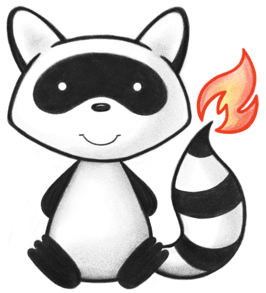
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * Describes the event of a patient being administered a vaccination or a record 048 * of a vaccination as reported by a patient, a clinician or another party and 049 * may include vaccine reaction information and what vaccination protocol was 050 * followed. 051 */ 052@ResourceDef(name = "Immunization", profile = "http://hl7.org/fhir/Profile/Immunization") 053public class Immunization extends DomainResource { 054 055 @Block() 056 public static class ImmunizationExplanationComponent extends BackboneElement implements IBaseBackboneElement { 057 /** 058 * Reasons why a vaccine was administered. 059 */ 060 @Child(name = "reason", type = { 061 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 062 @Description(shortDefinition = "Why immunization occurred", formalDefinition = "Reasons why a vaccine was administered.") 063 protected List<CodeableConcept> reason; 064 065 /** 066 * Reason why a vaccine was not administered. 067 */ 068 @Child(name = "reasonNotGiven", type = { 069 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 070 @Description(shortDefinition = "Why immunization did not occur", formalDefinition = "Reason why a vaccine was not administered.") 071 protected List<CodeableConcept> reasonNotGiven; 072 073 private static final long serialVersionUID = -539821866L; 074 075 /* 076 * Constructor 077 */ 078 public ImmunizationExplanationComponent() { 079 super(); 080 } 081 082 /** 083 * @return {@link #reason} (Reasons why a vaccine was administered.) 084 */ 085 public List<CodeableConcept> getReason() { 086 if (this.reason == null) 087 this.reason = new ArrayList<CodeableConcept>(); 088 return this.reason; 089 } 090 091 public boolean hasReason() { 092 if (this.reason == null) 093 return false; 094 for (CodeableConcept item : this.reason) 095 if (!item.isEmpty()) 096 return true; 097 return false; 098 } 099 100 /** 101 * @return {@link #reason} (Reasons why a vaccine was administered.) 102 */ 103 // syntactic sugar 104 public CodeableConcept addReason() { // 3 105 CodeableConcept t = new CodeableConcept(); 106 if (this.reason == null) 107 this.reason = new ArrayList<CodeableConcept>(); 108 this.reason.add(t); 109 return t; 110 } 111 112 // syntactic sugar 113 public ImmunizationExplanationComponent addReason(CodeableConcept t) { // 3 114 if (t == null) 115 return this; 116 if (this.reason == null) 117 this.reason = new ArrayList<CodeableConcept>(); 118 this.reason.add(t); 119 return this; 120 } 121 122 /** 123 * @return {@link #reasonNotGiven} (Reason why a vaccine was not administered.) 124 */ 125 public List<CodeableConcept> getReasonNotGiven() { 126 if (this.reasonNotGiven == null) 127 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 128 return this.reasonNotGiven; 129 } 130 131 public boolean hasReasonNotGiven() { 132 if (this.reasonNotGiven == null) 133 return false; 134 for (CodeableConcept item : this.reasonNotGiven) 135 if (!item.isEmpty()) 136 return true; 137 return false; 138 } 139 140 /** 141 * @return {@link #reasonNotGiven} (Reason why a vaccine was not administered.) 142 */ 143 // syntactic sugar 144 public CodeableConcept addReasonNotGiven() { // 3 145 CodeableConcept t = new CodeableConcept(); 146 if (this.reasonNotGiven == null) 147 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 148 this.reasonNotGiven.add(t); 149 return t; 150 } 151 152 // syntactic sugar 153 public ImmunizationExplanationComponent addReasonNotGiven(CodeableConcept t) { // 3 154 if (t == null) 155 return this; 156 if (this.reasonNotGiven == null) 157 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 158 this.reasonNotGiven.add(t); 159 return this; 160 } 161 162 protected void listChildren(List<Property> childrenList) { 163 super.listChildren(childrenList); 164 childrenList.add(new Property("reason", "CodeableConcept", "Reasons why a vaccine was administered.", 0, 165 java.lang.Integer.MAX_VALUE, reason)); 166 childrenList.add(new Property("reasonNotGiven", "CodeableConcept", "Reason why a vaccine was not administered.", 167 0, java.lang.Integer.MAX_VALUE, reasonNotGiven)); 168 } 169 170 @Override 171 public void setProperty(String name, Base value) throws FHIRException { 172 if (name.equals("reason")) 173 this.getReason().add(castToCodeableConcept(value)); 174 else if (name.equals("reasonNotGiven")) 175 this.getReasonNotGiven().add(castToCodeableConcept(value)); 176 else 177 super.setProperty(name, value); 178 } 179 180 @Override 181 public Base addChild(String name) throws FHIRException { 182 if (name.equals("reason")) { 183 return addReason(); 184 } else if (name.equals("reasonNotGiven")) { 185 return addReasonNotGiven(); 186 } else 187 return super.addChild(name); 188 } 189 190 public ImmunizationExplanationComponent copy() { 191 ImmunizationExplanationComponent dst = new ImmunizationExplanationComponent(); 192 copyValues(dst); 193 if (reason != null) { 194 dst.reason = new ArrayList<CodeableConcept>(); 195 for (CodeableConcept i : reason) 196 dst.reason.add(i.copy()); 197 } 198 ; 199 if (reasonNotGiven != null) { 200 dst.reasonNotGiven = new ArrayList<CodeableConcept>(); 201 for (CodeableConcept i : reasonNotGiven) 202 dst.reasonNotGiven.add(i.copy()); 203 } 204 ; 205 return dst; 206 } 207 208 @Override 209 public boolean equalsDeep(Base other) { 210 if (!super.equalsDeep(other)) 211 return false; 212 if (!(other instanceof ImmunizationExplanationComponent)) 213 return false; 214 ImmunizationExplanationComponent o = (ImmunizationExplanationComponent) other; 215 return compareDeep(reason, o.reason, true) && compareDeep(reasonNotGiven, o.reasonNotGiven, true); 216 } 217 218 @Override 219 public boolean equalsShallow(Base other) { 220 if (!super.equalsShallow(other)) 221 return false; 222 if (!(other instanceof ImmunizationExplanationComponent)) 223 return false; 224 ImmunizationExplanationComponent o = (ImmunizationExplanationComponent) other; 225 return true; 226 } 227 228 public boolean isEmpty() { 229 return super.isEmpty() && (reason == null || reason.isEmpty()) 230 && (reasonNotGiven == null || reasonNotGiven.isEmpty()); 231 } 232 233 public String fhirType() { 234 return "Immunization.explanation"; 235 236 } 237 238 } 239 240 @Block() 241 public static class ImmunizationReactionComponent extends BackboneElement implements IBaseBackboneElement { 242 /** 243 * Date of reaction to the immunization. 244 */ 245 @Child(name = "date", type = { DateTimeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 246 @Description(shortDefinition = "When reaction started", formalDefinition = "Date of reaction to the immunization.") 247 protected DateTimeType date; 248 249 /** 250 * Details of the reaction. 251 */ 252 @Child(name = "detail", type = { 253 Observation.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 254 @Description(shortDefinition = "Additional information on reaction", formalDefinition = "Details of the reaction.") 255 protected Reference detail; 256 257 /** 258 * The actual object that is the target of the reference (Details of the 259 * reaction.) 260 */ 261 protected Observation detailTarget; 262 263 /** 264 * Self-reported indicator. 265 */ 266 @Child(name = "reported", type = { 267 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 268 @Description(shortDefinition = "Indicates self-reported reaction", formalDefinition = "Self-reported indicator.") 269 protected BooleanType reported; 270 271 private static final long serialVersionUID = -1297668556L; 272 273 /* 274 * Constructor 275 */ 276 public ImmunizationReactionComponent() { 277 super(); 278 } 279 280 /** 281 * @return {@link #date} (Date of reaction to the immunization.). This is the 282 * underlying object with id, value and extensions. The accessor 283 * "getDate" gives direct access to the value 284 */ 285 public DateTimeType getDateElement() { 286 if (this.date == null) 287 if (Configuration.errorOnAutoCreate()) 288 throw new Error("Attempt to auto-create ImmunizationReactionComponent.date"); 289 else if (Configuration.doAutoCreate()) 290 this.date = new DateTimeType(); // bb 291 return this.date; 292 } 293 294 public boolean hasDateElement() { 295 return this.date != null && !this.date.isEmpty(); 296 } 297 298 public boolean hasDate() { 299 return this.date != null && !this.date.isEmpty(); 300 } 301 302 /** 303 * @param value {@link #date} (Date of reaction to the immunization.). This is 304 * the underlying object with id, value and extensions. The 305 * accessor "getDate" gives direct access to the value 306 */ 307 public ImmunizationReactionComponent setDateElement(DateTimeType value) { 308 this.date = value; 309 return this; 310 } 311 312 /** 313 * @return Date of reaction to the immunization. 314 */ 315 public Date getDate() { 316 return this.date == null ? null : this.date.getValue(); 317 } 318 319 /** 320 * @param value Date of reaction to the immunization. 321 */ 322 public ImmunizationReactionComponent setDate(Date value) { 323 if (value == null) 324 this.date = null; 325 else { 326 if (this.date == null) 327 this.date = new DateTimeType(); 328 this.date.setValue(value); 329 } 330 return this; 331 } 332 333 /** 334 * @return {@link #detail} (Details of the reaction.) 335 */ 336 public Reference getDetail() { 337 if (this.detail == null) 338 if (Configuration.errorOnAutoCreate()) 339 throw new Error("Attempt to auto-create ImmunizationReactionComponent.detail"); 340 else if (Configuration.doAutoCreate()) 341 this.detail = new Reference(); // cc 342 return this.detail; 343 } 344 345 public boolean hasDetail() { 346 return this.detail != null && !this.detail.isEmpty(); 347 } 348 349 /** 350 * @param value {@link #detail} (Details of the reaction.) 351 */ 352 public ImmunizationReactionComponent setDetail(Reference value) { 353 this.detail = value; 354 return this; 355 } 356 357 /** 358 * @return {@link #detail} The actual object that is the target of the 359 * reference. The reference library doesn't populate this, but you can 360 * use it to hold the resource if you resolve it. (Details of the 361 * reaction.) 362 */ 363 public Observation getDetailTarget() { 364 if (this.detailTarget == null) 365 if (Configuration.errorOnAutoCreate()) 366 throw new Error("Attempt to auto-create ImmunizationReactionComponent.detail"); 367 else if (Configuration.doAutoCreate()) 368 this.detailTarget = new Observation(); // aa 369 return this.detailTarget; 370 } 371 372 /** 373 * @param value {@link #detail} The actual object that is the target of the 374 * reference. The reference library doesn't use these, but you can 375 * use it to hold the resource if you resolve it. (Details of the 376 * reaction.) 377 */ 378 public ImmunizationReactionComponent setDetailTarget(Observation value) { 379 this.detailTarget = value; 380 return this; 381 } 382 383 /** 384 * @return {@link #reported} (Self-reported indicator.). This is the underlying 385 * object with id, value and extensions. The accessor "getReported" 386 * gives direct access to the value 387 */ 388 public BooleanType getReportedElement() { 389 if (this.reported == null) 390 if (Configuration.errorOnAutoCreate()) 391 throw new Error("Attempt to auto-create ImmunizationReactionComponent.reported"); 392 else if (Configuration.doAutoCreate()) 393 this.reported = new BooleanType(); // bb 394 return this.reported; 395 } 396 397 public boolean hasReportedElement() { 398 return this.reported != null && !this.reported.isEmpty(); 399 } 400 401 public boolean hasReported() { 402 return this.reported != null && !this.reported.isEmpty(); 403 } 404 405 /** 406 * @param value {@link #reported} (Self-reported indicator.). This is the 407 * underlying object with id, value and extensions. The accessor 408 * "getReported" gives direct access to the value 409 */ 410 public ImmunizationReactionComponent setReportedElement(BooleanType value) { 411 this.reported = value; 412 return this; 413 } 414 415 /** 416 * @return Self-reported indicator. 417 */ 418 public boolean getReported() { 419 return this.reported == null || this.reported.isEmpty() ? false : this.reported.getValue(); 420 } 421 422 /** 423 * @param value Self-reported indicator. 424 */ 425 public ImmunizationReactionComponent setReported(boolean value) { 426 if (this.reported == null) 427 this.reported = new BooleanType(); 428 this.reported.setValue(value); 429 return this; 430 } 431 432 protected void listChildren(List<Property> childrenList) { 433 super.listChildren(childrenList); 434 childrenList.add(new Property("date", "dateTime", "Date of reaction to the immunization.", 0, 435 java.lang.Integer.MAX_VALUE, date)); 436 childrenList.add(new Property("detail", "Reference(Observation)", "Details of the reaction.", 0, 437 java.lang.Integer.MAX_VALUE, detail)); 438 childrenList.add( 439 new Property("reported", "boolean", "Self-reported indicator.", 0, java.lang.Integer.MAX_VALUE, reported)); 440 } 441 442 @Override 443 public void setProperty(String name, Base value) throws FHIRException { 444 if (name.equals("date")) 445 this.date = castToDateTime(value); // DateTimeType 446 else if (name.equals("detail")) 447 this.detail = castToReference(value); // Reference 448 else if (name.equals("reported")) 449 this.reported = castToBoolean(value); // BooleanType 450 else 451 super.setProperty(name, value); 452 } 453 454 @Override 455 public Base addChild(String name) throws FHIRException { 456 if (name.equals("date")) { 457 throw new FHIRException("Cannot call addChild on a singleton property Immunization.date"); 458 } else if (name.equals("detail")) { 459 this.detail = new Reference(); 460 return this.detail; 461 } else if (name.equals("reported")) { 462 throw new FHIRException("Cannot call addChild on a singleton property Immunization.reported"); 463 } else 464 return super.addChild(name); 465 } 466 467 public ImmunizationReactionComponent copy() { 468 ImmunizationReactionComponent dst = new ImmunizationReactionComponent(); 469 copyValues(dst); 470 dst.date = date == null ? null : date.copy(); 471 dst.detail = detail == null ? null : detail.copy(); 472 dst.reported = reported == null ? null : reported.copy(); 473 return dst; 474 } 475 476 @Override 477 public boolean equalsDeep(Base other) { 478 if (!super.equalsDeep(other)) 479 return false; 480 if (!(other instanceof ImmunizationReactionComponent)) 481 return false; 482 ImmunizationReactionComponent o = (ImmunizationReactionComponent) other; 483 return compareDeep(date, o.date, true) && compareDeep(detail, o.detail, true) 484 && compareDeep(reported, o.reported, true); 485 } 486 487 @Override 488 public boolean equalsShallow(Base other) { 489 if (!super.equalsShallow(other)) 490 return false; 491 if (!(other instanceof ImmunizationReactionComponent)) 492 return false; 493 ImmunizationReactionComponent o = (ImmunizationReactionComponent) other; 494 return compareValues(date, o.date, true) && compareValues(reported, o.reported, true); 495 } 496 497 public boolean isEmpty() { 498 return super.isEmpty() && (date == null || date.isEmpty()) && (detail == null || detail.isEmpty()) 499 && (reported == null || reported.isEmpty()); 500 } 501 502 public String fhirType() { 503 return "Immunization.reaction"; 504 505 } 506 507 } 508 509 @Block() 510 public static class ImmunizationVaccinationProtocolComponent extends BackboneElement implements IBaseBackboneElement { 511 /** 512 * Nominal position in a series. 513 */ 514 @Child(name = "doseSequence", type = { 515 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 516 @Description(shortDefinition = "Dose number within series", formalDefinition = "Nominal position in a series.") 517 protected PositiveIntType doseSequence; 518 519 /** 520 * Contains the description about the protocol under which the vaccine was 521 * administered. 522 */ 523 @Child(name = "description", type = { 524 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 525 @Description(shortDefinition = "Details of vaccine protocol", formalDefinition = "Contains the description about the protocol under which the vaccine was administered.") 526 protected StringType description; 527 528 /** 529 * Indicates the authority who published the protocol. E.g. ACIP. 530 */ 531 @Child(name = "authority", type = { 532 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 533 @Description(shortDefinition = "Who is responsible for protocol", formalDefinition = "Indicates the authority who published the protocol. E.g. ACIP.") 534 protected Reference authority; 535 536 /** 537 * The actual object that is the target of the reference (Indicates the 538 * authority who published the protocol. E.g. ACIP.) 539 */ 540 protected Organization authorityTarget; 541 542 /** 543 * One possible path to achieve presumed immunity against a disease - within the 544 * context of an authority. 545 */ 546 @Child(name = "series", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 547 @Description(shortDefinition = "Name of vaccine series", formalDefinition = "One possible path to achieve presumed immunity against a disease - within the context of an authority.") 548 protected StringType series; 549 550 /** 551 * The recommended number of doses to achieve immunity. 552 */ 553 @Child(name = "seriesDoses", type = { 554 PositiveIntType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 555 @Description(shortDefinition = "Recommended number of doses for immunity", formalDefinition = "The recommended number of doses to achieve immunity.") 556 protected PositiveIntType seriesDoses; 557 558 /** 559 * The targeted disease. 560 */ 561 @Child(name = "targetDisease", type = { 562 CodeableConcept.class }, order = 6, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 563 @Description(shortDefinition = "Disease immunized against", formalDefinition = "The targeted disease.") 564 protected List<CodeableConcept> targetDisease; 565 566 /** 567 * Indicates if the immunization event should "count" against the protocol. 568 */ 569 @Child(name = "doseStatus", type = { 570 CodeableConcept.class }, order = 7, min = 1, max = 1, modifier = false, summary = false) 571 @Description(shortDefinition = "Indicates if dose counts towards immunity", formalDefinition = "Indicates if the immunization event should \"count\" against the protocol.") 572 protected CodeableConcept doseStatus; 573 574 /** 575 * Provides an explanation as to why an immunization event should or should not 576 * count against the protocol. 577 */ 578 @Child(name = "doseStatusReason", type = { 579 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 580 @Description(shortDefinition = "Why dose does (not) count", formalDefinition = "Provides an explanation as to why an immunization event should or should not count against the protocol.") 581 protected CodeableConcept doseStatusReason; 582 583 private static final long serialVersionUID = 386814037L; 584 585 /* 586 * Constructor 587 */ 588 public ImmunizationVaccinationProtocolComponent() { 589 super(); 590 } 591 592 /* 593 * Constructor 594 */ 595 public ImmunizationVaccinationProtocolComponent(PositiveIntType doseSequence, CodeableConcept doseStatus) { 596 super(); 597 this.doseSequence = doseSequence; 598 this.doseStatus = doseStatus; 599 } 600 601 /** 602 * @return {@link #doseSequence} (Nominal position in a series.). This is the 603 * underlying object with id, value and extensions. The accessor 604 * "getDoseSequence" gives direct access to the value 605 */ 606 public PositiveIntType getDoseSequenceElement() { 607 if (this.doseSequence == null) 608 if (Configuration.errorOnAutoCreate()) 609 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.doseSequence"); 610 else if (Configuration.doAutoCreate()) 611 this.doseSequence = new PositiveIntType(); // bb 612 return this.doseSequence; 613 } 614 615 public boolean hasDoseSequenceElement() { 616 return this.doseSequence != null && !this.doseSequence.isEmpty(); 617 } 618 619 public boolean hasDoseSequence() { 620 return this.doseSequence != null && !this.doseSequence.isEmpty(); 621 } 622 623 /** 624 * @param value {@link #doseSequence} (Nominal position in a series.). This is 625 * the underlying object with id, value and extensions. The 626 * accessor "getDoseSequence" gives direct access to the value 627 */ 628 public ImmunizationVaccinationProtocolComponent setDoseSequenceElement(PositiveIntType value) { 629 this.doseSequence = value; 630 return this; 631 } 632 633 /** 634 * @return Nominal position in a series. 635 */ 636 public int getDoseSequence() { 637 return this.doseSequence == null || this.doseSequence.isEmpty() ? 0 : this.doseSequence.getValue(); 638 } 639 640 /** 641 * @param value Nominal position in a series. 642 */ 643 public ImmunizationVaccinationProtocolComponent setDoseSequence(int value) { 644 if (this.doseSequence == null) 645 this.doseSequence = new PositiveIntType(); 646 this.doseSequence.setValue(value); 647 return this; 648 } 649 650 /** 651 * @return {@link #description} (Contains the description about the protocol 652 * under which the vaccine was administered.). This is the underlying 653 * object with id, value and extensions. The accessor "getDescription" 654 * gives direct access to the value 655 */ 656 public StringType getDescriptionElement() { 657 if (this.description == null) 658 if (Configuration.errorOnAutoCreate()) 659 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.description"); 660 else if (Configuration.doAutoCreate()) 661 this.description = new StringType(); // bb 662 return this.description; 663 } 664 665 public boolean hasDescriptionElement() { 666 return this.description != null && !this.description.isEmpty(); 667 } 668 669 public boolean hasDescription() { 670 return this.description != null && !this.description.isEmpty(); 671 } 672 673 /** 674 * @param value {@link #description} (Contains the description about the 675 * protocol under which the vaccine was administered.). This is the 676 * underlying object with id, value and extensions. The accessor 677 * "getDescription" gives direct access to the value 678 */ 679 public ImmunizationVaccinationProtocolComponent setDescriptionElement(StringType value) { 680 this.description = value; 681 return this; 682 } 683 684 /** 685 * @return Contains the description about the protocol under which the vaccine 686 * was administered. 687 */ 688 public String getDescription() { 689 return this.description == null ? null : this.description.getValue(); 690 } 691 692 /** 693 * @param value Contains the description about the protocol under which the 694 * vaccine was administered. 695 */ 696 public ImmunizationVaccinationProtocolComponent setDescription(String value) { 697 if (Utilities.noString(value)) 698 this.description = null; 699 else { 700 if (this.description == null) 701 this.description = new StringType(); 702 this.description.setValue(value); 703 } 704 return this; 705 } 706 707 /** 708 * @return {@link #authority} (Indicates the authority who published the 709 * protocol. E.g. ACIP.) 710 */ 711 public Reference getAuthority() { 712 if (this.authority == null) 713 if (Configuration.errorOnAutoCreate()) 714 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.authority"); 715 else if (Configuration.doAutoCreate()) 716 this.authority = new Reference(); // cc 717 return this.authority; 718 } 719 720 public boolean hasAuthority() { 721 return this.authority != null && !this.authority.isEmpty(); 722 } 723 724 /** 725 * @param value {@link #authority} (Indicates the authority who published the 726 * protocol. E.g. ACIP.) 727 */ 728 public ImmunizationVaccinationProtocolComponent setAuthority(Reference value) { 729 this.authority = value; 730 return this; 731 } 732 733 /** 734 * @return {@link #authority} The actual object that is the target of the 735 * reference. The reference library doesn't populate this, but you can 736 * use it to hold the resource if you resolve it. (Indicates the 737 * authority who published the protocol. E.g. ACIP.) 738 */ 739 public Organization getAuthorityTarget() { 740 if (this.authorityTarget == null) 741 if (Configuration.errorOnAutoCreate()) 742 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.authority"); 743 else if (Configuration.doAutoCreate()) 744 this.authorityTarget = new Organization(); // aa 745 return this.authorityTarget; 746 } 747 748 /** 749 * @param value {@link #authority} The actual object that is the target of the 750 * reference. The reference library doesn't use these, but you can 751 * use it to hold the resource if you resolve it. (Indicates the 752 * authority who published the protocol. E.g. ACIP.) 753 */ 754 public ImmunizationVaccinationProtocolComponent setAuthorityTarget(Organization value) { 755 this.authorityTarget = value; 756 return this; 757 } 758 759 /** 760 * @return {@link #series} (One possible path to achieve presumed immunity 761 * against a disease - within the context of an authority.). This is the 762 * underlying object with id, value and extensions. The accessor 763 * "getSeries" gives direct access to the value 764 */ 765 public StringType getSeriesElement() { 766 if (this.series == null) 767 if (Configuration.errorOnAutoCreate()) 768 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.series"); 769 else if (Configuration.doAutoCreate()) 770 this.series = new StringType(); // bb 771 return this.series; 772 } 773 774 public boolean hasSeriesElement() { 775 return this.series != null && !this.series.isEmpty(); 776 } 777 778 public boolean hasSeries() { 779 return this.series != null && !this.series.isEmpty(); 780 } 781 782 /** 783 * @param value {@link #series} (One possible path to achieve presumed immunity 784 * against a disease - within the context of an authority.). This 785 * is the underlying object with id, value and extensions. The 786 * accessor "getSeries" gives direct access to the value 787 */ 788 public ImmunizationVaccinationProtocolComponent setSeriesElement(StringType value) { 789 this.series = value; 790 return this; 791 } 792 793 /** 794 * @return One possible path to achieve presumed immunity against a disease - 795 * within the context of an authority. 796 */ 797 public String getSeries() { 798 return this.series == null ? null : this.series.getValue(); 799 } 800 801 /** 802 * @param value One possible path to achieve presumed immunity against a disease 803 * - within the context of an authority. 804 */ 805 public ImmunizationVaccinationProtocolComponent setSeries(String value) { 806 if (Utilities.noString(value)) 807 this.series = null; 808 else { 809 if (this.series == null) 810 this.series = new StringType(); 811 this.series.setValue(value); 812 } 813 return this; 814 } 815 816 /** 817 * @return {@link #seriesDoses} (The recommended number of doses to achieve 818 * immunity.). This is the underlying object with id, value and 819 * extensions. The accessor "getSeriesDoses" gives direct access to the 820 * value 821 */ 822 public PositiveIntType getSeriesDosesElement() { 823 if (this.seriesDoses == null) 824 if (Configuration.errorOnAutoCreate()) 825 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.seriesDoses"); 826 else if (Configuration.doAutoCreate()) 827 this.seriesDoses = new PositiveIntType(); // bb 828 return this.seriesDoses; 829 } 830 831 public boolean hasSeriesDosesElement() { 832 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 833 } 834 835 public boolean hasSeriesDoses() { 836 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 837 } 838 839 /** 840 * @param value {@link #seriesDoses} (The recommended number of doses to achieve 841 * immunity.). This is the underlying object with id, value and 842 * extensions. The accessor "getSeriesDoses" gives direct access to 843 * the value 844 */ 845 public ImmunizationVaccinationProtocolComponent setSeriesDosesElement(PositiveIntType value) { 846 this.seriesDoses = value; 847 return this; 848 } 849 850 /** 851 * @return The recommended number of doses to achieve immunity. 852 */ 853 public int getSeriesDoses() { 854 return this.seriesDoses == null || this.seriesDoses.isEmpty() ? 0 : this.seriesDoses.getValue(); 855 } 856 857 /** 858 * @param value The recommended number of doses to achieve immunity. 859 */ 860 public ImmunizationVaccinationProtocolComponent setSeriesDoses(int value) { 861 if (this.seriesDoses == null) 862 this.seriesDoses = new PositiveIntType(); 863 this.seriesDoses.setValue(value); 864 return this; 865 } 866 867 /** 868 * @return {@link #targetDisease} (The targeted disease.) 869 */ 870 public List<CodeableConcept> getTargetDisease() { 871 if (this.targetDisease == null) 872 this.targetDisease = new ArrayList<CodeableConcept>(); 873 return this.targetDisease; 874 } 875 876 public boolean hasTargetDisease() { 877 if (this.targetDisease == null) 878 return false; 879 for (CodeableConcept item : this.targetDisease) 880 if (!item.isEmpty()) 881 return true; 882 return false; 883 } 884 885 /** 886 * @return {@link #targetDisease} (The targeted disease.) 887 */ 888 // syntactic sugar 889 public CodeableConcept addTargetDisease() { // 3 890 CodeableConcept t = new CodeableConcept(); 891 if (this.targetDisease == null) 892 this.targetDisease = new ArrayList<CodeableConcept>(); 893 this.targetDisease.add(t); 894 return t; 895 } 896 897 // syntactic sugar 898 public ImmunizationVaccinationProtocolComponent addTargetDisease(CodeableConcept t) { // 3 899 if (t == null) 900 return this; 901 if (this.targetDisease == null) 902 this.targetDisease = new ArrayList<CodeableConcept>(); 903 this.targetDisease.add(t); 904 return this; 905 } 906 907 /** 908 * @return {@link #doseStatus} (Indicates if the immunization event should 909 * "count" against the protocol.) 910 */ 911 public CodeableConcept getDoseStatus() { 912 if (this.doseStatus == null) 913 if (Configuration.errorOnAutoCreate()) 914 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.doseStatus"); 915 else if (Configuration.doAutoCreate()) 916 this.doseStatus = new CodeableConcept(); // cc 917 return this.doseStatus; 918 } 919 920 public boolean hasDoseStatus() { 921 return this.doseStatus != null && !this.doseStatus.isEmpty(); 922 } 923 924 /** 925 * @param value {@link #doseStatus} (Indicates if the immunization event should 926 * "count" against the protocol.) 927 */ 928 public ImmunizationVaccinationProtocolComponent setDoseStatus(CodeableConcept value) { 929 this.doseStatus = value; 930 return this; 931 } 932 933 /** 934 * @return {@link #doseStatusReason} (Provides an explanation as to why an 935 * immunization event should or should not count against the protocol.) 936 */ 937 public CodeableConcept getDoseStatusReason() { 938 if (this.doseStatusReason == null) 939 if (Configuration.errorOnAutoCreate()) 940 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.doseStatusReason"); 941 else if (Configuration.doAutoCreate()) 942 this.doseStatusReason = new CodeableConcept(); // cc 943 return this.doseStatusReason; 944 } 945 946 public boolean hasDoseStatusReason() { 947 return this.doseStatusReason != null && !this.doseStatusReason.isEmpty(); 948 } 949 950 /** 951 * @param value {@link #doseStatusReason} (Provides an explanation as to why an 952 * immunization event should or should not count against the 953 * protocol.) 954 */ 955 public ImmunizationVaccinationProtocolComponent setDoseStatusReason(CodeableConcept value) { 956 this.doseStatusReason = value; 957 return this; 958 } 959 960 protected void listChildren(List<Property> childrenList) { 961 super.listChildren(childrenList); 962 childrenList.add(new Property("doseSequence", "positiveInt", "Nominal position in a series.", 0, 963 java.lang.Integer.MAX_VALUE, doseSequence)); 964 childrenList.add(new Property("description", "string", 965 "Contains the description about the protocol under which the vaccine was administered.", 0, 966 java.lang.Integer.MAX_VALUE, description)); 967 childrenList.add(new Property("authority", "Reference(Organization)", 968 "Indicates the authority who published the protocol. E.g. ACIP.", 0, java.lang.Integer.MAX_VALUE, 969 authority)); 970 childrenList.add(new Property("series", "string", 971 "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 972 java.lang.Integer.MAX_VALUE, series)); 973 childrenList.add(new Property("seriesDoses", "positiveInt", 974 "The recommended number of doses to achieve immunity.", 0, java.lang.Integer.MAX_VALUE, seriesDoses)); 975 childrenList.add(new Property("targetDisease", "CodeableConcept", "The targeted disease.", 0, 976 java.lang.Integer.MAX_VALUE, targetDisease)); 977 childrenList.add(new Property("doseStatus", "CodeableConcept", 978 "Indicates if the immunization event should \"count\" against the protocol.", 0, java.lang.Integer.MAX_VALUE, 979 doseStatus)); 980 childrenList.add(new Property("doseStatusReason", "CodeableConcept", 981 "Provides an explanation as to why an immunization event should or should not count against the protocol.", 0, 982 java.lang.Integer.MAX_VALUE, doseStatusReason)); 983 } 984 985 @Override 986 public void setProperty(String name, Base value) throws FHIRException { 987 if (name.equals("doseSequence")) 988 this.doseSequence = castToPositiveInt(value); // PositiveIntType 989 else if (name.equals("description")) 990 this.description = castToString(value); // StringType 991 else if (name.equals("authority")) 992 this.authority = castToReference(value); // Reference 993 else if (name.equals("series")) 994 this.series = castToString(value); // StringType 995 else if (name.equals("seriesDoses")) 996 this.seriesDoses = castToPositiveInt(value); // PositiveIntType 997 else if (name.equals("targetDisease")) 998 this.getTargetDisease().add(castToCodeableConcept(value)); 999 else if (name.equals("doseStatus")) 1000 this.doseStatus = castToCodeableConcept(value); // CodeableConcept 1001 else if (name.equals("doseStatusReason")) 1002 this.doseStatusReason = castToCodeableConcept(value); // CodeableConcept 1003 else 1004 super.setProperty(name, value); 1005 } 1006 1007 @Override 1008 public Base addChild(String name) throws FHIRException { 1009 if (name.equals("doseSequence")) { 1010 throw new FHIRException("Cannot call addChild on a singleton property Immunization.doseSequence"); 1011 } else if (name.equals("description")) { 1012 throw new FHIRException("Cannot call addChild on a singleton property Immunization.description"); 1013 } else if (name.equals("authority")) { 1014 this.authority = new Reference(); 1015 return this.authority; 1016 } else if (name.equals("series")) { 1017 throw new FHIRException("Cannot call addChild on a singleton property Immunization.series"); 1018 } else if (name.equals("seriesDoses")) { 1019 throw new FHIRException("Cannot call addChild on a singleton property Immunization.seriesDoses"); 1020 } else if (name.equals("targetDisease")) { 1021 return addTargetDisease(); 1022 } else if (name.equals("doseStatus")) { 1023 this.doseStatus = new CodeableConcept(); 1024 return this.doseStatus; 1025 } else if (name.equals("doseStatusReason")) { 1026 this.doseStatusReason = new CodeableConcept(); 1027 return this.doseStatusReason; 1028 } else 1029 return super.addChild(name); 1030 } 1031 1032 public ImmunizationVaccinationProtocolComponent copy() { 1033 ImmunizationVaccinationProtocolComponent dst = new ImmunizationVaccinationProtocolComponent(); 1034 copyValues(dst); 1035 dst.doseSequence = doseSequence == null ? null : doseSequence.copy(); 1036 dst.description = description == null ? null : description.copy(); 1037 dst.authority = authority == null ? null : authority.copy(); 1038 dst.series = series == null ? null : series.copy(); 1039 dst.seriesDoses = seriesDoses == null ? null : seriesDoses.copy(); 1040 if (targetDisease != null) { 1041 dst.targetDisease = new ArrayList<CodeableConcept>(); 1042 for (CodeableConcept i : targetDisease) 1043 dst.targetDisease.add(i.copy()); 1044 } 1045 ; 1046 dst.doseStatus = doseStatus == null ? null : doseStatus.copy(); 1047 dst.doseStatusReason = doseStatusReason == null ? null : doseStatusReason.copy(); 1048 return dst; 1049 } 1050 1051 @Override 1052 public boolean equalsDeep(Base other) { 1053 if (!super.equalsDeep(other)) 1054 return false; 1055 if (!(other instanceof ImmunizationVaccinationProtocolComponent)) 1056 return false; 1057 ImmunizationVaccinationProtocolComponent o = (ImmunizationVaccinationProtocolComponent) other; 1058 return compareDeep(doseSequence, o.doseSequence, true) && compareDeep(description, o.description, true) 1059 && compareDeep(authority, o.authority, true) && compareDeep(series, o.series, true) 1060 && compareDeep(seriesDoses, o.seriesDoses, true) && compareDeep(targetDisease, o.targetDisease, true) 1061 && compareDeep(doseStatus, o.doseStatus, true) && compareDeep(doseStatusReason, o.doseStatusReason, true); 1062 } 1063 1064 @Override 1065 public boolean equalsShallow(Base other) { 1066 if (!super.equalsShallow(other)) 1067 return false; 1068 if (!(other instanceof ImmunizationVaccinationProtocolComponent)) 1069 return false; 1070 ImmunizationVaccinationProtocolComponent o = (ImmunizationVaccinationProtocolComponent) other; 1071 return compareValues(doseSequence, o.doseSequence, true) && compareValues(description, o.description, true) 1072 && compareValues(series, o.series, true) && compareValues(seriesDoses, o.seriesDoses, true); 1073 } 1074 1075 public boolean isEmpty() { 1076 return super.isEmpty() && (doseSequence == null || doseSequence.isEmpty()) 1077 && (description == null || description.isEmpty()) && (authority == null || authority.isEmpty()) 1078 && (series == null || series.isEmpty()) && (seriesDoses == null || seriesDoses.isEmpty()) 1079 && (targetDisease == null || targetDisease.isEmpty()) && (doseStatus == null || doseStatus.isEmpty()) 1080 && (doseStatusReason == null || doseStatusReason.isEmpty()); 1081 } 1082 1083 public String fhirType() { 1084 return "Immunization.vaccinationProtocol"; 1085 1086 } 1087 1088 } 1089 1090 /** 1091 * A unique identifier assigned to this immunization record. 1092 */ 1093 @Child(name = "identifier", type = { 1094 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1095 @Description(shortDefinition = "Business identifier", formalDefinition = "A unique identifier assigned to this immunization record.") 1096 protected List<Identifier> identifier; 1097 1098 /** 1099 * Indicates the current status of the vaccination event. 1100 */ 1101 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 1102 @Description(shortDefinition = "in-progress | on-hold | completed | entered-in-error | stopped", formalDefinition = "Indicates the current status of the vaccination event.") 1103 protected CodeType status; 1104 1105 /** 1106 * Date vaccine administered or was to be administered. 1107 */ 1108 @Child(name = "date", type = { DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1109 @Description(shortDefinition = "Vaccination administration date", formalDefinition = "Date vaccine administered or was to be administered.") 1110 protected DateTimeType date; 1111 1112 /** 1113 * Vaccine that was administered or was to be administered. 1114 */ 1115 @Child(name = "vaccineCode", type = { 1116 CodeableConcept.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 1117 @Description(shortDefinition = "Vaccine product administered", formalDefinition = "Vaccine that was administered or was to be administered.") 1118 protected CodeableConcept vaccineCode; 1119 1120 /** 1121 * The patient who either received or did not receive the immunization. 1122 */ 1123 @Child(name = "patient", type = { Patient.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 1124 @Description(shortDefinition = "Who was immunized", formalDefinition = "The patient who either received or did not receive the immunization.") 1125 protected Reference patient; 1126 1127 /** 1128 * The actual object that is the target of the reference (The patient who either 1129 * received or did not receive the immunization.) 1130 */ 1131 protected Patient patientTarget; 1132 1133 /** 1134 * Indicates if the vaccination was or was not given. 1135 */ 1136 @Child(name = "wasNotGiven", type = { 1137 BooleanType.class }, order = 5, min = 1, max = 1, modifier = true, summary = false) 1138 @Description(shortDefinition = "Flag for whether immunization was given", formalDefinition = "Indicates if the vaccination was or was not given.") 1139 protected BooleanType wasNotGiven; 1140 1141 /** 1142 * True if this administration was reported rather than directly administered. 1143 */ 1144 @Child(name = "reported", type = { 1145 BooleanType.class }, order = 6, min = 1, max = 1, modifier = false, summary = false) 1146 @Description(shortDefinition = "Indicates a self-reported record", formalDefinition = "True if this administration was reported rather than directly administered.") 1147 protected BooleanType reported; 1148 1149 /** 1150 * Clinician who administered the vaccine. 1151 */ 1152 @Child(name = "performer", type = { 1153 Practitioner.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1154 @Description(shortDefinition = "Who administered vaccine", formalDefinition = "Clinician who administered the vaccine.") 1155 protected Reference performer; 1156 1157 /** 1158 * The actual object that is the target of the reference (Clinician who 1159 * administered the vaccine.) 1160 */ 1161 protected Practitioner performerTarget; 1162 1163 /** 1164 * Clinician who ordered the vaccination. 1165 */ 1166 @Child(name = "requester", type = { 1167 Practitioner.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1168 @Description(shortDefinition = "Who ordered vaccination", formalDefinition = "Clinician who ordered the vaccination.") 1169 protected Reference requester; 1170 1171 /** 1172 * The actual object that is the target of the reference (Clinician who ordered 1173 * the vaccination.) 1174 */ 1175 protected Practitioner requesterTarget; 1176 1177 /** 1178 * The visit or admission or other contact between patient and health care 1179 * provider the immunization was performed as part of. 1180 */ 1181 @Child(name = "encounter", type = { Encounter.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1182 @Description(shortDefinition = "Encounter administered as part of", formalDefinition = "The visit or admission or other contact between patient and health care provider the immunization was performed as part of.") 1183 protected Reference encounter; 1184 1185 /** 1186 * The actual object that is the target of the reference (The visit or admission 1187 * or other contact between patient and health care provider the immunization 1188 * was performed as part of.) 1189 */ 1190 protected Encounter encounterTarget; 1191 1192 /** 1193 * Name of vaccine manufacturer. 1194 */ 1195 @Child(name = "manufacturer", type = { 1196 Organization.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1197 @Description(shortDefinition = "Vaccine manufacturer", formalDefinition = "Name of vaccine manufacturer.") 1198 protected Reference manufacturer; 1199 1200 /** 1201 * The actual object that is the target of the reference (Name of vaccine 1202 * manufacturer.) 1203 */ 1204 protected Organization manufacturerTarget; 1205 1206 /** 1207 * The service delivery location where the vaccine administration occurred. 1208 */ 1209 @Child(name = "location", type = { Location.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 1210 @Description(shortDefinition = "Where vaccination occurred", formalDefinition = "The service delivery location where the vaccine administration occurred.") 1211 protected Reference location; 1212 1213 /** 1214 * The actual object that is the target of the reference (The service delivery 1215 * location where the vaccine administration occurred.) 1216 */ 1217 protected Location locationTarget; 1218 1219 /** 1220 * Lot number of the vaccine product. 1221 */ 1222 @Child(name = "lotNumber", type = { 1223 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1224 @Description(shortDefinition = "Vaccine lot number", formalDefinition = "Lot number of the vaccine product.") 1225 protected StringType lotNumber; 1226 1227 /** 1228 * Date vaccine batch expires. 1229 */ 1230 @Child(name = "expirationDate", type = { 1231 DateType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1232 @Description(shortDefinition = "Vaccine expiration date", formalDefinition = "Date vaccine batch expires.") 1233 protected DateType expirationDate; 1234 1235 /** 1236 * Body site where vaccine was administered. 1237 */ 1238 @Child(name = "site", type = { 1239 CodeableConcept.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 1240 @Description(shortDefinition = "Body site vaccine was administered", formalDefinition = "Body site where vaccine was administered.") 1241 protected CodeableConcept site; 1242 1243 /** 1244 * The path by which the vaccine product is taken into the body. 1245 */ 1246 @Child(name = "route", type = { 1247 CodeableConcept.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 1248 @Description(shortDefinition = "How vaccine entered body", formalDefinition = "The path by which the vaccine product is taken into the body.") 1249 protected CodeableConcept route; 1250 1251 /** 1252 * The quantity of vaccine product that was administered. 1253 */ 1254 @Child(name = "doseQuantity", type = { 1255 SimpleQuantity.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 1256 @Description(shortDefinition = "Amount of vaccine administered", formalDefinition = "The quantity of vaccine product that was administered.") 1257 protected SimpleQuantity doseQuantity; 1258 1259 /** 1260 * Extra information about the immunization that is not conveyed by the other 1261 * attributes. 1262 */ 1263 @Child(name = "note", type = { 1264 Annotation.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1265 @Description(shortDefinition = "Vaccination notes", formalDefinition = "Extra information about the immunization that is not conveyed by the other attributes.") 1266 protected List<Annotation> note; 1267 1268 /** 1269 * Reasons why a vaccine was or was not administered. 1270 */ 1271 @Child(name = "explanation", type = {}, order = 18, min = 0, max = 1, modifier = false, summary = false) 1272 @Description(shortDefinition = "Administration/non-administration reasons", formalDefinition = "Reasons why a vaccine was or was not administered.") 1273 protected ImmunizationExplanationComponent explanation; 1274 1275 /** 1276 * Categorical data indicating that an adverse event is associated in time to an 1277 * immunization. 1278 */ 1279 @Child(name = "reaction", type = {}, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1280 @Description(shortDefinition = "Details of a reaction that follows immunization", formalDefinition = "Categorical data indicating that an adverse event is associated in time to an immunization.") 1281 protected List<ImmunizationReactionComponent> reaction; 1282 1283 /** 1284 * Contains information about the protocol(s) under which the vaccine was 1285 * administered. 1286 */ 1287 @Child(name = "vaccinationProtocol", type = {}, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1288 @Description(shortDefinition = "What protocol was followed", formalDefinition = "Contains information about the protocol(s) under which the vaccine was administered.") 1289 protected List<ImmunizationVaccinationProtocolComponent> vaccinationProtocol; 1290 1291 private static final long serialVersionUID = 898786200L; 1292 1293 /* 1294 * Constructor 1295 */ 1296 public Immunization() { 1297 super(); 1298 } 1299 1300 /* 1301 * Constructor 1302 */ 1303 public Immunization(CodeType status, CodeableConcept vaccineCode, Reference patient, BooleanType wasNotGiven, 1304 BooleanType reported) { 1305 super(); 1306 this.status = status; 1307 this.vaccineCode = vaccineCode; 1308 this.patient = patient; 1309 this.wasNotGiven = wasNotGiven; 1310 this.reported = reported; 1311 } 1312 1313 /** 1314 * @return {@link #identifier} (A unique identifier assigned to this 1315 * immunization record.) 1316 */ 1317 public List<Identifier> getIdentifier() { 1318 if (this.identifier == null) 1319 this.identifier = new ArrayList<Identifier>(); 1320 return this.identifier; 1321 } 1322 1323 public boolean hasIdentifier() { 1324 if (this.identifier == null) 1325 return false; 1326 for (Identifier item : this.identifier) 1327 if (!item.isEmpty()) 1328 return true; 1329 return false; 1330 } 1331 1332 /** 1333 * @return {@link #identifier} (A unique identifier assigned to this 1334 * immunization record.) 1335 */ 1336 // syntactic sugar 1337 public Identifier addIdentifier() { // 3 1338 Identifier t = new Identifier(); 1339 if (this.identifier == null) 1340 this.identifier = new ArrayList<Identifier>(); 1341 this.identifier.add(t); 1342 return t; 1343 } 1344 1345 // syntactic sugar 1346 public Immunization addIdentifier(Identifier t) { // 3 1347 if (t == null) 1348 return this; 1349 if (this.identifier == null) 1350 this.identifier = new ArrayList<Identifier>(); 1351 this.identifier.add(t); 1352 return this; 1353 } 1354 1355 /** 1356 * @return {@link #status} (Indicates the current status of the vaccination 1357 * event.). This is the underlying object with id, value and extensions. 1358 * The accessor "getStatus" gives direct access to the value 1359 */ 1360 public CodeType getStatusElement() { 1361 if (this.status == null) 1362 if (Configuration.errorOnAutoCreate()) 1363 throw new Error("Attempt to auto-create Immunization.status"); 1364 else if (Configuration.doAutoCreate()) 1365 this.status = new CodeType(); // bb 1366 return this.status; 1367 } 1368 1369 public boolean hasStatusElement() { 1370 return this.status != null && !this.status.isEmpty(); 1371 } 1372 1373 public boolean hasStatus() { 1374 return this.status != null && !this.status.isEmpty(); 1375 } 1376 1377 /** 1378 * @param value {@link #status} (Indicates the current status of the vaccination 1379 * event.). This is the underlying object with id, value and 1380 * extensions. The accessor "getStatus" gives direct access to the 1381 * value 1382 */ 1383 public Immunization setStatusElement(CodeType value) { 1384 this.status = value; 1385 return this; 1386 } 1387 1388 /** 1389 * @return Indicates the current status of the vaccination event. 1390 */ 1391 public String getStatus() { 1392 return this.status == null ? null : this.status.getValue(); 1393 } 1394 1395 /** 1396 * @param value Indicates the current status of the vaccination event. 1397 */ 1398 public Immunization setStatus(String value) { 1399 if (this.status == null) 1400 this.status = new CodeType(); 1401 this.status.setValue(value); 1402 return this; 1403 } 1404 1405 /** 1406 * @return {@link #date} (Date vaccine administered or was to be administered.). 1407 * This is the underlying object with id, value and extensions. The 1408 * accessor "getDate" gives direct access to the value 1409 */ 1410 public DateTimeType getDateElement() { 1411 if (this.date == null) 1412 if (Configuration.errorOnAutoCreate()) 1413 throw new Error("Attempt to auto-create Immunization.date"); 1414 else if (Configuration.doAutoCreate()) 1415 this.date = new DateTimeType(); // bb 1416 return this.date; 1417 } 1418 1419 public boolean hasDateElement() { 1420 return this.date != null && !this.date.isEmpty(); 1421 } 1422 1423 public boolean hasDate() { 1424 return this.date != null && !this.date.isEmpty(); 1425 } 1426 1427 /** 1428 * @param value {@link #date} (Date vaccine administered or was to be 1429 * administered.). This is the underlying object with id, value and 1430 * extensions. The accessor "getDate" gives direct access to the 1431 * value 1432 */ 1433 public Immunization setDateElement(DateTimeType value) { 1434 this.date = value; 1435 return this; 1436 } 1437 1438 /** 1439 * @return Date vaccine administered or was to be administered. 1440 */ 1441 public Date getDate() { 1442 return this.date == null ? null : this.date.getValue(); 1443 } 1444 1445 /** 1446 * @param value Date vaccine administered or was to be administered. 1447 */ 1448 public Immunization setDate(Date value) { 1449 if (value == null) 1450 this.date = null; 1451 else { 1452 if (this.date == null) 1453 this.date = new DateTimeType(); 1454 this.date.setValue(value); 1455 } 1456 return this; 1457 } 1458 1459 /** 1460 * @return {@link #vaccineCode} (Vaccine that was administered or was to be 1461 * administered.) 1462 */ 1463 public CodeableConcept getVaccineCode() { 1464 if (this.vaccineCode == null) 1465 if (Configuration.errorOnAutoCreate()) 1466 throw new Error("Attempt to auto-create Immunization.vaccineCode"); 1467 else if (Configuration.doAutoCreate()) 1468 this.vaccineCode = new CodeableConcept(); // cc 1469 return this.vaccineCode; 1470 } 1471 1472 public boolean hasVaccineCode() { 1473 return this.vaccineCode != null && !this.vaccineCode.isEmpty(); 1474 } 1475 1476 /** 1477 * @param value {@link #vaccineCode} (Vaccine that was administered or was to be 1478 * administered.) 1479 */ 1480 public Immunization setVaccineCode(CodeableConcept value) { 1481 this.vaccineCode = value; 1482 return this; 1483 } 1484 1485 /** 1486 * @return {@link #patient} (The patient who either received or did not receive 1487 * the immunization.) 1488 */ 1489 public Reference getPatient() { 1490 if (this.patient == null) 1491 if (Configuration.errorOnAutoCreate()) 1492 throw new Error("Attempt to auto-create Immunization.patient"); 1493 else if (Configuration.doAutoCreate()) 1494 this.patient = new Reference(); // cc 1495 return this.patient; 1496 } 1497 1498 public boolean hasPatient() { 1499 return this.patient != null && !this.patient.isEmpty(); 1500 } 1501 1502 /** 1503 * @param value {@link #patient} (The patient who either received or did not 1504 * receive the immunization.) 1505 */ 1506 public Immunization setPatient(Reference value) { 1507 this.patient = value; 1508 return this; 1509 } 1510 1511 /** 1512 * @return {@link #patient} The actual object that is the target of the 1513 * reference. The reference library doesn't populate this, but you can 1514 * use it to hold the resource if you resolve it. (The patient who 1515 * either received or did not receive the immunization.) 1516 */ 1517 public Patient getPatientTarget() { 1518 if (this.patientTarget == null) 1519 if (Configuration.errorOnAutoCreate()) 1520 throw new Error("Attempt to auto-create Immunization.patient"); 1521 else if (Configuration.doAutoCreate()) 1522 this.patientTarget = new Patient(); // aa 1523 return this.patientTarget; 1524 } 1525 1526 /** 1527 * @param value {@link #patient} The actual object that is the target of the 1528 * reference. The reference library doesn't use these, but you can 1529 * use it to hold the resource if you resolve it. (The patient who 1530 * either received or did not receive the immunization.) 1531 */ 1532 public Immunization setPatientTarget(Patient value) { 1533 this.patientTarget = value; 1534 return this; 1535 } 1536 1537 /** 1538 * @return {@link #wasNotGiven} (Indicates if the vaccination was or was not 1539 * given.). This is the underlying object with id, value and extensions. 1540 * The accessor "getWasNotGiven" gives direct access to the value 1541 */ 1542 public BooleanType getWasNotGivenElement() { 1543 if (this.wasNotGiven == null) 1544 if (Configuration.errorOnAutoCreate()) 1545 throw new Error("Attempt to auto-create Immunization.wasNotGiven"); 1546 else if (Configuration.doAutoCreate()) 1547 this.wasNotGiven = new BooleanType(); // bb 1548 return this.wasNotGiven; 1549 } 1550 1551 public boolean hasWasNotGivenElement() { 1552 return this.wasNotGiven != null && !this.wasNotGiven.isEmpty(); 1553 } 1554 1555 public boolean hasWasNotGiven() { 1556 return this.wasNotGiven != null && !this.wasNotGiven.isEmpty(); 1557 } 1558 1559 /** 1560 * @param value {@link #wasNotGiven} (Indicates if the vaccination was or was 1561 * not given.). This is the underlying object with id, value and 1562 * extensions. The accessor "getWasNotGiven" gives direct access to 1563 * the value 1564 */ 1565 public Immunization setWasNotGivenElement(BooleanType value) { 1566 this.wasNotGiven = value; 1567 return this; 1568 } 1569 1570 /** 1571 * @return Indicates if the vaccination was or was not given. 1572 */ 1573 public boolean getWasNotGiven() { 1574 return this.wasNotGiven == null || this.wasNotGiven.isEmpty() ? false : this.wasNotGiven.getValue(); 1575 } 1576 1577 /** 1578 * @param value Indicates if the vaccination was or was not given. 1579 */ 1580 public Immunization setWasNotGiven(boolean value) { 1581 if (this.wasNotGiven == null) 1582 this.wasNotGiven = new BooleanType(); 1583 this.wasNotGiven.setValue(value); 1584 return this; 1585 } 1586 1587 /** 1588 * @return {@link #reported} (True if this administration was reported rather 1589 * than directly administered.). This is the underlying object with id, 1590 * value and extensions. The accessor "getReported" gives direct access 1591 * to the value 1592 */ 1593 public BooleanType getReportedElement() { 1594 if (this.reported == null) 1595 if (Configuration.errorOnAutoCreate()) 1596 throw new Error("Attempt to auto-create Immunization.reported"); 1597 else if (Configuration.doAutoCreate()) 1598 this.reported = new BooleanType(); // bb 1599 return this.reported; 1600 } 1601 1602 public boolean hasReportedElement() { 1603 return this.reported != null && !this.reported.isEmpty(); 1604 } 1605 1606 public boolean hasReported() { 1607 return this.reported != null && !this.reported.isEmpty(); 1608 } 1609 1610 /** 1611 * @param value {@link #reported} (True if this administration was reported 1612 * rather than directly administered.). This is the underlying 1613 * object with id, value and extensions. The accessor "getReported" 1614 * gives direct access to the value 1615 */ 1616 public Immunization setReportedElement(BooleanType value) { 1617 this.reported = value; 1618 return this; 1619 } 1620 1621 /** 1622 * @return True if this administration was reported rather than directly 1623 * administered. 1624 */ 1625 public boolean getReported() { 1626 return this.reported == null || this.reported.isEmpty() ? false : this.reported.getValue(); 1627 } 1628 1629 /** 1630 * @param value True if this administration was reported rather than directly 1631 * administered. 1632 */ 1633 public Immunization setReported(boolean value) { 1634 if (this.reported == null) 1635 this.reported = new BooleanType(); 1636 this.reported.setValue(value); 1637 return this; 1638 } 1639 1640 /** 1641 * @return {@link #performer} (Clinician who administered the vaccine.) 1642 */ 1643 public Reference getPerformer() { 1644 if (this.performer == null) 1645 if (Configuration.errorOnAutoCreate()) 1646 throw new Error("Attempt to auto-create Immunization.performer"); 1647 else if (Configuration.doAutoCreate()) 1648 this.performer = new Reference(); // cc 1649 return this.performer; 1650 } 1651 1652 public boolean hasPerformer() { 1653 return this.performer != null && !this.performer.isEmpty(); 1654 } 1655 1656 /** 1657 * @param value {@link #performer} (Clinician who administered the vaccine.) 1658 */ 1659 public Immunization setPerformer(Reference value) { 1660 this.performer = value; 1661 return this; 1662 } 1663 1664 /** 1665 * @return {@link #performer} The actual object that is the target of the 1666 * reference. The reference library doesn't populate this, but you can 1667 * use it to hold the resource if you resolve it. (Clinician who 1668 * administered the vaccine.) 1669 */ 1670 public Practitioner getPerformerTarget() { 1671 if (this.performerTarget == null) 1672 if (Configuration.errorOnAutoCreate()) 1673 throw new Error("Attempt to auto-create Immunization.performer"); 1674 else if (Configuration.doAutoCreate()) 1675 this.performerTarget = new Practitioner(); // aa 1676 return this.performerTarget; 1677 } 1678 1679 /** 1680 * @param value {@link #performer} The actual object that is the target of the 1681 * reference. The reference library doesn't use these, but you can 1682 * use it to hold the resource if you resolve it. (Clinician who 1683 * administered the vaccine.) 1684 */ 1685 public Immunization setPerformerTarget(Practitioner value) { 1686 this.performerTarget = value; 1687 return this; 1688 } 1689 1690 /** 1691 * @return {@link #requester} (Clinician who ordered the vaccination.) 1692 */ 1693 public Reference getRequester() { 1694 if (this.requester == null) 1695 if (Configuration.errorOnAutoCreate()) 1696 throw new Error("Attempt to auto-create Immunization.requester"); 1697 else if (Configuration.doAutoCreate()) 1698 this.requester = new Reference(); // cc 1699 return this.requester; 1700 } 1701 1702 public boolean hasRequester() { 1703 return this.requester != null && !this.requester.isEmpty(); 1704 } 1705 1706 /** 1707 * @param value {@link #requester} (Clinician who ordered the vaccination.) 1708 */ 1709 public Immunization setRequester(Reference value) { 1710 this.requester = value; 1711 return this; 1712 } 1713 1714 /** 1715 * @return {@link #requester} The actual object that is the target of the 1716 * reference. The reference library doesn't populate this, but you can 1717 * use it to hold the resource if you resolve it. (Clinician who ordered 1718 * the vaccination.) 1719 */ 1720 public Practitioner getRequesterTarget() { 1721 if (this.requesterTarget == null) 1722 if (Configuration.errorOnAutoCreate()) 1723 throw new Error("Attempt to auto-create Immunization.requester"); 1724 else if (Configuration.doAutoCreate()) 1725 this.requesterTarget = new Practitioner(); // aa 1726 return this.requesterTarget; 1727 } 1728 1729 /** 1730 * @param value {@link #requester} The actual object that is the target of the 1731 * reference. The reference library doesn't use these, but you can 1732 * use it to hold the resource if you resolve it. (Clinician who 1733 * ordered the vaccination.) 1734 */ 1735 public Immunization setRequesterTarget(Practitioner value) { 1736 this.requesterTarget = value; 1737 return this; 1738 } 1739 1740 /** 1741 * @return {@link #encounter} (The visit or admission or other contact between 1742 * patient and health care provider the immunization was performed as 1743 * part of.) 1744 */ 1745 public Reference getEncounter() { 1746 if (this.encounter == null) 1747 if (Configuration.errorOnAutoCreate()) 1748 throw new Error("Attempt to auto-create Immunization.encounter"); 1749 else if (Configuration.doAutoCreate()) 1750 this.encounter = new Reference(); // cc 1751 return this.encounter; 1752 } 1753 1754 public boolean hasEncounter() { 1755 return this.encounter != null && !this.encounter.isEmpty(); 1756 } 1757 1758 /** 1759 * @param value {@link #encounter} (The visit or admission or other contact 1760 * between patient and health care provider the immunization was 1761 * performed as part of.) 1762 */ 1763 public Immunization setEncounter(Reference value) { 1764 this.encounter = value; 1765 return this; 1766 } 1767 1768 /** 1769 * @return {@link #encounter} The actual object that is the target of the 1770 * reference. The reference library doesn't populate this, but you can 1771 * use it to hold the resource if you resolve it. (The visit or 1772 * admission or other contact between patient and health care provider 1773 * the immunization was performed as part of.) 1774 */ 1775 public Encounter getEncounterTarget() { 1776 if (this.encounterTarget == null) 1777 if (Configuration.errorOnAutoCreate()) 1778 throw new Error("Attempt to auto-create Immunization.encounter"); 1779 else if (Configuration.doAutoCreate()) 1780 this.encounterTarget = new Encounter(); // aa 1781 return this.encounterTarget; 1782 } 1783 1784 /** 1785 * @param value {@link #encounter} The actual object that is the target of the 1786 * reference. The reference library doesn't use these, but you can 1787 * use it to hold the resource if you resolve it. (The visit or 1788 * admission or other contact between patient and health care 1789 * provider the immunization was performed as part of.) 1790 */ 1791 public Immunization setEncounterTarget(Encounter value) { 1792 this.encounterTarget = value; 1793 return this; 1794 } 1795 1796 /** 1797 * @return {@link #manufacturer} (Name of vaccine manufacturer.) 1798 */ 1799 public Reference getManufacturer() { 1800 if (this.manufacturer == null) 1801 if (Configuration.errorOnAutoCreate()) 1802 throw new Error("Attempt to auto-create Immunization.manufacturer"); 1803 else if (Configuration.doAutoCreate()) 1804 this.manufacturer = new Reference(); // cc 1805 return this.manufacturer; 1806 } 1807 1808 public boolean hasManufacturer() { 1809 return this.manufacturer != null && !this.manufacturer.isEmpty(); 1810 } 1811 1812 /** 1813 * @param value {@link #manufacturer} (Name of vaccine manufacturer.) 1814 */ 1815 public Immunization setManufacturer(Reference value) { 1816 this.manufacturer = value; 1817 return this; 1818 } 1819 1820 /** 1821 * @return {@link #manufacturer} The actual object that is the target of the 1822 * reference. The reference library doesn't populate this, but you can 1823 * use it to hold the resource if you resolve it. (Name of vaccine 1824 * manufacturer.) 1825 */ 1826 public Organization getManufacturerTarget() { 1827 if (this.manufacturerTarget == null) 1828 if (Configuration.errorOnAutoCreate()) 1829 throw new Error("Attempt to auto-create Immunization.manufacturer"); 1830 else if (Configuration.doAutoCreate()) 1831 this.manufacturerTarget = new Organization(); // aa 1832 return this.manufacturerTarget; 1833 } 1834 1835 /** 1836 * @param value {@link #manufacturer} The actual object that is the target of 1837 * the reference. The reference library doesn't use these, but you 1838 * can use it to hold the resource if you resolve it. (Name of 1839 * vaccine manufacturer.) 1840 */ 1841 public Immunization setManufacturerTarget(Organization value) { 1842 this.manufacturerTarget = value; 1843 return this; 1844 } 1845 1846 /** 1847 * @return {@link #location} (The service delivery location where the vaccine 1848 * administration occurred.) 1849 */ 1850 public Reference getLocation() { 1851 if (this.location == null) 1852 if (Configuration.errorOnAutoCreate()) 1853 throw new Error("Attempt to auto-create Immunization.location"); 1854 else if (Configuration.doAutoCreate()) 1855 this.location = new Reference(); // cc 1856 return this.location; 1857 } 1858 1859 public boolean hasLocation() { 1860 return this.location != null && !this.location.isEmpty(); 1861 } 1862 1863 /** 1864 * @param value {@link #location} (The service delivery location where the 1865 * vaccine administration occurred.) 1866 */ 1867 public Immunization setLocation(Reference value) { 1868 this.location = value; 1869 return this; 1870 } 1871 1872 /** 1873 * @return {@link #location} The actual object that is the target of the 1874 * reference. The reference library doesn't populate this, but you can 1875 * use it to hold the resource if you resolve it. (The service delivery 1876 * location where the vaccine administration occurred.) 1877 */ 1878 public Location getLocationTarget() { 1879 if (this.locationTarget == null) 1880 if (Configuration.errorOnAutoCreate()) 1881 throw new Error("Attempt to auto-create Immunization.location"); 1882 else if (Configuration.doAutoCreate()) 1883 this.locationTarget = new Location(); // aa 1884 return this.locationTarget; 1885 } 1886 1887 /** 1888 * @param value {@link #location} The actual object that is the target of the 1889 * reference. The reference library doesn't use these, but you can 1890 * use it to hold the resource if you resolve it. (The service 1891 * delivery location where the vaccine administration occurred.) 1892 */ 1893 public Immunization setLocationTarget(Location value) { 1894 this.locationTarget = value; 1895 return this; 1896 } 1897 1898 /** 1899 * @return {@link #lotNumber} (Lot number of the vaccine product.). This is the 1900 * underlying object with id, value and extensions. The accessor 1901 * "getLotNumber" gives direct access to the value 1902 */ 1903 public StringType getLotNumberElement() { 1904 if (this.lotNumber == null) 1905 if (Configuration.errorOnAutoCreate()) 1906 throw new Error("Attempt to auto-create Immunization.lotNumber"); 1907 else if (Configuration.doAutoCreate()) 1908 this.lotNumber = new StringType(); // bb 1909 return this.lotNumber; 1910 } 1911 1912 public boolean hasLotNumberElement() { 1913 return this.lotNumber != null && !this.lotNumber.isEmpty(); 1914 } 1915 1916 public boolean hasLotNumber() { 1917 return this.lotNumber != null && !this.lotNumber.isEmpty(); 1918 } 1919 1920 /** 1921 * @param value {@link #lotNumber} (Lot number of the vaccine product.). This is 1922 * the underlying object with id, value and extensions. The 1923 * accessor "getLotNumber" gives direct access to the value 1924 */ 1925 public Immunization setLotNumberElement(StringType value) { 1926 this.lotNumber = value; 1927 return this; 1928 } 1929 1930 /** 1931 * @return Lot number of the vaccine product. 1932 */ 1933 public String getLotNumber() { 1934 return this.lotNumber == null ? null : this.lotNumber.getValue(); 1935 } 1936 1937 /** 1938 * @param value Lot number of the vaccine product. 1939 */ 1940 public Immunization setLotNumber(String value) { 1941 if (Utilities.noString(value)) 1942 this.lotNumber = null; 1943 else { 1944 if (this.lotNumber == null) 1945 this.lotNumber = new StringType(); 1946 this.lotNumber.setValue(value); 1947 } 1948 return this; 1949 } 1950 1951 /** 1952 * @return {@link #expirationDate} (Date vaccine batch expires.). This is the 1953 * underlying object with id, value and extensions. The accessor 1954 * "getExpirationDate" gives direct access to the value 1955 */ 1956 public DateType getExpirationDateElement() { 1957 if (this.expirationDate == null) 1958 if (Configuration.errorOnAutoCreate()) 1959 throw new Error("Attempt to auto-create Immunization.expirationDate"); 1960 else if (Configuration.doAutoCreate()) 1961 this.expirationDate = new DateType(); // bb 1962 return this.expirationDate; 1963 } 1964 1965 public boolean hasExpirationDateElement() { 1966 return this.expirationDate != null && !this.expirationDate.isEmpty(); 1967 } 1968 1969 public boolean hasExpirationDate() { 1970 return this.expirationDate != null && !this.expirationDate.isEmpty(); 1971 } 1972 1973 /** 1974 * @param value {@link #expirationDate} (Date vaccine batch expires.). This is 1975 * the underlying object with id, value and extensions. The 1976 * accessor "getExpirationDate" gives direct access to the value 1977 */ 1978 public Immunization setExpirationDateElement(DateType value) { 1979 this.expirationDate = value; 1980 return this; 1981 } 1982 1983 /** 1984 * @return Date vaccine batch expires. 1985 */ 1986 public Date getExpirationDate() { 1987 return this.expirationDate == null ? null : this.expirationDate.getValue(); 1988 } 1989 1990 /** 1991 * @param value Date vaccine batch expires. 1992 */ 1993 public Immunization setExpirationDate(Date value) { 1994 if (value == null) 1995 this.expirationDate = null; 1996 else { 1997 if (this.expirationDate == null) 1998 this.expirationDate = new DateType(); 1999 this.expirationDate.setValue(value); 2000 } 2001 return this; 2002 } 2003 2004 /** 2005 * @return {@link #site} (Body site where vaccine was administered.) 2006 */ 2007 public CodeableConcept getSite() { 2008 if (this.site == null) 2009 if (Configuration.errorOnAutoCreate()) 2010 throw new Error("Attempt to auto-create Immunization.site"); 2011 else if (Configuration.doAutoCreate()) 2012 this.site = new CodeableConcept(); // cc 2013 return this.site; 2014 } 2015 2016 public boolean hasSite() { 2017 return this.site != null && !this.site.isEmpty(); 2018 } 2019 2020 /** 2021 * @param value {@link #site} (Body site where vaccine was administered.) 2022 */ 2023 public Immunization setSite(CodeableConcept value) { 2024 this.site = value; 2025 return this; 2026 } 2027 2028 /** 2029 * @return {@link #route} (The path by which the vaccine product is taken into 2030 * the body.) 2031 */ 2032 public CodeableConcept getRoute() { 2033 if (this.route == null) 2034 if (Configuration.errorOnAutoCreate()) 2035 throw new Error("Attempt to auto-create Immunization.route"); 2036 else if (Configuration.doAutoCreate()) 2037 this.route = new CodeableConcept(); // cc 2038 return this.route; 2039 } 2040 2041 public boolean hasRoute() { 2042 return this.route != null && !this.route.isEmpty(); 2043 } 2044 2045 /** 2046 * @param value {@link #route} (The path by which the vaccine product is taken 2047 * into the body.) 2048 */ 2049 public Immunization setRoute(CodeableConcept value) { 2050 this.route = value; 2051 return this; 2052 } 2053 2054 /** 2055 * @return {@link #doseQuantity} (The quantity of vaccine product that was 2056 * administered.) 2057 */ 2058 public SimpleQuantity getDoseQuantity() { 2059 if (this.doseQuantity == null) 2060 if (Configuration.errorOnAutoCreate()) 2061 throw new Error("Attempt to auto-create Immunization.doseQuantity"); 2062 else if (Configuration.doAutoCreate()) 2063 this.doseQuantity = new SimpleQuantity(); // cc 2064 return this.doseQuantity; 2065 } 2066 2067 public boolean hasDoseQuantity() { 2068 return this.doseQuantity != null && !this.doseQuantity.isEmpty(); 2069 } 2070 2071 /** 2072 * @param value {@link #doseQuantity} (The quantity of vaccine product that was 2073 * administered.) 2074 */ 2075 public Immunization setDoseQuantity(SimpleQuantity value) { 2076 this.doseQuantity = value; 2077 return this; 2078 } 2079 2080 /** 2081 * @return {@link #note} (Extra information about the immunization that is not 2082 * conveyed by the other attributes.) 2083 */ 2084 public List<Annotation> getNote() { 2085 if (this.note == null) 2086 this.note = new ArrayList<Annotation>(); 2087 return this.note; 2088 } 2089 2090 public boolean hasNote() { 2091 if (this.note == null) 2092 return false; 2093 for (Annotation item : this.note) 2094 if (!item.isEmpty()) 2095 return true; 2096 return false; 2097 } 2098 2099 /** 2100 * @return {@link #note} (Extra information about the immunization that is not 2101 * conveyed by the other attributes.) 2102 */ 2103 // syntactic sugar 2104 public Annotation addNote() { // 3 2105 Annotation t = new Annotation(); 2106 if (this.note == null) 2107 this.note = new ArrayList<Annotation>(); 2108 this.note.add(t); 2109 return t; 2110 } 2111 2112 // syntactic sugar 2113 public Immunization addNote(Annotation t) { // 3 2114 if (t == null) 2115 return this; 2116 if (this.note == null) 2117 this.note = new ArrayList<Annotation>(); 2118 this.note.add(t); 2119 return this; 2120 } 2121 2122 /** 2123 * @return {@link #explanation} (Reasons why a vaccine was or was not 2124 * administered.) 2125 */ 2126 public ImmunizationExplanationComponent getExplanation() { 2127 if (this.explanation == null) 2128 if (Configuration.errorOnAutoCreate()) 2129 throw new Error("Attempt to auto-create Immunization.explanation"); 2130 else if (Configuration.doAutoCreate()) 2131 this.explanation = new ImmunizationExplanationComponent(); // cc 2132 return this.explanation; 2133 } 2134 2135 public boolean hasExplanation() { 2136 return this.explanation != null && !this.explanation.isEmpty(); 2137 } 2138 2139 /** 2140 * @param value {@link #explanation} (Reasons why a vaccine was or was not 2141 * administered.) 2142 */ 2143 public Immunization setExplanation(ImmunizationExplanationComponent value) { 2144 this.explanation = value; 2145 return this; 2146 } 2147 2148 /** 2149 * @return {@link #reaction} (Categorical data indicating that an adverse event 2150 * is associated in time to an immunization.) 2151 */ 2152 public List<ImmunizationReactionComponent> getReaction() { 2153 if (this.reaction == null) 2154 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 2155 return this.reaction; 2156 } 2157 2158 public boolean hasReaction() { 2159 if (this.reaction == null) 2160 return false; 2161 for (ImmunizationReactionComponent item : this.reaction) 2162 if (!item.isEmpty()) 2163 return true; 2164 return false; 2165 } 2166 2167 /** 2168 * @return {@link #reaction} (Categorical data indicating that an adverse event 2169 * is associated in time to an immunization.) 2170 */ 2171 // syntactic sugar 2172 public ImmunizationReactionComponent addReaction() { // 3 2173 ImmunizationReactionComponent t = new ImmunizationReactionComponent(); 2174 if (this.reaction == null) 2175 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 2176 this.reaction.add(t); 2177 return t; 2178 } 2179 2180 // syntactic sugar 2181 public Immunization addReaction(ImmunizationReactionComponent t) { // 3 2182 if (t == null) 2183 return this; 2184 if (this.reaction == null) 2185 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 2186 this.reaction.add(t); 2187 return this; 2188 } 2189 2190 /** 2191 * @return {@link #vaccinationProtocol} (Contains information about the 2192 * protocol(s) under which the vaccine was administered.) 2193 */ 2194 public List<ImmunizationVaccinationProtocolComponent> getVaccinationProtocol() { 2195 if (this.vaccinationProtocol == null) 2196 this.vaccinationProtocol = new ArrayList<ImmunizationVaccinationProtocolComponent>(); 2197 return this.vaccinationProtocol; 2198 } 2199 2200 public boolean hasVaccinationProtocol() { 2201 if (this.vaccinationProtocol == null) 2202 return false; 2203 for (ImmunizationVaccinationProtocolComponent item : this.vaccinationProtocol) 2204 if (!item.isEmpty()) 2205 return true; 2206 return false; 2207 } 2208 2209 /** 2210 * @return {@link #vaccinationProtocol} (Contains information about the 2211 * protocol(s) under which the vaccine was administered.) 2212 */ 2213 // syntactic sugar 2214 public ImmunizationVaccinationProtocolComponent addVaccinationProtocol() { // 3 2215 ImmunizationVaccinationProtocolComponent t = new ImmunizationVaccinationProtocolComponent(); 2216 if (this.vaccinationProtocol == null) 2217 this.vaccinationProtocol = new ArrayList<ImmunizationVaccinationProtocolComponent>(); 2218 this.vaccinationProtocol.add(t); 2219 return t; 2220 } 2221 2222 // syntactic sugar 2223 public Immunization addVaccinationProtocol(ImmunizationVaccinationProtocolComponent t) { // 3 2224 if (t == null) 2225 return this; 2226 if (this.vaccinationProtocol == null) 2227 this.vaccinationProtocol = new ArrayList<ImmunizationVaccinationProtocolComponent>(); 2228 this.vaccinationProtocol.add(t); 2229 return this; 2230 } 2231 2232 protected void listChildren(List<Property> childrenList) { 2233 super.listChildren(childrenList); 2234 childrenList.add(new Property("identifier", "Identifier", 2235 "A unique identifier assigned to this immunization record.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2236 childrenList.add(new Property("status", "code", "Indicates the current status of the vaccination event.", 0, 2237 java.lang.Integer.MAX_VALUE, status)); 2238 childrenList.add(new Property("date", "dateTime", "Date vaccine administered or was to be administered.", 0, 2239 java.lang.Integer.MAX_VALUE, date)); 2240 childrenList.add(new Property("vaccineCode", "CodeableConcept", 2241 "Vaccine that was administered or was to be administered.", 0, java.lang.Integer.MAX_VALUE, vaccineCode)); 2242 childrenList.add(new Property("patient", "Reference(Patient)", 2243 "The patient who either received or did not receive the immunization.", 0, java.lang.Integer.MAX_VALUE, 2244 patient)); 2245 childrenList.add(new Property("wasNotGiven", "boolean", "Indicates if the vaccination was or was not given.", 0, 2246 java.lang.Integer.MAX_VALUE, wasNotGiven)); 2247 childrenList.add(new Property("reported", "boolean", 2248 "True if this administration was reported rather than directly administered.", 0, java.lang.Integer.MAX_VALUE, 2249 reported)); 2250 childrenList.add(new Property("performer", "Reference(Practitioner)", "Clinician who administered the vaccine.", 0, 2251 java.lang.Integer.MAX_VALUE, performer)); 2252 childrenList.add(new Property("requester", "Reference(Practitioner)", "Clinician who ordered the vaccination.", 0, 2253 java.lang.Integer.MAX_VALUE, requester)); 2254 childrenList.add(new Property("encounter", "Reference(Encounter)", 2255 "The visit or admission or other contact between patient and health care provider the immunization was performed as part of.", 2256 0, java.lang.Integer.MAX_VALUE, encounter)); 2257 childrenList.add(new Property("manufacturer", "Reference(Organization)", "Name of vaccine manufacturer.", 0, 2258 java.lang.Integer.MAX_VALUE, manufacturer)); 2259 childrenList.add(new Property("location", "Reference(Location)", 2260 "The service delivery location where the vaccine administration occurred.", 0, java.lang.Integer.MAX_VALUE, 2261 location)); 2262 childrenList.add(new Property("lotNumber", "string", "Lot number of the vaccine product.", 0, 2263 java.lang.Integer.MAX_VALUE, lotNumber)); 2264 childrenList.add(new Property("expirationDate", "date", "Date vaccine batch expires.", 0, 2265 java.lang.Integer.MAX_VALUE, expirationDate)); 2266 childrenList.add(new Property("site", "CodeableConcept", "Body site where vaccine was administered.", 0, 2267 java.lang.Integer.MAX_VALUE, site)); 2268 childrenList.add(new Property("route", "CodeableConcept", 2269 "The path by which the vaccine product is taken into the body.", 0, java.lang.Integer.MAX_VALUE, route)); 2270 childrenList.add(new Property("doseQuantity", "SimpleQuantity", 2271 "The quantity of vaccine product that was administered.", 0, java.lang.Integer.MAX_VALUE, doseQuantity)); 2272 childrenList.add(new Property("note", "Annotation", 2273 "Extra information about the immunization that is not conveyed by the other attributes.", 0, 2274 java.lang.Integer.MAX_VALUE, note)); 2275 childrenList.add(new Property("explanation", "", "Reasons why a vaccine was or was not administered.", 0, 2276 java.lang.Integer.MAX_VALUE, explanation)); 2277 childrenList.add(new Property("reaction", "", 2278 "Categorical data indicating that an adverse event is associated in time to an immunization.", 0, 2279 java.lang.Integer.MAX_VALUE, reaction)); 2280 childrenList.add(new Property("vaccinationProtocol", "", 2281 "Contains information about the protocol(s) under which the vaccine was administered.", 0, 2282 java.lang.Integer.MAX_VALUE, vaccinationProtocol)); 2283 } 2284 2285 @Override 2286 public void setProperty(String name, Base value) throws FHIRException { 2287 if (name.equals("identifier")) 2288 this.getIdentifier().add(castToIdentifier(value)); 2289 else if (name.equals("status")) 2290 this.status = castToCode(value); // CodeType 2291 else if (name.equals("date")) 2292 this.date = castToDateTime(value); // DateTimeType 2293 else if (name.equals("vaccineCode")) 2294 this.vaccineCode = castToCodeableConcept(value); // CodeableConcept 2295 else if (name.equals("patient")) 2296 this.patient = castToReference(value); // Reference 2297 else if (name.equals("wasNotGiven")) 2298 this.wasNotGiven = castToBoolean(value); // BooleanType 2299 else if (name.equals("reported")) 2300 this.reported = castToBoolean(value); // BooleanType 2301 else if (name.equals("performer")) 2302 this.performer = castToReference(value); // Reference 2303 else if (name.equals("requester")) 2304 this.requester = castToReference(value); // Reference 2305 else if (name.equals("encounter")) 2306 this.encounter = castToReference(value); // Reference 2307 else if (name.equals("manufacturer")) 2308 this.manufacturer = castToReference(value); // Reference 2309 else if (name.equals("location")) 2310 this.location = castToReference(value); // Reference 2311 else if (name.equals("lotNumber")) 2312 this.lotNumber = castToString(value); // StringType 2313 else if (name.equals("expirationDate")) 2314 this.expirationDate = castToDate(value); // DateType 2315 else if (name.equals("site")) 2316 this.site = castToCodeableConcept(value); // CodeableConcept 2317 else if (name.equals("route")) 2318 this.route = castToCodeableConcept(value); // CodeableConcept 2319 else if (name.equals("doseQuantity")) 2320 this.doseQuantity = castToSimpleQuantity(value); // SimpleQuantity 2321 else if (name.equals("note")) 2322 this.getNote().add(castToAnnotation(value)); 2323 else if (name.equals("explanation")) 2324 this.explanation = (ImmunizationExplanationComponent) value; // ImmunizationExplanationComponent 2325 else if (name.equals("reaction")) 2326 this.getReaction().add((ImmunizationReactionComponent) value); 2327 else if (name.equals("vaccinationProtocol")) 2328 this.getVaccinationProtocol().add((ImmunizationVaccinationProtocolComponent) value); 2329 else 2330 super.setProperty(name, value); 2331 } 2332 2333 @Override 2334 public Base addChild(String name) throws FHIRException { 2335 if (name.equals("identifier")) { 2336 return addIdentifier(); 2337 } else if (name.equals("status")) { 2338 throw new FHIRException("Cannot call addChild on a singleton property Immunization.status"); 2339 } else if (name.equals("date")) { 2340 throw new FHIRException("Cannot call addChild on a singleton property Immunization.date"); 2341 } else if (name.equals("vaccineCode")) { 2342 this.vaccineCode = new CodeableConcept(); 2343 return this.vaccineCode; 2344 } else if (name.equals("patient")) { 2345 this.patient = new Reference(); 2346 return this.patient; 2347 } else if (name.equals("wasNotGiven")) { 2348 throw new FHIRException("Cannot call addChild on a singleton property Immunization.wasNotGiven"); 2349 } else if (name.equals("reported")) { 2350 throw new FHIRException("Cannot call addChild on a singleton property Immunization.reported"); 2351 } else if (name.equals("performer")) { 2352 this.performer = new Reference(); 2353 return this.performer; 2354 } else if (name.equals("requester")) { 2355 this.requester = new Reference(); 2356 return this.requester; 2357 } else if (name.equals("encounter")) { 2358 this.encounter = new Reference(); 2359 return this.encounter; 2360 } else if (name.equals("manufacturer")) { 2361 this.manufacturer = new Reference(); 2362 return this.manufacturer; 2363 } else if (name.equals("location")) { 2364 this.location = new Reference(); 2365 return this.location; 2366 } else if (name.equals("lotNumber")) { 2367 throw new FHIRException("Cannot call addChild on a singleton property Immunization.lotNumber"); 2368 } else if (name.equals("expirationDate")) { 2369 throw new FHIRException("Cannot call addChild on a singleton property Immunization.expirationDate"); 2370 } else if (name.equals("site")) { 2371 this.site = new CodeableConcept(); 2372 return this.site; 2373 } else if (name.equals("route")) { 2374 this.route = new CodeableConcept(); 2375 return this.route; 2376 } else if (name.equals("doseQuantity")) { 2377 this.doseQuantity = new SimpleQuantity(); 2378 return this.doseQuantity; 2379 } else if (name.equals("note")) { 2380 return addNote(); 2381 } else if (name.equals("explanation")) { 2382 this.explanation = new ImmunizationExplanationComponent(); 2383 return this.explanation; 2384 } else if (name.equals("reaction")) { 2385 return addReaction(); 2386 } else if (name.equals("vaccinationProtocol")) { 2387 return addVaccinationProtocol(); 2388 } else 2389 return super.addChild(name); 2390 } 2391 2392 public String fhirType() { 2393 return "Immunization"; 2394 2395 } 2396 2397 public Immunization copy() { 2398 Immunization dst = new Immunization(); 2399 copyValues(dst); 2400 if (identifier != null) { 2401 dst.identifier = new ArrayList<Identifier>(); 2402 for (Identifier i : identifier) 2403 dst.identifier.add(i.copy()); 2404 } 2405 ; 2406 dst.status = status == null ? null : status.copy(); 2407 dst.date = date == null ? null : date.copy(); 2408 dst.vaccineCode = vaccineCode == null ? null : vaccineCode.copy(); 2409 dst.patient = patient == null ? null : patient.copy(); 2410 dst.wasNotGiven = wasNotGiven == null ? null : wasNotGiven.copy(); 2411 dst.reported = reported == null ? null : reported.copy(); 2412 dst.performer = performer == null ? null : performer.copy(); 2413 dst.requester = requester == null ? null : requester.copy(); 2414 dst.encounter = encounter == null ? null : encounter.copy(); 2415 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 2416 dst.location = location == null ? null : location.copy(); 2417 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 2418 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 2419 dst.site = site == null ? null : site.copy(); 2420 dst.route = route == null ? null : route.copy(); 2421 dst.doseQuantity = doseQuantity == null ? null : doseQuantity.copy(); 2422 if (note != null) { 2423 dst.note = new ArrayList<Annotation>(); 2424 for (Annotation i : note) 2425 dst.note.add(i.copy()); 2426 } 2427 ; 2428 dst.explanation = explanation == null ? null : explanation.copy(); 2429 if (reaction != null) { 2430 dst.reaction = new ArrayList<ImmunizationReactionComponent>(); 2431 for (ImmunizationReactionComponent i : reaction) 2432 dst.reaction.add(i.copy()); 2433 } 2434 ; 2435 if (vaccinationProtocol != null) { 2436 dst.vaccinationProtocol = new ArrayList<ImmunizationVaccinationProtocolComponent>(); 2437 for (ImmunizationVaccinationProtocolComponent i : vaccinationProtocol) 2438 dst.vaccinationProtocol.add(i.copy()); 2439 } 2440 ; 2441 return dst; 2442 } 2443 2444 protected Immunization typedCopy() { 2445 return copy(); 2446 } 2447 2448 @Override 2449 public boolean equalsDeep(Base other) { 2450 if (!super.equalsDeep(other)) 2451 return false; 2452 if (!(other instanceof Immunization)) 2453 return false; 2454 Immunization o = (Immunization) other; 2455 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2456 && compareDeep(date, o.date, true) && compareDeep(vaccineCode, o.vaccineCode, true) 2457 && compareDeep(patient, o.patient, true) && compareDeep(wasNotGiven, o.wasNotGiven, true) 2458 && compareDeep(reported, o.reported, true) && compareDeep(performer, o.performer, true) 2459 && compareDeep(requester, o.requester, true) && compareDeep(encounter, o.encounter, true) 2460 && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(location, o.location, true) 2461 && compareDeep(lotNumber, o.lotNumber, true) && compareDeep(expirationDate, o.expirationDate, true) 2462 && compareDeep(site, o.site, true) && compareDeep(route, o.route, true) 2463 && compareDeep(doseQuantity, o.doseQuantity, true) && compareDeep(note, o.note, true) 2464 && compareDeep(explanation, o.explanation, true) && compareDeep(reaction, o.reaction, true) 2465 && compareDeep(vaccinationProtocol, o.vaccinationProtocol, true); 2466 } 2467 2468 @Override 2469 public boolean equalsShallow(Base other) { 2470 if (!super.equalsShallow(other)) 2471 return false; 2472 if (!(other instanceof Immunization)) 2473 return false; 2474 Immunization o = (Immunization) other; 2475 return compareValues(status, o.status, true) && compareValues(date, o.date, true) 2476 && compareValues(wasNotGiven, o.wasNotGiven, true) && compareValues(reported, o.reported, true) 2477 && compareValues(lotNumber, o.lotNumber, true) && compareValues(expirationDate, o.expirationDate, true); 2478 } 2479 2480 public boolean isEmpty() { 2481 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (status == null || status.isEmpty()) 2482 && (date == null || date.isEmpty()) && (vaccineCode == null || vaccineCode.isEmpty()) 2483 && (patient == null || patient.isEmpty()) && (wasNotGiven == null || wasNotGiven.isEmpty()) 2484 && (reported == null || reported.isEmpty()) && (performer == null || performer.isEmpty()) 2485 && (requester == null || requester.isEmpty()) && (encounter == null || encounter.isEmpty()) 2486 && (manufacturer == null || manufacturer.isEmpty()) && (location == null || location.isEmpty()) 2487 && (lotNumber == null || lotNumber.isEmpty()) && (expirationDate == null || expirationDate.isEmpty()) 2488 && (site == null || site.isEmpty()) && (route == null || route.isEmpty()) 2489 && (doseQuantity == null || doseQuantity.isEmpty()) && (note == null || note.isEmpty()) 2490 && (explanation == null || explanation.isEmpty()) && (reaction == null || reaction.isEmpty()) 2491 && (vaccinationProtocol == null || vaccinationProtocol.isEmpty()); 2492 } 2493 2494 @Override 2495 public ResourceType getResourceType() { 2496 return ResourceType.Immunization; 2497 } 2498 2499 @SearchParamDefinition(name = "date", path = "Immunization.date", description = "Vaccination (non)-Administration Date", type = "date") 2500 public static final String SP_DATE = "date"; 2501 @SearchParamDefinition(name = "requester", path = "Immunization.requester", description = "The practitioner who ordered the vaccination", type = "reference") 2502 public static final String SP_REQUESTER = "requester"; 2503 @SearchParamDefinition(name = "identifier", path = "Immunization.identifier", description = "Business identifier", type = "token") 2504 public static final String SP_IDENTIFIER = "identifier"; 2505 @SearchParamDefinition(name = "reason", path = "Immunization.explanation.reason", description = "Why immunization occurred", type = "token") 2506 public static final String SP_REASON = "reason"; 2507 @SearchParamDefinition(name = "performer", path = "Immunization.performer", description = "The practitioner who administered the vaccination", type = "reference") 2508 public static final String SP_PERFORMER = "performer"; 2509 @SearchParamDefinition(name = "reaction", path = "Immunization.reaction.detail", description = "Additional information on reaction", type = "reference") 2510 public static final String SP_REACTION = "reaction"; 2511 @SearchParamDefinition(name = "lot-number", path = "Immunization.lotNumber", description = "Vaccine Lot Number", type = "string") 2512 public static final String SP_LOTNUMBER = "lot-number"; 2513 @SearchParamDefinition(name = "notgiven", path = "Immunization.wasNotGiven", description = "Administrations which were not given", type = "token") 2514 public static final String SP_NOTGIVEN = "notgiven"; 2515 @SearchParamDefinition(name = "manufacturer", path = "Immunization.manufacturer", description = "Vaccine Manufacturer", type = "reference") 2516 public static final String SP_MANUFACTURER = "manufacturer"; 2517 @SearchParamDefinition(name = "dose-sequence", path = "Immunization.vaccinationProtocol.doseSequence", description = "Dose number within series", type = "number") 2518 public static final String SP_DOSESEQUENCE = "dose-sequence"; 2519 @SearchParamDefinition(name = "patient", path = "Immunization.patient", description = "The patient for the vaccination record", type = "reference") 2520 public static final String SP_PATIENT = "patient"; 2521 @SearchParamDefinition(name = "vaccine-code", path = "Immunization.vaccineCode", description = "Vaccine Product Administered", type = "token") 2522 public static final String SP_VACCINECODE = "vaccine-code"; 2523 @SearchParamDefinition(name = "reason-not-given", path = "Immunization.explanation.reasonNotGiven", description = "Explanation of reason vaccination was not administered", type = "token") 2524 public static final String SP_REASONNOTGIVEN = "reason-not-given"; 2525 @SearchParamDefinition(name = "location", path = "Immunization.location", description = "The service delivery location or facility in which the vaccine was / was to be administered", type = "reference") 2526 public static final String SP_LOCATION = "location"; 2527 @SearchParamDefinition(name = "reaction-date", path = "Immunization.reaction.date", description = "When reaction started", type = "date") 2528 public static final String SP_REACTIONDATE = "reaction-date"; 2529 @SearchParamDefinition(name = "status", path = "Immunization.status", description = "Immunization event status", type = "token") 2530 public static final String SP_STATUS = "status"; 2531 2532}