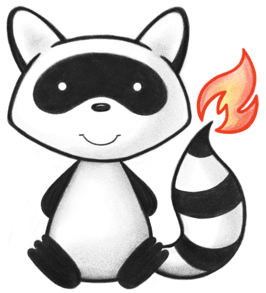
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * A set of information summarized from a list of other resources. 048 */ 049@ResourceDef(name = "List", profile = "http://hl7.org/fhir/Profile/List_") 050public class List_ extends DomainResource { 051 052 public enum ListStatus { 053 /** 054 * The list is considered to be an active part of the patient's record. 055 */ 056 CURRENT, 057 /** 058 * The list is "old" and should no longer be considered accurate or relevant. 059 */ 060 RETIRED, 061 /** 062 * The list was never accurate. It is retained for medico-legal purposes only. 063 */ 064 ENTEREDINERROR, 065 /** 066 * added to help the parsers 067 */ 068 NULL; 069 070 public static ListStatus fromCode(String codeString) throws FHIRException { 071 if (codeString == null || "".equals(codeString)) 072 return null; 073 if ("current".equals(codeString)) 074 return CURRENT; 075 if ("retired".equals(codeString)) 076 return RETIRED; 077 if ("entered-in-error".equals(codeString)) 078 return ENTEREDINERROR; 079 throw new FHIRException("Unknown ListStatus code '" + codeString + "'"); 080 } 081 082 public String toCode() { 083 switch (this) { 084 case CURRENT: 085 return "current"; 086 case RETIRED: 087 return "retired"; 088 case ENTEREDINERROR: 089 return "entered-in-error"; 090 case NULL: 091 return null; 092 default: 093 return "?"; 094 } 095 } 096 097 public String getSystem() { 098 switch (this) { 099 case CURRENT: 100 return "http://hl7.org/fhir/list-status"; 101 case RETIRED: 102 return "http://hl7.org/fhir/list-status"; 103 case ENTEREDINERROR: 104 return "http://hl7.org/fhir/list-status"; 105 case NULL: 106 return null; 107 default: 108 return "?"; 109 } 110 } 111 112 public String getDefinition() { 113 switch (this) { 114 case CURRENT: 115 return "The list is considered to be an active part of the patient's record."; 116 case RETIRED: 117 return "The list is \"old\" and should no longer be considered accurate or relevant."; 118 case ENTEREDINERROR: 119 return "The list was never accurate. It is retained for medico-legal purposes only."; 120 case NULL: 121 return null; 122 default: 123 return "?"; 124 } 125 } 126 127 public String getDisplay() { 128 switch (this) { 129 case CURRENT: 130 return "Current"; 131 case RETIRED: 132 return "Retired"; 133 case ENTEREDINERROR: 134 return "Entered In Error"; 135 case NULL: 136 return null; 137 default: 138 return "?"; 139 } 140 } 141 } 142 143 public static class ListStatusEnumFactory implements EnumFactory<ListStatus> { 144 public ListStatus fromCode(String codeString) throws IllegalArgumentException { 145 if (codeString == null || "".equals(codeString)) 146 if (codeString == null || "".equals(codeString)) 147 return null; 148 if ("current".equals(codeString)) 149 return ListStatus.CURRENT; 150 if ("retired".equals(codeString)) 151 return ListStatus.RETIRED; 152 if ("entered-in-error".equals(codeString)) 153 return ListStatus.ENTEREDINERROR; 154 throw new IllegalArgumentException("Unknown ListStatus code '" + codeString + "'"); 155 } 156 157 public Enumeration<ListStatus> fromType(Base code) throws FHIRException { 158 if (code == null || code.isEmpty()) 159 return null; 160 String codeString = ((PrimitiveType) code).asStringValue(); 161 if (codeString == null || "".equals(codeString)) 162 return null; 163 if ("current".equals(codeString)) 164 return new Enumeration<ListStatus>(this, ListStatus.CURRENT); 165 if ("retired".equals(codeString)) 166 return new Enumeration<ListStatus>(this, ListStatus.RETIRED); 167 if ("entered-in-error".equals(codeString)) 168 return new Enumeration<ListStatus>(this, ListStatus.ENTEREDINERROR); 169 throw new FHIRException("Unknown ListStatus code '" + codeString + "'"); 170 } 171 172 public String toCode(ListStatus code) { 173 if (code == ListStatus.CURRENT) 174 return "current"; 175 if (code == ListStatus.RETIRED) 176 return "retired"; 177 if (code == ListStatus.ENTEREDINERROR) 178 return "entered-in-error"; 179 return "?"; 180 } 181 } 182 183 public enum ListMode { 184 /** 185 * This list is the master list, maintained in an ongoing fashion with regular 186 * updates as the real world list it is tracking changes 187 */ 188 WORKING, 189 /** 190 * This list was prepared as a snapshot. It should not be assumed to be current 191 */ 192 SNAPSHOT, 193 /** 194 * A list that indicates where changes have been made or recommended 195 */ 196 CHANGES, 197 /** 198 * added to help the parsers 199 */ 200 NULL; 201 202 public static ListMode fromCode(String codeString) throws FHIRException { 203 if (codeString == null || "".equals(codeString)) 204 return null; 205 if ("working".equals(codeString)) 206 return WORKING; 207 if ("snapshot".equals(codeString)) 208 return SNAPSHOT; 209 if ("changes".equals(codeString)) 210 return CHANGES; 211 throw new FHIRException("Unknown ListMode code '" + codeString + "'"); 212 } 213 214 public String toCode() { 215 switch (this) { 216 case WORKING: 217 return "working"; 218 case SNAPSHOT: 219 return "snapshot"; 220 case CHANGES: 221 return "changes"; 222 case NULL: 223 return null; 224 default: 225 return "?"; 226 } 227 } 228 229 public String getSystem() { 230 switch (this) { 231 case WORKING: 232 return "http://hl7.org/fhir/list-mode"; 233 case SNAPSHOT: 234 return "http://hl7.org/fhir/list-mode"; 235 case CHANGES: 236 return "http://hl7.org/fhir/list-mode"; 237 case NULL: 238 return null; 239 default: 240 return "?"; 241 } 242 } 243 244 public String getDefinition() { 245 switch (this) { 246 case WORKING: 247 return "This list is the master list, maintained in an ongoing fashion with regular updates as the real world list it is tracking changes"; 248 case SNAPSHOT: 249 return "This list was prepared as a snapshot. It should not be assumed to be current"; 250 case CHANGES: 251 return "A list that indicates where changes have been made or recommended"; 252 case NULL: 253 return null; 254 default: 255 return "?"; 256 } 257 } 258 259 public String getDisplay() { 260 switch (this) { 261 case WORKING: 262 return "Working List"; 263 case SNAPSHOT: 264 return "Snapshot List"; 265 case CHANGES: 266 return "Change List"; 267 case NULL: 268 return null; 269 default: 270 return "?"; 271 } 272 } 273 } 274 275 public static class ListModeEnumFactory implements EnumFactory<ListMode> { 276 public ListMode fromCode(String codeString) throws IllegalArgumentException { 277 if (codeString == null || "".equals(codeString)) 278 if (codeString == null || "".equals(codeString)) 279 return null; 280 if ("working".equals(codeString)) 281 return ListMode.WORKING; 282 if ("snapshot".equals(codeString)) 283 return ListMode.SNAPSHOT; 284 if ("changes".equals(codeString)) 285 return ListMode.CHANGES; 286 throw new IllegalArgumentException("Unknown ListMode code '" + codeString + "'"); 287 } 288 289 public Enumeration<ListMode> fromType(Base code) throws FHIRException { 290 if (code == null || code.isEmpty()) 291 return null; 292 String codeString = ((PrimitiveType) code).asStringValue(); 293 if (codeString == null || "".equals(codeString)) 294 return null; 295 if ("working".equals(codeString)) 296 return new Enumeration<ListMode>(this, ListMode.WORKING); 297 if ("snapshot".equals(codeString)) 298 return new Enumeration<ListMode>(this, ListMode.SNAPSHOT); 299 if ("changes".equals(codeString)) 300 return new Enumeration<ListMode>(this, ListMode.CHANGES); 301 throw new FHIRException("Unknown ListMode code '" + codeString + "'"); 302 } 303 304 public String toCode(ListMode code) { 305 if (code == ListMode.WORKING) 306 return "working"; 307 if (code == ListMode.SNAPSHOT) 308 return "snapshot"; 309 if (code == ListMode.CHANGES) 310 return "changes"; 311 return "?"; 312 } 313 } 314 315 @Block() 316 public static class ListEntryComponent extends BackboneElement implements IBaseBackboneElement { 317 /** 318 * The flag allows the system constructing the list to indicate the role and 319 * significance of the item in the list. 320 */ 321 @Child(name = "flag", type = { 322 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 323 @Description(shortDefinition = "Status/Workflow information about this item", formalDefinition = "The flag allows the system constructing the list to indicate the role and significance of the item in the list.") 324 protected CodeableConcept flag; 325 326 /** 327 * True if this item is marked as deleted in the list. 328 */ 329 @Child(name = "deleted", type = { 330 BooleanType.class }, order = 2, min = 0, max = 1, modifier = true, summary = false) 331 @Description(shortDefinition = "If this item is actually marked as deleted", formalDefinition = "True if this item is marked as deleted in the list.") 332 protected BooleanType deleted; 333 334 /** 335 * When this item was added to the list. 336 */ 337 @Child(name = "date", type = { DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 338 @Description(shortDefinition = "When item added to list", formalDefinition = "When this item was added to the list.") 339 protected DateTimeType date; 340 341 /** 342 * A reference to the actual resource from which data was derived. 343 */ 344 @Child(name = "item", type = {}, order = 4, min = 1, max = 1, modifier = false, summary = false) 345 @Description(shortDefinition = "Actual entry", formalDefinition = "A reference to the actual resource from which data was derived.") 346 protected Reference item; 347 348 /** 349 * The actual object that is the target of the reference (A reference to the 350 * actual resource from which data was derived.) 351 */ 352 protected Resource itemTarget; 353 354 private static final long serialVersionUID = -758164425L; 355 356 /* 357 * Constructor 358 */ 359 public ListEntryComponent() { 360 super(); 361 } 362 363 /* 364 * Constructor 365 */ 366 public ListEntryComponent(Reference item) { 367 super(); 368 this.item = item; 369 } 370 371 /** 372 * @return {@link #flag} (The flag allows the system constructing the list to 373 * indicate the role and significance of the item in the list.) 374 */ 375 public CodeableConcept getFlag() { 376 if (this.flag == null) 377 if (Configuration.errorOnAutoCreate()) 378 throw new Error("Attempt to auto-create ListEntryComponent.flag"); 379 else if (Configuration.doAutoCreate()) 380 this.flag = new CodeableConcept(); // cc 381 return this.flag; 382 } 383 384 public boolean hasFlag() { 385 return this.flag != null && !this.flag.isEmpty(); 386 } 387 388 /** 389 * @param value {@link #flag} (The flag allows the system constructing the list 390 * to indicate the role and significance of the item in the list.) 391 */ 392 public ListEntryComponent setFlag(CodeableConcept value) { 393 this.flag = value; 394 return this; 395 } 396 397 /** 398 * @return {@link #deleted} (True if this item is marked as deleted in the 399 * list.). This is the underlying object with id, value and extensions. 400 * The accessor "getDeleted" gives direct access to the value 401 */ 402 public BooleanType getDeletedElement() { 403 if (this.deleted == null) 404 if (Configuration.errorOnAutoCreate()) 405 throw new Error("Attempt to auto-create ListEntryComponent.deleted"); 406 else if (Configuration.doAutoCreate()) 407 this.deleted = new BooleanType(); // bb 408 return this.deleted; 409 } 410 411 public boolean hasDeletedElement() { 412 return this.deleted != null && !this.deleted.isEmpty(); 413 } 414 415 public boolean hasDeleted() { 416 return this.deleted != null && !this.deleted.isEmpty(); 417 } 418 419 /** 420 * @param value {@link #deleted} (True if this item is marked as deleted in the 421 * list.). This is the underlying object with id, value and 422 * extensions. The accessor "getDeleted" gives direct access to the 423 * value 424 */ 425 public ListEntryComponent setDeletedElement(BooleanType value) { 426 this.deleted = value; 427 return this; 428 } 429 430 /** 431 * @return True if this item is marked as deleted in the list. 432 */ 433 public boolean getDeleted() { 434 return this.deleted == null || this.deleted.isEmpty() ? false : this.deleted.getValue(); 435 } 436 437 /** 438 * @param value True if this item is marked as deleted in the list. 439 */ 440 public ListEntryComponent setDeleted(boolean value) { 441 if (this.deleted == null) 442 this.deleted = new BooleanType(); 443 this.deleted.setValue(value); 444 return this; 445 } 446 447 /** 448 * @return {@link #date} (When this item was added to the list.). This is the 449 * underlying object with id, value and extensions. The accessor 450 * "getDate" gives direct access to the value 451 */ 452 public DateTimeType getDateElement() { 453 if (this.date == null) 454 if (Configuration.errorOnAutoCreate()) 455 throw new Error("Attempt to auto-create ListEntryComponent.date"); 456 else if (Configuration.doAutoCreate()) 457 this.date = new DateTimeType(); // bb 458 return this.date; 459 } 460 461 public boolean hasDateElement() { 462 return this.date != null && !this.date.isEmpty(); 463 } 464 465 public boolean hasDate() { 466 return this.date != null && !this.date.isEmpty(); 467 } 468 469 /** 470 * @param value {@link #date} (When this item was added to the list.). This is 471 * the underlying object with id, value and extensions. The 472 * accessor "getDate" gives direct access to the value 473 */ 474 public ListEntryComponent setDateElement(DateTimeType value) { 475 this.date = value; 476 return this; 477 } 478 479 /** 480 * @return When this item was added to the list. 481 */ 482 public Date getDate() { 483 return this.date == null ? null : this.date.getValue(); 484 } 485 486 /** 487 * @param value When this item was added to the list. 488 */ 489 public ListEntryComponent setDate(Date value) { 490 if (value == null) 491 this.date = null; 492 else { 493 if (this.date == null) 494 this.date = new DateTimeType(); 495 this.date.setValue(value); 496 } 497 return this; 498 } 499 500 /** 501 * @return {@link #item} (A reference to the actual resource from which data was 502 * derived.) 503 */ 504 public Reference getItem() { 505 if (this.item == null) 506 if (Configuration.errorOnAutoCreate()) 507 throw new Error("Attempt to auto-create ListEntryComponent.item"); 508 else if (Configuration.doAutoCreate()) 509 this.item = new Reference(); // cc 510 return this.item; 511 } 512 513 public boolean hasItem() { 514 return this.item != null && !this.item.isEmpty(); 515 } 516 517 /** 518 * @param value {@link #item} (A reference to the actual resource from which 519 * data was derived.) 520 */ 521 public ListEntryComponent setItem(Reference value) { 522 this.item = value; 523 return this; 524 } 525 526 /** 527 * @return {@link #item} The actual object that is the target of the reference. 528 * The reference library doesn't populate this, but you can use it to 529 * hold the resource if you resolve it. (A reference to the actual 530 * resource from which data was derived.) 531 */ 532 public Resource getItemTarget() { 533 return this.itemTarget; 534 } 535 536 /** 537 * @param value {@link #item} The actual object that is the target of the 538 * reference. The reference library doesn't use these, but you can 539 * use it to hold the resource if you resolve it. (A reference to 540 * the actual resource from which data was derived.) 541 */ 542 public ListEntryComponent setItemTarget(Resource value) { 543 this.itemTarget = value; 544 return this; 545 } 546 547 protected void listChildren(List<Property> childrenList) { 548 super.listChildren(childrenList); 549 childrenList.add(new Property("flag", "CodeableConcept", 550 "The flag allows the system constructing the list to indicate the role and significance of the item in the list.", 551 0, java.lang.Integer.MAX_VALUE, flag)); 552 childrenList.add(new Property("deleted", "boolean", "True if this item is marked as deleted in the list.", 0, 553 java.lang.Integer.MAX_VALUE, deleted)); 554 childrenList.add(new Property("date", "dateTime", "When this item was added to the list.", 0, 555 java.lang.Integer.MAX_VALUE, date)); 556 childrenList.add(new Property("item", "Reference(Any)", 557 "A reference to the actual resource from which data was derived.", 0, java.lang.Integer.MAX_VALUE, item)); 558 } 559 560 @Override 561 public void setProperty(String name, Base value) throws FHIRException { 562 if (name.equals("flag")) 563 this.flag = castToCodeableConcept(value); // CodeableConcept 564 else if (name.equals("deleted")) 565 this.deleted = castToBoolean(value); // BooleanType 566 else if (name.equals("date")) 567 this.date = castToDateTime(value); // DateTimeType 568 else if (name.equals("item")) 569 this.item = castToReference(value); // Reference 570 else 571 super.setProperty(name, value); 572 } 573 574 @Override 575 public Base addChild(String name) throws FHIRException { 576 if (name.equals("flag")) { 577 this.flag = new CodeableConcept(); 578 return this.flag; 579 } else if (name.equals("deleted")) { 580 throw new FHIRException("Cannot call addChild on a singleton property List_.deleted"); 581 } else if (name.equals("date")) { 582 throw new FHIRException("Cannot call addChild on a singleton property List_.date"); 583 } else if (name.equals("item")) { 584 this.item = new Reference(); 585 return this.item; 586 } else 587 return super.addChild(name); 588 } 589 590 public ListEntryComponent copy() { 591 ListEntryComponent dst = new ListEntryComponent(); 592 copyValues(dst); 593 dst.flag = flag == null ? null : flag.copy(); 594 dst.deleted = deleted == null ? null : deleted.copy(); 595 dst.date = date == null ? null : date.copy(); 596 dst.item = item == null ? null : item.copy(); 597 return dst; 598 } 599 600 @Override 601 public boolean equalsDeep(Base other) { 602 if (!super.equalsDeep(other)) 603 return false; 604 if (!(other instanceof ListEntryComponent)) 605 return false; 606 ListEntryComponent o = (ListEntryComponent) other; 607 return compareDeep(flag, o.flag, true) && compareDeep(deleted, o.deleted, true) && compareDeep(date, o.date, true) 608 && compareDeep(item, o.item, true); 609 } 610 611 @Override 612 public boolean equalsShallow(Base other) { 613 if (!super.equalsShallow(other)) 614 return false; 615 if (!(other instanceof ListEntryComponent)) 616 return false; 617 ListEntryComponent o = (ListEntryComponent) other; 618 return compareValues(deleted, o.deleted, true) && compareValues(date, o.date, true); 619 } 620 621 public boolean isEmpty() { 622 return super.isEmpty() && (flag == null || flag.isEmpty()) && (deleted == null || deleted.isEmpty()) 623 && (date == null || date.isEmpty()) && (item == null || item.isEmpty()); 624 } 625 626 public String fhirType() { 627 return "List.entry"; 628 629 } 630 631 } 632 633 /** 634 * Identifier for the List assigned for business purposes outside the context of 635 * FHIR. 636 */ 637 @Child(name = "identifier", type = { 638 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 639 @Description(shortDefinition = "Business identifier", formalDefinition = "Identifier for the List assigned for business purposes outside the context of FHIR.") 640 protected List<Identifier> identifier; 641 642 /** 643 * A label for the list assigned by the author. 644 */ 645 @Child(name = "title", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 646 @Description(shortDefinition = "Descriptive name for the list", formalDefinition = "A label for the list assigned by the author.") 647 protected StringType title; 648 649 /** 650 * This code defines the purpose of the list - why it was created. 651 */ 652 @Child(name = "code", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 653 @Description(shortDefinition = "What the purpose of this list is", formalDefinition = "This code defines the purpose of the list - why it was created.") 654 protected CodeableConcept code; 655 656 /** 657 * The common subject (or patient) of the resources that are in the list, if 658 * there is one. 659 */ 660 @Child(name = "subject", type = { Patient.class, Group.class, Device.class, 661 Location.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 662 @Description(shortDefinition = "If all resources have the same subject", formalDefinition = "The common subject (or patient) of the resources that are in the list, if there is one.") 663 protected Reference subject; 664 665 /** 666 * The actual object that is the target of the reference (The common subject (or 667 * patient) of the resources that are in the list, if there is one.) 668 */ 669 protected Resource subjectTarget; 670 671 /** 672 * The entity responsible for deciding what the contents of the list were. Where 673 * the list was created by a human, this is the same as the author of the list. 674 */ 675 @Child(name = "source", type = { Practitioner.class, Patient.class, 676 Device.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 677 @Description(shortDefinition = "Who and/or what defined the list contents (aka Author)", formalDefinition = "The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.") 678 protected Reference source; 679 680 /** 681 * The actual object that is the target of the reference (The entity responsible 682 * for deciding what the contents of the list were. Where the list was created 683 * by a human, this is the same as the author of the list.) 684 */ 685 protected Resource sourceTarget; 686 687 /** 688 * The encounter that is the context in which this list was created. 689 */ 690 @Child(name = "encounter", type = { Encounter.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 691 @Description(shortDefinition = "Context in which list created", formalDefinition = "The encounter that is the context in which this list was created.") 692 protected Reference encounter; 693 694 /** 695 * The actual object that is the target of the reference (The encounter that is 696 * the context in which this list was created.) 697 */ 698 protected Encounter encounterTarget; 699 700 /** 701 * Indicates the current state of this list. 702 */ 703 @Child(name = "status", type = { CodeType.class }, order = 6, min = 1, max = 1, modifier = true, summary = true) 704 @Description(shortDefinition = "current | retired | entered-in-error", formalDefinition = "Indicates the current state of this list.") 705 protected Enumeration<ListStatus> status; 706 707 /** 708 * The date that the list was prepared. 709 */ 710 @Child(name = "date", type = { DateTimeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 711 @Description(shortDefinition = "When the list was prepared", formalDefinition = "The date that the list was prepared.") 712 protected DateTimeType date; 713 714 /** 715 * What order applies to the items in the list. 716 */ 717 @Child(name = "orderedBy", type = { 718 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 719 @Description(shortDefinition = "What order the list has", formalDefinition = "What order applies to the items in the list.") 720 protected CodeableConcept orderedBy; 721 722 /** 723 * How this list was prepared - whether it is a working list that is suitable 724 * for being maintained on an ongoing basis, or if it represents a snapshot of a 725 * list of items from another source, or whether it is a prepared list where 726 * items may be marked as added, modified or deleted. 727 */ 728 @Child(name = "mode", type = { CodeType.class }, order = 9, min = 1, max = 1, modifier = true, summary = true) 729 @Description(shortDefinition = "working | snapshot | changes", formalDefinition = "How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.") 730 protected Enumeration<ListMode> mode; 731 732 /** 733 * Comments that apply to the overall list. 734 */ 735 @Child(name = "note", type = { StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 736 @Description(shortDefinition = "Comments about the list", formalDefinition = "Comments that apply to the overall list.") 737 protected StringType note; 738 739 /** 740 * Entries in this list. 741 */ 742 @Child(name = "entry", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 743 @Description(shortDefinition = "Entries in the list", formalDefinition = "Entries in this list.") 744 protected List<ListEntryComponent> entry; 745 746 /** 747 * If the list is empty, why the list is empty. 748 */ 749 @Child(name = "emptyReason", type = { 750 CodeableConcept.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 751 @Description(shortDefinition = "Why list is empty", formalDefinition = "If the list is empty, why the list is empty.") 752 protected CodeableConcept emptyReason; 753 754 private static final long serialVersionUID = 1819128642L; 755 756 /* 757 * Constructor 758 */ 759 public List_() { 760 super(); 761 } 762 763 /* 764 * Constructor 765 */ 766 public List_(Enumeration<ListStatus> status, Enumeration<ListMode> mode) { 767 super(); 768 this.status = status; 769 this.mode = mode; 770 } 771 772 /** 773 * @return {@link #identifier} (Identifier for the List assigned for business 774 * purposes outside the context of FHIR.) 775 */ 776 public List<Identifier> getIdentifier() { 777 if (this.identifier == null) 778 this.identifier = new ArrayList<Identifier>(); 779 return this.identifier; 780 } 781 782 public boolean hasIdentifier() { 783 if (this.identifier == null) 784 return false; 785 for (Identifier item : this.identifier) 786 if (!item.isEmpty()) 787 return true; 788 return false; 789 } 790 791 /** 792 * @return {@link #identifier} (Identifier for the List assigned for business 793 * purposes outside the context of FHIR.) 794 */ 795 // syntactic sugar 796 public Identifier addIdentifier() { // 3 797 Identifier t = new Identifier(); 798 if (this.identifier == null) 799 this.identifier = new ArrayList<Identifier>(); 800 this.identifier.add(t); 801 return t; 802 } 803 804 // syntactic sugar 805 public List_ addIdentifier(Identifier t) { // 3 806 if (t == null) 807 return this; 808 if (this.identifier == null) 809 this.identifier = new ArrayList<Identifier>(); 810 this.identifier.add(t); 811 return this; 812 } 813 814 /** 815 * @return {@link #title} (A label for the list assigned by the author.). This 816 * is the underlying object with id, value and extensions. The accessor 817 * "getTitle" gives direct access to the value 818 */ 819 public StringType getTitleElement() { 820 if (this.title == null) 821 if (Configuration.errorOnAutoCreate()) 822 throw new Error("Attempt to auto-create List_.title"); 823 else if (Configuration.doAutoCreate()) 824 this.title = new StringType(); // bb 825 return this.title; 826 } 827 828 public boolean hasTitleElement() { 829 return this.title != null && !this.title.isEmpty(); 830 } 831 832 public boolean hasTitle() { 833 return this.title != null && !this.title.isEmpty(); 834 } 835 836 /** 837 * @param value {@link #title} (A label for the list assigned by the author.). 838 * This is the underlying object with id, value and extensions. The 839 * accessor "getTitle" gives direct access to the value 840 */ 841 public List_ setTitleElement(StringType value) { 842 this.title = value; 843 return this; 844 } 845 846 /** 847 * @return A label for the list assigned by the author. 848 */ 849 public String getTitle() { 850 return this.title == null ? null : this.title.getValue(); 851 } 852 853 /** 854 * @param value A label for the list assigned by the author. 855 */ 856 public List_ setTitle(String value) { 857 if (Utilities.noString(value)) 858 this.title = null; 859 else { 860 if (this.title == null) 861 this.title = new StringType(); 862 this.title.setValue(value); 863 } 864 return this; 865 } 866 867 /** 868 * @return {@link #code} (This code defines the purpose of the list - why it was 869 * created.) 870 */ 871 public CodeableConcept getCode() { 872 if (this.code == null) 873 if (Configuration.errorOnAutoCreate()) 874 throw new Error("Attempt to auto-create List_.code"); 875 else if (Configuration.doAutoCreate()) 876 this.code = new CodeableConcept(); // cc 877 return this.code; 878 } 879 880 public boolean hasCode() { 881 return this.code != null && !this.code.isEmpty(); 882 } 883 884 /** 885 * @param value {@link #code} (This code defines the purpose of the list - why 886 * it was created.) 887 */ 888 public List_ setCode(CodeableConcept value) { 889 this.code = value; 890 return this; 891 } 892 893 /** 894 * @return {@link #subject} (The common subject (or patient) of the resources 895 * that are in the list, if there is one.) 896 */ 897 public Reference getSubject() { 898 if (this.subject == null) 899 if (Configuration.errorOnAutoCreate()) 900 throw new Error("Attempt to auto-create List_.subject"); 901 else if (Configuration.doAutoCreate()) 902 this.subject = new Reference(); // cc 903 return this.subject; 904 } 905 906 public boolean hasSubject() { 907 return this.subject != null && !this.subject.isEmpty(); 908 } 909 910 /** 911 * @param value {@link #subject} (The common subject (or patient) of the 912 * resources that are in the list, if there is one.) 913 */ 914 public List_ setSubject(Reference value) { 915 this.subject = value; 916 return this; 917 } 918 919 /** 920 * @return {@link #subject} The actual object that is the target of the 921 * reference. The reference library doesn't populate this, but you can 922 * use it to hold the resource if you resolve it. (The common subject 923 * (or patient) of the resources that are in the list, if there is one.) 924 */ 925 public Resource getSubjectTarget() { 926 return this.subjectTarget; 927 } 928 929 /** 930 * @param value {@link #subject} The actual object that is the target of the 931 * reference. The reference library doesn't use these, but you can 932 * use it to hold the resource if you resolve it. (The common 933 * subject (or patient) of the resources that are in the list, if 934 * there is one.) 935 */ 936 public List_ setSubjectTarget(Resource value) { 937 this.subjectTarget = value; 938 return this; 939 } 940 941 /** 942 * @return {@link #source} (The entity responsible for deciding what the 943 * contents of the list were. Where the list was created by a human, 944 * this is the same as the author of the list.) 945 */ 946 public Reference getSource() { 947 if (this.source == null) 948 if (Configuration.errorOnAutoCreate()) 949 throw new Error("Attempt to auto-create List_.source"); 950 else if (Configuration.doAutoCreate()) 951 this.source = new Reference(); // cc 952 return this.source; 953 } 954 955 public boolean hasSource() { 956 return this.source != null && !this.source.isEmpty(); 957 } 958 959 /** 960 * @param value {@link #source} (The entity responsible for deciding what the 961 * contents of the list were. Where the list was created by a 962 * human, this is the same as the author of the list.) 963 */ 964 public List_ setSource(Reference value) { 965 this.source = value; 966 return this; 967 } 968 969 /** 970 * @return {@link #source} The actual object that is the target of the 971 * reference. The reference library doesn't populate this, but you can 972 * use it to hold the resource if you resolve it. (The entity 973 * responsible for deciding what the contents of the list were. Where 974 * the list was created by a human, this is the same as the author of 975 * the list.) 976 */ 977 public Resource getSourceTarget() { 978 return this.sourceTarget; 979 } 980 981 /** 982 * @param value {@link #source} The actual object that is the target of the 983 * reference. The reference library doesn't use these, but you can 984 * use it to hold the resource if you resolve it. (The entity 985 * responsible for deciding what the contents of the list were. 986 * Where the list was created by a human, this is the same as the 987 * author of the list.) 988 */ 989 public List_ setSourceTarget(Resource value) { 990 this.sourceTarget = value; 991 return this; 992 } 993 994 /** 995 * @return {@link #encounter} (The encounter that is the context in which this 996 * list was created.) 997 */ 998 public Reference getEncounter() { 999 if (this.encounter == null) 1000 if (Configuration.errorOnAutoCreate()) 1001 throw new Error("Attempt to auto-create List_.encounter"); 1002 else if (Configuration.doAutoCreate()) 1003 this.encounter = new Reference(); // cc 1004 return this.encounter; 1005 } 1006 1007 public boolean hasEncounter() { 1008 return this.encounter != null && !this.encounter.isEmpty(); 1009 } 1010 1011 /** 1012 * @param value {@link #encounter} (The encounter that is the context in which 1013 * this list was created.) 1014 */ 1015 public List_ setEncounter(Reference value) { 1016 this.encounter = value; 1017 return this; 1018 } 1019 1020 /** 1021 * @return {@link #encounter} The actual object that is the target of the 1022 * reference. The reference library doesn't populate this, but you can 1023 * use it to hold the resource if you resolve it. (The encounter that is 1024 * the context in which this list was created.) 1025 */ 1026 public Encounter getEncounterTarget() { 1027 if (this.encounterTarget == null) 1028 if (Configuration.errorOnAutoCreate()) 1029 throw new Error("Attempt to auto-create List_.encounter"); 1030 else if (Configuration.doAutoCreate()) 1031 this.encounterTarget = new Encounter(); // aa 1032 return this.encounterTarget; 1033 } 1034 1035 /** 1036 * @param value {@link #encounter} The actual object that is the target of the 1037 * reference. The reference library doesn't use these, but you can 1038 * use it to hold the resource if you resolve it. (The encounter 1039 * that is the context in which this list was created.) 1040 */ 1041 public List_ setEncounterTarget(Encounter value) { 1042 this.encounterTarget = value; 1043 return this; 1044 } 1045 1046 /** 1047 * @return {@link #status} (Indicates the current state of this list.). This is 1048 * the underlying object with id, value and extensions. The accessor 1049 * "getStatus" gives direct access to the value 1050 */ 1051 public Enumeration<ListStatus> getStatusElement() { 1052 if (this.status == null) 1053 if (Configuration.errorOnAutoCreate()) 1054 throw new Error("Attempt to auto-create List_.status"); 1055 else if (Configuration.doAutoCreate()) 1056 this.status = new Enumeration<ListStatus>(new ListStatusEnumFactory()); // bb 1057 return this.status; 1058 } 1059 1060 public boolean hasStatusElement() { 1061 return this.status != null && !this.status.isEmpty(); 1062 } 1063 1064 public boolean hasStatus() { 1065 return this.status != null && !this.status.isEmpty(); 1066 } 1067 1068 /** 1069 * @param value {@link #status} (Indicates the current state of this list.). 1070 * This is the underlying object with id, value and extensions. The 1071 * accessor "getStatus" gives direct access to the value 1072 */ 1073 public List_ setStatusElement(Enumeration<ListStatus> value) { 1074 this.status = value; 1075 return this; 1076 } 1077 1078 /** 1079 * @return Indicates the current state of this list. 1080 */ 1081 public ListStatus getStatus() { 1082 return this.status == null ? null : this.status.getValue(); 1083 } 1084 1085 /** 1086 * @param value Indicates the current state of this list. 1087 */ 1088 public List_ setStatus(ListStatus value) { 1089 if (this.status == null) 1090 this.status = new Enumeration<ListStatus>(new ListStatusEnumFactory()); 1091 this.status.setValue(value); 1092 return this; 1093 } 1094 1095 /** 1096 * @return {@link #date} (The date that the list was prepared.). This is the 1097 * underlying object with id, value and extensions. The accessor 1098 * "getDate" gives direct access to the value 1099 */ 1100 public DateTimeType getDateElement() { 1101 if (this.date == null) 1102 if (Configuration.errorOnAutoCreate()) 1103 throw new Error("Attempt to auto-create List_.date"); 1104 else if (Configuration.doAutoCreate()) 1105 this.date = new DateTimeType(); // bb 1106 return this.date; 1107 } 1108 1109 public boolean hasDateElement() { 1110 return this.date != null && !this.date.isEmpty(); 1111 } 1112 1113 public boolean hasDate() { 1114 return this.date != null && !this.date.isEmpty(); 1115 } 1116 1117 /** 1118 * @param value {@link #date} (The date that the list was prepared.). This is 1119 * the underlying object with id, value and extensions. The 1120 * accessor "getDate" gives direct access to the value 1121 */ 1122 public List_ setDateElement(DateTimeType value) { 1123 this.date = value; 1124 return this; 1125 } 1126 1127 /** 1128 * @return The date that the list was prepared. 1129 */ 1130 public Date getDate() { 1131 return this.date == null ? null : this.date.getValue(); 1132 } 1133 1134 /** 1135 * @param value The date that the list was prepared. 1136 */ 1137 public List_ setDate(Date value) { 1138 if (value == null) 1139 this.date = null; 1140 else { 1141 if (this.date == null) 1142 this.date = new DateTimeType(); 1143 this.date.setValue(value); 1144 } 1145 return this; 1146 } 1147 1148 /** 1149 * @return {@link #orderedBy} (What order applies to the items in the list.) 1150 */ 1151 public CodeableConcept getOrderedBy() { 1152 if (this.orderedBy == null) 1153 if (Configuration.errorOnAutoCreate()) 1154 throw new Error("Attempt to auto-create List_.orderedBy"); 1155 else if (Configuration.doAutoCreate()) 1156 this.orderedBy = new CodeableConcept(); // cc 1157 return this.orderedBy; 1158 } 1159 1160 public boolean hasOrderedBy() { 1161 return this.orderedBy != null && !this.orderedBy.isEmpty(); 1162 } 1163 1164 /** 1165 * @param value {@link #orderedBy} (What order applies to the items in the 1166 * list.) 1167 */ 1168 public List_ setOrderedBy(CodeableConcept value) { 1169 this.orderedBy = value; 1170 return this; 1171 } 1172 1173 /** 1174 * @return {@link #mode} (How this list was prepared - whether it is a working 1175 * list that is suitable for being maintained on an ongoing basis, or if 1176 * it represents a snapshot of a list of items from another source, or 1177 * whether it is a prepared list where items may be marked as added, 1178 * modified or deleted.). This is the underlying object with id, value 1179 * and extensions. The accessor "getMode" gives direct access to the 1180 * value 1181 */ 1182 public Enumeration<ListMode> getModeElement() { 1183 if (this.mode == null) 1184 if (Configuration.errorOnAutoCreate()) 1185 throw new Error("Attempt to auto-create List_.mode"); 1186 else if (Configuration.doAutoCreate()) 1187 this.mode = new Enumeration<ListMode>(new ListModeEnumFactory()); // bb 1188 return this.mode; 1189 } 1190 1191 public boolean hasModeElement() { 1192 return this.mode != null && !this.mode.isEmpty(); 1193 } 1194 1195 public boolean hasMode() { 1196 return this.mode != null && !this.mode.isEmpty(); 1197 } 1198 1199 /** 1200 * @param value {@link #mode} (How this list was prepared - whether it is a 1201 * working list that is suitable for being maintained on an ongoing 1202 * basis, or if it represents a snapshot of a list of items from 1203 * another source, or whether it is a prepared list where items may 1204 * be marked as added, modified or deleted.). This is the 1205 * underlying object with id, value and extensions. The accessor 1206 * "getMode" gives direct access to the value 1207 */ 1208 public List_ setModeElement(Enumeration<ListMode> value) { 1209 this.mode = value; 1210 return this; 1211 } 1212 1213 /** 1214 * @return How this list was prepared - whether it is a working list that is 1215 * suitable for being maintained on an ongoing basis, or if it 1216 * represents a snapshot of a list of items from another source, or 1217 * whether it is a prepared list where items may be marked as added, 1218 * modified or deleted. 1219 */ 1220 public ListMode getMode() { 1221 return this.mode == null ? null : this.mode.getValue(); 1222 } 1223 1224 /** 1225 * @param value How this list was prepared - whether it is a working list that 1226 * is suitable for being maintained on an ongoing basis, or if it 1227 * represents a snapshot of a list of items from another source, or 1228 * whether it is a prepared list where items may be marked as 1229 * added, modified or deleted. 1230 */ 1231 public List_ setMode(ListMode value) { 1232 if (this.mode == null) 1233 this.mode = new Enumeration<ListMode>(new ListModeEnumFactory()); 1234 this.mode.setValue(value); 1235 return this; 1236 } 1237 1238 /** 1239 * @return {@link #note} (Comments that apply to the overall list.). This is the 1240 * underlying object with id, value and extensions. The accessor 1241 * "getNote" gives direct access to the value 1242 */ 1243 public StringType getNoteElement() { 1244 if (this.note == null) 1245 if (Configuration.errorOnAutoCreate()) 1246 throw new Error("Attempt to auto-create List_.note"); 1247 else if (Configuration.doAutoCreate()) 1248 this.note = new StringType(); // bb 1249 return this.note; 1250 } 1251 1252 public boolean hasNoteElement() { 1253 return this.note != null && !this.note.isEmpty(); 1254 } 1255 1256 public boolean hasNote() { 1257 return this.note != null && !this.note.isEmpty(); 1258 } 1259 1260 /** 1261 * @param value {@link #note} (Comments that apply to the overall list.). This 1262 * is the underlying object with id, value and extensions. The 1263 * accessor "getNote" gives direct access to the value 1264 */ 1265 public List_ setNoteElement(StringType value) { 1266 this.note = value; 1267 return this; 1268 } 1269 1270 /** 1271 * @return Comments that apply to the overall list. 1272 */ 1273 public String getNote() { 1274 return this.note == null ? null : this.note.getValue(); 1275 } 1276 1277 /** 1278 * @param value Comments that apply to the overall list. 1279 */ 1280 public List_ setNote(String value) { 1281 if (Utilities.noString(value)) 1282 this.note = null; 1283 else { 1284 if (this.note == null) 1285 this.note = new StringType(); 1286 this.note.setValue(value); 1287 } 1288 return this; 1289 } 1290 1291 /** 1292 * @return {@link #entry} (Entries in this list.) 1293 */ 1294 public List<ListEntryComponent> getEntry() { 1295 if (this.entry == null) 1296 this.entry = new ArrayList<ListEntryComponent>(); 1297 return this.entry; 1298 } 1299 1300 public boolean hasEntry() { 1301 if (this.entry == null) 1302 return false; 1303 for (ListEntryComponent item : this.entry) 1304 if (!item.isEmpty()) 1305 return true; 1306 return false; 1307 } 1308 1309 /** 1310 * @return {@link #entry} (Entries in this list.) 1311 */ 1312 // syntactic sugar 1313 public ListEntryComponent addEntry() { // 3 1314 ListEntryComponent t = new ListEntryComponent(); 1315 if (this.entry == null) 1316 this.entry = new ArrayList<ListEntryComponent>(); 1317 this.entry.add(t); 1318 return t; 1319 } 1320 1321 // syntactic sugar 1322 public List_ addEntry(ListEntryComponent t) { // 3 1323 if (t == null) 1324 return this; 1325 if (this.entry == null) 1326 this.entry = new ArrayList<ListEntryComponent>(); 1327 this.entry.add(t); 1328 return this; 1329 } 1330 1331 /** 1332 * @return {@link #emptyReason} (If the list is empty, why the list is empty.) 1333 */ 1334 public CodeableConcept getEmptyReason() { 1335 if (this.emptyReason == null) 1336 if (Configuration.errorOnAutoCreate()) 1337 throw new Error("Attempt to auto-create List_.emptyReason"); 1338 else if (Configuration.doAutoCreate()) 1339 this.emptyReason = new CodeableConcept(); // cc 1340 return this.emptyReason; 1341 } 1342 1343 public boolean hasEmptyReason() { 1344 return this.emptyReason != null && !this.emptyReason.isEmpty(); 1345 } 1346 1347 /** 1348 * @param value {@link #emptyReason} (If the list is empty, why the list is 1349 * empty.) 1350 */ 1351 public List_ setEmptyReason(CodeableConcept value) { 1352 this.emptyReason = value; 1353 return this; 1354 } 1355 1356 protected void listChildren(List<Property> childrenList) { 1357 super.listChildren(childrenList); 1358 childrenList.add(new Property("identifier", "Identifier", 1359 "Identifier for the List assigned for business purposes outside the context of FHIR.", 0, 1360 java.lang.Integer.MAX_VALUE, identifier)); 1361 childrenList.add(new Property("title", "string", "A label for the list assigned by the author.", 0, 1362 java.lang.Integer.MAX_VALUE, title)); 1363 childrenList.add(new Property("code", "CodeableConcept", 1364 "This code defines the purpose of the list - why it was created.", 0, java.lang.Integer.MAX_VALUE, code)); 1365 childrenList.add(new Property("subject", "Reference(Patient|Group|Device|Location)", 1366 "The common subject (or patient) of the resources that are in the list, if there is one.", 0, 1367 java.lang.Integer.MAX_VALUE, subject)); 1368 childrenList.add(new Property("source", "Reference(Practitioner|Patient|Device)", 1369 "The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.", 1370 0, java.lang.Integer.MAX_VALUE, source)); 1371 childrenList.add(new Property("encounter", "Reference(Encounter)", 1372 "The encounter that is the context in which this list was created.", 0, java.lang.Integer.MAX_VALUE, 1373 encounter)); 1374 childrenList.add(new Property("status", "code", "Indicates the current state of this list.", 0, 1375 java.lang.Integer.MAX_VALUE, status)); 1376 childrenList.add( 1377 new Property("date", "dateTime", "The date that the list was prepared.", 0, java.lang.Integer.MAX_VALUE, date)); 1378 childrenList.add(new Property("orderedBy", "CodeableConcept", "What order applies to the items in the list.", 0, 1379 java.lang.Integer.MAX_VALUE, orderedBy)); 1380 childrenList.add(new Property("mode", "code", 1381 "How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 1382 0, java.lang.Integer.MAX_VALUE, mode)); 1383 childrenList.add(new Property("note", "string", "Comments that apply to the overall list.", 0, 1384 java.lang.Integer.MAX_VALUE, note)); 1385 childrenList.add(new Property("entry", "", "Entries in this list.", 0, java.lang.Integer.MAX_VALUE, entry)); 1386 childrenList.add(new Property("emptyReason", "CodeableConcept", "If the list is empty, why the list is empty.", 0, 1387 java.lang.Integer.MAX_VALUE, emptyReason)); 1388 } 1389 1390 @Override 1391 public void setProperty(String name, Base value) throws FHIRException { 1392 if (name.equals("identifier")) 1393 this.getIdentifier().add(castToIdentifier(value)); 1394 else if (name.equals("title")) 1395 this.title = castToString(value); // StringType 1396 else if (name.equals("code")) 1397 this.code = castToCodeableConcept(value); // CodeableConcept 1398 else if (name.equals("subject")) 1399 this.subject = castToReference(value); // Reference 1400 else if (name.equals("source")) 1401 this.source = castToReference(value); // Reference 1402 else if (name.equals("encounter")) 1403 this.encounter = castToReference(value); // Reference 1404 else if (name.equals("status")) 1405 this.status = new ListStatusEnumFactory().fromType(value); // Enumeration<ListStatus> 1406 else if (name.equals("date")) 1407 this.date = castToDateTime(value); // DateTimeType 1408 else if (name.equals("orderedBy")) 1409 this.orderedBy = castToCodeableConcept(value); // CodeableConcept 1410 else if (name.equals("mode")) 1411 this.mode = new ListModeEnumFactory().fromType(value); // Enumeration<ListMode> 1412 else if (name.equals("note")) 1413 this.note = castToString(value); // StringType 1414 else if (name.equals("entry")) 1415 this.getEntry().add((ListEntryComponent) value); 1416 else if (name.equals("emptyReason")) 1417 this.emptyReason = castToCodeableConcept(value); // CodeableConcept 1418 else 1419 super.setProperty(name, value); 1420 } 1421 1422 @Override 1423 public Base addChild(String name) throws FHIRException { 1424 if (name.equals("identifier")) { 1425 return addIdentifier(); 1426 } else if (name.equals("title")) { 1427 throw new FHIRException("Cannot call addChild on a singleton property List_.title"); 1428 } else if (name.equals("code")) { 1429 this.code = new CodeableConcept(); 1430 return this.code; 1431 } else if (name.equals("subject")) { 1432 this.subject = new Reference(); 1433 return this.subject; 1434 } else if (name.equals("source")) { 1435 this.source = new Reference(); 1436 return this.source; 1437 } else if (name.equals("encounter")) { 1438 this.encounter = new Reference(); 1439 return this.encounter; 1440 } else if (name.equals("status")) { 1441 throw new FHIRException("Cannot call addChild on a singleton property List_.status"); 1442 } else if (name.equals("date")) { 1443 throw new FHIRException("Cannot call addChild on a singleton property List_.date"); 1444 } else if (name.equals("orderedBy")) { 1445 this.orderedBy = new CodeableConcept(); 1446 return this.orderedBy; 1447 } else if (name.equals("mode")) { 1448 throw new FHIRException("Cannot call addChild on a singleton property List_.mode"); 1449 } else if (name.equals("note")) { 1450 throw new FHIRException("Cannot call addChild on a singleton property List_.note"); 1451 } else if (name.equals("entry")) { 1452 return addEntry(); 1453 } else if (name.equals("emptyReason")) { 1454 this.emptyReason = new CodeableConcept(); 1455 return this.emptyReason; 1456 } else 1457 return super.addChild(name); 1458 } 1459 1460 public String fhirType() { 1461 return "List"; 1462 1463 } 1464 1465 public List_ copy() { 1466 List_ dst = new List_(); 1467 copyValues(dst); 1468 if (identifier != null) { 1469 dst.identifier = new ArrayList<Identifier>(); 1470 for (Identifier i : identifier) 1471 dst.identifier.add(i.copy()); 1472 } 1473 ; 1474 dst.title = title == null ? null : title.copy(); 1475 dst.code = code == null ? null : code.copy(); 1476 dst.subject = subject == null ? null : subject.copy(); 1477 dst.source = source == null ? null : source.copy(); 1478 dst.encounter = encounter == null ? null : encounter.copy(); 1479 dst.status = status == null ? null : status.copy(); 1480 dst.date = date == null ? null : date.copy(); 1481 dst.orderedBy = orderedBy == null ? null : orderedBy.copy(); 1482 dst.mode = mode == null ? null : mode.copy(); 1483 dst.note = note == null ? null : note.copy(); 1484 if (entry != null) { 1485 dst.entry = new ArrayList<ListEntryComponent>(); 1486 for (ListEntryComponent i : entry) 1487 dst.entry.add(i.copy()); 1488 } 1489 ; 1490 dst.emptyReason = emptyReason == null ? null : emptyReason.copy(); 1491 return dst; 1492 } 1493 1494 protected List_ typedCopy() { 1495 return copy(); 1496 } 1497 1498 @Override 1499 public boolean equalsDeep(Base other) { 1500 if (!super.equalsDeep(other)) 1501 return false; 1502 if (!(other instanceof List_)) 1503 return false; 1504 List_ o = (List_) other; 1505 return compareDeep(identifier, o.identifier, true) && compareDeep(title, o.title, true) 1506 && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 1507 && compareDeep(source, o.source, true) && compareDeep(encounter, o.encounter, true) 1508 && compareDeep(status, o.status, true) && compareDeep(date, o.date, true) 1509 && compareDeep(orderedBy, o.orderedBy, true) && compareDeep(mode, o.mode, true) 1510 && compareDeep(note, o.note, true) && compareDeep(entry, o.entry, true) 1511 && compareDeep(emptyReason, o.emptyReason, true); 1512 } 1513 1514 @Override 1515 public boolean equalsShallow(Base other) { 1516 if (!super.equalsShallow(other)) 1517 return false; 1518 if (!(other instanceof List_)) 1519 return false; 1520 List_ o = (List_) other; 1521 return compareValues(title, o.title, true) && compareValues(status, o.status, true) 1522 && compareValues(date, o.date, true) && compareValues(mode, o.mode, true) && compareValues(note, o.note, true); 1523 } 1524 1525 public boolean isEmpty() { 1526 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (title == null || title.isEmpty()) 1527 && (code == null || code.isEmpty()) && (subject == null || subject.isEmpty()) 1528 && (source == null || source.isEmpty()) && (encounter == null || encounter.isEmpty()) 1529 && (status == null || status.isEmpty()) && (date == null || date.isEmpty()) 1530 && (orderedBy == null || orderedBy.isEmpty()) && (mode == null || mode.isEmpty()) 1531 && (note == null || note.isEmpty()) && (entry == null || entry.isEmpty()) 1532 && (emptyReason == null || emptyReason.isEmpty()); 1533 } 1534 1535 @Override 1536 public ResourceType getResourceType() { 1537 return ResourceType.List; 1538 } 1539 1540 @SearchParamDefinition(name = "date", path = "List.date", description = "When the list was prepared", type = "date") 1541 public static final String SP_DATE = "date"; 1542 @SearchParamDefinition(name = "item", path = "List.entry.item", description = "Actual entry", type = "reference") 1543 public static final String SP_ITEM = "item"; 1544 @SearchParamDefinition(name = "empty-reason", path = "List.emptyReason", description = "Why list is empty", type = "token") 1545 public static final String SP_EMPTYREASON = "empty-reason"; 1546 @SearchParamDefinition(name = "code", path = "List.code", description = "What the purpose of this list is", type = "token") 1547 public static final String SP_CODE = "code"; 1548 @SearchParamDefinition(name = "notes", path = "List.note", description = "Comments about the list", type = "string") 1549 public static final String SP_NOTES = "notes"; 1550 @SearchParamDefinition(name = "subject", path = "List.subject", description = "If all resources have the same subject", type = "reference") 1551 public static final String SP_SUBJECT = "subject"; 1552 @SearchParamDefinition(name = "patient", path = "List.subject", description = "If all resources have the same subject", type = "reference") 1553 public static final String SP_PATIENT = "patient"; 1554 @SearchParamDefinition(name = "source", path = "List.source", description = "Who and/or what defined the list contents (aka Author)", type = "reference") 1555 public static final String SP_SOURCE = "source"; 1556 @SearchParamDefinition(name = "encounter", path = "List.encounter", description = "Context in which list created", type = "reference") 1557 public static final String SP_ENCOUNTER = "encounter"; 1558 @SearchParamDefinition(name = "title", path = "List.title", description = "Descriptive name for the list", type = "string") 1559 public static final String SP_TITLE = "title"; 1560 @SearchParamDefinition(name = "status", path = "List.status", description = "current | retired | entered-in-error", type = "token") 1561 public static final String SP_STATUS = "status"; 1562 1563}