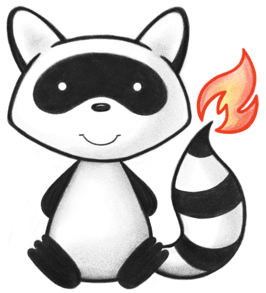
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * A manifest of a set of DICOM Service-Object Pair Instances (SOP Instances). 048 * The referenced SOP Instances (images or other content) are for a single 049 * patient, and may be from one or more studies. The referenced SOP Instances 050 * have been selected for a purpose, such as quality assurance, conference, or 051 * consult. Reflecting that range of purposes, typical ImagingObjectSelection 052 * resources may include all SOP Instances in a study (perhaps for sharing 053 * through a Health Information Exchange); key images from multiple studies (for 054 * reference by a referring or treating physician); a multi-frame ultrasound 055 * instance ("cine" video clip) and a set of measurements taken from that 056 * instance (for inclusion in a teaching file); and so on. 057 */ 058@ResourceDef(name = "ImagingObjectSelection", profile = "http://hl7.org/fhir/Profile/ImagingObjectSelection") 059public class ImagingObjectSelection extends DomainResource { 060 061 @Block() 062 public static class StudyComponent extends BackboneElement implements IBaseBackboneElement { 063 /** 064 * Study instance UID of the SOP instances in the selection. 065 */ 066 @Child(name = "uid", type = { OidType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 067 @Description(shortDefinition = "Study instance UID", formalDefinition = "Study instance UID of the SOP instances in the selection.") 068 protected OidType uid; 069 070 /** 071 * WADO-RS URL to retrieve the study. Note that this URL retrieves all SOP 072 * instances of the study, not only those in the selection. 073 */ 074 @Child(name = "url", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 075 @Description(shortDefinition = "Retrieve study URL", formalDefinition = "WADO-RS URL to retrieve the study. Note that this URL retrieves all SOP instances of the study, not only those in the selection.") 076 protected UriType url; 077 078 /** 079 * Reference to the Imaging Study in FHIR form. 080 */ 081 @Child(name = "imagingStudy", type = { 082 ImagingStudy.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 083 @Description(shortDefinition = "Reference to ImagingStudy", formalDefinition = "Reference to the Imaging Study in FHIR form.") 084 protected Reference imagingStudy; 085 086 /** 087 * The actual object that is the target of the reference (Reference to the 088 * Imaging Study in FHIR form.) 089 */ 090 protected ImagingStudy imagingStudyTarget; 091 092 /** 093 * Series identity and locating information of the DICOM SOP instances in the 094 * selection. 095 */ 096 @Child(name = "series", type = {}, order = 4, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 097 @Description(shortDefinition = "Series identity of the selected instances", formalDefinition = "Series identity and locating information of the DICOM SOP instances in the selection.") 098 protected List<SeriesComponent> series; 099 100 private static final long serialVersionUID = 341246743L; 101 102 /* 103 * Constructor 104 */ 105 public StudyComponent() { 106 super(); 107 } 108 109 /* 110 * Constructor 111 */ 112 public StudyComponent(OidType uid) { 113 super(); 114 this.uid = uid; 115 } 116 117 /** 118 * @return {@link #uid} (Study instance UID of the SOP instances in the 119 * selection.). This is the underlying object with id, value and 120 * extensions. The accessor "getUid" gives direct access to the value 121 */ 122 public OidType getUidElement() { 123 if (this.uid == null) 124 if (Configuration.errorOnAutoCreate()) 125 throw new Error("Attempt to auto-create StudyComponent.uid"); 126 else if (Configuration.doAutoCreate()) 127 this.uid = new OidType(); // bb 128 return this.uid; 129 } 130 131 public boolean hasUidElement() { 132 return this.uid != null && !this.uid.isEmpty(); 133 } 134 135 public boolean hasUid() { 136 return this.uid != null && !this.uid.isEmpty(); 137 } 138 139 /** 140 * @param value {@link #uid} (Study instance UID of the SOP instances in the 141 * selection.). This is the underlying object with id, value and 142 * extensions. The accessor "getUid" gives direct access to the 143 * value 144 */ 145 public StudyComponent setUidElement(OidType value) { 146 this.uid = value; 147 return this; 148 } 149 150 /** 151 * @return Study instance UID of the SOP instances in the selection. 152 */ 153 public String getUid() { 154 return this.uid == null ? null : this.uid.getValue(); 155 } 156 157 /** 158 * @param value Study instance UID of the SOP instances in the selection. 159 */ 160 public StudyComponent setUid(String value) { 161 if (this.uid == null) 162 this.uid = new OidType(); 163 this.uid.setValue(value); 164 return this; 165 } 166 167 /** 168 * @return {@link #url} (WADO-RS URL to retrieve the study. Note that this URL 169 * retrieves all SOP instances of the study, not only those in the 170 * selection.). This is the underlying object with id, value and 171 * extensions. The accessor "getUrl" gives direct access to the value 172 */ 173 public UriType getUrlElement() { 174 if (this.url == null) 175 if (Configuration.errorOnAutoCreate()) 176 throw new Error("Attempt to auto-create StudyComponent.url"); 177 else if (Configuration.doAutoCreate()) 178 this.url = new UriType(); // bb 179 return this.url; 180 } 181 182 public boolean hasUrlElement() { 183 return this.url != null && !this.url.isEmpty(); 184 } 185 186 public boolean hasUrl() { 187 return this.url != null && !this.url.isEmpty(); 188 } 189 190 /** 191 * @param value {@link #url} (WADO-RS URL to retrieve the study. Note that this 192 * URL retrieves all SOP instances of the study, not only those in 193 * the selection.). This is the underlying object with id, value 194 * and extensions. The accessor "getUrl" gives direct access to the 195 * value 196 */ 197 public StudyComponent setUrlElement(UriType value) { 198 this.url = value; 199 return this; 200 } 201 202 /** 203 * @return WADO-RS URL to retrieve the study. Note that this URL retrieves all 204 * SOP instances of the study, not only those in the selection. 205 */ 206 public String getUrl() { 207 return this.url == null ? null : this.url.getValue(); 208 } 209 210 /** 211 * @param value WADO-RS URL to retrieve the study. Note that this URL retrieves 212 * all SOP instances of the study, not only those in the selection. 213 */ 214 public StudyComponent setUrl(String value) { 215 if (Utilities.noString(value)) 216 this.url = null; 217 else { 218 if (this.url == null) 219 this.url = new UriType(); 220 this.url.setValue(value); 221 } 222 return this; 223 } 224 225 /** 226 * @return {@link #imagingStudy} (Reference to the Imaging Study in FHIR form.) 227 */ 228 public Reference getImagingStudy() { 229 if (this.imagingStudy == null) 230 if (Configuration.errorOnAutoCreate()) 231 throw new Error("Attempt to auto-create StudyComponent.imagingStudy"); 232 else if (Configuration.doAutoCreate()) 233 this.imagingStudy = new Reference(); // cc 234 return this.imagingStudy; 235 } 236 237 public boolean hasImagingStudy() { 238 return this.imagingStudy != null && !this.imagingStudy.isEmpty(); 239 } 240 241 /** 242 * @param value {@link #imagingStudy} (Reference to the Imaging Study in FHIR 243 * form.) 244 */ 245 public StudyComponent setImagingStudy(Reference value) { 246 this.imagingStudy = value; 247 return this; 248 } 249 250 /** 251 * @return {@link #imagingStudy} The actual object that is the target of the 252 * reference. The reference library doesn't populate this, but you can 253 * use it to hold the resource if you resolve it. (Reference to the 254 * Imaging Study in FHIR form.) 255 */ 256 public ImagingStudy getImagingStudyTarget() { 257 if (this.imagingStudyTarget == null) 258 if (Configuration.errorOnAutoCreate()) 259 throw new Error("Attempt to auto-create StudyComponent.imagingStudy"); 260 else if (Configuration.doAutoCreate()) 261 this.imagingStudyTarget = new ImagingStudy(); // aa 262 return this.imagingStudyTarget; 263 } 264 265 /** 266 * @param value {@link #imagingStudy} The actual object that is the target of 267 * the reference. The reference library doesn't use these, but you 268 * can use it to hold the resource if you resolve it. (Reference to 269 * the Imaging Study in FHIR form.) 270 */ 271 public StudyComponent setImagingStudyTarget(ImagingStudy value) { 272 this.imagingStudyTarget = value; 273 return this; 274 } 275 276 /** 277 * @return {@link #series} (Series identity and locating information of the 278 * DICOM SOP instances in the selection.) 279 */ 280 public List<SeriesComponent> getSeries() { 281 if (this.series == null) 282 this.series = new ArrayList<SeriesComponent>(); 283 return this.series; 284 } 285 286 public boolean hasSeries() { 287 if (this.series == null) 288 return false; 289 for (SeriesComponent item : this.series) 290 if (!item.isEmpty()) 291 return true; 292 return false; 293 } 294 295 /** 296 * @return {@link #series} (Series identity and locating information of the 297 * DICOM SOP instances in the selection.) 298 */ 299 // syntactic sugar 300 public SeriesComponent addSeries() { // 3 301 SeriesComponent t = new SeriesComponent(); 302 if (this.series == null) 303 this.series = new ArrayList<SeriesComponent>(); 304 this.series.add(t); 305 return t; 306 } 307 308 // syntactic sugar 309 public StudyComponent addSeries(SeriesComponent t) { // 3 310 if (t == null) 311 return this; 312 if (this.series == null) 313 this.series = new ArrayList<SeriesComponent>(); 314 this.series.add(t); 315 return this; 316 } 317 318 protected void listChildren(List<Property> childrenList) { 319 super.listChildren(childrenList); 320 childrenList.add(new Property("uid", "oid", "Study instance UID of the SOP instances in the selection.", 0, 321 java.lang.Integer.MAX_VALUE, uid)); 322 childrenList.add(new Property("url", "uri", 323 "WADO-RS URL to retrieve the study. Note that this URL retrieves all SOP instances of the study, not only those in the selection.", 324 0, java.lang.Integer.MAX_VALUE, url)); 325 childrenList.add(new Property("imagingStudy", "Reference(ImagingStudy)", 326 "Reference to the Imaging Study in FHIR form.", 0, java.lang.Integer.MAX_VALUE, imagingStudy)); 327 childrenList.add(new Property("series", "", 328 "Series identity and locating information of the DICOM SOP instances in the selection.", 0, 329 java.lang.Integer.MAX_VALUE, series)); 330 } 331 332 @Override 333 public void setProperty(String name, Base value) throws FHIRException { 334 if (name.equals("uid")) 335 this.uid = castToOid(value); // OidType 336 else if (name.equals("url")) 337 this.url = castToUri(value); // UriType 338 else if (name.equals("imagingStudy")) 339 this.imagingStudy = castToReference(value); // Reference 340 else if (name.equals("series")) 341 this.getSeries().add((SeriesComponent) value); 342 else 343 super.setProperty(name, value); 344 } 345 346 @Override 347 public Base addChild(String name) throws FHIRException { 348 if (name.equals("uid")) { 349 throw new FHIRException("Cannot call addChild on a singleton property ImagingObjectSelection.uid"); 350 } else if (name.equals("url")) { 351 throw new FHIRException("Cannot call addChild on a singleton property ImagingObjectSelection.url"); 352 } else if (name.equals("imagingStudy")) { 353 this.imagingStudy = new Reference(); 354 return this.imagingStudy; 355 } else if (name.equals("series")) { 356 return addSeries(); 357 } else 358 return super.addChild(name); 359 } 360 361 public StudyComponent copy() { 362 StudyComponent dst = new StudyComponent(); 363 copyValues(dst); 364 dst.uid = uid == null ? null : uid.copy(); 365 dst.url = url == null ? null : url.copy(); 366 dst.imagingStudy = imagingStudy == null ? null : imagingStudy.copy(); 367 if (series != null) { 368 dst.series = new ArrayList<SeriesComponent>(); 369 for (SeriesComponent i : series) 370 dst.series.add(i.copy()); 371 } 372 ; 373 return dst; 374 } 375 376 @Override 377 public boolean equalsDeep(Base other) { 378 if (!super.equalsDeep(other)) 379 return false; 380 if (!(other instanceof StudyComponent)) 381 return false; 382 StudyComponent o = (StudyComponent) other; 383 return compareDeep(uid, o.uid, true) && compareDeep(url, o.url, true) 384 && compareDeep(imagingStudy, o.imagingStudy, true) && compareDeep(series, o.series, true); 385 } 386 387 @Override 388 public boolean equalsShallow(Base other) { 389 if (!super.equalsShallow(other)) 390 return false; 391 if (!(other instanceof StudyComponent)) 392 return false; 393 StudyComponent o = (StudyComponent) other; 394 return compareValues(uid, o.uid, true) && compareValues(url, o.url, true); 395 } 396 397 public boolean isEmpty() { 398 return super.isEmpty() && (uid == null || uid.isEmpty()) && (url == null || url.isEmpty()) 399 && (imagingStudy == null || imagingStudy.isEmpty()) && (series == null || series.isEmpty()); 400 } 401 402 public String fhirType() { 403 return "ImagingObjectSelection.study"; 404 405 } 406 407 } 408 409 @Block() 410 public static class SeriesComponent extends BackboneElement implements IBaseBackboneElement { 411 /** 412 * Series instance UID of the SOP instances in the selection. 413 */ 414 @Child(name = "uid", type = { OidType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 415 @Description(shortDefinition = "Series instance UID", formalDefinition = "Series instance UID of the SOP instances in the selection.") 416 protected OidType uid; 417 418 /** 419 * WADO-RS URL to retrieve the series. Note that this URL retrieves all SOP 420 * instances of the series not only those in the selection. 421 */ 422 @Child(name = "url", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 423 @Description(shortDefinition = "Retrieve series URL", formalDefinition = "WADO-RS URL to retrieve the series. Note that this URL retrieves all SOP instances of the series not only those in the selection.") 424 protected UriType url; 425 426 /** 427 * Identity and locating information of the selected DICOM SOP instances. 428 */ 429 @Child(name = "instance", type = {}, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 430 @Description(shortDefinition = "The selected instance", formalDefinition = "Identity and locating information of the selected DICOM SOP instances.") 431 protected List<InstanceComponent> instance; 432 433 private static final long serialVersionUID = 229247770L; 434 435 /* 436 * Constructor 437 */ 438 public SeriesComponent() { 439 super(); 440 } 441 442 /** 443 * @return {@link #uid} (Series instance UID of the SOP instances in the 444 * selection.). This is the underlying object with id, value and 445 * extensions. The accessor "getUid" gives direct access to the value 446 */ 447 public OidType getUidElement() { 448 if (this.uid == null) 449 if (Configuration.errorOnAutoCreate()) 450 throw new Error("Attempt to auto-create SeriesComponent.uid"); 451 else if (Configuration.doAutoCreate()) 452 this.uid = new OidType(); // bb 453 return this.uid; 454 } 455 456 public boolean hasUidElement() { 457 return this.uid != null && !this.uid.isEmpty(); 458 } 459 460 public boolean hasUid() { 461 return this.uid != null && !this.uid.isEmpty(); 462 } 463 464 /** 465 * @param value {@link #uid} (Series instance UID of the SOP instances in the 466 * selection.). This is the underlying object with id, value and 467 * extensions. The accessor "getUid" gives direct access to the 468 * value 469 */ 470 public SeriesComponent setUidElement(OidType value) { 471 this.uid = value; 472 return this; 473 } 474 475 /** 476 * @return Series instance UID of the SOP instances in the selection. 477 */ 478 public String getUid() { 479 return this.uid == null ? null : this.uid.getValue(); 480 } 481 482 /** 483 * @param value Series instance UID of the SOP instances in the selection. 484 */ 485 public SeriesComponent setUid(String value) { 486 if (Utilities.noString(value)) 487 this.uid = null; 488 else { 489 if (this.uid == null) 490 this.uid = new OidType(); 491 this.uid.setValue(value); 492 } 493 return this; 494 } 495 496 /** 497 * @return {@link #url} (WADO-RS URL to retrieve the series. Note that this URL 498 * retrieves all SOP instances of the series not only those in the 499 * selection.). This is the underlying object with id, value and 500 * extensions. The accessor "getUrl" gives direct access to the value 501 */ 502 public UriType getUrlElement() { 503 if (this.url == null) 504 if (Configuration.errorOnAutoCreate()) 505 throw new Error("Attempt to auto-create SeriesComponent.url"); 506 else if (Configuration.doAutoCreate()) 507 this.url = new UriType(); // bb 508 return this.url; 509 } 510 511 public boolean hasUrlElement() { 512 return this.url != null && !this.url.isEmpty(); 513 } 514 515 public boolean hasUrl() { 516 return this.url != null && !this.url.isEmpty(); 517 } 518 519 /** 520 * @param value {@link #url} (WADO-RS URL to retrieve the series. Note that this 521 * URL retrieves all SOP instances of the series not only those in 522 * the selection.). This is the underlying object with id, value 523 * and extensions. The accessor "getUrl" gives direct access to the 524 * value 525 */ 526 public SeriesComponent setUrlElement(UriType value) { 527 this.url = value; 528 return this; 529 } 530 531 /** 532 * @return WADO-RS URL to retrieve the series. Note that this URL retrieves all 533 * SOP instances of the series not only those in the selection. 534 */ 535 public String getUrl() { 536 return this.url == null ? null : this.url.getValue(); 537 } 538 539 /** 540 * @param value WADO-RS URL to retrieve the series. Note that this URL retrieves 541 * all SOP instances of the series not only those in the selection. 542 */ 543 public SeriesComponent setUrl(String value) { 544 if (Utilities.noString(value)) 545 this.url = null; 546 else { 547 if (this.url == null) 548 this.url = new UriType(); 549 this.url.setValue(value); 550 } 551 return this; 552 } 553 554 /** 555 * @return {@link #instance} (Identity and locating information of the selected 556 * DICOM SOP instances.) 557 */ 558 public List<InstanceComponent> getInstance() { 559 if (this.instance == null) 560 this.instance = new ArrayList<InstanceComponent>(); 561 return this.instance; 562 } 563 564 public boolean hasInstance() { 565 if (this.instance == null) 566 return false; 567 for (InstanceComponent item : this.instance) 568 if (!item.isEmpty()) 569 return true; 570 return false; 571 } 572 573 /** 574 * @return {@link #instance} (Identity and locating information of the selected 575 * DICOM SOP instances.) 576 */ 577 // syntactic sugar 578 public InstanceComponent addInstance() { // 3 579 InstanceComponent t = new InstanceComponent(); 580 if (this.instance == null) 581 this.instance = new ArrayList<InstanceComponent>(); 582 this.instance.add(t); 583 return t; 584 } 585 586 // syntactic sugar 587 public SeriesComponent addInstance(InstanceComponent t) { // 3 588 if (t == null) 589 return this; 590 if (this.instance == null) 591 this.instance = new ArrayList<InstanceComponent>(); 592 this.instance.add(t); 593 return this; 594 } 595 596 protected void listChildren(List<Property> childrenList) { 597 super.listChildren(childrenList); 598 childrenList.add(new Property("uid", "oid", "Series instance UID of the SOP instances in the selection.", 0, 599 java.lang.Integer.MAX_VALUE, uid)); 600 childrenList.add(new Property("url", "uri", 601 "WADO-RS URL to retrieve the series. Note that this URL retrieves all SOP instances of the series not only those in the selection.", 602 0, java.lang.Integer.MAX_VALUE, url)); 603 childrenList 604 .add(new Property("instance", "", "Identity and locating information of the selected DICOM SOP instances.", 0, 605 java.lang.Integer.MAX_VALUE, instance)); 606 } 607 608 @Override 609 public void setProperty(String name, Base value) throws FHIRException { 610 if (name.equals("uid")) 611 this.uid = castToOid(value); // OidType 612 else if (name.equals("url")) 613 this.url = castToUri(value); // UriType 614 else if (name.equals("instance")) 615 this.getInstance().add((InstanceComponent) value); 616 else 617 super.setProperty(name, value); 618 } 619 620 @Override 621 public Base addChild(String name) throws FHIRException { 622 if (name.equals("uid")) { 623 throw new FHIRException("Cannot call addChild on a singleton property ImagingObjectSelection.uid"); 624 } else if (name.equals("url")) { 625 throw new FHIRException("Cannot call addChild on a singleton property ImagingObjectSelection.url"); 626 } else if (name.equals("instance")) { 627 return addInstance(); 628 } else 629 return super.addChild(name); 630 } 631 632 public SeriesComponent copy() { 633 SeriesComponent dst = new SeriesComponent(); 634 copyValues(dst); 635 dst.uid = uid == null ? null : uid.copy(); 636 dst.url = url == null ? null : url.copy(); 637 if (instance != null) { 638 dst.instance = new ArrayList<InstanceComponent>(); 639 for (InstanceComponent i : instance) 640 dst.instance.add(i.copy()); 641 } 642 ; 643 return dst; 644 } 645 646 @Override 647 public boolean equalsDeep(Base other) { 648 if (!super.equalsDeep(other)) 649 return false; 650 if (!(other instanceof SeriesComponent)) 651 return false; 652 SeriesComponent o = (SeriesComponent) other; 653 return compareDeep(uid, o.uid, true) && compareDeep(url, o.url, true) && compareDeep(instance, o.instance, true); 654 } 655 656 @Override 657 public boolean equalsShallow(Base other) { 658 if (!super.equalsShallow(other)) 659 return false; 660 if (!(other instanceof SeriesComponent)) 661 return false; 662 SeriesComponent o = (SeriesComponent) other; 663 return compareValues(uid, o.uid, true) && compareValues(url, o.url, true); 664 } 665 666 public boolean isEmpty() { 667 return super.isEmpty() && (uid == null || uid.isEmpty()) && (url == null || url.isEmpty()) 668 && (instance == null || instance.isEmpty()); 669 } 670 671 public String fhirType() { 672 return "ImagingObjectSelection.study.series"; 673 674 } 675 676 } 677 678 @Block() 679 public static class InstanceComponent extends BackboneElement implements IBaseBackboneElement { 680 /** 681 * SOP class UID of the selected instance. 682 */ 683 @Child(name = "sopClass", type = { OidType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 684 @Description(shortDefinition = "SOP class UID of instance", formalDefinition = "SOP class UID of the selected instance.") 685 protected OidType sopClass; 686 687 /** 688 * SOP Instance UID of the selected instance. 689 */ 690 @Child(name = "uid", type = { OidType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 691 @Description(shortDefinition = "Selected instance UID", formalDefinition = "SOP Instance UID of the selected instance.") 692 protected OidType uid; 693 694 /** 695 * WADO-RS URL to retrieve the DICOM SOP Instance. 696 */ 697 @Child(name = "url", type = { UriType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 698 @Description(shortDefinition = "Retrieve instance URL", formalDefinition = "WADO-RS URL to retrieve the DICOM SOP Instance.") 699 protected UriType url; 700 701 /** 702 * Identity and location information of the frames in the selected instance. 703 */ 704 @Child(name = "frames", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 705 @Description(shortDefinition = "The frame set", formalDefinition = "Identity and location information of the frames in the selected instance.") 706 protected List<FramesComponent> frames; 707 708 private static final long serialVersionUID = 1641180916L; 709 710 /* 711 * Constructor 712 */ 713 public InstanceComponent() { 714 super(); 715 } 716 717 /* 718 * Constructor 719 */ 720 public InstanceComponent(OidType sopClass, OidType uid, UriType url) { 721 super(); 722 this.sopClass = sopClass; 723 this.uid = uid; 724 this.url = url; 725 } 726 727 /** 728 * @return {@link #sopClass} (SOP class UID of the selected instance.). This is 729 * the underlying object with id, value and extensions. The accessor 730 * "getSopClass" gives direct access to the value 731 */ 732 public OidType getSopClassElement() { 733 if (this.sopClass == null) 734 if (Configuration.errorOnAutoCreate()) 735 throw new Error("Attempt to auto-create InstanceComponent.sopClass"); 736 else if (Configuration.doAutoCreate()) 737 this.sopClass = new OidType(); // bb 738 return this.sopClass; 739 } 740 741 public boolean hasSopClassElement() { 742 return this.sopClass != null && !this.sopClass.isEmpty(); 743 } 744 745 public boolean hasSopClass() { 746 return this.sopClass != null && !this.sopClass.isEmpty(); 747 } 748 749 /** 750 * @param value {@link #sopClass} (SOP class UID of the selected instance.). 751 * This is the underlying object with id, value and extensions. The 752 * accessor "getSopClass" gives direct access to the value 753 */ 754 public InstanceComponent setSopClassElement(OidType value) { 755 this.sopClass = value; 756 return this; 757 } 758 759 /** 760 * @return SOP class UID of the selected instance. 761 */ 762 public String getSopClass() { 763 return this.sopClass == null ? null : this.sopClass.getValue(); 764 } 765 766 /** 767 * @param value SOP class UID of the selected instance. 768 */ 769 public InstanceComponent setSopClass(String value) { 770 if (this.sopClass == null) 771 this.sopClass = new OidType(); 772 this.sopClass.setValue(value); 773 return this; 774 } 775 776 /** 777 * @return {@link #uid} (SOP Instance UID of the selected instance.). This is 778 * the underlying object with id, value and extensions. The accessor 779 * "getUid" gives direct access to the value 780 */ 781 public OidType getUidElement() { 782 if (this.uid == null) 783 if (Configuration.errorOnAutoCreate()) 784 throw new Error("Attempt to auto-create InstanceComponent.uid"); 785 else if (Configuration.doAutoCreate()) 786 this.uid = new OidType(); // bb 787 return this.uid; 788 } 789 790 public boolean hasUidElement() { 791 return this.uid != null && !this.uid.isEmpty(); 792 } 793 794 public boolean hasUid() { 795 return this.uid != null && !this.uid.isEmpty(); 796 } 797 798 /** 799 * @param value {@link #uid} (SOP Instance UID of the selected instance.). This 800 * is the underlying object with id, value and extensions. The 801 * accessor "getUid" gives direct access to the value 802 */ 803 public InstanceComponent setUidElement(OidType value) { 804 this.uid = value; 805 return this; 806 } 807 808 /** 809 * @return SOP Instance UID of the selected instance. 810 */ 811 public String getUid() { 812 return this.uid == null ? null : this.uid.getValue(); 813 } 814 815 /** 816 * @param value SOP Instance UID of the selected instance. 817 */ 818 public InstanceComponent setUid(String value) { 819 if (this.uid == null) 820 this.uid = new OidType(); 821 this.uid.setValue(value); 822 return this; 823 } 824 825 /** 826 * @return {@link #url} (WADO-RS URL to retrieve the DICOM SOP Instance.). This 827 * is the underlying object with id, value and extensions. The accessor 828 * "getUrl" gives direct access to the value 829 */ 830 public UriType getUrlElement() { 831 if (this.url == null) 832 if (Configuration.errorOnAutoCreate()) 833 throw new Error("Attempt to auto-create InstanceComponent.url"); 834 else if (Configuration.doAutoCreate()) 835 this.url = new UriType(); // bb 836 return this.url; 837 } 838 839 public boolean hasUrlElement() { 840 return this.url != null && !this.url.isEmpty(); 841 } 842 843 public boolean hasUrl() { 844 return this.url != null && !this.url.isEmpty(); 845 } 846 847 /** 848 * @param value {@link #url} (WADO-RS URL to retrieve the DICOM SOP Instance.). 849 * This is the underlying object with id, value and extensions. The 850 * accessor "getUrl" gives direct access to the value 851 */ 852 public InstanceComponent setUrlElement(UriType value) { 853 this.url = value; 854 return this; 855 } 856 857 /** 858 * @return WADO-RS URL to retrieve the DICOM SOP Instance. 859 */ 860 public String getUrl() { 861 return this.url == null ? null : this.url.getValue(); 862 } 863 864 /** 865 * @param value WADO-RS URL to retrieve the DICOM SOP Instance. 866 */ 867 public InstanceComponent setUrl(String value) { 868 if (this.url == null) 869 this.url = new UriType(); 870 this.url.setValue(value); 871 return this; 872 } 873 874 /** 875 * @return {@link #frames} (Identity and location information of the frames in 876 * the selected instance.) 877 */ 878 public List<FramesComponent> getFrames() { 879 if (this.frames == null) 880 this.frames = new ArrayList<FramesComponent>(); 881 return this.frames; 882 } 883 884 public boolean hasFrames() { 885 if (this.frames == null) 886 return false; 887 for (FramesComponent item : this.frames) 888 if (!item.isEmpty()) 889 return true; 890 return false; 891 } 892 893 /** 894 * @return {@link #frames} (Identity and location information of the frames in 895 * the selected instance.) 896 */ 897 // syntactic sugar 898 public FramesComponent addFrames() { // 3 899 FramesComponent t = new FramesComponent(); 900 if (this.frames == null) 901 this.frames = new ArrayList<FramesComponent>(); 902 this.frames.add(t); 903 return t; 904 } 905 906 // syntactic sugar 907 public InstanceComponent addFrames(FramesComponent t) { // 3 908 if (t == null) 909 return this; 910 if (this.frames == null) 911 this.frames = new ArrayList<FramesComponent>(); 912 this.frames.add(t); 913 return this; 914 } 915 916 protected void listChildren(List<Property> childrenList) { 917 super.listChildren(childrenList); 918 childrenList.add(new Property("sopClass", "oid", "SOP class UID of the selected instance.", 0, 919 java.lang.Integer.MAX_VALUE, sopClass)); 920 childrenList.add(new Property("uid", "oid", "SOP Instance UID of the selected instance.", 0, 921 java.lang.Integer.MAX_VALUE, uid)); 922 childrenList.add(new Property("url", "uri", "WADO-RS URL to retrieve the DICOM SOP Instance.", 0, 923 java.lang.Integer.MAX_VALUE, url)); 924 childrenList 925 .add(new Property("frames", "", "Identity and location information of the frames in the selected instance.", 926 0, java.lang.Integer.MAX_VALUE, frames)); 927 } 928 929 @Override 930 public void setProperty(String name, Base value) throws FHIRException { 931 if (name.equals("sopClass")) 932 this.sopClass = castToOid(value); // OidType 933 else if (name.equals("uid")) 934 this.uid = castToOid(value); // OidType 935 else if (name.equals("url")) 936 this.url = castToUri(value); // UriType 937 else if (name.equals("frames")) 938 this.getFrames().add((FramesComponent) value); 939 else 940 super.setProperty(name, value); 941 } 942 943 @Override 944 public Base addChild(String name) throws FHIRException { 945 if (name.equals("sopClass")) { 946 throw new FHIRException("Cannot call addChild on a singleton property ImagingObjectSelection.sopClass"); 947 } else if (name.equals("uid")) { 948 throw new FHIRException("Cannot call addChild on a singleton property ImagingObjectSelection.uid"); 949 } else if (name.equals("url")) { 950 throw new FHIRException("Cannot call addChild on a singleton property ImagingObjectSelection.url"); 951 } else if (name.equals("frames")) { 952 return addFrames(); 953 } else 954 return super.addChild(name); 955 } 956 957 public InstanceComponent copy() { 958 InstanceComponent dst = new InstanceComponent(); 959 copyValues(dst); 960 dst.sopClass = sopClass == null ? null : sopClass.copy(); 961 dst.uid = uid == null ? null : uid.copy(); 962 dst.url = url == null ? null : url.copy(); 963 if (frames != null) { 964 dst.frames = new ArrayList<FramesComponent>(); 965 for (FramesComponent i : frames) 966 dst.frames.add(i.copy()); 967 } 968 ; 969 return dst; 970 } 971 972 @Override 973 public boolean equalsDeep(Base other) { 974 if (!super.equalsDeep(other)) 975 return false; 976 if (!(other instanceof InstanceComponent)) 977 return false; 978 InstanceComponent o = (InstanceComponent) other; 979 return compareDeep(sopClass, o.sopClass, true) && compareDeep(uid, o.uid, true) && compareDeep(url, o.url, true) 980 && compareDeep(frames, o.frames, true); 981 } 982 983 @Override 984 public boolean equalsShallow(Base other) { 985 if (!super.equalsShallow(other)) 986 return false; 987 if (!(other instanceof InstanceComponent)) 988 return false; 989 InstanceComponent o = (InstanceComponent) other; 990 return compareValues(sopClass, o.sopClass, true) && compareValues(uid, o.uid, true) 991 && compareValues(url, o.url, true); 992 } 993 994 public boolean isEmpty() { 995 return super.isEmpty() && (sopClass == null || sopClass.isEmpty()) && (uid == null || uid.isEmpty()) 996 && (url == null || url.isEmpty()) && (frames == null || frames.isEmpty()); 997 } 998 999 public String fhirType() { 1000 return "ImagingObjectSelection.study.series.instance"; 1001 1002 } 1003 1004 } 1005 1006 @Block() 1007 public static class FramesComponent extends BackboneElement implements IBaseBackboneElement { 1008 /** 1009 * The frame numbers in the frame set. 1010 */ 1011 @Child(name = "frameNumbers", type = { 1012 UnsignedIntType.class }, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1013 @Description(shortDefinition = "Frame numbers", formalDefinition = "The frame numbers in the frame set.") 1014 protected List<UnsignedIntType> frameNumbers; 1015 1016 /** 1017 * WADO-RS URL to retrieve the DICOM frames. 1018 */ 1019 @Child(name = "url", type = { UriType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1020 @Description(shortDefinition = "Retrieve frame URL", formalDefinition = "WADO-RS URL to retrieve the DICOM frames.") 1021 protected UriType url; 1022 1023 private static final long serialVersionUID = -2068206970L; 1024 1025 /* 1026 * Constructor 1027 */ 1028 public FramesComponent() { 1029 super(); 1030 } 1031 1032 /* 1033 * Constructor 1034 */ 1035 public FramesComponent(UriType url) { 1036 super(); 1037 this.url = url; 1038 } 1039 1040 /** 1041 * @return {@link #frameNumbers} (The frame numbers in the frame set.) 1042 */ 1043 public List<UnsignedIntType> getFrameNumbers() { 1044 if (this.frameNumbers == null) 1045 this.frameNumbers = new ArrayList<UnsignedIntType>(); 1046 return this.frameNumbers; 1047 } 1048 1049 public boolean hasFrameNumbers() { 1050 if (this.frameNumbers == null) 1051 return false; 1052 for (UnsignedIntType item : this.frameNumbers) 1053 if (!item.isEmpty()) 1054 return true; 1055 return false; 1056 } 1057 1058 /** 1059 * @return {@link #frameNumbers} (The frame numbers in the frame set.) 1060 */ 1061 // syntactic sugar 1062 public UnsignedIntType addFrameNumbersElement() {// 2 1063 UnsignedIntType t = new UnsignedIntType(); 1064 if (this.frameNumbers == null) 1065 this.frameNumbers = new ArrayList<UnsignedIntType>(); 1066 this.frameNumbers.add(t); 1067 return t; 1068 } 1069 1070 /** 1071 * @param value {@link #frameNumbers} (The frame numbers in the frame set.) 1072 */ 1073 public FramesComponent addFrameNumbers(int value) { // 1 1074 UnsignedIntType t = new UnsignedIntType(); 1075 t.setValue(value); 1076 if (this.frameNumbers == null) 1077 this.frameNumbers = new ArrayList<UnsignedIntType>(); 1078 this.frameNumbers.add(t); 1079 return this; 1080 } 1081 1082 /** 1083 * @param value {@link #frameNumbers} (The frame numbers in the frame set.) 1084 */ 1085 public boolean hasFrameNumbers(int value) { 1086 if (this.frameNumbers == null) 1087 return false; 1088 for (UnsignedIntType v : this.frameNumbers) 1089 if (v.equals(value)) // unsignedInt 1090 return true; 1091 return false; 1092 } 1093 1094 /** 1095 * @return {@link #url} (WADO-RS URL to retrieve the DICOM frames.). This is the 1096 * underlying object with id, value and extensions. The accessor 1097 * "getUrl" gives direct access to the value 1098 */ 1099 public UriType getUrlElement() { 1100 if (this.url == null) 1101 if (Configuration.errorOnAutoCreate()) 1102 throw new Error("Attempt to auto-create FramesComponent.url"); 1103 else if (Configuration.doAutoCreate()) 1104 this.url = new UriType(); // bb 1105 return this.url; 1106 } 1107 1108 public boolean hasUrlElement() { 1109 return this.url != null && !this.url.isEmpty(); 1110 } 1111 1112 public boolean hasUrl() { 1113 return this.url != null && !this.url.isEmpty(); 1114 } 1115 1116 /** 1117 * @param value {@link #url} (WADO-RS URL to retrieve the DICOM frames.). This 1118 * is the underlying object with id, value and extensions. The 1119 * accessor "getUrl" gives direct access to the value 1120 */ 1121 public FramesComponent setUrlElement(UriType value) { 1122 this.url = value; 1123 return this; 1124 } 1125 1126 /** 1127 * @return WADO-RS URL to retrieve the DICOM frames. 1128 */ 1129 public String getUrl() { 1130 return this.url == null ? null : this.url.getValue(); 1131 } 1132 1133 /** 1134 * @param value WADO-RS URL to retrieve the DICOM frames. 1135 */ 1136 public FramesComponent setUrl(String value) { 1137 if (this.url == null) 1138 this.url = new UriType(); 1139 this.url.setValue(value); 1140 return this; 1141 } 1142 1143 protected void listChildren(List<Property> childrenList) { 1144 super.listChildren(childrenList); 1145 childrenList.add(new Property("frameNumbers", "unsignedInt", "The frame numbers in the frame set.", 0, 1146 java.lang.Integer.MAX_VALUE, frameNumbers)); 1147 childrenList.add( 1148 new Property("url", "uri", "WADO-RS URL to retrieve the DICOM frames.", 0, java.lang.Integer.MAX_VALUE, url)); 1149 } 1150 1151 @Override 1152 public void setProperty(String name, Base value) throws FHIRException { 1153 if (name.equals("frameNumbers")) 1154 this.getFrameNumbers().add(castToUnsignedInt(value)); 1155 else if (name.equals("url")) 1156 this.url = castToUri(value); // UriType 1157 else 1158 super.setProperty(name, value); 1159 } 1160 1161 @Override 1162 public Base addChild(String name) throws FHIRException { 1163 if (name.equals("frameNumbers")) { 1164 throw new FHIRException("Cannot call addChild on a singleton property ImagingObjectSelection.frameNumbers"); 1165 } else if (name.equals("url")) { 1166 throw new FHIRException("Cannot call addChild on a singleton property ImagingObjectSelection.url"); 1167 } else 1168 return super.addChild(name); 1169 } 1170 1171 public FramesComponent copy() { 1172 FramesComponent dst = new FramesComponent(); 1173 copyValues(dst); 1174 if (frameNumbers != null) { 1175 dst.frameNumbers = new ArrayList<UnsignedIntType>(); 1176 for (UnsignedIntType i : frameNumbers) 1177 dst.frameNumbers.add(i.copy()); 1178 } 1179 ; 1180 dst.url = url == null ? null : url.copy(); 1181 return dst; 1182 } 1183 1184 @Override 1185 public boolean equalsDeep(Base other) { 1186 if (!super.equalsDeep(other)) 1187 return false; 1188 if (!(other instanceof FramesComponent)) 1189 return false; 1190 FramesComponent o = (FramesComponent) other; 1191 return compareDeep(frameNumbers, o.frameNumbers, true) && compareDeep(url, o.url, true); 1192 } 1193 1194 @Override 1195 public boolean equalsShallow(Base other) { 1196 if (!super.equalsShallow(other)) 1197 return false; 1198 if (!(other instanceof FramesComponent)) 1199 return false; 1200 FramesComponent o = (FramesComponent) other; 1201 return compareValues(frameNumbers, o.frameNumbers, true) && compareValues(url, o.url, true); 1202 } 1203 1204 public boolean isEmpty() { 1205 return super.isEmpty() && (frameNumbers == null || frameNumbers.isEmpty()) && (url == null || url.isEmpty()); 1206 } 1207 1208 public String fhirType() { 1209 return "ImagingObjectSelection.study.series.instance.frames"; 1210 1211 } 1212 1213 } 1214 1215 /** 1216 * Instance UID of the DICOM KOS SOP Instances represented in this resource. 1217 */ 1218 @Child(name = "uid", type = { OidType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 1219 @Description(shortDefinition = "Instance UID", formalDefinition = "Instance UID of the DICOM KOS SOP Instances represented in this resource.") 1220 protected OidType uid; 1221 1222 /** 1223 * A patient resource reference which is the patient subject of all DICOM SOP 1224 * Instances in this ImagingObjectSelection. 1225 */ 1226 @Child(name = "patient", type = { Patient.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1227 @Description(shortDefinition = "Patient of the selected objects", formalDefinition = "A patient resource reference which is the patient subject of all DICOM SOP Instances in this ImagingObjectSelection.") 1228 protected Reference patient; 1229 1230 /** 1231 * The actual object that is the target of the reference (A patient resource 1232 * reference which is the patient subject of all DICOM SOP Instances in this 1233 * ImagingObjectSelection.) 1234 */ 1235 protected Patient patientTarget; 1236 1237 /** 1238 * The reason for, or significance of, the selection of objects referenced in 1239 * the resource. 1240 */ 1241 @Child(name = "title", type = { 1242 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1243 @Description(shortDefinition = "Reason for selection", formalDefinition = "The reason for, or significance of, the selection of objects referenced in the resource.") 1244 protected CodeableConcept title; 1245 1246 /** 1247 * Text description of the DICOM SOP instances selected in the 1248 * ImagingObjectSelection. This should be aligned with the content of the title 1249 * element, and can provide further explanation of the SOP instances in the 1250 * selection. 1251 */ 1252 @Child(name = "description", type = { 1253 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1254 @Description(shortDefinition = "Description text", formalDefinition = "Text description of the DICOM SOP instances selected in the ImagingObjectSelection. This should be aligned with the content of the title element, and can provide further explanation of the SOP instances in the selection.") 1255 protected StringType description; 1256 1257 /** 1258 * Author of ImagingObjectSelection. It can be a human author or a device which 1259 * made the decision of the SOP instances selected. For example, a radiologist 1260 * selected a set of imaging SOP instances to attach in a diagnostic report, and 1261 * a CAD application may author a selection to describe SOP instances it used to 1262 * generate a detection conclusion. 1263 */ 1264 @Child(name = "author", type = { Practitioner.class, Device.class, Organization.class, Patient.class, 1265 RelatedPerson.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1266 @Description(shortDefinition = "Author (human or machine)", formalDefinition = "Author of ImagingObjectSelection. It can be a human author or a device which made the decision of the SOP instances selected. For example, a radiologist selected a set of imaging SOP instances to attach in a diagnostic report, and a CAD application may author a selection to describe SOP instances it used to generate a detection conclusion.") 1267 protected Reference author; 1268 1269 /** 1270 * The actual object that is the target of the reference (Author of 1271 * ImagingObjectSelection. It can be a human author or a device which made the 1272 * decision of the SOP instances selected. For example, a radiologist selected a 1273 * set of imaging SOP instances to attach in a diagnostic report, and a CAD 1274 * application may author a selection to describe SOP instances it used to 1275 * generate a detection conclusion.) 1276 */ 1277 protected Resource authorTarget; 1278 1279 /** 1280 * Date and time when the selection of the referenced instances were made. It is 1281 * (typically) different from the creation date of the selection resource, and 1282 * from dates associated with the referenced instances (e.g. capture time of the 1283 * referenced image). 1284 */ 1285 @Child(name = "authoringTime", type = { 1286 DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1287 @Description(shortDefinition = "Authoring time of the selection", formalDefinition = "Date and time when the selection of the referenced instances were made. It is (typically) different from the creation date of the selection resource, and from dates associated with the referenced instances (e.g. capture time of the referenced image).") 1288 protected DateTimeType authoringTime; 1289 1290 /** 1291 * Study identity and locating information of the DICOM SOP instances in the 1292 * selection. 1293 */ 1294 @Child(name = "study", type = {}, order = 6, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1295 @Description(shortDefinition = "Study identity of the selected instances", formalDefinition = "Study identity and locating information of the DICOM SOP instances in the selection.") 1296 protected List<StudyComponent> study; 1297 1298 private static final long serialVersionUID = -1961832713L; 1299 1300 /* 1301 * Constructor 1302 */ 1303 public ImagingObjectSelection() { 1304 super(); 1305 } 1306 1307 /* 1308 * Constructor 1309 */ 1310 public ImagingObjectSelection(OidType uid, Reference patient, CodeableConcept title) { 1311 super(); 1312 this.uid = uid; 1313 this.patient = patient; 1314 this.title = title; 1315 } 1316 1317 /** 1318 * @return {@link #uid} (Instance UID of the DICOM KOS SOP Instances represented 1319 * in this resource.). This is the underlying object with id, value and 1320 * extensions. The accessor "getUid" gives direct access to the value 1321 */ 1322 public OidType getUidElement() { 1323 if (this.uid == null) 1324 if (Configuration.errorOnAutoCreate()) 1325 throw new Error("Attempt to auto-create ImagingObjectSelection.uid"); 1326 else if (Configuration.doAutoCreate()) 1327 this.uid = new OidType(); // bb 1328 return this.uid; 1329 } 1330 1331 public boolean hasUidElement() { 1332 return this.uid != null && !this.uid.isEmpty(); 1333 } 1334 1335 public boolean hasUid() { 1336 return this.uid != null && !this.uid.isEmpty(); 1337 } 1338 1339 /** 1340 * @param value {@link #uid} (Instance UID of the DICOM KOS SOP Instances 1341 * represented in this resource.). This is the underlying object 1342 * with id, value and extensions. The accessor "getUid" gives 1343 * direct access to the value 1344 */ 1345 public ImagingObjectSelection setUidElement(OidType value) { 1346 this.uid = value; 1347 return this; 1348 } 1349 1350 /** 1351 * @return Instance UID of the DICOM KOS SOP Instances represented in this 1352 * resource. 1353 */ 1354 public String getUid() { 1355 return this.uid == null ? null : this.uid.getValue(); 1356 } 1357 1358 /** 1359 * @param value Instance UID of the DICOM KOS SOP Instances represented in this 1360 * resource. 1361 */ 1362 public ImagingObjectSelection setUid(String value) { 1363 if (this.uid == null) 1364 this.uid = new OidType(); 1365 this.uid.setValue(value); 1366 return this; 1367 } 1368 1369 /** 1370 * @return {@link #patient} (A patient resource reference which is the patient 1371 * subject of all DICOM SOP Instances in this ImagingObjectSelection.) 1372 */ 1373 public Reference getPatient() { 1374 if (this.patient == null) 1375 if (Configuration.errorOnAutoCreate()) 1376 throw new Error("Attempt to auto-create ImagingObjectSelection.patient"); 1377 else if (Configuration.doAutoCreate()) 1378 this.patient = new Reference(); // cc 1379 return this.patient; 1380 } 1381 1382 public boolean hasPatient() { 1383 return this.patient != null && !this.patient.isEmpty(); 1384 } 1385 1386 /** 1387 * @param value {@link #patient} (A patient resource reference which is the 1388 * patient subject of all DICOM SOP Instances in this 1389 * ImagingObjectSelection.) 1390 */ 1391 public ImagingObjectSelection setPatient(Reference value) { 1392 this.patient = value; 1393 return this; 1394 } 1395 1396 /** 1397 * @return {@link #patient} The actual object that is the target of the 1398 * reference. The reference library doesn't populate this, but you can 1399 * use it to hold the resource if you resolve it. (A patient resource 1400 * reference which is the patient subject of all DICOM SOP Instances in 1401 * this ImagingObjectSelection.) 1402 */ 1403 public Patient getPatientTarget() { 1404 if (this.patientTarget == null) 1405 if (Configuration.errorOnAutoCreate()) 1406 throw new Error("Attempt to auto-create ImagingObjectSelection.patient"); 1407 else if (Configuration.doAutoCreate()) 1408 this.patientTarget = new Patient(); // aa 1409 return this.patientTarget; 1410 } 1411 1412 /** 1413 * @param value {@link #patient} The actual object that is the target of the 1414 * reference. The reference library doesn't use these, but you can 1415 * use it to hold the resource if you resolve it. (A patient 1416 * resource reference which is the patient subject of all DICOM SOP 1417 * Instances in this ImagingObjectSelection.) 1418 */ 1419 public ImagingObjectSelection setPatientTarget(Patient value) { 1420 this.patientTarget = value; 1421 return this; 1422 } 1423 1424 /** 1425 * @return {@link #title} (The reason for, or significance of, the selection of 1426 * objects referenced in the resource.) 1427 */ 1428 public CodeableConcept getTitle() { 1429 if (this.title == null) 1430 if (Configuration.errorOnAutoCreate()) 1431 throw new Error("Attempt to auto-create ImagingObjectSelection.title"); 1432 else if (Configuration.doAutoCreate()) 1433 this.title = new CodeableConcept(); // cc 1434 return this.title; 1435 } 1436 1437 public boolean hasTitle() { 1438 return this.title != null && !this.title.isEmpty(); 1439 } 1440 1441 /** 1442 * @param value {@link #title} (The reason for, or significance of, the 1443 * selection of objects referenced in the resource.) 1444 */ 1445 public ImagingObjectSelection setTitle(CodeableConcept value) { 1446 this.title = value; 1447 return this; 1448 } 1449 1450 /** 1451 * @return {@link #description} (Text description of the DICOM SOP instances 1452 * selected in the ImagingObjectSelection. This should be aligned with 1453 * the content of the title element, and can provide further explanation 1454 * of the SOP instances in the selection.). This is the underlying 1455 * object with id, value and extensions. The accessor "getDescription" 1456 * gives direct access to the value 1457 */ 1458 public StringType getDescriptionElement() { 1459 if (this.description == null) 1460 if (Configuration.errorOnAutoCreate()) 1461 throw new Error("Attempt to auto-create ImagingObjectSelection.description"); 1462 else if (Configuration.doAutoCreate()) 1463 this.description = new StringType(); // bb 1464 return this.description; 1465 } 1466 1467 public boolean hasDescriptionElement() { 1468 return this.description != null && !this.description.isEmpty(); 1469 } 1470 1471 public boolean hasDescription() { 1472 return this.description != null && !this.description.isEmpty(); 1473 } 1474 1475 /** 1476 * @param value {@link #description} (Text description of the DICOM SOP 1477 * instances selected in the ImagingObjectSelection. This should be 1478 * aligned with the content of the title element, and can provide 1479 * further explanation of the SOP instances in the selection.). 1480 * This is the underlying object with id, value and extensions. The 1481 * accessor "getDescription" gives direct access to the value 1482 */ 1483 public ImagingObjectSelection setDescriptionElement(StringType value) { 1484 this.description = value; 1485 return this; 1486 } 1487 1488 /** 1489 * @return Text description of the DICOM SOP instances selected in the 1490 * ImagingObjectSelection. This should be aligned with the content of 1491 * the title element, and can provide further explanation of the SOP 1492 * instances in the selection. 1493 */ 1494 public String getDescription() { 1495 return this.description == null ? null : this.description.getValue(); 1496 } 1497 1498 /** 1499 * @param value Text description of the DICOM SOP instances selected in the 1500 * ImagingObjectSelection. This should be aligned with the content 1501 * of the title element, and can provide further explanation of the 1502 * SOP instances in the selection. 1503 */ 1504 public ImagingObjectSelection setDescription(String value) { 1505 if (Utilities.noString(value)) 1506 this.description = null; 1507 else { 1508 if (this.description == null) 1509 this.description = new StringType(); 1510 this.description.setValue(value); 1511 } 1512 return this; 1513 } 1514 1515 /** 1516 * @return {@link #author} (Author of ImagingObjectSelection. It can be a human 1517 * author or a device which made the decision of the SOP instances 1518 * selected. For example, a radiologist selected a set of imaging SOP 1519 * instances to attach in a diagnostic report, and a CAD application may 1520 * author a selection to describe SOP instances it used to generate a 1521 * detection conclusion.) 1522 */ 1523 public Reference getAuthor() { 1524 if (this.author == null) 1525 if (Configuration.errorOnAutoCreate()) 1526 throw new Error("Attempt to auto-create ImagingObjectSelection.author"); 1527 else if (Configuration.doAutoCreate()) 1528 this.author = new Reference(); // cc 1529 return this.author; 1530 } 1531 1532 public boolean hasAuthor() { 1533 return this.author != null && !this.author.isEmpty(); 1534 } 1535 1536 /** 1537 * @param value {@link #author} (Author of ImagingObjectSelection. It can be a 1538 * human author or a device which made the decision of the SOP 1539 * instances selected. For example, a radiologist selected a set of 1540 * imaging SOP instances to attach in a diagnostic report, and a 1541 * CAD application may author a selection to describe SOP instances 1542 * it used to generate a detection conclusion.) 1543 */ 1544 public ImagingObjectSelection setAuthor(Reference value) { 1545 this.author = value; 1546 return this; 1547 } 1548 1549 /** 1550 * @return {@link #author} The actual object that is the target of the 1551 * reference. The reference library doesn't populate this, but you can 1552 * use it to hold the resource if you resolve it. (Author of 1553 * ImagingObjectSelection. It can be a human author or a device which 1554 * made the decision of the SOP instances selected. For example, a 1555 * radiologist selected a set of imaging SOP instances to attach in a 1556 * diagnostic report, and a CAD application may author a selection to 1557 * describe SOP instances it used to generate a detection conclusion.) 1558 */ 1559 public Resource getAuthorTarget() { 1560 return this.authorTarget; 1561 } 1562 1563 /** 1564 * @param value {@link #author} The actual object that is the target of the 1565 * reference. The reference library doesn't use these, but you can 1566 * use it to hold the resource if you resolve it. (Author of 1567 * ImagingObjectSelection. It can be a human author or a device 1568 * which made the decision of the SOP instances selected. For 1569 * example, a radiologist selected a set of imaging SOP instances 1570 * to attach in a diagnostic report, and a CAD application may 1571 * author a selection to describe SOP instances it used to generate 1572 * a detection conclusion.) 1573 */ 1574 public ImagingObjectSelection setAuthorTarget(Resource value) { 1575 this.authorTarget = value; 1576 return this; 1577 } 1578 1579 /** 1580 * @return {@link #authoringTime} (Date and time when the selection of the 1581 * referenced instances were made. It is (typically) different from the 1582 * creation date of the selection resource, and from dates associated 1583 * with the referenced instances (e.g. capture time of the referenced 1584 * image).). This is the underlying object with id, value and 1585 * extensions. The accessor "getAuthoringTime" gives direct access to 1586 * the value 1587 */ 1588 public DateTimeType getAuthoringTimeElement() { 1589 if (this.authoringTime == null) 1590 if (Configuration.errorOnAutoCreate()) 1591 throw new Error("Attempt to auto-create ImagingObjectSelection.authoringTime"); 1592 else if (Configuration.doAutoCreate()) 1593 this.authoringTime = new DateTimeType(); // bb 1594 return this.authoringTime; 1595 } 1596 1597 public boolean hasAuthoringTimeElement() { 1598 return this.authoringTime != null && !this.authoringTime.isEmpty(); 1599 } 1600 1601 public boolean hasAuthoringTime() { 1602 return this.authoringTime != null && !this.authoringTime.isEmpty(); 1603 } 1604 1605 /** 1606 * @param value {@link #authoringTime} (Date and time when the selection of the 1607 * referenced instances were made. It is (typically) different from 1608 * the creation date of the selection resource, and from dates 1609 * associated with the referenced instances (e.g. capture time of 1610 * the referenced image).). This is the underlying object with id, 1611 * value and extensions. The accessor "getAuthoringTime" gives 1612 * direct access to the value 1613 */ 1614 public ImagingObjectSelection setAuthoringTimeElement(DateTimeType value) { 1615 this.authoringTime = value; 1616 return this; 1617 } 1618 1619 /** 1620 * @return Date and time when the selection of the referenced instances were 1621 * made. It is (typically) different from the creation date of the 1622 * selection resource, and from dates associated with the referenced 1623 * instances (e.g. capture time of the referenced image). 1624 */ 1625 public Date getAuthoringTime() { 1626 return this.authoringTime == null ? null : this.authoringTime.getValue(); 1627 } 1628 1629 /** 1630 * @param value Date and time when the selection of the referenced instances 1631 * were made. It is (typically) different from the creation date of 1632 * the selection resource, and from dates associated with the 1633 * referenced instances (e.g. capture time of the referenced 1634 * image). 1635 */ 1636 public ImagingObjectSelection setAuthoringTime(Date value) { 1637 if (value == null) 1638 this.authoringTime = null; 1639 else { 1640 if (this.authoringTime == null) 1641 this.authoringTime = new DateTimeType(); 1642 this.authoringTime.setValue(value); 1643 } 1644 return this; 1645 } 1646 1647 /** 1648 * @return {@link #study} (Study identity and locating information of the DICOM 1649 * SOP instances in the selection.) 1650 */ 1651 public List<StudyComponent> getStudy() { 1652 if (this.study == null) 1653 this.study = new ArrayList<StudyComponent>(); 1654 return this.study; 1655 } 1656 1657 public boolean hasStudy() { 1658 if (this.study == null) 1659 return false; 1660 for (StudyComponent item : this.study) 1661 if (!item.isEmpty()) 1662 return true; 1663 return false; 1664 } 1665 1666 /** 1667 * @return {@link #study} (Study identity and locating information of the DICOM 1668 * SOP instances in the selection.) 1669 */ 1670 // syntactic sugar 1671 public StudyComponent addStudy() { // 3 1672 StudyComponent t = new StudyComponent(); 1673 if (this.study == null) 1674 this.study = new ArrayList<StudyComponent>(); 1675 this.study.add(t); 1676 return t; 1677 } 1678 1679 // syntactic sugar 1680 public ImagingObjectSelection addStudy(StudyComponent t) { // 3 1681 if (t == null) 1682 return this; 1683 if (this.study == null) 1684 this.study = new ArrayList<StudyComponent>(); 1685 this.study.add(t); 1686 return this; 1687 } 1688 1689 protected void listChildren(List<Property> childrenList) { 1690 super.listChildren(childrenList); 1691 childrenList 1692 .add(new Property("uid", "oid", "Instance UID of the DICOM KOS SOP Instances represented in this resource.", 0, 1693 java.lang.Integer.MAX_VALUE, uid)); 1694 childrenList.add(new Property("patient", "Reference(Patient)", 1695 "A patient resource reference which is the patient subject of all DICOM SOP Instances in this ImagingObjectSelection.", 1696 0, java.lang.Integer.MAX_VALUE, patient)); 1697 childrenList.add(new Property("title", "CodeableConcept", 1698 "The reason for, or significance of, the selection of objects referenced in the resource.", 0, 1699 java.lang.Integer.MAX_VALUE, title)); 1700 childrenList.add(new Property("description", "string", 1701 "Text description of the DICOM SOP instances selected in the ImagingObjectSelection. This should be aligned with the content of the title element, and can provide further explanation of the SOP instances in the selection.", 1702 0, java.lang.Integer.MAX_VALUE, description)); 1703 childrenList.add(new Property("author", "Reference(Practitioner|Device|Organization|Patient|RelatedPerson)", 1704 "Author of ImagingObjectSelection. It can be a human author or a device which made the decision of the SOP instances selected. For example, a radiologist selected a set of imaging SOP instances to attach in a diagnostic report, and a CAD application may author a selection to describe SOP instances it used to generate a detection conclusion.", 1705 0, java.lang.Integer.MAX_VALUE, author)); 1706 childrenList.add(new Property("authoringTime", "dateTime", 1707 "Date and time when the selection of the referenced instances were made. It is (typically) different from the creation date of the selection resource, and from dates associated with the referenced instances (e.g. capture time of the referenced image).", 1708 0, java.lang.Integer.MAX_VALUE, authoringTime)); 1709 childrenList.add(new Property("study", "", 1710 "Study identity and locating information of the DICOM SOP instances in the selection.", 0, 1711 java.lang.Integer.MAX_VALUE, study)); 1712 } 1713 1714 @Override 1715 public void setProperty(String name, Base value) throws FHIRException { 1716 if (name.equals("uid")) 1717 this.uid = castToOid(value); // OidType 1718 else if (name.equals("patient")) 1719 this.patient = castToReference(value); // Reference 1720 else if (name.equals("title")) 1721 this.title = castToCodeableConcept(value); // CodeableConcept 1722 else if (name.equals("description")) 1723 this.description = castToString(value); // StringType 1724 else if (name.equals("author")) 1725 this.author = castToReference(value); // Reference 1726 else if (name.equals("authoringTime")) 1727 this.authoringTime = castToDateTime(value); // DateTimeType 1728 else if (name.equals("study")) 1729 this.getStudy().add((StudyComponent) value); 1730 else 1731 super.setProperty(name, value); 1732 } 1733 1734 @Override 1735 public Base addChild(String name) throws FHIRException { 1736 if (name.equals("uid")) { 1737 throw new FHIRException("Cannot call addChild on a singleton property ImagingObjectSelection.uid"); 1738 } else if (name.equals("patient")) { 1739 this.patient = new Reference(); 1740 return this.patient; 1741 } else if (name.equals("title")) { 1742 this.title = new CodeableConcept(); 1743 return this.title; 1744 } else if (name.equals("description")) { 1745 throw new FHIRException("Cannot call addChild on a singleton property ImagingObjectSelection.description"); 1746 } else if (name.equals("author")) { 1747 this.author = new Reference(); 1748 return this.author; 1749 } else if (name.equals("authoringTime")) { 1750 throw new FHIRException("Cannot call addChild on a singleton property ImagingObjectSelection.authoringTime"); 1751 } else if (name.equals("study")) { 1752 return addStudy(); 1753 } else 1754 return super.addChild(name); 1755 } 1756 1757 public String fhirType() { 1758 return "ImagingObjectSelection"; 1759 1760 } 1761 1762 public ImagingObjectSelection copy() { 1763 ImagingObjectSelection dst = new ImagingObjectSelection(); 1764 copyValues(dst); 1765 dst.uid = uid == null ? null : uid.copy(); 1766 dst.patient = patient == null ? null : patient.copy(); 1767 dst.title = title == null ? null : title.copy(); 1768 dst.description = description == null ? null : description.copy(); 1769 dst.author = author == null ? null : author.copy(); 1770 dst.authoringTime = authoringTime == null ? null : authoringTime.copy(); 1771 if (study != null) { 1772 dst.study = new ArrayList<StudyComponent>(); 1773 for (StudyComponent i : study) 1774 dst.study.add(i.copy()); 1775 } 1776 ; 1777 return dst; 1778 } 1779 1780 protected ImagingObjectSelection typedCopy() { 1781 return copy(); 1782 } 1783 1784 @Override 1785 public boolean equalsDeep(Base other) { 1786 if (!super.equalsDeep(other)) 1787 return false; 1788 if (!(other instanceof ImagingObjectSelection)) 1789 return false; 1790 ImagingObjectSelection o = (ImagingObjectSelection) other; 1791 return compareDeep(uid, o.uid, true) && compareDeep(patient, o.patient, true) && compareDeep(title, o.title, true) 1792 && compareDeep(description, o.description, true) && compareDeep(author, o.author, true) 1793 && compareDeep(authoringTime, o.authoringTime, true) && compareDeep(study, o.study, true); 1794 } 1795 1796 @Override 1797 public boolean equalsShallow(Base other) { 1798 if (!super.equalsShallow(other)) 1799 return false; 1800 if (!(other instanceof ImagingObjectSelection)) 1801 return false; 1802 ImagingObjectSelection o = (ImagingObjectSelection) other; 1803 return compareValues(uid, o.uid, true) && compareValues(description, o.description, true) 1804 && compareValues(authoringTime, o.authoringTime, true); 1805 } 1806 1807 public boolean isEmpty() { 1808 return super.isEmpty() && (uid == null || uid.isEmpty()) && (patient == null || patient.isEmpty()) 1809 && (title == null || title.isEmpty()) && (description == null || description.isEmpty()) 1810 && (author == null || author.isEmpty()) && (authoringTime == null || authoringTime.isEmpty()) 1811 && (study == null || study.isEmpty()); 1812 } 1813 1814 @Override 1815 public ResourceType getResourceType() { 1816 return ResourceType.ImagingObjectSelection; 1817 } 1818 1819 @SearchParamDefinition(name = "identifier", path = "ImagingObjectSelection.uid", description = "UID of key DICOM object selection", type = "uri") 1820 public static final String SP_IDENTIFIER = "identifier"; 1821 @SearchParamDefinition(name = "authoring-time", path = "ImagingObjectSelection.authoringTime", description = "Time of key DICOM object selection authoring", type = "date") 1822 public static final String SP_AUTHORINGTIME = "authoring-time"; 1823 @SearchParamDefinition(name = "selected-study", path = "ImagingObjectSelection.study.uid", description = "Study selected in key DICOM object selection", type = "uri") 1824 public static final String SP_SELECTEDSTUDY = "selected-study"; 1825 @SearchParamDefinition(name = "author", path = "ImagingObjectSelection.author", description = "Author of key DICOM object selection", type = "reference") 1826 public static final String SP_AUTHOR = "author"; 1827 @SearchParamDefinition(name = "patient", path = "ImagingObjectSelection.patient", description = "Subject of key DICOM object selection", type = "reference") 1828 public static final String SP_PATIENT = "patient"; 1829 @SearchParamDefinition(name = "title", path = "ImagingObjectSelection.title", description = "Title of key DICOM object selection", type = "token") 1830 public static final String SP_TITLE = "title"; 1831 1832}