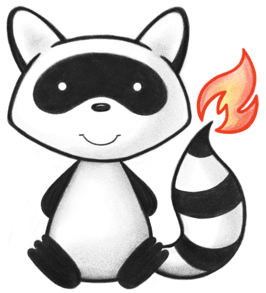
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Child; 037import ca.uhn.fhir.model.api.annotation.DatatypeDef; 038import ca.uhn.fhir.model.api.annotation.Description; 039import org.hl7.fhir.instance.model.api.ICompositeType; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.utilities.Utilities; 042 043/** 044 * A human's name with the ability to identify parts and usage. 045 */ 046@DatatypeDef(name = "HumanName") 047public class HumanName extends Type implements ICompositeType { 048 049 public enum NameUse { 050 /** 051 * Known as/conventional/the one you normally use 052 */ 053 USUAL, 054 /** 055 * The formal name as registered in an official (government) registry, but which 056 * name might not be commonly used. May be called "legal name". 057 */ 058 OFFICIAL, 059 /** 060 * A temporary name. Name.period can provide more detailed information. This may 061 * also be used for temporary names assigned at birth or in emergency 062 * situations. 063 */ 064 TEMP, 065 /** 066 * A name that is used to address the person in an informal manner, but is not 067 * part of their formal or usual name 068 */ 069 NICKNAME, 070 /** 071 * Anonymous assigned name, alias, or pseudonym (used to protect a person's 072 * identity for privacy reasons) 073 */ 074 ANONYMOUS, 075 /** 076 * This name is no longer in use (or was never correct, but retained for 077 * records) 078 */ 079 OLD, 080 /** 081 * A name used prior to marriage. Marriage naming customs vary greatly around 082 * the world. This name use is for use by applications that collect and store 083 * "maiden" names. Though the concept of maiden name is often gender specific, 084 * the use of this term is not gender specific. The use of this term does not 085 * imply any particular history for a person's name, nor should the maiden name 086 * be determined algorithmically. 087 */ 088 MAIDEN, 089 /** 090 * added to help the parsers 091 */ 092 NULL; 093 094 public static NameUse fromCode(String codeString) throws FHIRException { 095 if (codeString == null || "".equals(codeString)) 096 return null; 097 if ("usual".equals(codeString)) 098 return USUAL; 099 if ("official".equals(codeString)) 100 return OFFICIAL; 101 if ("temp".equals(codeString)) 102 return TEMP; 103 if ("nickname".equals(codeString)) 104 return NICKNAME; 105 if ("anonymous".equals(codeString)) 106 return ANONYMOUS; 107 if ("old".equals(codeString)) 108 return OLD; 109 if ("maiden".equals(codeString)) 110 return MAIDEN; 111 throw new FHIRException("Unknown NameUse code '" + codeString + "'"); 112 } 113 114 public String toCode() { 115 switch (this) { 116 case USUAL: 117 return "usual"; 118 case OFFICIAL: 119 return "official"; 120 case TEMP: 121 return "temp"; 122 case NICKNAME: 123 return "nickname"; 124 case ANONYMOUS: 125 return "anonymous"; 126 case OLD: 127 return "old"; 128 case MAIDEN: 129 return "maiden"; 130 case NULL: 131 return null; 132 default: 133 return "?"; 134 } 135 } 136 137 public String getSystem() { 138 switch (this) { 139 case USUAL: 140 return "http://hl7.org/fhir/name-use"; 141 case OFFICIAL: 142 return "http://hl7.org/fhir/name-use"; 143 case TEMP: 144 return "http://hl7.org/fhir/name-use"; 145 case NICKNAME: 146 return "http://hl7.org/fhir/name-use"; 147 case ANONYMOUS: 148 return "http://hl7.org/fhir/name-use"; 149 case OLD: 150 return "http://hl7.org/fhir/name-use"; 151 case MAIDEN: 152 return "http://hl7.org/fhir/name-use"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 160 public String getDefinition() { 161 switch (this) { 162 case USUAL: 163 return "Known as/conventional/the one you normally use"; 164 case OFFICIAL: 165 return "The formal name as registered in an official (government) registry, but which name might not be commonly used. May be called \"legal name\"."; 166 case TEMP: 167 return "A temporary name. Name.period can provide more detailed information. This may also be used for temporary names assigned at birth or in emergency situations."; 168 case NICKNAME: 169 return "A name that is used to address the person in an informal manner, but is not part of their formal or usual name"; 170 case ANONYMOUS: 171 return "Anonymous assigned name, alias, or pseudonym (used to protect a person's identity for privacy reasons)"; 172 case OLD: 173 return "This name is no longer in use (or was never correct, but retained for records)"; 174 case MAIDEN: 175 return "A name used prior to marriage. Marriage naming customs vary greatly around the world. This name use is for use by applications that collect and store \"maiden\" names. Though the concept of maiden name is often gender specific, the use of this term is not gender specific. The use of this term does not imply any particular history for a person's name, nor should the maiden name be determined algorithmically."; 176 case NULL: 177 return null; 178 default: 179 return "?"; 180 } 181 } 182 183 public String getDisplay() { 184 switch (this) { 185 case USUAL: 186 return "Usual"; 187 case OFFICIAL: 188 return "Official"; 189 case TEMP: 190 return "Temp"; 191 case NICKNAME: 192 return "Nickname"; 193 case ANONYMOUS: 194 return "Anonymous"; 195 case OLD: 196 return "Old"; 197 case MAIDEN: 198 return "Maiden"; 199 case NULL: 200 return null; 201 default: 202 return "?"; 203 } 204 } 205 } 206 207 public static class NameUseEnumFactory implements EnumFactory<NameUse> { 208 public NameUse fromCode(String codeString) throws IllegalArgumentException { 209 if (codeString == null || "".equals(codeString)) 210 if (codeString == null || "".equals(codeString)) 211 return null; 212 if ("usual".equals(codeString)) 213 return NameUse.USUAL; 214 if ("official".equals(codeString)) 215 return NameUse.OFFICIAL; 216 if ("temp".equals(codeString)) 217 return NameUse.TEMP; 218 if ("nickname".equals(codeString)) 219 return NameUse.NICKNAME; 220 if ("anonymous".equals(codeString)) 221 return NameUse.ANONYMOUS; 222 if ("old".equals(codeString)) 223 return NameUse.OLD; 224 if ("maiden".equals(codeString)) 225 return NameUse.MAIDEN; 226 throw new IllegalArgumentException("Unknown NameUse code '" + codeString + "'"); 227 } 228 229 public Enumeration<NameUse> fromType(Base code) throws FHIRException { 230 if (code == null || code.isEmpty()) 231 return null; 232 String codeString = ((PrimitiveType) code).asStringValue(); 233 if (codeString == null || "".equals(codeString)) 234 return null; 235 if ("usual".equals(codeString)) 236 return new Enumeration<NameUse>(this, NameUse.USUAL); 237 if ("official".equals(codeString)) 238 return new Enumeration<NameUse>(this, NameUse.OFFICIAL); 239 if ("temp".equals(codeString)) 240 return new Enumeration<NameUse>(this, NameUse.TEMP); 241 if ("nickname".equals(codeString)) 242 return new Enumeration<NameUse>(this, NameUse.NICKNAME); 243 if ("anonymous".equals(codeString)) 244 return new Enumeration<NameUse>(this, NameUse.ANONYMOUS); 245 if ("old".equals(codeString)) 246 return new Enumeration<NameUse>(this, NameUse.OLD); 247 if ("maiden".equals(codeString)) 248 return new Enumeration<NameUse>(this, NameUse.MAIDEN); 249 throw new FHIRException("Unknown NameUse code '" + codeString + "'"); 250 } 251 252 public String toCode(NameUse code) 253 { 254 if (code == NameUse.NULL) 255 return null; 256 if (code == NameUse.USUAL) 257 return "usual"; 258 if (code == NameUse.OFFICIAL) 259 return "official"; 260 if (code == NameUse.TEMP) 261 return "temp"; 262 if (code == NameUse.NICKNAME) 263 return "nickname"; 264 if (code == NameUse.ANONYMOUS) 265 return "anonymous"; 266 if (code == NameUse.OLD) 267 return "old"; 268 if (code == NameUse.MAIDEN) 269 return "maiden"; 270 return "?"; 271 } 272 } 273 274 /** 275 * Identifies the purpose for this name. 276 */ 277 @Child(name = "use", type = { CodeType.class }, order = 0, min = 0, max = 1, modifier = true, summary = true) 278 @Description(shortDefinition = "usual | official | temp | nickname | anonymous | old | maiden", formalDefinition = "Identifies the purpose for this name.") 279 protected Enumeration<NameUse> use; 280 281 /** 282 * A full text representation of the name. 283 */ 284 @Child(name = "text", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 285 @Description(shortDefinition = "Text representation of the full name", formalDefinition = "A full text representation of the name.") 286 protected StringType text; 287 288 /** 289 * The part of a name that links to the genealogy. In some cultures (e.g. 290 * Eritrea) the family name of a son is the first name of his father. 291 */ 292 @Child(name = "family", type = { 293 StringType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 294 @Description(shortDefinition = "Family name (often called 'Surname')", formalDefinition = "The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.") 295 protected List<StringType> family; 296 297 /** 298 * Given name. 299 */ 300 @Child(name = "given", type = { 301 StringType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 302 @Description(shortDefinition = "Given names (not always 'first'). Includes middle names", formalDefinition = "Given name.") 303 protected List<StringType> given; 304 305 /** 306 * Part of the name that is acquired as a title due to academic, legal, 307 * employment or nobility status, etc. and that appears at the start of the 308 * name. 309 */ 310 @Child(name = "prefix", type = { 311 StringType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 312 @Description(shortDefinition = "Parts that come before the name", formalDefinition = "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.") 313 protected List<StringType> prefix; 314 315 /** 316 * Part of the name that is acquired as a title due to academic, legal, 317 * employment or nobility status, etc. and that appears at the end of the name. 318 */ 319 @Child(name = "suffix", type = { 320 StringType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 321 @Description(shortDefinition = "Parts that come after the name", formalDefinition = "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.") 322 protected List<StringType> suffix; 323 324 /** 325 * Indicates the period of time when this name was valid for the named person. 326 */ 327 @Child(name = "period", type = { Period.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 328 @Description(shortDefinition = "Time period when name was/is in use", formalDefinition = "Indicates the period of time when this name was valid for the named person.") 329 protected Period period; 330 331 private static final long serialVersionUID = -210174642L; 332 333 /* 334 * Constructor 335 */ 336 public HumanName() { 337 super(); 338 } 339 340 /** 341 * @return {@link #use} (Identifies the purpose for this name.). This is the 342 * underlying object with id, value and extensions. The accessor 343 * "getUse" gives direct access to the value 344 */ 345 public Enumeration<NameUse> getUseElement() { 346 if (this.use == null) 347 if (Configuration.errorOnAutoCreate()) 348 throw new Error("Attempt to auto-create HumanName.use"); 349 else if (Configuration.doAutoCreate()) 350 this.use = new Enumeration<NameUse>(new NameUseEnumFactory()); // bb 351 return this.use; 352 } 353 354 public boolean hasUseElement() { 355 return this.use != null && !this.use.isEmpty(); 356 } 357 358 public boolean hasUse() { 359 return this.use != null && !this.use.isEmpty(); 360 } 361 362 /** 363 * @param value {@link #use} (Identifies the purpose for this name.). This is 364 * the underlying object with id, value and extensions. The 365 * accessor "getUse" gives direct access to the value 366 */ 367 public HumanName setUseElement(Enumeration<NameUse> value) { 368 this.use = value; 369 return this; 370 } 371 372 /** 373 * @return Identifies the purpose for this name. 374 */ 375 public NameUse getUse() { 376 return this.use == null ? null : this.use.getValue(); 377 } 378 379 /** 380 * @param value Identifies the purpose for this name. 381 */ 382 public HumanName setUse(NameUse value) { 383 if (value == null) 384 this.use = null; 385 else { 386 if (this.use == null) 387 this.use = new Enumeration<NameUse>(new NameUseEnumFactory()); 388 this.use.setValue(value); 389 } 390 return this; 391 } 392 393 /** 394 * @return {@link #text} (A full text representation of the name.). This is the 395 * underlying object with id, value and extensions. The accessor 396 * "getText" gives direct access to the value 397 */ 398 public StringType getTextElement() { 399 if (this.text == null) 400 if (Configuration.errorOnAutoCreate()) 401 throw new Error("Attempt to auto-create HumanName.text"); 402 else if (Configuration.doAutoCreate()) 403 this.text = new StringType(); // bb 404 return this.text; 405 } 406 407 public boolean hasTextElement() { 408 return this.text != null && !this.text.isEmpty(); 409 } 410 411 public boolean hasText() { 412 return this.text != null && !this.text.isEmpty(); 413 } 414 415 /** 416 * @param value {@link #text} (A full text representation of the name.). This is 417 * the underlying object with id, value and extensions. The 418 * accessor "getText" gives direct access to the value 419 */ 420 public HumanName setTextElement(StringType value) { 421 this.text = value; 422 return this; 423 } 424 425 /** 426 * @return A full text representation of the name. 427 */ 428 public String getText() { 429 return this.text == null ? null : this.text.getValue(); 430 } 431 432 /** 433 * @param value A full text representation of the name. 434 */ 435 public HumanName setText(String value) { 436 if (Utilities.noString(value)) 437 this.text = null; 438 else { 439 if (this.text == null) 440 this.text = new StringType(); 441 this.text.setValue(value); 442 } 443 return this; 444 } 445 446 /** 447 * @return {@link #family} (The part of a name that links to the genealogy. In 448 * some cultures (e.g. Eritrea) the family name of a son is the first 449 * name of his father.) 450 */ 451 public List<StringType> getFamily() { 452 if (this.family == null) 453 this.family = new ArrayList<StringType>(); 454 return this.family; 455 } 456 457 public boolean hasFamily() { 458 if (this.family == null) 459 return false; 460 for (StringType item : this.family) 461 if (!item.isEmpty()) 462 return true; 463 return false; 464 } 465 466 /** 467 * @return {@link #family} (The part of a name that links to the genealogy. In 468 * some cultures (e.g. Eritrea) the family name of a son is the first 469 * name of his father.) 470 */ 471 // syntactic sugar 472 public StringType addFamilyElement() {// 2 473 StringType t = new StringType(); 474 if (this.family == null) 475 this.family = new ArrayList<StringType>(); 476 this.family.add(t); 477 return t; 478 } 479 480 /** 481 * @param value {@link #family} (The part of a name that links to the genealogy. 482 * In some cultures (e.g. Eritrea) the family name of a son is the 483 * first name of his father.) 484 */ 485 public HumanName addFamily(String value) { // 1 486 StringType t = new StringType(); 487 t.setValue(value); 488 if (this.family == null) 489 this.family = new ArrayList<StringType>(); 490 this.family.add(t); 491 return this; 492 } 493 494 /** 495 * @param value {@link #family} (The part of a name that links to the genealogy. 496 * In some cultures (e.g. Eritrea) the family name of a son is the 497 * first name of his father.) 498 */ 499 public boolean hasFamily(String value) { 500 if (this.family == null) 501 return false; 502 for (StringType v : this.family) 503 if (v.equals(value)) // string 504 return true; 505 return false; 506 } 507 508 /** 509 * @return {@link #given} (Given name.) 510 */ 511 public List<StringType> getGiven() { 512 if (this.given == null) 513 this.given = new ArrayList<StringType>(); 514 return this.given; 515 } 516 517 public boolean hasGiven() { 518 if (this.given == null) 519 return false; 520 for (StringType item : this.given) 521 if (!item.isEmpty()) 522 return true; 523 return false; 524 } 525 526 /** 527 * @return {@link #given} (Given name.) 528 */ 529 // syntactic sugar 530 public StringType addGivenElement() {// 2 531 StringType t = new StringType(); 532 if (this.given == null) 533 this.given = new ArrayList<StringType>(); 534 this.given.add(t); 535 return t; 536 } 537 538 /** 539 * @param value {@link #given} (Given name.) 540 */ 541 public HumanName addGiven(String value) { // 1 542 StringType t = new StringType(); 543 t.setValue(value); 544 if (this.given == null) 545 this.given = new ArrayList<StringType>(); 546 this.given.add(t); 547 return this; 548 } 549 550 /** 551 * @param value {@link #given} (Given name.) 552 */ 553 public boolean hasGiven(String value) { 554 if (this.given == null) 555 return false; 556 for (StringType v : this.given) 557 if (v.equals(value)) // string 558 return true; 559 return false; 560 } 561 562 /** 563 * @return {@link #prefix} (Part of the name that is acquired as a title due to 564 * academic, legal, employment or nobility status, etc. and that appears 565 * at the start of the name.) 566 */ 567 public List<StringType> getPrefix() { 568 if (this.prefix == null) 569 this.prefix = new ArrayList<StringType>(); 570 return this.prefix; 571 } 572 573 public boolean hasPrefix() { 574 if (this.prefix == null) 575 return false; 576 for (StringType item : this.prefix) 577 if (!item.isEmpty()) 578 return true; 579 return false; 580 } 581 582 /** 583 * @return {@link #prefix} (Part of the name that is acquired as a title due to 584 * academic, legal, employment or nobility status, etc. and that appears 585 * at the start of the name.) 586 */ 587 // syntactic sugar 588 public StringType addPrefixElement() {// 2 589 StringType t = new StringType(); 590 if (this.prefix == null) 591 this.prefix = new ArrayList<StringType>(); 592 this.prefix.add(t); 593 return t; 594 } 595 596 /** 597 * @param value {@link #prefix} (Part of the name that is acquired as a title 598 * due to academic, legal, employment or nobility status, etc. and 599 * that appears at the start of the name.) 600 */ 601 public HumanName addPrefix(String value) { // 1 602 StringType t = new StringType(); 603 t.setValue(value); 604 if (this.prefix == null) 605 this.prefix = new ArrayList<StringType>(); 606 this.prefix.add(t); 607 return this; 608 } 609 610 /** 611 * @param value {@link #prefix} (Part of the name that is acquired as a title 612 * due to academic, legal, employment or nobility status, etc. and 613 * that appears at the start of the name.) 614 */ 615 public boolean hasPrefix(String value) { 616 if (this.prefix == null) 617 return false; 618 for (StringType v : this.prefix) 619 if (v.equals(value)) // string 620 return true; 621 return false; 622 } 623 624 /** 625 * @return {@link #suffix} (Part of the name that is acquired as a title due to 626 * academic, legal, employment or nobility status, etc. and that appears 627 * at the end of the name.) 628 */ 629 public List<StringType> getSuffix() { 630 if (this.suffix == null) 631 this.suffix = new ArrayList<StringType>(); 632 return this.suffix; 633 } 634 635 public boolean hasSuffix() { 636 if (this.suffix == null) 637 return false; 638 for (StringType item : this.suffix) 639 if (!item.isEmpty()) 640 return true; 641 return false; 642 } 643 644 /** 645 * @return {@link #suffix} (Part of the name that is acquired as a title due to 646 * academic, legal, employment or nobility status, etc. and that appears 647 * at the end of the name.) 648 */ 649 // syntactic sugar 650 public StringType addSuffixElement() {// 2 651 StringType t = new StringType(); 652 if (this.suffix == null) 653 this.suffix = new ArrayList<StringType>(); 654 this.suffix.add(t); 655 return t; 656 } 657 658 /** 659 * @param value {@link #suffix} (Part of the name that is acquired as a title 660 * due to academic, legal, employment or nobility status, etc. and 661 * that appears at the end of the name.) 662 */ 663 public HumanName addSuffix(String value) { // 1 664 StringType t = new StringType(); 665 t.setValue(value); 666 if (this.suffix == null) 667 this.suffix = new ArrayList<StringType>(); 668 this.suffix.add(t); 669 return this; 670 } 671 672 /** 673 * @param value {@link #suffix} (Part of the name that is acquired as a title 674 * due to academic, legal, employment or nobility status, etc. and 675 * that appears at the end of the name.) 676 */ 677 public boolean hasSuffix(String value) { 678 if (this.suffix == null) 679 return false; 680 for (StringType v : this.suffix) 681 if (v.equals(value)) // string 682 return true; 683 return false; 684 } 685 686 /** 687 * @return {@link #period} (Indicates the period of time when this name was 688 * valid for the named person.) 689 */ 690 public Period getPeriod() { 691 if (this.period == null) 692 if (Configuration.errorOnAutoCreate()) 693 throw new Error("Attempt to auto-create HumanName.period"); 694 else if (Configuration.doAutoCreate()) 695 this.period = new Period(); // cc 696 return this.period; 697 } 698 699 public boolean hasPeriod() { 700 return this.period != null && !this.period.isEmpty(); 701 } 702 703 /** 704 * @param value {@link #period} (Indicates the period of time when this name was 705 * valid for the named person.) 706 */ 707 public HumanName setPeriod(Period value) { 708 this.period = value; 709 return this; 710 } 711 712 protected void listChildren(List<Property> childrenList) { 713 super.listChildren(childrenList); 714 childrenList 715 .add(new Property("use", "code", "Identifies the purpose for this name.", 0, java.lang.Integer.MAX_VALUE, use)); 716 childrenList.add(new Property("text", "string", "A full text representation of the name.", 0, 717 java.lang.Integer.MAX_VALUE, text)); 718 childrenList.add(new Property("family", "string", 719 "The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.", 720 0, java.lang.Integer.MAX_VALUE, family)); 721 childrenList.add(new Property("given", "string", "Given name.", 0, java.lang.Integer.MAX_VALUE, given)); 722 childrenList.add(new Property("prefix", "string", 723 "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.", 724 0, java.lang.Integer.MAX_VALUE, prefix)); 725 childrenList.add(new Property("suffix", "string", 726 "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.", 727 0, java.lang.Integer.MAX_VALUE, suffix)); 728 childrenList.add( 729 new Property("period", "Period", "Indicates the period of time when this name was valid for the named person.", 730 0, java.lang.Integer.MAX_VALUE, period)); 731 } 732 733 @Override 734 public void setProperty(String name, Base value) throws FHIRException { 735 if (name.equals("use")) 736 this.use = new NameUseEnumFactory().fromType(value); // Enumeration<NameUse> 737 else if (name.equals("text")) 738 this.text = castToString(value); // StringType 739 else if (name.equals("family")) 740 this.getFamily().add(castToString(value)); 741 else if (name.equals("given")) 742 this.getGiven().add(castToString(value)); 743 else if (name.equals("prefix")) 744 this.getPrefix().add(castToString(value)); 745 else if (name.equals("suffix")) 746 this.getSuffix().add(castToString(value)); 747 else if (name.equals("period")) 748 this.period = castToPeriod(value); // Period 749 else 750 super.setProperty(name, value); 751 } 752 753 @Override 754 public Base addChild(String name) throws FHIRException { 755 if (name.equals("use")) { 756 throw new FHIRException("Cannot call addChild on a singleton property HumanName.use"); 757 } else if (name.equals("text")) { 758 throw new FHIRException("Cannot call addChild on a singleton property HumanName.text"); 759 } else if (name.equals("family")) { 760 throw new FHIRException("Cannot call addChild on a singleton property HumanName.family"); 761 } else if (name.equals("given")) { 762 throw new FHIRException("Cannot call addChild on a singleton property HumanName.given"); 763 } else if (name.equals("prefix")) { 764 throw new FHIRException("Cannot call addChild on a singleton property HumanName.prefix"); 765 } else if (name.equals("suffix")) { 766 throw new FHIRException("Cannot call addChild on a singleton property HumanName.suffix"); 767 } else if (name.equals("period")) { 768 this.period = new Period(); 769 return this.period; 770 } else 771 return super.addChild(name); 772 } 773 774 public String fhirType() { 775 return "HumanName"; 776 777 } 778 779 public HumanName copy() { 780 HumanName dst = new HumanName(); 781 copyValues(dst); 782 dst.use = use == null ? null : use.copy(); 783 dst.text = text == null ? null : text.copy(); 784 if (family != null) { 785 dst.family = new ArrayList<StringType>(); 786 for (StringType i : family) 787 dst.family.add(i.copy()); 788 } 789 ; 790 if (given != null) { 791 dst.given = new ArrayList<StringType>(); 792 for (StringType i : given) 793 dst.given.add(i.copy()); 794 } 795 ; 796 if (prefix != null) { 797 dst.prefix = new ArrayList<StringType>(); 798 for (StringType i : prefix) 799 dst.prefix.add(i.copy()); 800 } 801 ; 802 if (suffix != null) { 803 dst.suffix = new ArrayList<StringType>(); 804 for (StringType i : suffix) 805 dst.suffix.add(i.copy()); 806 } 807 ; 808 dst.period = period == null ? null : period.copy(); 809 return dst; 810 } 811 812 protected HumanName typedCopy() { 813 return copy(); 814 } 815 816 @Override 817 public boolean equalsDeep(Base other) { 818 if (!super.equalsDeep(other)) 819 return false; 820 if (!(other instanceof HumanName)) 821 return false; 822 HumanName o = (HumanName) other; 823 return compareDeep(use, o.use, true) && compareDeep(text, o.text, true) && compareDeep(family, o.family, true) 824 && compareDeep(given, o.given, true) && compareDeep(prefix, o.prefix, true) 825 && compareDeep(suffix, o.suffix, true) && compareDeep(period, o.period, true); 826 } 827 828 @Override 829 public boolean equalsShallow(Base other) { 830 if (!super.equalsShallow(other)) 831 return false; 832 if (!(other instanceof HumanName)) 833 return false; 834 HumanName o = (HumanName) other; 835 return compareValues(use, o.use, true) && compareValues(text, o.text, true) && compareValues(family, o.family, true) 836 && compareValues(given, o.given, true) && compareValues(prefix, o.prefix, true) 837 && compareValues(suffix, o.suffix, true); 838 } 839 840 public boolean isEmpty() { 841 return super.isEmpty() && (use == null || use.isEmpty()) && (text == null || text.isEmpty()) 842 && (family == null || family.isEmpty()) && (given == null || given.isEmpty()) 843 && (prefix == null || prefix.isEmpty()) && (suffix == null || suffix.isEmpty()) 844 && (period == null || period.isEmpty()); 845 } 846 847}