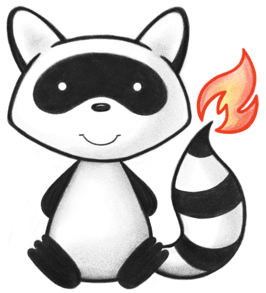
001package org.hl7.fhir.dstu2.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 035import java.util.ArrayList; 036import java.util.List; 037 038import ca.uhn.fhir.model.api.annotation.Block; 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.Description; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import org.hl7.fhir.exceptions.FHIRException; 045import org.hl7.fhir.utilities.Utilities; 046 047/** 048 * Details and position information for a physical place where services are 049 * provided and resources and participants may be stored, found, contained or 050 * accommodated. 051 */ 052@ResourceDef(name = "Location", profile = "http://hl7.org/fhir/Profile/Location") 053public class Location extends DomainResource { 054 055 public enum LocationStatus { 056 /** 057 * The location is operational. 058 */ 059 ACTIVE, 060 /** 061 * The location is temporarily closed. 062 */ 063 SUSPENDED, 064 /** 065 * The location is no longer used. 066 */ 067 INACTIVE, 068 /** 069 * added to help the parsers 070 */ 071 NULL; 072 073 public static LocationStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("suspended".equals(codeString)) 079 return SUSPENDED; 080 if ("inactive".equals(codeString)) 081 return INACTIVE; 082 throw new FHIRException("Unknown LocationStatus code '" + codeString + "'"); 083 } 084 085 public String toCode() { 086 switch (this) { 087 case ACTIVE: 088 return "active"; 089 case SUSPENDED: 090 return "suspended"; 091 case INACTIVE: 092 return "inactive"; 093 case NULL: 094 return null; 095 default: 096 return "?"; 097 } 098 } 099 100 public String getSystem() { 101 switch (this) { 102 case ACTIVE: 103 return "http://hl7.org/fhir/location-status"; 104 case SUSPENDED: 105 return "http://hl7.org/fhir/location-status"; 106 case INACTIVE: 107 return "http://hl7.org/fhir/location-status"; 108 case NULL: 109 return null; 110 default: 111 return "?"; 112 } 113 } 114 115 public String getDefinition() { 116 switch (this) { 117 case ACTIVE: 118 return "The location is operational."; 119 case SUSPENDED: 120 return "The location is temporarily closed."; 121 case INACTIVE: 122 return "The location is no longer used."; 123 case NULL: 124 return null; 125 default: 126 return "?"; 127 } 128 } 129 130 public String getDisplay() { 131 switch (this) { 132 case ACTIVE: 133 return "Active"; 134 case SUSPENDED: 135 return "Suspended"; 136 case INACTIVE: 137 return "Inactive"; 138 case NULL: 139 return null; 140 default: 141 return "?"; 142 } 143 } 144 } 145 146 public static class LocationStatusEnumFactory implements EnumFactory<LocationStatus> { 147 public LocationStatus fromCode(String codeString) throws IllegalArgumentException { 148 if (codeString == null || "".equals(codeString)) 149 if (codeString == null || "".equals(codeString)) 150 return null; 151 if ("active".equals(codeString)) 152 return LocationStatus.ACTIVE; 153 if ("suspended".equals(codeString)) 154 return LocationStatus.SUSPENDED; 155 if ("inactive".equals(codeString)) 156 return LocationStatus.INACTIVE; 157 throw new IllegalArgumentException("Unknown LocationStatus code '" + codeString + "'"); 158 } 159 160 public Enumeration<LocationStatus> fromType(Base code) throws FHIRException { 161 if (code == null || code.isEmpty()) 162 return null; 163 String codeString = ((PrimitiveType) code).asStringValue(); 164 if (codeString == null || "".equals(codeString)) 165 return null; 166 if ("active".equals(codeString)) 167 return new Enumeration<LocationStatus>(this, LocationStatus.ACTIVE); 168 if ("suspended".equals(codeString)) 169 return new Enumeration<LocationStatus>(this, LocationStatus.SUSPENDED); 170 if ("inactive".equals(codeString)) 171 return new Enumeration<LocationStatus>(this, LocationStatus.INACTIVE); 172 throw new FHIRException("Unknown LocationStatus code '" + codeString + "'"); 173 } 174 175 public String toCode(LocationStatus code) 176 { 177 if (code == LocationStatus.NULL) 178 return null; 179 if (code == LocationStatus.ACTIVE) 180 return "active"; 181 if (code == LocationStatus.SUSPENDED) 182 return "suspended"; 183 if (code == LocationStatus.INACTIVE) 184 return "inactive"; 185 return "?"; 186 } 187 } 188 189 public enum LocationMode { 190 /** 191 * The Location resource represents a specific instance of a location (e.g. 192 * Operating Theatre 1A). 193 */ 194 INSTANCE, 195 /** 196 * The Location represents a class of locations (e.g. Any Operating Theatre) 197 * although this class of locations could be constrained within a specific 198 * boundary (such as organization, or parent location, address etc.). 199 */ 200 KIND, 201 /** 202 * added to help the parsers 203 */ 204 NULL; 205 206 public static LocationMode fromCode(String codeString) throws FHIRException { 207 if (codeString == null || "".equals(codeString)) 208 return null; 209 if ("instance".equals(codeString)) 210 return INSTANCE; 211 if ("kind".equals(codeString)) 212 return KIND; 213 throw new FHIRException("Unknown LocationMode code '" + codeString + "'"); 214 } 215 216 public String toCode() { 217 switch (this) { 218 case INSTANCE: 219 return "instance"; 220 case KIND: 221 return "kind"; 222 case NULL: 223 return null; 224 default: 225 return "?"; 226 } 227 } 228 229 public String getSystem() { 230 switch (this) { 231 case INSTANCE: 232 return "http://hl7.org/fhir/location-mode"; 233 case KIND: 234 return "http://hl7.org/fhir/location-mode"; 235 case NULL: 236 return null; 237 default: 238 return "?"; 239 } 240 } 241 242 public String getDefinition() { 243 switch (this) { 244 case INSTANCE: 245 return "The Location resource represents a specific instance of a location (e.g. Operating Theatre 1A)."; 246 case KIND: 247 return "The Location represents a class of locations (e.g. Any Operating Theatre) although this class of locations could be constrained within a specific boundary (such as organization, or parent location, address etc.)."; 248 case NULL: 249 return null; 250 default: 251 return "?"; 252 } 253 } 254 255 public String getDisplay() { 256 switch (this) { 257 case INSTANCE: 258 return "Instance"; 259 case KIND: 260 return "Kind"; 261 case NULL: 262 return null; 263 default: 264 return "?"; 265 } 266 } 267 } 268 269 public static class LocationModeEnumFactory implements EnumFactory<LocationMode> { 270 public LocationMode fromCode(String codeString) throws IllegalArgumentException { 271 if (codeString == null || "".equals(codeString)) 272 if (codeString == null || "".equals(codeString)) 273 return null; 274 if ("instance".equals(codeString)) 275 return LocationMode.INSTANCE; 276 if ("kind".equals(codeString)) 277 return LocationMode.KIND; 278 throw new IllegalArgumentException("Unknown LocationMode code '" + codeString + "'"); 279 } 280 281 public Enumeration<LocationMode> fromType(Base code) throws FHIRException { 282 if (code == null || code.isEmpty()) 283 return null; 284 String codeString = ((PrimitiveType) code).asStringValue(); 285 if (codeString == null || "".equals(codeString)) 286 return null; 287 if ("instance".equals(codeString)) 288 return new Enumeration<LocationMode>(this, LocationMode.INSTANCE); 289 if ("kind".equals(codeString)) 290 return new Enumeration<LocationMode>(this, LocationMode.KIND); 291 throw new FHIRException("Unknown LocationMode code '" + codeString + "'"); 292 } 293 294 public String toCode(LocationMode code) 295 { 296 if (code == LocationMode.NULL) 297 return null; 298 if (code == LocationMode.INSTANCE) 299 return "instance"; 300 if (code == LocationMode.KIND) 301 return "kind"; 302 return "?"; 303 } 304 } 305 306 @Block() 307 public static class LocationPositionComponent extends BackboneElement implements IBaseBackboneElement { 308 /** 309 * Longitude. The value domain and the interpretation are the same as for the 310 * text of the longitude element in KML (see notes below). 311 */ 312 @Child(name = "longitude", type = { 313 DecimalType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 314 @Description(shortDefinition = "Longitude with WGS84 datum", formalDefinition = "Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below).") 315 protected DecimalType longitude; 316 317 /** 318 * Latitude. The value domain and the interpretation are the same as for the 319 * text of the latitude element in KML (see notes below). 320 */ 321 @Child(name = "latitude", type = { 322 DecimalType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 323 @Description(shortDefinition = "Latitude with WGS84 datum", formalDefinition = "Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below).") 324 protected DecimalType latitude; 325 326 /** 327 * Altitude. The value domain and the interpretation are the same as for the 328 * text of the altitude element in KML (see notes below). 329 */ 330 @Child(name = "altitude", type = { 331 DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 332 @Description(shortDefinition = "Altitude with WGS84 datum", formalDefinition = "Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below).") 333 protected DecimalType altitude; 334 335 private static final long serialVersionUID = -74276134L; 336 337 /* 338 * Constructor 339 */ 340 public LocationPositionComponent() { 341 super(); 342 } 343 344 /* 345 * Constructor 346 */ 347 public LocationPositionComponent(DecimalType longitude, DecimalType latitude) { 348 super(); 349 this.longitude = longitude; 350 this.latitude = latitude; 351 } 352 353 /** 354 * @return {@link #longitude} (Longitude. The value domain and the 355 * interpretation are the same as for the text of the longitude element 356 * in KML (see notes below).). This is the underlying object with id, 357 * value and extensions. The accessor "getLongitude" gives direct access 358 * to the value 359 */ 360 public DecimalType getLongitudeElement() { 361 if (this.longitude == null) 362 if (Configuration.errorOnAutoCreate()) 363 throw new Error("Attempt to auto-create LocationPositionComponent.longitude"); 364 else if (Configuration.doAutoCreate()) 365 this.longitude = new DecimalType(); // bb 366 return this.longitude; 367 } 368 369 public boolean hasLongitudeElement() { 370 return this.longitude != null && !this.longitude.isEmpty(); 371 } 372 373 public boolean hasLongitude() { 374 return this.longitude != null && !this.longitude.isEmpty(); 375 } 376 377 /** 378 * @param value {@link #longitude} (Longitude. The value domain and the 379 * interpretation are the same as for the text of the longitude 380 * element in KML (see notes below).). This is the underlying 381 * object with id, value and extensions. The accessor 382 * "getLongitude" gives direct access to the value 383 */ 384 public LocationPositionComponent setLongitudeElement(DecimalType value) { 385 this.longitude = value; 386 return this; 387 } 388 389 /** 390 * @return Longitude. The value domain and the interpretation are the same as 391 * for the text of the longitude element in KML (see notes below). 392 */ 393 public BigDecimal getLongitude() { 394 return this.longitude == null ? null : this.longitude.getValue(); 395 } 396 397 /** 398 * @param value Longitude. The value domain and the interpretation are the same 399 * as for the text of the longitude element in KML (see notes 400 * below). 401 */ 402 public LocationPositionComponent setLongitude(BigDecimal value) { 403 if (this.longitude == null) 404 this.longitude = new DecimalType(); 405 this.longitude.setValue(value); 406 return this; 407 } 408 409 /** 410 * @return {@link #latitude} (Latitude. The value domain and the interpretation 411 * are the same as for the text of the latitude element in KML (see 412 * notes below).). This is the underlying object with id, value and 413 * extensions. The accessor "getLatitude" gives direct access to the 414 * value 415 */ 416 public DecimalType getLatitudeElement() { 417 if (this.latitude == null) 418 if (Configuration.errorOnAutoCreate()) 419 throw new Error("Attempt to auto-create LocationPositionComponent.latitude"); 420 else if (Configuration.doAutoCreate()) 421 this.latitude = new DecimalType(); // bb 422 return this.latitude; 423 } 424 425 public boolean hasLatitudeElement() { 426 return this.latitude != null && !this.latitude.isEmpty(); 427 } 428 429 public boolean hasLatitude() { 430 return this.latitude != null && !this.latitude.isEmpty(); 431 } 432 433 /** 434 * @param value {@link #latitude} (Latitude. The value domain and the 435 * interpretation are the same as for the text of the latitude 436 * element in KML (see notes below).). This is the underlying 437 * object with id, value and extensions. The accessor "getLatitude" 438 * gives direct access to the value 439 */ 440 public LocationPositionComponent setLatitudeElement(DecimalType value) { 441 this.latitude = value; 442 return this; 443 } 444 445 /** 446 * @return Latitude. The value domain and the interpretation are the same as for 447 * the text of the latitude element in KML (see notes below). 448 */ 449 public BigDecimal getLatitude() { 450 return this.latitude == null ? null : this.latitude.getValue(); 451 } 452 453 /** 454 * @param value Latitude. The value domain and the interpretation are the same 455 * as for the text of the latitude element in KML (see notes 456 * below). 457 */ 458 public LocationPositionComponent setLatitude(BigDecimal value) { 459 if (this.latitude == null) 460 this.latitude = new DecimalType(); 461 this.latitude.setValue(value); 462 return this; 463 } 464 465 /** 466 * @return {@link #altitude} (Altitude. The value domain and the interpretation 467 * are the same as for the text of the altitude element in KML (see 468 * notes below).). This is the underlying object with id, value and 469 * extensions. The accessor "getAltitude" gives direct access to the 470 * value 471 */ 472 public DecimalType getAltitudeElement() { 473 if (this.altitude == null) 474 if (Configuration.errorOnAutoCreate()) 475 throw new Error("Attempt to auto-create LocationPositionComponent.altitude"); 476 else if (Configuration.doAutoCreate()) 477 this.altitude = new DecimalType(); // bb 478 return this.altitude; 479 } 480 481 public boolean hasAltitudeElement() { 482 return this.altitude != null && !this.altitude.isEmpty(); 483 } 484 485 public boolean hasAltitude() { 486 return this.altitude != null && !this.altitude.isEmpty(); 487 } 488 489 /** 490 * @param value {@link #altitude} (Altitude. The value domain and the 491 * interpretation are the same as for the text of the altitude 492 * element in KML (see notes below).). This is the underlying 493 * object with id, value and extensions. The accessor "getAltitude" 494 * gives direct access to the value 495 */ 496 public LocationPositionComponent setAltitudeElement(DecimalType value) { 497 this.altitude = value; 498 return this; 499 } 500 501 /** 502 * @return Altitude. The value domain and the interpretation are the same as for 503 * the text of the altitude element in KML (see notes below). 504 */ 505 public BigDecimal getAltitude() { 506 return this.altitude == null ? null : this.altitude.getValue(); 507 } 508 509 /** 510 * @param value Altitude. The value domain and the interpretation are the same 511 * as for the text of the altitude element in KML (see notes 512 * below). 513 */ 514 public LocationPositionComponent setAltitude(BigDecimal value) { 515 if (value == null) 516 this.altitude = null; 517 else { 518 if (this.altitude == null) 519 this.altitude = new DecimalType(); 520 this.altitude.setValue(value); 521 } 522 return this; 523 } 524 525 protected void listChildren(List<Property> childrenList) { 526 super.listChildren(childrenList); 527 childrenList.add(new Property("longitude", "decimal", 528 "Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below).", 529 0, java.lang.Integer.MAX_VALUE, longitude)); 530 childrenList.add(new Property("latitude", "decimal", 531 "Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below).", 532 0, java.lang.Integer.MAX_VALUE, latitude)); 533 childrenList.add(new Property("altitude", "decimal", 534 "Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below).", 535 0, java.lang.Integer.MAX_VALUE, altitude)); 536 } 537 538 @Override 539 public void setProperty(String name, Base value) throws FHIRException { 540 if (name.equals("longitude")) 541 this.longitude = castToDecimal(value); // DecimalType 542 else if (name.equals("latitude")) 543 this.latitude = castToDecimal(value); // DecimalType 544 else if (name.equals("altitude")) 545 this.altitude = castToDecimal(value); // DecimalType 546 else 547 super.setProperty(name, value); 548 } 549 550 @Override 551 public Base addChild(String name) throws FHIRException { 552 if (name.equals("longitude")) { 553 throw new FHIRException("Cannot call addChild on a singleton property Location.longitude"); 554 } else if (name.equals("latitude")) { 555 throw new FHIRException("Cannot call addChild on a singleton property Location.latitude"); 556 } else if (name.equals("altitude")) { 557 throw new FHIRException("Cannot call addChild on a singleton property Location.altitude"); 558 } else 559 return super.addChild(name); 560 } 561 562 public LocationPositionComponent copy() { 563 LocationPositionComponent dst = new LocationPositionComponent(); 564 copyValues(dst); 565 dst.longitude = longitude == null ? null : longitude.copy(); 566 dst.latitude = latitude == null ? null : latitude.copy(); 567 dst.altitude = altitude == null ? null : altitude.copy(); 568 return dst; 569 } 570 571 @Override 572 public boolean equalsDeep(Base other) { 573 if (!super.equalsDeep(other)) 574 return false; 575 if (!(other instanceof LocationPositionComponent)) 576 return false; 577 LocationPositionComponent o = (LocationPositionComponent) other; 578 return compareDeep(longitude, o.longitude, true) && compareDeep(latitude, o.latitude, true) 579 && compareDeep(altitude, o.altitude, true); 580 } 581 582 @Override 583 public boolean equalsShallow(Base other) { 584 if (!super.equalsShallow(other)) 585 return false; 586 if (!(other instanceof LocationPositionComponent)) 587 return false; 588 LocationPositionComponent o = (LocationPositionComponent) other; 589 return compareValues(longitude, o.longitude, true) && compareValues(latitude, o.latitude, true) 590 && compareValues(altitude, o.altitude, true); 591 } 592 593 public boolean isEmpty() { 594 return super.isEmpty() && (longitude == null || longitude.isEmpty()) && (latitude == null || latitude.isEmpty()) 595 && (altitude == null || altitude.isEmpty()); 596 } 597 598 public String fhirType() { 599 return "Location.position"; 600 601 } 602 603 } 604 605 /** 606 * Unique code or number identifying the location to its users. 607 */ 608 @Child(name = "identifier", type = { 609 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 610 @Description(shortDefinition = "Unique code or number identifying the location to its users", formalDefinition = "Unique code or number identifying the location to its users.") 611 protected List<Identifier> identifier; 612 613 /** 614 * active | suspended | inactive. 615 */ 616 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 617 @Description(shortDefinition = "active | suspended | inactive", formalDefinition = "active | suspended | inactive.") 618 protected Enumeration<LocationStatus> status; 619 620 /** 621 * Name of the location as used by humans. Does not need to be unique. 622 */ 623 @Child(name = "name", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 624 @Description(shortDefinition = "Name of the location as used by humans", formalDefinition = "Name of the location as used by humans. Does not need to be unique.") 625 protected StringType name; 626 627 /** 628 * Description of the Location, which helps in finding or referencing the place. 629 */ 630 @Child(name = "description", type = { 631 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 632 @Description(shortDefinition = "Description of the location", formalDefinition = "Description of the Location, which helps in finding or referencing the place.") 633 protected StringType description; 634 635 /** 636 * Indicates whether a resource instance represents a specific location or a 637 * class of locations. 638 */ 639 @Child(name = "mode", type = { CodeType.class }, order = 4, min = 0, max = 1, modifier = true, summary = true) 640 @Description(shortDefinition = "instance | kind", formalDefinition = "Indicates whether a resource instance represents a specific location or a class of locations.") 641 protected Enumeration<LocationMode> mode; 642 643 /** 644 * Indicates the type of function performed at the location. 645 */ 646 @Child(name = "type", type = { CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 647 @Description(shortDefinition = "Type of function performed", formalDefinition = "Indicates the type of function performed at the location.") 648 protected CodeableConcept type; 649 650 /** 651 * The contact details of communication devices available at the location. This 652 * can include phone numbers, fax numbers, mobile numbers, email addresses and 653 * web sites. 654 */ 655 @Child(name = "telecom", type = { 656 ContactPoint.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 657 @Description(shortDefinition = "Contact details of the location", formalDefinition = "The contact details of communication devices available at the location. This can include phone numbers, fax numbers, mobile numbers, email addresses and web sites.") 658 protected List<ContactPoint> telecom; 659 660 /** 661 * Physical location. 662 */ 663 @Child(name = "address", type = { Address.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 664 @Description(shortDefinition = "Physical location", formalDefinition = "Physical location.") 665 protected Address address; 666 667 /** 668 * Physical form of the location, e.g. building, room, vehicle, road. 669 */ 670 @Child(name = "physicalType", type = { 671 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 672 @Description(shortDefinition = "Physical form of the location", formalDefinition = "Physical form of the location, e.g. building, room, vehicle, road.") 673 protected CodeableConcept physicalType; 674 675 /** 676 * The absolute geographic location of the Location, expressed using the WGS84 677 * datum (This is the same co-ordinate system used in KML). 678 */ 679 @Child(name = "position", type = {}, order = 9, min = 0, max = 1, modifier = false, summary = false) 680 @Description(shortDefinition = "The absolute geographic location", formalDefinition = "The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML).") 681 protected LocationPositionComponent position; 682 683 /** 684 * The organization responsible for the provisioning and upkeep of the location. 685 */ 686 @Child(name = "managingOrganization", type = { 687 Organization.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 688 @Description(shortDefinition = "Organization responsible for provisioning and upkeep", formalDefinition = "The organization responsible for the provisioning and upkeep of the location.") 689 protected Reference managingOrganization; 690 691 /** 692 * The actual object that is the target of the reference (The organization 693 * responsible for the provisioning and upkeep of the location.) 694 */ 695 protected Organization managingOrganizationTarget; 696 697 /** 698 * Another Location which this Location is physically part of. 699 */ 700 @Child(name = "partOf", type = { Location.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 701 @Description(shortDefinition = "Another Location this one is physically part of", formalDefinition = "Another Location which this Location is physically part of.") 702 protected Reference partOf; 703 704 /** 705 * The actual object that is the target of the reference (Another Location which 706 * this Location is physically part of.) 707 */ 708 protected Location partOfTarget; 709 710 private static final long serialVersionUID = -2100435761L; 711 712 /* 713 * Constructor 714 */ 715 public Location() { 716 super(); 717 } 718 719 /** 720 * @return {@link #identifier} (Unique code or number identifying the location 721 * to its users.) 722 */ 723 public List<Identifier> getIdentifier() { 724 if (this.identifier == null) 725 this.identifier = new ArrayList<Identifier>(); 726 return this.identifier; 727 } 728 729 public boolean hasIdentifier() { 730 if (this.identifier == null) 731 return false; 732 for (Identifier item : this.identifier) 733 if (!item.isEmpty()) 734 return true; 735 return false; 736 } 737 738 /** 739 * @return {@link #identifier} (Unique code or number identifying the location 740 * to its users.) 741 */ 742 // syntactic sugar 743 public Identifier addIdentifier() { // 3 744 Identifier t = new Identifier(); 745 if (this.identifier == null) 746 this.identifier = new ArrayList<Identifier>(); 747 this.identifier.add(t); 748 return t; 749 } 750 751 // syntactic sugar 752 public Location addIdentifier(Identifier t) { // 3 753 if (t == null) 754 return this; 755 if (this.identifier == null) 756 this.identifier = new ArrayList<Identifier>(); 757 this.identifier.add(t); 758 return this; 759 } 760 761 /** 762 * @return {@link #status} (active | suspended | inactive.). This is the 763 * underlying object with id, value and extensions. The accessor 764 * "getStatus" gives direct access to the value 765 */ 766 public Enumeration<LocationStatus> getStatusElement() { 767 if (this.status == null) 768 if (Configuration.errorOnAutoCreate()) 769 throw new Error("Attempt to auto-create Location.status"); 770 else if (Configuration.doAutoCreate()) 771 this.status = new Enumeration<LocationStatus>(new LocationStatusEnumFactory()); // bb 772 return this.status; 773 } 774 775 public boolean hasStatusElement() { 776 return this.status != null && !this.status.isEmpty(); 777 } 778 779 public boolean hasStatus() { 780 return this.status != null && !this.status.isEmpty(); 781 } 782 783 /** 784 * @param value {@link #status} (active | suspended | inactive.). This is the 785 * underlying object with id, value and extensions. The accessor 786 * "getStatus" gives direct access to the value 787 */ 788 public Location setStatusElement(Enumeration<LocationStatus> value) { 789 this.status = value; 790 return this; 791 } 792 793 /** 794 * @return active | suspended | inactive. 795 */ 796 public LocationStatus getStatus() { 797 return this.status == null ? null : this.status.getValue(); 798 } 799 800 /** 801 * @param value active | suspended | inactive. 802 */ 803 public Location setStatus(LocationStatus value) { 804 if (value == null) 805 this.status = null; 806 else { 807 if (this.status == null) 808 this.status = new Enumeration<LocationStatus>(new LocationStatusEnumFactory()); 809 this.status.setValue(value); 810 } 811 return this; 812 } 813 814 /** 815 * @return {@link #name} (Name of the location as used by humans. Does not need 816 * to be unique.). This is the underlying object with id, value and 817 * extensions. The accessor "getName" gives direct access to the value 818 */ 819 public StringType getNameElement() { 820 if (this.name == null) 821 if (Configuration.errorOnAutoCreate()) 822 throw new Error("Attempt to auto-create Location.name"); 823 else if (Configuration.doAutoCreate()) 824 this.name = new StringType(); // bb 825 return this.name; 826 } 827 828 public boolean hasNameElement() { 829 return this.name != null && !this.name.isEmpty(); 830 } 831 832 public boolean hasName() { 833 return this.name != null && !this.name.isEmpty(); 834 } 835 836 /** 837 * @param value {@link #name} (Name of the location as used by humans. Does not 838 * need to be unique.). This is the underlying object with id, 839 * value and extensions. The accessor "getName" gives direct access 840 * to the value 841 */ 842 public Location setNameElement(StringType value) { 843 this.name = value; 844 return this; 845 } 846 847 /** 848 * @return Name of the location as used by humans. Does not need to be unique. 849 */ 850 public String getName() { 851 return this.name == null ? null : this.name.getValue(); 852 } 853 854 /** 855 * @param value Name of the location as used by humans. Does not need to be 856 * unique. 857 */ 858 public Location setName(String value) { 859 if (Utilities.noString(value)) 860 this.name = null; 861 else { 862 if (this.name == null) 863 this.name = new StringType(); 864 this.name.setValue(value); 865 } 866 return this; 867 } 868 869 /** 870 * @return {@link #description} (Description of the Location, which helps in 871 * finding or referencing the place.). This is the underlying object 872 * with id, value and extensions. The accessor "getDescription" gives 873 * direct access to the value 874 */ 875 public StringType getDescriptionElement() { 876 if (this.description == null) 877 if (Configuration.errorOnAutoCreate()) 878 throw new Error("Attempt to auto-create Location.description"); 879 else if (Configuration.doAutoCreate()) 880 this.description = new StringType(); // bb 881 return this.description; 882 } 883 884 public boolean hasDescriptionElement() { 885 return this.description != null && !this.description.isEmpty(); 886 } 887 888 public boolean hasDescription() { 889 return this.description != null && !this.description.isEmpty(); 890 } 891 892 /** 893 * @param value {@link #description} (Description of the Location, which helps 894 * in finding or referencing the place.). This is the underlying 895 * object with id, value and extensions. The accessor 896 * "getDescription" gives direct access to the value 897 */ 898 public Location setDescriptionElement(StringType value) { 899 this.description = value; 900 return this; 901 } 902 903 /** 904 * @return Description of the Location, which helps in finding or referencing 905 * the place. 906 */ 907 public String getDescription() { 908 return this.description == null ? null : this.description.getValue(); 909 } 910 911 /** 912 * @param value Description of the Location, which helps in finding or 913 * referencing the place. 914 */ 915 public Location setDescription(String value) { 916 if (Utilities.noString(value)) 917 this.description = null; 918 else { 919 if (this.description == null) 920 this.description = new StringType(); 921 this.description.setValue(value); 922 } 923 return this; 924 } 925 926 /** 927 * @return {@link #mode} (Indicates whether a resource instance represents a 928 * specific location or a class of locations.). This is the underlying 929 * object with id, value and extensions. The accessor "getMode" gives 930 * direct access to the value 931 */ 932 public Enumeration<LocationMode> getModeElement() { 933 if (this.mode == null) 934 if (Configuration.errorOnAutoCreate()) 935 throw new Error("Attempt to auto-create Location.mode"); 936 else if (Configuration.doAutoCreate()) 937 this.mode = new Enumeration<LocationMode>(new LocationModeEnumFactory()); // bb 938 return this.mode; 939 } 940 941 public boolean hasModeElement() { 942 return this.mode != null && !this.mode.isEmpty(); 943 } 944 945 public boolean hasMode() { 946 return this.mode != null && !this.mode.isEmpty(); 947 } 948 949 /** 950 * @param value {@link #mode} (Indicates whether a resource instance represents 951 * a specific location or a class of locations.). This is the 952 * underlying object with id, value and extensions. The accessor 953 * "getMode" gives direct access to the value 954 */ 955 public Location setModeElement(Enumeration<LocationMode> value) { 956 this.mode = value; 957 return this; 958 } 959 960 /** 961 * @return Indicates whether a resource instance represents a specific location 962 * or a class of locations. 963 */ 964 public LocationMode getMode() { 965 return this.mode == null ? null : this.mode.getValue(); 966 } 967 968 /** 969 * @param value Indicates whether a resource instance represents a specific 970 * location or a class of locations. 971 */ 972 public Location setMode(LocationMode value) { 973 if (value == null) 974 this.mode = null; 975 else { 976 if (this.mode == null) 977 this.mode = new Enumeration<LocationMode>(new LocationModeEnumFactory()); 978 this.mode.setValue(value); 979 } 980 return this; 981 } 982 983 /** 984 * @return {@link #type} (Indicates the type of function performed at the 985 * location.) 986 */ 987 public CodeableConcept getType() { 988 if (this.type == null) 989 if (Configuration.errorOnAutoCreate()) 990 throw new Error("Attempt to auto-create Location.type"); 991 else if (Configuration.doAutoCreate()) 992 this.type = new CodeableConcept(); // cc 993 return this.type; 994 } 995 996 public boolean hasType() { 997 return this.type != null && !this.type.isEmpty(); 998 } 999 1000 /** 1001 * @param value {@link #type} (Indicates the type of function performed at the 1002 * location.) 1003 */ 1004 public Location setType(CodeableConcept value) { 1005 this.type = value; 1006 return this; 1007 } 1008 1009 /** 1010 * @return {@link #telecom} (The contact details of communication devices 1011 * available at the location. This can include phone numbers, fax 1012 * numbers, mobile numbers, email addresses and web sites.) 1013 */ 1014 public List<ContactPoint> getTelecom() { 1015 if (this.telecom == null) 1016 this.telecom = new ArrayList<ContactPoint>(); 1017 return this.telecom; 1018 } 1019 1020 public boolean hasTelecom() { 1021 if (this.telecom == null) 1022 return false; 1023 for (ContactPoint item : this.telecom) 1024 if (!item.isEmpty()) 1025 return true; 1026 return false; 1027 } 1028 1029 /** 1030 * @return {@link #telecom} (The contact details of communication devices 1031 * available at the location. This can include phone numbers, fax 1032 * numbers, mobile numbers, email addresses and web sites.) 1033 */ 1034 // syntactic sugar 1035 public ContactPoint addTelecom() { // 3 1036 ContactPoint t = new ContactPoint(); 1037 if (this.telecom == null) 1038 this.telecom = new ArrayList<ContactPoint>(); 1039 this.telecom.add(t); 1040 return t; 1041 } 1042 1043 // syntactic sugar 1044 public Location addTelecom(ContactPoint t) { // 3 1045 if (t == null) 1046 return this; 1047 if (this.telecom == null) 1048 this.telecom = new ArrayList<ContactPoint>(); 1049 this.telecom.add(t); 1050 return this; 1051 } 1052 1053 /** 1054 * @return {@link #address} (Physical location.) 1055 */ 1056 public Address getAddress() { 1057 if (this.address == null) 1058 if (Configuration.errorOnAutoCreate()) 1059 throw new Error("Attempt to auto-create Location.address"); 1060 else if (Configuration.doAutoCreate()) 1061 this.address = new Address(); // cc 1062 return this.address; 1063 } 1064 1065 public boolean hasAddress() { 1066 return this.address != null && !this.address.isEmpty(); 1067 } 1068 1069 /** 1070 * @param value {@link #address} (Physical location.) 1071 */ 1072 public Location setAddress(Address value) { 1073 this.address = value; 1074 return this; 1075 } 1076 1077 /** 1078 * @return {@link #physicalType} (Physical form of the location, e.g. building, 1079 * room, vehicle, road.) 1080 */ 1081 public CodeableConcept getPhysicalType() { 1082 if (this.physicalType == null) 1083 if (Configuration.errorOnAutoCreate()) 1084 throw new Error("Attempt to auto-create Location.physicalType"); 1085 else if (Configuration.doAutoCreate()) 1086 this.physicalType = new CodeableConcept(); // cc 1087 return this.physicalType; 1088 } 1089 1090 public boolean hasPhysicalType() { 1091 return this.physicalType != null && !this.physicalType.isEmpty(); 1092 } 1093 1094 /** 1095 * @param value {@link #physicalType} (Physical form of the location, e.g. 1096 * building, room, vehicle, road.) 1097 */ 1098 public Location setPhysicalType(CodeableConcept value) { 1099 this.physicalType = value; 1100 return this; 1101 } 1102 1103 /** 1104 * @return {@link #position} (The absolute geographic location of the Location, 1105 * expressed using the WGS84 datum (This is the same co-ordinate system 1106 * used in KML).) 1107 */ 1108 public LocationPositionComponent getPosition() { 1109 if (this.position == null) 1110 if (Configuration.errorOnAutoCreate()) 1111 throw new Error("Attempt to auto-create Location.position"); 1112 else if (Configuration.doAutoCreate()) 1113 this.position = new LocationPositionComponent(); // cc 1114 return this.position; 1115 } 1116 1117 public boolean hasPosition() { 1118 return this.position != null && !this.position.isEmpty(); 1119 } 1120 1121 /** 1122 * @param value {@link #position} (The absolute geographic location of the 1123 * Location, expressed using the WGS84 datum (This is the same 1124 * co-ordinate system used in KML).) 1125 */ 1126 public Location setPosition(LocationPositionComponent value) { 1127 this.position = value; 1128 return this; 1129 } 1130 1131 /** 1132 * @return {@link #managingOrganization} (The organization responsible for the 1133 * provisioning and upkeep of the location.) 1134 */ 1135 public Reference getManagingOrganization() { 1136 if (this.managingOrganization == null) 1137 if (Configuration.errorOnAutoCreate()) 1138 throw new Error("Attempt to auto-create Location.managingOrganization"); 1139 else if (Configuration.doAutoCreate()) 1140 this.managingOrganization = new Reference(); // cc 1141 return this.managingOrganization; 1142 } 1143 1144 public boolean hasManagingOrganization() { 1145 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 1146 } 1147 1148 /** 1149 * @param value {@link #managingOrganization} (The organization responsible for 1150 * the provisioning and upkeep of the location.) 1151 */ 1152 public Location setManagingOrganization(Reference value) { 1153 this.managingOrganization = value; 1154 return this; 1155 } 1156 1157 /** 1158 * @return {@link #managingOrganization} The actual object that is the target of 1159 * the reference. The reference library doesn't populate this, but you 1160 * can use it to hold the resource if you resolve it. (The organization 1161 * responsible for the provisioning and upkeep of the location.) 1162 */ 1163 public Organization getManagingOrganizationTarget() { 1164 if (this.managingOrganizationTarget == null) 1165 if (Configuration.errorOnAutoCreate()) 1166 throw new Error("Attempt to auto-create Location.managingOrganization"); 1167 else if (Configuration.doAutoCreate()) 1168 this.managingOrganizationTarget = new Organization(); // aa 1169 return this.managingOrganizationTarget; 1170 } 1171 1172 /** 1173 * @param value {@link #managingOrganization} The actual object that is the 1174 * target of the reference. The reference library doesn't use 1175 * these, but you can use it to hold the resource if you resolve 1176 * it. (The organization responsible for the provisioning and 1177 * upkeep of the location.) 1178 */ 1179 public Location setManagingOrganizationTarget(Organization value) { 1180 this.managingOrganizationTarget = value; 1181 return this; 1182 } 1183 1184 /** 1185 * @return {@link #partOf} (Another Location which this Location is physically 1186 * part of.) 1187 */ 1188 public Reference getPartOf() { 1189 if (this.partOf == null) 1190 if (Configuration.errorOnAutoCreate()) 1191 throw new Error("Attempt to auto-create Location.partOf"); 1192 else if (Configuration.doAutoCreate()) 1193 this.partOf = new Reference(); // cc 1194 return this.partOf; 1195 } 1196 1197 public boolean hasPartOf() { 1198 return this.partOf != null && !this.partOf.isEmpty(); 1199 } 1200 1201 /** 1202 * @param value {@link #partOf} (Another Location which this Location is 1203 * physically part of.) 1204 */ 1205 public Location setPartOf(Reference value) { 1206 this.partOf = value; 1207 return this; 1208 } 1209 1210 /** 1211 * @return {@link #partOf} The actual object that is the target of the 1212 * reference. The reference library doesn't populate this, but you can 1213 * use it to hold the resource if you resolve it. (Another Location 1214 * which this Location is physically part of.) 1215 */ 1216 public Location getPartOfTarget() { 1217 if (this.partOfTarget == null) 1218 if (Configuration.errorOnAutoCreate()) 1219 throw new Error("Attempt to auto-create Location.partOf"); 1220 else if (Configuration.doAutoCreate()) 1221 this.partOfTarget = new Location(); // aa 1222 return this.partOfTarget; 1223 } 1224 1225 /** 1226 * @param value {@link #partOf} The actual object that is the target of the 1227 * reference. The reference library doesn't use these, but you can 1228 * use it to hold the resource if you resolve it. (Another Location 1229 * which this Location is physically part of.) 1230 */ 1231 public Location setPartOfTarget(Location value) { 1232 this.partOfTarget = value; 1233 return this; 1234 } 1235 1236 protected void listChildren(List<Property> childrenList) { 1237 super.listChildren(childrenList); 1238 childrenList.add(new Property("identifier", "Identifier", 1239 "Unique code or number identifying the location to its users.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1240 childrenList 1241 .add(new Property("status", "code", "active | suspended | inactive.", 0, java.lang.Integer.MAX_VALUE, status)); 1242 childrenList.add(new Property("name", "string", 1243 "Name of the location as used by humans. Does not need to be unique.", 0, java.lang.Integer.MAX_VALUE, name)); 1244 childrenList.add(new Property("description", "string", 1245 "Description of the Location, which helps in finding or referencing the place.", 0, java.lang.Integer.MAX_VALUE, 1246 description)); 1247 childrenList.add(new Property("mode", "code", 1248 "Indicates whether a resource instance represents a specific location or a class of locations.", 0, 1249 java.lang.Integer.MAX_VALUE, mode)); 1250 childrenList.add(new Property("type", "CodeableConcept", 1251 "Indicates the type of function performed at the location.", 0, java.lang.Integer.MAX_VALUE, type)); 1252 childrenList.add(new Property("telecom", "ContactPoint", 1253 "The contact details of communication devices available at the location. This can include phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 1254 0, java.lang.Integer.MAX_VALUE, telecom)); 1255 childrenList.add(new Property("address", "Address", "Physical location.", 0, java.lang.Integer.MAX_VALUE, address)); 1256 childrenList.add(new Property("physicalType", "CodeableConcept", 1257 "Physical form of the location, e.g. building, room, vehicle, road.", 0, java.lang.Integer.MAX_VALUE, 1258 physicalType)); 1259 childrenList.add(new Property("position", "", 1260 "The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML).", 1261 0, java.lang.Integer.MAX_VALUE, position)); 1262 childrenList.add(new Property("managingOrganization", "Reference(Organization)", 1263 "The organization responsible for the provisioning and upkeep of the location.", 0, java.lang.Integer.MAX_VALUE, 1264 managingOrganization)); 1265 childrenList.add(new Property("partOf", "Reference(Location)", 1266 "Another Location which this Location is physically part of.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1267 } 1268 1269 @Override 1270 public void setProperty(String name, Base value) throws FHIRException { 1271 if (name.equals("identifier")) 1272 this.getIdentifier().add(castToIdentifier(value)); 1273 else if (name.equals("status")) 1274 this.status = new LocationStatusEnumFactory().fromType(value); // Enumeration<LocationStatus> 1275 else if (name.equals("name")) 1276 this.name = castToString(value); // StringType 1277 else if (name.equals("description")) 1278 this.description = castToString(value); // StringType 1279 else if (name.equals("mode")) 1280 this.mode = new LocationModeEnumFactory().fromType(value); // Enumeration<LocationMode> 1281 else if (name.equals("type")) 1282 this.type = castToCodeableConcept(value); // CodeableConcept 1283 else if (name.equals("telecom")) 1284 this.getTelecom().add(castToContactPoint(value)); 1285 else if (name.equals("address")) 1286 this.address = castToAddress(value); // Address 1287 else if (name.equals("physicalType")) 1288 this.physicalType = castToCodeableConcept(value); // CodeableConcept 1289 else if (name.equals("position")) 1290 this.position = (LocationPositionComponent) value; // LocationPositionComponent 1291 else if (name.equals("managingOrganization")) 1292 this.managingOrganization = castToReference(value); // Reference 1293 else if (name.equals("partOf")) 1294 this.partOf = castToReference(value); // Reference 1295 else 1296 super.setProperty(name, value); 1297 } 1298 1299 @Override 1300 public Base addChild(String name) throws FHIRException { 1301 if (name.equals("identifier")) { 1302 return addIdentifier(); 1303 } else if (name.equals("status")) { 1304 throw new FHIRException("Cannot call addChild on a singleton property Location.status"); 1305 } else if (name.equals("name")) { 1306 throw new FHIRException("Cannot call addChild on a singleton property Location.name"); 1307 } else if (name.equals("description")) { 1308 throw new FHIRException("Cannot call addChild on a singleton property Location.description"); 1309 } else if (name.equals("mode")) { 1310 throw new FHIRException("Cannot call addChild on a singleton property Location.mode"); 1311 } else if (name.equals("type")) { 1312 this.type = new CodeableConcept(); 1313 return this.type; 1314 } else if (name.equals("telecom")) { 1315 return addTelecom(); 1316 } else if (name.equals("address")) { 1317 this.address = new Address(); 1318 return this.address; 1319 } else if (name.equals("physicalType")) { 1320 this.physicalType = new CodeableConcept(); 1321 return this.physicalType; 1322 } else if (name.equals("position")) { 1323 this.position = new LocationPositionComponent(); 1324 return this.position; 1325 } else if (name.equals("managingOrganization")) { 1326 this.managingOrganization = new Reference(); 1327 return this.managingOrganization; 1328 } else if (name.equals("partOf")) { 1329 this.partOf = new Reference(); 1330 return this.partOf; 1331 } else 1332 return super.addChild(name); 1333 } 1334 1335 public String fhirType() { 1336 return "Location"; 1337 1338 } 1339 1340 public Location copy() { 1341 Location dst = new Location(); 1342 copyValues(dst); 1343 if (identifier != null) { 1344 dst.identifier = new ArrayList<Identifier>(); 1345 for (Identifier i : identifier) 1346 dst.identifier.add(i.copy()); 1347 } 1348 ; 1349 dst.status = status == null ? null : status.copy(); 1350 dst.name = name == null ? null : name.copy(); 1351 dst.description = description == null ? null : description.copy(); 1352 dst.mode = mode == null ? null : mode.copy(); 1353 dst.type = type == null ? null : type.copy(); 1354 if (telecom != null) { 1355 dst.telecom = new ArrayList<ContactPoint>(); 1356 for (ContactPoint i : telecom) 1357 dst.telecom.add(i.copy()); 1358 } 1359 ; 1360 dst.address = address == null ? null : address.copy(); 1361 dst.physicalType = physicalType == null ? null : physicalType.copy(); 1362 dst.position = position == null ? null : position.copy(); 1363 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 1364 dst.partOf = partOf == null ? null : partOf.copy(); 1365 return dst; 1366 } 1367 1368 protected Location typedCopy() { 1369 return copy(); 1370 } 1371 1372 @Override 1373 public boolean equalsDeep(Base other) { 1374 if (!super.equalsDeep(other)) 1375 return false; 1376 if (!(other instanceof Location)) 1377 return false; 1378 Location o = (Location) other; 1379 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1380 && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 1381 && compareDeep(mode, o.mode, true) && compareDeep(type, o.type, true) && compareDeep(telecom, o.telecom, true) 1382 && compareDeep(address, o.address, true) && compareDeep(physicalType, o.physicalType, true) 1383 && compareDeep(position, o.position, true) && compareDeep(managingOrganization, o.managingOrganization, true) 1384 && compareDeep(partOf, o.partOf, true); 1385 } 1386 1387 @Override 1388 public boolean equalsShallow(Base other) { 1389 if (!super.equalsShallow(other)) 1390 return false; 1391 if (!(other instanceof Location)) 1392 return false; 1393 Location o = (Location) other; 1394 return compareValues(status, o.status, true) && compareValues(name, o.name, true) 1395 && compareValues(description, o.description, true) && compareValues(mode, o.mode, true); 1396 } 1397 1398 public boolean isEmpty() { 1399 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (status == null || status.isEmpty()) 1400 && (name == null || name.isEmpty()) && (description == null || description.isEmpty()) 1401 && (mode == null || mode.isEmpty()) && (type == null || type.isEmpty()) 1402 && (telecom == null || telecom.isEmpty()) && (address == null || address.isEmpty()) 1403 && (physicalType == null || physicalType.isEmpty()) && (position == null || position.isEmpty()) 1404 && (managingOrganization == null || managingOrganization.isEmpty()) && (partOf == null || partOf.isEmpty()); 1405 } 1406 1407 @Override 1408 public ResourceType getResourceType() { 1409 return ResourceType.Location; 1410 } 1411 1412 @SearchParamDefinition(name = "identifier", path = "Location.identifier", description = "Unique code or number identifying the location to its users", type = "token") 1413 public static final String SP_IDENTIFIER = "identifier"; 1414 @SearchParamDefinition(name = "partof", path = "Location.partOf", description = "The location of which this location is a part", type = "reference") 1415 public static final String SP_PARTOF = "partof"; 1416 @SearchParamDefinition(name = "near-distance", path = "Location.position", description = "A distance quantity to limit the near search to locations within a specific distance", type = "token") 1417 public static final String SP_NEARDISTANCE = "near-distance"; 1418 @SearchParamDefinition(name = "address", path = "Location.address", description = "A (part of the) address of the location", type = "string") 1419 public static final String SP_ADDRESS = "address"; 1420 @SearchParamDefinition(name = "address-state", path = "Location.address.state", description = "A state specified in an address", type = "string") 1421 public static final String SP_ADDRESSSTATE = "address-state"; 1422 @SearchParamDefinition(name = "type", path = "Location.type", description = "A code for the type of location", type = "token") 1423 public static final String SP_TYPE = "type"; 1424 @SearchParamDefinition(name = "address-postalcode", path = "Location.address.postalCode", description = "A postal code specified in an address", type = "string") 1425 public static final String SP_ADDRESSPOSTALCODE = "address-postalcode"; 1426 @SearchParamDefinition(name = "address-country", path = "Location.address.country", description = "A country specified in an address", type = "string") 1427 public static final String SP_ADDRESSCOUNTRY = "address-country"; 1428 @SearchParamDefinition(name = "organization", path = "Location.managingOrganization", description = "Searches for locations that are managed by the provided organization", type = "reference") 1429 public static final String SP_ORGANIZATION = "organization"; 1430 @SearchParamDefinition(name = "name", path = "Location.name", description = "A (portion of the) name of the location", type = "string") 1431 public static final String SP_NAME = "name"; 1432 @SearchParamDefinition(name = "address-use", path = "Location.address.use", description = "A use code specified in an address", type = "token") 1433 public static final String SP_ADDRESSUSE = "address-use"; 1434 @SearchParamDefinition(name = "near", path = "Location.position", description = "The coordinates expressed as [lat],[long] (using the WGS84 datum, see notes) to find locations near to (servers may search using a square rather than a circle for efficiency)", type = "token") 1435 public static final String SP_NEAR = "near"; 1436 @SearchParamDefinition(name = "address-city", path = "Location.address.city", description = "A city specified in an address", type = "string") 1437 public static final String SP_ADDRESSCITY = "address-city"; 1438 @SearchParamDefinition(name = "status", path = "Location.status", description = "Searches for locations with a specific kind of status", type = "token") 1439 public static final String SP_STATUS = "status"; 1440 1441}