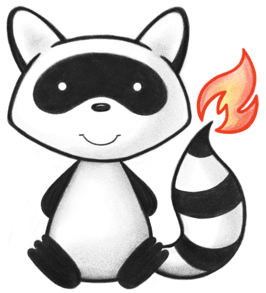
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.List; 034 035import ca.uhn.fhir.model.api.annotation.Child; 036import ca.uhn.fhir.model.api.annotation.DatatypeDef; 037import ca.uhn.fhir.model.api.annotation.Description; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.utilities.Utilities; 041 042/** 043 * A technical identifier - identifies some entity uniquely and unambiguously. 044 */ 045@DatatypeDef(name = "Identifier") 046public class Identifier extends Type implements ICompositeType { 047 048 public enum IdentifierUse { 049 /** 050 * The identifier recommended for display and use in real-world interactions. 051 */ 052 USUAL, 053 /** 054 * The identifier considered to be most trusted for the identification of this 055 * item. 056 */ 057 OFFICIAL, 058 /** 059 * A temporary identifier. 060 */ 061 TEMP, 062 /** 063 * An identifier that was assigned in secondary use - it serves to identify the 064 * object in a relative context, but cannot be consistently assigned to the same 065 * object again in a different context. 066 */ 067 SECONDARY, 068 /** 069 * added to help the parsers 070 */ 071 NULL; 072 073 public static IdentifierUse fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("usual".equals(codeString)) 077 return USUAL; 078 if ("official".equals(codeString)) 079 return OFFICIAL; 080 if ("temp".equals(codeString)) 081 return TEMP; 082 if ("secondary".equals(codeString)) 083 return SECONDARY; 084 throw new FHIRException("Unknown IdentifierUse code '" + codeString + "'"); 085 } 086 087 public String toCode() { 088 switch (this) { 089 case USUAL: 090 return "usual"; 091 case OFFICIAL: 092 return "official"; 093 case TEMP: 094 return "temp"; 095 case SECONDARY: 096 return "secondary"; 097 case NULL: 098 return null; 099 default: 100 return "?"; 101 } 102 } 103 104 public String getSystem() { 105 switch (this) { 106 case USUAL: 107 return "http://hl7.org/fhir/identifier-use"; 108 case OFFICIAL: 109 return "http://hl7.org/fhir/identifier-use"; 110 case TEMP: 111 return "http://hl7.org/fhir/identifier-use"; 112 case SECONDARY: 113 return "http://hl7.org/fhir/identifier-use"; 114 case NULL: 115 return null; 116 default: 117 return "?"; 118 } 119 } 120 121 public String getDefinition() { 122 switch (this) { 123 case USUAL: 124 return "The identifier recommended for display and use in real-world interactions."; 125 case OFFICIAL: 126 return "The identifier considered to be most trusted for the identification of this item."; 127 case TEMP: 128 return "A temporary identifier."; 129 case SECONDARY: 130 return "An identifier that was assigned in secondary use - it serves to identify the object in a relative context, but cannot be consistently assigned to the same object again in a different context."; 131 case NULL: 132 return null; 133 default: 134 return "?"; 135 } 136 } 137 138 public String getDisplay() { 139 switch (this) { 140 case USUAL: 141 return "Usual"; 142 case OFFICIAL: 143 return "Official"; 144 case TEMP: 145 return "Temp"; 146 case SECONDARY: 147 return "Secondary"; 148 case NULL: 149 return null; 150 default: 151 return "?"; 152 } 153 } 154 } 155 156 public static class IdentifierUseEnumFactory implements EnumFactory<IdentifierUse> { 157 public IdentifierUse fromCode(String codeString) throws IllegalArgumentException { 158 if (codeString == null || "".equals(codeString)) 159 if (codeString == null || "".equals(codeString)) 160 return null; 161 if ("usual".equals(codeString)) 162 return IdentifierUse.USUAL; 163 if ("official".equals(codeString)) 164 return IdentifierUse.OFFICIAL; 165 if ("temp".equals(codeString)) 166 return IdentifierUse.TEMP; 167 if ("secondary".equals(codeString)) 168 return IdentifierUse.SECONDARY; 169 throw new IllegalArgumentException("Unknown IdentifierUse code '" + codeString + "'"); 170 } 171 172 public Enumeration<IdentifierUse> fromType(Base code) throws FHIRException { 173 if (code == null || code.isEmpty()) 174 return null; 175 String codeString = ((PrimitiveType) code).asStringValue(); 176 if (codeString == null || "".equals(codeString)) 177 return null; 178 if ("usual".equals(codeString)) 179 return new Enumeration<IdentifierUse>(this, IdentifierUse.USUAL); 180 if ("official".equals(codeString)) 181 return new Enumeration<IdentifierUse>(this, IdentifierUse.OFFICIAL); 182 if ("temp".equals(codeString)) 183 return new Enumeration<IdentifierUse>(this, IdentifierUse.TEMP); 184 if ("secondary".equals(codeString)) 185 return new Enumeration<IdentifierUse>(this, IdentifierUse.SECONDARY); 186 throw new FHIRException("Unknown IdentifierUse code '" + codeString + "'"); 187 } 188 189 public String toCode(IdentifierUse code) 190 { 191 if (code == IdentifierUse.NULL) 192 return null; 193 if (code == IdentifierUse.USUAL) 194 return "usual"; 195 if (code == IdentifierUse.OFFICIAL) 196 return "official"; 197 if (code == IdentifierUse.TEMP) 198 return "temp"; 199 if (code == IdentifierUse.SECONDARY) 200 return "secondary"; 201 return "?"; 202 } 203 } 204 205 /** 206 * The purpose of this identifier. 207 */ 208 @Child(name = "use", type = { CodeType.class }, order = 0, min = 0, max = 1, modifier = true, summary = true) 209 @Description(shortDefinition = "usual | official | temp | secondary (If known)", formalDefinition = "The purpose of this identifier.") 210 protected Enumeration<IdentifierUse> use; 211 212 /** 213 * A coded type for the identifier that can be used to determine which 214 * identifier to use for a specific purpose. 215 */ 216 @Child(name = "type", type = { CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 217 @Description(shortDefinition = "Description of identifier", formalDefinition = "A coded type for the identifier that can be used to determine which identifier to use for a specific purpose.") 218 protected CodeableConcept type; 219 220 /** 221 * Establishes the namespace in which set of possible id values is unique. 222 */ 223 @Child(name = "system", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 224 @Description(shortDefinition = "The namespace for the identifier", formalDefinition = "Establishes the namespace in which set of possible id values is unique.") 225 protected UriType system; 226 227 /** 228 * The portion of the identifier typically displayed to the user and which is 229 * unique within the context of the system. 230 */ 231 @Child(name = "value", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 232 @Description(shortDefinition = "The value that is unique", formalDefinition = "The portion of the identifier typically displayed to the user and which is unique within the context of the system.") 233 protected StringType value; 234 235 /** 236 * Time period during which identifier is/was valid for use. 237 */ 238 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 239 @Description(shortDefinition = "Time period when id is/was valid for use", formalDefinition = "Time period during which identifier is/was valid for use.") 240 protected Period period; 241 242 /** 243 * Organization that issued/manages the identifier. 244 */ 245 @Child(name = "assigner", type = { 246 Organization.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 247 @Description(shortDefinition = "Organization that issued id (may be just text)", formalDefinition = "Organization that issued/manages the identifier.") 248 protected Reference assigner; 249 250 /** 251 * The actual object that is the target of the reference (Organization that 252 * issued/manages the identifier.) 253 */ 254 protected Organization assignerTarget; 255 256 private static final long serialVersionUID = -478840981L; 257 258 /* 259 * Constructor 260 */ 261 public Identifier() { 262 super(); 263 } 264 265 /** 266 * @return {@link #use} (The purpose of this identifier.). This is the 267 * underlying object with id, value and extensions. The accessor 268 * "getUse" gives direct access to the value 269 */ 270 public Enumeration<IdentifierUse> getUseElement() { 271 if (this.use == null) 272 if (Configuration.errorOnAutoCreate()) 273 throw new Error("Attempt to auto-create Identifier.use"); 274 else if (Configuration.doAutoCreate()) 275 this.use = new Enumeration<IdentifierUse>(new IdentifierUseEnumFactory()); // bb 276 return this.use; 277 } 278 279 public boolean hasUseElement() { 280 return this.use != null && !this.use.isEmpty(); 281 } 282 283 public boolean hasUse() { 284 return this.use != null && !this.use.isEmpty(); 285 } 286 287 /** 288 * @param value {@link #use} (The purpose of this identifier.). This is the 289 * underlying object with id, value and extensions. The accessor 290 * "getUse" gives direct access to the value 291 */ 292 public Identifier setUseElement(Enumeration<IdentifierUse> value) { 293 this.use = value; 294 return this; 295 } 296 297 /** 298 * @return The purpose of this identifier. 299 */ 300 public IdentifierUse getUse() { 301 return this.use == null ? null : this.use.getValue(); 302 } 303 304 /** 305 * @param value The purpose of this identifier. 306 */ 307 public Identifier setUse(IdentifierUse value) { 308 if (value == null) 309 this.use = null; 310 else { 311 if (this.use == null) 312 this.use = new Enumeration<IdentifierUse>(new IdentifierUseEnumFactory()); 313 this.use.setValue(value); 314 } 315 return this; 316 } 317 318 /** 319 * @return {@link #type} (A coded type for the identifier that can be used to 320 * determine which identifier to use for a specific purpose.) 321 */ 322 public CodeableConcept getType() { 323 if (this.type == null) 324 if (Configuration.errorOnAutoCreate()) 325 throw new Error("Attempt to auto-create Identifier.type"); 326 else if (Configuration.doAutoCreate()) 327 this.type = new CodeableConcept(); // cc 328 return this.type; 329 } 330 331 public boolean hasType() { 332 return this.type != null && !this.type.isEmpty(); 333 } 334 335 /** 336 * @param value {@link #type} (A coded type for the identifier that can be used 337 * to determine which identifier to use for a specific purpose.) 338 */ 339 public Identifier setType(CodeableConcept value) { 340 this.type = value; 341 return this; 342 } 343 344 /** 345 * @return {@link #system} (Establishes the namespace in which set of possible 346 * id values is unique.). This is the underlying object with id, value 347 * and extensions. The accessor "getSystem" gives direct access to the 348 * value 349 */ 350 public UriType getSystemElement() { 351 if (this.system == null) 352 if (Configuration.errorOnAutoCreate()) 353 throw new Error("Attempt to auto-create Identifier.system"); 354 else if (Configuration.doAutoCreate()) 355 this.system = new UriType(); // bb 356 return this.system; 357 } 358 359 public boolean hasSystemElement() { 360 return this.system != null && !this.system.isEmpty(); 361 } 362 363 public boolean hasSystem() { 364 return this.system != null && !this.system.isEmpty(); 365 } 366 367 /** 368 * @param value {@link #system} (Establishes the namespace in which set of 369 * possible id values is unique.). This is the underlying object 370 * with id, value and extensions. The accessor "getSystem" gives 371 * direct access to the value 372 */ 373 public Identifier setSystemElement(UriType value) { 374 this.system = value; 375 return this; 376 } 377 378 /** 379 * @return Establishes the namespace in which set of possible id values is 380 * unique. 381 */ 382 public String getSystem() { 383 return this.system == null ? null : this.system.getValue(); 384 } 385 386 /** 387 * @param value Establishes the namespace in which set of possible id values is 388 * unique. 389 */ 390 public Identifier setSystem(String value) { 391 if (Utilities.noString(value)) 392 this.system = null; 393 else { 394 if (this.system == null) 395 this.system = new UriType(); 396 this.system.setValue(value); 397 } 398 return this; 399 } 400 401 /** 402 * @return {@link #value} (The portion of the identifier typically displayed to 403 * the user and which is unique within the context of the system.). This 404 * is the underlying object with id, value and extensions. The accessor 405 * "getValue" gives direct access to the value 406 */ 407 public StringType getValueElement() { 408 if (this.value == null) 409 if (Configuration.errorOnAutoCreate()) 410 throw new Error("Attempt to auto-create Identifier.value"); 411 else if (Configuration.doAutoCreate()) 412 this.value = new StringType(); // bb 413 return this.value; 414 } 415 416 public boolean hasValueElement() { 417 return this.value != null && !this.value.isEmpty(); 418 } 419 420 public boolean hasValue() { 421 return this.value != null && !this.value.isEmpty(); 422 } 423 424 /** 425 * @param value {@link #value} (The portion of the identifier typically 426 * displayed to the user and which is unique within the context of 427 * the system.). This is the underlying object with id, value and 428 * extensions. The accessor "getValue" gives direct access to the 429 * value 430 */ 431 public Identifier setValueElement(StringType value) { 432 this.value = value; 433 return this; 434 } 435 436 /** 437 * @return The portion of the identifier typically displayed to the user and 438 * which is unique within the context of the system. 439 */ 440 public String getValue() { 441 return this.value == null ? null : this.value.getValue(); 442 } 443 444 /** 445 * @param value The portion of the identifier typically displayed to the user 446 * and which is unique within the context of the system. 447 */ 448 public Identifier setValue(String value) { 449 if (Utilities.noString(value)) 450 this.value = null; 451 else { 452 if (this.value == null) 453 this.value = new StringType(); 454 this.value.setValue(value); 455 } 456 return this; 457 } 458 459 /** 460 * @return {@link #period} (Time period during which identifier is/was valid for 461 * use.) 462 */ 463 public Period getPeriod() { 464 if (this.period == null) 465 if (Configuration.errorOnAutoCreate()) 466 throw new Error("Attempt to auto-create Identifier.period"); 467 else if (Configuration.doAutoCreate()) 468 this.period = new Period(); // cc 469 return this.period; 470 } 471 472 public boolean hasPeriod() { 473 return this.period != null && !this.period.isEmpty(); 474 } 475 476 /** 477 * @param value {@link #period} (Time period during which identifier is/was 478 * valid for use.) 479 */ 480 public Identifier setPeriod(Period value) { 481 this.period = value; 482 return this; 483 } 484 485 /** 486 * @return {@link #assigner} (Organization that issued/manages the identifier.) 487 */ 488 public Reference getAssigner() { 489 if (this.assigner == null) 490 if (Configuration.errorOnAutoCreate()) 491 throw new Error("Attempt to auto-create Identifier.assigner"); 492 else if (Configuration.doAutoCreate()) 493 this.assigner = new Reference(); // cc 494 return this.assigner; 495 } 496 497 public boolean hasAssigner() { 498 return this.assigner != null && !this.assigner.isEmpty(); 499 } 500 501 /** 502 * @param value {@link #assigner} (Organization that issued/manages the 503 * identifier.) 504 */ 505 public Identifier setAssigner(Reference value) { 506 this.assigner = value; 507 return this; 508 } 509 510 /** 511 * @return {@link #assigner} The actual object that is the target of the 512 * reference. The reference library doesn't populate this, but you can 513 * use it to hold the resource if you resolve it. (Organization that 514 * issued/manages the identifier.) 515 */ 516 public Organization getAssignerTarget() { 517 if (this.assignerTarget == null) 518 if (Configuration.errorOnAutoCreate()) 519 throw new Error("Attempt to auto-create Identifier.assigner"); 520 else if (Configuration.doAutoCreate()) 521 this.assignerTarget = new Organization(); // aa 522 return this.assignerTarget; 523 } 524 525 /** 526 * @param value {@link #assigner} The actual object that is the target of the 527 * reference. The reference library doesn't use these, but you can 528 * use it to hold the resource if you resolve it. (Organization 529 * that issued/manages the identifier.) 530 */ 531 public Identifier setAssignerTarget(Organization value) { 532 this.assignerTarget = value; 533 return this; 534 } 535 536 protected void listChildren(List<Property> childrenList) { 537 super.listChildren(childrenList); 538 childrenList 539 .add(new Property("use", "code", "The purpose of this identifier.", 0, java.lang.Integer.MAX_VALUE, use)); 540 childrenList.add(new Property("type", "CodeableConcept", 541 "A coded type for the identifier that can be used to determine which identifier to use for a specific purpose.", 542 0, java.lang.Integer.MAX_VALUE, type)); 543 childrenList 544 .add(new Property("system", "uri", "Establishes the namespace in which set of possible id values is unique.", 0, 545 java.lang.Integer.MAX_VALUE, system)); 546 childrenList.add(new Property("value", "string", 547 "The portion of the identifier typically displayed to the user and which is unique within the context of the system.", 548 0, java.lang.Integer.MAX_VALUE, value)); 549 childrenList.add(new Property("period", "Period", "Time period during which identifier is/was valid for use.", 0, 550 java.lang.Integer.MAX_VALUE, period)); 551 childrenList.add(new Property("assigner", "Reference(Organization)", 552 "Organization that issued/manages the identifier.", 0, java.lang.Integer.MAX_VALUE, assigner)); 553 } 554 555 @Override 556 public void setProperty(String name, Base value) throws FHIRException { 557 if (name.equals("use")) 558 this.use = new IdentifierUseEnumFactory().fromType(value); // Enumeration<IdentifierUse> 559 else if (name.equals("type")) 560 this.type = castToCodeableConcept(value); // CodeableConcept 561 else if (name.equals("system")) 562 this.system = castToUri(value); // UriType 563 else if (name.equals("value")) 564 this.value = castToString(value); // StringType 565 else if (name.equals("period")) 566 this.period = castToPeriod(value); // Period 567 else if (name.equals("assigner")) 568 this.assigner = castToReference(value); // Reference 569 else 570 super.setProperty(name, value); 571 } 572 573 @Override 574 public Base addChild(String name) throws FHIRException { 575 if (name.equals("use")) { 576 throw new FHIRException("Cannot call addChild on a singleton property Identifier.use"); 577 } else if (name.equals("type")) { 578 this.type = new CodeableConcept(); 579 return this.type; 580 } else if (name.equals("system")) { 581 throw new FHIRException("Cannot call addChild on a singleton property Identifier.system"); 582 } else if (name.equals("value")) { 583 throw new FHIRException("Cannot call addChild on a singleton property Identifier.value"); 584 } else if (name.equals("period")) { 585 this.period = new Period(); 586 return this.period; 587 } else if (name.equals("assigner")) { 588 this.assigner = new Reference(); 589 return this.assigner; 590 } else 591 return super.addChild(name); 592 } 593 594 public String fhirType() { 595 return "Identifier"; 596 597 } 598 599 public Identifier copy() { 600 Identifier dst = new Identifier(); 601 copyValues(dst); 602 dst.use = use == null ? null : use.copy(); 603 dst.type = type == null ? null : type.copy(); 604 dst.system = system == null ? null : system.copy(); 605 dst.value = value == null ? null : value.copy(); 606 dst.period = period == null ? null : period.copy(); 607 dst.assigner = assigner == null ? null : assigner.copy(); 608 return dst; 609 } 610 611 protected Identifier typedCopy() { 612 return copy(); 613 } 614 615 @Override 616 public boolean equalsDeep(Base other) { 617 if (!super.equalsDeep(other)) 618 return false; 619 if (!(other instanceof Identifier)) 620 return false; 621 Identifier o = (Identifier) other; 622 return compareDeep(use, o.use, true) && compareDeep(type, o.type, true) && compareDeep(system, o.system, true) 623 && compareDeep(value, o.value, true) && compareDeep(period, o.period, true) 624 && compareDeep(assigner, o.assigner, true); 625 } 626 627 @Override 628 public boolean equalsShallow(Base other) { 629 if (!super.equalsShallow(other)) 630 return false; 631 if (!(other instanceof Identifier)) 632 return false; 633 Identifier o = (Identifier) other; 634 return compareValues(use, o.use, true) && compareValues(system, o.system, true) 635 && compareValues(value, o.value, true); 636 } 637 638 public boolean isEmpty() { 639 return super.isEmpty() && (use == null || use.isEmpty()) && (type == null || type.isEmpty()) 640 && (system == null || system.isEmpty()) && (value == null || value.isEmpty()) 641 && (period == null || period.isEmpty()) && (assigner == null || assigner.isEmpty()); 642 } 643 644}