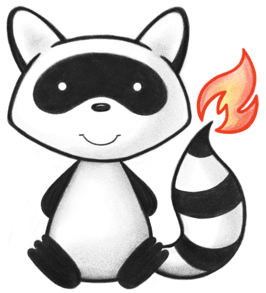
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * Representation of the content produced in a DICOM imaging study. A study 048 * comprises a set of series, each of which includes a set of Service-Object 049 * Pair Instances (SOP Instances - images or other data) acquired or produced in 050 * a common context. A series is of only one modality (e.g. X-ray, CT, MR, 051 * ultrasound), but a study may have multiple series of different modalities. 052 */ 053@ResourceDef(name = "ImagingStudy", profile = "http://hl7.org/fhir/Profile/ImagingStudy") 054public class ImagingStudy extends DomainResource { 055 056 public enum InstanceAvailability { 057 /** 058 * null 059 */ 060 ONLINE, 061 /** 062 * null 063 */ 064 OFFLINE, 065 /** 066 * null 067 */ 068 NEARLINE, 069 /** 070 * null 071 */ 072 UNAVAILABLE, 073 /** 074 * added to help the parsers 075 */ 076 NULL; 077 078 public static InstanceAvailability fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("ONLINE".equals(codeString)) 082 return ONLINE; 083 if ("OFFLINE".equals(codeString)) 084 return OFFLINE; 085 if ("NEARLINE".equals(codeString)) 086 return NEARLINE; 087 if ("UNAVAILABLE".equals(codeString)) 088 return UNAVAILABLE; 089 throw new FHIRException("Unknown InstanceAvailability code '" + codeString + "'"); 090 } 091 092 public String toCode() { 093 switch (this) { 094 case ONLINE: 095 return "ONLINE"; 096 case OFFLINE: 097 return "OFFLINE"; 098 case NEARLINE: 099 return "NEARLINE"; 100 case UNAVAILABLE: 101 return "UNAVAILABLE"; 102 case NULL: 103 return null; 104 default: 105 return "?"; 106 } 107 } 108 109 public String getSystem() { 110 switch (this) { 111 case ONLINE: 112 return "http://nema.org/dicom/dicm"; 113 case OFFLINE: 114 return "http://nema.org/dicom/dicm"; 115 case NEARLINE: 116 return "http://nema.org/dicom/dicm"; 117 case UNAVAILABLE: 118 return "http://nema.org/dicom/dicm"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getDefinition() { 127 switch (this) { 128 case ONLINE: 129 return ""; 130 case OFFLINE: 131 return ""; 132 case NEARLINE: 133 return ""; 134 case UNAVAILABLE: 135 return ""; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getDisplay() { 144 switch (this) { 145 case ONLINE: 146 return "ONLINE"; 147 case OFFLINE: 148 return "OFFLINE"; 149 case NEARLINE: 150 return "NEARLINE"; 151 case UNAVAILABLE: 152 return "UNAVAILABLE"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 } 160 161 public static class InstanceAvailabilityEnumFactory implements EnumFactory<InstanceAvailability> { 162 public InstanceAvailability fromCode(String codeString) throws IllegalArgumentException { 163 if (codeString == null || "".equals(codeString)) 164 if (codeString == null || "".equals(codeString)) 165 return null; 166 if ("ONLINE".equals(codeString)) 167 return InstanceAvailability.ONLINE; 168 if ("OFFLINE".equals(codeString)) 169 return InstanceAvailability.OFFLINE; 170 if ("NEARLINE".equals(codeString)) 171 return InstanceAvailability.NEARLINE; 172 if ("UNAVAILABLE".equals(codeString)) 173 return InstanceAvailability.UNAVAILABLE; 174 throw new IllegalArgumentException("Unknown InstanceAvailability code '" + codeString + "'"); 175 } 176 177 public Enumeration<InstanceAvailability> fromType(Base code) throws FHIRException { 178 if (code == null || code.isEmpty()) 179 return null; 180 String codeString = ((PrimitiveType) code).asStringValue(); 181 if (codeString == null || "".equals(codeString)) 182 return null; 183 if ("ONLINE".equals(codeString)) 184 return new Enumeration<InstanceAvailability>(this, InstanceAvailability.ONLINE); 185 if ("OFFLINE".equals(codeString)) 186 return new Enumeration<InstanceAvailability>(this, InstanceAvailability.OFFLINE); 187 if ("NEARLINE".equals(codeString)) 188 return new Enumeration<InstanceAvailability>(this, InstanceAvailability.NEARLINE); 189 if ("UNAVAILABLE".equals(codeString)) 190 return new Enumeration<InstanceAvailability>(this, InstanceAvailability.UNAVAILABLE); 191 throw new FHIRException("Unknown InstanceAvailability code '" + codeString + "'"); 192 } 193 194 public String toCode(InstanceAvailability code) 195 { 196 if (code == InstanceAvailability.NULL) 197 return null; 198 if (code == InstanceAvailability.ONLINE) 199 return "ONLINE"; 200 if (code == InstanceAvailability.OFFLINE) 201 return "OFFLINE"; 202 if (code == InstanceAvailability.NEARLINE) 203 return "NEARLINE"; 204 if (code == InstanceAvailability.UNAVAILABLE) 205 return "UNAVAILABLE"; 206 return "?"; 207 } 208 } 209 210 @Block() 211 public static class ImagingStudySeriesComponent extends BackboneElement implements IBaseBackboneElement { 212 /** 213 * The Numeric identifier of this series in the study. 214 */ 215 @Child(name = "number", type = { 216 UnsignedIntType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 217 @Description(shortDefinition = "Numeric identifier of this series", formalDefinition = "The Numeric identifier of this series in the study.") 218 protected UnsignedIntType number; 219 220 /** 221 * The modality of this series sequence. 222 */ 223 @Child(name = "modality", type = { Coding.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 224 @Description(shortDefinition = "The modality of the instances in the series", formalDefinition = "The modality of this series sequence.") 225 protected Coding modality; 226 227 /** 228 * Formal identifier for this series. 229 */ 230 @Child(name = "uid", type = { OidType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 231 @Description(shortDefinition = "Formal identifier for this series", formalDefinition = "Formal identifier for this series.") 232 protected OidType uid; 233 234 /** 235 * A description of the series. 236 */ 237 @Child(name = "description", type = { 238 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 239 @Description(shortDefinition = "A description of the series", formalDefinition = "A description of the series.") 240 protected StringType description; 241 242 /** 243 * Number of SOP Instances in Series. 244 */ 245 @Child(name = "numberOfInstances", type = { 246 UnsignedIntType.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 247 @Description(shortDefinition = "Number of Series Related Instances", formalDefinition = "Number of SOP Instances in Series.") 248 protected UnsignedIntType numberOfInstances; 249 250 /** 251 * Availability of series (online, offline or nearline). 252 */ 253 @Child(name = "availability", type = { 254 CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 255 @Description(shortDefinition = "ONLINE | OFFLINE | NEARLINE | UNAVAILABLE", formalDefinition = "Availability of series (online, offline or nearline).") 256 protected Enumeration<InstanceAvailability> availability; 257 258 /** 259 * URI/URL specifying the location of the referenced series using WADO-RS. 260 */ 261 @Child(name = "url", type = { UriType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 262 @Description(shortDefinition = "Location of the referenced instance(s)", formalDefinition = "URI/URL specifying the location of the referenced series using WADO-RS.") 263 protected UriType url; 264 265 /** 266 * Body part examined. See DICOM Part 16 Annex L for the mapping from DICOM to 267 * Snomed CT. 268 */ 269 @Child(name = "bodySite", type = { Coding.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 270 @Description(shortDefinition = "Body part examined", formalDefinition = "Body part examined. See DICOM Part 16 Annex L for the mapping from DICOM to Snomed CT.") 271 protected Coding bodySite; 272 273 /** 274 * Laterality if body site is paired anatomic structure and laterality is not 275 * pre-coordinated in body site code. 276 */ 277 @Child(name = "laterality", type = { Coding.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 278 @Description(shortDefinition = "Body part laterality", formalDefinition = "Laterality if body site is paired anatomic structure and laterality is not pre-coordinated in body site code.") 279 protected Coding laterality; 280 281 /** 282 * The date and time the series was started. 283 */ 284 @Child(name = "started", type = { 285 DateTimeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 286 @Description(shortDefinition = "When the series started", formalDefinition = "The date and time the series was started.") 287 protected DateTimeType started; 288 289 /** 290 * A single SOP Instance within the series, e.g. an image, or presentation 291 * state. 292 */ 293 @Child(name = "instance", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 294 @Description(shortDefinition = "A single SOP instance from the series", formalDefinition = "A single SOP Instance within the series, e.g. an image, or presentation state.") 295 protected List<ImagingStudySeriesInstanceComponent> instance; 296 297 private static final long serialVersionUID = -1798366943L; 298 299 /* 300 * Constructor 301 */ 302 public ImagingStudySeriesComponent() { 303 super(); 304 } 305 306 /* 307 * Constructor 308 */ 309 public ImagingStudySeriesComponent(Coding modality, OidType uid, UnsignedIntType numberOfInstances) { 310 super(); 311 this.modality = modality; 312 this.uid = uid; 313 this.numberOfInstances = numberOfInstances; 314 } 315 316 /** 317 * @return {@link #number} (The Numeric identifier of this series in the 318 * study.). This is the underlying object with id, value and extensions. 319 * The accessor "getNumber" gives direct access to the value 320 */ 321 public UnsignedIntType getNumberElement() { 322 if (this.number == null) 323 if (Configuration.errorOnAutoCreate()) 324 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.number"); 325 else if (Configuration.doAutoCreate()) 326 this.number = new UnsignedIntType(); // bb 327 return this.number; 328 } 329 330 public boolean hasNumberElement() { 331 return this.number != null && !this.number.isEmpty(); 332 } 333 334 public boolean hasNumber() { 335 return this.number != null && !this.number.isEmpty(); 336 } 337 338 /** 339 * @param value {@link #number} (The Numeric identifier of this series in the 340 * study.). This is the underlying object with id, value and 341 * extensions. The accessor "getNumber" gives direct access to the 342 * value 343 */ 344 public ImagingStudySeriesComponent setNumberElement(UnsignedIntType value) { 345 this.number = value; 346 return this; 347 } 348 349 /** 350 * @return The Numeric identifier of this series in the study. 351 */ 352 public int getNumber() { 353 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 354 } 355 356 /** 357 * @param value The Numeric identifier of this series in the study. 358 */ 359 public ImagingStudySeriesComponent setNumber(int value) { 360 if (this.number == null) 361 this.number = new UnsignedIntType(); 362 this.number.setValue(value); 363 return this; 364 } 365 366 /** 367 * @return {@link #modality} (The modality of this series sequence.) 368 */ 369 public Coding getModality() { 370 if (this.modality == null) 371 if (Configuration.errorOnAutoCreate()) 372 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.modality"); 373 else if (Configuration.doAutoCreate()) 374 this.modality = new Coding(); // cc 375 return this.modality; 376 } 377 378 public boolean hasModality() { 379 return this.modality != null && !this.modality.isEmpty(); 380 } 381 382 /** 383 * @param value {@link #modality} (The modality of this series sequence.) 384 */ 385 public ImagingStudySeriesComponent setModality(Coding value) { 386 this.modality = value; 387 return this; 388 } 389 390 /** 391 * @return {@link #uid} (Formal identifier for this series.). This is the 392 * underlying object with id, value and extensions. The accessor 393 * "getUid" gives direct access to the value 394 */ 395 public OidType getUidElement() { 396 if (this.uid == null) 397 if (Configuration.errorOnAutoCreate()) 398 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.uid"); 399 else if (Configuration.doAutoCreate()) 400 this.uid = new OidType(); // bb 401 return this.uid; 402 } 403 404 public boolean hasUidElement() { 405 return this.uid != null && !this.uid.isEmpty(); 406 } 407 408 public boolean hasUid() { 409 return this.uid != null && !this.uid.isEmpty(); 410 } 411 412 /** 413 * @param value {@link #uid} (Formal identifier for this series.). This is the 414 * underlying object with id, value and extensions. The accessor 415 * "getUid" gives direct access to the value 416 */ 417 public ImagingStudySeriesComponent setUidElement(OidType value) { 418 this.uid = value; 419 return this; 420 } 421 422 /** 423 * @return Formal identifier for this series. 424 */ 425 public String getUid() { 426 return this.uid == null ? null : this.uid.getValue(); 427 } 428 429 /** 430 * @param value Formal identifier for this series. 431 */ 432 public ImagingStudySeriesComponent setUid(String value) { 433 if (this.uid == null) 434 this.uid = new OidType(); 435 this.uid.setValue(value); 436 return this; 437 } 438 439 /** 440 * @return {@link #description} (A description of the series.). This is the 441 * underlying object with id, value and extensions. The accessor 442 * "getDescription" gives direct access to the value 443 */ 444 public StringType getDescriptionElement() { 445 if (this.description == null) 446 if (Configuration.errorOnAutoCreate()) 447 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.description"); 448 else if (Configuration.doAutoCreate()) 449 this.description = new StringType(); // bb 450 return this.description; 451 } 452 453 public boolean hasDescriptionElement() { 454 return this.description != null && !this.description.isEmpty(); 455 } 456 457 public boolean hasDescription() { 458 return this.description != null && !this.description.isEmpty(); 459 } 460 461 /** 462 * @param value {@link #description} (A description of the series.). This is the 463 * underlying object with id, value and extensions. The accessor 464 * "getDescription" gives direct access to the value 465 */ 466 public ImagingStudySeriesComponent setDescriptionElement(StringType value) { 467 this.description = value; 468 return this; 469 } 470 471 /** 472 * @return A description of the series. 473 */ 474 public String getDescription() { 475 return this.description == null ? null : this.description.getValue(); 476 } 477 478 /** 479 * @param value A description of the series. 480 */ 481 public ImagingStudySeriesComponent setDescription(String value) { 482 if (Utilities.noString(value)) 483 this.description = null; 484 else { 485 if (this.description == null) 486 this.description = new StringType(); 487 this.description.setValue(value); 488 } 489 return this; 490 } 491 492 /** 493 * @return {@link #numberOfInstances} (Number of SOP Instances in Series.). This 494 * is the underlying object with id, value and extensions. The accessor 495 * "getNumberOfInstances" gives direct access to the value 496 */ 497 public UnsignedIntType getNumberOfInstancesElement() { 498 if (this.numberOfInstances == null) 499 if (Configuration.errorOnAutoCreate()) 500 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.numberOfInstances"); 501 else if (Configuration.doAutoCreate()) 502 this.numberOfInstances = new UnsignedIntType(); // bb 503 return this.numberOfInstances; 504 } 505 506 public boolean hasNumberOfInstancesElement() { 507 return this.numberOfInstances != null && !this.numberOfInstances.isEmpty(); 508 } 509 510 public boolean hasNumberOfInstances() { 511 return this.numberOfInstances != null && !this.numberOfInstances.isEmpty(); 512 } 513 514 /** 515 * @param value {@link #numberOfInstances} (Number of SOP Instances in Series.). 516 * This is the underlying object with id, value and extensions. The 517 * accessor "getNumberOfInstances" gives direct access to the value 518 */ 519 public ImagingStudySeriesComponent setNumberOfInstancesElement(UnsignedIntType value) { 520 this.numberOfInstances = value; 521 return this; 522 } 523 524 /** 525 * @return Number of SOP Instances in Series. 526 */ 527 public int getNumberOfInstances() { 528 return this.numberOfInstances == null || this.numberOfInstances.isEmpty() ? 0 : this.numberOfInstances.getValue(); 529 } 530 531 /** 532 * @param value Number of SOP Instances in Series. 533 */ 534 public ImagingStudySeriesComponent setNumberOfInstances(int value) { 535 if (this.numberOfInstances == null) 536 this.numberOfInstances = new UnsignedIntType(); 537 this.numberOfInstances.setValue(value); 538 return this; 539 } 540 541 /** 542 * @return {@link #availability} (Availability of series (online, offline or 543 * nearline).). This is the underlying object with id, value and 544 * extensions. The accessor "getAvailability" gives direct access to the 545 * value 546 */ 547 public Enumeration<InstanceAvailability> getAvailabilityElement() { 548 if (this.availability == null) 549 if (Configuration.errorOnAutoCreate()) 550 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.availability"); 551 else if (Configuration.doAutoCreate()) 552 this.availability = new Enumeration<InstanceAvailability>(new InstanceAvailabilityEnumFactory()); // bb 553 return this.availability; 554 } 555 556 public boolean hasAvailabilityElement() { 557 return this.availability != null && !this.availability.isEmpty(); 558 } 559 560 public boolean hasAvailability() { 561 return this.availability != null && !this.availability.isEmpty(); 562 } 563 564 /** 565 * @param value {@link #availability} (Availability of series (online, offline 566 * or nearline).). This is the underlying object with id, value and 567 * extensions. The accessor "getAvailability" gives direct access 568 * to the value 569 */ 570 public ImagingStudySeriesComponent setAvailabilityElement(Enumeration<InstanceAvailability> value) { 571 this.availability = value; 572 return this; 573 } 574 575 /** 576 * @return Availability of series (online, offline or nearline). 577 */ 578 public InstanceAvailability getAvailability() { 579 return this.availability == null ? null : this.availability.getValue(); 580 } 581 582 /** 583 * @param value Availability of series (online, offline or nearline). 584 */ 585 public ImagingStudySeriesComponent setAvailability(InstanceAvailability value) { 586 if (value == null) 587 this.availability = null; 588 else { 589 if (this.availability == null) 590 this.availability = new Enumeration<InstanceAvailability>(new InstanceAvailabilityEnumFactory()); 591 this.availability.setValue(value); 592 } 593 return this; 594 } 595 596 /** 597 * @return {@link #url} (URI/URL specifying the location of the referenced 598 * series using WADO-RS.). This is the underlying object with id, value 599 * and extensions. The accessor "getUrl" gives direct access to the 600 * value 601 */ 602 public UriType getUrlElement() { 603 if (this.url == null) 604 if (Configuration.errorOnAutoCreate()) 605 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.url"); 606 else if (Configuration.doAutoCreate()) 607 this.url = new UriType(); // bb 608 return this.url; 609 } 610 611 public boolean hasUrlElement() { 612 return this.url != null && !this.url.isEmpty(); 613 } 614 615 public boolean hasUrl() { 616 return this.url != null && !this.url.isEmpty(); 617 } 618 619 /** 620 * @param value {@link #url} (URI/URL specifying the location of the referenced 621 * series using WADO-RS.). This is the underlying object with id, 622 * value and extensions. The accessor "getUrl" gives direct access 623 * to the value 624 */ 625 public ImagingStudySeriesComponent setUrlElement(UriType value) { 626 this.url = value; 627 return this; 628 } 629 630 /** 631 * @return URI/URL specifying the location of the referenced series using 632 * WADO-RS. 633 */ 634 public String getUrl() { 635 return this.url == null ? null : this.url.getValue(); 636 } 637 638 /** 639 * @param value URI/URL specifying the location of the referenced series using 640 * WADO-RS. 641 */ 642 public ImagingStudySeriesComponent setUrl(String value) { 643 if (Utilities.noString(value)) 644 this.url = null; 645 else { 646 if (this.url == null) 647 this.url = new UriType(); 648 this.url.setValue(value); 649 } 650 return this; 651 } 652 653 /** 654 * @return {@link #bodySite} (Body part examined. See DICOM Part 16 Annex L for 655 * the mapping from DICOM to Snomed CT.) 656 */ 657 public Coding getBodySite() { 658 if (this.bodySite == null) 659 if (Configuration.errorOnAutoCreate()) 660 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.bodySite"); 661 else if (Configuration.doAutoCreate()) 662 this.bodySite = new Coding(); // cc 663 return this.bodySite; 664 } 665 666 public boolean hasBodySite() { 667 return this.bodySite != null && !this.bodySite.isEmpty(); 668 } 669 670 /** 671 * @param value {@link #bodySite} (Body part examined. See DICOM Part 16 Annex L 672 * for the mapping from DICOM to Snomed CT.) 673 */ 674 public ImagingStudySeriesComponent setBodySite(Coding value) { 675 this.bodySite = value; 676 return this; 677 } 678 679 /** 680 * @return {@link #laterality} (Laterality if body site is paired anatomic 681 * structure and laterality is not pre-coordinated in body site code.) 682 */ 683 public Coding getLaterality() { 684 if (this.laterality == null) 685 if (Configuration.errorOnAutoCreate()) 686 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.laterality"); 687 else if (Configuration.doAutoCreate()) 688 this.laterality = new Coding(); // cc 689 return this.laterality; 690 } 691 692 public boolean hasLaterality() { 693 return this.laterality != null && !this.laterality.isEmpty(); 694 } 695 696 /** 697 * @param value {@link #laterality} (Laterality if body site is paired anatomic 698 * structure and laterality is not pre-coordinated in body site 699 * code.) 700 */ 701 public ImagingStudySeriesComponent setLaterality(Coding value) { 702 this.laterality = value; 703 return this; 704 } 705 706 /** 707 * @return {@link #started} (The date and time the series was started.). This is 708 * the underlying object with id, value and extensions. The accessor 709 * "getStarted" gives direct access to the value 710 */ 711 public DateTimeType getStartedElement() { 712 if (this.started == null) 713 if (Configuration.errorOnAutoCreate()) 714 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.started"); 715 else if (Configuration.doAutoCreate()) 716 this.started = new DateTimeType(); // bb 717 return this.started; 718 } 719 720 public boolean hasStartedElement() { 721 return this.started != null && !this.started.isEmpty(); 722 } 723 724 public boolean hasStarted() { 725 return this.started != null && !this.started.isEmpty(); 726 } 727 728 /** 729 * @param value {@link #started} (The date and time the series was started.). 730 * This is the underlying object with id, value and extensions. The 731 * accessor "getStarted" gives direct access to the value 732 */ 733 public ImagingStudySeriesComponent setStartedElement(DateTimeType value) { 734 this.started = value; 735 return this; 736 } 737 738 /** 739 * @return The date and time the series was started. 740 */ 741 public Date getStarted() { 742 return this.started == null ? null : this.started.getValue(); 743 } 744 745 /** 746 * @param value The date and time the series was started. 747 */ 748 public ImagingStudySeriesComponent setStarted(Date value) { 749 if (value == null) 750 this.started = null; 751 else { 752 if (this.started == null) 753 this.started = new DateTimeType(); 754 this.started.setValue(value); 755 } 756 return this; 757 } 758 759 /** 760 * @return {@link #instance} (A single SOP Instance within the series, e.g. an 761 * image, or presentation state.) 762 */ 763 public List<ImagingStudySeriesInstanceComponent> getInstance() { 764 if (this.instance == null) 765 this.instance = new ArrayList<ImagingStudySeriesInstanceComponent>(); 766 return this.instance; 767 } 768 769 public boolean hasInstance() { 770 if (this.instance == null) 771 return false; 772 for (ImagingStudySeriesInstanceComponent item : this.instance) 773 if (!item.isEmpty()) 774 return true; 775 return false; 776 } 777 778 /** 779 * @return {@link #instance} (A single SOP Instance within the series, e.g. an 780 * image, or presentation state.) 781 */ 782 // syntactic sugar 783 public ImagingStudySeriesInstanceComponent addInstance() { // 3 784 ImagingStudySeriesInstanceComponent t = new ImagingStudySeriesInstanceComponent(); 785 if (this.instance == null) 786 this.instance = new ArrayList<ImagingStudySeriesInstanceComponent>(); 787 this.instance.add(t); 788 return t; 789 } 790 791 // syntactic sugar 792 public ImagingStudySeriesComponent addInstance(ImagingStudySeriesInstanceComponent t) { // 3 793 if (t == null) 794 return this; 795 if (this.instance == null) 796 this.instance = new ArrayList<ImagingStudySeriesInstanceComponent>(); 797 this.instance.add(t); 798 return this; 799 } 800 801 protected void listChildren(List<Property> childrenList) { 802 super.listChildren(childrenList); 803 childrenList.add(new Property("number", "unsignedInt", "The Numeric identifier of this series in the study.", 0, 804 java.lang.Integer.MAX_VALUE, number)); 805 childrenList.add(new Property("modality", "Coding", "The modality of this series sequence.", 0, 806 java.lang.Integer.MAX_VALUE, modality)); 807 childrenList 808 .add(new Property("uid", "oid", "Formal identifier for this series.", 0, java.lang.Integer.MAX_VALUE, uid)); 809 childrenList.add(new Property("description", "string", "A description of the series.", 0, 810 java.lang.Integer.MAX_VALUE, description)); 811 childrenList.add(new Property("numberOfInstances", "unsignedInt", "Number of SOP Instances in Series.", 0, 812 java.lang.Integer.MAX_VALUE, numberOfInstances)); 813 childrenList.add(new Property("availability", "code", "Availability of series (online, offline or nearline).", 0, 814 java.lang.Integer.MAX_VALUE, availability)); 815 childrenList 816 .add(new Property("url", "uri", "URI/URL specifying the location of the referenced series using WADO-RS.", 0, 817 java.lang.Integer.MAX_VALUE, url)); 818 childrenList.add(new Property("bodySite", "Coding", 819 "Body part examined. See DICOM Part 16 Annex L for the mapping from DICOM to Snomed CT.", 0, 820 java.lang.Integer.MAX_VALUE, bodySite)); 821 childrenList.add(new Property("laterality", "Coding", 822 "Laterality if body site is paired anatomic structure and laterality is not pre-coordinated in body site code.", 823 0, java.lang.Integer.MAX_VALUE, laterality)); 824 childrenList.add(new Property("started", "dateTime", "The date and time the series was started.", 0, 825 java.lang.Integer.MAX_VALUE, started)); 826 childrenList.add( 827 new Property("instance", "", "A single SOP Instance within the series, e.g. an image, or presentation state.", 828 0, java.lang.Integer.MAX_VALUE, instance)); 829 } 830 831 @Override 832 public void setProperty(String name, Base value) throws FHIRException { 833 if (name.equals("number")) 834 this.number = castToUnsignedInt(value); // UnsignedIntType 835 else if (name.equals("modality")) 836 this.modality = castToCoding(value); // Coding 837 else if (name.equals("uid")) 838 this.uid = castToOid(value); // OidType 839 else if (name.equals("description")) 840 this.description = castToString(value); // StringType 841 else if (name.equals("numberOfInstances")) 842 this.numberOfInstances = castToUnsignedInt(value); // UnsignedIntType 843 else if (name.equals("availability")) 844 this.availability = new InstanceAvailabilityEnumFactory().fromType(value); // Enumeration<InstanceAvailability> 845 else if (name.equals("url")) 846 this.url = castToUri(value); // UriType 847 else if (name.equals("bodySite")) 848 this.bodySite = castToCoding(value); // Coding 849 else if (name.equals("laterality")) 850 this.laterality = castToCoding(value); // Coding 851 else if (name.equals("started")) 852 this.started = castToDateTime(value); // DateTimeType 853 else if (name.equals("instance")) 854 this.getInstance().add((ImagingStudySeriesInstanceComponent) value); 855 else 856 super.setProperty(name, value); 857 } 858 859 @Override 860 public Base addChild(String name) throws FHIRException { 861 if (name.equals("number")) { 862 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.number"); 863 } else if (name.equals("modality")) { 864 this.modality = new Coding(); 865 return this.modality; 866 } else if (name.equals("uid")) { 867 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.uid"); 868 } else if (name.equals("description")) { 869 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.description"); 870 } else if (name.equals("numberOfInstances")) { 871 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.numberOfInstances"); 872 } else if (name.equals("availability")) { 873 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.availability"); 874 } else if (name.equals("url")) { 875 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.url"); 876 } else if (name.equals("bodySite")) { 877 this.bodySite = new Coding(); 878 return this.bodySite; 879 } else if (name.equals("laterality")) { 880 this.laterality = new Coding(); 881 return this.laterality; 882 } else if (name.equals("started")) { 883 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.started"); 884 } else if (name.equals("instance")) { 885 return addInstance(); 886 } else 887 return super.addChild(name); 888 } 889 890 public ImagingStudySeriesComponent copy() { 891 ImagingStudySeriesComponent dst = new ImagingStudySeriesComponent(); 892 copyValues(dst); 893 dst.number = number == null ? null : number.copy(); 894 dst.modality = modality == null ? null : modality.copy(); 895 dst.uid = uid == null ? null : uid.copy(); 896 dst.description = description == null ? null : description.copy(); 897 dst.numberOfInstances = numberOfInstances == null ? null : numberOfInstances.copy(); 898 dst.availability = availability == null ? null : availability.copy(); 899 dst.url = url == null ? null : url.copy(); 900 dst.bodySite = bodySite == null ? null : bodySite.copy(); 901 dst.laterality = laterality == null ? null : laterality.copy(); 902 dst.started = started == null ? null : started.copy(); 903 if (instance != null) { 904 dst.instance = new ArrayList<ImagingStudySeriesInstanceComponent>(); 905 for (ImagingStudySeriesInstanceComponent i : instance) 906 dst.instance.add(i.copy()); 907 } 908 ; 909 return dst; 910 } 911 912 @Override 913 public boolean equalsDeep(Base other) { 914 if (!super.equalsDeep(other)) 915 return false; 916 if (!(other instanceof ImagingStudySeriesComponent)) 917 return false; 918 ImagingStudySeriesComponent o = (ImagingStudySeriesComponent) other; 919 return compareDeep(number, o.number, true) && compareDeep(modality, o.modality, true) 920 && compareDeep(uid, o.uid, true) && compareDeep(description, o.description, true) 921 && compareDeep(numberOfInstances, o.numberOfInstances, true) 922 && compareDeep(availability, o.availability, true) && compareDeep(url, o.url, true) 923 && compareDeep(bodySite, o.bodySite, true) && compareDeep(laterality, o.laterality, true) 924 && compareDeep(started, o.started, true) && compareDeep(instance, o.instance, true); 925 } 926 927 @Override 928 public boolean equalsShallow(Base other) { 929 if (!super.equalsShallow(other)) 930 return false; 931 if (!(other instanceof ImagingStudySeriesComponent)) 932 return false; 933 ImagingStudySeriesComponent o = (ImagingStudySeriesComponent) other; 934 return compareValues(number, o.number, true) && compareValues(uid, o.uid, true) 935 && compareValues(description, o.description, true) 936 && compareValues(numberOfInstances, o.numberOfInstances, true) 937 && compareValues(availability, o.availability, true) && compareValues(url, o.url, true) 938 && compareValues(started, o.started, true); 939 } 940 941 public boolean isEmpty() { 942 return super.isEmpty() && (number == null || number.isEmpty()) && (modality == null || modality.isEmpty()) 943 && (uid == null || uid.isEmpty()) && (description == null || description.isEmpty()) 944 && (numberOfInstances == null || numberOfInstances.isEmpty()) 945 && (availability == null || availability.isEmpty()) && (url == null || url.isEmpty()) 946 && (bodySite == null || bodySite.isEmpty()) && (laterality == null || laterality.isEmpty()) 947 && (started == null || started.isEmpty()) && (instance == null || instance.isEmpty()); 948 } 949 950 public String fhirType() { 951 return "ImagingStudy.series"; 952 953 } 954 955 } 956 957 @Block() 958 public static class ImagingStudySeriesInstanceComponent extends BackboneElement implements IBaseBackboneElement { 959 /** 960 * The number of instance in the series. 961 */ 962 @Child(name = "number", type = { 963 UnsignedIntType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 964 @Description(shortDefinition = "The number of this instance in the series", formalDefinition = "The number of instance in the series.") 965 protected UnsignedIntType number; 966 967 /** 968 * Formal identifier for this image or other content. 969 */ 970 @Child(name = "uid", type = { OidType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 971 @Description(shortDefinition = "Formal identifier for this instance", formalDefinition = "Formal identifier for this image or other content.") 972 protected OidType uid; 973 974 /** 975 * DICOM instance type. 976 */ 977 @Child(name = "sopClass", type = { OidType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 978 @Description(shortDefinition = "DICOM class type", formalDefinition = "DICOM instance type.") 979 protected OidType sopClass; 980 981 /** 982 * A human-friendly SOP Class name. 983 */ 984 @Child(name = "type", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 985 @Description(shortDefinition = "Type of instance (image etc.)", formalDefinition = "A human-friendly SOP Class name.") 986 protected StringType type; 987 988 /** 989 * The description of the instance. 990 */ 991 @Child(name = "title", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 992 @Description(shortDefinition = "Description of instance", formalDefinition = "The description of the instance.") 993 protected StringType title; 994 995 /** 996 * Content of the instance or a rendering thereof (e.g. a JPEG of an image, or 997 * an XML of a structured report). May be represented for example by inline 998 * encoding; by a URL reference to a WADO-RS service that makes the instance 999 * available; or to a FHIR Resource (e.g. Media, Document, etc.). Multiple 1000 * content attachments may be used for alternate representations of the 1001 * instance. 1002 */ 1003 @Child(name = "content", type = { 1004 Attachment.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1005 @Description(shortDefinition = "Content of the instance", formalDefinition = "Content of the instance or a rendering thereof (e.g. a JPEG of an image, or an XML of a structured report). May be represented for example by inline encoding; by a URL reference to a WADO-RS service that makes the instance available; or to a FHIR Resource (e.g. Media, Document, etc.). Multiple content attachments may be used for alternate representations of the instance.") 1006 protected List<Attachment> content; 1007 1008 private static final long serialVersionUID = -1450403705L; 1009 1010 /* 1011 * Constructor 1012 */ 1013 public ImagingStudySeriesInstanceComponent() { 1014 super(); 1015 } 1016 1017 /* 1018 * Constructor 1019 */ 1020 public ImagingStudySeriesInstanceComponent(OidType uid, OidType sopClass) { 1021 super(); 1022 this.uid = uid; 1023 this.sopClass = sopClass; 1024 } 1025 1026 /** 1027 * @return {@link #number} (The number of instance in the series.). This is the 1028 * underlying object with id, value and extensions. The accessor 1029 * "getNumber" gives direct access to the value 1030 */ 1031 public UnsignedIntType getNumberElement() { 1032 if (this.number == null) 1033 if (Configuration.errorOnAutoCreate()) 1034 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.number"); 1035 else if (Configuration.doAutoCreate()) 1036 this.number = new UnsignedIntType(); // bb 1037 return this.number; 1038 } 1039 1040 public boolean hasNumberElement() { 1041 return this.number != null && !this.number.isEmpty(); 1042 } 1043 1044 public boolean hasNumber() { 1045 return this.number != null && !this.number.isEmpty(); 1046 } 1047 1048 /** 1049 * @param value {@link #number} (The number of instance in the series.). This is 1050 * the underlying object with id, value and extensions. The 1051 * accessor "getNumber" gives direct access to the value 1052 */ 1053 public ImagingStudySeriesInstanceComponent setNumberElement(UnsignedIntType value) { 1054 this.number = value; 1055 return this; 1056 } 1057 1058 /** 1059 * @return The number of instance in the series. 1060 */ 1061 public int getNumber() { 1062 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 1063 } 1064 1065 /** 1066 * @param value The number of instance in the series. 1067 */ 1068 public ImagingStudySeriesInstanceComponent setNumber(int value) { 1069 if (this.number == null) 1070 this.number = new UnsignedIntType(); 1071 this.number.setValue(value); 1072 return this; 1073 } 1074 1075 /** 1076 * @return {@link #uid} (Formal identifier for this image or other content.). 1077 * This is the underlying object with id, value and extensions. The 1078 * accessor "getUid" gives direct access to the value 1079 */ 1080 public OidType getUidElement() { 1081 if (this.uid == null) 1082 if (Configuration.errorOnAutoCreate()) 1083 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.uid"); 1084 else if (Configuration.doAutoCreate()) 1085 this.uid = new OidType(); // bb 1086 return this.uid; 1087 } 1088 1089 public boolean hasUidElement() { 1090 return this.uid != null && !this.uid.isEmpty(); 1091 } 1092 1093 public boolean hasUid() { 1094 return this.uid != null && !this.uid.isEmpty(); 1095 } 1096 1097 /** 1098 * @param value {@link #uid} (Formal identifier for this image or other 1099 * content.). This is the underlying object with id, value and 1100 * extensions. The accessor "getUid" gives direct access to the 1101 * value 1102 */ 1103 public ImagingStudySeriesInstanceComponent setUidElement(OidType value) { 1104 this.uid = value; 1105 return this; 1106 } 1107 1108 /** 1109 * @return Formal identifier for this image or other content. 1110 */ 1111 public String getUid() { 1112 return this.uid == null ? null : this.uid.getValue(); 1113 } 1114 1115 /** 1116 * @param value Formal identifier for this image or other content. 1117 */ 1118 public ImagingStudySeriesInstanceComponent setUid(String value) { 1119 if (this.uid == null) 1120 this.uid = new OidType(); 1121 this.uid.setValue(value); 1122 return this; 1123 } 1124 1125 /** 1126 * @return {@link #sopClass} (DICOM instance type.). This is the underlying 1127 * object with id, value and extensions. The accessor "getSopClass" 1128 * gives direct access to the value 1129 */ 1130 public OidType getSopClassElement() { 1131 if (this.sopClass == null) 1132 if (Configuration.errorOnAutoCreate()) 1133 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.sopClass"); 1134 else if (Configuration.doAutoCreate()) 1135 this.sopClass = new OidType(); // bb 1136 return this.sopClass; 1137 } 1138 1139 public boolean hasSopClassElement() { 1140 return this.sopClass != null && !this.sopClass.isEmpty(); 1141 } 1142 1143 public boolean hasSopClass() { 1144 return this.sopClass != null && !this.sopClass.isEmpty(); 1145 } 1146 1147 /** 1148 * @param value {@link #sopClass} (DICOM instance type.). This is the underlying 1149 * object with id, value and extensions. The accessor "getSopClass" 1150 * gives direct access to the value 1151 */ 1152 public ImagingStudySeriesInstanceComponent setSopClassElement(OidType value) { 1153 this.sopClass = value; 1154 return this; 1155 } 1156 1157 /** 1158 * @return DICOM instance type. 1159 */ 1160 public String getSopClass() { 1161 return this.sopClass == null ? null : this.sopClass.getValue(); 1162 } 1163 1164 /** 1165 * @param value DICOM instance type. 1166 */ 1167 public ImagingStudySeriesInstanceComponent setSopClass(String value) { 1168 if (this.sopClass == null) 1169 this.sopClass = new OidType(); 1170 this.sopClass.setValue(value); 1171 return this; 1172 } 1173 1174 /** 1175 * @return {@link #type} (A human-friendly SOP Class name.). This is the 1176 * underlying object with id, value and extensions. The accessor 1177 * "getType" gives direct access to the value 1178 */ 1179 public StringType getTypeElement() { 1180 if (this.type == null) 1181 if (Configuration.errorOnAutoCreate()) 1182 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.type"); 1183 else if (Configuration.doAutoCreate()) 1184 this.type = new StringType(); // bb 1185 return this.type; 1186 } 1187 1188 public boolean hasTypeElement() { 1189 return this.type != null && !this.type.isEmpty(); 1190 } 1191 1192 public boolean hasType() { 1193 return this.type != null && !this.type.isEmpty(); 1194 } 1195 1196 /** 1197 * @param value {@link #type} (A human-friendly SOP Class name.). This is the 1198 * underlying object with id, value and extensions. The accessor 1199 * "getType" gives direct access to the value 1200 */ 1201 public ImagingStudySeriesInstanceComponent setTypeElement(StringType value) { 1202 this.type = value; 1203 return this; 1204 } 1205 1206 /** 1207 * @return A human-friendly SOP Class name. 1208 */ 1209 public String getType() { 1210 return this.type == null ? null : this.type.getValue(); 1211 } 1212 1213 /** 1214 * @param value A human-friendly SOP Class name. 1215 */ 1216 public ImagingStudySeriesInstanceComponent setType(String value) { 1217 if (Utilities.noString(value)) 1218 this.type = null; 1219 else { 1220 if (this.type == null) 1221 this.type = new StringType(); 1222 this.type.setValue(value); 1223 } 1224 return this; 1225 } 1226 1227 /** 1228 * @return {@link #title} (The description of the instance.). This is the 1229 * underlying object with id, value and extensions. The accessor 1230 * "getTitle" gives direct access to the value 1231 */ 1232 public StringType getTitleElement() { 1233 if (this.title == null) 1234 if (Configuration.errorOnAutoCreate()) 1235 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.title"); 1236 else if (Configuration.doAutoCreate()) 1237 this.title = new StringType(); // bb 1238 return this.title; 1239 } 1240 1241 public boolean hasTitleElement() { 1242 return this.title != null && !this.title.isEmpty(); 1243 } 1244 1245 public boolean hasTitle() { 1246 return this.title != null && !this.title.isEmpty(); 1247 } 1248 1249 /** 1250 * @param value {@link #title} (The description of the instance.). This is the 1251 * underlying object with id, value and extensions. The accessor 1252 * "getTitle" gives direct access to the value 1253 */ 1254 public ImagingStudySeriesInstanceComponent setTitleElement(StringType value) { 1255 this.title = value; 1256 return this; 1257 } 1258 1259 /** 1260 * @return The description of the instance. 1261 */ 1262 public String getTitle() { 1263 return this.title == null ? null : this.title.getValue(); 1264 } 1265 1266 /** 1267 * @param value The description of the instance. 1268 */ 1269 public ImagingStudySeriesInstanceComponent setTitle(String value) { 1270 if (Utilities.noString(value)) 1271 this.title = null; 1272 else { 1273 if (this.title == null) 1274 this.title = new StringType(); 1275 this.title.setValue(value); 1276 } 1277 return this; 1278 } 1279 1280 /** 1281 * @return {@link #content} (Content of the instance or a rendering thereof 1282 * (e.g. a JPEG of an image, or an XML of a structured report). May be 1283 * represented for example by inline encoding; by a URL reference to a 1284 * WADO-RS service that makes the instance available; or to a FHIR 1285 * Resource (e.g. Media, Document, etc.). Multiple content attachments 1286 * may be used for alternate representations of the instance.) 1287 */ 1288 public List<Attachment> getContent() { 1289 if (this.content == null) 1290 this.content = new ArrayList<Attachment>(); 1291 return this.content; 1292 } 1293 1294 public boolean hasContent() { 1295 if (this.content == null) 1296 return false; 1297 for (Attachment item : this.content) 1298 if (!item.isEmpty()) 1299 return true; 1300 return false; 1301 } 1302 1303 /** 1304 * @return {@link #content} (Content of the instance or a rendering thereof 1305 * (e.g. a JPEG of an image, or an XML of a structured report). May be 1306 * represented for example by inline encoding; by a URL reference to a 1307 * WADO-RS service that makes the instance available; or to a FHIR 1308 * Resource (e.g. Media, Document, etc.). Multiple content attachments 1309 * may be used for alternate representations of the instance.) 1310 */ 1311 // syntactic sugar 1312 public Attachment addContent() { // 3 1313 Attachment t = new Attachment(); 1314 if (this.content == null) 1315 this.content = new ArrayList<Attachment>(); 1316 this.content.add(t); 1317 return t; 1318 } 1319 1320 // syntactic sugar 1321 public ImagingStudySeriesInstanceComponent addContent(Attachment t) { // 3 1322 if (t == null) 1323 return this; 1324 if (this.content == null) 1325 this.content = new ArrayList<Attachment>(); 1326 this.content.add(t); 1327 return this; 1328 } 1329 1330 protected void listChildren(List<Property> childrenList) { 1331 super.listChildren(childrenList); 1332 childrenList.add(new Property("number", "unsignedInt", "The number of instance in the series.", 0, 1333 java.lang.Integer.MAX_VALUE, number)); 1334 childrenList.add(new Property("uid", "oid", "Formal identifier for this image or other content.", 0, 1335 java.lang.Integer.MAX_VALUE, uid)); 1336 childrenList 1337 .add(new Property("sopClass", "oid", "DICOM instance type.", 0, java.lang.Integer.MAX_VALUE, sopClass)); 1338 childrenList.add( 1339 new Property("type", "string", "A human-friendly SOP Class name.", 0, java.lang.Integer.MAX_VALUE, type)); 1340 childrenList.add( 1341 new Property("title", "string", "The description of the instance.", 0, java.lang.Integer.MAX_VALUE, title)); 1342 childrenList.add(new Property("content", "Attachment", 1343 "Content of the instance or a rendering thereof (e.g. a JPEG of an image, or an XML of a structured report). May be represented for example by inline encoding; by a URL reference to a WADO-RS service that makes the instance available; or to a FHIR Resource (e.g. Media, Document, etc.). Multiple content attachments may be used for alternate representations of the instance.", 1344 0, java.lang.Integer.MAX_VALUE, content)); 1345 } 1346 1347 @Override 1348 public void setProperty(String name, Base value) throws FHIRException { 1349 if (name.equals("number")) 1350 this.number = castToUnsignedInt(value); // UnsignedIntType 1351 else if (name.equals("uid")) 1352 this.uid = castToOid(value); // OidType 1353 else if (name.equals("sopClass")) 1354 this.sopClass = castToOid(value); // OidType 1355 else if (name.equals("type")) 1356 this.type = castToString(value); // StringType 1357 else if (name.equals("title")) 1358 this.title = castToString(value); // StringType 1359 else if (name.equals("content")) 1360 this.getContent().add(castToAttachment(value)); 1361 else 1362 super.setProperty(name, value); 1363 } 1364 1365 @Override 1366 public Base addChild(String name) throws FHIRException { 1367 if (name.equals("number")) { 1368 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.number"); 1369 } else if (name.equals("uid")) { 1370 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.uid"); 1371 } else if (name.equals("sopClass")) { 1372 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.sopClass"); 1373 } else if (name.equals("type")) { 1374 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.type"); 1375 } else if (name.equals("title")) { 1376 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.title"); 1377 } else if (name.equals("content")) { 1378 return addContent(); 1379 } else 1380 return super.addChild(name); 1381 } 1382 1383 public ImagingStudySeriesInstanceComponent copy() { 1384 ImagingStudySeriesInstanceComponent dst = new ImagingStudySeriesInstanceComponent(); 1385 copyValues(dst); 1386 dst.number = number == null ? null : number.copy(); 1387 dst.uid = uid == null ? null : uid.copy(); 1388 dst.sopClass = sopClass == null ? null : sopClass.copy(); 1389 dst.type = type == null ? null : type.copy(); 1390 dst.title = title == null ? null : title.copy(); 1391 if (content != null) { 1392 dst.content = new ArrayList<Attachment>(); 1393 for (Attachment i : content) 1394 dst.content.add(i.copy()); 1395 } 1396 ; 1397 return dst; 1398 } 1399 1400 @Override 1401 public boolean equalsDeep(Base other) { 1402 if (!super.equalsDeep(other)) 1403 return false; 1404 if (!(other instanceof ImagingStudySeriesInstanceComponent)) 1405 return false; 1406 ImagingStudySeriesInstanceComponent o = (ImagingStudySeriesInstanceComponent) other; 1407 return compareDeep(number, o.number, true) && compareDeep(uid, o.uid, true) 1408 && compareDeep(sopClass, o.sopClass, true) && compareDeep(type, o.type, true) 1409 && compareDeep(title, o.title, true) && compareDeep(content, o.content, true); 1410 } 1411 1412 @Override 1413 public boolean equalsShallow(Base other) { 1414 if (!super.equalsShallow(other)) 1415 return false; 1416 if (!(other instanceof ImagingStudySeriesInstanceComponent)) 1417 return false; 1418 ImagingStudySeriesInstanceComponent o = (ImagingStudySeriesInstanceComponent) other; 1419 return compareValues(number, o.number, true) && compareValues(uid, o.uid, true) 1420 && compareValues(sopClass, o.sopClass, true) && compareValues(type, o.type, true) 1421 && compareValues(title, o.title, true); 1422 } 1423 1424 public boolean isEmpty() { 1425 return super.isEmpty() && (number == null || number.isEmpty()) && (uid == null || uid.isEmpty()) 1426 && (sopClass == null || sopClass.isEmpty()) && (type == null || type.isEmpty()) 1427 && (title == null || title.isEmpty()) && (content == null || content.isEmpty()); 1428 } 1429 1430 public String fhirType() { 1431 return "ImagingStudy.series.instance"; 1432 1433 } 1434 1435 } 1436 1437 /** 1438 * Date and Time the study started. 1439 */ 1440 @Child(name = "started", type = { DateTimeType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 1441 @Description(shortDefinition = "When the study was started", formalDefinition = "Date and Time the study started.") 1442 protected DateTimeType started; 1443 1444 /** 1445 * The patient imaged in the study. 1446 */ 1447 @Child(name = "patient", type = { Patient.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1448 @Description(shortDefinition = "Who the images are of", formalDefinition = "The patient imaged in the study.") 1449 protected Reference patient; 1450 1451 /** 1452 * The actual object that is the target of the reference (The patient imaged in 1453 * the study.) 1454 */ 1455 protected Patient patientTarget; 1456 1457 /** 1458 * Formal identifier for the study. 1459 */ 1460 @Child(name = "uid", type = { OidType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1461 @Description(shortDefinition = "Formal identifier for the study", formalDefinition = "Formal identifier for the study.") 1462 protected OidType uid; 1463 1464 /** 1465 * Accession Number is an identifier related to some aspect of imaging workflow 1466 * and data management. Usage may vary across different institutions. See for 1467 * instance [IHE Radiology Technical Framework Volume 1 Appendix 1468 * A](http://www.ihe.net/uploadedFiles/Documents/Radiology/IHE_RAD_TF_Rev13.0_Vol1_FT_2014-07-30.pdf). 1469 */ 1470 @Child(name = "accession", type = { Identifier.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1471 @Description(shortDefinition = "Related workflow identifier (\"Accession Number\")", formalDefinition = "Accession Number is an identifier related to some aspect of imaging workflow and data management. Usage may vary across different institutions. See for instance [IHE Radiology Technical Framework Volume 1 Appendix A](http://www.ihe.net/uploadedFiles/Documents/Radiology/IHE_RAD_TF_Rev13.0_Vol1_FT_2014-07-30.pdf).") 1472 protected Identifier accession; 1473 1474 /** 1475 * Other identifiers for the study. 1476 */ 1477 @Child(name = "identifier", type = { 1478 Identifier.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1479 @Description(shortDefinition = "Other identifiers for the study", formalDefinition = "Other identifiers for the study.") 1480 protected List<Identifier> identifier; 1481 1482 /** 1483 * A list of the diagnostic orders that resulted in this imaging study being 1484 * performed. 1485 */ 1486 @Child(name = "order", type = { 1487 DiagnosticOrder.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1488 @Description(shortDefinition = "Order(s) that caused this study to be performed", formalDefinition = "A list of the diagnostic orders that resulted in this imaging study being performed.") 1489 protected List<Reference> order; 1490 /** 1491 * The actual objects that are the target of the reference (A list of the 1492 * diagnostic orders that resulted in this imaging study being performed.) 1493 */ 1494 protected List<DiagnosticOrder> orderTarget; 1495 1496 /** 1497 * A list of all the Series.ImageModality values that are actual acquisition 1498 * modalities, i.e. those in the DICOM Context Group 29 (value set OID 1499 * 1.2.840.10008.6.1.19). 1500 */ 1501 @Child(name = "modalityList", type = { 1502 Coding.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1503 @Description(shortDefinition = "All series modality if actual acquisition modalities", formalDefinition = "A list of all the Series.ImageModality values that are actual acquisition modalities, i.e. those in the DICOM Context Group 29 (value set OID 1.2.840.10008.6.1.19).") 1504 protected List<Coding> modalityList; 1505 1506 /** 1507 * The requesting/referring physician. 1508 */ 1509 @Child(name = "referrer", type = { 1510 Practitioner.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1511 @Description(shortDefinition = "Referring physician (0008,0090)", formalDefinition = "The requesting/referring physician.") 1512 protected Reference referrer; 1513 1514 /** 1515 * The actual object that is the target of the reference (The 1516 * requesting/referring physician.) 1517 */ 1518 protected Practitioner referrerTarget; 1519 1520 /** 1521 * Availability of study (online, offline or nearline). 1522 */ 1523 @Child(name = "availability", type = { 1524 CodeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1525 @Description(shortDefinition = "ONLINE | OFFLINE | NEARLINE | UNAVAILABLE (0008,0056)", formalDefinition = "Availability of study (online, offline or nearline).") 1526 protected Enumeration<InstanceAvailability> availability; 1527 1528 /** 1529 * WADO-RS resource where Study is available. 1530 */ 1531 @Child(name = "url", type = { UriType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1532 @Description(shortDefinition = "Retrieve URI", formalDefinition = "WADO-RS resource where Study is available.") 1533 protected UriType url; 1534 1535 /** 1536 * Number of Series in Study. 1537 */ 1538 @Child(name = "numberOfSeries", type = { 1539 UnsignedIntType.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 1540 @Description(shortDefinition = "Number of Study Related Series", formalDefinition = "Number of Series in Study.") 1541 protected UnsignedIntType numberOfSeries; 1542 1543 /** 1544 * Number of SOP Instances in Study. 1545 */ 1546 @Child(name = "numberOfInstances", type = { 1547 UnsignedIntType.class }, order = 11, min = 1, max = 1, modifier = false, summary = true) 1548 @Description(shortDefinition = "Number of Study Related Instances", formalDefinition = "Number of SOP Instances in Study.") 1549 protected UnsignedIntType numberOfInstances; 1550 1551 /** 1552 * Type of procedure performed. 1553 */ 1554 @Child(name = "procedure", type = { 1555 Procedure.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1556 @Description(shortDefinition = "Type of procedure performed", formalDefinition = "Type of procedure performed.") 1557 protected List<Reference> procedure; 1558 /** 1559 * The actual objects that are the target of the reference (Type of procedure 1560 * performed.) 1561 */ 1562 protected List<Procedure> procedureTarget; 1563 1564 /** 1565 * Who read the study and interpreted the images or other content. 1566 */ 1567 @Child(name = "interpreter", type = { 1568 Practitioner.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 1569 @Description(shortDefinition = "Who interpreted images", formalDefinition = "Who read the study and interpreted the images or other content.") 1570 protected Reference interpreter; 1571 1572 /** 1573 * The actual object that is the target of the reference (Who read the study and 1574 * interpreted the images or other content.) 1575 */ 1576 protected Practitioner interpreterTarget; 1577 1578 /** 1579 * Institution-generated description or classification of the Study performed. 1580 */ 1581 @Child(name = "description", type = { 1582 StringType.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 1583 @Description(shortDefinition = "Institution-generated description", formalDefinition = "Institution-generated description or classification of the Study performed.") 1584 protected StringType description; 1585 1586 /** 1587 * Each study has one or more series of images or other content. 1588 */ 1589 @Child(name = "series", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1590 @Description(shortDefinition = "Each study has one or more series of instances", formalDefinition = "Each study has one or more series of images or other content.") 1591 protected List<ImagingStudySeriesComponent> series; 1592 1593 private static final long serialVersionUID = 1895046380L; 1594 1595 /* 1596 * Constructor 1597 */ 1598 public ImagingStudy() { 1599 super(); 1600 } 1601 1602 /* 1603 * Constructor 1604 */ 1605 public ImagingStudy(Reference patient, OidType uid, UnsignedIntType numberOfSeries, 1606 UnsignedIntType numberOfInstances) { 1607 super(); 1608 this.patient = patient; 1609 this.uid = uid; 1610 this.numberOfSeries = numberOfSeries; 1611 this.numberOfInstances = numberOfInstances; 1612 } 1613 1614 /** 1615 * @return {@link #started} (Date and Time the study started.). This is the 1616 * underlying object with id, value and extensions. The accessor 1617 * "getStarted" gives direct access to the value 1618 */ 1619 public DateTimeType getStartedElement() { 1620 if (this.started == null) 1621 if (Configuration.errorOnAutoCreate()) 1622 throw new Error("Attempt to auto-create ImagingStudy.started"); 1623 else if (Configuration.doAutoCreate()) 1624 this.started = new DateTimeType(); // bb 1625 return this.started; 1626 } 1627 1628 public boolean hasStartedElement() { 1629 return this.started != null && !this.started.isEmpty(); 1630 } 1631 1632 public boolean hasStarted() { 1633 return this.started != null && !this.started.isEmpty(); 1634 } 1635 1636 /** 1637 * @param value {@link #started} (Date and Time the study started.). This is the 1638 * underlying object with id, value and extensions. The accessor 1639 * "getStarted" gives direct access to the value 1640 */ 1641 public ImagingStudy setStartedElement(DateTimeType value) { 1642 this.started = value; 1643 return this; 1644 } 1645 1646 /** 1647 * @return Date and Time the study started. 1648 */ 1649 public Date getStarted() { 1650 return this.started == null ? null : this.started.getValue(); 1651 } 1652 1653 /** 1654 * @param value Date and Time the study started. 1655 */ 1656 public ImagingStudy setStarted(Date value) { 1657 if (value == null) 1658 this.started = null; 1659 else { 1660 if (this.started == null) 1661 this.started = new DateTimeType(); 1662 this.started.setValue(value); 1663 } 1664 return this; 1665 } 1666 1667 /** 1668 * @return {@link #patient} (The patient imaged in the study.) 1669 */ 1670 public Reference getPatient() { 1671 if (this.patient == null) 1672 if (Configuration.errorOnAutoCreate()) 1673 throw new Error("Attempt to auto-create ImagingStudy.patient"); 1674 else if (Configuration.doAutoCreate()) 1675 this.patient = new Reference(); // cc 1676 return this.patient; 1677 } 1678 1679 public boolean hasPatient() { 1680 return this.patient != null && !this.patient.isEmpty(); 1681 } 1682 1683 /** 1684 * @param value {@link #patient} (The patient imaged in the study.) 1685 */ 1686 public ImagingStudy setPatient(Reference value) { 1687 this.patient = value; 1688 return this; 1689 } 1690 1691 /** 1692 * @return {@link #patient} The actual object that is the target of the 1693 * reference. The reference library doesn't populate this, but you can 1694 * use it to hold the resource if you resolve it. (The patient imaged in 1695 * the study.) 1696 */ 1697 public Patient getPatientTarget() { 1698 if (this.patientTarget == null) 1699 if (Configuration.errorOnAutoCreate()) 1700 throw new Error("Attempt to auto-create ImagingStudy.patient"); 1701 else if (Configuration.doAutoCreate()) 1702 this.patientTarget = new Patient(); // aa 1703 return this.patientTarget; 1704 } 1705 1706 /** 1707 * @param value {@link #patient} The actual object that is the target of the 1708 * reference. The reference library doesn't use these, but you can 1709 * use it to hold the resource if you resolve it. (The patient 1710 * imaged in the study.) 1711 */ 1712 public ImagingStudy setPatientTarget(Patient value) { 1713 this.patientTarget = value; 1714 return this; 1715 } 1716 1717 /** 1718 * @return {@link #uid} (Formal identifier for the study.). This is the 1719 * underlying object with id, value and extensions. The accessor 1720 * "getUid" gives direct access to the value 1721 */ 1722 public OidType getUidElement() { 1723 if (this.uid == null) 1724 if (Configuration.errorOnAutoCreate()) 1725 throw new Error("Attempt to auto-create ImagingStudy.uid"); 1726 else if (Configuration.doAutoCreate()) 1727 this.uid = new OidType(); // bb 1728 return this.uid; 1729 } 1730 1731 public boolean hasUidElement() { 1732 return this.uid != null && !this.uid.isEmpty(); 1733 } 1734 1735 public boolean hasUid() { 1736 return this.uid != null && !this.uid.isEmpty(); 1737 } 1738 1739 /** 1740 * @param value {@link #uid} (Formal identifier for the study.). This is the 1741 * underlying object with id, value and extensions. The accessor 1742 * "getUid" gives direct access to the value 1743 */ 1744 public ImagingStudy setUidElement(OidType value) { 1745 this.uid = value; 1746 return this; 1747 } 1748 1749 /** 1750 * @return Formal identifier for the study. 1751 */ 1752 public String getUid() { 1753 return this.uid == null ? null : this.uid.getValue(); 1754 } 1755 1756 /** 1757 * @param value Formal identifier for the study. 1758 */ 1759 public ImagingStudy setUid(String value) { 1760 if (this.uid == null) 1761 this.uid = new OidType(); 1762 this.uid.setValue(value); 1763 return this; 1764 } 1765 1766 /** 1767 * @return {@link #accession} (Accession Number is an identifier related to some 1768 * aspect of imaging workflow and data management. Usage may vary across 1769 * different institutions. See for instance [IHE Radiology Technical 1770 * Framework Volume 1 Appendix 1771 * A](http://www.ihe.net/uploadedFiles/Documents/Radiology/IHE_RAD_TF_Rev13.0_Vol1_FT_2014-07-30.pdf).) 1772 */ 1773 public Identifier getAccession() { 1774 if (this.accession == null) 1775 if (Configuration.errorOnAutoCreate()) 1776 throw new Error("Attempt to auto-create ImagingStudy.accession"); 1777 else if (Configuration.doAutoCreate()) 1778 this.accession = new Identifier(); // cc 1779 return this.accession; 1780 } 1781 1782 public boolean hasAccession() { 1783 return this.accession != null && !this.accession.isEmpty(); 1784 } 1785 1786 /** 1787 * @param value {@link #accession} (Accession Number is an identifier related to 1788 * some aspect of imaging workflow and data management. Usage may 1789 * vary across different institutions. See for instance [IHE 1790 * Radiology Technical Framework Volume 1 Appendix 1791 * A](http://www.ihe.net/uploadedFiles/Documents/Radiology/IHE_RAD_TF_Rev13.0_Vol1_FT_2014-07-30.pdf).) 1792 */ 1793 public ImagingStudy setAccession(Identifier value) { 1794 this.accession = value; 1795 return this; 1796 } 1797 1798 /** 1799 * @return {@link #identifier} (Other identifiers for the study.) 1800 */ 1801 public List<Identifier> getIdentifier() { 1802 if (this.identifier == null) 1803 this.identifier = new ArrayList<Identifier>(); 1804 return this.identifier; 1805 } 1806 1807 public boolean hasIdentifier() { 1808 if (this.identifier == null) 1809 return false; 1810 for (Identifier item : this.identifier) 1811 if (!item.isEmpty()) 1812 return true; 1813 return false; 1814 } 1815 1816 /** 1817 * @return {@link #identifier} (Other identifiers for the study.) 1818 */ 1819 // syntactic sugar 1820 public Identifier addIdentifier() { // 3 1821 Identifier t = new Identifier(); 1822 if (this.identifier == null) 1823 this.identifier = new ArrayList<Identifier>(); 1824 this.identifier.add(t); 1825 return t; 1826 } 1827 1828 // syntactic sugar 1829 public ImagingStudy addIdentifier(Identifier t) { // 3 1830 if (t == null) 1831 return this; 1832 if (this.identifier == null) 1833 this.identifier = new ArrayList<Identifier>(); 1834 this.identifier.add(t); 1835 return this; 1836 } 1837 1838 /** 1839 * @return {@link #order} (A list of the diagnostic orders that resulted in this 1840 * imaging study being performed.) 1841 */ 1842 public List<Reference> getOrder() { 1843 if (this.order == null) 1844 this.order = new ArrayList<Reference>(); 1845 return this.order; 1846 } 1847 1848 public boolean hasOrder() { 1849 if (this.order == null) 1850 return false; 1851 for (Reference item : this.order) 1852 if (!item.isEmpty()) 1853 return true; 1854 return false; 1855 } 1856 1857 /** 1858 * @return {@link #order} (A list of the diagnostic orders that resulted in this 1859 * imaging study being performed.) 1860 */ 1861 // syntactic sugar 1862 public Reference addOrder() { // 3 1863 Reference t = new Reference(); 1864 if (this.order == null) 1865 this.order = new ArrayList<Reference>(); 1866 this.order.add(t); 1867 return t; 1868 } 1869 1870 // syntactic sugar 1871 public ImagingStudy addOrder(Reference t) { // 3 1872 if (t == null) 1873 return this; 1874 if (this.order == null) 1875 this.order = new ArrayList<Reference>(); 1876 this.order.add(t); 1877 return this; 1878 } 1879 1880 /** 1881 * @return {@link #order} (The actual objects that are the target of the 1882 * reference. The reference library doesn't populate this, but you can 1883 * use this to hold the resources if you resolvethemt. A list of the 1884 * diagnostic orders that resulted in this imaging study being 1885 * performed.) 1886 */ 1887 public List<DiagnosticOrder> getOrderTarget() { 1888 if (this.orderTarget == null) 1889 this.orderTarget = new ArrayList<DiagnosticOrder>(); 1890 return this.orderTarget; 1891 } 1892 1893 // syntactic sugar 1894 /** 1895 * @return {@link #order} (Add an actual object that is the target of the 1896 * reference. The reference library doesn't use these, but you can use 1897 * this to hold the resources if you resolvethemt. A list of the 1898 * diagnostic orders that resulted in this imaging study being 1899 * performed.) 1900 */ 1901 public DiagnosticOrder addOrderTarget() { 1902 DiagnosticOrder r = new DiagnosticOrder(); 1903 if (this.orderTarget == null) 1904 this.orderTarget = new ArrayList<DiagnosticOrder>(); 1905 this.orderTarget.add(r); 1906 return r; 1907 } 1908 1909 /** 1910 * @return {@link #modalityList} (A list of all the Series.ImageModality values 1911 * that are actual acquisition modalities, i.e. those in the DICOM 1912 * Context Group 29 (value set OID 1.2.840.10008.6.1.19).) 1913 */ 1914 public List<Coding> getModalityList() { 1915 if (this.modalityList == null) 1916 this.modalityList = new ArrayList<Coding>(); 1917 return this.modalityList; 1918 } 1919 1920 public boolean hasModalityList() { 1921 if (this.modalityList == null) 1922 return false; 1923 for (Coding item : this.modalityList) 1924 if (!item.isEmpty()) 1925 return true; 1926 return false; 1927 } 1928 1929 /** 1930 * @return {@link #modalityList} (A list of all the Series.ImageModality values 1931 * that are actual acquisition modalities, i.e. those in the DICOM 1932 * Context Group 29 (value set OID 1.2.840.10008.6.1.19).) 1933 */ 1934 // syntactic sugar 1935 public Coding addModalityList() { // 3 1936 Coding t = new Coding(); 1937 if (this.modalityList == null) 1938 this.modalityList = new ArrayList<Coding>(); 1939 this.modalityList.add(t); 1940 return t; 1941 } 1942 1943 // syntactic sugar 1944 public ImagingStudy addModalityList(Coding t) { // 3 1945 if (t == null) 1946 return this; 1947 if (this.modalityList == null) 1948 this.modalityList = new ArrayList<Coding>(); 1949 this.modalityList.add(t); 1950 return this; 1951 } 1952 1953 /** 1954 * @return {@link #referrer} (The requesting/referring physician.) 1955 */ 1956 public Reference getReferrer() { 1957 if (this.referrer == null) 1958 if (Configuration.errorOnAutoCreate()) 1959 throw new Error("Attempt to auto-create ImagingStudy.referrer"); 1960 else if (Configuration.doAutoCreate()) 1961 this.referrer = new Reference(); // cc 1962 return this.referrer; 1963 } 1964 1965 public boolean hasReferrer() { 1966 return this.referrer != null && !this.referrer.isEmpty(); 1967 } 1968 1969 /** 1970 * @param value {@link #referrer} (The requesting/referring physician.) 1971 */ 1972 public ImagingStudy setReferrer(Reference value) { 1973 this.referrer = value; 1974 return this; 1975 } 1976 1977 /** 1978 * @return {@link #referrer} The actual object that is the target of the 1979 * reference. The reference library doesn't populate this, but you can 1980 * use it to hold the resource if you resolve it. (The 1981 * requesting/referring physician.) 1982 */ 1983 public Practitioner getReferrerTarget() { 1984 if (this.referrerTarget == null) 1985 if (Configuration.errorOnAutoCreate()) 1986 throw new Error("Attempt to auto-create ImagingStudy.referrer"); 1987 else if (Configuration.doAutoCreate()) 1988 this.referrerTarget = new Practitioner(); // aa 1989 return this.referrerTarget; 1990 } 1991 1992 /** 1993 * @param value {@link #referrer} The actual object that is the target of the 1994 * reference. The reference library doesn't use these, but you can 1995 * use it to hold the resource if you resolve it. (The 1996 * requesting/referring physician.) 1997 */ 1998 public ImagingStudy setReferrerTarget(Practitioner value) { 1999 this.referrerTarget = value; 2000 return this; 2001 } 2002 2003 /** 2004 * @return {@link #availability} (Availability of study (online, offline or 2005 * nearline).). This is the underlying object with id, value and 2006 * extensions. The accessor "getAvailability" gives direct access to the 2007 * value 2008 */ 2009 public Enumeration<InstanceAvailability> getAvailabilityElement() { 2010 if (this.availability == null) 2011 if (Configuration.errorOnAutoCreate()) 2012 throw new Error("Attempt to auto-create ImagingStudy.availability"); 2013 else if (Configuration.doAutoCreate()) 2014 this.availability = new Enumeration<InstanceAvailability>(new InstanceAvailabilityEnumFactory()); // bb 2015 return this.availability; 2016 } 2017 2018 public boolean hasAvailabilityElement() { 2019 return this.availability != null && !this.availability.isEmpty(); 2020 } 2021 2022 public boolean hasAvailability() { 2023 return this.availability != null && !this.availability.isEmpty(); 2024 } 2025 2026 /** 2027 * @param value {@link #availability} (Availability of study (online, offline or 2028 * nearline).). This is the underlying object with id, value and 2029 * extensions. The accessor "getAvailability" gives direct access 2030 * to the value 2031 */ 2032 public ImagingStudy setAvailabilityElement(Enumeration<InstanceAvailability> value) { 2033 this.availability = value; 2034 return this; 2035 } 2036 2037 /** 2038 * @return Availability of study (online, offline or nearline). 2039 */ 2040 public InstanceAvailability getAvailability() { 2041 return this.availability == null ? null : this.availability.getValue(); 2042 } 2043 2044 /** 2045 * @param value Availability of study (online, offline or nearline). 2046 */ 2047 public ImagingStudy setAvailability(InstanceAvailability value) { 2048 if (value == null) 2049 this.availability = null; 2050 else { 2051 if (this.availability == null) 2052 this.availability = new Enumeration<InstanceAvailability>(new InstanceAvailabilityEnumFactory()); 2053 this.availability.setValue(value); 2054 } 2055 return this; 2056 } 2057 2058 /** 2059 * @return {@link #url} (WADO-RS resource where Study is available.). This is 2060 * the underlying object with id, value and extensions. The accessor 2061 * "getUrl" gives direct access to the value 2062 */ 2063 public UriType getUrlElement() { 2064 if (this.url == null) 2065 if (Configuration.errorOnAutoCreate()) 2066 throw new Error("Attempt to auto-create ImagingStudy.url"); 2067 else if (Configuration.doAutoCreate()) 2068 this.url = new UriType(); // bb 2069 return this.url; 2070 } 2071 2072 public boolean hasUrlElement() { 2073 return this.url != null && !this.url.isEmpty(); 2074 } 2075 2076 public boolean hasUrl() { 2077 return this.url != null && !this.url.isEmpty(); 2078 } 2079 2080 /** 2081 * @param value {@link #url} (WADO-RS resource where Study is available.). This 2082 * is the underlying object with id, value and extensions. The 2083 * accessor "getUrl" gives direct access to the value 2084 */ 2085 public ImagingStudy setUrlElement(UriType value) { 2086 this.url = value; 2087 return this; 2088 } 2089 2090 /** 2091 * @return WADO-RS resource where Study is available. 2092 */ 2093 public String getUrl() { 2094 return this.url == null ? null : this.url.getValue(); 2095 } 2096 2097 /** 2098 * @param value WADO-RS resource where Study is available. 2099 */ 2100 public ImagingStudy setUrl(String value) { 2101 if (Utilities.noString(value)) 2102 this.url = null; 2103 else { 2104 if (this.url == null) 2105 this.url = new UriType(); 2106 this.url.setValue(value); 2107 } 2108 return this; 2109 } 2110 2111 /** 2112 * @return {@link #numberOfSeries} (Number of Series in Study.). This is the 2113 * underlying object with id, value and extensions. The accessor 2114 * "getNumberOfSeries" gives direct access to the value 2115 */ 2116 public UnsignedIntType getNumberOfSeriesElement() { 2117 if (this.numberOfSeries == null) 2118 if (Configuration.errorOnAutoCreate()) 2119 throw new Error("Attempt to auto-create ImagingStudy.numberOfSeries"); 2120 else if (Configuration.doAutoCreate()) 2121 this.numberOfSeries = new UnsignedIntType(); // bb 2122 return this.numberOfSeries; 2123 } 2124 2125 public boolean hasNumberOfSeriesElement() { 2126 return this.numberOfSeries != null && !this.numberOfSeries.isEmpty(); 2127 } 2128 2129 public boolean hasNumberOfSeries() { 2130 return this.numberOfSeries != null && !this.numberOfSeries.isEmpty(); 2131 } 2132 2133 /** 2134 * @param value {@link #numberOfSeries} (Number of Series in Study.). This is 2135 * the underlying object with id, value and extensions. The 2136 * accessor "getNumberOfSeries" gives direct access to the value 2137 */ 2138 public ImagingStudy setNumberOfSeriesElement(UnsignedIntType value) { 2139 this.numberOfSeries = value; 2140 return this; 2141 } 2142 2143 /** 2144 * @return Number of Series in Study. 2145 */ 2146 public int getNumberOfSeries() { 2147 return this.numberOfSeries == null || this.numberOfSeries.isEmpty() ? 0 : this.numberOfSeries.getValue(); 2148 } 2149 2150 /** 2151 * @param value Number of Series in Study. 2152 */ 2153 public ImagingStudy setNumberOfSeries(int value) { 2154 if (this.numberOfSeries == null) 2155 this.numberOfSeries = new UnsignedIntType(); 2156 this.numberOfSeries.setValue(value); 2157 return this; 2158 } 2159 2160 /** 2161 * @return {@link #numberOfInstances} (Number of SOP Instances in Study.). This 2162 * is the underlying object with id, value and extensions. The accessor 2163 * "getNumberOfInstances" gives direct access to the value 2164 */ 2165 public UnsignedIntType getNumberOfInstancesElement() { 2166 if (this.numberOfInstances == null) 2167 if (Configuration.errorOnAutoCreate()) 2168 throw new Error("Attempt to auto-create ImagingStudy.numberOfInstances"); 2169 else if (Configuration.doAutoCreate()) 2170 this.numberOfInstances = new UnsignedIntType(); // bb 2171 return this.numberOfInstances; 2172 } 2173 2174 public boolean hasNumberOfInstancesElement() { 2175 return this.numberOfInstances != null && !this.numberOfInstances.isEmpty(); 2176 } 2177 2178 public boolean hasNumberOfInstances() { 2179 return this.numberOfInstances != null && !this.numberOfInstances.isEmpty(); 2180 } 2181 2182 /** 2183 * @param value {@link #numberOfInstances} (Number of SOP Instances in Study.). 2184 * This is the underlying object with id, value and extensions. The 2185 * accessor "getNumberOfInstances" gives direct access to the value 2186 */ 2187 public ImagingStudy setNumberOfInstancesElement(UnsignedIntType value) { 2188 this.numberOfInstances = value; 2189 return this; 2190 } 2191 2192 /** 2193 * @return Number of SOP Instances in Study. 2194 */ 2195 public int getNumberOfInstances() { 2196 return this.numberOfInstances == null || this.numberOfInstances.isEmpty() ? 0 : this.numberOfInstances.getValue(); 2197 } 2198 2199 /** 2200 * @param value Number of SOP Instances in Study. 2201 */ 2202 public ImagingStudy setNumberOfInstances(int value) { 2203 if (this.numberOfInstances == null) 2204 this.numberOfInstances = new UnsignedIntType(); 2205 this.numberOfInstances.setValue(value); 2206 return this; 2207 } 2208 2209 /** 2210 * @return {@link #procedure} (Type of procedure performed.) 2211 */ 2212 public List<Reference> getProcedure() { 2213 if (this.procedure == null) 2214 this.procedure = new ArrayList<Reference>(); 2215 return this.procedure; 2216 } 2217 2218 public boolean hasProcedure() { 2219 if (this.procedure == null) 2220 return false; 2221 for (Reference item : this.procedure) 2222 if (!item.isEmpty()) 2223 return true; 2224 return false; 2225 } 2226 2227 /** 2228 * @return {@link #procedure} (Type of procedure performed.) 2229 */ 2230 // syntactic sugar 2231 public Reference addProcedure() { // 3 2232 Reference t = new Reference(); 2233 if (this.procedure == null) 2234 this.procedure = new ArrayList<Reference>(); 2235 this.procedure.add(t); 2236 return t; 2237 } 2238 2239 // syntactic sugar 2240 public ImagingStudy addProcedure(Reference t) { // 3 2241 if (t == null) 2242 return this; 2243 if (this.procedure == null) 2244 this.procedure = new ArrayList<Reference>(); 2245 this.procedure.add(t); 2246 return this; 2247 } 2248 2249 /** 2250 * @return {@link #procedure} (The actual objects that are the target of the 2251 * reference. The reference library doesn't populate this, but you can 2252 * use this to hold the resources if you resolvethemt. Type of procedure 2253 * performed.) 2254 */ 2255 public List<Procedure> getProcedureTarget() { 2256 if (this.procedureTarget == null) 2257 this.procedureTarget = new ArrayList<Procedure>(); 2258 return this.procedureTarget; 2259 } 2260 2261 // syntactic sugar 2262 /** 2263 * @return {@link #procedure} (Add an actual object that is the target of the 2264 * reference. The reference library doesn't use these, but you can use 2265 * this to hold the resources if you resolvethemt. Type of procedure 2266 * performed.) 2267 */ 2268 public Procedure addProcedureTarget() { 2269 Procedure r = new Procedure(); 2270 if (this.procedureTarget == null) 2271 this.procedureTarget = new ArrayList<Procedure>(); 2272 this.procedureTarget.add(r); 2273 return r; 2274 } 2275 2276 /** 2277 * @return {@link #interpreter} (Who read the study and interpreted the images 2278 * or other content.) 2279 */ 2280 public Reference getInterpreter() { 2281 if (this.interpreter == null) 2282 if (Configuration.errorOnAutoCreate()) 2283 throw new Error("Attempt to auto-create ImagingStudy.interpreter"); 2284 else if (Configuration.doAutoCreate()) 2285 this.interpreter = new Reference(); // cc 2286 return this.interpreter; 2287 } 2288 2289 public boolean hasInterpreter() { 2290 return this.interpreter != null && !this.interpreter.isEmpty(); 2291 } 2292 2293 /** 2294 * @param value {@link #interpreter} (Who read the study and interpreted the 2295 * images or other content.) 2296 */ 2297 public ImagingStudy setInterpreter(Reference value) { 2298 this.interpreter = value; 2299 return this; 2300 } 2301 2302 /** 2303 * @return {@link #interpreter} The actual object that is the target of the 2304 * reference. The reference library doesn't populate this, but you can 2305 * use it to hold the resource if you resolve it. (Who read the study 2306 * and interpreted the images or other content.) 2307 */ 2308 public Practitioner getInterpreterTarget() { 2309 if (this.interpreterTarget == null) 2310 if (Configuration.errorOnAutoCreate()) 2311 throw new Error("Attempt to auto-create ImagingStudy.interpreter"); 2312 else if (Configuration.doAutoCreate()) 2313 this.interpreterTarget = new Practitioner(); // aa 2314 return this.interpreterTarget; 2315 } 2316 2317 /** 2318 * @param value {@link #interpreter} The actual object that is the target of the 2319 * reference. The reference library doesn't use these, but you can 2320 * use it to hold the resource if you resolve it. (Who read the 2321 * study and interpreted the images or other content.) 2322 */ 2323 public ImagingStudy setInterpreterTarget(Practitioner value) { 2324 this.interpreterTarget = value; 2325 return this; 2326 } 2327 2328 /** 2329 * @return {@link #description} (Institution-generated description or 2330 * classification of the Study performed.). This is the underlying 2331 * object with id, value and extensions. The accessor "getDescription" 2332 * gives direct access to the value 2333 */ 2334 public StringType getDescriptionElement() { 2335 if (this.description == null) 2336 if (Configuration.errorOnAutoCreate()) 2337 throw new Error("Attempt to auto-create ImagingStudy.description"); 2338 else if (Configuration.doAutoCreate()) 2339 this.description = new StringType(); // bb 2340 return this.description; 2341 } 2342 2343 public boolean hasDescriptionElement() { 2344 return this.description != null && !this.description.isEmpty(); 2345 } 2346 2347 public boolean hasDescription() { 2348 return this.description != null && !this.description.isEmpty(); 2349 } 2350 2351 /** 2352 * @param value {@link #description} (Institution-generated description or 2353 * classification of the Study performed.). This is the underlying 2354 * object with id, value and extensions. The accessor 2355 * "getDescription" gives direct access to the value 2356 */ 2357 public ImagingStudy setDescriptionElement(StringType value) { 2358 this.description = value; 2359 return this; 2360 } 2361 2362 /** 2363 * @return Institution-generated description or classification of the Study 2364 * performed. 2365 */ 2366 public String getDescription() { 2367 return this.description == null ? null : this.description.getValue(); 2368 } 2369 2370 /** 2371 * @param value Institution-generated description or classification of the Study 2372 * performed. 2373 */ 2374 public ImagingStudy setDescription(String value) { 2375 if (Utilities.noString(value)) 2376 this.description = null; 2377 else { 2378 if (this.description == null) 2379 this.description = new StringType(); 2380 this.description.setValue(value); 2381 } 2382 return this; 2383 } 2384 2385 /** 2386 * @return {@link #series} (Each study has one or more series of images or other 2387 * content.) 2388 */ 2389 public List<ImagingStudySeriesComponent> getSeries() { 2390 if (this.series == null) 2391 this.series = new ArrayList<ImagingStudySeriesComponent>(); 2392 return this.series; 2393 } 2394 2395 public boolean hasSeries() { 2396 if (this.series == null) 2397 return false; 2398 for (ImagingStudySeriesComponent item : this.series) 2399 if (!item.isEmpty()) 2400 return true; 2401 return false; 2402 } 2403 2404 /** 2405 * @return {@link #series} (Each study has one or more series of images or other 2406 * content.) 2407 */ 2408 // syntactic sugar 2409 public ImagingStudySeriesComponent addSeries() { // 3 2410 ImagingStudySeriesComponent t = new ImagingStudySeriesComponent(); 2411 if (this.series == null) 2412 this.series = new ArrayList<ImagingStudySeriesComponent>(); 2413 this.series.add(t); 2414 return t; 2415 } 2416 2417 // syntactic sugar 2418 public ImagingStudy addSeries(ImagingStudySeriesComponent t) { // 3 2419 if (t == null) 2420 return this; 2421 if (this.series == null) 2422 this.series = new ArrayList<ImagingStudySeriesComponent>(); 2423 this.series.add(t); 2424 return this; 2425 } 2426 2427 protected void listChildren(List<Property> childrenList) { 2428 super.listChildren(childrenList); 2429 childrenList.add(new Property("started", "dateTime", "Date and Time the study started.", 0, 2430 java.lang.Integer.MAX_VALUE, started)); 2431 childrenList.add(new Property("patient", "Reference(Patient)", "The patient imaged in the study.", 0, 2432 java.lang.Integer.MAX_VALUE, patient)); 2433 childrenList 2434 .add(new Property("uid", "oid", "Formal identifier for the study.", 0, java.lang.Integer.MAX_VALUE, uid)); 2435 childrenList.add(new Property("accession", "Identifier", 2436 "Accession Number is an identifier related to some aspect of imaging workflow and data management. Usage may vary across different institutions. See for instance [IHE Radiology Technical Framework Volume 1 Appendix A](http://www.ihe.net/uploadedFiles/Documents/Radiology/IHE_RAD_TF_Rev13.0_Vol1_FT_2014-07-30.pdf).", 2437 0, java.lang.Integer.MAX_VALUE, accession)); 2438 childrenList.add(new Property("identifier", "Identifier", "Other identifiers for the study.", 0, 2439 java.lang.Integer.MAX_VALUE, identifier)); 2440 childrenList.add(new Property("order", "Reference(DiagnosticOrder)", 2441 "A list of the diagnostic orders that resulted in this imaging study being performed.", 0, 2442 java.lang.Integer.MAX_VALUE, order)); 2443 childrenList.add(new Property("modalityList", "Coding", 2444 "A list of all the Series.ImageModality values that are actual acquisition modalities, i.e. those in the DICOM Context Group 29 (value set OID 1.2.840.10008.6.1.19).", 2445 0, java.lang.Integer.MAX_VALUE, modalityList)); 2446 childrenList.add(new Property("referrer", "Reference(Practitioner)", "The requesting/referring physician.", 0, 2447 java.lang.Integer.MAX_VALUE, referrer)); 2448 childrenList.add(new Property("availability", "code", "Availability of study (online, offline or nearline).", 0, 2449 java.lang.Integer.MAX_VALUE, availability)); 2450 childrenList.add( 2451 new Property("url", "uri", "WADO-RS resource where Study is available.", 0, java.lang.Integer.MAX_VALUE, url)); 2452 childrenList.add(new Property("numberOfSeries", "unsignedInt", "Number of Series in Study.", 0, 2453 java.lang.Integer.MAX_VALUE, numberOfSeries)); 2454 childrenList.add(new Property("numberOfInstances", "unsignedInt", "Number of SOP Instances in Study.", 0, 2455 java.lang.Integer.MAX_VALUE, numberOfInstances)); 2456 childrenList.add(new Property("procedure", "Reference(Procedure)", "Type of procedure performed.", 0, 2457 java.lang.Integer.MAX_VALUE, procedure)); 2458 childrenList.add(new Property("interpreter", "Reference(Practitioner)", 2459 "Who read the study and interpreted the images or other content.", 0, java.lang.Integer.MAX_VALUE, 2460 interpreter)); 2461 childrenList.add(new Property("description", "string", 2462 "Institution-generated description or classification of the Study performed.", 0, java.lang.Integer.MAX_VALUE, 2463 description)); 2464 childrenList.add(new Property("series", "", "Each study has one or more series of images or other content.", 0, 2465 java.lang.Integer.MAX_VALUE, series)); 2466 } 2467 2468 @Override 2469 public void setProperty(String name, Base value) throws FHIRException { 2470 if (name.equals("started")) 2471 this.started = castToDateTime(value); // DateTimeType 2472 else if (name.equals("patient")) 2473 this.patient = castToReference(value); // Reference 2474 else if (name.equals("uid")) 2475 this.uid = castToOid(value); // OidType 2476 else if (name.equals("accession")) 2477 this.accession = castToIdentifier(value); // Identifier 2478 else if (name.equals("identifier")) 2479 this.getIdentifier().add(castToIdentifier(value)); 2480 else if (name.equals("order")) 2481 this.getOrder().add(castToReference(value)); 2482 else if (name.equals("modalityList")) 2483 this.getModalityList().add(castToCoding(value)); 2484 else if (name.equals("referrer")) 2485 this.referrer = castToReference(value); // Reference 2486 else if (name.equals("availability")) 2487 this.availability = new InstanceAvailabilityEnumFactory().fromType(value); // Enumeration<InstanceAvailability> 2488 else if (name.equals("url")) 2489 this.url = castToUri(value); // UriType 2490 else if (name.equals("numberOfSeries")) 2491 this.numberOfSeries = castToUnsignedInt(value); // UnsignedIntType 2492 else if (name.equals("numberOfInstances")) 2493 this.numberOfInstances = castToUnsignedInt(value); // UnsignedIntType 2494 else if (name.equals("procedure")) 2495 this.getProcedure().add(castToReference(value)); 2496 else if (name.equals("interpreter")) 2497 this.interpreter = castToReference(value); // Reference 2498 else if (name.equals("description")) 2499 this.description = castToString(value); // StringType 2500 else if (name.equals("series")) 2501 this.getSeries().add((ImagingStudySeriesComponent) value); 2502 else 2503 super.setProperty(name, value); 2504 } 2505 2506 @Override 2507 public Base addChild(String name) throws FHIRException { 2508 if (name.equals("started")) { 2509 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.started"); 2510 } else if (name.equals("patient")) { 2511 this.patient = new Reference(); 2512 return this.patient; 2513 } else if (name.equals("uid")) { 2514 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.uid"); 2515 } else if (name.equals("accession")) { 2516 this.accession = new Identifier(); 2517 return this.accession; 2518 } else if (name.equals("identifier")) { 2519 return addIdentifier(); 2520 } else if (name.equals("order")) { 2521 return addOrder(); 2522 } else if (name.equals("modalityList")) { 2523 return addModalityList(); 2524 } else if (name.equals("referrer")) { 2525 this.referrer = new Reference(); 2526 return this.referrer; 2527 } else if (name.equals("availability")) { 2528 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.availability"); 2529 } else if (name.equals("url")) { 2530 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.url"); 2531 } else if (name.equals("numberOfSeries")) { 2532 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.numberOfSeries"); 2533 } else if (name.equals("numberOfInstances")) { 2534 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.numberOfInstances"); 2535 } else if (name.equals("procedure")) { 2536 return addProcedure(); 2537 } else if (name.equals("interpreter")) { 2538 this.interpreter = new Reference(); 2539 return this.interpreter; 2540 } else if (name.equals("description")) { 2541 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.description"); 2542 } else if (name.equals("series")) { 2543 return addSeries(); 2544 } else 2545 return super.addChild(name); 2546 } 2547 2548 public String fhirType() { 2549 return "ImagingStudy"; 2550 2551 } 2552 2553 public ImagingStudy copy() { 2554 ImagingStudy dst = new ImagingStudy(); 2555 copyValues(dst); 2556 dst.started = started == null ? null : started.copy(); 2557 dst.patient = patient == null ? null : patient.copy(); 2558 dst.uid = uid == null ? null : uid.copy(); 2559 dst.accession = accession == null ? null : accession.copy(); 2560 if (identifier != null) { 2561 dst.identifier = new ArrayList<Identifier>(); 2562 for (Identifier i : identifier) 2563 dst.identifier.add(i.copy()); 2564 } 2565 ; 2566 if (order != null) { 2567 dst.order = new ArrayList<Reference>(); 2568 for (Reference i : order) 2569 dst.order.add(i.copy()); 2570 } 2571 ; 2572 if (modalityList != null) { 2573 dst.modalityList = new ArrayList<Coding>(); 2574 for (Coding i : modalityList) 2575 dst.modalityList.add(i.copy()); 2576 } 2577 ; 2578 dst.referrer = referrer == null ? null : referrer.copy(); 2579 dst.availability = availability == null ? null : availability.copy(); 2580 dst.url = url == null ? null : url.copy(); 2581 dst.numberOfSeries = numberOfSeries == null ? null : numberOfSeries.copy(); 2582 dst.numberOfInstances = numberOfInstances == null ? null : numberOfInstances.copy(); 2583 if (procedure != null) { 2584 dst.procedure = new ArrayList<Reference>(); 2585 for (Reference i : procedure) 2586 dst.procedure.add(i.copy()); 2587 } 2588 ; 2589 dst.interpreter = interpreter == null ? null : interpreter.copy(); 2590 dst.description = description == null ? null : description.copy(); 2591 if (series != null) { 2592 dst.series = new ArrayList<ImagingStudySeriesComponent>(); 2593 for (ImagingStudySeriesComponent i : series) 2594 dst.series.add(i.copy()); 2595 } 2596 ; 2597 return dst; 2598 } 2599 2600 protected ImagingStudy typedCopy() { 2601 return copy(); 2602 } 2603 2604 @Override 2605 public boolean equalsDeep(Base other) { 2606 if (!super.equalsDeep(other)) 2607 return false; 2608 if (!(other instanceof ImagingStudy)) 2609 return false; 2610 ImagingStudy o = (ImagingStudy) other; 2611 return compareDeep(started, o.started, true) && compareDeep(patient, o.patient, true) 2612 && compareDeep(uid, o.uid, true) && compareDeep(accession, o.accession, true) 2613 && compareDeep(identifier, o.identifier, true) && compareDeep(order, o.order, true) 2614 && compareDeep(modalityList, o.modalityList, true) && compareDeep(referrer, o.referrer, true) 2615 && compareDeep(availability, o.availability, true) && compareDeep(url, o.url, true) 2616 && compareDeep(numberOfSeries, o.numberOfSeries, true) 2617 && compareDeep(numberOfInstances, o.numberOfInstances, true) && compareDeep(procedure, o.procedure, true) 2618 && compareDeep(interpreter, o.interpreter, true) && compareDeep(description, o.description, true) 2619 && compareDeep(series, o.series, true); 2620 } 2621 2622 @Override 2623 public boolean equalsShallow(Base other) { 2624 if (!super.equalsShallow(other)) 2625 return false; 2626 if (!(other instanceof ImagingStudy)) 2627 return false; 2628 ImagingStudy o = (ImagingStudy) other; 2629 return compareValues(started, o.started, true) && compareValues(uid, o.uid, true) 2630 && compareValues(availability, o.availability, true) && compareValues(url, o.url, true) 2631 && compareValues(numberOfSeries, o.numberOfSeries, true) 2632 && compareValues(numberOfInstances, o.numberOfInstances, true) 2633 && compareValues(description, o.description, true); 2634 } 2635 2636 public boolean isEmpty() { 2637 return super.isEmpty() && (started == null || started.isEmpty()) && (patient == null || patient.isEmpty()) 2638 && (uid == null || uid.isEmpty()) && (accession == null || accession.isEmpty()) 2639 && (identifier == null || identifier.isEmpty()) && (order == null || order.isEmpty()) 2640 && (modalityList == null || modalityList.isEmpty()) && (referrer == null || referrer.isEmpty()) 2641 && (availability == null || availability.isEmpty()) && (url == null || url.isEmpty()) 2642 && (numberOfSeries == null || numberOfSeries.isEmpty()) 2643 && (numberOfInstances == null || numberOfInstances.isEmpty()) && (procedure == null || procedure.isEmpty()) 2644 && (interpreter == null || interpreter.isEmpty()) && (description == null || description.isEmpty()) 2645 && (series == null || series.isEmpty()); 2646 } 2647 2648 @Override 2649 public ResourceType getResourceType() { 2650 return ResourceType.ImagingStudy; 2651 } 2652 2653 @SearchParamDefinition(name = "uid", path = "ImagingStudy.series.instance.uid", description = "The instance unique identifier", type = "uri") 2654 public static final String SP_UID = "uid"; 2655 @SearchParamDefinition(name = "study", path = "ImagingStudy.uid", description = "The study identifier for the image", type = "uri") 2656 public static final String SP_STUDY = "study"; 2657 @SearchParamDefinition(name = "dicom-class", path = "ImagingStudy.series.instance.sopClass", description = "The type of the instance", type = "uri") 2658 public static final String SP_DICOMCLASS = "dicom-class"; 2659 @SearchParamDefinition(name = "modality", path = "ImagingStudy.series.modality", description = "The modality of the series", type = "token") 2660 public static final String SP_MODALITY = "modality"; 2661 @SearchParamDefinition(name = "bodysite", path = "ImagingStudy.series.bodySite", description = "The body site studied", type = "token") 2662 public static final String SP_BODYSITE = "bodysite"; 2663 @SearchParamDefinition(name = "patient", path = "ImagingStudy.patient", description = "Who the study is about", type = "reference") 2664 public static final String SP_PATIENT = "patient"; 2665 @SearchParamDefinition(name = "series", path = "ImagingStudy.series.uid", description = "The identifier of the series of images", type = "uri") 2666 public static final String SP_SERIES = "series"; 2667 @SearchParamDefinition(name = "started", path = "ImagingStudy.started", description = "When the study was started", type = "date") 2668 public static final String SP_STARTED = "started"; 2669 @SearchParamDefinition(name = "accession", path = "ImagingStudy.accession", description = "The accession identifier for the study", type = "token") 2670 public static final String SP_ACCESSION = "accession"; 2671 @SearchParamDefinition(name = "order", path = "ImagingStudy.order", description = "The order for the image", type = "reference") 2672 public static final String SP_ORDER = "order"; 2673 2674}