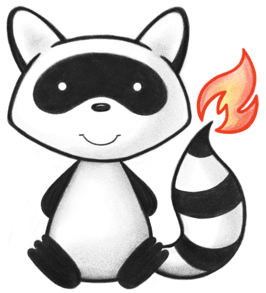
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus; 038import org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory; 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.utilities.Utilities; 047 048/** 049 * A set of rules or how FHIR is used to solve a particular problem. This 050 * resource is used to gather all the parts of an implementation guide into a 051 * logical whole, and to publish a computable definition of all the parts. 052 */ 053@ResourceDef(name = "ImplementationGuide", profile = "http://hl7.org/fhir/Profile/ImplementationGuide") 054public class ImplementationGuide extends DomainResource { 055 056 public enum GuideDependencyType { 057 /** 058 * The guide is referred to by URL. 059 */ 060 REFERENCE, 061 /** 062 * The guide is embedded in this guide when published. 063 */ 064 INCLUSION, 065 /** 066 * added to help the parsers 067 */ 068 NULL; 069 070 public static GuideDependencyType fromCode(String codeString) throws FHIRException { 071 if (codeString == null || "".equals(codeString)) 072 return null; 073 if ("reference".equals(codeString)) 074 return REFERENCE; 075 if ("inclusion".equals(codeString)) 076 return INCLUSION; 077 throw new FHIRException("Unknown GuideDependencyType code '" + codeString + "'"); 078 } 079 080 public String toCode() { 081 switch (this) { 082 case REFERENCE: 083 return "reference"; 084 case INCLUSION: 085 return "inclusion"; 086 case NULL: 087 return null; 088 default: 089 return "?"; 090 } 091 } 092 093 public String getSystem() { 094 switch (this) { 095 case REFERENCE: 096 return "http://hl7.org/fhir/guide-dependency-type"; 097 case INCLUSION: 098 return "http://hl7.org/fhir/guide-dependency-type"; 099 case NULL: 100 return null; 101 default: 102 return "?"; 103 } 104 } 105 106 public String getDefinition() { 107 switch (this) { 108 case REFERENCE: 109 return "The guide is referred to by URL."; 110 case INCLUSION: 111 return "The guide is embedded in this guide when published."; 112 case NULL: 113 return null; 114 default: 115 return "?"; 116 } 117 } 118 119 public String getDisplay() { 120 switch (this) { 121 case REFERENCE: 122 return "Reference"; 123 case INCLUSION: 124 return "Inclusion"; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 } 132 133 public static class GuideDependencyTypeEnumFactory implements EnumFactory<GuideDependencyType> { 134 public GuideDependencyType fromCode(String codeString) throws IllegalArgumentException { 135 if (codeString == null || "".equals(codeString)) 136 if (codeString == null || "".equals(codeString)) 137 return null; 138 if ("reference".equals(codeString)) 139 return GuideDependencyType.REFERENCE; 140 if ("inclusion".equals(codeString)) 141 return GuideDependencyType.INCLUSION; 142 throw new IllegalArgumentException("Unknown GuideDependencyType code '" + codeString + "'"); 143 } 144 145 public Enumeration<GuideDependencyType> fromType(Base code) throws FHIRException { 146 if (code == null || code.isEmpty()) 147 return null; 148 String codeString = ((PrimitiveType) code).asStringValue(); 149 if (codeString == null || "".equals(codeString)) 150 return null; 151 if ("reference".equals(codeString)) 152 return new Enumeration<GuideDependencyType>(this, GuideDependencyType.REFERENCE); 153 if ("inclusion".equals(codeString)) 154 return new Enumeration<GuideDependencyType>(this, GuideDependencyType.INCLUSION); 155 throw new FHIRException("Unknown GuideDependencyType code '" + codeString + "'"); 156 } 157 158 public String toCode(GuideDependencyType code) 159 { 160 if (code == GuideDependencyType.NULL) 161 return null; 162 if (code == GuideDependencyType.REFERENCE) 163 return "reference"; 164 if (code == GuideDependencyType.INCLUSION) 165 return "inclusion"; 166 return "?"; 167 } 168 } 169 170 public enum GuideResourcePurpose { 171 /** 172 * The resource is intended as an example. 173 */ 174 EXAMPLE, 175 /** 176 * The resource defines a value set or concept map used in the implementation 177 * guide. 178 */ 179 TERMINOLOGY, 180 /** 181 * The resource defines a profile (StructureDefinition) that is used in the 182 * implementation guide. 183 */ 184 PROFILE, 185 /** 186 * The resource defines an extension (StructureDefinition) that is used in the 187 * implementation guide. 188 */ 189 EXTENSION, 190 /** 191 * The resource contains a dictionary that is part of the implementation guide. 192 */ 193 DICTIONARY, 194 /** 195 * The resource defines a logical model (in a StructureDefinition) that is used 196 * in the implementation guide. 197 */ 198 LOGICAL, 199 /** 200 * added to help the parsers 201 */ 202 NULL; 203 204 public static GuideResourcePurpose fromCode(String codeString) throws FHIRException { 205 if (codeString == null || "".equals(codeString)) 206 return null; 207 if ("example".equals(codeString)) 208 return EXAMPLE; 209 if ("terminology".equals(codeString)) 210 return TERMINOLOGY; 211 if ("profile".equals(codeString)) 212 return PROFILE; 213 if ("extension".equals(codeString)) 214 return EXTENSION; 215 if ("dictionary".equals(codeString)) 216 return DICTIONARY; 217 if ("logical".equals(codeString)) 218 return LOGICAL; 219 throw new FHIRException("Unknown GuideResourcePurpose code '" + codeString + "'"); 220 } 221 222 public String toCode() { 223 switch (this) { 224 case EXAMPLE: 225 return "example"; 226 case TERMINOLOGY: 227 return "terminology"; 228 case PROFILE: 229 return "profile"; 230 case EXTENSION: 231 return "extension"; 232 case DICTIONARY: 233 return "dictionary"; 234 case LOGICAL: 235 return "logical"; 236 case NULL: 237 return null; 238 default: 239 return "?"; 240 } 241 } 242 243 public String getSystem() { 244 switch (this) { 245 case EXAMPLE: 246 return "http://hl7.org/fhir/guide-resource-purpose"; 247 case TERMINOLOGY: 248 return "http://hl7.org/fhir/guide-resource-purpose"; 249 case PROFILE: 250 return "http://hl7.org/fhir/guide-resource-purpose"; 251 case EXTENSION: 252 return "http://hl7.org/fhir/guide-resource-purpose"; 253 case DICTIONARY: 254 return "http://hl7.org/fhir/guide-resource-purpose"; 255 case LOGICAL: 256 return "http://hl7.org/fhir/guide-resource-purpose"; 257 case NULL: 258 return null; 259 default: 260 return "?"; 261 } 262 } 263 264 public String getDefinition() { 265 switch (this) { 266 case EXAMPLE: 267 return "The resource is intended as an example."; 268 case TERMINOLOGY: 269 return "The resource defines a value set or concept map used in the implementation guide."; 270 case PROFILE: 271 return "The resource defines a profile (StructureDefinition) that is used in the implementation guide."; 272 case EXTENSION: 273 return "The resource defines an extension (StructureDefinition) that is used in the implementation guide."; 274 case DICTIONARY: 275 return "The resource contains a dictionary that is part of the implementation guide."; 276 case LOGICAL: 277 return "The resource defines a logical model (in a StructureDefinition) that is used in the implementation guide."; 278 case NULL: 279 return null; 280 default: 281 return "?"; 282 } 283 } 284 285 public String getDisplay() { 286 switch (this) { 287 case EXAMPLE: 288 return "Example"; 289 case TERMINOLOGY: 290 return "Terminology"; 291 case PROFILE: 292 return "Profile"; 293 case EXTENSION: 294 return "Extension"; 295 case DICTIONARY: 296 return "Dictionary"; 297 case LOGICAL: 298 return "Logical Model"; 299 case NULL: 300 return null; 301 default: 302 return "?"; 303 } 304 } 305 } 306 307 public static class GuideResourcePurposeEnumFactory implements EnumFactory<GuideResourcePurpose> { 308 public GuideResourcePurpose fromCode(String codeString) throws IllegalArgumentException { 309 if (codeString == null || "".equals(codeString)) 310 if (codeString == null || "".equals(codeString)) 311 return null; 312 if ("example".equals(codeString)) 313 return GuideResourcePurpose.EXAMPLE; 314 if ("terminology".equals(codeString)) 315 return GuideResourcePurpose.TERMINOLOGY; 316 if ("profile".equals(codeString)) 317 return GuideResourcePurpose.PROFILE; 318 if ("extension".equals(codeString)) 319 return GuideResourcePurpose.EXTENSION; 320 if ("dictionary".equals(codeString)) 321 return GuideResourcePurpose.DICTIONARY; 322 if ("logical".equals(codeString)) 323 return GuideResourcePurpose.LOGICAL; 324 throw new IllegalArgumentException("Unknown GuideResourcePurpose code '" + codeString + "'"); 325 } 326 327 public Enumeration<GuideResourcePurpose> fromType(Base code) throws FHIRException { 328 if (code == null || code.isEmpty()) 329 return null; 330 String codeString = ((PrimitiveType) code).asStringValue(); 331 if (codeString == null || "".equals(codeString)) 332 return null; 333 if ("example".equals(codeString)) 334 return new Enumeration<GuideResourcePurpose>(this, GuideResourcePurpose.EXAMPLE); 335 if ("terminology".equals(codeString)) 336 return new Enumeration<GuideResourcePurpose>(this, GuideResourcePurpose.TERMINOLOGY); 337 if ("profile".equals(codeString)) 338 return new Enumeration<GuideResourcePurpose>(this, GuideResourcePurpose.PROFILE); 339 if ("extension".equals(codeString)) 340 return new Enumeration<GuideResourcePurpose>(this, GuideResourcePurpose.EXTENSION); 341 if ("dictionary".equals(codeString)) 342 return new Enumeration<GuideResourcePurpose>(this, GuideResourcePurpose.DICTIONARY); 343 if ("logical".equals(codeString)) 344 return new Enumeration<GuideResourcePurpose>(this, GuideResourcePurpose.LOGICAL); 345 throw new FHIRException("Unknown GuideResourcePurpose code '" + codeString + "'"); 346 } 347 348 public String toCode(GuideResourcePurpose code) 349 { 350 if (code == GuideResourcePurpose.NULL) 351 return null; 352 if (code == GuideResourcePurpose.EXAMPLE) 353 return "example"; 354 if (code == GuideResourcePurpose.TERMINOLOGY) 355 return "terminology"; 356 if (code == GuideResourcePurpose.PROFILE) 357 return "profile"; 358 if (code == GuideResourcePurpose.EXTENSION) 359 return "extension"; 360 if (code == GuideResourcePurpose.DICTIONARY) 361 return "dictionary"; 362 if (code == GuideResourcePurpose.LOGICAL) 363 return "logical"; 364 return "?"; 365 } 366 } 367 368 public enum GuidePageKind { 369 /** 370 * This is a page of content that is included in the implementation guide. It 371 * has no particular function. 372 */ 373 PAGE, 374 /** 375 * This is a page that represents a human readable rendering of an example. 376 */ 377 EXAMPLE, 378 /** 379 * This is a page that represents a list of resources of one or more types. 380 */ 381 LIST, 382 /** 383 * This is a page showing where an included guide is injected. 384 */ 385 INCLUDE, 386 /** 387 * This is a page that lists the resources of a given type, and also creates 388 * pages for all the listed types as other pages in the section. 389 */ 390 DIRECTORY, 391 /** 392 * This is a page that creates the listed resources as a dictionary. 393 */ 394 DICTIONARY, 395 /** 396 * This is a generated page that contains the table of contents. 397 */ 398 TOC, 399 /** 400 * This is a page that represents a presented resource. This is typically used 401 * for generated conformance resource presentations. 402 */ 403 RESOURCE, 404 /** 405 * added to help the parsers 406 */ 407 NULL; 408 409 public static GuidePageKind fromCode(String codeString) throws FHIRException { 410 if (codeString == null || "".equals(codeString)) 411 return null; 412 if ("page".equals(codeString)) 413 return PAGE; 414 if ("example".equals(codeString)) 415 return EXAMPLE; 416 if ("list".equals(codeString)) 417 return LIST; 418 if ("include".equals(codeString)) 419 return INCLUDE; 420 if ("directory".equals(codeString)) 421 return DIRECTORY; 422 if ("dictionary".equals(codeString)) 423 return DICTIONARY; 424 if ("toc".equals(codeString)) 425 return TOC; 426 if ("resource".equals(codeString)) 427 return RESOURCE; 428 throw new FHIRException("Unknown GuidePageKind code '" + codeString + "'"); 429 } 430 431 public String toCode() { 432 switch (this) { 433 case PAGE: 434 return "page"; 435 case EXAMPLE: 436 return "example"; 437 case LIST: 438 return "list"; 439 case INCLUDE: 440 return "include"; 441 case DIRECTORY: 442 return "directory"; 443 case DICTIONARY: 444 return "dictionary"; 445 case TOC: 446 return "toc"; 447 case RESOURCE: 448 return "resource"; 449 case NULL: 450 return null; 451 default: 452 return "?"; 453 } 454 } 455 456 public String getSystem() { 457 switch (this) { 458 case PAGE: 459 return "http://hl7.org/fhir/guide-page-kind"; 460 case EXAMPLE: 461 return "http://hl7.org/fhir/guide-page-kind"; 462 case LIST: 463 return "http://hl7.org/fhir/guide-page-kind"; 464 case INCLUDE: 465 return "http://hl7.org/fhir/guide-page-kind"; 466 case DIRECTORY: 467 return "http://hl7.org/fhir/guide-page-kind"; 468 case DICTIONARY: 469 return "http://hl7.org/fhir/guide-page-kind"; 470 case TOC: 471 return "http://hl7.org/fhir/guide-page-kind"; 472 case RESOURCE: 473 return "http://hl7.org/fhir/guide-page-kind"; 474 case NULL: 475 return null; 476 default: 477 return "?"; 478 } 479 } 480 481 public String getDefinition() { 482 switch (this) { 483 case PAGE: 484 return "This is a page of content that is included in the implementation guide. It has no particular function."; 485 case EXAMPLE: 486 return "This is a page that represents a human readable rendering of an example."; 487 case LIST: 488 return "This is a page that represents a list of resources of one or more types."; 489 case INCLUDE: 490 return "This is a page showing where an included guide is injected."; 491 case DIRECTORY: 492 return "This is a page that lists the resources of a given type, and also creates pages for all the listed types as other pages in the section."; 493 case DICTIONARY: 494 return "This is a page that creates the listed resources as a dictionary."; 495 case TOC: 496 return "This is a generated page that contains the table of contents."; 497 case RESOURCE: 498 return "This is a page that represents a presented resource. This is typically used for generated conformance resource presentations."; 499 case NULL: 500 return null; 501 default: 502 return "?"; 503 } 504 } 505 506 public String getDisplay() { 507 switch (this) { 508 case PAGE: 509 return "Page"; 510 case EXAMPLE: 511 return "Example"; 512 case LIST: 513 return "List"; 514 case INCLUDE: 515 return "Include"; 516 case DIRECTORY: 517 return "Directory"; 518 case DICTIONARY: 519 return "Dictionary"; 520 case TOC: 521 return "Table Of Contents"; 522 case RESOURCE: 523 return "Resource"; 524 case NULL: 525 return null; 526 default: 527 return "?"; 528 } 529 } 530 } 531 532 public static class GuidePageKindEnumFactory implements EnumFactory<GuidePageKind> { 533 public GuidePageKind fromCode(String codeString) throws IllegalArgumentException { 534 if (codeString == null || "".equals(codeString)) 535 if (codeString == null || "".equals(codeString)) 536 return null; 537 if ("page".equals(codeString)) 538 return GuidePageKind.PAGE; 539 if ("example".equals(codeString)) 540 return GuidePageKind.EXAMPLE; 541 if ("list".equals(codeString)) 542 return GuidePageKind.LIST; 543 if ("include".equals(codeString)) 544 return GuidePageKind.INCLUDE; 545 if ("directory".equals(codeString)) 546 return GuidePageKind.DIRECTORY; 547 if ("dictionary".equals(codeString)) 548 return GuidePageKind.DICTIONARY; 549 if ("toc".equals(codeString)) 550 return GuidePageKind.TOC; 551 if ("resource".equals(codeString)) 552 return GuidePageKind.RESOURCE; 553 throw new IllegalArgumentException("Unknown GuidePageKind code '" + codeString + "'"); 554 } 555 556 public Enumeration<GuidePageKind> fromType(Base code) throws FHIRException { 557 if (code == null || code.isEmpty()) 558 return null; 559 String codeString = ((PrimitiveType) code).asStringValue(); 560 if (codeString == null || "".equals(codeString)) 561 return null; 562 if ("page".equals(codeString)) 563 return new Enumeration<GuidePageKind>(this, GuidePageKind.PAGE); 564 if ("example".equals(codeString)) 565 return new Enumeration<GuidePageKind>(this, GuidePageKind.EXAMPLE); 566 if ("list".equals(codeString)) 567 return new Enumeration<GuidePageKind>(this, GuidePageKind.LIST); 568 if ("include".equals(codeString)) 569 return new Enumeration<GuidePageKind>(this, GuidePageKind.INCLUDE); 570 if ("directory".equals(codeString)) 571 return new Enumeration<GuidePageKind>(this, GuidePageKind.DIRECTORY); 572 if ("dictionary".equals(codeString)) 573 return new Enumeration<GuidePageKind>(this, GuidePageKind.DICTIONARY); 574 if ("toc".equals(codeString)) 575 return new Enumeration<GuidePageKind>(this, GuidePageKind.TOC); 576 if ("resource".equals(codeString)) 577 return new Enumeration<GuidePageKind>(this, GuidePageKind.RESOURCE); 578 throw new FHIRException("Unknown GuidePageKind code '" + codeString + "'"); 579 } 580 581 public String toCode(GuidePageKind code) 582 { 583 if (code == GuidePageKind.NULL) 584 return null; 585 if (code == GuidePageKind.PAGE) 586 return "page"; 587 if (code == GuidePageKind.EXAMPLE) 588 return "example"; 589 if (code == GuidePageKind.LIST) 590 return "list"; 591 if (code == GuidePageKind.INCLUDE) 592 return "include"; 593 if (code == GuidePageKind.DIRECTORY) 594 return "directory"; 595 if (code == GuidePageKind.DICTIONARY) 596 return "dictionary"; 597 if (code == GuidePageKind.TOC) 598 return "toc"; 599 if (code == GuidePageKind.RESOURCE) 600 return "resource"; 601 return "?"; 602 } 603 } 604 605 @Block() 606 public static class ImplementationGuideContactComponent extends BackboneElement implements IBaseBackboneElement { 607 /** 608 * The name of an individual to contact regarding the implementation guide. 609 */ 610 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 611 @Description(shortDefinition = "Name of a individual to contact", formalDefinition = "The name of an individual to contact regarding the implementation guide.") 612 protected StringType name; 613 614 /** 615 * Contact details for individual (if a name was provided) or the publisher. 616 */ 617 @Child(name = "telecom", type = { 618 ContactPoint.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 619 @Description(shortDefinition = "Contact details for individual or publisher", formalDefinition = "Contact details for individual (if a name was provided) or the publisher.") 620 protected List<ContactPoint> telecom; 621 622 private static final long serialVersionUID = -1179697803L; 623 624 /* 625 * Constructor 626 */ 627 public ImplementationGuideContactComponent() { 628 super(); 629 } 630 631 /** 632 * @return {@link #name} (The name of an individual to contact regarding the 633 * implementation guide.). This is the underlying object with id, value 634 * and extensions. The accessor "getName" gives direct access to the 635 * value 636 */ 637 public StringType getNameElement() { 638 if (this.name == null) 639 if (Configuration.errorOnAutoCreate()) 640 throw new Error("Attempt to auto-create ImplementationGuideContactComponent.name"); 641 else if (Configuration.doAutoCreate()) 642 this.name = new StringType(); // bb 643 return this.name; 644 } 645 646 public boolean hasNameElement() { 647 return this.name != null && !this.name.isEmpty(); 648 } 649 650 public boolean hasName() { 651 return this.name != null && !this.name.isEmpty(); 652 } 653 654 /** 655 * @param value {@link #name} (The name of an individual to contact regarding 656 * the implementation guide.). This is the underlying object with 657 * id, value and extensions. The accessor "getName" gives direct 658 * access to the value 659 */ 660 public ImplementationGuideContactComponent setNameElement(StringType value) { 661 this.name = value; 662 return this; 663 } 664 665 /** 666 * @return The name of an individual to contact regarding the implementation 667 * guide. 668 */ 669 public String getName() { 670 return this.name == null ? null : this.name.getValue(); 671 } 672 673 /** 674 * @param value The name of an individual to contact regarding the 675 * implementation guide. 676 */ 677 public ImplementationGuideContactComponent setName(String value) { 678 if (Utilities.noString(value)) 679 this.name = null; 680 else { 681 if (this.name == null) 682 this.name = new StringType(); 683 this.name.setValue(value); 684 } 685 return this; 686 } 687 688 /** 689 * @return {@link #telecom} (Contact details for individual (if a name was 690 * provided) or the publisher.) 691 */ 692 public List<ContactPoint> getTelecom() { 693 if (this.telecom == null) 694 this.telecom = new ArrayList<ContactPoint>(); 695 return this.telecom; 696 } 697 698 public boolean hasTelecom() { 699 if (this.telecom == null) 700 return false; 701 for (ContactPoint item : this.telecom) 702 if (!item.isEmpty()) 703 return true; 704 return false; 705 } 706 707 /** 708 * @return {@link #telecom} (Contact details for individual (if a name was 709 * provided) or the publisher.) 710 */ 711 // syntactic sugar 712 public ContactPoint addTelecom() { // 3 713 ContactPoint t = new ContactPoint(); 714 if (this.telecom == null) 715 this.telecom = new ArrayList<ContactPoint>(); 716 this.telecom.add(t); 717 return t; 718 } 719 720 // syntactic sugar 721 public ImplementationGuideContactComponent addTelecom(ContactPoint t) { // 3 722 if (t == null) 723 return this; 724 if (this.telecom == null) 725 this.telecom = new ArrayList<ContactPoint>(); 726 this.telecom.add(t); 727 return this; 728 } 729 730 protected void listChildren(List<Property> childrenList) { 731 super.listChildren(childrenList); 732 childrenList.add( 733 new Property("name", "string", "The name of an individual to contact regarding the implementation guide.", 0, 734 java.lang.Integer.MAX_VALUE, name)); 735 childrenList.add(new Property("telecom", "ContactPoint", 736 "Contact details for individual (if a name was provided) or the publisher.", 0, java.lang.Integer.MAX_VALUE, 737 telecom)); 738 } 739 740 @Override 741 public void setProperty(String name, Base value) throws FHIRException { 742 if (name.equals("name")) 743 this.name = castToString(value); // StringType 744 else if (name.equals("telecom")) 745 this.getTelecom().add(castToContactPoint(value)); 746 else 747 super.setProperty(name, value); 748 } 749 750 @Override 751 public Base addChild(String name) throws FHIRException { 752 if (name.equals("name")) { 753 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.name"); 754 } else if (name.equals("telecom")) { 755 return addTelecom(); 756 } else 757 return super.addChild(name); 758 } 759 760 public ImplementationGuideContactComponent copy() { 761 ImplementationGuideContactComponent dst = new ImplementationGuideContactComponent(); 762 copyValues(dst); 763 dst.name = name == null ? null : name.copy(); 764 if (telecom != null) { 765 dst.telecom = new ArrayList<ContactPoint>(); 766 for (ContactPoint i : telecom) 767 dst.telecom.add(i.copy()); 768 } 769 ; 770 return dst; 771 } 772 773 @Override 774 public boolean equalsDeep(Base other) { 775 if (!super.equalsDeep(other)) 776 return false; 777 if (!(other instanceof ImplementationGuideContactComponent)) 778 return false; 779 ImplementationGuideContactComponent o = (ImplementationGuideContactComponent) other; 780 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 781 } 782 783 @Override 784 public boolean equalsShallow(Base other) { 785 if (!super.equalsShallow(other)) 786 return false; 787 if (!(other instanceof ImplementationGuideContactComponent)) 788 return false; 789 ImplementationGuideContactComponent o = (ImplementationGuideContactComponent) other; 790 return compareValues(name, o.name, true); 791 } 792 793 public boolean isEmpty() { 794 return super.isEmpty() && (name == null || name.isEmpty()) && (telecom == null || telecom.isEmpty()); 795 } 796 797 public String fhirType() { 798 return "ImplementationGuide.contact"; 799 800 } 801 802 } 803 804 @Block() 805 public static class ImplementationGuideDependencyComponent extends BackboneElement implements IBaseBackboneElement { 806 /** 807 * How the dependency is represented when the guide is published. 808 */ 809 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 810 @Description(shortDefinition = "reference | inclusion", formalDefinition = "How the dependency is represented when the guide is published.") 811 protected Enumeration<GuideDependencyType> type; 812 813 /** 814 * Where the dependency is located. 815 */ 816 @Child(name = "uri", type = { UriType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 817 @Description(shortDefinition = "Where to find dependency", formalDefinition = "Where the dependency is located.") 818 protected UriType uri; 819 820 private static final long serialVersionUID = 162447098L; 821 822 /* 823 * Constructor 824 */ 825 public ImplementationGuideDependencyComponent() { 826 super(); 827 } 828 829 /* 830 * Constructor 831 */ 832 public ImplementationGuideDependencyComponent(Enumeration<GuideDependencyType> type, UriType uri) { 833 super(); 834 this.type = type; 835 this.uri = uri; 836 } 837 838 /** 839 * @return {@link #type} (How the dependency is represented when the guide is 840 * published.). This is the underlying object with id, value and 841 * extensions. The accessor "getType" gives direct access to the value 842 */ 843 public Enumeration<GuideDependencyType> getTypeElement() { 844 if (this.type == null) 845 if (Configuration.errorOnAutoCreate()) 846 throw new Error("Attempt to auto-create ImplementationGuideDependencyComponent.type"); 847 else if (Configuration.doAutoCreate()) 848 this.type = new Enumeration<GuideDependencyType>(new GuideDependencyTypeEnumFactory()); // bb 849 return this.type; 850 } 851 852 public boolean hasTypeElement() { 853 return this.type != null && !this.type.isEmpty(); 854 } 855 856 public boolean hasType() { 857 return this.type != null && !this.type.isEmpty(); 858 } 859 860 /** 861 * @param value {@link #type} (How the dependency is represented when the guide 862 * is published.). This is the underlying object with id, value and 863 * extensions. The accessor "getType" gives direct access to the 864 * value 865 */ 866 public ImplementationGuideDependencyComponent setTypeElement(Enumeration<GuideDependencyType> value) { 867 this.type = value; 868 return this; 869 } 870 871 /** 872 * @return How the dependency is represented when the guide is published. 873 */ 874 public GuideDependencyType getType() { 875 return this.type == null ? null : this.type.getValue(); 876 } 877 878 /** 879 * @param value How the dependency is represented when the guide is published. 880 */ 881 public ImplementationGuideDependencyComponent setType(GuideDependencyType value) { 882 if (this.type == null) 883 this.type = new Enumeration<GuideDependencyType>(new GuideDependencyTypeEnumFactory()); 884 this.type.setValue(value); 885 return this; 886 } 887 888 /** 889 * @return {@link #uri} (Where the dependency is located.). This is the 890 * underlying object with id, value and extensions. The accessor 891 * "getUri" gives direct access to the value 892 */ 893 public UriType getUriElement() { 894 if (this.uri == null) 895 if (Configuration.errorOnAutoCreate()) 896 throw new Error("Attempt to auto-create ImplementationGuideDependencyComponent.uri"); 897 else if (Configuration.doAutoCreate()) 898 this.uri = new UriType(); // bb 899 return this.uri; 900 } 901 902 public boolean hasUriElement() { 903 return this.uri != null && !this.uri.isEmpty(); 904 } 905 906 public boolean hasUri() { 907 return this.uri != null && !this.uri.isEmpty(); 908 } 909 910 /** 911 * @param value {@link #uri} (Where the dependency is located.). This is the 912 * underlying object with id, value and extensions. The accessor 913 * "getUri" gives direct access to the value 914 */ 915 public ImplementationGuideDependencyComponent setUriElement(UriType value) { 916 this.uri = value; 917 return this; 918 } 919 920 /** 921 * @return Where the dependency is located. 922 */ 923 public String getUri() { 924 return this.uri == null ? null : this.uri.getValue(); 925 } 926 927 /** 928 * @param value Where the dependency is located. 929 */ 930 public ImplementationGuideDependencyComponent setUri(String value) { 931 if (this.uri == null) 932 this.uri = new UriType(); 933 this.uri.setValue(value); 934 return this; 935 } 936 937 protected void listChildren(List<Property> childrenList) { 938 super.listChildren(childrenList); 939 childrenList.add(new Property("type", "code", "How the dependency is represented when the guide is published.", 0, 940 java.lang.Integer.MAX_VALUE, type)); 941 childrenList 942 .add(new Property("uri", "uri", "Where the dependency is located.", 0, java.lang.Integer.MAX_VALUE, uri)); 943 } 944 945 @Override 946 public void setProperty(String name, Base value) throws FHIRException { 947 if (name.equals("type")) 948 this.type = new GuideDependencyTypeEnumFactory().fromType(value); // Enumeration<GuideDependencyType> 949 else if (name.equals("uri")) 950 this.uri = castToUri(value); // UriType 951 else 952 super.setProperty(name, value); 953 } 954 955 @Override 956 public Base addChild(String name) throws FHIRException { 957 if (name.equals("type")) { 958 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.type"); 959 } else if (name.equals("uri")) { 960 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.uri"); 961 } else 962 return super.addChild(name); 963 } 964 965 public ImplementationGuideDependencyComponent copy() { 966 ImplementationGuideDependencyComponent dst = new ImplementationGuideDependencyComponent(); 967 copyValues(dst); 968 dst.type = type == null ? null : type.copy(); 969 dst.uri = uri == null ? null : uri.copy(); 970 return dst; 971 } 972 973 @Override 974 public boolean equalsDeep(Base other) { 975 if (!super.equalsDeep(other)) 976 return false; 977 if (!(other instanceof ImplementationGuideDependencyComponent)) 978 return false; 979 ImplementationGuideDependencyComponent o = (ImplementationGuideDependencyComponent) other; 980 return compareDeep(type, o.type, true) && compareDeep(uri, o.uri, true); 981 } 982 983 @Override 984 public boolean equalsShallow(Base other) { 985 if (!super.equalsShallow(other)) 986 return false; 987 if (!(other instanceof ImplementationGuideDependencyComponent)) 988 return false; 989 ImplementationGuideDependencyComponent o = (ImplementationGuideDependencyComponent) other; 990 return compareValues(type, o.type, true) && compareValues(uri, o.uri, true); 991 } 992 993 public boolean isEmpty() { 994 return super.isEmpty() && (type == null || type.isEmpty()) && (uri == null || uri.isEmpty()); 995 } 996 997 public String fhirType() { 998 return "ImplementationGuide.dependency"; 999 1000 } 1001 1002 } 1003 1004 @Block() 1005 public static class ImplementationGuidePackageComponent extends BackboneElement implements IBaseBackboneElement { 1006 /** 1007 * The name for the group, as used in page.package. 1008 */ 1009 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1010 @Description(shortDefinition = "Name used .page.package", formalDefinition = "The name for the group, as used in page.package.") 1011 protected StringType name; 1012 1013 /** 1014 * Human readable text describing the package. 1015 */ 1016 @Child(name = "description", type = { 1017 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1018 @Description(shortDefinition = "Human readable text describing the package", formalDefinition = "Human readable text describing the package.") 1019 protected StringType description; 1020 1021 /** 1022 * A resource that is part of the implementation guide. Conformance resources 1023 * (value set, structure definition, conformance statements etc.) are obvious 1024 * candidates for inclusion, but any kind of resource can be included as an 1025 * example resource. 1026 */ 1027 @Child(name = "resource", type = {}, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1028 @Description(shortDefinition = "Resource in the implementation guide", formalDefinition = "A resource that is part of the implementation guide. Conformance resources (value set, structure definition, conformance statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.") 1029 protected List<ImplementationGuidePackageResourceComponent> resource; 1030 1031 private static final long serialVersionUID = -701846580L; 1032 1033 /* 1034 * Constructor 1035 */ 1036 public ImplementationGuidePackageComponent() { 1037 super(); 1038 } 1039 1040 /* 1041 * Constructor 1042 */ 1043 public ImplementationGuidePackageComponent(StringType name) { 1044 super(); 1045 this.name = name; 1046 } 1047 1048 /** 1049 * @return {@link #name} (The name for the group, as used in page.package.). 1050 * This is the underlying object with id, value and extensions. The 1051 * accessor "getName" gives direct access to the value 1052 */ 1053 public StringType getNameElement() { 1054 if (this.name == null) 1055 if (Configuration.errorOnAutoCreate()) 1056 throw new Error("Attempt to auto-create ImplementationGuidePackageComponent.name"); 1057 else if (Configuration.doAutoCreate()) 1058 this.name = new StringType(); // bb 1059 return this.name; 1060 } 1061 1062 public boolean hasNameElement() { 1063 return this.name != null && !this.name.isEmpty(); 1064 } 1065 1066 public boolean hasName() { 1067 return this.name != null && !this.name.isEmpty(); 1068 } 1069 1070 /** 1071 * @param value {@link #name} (The name for the group, as used in 1072 * page.package.). This is the underlying object with id, value and 1073 * extensions. The accessor "getName" gives direct access to the 1074 * value 1075 */ 1076 public ImplementationGuidePackageComponent setNameElement(StringType value) { 1077 this.name = value; 1078 return this; 1079 } 1080 1081 /** 1082 * @return The name for the group, as used in page.package. 1083 */ 1084 public String getName() { 1085 return this.name == null ? null : this.name.getValue(); 1086 } 1087 1088 /** 1089 * @param value The name for the group, as used in page.package. 1090 */ 1091 public ImplementationGuidePackageComponent setName(String value) { 1092 if (this.name == null) 1093 this.name = new StringType(); 1094 this.name.setValue(value); 1095 return this; 1096 } 1097 1098 /** 1099 * @return {@link #description} (Human readable text describing the package.). 1100 * This is the underlying object with id, value and extensions. The 1101 * accessor "getDescription" gives direct access to the value 1102 */ 1103 public StringType getDescriptionElement() { 1104 if (this.description == null) 1105 if (Configuration.errorOnAutoCreate()) 1106 throw new Error("Attempt to auto-create ImplementationGuidePackageComponent.description"); 1107 else if (Configuration.doAutoCreate()) 1108 this.description = new StringType(); // bb 1109 return this.description; 1110 } 1111 1112 public boolean hasDescriptionElement() { 1113 return this.description != null && !this.description.isEmpty(); 1114 } 1115 1116 public boolean hasDescription() { 1117 return this.description != null && !this.description.isEmpty(); 1118 } 1119 1120 /** 1121 * @param value {@link #description} (Human readable text describing the 1122 * package.). This is the underlying object with id, value and 1123 * extensions. The accessor "getDescription" gives direct access to 1124 * the value 1125 */ 1126 public ImplementationGuidePackageComponent setDescriptionElement(StringType value) { 1127 this.description = value; 1128 return this; 1129 } 1130 1131 /** 1132 * @return Human readable text describing the package. 1133 */ 1134 public String getDescription() { 1135 return this.description == null ? null : this.description.getValue(); 1136 } 1137 1138 /** 1139 * @param value Human readable text describing the package. 1140 */ 1141 public ImplementationGuidePackageComponent setDescription(String value) { 1142 if (Utilities.noString(value)) 1143 this.description = null; 1144 else { 1145 if (this.description == null) 1146 this.description = new StringType(); 1147 this.description.setValue(value); 1148 } 1149 return this; 1150 } 1151 1152 /** 1153 * @return {@link #resource} (A resource that is part of the implementation 1154 * guide. Conformance resources (value set, structure definition, 1155 * conformance statements etc.) are obvious candidates for inclusion, 1156 * but any kind of resource can be included as an example resource.) 1157 */ 1158 public List<ImplementationGuidePackageResourceComponent> getResource() { 1159 if (this.resource == null) 1160 this.resource = new ArrayList<ImplementationGuidePackageResourceComponent>(); 1161 return this.resource; 1162 } 1163 1164 public boolean hasResource() { 1165 if (this.resource == null) 1166 return false; 1167 for (ImplementationGuidePackageResourceComponent item : this.resource) 1168 if (!item.isEmpty()) 1169 return true; 1170 return false; 1171 } 1172 1173 /** 1174 * @return {@link #resource} (A resource that is part of the implementation 1175 * guide. Conformance resources (value set, structure definition, 1176 * conformance statements etc.) are obvious candidates for inclusion, 1177 * but any kind of resource can be included as an example resource.) 1178 */ 1179 // syntactic sugar 1180 public ImplementationGuidePackageResourceComponent addResource() { // 3 1181 ImplementationGuidePackageResourceComponent t = new ImplementationGuidePackageResourceComponent(); 1182 if (this.resource == null) 1183 this.resource = new ArrayList<ImplementationGuidePackageResourceComponent>(); 1184 this.resource.add(t); 1185 return t; 1186 } 1187 1188 // syntactic sugar 1189 public ImplementationGuidePackageComponent addResource(ImplementationGuidePackageResourceComponent t) { // 3 1190 if (t == null) 1191 return this; 1192 if (this.resource == null) 1193 this.resource = new ArrayList<ImplementationGuidePackageResourceComponent>(); 1194 this.resource.add(t); 1195 return this; 1196 } 1197 1198 protected void listChildren(List<Property> childrenList) { 1199 super.listChildren(childrenList); 1200 childrenList.add(new Property("name", "string", "The name for the group, as used in page.package.", 0, 1201 java.lang.Integer.MAX_VALUE, name)); 1202 childrenList.add(new Property("description", "string", "Human readable text describing the package.", 0, 1203 java.lang.Integer.MAX_VALUE, description)); 1204 childrenList.add(new Property("resource", "", 1205 "A resource that is part of the implementation guide. Conformance resources (value set, structure definition, conformance statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.", 1206 0, java.lang.Integer.MAX_VALUE, resource)); 1207 } 1208 1209 @Override 1210 public void setProperty(String name, Base value) throws FHIRException { 1211 if (name.equals("name")) 1212 this.name = castToString(value); // StringType 1213 else if (name.equals("description")) 1214 this.description = castToString(value); // StringType 1215 else if (name.equals("resource")) 1216 this.getResource().add((ImplementationGuidePackageResourceComponent) value); 1217 else 1218 super.setProperty(name, value); 1219 } 1220 1221 @Override 1222 public Base addChild(String name) throws FHIRException { 1223 if (name.equals("name")) { 1224 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.name"); 1225 } else if (name.equals("description")) { 1226 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.description"); 1227 } else if (name.equals("resource")) { 1228 return addResource(); 1229 } else 1230 return super.addChild(name); 1231 } 1232 1233 public ImplementationGuidePackageComponent copy() { 1234 ImplementationGuidePackageComponent dst = new ImplementationGuidePackageComponent(); 1235 copyValues(dst); 1236 dst.name = name == null ? null : name.copy(); 1237 dst.description = description == null ? null : description.copy(); 1238 if (resource != null) { 1239 dst.resource = new ArrayList<ImplementationGuidePackageResourceComponent>(); 1240 for (ImplementationGuidePackageResourceComponent i : resource) 1241 dst.resource.add(i.copy()); 1242 } 1243 ; 1244 return dst; 1245 } 1246 1247 @Override 1248 public boolean equalsDeep(Base other) { 1249 if (!super.equalsDeep(other)) 1250 return false; 1251 if (!(other instanceof ImplementationGuidePackageComponent)) 1252 return false; 1253 ImplementationGuidePackageComponent o = (ImplementationGuidePackageComponent) other; 1254 return compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 1255 && compareDeep(resource, o.resource, true); 1256 } 1257 1258 @Override 1259 public boolean equalsShallow(Base other) { 1260 if (!super.equalsShallow(other)) 1261 return false; 1262 if (!(other instanceof ImplementationGuidePackageComponent)) 1263 return false; 1264 ImplementationGuidePackageComponent o = (ImplementationGuidePackageComponent) other; 1265 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 1266 } 1267 1268 public boolean isEmpty() { 1269 return super.isEmpty() && (name == null || name.isEmpty()) && (description == null || description.isEmpty()) 1270 && (resource == null || resource.isEmpty()); 1271 } 1272 1273 public String fhirType() { 1274 return "ImplementationGuide.package"; 1275 1276 } 1277 1278 } 1279 1280 @Block() 1281 public static class ImplementationGuidePackageResourceComponent extends BackboneElement 1282 implements IBaseBackboneElement { 1283 /** 1284 * Why the resource is included in the guide. 1285 */ 1286 @Child(name = "purpose", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1287 @Description(shortDefinition = "example | terminology | profile | extension | dictionary | logical", formalDefinition = "Why the resource is included in the guide.") 1288 protected Enumeration<GuideResourcePurpose> purpose; 1289 1290 /** 1291 * A human assigned name for the resource. All resources SHOULD have a name, but 1292 * the name may be extracted from the resource (e.g. ValueSet.name). 1293 */ 1294 @Child(name = "name", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1295 @Description(shortDefinition = "Human Name for the resource", formalDefinition = "A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name).") 1296 protected StringType name; 1297 1298 /** 1299 * A description of the reason that a resource has been included in the 1300 * implementation guide. 1301 */ 1302 @Child(name = "description", type = { 1303 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1304 @Description(shortDefinition = "Reason why included in guide", formalDefinition = "A description of the reason that a resource has been included in the implementation guide.") 1305 protected StringType description; 1306 1307 /** 1308 * A short code that may be used to identify the resource throughout the 1309 * implementation guide. 1310 */ 1311 @Child(name = "acronym", type = { 1312 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1313 @Description(shortDefinition = "Short code to identify the resource", formalDefinition = "A short code that may be used to identify the resource throughout the implementation guide.") 1314 protected StringType acronym; 1315 1316 /** 1317 * Where this resource is found. 1318 */ 1319 @Child(name = "source", type = { UriType.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 1320 @Description(shortDefinition = "Location of the resource", formalDefinition = "Where this resource is found.") 1321 protected Type source; 1322 1323 /** 1324 * Another resource that this resource is an example for. This is mostly used 1325 * for resources that are included as examples of StructureDefinitions. 1326 */ 1327 @Child(name = "exampleFor", type = { 1328 StructureDefinition.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1329 @Description(shortDefinition = "Resource this is an example of (if applicable)", formalDefinition = "Another resource that this resource is an example for. This is mostly used for resources that are included as examples of StructureDefinitions.") 1330 protected Reference exampleFor; 1331 1332 /** 1333 * The actual object that is the target of the reference (Another resource that 1334 * this resource is an example for. This is mostly used for resources that are 1335 * included as examples of StructureDefinitions.) 1336 */ 1337 protected StructureDefinition exampleForTarget; 1338 1339 private static final long serialVersionUID = 428339533L; 1340 1341 /* 1342 * Constructor 1343 */ 1344 public ImplementationGuidePackageResourceComponent() { 1345 super(); 1346 } 1347 1348 /* 1349 * Constructor 1350 */ 1351 public ImplementationGuidePackageResourceComponent(Enumeration<GuideResourcePurpose> purpose, Type source) { 1352 super(); 1353 this.purpose = purpose; 1354 this.source = source; 1355 } 1356 1357 /** 1358 * @return {@link #purpose} (Why the resource is included in the guide.). This 1359 * is the underlying object with id, value and extensions. The accessor 1360 * "getPurpose" gives direct access to the value 1361 */ 1362 public Enumeration<GuideResourcePurpose> getPurposeElement() { 1363 if (this.purpose == null) 1364 if (Configuration.errorOnAutoCreate()) 1365 throw new Error("Attempt to auto-create ImplementationGuidePackageResourceComponent.purpose"); 1366 else if (Configuration.doAutoCreate()) 1367 this.purpose = new Enumeration<GuideResourcePurpose>(new GuideResourcePurposeEnumFactory()); // bb 1368 return this.purpose; 1369 } 1370 1371 public boolean hasPurposeElement() { 1372 return this.purpose != null && !this.purpose.isEmpty(); 1373 } 1374 1375 public boolean hasPurpose() { 1376 return this.purpose != null && !this.purpose.isEmpty(); 1377 } 1378 1379 /** 1380 * @param value {@link #purpose} (Why the resource is included in the guide.). 1381 * This is the underlying object with id, value and extensions. The 1382 * accessor "getPurpose" gives direct access to the value 1383 */ 1384 public ImplementationGuidePackageResourceComponent setPurposeElement(Enumeration<GuideResourcePurpose> value) { 1385 this.purpose = value; 1386 return this; 1387 } 1388 1389 /** 1390 * @return Why the resource is included in the guide. 1391 */ 1392 public GuideResourcePurpose getPurpose() { 1393 return this.purpose == null ? null : this.purpose.getValue(); 1394 } 1395 1396 /** 1397 * @param value Why the resource is included in the guide. 1398 */ 1399 public ImplementationGuidePackageResourceComponent setPurpose(GuideResourcePurpose value) { 1400 if (this.purpose == null) 1401 this.purpose = new Enumeration<GuideResourcePurpose>(new GuideResourcePurposeEnumFactory()); 1402 this.purpose.setValue(value); 1403 return this; 1404 } 1405 1406 /** 1407 * @return {@link #name} (A human assigned name for the resource. All resources 1408 * SHOULD have a name, but the name may be extracted from the resource 1409 * (e.g. ValueSet.name).). This is the underlying object with id, value 1410 * and extensions. The accessor "getName" gives direct access to the 1411 * value 1412 */ 1413 public StringType getNameElement() { 1414 if (this.name == null) 1415 if (Configuration.errorOnAutoCreate()) 1416 throw new Error("Attempt to auto-create ImplementationGuidePackageResourceComponent.name"); 1417 else if (Configuration.doAutoCreate()) 1418 this.name = new StringType(); // bb 1419 return this.name; 1420 } 1421 1422 public boolean hasNameElement() { 1423 return this.name != null && !this.name.isEmpty(); 1424 } 1425 1426 public boolean hasName() { 1427 return this.name != null && !this.name.isEmpty(); 1428 } 1429 1430 /** 1431 * @param value {@link #name} (A human assigned name for the resource. All 1432 * resources SHOULD have a name, but the name may be extracted from 1433 * the resource (e.g. ValueSet.name).). This is the underlying 1434 * object with id, value and extensions. The accessor "getName" 1435 * gives direct access to the value 1436 */ 1437 public ImplementationGuidePackageResourceComponent setNameElement(StringType value) { 1438 this.name = value; 1439 return this; 1440 } 1441 1442 /** 1443 * @return A human assigned name for the resource. All resources SHOULD have a 1444 * name, but the name may be extracted from the resource (e.g. 1445 * ValueSet.name). 1446 */ 1447 public String getName() { 1448 return this.name == null ? null : this.name.getValue(); 1449 } 1450 1451 /** 1452 * @param value A human assigned name for the resource. All resources SHOULD 1453 * have a name, but the name may be extracted from the resource 1454 * (e.g. ValueSet.name). 1455 */ 1456 public ImplementationGuidePackageResourceComponent setName(String value) { 1457 if (Utilities.noString(value)) 1458 this.name = null; 1459 else { 1460 if (this.name == null) 1461 this.name = new StringType(); 1462 this.name.setValue(value); 1463 } 1464 return this; 1465 } 1466 1467 /** 1468 * @return {@link #description} (A description of the reason that a resource has 1469 * been included in the implementation guide.). This is the underlying 1470 * object with id, value and extensions. The accessor "getDescription" 1471 * gives direct access to the value 1472 */ 1473 public StringType getDescriptionElement() { 1474 if (this.description == null) 1475 if (Configuration.errorOnAutoCreate()) 1476 throw new Error("Attempt to auto-create ImplementationGuidePackageResourceComponent.description"); 1477 else if (Configuration.doAutoCreate()) 1478 this.description = new StringType(); // bb 1479 return this.description; 1480 } 1481 1482 public boolean hasDescriptionElement() { 1483 return this.description != null && !this.description.isEmpty(); 1484 } 1485 1486 public boolean hasDescription() { 1487 return this.description != null && !this.description.isEmpty(); 1488 } 1489 1490 /** 1491 * @param value {@link #description} (A description of the reason that a 1492 * resource has been included in the implementation guide.). This 1493 * is the underlying object with id, value and extensions. The 1494 * accessor "getDescription" gives direct access to the value 1495 */ 1496 public ImplementationGuidePackageResourceComponent setDescriptionElement(StringType value) { 1497 this.description = value; 1498 return this; 1499 } 1500 1501 /** 1502 * @return A description of the reason that a resource has been included in the 1503 * implementation guide. 1504 */ 1505 public String getDescription() { 1506 return this.description == null ? null : this.description.getValue(); 1507 } 1508 1509 /** 1510 * @param value A description of the reason that a resource has been included in 1511 * the implementation guide. 1512 */ 1513 public ImplementationGuidePackageResourceComponent setDescription(String value) { 1514 if (Utilities.noString(value)) 1515 this.description = null; 1516 else { 1517 if (this.description == null) 1518 this.description = new StringType(); 1519 this.description.setValue(value); 1520 } 1521 return this; 1522 } 1523 1524 /** 1525 * @return {@link #acronym} (A short code that may be used to identify the 1526 * resource throughout the implementation guide.). This is the 1527 * underlying object with id, value and extensions. The accessor 1528 * "getAcronym" gives direct access to the value 1529 */ 1530 public StringType getAcronymElement() { 1531 if (this.acronym == null) 1532 if (Configuration.errorOnAutoCreate()) 1533 throw new Error("Attempt to auto-create ImplementationGuidePackageResourceComponent.acronym"); 1534 else if (Configuration.doAutoCreate()) 1535 this.acronym = new StringType(); // bb 1536 return this.acronym; 1537 } 1538 1539 public boolean hasAcronymElement() { 1540 return this.acronym != null && !this.acronym.isEmpty(); 1541 } 1542 1543 public boolean hasAcronym() { 1544 return this.acronym != null && !this.acronym.isEmpty(); 1545 } 1546 1547 /** 1548 * @param value {@link #acronym} (A short code that may be used to identify the 1549 * resource throughout the implementation guide.). This is the 1550 * underlying object with id, value and extensions. The accessor 1551 * "getAcronym" gives direct access to the value 1552 */ 1553 public ImplementationGuidePackageResourceComponent setAcronymElement(StringType value) { 1554 this.acronym = value; 1555 return this; 1556 } 1557 1558 /** 1559 * @return A short code that may be used to identify the resource throughout the 1560 * implementation guide. 1561 */ 1562 public String getAcronym() { 1563 return this.acronym == null ? null : this.acronym.getValue(); 1564 } 1565 1566 /** 1567 * @param value A short code that may be used to identify the resource 1568 * throughout the implementation guide. 1569 */ 1570 public ImplementationGuidePackageResourceComponent setAcronym(String value) { 1571 if (Utilities.noString(value)) 1572 this.acronym = null; 1573 else { 1574 if (this.acronym == null) 1575 this.acronym = new StringType(); 1576 this.acronym.setValue(value); 1577 } 1578 return this; 1579 } 1580 1581 /** 1582 * @return {@link #source} (Where this resource is found.) 1583 */ 1584 public Type getSource() { 1585 return this.source; 1586 } 1587 1588 /** 1589 * @return {@link #source} (Where this resource is found.) 1590 */ 1591 public UriType getSourceUriType() throws FHIRException { 1592 if (!(this.source instanceof UriType)) 1593 throw new FHIRException("Type mismatch: the type UriType was expected, but " + this.source.getClass().getName() 1594 + " was encountered"); 1595 return (UriType) this.source; 1596 } 1597 1598 public boolean hasSourceUriType() { 1599 return this.source instanceof UriType; 1600 } 1601 1602 /** 1603 * @return {@link #source} (Where this resource is found.) 1604 */ 1605 public Reference getSourceReference() throws FHIRException { 1606 if (!(this.source instanceof Reference)) 1607 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1608 + this.source.getClass().getName() + " was encountered"); 1609 return (Reference) this.source; 1610 } 1611 1612 public boolean hasSourceReference() { 1613 return this.source instanceof Reference; 1614 } 1615 1616 public boolean hasSource() { 1617 return this.source != null && !this.source.isEmpty(); 1618 } 1619 1620 /** 1621 * @param value {@link #source} (Where this resource is found.) 1622 */ 1623 public ImplementationGuidePackageResourceComponent setSource(Type value) { 1624 this.source = value; 1625 return this; 1626 } 1627 1628 /** 1629 * @return {@link #exampleFor} (Another resource that this resource is an 1630 * example for. This is mostly used for resources that are included as 1631 * examples of StructureDefinitions.) 1632 */ 1633 public Reference getExampleFor() { 1634 if (this.exampleFor == null) 1635 if (Configuration.errorOnAutoCreate()) 1636 throw new Error("Attempt to auto-create ImplementationGuidePackageResourceComponent.exampleFor"); 1637 else if (Configuration.doAutoCreate()) 1638 this.exampleFor = new Reference(); // cc 1639 return this.exampleFor; 1640 } 1641 1642 public boolean hasExampleFor() { 1643 return this.exampleFor != null && !this.exampleFor.isEmpty(); 1644 } 1645 1646 /** 1647 * @param value {@link #exampleFor} (Another resource that this resource is an 1648 * example for. This is mostly used for resources that are included 1649 * as examples of StructureDefinitions.) 1650 */ 1651 public ImplementationGuidePackageResourceComponent setExampleFor(Reference value) { 1652 this.exampleFor = value; 1653 return this; 1654 } 1655 1656 /** 1657 * @return {@link #exampleFor} The actual object that is the target of the 1658 * reference. The reference library doesn't populate this, but you can 1659 * use it to hold the resource if you resolve it. (Another resource that 1660 * this resource is an example for. This is mostly used for resources 1661 * that are included as examples of StructureDefinitions.) 1662 */ 1663 public StructureDefinition getExampleForTarget() { 1664 if (this.exampleForTarget == null) 1665 if (Configuration.errorOnAutoCreate()) 1666 throw new Error("Attempt to auto-create ImplementationGuidePackageResourceComponent.exampleFor"); 1667 else if (Configuration.doAutoCreate()) 1668 this.exampleForTarget = new StructureDefinition(); // aa 1669 return this.exampleForTarget; 1670 } 1671 1672 /** 1673 * @param value {@link #exampleFor} The actual object that is the target of the 1674 * reference. The reference library doesn't use these, but you can 1675 * use it to hold the resource if you resolve it. (Another resource 1676 * that this resource is an example for. This is mostly used for 1677 * resources that are included as examples of 1678 * StructureDefinitions.) 1679 */ 1680 public ImplementationGuidePackageResourceComponent setExampleForTarget(StructureDefinition value) { 1681 this.exampleForTarget = value; 1682 return this; 1683 } 1684 1685 protected void listChildren(List<Property> childrenList) { 1686 super.listChildren(childrenList); 1687 childrenList.add(new Property("purpose", "code", "Why the resource is included in the guide.", 0, 1688 java.lang.Integer.MAX_VALUE, purpose)); 1689 childrenList.add(new Property("name", "string", 1690 "A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name).", 1691 0, java.lang.Integer.MAX_VALUE, name)); 1692 childrenList.add(new Property("description", "string", 1693 "A description of the reason that a resource has been included in the implementation guide.", 0, 1694 java.lang.Integer.MAX_VALUE, description)); 1695 childrenList.add(new Property("acronym", "string", 1696 "A short code that may be used to identify the resource throughout the implementation guide.", 0, 1697 java.lang.Integer.MAX_VALUE, acronym)); 1698 childrenList.add(new Property("source[x]", "uri|Reference(Any)", "Where this resource is found.", 0, 1699 java.lang.Integer.MAX_VALUE, source)); 1700 childrenList.add(new Property("exampleFor", "Reference(StructureDefinition)", 1701 "Another resource that this resource is an example for. This is mostly used for resources that are included as examples of StructureDefinitions.", 1702 0, java.lang.Integer.MAX_VALUE, exampleFor)); 1703 } 1704 1705 @Override 1706 public void setProperty(String name, Base value) throws FHIRException { 1707 if (name.equals("purpose")) 1708 this.purpose = new GuideResourcePurposeEnumFactory().fromType(value); // Enumeration<GuideResourcePurpose> 1709 else if (name.equals("name")) 1710 this.name = castToString(value); // StringType 1711 else if (name.equals("description")) 1712 this.description = castToString(value); // StringType 1713 else if (name.equals("acronym")) 1714 this.acronym = castToString(value); // StringType 1715 else if (name.equals("source[x]")) 1716 this.source = (Type) value; // Type 1717 else if (name.equals("exampleFor")) 1718 this.exampleFor = castToReference(value); // Reference 1719 else 1720 super.setProperty(name, value); 1721 } 1722 1723 @Override 1724 public Base addChild(String name) throws FHIRException { 1725 if (name.equals("purpose")) { 1726 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.purpose"); 1727 } else if (name.equals("name")) { 1728 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.name"); 1729 } else if (name.equals("description")) { 1730 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.description"); 1731 } else if (name.equals("acronym")) { 1732 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.acronym"); 1733 } else if (name.equals("sourceUri")) { 1734 this.source = new UriType(); 1735 return this.source; 1736 } else if (name.equals("sourceReference")) { 1737 this.source = new Reference(); 1738 return this.source; 1739 } else if (name.equals("exampleFor")) { 1740 this.exampleFor = new Reference(); 1741 return this.exampleFor; 1742 } else 1743 return super.addChild(name); 1744 } 1745 1746 public ImplementationGuidePackageResourceComponent copy() { 1747 ImplementationGuidePackageResourceComponent dst = new ImplementationGuidePackageResourceComponent(); 1748 copyValues(dst); 1749 dst.purpose = purpose == null ? null : purpose.copy(); 1750 dst.name = name == null ? null : name.copy(); 1751 dst.description = description == null ? null : description.copy(); 1752 dst.acronym = acronym == null ? null : acronym.copy(); 1753 dst.source = source == null ? null : source.copy(); 1754 dst.exampleFor = exampleFor == null ? null : exampleFor.copy(); 1755 return dst; 1756 } 1757 1758 @Override 1759 public boolean equalsDeep(Base other) { 1760 if (!super.equalsDeep(other)) 1761 return false; 1762 if (!(other instanceof ImplementationGuidePackageResourceComponent)) 1763 return false; 1764 ImplementationGuidePackageResourceComponent o = (ImplementationGuidePackageResourceComponent) other; 1765 return compareDeep(purpose, o.purpose, true) && compareDeep(name, o.name, true) 1766 && compareDeep(description, o.description, true) && compareDeep(acronym, o.acronym, true) 1767 && compareDeep(source, o.source, true) && compareDeep(exampleFor, o.exampleFor, true); 1768 } 1769 1770 @Override 1771 public boolean equalsShallow(Base other) { 1772 if (!super.equalsShallow(other)) 1773 return false; 1774 if (!(other instanceof ImplementationGuidePackageResourceComponent)) 1775 return false; 1776 ImplementationGuidePackageResourceComponent o = (ImplementationGuidePackageResourceComponent) other; 1777 return compareValues(purpose, o.purpose, true) && compareValues(name, o.name, true) 1778 && compareValues(description, o.description, true) && compareValues(acronym, o.acronym, true); 1779 } 1780 1781 public boolean isEmpty() { 1782 return super.isEmpty() && (purpose == null || purpose.isEmpty()) && (name == null || name.isEmpty()) 1783 && (description == null || description.isEmpty()) && (acronym == null || acronym.isEmpty()) 1784 && (source == null || source.isEmpty()) && (exampleFor == null || exampleFor.isEmpty()); 1785 } 1786 1787 public String fhirType() { 1788 return "ImplementationGuide.package.resource"; 1789 1790 } 1791 1792 } 1793 1794 @Block() 1795 public static class ImplementationGuideGlobalComponent extends BackboneElement implements IBaseBackboneElement { 1796 /** 1797 * The type of resource that all instances must conform to. 1798 */ 1799 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1800 @Description(shortDefinition = "Type this profiles applies to", formalDefinition = "The type of resource that all instances must conform to.") 1801 protected CodeType type; 1802 1803 /** 1804 * A reference to the profile that all instances must conform to. 1805 */ 1806 @Child(name = "profile", type = { 1807 StructureDefinition.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1808 @Description(shortDefinition = "Profile that all resources must conform to", formalDefinition = "A reference to the profile that all instances must conform to.") 1809 protected Reference profile; 1810 1811 /** 1812 * The actual object that is the target of the reference (A reference to the 1813 * profile that all instances must conform to.) 1814 */ 1815 protected StructureDefinition profileTarget; 1816 1817 private static final long serialVersionUID = 2011731959L; 1818 1819 /* 1820 * Constructor 1821 */ 1822 public ImplementationGuideGlobalComponent() { 1823 super(); 1824 } 1825 1826 /* 1827 * Constructor 1828 */ 1829 public ImplementationGuideGlobalComponent(CodeType type, Reference profile) { 1830 super(); 1831 this.type = type; 1832 this.profile = profile; 1833 } 1834 1835 /** 1836 * @return {@link #type} (The type of resource that all instances must conform 1837 * to.). This is the underlying object with id, value and extensions. 1838 * The accessor "getType" gives direct access to the value 1839 */ 1840 public CodeType getTypeElement() { 1841 if (this.type == null) 1842 if (Configuration.errorOnAutoCreate()) 1843 throw new Error("Attempt to auto-create ImplementationGuideGlobalComponent.type"); 1844 else if (Configuration.doAutoCreate()) 1845 this.type = new CodeType(); // bb 1846 return this.type; 1847 } 1848 1849 public boolean hasTypeElement() { 1850 return this.type != null && !this.type.isEmpty(); 1851 } 1852 1853 public boolean hasType() { 1854 return this.type != null && !this.type.isEmpty(); 1855 } 1856 1857 /** 1858 * @param value {@link #type} (The type of resource that all instances must 1859 * conform to.). This is the underlying object with id, value and 1860 * extensions. The accessor "getType" gives direct access to the 1861 * value 1862 */ 1863 public ImplementationGuideGlobalComponent setTypeElement(CodeType value) { 1864 this.type = value; 1865 return this; 1866 } 1867 1868 /** 1869 * @return The type of resource that all instances must conform to. 1870 */ 1871 public String getType() { 1872 return this.type == null ? null : this.type.getValue(); 1873 } 1874 1875 /** 1876 * @param value The type of resource that all instances must conform to. 1877 */ 1878 public ImplementationGuideGlobalComponent setType(String value) { 1879 if (this.type == null) 1880 this.type = new CodeType(); 1881 this.type.setValue(value); 1882 return this; 1883 } 1884 1885 /** 1886 * @return {@link #profile} (A reference to the profile that all instances must 1887 * conform to.) 1888 */ 1889 public Reference getProfile() { 1890 if (this.profile == null) 1891 if (Configuration.errorOnAutoCreate()) 1892 throw new Error("Attempt to auto-create ImplementationGuideGlobalComponent.profile"); 1893 else if (Configuration.doAutoCreate()) 1894 this.profile = new Reference(); // cc 1895 return this.profile; 1896 } 1897 1898 public boolean hasProfile() { 1899 return this.profile != null && !this.profile.isEmpty(); 1900 } 1901 1902 /** 1903 * @param value {@link #profile} (A reference to the profile that all instances 1904 * must conform to.) 1905 */ 1906 public ImplementationGuideGlobalComponent setProfile(Reference value) { 1907 this.profile = value; 1908 return this; 1909 } 1910 1911 /** 1912 * @return {@link #profile} The actual object that is the target of the 1913 * reference. The reference library doesn't populate this, but you can 1914 * use it to hold the resource if you resolve it. (A reference to the 1915 * profile that all instances must conform to.) 1916 */ 1917 public StructureDefinition getProfileTarget() { 1918 if (this.profileTarget == null) 1919 if (Configuration.errorOnAutoCreate()) 1920 throw new Error("Attempt to auto-create ImplementationGuideGlobalComponent.profile"); 1921 else if (Configuration.doAutoCreate()) 1922 this.profileTarget = new StructureDefinition(); // aa 1923 return this.profileTarget; 1924 } 1925 1926 /** 1927 * @param value {@link #profile} The actual object that is the target of the 1928 * reference. The reference library doesn't use these, but you can 1929 * use it to hold the resource if you resolve it. (A reference to 1930 * the profile that all instances must conform to.) 1931 */ 1932 public ImplementationGuideGlobalComponent setProfileTarget(StructureDefinition value) { 1933 this.profileTarget = value; 1934 return this; 1935 } 1936 1937 protected void listChildren(List<Property> childrenList) { 1938 super.listChildren(childrenList); 1939 childrenList.add(new Property("type", "code", "The type of resource that all instances must conform to.", 0, 1940 java.lang.Integer.MAX_VALUE, type)); 1941 childrenList.add(new Property("profile", "Reference(StructureDefinition)", 1942 "A reference to the profile that all instances must conform to.", 0, java.lang.Integer.MAX_VALUE, profile)); 1943 } 1944 1945 @Override 1946 public void setProperty(String name, Base value) throws FHIRException { 1947 if (name.equals("type")) 1948 this.type = castToCode(value); // CodeType 1949 else if (name.equals("profile")) 1950 this.profile = castToReference(value); // Reference 1951 else 1952 super.setProperty(name, value); 1953 } 1954 1955 @Override 1956 public Base addChild(String name) throws FHIRException { 1957 if (name.equals("type")) { 1958 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.type"); 1959 } else if (name.equals("profile")) { 1960 this.profile = new Reference(); 1961 return this.profile; 1962 } else 1963 return super.addChild(name); 1964 } 1965 1966 public ImplementationGuideGlobalComponent copy() { 1967 ImplementationGuideGlobalComponent dst = new ImplementationGuideGlobalComponent(); 1968 copyValues(dst); 1969 dst.type = type == null ? null : type.copy(); 1970 dst.profile = profile == null ? null : profile.copy(); 1971 return dst; 1972 } 1973 1974 @Override 1975 public boolean equalsDeep(Base other) { 1976 if (!super.equalsDeep(other)) 1977 return false; 1978 if (!(other instanceof ImplementationGuideGlobalComponent)) 1979 return false; 1980 ImplementationGuideGlobalComponent o = (ImplementationGuideGlobalComponent) other; 1981 return compareDeep(type, o.type, true) && compareDeep(profile, o.profile, true); 1982 } 1983 1984 @Override 1985 public boolean equalsShallow(Base other) { 1986 if (!super.equalsShallow(other)) 1987 return false; 1988 if (!(other instanceof ImplementationGuideGlobalComponent)) 1989 return false; 1990 ImplementationGuideGlobalComponent o = (ImplementationGuideGlobalComponent) other; 1991 return compareValues(type, o.type, true); 1992 } 1993 1994 public boolean isEmpty() { 1995 return super.isEmpty() && (type == null || type.isEmpty()) && (profile == null || profile.isEmpty()); 1996 } 1997 1998 public String fhirType() { 1999 return "ImplementationGuide.global"; 2000 2001 } 2002 2003 } 2004 2005 @Block() 2006 public static class ImplementationGuidePageComponent extends BackboneElement implements IBaseBackboneElement { 2007 /** 2008 * The source address for the page. 2009 */ 2010 @Child(name = "source", type = { UriType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2011 @Description(shortDefinition = "Where to find that page", formalDefinition = "The source address for the page.") 2012 protected UriType source; 2013 2014 /** 2015 * A short name used to represent this page in navigational structures such as 2016 * table of contents, bread crumbs, etc. 2017 */ 2018 @Child(name = "name", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 2019 @Description(shortDefinition = "Short name shown for navigational assistance", formalDefinition = "A short name used to represent this page in navigational structures such as table of contents, bread crumbs, etc.") 2020 protected StringType name; 2021 2022 /** 2023 * The kind of page that this is. Some pages are autogenerated (list, example), 2024 * and other kinds are of interest so that tools can navigate the user to the 2025 * page of interest. 2026 */ 2027 @Child(name = "kind", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 2028 @Description(shortDefinition = "page | example | list | include | directory | dictionary | toc | resource", formalDefinition = "The kind of page that this is. Some pages are autogenerated (list, example), and other kinds are of interest so that tools can navigate the user to the page of interest.") 2029 protected Enumeration<GuidePageKind> kind; 2030 2031 /** 2032 * For constructed pages, what kind of resources to include in the list. 2033 */ 2034 @Child(name = "type", type = { 2035 CodeType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2036 @Description(shortDefinition = "Kind of resource to include in the list", formalDefinition = "For constructed pages, what kind of resources to include in the list.") 2037 protected List<CodeType> type; 2038 2039 /** 2040 * For constructed pages, a list of packages to include in the page (or else 2041 * empty for everything). 2042 */ 2043 @Child(name = "package", type = { 2044 StringType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2045 @Description(shortDefinition = "Name of package to include", formalDefinition = "For constructed pages, a list of packages to include in the page (or else empty for everything).") 2046 protected List<StringType> package_; 2047 2048 /** 2049 * The format of the page. 2050 */ 2051 @Child(name = "format", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 2052 @Description(shortDefinition = "Format of the page (e.g. html, markdown, etc.)", formalDefinition = "The format of the page.") 2053 protected CodeType format; 2054 2055 /** 2056 * Nested Pages/Sections under this page. 2057 */ 2058 @Child(name = "page", type = { 2059 ImplementationGuidePageComponent.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2060 @Description(shortDefinition = "Nested Pages / Sections", formalDefinition = "Nested Pages/Sections under this page.") 2061 protected List<ImplementationGuidePageComponent> page; 2062 2063 private static final long serialVersionUID = -1620890043L; 2064 2065 /* 2066 * Constructor 2067 */ 2068 public ImplementationGuidePageComponent() { 2069 super(); 2070 } 2071 2072 /* 2073 * Constructor 2074 */ 2075 public ImplementationGuidePageComponent(UriType source, StringType name, Enumeration<GuidePageKind> kind) { 2076 super(); 2077 this.source = source; 2078 this.name = name; 2079 this.kind = kind; 2080 } 2081 2082 /** 2083 * @return {@link #source} (The source address for the page.). This is the 2084 * underlying object with id, value and extensions. The accessor 2085 * "getSource" gives direct access to the value 2086 */ 2087 public UriType getSourceElement() { 2088 if (this.source == null) 2089 if (Configuration.errorOnAutoCreate()) 2090 throw new Error("Attempt to auto-create ImplementationGuidePageComponent.source"); 2091 else if (Configuration.doAutoCreate()) 2092 this.source = new UriType(); // bb 2093 return this.source; 2094 } 2095 2096 public boolean hasSourceElement() { 2097 return this.source != null && !this.source.isEmpty(); 2098 } 2099 2100 public boolean hasSource() { 2101 return this.source != null && !this.source.isEmpty(); 2102 } 2103 2104 /** 2105 * @param value {@link #source} (The source address for the page.). This is the 2106 * underlying object with id, value and extensions. The accessor 2107 * "getSource" gives direct access to the value 2108 */ 2109 public ImplementationGuidePageComponent setSourceElement(UriType value) { 2110 this.source = value; 2111 return this; 2112 } 2113 2114 /** 2115 * @return The source address for the page. 2116 */ 2117 public String getSource() { 2118 return this.source == null ? null : this.source.getValue(); 2119 } 2120 2121 /** 2122 * @param value The source address for the page. 2123 */ 2124 public ImplementationGuidePageComponent setSource(String value) { 2125 if (this.source == null) 2126 this.source = new UriType(); 2127 this.source.setValue(value); 2128 return this; 2129 } 2130 2131 /** 2132 * @return {@link #name} (A short name used to represent this page in 2133 * navigational structures such as table of contents, bread crumbs, 2134 * etc.). This is the underlying object with id, value and extensions. 2135 * The accessor "getName" gives direct access to the value 2136 */ 2137 public StringType getNameElement() { 2138 if (this.name == null) 2139 if (Configuration.errorOnAutoCreate()) 2140 throw new Error("Attempt to auto-create ImplementationGuidePageComponent.name"); 2141 else if (Configuration.doAutoCreate()) 2142 this.name = new StringType(); // bb 2143 return this.name; 2144 } 2145 2146 public boolean hasNameElement() { 2147 return this.name != null && !this.name.isEmpty(); 2148 } 2149 2150 public boolean hasName() { 2151 return this.name != null && !this.name.isEmpty(); 2152 } 2153 2154 /** 2155 * @param value {@link #name} (A short name used to represent this page in 2156 * navigational structures such as table of contents, bread crumbs, 2157 * etc.). This is the underlying object with id, value and 2158 * extensions. The accessor "getName" gives direct access to the 2159 * value 2160 */ 2161 public ImplementationGuidePageComponent setNameElement(StringType value) { 2162 this.name = value; 2163 return this; 2164 } 2165 2166 /** 2167 * @return A short name used to represent this page in navigational structures 2168 * such as table of contents, bread crumbs, etc. 2169 */ 2170 public String getName() { 2171 return this.name == null ? null : this.name.getValue(); 2172 } 2173 2174 /** 2175 * @param value A short name used to represent this page in navigational 2176 * structures such as table of contents, bread crumbs, etc. 2177 */ 2178 public ImplementationGuidePageComponent setName(String value) { 2179 if (this.name == null) 2180 this.name = new StringType(); 2181 this.name.setValue(value); 2182 return this; 2183 } 2184 2185 /** 2186 * @return {@link #kind} (The kind of page that this is. Some pages are 2187 * autogenerated (list, example), and other kinds are of interest so 2188 * that tools can navigate the user to the page of interest.). This is 2189 * the underlying object with id, value and extensions. The accessor 2190 * "getKind" gives direct access to the value 2191 */ 2192 public Enumeration<GuidePageKind> getKindElement() { 2193 if (this.kind == null) 2194 if (Configuration.errorOnAutoCreate()) 2195 throw new Error("Attempt to auto-create ImplementationGuidePageComponent.kind"); 2196 else if (Configuration.doAutoCreate()) 2197 this.kind = new Enumeration<GuidePageKind>(new GuidePageKindEnumFactory()); // bb 2198 return this.kind; 2199 } 2200 2201 public boolean hasKindElement() { 2202 return this.kind != null && !this.kind.isEmpty(); 2203 } 2204 2205 public boolean hasKind() { 2206 return this.kind != null && !this.kind.isEmpty(); 2207 } 2208 2209 /** 2210 * @param value {@link #kind} (The kind of page that this is. Some pages are 2211 * autogenerated (list, example), and other kinds are of interest 2212 * so that tools can navigate the user to the page of interest.). 2213 * This is the underlying object with id, value and extensions. The 2214 * accessor "getKind" gives direct access to the value 2215 */ 2216 public ImplementationGuidePageComponent setKindElement(Enumeration<GuidePageKind> value) { 2217 this.kind = value; 2218 return this; 2219 } 2220 2221 /** 2222 * @return The kind of page that this is. Some pages are autogenerated (list, 2223 * example), and other kinds are of interest so that tools can navigate 2224 * the user to the page of interest. 2225 */ 2226 public GuidePageKind getKind() { 2227 return this.kind == null ? null : this.kind.getValue(); 2228 } 2229 2230 /** 2231 * @param value The kind of page that this is. Some pages are autogenerated 2232 * (list, example), and other kinds are of interest so that tools 2233 * can navigate the user to the page of interest. 2234 */ 2235 public ImplementationGuidePageComponent setKind(GuidePageKind value) { 2236 if (this.kind == null) 2237 this.kind = new Enumeration<GuidePageKind>(new GuidePageKindEnumFactory()); 2238 this.kind.setValue(value); 2239 return this; 2240 } 2241 2242 /** 2243 * @return {@link #type} (For constructed pages, what kind of resources to 2244 * include in the list.) 2245 */ 2246 public List<CodeType> getType() { 2247 if (this.type == null) 2248 this.type = new ArrayList<CodeType>(); 2249 return this.type; 2250 } 2251 2252 public boolean hasType() { 2253 if (this.type == null) 2254 return false; 2255 for (CodeType item : this.type) 2256 if (!item.isEmpty()) 2257 return true; 2258 return false; 2259 } 2260 2261 /** 2262 * @return {@link #type} (For constructed pages, what kind of resources to 2263 * include in the list.) 2264 */ 2265 // syntactic sugar 2266 public CodeType addTypeElement() {// 2 2267 CodeType t = new CodeType(); 2268 if (this.type == null) 2269 this.type = new ArrayList<CodeType>(); 2270 this.type.add(t); 2271 return t; 2272 } 2273 2274 /** 2275 * @param value {@link #type} (For constructed pages, what kind of resources to 2276 * include in the list.) 2277 */ 2278 public ImplementationGuidePageComponent addType(String value) { // 1 2279 CodeType t = new CodeType(); 2280 t.setValue(value); 2281 if (this.type == null) 2282 this.type = new ArrayList<CodeType>(); 2283 this.type.add(t); 2284 return this; 2285 } 2286 2287 /** 2288 * @param value {@link #type} (For constructed pages, what kind of resources to 2289 * include in the list.) 2290 */ 2291 public boolean hasType(String value) { 2292 if (this.type == null) 2293 return false; 2294 for (CodeType v : this.type) 2295 if (v.equals(value)) // code 2296 return true; 2297 return false; 2298 } 2299 2300 /** 2301 * @return {@link #package_} (For constructed pages, a list of packages to 2302 * include in the page (or else empty for everything).) 2303 */ 2304 public List<StringType> getPackage() { 2305 if (this.package_ == null) 2306 this.package_ = new ArrayList<StringType>(); 2307 return this.package_; 2308 } 2309 2310 public boolean hasPackage() { 2311 if (this.package_ == null) 2312 return false; 2313 for (StringType item : this.package_) 2314 if (!item.isEmpty()) 2315 return true; 2316 return false; 2317 } 2318 2319 /** 2320 * @return {@link #package_} (For constructed pages, a list of packages to 2321 * include in the page (or else empty for everything).) 2322 */ 2323 // syntactic sugar 2324 public StringType addPackageElement() {// 2 2325 StringType t = new StringType(); 2326 if (this.package_ == null) 2327 this.package_ = new ArrayList<StringType>(); 2328 this.package_.add(t); 2329 return t; 2330 } 2331 2332 /** 2333 * @param value {@link #package_} (For constructed pages, a list of packages to 2334 * include in the page (or else empty for everything).) 2335 */ 2336 public ImplementationGuidePageComponent addPackage(String value) { // 1 2337 StringType t = new StringType(); 2338 t.setValue(value); 2339 if (this.package_ == null) 2340 this.package_ = new ArrayList<StringType>(); 2341 this.package_.add(t); 2342 return this; 2343 } 2344 2345 /** 2346 * @param value {@link #package_} (For constructed pages, a list of packages to 2347 * include in the page (or else empty for everything).) 2348 */ 2349 public boolean hasPackage(String value) { 2350 if (this.package_ == null) 2351 return false; 2352 for (StringType v : this.package_) 2353 if (v.equals(value)) // string 2354 return true; 2355 return false; 2356 } 2357 2358 /** 2359 * @return {@link #format} (The format of the page.). This is the underlying 2360 * object with id, value and extensions. The accessor "getFormat" gives 2361 * direct access to the value 2362 */ 2363 public CodeType getFormatElement() { 2364 if (this.format == null) 2365 if (Configuration.errorOnAutoCreate()) 2366 throw new Error("Attempt to auto-create ImplementationGuidePageComponent.format"); 2367 else if (Configuration.doAutoCreate()) 2368 this.format = new CodeType(); // bb 2369 return this.format; 2370 } 2371 2372 public boolean hasFormatElement() { 2373 return this.format != null && !this.format.isEmpty(); 2374 } 2375 2376 public boolean hasFormat() { 2377 return this.format != null && !this.format.isEmpty(); 2378 } 2379 2380 /** 2381 * @param value {@link #format} (The format of the page.). This is the 2382 * underlying object with id, value and extensions. The accessor 2383 * "getFormat" gives direct access to the value 2384 */ 2385 public ImplementationGuidePageComponent setFormatElement(CodeType value) { 2386 this.format = value; 2387 return this; 2388 } 2389 2390 /** 2391 * @return The format of the page. 2392 */ 2393 public String getFormat() { 2394 return this.format == null ? null : this.format.getValue(); 2395 } 2396 2397 /** 2398 * @param value The format of the page. 2399 */ 2400 public ImplementationGuidePageComponent setFormat(String value) { 2401 if (Utilities.noString(value)) 2402 this.format = null; 2403 else { 2404 if (this.format == null) 2405 this.format = new CodeType(); 2406 this.format.setValue(value); 2407 } 2408 return this; 2409 } 2410 2411 /** 2412 * @return {@link #page} (Nested Pages/Sections under this page.) 2413 */ 2414 public List<ImplementationGuidePageComponent> getPage() { 2415 if (this.page == null) 2416 this.page = new ArrayList<ImplementationGuidePageComponent>(); 2417 return this.page; 2418 } 2419 2420 public boolean hasPage() { 2421 if (this.page == null) 2422 return false; 2423 for (ImplementationGuidePageComponent item : this.page) 2424 if (!item.isEmpty()) 2425 return true; 2426 return false; 2427 } 2428 2429 /** 2430 * @return {@link #page} (Nested Pages/Sections under this page.) 2431 */ 2432 // syntactic sugar 2433 public ImplementationGuidePageComponent addPage() { // 3 2434 ImplementationGuidePageComponent t = new ImplementationGuidePageComponent(); 2435 if (this.page == null) 2436 this.page = new ArrayList<ImplementationGuidePageComponent>(); 2437 this.page.add(t); 2438 return t; 2439 } 2440 2441 // syntactic sugar 2442 public ImplementationGuidePageComponent addPage(ImplementationGuidePageComponent t) { // 3 2443 if (t == null) 2444 return this; 2445 if (this.page == null) 2446 this.page = new ArrayList<ImplementationGuidePageComponent>(); 2447 this.page.add(t); 2448 return this; 2449 } 2450 2451 protected void listChildren(List<Property> childrenList) { 2452 super.listChildren(childrenList); 2453 childrenList.add( 2454 new Property("source", "uri", "The source address for the page.", 0, java.lang.Integer.MAX_VALUE, source)); 2455 childrenList.add(new Property("name", "string", 2456 "A short name used to represent this page in navigational structures such as table of contents, bread crumbs, etc.", 2457 0, java.lang.Integer.MAX_VALUE, name)); 2458 childrenList.add(new Property("kind", "code", 2459 "The kind of page that this is. Some pages are autogenerated (list, example), and other kinds are of interest so that tools can navigate the user to the page of interest.", 2460 0, java.lang.Integer.MAX_VALUE, kind)); 2461 childrenList 2462 .add(new Property("type", "code", "For constructed pages, what kind of resources to include in the list.", 0, 2463 java.lang.Integer.MAX_VALUE, type)); 2464 childrenList.add(new Property("package", "string", 2465 "For constructed pages, a list of packages to include in the page (or else empty for everything).", 0, 2466 java.lang.Integer.MAX_VALUE, package_)); 2467 childrenList 2468 .add(new Property("format", "code", "The format of the page.", 0, java.lang.Integer.MAX_VALUE, format)); 2469 childrenList.add(new Property("page", "@ImplementationGuide.page", "Nested Pages/Sections under this page.", 0, 2470 java.lang.Integer.MAX_VALUE, page)); 2471 } 2472 2473 @Override 2474 public void setProperty(String name, Base value) throws FHIRException { 2475 if (name.equals("source")) 2476 this.source = castToUri(value); // UriType 2477 else if (name.equals("name")) 2478 this.name = castToString(value); // StringType 2479 else if (name.equals("kind")) 2480 this.kind = new GuidePageKindEnumFactory().fromType(value); // Enumeration<GuidePageKind> 2481 else if (name.equals("type")) 2482 this.getType().add(castToCode(value)); 2483 else if (name.equals("package")) 2484 this.getPackage().add(castToString(value)); 2485 else if (name.equals("format")) 2486 this.format = castToCode(value); // CodeType 2487 else if (name.equals("page")) 2488 this.getPage().add((ImplementationGuidePageComponent) value); 2489 else 2490 super.setProperty(name, value); 2491 } 2492 2493 @Override 2494 public Base addChild(String name) throws FHIRException { 2495 if (name.equals("source")) { 2496 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.source"); 2497 } else if (name.equals("name")) { 2498 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.name"); 2499 } else if (name.equals("kind")) { 2500 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.kind"); 2501 } else if (name.equals("type")) { 2502 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.type"); 2503 } else if (name.equals("package")) { 2504 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.package"); 2505 } else if (name.equals("format")) { 2506 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.format"); 2507 } else if (name.equals("page")) { 2508 return addPage(); 2509 } else 2510 return super.addChild(name); 2511 } 2512 2513 public ImplementationGuidePageComponent copy() { 2514 ImplementationGuidePageComponent dst = new ImplementationGuidePageComponent(); 2515 copyValues(dst); 2516 dst.source = source == null ? null : source.copy(); 2517 dst.name = name == null ? null : name.copy(); 2518 dst.kind = kind == null ? null : kind.copy(); 2519 if (type != null) { 2520 dst.type = new ArrayList<CodeType>(); 2521 for (CodeType i : type) 2522 dst.type.add(i.copy()); 2523 } 2524 ; 2525 if (package_ != null) { 2526 dst.package_ = new ArrayList<StringType>(); 2527 for (StringType i : package_) 2528 dst.package_.add(i.copy()); 2529 } 2530 ; 2531 dst.format = format == null ? null : format.copy(); 2532 if (page != null) { 2533 dst.page = new ArrayList<ImplementationGuidePageComponent>(); 2534 for (ImplementationGuidePageComponent i : page) 2535 dst.page.add(i.copy()); 2536 } 2537 ; 2538 return dst; 2539 } 2540 2541 @Override 2542 public boolean equalsDeep(Base other) { 2543 if (!super.equalsDeep(other)) 2544 return false; 2545 if (!(other instanceof ImplementationGuidePageComponent)) 2546 return false; 2547 ImplementationGuidePageComponent o = (ImplementationGuidePageComponent) other; 2548 return compareDeep(source, o.source, true) && compareDeep(name, o.name, true) && compareDeep(kind, o.kind, true) 2549 && compareDeep(type, o.type, true) && compareDeep(package_, o.package_, true) 2550 && compareDeep(format, o.format, true) && compareDeep(page, o.page, true); 2551 } 2552 2553 @Override 2554 public boolean equalsShallow(Base other) { 2555 if (!super.equalsShallow(other)) 2556 return false; 2557 if (!(other instanceof ImplementationGuidePageComponent)) 2558 return false; 2559 ImplementationGuidePageComponent o = (ImplementationGuidePageComponent) other; 2560 return compareValues(source, o.source, true) && compareValues(name, o.name, true) 2561 && compareValues(kind, o.kind, true) && compareValues(type, o.type, true) 2562 && compareValues(package_, o.package_, true) && compareValues(format, o.format, true); 2563 } 2564 2565 public boolean isEmpty() { 2566 return super.isEmpty() && (source == null || source.isEmpty()) && (name == null || name.isEmpty()) 2567 && (kind == null || kind.isEmpty()) && (type == null || type.isEmpty()) 2568 && (package_ == null || package_.isEmpty()) && (format == null || format.isEmpty()) 2569 && (page == null || page.isEmpty()); 2570 } 2571 2572 public String fhirType() { 2573 return "ImplementationGuide.page"; 2574 2575 } 2576 2577 } 2578 2579 /** 2580 * An absolute URL that is used to identify this implementation guide when it is 2581 * referenced in a specification, model, design or an instance. This SHALL be a 2582 * URL, SHOULD be globally unique, and SHOULD be an address at which this 2583 * implementation guide is (or will be) published. 2584 */ 2585 @Child(name = "url", type = { UriType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 2586 @Description(shortDefinition = "Absolute URL used to reference this Implementation Guide", formalDefinition = "An absolute URL that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this implementation guide is (or will be) published.") 2587 protected UriType url; 2588 2589 /** 2590 * The identifier that is used to identify this version of the Implementation 2591 * Guide when it is referenced in a specification, model, design or instance. 2592 * This is an arbitrary value managed by the Implementation Guide author 2593 * manually. 2594 */ 2595 @Child(name = "version", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2596 @Description(shortDefinition = "Logical id for this version of the Implementation Guide", formalDefinition = "The identifier that is used to identify this version of the Implementation Guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Implementation Guide author manually.") 2597 protected StringType version; 2598 2599 /** 2600 * A free text natural language name identifying the Implementation Guide. 2601 */ 2602 @Child(name = "name", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 2603 @Description(shortDefinition = "Informal name for this Implementation Guide", formalDefinition = "A free text natural language name identifying the Implementation Guide.") 2604 protected StringType name; 2605 2606 /** 2607 * The status of the Implementation Guide. 2608 */ 2609 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = true) 2610 @Description(shortDefinition = "draft | active | retired", formalDefinition = "The status of the Implementation Guide.") 2611 protected Enumeration<ConformanceResourceStatus> status; 2612 2613 /** 2614 * This Implementation Guide was authored for testing purposes (or 2615 * education/evaluation/marketing), and is not intended to be used for genuine 2616 * usage. 2617 */ 2618 @Child(name = "experimental", type = { 2619 BooleanType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2620 @Description(shortDefinition = "If for testing purposes, not real usage", formalDefinition = "This Implementation Guide was authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.") 2621 protected BooleanType experimental; 2622 2623 /** 2624 * The name of the individual or organization that published the implementation 2625 * guide. 2626 */ 2627 @Child(name = "publisher", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2628 @Description(shortDefinition = "Name of the publisher (Organization or individual)", formalDefinition = "The name of the individual or organization that published the implementation guide.") 2629 protected StringType publisher; 2630 2631 /** 2632 * Contacts to assist a user in finding and communicating with the publisher. 2633 */ 2634 @Child(name = "contact", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2635 @Description(shortDefinition = "Contact details of the publisher", formalDefinition = "Contacts to assist a user in finding and communicating with the publisher.") 2636 protected List<ImplementationGuideContactComponent> contact; 2637 2638 /** 2639 * The date this version of the implementation guide was published. The date 2640 * must change when the business version changes, if it does, and it must change 2641 * if the status code changes. In addition, it should change when the 2642 * substantive content of the implementation guide changes. 2643 */ 2644 @Child(name = "date", type = { DateTimeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 2645 @Description(shortDefinition = "Date for this version of the Implementation Guide", formalDefinition = "The date this version of the implementation guide was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes.") 2646 protected DateTimeType date; 2647 2648 /** 2649 * A free text natural language description of the Implementation Guide and its 2650 * use. 2651 */ 2652 @Child(name = "description", type = { 2653 StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 2654 @Description(shortDefinition = "Natural language description of the Implementation Guide", formalDefinition = "A free text natural language description of the Implementation Guide and its use.") 2655 protected StringType description; 2656 2657 /** 2658 * The content was developed with a focus and intent of supporting the contexts 2659 * that are listed. These terms may be used to assist with indexing and 2660 * searching of implementation guides. The most common use of this element is to 2661 * represent the country / jurisdiction for which this implementation guide was 2662 * defined. 2663 */ 2664 @Child(name = "useContext", type = { 2665 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2666 @Description(shortDefinition = "The implementation guide is intended to support these contexts", formalDefinition = "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of implementation guides. The most common use of this element is to represent the country / jurisdiction for which this implementation guide was defined.") 2667 protected List<CodeableConcept> useContext; 2668 2669 /** 2670 * A copyright statement relating to the implementation guide and/or its 2671 * contents. Copyright statements are generally legal restrictions on the use 2672 * and publishing of the details of the constraints and mappings. 2673 */ 2674 @Child(name = "copyright", type = { 2675 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 2676 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the details of the constraints and mappings.") 2677 protected StringType copyright; 2678 2679 /** 2680 * The version of the FHIR specification on which this ImplementationGuide is 2681 * based - this is the formal version of the specification, without the revision 2682 * number, e.g. [publication].[major].[minor], which is 1.0.2 for this version. 2683 */ 2684 @Child(name = "fhirVersion", type = { IdType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 2685 @Description(shortDefinition = "FHIR Version this Implementation Guide targets", formalDefinition = "The version of the FHIR specification on which this ImplementationGuide is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 1.0.2 for this version.") 2686 protected IdType fhirVersion; 2687 2688 /** 2689 * Another implementation guide that this implementation depends on. Typically, 2690 * an implementation guide uses value sets, profiles etc.defined in other 2691 * implementation guides. 2692 */ 2693 @Child(name = "dependency", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2694 @Description(shortDefinition = "Another Implementation guide this depends on", formalDefinition = "Another implementation guide that this implementation depends on. Typically, an implementation guide uses value sets, profiles etc.defined in other implementation guides.") 2695 protected List<ImplementationGuideDependencyComponent> dependency; 2696 2697 /** 2698 * A logical group of resources. Logical groups can be used when building pages. 2699 */ 2700 @Child(name = "package", type = {}, order = 13, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2701 @Description(shortDefinition = "Group of resources as used in .page.package", formalDefinition = "A logical group of resources. Logical groups can be used when building pages.") 2702 protected List<ImplementationGuidePackageComponent> package_; 2703 2704 /** 2705 * A set of profiles that all resources covered by this implementation guide 2706 * must conform to. 2707 */ 2708 @Child(name = "global", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2709 @Description(shortDefinition = "Profiles that apply globally", formalDefinition = "A set of profiles that all resources covered by this implementation guide must conform to.") 2710 protected List<ImplementationGuideGlobalComponent> global; 2711 2712 /** 2713 * A binary file that is included in the implementation guide when it is 2714 * published. 2715 */ 2716 @Child(name = "binary", type = { 2717 UriType.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2718 @Description(shortDefinition = "Image, css, script, etc.", formalDefinition = "A binary file that is included in the implementation guide when it is published.") 2719 protected List<UriType> binary; 2720 2721 /** 2722 * A page / section in the implementation guide. The root page is the 2723 * implementation guide home page. 2724 */ 2725 @Child(name = "page", type = {}, order = 16, min = 1, max = 1, modifier = false, summary = true) 2726 @Description(shortDefinition = "Page/Section in the Guide", formalDefinition = "A page / section in the implementation guide. The root page is the implementation guide home page.") 2727 protected ImplementationGuidePageComponent page; 2728 2729 private static final long serialVersionUID = 1150122415L; 2730 2731 /* 2732 * Constructor 2733 */ 2734 public ImplementationGuide() { 2735 super(); 2736 } 2737 2738 /* 2739 * Constructor 2740 */ 2741 public ImplementationGuide(UriType url, StringType name, Enumeration<ConformanceResourceStatus> status, 2742 ImplementationGuidePageComponent page) { 2743 super(); 2744 this.url = url; 2745 this.name = name; 2746 this.status = status; 2747 this.page = page; 2748 } 2749 2750 /** 2751 * @return {@link #url} (An absolute URL that is used to identify this 2752 * implementation guide when it is referenced in a specification, model, 2753 * design or an instance. This SHALL be a URL, SHOULD be globally 2754 * unique, and SHOULD be an address at which this implementation guide 2755 * is (or will be) published.). This is the underlying object with id, 2756 * value and extensions. The accessor "getUrl" gives direct access to 2757 * the value 2758 */ 2759 public UriType getUrlElement() { 2760 if (this.url == null) 2761 if (Configuration.errorOnAutoCreate()) 2762 throw new Error("Attempt to auto-create ImplementationGuide.url"); 2763 else if (Configuration.doAutoCreate()) 2764 this.url = new UriType(); // bb 2765 return this.url; 2766 } 2767 2768 public boolean hasUrlElement() { 2769 return this.url != null && !this.url.isEmpty(); 2770 } 2771 2772 public boolean hasUrl() { 2773 return this.url != null && !this.url.isEmpty(); 2774 } 2775 2776 /** 2777 * @param value {@link #url} (An absolute URL that is used to identify this 2778 * implementation guide when it is referenced in a specification, 2779 * model, design or an instance. This SHALL be a URL, SHOULD be 2780 * globally unique, and SHOULD be an address at which this 2781 * implementation guide is (or will be) published.). This is the 2782 * underlying object with id, value and extensions. The accessor 2783 * "getUrl" gives direct access to the value 2784 */ 2785 public ImplementationGuide setUrlElement(UriType value) { 2786 this.url = value; 2787 return this; 2788 } 2789 2790 /** 2791 * @return An absolute URL that is used to identify this implementation guide 2792 * when it is referenced in a specification, model, design or an 2793 * instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD 2794 * be an address at which this implementation guide is (or will be) 2795 * published. 2796 */ 2797 public String getUrl() { 2798 return this.url == null ? null : this.url.getValue(); 2799 } 2800 2801 /** 2802 * @param value An absolute URL that is used to identify this implementation 2803 * guide when it is referenced in a specification, model, design or 2804 * an instance. This SHALL be a URL, SHOULD be globally unique, and 2805 * SHOULD be an address at which this implementation guide is (or 2806 * will be) published. 2807 */ 2808 public ImplementationGuide setUrl(String value) { 2809 if (this.url == null) 2810 this.url = new UriType(); 2811 this.url.setValue(value); 2812 return this; 2813 } 2814 2815 /** 2816 * @return {@link #version} (The identifier that is used to identify this 2817 * version of the Implementation Guide when it is referenced in a 2818 * specification, model, design or instance. This is an arbitrary value 2819 * managed by the Implementation Guide author manually.). This is the 2820 * underlying object with id, value and extensions. The accessor 2821 * "getVersion" gives direct access to the value 2822 */ 2823 public StringType getVersionElement() { 2824 if (this.version == null) 2825 if (Configuration.errorOnAutoCreate()) 2826 throw new Error("Attempt to auto-create ImplementationGuide.version"); 2827 else if (Configuration.doAutoCreate()) 2828 this.version = new StringType(); // bb 2829 return this.version; 2830 } 2831 2832 public boolean hasVersionElement() { 2833 return this.version != null && !this.version.isEmpty(); 2834 } 2835 2836 public boolean hasVersion() { 2837 return this.version != null && !this.version.isEmpty(); 2838 } 2839 2840 /** 2841 * @param value {@link #version} (The identifier that is used to identify this 2842 * version of the Implementation Guide when it is referenced in a 2843 * specification, model, design or instance. This is an arbitrary 2844 * value managed by the Implementation Guide author manually.). 2845 * This is the underlying object with id, value and extensions. The 2846 * accessor "getVersion" gives direct access to the value 2847 */ 2848 public ImplementationGuide setVersionElement(StringType value) { 2849 this.version = value; 2850 return this; 2851 } 2852 2853 /** 2854 * @return The identifier that is used to identify this version of the 2855 * Implementation Guide when it is referenced in a specification, model, 2856 * design or instance. This is an arbitrary value managed by the 2857 * Implementation Guide author manually. 2858 */ 2859 public String getVersion() { 2860 return this.version == null ? null : this.version.getValue(); 2861 } 2862 2863 /** 2864 * @param value The identifier that is used to identify this version of the 2865 * Implementation Guide when it is referenced in a specification, 2866 * model, design or instance. This is an arbitrary value managed by 2867 * the Implementation Guide author manually. 2868 */ 2869 public ImplementationGuide setVersion(String value) { 2870 if (Utilities.noString(value)) 2871 this.version = null; 2872 else { 2873 if (this.version == null) 2874 this.version = new StringType(); 2875 this.version.setValue(value); 2876 } 2877 return this; 2878 } 2879 2880 /** 2881 * @return {@link #name} (A free text natural language name identifying the 2882 * Implementation Guide.). This is the underlying object with id, value 2883 * and extensions. The accessor "getName" gives direct access to the 2884 * value 2885 */ 2886 public StringType getNameElement() { 2887 if (this.name == null) 2888 if (Configuration.errorOnAutoCreate()) 2889 throw new Error("Attempt to auto-create ImplementationGuide.name"); 2890 else if (Configuration.doAutoCreate()) 2891 this.name = new StringType(); // bb 2892 return this.name; 2893 } 2894 2895 public boolean hasNameElement() { 2896 return this.name != null && !this.name.isEmpty(); 2897 } 2898 2899 public boolean hasName() { 2900 return this.name != null && !this.name.isEmpty(); 2901 } 2902 2903 /** 2904 * @param value {@link #name} (A free text natural language name identifying the 2905 * Implementation Guide.). This is the underlying object with id, 2906 * value and extensions. The accessor "getName" gives direct access 2907 * to the value 2908 */ 2909 public ImplementationGuide setNameElement(StringType value) { 2910 this.name = value; 2911 return this; 2912 } 2913 2914 /** 2915 * @return A free text natural language name identifying the Implementation 2916 * Guide. 2917 */ 2918 public String getName() { 2919 return this.name == null ? null : this.name.getValue(); 2920 } 2921 2922 /** 2923 * @param value A free text natural language name identifying the Implementation 2924 * Guide. 2925 */ 2926 public ImplementationGuide setName(String value) { 2927 if (this.name == null) 2928 this.name = new StringType(); 2929 this.name.setValue(value); 2930 return this; 2931 } 2932 2933 /** 2934 * @return {@link #status} (The status of the Implementation Guide.). This is 2935 * the underlying object with id, value and extensions. The accessor 2936 * "getStatus" gives direct access to the value 2937 */ 2938 public Enumeration<ConformanceResourceStatus> getStatusElement() { 2939 if (this.status == null) 2940 if (Configuration.errorOnAutoCreate()) 2941 throw new Error("Attempt to auto-create ImplementationGuide.status"); 2942 else if (Configuration.doAutoCreate()) 2943 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); // bb 2944 return this.status; 2945 } 2946 2947 public boolean hasStatusElement() { 2948 return this.status != null && !this.status.isEmpty(); 2949 } 2950 2951 public boolean hasStatus() { 2952 return this.status != null && !this.status.isEmpty(); 2953 } 2954 2955 /** 2956 * @param value {@link #status} (The status of the Implementation Guide.). This 2957 * is the underlying object with id, value and extensions. The 2958 * accessor "getStatus" gives direct access to the value 2959 */ 2960 public ImplementationGuide setStatusElement(Enumeration<ConformanceResourceStatus> value) { 2961 this.status = value; 2962 return this; 2963 } 2964 2965 /** 2966 * @return The status of the Implementation Guide. 2967 */ 2968 public ConformanceResourceStatus getStatus() { 2969 return this.status == null ? null : this.status.getValue(); 2970 } 2971 2972 /** 2973 * @param value The status of the Implementation Guide. 2974 */ 2975 public ImplementationGuide setStatus(ConformanceResourceStatus value) { 2976 if (this.status == null) 2977 this.status = new Enumeration<ConformanceResourceStatus>(new ConformanceResourceStatusEnumFactory()); 2978 this.status.setValue(value); 2979 return this; 2980 } 2981 2982 /** 2983 * @return {@link #experimental} (This Implementation Guide was authored for 2984 * testing purposes (or education/evaluation/marketing), and is not 2985 * intended to be used for genuine usage.). This is the underlying 2986 * object with id, value and extensions. The accessor "getExperimental" 2987 * gives direct access to the value 2988 */ 2989 public BooleanType getExperimentalElement() { 2990 if (this.experimental == null) 2991 if (Configuration.errorOnAutoCreate()) 2992 throw new Error("Attempt to auto-create ImplementationGuide.experimental"); 2993 else if (Configuration.doAutoCreate()) 2994 this.experimental = new BooleanType(); // bb 2995 return this.experimental; 2996 } 2997 2998 public boolean hasExperimentalElement() { 2999 return this.experimental != null && !this.experimental.isEmpty(); 3000 } 3001 3002 public boolean hasExperimental() { 3003 return this.experimental != null && !this.experimental.isEmpty(); 3004 } 3005 3006 /** 3007 * @param value {@link #experimental} (This Implementation Guide was authored 3008 * for testing purposes (or education/evaluation/marketing), and is 3009 * not intended to be used for genuine usage.). This is the 3010 * underlying object with id, value and extensions. The accessor 3011 * "getExperimental" gives direct access to the value 3012 */ 3013 public ImplementationGuide setExperimentalElement(BooleanType value) { 3014 this.experimental = value; 3015 return this; 3016 } 3017 3018 /** 3019 * @return This Implementation Guide was authored for testing purposes (or 3020 * education/evaluation/marketing), and is not intended to be used for 3021 * genuine usage. 3022 */ 3023 public boolean getExperimental() { 3024 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 3025 } 3026 3027 /** 3028 * @param value This Implementation Guide was authored for testing purposes (or 3029 * education/evaluation/marketing), and is not intended to be used 3030 * for genuine usage. 3031 */ 3032 public ImplementationGuide setExperimental(boolean value) { 3033 if (this.experimental == null) 3034 this.experimental = new BooleanType(); 3035 this.experimental.setValue(value); 3036 return this; 3037 } 3038 3039 /** 3040 * @return {@link #publisher} (The name of the individual or organization that 3041 * published the implementation guide.). This is the underlying object 3042 * with id, value and extensions. The accessor "getPublisher" gives 3043 * direct access to the value 3044 */ 3045 public StringType getPublisherElement() { 3046 if (this.publisher == null) 3047 if (Configuration.errorOnAutoCreate()) 3048 throw new Error("Attempt to auto-create ImplementationGuide.publisher"); 3049 else if (Configuration.doAutoCreate()) 3050 this.publisher = new StringType(); // bb 3051 return this.publisher; 3052 } 3053 3054 public boolean hasPublisherElement() { 3055 return this.publisher != null && !this.publisher.isEmpty(); 3056 } 3057 3058 public boolean hasPublisher() { 3059 return this.publisher != null && !this.publisher.isEmpty(); 3060 } 3061 3062 /** 3063 * @param value {@link #publisher} (The name of the individual or organization 3064 * that published the implementation guide.). This is the 3065 * underlying object with id, value and extensions. The accessor 3066 * "getPublisher" gives direct access to the value 3067 */ 3068 public ImplementationGuide setPublisherElement(StringType value) { 3069 this.publisher = value; 3070 return this; 3071 } 3072 3073 /** 3074 * @return The name of the individual or organization that published the 3075 * implementation guide. 3076 */ 3077 public String getPublisher() { 3078 return this.publisher == null ? null : this.publisher.getValue(); 3079 } 3080 3081 /** 3082 * @param value The name of the individual or organization that published the 3083 * implementation guide. 3084 */ 3085 public ImplementationGuide setPublisher(String value) { 3086 if (Utilities.noString(value)) 3087 this.publisher = null; 3088 else { 3089 if (this.publisher == null) 3090 this.publisher = new StringType(); 3091 this.publisher.setValue(value); 3092 } 3093 return this; 3094 } 3095 3096 /** 3097 * @return {@link #contact} (Contacts to assist a user in finding and 3098 * communicating with the publisher.) 3099 */ 3100 public List<ImplementationGuideContactComponent> getContact() { 3101 if (this.contact == null) 3102 this.contact = new ArrayList<ImplementationGuideContactComponent>(); 3103 return this.contact; 3104 } 3105 3106 public boolean hasContact() { 3107 if (this.contact == null) 3108 return false; 3109 for (ImplementationGuideContactComponent item : this.contact) 3110 if (!item.isEmpty()) 3111 return true; 3112 return false; 3113 } 3114 3115 /** 3116 * @return {@link #contact} (Contacts to assist a user in finding and 3117 * communicating with the publisher.) 3118 */ 3119 // syntactic sugar 3120 public ImplementationGuideContactComponent addContact() { // 3 3121 ImplementationGuideContactComponent t = new ImplementationGuideContactComponent(); 3122 if (this.contact == null) 3123 this.contact = new ArrayList<ImplementationGuideContactComponent>(); 3124 this.contact.add(t); 3125 return t; 3126 } 3127 3128 // syntactic sugar 3129 public ImplementationGuide addContact(ImplementationGuideContactComponent t) { // 3 3130 if (t == null) 3131 return this; 3132 if (this.contact == null) 3133 this.contact = new ArrayList<ImplementationGuideContactComponent>(); 3134 this.contact.add(t); 3135 return this; 3136 } 3137 3138 /** 3139 * @return {@link #date} (The date this version of the implementation guide was 3140 * published. The date must change when the business version changes, if 3141 * it does, and it must change if the status code changes. In addition, 3142 * it should change when the substantive content of the implementation 3143 * guide changes.). This is the underlying object with id, value and 3144 * extensions. The accessor "getDate" gives direct access to the value 3145 */ 3146 public DateTimeType getDateElement() { 3147 if (this.date == null) 3148 if (Configuration.errorOnAutoCreate()) 3149 throw new Error("Attempt to auto-create ImplementationGuide.date"); 3150 else if (Configuration.doAutoCreate()) 3151 this.date = new DateTimeType(); // bb 3152 return this.date; 3153 } 3154 3155 public boolean hasDateElement() { 3156 return this.date != null && !this.date.isEmpty(); 3157 } 3158 3159 public boolean hasDate() { 3160 return this.date != null && !this.date.isEmpty(); 3161 } 3162 3163 /** 3164 * @param value {@link #date} (The date this version of the implementation guide 3165 * was published. The date must change when the business version 3166 * changes, if it does, and it must change if the status code 3167 * changes. In addition, it should change when the substantive 3168 * content of the implementation guide changes.). This is the 3169 * underlying object with id, value and extensions. The accessor 3170 * "getDate" gives direct access to the value 3171 */ 3172 public ImplementationGuide setDateElement(DateTimeType value) { 3173 this.date = value; 3174 return this; 3175 } 3176 3177 /** 3178 * @return The date this version of the implementation guide was published. The 3179 * date must change when the business version changes, if it does, and 3180 * it must change if the status code changes. In addition, it should 3181 * change when the substantive content of the implementation guide 3182 * changes. 3183 */ 3184 public Date getDate() { 3185 return this.date == null ? null : this.date.getValue(); 3186 } 3187 3188 /** 3189 * @param value The date this version of the implementation guide was published. 3190 * The date must change when the business version changes, if it 3191 * does, and it must change if the status code changes. In 3192 * addition, it should change when the substantive content of the 3193 * implementation guide changes. 3194 */ 3195 public ImplementationGuide setDate(Date value) { 3196 if (value == null) 3197 this.date = null; 3198 else { 3199 if (this.date == null) 3200 this.date = new DateTimeType(); 3201 this.date.setValue(value); 3202 } 3203 return this; 3204 } 3205 3206 /** 3207 * @return {@link #description} (A free text natural language description of the 3208 * Implementation Guide and its use.). This is the underlying object 3209 * with id, value and extensions. The accessor "getDescription" gives 3210 * direct access to the value 3211 */ 3212 public StringType getDescriptionElement() { 3213 if (this.description == null) 3214 if (Configuration.errorOnAutoCreate()) 3215 throw new Error("Attempt to auto-create ImplementationGuide.description"); 3216 else if (Configuration.doAutoCreate()) 3217 this.description = new StringType(); // bb 3218 return this.description; 3219 } 3220 3221 public boolean hasDescriptionElement() { 3222 return this.description != null && !this.description.isEmpty(); 3223 } 3224 3225 public boolean hasDescription() { 3226 return this.description != null && !this.description.isEmpty(); 3227 } 3228 3229 /** 3230 * @param value {@link #description} (A free text natural language description 3231 * of the Implementation Guide and its use.). This is the 3232 * underlying object with id, value and extensions. The accessor 3233 * "getDescription" gives direct access to the value 3234 */ 3235 public ImplementationGuide setDescriptionElement(StringType value) { 3236 this.description = value; 3237 return this; 3238 } 3239 3240 /** 3241 * @return A free text natural language description of the Implementation Guide 3242 * and its use. 3243 */ 3244 public String getDescription() { 3245 return this.description == null ? null : this.description.getValue(); 3246 } 3247 3248 /** 3249 * @param value A free text natural language description of the Implementation 3250 * Guide and its use. 3251 */ 3252 public ImplementationGuide setDescription(String value) { 3253 if (Utilities.noString(value)) 3254 this.description = null; 3255 else { 3256 if (this.description == null) 3257 this.description = new StringType(); 3258 this.description.setValue(value); 3259 } 3260 return this; 3261 } 3262 3263 /** 3264 * @return {@link #useContext} (The content was developed with a focus and 3265 * intent of supporting the contexts that are listed. These terms may be 3266 * used to assist with indexing and searching of implementation guides. 3267 * The most common use of this element is to represent the country / 3268 * jurisdiction for which this implementation guide was defined.) 3269 */ 3270 public List<CodeableConcept> getUseContext() { 3271 if (this.useContext == null) 3272 this.useContext = new ArrayList<CodeableConcept>(); 3273 return this.useContext; 3274 } 3275 3276 public boolean hasUseContext() { 3277 if (this.useContext == null) 3278 return false; 3279 for (CodeableConcept item : this.useContext) 3280 if (!item.isEmpty()) 3281 return true; 3282 return false; 3283 } 3284 3285 /** 3286 * @return {@link #useContext} (The content was developed with a focus and 3287 * intent of supporting the contexts that are listed. These terms may be 3288 * used to assist with indexing and searching of implementation guides. 3289 * The most common use of this element is to represent the country / 3290 * jurisdiction for which this implementation guide was defined.) 3291 */ 3292 // syntactic sugar 3293 public CodeableConcept addUseContext() { // 3 3294 CodeableConcept t = new CodeableConcept(); 3295 if (this.useContext == null) 3296 this.useContext = new ArrayList<CodeableConcept>(); 3297 this.useContext.add(t); 3298 return t; 3299 } 3300 3301 // syntactic sugar 3302 public ImplementationGuide addUseContext(CodeableConcept t) { // 3 3303 if (t == null) 3304 return this; 3305 if (this.useContext == null) 3306 this.useContext = new ArrayList<CodeableConcept>(); 3307 this.useContext.add(t); 3308 return this; 3309 } 3310 3311 /** 3312 * @return {@link #copyright} (A copyright statement relating to the 3313 * implementation guide and/or its contents. Copyright statements are 3314 * generally legal restrictions on the use and publishing of the details 3315 * of the constraints and mappings.). This is the underlying object with 3316 * id, value and extensions. The accessor "getCopyright" gives direct 3317 * access to the value 3318 */ 3319 public StringType getCopyrightElement() { 3320 if (this.copyright == null) 3321 if (Configuration.errorOnAutoCreate()) 3322 throw new Error("Attempt to auto-create ImplementationGuide.copyright"); 3323 else if (Configuration.doAutoCreate()) 3324 this.copyright = new StringType(); // bb 3325 return this.copyright; 3326 } 3327 3328 public boolean hasCopyrightElement() { 3329 return this.copyright != null && !this.copyright.isEmpty(); 3330 } 3331 3332 public boolean hasCopyright() { 3333 return this.copyright != null && !this.copyright.isEmpty(); 3334 } 3335 3336 /** 3337 * @param value {@link #copyright} (A copyright statement relating to the 3338 * implementation guide and/or its contents. Copyright statements 3339 * are generally legal restrictions on the use and publishing of 3340 * the details of the constraints and mappings.). This is the 3341 * underlying object with id, value and extensions. The accessor 3342 * "getCopyright" gives direct access to the value 3343 */ 3344 public ImplementationGuide setCopyrightElement(StringType value) { 3345 this.copyright = value; 3346 return this; 3347 } 3348 3349 /** 3350 * @return A copyright statement relating to the implementation guide and/or its 3351 * contents. Copyright statements are generally legal restrictions on 3352 * the use and publishing of the details of the constraints and 3353 * mappings. 3354 */ 3355 public String getCopyright() { 3356 return this.copyright == null ? null : this.copyright.getValue(); 3357 } 3358 3359 /** 3360 * @param value A copyright statement relating to the implementation guide 3361 * and/or its contents. Copyright statements are generally legal 3362 * restrictions on the use and publishing of the details of the 3363 * constraints and mappings. 3364 */ 3365 public ImplementationGuide setCopyright(String value) { 3366 if (Utilities.noString(value)) 3367 this.copyright = null; 3368 else { 3369 if (this.copyright == null) 3370 this.copyright = new StringType(); 3371 this.copyright.setValue(value); 3372 } 3373 return this; 3374 } 3375 3376 /** 3377 * @return {@link #fhirVersion} (The version of the FHIR specification on which 3378 * this ImplementationGuide is based - this is the formal version of the 3379 * specification, without the revision number, e.g. 3380 * [publication].[major].[minor], which is 1.0.2 for this version.). 3381 * This is the underlying object with id, value and extensions. The 3382 * accessor "getFhirVersion" gives direct access to the value 3383 */ 3384 public IdType getFhirVersionElement() { 3385 if (this.fhirVersion == null) 3386 if (Configuration.errorOnAutoCreate()) 3387 throw new Error("Attempt to auto-create ImplementationGuide.fhirVersion"); 3388 else if (Configuration.doAutoCreate()) 3389 this.fhirVersion = new IdType(); // bb 3390 return this.fhirVersion; 3391 } 3392 3393 public boolean hasFhirVersionElement() { 3394 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 3395 } 3396 3397 public boolean hasFhirVersion() { 3398 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 3399 } 3400 3401 /** 3402 * @param value {@link #fhirVersion} (The version of the FHIR specification on 3403 * which this ImplementationGuide is based - this is the formal 3404 * version of the specification, without the revision number, e.g. 3405 * [publication].[major].[minor], which is 1.0.2 for this 3406 * version.). This is the underlying object with id, value and 3407 * extensions. The accessor "getFhirVersion" gives direct access to 3408 * the value 3409 */ 3410 public ImplementationGuide setFhirVersionElement(IdType value) { 3411 this.fhirVersion = value; 3412 return this; 3413 } 3414 3415 /** 3416 * @return The version of the FHIR specification on which this 3417 * ImplementationGuide is based - this is the formal version of the 3418 * specification, without the revision number, e.g. 3419 * [publication].[major].[minor], which is 1.0.2 for this version. 3420 */ 3421 public String getFhirVersion() { 3422 return this.fhirVersion == null ? null : this.fhirVersion.getValue(); 3423 } 3424 3425 /** 3426 * @param value The version of the FHIR specification on which this 3427 * ImplementationGuide is based - this is the formal version of the 3428 * specification, without the revision number, e.g. 3429 * [publication].[major].[minor], which is 1.0.2 for this version. 3430 */ 3431 public ImplementationGuide setFhirVersion(String value) { 3432 if (Utilities.noString(value)) 3433 this.fhirVersion = null; 3434 else { 3435 if (this.fhirVersion == null) 3436 this.fhirVersion = new IdType(); 3437 this.fhirVersion.setValue(value); 3438 } 3439 return this; 3440 } 3441 3442 /** 3443 * @return {@link #dependency} (Another implementation guide that this 3444 * implementation depends on. Typically, an implementation guide uses 3445 * value sets, profiles etc.defined in other implementation guides.) 3446 */ 3447 public List<ImplementationGuideDependencyComponent> getDependency() { 3448 if (this.dependency == null) 3449 this.dependency = new ArrayList<ImplementationGuideDependencyComponent>(); 3450 return this.dependency; 3451 } 3452 3453 public boolean hasDependency() { 3454 if (this.dependency == null) 3455 return false; 3456 for (ImplementationGuideDependencyComponent item : this.dependency) 3457 if (!item.isEmpty()) 3458 return true; 3459 return false; 3460 } 3461 3462 /** 3463 * @return {@link #dependency} (Another implementation guide that this 3464 * implementation depends on. Typically, an implementation guide uses 3465 * value sets, profiles etc.defined in other implementation guides.) 3466 */ 3467 // syntactic sugar 3468 public ImplementationGuideDependencyComponent addDependency() { // 3 3469 ImplementationGuideDependencyComponent t = new ImplementationGuideDependencyComponent(); 3470 if (this.dependency == null) 3471 this.dependency = new ArrayList<ImplementationGuideDependencyComponent>(); 3472 this.dependency.add(t); 3473 return t; 3474 } 3475 3476 // syntactic sugar 3477 public ImplementationGuide addDependency(ImplementationGuideDependencyComponent t) { // 3 3478 if (t == null) 3479 return this; 3480 if (this.dependency == null) 3481 this.dependency = new ArrayList<ImplementationGuideDependencyComponent>(); 3482 this.dependency.add(t); 3483 return this; 3484 } 3485 3486 /** 3487 * @return {@link #package_} (A logical group of resources. Logical groups can 3488 * be used when building pages.) 3489 */ 3490 public List<ImplementationGuidePackageComponent> getPackage() { 3491 if (this.package_ == null) 3492 this.package_ = new ArrayList<ImplementationGuidePackageComponent>(); 3493 return this.package_; 3494 } 3495 3496 public boolean hasPackage() { 3497 if (this.package_ == null) 3498 return false; 3499 for (ImplementationGuidePackageComponent item : this.package_) 3500 if (!item.isEmpty()) 3501 return true; 3502 return false; 3503 } 3504 3505 /** 3506 * @return {@link #package_} (A logical group of resources. Logical groups can 3507 * be used when building pages.) 3508 */ 3509 // syntactic sugar 3510 public ImplementationGuidePackageComponent addPackage() { // 3 3511 ImplementationGuidePackageComponent t = new ImplementationGuidePackageComponent(); 3512 if (this.package_ == null) 3513 this.package_ = new ArrayList<ImplementationGuidePackageComponent>(); 3514 this.package_.add(t); 3515 return t; 3516 } 3517 3518 // syntactic sugar 3519 public ImplementationGuide addPackage(ImplementationGuidePackageComponent t) { // 3 3520 if (t == null) 3521 return this; 3522 if (this.package_ == null) 3523 this.package_ = new ArrayList<ImplementationGuidePackageComponent>(); 3524 this.package_.add(t); 3525 return this; 3526 } 3527 3528 /** 3529 * @return {@link #global} (A set of profiles that all resources covered by this 3530 * implementation guide must conform to.) 3531 */ 3532 public List<ImplementationGuideGlobalComponent> getGlobal() { 3533 if (this.global == null) 3534 this.global = new ArrayList<ImplementationGuideGlobalComponent>(); 3535 return this.global; 3536 } 3537 3538 public boolean hasGlobal() { 3539 if (this.global == null) 3540 return false; 3541 for (ImplementationGuideGlobalComponent item : this.global) 3542 if (!item.isEmpty()) 3543 return true; 3544 return false; 3545 } 3546 3547 /** 3548 * @return {@link #global} (A set of profiles that all resources covered by this 3549 * implementation guide must conform to.) 3550 */ 3551 // syntactic sugar 3552 public ImplementationGuideGlobalComponent addGlobal() { // 3 3553 ImplementationGuideGlobalComponent t = new ImplementationGuideGlobalComponent(); 3554 if (this.global == null) 3555 this.global = new ArrayList<ImplementationGuideGlobalComponent>(); 3556 this.global.add(t); 3557 return t; 3558 } 3559 3560 // syntactic sugar 3561 public ImplementationGuide addGlobal(ImplementationGuideGlobalComponent t) { // 3 3562 if (t == null) 3563 return this; 3564 if (this.global == null) 3565 this.global = new ArrayList<ImplementationGuideGlobalComponent>(); 3566 this.global.add(t); 3567 return this; 3568 } 3569 3570 /** 3571 * @return {@link #binary} (A binary file that is included in the implementation 3572 * guide when it is published.) 3573 */ 3574 public List<UriType> getBinary() { 3575 if (this.binary == null) 3576 this.binary = new ArrayList<UriType>(); 3577 return this.binary; 3578 } 3579 3580 public boolean hasBinary() { 3581 if (this.binary == null) 3582 return false; 3583 for (UriType item : this.binary) 3584 if (!item.isEmpty()) 3585 return true; 3586 return false; 3587 } 3588 3589 /** 3590 * @return {@link #binary} (A binary file that is included in the implementation 3591 * guide when it is published.) 3592 */ 3593 // syntactic sugar 3594 public UriType addBinaryElement() {// 2 3595 UriType t = new UriType(); 3596 if (this.binary == null) 3597 this.binary = new ArrayList<UriType>(); 3598 this.binary.add(t); 3599 return t; 3600 } 3601 3602 /** 3603 * @param value {@link #binary} (A binary file that is included in the 3604 * implementation guide when it is published.) 3605 */ 3606 public ImplementationGuide addBinary(String value) { // 1 3607 UriType t = new UriType(); 3608 t.setValue(value); 3609 if (this.binary == null) 3610 this.binary = new ArrayList<UriType>(); 3611 this.binary.add(t); 3612 return this; 3613 } 3614 3615 /** 3616 * @param value {@link #binary} (A binary file that is included in the 3617 * implementation guide when it is published.) 3618 */ 3619 public boolean hasBinary(String value) { 3620 if (this.binary == null) 3621 return false; 3622 for (UriType v : this.binary) 3623 if (v.equals(value)) // uri 3624 return true; 3625 return false; 3626 } 3627 3628 /** 3629 * @return {@link #page} (A page / section in the implementation guide. The root 3630 * page is the implementation guide home page.) 3631 */ 3632 public ImplementationGuidePageComponent getPage() { 3633 if (this.page == null) 3634 if (Configuration.errorOnAutoCreate()) 3635 throw new Error("Attempt to auto-create ImplementationGuide.page"); 3636 else if (Configuration.doAutoCreate()) 3637 this.page = new ImplementationGuidePageComponent(); // cc 3638 return this.page; 3639 } 3640 3641 public boolean hasPage() { 3642 return this.page != null && !this.page.isEmpty(); 3643 } 3644 3645 /** 3646 * @param value {@link #page} (A page / section in the implementation guide. The 3647 * root page is the implementation guide home page.) 3648 */ 3649 public ImplementationGuide setPage(ImplementationGuidePageComponent value) { 3650 this.page = value; 3651 return this; 3652 } 3653 3654 protected void listChildren(List<Property> childrenList) { 3655 super.listChildren(childrenList); 3656 childrenList.add(new Property("url", "uri", 3657 "An absolute URL that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this implementation guide is (or will be) published.", 3658 0, java.lang.Integer.MAX_VALUE, url)); 3659 childrenList.add(new Property("version", "string", 3660 "The identifier that is used to identify this version of the Implementation Guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Implementation Guide author manually.", 3661 0, java.lang.Integer.MAX_VALUE, version)); 3662 childrenList 3663 .add(new Property("name", "string", "A free text natural language name identifying the Implementation Guide.", 3664 0, java.lang.Integer.MAX_VALUE, name)); 3665 childrenList.add(new Property("status", "code", "The status of the Implementation Guide.", 0, 3666 java.lang.Integer.MAX_VALUE, status)); 3667 childrenList.add(new Property("experimental", "boolean", 3668 "This Implementation Guide was authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 3669 0, java.lang.Integer.MAX_VALUE, experimental)); 3670 childrenList.add(new Property("publisher", "string", 3671 "The name of the individual or organization that published the implementation guide.", 0, 3672 java.lang.Integer.MAX_VALUE, publisher)); 3673 childrenList 3674 .add(new Property("contact", "", "Contacts to assist a user in finding and communicating with the publisher.", 3675 0, java.lang.Integer.MAX_VALUE, contact)); 3676 childrenList.add(new Property("date", "dateTime", 3677 "The date this version of the implementation guide was published. The date must change when the business version changes, if it does, and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes.", 3678 0, java.lang.Integer.MAX_VALUE, date)); 3679 childrenList.add(new Property("description", "string", 3680 "A free text natural language description of the Implementation Guide and its use.", 0, 3681 java.lang.Integer.MAX_VALUE, description)); 3682 childrenList.add(new Property("useContext", "CodeableConcept", 3683 "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of implementation guides. The most common use of this element is to represent the country / jurisdiction for which this implementation guide was defined.", 3684 0, java.lang.Integer.MAX_VALUE, useContext)); 3685 childrenList.add(new Property("copyright", "string", 3686 "A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the details of the constraints and mappings.", 3687 0, java.lang.Integer.MAX_VALUE, copyright)); 3688 childrenList.add(new Property("fhirVersion", "id", 3689 "The version of the FHIR specification on which this ImplementationGuide is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 1.0.2 for this version.", 3690 0, java.lang.Integer.MAX_VALUE, fhirVersion)); 3691 childrenList.add(new Property("dependency", "", 3692 "Another implementation guide that this implementation depends on. Typically, an implementation guide uses value sets, profiles etc.defined in other implementation guides.", 3693 0, java.lang.Integer.MAX_VALUE, dependency)); 3694 childrenList.add( 3695 new Property("package", "", "A logical group of resources. Logical groups can be used when building pages.", 0, 3696 java.lang.Integer.MAX_VALUE, package_)); 3697 childrenList.add(new Property("global", "", 3698 "A set of profiles that all resources covered by this implementation guide must conform to.", 0, 3699 java.lang.Integer.MAX_VALUE, global)); 3700 childrenList.add(new Property("binary", "uri", 3701 "A binary file that is included in the implementation guide when it is published.", 0, 3702 java.lang.Integer.MAX_VALUE, binary)); 3703 childrenList.add(new Property("page", "", 3704 "A page / section in the implementation guide. The root page is the implementation guide home page.", 0, 3705 java.lang.Integer.MAX_VALUE, page)); 3706 } 3707 3708 @Override 3709 public void setProperty(String name, Base value) throws FHIRException { 3710 if (name.equals("url")) 3711 this.url = castToUri(value); // UriType 3712 else if (name.equals("version")) 3713 this.version = castToString(value); // StringType 3714 else if (name.equals("name")) 3715 this.name = castToString(value); // StringType 3716 else if (name.equals("status")) 3717 this.status = new ConformanceResourceStatusEnumFactory().fromType(value); // Enumeration<ConformanceResourceStatus> 3718 else if (name.equals("experimental")) 3719 this.experimental = castToBoolean(value); // BooleanType 3720 else if (name.equals("publisher")) 3721 this.publisher = castToString(value); // StringType 3722 else if (name.equals("contact")) 3723 this.getContact().add((ImplementationGuideContactComponent) value); 3724 else if (name.equals("date")) 3725 this.date = castToDateTime(value); // DateTimeType 3726 else if (name.equals("description")) 3727 this.description = castToString(value); // StringType 3728 else if (name.equals("useContext")) 3729 this.getUseContext().add(castToCodeableConcept(value)); 3730 else if (name.equals("copyright")) 3731 this.copyright = castToString(value); // StringType 3732 else if (name.equals("fhirVersion")) 3733 this.fhirVersion = castToId(value); // IdType 3734 else if (name.equals("dependency")) 3735 this.getDependency().add((ImplementationGuideDependencyComponent) value); 3736 else if (name.equals("package")) 3737 this.getPackage().add((ImplementationGuidePackageComponent) value); 3738 else if (name.equals("global")) 3739 this.getGlobal().add((ImplementationGuideGlobalComponent) value); 3740 else if (name.equals("binary")) 3741 this.getBinary().add(castToUri(value)); 3742 else if (name.equals("page")) 3743 this.page = (ImplementationGuidePageComponent) value; // ImplementationGuidePageComponent 3744 else 3745 super.setProperty(name, value); 3746 } 3747 3748 @Override 3749 public Base addChild(String name) throws FHIRException { 3750 if (name.equals("url")) { 3751 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.url"); 3752 } else if (name.equals("version")) { 3753 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.version"); 3754 } else if (name.equals("name")) { 3755 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.name"); 3756 } else if (name.equals("status")) { 3757 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.status"); 3758 } else if (name.equals("experimental")) { 3759 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.experimental"); 3760 } else if (name.equals("publisher")) { 3761 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.publisher"); 3762 } else if (name.equals("contact")) { 3763 return addContact(); 3764 } else if (name.equals("date")) { 3765 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.date"); 3766 } else if (name.equals("description")) { 3767 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.description"); 3768 } else if (name.equals("useContext")) { 3769 return addUseContext(); 3770 } else if (name.equals("copyright")) { 3771 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.copyright"); 3772 } else if (name.equals("fhirVersion")) { 3773 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.fhirVersion"); 3774 } else if (name.equals("dependency")) { 3775 return addDependency(); 3776 } else if (name.equals("package")) { 3777 return addPackage(); 3778 } else if (name.equals("global")) { 3779 return addGlobal(); 3780 } else if (name.equals("binary")) { 3781 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.binary"); 3782 } else if (name.equals("page")) { 3783 this.page = new ImplementationGuidePageComponent(); 3784 return this.page; 3785 } else 3786 return super.addChild(name); 3787 } 3788 3789 public String fhirType() { 3790 return "ImplementationGuide"; 3791 3792 } 3793 3794 public ImplementationGuide copy() { 3795 ImplementationGuide dst = new ImplementationGuide(); 3796 copyValues(dst); 3797 dst.url = url == null ? null : url.copy(); 3798 dst.version = version == null ? null : version.copy(); 3799 dst.name = name == null ? null : name.copy(); 3800 dst.status = status == null ? null : status.copy(); 3801 dst.experimental = experimental == null ? null : experimental.copy(); 3802 dst.publisher = publisher == null ? null : publisher.copy(); 3803 if (contact != null) { 3804 dst.contact = new ArrayList<ImplementationGuideContactComponent>(); 3805 for (ImplementationGuideContactComponent i : contact) 3806 dst.contact.add(i.copy()); 3807 } 3808 ; 3809 dst.date = date == null ? null : date.copy(); 3810 dst.description = description == null ? null : description.copy(); 3811 if (useContext != null) { 3812 dst.useContext = new ArrayList<CodeableConcept>(); 3813 for (CodeableConcept i : useContext) 3814 dst.useContext.add(i.copy()); 3815 } 3816 ; 3817 dst.copyright = copyright == null ? null : copyright.copy(); 3818 dst.fhirVersion = fhirVersion == null ? null : fhirVersion.copy(); 3819 if (dependency != null) { 3820 dst.dependency = new ArrayList<ImplementationGuideDependencyComponent>(); 3821 for (ImplementationGuideDependencyComponent i : dependency) 3822 dst.dependency.add(i.copy()); 3823 } 3824 ; 3825 if (package_ != null) { 3826 dst.package_ = new ArrayList<ImplementationGuidePackageComponent>(); 3827 for (ImplementationGuidePackageComponent i : package_) 3828 dst.package_.add(i.copy()); 3829 } 3830 ; 3831 if (global != null) { 3832 dst.global = new ArrayList<ImplementationGuideGlobalComponent>(); 3833 for (ImplementationGuideGlobalComponent i : global) 3834 dst.global.add(i.copy()); 3835 } 3836 ; 3837 if (binary != null) { 3838 dst.binary = new ArrayList<UriType>(); 3839 for (UriType i : binary) 3840 dst.binary.add(i.copy()); 3841 } 3842 ; 3843 dst.page = page == null ? null : page.copy(); 3844 return dst; 3845 } 3846 3847 protected ImplementationGuide typedCopy() { 3848 return copy(); 3849 } 3850 3851 @Override 3852 public boolean equalsDeep(Base other) { 3853 if (!super.equalsDeep(other)) 3854 return false; 3855 if (!(other instanceof ImplementationGuide)) 3856 return false; 3857 ImplementationGuide o = (ImplementationGuide) other; 3858 return compareDeep(url, o.url, true) && compareDeep(version, o.version, true) && compareDeep(name, o.name, true) 3859 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 3860 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 3861 && compareDeep(date, o.date, true) && compareDeep(description, o.description, true) 3862 && compareDeep(useContext, o.useContext, true) && compareDeep(copyright, o.copyright, true) 3863 && compareDeep(fhirVersion, o.fhirVersion, true) && compareDeep(dependency, o.dependency, true) 3864 && compareDeep(package_, o.package_, true) && compareDeep(global, o.global, true) 3865 && compareDeep(binary, o.binary, true) && compareDeep(page, o.page, true); 3866 } 3867 3868 @Override 3869 public boolean equalsShallow(Base other) { 3870 if (!super.equalsShallow(other)) 3871 return false; 3872 if (!(other instanceof ImplementationGuide)) 3873 return false; 3874 ImplementationGuide o = (ImplementationGuide) other; 3875 return compareValues(url, o.url, true) && compareValues(version, o.version, true) 3876 && compareValues(name, o.name, true) && compareValues(status, o.status, true) 3877 && compareValues(experimental, o.experimental, true) && compareValues(publisher, o.publisher, true) 3878 && compareValues(date, o.date, true) && compareValues(description, o.description, true) 3879 && compareValues(copyright, o.copyright, true) && compareValues(fhirVersion, o.fhirVersion, true) 3880 && compareValues(binary, o.binary, true); 3881 } 3882 3883 public boolean isEmpty() { 3884 return super.isEmpty() && (url == null || url.isEmpty()) && (version == null || version.isEmpty()) 3885 && (name == null || name.isEmpty()) && (status == null || status.isEmpty()) 3886 && (experimental == null || experimental.isEmpty()) && (publisher == null || publisher.isEmpty()) 3887 && (contact == null || contact.isEmpty()) && (date == null || date.isEmpty()) 3888 && (description == null || description.isEmpty()) && (useContext == null || useContext.isEmpty()) 3889 && (copyright == null || copyright.isEmpty()) && (fhirVersion == null || fhirVersion.isEmpty()) 3890 && (dependency == null || dependency.isEmpty()) && (package_ == null || package_.isEmpty()) 3891 && (global == null || global.isEmpty()) && (binary == null || binary.isEmpty()) 3892 && (page == null || page.isEmpty()); 3893 } 3894 3895 @Override 3896 public ResourceType getResourceType() { 3897 return ResourceType.ImplementationGuide; 3898 } 3899 3900 @SearchParamDefinition(name = "date", path = "ImplementationGuide.date", description = "The implementation guide publication date", type = "date") 3901 public static final String SP_DATE = "date"; 3902 @SearchParamDefinition(name = "dependency", path = "ImplementationGuide.dependency.uri", description = "Where to find dependency", type = "uri") 3903 public static final String SP_DEPENDENCY = "dependency"; 3904 @SearchParamDefinition(name = "name", path = "ImplementationGuide.name", description = "Name of the implementation guide", type = "string") 3905 public static final String SP_NAME = "name"; 3906 @SearchParamDefinition(name = "context", path = "ImplementationGuide.useContext", description = "A use context assigned to the structure", type = "token") 3907 public static final String SP_CONTEXT = "context"; 3908 @SearchParamDefinition(name = "publisher", path = "ImplementationGuide.publisher", description = "Name of the publisher of the implementation guide", type = "string") 3909 public static final String SP_PUBLISHER = "publisher"; 3910 @SearchParamDefinition(name = "description", path = "ImplementationGuide.description", description = "Text search in the description of the implementation guide", type = "string") 3911 public static final String SP_DESCRIPTION = "description"; 3912 @SearchParamDefinition(name = "experimental", path = "ImplementationGuide.experimental", description = "If for testing purposes, not real usage", type = "token") 3913 public static final String SP_EXPERIMENTAL = "experimental"; 3914 @SearchParamDefinition(name = "version", path = "ImplementationGuide.version", description = "The version identifier of the implementation guide", type = "token") 3915 public static final String SP_VERSION = "version"; 3916 @SearchParamDefinition(name = "url", path = "ImplementationGuide.url", description = "Absolute URL used to reference this Implementation Guide", type = "uri") 3917 public static final String SP_URL = "url"; 3918 @SearchParamDefinition(name = "status", path = "ImplementationGuide.status", description = "The current status of the implementation guide", type = "token") 3919 public static final String SP_STATUS = "status"; 3920 3921}