligibility == null) 1794 if (Configuration.errorOnAutoCreate()) 1795 throw new Error("Attempt to auto-create HealthcareService.eligibility"); 1796 else if (Configuration.doAutoCreate()) 1797 this.eligibility = new CodeableConcept(); // cc 1798 return this.eligibility; 1799 } 1800 1801 public boolean hasEligibility() { 1802 return this.eligibility != null && !this.eligibility.isEmpty(); 1803 } 1804 1805 /** 1806 * @param value {@link #eligibility} (Does this service have specific 1807 * eligibility requirements that need to be met in order to use the 1808 * service?) 1809 */ 1810 public HealthcareService setEligibility(CodeableConcept value) { 1811 this.eligibility = value; 1812 return this; 1813 } 1814 1815 /** 1816 * @return {@link #eligibilityNote} (Describes the eligibility conditions for 1817 * the service.). This is the underlying object with id, value and 1818 * extensions. The accessor "getEligibilityNote" gives direct access to 1819 * the value 1820 */ 1821 public StringType getEligibilityNoteElement() { 1822 if (this.eligibilityNote == null) 1823 if (Configuration.errorOnAutoCreate()) 1824 throw new Error("Attempt to auto-create HealthcareService.eligibilityNote"); 1825 else if (Configuration.doAutoCreate()) 1826 this.eligibilityNote = new StringType(); // bb 1827 return this.eligibilityNote; 1828 } 1829 1830 public boolean hasEligibilityNoteElement() { 1831 return this.eligibilityNote != null && !this.eligibilityNote.isEmpty(); 1832 } 1833 1834 public boolean hasEligibilityNote() { 1835 return this.eligibilityNote != null && !this.eligibilityNote.isEmpty(); 1836 } 1837 1838 /** 1839 * @param value {@link #eligibilityNote} (Describes the eligibility conditions 1840 * for the service.). This is the underlying object with id, value 1841 * and extensions. The accessor "getEligibilityNote" gives direct 1842 * access to the value 1843 */ 1844 public HealthcareService setEligibilityNoteElement(StringType value) { 1845 this.eligibilityNote = value; 1846 return this; 1847 } 1848 1849 /** 1850 * @return Describes the eligibility conditions for the service. 1851 */ 1852 public String getEligibilityNote() { 1853 return this.eligibilityNote == null ? null : this.eligibilityNote.getValue(); 1854 } 1855 1856 /** 1857 * @param value Describes the eligibility conditions for the service. 1858 */ 1859 public HealthcareService setEligibilityNote(String value) { 1860 if (Utilities.noString(value)) 1861 this.eligibilityNote = null; 1862 else { 1863 if (this.eligibilityNote == null) 1864 this.eligibilityNote = new StringType(); 1865 this.eligibilityNote.setValue(value); 1866 } 1867 return this; 1868 } 1869 1870 /** 1871 * @return {@link #programName} (Program Names that can be used to categorize 1872 * the service.) 1873 */ 1874 public List<StringType> getProgramName() { 1875 if (this.programName == null) 1876 this.programName = new ArrayList<StringType>(); 1877 return this.programName; 1878 } 1879 1880 public boolean hasProgramName() { 1881 if (this.programName == null) 1882 return false; 1883 for (StringType item : this.programName) 1884 if (!item.isEmpty()) 1885 return true; 1886 return false; 1887 } 1888 1889 /** 1890 * @return {@link #programName} (Program Names that can be used to categorize 1891 * the service.) 1892 */ 1893 // syntactic sugar 1894 public StringType addProgramNameElement() {// 2 1895 StringType t = new StringType(); 1896 if (this.programName == null) 1897 this.programName = new ArrayList<StringType>(); 1898 this.programName.add(t); 1899 return t; 1900 } 1901 1902 /** 1903 * @param value {@link #programName} (Program Names that can be used to 1904 * categorize the service.) 1905 */ 1906 public HealthcareService addProgramName(String value) { // 1 1907 StringType t = new StringType(); 1908 t.setValue(value); 1909 if (this.programName == null) 1910 this.programName = new ArrayList<StringType>(); 1911 this.programName.add(t); 1912 return this; 1913 } 1914 1915 /** 1916 * @param value {@link #programName} (Program Names that can be used to 1917 * categorize the service.) 1918 */ 1919 public boolean hasProgramName(String value) { 1920 if (this.programName == null) 1921 return false; 1922 for (StringType v : this.programName) 1923 if (v.equals(value)) // string 1924 return true; 1925 return false; 1926 } 1927 1928 /** 1929 * @return {@link #characteristic} (Collection of characteristics (attributes).) 1930 */ 1931 public List<CodeableConcept> getCharacteristic() { 1932 if (this.characteristic == null) 1933 this.characteristic = new ArrayList<CodeableConcept>(); 1934 return this.characteristic; 1935 } 1936 1937 public boolean hasCharacteristic() { 1938 if (this.characteristic == null) 1939 return false; 1940 for (CodeableConcept item : this.characteristic) 1941 if (!item.isEmpty()) 1942 return true; 1943 return false; 1944 } 1945 1946 /** 1947 * @return {@link #characteristic} (Collection of characteristics (attributes).) 1948 */ 1949 // syntactic sugar 1950 public CodeableConcept addCharacteristic() { // 3 1951 CodeableConcept t = new CodeableConcept(); 1952 if (this.characteristic == null) 1953 this.characteristic = new ArrayList<CodeableConcept>(); 1954 this.characteristic.add(t); 1955 return t; 1956 } 1957 1958 // syntactic sugar 1959 public HealthcareService addCharacteristic(CodeableConcept t) { // 3 1960 if (t == null) 1961 return this; 1962 if (this.characteristic == null) 1963 this.characteristic = new ArrayList<CodeableConcept>(); 1964 this.characteristic.add(t); 1965 return this; 1966 } 1967 1968 /** 1969 * @return {@link #referralMethod} (Ways that the service accepts referrals, if 1970 * this is not provided then it is implied that no referral is 1971 * required.) 1972 */ 1973 public List<CodeableConcept> getReferralMethod() { 1974 if (this.referralMethod == null) 1975 this.referralMethod = new ArrayList<CodeableConcept>(); 1976 return this.referralMethod; 1977 } 1978 1979 public boolean hasReferralMethod() { 1980 if (this.referralMethod == null) 1981 return false; 1982 for (CodeableConcept item : this.referralMethod) 1983 if (!item.isEmpty()) 1984 return true; 1985 return false; 1986 } 1987 1988 /** 1989 * @return {@link #referralMethod} (Ways that the service accepts referrals, if 1990 * this is not provided then it is implied that no referral is 1991 * required.) 1992 */ 1993 // syntactic sugar 1994 public CodeableConcept addReferralMethod() { // 3 1995 CodeableConcept t = new CodeableConcept(); 1996 if (this.referralMethod == null) 1997 this.referralMethod = new ArrayList<CodeableConcept>(); 1998 this.referralMethod.add(t); 1999 return t; 2000 } 2001 2002 // syntactic sugar 2003 public HealthcareService addReferralMethod(CodeableConcept t) { // 3 2004 if (t == null) 2005 return this; 2006 if (this.referralMethod == null) 2007 this.referralMethod = new ArrayList<CodeableConcept>(); 2008 this.referralMethod.add(t); 2009 return this; 2010 } 2011 2012 /** 2013 * @return {@link #publicKey} (The public part of the 'keys' allocated to an 2014 * Organization by an accredited body to support secure exchange of data 2015 * over the internet. To be provided by the Organization, where 2016 * available.). This is the underlying object with id, value and 2017 * extensions. The accessor "getPublicKey" gives direct access to the 2018 * value 2019 */ 2020 public StringType getPublicKeyElement() { 2021 if (this.publicKey == null) 2022 if (Configuration.errorOnAutoCreate()) 2023 throw new Error("Attempt to auto-create HealthcareService.publicKey"); 2024 else if (Configuration.doAutoCreate()) 2025 this.publicKey = new StringType(); // bb 2026 return this.publicKey; 2027 } 2028 2029 public boolean hasPublicKeyElement() { 2030 return this.publicKey != null && !this.publicKey.isEmpty(); 2031 } 2032 2033 public boolean hasPublicKey() { 2034 return this.publicKey != null && !this.publicKey.isEmpty(); 2035 } 2036 2037 /** 2038 * @param value {@link #publicKey} (The public part of the 'keys' allocated to 2039 * an Organization by an accredited body to support secure exchange 2040 * of data over the internet. To be provided by the Organization, 2041 * where available.). This is the underlying object with id, value 2042 * and extensions. The accessor "getPublicKey" gives direct access 2043 * to the value 2044 */ 2045 public HealthcareService setPublicKeyElement(StringType value) { 2046 this.publicKey = value; 2047 return this; 2048 } 2049 2050 /** 2051 * @return The public part of the 'keys' allocated to an Organization by an 2052 * accredited body to support secure exchange of data over the internet. 2053 * To be provided by the Organization, where available. 2054 */ 2055 public String getPublicKey() { 2056 return this.publicKey == null ? null : this.publicKey.getValue(); 2057 } 2058 2059 /** 2060 * @param value The public part of the 'keys' allocated to an Organization by an 2061 * accredited body to support secure exchange of data over the 2062 * internet. To be provided by the Organization, where available. 2063 */ 2064 public HealthcareService setPublicKey(String value) { 2065 if (Utilities.noString(value)) 2066 this.publicKey = null; 2067 else { 2068 if (this.publicKey == null) 2069 this.publicKey = new StringType(); 2070 this.publicKey.setValue(value); 2071 } 2072 return this; 2073 } 2074 2075 /** 2076 * @return {@link #appointmentRequired} (Indicates whether or not a prospective 2077 * consumer will require an appointment for a particular service at a 2078 * site to be provided by the Organization. Indicates if an appointment 2079 * is required for access to this service.). This is the underlying 2080 * object with id, value and extensions. The accessor 2081 * "getAppointmentRequired" gives direct access to the value 2082 */ 2083 public BooleanType getAppointmentRequiredElement() { 2084 if (this.appointmentRequired == null) 2085 if (Configuration.errorOnAutoCreate()) 2086 throw new Error("Attempt to auto-create HealthcareService.appointmentRequired"); 2087 else if (Configuration.doAutoCreate()) 2088 this.appointmentRequired = new BooleanType(); // bb 2089 return this.appointmentRequired; 2090 } 2091 2092 public boolean hasAppointmentRequiredElement() { 2093 return this.appointmentRequired != null && !this.appointmentRequired.isEmpty(); 2094 } 2095 2096 public boolean hasAppointmentRequired() { 2097 return this.appointmentRequired != null && !this.appointmentRequired.isEmpty(); 2098 } 2099 2100 /** 2101 * @param value {@link #appointmentRequired} (Indicates whether or not a 2102 * prospective consumer will require an appointment for a 2103 * particular service at a site to be provided by the Organization. 2104 * Indicates if an appointment is required for access to this 2105 * service.). This is the underlying object with id, value and 2106 * extensions. The accessor "getAppointmentRequired" gives direct 2107 * access to the value 2108 */ 2109 public HealthcareService setAppointmentRequiredElement(BooleanType value) { 2110 this.appointmentRequired = value; 2111 return this; 2112 } 2113 2114 /** 2115 * @return Indicates whether or not a prospective consumer will require an 2116 * appointment for a particular service at a site to be provided by the 2117 * Organization. Indicates if an appointment is required for access to 2118 * this service. 2119 */ 2120 public boolean getAppointmentRequired() { 2121 return this.appointmentRequired == null || this.appointmentRequired.isEmpty() ? false 2122 : this.appointmentRequired.getValue(); 2123 } 2124 2125 /** 2126 * @param value Indicates whether or not a prospective consumer will require an 2127 * appointment for a particular service at a site to be provided by 2128 * the Organization. Indicates if an appointment is required for 2129 * access to this service. 2130 */ 2131 public HealthcareService setAppointmentRequired(boolean value) { 2132 if (this.appointmentRequired == null) 2133 this.appointmentRequired = new BooleanType(); 2134 this.appointmentRequired.setValue(value); 2135 return this; 2136 } 2137 2138 /** 2139 * @return {@link #availableTime} (A collection of times that the Service Site 2140 * is available.) 2141 */ 2142 public List<HealthcareServiceAvailableTimeComponent> getAvailableTime() { 2143 if (this.availableTime == null) 2144 this.availableTime = new ArrayList<HealthcareServiceAvailableTimeComponent>(); 2145 return this.availableTime; 2146 } 2147 2148 public boolean hasAvailableTime() { 2149 if (this.availableTime == null) 2150 return false; 2151 for (HealthcareServiceAvailableTimeComponent item : this.availableTime) 2152 if (!item.isEmpty()) 2153 return true; 2154 return false; 2155 } 2156 2157 /** 2158 * @return {@link #availableTime} (A collection of times that the Service Site 2159 * is available.) 2160 */ 2161 // syntactic sugar 2162 public HealthcareServiceAvailableTimeComponent addAvailableTime() { // 3 2163 HealthcareServiceAvailableTimeComponent t = new HealthcareServiceAvailableTimeComponent(); 2164 if (this.availableTime == null) 2165 this.availableTime = new ArrayList<HealthcareServiceAvailableTimeComponent>(); 2166 this.availableTime.add(t); 2167 return t; 2168 } 2169 2170 // syntactic sugar 2171 public HealthcareService addAvailableTime(HealthcareServiceAvailableTimeComponent t) { // 3 2172 if (t == null) 2173 return this; 2174 if (this.availableTime == null) 2175 this.availableTime = new ArrayList<HealthcareServiceAvailableTimeComponent>(); 2176 this.availableTime.add(t); 2177 return this; 2178 } 2179 2180 /** 2181 * @return {@link #notAvailable} (The HealthcareService is not available during 2182 * this period of time due to the provided reason.) 2183 */ 2184 public List<HealthcareServiceNotAvailableComponent> getNotAvailable() { 2185 if (this.notAvailable == null) 2186 this.notAvailable = new ArrayList<HealthcareServiceNotAvailableComponent>(); 2187 return this.notAvailable; 2188 } 2189 2190 public boolean hasNotAvailable() { 2191 if (this.notAvailable == null) 2192 return false; 2193 for (HealthcareServiceNotAvailableComponent item : this.notAvailable) 2194 if (!item.isEmpty()) 2195 return true; 2196 return false; 2197 } 2198 2199 /** 2200 * @return {@link #notAvailable} (The HealthcareService is not available during 2201 * this period of time due to the provided reason.) 2202 */ 2203 // syntactic sugar 2204 public HealthcareServiceNotAvailableComponent addNotAvailable() { // 3 2205 HealthcareServiceNotAvailableComponent t = new HealthcareServiceNotAvailableComponent(); 2206 if (this.notAvailable == null) 2207 this.notAvailable = new ArrayList<HealthcareServiceNotAvailableComponent>(); 2208 this.notAvailable.add(t); 2209 return t; 2210 } 2211 2212 // syntactic sugar 2213 public HealthcareService addNotAvailable(HealthcareServiceNotAvailableComponent t) { // 3 2214 if (t == null) 2215 return this; 2216 if (this.notAvailable == null) 2217 this.notAvailable = new ArrayList<HealthcareServiceNotAvailableComponent>(); 2218 this.notAvailable.add(t); 2219 return this; 2220 } 2221 2222 /** 2223 * @return {@link #availabilityExceptions} (A description of site availability 2224 * exceptions, e.g. public holiday availability. Succinctly describing 2225 * all possible exceptions to normal site availability as details in the 2226 * available Times and not available Times.). This is the underlying 2227 * object with id, value and extensions. The accessor 2228 * "getAvailabilityExceptions" gives direct access to the value 2229 */ 2230 public StringType getAvailabilityExceptionsElement() { 2231 if (this.availabilityExceptions == null) 2232 if (Configuration.errorOnAutoCreate()) 2233 throw new Error("Attempt to auto-create HealthcareService.availabilityExceptions"); 2234 else if (Configuration.doAutoCreate()) 2235 this.availabilityExceptions = new StringType(); // bb 2236 return this.availabilityExceptions; 2237 } 2238 2239 public boolean hasAvailabilityExceptionsElement() { 2240 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 2241 } 2242 2243 public boolean hasAvailabilityExceptions() { 2244 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 2245 } 2246 2247 /** 2248 * @param value {@link #availabilityExceptions} (A description of site 2249 * availability exceptions, e.g. public holiday availability. 2250 * Succinctly describing all possible exceptions to normal site 2251 * availability as details in the available Times and not available 2252 * Times.). This is the underlying object with id, value and 2253 * extensions. The accessor "getAvailabilityExceptions" gives 2254 * direct access to the value 2255 */ 2256 public HealthcareService setAvailabilityExceptionsElement(StringType value) { 2257 this.availabilityExceptions = value; 2258 return this; 2259 } 2260 2261 /** 2262 * @return A description of site availability exceptions, e.g. public holiday 2263 * availability. Succinctly describing all possible exceptions to normal 2264 * site availability as details in the available Times and not available 2265 * Times. 2266 */ 2267 public String getAvailabilityExceptions() { 2268 return this.availabilityExceptions == null ? null : this.availabilityExceptions.getValue(); 2269 } 2270 2271 /** 2272 * @param value A description of site availability exceptions, e.g. public 2273 * holiday availability. Succinctly describing all possible 2274 * exceptions to normal site availability as details in the 2275 * available Times and not available Times. 2276 */ 2277 public HealthcareService setAvailabilityExceptions(String value) { 2278 if (Utilities.noString(value)) 2279 this.availabilityExceptions = null; 2280 else { 2281 if (this.availabilityExceptions == null) 2282 this.availabilityExceptions = new StringType(); 2283 this.availabilityExceptions.setValue(value); 2284 } 2285 return this; 2286 } 2287 2288 protected void listChildren(List<Property> childrenList) { 2289 super.listChildren(childrenList); 2290 childrenList.add(new Property("identifier", "Identifier", "External identifiers for this item.", 0, 2291 java.lang.Integer.MAX_VALUE, identifier)); 2292 childrenList.add(new Property("providedBy", "Reference(Organization)", 2293 "The organization that provides this healthcare service.", 0, java.lang.Integer.MAX_VALUE, providedBy)); 2294 childrenList.add(new Property("serviceCategory", "CodeableConcept", 2295 "Identifies the broad category of service being performed or delivered.", 0, java.lang.Integer.MAX_VALUE, 2296 serviceCategory)); 2297 childrenList.add(new Property("serviceType", "", "A specific type of service that may be delivered or performed.", 2298 0, java.lang.Integer.MAX_VALUE, serviceType)); 2299 childrenList.add(new Property("location", "Reference(Location)", 2300 "The location where this healthcare service may be provided.", 0, java.lang.Integer.MAX_VALUE, location)); 2301 childrenList.add(new Property("serviceName", "string", 2302 "Further description of the service as it would be presented to a consumer while searching.", 0, 2303 java.lang.Integer.MAX_VALUE, serviceName)); 2304 childrenList.add(new Property("comment", "string", 2305 "Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName.", 2306 0, java.lang.Integer.MAX_VALUE, comment)); 2307 childrenList.add(new Property("extraDetails", "string", 2308 "Extra details about the service that can't be placed in the other fields.", 0, java.lang.Integer.MAX_VALUE, 2309 extraDetails)); 2310 childrenList.add(new Property("photo", "Attachment", 2311 "If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list.", 2312 0, java.lang.Integer.MAX_VALUE, photo)); 2313 childrenList.add(new Property("telecom", "ContactPoint", 2314 "List of contacts related to this specific healthcare service.", 0, java.lang.Integer.MAX_VALUE, telecom)); 2315 childrenList.add(new Property("coverageArea", "Reference(Location)", 2316 "The location(s) that this service is available to (not where the service is provided).", 0, 2317 java.lang.Integer.MAX_VALUE, coverageArea)); 2318 childrenList.add(new Property("serviceProvisionCode", "CodeableConcept", 2319 "The code(s) that detail the conditions under which the healthcare service is available/offered.", 0, 2320 java.lang.Integer.MAX_VALUE, serviceProvisionCode)); 2321 childrenList.add(new Property("eligibility", "CodeableConcept", 2322 "Does this service have specific eligibility requirements that need to be met in order to use the service?", 0, 2323 java.lang.Integer.MAX_VALUE, eligibility)); 2324 childrenList.add(new Property("eligibilityNote", "string", "Describes the eligibility conditions for the service.", 2325 0, java.lang.Integer.MAX_VALUE, eligibilityNote)); 2326 childrenList.add(new Property("programName", "string", "Program Names that can be used to categorize the service.", 2327 0, java.lang.Integer.MAX_VALUE, programName)); 2328 childrenList.add(new Property("characteristic", "CodeableConcept", "Collection of characteristics (attributes).", 0, 2329 java.lang.Integer.MAX_VALUE, characteristic)); 2330 childrenList.add(new Property("referralMethod", "CodeableConcept", 2331 "Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required.", 2332 0, java.lang.Integer.MAX_VALUE, referralMethod)); 2333 childrenList.add(new Property("publicKey", "string", 2334 "The public part of the 'keys' allocated to an Organization by an accredited body to support secure exchange of data over the internet. To be provided by the Organization, where available.", 2335 0, java.lang.Integer.MAX_VALUE, publicKey)); 2336 childrenList.add(new Property("appointmentRequired", "boolean", 2337 "Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service.", 2338 0, java.lang.Integer.MAX_VALUE, appointmentRequired)); 2339 childrenList.add(new Property("availableTime", "", "A collection of times that the Service Site is available.", 0, 2340 java.lang.Integer.MAX_VALUE, availableTime)); 2341 childrenList.add(new Property("notAvailable", "", 2342 "The HealthcareService is not available during this period of time due to the provided reason.", 0, 2343 java.lang.Integer.MAX_VALUE, notAvailable)); 2344 childrenList.add(new Property("availabilityExceptions", "string", 2345 "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.", 2346 0, java.lang.Integer.MAX_VALUE, availabilityExceptions)); 2347 } 2348 2349 @Override 2350 public void setProperty(String name, Base value) throws FHIRException { 2351 if (name.equals("identifier")) 2352 this.getIdentifier().add(castToIdentifier(value)); 2353 else if (name.equals("providedBy")) 2354 this.providedBy = castToReference(value); // Reference 2355 else if (name.equals("serviceCategory")) 2356 this.serviceCategory = castToCodeableConcept(value); // CodeableConcept 2357 else if (name.equals("serviceType")) 2358 this.getServiceType().add((ServiceTypeComponent) value); 2359 else if (name.equals("location")) 2360 this.location = castToReference(value); // Reference 2361 else if (name.equals("serviceName")) 2362 this.serviceName = castToString(value); // StringType 2363 else if (name.equals("comment")) 2364 this.comment = castToString(value); // StringType 2365 else if (name.equals("extraDetails")) 2366 this.extraDetails = castToString(value); // StringType 2367 else if (name.equals("photo")) 2368 this.photo = castToAttachment(value); // Attachment 2369 else if (name.equals("telecom")) 2370 this.getTelecom().add(castToContactPoint(value)); 2371 else if (name.equals("coverageArea")) 2372 this.getCoverageArea().add(castToReference(value)); 2373 else if (name.equals("serviceProvisionCode")) 2374 this.getServiceProvisionCode().add(castToCodeableConcept(value)); 2375 else if (name.equals("eligibility")) 2376 this.eligibility = castToCodeableConcept(value); // CodeableConcept 2377 else if (name.equals("eligibilityNote")) 2378 this.eligibilityNote = castToString(value); // StringType 2379 else if (name.equals("programName")) 2380 this.getProgramName().add(castToString(value)); 2381 else if (name.equals("characteristic")) 2382 this.getCharacteristic().add(castToCodeableConcept(value)); 2383 else if (name.equals("referralMethod")) 2384 this.getReferralMethod().add(castToCodeableConcept(value)); 2385 else if (name.equals("publicKey")) 2386 this.publicKey = castToString(value); // StringType 2387 else if (name.equals("appointmentRequired")) 2388 this.appointmentRequired = castToBoolean(value); // BooleanType 2389 else if (name.equals("availableTime")) 2390 this.getAvailableTime().add((HealthcareServiceAvailableTimeComponent) value); 2391 else if (name.equals("notAvailable")) 2392 this.getNotAvailable().add((HealthcareServiceNotAvailableComponent) value); 2393 else if (name.equals("availabilityExceptions")) 2394 this.availabilityExceptions = castToString(value); // StringType 2395 else 2396 super.setProperty(name, value); 2397 } 2398 2399 @Override 2400 public Base addChild(String name) throws FHIRException { 2401 if (name.equals("identifier")) { 2402 return addIdentifier(); 2403 } else if (name.equals("providedBy")) { 2404 this.providedBy = new Reference(); 2405 return this.providedBy; 2406 } else if (name.equals("serviceCategory")) { 2407 this.serviceCategory = new CodeableConcept(); 2408 return this.serviceCategory; 2409 } else if (name.equals("serviceType")) { 2410 return addServiceType(); 2411 } else if (name.equals("location")) { 2412 this.location = new Reference(); 2413 return this.location; 2414 } else if (name.equals("serviceName")) { 2415 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.serviceName"); 2416 } else if (name.equals("comment")) { 2417 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.comment"); 2418 } else if (name.equals("extraDetails")) { 2419 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.extraDetails"); 2420 } else if (name.equals("photo")) { 2421 this.photo = new Attachment(); 2422 return this.photo; 2423 } else if (name.equals("telecom")) { 2424 return addTelecom(); 2425 } else if (name.equals("coverageArea")) { 2426 return addCoverageArea(); 2427 } else if (name.equals("serviceProvisionCode")) { 2428 return addServiceProvisionCode(); 2429 } else if (name.equals("eligibility")) { 2430 this.eligibility = new CodeableConcept(); 2431 return this.eligibility; 2432 } else if (name.equals("eligibilityNote")) { 2433 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.eligibilityNote"); 2434 } else if (name.equals("programName")) { 2435 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.programName"); 2436 } else if (name.equals("characteristic")) { 2437 return addCharacteristic(); 2438 } else if (name.equals("referralMethod")) { 2439 return addReferralMethod(); 2440 } else if (name.equals("publicKey")) { 2441 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.publicKey"); 2442 } else if (name.equals("appointmentRequired")) { 2443 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.appointmentRequired"); 2444 } else if (name.equals("availableTime")) { 2445 return addAvailableTime(); 2446 } else if (name.equals("notAvailable")) { 2447 return addNotAvailable(); 2448 } else if (name.equals("availabilityExceptions")) { 2449 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.availabilityExceptions"); 2450 } else 2451 return super.addChild(name); 2452 } 2453 2454 public String fhirType() { 2455 return "HealthcareService"; 2456 2457 } 2458 2459 public HealthcareService copy() { 2460 HealthcareService dst = new HealthcareService(); 2461 copyValues(dst); 2462 if (identifier != null) { 2463 dst.identifier = new ArrayList<Identifier>(); 2464 for (Identifier i : identifier) 2465 dst.identifier.add(i.copy()); 2466 } 2467 ; 2468 dst.providedBy = providedBy == null ? null : providedBy.copy(); 2469 dst.serviceCategory = serviceCategory == null ? null : serviceCategory.copy(); 2470 if (serviceType != null) { 2471 dst.serviceType = new ArrayList<ServiceTypeComponent>(); 2472 for (ServiceTypeComponent i : serviceType) 2473 dst.serviceType.add(i.copy()); 2474 } 2475 ; 2476 dst.location = location == null ? null : location.copy(); 2477 dst.serviceName = serviceName == null ? null : serviceName.copy(); 2478 dst.comment = comment == null ? null : comment.copy(); 2479 dst.extraDetails = extraDetails == null ? null : extraDetails.copy(); 2480 dst.photo = photo == null ? null : photo.copy(); 2481 if (telecom != null) { 2482 dst.telecom = new ArrayList<ContactPoint>(); 2483 for (ContactPoint i : telecom) 2484 dst.telecom.add(i.copy()); 2485 } 2486 ; 2487 if (coverageArea != null) { 2488 dst.coverageArea = new ArrayList<Reference>(); 2489 for (Reference i : coverageArea) 2490 dst.coverageArea.add(i.copy()); 2491 } 2492 ; 2493 if (serviceProvisionCode != null) { 2494 dst.serviceProvisionCode = new ArrayList<CodeableConcept>(); 2495 for (CodeableConcept i : serviceProvisionCode) 2496 dst.serviceProvisionCode.add(i.copy()); 2497 } 2498 ; 2499 dst.eligibility = eligibility == null ? null : eligibility.copy(); 2500 dst.eligibilityNote = eligibilityNote == null ? null : eligibilityNote.copy(); 2501 if (programName != null) { 2502 dst.programName = new ArrayList<StringType>(); 2503 for (StringType i : programName) 2504 dst.programName.add(i.copy()); 2505 } 2506 ; 2507 if (characteristic != null) { 2508 dst.characteristic = new ArrayList<CodeableConcept>(); 2509 for (CodeableConcept i : characteristic) 2510 dst.characteristic.add(i.copy()); 2511 } 2512 ; 2513 if (referralMethod != null) { 2514 dst.referralMethod = new ArrayList<CodeableConcept>(); 2515 for (CodeableConcept i : referralMethod) 2516 dst.referralMethod.add(i.copy()); 2517 } 2518 ; 2519 dst.publicKey = publicKey == null ? null : publicKey.copy(); 2520 dst.appointmentRequired = appointmentRequired == null ? null : appointmentRequired.copy(); 2521 if (availableTime != null) { 2522 dst.availableTime = new ArrayList<HealthcareServiceAvailableTimeComponent>(); 2523 for (HealthcareServiceAvailableTimeComponent i : availableTime) 2524 dst.availableTime.add(i.copy()); 2525 } 2526 ; 2527 if (notAvailable != null) { 2528 dst.notAvailable = new ArrayList<HealthcareServiceNotAvailableComponent>(); 2529 for (HealthcareServiceNotAvailableComponent i : notAvailable) 2530 dst.notAvailable.add(i.copy()); 2531 } 2532 ; 2533 dst.availabilityExceptions = availabilityExceptions == null ? null : availabilityExceptions.copy(); 2534 return dst; 2535 } 2536 2537 protected HealthcareService typedCopy() { 2538 return copy(); 2539 } 2540 2541 @Override 2542 public boolean equalsDeep(Base other) { 2543 if (!super.equalsDeep(other)) 2544 return false; 2545 if (!(other instanceof HealthcareService)) 2546 return false; 2547 HealthcareService o = (HealthcareService) other; 2548 return compareDeep(identifier, o.identifier, true) && compareDeep(providedBy, o.providedBy, true) 2549 && compareDeep(serviceCategory, o.serviceCategory, true) && compareDeep(serviceType, o.serviceType, true) 2550 && compareDeep(location, o.location, true) && compareDeep(serviceName, o.serviceName, true) 2551 && compareDeep(comment, o.comment, true) && compareDeep(extraDetails, o.extraDetails, true) 2552 && compareDeep(photo, o.photo, true) && compareDeep(telecom, o.telecom, true) 2553 && compareDeep(coverageArea, o.coverageArea, true) 2554 && compareDeep(serviceProvisionCode, o.serviceProvisionCode, true) 2555 && compareDeep(eligibility, o.eligibility, true) && compareDeep(eligibilityNote, o.eligibilityNote, true) 2556 && compareDeep(programName, o.programName, true) && compareDeep(characteristic, o.characteristic, true) 2557 && compareDeep(referralMethod, o.referralMethod, true) && compareDeep(publicKey, o.publicKey, true) 2558 && compareDeep(appointmentRequired, o.appointmentRequired, true) 2559 && compareDeep(availableTime, o.availableTime, true) && compareDeep(notAvailable, o.notAvailable, true) 2560 && compareDeep(availabilityExceptions, o.availabilityExceptions, true); 2561 } 2562 2563 @Override 2564 public boolean equalsShallow(Base other) { 2565 if (!super.equalsShallow(other)) 2566 return false; 2567 if (!(other instanceof HealthcareService)) 2568 return false; 2569 HealthcareService o = (HealthcareService) other; 2570 return compareValues(serviceName, o.serviceName, true) && compareValues(comment, o.comment, true) 2571 && compareValues(extraDetails, o.extraDetails, true) && compareValues(eligibilityNote, o.eligibilityNote, true) 2572 && compareValues(programName, o.programName, true) && compareValues(publicKey, o.publicKey, true) 2573 && compareValues(appointmentRequired, o.appointmentRequired, true) 2574 && compareValues(availabilityExceptions, o.availabilityExceptions, true); 2575 } 2576 2577 public boolean isEmpty() { 2578 return super.isEmpty() && (identifier == null || identifier.isEmpty()) 2579 && (providedBy == null || providedBy.isEmpty()) && (serviceCategory == null || serviceCategory.isEmpty()) 2580 && (serviceType == null || serviceType.isEmpty()) && (location == null || location.isEmpty()) 2581 && (serviceName == null || serviceName.isEmpty()) && (comment == null || comment.isEmpty()) 2582 && (extraDetails == null || extraDetails.isEmpty()) && (photo == null || photo.isEmpty()) 2583 && (telecom == null || telecom.isEmpty()) && (coverageArea == null || coverageArea.isEmpty()) 2584 && (serviceProvisionCode == null || serviceProvisionCode.isEmpty()) 2585 && (eligibility == null || eligibility.isEmpty()) && (eligibilityNote == null || eligibilityNote.isEmpty()) 2586 && (programName == null || programName.isEmpty()) && (characteristic == null || characteristic.isEmpty()) 2587 && (referralMethod == null || referralMethod.isEmpty()) && (publicKey == null || publicKey.isEmpty()) 2588 && (appointmentRequired == null || appointmentRequired.isEmpty()) 2589 && (availableTime == null || availableTime.isEmpty()) && (notAvailable == null || notAvailable.isEmpty()) 2590 && (availabilityExceptions == null || availabilityExceptions.isEmpty()); 2591 } 2592 2593 @Override 2594 public ResourceType getResourceType() { 2595 return ResourceType.HealthcareService; 2596 } 2597 2598 @SearchParamDefinition(name = "identifier", path = "HealthcareService.identifier", description = "External identifiers for this item", type = "token") 2599 public static final String SP_IDENTIFIER = "identifier"; 2600 @SearchParamDefinition(name = "servicecategory", path = "HealthcareService.serviceCategory", description = "Service Category of the Healthcare Service", type = "token") 2601 public static final String SP_SERVICECATEGORY = "servicecategory"; 2602 @SearchParamDefinition(name = "servicetype", path = "HealthcareService.serviceType.type", description = "The type of service provided by this healthcare service", type = "token") 2603 public static final String SP_SERVICETYPE = "servicetype"; 2604 @SearchParamDefinition(name = "organization", path = "HealthcareService.providedBy", description = "The organization that provides this Healthcare Service", type = "reference") 2605 public static final String SP_ORGANIZATION = "organization"; 2606 @SearchParamDefinition(name = "name", path = "HealthcareService.serviceName", description = "A portion of the Healthcare service name", type = "string") 2607 public static final String SP_NAME = "name"; 2608 @SearchParamDefinition(name = "programname", path = "HealthcareService.programName", description = "One of the Program Names serviced by this HealthcareService", type = "string") 2609 public static final String SP_PROGRAMNAME = "programname"; 2610 @SearchParamDefinition(name = "location", path = "HealthcareService.location", description = "The location of the Healthcare Service", type = "reference") 2611 public static final String SP_LOCATION = "location"; 2612 @SearchParamDefinition(name = "characteristic", path = "HealthcareService.characteristic", description = "One of the HealthcareService's characteristics", type = "token") 2613 public static final String SP_CHARACTERISTIC = "characteristic"; 2614 2615}