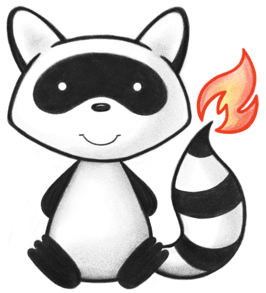
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * This resource is primarily used for the identification and definition of a 048 * medication. It covers the ingredients and the packaging for a medication. 049 */ 050@ResourceDef(name = "Medication", profile = "http://hl7.org/fhir/Profile/Medication") 051public class Medication extends DomainResource { 052 053 @Block() 054 public static class MedicationProductComponent extends BackboneElement implements IBaseBackboneElement { 055 /** 056 * Describes the form of the item. Powder; tablets; carton. 057 */ 058 @Child(name = "form", type = { 059 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 060 @Description(shortDefinition = "powder | tablets | carton +", formalDefinition = "Describes the form of the item. Powder; tablets; carton.") 061 protected CodeableConcept form; 062 063 /** 064 * Identifies a particular constituent of interest in the product. 065 */ 066 @Child(name = "ingredient", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 067 @Description(shortDefinition = "Active or inactive ingredient", formalDefinition = "Identifies a particular constituent of interest in the product.") 068 protected List<MedicationProductIngredientComponent> ingredient; 069 070 /** 071 * Information about a group of medication produced or packaged from one 072 * production run. 073 */ 074 @Child(name = "batch", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 075 @Description(shortDefinition = "", formalDefinition = "Information about a group of medication produced or packaged from one production run.") 076 protected List<MedicationProductBatchComponent> batch; 077 078 private static final long serialVersionUID = 1132853671L; 079 080 /* 081 * Constructor 082 */ 083 public MedicationProductComponent() { 084 super(); 085 } 086 087 /** 088 * @return {@link #form} (Describes the form of the item. Powder; tablets; 089 * carton.) 090 */ 091 public CodeableConcept getForm() { 092 if (this.form == null) 093 if (Configuration.errorOnAutoCreate()) 094 throw new Error("Attempt to auto-create MedicationProductComponent.form"); 095 else if (Configuration.doAutoCreate()) 096 this.form = new CodeableConcept(); // cc 097 return this.form; 098 } 099 100 public boolean hasForm() { 101 return this.form != null && !this.form.isEmpty(); 102 } 103 104 /** 105 * @param value {@link #form} (Describes the form of the item. Powder; tablets; 106 * carton.) 107 */ 108 public MedicationProductComponent setForm(CodeableConcept value) { 109 this.form = value; 110 return this; 111 } 112 113 /** 114 * @return {@link #ingredient} (Identifies a particular constituent of interest 115 * in the product.) 116 */ 117 public List<MedicationProductIngredientComponent> getIngredient() { 118 if (this.ingredient == null) 119 this.ingredient = new ArrayList<MedicationProductIngredientComponent>(); 120 return this.ingredient; 121 } 122 123 public boolean hasIngredient() { 124 if (this.ingredient == null) 125 return false; 126 for (MedicationProductIngredientComponent item : this.ingredient) 127 if (!item.isEmpty()) 128 return true; 129 return false; 130 } 131 132 /** 133 * @return {@link #ingredient} (Identifies a particular constituent of interest 134 * in the product.) 135 */ 136 // syntactic sugar 137 public MedicationProductIngredientComponent addIngredient() { // 3 138 MedicationProductIngredientComponent t = new MedicationProductIngredientComponent(); 139 if (this.ingredient == null) 140 this.ingredient = new ArrayList<MedicationProductIngredientComponent>(); 141 this.ingredient.add(t); 142 return t; 143 } 144 145 // syntactic sugar 146 public MedicationProductComponent addIngredient(MedicationProductIngredientComponent t) { // 3 147 if (t == null) 148 return this; 149 if (this.ingredient == null) 150 this.ingredient = new ArrayList<MedicationProductIngredientComponent>(); 151 this.ingredient.add(t); 152 return this; 153 } 154 155 /** 156 * @return {@link #batch} (Information about a group of medication produced or 157 * packaged from one production run.) 158 */ 159 public List<MedicationProductBatchComponent> getBatch() { 160 if (this.batch == null) 161 this.batch = new ArrayList<MedicationProductBatchComponent>(); 162 return this.batch; 163 } 164 165 public boolean hasBatch() { 166 if (this.batch == null) 167 return false; 168 for (MedicationProductBatchComponent item : this.batch) 169 if (!item.isEmpty()) 170 return true; 171 return false; 172 } 173 174 /** 175 * @return {@link #batch} (Information about a group of medication produced or 176 * packaged from one production run.) 177 */ 178 // syntactic sugar 179 public MedicationProductBatchComponent addBatch() { // 3 180 MedicationProductBatchComponent t = new MedicationProductBatchComponent(); 181 if (this.batch == null) 182 this.batch = new ArrayList<MedicationProductBatchComponent>(); 183 this.batch.add(t); 184 return t; 185 } 186 187 // syntactic sugar 188 public MedicationProductComponent addBatch(MedicationProductBatchComponent t) { // 3 189 if (t == null) 190 return this; 191 if (this.batch == null) 192 this.batch = new ArrayList<MedicationProductBatchComponent>(); 193 this.batch.add(t); 194 return this; 195 } 196 197 protected void listChildren(List<Property> childrenList) { 198 super.listChildren(childrenList); 199 childrenList.add(new Property("form", "CodeableConcept", 200 "Describes the form of the item. Powder; tablets; carton.", 0, java.lang.Integer.MAX_VALUE, form)); 201 childrenList.add(new Property("ingredient", "", "Identifies a particular constituent of interest in the product.", 202 0, java.lang.Integer.MAX_VALUE, ingredient)); 203 childrenList.add(new Property("batch", "", 204 "Information about a group of medication produced or packaged from one production run.", 0, 205 java.lang.Integer.MAX_VALUE, batch)); 206 } 207 208 @Override 209 public void setProperty(String name, Base value) throws FHIRException { 210 if (name.equals("form")) 211 this.form = castToCodeableConcept(value); // CodeableConcept 212 else if (name.equals("ingredient")) 213 this.getIngredient().add((MedicationProductIngredientComponent) value); 214 else if (name.equals("batch")) 215 this.getBatch().add((MedicationProductBatchComponent) value); 216 else 217 super.setProperty(name, value); 218 } 219 220 @Override 221 public Base addChild(String name) throws FHIRException { 222 if (name.equals("form")) { 223 this.form = new CodeableConcept(); 224 return this.form; 225 } else if (name.equals("ingredient")) { 226 return addIngredient(); 227 } else if (name.equals("batch")) { 228 return addBatch(); 229 } else 230 return super.addChild(name); 231 } 232 233 public MedicationProductComponent copy() { 234 MedicationProductComponent dst = new MedicationProductComponent(); 235 copyValues(dst); 236 dst.form = form == null ? null : form.copy(); 237 if (ingredient != null) { 238 dst.ingredient = new ArrayList<MedicationProductIngredientComponent>(); 239 for (MedicationProductIngredientComponent i : ingredient) 240 dst.ingredient.add(i.copy()); 241 } 242 ; 243 if (batch != null) { 244 dst.batch = new ArrayList<MedicationProductBatchComponent>(); 245 for (MedicationProductBatchComponent i : batch) 246 dst.batch.add(i.copy()); 247 } 248 ; 249 return dst; 250 } 251 252 @Override 253 public boolean equalsDeep(Base other) { 254 if (!super.equalsDeep(other)) 255 return false; 256 if (!(other instanceof MedicationProductComponent)) 257 return false; 258 MedicationProductComponent o = (MedicationProductComponent) other; 259 return compareDeep(form, o.form, true) && compareDeep(ingredient, o.ingredient, true) 260 && compareDeep(batch, o.batch, true); 261 } 262 263 @Override 264 public boolean equalsShallow(Base other) { 265 if (!super.equalsShallow(other)) 266 return false; 267 if (!(other instanceof MedicationProductComponent)) 268 return false; 269 MedicationProductComponent o = (MedicationProductComponent) other; 270 return true; 271 } 272 273 public boolean isEmpty() { 274 return super.isEmpty() && (form == null || form.isEmpty()) && (ingredient == null || ingredient.isEmpty()) 275 && (batch == null || batch.isEmpty()); 276 } 277 278 public String fhirType() { 279 return "Medication.product"; 280 281 } 282 283 } 284 285 @Block() 286 public static class MedicationProductIngredientComponent extends BackboneElement implements IBaseBackboneElement { 287 /** 288 * The actual ingredient - either a substance (simple ingredient) or another 289 * medication. 290 */ 291 @Child(name = "item", type = { Substance.class, 292 Medication.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 293 @Description(shortDefinition = "The product contained", formalDefinition = "The actual ingredient - either a substance (simple ingredient) or another medication.") 294 protected Reference item; 295 296 /** 297 * The actual object that is the target of the reference (The actual ingredient 298 * - either a substance (simple ingredient) or another medication.) 299 */ 300 protected Resource itemTarget; 301 302 /** 303 * Specifies how many (or how much) of the items there are in this Medication. 304 * For example, 250 mg per tablet. 305 */ 306 @Child(name = "amount", type = { Ratio.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 307 @Description(shortDefinition = "Quantity of ingredient present", formalDefinition = "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet.") 308 protected Ratio amount; 309 310 private static final long serialVersionUID = -1217232889L; 311 312 /* 313 * Constructor 314 */ 315 public MedicationProductIngredientComponent() { 316 super(); 317 } 318 319 /* 320 * Constructor 321 */ 322 public MedicationProductIngredientComponent(Reference item) { 323 super(); 324 this.item = item; 325 } 326 327 /** 328 * @return {@link #item} (The actual ingredient - either a substance (simple 329 * ingredient) or another medication.) 330 */ 331 public Reference getItem() { 332 if (this.item == null) 333 if (Configuration.errorOnAutoCreate()) 334 throw new Error("Attempt to auto-create MedicationProductIngredientComponent.item"); 335 else if (Configuration.doAutoCreate()) 336 this.item = new Reference(); // cc 337 return this.item; 338 } 339 340 public boolean hasItem() { 341 return this.item != null && !this.item.isEmpty(); 342 } 343 344 /** 345 * @param value {@link #item} (The actual ingredient - either a substance 346 * (simple ingredient) or another medication.) 347 */ 348 public MedicationProductIngredientComponent setItem(Reference value) { 349 this.item = value; 350 return this; 351 } 352 353 /** 354 * @return {@link #item} The actual object that is the target of the reference. 355 * The reference library doesn't populate this, but you can use it to 356 * hold the resource if you resolve it. (The actual ingredient - either 357 * a substance (simple ingredient) or another medication.) 358 */ 359 public Resource getItemTarget() { 360 return this.itemTarget; 361 } 362 363 /** 364 * @param value {@link #item} The actual object that is the target of the 365 * reference. The reference library doesn't use these, but you can 366 * use it to hold the resource if you resolve it. (The actual 367 * ingredient - either a substance (simple ingredient) or another 368 * medication.) 369 */ 370 public MedicationProductIngredientComponent setItemTarget(Resource value) { 371 this.itemTarget = value; 372 return this; 373 } 374 375 /** 376 * @return {@link #amount} (Specifies how many (or how much) of the items there 377 * are in this Medication. For example, 250 mg per tablet.) 378 */ 379 public Ratio getAmount() { 380 if (this.amount == null) 381 if (Configuration.errorOnAutoCreate()) 382 throw new Error("Attempt to auto-create MedicationProductIngredientComponent.amount"); 383 else if (Configuration.doAutoCreate()) 384 this.amount = new Ratio(); // cc 385 return this.amount; 386 } 387 388 public boolean hasAmount() { 389 return this.amount != null && !this.amount.isEmpty(); 390 } 391 392 /** 393 * @param value {@link #amount} (Specifies how many (or how much) of the items 394 * there are in this Medication. For example, 250 mg per tablet.) 395 */ 396 public MedicationProductIngredientComponent setAmount(Ratio value) { 397 this.amount = value; 398 return this; 399 } 400 401 protected void listChildren(List<Property> childrenList) { 402 super.listChildren(childrenList); 403 childrenList.add(new Property("item", "Reference(Substance|Medication)", 404 "The actual ingredient - either a substance (simple ingredient) or another medication.", 0, 405 java.lang.Integer.MAX_VALUE, item)); 406 childrenList.add(new Property("amount", "Ratio", 407 "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet.", 408 0, java.lang.Integer.MAX_VALUE, amount)); 409 } 410 411 @Override 412 public void setProperty(String name, Base value) throws FHIRException { 413 if (name.equals("item")) 414 this.item = castToReference(value); // Reference 415 else if (name.equals("amount")) 416 this.amount = castToRatio(value); // Ratio 417 else 418 super.setProperty(name, value); 419 } 420 421 @Override 422 public Base addChild(String name) throws FHIRException { 423 if (name.equals("item")) { 424 this.item = new Reference(); 425 return this.item; 426 } else if (name.equals("amount")) { 427 this.amount = new Ratio(); 428 return this.amount; 429 } else 430 return super.addChild(name); 431 } 432 433 public MedicationProductIngredientComponent copy() { 434 MedicationProductIngredientComponent dst = new MedicationProductIngredientComponent(); 435 copyValues(dst); 436 dst.item = item == null ? null : item.copy(); 437 dst.amount = amount == null ? null : amount.copy(); 438 return dst; 439 } 440 441 @Override 442 public boolean equalsDeep(Base other) { 443 if (!super.equalsDeep(other)) 444 return false; 445 if (!(other instanceof MedicationProductIngredientComponent)) 446 return false; 447 MedicationProductIngredientComponent o = (MedicationProductIngredientComponent) other; 448 return compareDeep(item, o.item, true) && compareDeep(amount, o.amount, true); 449 } 450 451 @Override 452 public boolean equalsShallow(Base other) { 453 if (!super.equalsShallow(other)) 454 return false; 455 if (!(other instanceof MedicationProductIngredientComponent)) 456 return false; 457 MedicationProductIngredientComponent o = (MedicationProductIngredientComponent) other; 458 return true; 459 } 460 461 public boolean isEmpty() { 462 return super.isEmpty() && (item == null || item.isEmpty()) && (amount == null || amount.isEmpty()); 463 } 464 465 public String fhirType() { 466 return "Medication.product.ingredient"; 467 468 } 469 470 } 471 472 @Block() 473 public static class MedicationProductBatchComponent extends BackboneElement implements IBaseBackboneElement { 474 /** 475 * The assigned lot number of a batch of the specified product. 476 */ 477 @Child(name = "lotNumber", type = { 478 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 479 @Description(shortDefinition = "", formalDefinition = "The assigned lot number of a batch of the specified product.") 480 protected StringType lotNumber; 481 482 /** 483 * When this specific batch of product will expire. 484 */ 485 @Child(name = "expirationDate", type = { 486 DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 487 @Description(shortDefinition = "", formalDefinition = "When this specific batch of product will expire.") 488 protected DateTimeType expirationDate; 489 490 private static final long serialVersionUID = 1982738755L; 491 492 /* 493 * Constructor 494 */ 495 public MedicationProductBatchComponent() { 496 super(); 497 } 498 499 /** 500 * @return {@link #lotNumber} (The assigned lot number of a batch of the 501 * specified product.). This is the underlying object with id, value and 502 * extensions. The accessor "getLotNumber" gives direct access to the 503 * value 504 */ 505 public StringType getLotNumberElement() { 506 if (this.lotNumber == null) 507 if (Configuration.errorOnAutoCreate()) 508 throw new Error("Attempt to auto-create MedicationProductBatchComponent.lotNumber"); 509 else if (Configuration.doAutoCreate()) 510 this.lotNumber = new StringType(); // bb 511 return this.lotNumber; 512 } 513 514 public boolean hasLotNumberElement() { 515 return this.lotNumber != null && !this.lotNumber.isEmpty(); 516 } 517 518 public boolean hasLotNumber() { 519 return this.lotNumber != null && !this.lotNumber.isEmpty(); 520 } 521 522 /** 523 * @param value {@link #lotNumber} (The assigned lot number of a batch of the 524 * specified product.). This is the underlying object with id, 525 * value and extensions. The accessor "getLotNumber" gives direct 526 * access to the value 527 */ 528 public MedicationProductBatchComponent setLotNumberElement(StringType value) { 529 this.lotNumber = value; 530 return this; 531 } 532 533 /** 534 * @return The assigned lot number of a batch of the specified product. 535 */ 536 public String getLotNumber() { 537 return this.lotNumber == null ? null : this.lotNumber.getValue(); 538 } 539 540 /** 541 * @param value The assigned lot number of a batch of the specified product. 542 */ 543 public MedicationProductBatchComponent setLotNumber(String value) { 544 if (Utilities.noString(value)) 545 this.lotNumber = null; 546 else { 547 if (this.lotNumber == null) 548 this.lotNumber = new StringType(); 549 this.lotNumber.setValue(value); 550 } 551 return this; 552 } 553 554 /** 555 * @return {@link #expirationDate} (When this specific batch of product will 556 * expire.). This is the underlying object with id, value and 557 * extensions. The accessor "getExpirationDate" gives direct access to 558 * the value 559 */ 560 public DateTimeType getExpirationDateElement() { 561 if (this.expirationDate == null) 562 if (Configuration.errorOnAutoCreate()) 563 throw new Error("Attempt to auto-create MedicationProductBatchComponent.expirationDate"); 564 else if (Configuration.doAutoCreate()) 565 this.expirationDate = new DateTimeType(); // bb 566 return this.expirationDate; 567 } 568 569 public boolean hasExpirationDateElement() { 570 return this.expirationDate != null && !this.expirationDate.isEmpty(); 571 } 572 573 public boolean hasExpirationDate() { 574 return this.expirationDate != null && !this.expirationDate.isEmpty(); 575 } 576 577 /** 578 * @param value {@link #expirationDate} (When this specific batch of product 579 * will expire.). This is the underlying object with id, value and 580 * extensions. The accessor "getExpirationDate" gives direct access 581 * to the value 582 */ 583 public MedicationProductBatchComponent setExpirationDateElement(DateTimeType value) { 584 this.expirationDate = value; 585 return this; 586 } 587 588 /** 589 * @return When this specific batch of product will expire. 590 */ 591 public Date getExpirationDate() { 592 return this.expirationDate == null ? null : this.expirationDate.getValue(); 593 } 594 595 /** 596 * @param value When this specific batch of product will expire. 597 */ 598 public MedicationProductBatchComponent setExpirationDate(Date value) { 599 if (value == null) 600 this.expirationDate = null; 601 else { 602 if (this.expirationDate == null) 603 this.expirationDate = new DateTimeType(); 604 this.expirationDate.setValue(value); 605 } 606 return this; 607 } 608 609 protected void listChildren(List<Property> childrenList) { 610 super.listChildren(childrenList); 611 childrenList.add(new Property("lotNumber", "string", 612 "The assigned lot number of a batch of the specified product.", 0, java.lang.Integer.MAX_VALUE, lotNumber)); 613 childrenList.add(new Property("expirationDate", "dateTime", "When this specific batch of product will expire.", 0, 614 java.lang.Integer.MAX_VALUE, expirationDate)); 615 } 616 617 @Override 618 public void setProperty(String name, Base value) throws FHIRException { 619 if (name.equals("lotNumber")) 620 this.lotNumber = castToString(value); // StringType 621 else if (name.equals("expirationDate")) 622 this.expirationDate = castToDateTime(value); // DateTimeType 623 else 624 super.setProperty(name, value); 625 } 626 627 @Override 628 public Base addChild(String name) throws FHIRException { 629 if (name.equals("lotNumber")) { 630 throw new FHIRException("Cannot call addChild on a singleton property Medication.lotNumber"); 631 } else if (name.equals("expirationDate")) { 632 throw new FHIRException("Cannot call addChild on a singleton property Medication.expirationDate"); 633 } else 634 return super.addChild(name); 635 } 636 637 public MedicationProductBatchComponent copy() { 638 MedicationProductBatchComponent dst = new MedicationProductBatchComponent(); 639 copyValues(dst); 640 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 641 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 642 return dst; 643 } 644 645 @Override 646 public boolean equalsDeep(Base other) { 647 if (!super.equalsDeep(other)) 648 return false; 649 if (!(other instanceof MedicationProductBatchComponent)) 650 return false; 651 MedicationProductBatchComponent o = (MedicationProductBatchComponent) other; 652 return compareDeep(lotNumber, o.lotNumber, true) && compareDeep(expirationDate, o.expirationDate, true); 653 } 654 655 @Override 656 public boolean equalsShallow(Base other) { 657 if (!super.equalsShallow(other)) 658 return false; 659 if (!(other instanceof MedicationProductBatchComponent)) 660 return false; 661 MedicationProductBatchComponent o = (MedicationProductBatchComponent) other; 662 return compareValues(lotNumber, o.lotNumber, true) && compareValues(expirationDate, o.expirationDate, true); 663 } 664 665 public boolean isEmpty() { 666 return super.isEmpty() && (lotNumber == null || lotNumber.isEmpty()) 667 && (expirationDate == null || expirationDate.isEmpty()); 668 } 669 670 public String fhirType() { 671 return "Medication.product.batch"; 672 673 } 674 675 } 676 677 @Block() 678 public static class MedicationPackageComponent extends BackboneElement implements IBaseBackboneElement { 679 /** 680 * The kind of container that this package comes as. 681 */ 682 @Child(name = "container", type = { 683 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 684 @Description(shortDefinition = "E.g. box, vial, blister-pack", formalDefinition = "The kind of container that this package comes as.") 685 protected CodeableConcept container; 686 687 /** 688 * A set of components that go to make up the described item. 689 */ 690 @Child(name = "content", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 691 @Description(shortDefinition = "What is in the package", formalDefinition = "A set of components that go to make up the described item.") 692 protected List<MedicationPackageContentComponent> content; 693 694 private static final long serialVersionUID = 503772472L; 695 696 /* 697 * Constructor 698 */ 699 public MedicationPackageComponent() { 700 super(); 701 } 702 703 /** 704 * @return {@link #container} (The kind of container that this package comes 705 * as.) 706 */ 707 public CodeableConcept getContainer() { 708 if (this.container == null) 709 if (Configuration.errorOnAutoCreate()) 710 throw new Error("Attempt to auto-create MedicationPackageComponent.container"); 711 else if (Configuration.doAutoCreate()) 712 this.container = new CodeableConcept(); // cc 713 return this.container; 714 } 715 716 public boolean hasContainer() { 717 return this.container != null && !this.container.isEmpty(); 718 } 719 720 /** 721 * @param value {@link #container} (The kind of container that this package 722 * comes as.) 723 */ 724 public MedicationPackageComponent setContainer(CodeableConcept value) { 725 this.container = value; 726 return this; 727 } 728 729 /** 730 * @return {@link #content} (A set of components that go to make up the 731 * described item.) 732 */ 733 public List<MedicationPackageContentComponent> getContent() { 734 if (this.content == null) 735 this.content = new ArrayList<MedicationPackageContentComponent>(); 736 return this.content; 737 } 738 739 public boolean hasContent() { 740 if (this.content == null) 741 return false; 742 for (MedicationPackageContentComponent item : this.content) 743 if (!item.isEmpty()) 744 return true; 745 return false; 746 } 747 748 /** 749 * @return {@link #content} (A set of components that go to make up the 750 * described item.) 751 */ 752 // syntactic sugar 753 public MedicationPackageContentComponent addContent() { // 3 754 MedicationPackageContentComponent t = new MedicationPackageContentComponent(); 755 if (this.content == null) 756 this.content = new ArrayList<MedicationPackageContentComponent>(); 757 this.content.add(t); 758 return t; 759 } 760 761 // syntactic sugar 762 public MedicationPackageComponent addContent(MedicationPackageContentComponent t) { // 3 763 if (t == null) 764 return this; 765 if (this.content == null) 766 this.content = new ArrayList<MedicationPackageContentComponent>(); 767 this.content.add(t); 768 return this; 769 } 770 771 protected void listChildren(List<Property> childrenList) { 772 super.listChildren(childrenList); 773 childrenList.add(new Property("container", "CodeableConcept", "The kind of container that this package comes as.", 774 0, java.lang.Integer.MAX_VALUE, container)); 775 childrenList.add(new Property("content", "", "A set of components that go to make up the described item.", 0, 776 java.lang.Integer.MAX_VALUE, content)); 777 } 778 779 @Override 780 public void setProperty(String name, Base value) throws FHIRException { 781 if (name.equals("container")) 782 this.container = castToCodeableConcept(value); // CodeableConcept 783 else if (name.equals("content")) 784 this.getContent().add((MedicationPackageContentComponent) value); 785 else 786 super.setProperty(name, value); 787 } 788 789 @Override 790 public Base addChild(String name) throws FHIRException { 791 if (name.equals("container")) { 792 this.container = new CodeableConcept(); 793 return this.container; 794 } else if (name.equals("content")) { 795 return addContent(); 796 } else 797 return super.addChild(name); 798 } 799 800 public MedicationPackageComponent copy() { 801 MedicationPackageComponent dst = new MedicationPackageComponent(); 802 copyValues(dst); 803 dst.container = container == null ? null : container.copy(); 804 if (content != null) { 805 dst.content = new ArrayList<MedicationPackageContentComponent>(); 806 for (MedicationPackageContentComponent i : content) 807 dst.content.add(i.copy()); 808 } 809 ; 810 return dst; 811 } 812 813 @Override 814 public boolean equalsDeep(Base other) { 815 if (!super.equalsDeep(other)) 816 return false; 817 if (!(other instanceof MedicationPackageComponent)) 818 return false; 819 MedicationPackageComponent o = (MedicationPackageComponent) other; 820 return compareDeep(container, o.container, true) && compareDeep(content, o.content, true); 821 } 822 823 @Override 824 public boolean equalsShallow(Base other) { 825 if (!super.equalsShallow(other)) 826 return false; 827 if (!(other instanceof MedicationPackageComponent)) 828 return false; 829 MedicationPackageComponent o = (MedicationPackageComponent) other; 830 return true; 831 } 832 833 public boolean isEmpty() { 834 return super.isEmpty() && (container == null || container.isEmpty()) && (content == null || content.isEmpty()); 835 } 836 837 public String fhirType() { 838 return "Medication.package"; 839 840 } 841 842 } 843 844 @Block() 845 public static class MedicationPackageContentComponent extends BackboneElement implements IBaseBackboneElement { 846 /** 847 * Identifies one of the items in the package. 848 */ 849 @Child(name = "item", type = { Medication.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 850 @Description(shortDefinition = "A product in the package", formalDefinition = "Identifies one of the items in the package.") 851 protected Reference item; 852 853 /** 854 * The actual object that is the target of the reference (Identifies one of the 855 * items in the package.) 856 */ 857 protected Medication itemTarget; 858 859 /** 860 * The amount of the product that is in the package. 861 */ 862 @Child(name = "amount", type = { 863 SimpleQuantity.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 864 @Description(shortDefinition = "Quantity present in the package", formalDefinition = "The amount of the product that is in the package.") 865 protected SimpleQuantity amount; 866 867 private static final long serialVersionUID = -1150048030L; 868 869 /* 870 * Constructor 871 */ 872 public MedicationPackageContentComponent() { 873 super(); 874 } 875 876 /* 877 * Constructor 878 */ 879 public MedicationPackageContentComponent(Reference item) { 880 super(); 881 this.item = item; 882 } 883 884 /** 885 * @return {@link #item} (Identifies one of the items in the package.) 886 */ 887 public Reference getItem() { 888 if (this.item == null) 889 if (Configuration.errorOnAutoCreate()) 890 throw new Error("Attempt to auto-create MedicationPackageContentComponent.item"); 891 else if (Configuration.doAutoCreate()) 892 this.item = new Reference(); // cc 893 return this.item; 894 } 895 896 public boolean hasItem() { 897 return this.item != null && !this.item.isEmpty(); 898 } 899 900 /** 901 * @param value {@link #item} (Identifies one of the items in the package.) 902 */ 903 public MedicationPackageContentComponent setItem(Reference value) { 904 this.item = value; 905 return this; 906 } 907 908 /** 909 * @return {@link #item} The actual object that is the target of the reference. 910 * The reference library doesn't populate this, but you can use it to 911 * hold the resource if you resolve it. (Identifies one of the items in 912 * the package.) 913 */ 914 public Medication getItemTarget() { 915 if (this.itemTarget == null) 916 if (Configuration.errorOnAutoCreate()) 917 throw new Error("Attempt to auto-create MedicationPackageContentComponent.item"); 918 else if (Configuration.doAutoCreate()) 919 this.itemTarget = new Medication(); // aa 920 return this.itemTarget; 921 } 922 923 /** 924 * @param value {@link #item} The actual object that is the target of the 925 * reference. The reference library doesn't use these, but you can 926 * use it to hold the resource if you resolve it. (Identifies one 927 * of the items in the package.) 928 */ 929 public MedicationPackageContentComponent setItemTarget(Medication value) { 930 this.itemTarget = value; 931 return this; 932 } 933 934 /** 935 * @return {@link #amount} (The amount of the product that is in the package.) 936 */ 937 public SimpleQuantity getAmount() { 938 if (this.amount == null) 939 if (Configuration.errorOnAutoCreate()) 940 throw new Error("Attempt to auto-create MedicationPackageContentComponent.amount"); 941 else if (Configuration.doAutoCreate()) 942 this.amount = new SimpleQuantity(); // cc 943 return this.amount; 944 } 945 946 public boolean hasAmount() { 947 return this.amount != null && !this.amount.isEmpty(); 948 } 949 950 /** 951 * @param value {@link #amount} (The amount of the product that is in the 952 * package.) 953 */ 954 public MedicationPackageContentComponent setAmount(SimpleQuantity value) { 955 this.amount = value; 956 return this; 957 } 958 959 protected void listChildren(List<Property> childrenList) { 960 super.listChildren(childrenList); 961 childrenList.add(new Property("item", "Reference(Medication)", "Identifies one of the items in the package.", 0, 962 java.lang.Integer.MAX_VALUE, item)); 963 childrenList.add(new Property("amount", "SimpleQuantity", "The amount of the product that is in the package.", 0, 964 java.lang.Integer.MAX_VALUE, amount)); 965 } 966 967 @Override 968 public void setProperty(String name, Base value) throws FHIRException { 969 if (name.equals("item")) 970 this.item = castToReference(value); // Reference 971 else if (name.equals("amount")) 972 this.amount = castToSimpleQuantity(value); // SimpleQuantity 973 else 974 super.setProperty(name, value); 975 } 976 977 @Override 978 public Base addChild(String name) throws FHIRException { 979 if (name.equals("item")) { 980 this.item = new Reference(); 981 return this.item; 982 } else if (name.equals("amount")) { 983 this.amount = new SimpleQuantity(); 984 return this.amount; 985 } else 986 return super.addChild(name); 987 } 988 989 public MedicationPackageContentComponent copy() { 990 MedicationPackageContentComponent dst = new MedicationPackageContentComponent(); 991 copyValues(dst); 992 dst.item = item == null ? null : item.copy(); 993 dst.amount = amount == null ? null : amount.copy(); 994 return dst; 995 } 996 997 @Override 998 public boolean equalsDeep(Base other) { 999 if (!super.equalsDeep(other)) 1000 return false; 1001 if (!(other instanceof MedicationPackageContentComponent)) 1002 return false; 1003 MedicationPackageContentComponent o = (MedicationPackageContentComponent) other; 1004 return compareDeep(item, o.item, true) && compareDeep(amount, o.amount, true); 1005 } 1006 1007 @Override 1008 public boolean equalsShallow(Base other) { 1009 if (!super.equalsShallow(other)) 1010 return false; 1011 if (!(other instanceof MedicationPackageContentComponent)) 1012 return false; 1013 MedicationPackageContentComponent o = (MedicationPackageContentComponent) other; 1014 return true; 1015 } 1016 1017 public boolean isEmpty() { 1018 return super.isEmpty() && (item == null || item.isEmpty()) && (amount == null || amount.isEmpty()); 1019 } 1020 1021 public String fhirType() { 1022 return "Medication.package.content"; 1023 1024 } 1025 1026 } 1027 1028 /** 1029 * A code (or set of codes) that specify this medication, or a textual 1030 * description if no code is available. Usage note: This could be a standard 1031 * medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could 1032 * also be a national or local formulary code, optionally with translations to 1033 * other code systems. 1034 */ 1035 @Child(name = "code", type = { CodeableConcept.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 1036 @Description(shortDefinition = "Codes that identify this medication", formalDefinition = "A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.") 1037 protected CodeableConcept code; 1038 1039 /** 1040 * Set to true if the item is attributable to a specific manufacturer. 1041 */ 1042 @Child(name = "isBrand", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1043 @Description(shortDefinition = "True if a brand", formalDefinition = "Set to true if the item is attributable to a specific manufacturer.") 1044 protected BooleanType isBrand; 1045 1046 /** 1047 * Describes the details of the manufacturer. 1048 */ 1049 @Child(name = "manufacturer", type = { 1050 Organization.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1051 @Description(shortDefinition = "Manufacturer of the item", formalDefinition = "Describes the details of the manufacturer.") 1052 protected Reference manufacturer; 1053 1054 /** 1055 * The actual object that is the target of the reference (Describes the details 1056 * of the manufacturer.) 1057 */ 1058 protected Organization manufacturerTarget; 1059 1060 /** 1061 * Information that only applies to products (not packages). 1062 */ 1063 @Child(name = "product", type = {}, order = 3, min = 0, max = 1, modifier = false, summary = false) 1064 @Description(shortDefinition = "Administrable medication details", formalDefinition = "Information that only applies to products (not packages).") 1065 protected MedicationProductComponent product; 1066 1067 /** 1068 * Information that only applies to packages (not products). 1069 */ 1070 @Child(name = "package", type = {}, order = 4, min = 0, max = 1, modifier = false, summary = false) 1071 @Description(shortDefinition = "Details about packaged medications", formalDefinition = "Information that only applies to packages (not products).") 1072 protected MedicationPackageComponent package_; 1073 1074 private static final long serialVersionUID = 859308699L; 1075 1076 /* 1077 * Constructor 1078 */ 1079 public Medication() { 1080 super(); 1081 } 1082 1083 /** 1084 * @return {@link #code} (A code (or set of codes) that specify this medication, 1085 * or a textual description if no code is available. Usage note: This 1086 * could be a standard medication code such as a code from RxNorm, 1087 * SNOMED CT, IDMP etc. It could also be a national or local formulary 1088 * code, optionally with translations to other code systems.) 1089 */ 1090 public CodeableConcept getCode() { 1091 if (this.code == null) 1092 if (Configuration.errorOnAutoCreate()) 1093 throw new Error("Attempt to auto-create Medication.code"); 1094 else if (Configuration.doAutoCreate()) 1095 this.code = new CodeableConcept(); // cc 1096 return this.code; 1097 } 1098 1099 public boolean hasCode() { 1100 return this.code != null && !this.code.isEmpty(); 1101 } 1102 1103 /** 1104 * @param value {@link #code} (A code (or set of codes) that specify this 1105 * medication, or a textual description if no code is available. 1106 * Usage note: This could be a standard medication code such as a 1107 * code from RxNorm, SNOMED CT, IDMP etc. It could also be a 1108 * national or local formulary code, optionally with translations 1109 * to other code systems.) 1110 */ 1111 public Medication setCode(CodeableConcept value) { 1112 this.code = value; 1113 return this; 1114 } 1115 1116 /** 1117 * @return {@link #isBrand} (Set to true if the item is attributable to a 1118 * specific manufacturer.). This is the underlying object with id, value 1119 * and extensions. The accessor "getIsBrand" gives direct access to the 1120 * value 1121 */ 1122 public BooleanType getIsBrandElement() { 1123 if (this.isBrand == null) 1124 if (Configuration.errorOnAutoCreate()) 1125 throw new Error("Attempt to auto-create Medication.isBrand"); 1126 else if (Configuration.doAutoCreate()) 1127 this.isBrand = new BooleanType(); // bb 1128 return this.isBrand; 1129 } 1130 1131 public boolean hasIsBrandElement() { 1132 return this.isBrand != null && !this.isBrand.isEmpty(); 1133 } 1134 1135 public boolean hasIsBrand() { 1136 return this.isBrand != null && !this.isBrand.isEmpty(); 1137 } 1138 1139 /** 1140 * @param value {@link #isBrand} (Set to true if the item is attributable to a 1141 * specific manufacturer.). This is the underlying object with id, 1142 * value and extensions. The accessor "getIsBrand" gives direct 1143 * access to the value 1144 */ 1145 public Medication setIsBrandElement(BooleanType value) { 1146 this.isBrand = value; 1147 return this; 1148 } 1149 1150 /** 1151 * @return Set to true if the item is attributable to a specific manufacturer. 1152 */ 1153 public boolean getIsBrand() { 1154 return this.isBrand == null || this.isBrand.isEmpty() ? false : this.isBrand.getValue(); 1155 } 1156 1157 /** 1158 * @param value Set to true if the item is attributable to a specific 1159 * manufacturer. 1160 */ 1161 public Medication setIsBrand(boolean value) { 1162 if (this.isBrand == null) 1163 this.isBrand = new BooleanType(); 1164 this.isBrand.setValue(value); 1165 return this; 1166 } 1167 1168 /** 1169 * @return {@link #manufacturer} (Describes the details of the manufacturer.) 1170 */ 1171 public Reference getManufacturer() { 1172 if (this.manufacturer == null) 1173 if (Configuration.errorOnAutoCreate()) 1174 throw new Error("Attempt to auto-create Medication.manufacturer"); 1175 else if (Configuration.doAutoCreate()) 1176 this.manufacturer = new Reference(); // cc 1177 return this.manufacturer; 1178 } 1179 1180 public boolean hasManufacturer() { 1181 return this.manufacturer != null && !this.manufacturer.isEmpty(); 1182 } 1183 1184 /** 1185 * @param value {@link #manufacturer} (Describes the details of the 1186 * manufacturer.) 1187 */ 1188 public Medication setManufacturer(Reference value) { 1189 this.manufacturer = value; 1190 return this; 1191 } 1192 1193 /** 1194 * @return {@link #manufacturer} The actual object that is the target of the 1195 * reference. The reference library doesn't populate this, but you can 1196 * use it to hold the resource if you resolve it. (Describes the details 1197 * of the manufacturer.) 1198 */ 1199 public Organization getManufacturerTarget() { 1200 if (this.manufacturerTarget == null) 1201 if (Configuration.errorOnAutoCreate()) 1202 throw new Error("Attempt to auto-create Medication.manufacturer"); 1203 else if (Configuration.doAutoCreate()) 1204 this.manufacturerTarget = new Organization(); // aa 1205 return this.manufacturerTarget; 1206 } 1207 1208 /** 1209 * @param value {@link #manufacturer} The actual object that is the target of 1210 * the reference. The reference library doesn't use these, but you 1211 * can use it to hold the resource if you resolve it. (Describes 1212 * the details of the manufacturer.) 1213 */ 1214 public Medication setManufacturerTarget(Organization value) { 1215 this.manufacturerTarget = value; 1216 return this; 1217 } 1218 1219 /** 1220 * @return {@link #product} (Information that only applies to products (not 1221 * packages).) 1222 */ 1223 public MedicationProductComponent getProduct() { 1224 if (this.product == null) 1225 if (Configuration.errorOnAutoCreate()) 1226 throw new Error("Attempt to auto-create Medication.product"); 1227 else if (Configuration.doAutoCreate()) 1228 this.product = new MedicationProductComponent(); // cc 1229 return this.product; 1230 } 1231 1232 public boolean hasProduct() { 1233 return this.product != null && !this.product.isEmpty(); 1234 } 1235 1236 /** 1237 * @param value {@link #product} (Information that only applies to products (not 1238 * packages).) 1239 */ 1240 public Medication setProduct(MedicationProductComponent value) { 1241 this.product = value; 1242 return this; 1243 } 1244 1245 /** 1246 * @return {@link #package_} (Information that only applies to packages (not 1247 * products).) 1248 */ 1249 public MedicationPackageComponent getPackage() { 1250 if (this.package_ == null) 1251 if (Configuration.errorOnAutoCreate()) 1252 throw new Error("Attempt to auto-create Medication.package_"); 1253 else if (Configuration.doAutoCreate()) 1254 this.package_ = new MedicationPackageComponent(); // cc 1255 return this.package_; 1256 } 1257 1258 public boolean hasPackage() { 1259 return this.package_ != null && !this.package_.isEmpty(); 1260 } 1261 1262 /** 1263 * @param value {@link #package_} (Information that only applies to packages 1264 * (not products).) 1265 */ 1266 public Medication setPackage(MedicationPackageComponent value) { 1267 this.package_ = value; 1268 return this; 1269 } 1270 1271 protected void listChildren(List<Property> childrenList) { 1272 super.listChildren(childrenList); 1273 childrenList.add(new Property("code", "CodeableConcept", 1274 "A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.", 1275 0, java.lang.Integer.MAX_VALUE, code)); 1276 childrenList 1277 .add(new Property("isBrand", "boolean", "Set to true if the item is attributable to a specific manufacturer.", 1278 0, java.lang.Integer.MAX_VALUE, isBrand)); 1279 childrenList.add(new Property("manufacturer", "Reference(Organization)", 1280 "Describes the details of the manufacturer.", 0, java.lang.Integer.MAX_VALUE, manufacturer)); 1281 childrenList.add(new Property("product", "", "Information that only applies to products (not packages).", 0, 1282 java.lang.Integer.MAX_VALUE, product)); 1283 childrenList.add(new Property("package", "", "Information that only applies to packages (not products).", 0, 1284 java.lang.Integer.MAX_VALUE, package_)); 1285 } 1286 1287 @Override 1288 public void setProperty(String name, Base value) throws FHIRException { 1289 if (name.equals("code")) 1290 this.code = castToCodeableConcept(value); // CodeableConcept 1291 else if (name.equals("isBrand")) 1292 this.isBrand = castToBoolean(value); // BooleanType 1293 else if (name.equals("manufacturer")) 1294 this.manufacturer = castToReference(value); // Reference 1295 else if (name.equals("product")) 1296 this.product = (MedicationProductComponent) value; // MedicationProductComponent 1297 else if (name.equals("package")) 1298 this.package_ = (MedicationPackageComponent) value; // MedicationPackageComponent 1299 else 1300 super.setProperty(name, value); 1301 } 1302 1303 @Override 1304 public Base addChild(String name) throws FHIRException { 1305 if (name.equals("code")) { 1306 this.code = new CodeableConcept(); 1307 return this.code; 1308 } else if (name.equals("isBrand")) { 1309 throw new FHIRException("Cannot call addChild on a singleton property Medication.isBrand"); 1310 } else if (name.equals("manufacturer")) { 1311 this.manufacturer = new Reference(); 1312 return this.manufacturer; 1313 } else if (name.equals("product")) { 1314 this.product = new MedicationProductComponent(); 1315 return this.product; 1316 } else if (name.equals("package")) { 1317 this.package_ = new MedicationPackageComponent(); 1318 return this.package_; 1319 } else 1320 return super.addChild(name); 1321 } 1322 1323 public String fhirType() { 1324 return "Medication"; 1325 1326 } 1327 1328 public Medication copy() { 1329 Medication dst = new Medication(); 1330 copyValues(dst); 1331 dst.code = code == null ? null : code.copy(); 1332 dst.isBrand = isBrand == null ? null : isBrand.copy(); 1333 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 1334 dst.product = product == null ? null : product.copy(); 1335 dst.package_ = package_ == null ? null : package_.copy(); 1336 return dst; 1337 } 1338 1339 protected Medication typedCopy() { 1340 return copy(); 1341 } 1342 1343 @Override 1344 public boolean equalsDeep(Base other) { 1345 if (!super.equalsDeep(other)) 1346 return false; 1347 if (!(other instanceof Medication)) 1348 return false; 1349 Medication o = (Medication) other; 1350 return compareDeep(code, o.code, true) && compareDeep(isBrand, o.isBrand, true) 1351 && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(product, o.product, true) 1352 && compareDeep(package_, o.package_, true); 1353 } 1354 1355 @Override 1356 public boolean equalsShallow(Base other) { 1357 if (!super.equalsShallow(other)) 1358 return false; 1359 if (!(other instanceof Medication)) 1360 return false; 1361 Medication o = (Medication) other; 1362 return compareValues(isBrand, o.isBrand, true); 1363 } 1364 1365 public boolean isEmpty() { 1366 return super.isEmpty() && (code == null || code.isEmpty()) && (isBrand == null || isBrand.isEmpty()) 1367 && (manufacturer == null || manufacturer.isEmpty()) && (product == null || product.isEmpty()) 1368 && (package_ == null || package_.isEmpty()); 1369 } 1370 1371 @Override 1372 public ResourceType getResourceType() { 1373 return ResourceType.Medication; 1374 } 1375 1376 @SearchParamDefinition(name = "container", path = "Medication.package.container", description = "E.g. box, vial, blister-pack", type = "token") 1377 public static final String SP_CONTAINER = "container"; 1378 @SearchParamDefinition(name = "code", path = "Medication.code", description = "Codes that identify this medication", type = "token") 1379 public static final String SP_CODE = "code"; 1380 @SearchParamDefinition(name = "ingredient", path = "Medication.product.ingredient.item", description = "The product contained", type = "reference") 1381 public static final String SP_INGREDIENT = "ingredient"; 1382 @SearchParamDefinition(name = "form", path = "Medication.product.form", description = "powder | tablets | carton +", type = "token") 1383 public static final String SP_FORM = "form"; 1384 @SearchParamDefinition(name = "content", path = "Medication.package.content.item", description = "A product in the package", type = "reference") 1385 public static final String SP_CONTENT = "content"; 1386 @SearchParamDefinition(name = "manufacturer", path = "Medication.manufacturer", description = "Manufacturer of the item", type = "reference") 1387 public static final String SP_MANUFACTURER = "manufacturer"; 1388 1389}