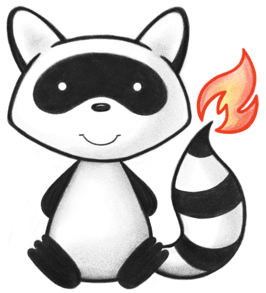
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Child; 037import ca.uhn.fhir.model.api.annotation.Description; 038import ca.uhn.fhir.model.api.annotation.ResourceDef; 039import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.utilities.Utilities; 042 043/** 044 * A photo, video, or audio recording acquired or used in healthcare. The actual 045 * content may be inline or provided by direct reference. 046 */ 047@ResourceDef(name = "Media", profile = "http://hl7.org/fhir/Profile/Media") 048public class Media extends DomainResource { 049 050 public enum DigitalMediaType { 051 /** 052 * The media consists of one or more unmoving images, including photographs, 053 * computer-generated graphs and charts, and scanned documents 054 */ 055 PHOTO, 056 /** 057 * The media consists of a series of frames that capture a moving image 058 */ 059 VIDEO, 060 /** 061 * The media consists of a sound recording 062 */ 063 AUDIO, 064 /** 065 * added to help the parsers 066 */ 067 NULL; 068 069 public static DigitalMediaType fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("photo".equals(codeString)) 073 return PHOTO; 074 if ("video".equals(codeString)) 075 return VIDEO; 076 if ("audio".equals(codeString)) 077 return AUDIO; 078 throw new FHIRException("Unknown DigitalMediaType code '" + codeString + "'"); 079 } 080 081 public String toCode() { 082 switch (this) { 083 case PHOTO: 084 return "photo"; 085 case VIDEO: 086 return "video"; 087 case AUDIO: 088 return "audio"; 089 case NULL: 090 return null; 091 default: 092 return "?"; 093 } 094 } 095 096 public String getSystem() { 097 switch (this) { 098 case PHOTO: 099 return "http://hl7.org/fhir/digital-media-type"; 100 case VIDEO: 101 return "http://hl7.org/fhir/digital-media-type"; 102 case AUDIO: 103 return "http://hl7.org/fhir/digital-media-type"; 104 case NULL: 105 return null; 106 default: 107 return "?"; 108 } 109 } 110 111 public String getDefinition() { 112 switch (this) { 113 case PHOTO: 114 return "The media consists of one or more unmoving images, including photographs, computer-generated graphs and charts, and scanned documents"; 115 case VIDEO: 116 return "The media consists of a series of frames that capture a moving image"; 117 case AUDIO: 118 return "The media consists of a sound recording"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getDisplay() { 127 switch (this) { 128 case PHOTO: 129 return "Photo"; 130 case VIDEO: 131 return "Video"; 132 case AUDIO: 133 return "Audio"; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 } 141 142 public static class DigitalMediaTypeEnumFactory implements EnumFactory<DigitalMediaType> { 143 public DigitalMediaType fromCode(String codeString) throws IllegalArgumentException { 144 if (codeString == null || "".equals(codeString)) 145 if (codeString == null || "".equals(codeString)) 146 return null; 147 if ("photo".equals(codeString)) 148 return DigitalMediaType.PHOTO; 149 if ("video".equals(codeString)) 150 return DigitalMediaType.VIDEO; 151 if ("audio".equals(codeString)) 152 return DigitalMediaType.AUDIO; 153 throw new IllegalArgumentException("Unknown DigitalMediaType code '" + codeString + "'"); 154 } 155 156 public Enumeration<DigitalMediaType> fromType(Base code) throws FHIRException { 157 if (code == null || code.isEmpty()) 158 return null; 159 String codeString = ((PrimitiveType) code).asStringValue(); 160 if (codeString == null || "".equals(codeString)) 161 return null; 162 if ("photo".equals(codeString)) 163 return new Enumeration<DigitalMediaType>(this, DigitalMediaType.PHOTO); 164 if ("video".equals(codeString)) 165 return new Enumeration<DigitalMediaType>(this, DigitalMediaType.VIDEO); 166 if ("audio".equals(codeString)) 167 return new Enumeration<DigitalMediaType>(this, DigitalMediaType.AUDIO); 168 throw new FHIRException("Unknown DigitalMediaType code '" + codeString + "'"); 169 } 170 171 public String toCode(DigitalMediaType code) 172 { 173 if (code == DigitalMediaType.NULL) 174 return null; 175 if (code == DigitalMediaType.PHOTO) 176 return "photo"; 177 if (code == DigitalMediaType.VIDEO) 178 return "video"; 179 if (code == DigitalMediaType.AUDIO) 180 return "audio"; 181 return "?"; 182 } 183 } 184 185 /** 186 * Whether the media is a photo (still image), an audio recording, or a video 187 * recording. 188 */ 189 @Child(name = "type", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 190 @Description(shortDefinition = "photo | video | audio", formalDefinition = "Whether the media is a photo (still image), an audio recording, or a video recording.") 191 protected Enumeration<DigitalMediaType> type; 192 193 /** 194 * Details of the type of the media - usually, how it was acquired (what type of 195 * device). If images sourced from a DICOM system, are wrapped in a Media 196 * resource, then this is the modality. 197 */ 198 @Child(name = "subtype", type = { 199 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 200 @Description(shortDefinition = "The type of acquisition equipment/process", formalDefinition = "Details of the type of the media - usually, how it was acquired (what type of device). If images sourced from a DICOM system, are wrapped in a Media resource, then this is the modality.") 201 protected CodeableConcept subtype; 202 203 /** 204 * Identifiers associated with the image - these may include identifiers for the 205 * image itself, identifiers for the context of its collection (e.g. series ids) 206 * and context ids such as accession numbers or other workflow identifiers. 207 */ 208 @Child(name = "identifier", type = { 209 Identifier.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 210 @Description(shortDefinition = "Identifier(s) for the image", formalDefinition = "Identifiers associated with the image - these may include identifiers for the image itself, identifiers for the context of its collection (e.g. series ids) and context ids such as accession numbers or other workflow identifiers.") 211 protected List<Identifier> identifier; 212 213 /** 214 * Who/What this Media is a record of. 215 */ 216 @Child(name = "subject", type = { Patient.class, Practitioner.class, Group.class, Device.class, 217 Specimen.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 218 @Description(shortDefinition = "Who/What this Media is a record of", formalDefinition = "Who/What this Media is a record of.") 219 protected Reference subject; 220 221 /** 222 * The actual object that is the target of the reference (Who/What this Media is 223 * a record of.) 224 */ 225 protected Resource subjectTarget; 226 227 /** 228 * The person who administered the collection of the image. 229 */ 230 @Child(name = "operator", type = { 231 Practitioner.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 232 @Description(shortDefinition = "The person who generated the image", formalDefinition = "The person who administered the collection of the image.") 233 protected Reference operator; 234 235 /** 236 * The actual object that is the target of the reference (The person who 237 * administered the collection of the image.) 238 */ 239 protected Practitioner operatorTarget; 240 241 /** 242 * The name of the imaging view e.g. Lateral or Antero-posterior (AP). 243 */ 244 @Child(name = "view", type = { CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 245 @Description(shortDefinition = "Imaging view, e.g. Lateral or Antero-posterior", formalDefinition = "The name of the imaging view e.g. Lateral or Antero-posterior (AP).") 246 protected CodeableConcept view; 247 248 /** 249 * The name of the device / manufacturer of the device that was used to make the 250 * recording. 251 */ 252 @Child(name = "deviceName", type = { 253 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 254 @Description(shortDefinition = "Name of the device/manufacturer", formalDefinition = "The name of the device / manufacturer of the device that was used to make the recording.") 255 protected StringType deviceName; 256 257 /** 258 * Height of the image in pixels (photo/video). 259 */ 260 @Child(name = "height", type = { 261 PositiveIntType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 262 @Description(shortDefinition = "Height of the image in pixels (photo/video)", formalDefinition = "Height of the image in pixels (photo/video).") 263 protected PositiveIntType height; 264 265 /** 266 * Width of the image in pixels (photo/video). 267 */ 268 @Child(name = "width", type = { 269 PositiveIntType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 270 @Description(shortDefinition = "Width of the image in pixels (photo/video)", formalDefinition = "Width of the image in pixels (photo/video).") 271 protected PositiveIntType width; 272 273 /** 274 * The number of frames in a photo. This is used with a multi-page fax, or an 275 * imaging acquisition context that takes multiple slices in a single image, or 276 * an animated gif. If there is more than one frame, this SHALL have a value in 277 * order to alert interface software that a multi-frame capable rendering widget 278 * is required. 279 */ 280 @Child(name = "frames", type = { 281 PositiveIntType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 282 @Description(shortDefinition = "Number of frames if > 1 (photo)", formalDefinition = "The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required.") 283 protected PositiveIntType frames; 284 285 /** 286 * The duration of the recording in seconds - for audio and video. 287 */ 288 @Child(name = "duration", type = { 289 UnsignedIntType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 290 @Description(shortDefinition = "Length in seconds (audio / video)", formalDefinition = "The duration of the recording in seconds - for audio and video.") 291 protected UnsignedIntType duration; 292 293 /** 294 * The actual content of the media - inline or by direct reference to the media 295 * source file. 296 */ 297 @Child(name = "content", type = { Attachment.class }, order = 11, min = 1, max = 1, modifier = false, summary = false) 298 @Description(shortDefinition = "Actual Media - reference or data", formalDefinition = "The actual content of the media - inline or by direct reference to the media source file.") 299 protected Attachment content; 300 301 private static final long serialVersionUID = -280764739L; 302 303 /* 304 * Constructor 305 */ 306 public Media() { 307 super(); 308 } 309 310 /* 311 * Constructor 312 */ 313 public Media(Enumeration<DigitalMediaType> type, Attachment content) { 314 super(); 315 this.type = type; 316 this.content = content; 317 } 318 319 /** 320 * @return {@link #type} (Whether the media is a photo (still image), an audio 321 * recording, or a video recording.). This is the underlying object with 322 * id, value and extensions. The accessor "getType" gives direct access 323 * to the value 324 */ 325 public Enumeration<DigitalMediaType> getTypeElement() { 326 if (this.type == null) 327 if (Configuration.errorOnAutoCreate()) 328 throw new Error("Attempt to auto-create Media.type"); 329 else if (Configuration.doAutoCreate()) 330 this.type = new Enumeration<DigitalMediaType>(new DigitalMediaTypeEnumFactory()); // bb 331 return this.type; 332 } 333 334 public boolean hasTypeElement() { 335 return this.type != null && !this.type.isEmpty(); 336 } 337 338 public boolean hasType() { 339 return this.type != null && !this.type.isEmpty(); 340 } 341 342 /** 343 * @param value {@link #type} (Whether the media is a photo (still image), an 344 * audio recording, or a video recording.). This is the underlying 345 * object with id, value and extensions. The accessor "getType" 346 * gives direct access to the value 347 */ 348 public Media setTypeElement(Enumeration<DigitalMediaType> value) { 349 this.type = value; 350 return this; 351 } 352 353 /** 354 * @return Whether the media is a photo (still image), an audio recording, or a 355 * video recording. 356 */ 357 public DigitalMediaType getType() { 358 return this.type == null ? null : this.type.getValue(); 359 } 360 361 /** 362 * @param value Whether the media is a photo (still image), an audio recording, 363 * or a video recording. 364 */ 365 public Media setType(DigitalMediaType value) { 366 if (this.type == null) 367 this.type = new Enumeration<DigitalMediaType>(new DigitalMediaTypeEnumFactory()); 368 this.type.setValue(value); 369 return this; 370 } 371 372 /** 373 * @return {@link #subtype} (Details of the type of the media - usually, how it 374 * was acquired (what type of device). If images sourced from a DICOM 375 * system, are wrapped in a Media resource, then this is the modality.) 376 */ 377 public CodeableConcept getSubtype() { 378 if (this.subtype == null) 379 if (Configuration.errorOnAutoCreate()) 380 throw new Error("Attempt to auto-create Media.subtype"); 381 else if (Configuration.doAutoCreate()) 382 this.subtype = new CodeableConcept(); // cc 383 return this.subtype; 384 } 385 386 public boolean hasSubtype() { 387 return this.subtype != null && !this.subtype.isEmpty(); 388 } 389 390 /** 391 * @param value {@link #subtype} (Details of the type of the media - usually, 392 * how it was acquired (what type of device). If images sourced 393 * from a DICOM system, are wrapped in a Media resource, then this 394 * is the modality.) 395 */ 396 public Media setSubtype(CodeableConcept value) { 397 this.subtype = value; 398 return this; 399 } 400 401 /** 402 * @return {@link #identifier} (Identifiers associated with the image - these 403 * may include identifiers for the image itself, identifiers for the 404 * context of its collection (e.g. series ids) and context ids such as 405 * accession numbers or other workflow identifiers.) 406 */ 407 public List<Identifier> getIdentifier() { 408 if (this.identifier == null) 409 this.identifier = new ArrayList<Identifier>(); 410 return this.identifier; 411 } 412 413 public boolean hasIdentifier() { 414 if (this.identifier == null) 415 return false; 416 for (Identifier item : this.identifier) 417 if (!item.isEmpty()) 418 return true; 419 return false; 420 } 421 422 /** 423 * @return {@link #identifier} (Identifiers associated with the image - these 424 * may include identifiers for the image itself, identifiers for the 425 * context of its collection (e.g. series ids) and context ids such as 426 * accession numbers or other workflow identifiers.) 427 */ 428 // syntactic sugar 429 public Identifier addIdentifier() { // 3 430 Identifier t = new Identifier(); 431 if (this.identifier == null) 432 this.identifier = new ArrayList<Identifier>(); 433 this.identifier.add(t); 434 return t; 435 } 436 437 // syntactic sugar 438 public Media addIdentifier(Identifier t) { // 3 439 if (t == null) 440 return this; 441 if (this.identifier == null) 442 this.identifier = new ArrayList<Identifier>(); 443 this.identifier.add(t); 444 return this; 445 } 446 447 /** 448 * @return {@link #subject} (Who/What this Media is a record of.) 449 */ 450 public Reference getSubject() { 451 if (this.subject == null) 452 if (Configuration.errorOnAutoCreate()) 453 throw new Error("Attempt to auto-create Media.subject"); 454 else if (Configuration.doAutoCreate()) 455 this.subject = new Reference(); // cc 456 return this.subject; 457 } 458 459 public boolean hasSubject() { 460 return this.subject != null && !this.subject.isEmpty(); 461 } 462 463 /** 464 * @param value {@link #subject} (Who/What this Media is a record of.) 465 */ 466 public Media setSubject(Reference value) { 467 this.subject = value; 468 return this; 469 } 470 471 /** 472 * @return {@link #subject} The actual object that is the target of the 473 * reference. The reference library doesn't populate this, but you can 474 * use it to hold the resource if you resolve it. (Who/What this Media 475 * is a record of.) 476 */ 477 public Resource getSubjectTarget() { 478 return this.subjectTarget; 479 } 480 481 /** 482 * @param value {@link #subject} The actual object that is the target of the 483 * reference. The reference library doesn't use these, but you can 484 * use it to hold the resource if you resolve it. (Who/What this 485 * Media is a record of.) 486 */ 487 public Media setSubjectTarget(Resource value) { 488 this.subjectTarget = value; 489 return this; 490 } 491 492 /** 493 * @return {@link #operator} (The person who administered the collection of the 494 * image.) 495 */ 496 public Reference getOperator() { 497 if (this.operator == null) 498 if (Configuration.errorOnAutoCreate()) 499 throw new Error("Attempt to auto-create Media.operator"); 500 else if (Configuration.doAutoCreate()) 501 this.operator = new Reference(); // cc 502 return this.operator; 503 } 504 505 public boolean hasOperator() { 506 return this.operator != null && !this.operator.isEmpty(); 507 } 508 509 /** 510 * @param value {@link #operator} (The person who administered the collection of 511 * the image.) 512 */ 513 public Media setOperator(Reference value) { 514 this.operator = value; 515 return this; 516 } 517 518 /** 519 * @return {@link #operator} The actual object that is the target of the 520 * reference. The reference library doesn't populate this, but you can 521 * use it to hold the resource if you resolve it. (The person who 522 * administered the collection of the image.) 523 */ 524 public Practitioner getOperatorTarget() { 525 if (this.operatorTarget == null) 526 if (Configuration.errorOnAutoCreate()) 527 throw new Error("Attempt to auto-create Media.operator"); 528 else if (Configuration.doAutoCreate()) 529 this.operatorTarget = new Practitioner(); // aa 530 return this.operatorTarget; 531 } 532 533 /** 534 * @param value {@link #operator} The actual object that is the target of the 535 * reference. The reference library doesn't use these, but you can 536 * use it to hold the resource if you resolve it. (The person who 537 * administered the collection of the image.) 538 */ 539 public Media setOperatorTarget(Practitioner value) { 540 this.operatorTarget = value; 541 return this; 542 } 543 544 /** 545 * @return {@link #view} (The name of the imaging view e.g. Lateral or 546 * Antero-posterior (AP).) 547 */ 548 public CodeableConcept getView() { 549 if (this.view == null) 550 if (Configuration.errorOnAutoCreate()) 551 throw new Error("Attempt to auto-create Media.view"); 552 else if (Configuration.doAutoCreate()) 553 this.view = new CodeableConcept(); // cc 554 return this.view; 555 } 556 557 public boolean hasView() { 558 return this.view != null && !this.view.isEmpty(); 559 } 560 561 /** 562 * @param value {@link #view} (The name of the imaging view e.g. Lateral or 563 * Antero-posterior (AP).) 564 */ 565 public Media setView(CodeableConcept value) { 566 this.view = value; 567 return this; 568 } 569 570 /** 571 * @return {@link #deviceName} (The name of the device / manufacturer of the 572 * device that was used to make the recording.). This is the underlying 573 * object with id, value and extensions. The accessor "getDeviceName" 574 * gives direct access to the value 575 */ 576 public StringType getDeviceNameElement() { 577 if (this.deviceName == null) 578 if (Configuration.errorOnAutoCreate()) 579 throw new Error("Attempt to auto-create Media.deviceName"); 580 else if (Configuration.doAutoCreate()) 581 this.deviceName = new StringType(); // bb 582 return this.deviceName; 583 } 584 585 public boolean hasDeviceNameElement() { 586 return this.deviceName != null && !this.deviceName.isEmpty(); 587 } 588 589 public boolean hasDeviceName() { 590 return this.deviceName != null && !this.deviceName.isEmpty(); 591 } 592 593 /** 594 * @param value {@link #deviceName} (The name of the device / manufacturer of 595 * the device that was used to make the recording.). This is the 596 * underlying object with id, value and extensions. The accessor 597 * "getDeviceName" gives direct access to the value 598 */ 599 public Media setDeviceNameElement(StringType value) { 600 this.deviceName = value; 601 return this; 602 } 603 604 /** 605 * @return The name of the device / manufacturer of the device that was used to 606 * make the recording. 607 */ 608 public String getDeviceName() { 609 return this.deviceName == null ? null : this.deviceName.getValue(); 610 } 611 612 /** 613 * @param value The name of the device / manufacturer of the device that was 614 * used to make the recording. 615 */ 616 public Media setDeviceName(String value) { 617 if (Utilities.noString(value)) 618 this.deviceName = null; 619 else { 620 if (this.deviceName == null) 621 this.deviceName = new StringType(); 622 this.deviceName.setValue(value); 623 } 624 return this; 625 } 626 627 /** 628 * @return {@link #height} (Height of the image in pixels (photo/video).). This 629 * is the underlying object with id, value and extensions. The accessor 630 * "getHeight" gives direct access to the value 631 */ 632 public PositiveIntType getHeightElement() { 633 if (this.height == null) 634 if (Configuration.errorOnAutoCreate()) 635 throw new Error("Attempt to auto-create Media.height"); 636 else if (Configuration.doAutoCreate()) 637 this.height = new PositiveIntType(); // bb 638 return this.height; 639 } 640 641 public boolean hasHeightElement() { 642 return this.height != null && !this.height.isEmpty(); 643 } 644 645 public boolean hasHeight() { 646 return this.height != null && !this.height.isEmpty(); 647 } 648 649 /** 650 * @param value {@link #height} (Height of the image in pixels (photo/video).). 651 * This is the underlying object with id, value and extensions. The 652 * accessor "getHeight" gives direct access to the value 653 */ 654 public Media setHeightElement(PositiveIntType value) { 655 this.height = value; 656 return this; 657 } 658 659 /** 660 * @return Height of the image in pixels (photo/video). 661 */ 662 public int getHeight() { 663 return this.height == null || this.height.isEmpty() ? 0 : this.height.getValue(); 664 } 665 666 /** 667 * @param value Height of the image in pixels (photo/video). 668 */ 669 public Media setHeight(int value) { 670 if (this.height == null) 671 this.height = new PositiveIntType(); 672 this.height.setValue(value); 673 return this; 674 } 675 676 /** 677 * @return {@link #width} (Width of the image in pixels (photo/video).). This is 678 * the underlying object with id, value and extensions. The accessor 679 * "getWidth" gives direct access to the value 680 */ 681 public PositiveIntType getWidthElement() { 682 if (this.width == null) 683 if (Configuration.errorOnAutoCreate()) 684 throw new Error("Attempt to auto-create Media.width"); 685 else if (Configuration.doAutoCreate()) 686 this.width = new PositiveIntType(); // bb 687 return this.width; 688 } 689 690 public boolean hasWidthElement() { 691 return this.width != null && !this.width.isEmpty(); 692 } 693 694 public boolean hasWidth() { 695 return this.width != null && !this.width.isEmpty(); 696 } 697 698 /** 699 * @param value {@link #width} (Width of the image in pixels (photo/video).). 700 * This is the underlying object with id, value and extensions. The 701 * accessor "getWidth" gives direct access to the value 702 */ 703 public Media setWidthElement(PositiveIntType value) { 704 this.width = value; 705 return this; 706 } 707 708 /** 709 * @return Width of the image in pixels (photo/video). 710 */ 711 public int getWidth() { 712 return this.width == null || this.width.isEmpty() ? 0 : this.width.getValue(); 713 } 714 715 /** 716 * @param value Width of the image in pixels (photo/video). 717 */ 718 public Media setWidth(int value) { 719 if (this.width == null) 720 this.width = new PositiveIntType(); 721 this.width.setValue(value); 722 return this; 723 } 724 725 /** 726 * @return {@link #frames} (The number of frames in a photo. This is used with a 727 * multi-page fax, or an imaging acquisition context that takes multiple 728 * slices in a single image, or an animated gif. If there is more than 729 * one frame, this SHALL have a value in order to alert interface 730 * software that a multi-frame capable rendering widget is required.). 731 * This is the underlying object with id, value and extensions. The 732 * accessor "getFrames" gives direct access to the value 733 */ 734 public PositiveIntType getFramesElement() { 735 if (this.frames == null) 736 if (Configuration.errorOnAutoCreate()) 737 throw new Error("Attempt to auto-create Media.frames"); 738 else if (Configuration.doAutoCreate()) 739 this.frames = new PositiveIntType(); // bb 740 return this.frames; 741 } 742 743 public boolean hasFramesElement() { 744 return this.frames != null && !this.frames.isEmpty(); 745 } 746 747 public boolean hasFrames() { 748 return this.frames != null && !this.frames.isEmpty(); 749 } 750 751 /** 752 * @param value {@link #frames} (The number of frames in a photo. This is used 753 * with a multi-page fax, or an imaging acquisition context that 754 * takes multiple slices in a single image, or an animated gif. If 755 * there is more than one frame, this SHALL have a value in order 756 * to alert interface software that a multi-frame capable rendering 757 * widget is required.). This is the underlying object with id, 758 * value and extensions. The accessor "getFrames" gives direct 759 * access to the value 760 */ 761 public Media setFramesElement(PositiveIntType value) { 762 this.frames = value; 763 return this; 764 } 765 766 /** 767 * @return The number of frames in a photo. This is used with a multi-page fax, 768 * or an imaging acquisition context that takes multiple slices in a 769 * single image, or an animated gif. If there is more than one frame, 770 * this SHALL have a value in order to alert interface software that a 771 * multi-frame capable rendering widget is required. 772 */ 773 public int getFrames() { 774 return this.frames == null || this.frames.isEmpty() ? 0 : this.frames.getValue(); 775 } 776 777 /** 778 * @param value The number of frames in a photo. This is used with a multi-page 779 * fax, or an imaging acquisition context that takes multiple 780 * slices in a single image, or an animated gif. If there is more 781 * than one frame, this SHALL have a value in order to alert 782 * interface software that a multi-frame capable rendering widget 783 * is required. 784 */ 785 public Media setFrames(int value) { 786 if (this.frames == null) 787 this.frames = new PositiveIntType(); 788 this.frames.setValue(value); 789 return this; 790 } 791 792 /** 793 * @return {@link #duration} (The duration of the recording in seconds - for 794 * audio and video.). This is the underlying object with id, value and 795 * extensions. The accessor "getDuration" gives direct access to the 796 * value 797 */ 798 public UnsignedIntType getDurationElement() { 799 if (this.duration == null) 800 if (Configuration.errorOnAutoCreate()) 801 throw new Error("Attempt to auto-create Media.duration"); 802 else if (Configuration.doAutoCreate()) 803 this.duration = new UnsignedIntType(); // bb 804 return this.duration; 805 } 806 807 public boolean hasDurationElement() { 808 return this.duration != null && !this.duration.isEmpty(); 809 } 810 811 public boolean hasDuration() { 812 return this.duration != null && !this.duration.isEmpty(); 813 } 814 815 /** 816 * @param value {@link #duration} (The duration of the recording in seconds - 817 * for audio and video.). This is the underlying object with id, 818 * value and extensions. The accessor "getDuration" gives direct 819 * access to the value 820 */ 821 public Media setDurationElement(UnsignedIntType value) { 822 this.duration = value; 823 return this; 824 } 825 826 /** 827 * @return The duration of the recording in seconds - for audio and video. 828 */ 829 public int getDuration() { 830 return this.duration == null || this.duration.isEmpty() ? 0 : this.duration.getValue(); 831 } 832 833 /** 834 * @param value The duration of the recording in seconds - for audio and video. 835 */ 836 public Media setDuration(int value) { 837 if (this.duration == null) 838 this.duration = new UnsignedIntType(); 839 this.duration.setValue(value); 840 return this; 841 } 842 843 /** 844 * @return {@link #content} (The actual content of the media - inline or by 845 * direct reference to the media source file.) 846 */ 847 public Attachment getContent() { 848 if (this.content == null) 849 if (Configuration.errorOnAutoCreate()) 850 throw new Error("Attempt to auto-create Media.content"); 851 else if (Configuration.doAutoCreate()) 852 this.content = new Attachment(); // cc 853 return this.content; 854 } 855 856 public boolean hasContent() { 857 return this.content != null && !this.content.isEmpty(); 858 } 859 860 /** 861 * @param value {@link #content} (The actual content of the media - inline or by 862 * direct reference to the media source file.) 863 */ 864 public Media setContent(Attachment value) { 865 this.content = value; 866 return this; 867 } 868 869 protected void listChildren(List<Property> childrenList) { 870 super.listChildren(childrenList); 871 childrenList.add(new Property("type", "code", 872 "Whether the media is a photo (still image), an audio recording, or a video recording.", 0, 873 java.lang.Integer.MAX_VALUE, type)); 874 childrenList.add(new Property("subtype", "CodeableConcept", 875 "Details of the type of the media - usually, how it was acquired (what type of device). If images sourced from a DICOM system, are wrapped in a Media resource, then this is the modality.", 876 0, java.lang.Integer.MAX_VALUE, subtype)); 877 childrenList.add(new Property("identifier", "Identifier", 878 "Identifiers associated with the image - these may include identifiers for the image itself, identifiers for the context of its collection (e.g. series ids) and context ids such as accession numbers or other workflow identifiers.", 879 0, java.lang.Integer.MAX_VALUE, identifier)); 880 childrenList.add(new Property("subject", "Reference(Patient|Practitioner|Group|Device|Specimen)", 881 "Who/What this Media is a record of.", 0, java.lang.Integer.MAX_VALUE, subject)); 882 childrenList.add(new Property("operator", "Reference(Practitioner)", 883 "The person who administered the collection of the image.", 0, java.lang.Integer.MAX_VALUE, operator)); 884 childrenList.add(new Property("view", "CodeableConcept", 885 "The name of the imaging view e.g. Lateral or Antero-posterior (AP).", 0, java.lang.Integer.MAX_VALUE, view)); 886 childrenList.add(new Property("deviceName", "string", 887 "The name of the device / manufacturer of the device that was used to make the recording.", 0, 888 java.lang.Integer.MAX_VALUE, deviceName)); 889 childrenList.add(new Property("height", "positiveInt", "Height of the image in pixels (photo/video).", 0, 890 java.lang.Integer.MAX_VALUE, height)); 891 childrenList.add(new Property("width", "positiveInt", "Width of the image in pixels (photo/video).", 0, 892 java.lang.Integer.MAX_VALUE, width)); 893 childrenList.add(new Property("frames", "positiveInt", 894 "The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required.", 895 0, java.lang.Integer.MAX_VALUE, frames)); 896 childrenList.add(new Property("duration", "unsignedInt", 897 "The duration of the recording in seconds - for audio and video.", 0, java.lang.Integer.MAX_VALUE, duration)); 898 childrenList.add(new Property("content", "Attachment", 899 "The actual content of the media - inline or by direct reference to the media source file.", 0, 900 java.lang.Integer.MAX_VALUE, content)); 901 } 902 903 @Override 904 public void setProperty(String name, Base value) throws FHIRException { 905 if (name.equals("type")) 906 this.type = new DigitalMediaTypeEnumFactory().fromType(value); // Enumeration<DigitalMediaType> 907 else if (name.equals("subtype")) 908 this.subtype = castToCodeableConcept(value); // CodeableConcept 909 else if (name.equals("identifier")) 910 this.getIdentifier().add(castToIdentifier(value)); 911 else if (name.equals("subject")) 912 this.subject = castToReference(value); // Reference 913 else if (name.equals("operator")) 914 this.operator = castToReference(value); // Reference 915 else if (name.equals("view")) 916 this.view = castToCodeableConcept(value); // CodeableConcept 917 else if (name.equals("deviceName")) 918 this.deviceName = castToString(value); // StringType 919 else if (name.equals("height")) 920 this.height = castToPositiveInt(value); // PositiveIntType 921 else if (name.equals("width")) 922 this.width = castToPositiveInt(value); // PositiveIntType 923 else if (name.equals("frames")) 924 this.frames = castToPositiveInt(value); // PositiveIntType 925 else if (name.equals("duration")) 926 this.duration = castToUnsignedInt(value); // UnsignedIntType 927 else if (name.equals("content")) 928 this.content = castToAttachment(value); // Attachment 929 else 930 super.setProperty(name, value); 931 } 932 933 @Override 934 public Base addChild(String name) throws FHIRException { 935 if (name.equals("type")) { 936 throw new FHIRException("Cannot call addChild on a singleton property Media.type"); 937 } else if (name.equals("subtype")) { 938 this.subtype = new CodeableConcept(); 939 return this.subtype; 940 } else if (name.equals("identifier")) { 941 return addIdentifier(); 942 } else if (name.equals("subject")) { 943 this.subject = new Reference(); 944 return this.subject; 945 } else if (name.equals("operator")) { 946 this.operator = new Reference(); 947 return this.operator; 948 } else if (name.equals("view")) { 949 this.view = new CodeableConcept(); 950 return this.view; 951 } else if (name.equals("deviceName")) { 952 throw new FHIRException("Cannot call addChild on a singleton property Media.deviceName"); 953 } else if (name.equals("height")) { 954 throw new FHIRException("Cannot call addChild on a singleton property Media.height"); 955 } else if (name.equals("width")) { 956 throw new FHIRException("Cannot call addChild on a singleton property Media.width"); 957 } else if (name.equals("frames")) { 958 throw new FHIRException("Cannot call addChild on a singleton property Media.frames"); 959 } else if (name.equals("duration")) { 960 throw new FHIRException("Cannot call addChild on a singleton property Media.duration"); 961 } else if (name.equals("content")) { 962 this.content = new Attachment(); 963 return this.content; 964 } else 965 return super.addChild(name); 966 } 967 968 public String fhirType() { 969 return "Media"; 970 971 } 972 973 public Media copy() { 974 Media dst = new Media(); 975 copyValues(dst); 976 dst.type = type == null ? null : type.copy(); 977 dst.subtype = subtype == null ? null : subtype.copy(); 978 if (identifier != null) { 979 dst.identifier = new ArrayList<Identifier>(); 980 for (Identifier i : identifier) 981 dst.identifier.add(i.copy()); 982 } 983 ; 984 dst.subject = subject == null ? null : subject.copy(); 985 dst.operator = operator == null ? null : operator.copy(); 986 dst.view = view == null ? null : view.copy(); 987 dst.deviceName = deviceName == null ? null : deviceName.copy(); 988 dst.height = height == null ? null : height.copy(); 989 dst.width = width == null ? null : width.copy(); 990 dst.frames = frames == null ? null : frames.copy(); 991 dst.duration = duration == null ? null : duration.copy(); 992 dst.content = content == null ? null : content.copy(); 993 return dst; 994 } 995 996 protected Media typedCopy() { 997 return copy(); 998 } 999 1000 @Override 1001 public boolean equalsDeep(Base other) { 1002 if (!super.equalsDeep(other)) 1003 return false; 1004 if (!(other instanceof Media)) 1005 return false; 1006 Media o = (Media) other; 1007 return compareDeep(type, o.type, true) && compareDeep(subtype, o.subtype, true) 1008 && compareDeep(identifier, o.identifier, true) && compareDeep(subject, o.subject, true) 1009 && compareDeep(operator, o.operator, true) && compareDeep(view, o.view, true) 1010 && compareDeep(deviceName, o.deviceName, true) && compareDeep(height, o.height, true) 1011 && compareDeep(width, o.width, true) && compareDeep(frames, o.frames, true) 1012 && compareDeep(duration, o.duration, true) && compareDeep(content, o.content, true); 1013 } 1014 1015 @Override 1016 public boolean equalsShallow(Base other) { 1017 if (!super.equalsShallow(other)) 1018 return false; 1019 if (!(other instanceof Media)) 1020 return false; 1021 Media o = (Media) other; 1022 return compareValues(type, o.type, true) && compareValues(deviceName, o.deviceName, true) 1023 && compareValues(height, o.height, true) && compareValues(width, o.width, true) 1024 && compareValues(frames, o.frames, true) && compareValues(duration, o.duration, true); 1025 } 1026 1027 public boolean isEmpty() { 1028 return super.isEmpty() && (type == null || type.isEmpty()) && (subtype == null || subtype.isEmpty()) 1029 && (identifier == null || identifier.isEmpty()) && (subject == null || subject.isEmpty()) 1030 && (operator == null || operator.isEmpty()) && (view == null || view.isEmpty()) 1031 && (deviceName == null || deviceName.isEmpty()) && (height == null || height.isEmpty()) 1032 && (width == null || width.isEmpty()) && (frames == null || frames.isEmpty()) 1033 && (duration == null || duration.isEmpty()) && (content == null || content.isEmpty()); 1034 } 1035 1036 @Override 1037 public ResourceType getResourceType() { 1038 return ResourceType.Media; 1039 } 1040 1041 @SearchParamDefinition(name = "identifier", path = "Media.identifier", description = "Identifier(s) for the image", type = "token") 1042 public static final String SP_IDENTIFIER = "identifier"; 1043 @SearchParamDefinition(name = "view", path = "Media.view", description = "Imaging view, e.g. Lateral or Antero-posterior", type = "token") 1044 public static final String SP_VIEW = "view"; 1045 @SearchParamDefinition(name = "subtype", path = "Media.subtype", description = "The type of acquisition equipment/process", type = "token") 1046 public static final String SP_SUBTYPE = "subtype"; 1047 @SearchParamDefinition(name = "created", path = "Media.content.creation", description = "Date attachment was first created", type = "date") 1048 public static final String SP_CREATED = "created"; 1049 @SearchParamDefinition(name = "subject", path = "Media.subject", description = "Who/What this Media is a record of", type = "reference") 1050 public static final String SP_SUBJECT = "subject"; 1051 @SearchParamDefinition(name = "patient", path = "Media.subject", description = "Who/What this Media is a record of", type = "reference") 1052 public static final String SP_PATIENT = "patient"; 1053 @SearchParamDefinition(name = "type", path = "Media.type", description = "photo | video | audio", type = "token") 1054 public static final String SP_TYPE = "type"; 1055 @SearchParamDefinition(name = "operator", path = "Media.operator", description = "The person who generated the image", type = "reference") 1056 public static final String SP_OPERATOR = "operator"; 1057 1058}