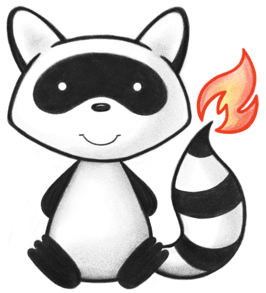
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * A patient's point-in-time immunization and recommendation (i.e. forecasting a 048 * patient's immunization eligibility according to a published schedule) with 049 * optional supporting justification. 050 */ 051@ResourceDef(name = "ImmunizationRecommendation", profile = "http://hl7.org/fhir/Profile/ImmunizationRecommendation") 052public class ImmunizationRecommendation extends DomainResource { 053 054 @Block() 055 public static class ImmunizationRecommendationRecommendationComponent extends BackboneElement 056 implements IBaseBackboneElement { 057 /** 058 * The date the immunization recommendation was created. 059 */ 060 @Child(name = "date", type = { DateTimeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 061 @Description(shortDefinition = "Date recommendation created", formalDefinition = "The date the immunization recommendation was created.") 062 protected DateTimeType date; 063 064 /** 065 * Vaccine that pertains to the recommendation. 066 */ 067 @Child(name = "vaccineCode", type = { 068 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 069 @Description(shortDefinition = "Vaccine recommendation applies to", formalDefinition = "Vaccine that pertains to the recommendation.") 070 protected CodeableConcept vaccineCode; 071 072 /** 073 * This indicates the next recommended dose number (e.g. dose 2 is the next 074 * recommended dose). 075 */ 076 @Child(name = "doseNumber", type = { 077 PositiveIntType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 078 @Description(shortDefinition = "Recommended dose number", formalDefinition = "This indicates the next recommended dose number (e.g. dose 2 is the next recommended dose).") 079 protected PositiveIntType doseNumber; 080 081 /** 082 * Vaccine administration status. 083 */ 084 @Child(name = "forecastStatus", type = { 085 CodeableConcept.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 086 @Description(shortDefinition = "Vaccine administration status", formalDefinition = "Vaccine administration status.") 087 protected CodeableConcept forecastStatus; 088 089 /** 090 * Vaccine date recommendations. For example, earliest date to administer, 091 * latest date to administer, etc. 092 */ 093 @Child(name = "dateCriterion", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 094 @Description(shortDefinition = "Dates governing proposed immunization", formalDefinition = "Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc.") 095 protected List<ImmunizationRecommendationRecommendationDateCriterionComponent> dateCriterion; 096 097 /** 098 * Contains information about the protocol under which the vaccine was 099 * administered. 100 */ 101 @Child(name = "protocol", type = {}, order = 6, min = 0, max = 1, modifier = false, summary = false) 102 @Description(shortDefinition = "Protocol used by recommendation", formalDefinition = "Contains information about the protocol under which the vaccine was administered.") 103 protected ImmunizationRecommendationRecommendationProtocolComponent protocol; 104 105 /** 106 * Immunization event history that supports the status and recommendation. 107 */ 108 @Child(name = "supportingImmunization", type = { 109 Immunization.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 110 @Description(shortDefinition = "Past immunizations supporting recommendation", formalDefinition = "Immunization event history that supports the status and recommendation.") 111 protected List<Reference> supportingImmunization; 112 /** 113 * The actual objects that are the target of the reference (Immunization event 114 * history that supports the status and recommendation.) 115 */ 116 protected List<Immunization> supportingImmunizationTarget; 117 118 /** 119 * Patient Information that supports the status and recommendation. This 120 * includes patient observations, adverse reactions and allergy/intolerance 121 * information. 122 */ 123 @Child(name = "supportingPatientInformation", type = { Observation.class, 124 AllergyIntolerance.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 125 @Description(shortDefinition = "Patient observations supporting recommendation", formalDefinition = "Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information.") 126 protected List<Reference> supportingPatientInformation; 127 /** 128 * The actual objects that are the target of the reference (Patient Information 129 * that supports the status and recommendation. This includes patient 130 * observations, adverse reactions and allergy/intolerance information.) 131 */ 132 protected List<Resource> supportingPatientInformationTarget; 133 134 private static final long serialVersionUID = 1501347482L; 135 136 /* 137 * Constructor 138 */ 139 public ImmunizationRecommendationRecommendationComponent() { 140 super(); 141 } 142 143 /* 144 * Constructor 145 */ 146 public ImmunizationRecommendationRecommendationComponent(DateTimeType date, CodeableConcept vaccineCode, 147 CodeableConcept forecastStatus) { 148 super(); 149 this.date = date; 150 this.vaccineCode = vaccineCode; 151 this.forecastStatus = forecastStatus; 152 } 153 154 /** 155 * @return {@link #date} (The date the immunization recommendation was 156 * created.). This is the underlying object with id, value and 157 * extensions. The accessor "getDate" gives direct access to the value 158 */ 159 public DateTimeType getDateElement() { 160 if (this.date == null) 161 if (Configuration.errorOnAutoCreate()) 162 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.date"); 163 else if (Configuration.doAutoCreate()) 164 this.date = new DateTimeType(); // bb 165 return this.date; 166 } 167 168 public boolean hasDateElement() { 169 return this.date != null && !this.date.isEmpty(); 170 } 171 172 public boolean hasDate() { 173 return this.date != null && !this.date.isEmpty(); 174 } 175 176 /** 177 * @param value {@link #date} (The date the immunization recommendation was 178 * created.). This is the underlying object with id, value and 179 * extensions. The accessor "getDate" gives direct access to the 180 * value 181 */ 182 public ImmunizationRecommendationRecommendationComponent setDateElement(DateTimeType value) { 183 this.date = value; 184 return this; 185 } 186 187 /** 188 * @return The date the immunization recommendation was created. 189 */ 190 public Date getDate() { 191 return this.date == null ? null : this.date.getValue(); 192 } 193 194 /** 195 * @param value The date the immunization recommendation was created. 196 */ 197 public ImmunizationRecommendationRecommendationComponent setDate(Date value) { 198 if (this.date == null) 199 this.date = new DateTimeType(); 200 this.date.setValue(value); 201 return this; 202 } 203 204 /** 205 * @return {@link #vaccineCode} (Vaccine that pertains to the recommendation.) 206 */ 207 public CodeableConcept getVaccineCode() { 208 if (this.vaccineCode == null) 209 if (Configuration.errorOnAutoCreate()) 210 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.vaccineCode"); 211 else if (Configuration.doAutoCreate()) 212 this.vaccineCode = new CodeableConcept(); // cc 213 return this.vaccineCode; 214 } 215 216 public boolean hasVaccineCode() { 217 return this.vaccineCode != null && !this.vaccineCode.isEmpty(); 218 } 219 220 /** 221 * @param value {@link #vaccineCode} (Vaccine that pertains to the 222 * recommendation.) 223 */ 224 public ImmunizationRecommendationRecommendationComponent setVaccineCode(CodeableConcept value) { 225 this.vaccineCode = value; 226 return this; 227 } 228 229 /** 230 * @return {@link #doseNumber} (This indicates the next recommended dose number 231 * (e.g. dose 2 is the next recommended dose).). This is the underlying 232 * object with id, value and extensions. The accessor "getDoseNumber" 233 * gives direct access to the value 234 */ 235 public PositiveIntType getDoseNumberElement() { 236 if (this.doseNumber == null) 237 if (Configuration.errorOnAutoCreate()) 238 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.doseNumber"); 239 else if (Configuration.doAutoCreate()) 240 this.doseNumber = new PositiveIntType(); // bb 241 return this.doseNumber; 242 } 243 244 public boolean hasDoseNumberElement() { 245 return this.doseNumber != null && !this.doseNumber.isEmpty(); 246 } 247 248 public boolean hasDoseNumber() { 249 return this.doseNumber != null && !this.doseNumber.isEmpty(); 250 } 251 252 /** 253 * @param value {@link #doseNumber} (This indicates the next recommended dose 254 * number (e.g. dose 2 is the next recommended dose).). This is the 255 * underlying object with id, value and extensions. The accessor 256 * "getDoseNumber" gives direct access to the value 257 */ 258 public ImmunizationRecommendationRecommendationComponent setDoseNumberElement(PositiveIntType value) { 259 this.doseNumber = value; 260 return this; 261 } 262 263 /** 264 * @return This indicates the next recommended dose number (e.g. dose 2 is the 265 * next recommended dose). 266 */ 267 public int getDoseNumber() { 268 return this.doseNumber == null || this.doseNumber.isEmpty() ? 0 : this.doseNumber.getValue(); 269 } 270 271 /** 272 * @param value This indicates the next recommended dose number (e.g. dose 2 is 273 * the next recommended dose). 274 */ 275 public ImmunizationRecommendationRecommendationComponent setDoseNumber(int value) { 276 if (this.doseNumber == null) 277 this.doseNumber = new PositiveIntType(); 278 this.doseNumber.setValue(value); 279 return this; 280 } 281 282 /** 283 * @return {@link #forecastStatus} (Vaccine administration status.) 284 */ 285 public CodeableConcept getForecastStatus() { 286 if (this.forecastStatus == null) 287 if (Configuration.errorOnAutoCreate()) 288 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.forecastStatus"); 289 else if (Configuration.doAutoCreate()) 290 this.forecastStatus = new CodeableConcept(); // cc 291 return this.forecastStatus; 292 } 293 294 public boolean hasForecastStatus() { 295 return this.forecastStatus != null && !this.forecastStatus.isEmpty(); 296 } 297 298 /** 299 * @param value {@link #forecastStatus} (Vaccine administration status.) 300 */ 301 public ImmunizationRecommendationRecommendationComponent setForecastStatus(CodeableConcept value) { 302 this.forecastStatus = value; 303 return this; 304 } 305 306 /** 307 * @return {@link #dateCriterion} (Vaccine date recommendations. For example, 308 * earliest date to administer, latest date to administer, etc.) 309 */ 310 public List<ImmunizationRecommendationRecommendationDateCriterionComponent> getDateCriterion() { 311 if (this.dateCriterion == null) 312 this.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 313 return this.dateCriterion; 314 } 315 316 public boolean hasDateCriterion() { 317 if (this.dateCriterion == null) 318 return false; 319 for (ImmunizationRecommendationRecommendationDateCriterionComponent item : this.dateCriterion) 320 if (!item.isEmpty()) 321 return true; 322 return false; 323 } 324 325 /** 326 * @return {@link #dateCriterion} (Vaccine date recommendations. For example, 327 * earliest date to administer, latest date to administer, etc.) 328 */ 329 // syntactic sugar 330 public ImmunizationRecommendationRecommendationDateCriterionComponent addDateCriterion() { // 3 331 ImmunizationRecommendationRecommendationDateCriterionComponent t = new ImmunizationRecommendationRecommendationDateCriterionComponent(); 332 if (this.dateCriterion == null) 333 this.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 334 this.dateCriterion.add(t); 335 return t; 336 } 337 338 // syntactic sugar 339 public ImmunizationRecommendationRecommendationComponent addDateCriterion( 340 ImmunizationRecommendationRecommendationDateCriterionComponent t) { // 3 341 if (t == null) 342 return this; 343 if (this.dateCriterion == null) 344 this.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 345 this.dateCriterion.add(t); 346 return this; 347 } 348 349 /** 350 * @return {@link #protocol} (Contains information about the protocol under 351 * which the vaccine was administered.) 352 */ 353 public ImmunizationRecommendationRecommendationProtocolComponent getProtocol() { 354 if (this.protocol == null) 355 if (Configuration.errorOnAutoCreate()) 356 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.protocol"); 357 else if (Configuration.doAutoCreate()) 358 this.protocol = new ImmunizationRecommendationRecommendationProtocolComponent(); // cc 359 return this.protocol; 360 } 361 362 public boolean hasProtocol() { 363 return this.protocol != null && !this.protocol.isEmpty(); 364 } 365 366 /** 367 * @param value {@link #protocol} (Contains information about the protocol under 368 * which the vaccine was administered.) 369 */ 370 public ImmunizationRecommendationRecommendationComponent setProtocol( 371 ImmunizationRecommendationRecommendationProtocolComponent value) { 372 this.protocol = value; 373 return this; 374 } 375 376 /** 377 * @return {@link #supportingImmunization} (Immunization event history that 378 * supports the status and recommendation.) 379 */ 380 public List<Reference> getSupportingImmunization() { 381 if (this.supportingImmunization == null) 382 this.supportingImmunization = new ArrayList<Reference>(); 383 return this.supportingImmunization; 384 } 385 386 public boolean hasSupportingImmunization() { 387 if (this.supportingImmunization == null) 388 return false; 389 for (Reference item : this.supportingImmunization) 390 if (!item.isEmpty()) 391 return true; 392 return false; 393 } 394 395 /** 396 * @return {@link #supportingImmunization} (Immunization event history that 397 * supports the status and recommendation.) 398 */ 399 // syntactic sugar 400 public Reference addSupportingImmunization() { // 3 401 Reference t = new Reference(); 402 if (this.supportingImmunization == null) 403 this.supportingImmunization = new ArrayList<Reference>(); 404 this.supportingImmunization.add(t); 405 return t; 406 } 407 408 // syntactic sugar 409 public ImmunizationRecommendationRecommendationComponent addSupportingImmunization(Reference t) { // 3 410 if (t == null) 411 return this; 412 if (this.supportingImmunization == null) 413 this.supportingImmunization = new ArrayList<Reference>(); 414 this.supportingImmunization.add(t); 415 return this; 416 } 417 418 /** 419 * @return {@link #supportingImmunization} (The actual objects that are the 420 * target of the reference. The reference library doesn't populate this, 421 * but you can use this to hold the resources if you resolvethemt. 422 * Immunization event history that supports the status and 423 * recommendation.) 424 */ 425 public List<Immunization> getSupportingImmunizationTarget() { 426 if (this.supportingImmunizationTarget == null) 427 this.supportingImmunizationTarget = new ArrayList<Immunization>(); 428 return this.supportingImmunizationTarget; 429 } 430 431 // syntactic sugar 432 /** 433 * @return {@link #supportingImmunization} (Add an actual object that is the 434 * target of the reference. The reference library doesn't use these, but 435 * you can use this to hold the resources if you resolvethemt. 436 * Immunization event history that supports the status and 437 * recommendation.) 438 */ 439 public Immunization addSupportingImmunizationTarget() { 440 Immunization r = new Immunization(); 441 if (this.supportingImmunizationTarget == null) 442 this.supportingImmunizationTarget = new ArrayList<Immunization>(); 443 this.supportingImmunizationTarget.add(r); 444 return r; 445 } 446 447 /** 448 * @return {@link #supportingPatientInformation} (Patient Information that 449 * supports the status and recommendation. This includes patient 450 * observations, adverse reactions and allergy/intolerance information.) 451 */ 452 public List<Reference> getSupportingPatientInformation() { 453 if (this.supportingPatientInformation == null) 454 this.supportingPatientInformation = new ArrayList<Reference>(); 455 return this.supportingPatientInformation; 456 } 457 458 public boolean hasSupportingPatientInformation() { 459 if (this.supportingPatientInformation == null) 460 return false; 461 for (Reference item : this.supportingPatientInformation) 462 if (!item.isEmpty()) 463 return true; 464 return false; 465 } 466 467 /** 468 * @return {@link #supportingPatientInformation} (Patient Information that 469 * supports the status and recommendation. This includes patient 470 * observations, adverse reactions and allergy/intolerance information.) 471 */ 472 // syntactic sugar 473 public Reference addSupportingPatientInformation() { // 3 474 Reference t = new Reference(); 475 if (this.supportingPatientInformation == null) 476 this.supportingPatientInformation = new ArrayList<Reference>(); 477 this.supportingPatientInformation.add(t); 478 return t; 479 } 480 481 // syntactic sugar 482 public ImmunizationRecommendationRecommendationComponent addSupportingPatientInformation(Reference t) { // 3 483 if (t == null) 484 return this; 485 if (this.supportingPatientInformation == null) 486 this.supportingPatientInformation = new ArrayList<Reference>(); 487 this.supportingPatientInformation.add(t); 488 return this; 489 } 490 491 /** 492 * @return {@link #supportingPatientInformation} (The actual objects that are 493 * the target of the reference. The reference library doesn't populate 494 * this, but you can use this to hold the resources if you resolvethemt. 495 * Patient Information that supports the status and recommendation. This 496 * includes patient observations, adverse reactions and 497 * allergy/intolerance information.) 498 */ 499 public List<Resource> getSupportingPatientInformationTarget() { 500 if (this.supportingPatientInformationTarget == null) 501 this.supportingPatientInformationTarget = new ArrayList<Resource>(); 502 return this.supportingPatientInformationTarget; 503 } 504 505 protected void listChildren(List<Property> childrenList) { 506 super.listChildren(childrenList); 507 childrenList.add(new Property("date", "dateTime", "The date the immunization recommendation was created.", 0, 508 java.lang.Integer.MAX_VALUE, date)); 509 childrenList.add(new Property("vaccineCode", "CodeableConcept", "Vaccine that pertains to the recommendation.", 0, 510 java.lang.Integer.MAX_VALUE, vaccineCode)); 511 childrenList.add(new Property("doseNumber", "positiveInt", 512 "This indicates the next recommended dose number (e.g. dose 2 is the next recommended dose).", 0, 513 java.lang.Integer.MAX_VALUE, doseNumber)); 514 childrenList.add(new Property("forecastStatus", "CodeableConcept", "Vaccine administration status.", 0, 515 java.lang.Integer.MAX_VALUE, forecastStatus)); 516 childrenList.add(new Property("dateCriterion", "", 517 "Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc.", 0, 518 java.lang.Integer.MAX_VALUE, dateCriterion)); 519 childrenList.add(new Property("protocol", "", 520 "Contains information about the protocol under which the vaccine was administered.", 0, 521 java.lang.Integer.MAX_VALUE, protocol)); 522 childrenList.add(new Property("supportingImmunization", "Reference(Immunization)", 523 "Immunization event history that supports the status and recommendation.", 0, java.lang.Integer.MAX_VALUE, 524 supportingImmunization)); 525 childrenList.add(new Property("supportingPatientInformation", "Reference(Observation|AllergyIntolerance)", 526 "Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information.", 527 0, java.lang.Integer.MAX_VALUE, supportingPatientInformation)); 528 } 529 530 @Override 531 public void setProperty(String name, Base value) throws FHIRException { 532 if (name.equals("date")) 533 this.date = castToDateTime(value); // DateTimeType 534 else if (name.equals("vaccineCode")) 535 this.vaccineCode = castToCodeableConcept(value); // CodeableConcept 536 else if (name.equals("doseNumber")) 537 this.doseNumber = castToPositiveInt(value); // PositiveIntType 538 else if (name.equals("forecastStatus")) 539 this.forecastStatus = castToCodeableConcept(value); // CodeableConcept 540 else if (name.equals("dateCriterion")) 541 this.getDateCriterion().add((ImmunizationRecommendationRecommendationDateCriterionComponent) value); 542 else if (name.equals("protocol")) 543 this.protocol = (ImmunizationRecommendationRecommendationProtocolComponent) value; // ImmunizationRecommendationRecommendationProtocolComponent 544 else if (name.equals("supportingImmunization")) 545 this.getSupportingImmunization().add(castToReference(value)); 546 else if (name.equals("supportingPatientInformation")) 547 this.getSupportingPatientInformation().add(castToReference(value)); 548 else 549 super.setProperty(name, value); 550 } 551 552 @Override 553 public Base addChild(String name) throws FHIRException { 554 if (name.equals("date")) { 555 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.date"); 556 } else if (name.equals("vaccineCode")) { 557 this.vaccineCode = new CodeableConcept(); 558 return this.vaccineCode; 559 } else if (name.equals("doseNumber")) { 560 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.doseNumber"); 561 } else if (name.equals("forecastStatus")) { 562 this.forecastStatus = new CodeableConcept(); 563 return this.forecastStatus; 564 } else if (name.equals("dateCriterion")) { 565 return addDateCriterion(); 566 } else if (name.equals("protocol")) { 567 this.protocol = new ImmunizationRecommendationRecommendationProtocolComponent(); 568 return this.protocol; 569 } else if (name.equals("supportingImmunization")) { 570 return addSupportingImmunization(); 571 } else if (name.equals("supportingPatientInformation")) { 572 return addSupportingPatientInformation(); 573 } else 574 return super.addChild(name); 575 } 576 577 public ImmunizationRecommendationRecommendationComponent copy() { 578 ImmunizationRecommendationRecommendationComponent dst = new ImmunizationRecommendationRecommendationComponent(); 579 copyValues(dst); 580 dst.date = date == null ? null : date.copy(); 581 dst.vaccineCode = vaccineCode == null ? null : vaccineCode.copy(); 582 dst.doseNumber = doseNumber == null ? null : doseNumber.copy(); 583 dst.forecastStatus = forecastStatus == null ? null : forecastStatus.copy(); 584 if (dateCriterion != null) { 585 dst.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 586 for (ImmunizationRecommendationRecommendationDateCriterionComponent i : dateCriterion) 587 dst.dateCriterion.add(i.copy()); 588 } 589 ; 590 dst.protocol = protocol == null ? null : protocol.copy(); 591 if (supportingImmunization != null) { 592 dst.supportingImmunization = new ArrayList<Reference>(); 593 for (Reference i : supportingImmunization) 594 dst.supportingImmunization.add(i.copy()); 595 } 596 ; 597 if (supportingPatientInformation != null) { 598 dst.supportingPatientInformation = new ArrayList<Reference>(); 599 for (Reference i : supportingPatientInformation) 600 dst.supportingPatientInformation.add(i.copy()); 601 } 602 ; 603 return dst; 604 } 605 606 @Override 607 public boolean equalsDeep(Base other) { 608 if (!super.equalsDeep(other)) 609 return false; 610 if (!(other instanceof ImmunizationRecommendationRecommendationComponent)) 611 return false; 612 ImmunizationRecommendationRecommendationComponent o = (ImmunizationRecommendationRecommendationComponent) other; 613 return compareDeep(date, o.date, true) && compareDeep(vaccineCode, o.vaccineCode, true) 614 && compareDeep(doseNumber, o.doseNumber, true) && compareDeep(forecastStatus, o.forecastStatus, true) 615 && compareDeep(dateCriterion, o.dateCriterion, true) && compareDeep(protocol, o.protocol, true) 616 && compareDeep(supportingImmunization, o.supportingImmunization, true) 617 && compareDeep(supportingPatientInformation, o.supportingPatientInformation, true); 618 } 619 620 @Override 621 public boolean equalsShallow(Base other) { 622 if (!super.equalsShallow(other)) 623 return false; 624 if (!(other instanceof ImmunizationRecommendationRecommendationComponent)) 625 return false; 626 ImmunizationRecommendationRecommendationComponent o = (ImmunizationRecommendationRecommendationComponent) other; 627 return compareValues(date, o.date, true) && compareValues(doseNumber, o.doseNumber, true); 628 } 629 630 public boolean isEmpty() { 631 return super.isEmpty() && (date == null || date.isEmpty()) && (vaccineCode == null || vaccineCode.isEmpty()) 632 && (doseNumber == null || doseNumber.isEmpty()) && (forecastStatus == null || forecastStatus.isEmpty()) 633 && (dateCriterion == null || dateCriterion.isEmpty()) && (protocol == null || protocol.isEmpty()) 634 && (supportingImmunization == null || supportingImmunization.isEmpty()) 635 && (supportingPatientInformation == null || supportingPatientInformation.isEmpty()); 636 } 637 638 public String fhirType() { 639 return "ImmunizationRecommendation.recommendation"; 640 641 } 642 643 } 644 645 @Block() 646 public static class ImmunizationRecommendationRecommendationDateCriterionComponent extends BackboneElement 647 implements IBaseBackboneElement { 648 /** 649 * Date classification of recommendation. For example, earliest date to give, 650 * latest date to give, etc. 651 */ 652 @Child(name = "code", type = { 653 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 654 @Description(shortDefinition = "Type of date", formalDefinition = "Date classification of recommendation. For example, earliest date to give, latest date to give, etc.") 655 protected CodeableConcept code; 656 657 /** 658 * The date whose meaning is specified by dateCriterion.code. 659 */ 660 @Child(name = "value", type = { 661 DateTimeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 662 @Description(shortDefinition = "Recommended date", formalDefinition = "The date whose meaning is specified by dateCriterion.code.") 663 protected DateTimeType value; 664 665 private static final long serialVersionUID = 1036994566L; 666 667 /* 668 * Constructor 669 */ 670 public ImmunizationRecommendationRecommendationDateCriterionComponent() { 671 super(); 672 } 673 674 /* 675 * Constructor 676 */ 677 public ImmunizationRecommendationRecommendationDateCriterionComponent(CodeableConcept code, DateTimeType value) { 678 super(); 679 this.code = code; 680 this.value = value; 681 } 682 683 /** 684 * @return {@link #code} (Date classification of recommendation. For example, 685 * earliest date to give, latest date to give, etc.) 686 */ 687 public CodeableConcept getCode() { 688 if (this.code == null) 689 if (Configuration.errorOnAutoCreate()) 690 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationDateCriterionComponent.code"); 691 else if (Configuration.doAutoCreate()) 692 this.code = new CodeableConcept(); // cc 693 return this.code; 694 } 695 696 public boolean hasCode() { 697 return this.code != null && !this.code.isEmpty(); 698 } 699 700 /** 701 * @param value {@link #code} (Date classification of recommendation. For 702 * example, earliest date to give, latest date to give, etc.) 703 */ 704 public ImmunizationRecommendationRecommendationDateCriterionComponent setCode(CodeableConcept value) { 705 this.code = value; 706 return this; 707 } 708 709 /** 710 * @return {@link #value} (The date whose meaning is specified by 711 * dateCriterion.code.). This is the underlying object with id, value 712 * and extensions. The accessor "getValue" gives direct access to the 713 * value 714 */ 715 public DateTimeType getValueElement() { 716 if (this.value == null) 717 if (Configuration.errorOnAutoCreate()) 718 throw new Error( 719 "Attempt to auto-create ImmunizationRecommendationRecommendationDateCriterionComponent.value"); 720 else if (Configuration.doAutoCreate()) 721 this.value = new DateTimeType(); // bb 722 return this.value; 723 } 724 725 public boolean hasValueElement() { 726 return this.value != null && !this.value.isEmpty(); 727 } 728 729 public boolean hasValue() { 730 return this.value != null && !this.value.isEmpty(); 731 } 732 733 /** 734 * @param value {@link #value} (The date whose meaning is specified by 735 * dateCriterion.code.). This is the underlying object with id, 736 * value and extensions. The accessor "getValue" gives direct 737 * access to the value 738 */ 739 public ImmunizationRecommendationRecommendationDateCriterionComponent setValueElement(DateTimeType value) { 740 this.value = value; 741 return this; 742 } 743 744 /** 745 * @return The date whose meaning is specified by dateCriterion.code. 746 */ 747 public Date getValue() { 748 return this.value == null ? null : this.value.getValue(); 749 } 750 751 /** 752 * @param value The date whose meaning is specified by dateCriterion.code. 753 */ 754 public ImmunizationRecommendationRecommendationDateCriterionComponent setValue(Date value) { 755 if (this.value == null) 756 this.value = new DateTimeType(); 757 this.value.setValue(value); 758 return this; 759 } 760 761 protected void listChildren(List<Property> childrenList) { 762 super.listChildren(childrenList); 763 childrenList.add(new Property("code", "CodeableConcept", 764 "Date classification of recommendation. For example, earliest date to give, latest date to give, etc.", 0, 765 java.lang.Integer.MAX_VALUE, code)); 766 childrenList.add(new Property("value", "dateTime", "The date whose meaning is specified by dateCriterion.code.", 767 0, java.lang.Integer.MAX_VALUE, value)); 768 } 769 770 @Override 771 public void setProperty(String name, Base value) throws FHIRException { 772 if (name.equals("code")) 773 this.code = castToCodeableConcept(value); // CodeableConcept 774 else if (name.equals("value")) 775 this.value = castToDateTime(value); // DateTimeType 776 else 777 super.setProperty(name, value); 778 } 779 780 @Override 781 public Base addChild(String name) throws FHIRException { 782 if (name.equals("code")) { 783 this.code = new CodeableConcept(); 784 return this.code; 785 } else if (name.equals("value")) { 786 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.value"); 787 } else 788 return super.addChild(name); 789 } 790 791 public ImmunizationRecommendationRecommendationDateCriterionComponent copy() { 792 ImmunizationRecommendationRecommendationDateCriterionComponent dst = new ImmunizationRecommendationRecommendationDateCriterionComponent(); 793 copyValues(dst); 794 dst.code = code == null ? null : code.copy(); 795 dst.value = value == null ? null : value.copy(); 796 return dst; 797 } 798 799 @Override 800 public boolean equalsDeep(Base other) { 801 if (!super.equalsDeep(other)) 802 return false; 803 if (!(other instanceof ImmunizationRecommendationRecommendationDateCriterionComponent)) 804 return false; 805 ImmunizationRecommendationRecommendationDateCriterionComponent o = (ImmunizationRecommendationRecommendationDateCriterionComponent) other; 806 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 807 } 808 809 @Override 810 public boolean equalsShallow(Base other) { 811 if (!super.equalsShallow(other)) 812 return false; 813 if (!(other instanceof ImmunizationRecommendationRecommendationDateCriterionComponent)) 814 return false; 815 ImmunizationRecommendationRecommendationDateCriterionComponent o = (ImmunizationRecommendationRecommendationDateCriterionComponent) other; 816 return compareValues(value, o.value, true); 817 } 818 819 public boolean isEmpty() { 820 return super.isEmpty() && (code == null || code.isEmpty()) && (value == null || value.isEmpty()); 821 } 822 823 public String fhirType() { 824 return "ImmunizationRecommendation.recommendation.dateCriterion"; 825 826 } 827 828 } 829 830 @Block() 831 public static class ImmunizationRecommendationRecommendationProtocolComponent extends BackboneElement 832 implements IBaseBackboneElement { 833 /** 834 * Indicates the nominal position in a series of the next dose. This is the 835 * recommended dose number as per a specified protocol. 836 */ 837 @Child(name = "doseSequence", type = { 838 IntegerType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 839 @Description(shortDefinition = "Dose number within sequence", formalDefinition = "Indicates the nominal position in a series of the next dose. This is the recommended dose number as per a specified protocol.") 840 protected IntegerType doseSequence; 841 842 /** 843 * Contains the description about the protocol under which the vaccine was 844 * administered. 845 */ 846 @Child(name = "description", type = { 847 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 848 @Description(shortDefinition = "Protocol details", formalDefinition = "Contains the description about the protocol under which the vaccine was administered.") 849 protected StringType description; 850 851 /** 852 * Indicates the authority who published the protocol. For example, ACIP. 853 */ 854 @Child(name = "authority", type = { 855 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 856 @Description(shortDefinition = "Who is responsible for protocol", formalDefinition = "Indicates the authority who published the protocol. For example, ACIP.") 857 protected Reference authority; 858 859 /** 860 * The actual object that is the target of the reference (Indicates the 861 * authority who published the protocol. For example, ACIP.) 862 */ 863 protected Organization authorityTarget; 864 865 /** 866 * One possible path to achieve presumed immunity against a disease - within the 867 * context of an authority. 868 */ 869 @Child(name = "series", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 870 @Description(shortDefinition = "Name of vaccination series", formalDefinition = "One possible path to achieve presumed immunity against a disease - within the context of an authority.") 871 protected StringType series; 872 873 private static final long serialVersionUID = -512702014L; 874 875 /* 876 * Constructor 877 */ 878 public ImmunizationRecommendationRecommendationProtocolComponent() { 879 super(); 880 } 881 882 /** 883 * @return {@link #doseSequence} (Indicates the nominal position in a series of 884 * the next dose. This is the recommended dose number as per a specified 885 * protocol.). This is the underlying object with id, value and 886 * extensions. The accessor "getDoseSequence" gives direct access to the 887 * value 888 */ 889 public IntegerType getDoseSequenceElement() { 890 if (this.doseSequence == null) 891 if (Configuration.errorOnAutoCreate()) 892 throw new Error( 893 "Attempt to auto-create ImmunizationRecommendationRecommendationProtocolComponent.doseSequence"); 894 else if (Configuration.doAutoCreate()) 895 this.doseSequence = new IntegerType(); // bb 896 return this.doseSequence; 897 } 898 899 public boolean hasDoseSequenceElement() { 900 return this.doseSequence != null && !this.doseSequence.isEmpty(); 901 } 902 903 public boolean hasDoseSequence() { 904 return this.doseSequence != null && !this.doseSequence.isEmpty(); 905 } 906 907 /** 908 * @param value {@link #doseSequence} (Indicates the nominal position in a 909 * series of the next dose. This is the recommended dose number as 910 * per a specified protocol.). This is the underlying object with 911 * id, value and extensions. The accessor "getDoseSequence" gives 912 * direct access to the value 913 */ 914 public ImmunizationRecommendationRecommendationProtocolComponent setDoseSequenceElement(IntegerType value) { 915 this.doseSequence = value; 916 return this; 917 } 918 919 /** 920 * @return Indicates the nominal position in a series of the next dose. This is 921 * the recommended dose number as per a specified protocol. 922 */ 923 public int getDoseSequence() { 924 return this.doseSequence == null || this.doseSequence.isEmpty() ? 0 : this.doseSequence.getValue(); 925 } 926 927 /** 928 * @param value Indicates the nominal position in a series of the next dose. 929 * This is the recommended dose number as per a specified protocol. 930 */ 931 public ImmunizationRecommendationRecommendationProtocolComponent setDoseSequence(int value) { 932 if (this.doseSequence == null) 933 this.doseSequence = new IntegerType(); 934 this.doseSequence.setValue(value); 935 return this; 936 } 937 938 /** 939 * @return {@link #description} (Contains the description about the protocol 940 * under which the vaccine was administered.). This is the underlying 941 * object with id, value and extensions. The accessor "getDescription" 942 * gives direct access to the value 943 */ 944 public StringType getDescriptionElement() { 945 if (this.description == null) 946 if (Configuration.errorOnAutoCreate()) 947 throw new Error( 948 "Attempt to auto-create ImmunizationRecommendationRecommendationProtocolComponent.description"); 949 else if (Configuration.doAutoCreate()) 950 this.description = new StringType(); // bb 951 return this.description; 952 } 953 954 public boolean hasDescriptionElement() { 955 return this.description != null && !this.description.isEmpty(); 956 } 957 958 public boolean hasDescription() { 959 return this.description != null && !this.description.isEmpty(); 960 } 961 962 /** 963 * @param value {@link #description} (Contains the description about the 964 * protocol under which the vaccine was administered.). This is the 965 * underlying object with id, value and extensions. The accessor 966 * "getDescription" gives direct access to the value 967 */ 968 public ImmunizationRecommendationRecommendationProtocolComponent setDescriptionElement(StringType value) { 969 this.description = value; 970 return this; 971 } 972 973 /** 974 * @return Contains the description about the protocol under which the vaccine 975 * was administered. 976 */ 977 public String getDescription() { 978 return this.description == null ? null : this.description.getValue(); 979 } 980 981 /** 982 * @param value Contains the description about the protocol under which the 983 * vaccine was administered. 984 */ 985 public ImmunizationRecommendationRecommendationProtocolComponent setDescription(String value) { 986 if (Utilities.noString(value)) 987 this.description = null; 988 else { 989 if (this.description == null) 990 this.description = new StringType(); 991 this.description.setValue(value); 992 } 993 return this; 994 } 995 996 /** 997 * @return {@link #authority} (Indicates the authority who published the 998 * protocol. For example, ACIP.) 999 */ 1000 public Reference getAuthority() { 1001 if (this.authority == null) 1002 if (Configuration.errorOnAutoCreate()) 1003 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationProtocolComponent.authority"); 1004 else if (Configuration.doAutoCreate()) 1005 this.authority = new Reference(); // cc 1006 return this.authority; 1007 } 1008 1009 public boolean hasAuthority() { 1010 return this.authority != null && !this.authority.isEmpty(); 1011 } 1012 1013 /** 1014 * @param value {@link #authority} (Indicates the authority who published the 1015 * protocol. For example, ACIP.) 1016 */ 1017 public ImmunizationRecommendationRecommendationProtocolComponent setAuthority(Reference value) { 1018 this.authority = value; 1019 return this; 1020 } 1021 1022 /** 1023 * @return {@link #authority} The actual object that is the target of the 1024 * reference. The reference library doesn't populate this, but you can 1025 * use it to hold the resource if you resolve it. (Indicates the 1026 * authority who published the protocol. For example, ACIP.) 1027 */ 1028 public Organization getAuthorityTarget() { 1029 if (this.authorityTarget == null) 1030 if (Configuration.errorOnAutoCreate()) 1031 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationProtocolComponent.authority"); 1032 else if (Configuration.doAutoCreate()) 1033 this.authorityTarget = new Organization(); // aa 1034 return this.authorityTarget; 1035 } 1036 1037 /** 1038 * @param value {@link #authority} The actual object that is the target of the 1039 * reference. The reference library doesn't use these, but you can 1040 * use it to hold the resource if you resolve it. (Indicates the 1041 * authority who published the protocol. For example, ACIP.) 1042 */ 1043 public ImmunizationRecommendationRecommendationProtocolComponent setAuthorityTarget(Organization value) { 1044 this.authorityTarget = value; 1045 return this; 1046 } 1047 1048 /** 1049 * @return {@link #series} (One possible path to achieve presumed immunity 1050 * against a disease - within the context of an authority.). This is the 1051 * underlying object with id, value and extensions. The accessor 1052 * "getSeries" gives direct access to the value 1053 */ 1054 public StringType getSeriesElement() { 1055 if (this.series == null) 1056 if (Configuration.errorOnAutoCreate()) 1057 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationProtocolComponent.series"); 1058 else if (Configuration.doAutoCreate()) 1059 this.series = new StringType(); // bb 1060 return this.series; 1061 } 1062 1063 public boolean hasSeriesElement() { 1064 return this.series != null && !this.series.isEmpty(); 1065 } 1066 1067 public boolean hasSeries() { 1068 return this.series != null && !this.series.isEmpty(); 1069 } 1070 1071 /** 1072 * @param value {@link #series} (One possible path to achieve presumed immunity 1073 * against a disease - within the context of an authority.). This 1074 * is the underlying object with id, value and extensions. The 1075 * accessor "getSeries" gives direct access to the value 1076 */ 1077 public ImmunizationRecommendationRecommendationProtocolComponent setSeriesElement(StringType value) { 1078 this.series = value; 1079 return this; 1080 } 1081 1082 /** 1083 * @return One possible path to achieve presumed immunity against a disease - 1084 * within the context of an authority. 1085 */ 1086 public String getSeries() { 1087 return this.series == null ? null : this.series.getValue(); 1088 } 1089 1090 /** 1091 * @param value One possible path to achieve presumed immunity against a disease 1092 * - within the context of an authority. 1093 */ 1094 public ImmunizationRecommendationRecommendationProtocolComponent setSeries(String value) { 1095 if (Utilities.noString(value)) 1096 this.series = null; 1097 else { 1098 if (this.series == null) 1099 this.series = new StringType(); 1100 this.series.setValue(value); 1101 } 1102 return this; 1103 } 1104 1105 protected void listChildren(List<Property> childrenList) { 1106 super.listChildren(childrenList); 1107 childrenList.add(new Property("doseSequence", "integer", 1108 "Indicates the nominal position in a series of the next dose. This is the recommended dose number as per a specified protocol.", 1109 0, java.lang.Integer.MAX_VALUE, doseSequence)); 1110 childrenList.add(new Property("description", "string", 1111 "Contains the description about the protocol under which the vaccine was administered.", 0, 1112 java.lang.Integer.MAX_VALUE, description)); 1113 childrenList.add(new Property("authority", "Reference(Organization)", 1114 "Indicates the authority who published the protocol. For example, ACIP.", 0, java.lang.Integer.MAX_VALUE, 1115 authority)); 1116 childrenList.add(new Property("series", "string", 1117 "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1118 java.lang.Integer.MAX_VALUE, series)); 1119 } 1120 1121 @Override 1122 public void setProperty(String name, Base value) throws FHIRException { 1123 if (name.equals("doseSequence")) 1124 this.doseSequence = castToInteger(value); // IntegerType 1125 else if (name.equals("description")) 1126 this.description = castToString(value); // StringType 1127 else if (name.equals("authority")) 1128 this.authority = castToReference(value); // Reference 1129 else if (name.equals("series")) 1130 this.series = castToString(value); // StringType 1131 else 1132 super.setProperty(name, value); 1133 } 1134 1135 @Override 1136 public Base addChild(String name) throws FHIRException { 1137 if (name.equals("doseSequence")) { 1138 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.doseSequence"); 1139 } else if (name.equals("description")) { 1140 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.description"); 1141 } else if (name.equals("authority")) { 1142 this.authority = new Reference(); 1143 return this.authority; 1144 } else if (name.equals("series")) { 1145 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.series"); 1146 } else 1147 return super.addChild(name); 1148 } 1149 1150 public ImmunizationRecommendationRecommendationProtocolComponent copy() { 1151 ImmunizationRecommendationRecommendationProtocolComponent dst = new ImmunizationRecommendationRecommendationProtocolComponent(); 1152 copyValues(dst); 1153 dst.doseSequence = doseSequence == null ? null : doseSequence.copy(); 1154 dst.description = description == null ? null : description.copy(); 1155 dst.authority = authority == null ? null : authority.copy(); 1156 dst.series = series == null ? null : series.copy(); 1157 return dst; 1158 } 1159 1160 @Override 1161 public boolean equalsDeep(Base other) { 1162 if (!super.equalsDeep(other)) 1163 return false; 1164 if (!(other instanceof ImmunizationRecommendationRecommendationProtocolComponent)) 1165 return false; 1166 ImmunizationRecommendationRecommendationProtocolComponent o = (ImmunizationRecommendationRecommendationProtocolComponent) other; 1167 return compareDeep(doseSequence, o.doseSequence, true) && compareDeep(description, o.description, true) 1168 && compareDeep(authority, o.authority, true) && compareDeep(series, o.series, true); 1169 } 1170 1171 @Override 1172 public boolean equalsShallow(Base other) { 1173 if (!super.equalsShallow(other)) 1174 return false; 1175 if (!(other instanceof ImmunizationRecommendationRecommendationProtocolComponent)) 1176 return false; 1177 ImmunizationRecommendationRecommendationProtocolComponent o = (ImmunizationRecommendationRecommendationProtocolComponent) other; 1178 return compareValues(doseSequence, o.doseSequence, true) && compareValues(description, o.description, true) 1179 && compareValues(series, o.series, true); 1180 } 1181 1182 public boolean isEmpty() { 1183 return super.isEmpty() && (doseSequence == null || doseSequence.isEmpty()) 1184 && (description == null || description.isEmpty()) && (authority == null || authority.isEmpty()) 1185 && (series == null || series.isEmpty()); 1186 } 1187 1188 public String fhirType() { 1189 return "ImmunizationRecommendation.recommendation.protocol"; 1190 1191 } 1192 1193 } 1194 1195 /** 1196 * A unique identifier assigned to this particular recommendation record. 1197 */ 1198 @Child(name = "identifier", type = { 1199 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1200 @Description(shortDefinition = "Business identifier", formalDefinition = "A unique identifier assigned to this particular recommendation record.") 1201 protected List<Identifier> identifier; 1202 1203 /** 1204 * The patient for whom the recommendations are for. 1205 */ 1206 @Child(name = "patient", type = { Patient.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1207 @Description(shortDefinition = "Who this profile is for", formalDefinition = "The patient for whom the recommendations are for.") 1208 protected Reference patient; 1209 1210 /** 1211 * The actual object that is the target of the reference (The patient for whom 1212 * the recommendations are for.) 1213 */ 1214 protected Patient patientTarget; 1215 1216 /** 1217 * Vaccine administration recommendations. 1218 */ 1219 @Child(name = "recommendation", type = {}, order = 2, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1220 @Description(shortDefinition = "Vaccine administration recommendations", formalDefinition = "Vaccine administration recommendations.") 1221 protected List<ImmunizationRecommendationRecommendationComponent> recommendation; 1222 1223 private static final long serialVersionUID = 641058495L; 1224 1225 /* 1226 * Constructor 1227 */ 1228 public ImmunizationRecommendation() { 1229 super(); 1230 } 1231 1232 /* 1233 * Constructor 1234 */ 1235 public ImmunizationRecommendation(Reference patient) { 1236 super(); 1237 this.patient = patient; 1238 } 1239 1240 /** 1241 * @return {@link #identifier} (A unique identifier assigned to this particular 1242 * recommendation record.) 1243 */ 1244 public List<Identifier> getIdentifier() { 1245 if (this.identifier == null) 1246 this.identifier = new ArrayList<Identifier>(); 1247 return this.identifier; 1248 } 1249 1250 public boolean hasIdentifier() { 1251 if (this.identifier == null) 1252 return false; 1253 for (Identifier item : this.identifier) 1254 if (!item.isEmpty()) 1255 return true; 1256 return false; 1257 } 1258 1259 /** 1260 * @return {@link #identifier} (A unique identifier assigned to this particular 1261 * recommendation record.) 1262 */ 1263 // syntactic sugar 1264 public Identifier addIdentifier() { // 3 1265 Identifier t = new Identifier(); 1266 if (this.identifier == null) 1267 this.identifier = new ArrayList<Identifier>(); 1268 this.identifier.add(t); 1269 return t; 1270 } 1271 1272 // syntactic sugar 1273 public ImmunizationRecommendation addIdentifier(Identifier t) { // 3 1274 if (t == null) 1275 return this; 1276 if (this.identifier == null) 1277 this.identifier = new ArrayList<Identifier>(); 1278 this.identifier.add(t); 1279 return this; 1280 } 1281 1282 /** 1283 * @return {@link #patient} (The patient for whom the recommendations are for.) 1284 */ 1285 public Reference getPatient() { 1286 if (this.patient == null) 1287 if (Configuration.errorOnAutoCreate()) 1288 throw new Error("Attempt to auto-create ImmunizationRecommendation.patient"); 1289 else if (Configuration.doAutoCreate()) 1290 this.patient = new Reference(); // cc 1291 return this.patient; 1292 } 1293 1294 public boolean hasPatient() { 1295 return this.patient != null && !this.patient.isEmpty(); 1296 } 1297 1298 /** 1299 * @param value {@link #patient} (The patient for whom the recommendations are 1300 * for.) 1301 */ 1302 public ImmunizationRecommendation setPatient(Reference value) { 1303 this.patient = value; 1304 return this; 1305 } 1306 1307 /** 1308 * @return {@link #patient} The actual object that is the target of the 1309 * reference. The reference library doesn't populate this, but you can 1310 * use it to hold the resource if you resolve it. (The patient for whom 1311 * the recommendations are for.) 1312 */ 1313 public Patient getPatientTarget() { 1314 if (this.patientTarget == null) 1315 if (Configuration.errorOnAutoCreate()) 1316 throw new Error("Attempt to auto-create ImmunizationRecommendation.patient"); 1317 else if (Configuration.doAutoCreate()) 1318 this.patientTarget = new Patient(); // aa 1319 return this.patientTarget; 1320 } 1321 1322 /** 1323 * @param value {@link #patient} The actual object that is the target of the 1324 * reference. The reference library doesn't use these, but you can 1325 * use it to hold the resource if you resolve it. (The patient for 1326 * whom the recommendations are for.) 1327 */ 1328 public ImmunizationRecommendation setPatientTarget(Patient value) { 1329 this.patientTarget = value; 1330 return this; 1331 } 1332 1333 /** 1334 * @return {@link #recommendation} (Vaccine administration recommendations.) 1335 */ 1336 public List<ImmunizationRecommendationRecommendationComponent> getRecommendation() { 1337 if (this.recommendation == null) 1338 this.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1339 return this.recommendation; 1340 } 1341 1342 public boolean hasRecommendation() { 1343 if (this.recommendation == null) 1344 return false; 1345 for (ImmunizationRecommendationRecommendationComponent item : this.recommendation) 1346 if (!item.isEmpty()) 1347 return true; 1348 return false; 1349 } 1350 1351 /** 1352 * @return {@link #recommendation} (Vaccine administration recommendations.) 1353 */ 1354 // syntactic sugar 1355 public ImmunizationRecommendationRecommendationComponent addRecommendation() { // 3 1356 ImmunizationRecommendationRecommendationComponent t = new ImmunizationRecommendationRecommendationComponent(); 1357 if (this.recommendation == null) 1358 this.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1359 this.recommendation.add(t); 1360 return t; 1361 } 1362 1363 // syntactic sugar 1364 public ImmunizationRecommendation addRecommendation(ImmunizationRecommendationRecommendationComponent t) { // 3 1365 if (t == null) 1366 return this; 1367 if (this.recommendation == null) 1368 this.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1369 this.recommendation.add(t); 1370 return this; 1371 } 1372 1373 protected void listChildren(List<Property> childrenList) { 1374 super.listChildren(childrenList); 1375 childrenList.add(new Property("identifier", "Identifier", 1376 "A unique identifier assigned to this particular recommendation record.", 0, java.lang.Integer.MAX_VALUE, 1377 identifier)); 1378 childrenList.add(new Property("patient", "Reference(Patient)", "The patient for whom the recommendations are for.", 1379 0, java.lang.Integer.MAX_VALUE, patient)); 1380 childrenList.add(new Property("recommendation", "", "Vaccine administration recommendations.", 0, 1381 java.lang.Integer.MAX_VALUE, recommendation)); 1382 } 1383 1384 @Override 1385 public void setProperty(String name, Base value) throws FHIRException { 1386 if (name.equals("identifier")) 1387 this.getIdentifier().add(castToIdentifier(value)); 1388 else if (name.equals("patient")) 1389 this.patient = castToReference(value); // Reference 1390 else if (name.equals("recommendation")) 1391 this.getRecommendation().add((ImmunizationRecommendationRecommendationComponent) value); 1392 else 1393 super.setProperty(name, value); 1394 } 1395 1396 @Override 1397 public Base addChild(String name) throws FHIRException { 1398 if (name.equals("identifier")) { 1399 return addIdentifier(); 1400 } else if (name.equals("patient")) { 1401 this.patient = new Reference(); 1402 return this.patient; 1403 } else if (name.equals("recommendation")) { 1404 return addRecommendation(); 1405 } else 1406 return super.addChild(name); 1407 } 1408 1409 public String fhirType() { 1410 return "ImmunizationRecommendation"; 1411 1412 } 1413 1414 public ImmunizationRecommendation copy() { 1415 ImmunizationRecommendation dst = new ImmunizationRecommendation(); 1416 copyValues(dst); 1417 if (identifier != null) { 1418 dst.identifier = new ArrayList<Identifier>(); 1419 for (Identifier i : identifier) 1420 dst.identifier.add(i.copy()); 1421 } 1422 ; 1423 dst.patient = patient == null ? null : patient.copy(); 1424 if (recommendation != null) { 1425 dst.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1426 for (ImmunizationRecommendationRecommendationComponent i : recommendation) 1427 dst.recommendation.add(i.copy()); 1428 } 1429 ; 1430 return dst; 1431 } 1432 1433 protected ImmunizationRecommendation typedCopy() { 1434 return copy(); 1435 } 1436 1437 @Override 1438 public boolean equalsDeep(Base other) { 1439 if (!super.equalsDeep(other)) 1440 return false; 1441 if (!(other instanceof ImmunizationRecommendation)) 1442 return false; 1443 ImmunizationRecommendation o = (ImmunizationRecommendation) other; 1444 return compareDeep(identifier, o.identifier, true) && compareDeep(patient, o.patient, true) 1445 && compareDeep(recommendation, o.recommendation, true); 1446 } 1447 1448 @Override 1449 public boolean equalsShallow(Base other) { 1450 if (!super.equalsShallow(other)) 1451 return false; 1452 if (!(other instanceof ImmunizationRecommendation)) 1453 return false; 1454 ImmunizationRecommendation o = (ImmunizationRecommendation) other; 1455 return true; 1456 } 1457 1458 public boolean isEmpty() { 1459 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (patient == null || patient.isEmpty()) 1460 && (recommendation == null || recommendation.isEmpty()); 1461 } 1462 1463 @Override 1464 public ResourceType getResourceType() { 1465 return ResourceType.ImmunizationRecommendation; 1466 } 1467 1468 @SearchParamDefinition(name = "date", path = "ImmunizationRecommendation.recommendation.date", description = "Date recommendation created", type = "date") 1469 public static final String SP_DATE = "date"; 1470 @SearchParamDefinition(name = "identifier", path = "ImmunizationRecommendation.identifier", description = "Business identifier", type = "token") 1471 public static final String SP_IDENTIFIER = "identifier"; 1472 @SearchParamDefinition(name = "dose-sequence", path = "ImmunizationRecommendation.recommendation.protocol.doseSequence", description = "Dose number within sequence", type = "number") 1473 public static final String SP_DOSESEQUENCE = "dose-sequence"; 1474 @SearchParamDefinition(name = "patient", path = "ImmunizationRecommendation.patient", description = "Who this profile is for", type = "reference") 1475 public static final String SP_PATIENT = "patient"; 1476 @SearchParamDefinition(name = "vaccine-type", path = "ImmunizationRecommendation.recommendation.vaccineCode", description = "Vaccine recommendation applies to", type = "token") 1477 public static final String SP_VACCINETYPE = "vaccine-type"; 1478 @SearchParamDefinition(name = "dose-number", path = "ImmunizationRecommendation.recommendation.doseNumber", description = "Recommended dose number", type = "number") 1479 public static final String SP_DOSENUMBER = "dose-number"; 1480 @SearchParamDefinition(name = "information", path = "ImmunizationRecommendation.recommendation.supportingPatientInformation", description = "Patient observations supporting recommendation", type = "reference") 1481 public static final String SP_INFORMATION = "information"; 1482 @SearchParamDefinition(name = "support", path = "ImmunizationRecommendation.recommendation.supportingImmunization", description = "Past immunizations supporting recommendation", type = "reference") 1483 public static final String SP_SUPPORT = "support"; 1484 @SearchParamDefinition(name = "status", path = "ImmunizationRecommendation.recommendation.forecastStatus", description = "Vaccine administration status", type = "token") 1485 public static final String SP_STATUS = "status"; 1486 1487}